title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
[VIDEO] Step-by-Step Visualization - Checking for Overflow | reverse-integer | 0 | 1 | ERROR: type should be string, got "https://youtu.be/YCxDTkWqcxw\\n\\nStep 1: Extract the digit in the ones place of `x` by using the modulo operator and store it in `digit`\\n\\nStep 2: Add that digit to `reverse` as the rightmost digit\\n\\nStep 3: Remove the ones digit from `x` and continue until `x` equals 0.\\n\\nIn Python, the modulo operator works slightly differently than other languages (such as Java or C) when it comes to negative numbers. Basically, you will get weird results if you try to do [positive number] mod [negative number]. If you want the modulo to behave the same way with negative numbers as it does with positive numbers, but just have the result be negative, then you need to make sure the divisor is also negative, since the modulo operation will always return a number with the same sign as the divisor.\\n\\nLastly, I use `math.trunc` instead of just using floor division `//` because of negative numbers. When dividing `x` by 10 and truncating the decimal, if the number is negative, then it would round down <i>away</i> from zero, when really, we want it to round up <i>towards</i> zero.\\n\\n# Code\\n```\\nclass Solution:\\n def reverse(self, x: int) -> int:\\n MAX_INT = 2 ** 31 - 1 # 2,147,483,647\\n MIN_INT = -2 ** 31 #-2,147,483,648\\n reverse = 0\\n\\n while x != 0:\\n if reverse > MAX_INT / 10 or reverse < MIN_INT / 10:\\n return 0\\n digit = x % 10 if x > 0 else x % -10\\n reverse = reverse * 10 + digit\\n x = math.trunc(x / 10)\\n\\n return reverse\\n\\n```" | 5 | Given a signed 32-bit integer `x`, return `x` _with its digits reversed_. If reversing `x` causes the value to go outside the signed 32-bit integer range `[-231, 231 - 1]`, then return `0`.
**Assume the environment does not allow you to store 64-bit integers (signed or unsigned).**
**Example 1:**
**Input:** x = 123
**Output:** 321
**Example 2:**
**Input:** x = -123
**Output:** -321
**Example 3:**
**Input:** x = 120
**Output:** 21
**Constraints:**
* `-231 <= x <= 231 - 1` | null |
Guys check my solution and tell me if it is wrong || and comment the Solution | reverse-integer | 0 | 1 | ***Guys PLEASE UPVOTE***\n\n\n# Code\n```\nclass Solution:\n def reverse(self, x: int) -> int:\n\n MIN=-2**31\n MAX=(2**31)-1\n k=0\n while x!=0:\n if k> MAX/10 or k<MIN/10 :\n return 0\n digit = x%10 if x>0 else x%-10\n k=k*10 + digit\n x=math.trunc(x/10)\n\n return k\n \n```\n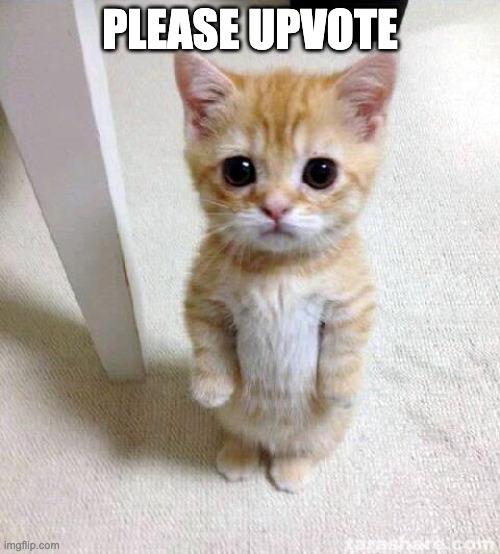 | 3 | Given a signed 32-bit integer `x`, return `x` _with its digits reversed_. If reversing `x` causes the value to go outside the signed 32-bit integer range `[-231, 231 - 1]`, then return `0`.
**Assume the environment does not allow you to store 64-bit integers (signed or unsigned).**
**Example 1:**
**Input:** x = 123
**Output:** 321
**Example 2:**
**Input:** x = -123
**Output:** -321
**Example 3:**
**Input:** x = 120
**Output:** 21
**Constraints:**
* `-231 <= x <= 231 - 1` | null |
Python | Simple Reverse String | O(n) | 90% T 87% S | reverse-integer | 0 | 1 | **Simple Reverse String Conversion**\n\n```\nclass Solution:\n def reverse(self, x: int) -> int:\n # positive case\n if x > 0:\n \n if int(str(x)[::-1]) > pow(2, 31) - 1: # out of bounds\n return 0\n \n return(int(str(x)[::-1])) # return reversed x\n \n # negative case\n x *= -1\n \n if int(str(x)[::-1]) > pow(2, 31) - 1: # out of bounds\n return 0\n \n return(int(str(x)[::-1]) * -1) # return reversed x * -1\n``` | 1 | Given a signed 32-bit integer `x`, return `x` _with its digits reversed_. If reversing `x` causes the value to go outside the signed 32-bit integer range `[-231, 231 - 1]`, then return `0`.
**Assume the environment does not allow you to store 64-bit integers (signed or unsigned).**
**Example 1:**
**Input:** x = 123
**Output:** 321
**Example 2:**
**Input:** x = -123
**Output:** -321
**Example 3:**
**Input:** x = 120
**Output:** 21
**Constraints:**
* `-231 <= x <= 231 - 1` | null |
Reverse Integer | reverse-integer | 0 | 1 | # Code\n```\nclass Solution:\n def reverse(self, x: int) -> int:\n x=list(str(x))\n x.reverse()\n k=""\n f=0\n for i in x:\n if(i!="-"):\n k+=i\n else:\n f=1\n k=int(k)\n if(k>(2**31)-1):\n return 0\n if(f==0):\n return k\n else:\n return -k\n``` | 2 | Given a signed 32-bit integer `x`, return `x` _with its digits reversed_. If reversing `x` causes the value to go outside the signed 32-bit integer range `[-231, 231 - 1]`, then return `0`.
**Assume the environment does not allow you to store 64-bit integers (signed or unsigned).**
**Example 1:**
**Input:** x = 123
**Output:** 321
**Example 2:**
**Input:** x = -123
**Output:** -321
**Example 3:**
**Input:** x = 120
**Output:** 21
**Constraints:**
* `-231 <= x <= 231 - 1` | null |
PYTHON SOLUTION | reverse-integer | 0 | 1 | # Intuition\nEasy solution in python\n\n# Code\n```\nMIN=-2**31\nMAX=(2**31)-1\nclass Solution:\n def __init__(self):\n self.rev=0\n self.is_neg=False\n def reverse(self, x: int) -> int:\n if x < 0:\n self.is_neg=True\n x=abs(x)\n while(x!=0):\n digit=x%10\n x=x//10\n\n if self.rev > MAX//10 or (self.rev==MAX//10 and digit>MAX%10):\n return 0\n if self.rev<MIN//10 or (self.rev==MIN//10 and digit <MIN%10):\n return 0\n \n self.rev=10*self.rev+digit\n if self.is_neg:\n self.rev=-self.rev\n return self.rev\n \n```\n\n\n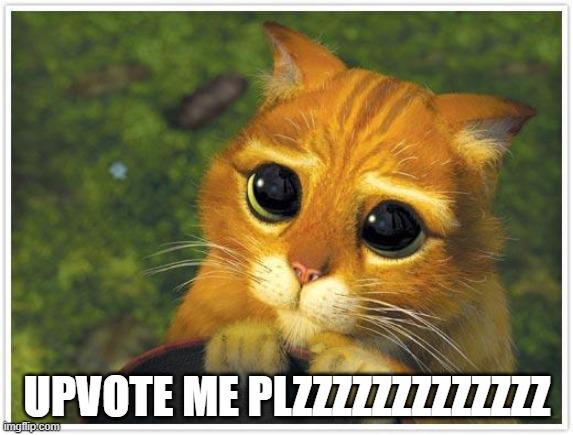\n | 12 | Given a signed 32-bit integer `x`, return `x` _with its digits reversed_. If reversing `x` causes the value to go outside the signed 32-bit integer range `[-231, 231 - 1]`, then return `0`.
**Assume the environment does not allow you to store 64-bit integers (signed or unsigned).**
**Example 1:**
**Input:** x = 123
**Output:** 321
**Example 2:**
**Input:** x = -123
**Output:** -321
**Example 3:**
**Input:** x = 120
**Output:** 21
**Constraints:**
* `-231 <= x <= 231 - 1` | null |
Iterative Python3 Solution | reverse-integer | 0 | 1 | # Intuition\nUtilize `//` (floor) and `%` (modulus) functions to break down the integer into its base-10 digits.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Instantiate DS to store digits of reversed number.\n2. Store digits in array as you use floor and modulus to break the number down, iteratively using a while loop.\n3. Store sign of final integer to return.\n4. Make sure integer meets constraints of problem statement.\n# Complexity\nTime complexity: $$O(log(n))$$\nSpace complexity: $$O(log(n))$$\n\n# Code\n```\nclass Solution:\n def reverse(self, x: int) -> int:\n arr = []\n should_reverse = x < 0\n if should_reverse:\n x *= -1\n while (x // 10 > 0):\n base = x % 10\n arr.append(base)\n x = x // 10\n arr.append(x)\n sum = 0\n for i in range(len(arr)):\n sum += ((10**(len(arr) - i - 1)) * arr[i])\n if should_reverse:\n sum *= -1\n meets_constraints = -2**31 <= sum and sum <= 2**31 - 1\n return sum if meets_constraints else 0\n\n \n``` | 1 | Given a signed 32-bit integer `x`, return `x` _with its digits reversed_. If reversing `x` causes the value to go outside the signed 32-bit integer range `[-231, 231 - 1]`, then return `0`.
**Assume the environment does not allow you to store 64-bit integers (signed or unsigned).**
**Example 1:**
**Input:** x = 123
**Output:** 321
**Example 2:**
**Input:** x = -123
**Output:** -321
**Example 3:**
**Input:** x = 120
**Output:** 21
**Constraints:**
* `-231 <= x <= 231 - 1` | null |
easy python code | reverse-integer | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def reverse(self, x: int) -> int:\n if(x<0):\n x=x*-1\n a=str(x)[::-1]\n if( int(a)<((2**31)-1) and int(a)>=(-(2**31))):\n return -int(a)\n else:\n return 0\n else:\n a=str(x)[::-1]\n if( int(a)<((2**31)-1) and int(a)>=(-2**31)):\n return int(a)\n else:\n return 0\n\n\n\n``` | 1 | Given a signed 32-bit integer `x`, return `x` _with its digits reversed_. If reversing `x` causes the value to go outside the signed 32-bit integer range `[-231, 231 - 1]`, then return `0`.
**Assume the environment does not allow you to store 64-bit integers (signed or unsigned).**
**Example 1:**
**Input:** x = 123
**Output:** 321
**Example 2:**
**Input:** x = -123
**Output:** -321
**Example 3:**
**Input:** x = 120
**Output:** 21
**Constraints:**
* `-231 <= x <= 231 - 1` | null |
Easy in Python | reverse-integer | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def reverse(self, x: int) -> int:\n MAX_INT = 2 ** 31 - 1 # 2,147,483,647\n MIN_INT = -2 ** 31 #-2,147,483,648\n reverse = 0\n\n while x != 0:\n if reverse > MAX_INT / 10 or reverse < MIN_INT / 10:\n return 0\n digit = x % 10 if x > 0 else x % -10\n reverse = reverse * 10 + digit\n x = math.trunc(x / 10)\n\n return reverse\n``` | 3 | Given a signed 32-bit integer `x`, return `x` _with its digits reversed_. If reversing `x` causes the value to go outside the signed 32-bit integer range `[-231, 231 - 1]`, then return `0`.
**Assume the environment does not allow you to store 64-bit integers (signed or unsigned).**
**Example 1:**
**Input:** x = 123
**Output:** 321
**Example 2:**
**Input:** x = -123
**Output:** -321
**Example 3:**
**Input:** x = 120
**Output:** 21
**Constraints:**
* `-231 <= x <= 231 - 1` | null |
Solution | string-to-integer-atoi | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def myAtoi(self, s: str) -> int:\n i = 0\n while i < len(s) and s[i].isspace():\n i += 1\n\n if i < len(s) and (s[i] == \'+\' or s[i] == \'-\'):\n sign = -1 if s[i] == \'-\' else 1\n i += 1\n else:\n sign = 1\n\n result = 0\n max_int = 2**31 - 1\n min_int = -2**31\n\n while i < len(s) and s[i].isdigit():\n digit = int(s[i])\n if result > (max_int - digit) // 10:\n return max_int if sign == 1 else min_int\n result = result * 10 + digit\n i += 1\n\n return sign * result\n\n``` | 1 | Implement the `myAtoi(string s)` function, which converts a string to a 32-bit signed integer (similar to C/C++'s `atoi` function).
The algorithm for `myAtoi(string s)` is as follows:
1. Read in and ignore any leading whitespace.
2. Check if the next character (if not already at the end of the string) is `'-'` or `'+'`. Read this character in if it is either. This determines if the final result is negative or positive respectively. Assume the result is positive if neither is present.
3. Read in next the characters until the next non-digit character or the end of the input is reached. The rest of the string is ignored.
4. Convert these digits into an integer (i.e. `"123 " -> 123`, `"0032 " -> 32`). If no digits were read, then the integer is `0`. Change the sign as necessary (from step 2).
5. If the integer is out of the 32-bit signed integer range `[-231, 231 - 1]`, then clamp the integer so that it remains in the range. Specifically, integers less than `-231` should be clamped to `-231`, and integers greater than `231 - 1` should be clamped to `231 - 1`.
6. Return the integer as the final result.
**Note:**
* Only the space character `' '` is considered a whitespace character.
* **Do not ignore** any characters other than the leading whitespace or the rest of the string after the digits.
**Example 1:**
**Input:** s = "42 "
**Output:** 42
**Explanation:** The underlined characters are what is read in, the caret is the current reader position.
Step 1: "42 " (no characters read because there is no leading whitespace)
^
Step 2: "42 " (no characters read because there is neither a '-' nor '+')
^
Step 3: "42 " ( "42 " is read in)
^
The parsed integer is 42.
Since 42 is in the range \[-231, 231 - 1\], the final result is 42.
**Example 2:**
**Input:** s = " -42 "
**Output:** -42
**Explanation:**
Step 1: " \-42 " (leading whitespace is read and ignored)
^
Step 2: " \-42 " ('-' is read, so the result should be negative)
^
Step 3: " -42 " ( "42 " is read in)
^
The parsed integer is -42.
Since -42 is in the range \[-231, 231 - 1\], the final result is -42.
**Example 3:**
**Input:** s = "4193 with words "
**Output:** 4193
**Explanation:**
Step 1: "4193 with words " (no characters read because there is no leading whitespace)
^
Step 2: "4193 with words " (no characters read because there is neither a '-' nor '+')
^
Step 3: "4193 with words " ( "4193 " is read in; reading stops because the next character is a non-digit)
^
The parsed integer is 4193.
Since 4193 is in the range \[-231, 231 - 1\], the final result is 4193.
**Constraints:**
* `0 <= s.length <= 200`
* `s` consists of English letters (lower-case and upper-case), digits (`0-9`), `' '`, `'+'`, `'-'`, and `'.'`. | null |
Fast and simpler DFA approach (Python 3) | string-to-integer-atoi | 0 | 1 | A fast and (probably) **much simpler and easier to understand DFA solution** than the others when you search for the keyword `DFA`:\n\n```python\nclass Solution:\n def myAtoi(self, str: str) -> int:\n value, state, pos, sign = 0, 0, 0, 1\n\n if len(str) == 0:\n return 0\n\n while pos < len(str):\n current_char = str[pos]\n if state == 0:\n if current_char == " ":\n state = 0\n elif current_char == "+" or current_char == "-":\n state = 1\n sign = 1 if current_char == "+" else -1\n elif current_char.isdigit():\n state = 2\n value = value * 10 + int(current_char)\n else:\n return 0\n elif state == 1:\n if current_char.isdigit():\n state = 2\n value = value * 10 + int(current_char)\n else:\n return 0\n elif state == 2:\n if current_char.isdigit():\n state = 2\n value = value * 10 + int(current_char)\n else:\n break\n else:\n return 0\n pos += 1\n\n value = sign * value\n value = min(value, 2 ** 31 - 1)\n value = max(-(2 ** 31), value)\n\n return value\n```\n\nDFA, which stands for Deterministic finite automaton, is a state machine that either accepts or rejects a sequence of symbols by running through a state sequence uniquely determined by the string. The DFA I used to implement this answer is very simple:\n\n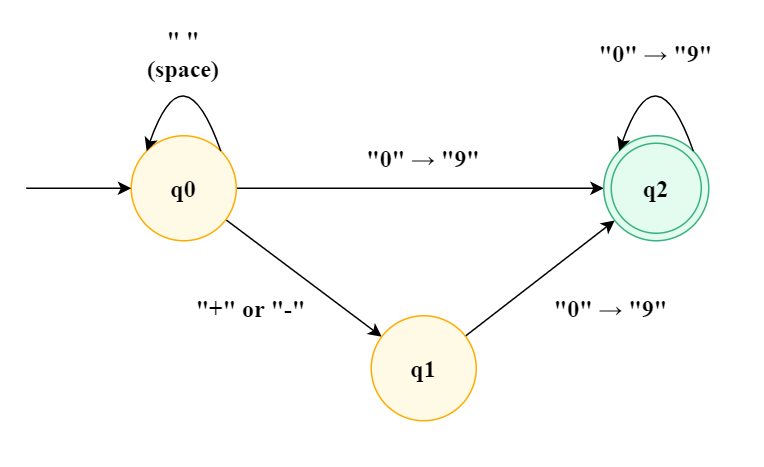\n\n | 286 | Implement the `myAtoi(string s)` function, which converts a string to a 32-bit signed integer (similar to C/C++'s `atoi` function).
The algorithm for `myAtoi(string s)` is as follows:
1. Read in and ignore any leading whitespace.
2. Check if the next character (if not already at the end of the string) is `'-'` or `'+'`. Read this character in if it is either. This determines if the final result is negative or positive respectively. Assume the result is positive if neither is present.
3. Read in next the characters until the next non-digit character or the end of the input is reached. The rest of the string is ignored.
4. Convert these digits into an integer (i.e. `"123 " -> 123`, `"0032 " -> 32`). If no digits were read, then the integer is `0`. Change the sign as necessary (from step 2).
5. If the integer is out of the 32-bit signed integer range `[-231, 231 - 1]`, then clamp the integer so that it remains in the range. Specifically, integers less than `-231` should be clamped to `-231`, and integers greater than `231 - 1` should be clamped to `231 - 1`.
6. Return the integer as the final result.
**Note:**
* Only the space character `' '` is considered a whitespace character.
* **Do not ignore** any characters other than the leading whitespace or the rest of the string after the digits.
**Example 1:**
**Input:** s = "42 "
**Output:** 42
**Explanation:** The underlined characters are what is read in, the caret is the current reader position.
Step 1: "42 " (no characters read because there is no leading whitespace)
^
Step 2: "42 " (no characters read because there is neither a '-' nor '+')
^
Step 3: "42 " ( "42 " is read in)
^
The parsed integer is 42.
Since 42 is in the range \[-231, 231 - 1\], the final result is 42.
**Example 2:**
**Input:** s = " -42 "
**Output:** -42
**Explanation:**
Step 1: " \-42 " (leading whitespace is read and ignored)
^
Step 2: " \-42 " ('-' is read, so the result should be negative)
^
Step 3: " -42 " ( "42 " is read in)
^
The parsed integer is -42.
Since -42 is in the range \[-231, 231 - 1\], the final result is -42.
**Example 3:**
**Input:** s = "4193 with words "
**Output:** 4193
**Explanation:**
Step 1: "4193 with words " (no characters read because there is no leading whitespace)
^
Step 2: "4193 with words " (no characters read because there is neither a '-' nor '+')
^
Step 3: "4193 with words " ( "4193 " is read in; reading stops because the next character is a non-digit)
^
The parsed integer is 4193.
Since 4193 is in the range \[-231, 231 - 1\], the final result is 4193.
**Constraints:**
* `0 <= s.length <= 200`
* `s` consists of English letters (lower-case and upper-case), digits (`0-9`), `' '`, `'+'`, `'-'`, and `'.'`. | null |
C++/Python beats 100% dealing with boundary cases | string-to-integer-atoi | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nAfter a lot of tries, it is solved, with many if-clauses and beats 100%.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Using do-while loop to deal with substring blank```\' \'```\'s at the beginning\n2. After the blank, the first occurring charater can either \'+\', \'-\' or digit, otherwise return 0\n3. Since the string s may be very long, it is a must to deal with overflow. Alone with long long it is not enough, use variable digit_len to track the actual length of number x.\n4. After the proceeding the major while loop, check weather x is positive or negative. Then everything is OK!\n \nLet\'s consider some examples\n```\n".1"->x=0\n```\n```\n" 0000000000012345678"->"0000000000012345678"->digit="12345678"\n=>digit_len=8<12\n=>x=12345678\n```\n```\n"-91283472332"->sgn=-1, digit="91283472332" ,\n digit_len=11<12 \n=> x=-91283472332<INT_MIN\n=> x=INT_MIN\n```\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(1)$$\n# C++ Code Runtime 0 ms Beats 100%\n```\nclass Solution {\npublic:\n int myAtoi(string s) {\n int n=s.size();\n char c;\n int i=0;\n //deal with \' \' at the beginning\n do{\n c=s[i++];\n }while (c==\' \');\n i--;\n\n //either \'+\', \'-\' or digit, otherwise return 0\n int sgn=1;\n long long x=0;\n int digit_len=0;\n c=s[i];\n if (c==\'-\') sgn=-1;\n else if (c==\'+\') sgn=1;\n else if (!isdigit(c)) return 0;\n else {//isdigit\n x=c-\'0\';\n if (x>0) digit_len++;\n }\n i++;\n\n //major loop. For dealing with overflow, use digit_len \n //to track the actual length of x\n while(i<n && digit_len<=12){\n c=s[i];\n if (!isdigit(c)) break;\n x=10*x+(c-\'0\');\n i++;\n if (x>0) digit_len++;\n }\n x=sgn*x;\n\n //Everything is OK!\n if (x>INT_MAX) x=INT_MAX;\n else if (x<INT_MIN) x=INT_MIN;\n return x; \n }\n};\n```\n\n# Python code, since there is no do-while loop, a little bit more tricky than C++\n```\nimport numpy as np\nclass Solution:\n def myAtoi(self, s: str) -> int:\n n=len(s)\n if n==0: return 0\n c=\' \'\n i=0\n while c==\' \' and i<n:\n c=s[i]\n i+=1\n i-=1\n\n sgn=1\n x=0\n digit_len=0\n c=s[i]\n if c==\'-\': sgn=-1\n elif c==\'+\': sgn=1\n elif not np.char.isdigit(c): return 0\n else:\n x=ord(c)-ord(\'0\')\n if x>0: digit_len+=1\n i+=1\n\n while i<n and digit_len<=12:\n c=s[i]\n if not np.char.isdigit(c): break\n x=10*x+ord(c)-ord(\'0\')\n i+=1\n if x>0: digit_len+=1\n x=sgn*x\n\n INT_MAX=2**31-1\n INT_MIN=-2**31\n if x>INT_MAX: x=INT_MAX\n elif x<INT_MIN: x=INT_MIN\n return x\n \n``` | 4 | Implement the `myAtoi(string s)` function, which converts a string to a 32-bit signed integer (similar to C/C++'s `atoi` function).
The algorithm for `myAtoi(string s)` is as follows:
1. Read in and ignore any leading whitespace.
2. Check if the next character (if not already at the end of the string) is `'-'` or `'+'`. Read this character in if it is either. This determines if the final result is negative or positive respectively. Assume the result is positive if neither is present.
3. Read in next the characters until the next non-digit character or the end of the input is reached. The rest of the string is ignored.
4. Convert these digits into an integer (i.e. `"123 " -> 123`, `"0032 " -> 32`). If no digits were read, then the integer is `0`. Change the sign as necessary (from step 2).
5. If the integer is out of the 32-bit signed integer range `[-231, 231 - 1]`, then clamp the integer so that it remains in the range. Specifically, integers less than `-231` should be clamped to `-231`, and integers greater than `231 - 1` should be clamped to `231 - 1`.
6. Return the integer as the final result.
**Note:**
* Only the space character `' '` is considered a whitespace character.
* **Do not ignore** any characters other than the leading whitespace or the rest of the string after the digits.
**Example 1:**
**Input:** s = "42 "
**Output:** 42
**Explanation:** The underlined characters are what is read in, the caret is the current reader position.
Step 1: "42 " (no characters read because there is no leading whitespace)
^
Step 2: "42 " (no characters read because there is neither a '-' nor '+')
^
Step 3: "42 " ( "42 " is read in)
^
The parsed integer is 42.
Since 42 is in the range \[-231, 231 - 1\], the final result is 42.
**Example 2:**
**Input:** s = " -42 "
**Output:** -42
**Explanation:**
Step 1: " \-42 " (leading whitespace is read and ignored)
^
Step 2: " \-42 " ('-' is read, so the result should be negative)
^
Step 3: " -42 " ( "42 " is read in)
^
The parsed integer is -42.
Since -42 is in the range \[-231, 231 - 1\], the final result is -42.
**Example 3:**
**Input:** s = "4193 with words "
**Output:** 4193
**Explanation:**
Step 1: "4193 with words " (no characters read because there is no leading whitespace)
^
Step 2: "4193 with words " (no characters read because there is neither a '-' nor '+')
^
Step 3: "4193 with words " ( "4193 " is read in; reading stops because the next character is a non-digit)
^
The parsed integer is 4193.
Since 4193 is in the range \[-231, 231 - 1\], the final result is 4193.
**Constraints:**
* `0 <= s.length <= 200`
* `s` consists of English letters (lower-case and upper-case), digits (`0-9`), `' '`, `'+'`, `'-'`, and `'.'`. | null |
Python Solution - Passes all test cases | string-to-integer-atoi | 0 | 1 | # Intuition\nSimple problem itself, just need to pass test cases.\n\n# Approach\nSolve each test case one by one, submit, solve next failed test case.\n\n# Complexity\n- Time complexity:\n $$O(n)$$\n\n- Space complexity:\n $$O(n)$$\n\n# Code\n```\nclass Solution:\n def myAtoi(self, s: str) -> int:\n s=s.strip()\n sign=1\n l=""\n res=""\n num=False\n\n for i in s:\n if i==\'-\' and num!= True:\n sign*=-1\n l+=i\n elif i==\'+\' and num!=True:\n l+=i\n continue\n elif ord(\'0\')<=ord(i)<=ord(\'9\'):\n res+=i\n num=True\n elif not ord(\'0\')<=ord(i)<=ord(\'9\'):\n break\n \n print(res)\n if res=="" or len(l)>1:\n return 0\n else:\n\n if (int(res)*sign)>2**31 -1:\n return 2**31 - 1\n elif (int(res)*sign)<(-2)**31:\n return (-2)**31\n return int(res)*sign\n\n\n\n``` | 1 | Implement the `myAtoi(string s)` function, which converts a string to a 32-bit signed integer (similar to C/C++'s `atoi` function).
The algorithm for `myAtoi(string s)` is as follows:
1. Read in and ignore any leading whitespace.
2. Check if the next character (if not already at the end of the string) is `'-'` or `'+'`. Read this character in if it is either. This determines if the final result is negative or positive respectively. Assume the result is positive if neither is present.
3. Read in next the characters until the next non-digit character or the end of the input is reached. The rest of the string is ignored.
4. Convert these digits into an integer (i.e. `"123 " -> 123`, `"0032 " -> 32`). If no digits were read, then the integer is `0`. Change the sign as necessary (from step 2).
5. If the integer is out of the 32-bit signed integer range `[-231, 231 - 1]`, then clamp the integer so that it remains in the range. Specifically, integers less than `-231` should be clamped to `-231`, and integers greater than `231 - 1` should be clamped to `231 - 1`.
6. Return the integer as the final result.
**Note:**
* Only the space character `' '` is considered a whitespace character.
* **Do not ignore** any characters other than the leading whitespace or the rest of the string after the digits.
**Example 1:**
**Input:** s = "42 "
**Output:** 42
**Explanation:** The underlined characters are what is read in, the caret is the current reader position.
Step 1: "42 " (no characters read because there is no leading whitespace)
^
Step 2: "42 " (no characters read because there is neither a '-' nor '+')
^
Step 3: "42 " ( "42 " is read in)
^
The parsed integer is 42.
Since 42 is in the range \[-231, 231 - 1\], the final result is 42.
**Example 2:**
**Input:** s = " -42 "
**Output:** -42
**Explanation:**
Step 1: " \-42 " (leading whitespace is read and ignored)
^
Step 2: " \-42 " ('-' is read, so the result should be negative)
^
Step 3: " -42 " ( "42 " is read in)
^
The parsed integer is -42.
Since -42 is in the range \[-231, 231 - 1\], the final result is -42.
**Example 3:**
**Input:** s = "4193 with words "
**Output:** 4193
**Explanation:**
Step 1: "4193 with words " (no characters read because there is no leading whitespace)
^
Step 2: "4193 with words " (no characters read because there is neither a '-' nor '+')
^
Step 3: "4193 with words " ( "4193 " is read in; reading stops because the next character is a non-digit)
^
The parsed integer is 4193.
Since 4193 is in the range \[-231, 231 - 1\], the final result is 4193.
**Constraints:**
* `0 <= s.length <= 200`
* `s` consists of English letters (lower-case and upper-case), digits (`0-9`), `' '`, `'+'`, `'-'`, and `'.'`. | null |
Python Very Intuitive with Comments | string-to-integer-atoi | 0 | 1 | ```\nclass Solution:\n def myAtoi(self, s: str) -> int:\n if not s:\n return 0\n\n # remove leading and trailing whitespace\n s = s.strip()\n\n # save sign if one exists\n pos = True\n if s and s[0] == \'-\':\n pos = False\n s = s[1:]\n elif s and s[0] == \'+\':\n s = s[1:]\n \n # ignore leading zeros\n i = 0\n while i < len(s) and s[i] == \'0\':\n i += 1\n\n # apply relevant digits\n res = None\n while i < len(s) and s[i] in \'0123456789\':\n if res is None:\n res = int(s[i])\n else:\n res = (res * 10) + int(s[i])\n i += 1\n res = 0 if res is None else res\n\n # apply sign\n res = res if pos else -res\n\n # clip result\n res = max(res, -2**31)\n res = min(res, (2**31)-1)\n\n return res\n\n``` | 5 | Implement the `myAtoi(string s)` function, which converts a string to a 32-bit signed integer (similar to C/C++'s `atoi` function).
The algorithm for `myAtoi(string s)` is as follows:
1. Read in and ignore any leading whitespace.
2. Check if the next character (if not already at the end of the string) is `'-'` or `'+'`. Read this character in if it is either. This determines if the final result is negative or positive respectively. Assume the result is positive if neither is present.
3. Read in next the characters until the next non-digit character or the end of the input is reached. The rest of the string is ignored.
4. Convert these digits into an integer (i.e. `"123 " -> 123`, `"0032 " -> 32`). If no digits were read, then the integer is `0`. Change the sign as necessary (from step 2).
5. If the integer is out of the 32-bit signed integer range `[-231, 231 - 1]`, then clamp the integer so that it remains in the range. Specifically, integers less than `-231` should be clamped to `-231`, and integers greater than `231 - 1` should be clamped to `231 - 1`.
6. Return the integer as the final result.
**Note:**
* Only the space character `' '` is considered a whitespace character.
* **Do not ignore** any characters other than the leading whitespace or the rest of the string after the digits.
**Example 1:**
**Input:** s = "42 "
**Output:** 42
**Explanation:** The underlined characters are what is read in, the caret is the current reader position.
Step 1: "42 " (no characters read because there is no leading whitespace)
^
Step 2: "42 " (no characters read because there is neither a '-' nor '+')
^
Step 3: "42 " ( "42 " is read in)
^
The parsed integer is 42.
Since 42 is in the range \[-231, 231 - 1\], the final result is 42.
**Example 2:**
**Input:** s = " -42 "
**Output:** -42
**Explanation:**
Step 1: " \-42 " (leading whitespace is read and ignored)
^
Step 2: " \-42 " ('-' is read, so the result should be negative)
^
Step 3: " -42 " ( "42 " is read in)
^
The parsed integer is -42.
Since -42 is in the range \[-231, 231 - 1\], the final result is -42.
**Example 3:**
**Input:** s = "4193 with words "
**Output:** 4193
**Explanation:**
Step 1: "4193 with words " (no characters read because there is no leading whitespace)
^
Step 2: "4193 with words " (no characters read because there is neither a '-' nor '+')
^
Step 3: "4193 with words " ( "4193 " is read in; reading stops because the next character is a non-digit)
^
The parsed integer is 4193.
Since 4193 is in the range \[-231, 231 - 1\], the final result is 4193.
**Constraints:**
* `0 <= s.length <= 200`
* `s` consists of English letters (lower-case and upper-case), digits (`0-9`), `' '`, `'+'`, `'-'`, and `'.'`. | null |
Beats : 90.02% [42/145 Top Interview Question] | string-to-integer-atoi | 0 | 1 | # Intuition\n*Paper and pen and try to figure out all the possible edge cases, The question was easy, but the question explanation was poor.No need to worry about the **least acceptance rate** that the question has.*\n\n# Approach\nThis code defines a class called Solution with a method named `myAtoi` which takes a string `s` as input and returns an integer as output. The method has some considerations which are mentioned in its docstring ***`(please do read)`***. The method performs the following steps:\n\n1. Define two constants `maxInt` and `minInt` as the maximum and minimum integer values that can be represented using 32 bits.\n\n2. Initialize the integer variables `result`, `startIdx`, and `sign` to 0, 0, and 1, respectively. \n\n3. Remove any leading whitespace characters from the input string `s` using the `lstrip()` method and store it in a variable called `cleanStr`. If `cleanStr` is an empty string, return `result`.\n\n4. Check if the first character of `cleanStr` at `startIdx` is either `"+"` or `"-"`. If it is `"-"`, set the `sign` variable to -1, otherwise, leave it as 1. If the first character is a sign, increment `startIdx` by 1.\n\n5. Iterate through the remaining characters in `cleanStr` starting at index `startIdx`. If a non-digit character is encountered, break the loop. If a digit is encountered, add it to the `result` variable by multiplying it by 10 and adding the integer value of the character.\n\n6. Check if the final result multiplied by `sign` is greater than `maxInt`. If it is, return `maxInt`. If it is less than or equal to `minInt`, return `minInt`.\n\n7. If the value is within the range of `maxInt` and `minInt`, return the value multiplied by `sign`.\n\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(1)\n\n`Time complexity`:\n- The method performs a single pass through the input string, which takes `O(n)` time, where `n` is the length of the input string.\n- The string operations such as `lstrip()` and `isdigit()` take constant time per character, so they don\'t affect the overall time complexity of the algorithm.\n- Therefore, the `time complexity` of the method is `O(n)`.\n\n`Space complexity`:\n- The method uses a constant amount of extra space to store integer variables and constants, so the `space complexity` is `O(1)`.\n- The additional space required by the method doesn\'t depend on the input size, so it is considered `constant`.\n\nTherefore, the overall `time complexity` is `O(n)` and the `space complexity` is `O(1)`.\n\n# Code\n```\nclass Solution:\n def myAtoi(self, s: str) -> int:\n """\n Considerations: \n 1. If there is a leading whitespace at start, remove it.\n 2. Check the sign and store it in a varible.\n 3. try adding the digits to the result.\n 4. witnessing anything other than a digit break the loop.\n\t 5. check the range and return accordingly.\n """\n\n maxInt, minInt = 2**31 - 1 , -2**31\n result, startIdx, sign = 0,0,1\n cleanStr = s.lstrip()\n \n if not cleanStr: return result\n\n if cleanStr[startIdx] in ("-", "+"):\n sign = -1 if cleanStr[startIdx] == "-" else 1 \n startIdx += 1\n \n for i in range(startIdx, len(cleanStr)):\n char = cleanStr[i]\n if not char.isdigit():\n break\n else:\n # read note at the end, if confusing\n result = (result * 10) + int(char)\n\n if result * sign > maxInt:\n return maxInt\n elif result * sign <= minInt:\n return minInt\n \n return result * sign\n\n"""\nNote: \nQ1. why int(char)?\nAns: The char will be x, where x is a digit in string format\n\nQ2. why result * 10?\nAns: We need to shift the current value of result\n to the left by one decimal place (i.e., multiply it by 10) \n and then add the integer value of the new digit to the result\n"""\n \n``` | 5 | Implement the `myAtoi(string s)` function, which converts a string to a 32-bit signed integer (similar to C/C++'s `atoi` function).
The algorithm for `myAtoi(string s)` is as follows:
1. Read in and ignore any leading whitespace.
2. Check if the next character (if not already at the end of the string) is `'-'` or `'+'`. Read this character in if it is either. This determines if the final result is negative or positive respectively. Assume the result is positive if neither is present.
3. Read in next the characters until the next non-digit character or the end of the input is reached. The rest of the string is ignored.
4. Convert these digits into an integer (i.e. `"123 " -> 123`, `"0032 " -> 32`). If no digits were read, then the integer is `0`. Change the sign as necessary (from step 2).
5. If the integer is out of the 32-bit signed integer range `[-231, 231 - 1]`, then clamp the integer so that it remains in the range. Specifically, integers less than `-231` should be clamped to `-231`, and integers greater than `231 - 1` should be clamped to `231 - 1`.
6. Return the integer as the final result.
**Note:**
* Only the space character `' '` is considered a whitespace character.
* **Do not ignore** any characters other than the leading whitespace or the rest of the string after the digits.
**Example 1:**
**Input:** s = "42 "
**Output:** 42
**Explanation:** The underlined characters are what is read in, the caret is the current reader position.
Step 1: "42 " (no characters read because there is no leading whitespace)
^
Step 2: "42 " (no characters read because there is neither a '-' nor '+')
^
Step 3: "42 " ( "42 " is read in)
^
The parsed integer is 42.
Since 42 is in the range \[-231, 231 - 1\], the final result is 42.
**Example 2:**
**Input:** s = " -42 "
**Output:** -42
**Explanation:**
Step 1: " \-42 " (leading whitespace is read and ignored)
^
Step 2: " \-42 " ('-' is read, so the result should be negative)
^
Step 3: " -42 " ( "42 " is read in)
^
The parsed integer is -42.
Since -42 is in the range \[-231, 231 - 1\], the final result is -42.
**Example 3:**
**Input:** s = "4193 with words "
**Output:** 4193
**Explanation:**
Step 1: "4193 with words " (no characters read because there is no leading whitespace)
^
Step 2: "4193 with words " (no characters read because there is neither a '-' nor '+')
^
Step 3: "4193 with words " ( "4193 " is read in; reading stops because the next character is a non-digit)
^
The parsed integer is 4193.
Since 4193 is in the range \[-231, 231 - 1\], the final result is 4193.
**Constraints:**
* `0 <= s.length <= 200`
* `s` consists of English letters (lower-case and upper-case), digits (`0-9`), `' '`, `'+'`, `'-'`, and `'.'`. | null |
Best Solution || Python3 || 100% Working || Using Regex || | string-to-integer-atoi | 0 | 1 | # Approach\nUsed Regular Expression approach to solve..!\n\n# Code\n```\nimport re\nclass Solution:\n def myAtoi(self, s: str) -> int:\n s = s.strip()\n if len(s):\n if not s[0].isalpha():\n i = re.search(r"^(-\\d+)|^(\\+\\d+)|^(\\d+)",s)\n if i is not None:\n i = int(i.group())\n if i < 0:\n return max(-2**31, i)\n return min(2**31 - 1, i)\n return 0\n \n``` | 2 | Implement the `myAtoi(string s)` function, which converts a string to a 32-bit signed integer (similar to C/C++'s `atoi` function).
The algorithm for `myAtoi(string s)` is as follows:
1. Read in and ignore any leading whitespace.
2. Check if the next character (if not already at the end of the string) is `'-'` or `'+'`. Read this character in if it is either. This determines if the final result is negative or positive respectively. Assume the result is positive if neither is present.
3. Read in next the characters until the next non-digit character or the end of the input is reached. The rest of the string is ignored.
4. Convert these digits into an integer (i.e. `"123 " -> 123`, `"0032 " -> 32`). If no digits were read, then the integer is `0`. Change the sign as necessary (from step 2).
5. If the integer is out of the 32-bit signed integer range `[-231, 231 - 1]`, then clamp the integer so that it remains in the range. Specifically, integers less than `-231` should be clamped to `-231`, and integers greater than `231 - 1` should be clamped to `231 - 1`.
6. Return the integer as the final result.
**Note:**
* Only the space character `' '` is considered a whitespace character.
* **Do not ignore** any characters other than the leading whitespace or the rest of the string after the digits.
**Example 1:**
**Input:** s = "42 "
**Output:** 42
**Explanation:** The underlined characters are what is read in, the caret is the current reader position.
Step 1: "42 " (no characters read because there is no leading whitespace)
^
Step 2: "42 " (no characters read because there is neither a '-' nor '+')
^
Step 3: "42 " ( "42 " is read in)
^
The parsed integer is 42.
Since 42 is in the range \[-231, 231 - 1\], the final result is 42.
**Example 2:**
**Input:** s = " -42 "
**Output:** -42
**Explanation:**
Step 1: " \-42 " (leading whitespace is read and ignored)
^
Step 2: " \-42 " ('-' is read, so the result should be negative)
^
Step 3: " -42 " ( "42 " is read in)
^
The parsed integer is -42.
Since -42 is in the range \[-231, 231 - 1\], the final result is -42.
**Example 3:**
**Input:** s = "4193 with words "
**Output:** 4193
**Explanation:**
Step 1: "4193 with words " (no characters read because there is no leading whitespace)
^
Step 2: "4193 with words " (no characters read because there is neither a '-' nor '+')
^
Step 3: "4193 with words " ( "4193 " is read in; reading stops because the next character is a non-digit)
^
The parsed integer is 4193.
Since 4193 is in the range \[-231, 231 - 1\], the final result is 4193.
**Constraints:**
* `0 <= s.length <= 200`
* `s` consists of English letters (lower-case and upper-case), digits (`0-9`), `' '`, `'+'`, `'-'`, and `'.'`. | null |
Python Easy Solution | string-to-integer-atoi | 0 | 1 | ```\nclass Solution:\n def myAtoi(self, s: str) -> int:\n su,num,flag = 1,0,0\n s = s.strip()\n if len(s) == 0: return 0\n if s[0] == "-":\n su = -1\n for i in s:\n if i.isdigit():\n num = num*10 + int(i)\n flag = 1\n elif (i == "+" or i == "-") and (flag == 0):\n flag = 1\n pass\n else: break\n num = num*su\n if (-2**31<=num<=(2**31)-1): return num\n if num<0: return -2**31\n else: return 2**31-1 | 5 | Implement the `myAtoi(string s)` function, which converts a string to a 32-bit signed integer (similar to C/C++'s `atoi` function).
The algorithm for `myAtoi(string s)` is as follows:
1. Read in and ignore any leading whitespace.
2. Check if the next character (if not already at the end of the string) is `'-'` or `'+'`. Read this character in if it is either. This determines if the final result is negative or positive respectively. Assume the result is positive if neither is present.
3. Read in next the characters until the next non-digit character or the end of the input is reached. The rest of the string is ignored.
4. Convert these digits into an integer (i.e. `"123 " -> 123`, `"0032 " -> 32`). If no digits were read, then the integer is `0`. Change the sign as necessary (from step 2).
5. If the integer is out of the 32-bit signed integer range `[-231, 231 - 1]`, then clamp the integer so that it remains in the range. Specifically, integers less than `-231` should be clamped to `-231`, and integers greater than `231 - 1` should be clamped to `231 - 1`.
6. Return the integer as the final result.
**Note:**
* Only the space character `' '` is considered a whitespace character.
* **Do not ignore** any characters other than the leading whitespace or the rest of the string after the digits.
**Example 1:**
**Input:** s = "42 "
**Output:** 42
**Explanation:** The underlined characters are what is read in, the caret is the current reader position.
Step 1: "42 " (no characters read because there is no leading whitespace)
^
Step 2: "42 " (no characters read because there is neither a '-' nor '+')
^
Step 3: "42 " ( "42 " is read in)
^
The parsed integer is 42.
Since 42 is in the range \[-231, 231 - 1\], the final result is 42.
**Example 2:**
**Input:** s = " -42 "
**Output:** -42
**Explanation:**
Step 1: " \-42 " (leading whitespace is read and ignored)
^
Step 2: " \-42 " ('-' is read, so the result should be negative)
^
Step 3: " -42 " ( "42 " is read in)
^
The parsed integer is -42.
Since -42 is in the range \[-231, 231 - 1\], the final result is -42.
**Example 3:**
**Input:** s = "4193 with words "
**Output:** 4193
**Explanation:**
Step 1: "4193 with words " (no characters read because there is no leading whitespace)
^
Step 2: "4193 with words " (no characters read because there is neither a '-' nor '+')
^
Step 3: "4193 with words " ( "4193 " is read in; reading stops because the next character is a non-digit)
^
The parsed integer is 4193.
Since 4193 is in the range \[-231, 231 - 1\], the final result is 4193.
**Constraints:**
* `0 <= s.length <= 200`
* `s` consists of English letters (lower-case and upper-case), digits (`0-9`), `' '`, `'+'`, `'-'`, and `'.'`. | null |
Python 3 step by step solution in code EASY | string-to-integer-atoi | 0 | 1 | \n```\nclass Solution:\n def myAtoi(self, s: str) -> int:\n # remove leading and trailing white space\n s = s.strip()\n if not s:\n return 0\n \n # determine the sign of the number\n sign = 1\n if s[0] == \'-\':\n sign = -1\n s = s[1:]\n elif s[0] == \'+\':\n s = s[1:]\n \n # read the digits until a non-digit character is encountered\n digits = []\n for c in s:\n if not c.isdigit():\n break\n digits.append(c)\n \n # convert the digits to an integer\n if not digits:\n return 0\n num = int(\'\'.join(digits)) * sign\n \n # clamp the number to the 32-bit range\n num = max(min(num, 2**31 - 1), -2**31)\n \n return num\n``` | 1 | Implement the `myAtoi(string s)` function, which converts a string to a 32-bit signed integer (similar to C/C++'s `atoi` function).
The algorithm for `myAtoi(string s)` is as follows:
1. Read in and ignore any leading whitespace.
2. Check if the next character (if not already at the end of the string) is `'-'` or `'+'`. Read this character in if it is either. This determines if the final result is negative or positive respectively. Assume the result is positive if neither is present.
3. Read in next the characters until the next non-digit character or the end of the input is reached. The rest of the string is ignored.
4. Convert these digits into an integer (i.e. `"123 " -> 123`, `"0032 " -> 32`). If no digits were read, then the integer is `0`. Change the sign as necessary (from step 2).
5. If the integer is out of the 32-bit signed integer range `[-231, 231 - 1]`, then clamp the integer so that it remains in the range. Specifically, integers less than `-231` should be clamped to `-231`, and integers greater than `231 - 1` should be clamped to `231 - 1`.
6. Return the integer as the final result.
**Note:**
* Only the space character `' '` is considered a whitespace character.
* **Do not ignore** any characters other than the leading whitespace or the rest of the string after the digits.
**Example 1:**
**Input:** s = "42 "
**Output:** 42
**Explanation:** The underlined characters are what is read in, the caret is the current reader position.
Step 1: "42 " (no characters read because there is no leading whitespace)
^
Step 2: "42 " (no characters read because there is neither a '-' nor '+')
^
Step 3: "42 " ( "42 " is read in)
^
The parsed integer is 42.
Since 42 is in the range \[-231, 231 - 1\], the final result is 42.
**Example 2:**
**Input:** s = " -42 "
**Output:** -42
**Explanation:**
Step 1: " \-42 " (leading whitespace is read and ignored)
^
Step 2: " \-42 " ('-' is read, so the result should be negative)
^
Step 3: " -42 " ( "42 " is read in)
^
The parsed integer is -42.
Since -42 is in the range \[-231, 231 - 1\], the final result is -42.
**Example 3:**
**Input:** s = "4193 with words "
**Output:** 4193
**Explanation:**
Step 1: "4193 with words " (no characters read because there is no leading whitespace)
^
Step 2: "4193 with words " (no characters read because there is neither a '-' nor '+')
^
Step 3: "4193 with words " ( "4193 " is read in; reading stops because the next character is a non-digit)
^
The parsed integer is 4193.
Since 4193 is in the range \[-231, 231 - 1\], the final result is 4193.
**Constraints:**
* `0 <= s.length <= 200`
* `s` consists of English letters (lower-case and upper-case), digits (`0-9`), `' '`, `'+'`, `'-'`, and `'.'`. | null |
✅2 Method's || C++ || JAVA || PYTHON || Beginner Friendly🔥🔥🔥 | palindrome-number | 1 | 1 | # Intuition:\nThe intuition behind this code is to reverse the entire input number and check if the reversed number is equal to the original number. If they are the same, then the number is a palindrome.\n\n# Approach 1: Reversing the Entire Number\n# Explanation:\n1. We begin by performing an initial check. If the input number `x` is negative, it cannot be a palindrome since palindromes are typically defined for positive numbers. In such cases, we immediately return `false`.\n\n2. We initialize two variables:\n - `reversed`: This variable will store the reversed value of the number `x`.\n - `temp`: This variable is a temporary placeholder to manipulate the input number without modifying the original value.\n\n3. We enter a loop that continues until `temp` becomes zero:\n - Inside the loop, we extract the last digit of `temp` using the modulo operator `%` and store it in the `digit` variable.\n - To reverse the number, we multiply the current value of `reversed` by 10 and add the extracted `digit`.\n - We then divide `temp` by 10 to remove the last digit and move on to the next iteration.\n\n4. Once the loop is completed, we have reversed the entire number. Now, we compare the reversed value `reversed` with the original input value `x`.\n - If they are equal, it means the number is a palindrome, so we return `true`.\n - If they are not equal, it means the number is not a palindrome, so we return `false`.\n\nThe code uses a `long long` data type for the `reversed` variable to handle potential overflow in case of large input numbers.\n\n# Code\n\n```C++ []\nclass Solution {\npublic:\n bool isPalindrome(int x) {\n if (x < 0) {\n return false;\n }\n\n long long reversed = 0;\n long long temp = x;\n\n while (temp != 0) {\n int digit = temp % 10;\n reversed = reversed * 10 + digit;\n temp /= 10;\n }\n\n return (reversed == x);\n }\n};\n\n```\n```Java []\nclass Solution {\n public boolean isPalindrome(int x) {\n if (x < 0) {\n return false;\n }\n\n long reversed = 0;\n long temp = x;\n\n while (temp != 0) {\n int digit = (int) (temp % 10);\n reversed = reversed * 10 + digit;\n temp /= 10;\n }\n\n return (reversed == x);\n }\n}\n\n```\n```Python3 []\nclass Solution:\n def isPalindrome(self, x: int) -> bool:\n if x < 0:\n return False\n\n reversed_num = 0\n temp = x\n\n while temp != 0:\n digit = temp % 10\n reversed_num = reversed_num * 10 + digit\n temp //= 10\n\n return reversed_num == x\n\n```\n\n# Approach 2: Reversing Half of the Number\nInstead of reversing the entire number, we can reverse only the last half of the number. This approach is tricky because when we reverse the last half of the number, we don\'t want the middle digit to be reversed back to its original value. This can happen if the number has an odd number of digits. To resolve this, we can compare the first half of the number with the reversed second half of the number.\n# Explanation:\n1. We begin with an initial check to handle special cases:\n - If the input number `x` is negative, it cannot be a palindrome since palindromes are typically defined for positive numbers. In such cases, we immediately return `false`.\n - If `x` is non-zero and ends with a zero, it cannot be a palindrome because leading zeros are not allowed in palindromes. We return `false` for such cases.\n\n2. We initialize two variables:\n - `reversed`: This variable will store the reversed second half of the digits of the number.\n - `temp`: This variable is a temporary placeholder to manipulate the input number without modifying the original value.\n\n3. We enter a loop that continues until the first half of the digits (`x`) becomes less than or equal to the reversed second half (`reversed`):\n - Inside the loop, we extract the last digit of `x` using the modulo operator `%` and add it to the `reversed` variable after multiplying it by 10 (shifting the existing digits to the left).\n - We then divide `x` by 10 to remove the last digit and move towards the center of the number.\n\n4. Once the loop is completed, we have reversed the second half of the digits. Now, we compare the first half of the digits (`x`) with the reversed second half (`reversed`) to determine if the number is a palindrome:\n - For an even number of digits, if `x` is equal to `reversed`, then the number is a palindrome. We return `true`.\n - For an odd number of digits, if `x` is equal to `reversed / 10` (ignoring the middle digit), then the number is a palindrome. We return `true`.\n - If none of the above conditions are met, it means the number is not a palindrome, so we return `false`.\n\nThe code avoids the need for reversing the entire number by comparing only the necessary parts. This approach reduces both time complexity and memory usage, resulting in a more efficient solution.\n\n# Code\n\n```C++ []\nclass Solution {\npublic:\n bool isPalindrome(int x) {\n if (x < 0 || (x != 0 && x % 10 == 0)) {\n return false;\n }\n\n int reversed = 0;\n while (x > reversed) {\n reversed = reversed * 10 + x % 10;\n x /= 10;\n }\n return (x == reversed) || (x == reversed / 10);\n }\n};\n```\n```Java []\nclass Solution {\n public boolean isPalindrome(int x) {\n if (x < 0 || (x != 0 && x % 10 == 0)) {\n return false;\n }\n\n int reversed = 0;\n int original = x;\n\n while (x > reversed) {\n reversed = reversed * 10 + x % 10;\n x /= 10;\n }\n\n return (x == reversed) || (x == reversed / 10);\n }\n}\n```\n```Python3 []\nclass Solution:\n def isPalindrome(self, x: int) -> bool:\n if x < 0 or (x != 0 and x % 10 == 0):\n return False\n\n reversed_num = 0\n original = x\n\n while x > reversed_num:\n reversed_num = reversed_num * 10 + x % 10\n x //= 10\n\n return x == reversed_num or x == reversed_num // 10\n```\n\n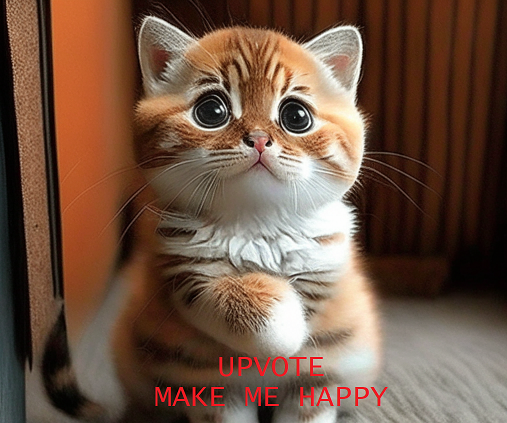\n\n**If you are a beginner solve these problems which makes concepts clear for future coding:**\n1. [Two Sum](https://leetcode.com/problems/two-sum/solutions/3619262/3-method-s-c-java-python-beginner-friendly/)\n2. [Roman to Integer](https://leetcode.com/problems/roman-to-integer/solutions/3651672/best-method-c-java-python-beginner-friendly/)\n3. [Palindrome Number](https://leetcode.com/problems/palindrome-number/solutions/3651712/2-method-s-c-java-python-beginner-friendly/)\n4. [Maximum Subarray](https://leetcode.com/problems/maximum-subarray/solutions/3666304/beats-100-c-java-python-beginner-friendly/)\n5. [Remove Element](https://leetcode.com/problems/remove-element/solutions/3670940/best-100-c-java-python-beginner-friendly/)\n6. [Contains Duplicate](https://leetcode.com/problems/contains-duplicate/solutions/3672475/4-method-s-c-java-python-beginner-friendly/)\n7. [Add Two Numbers](https://leetcode.com/problems/add-two-numbers/solutions/3675747/beats-100-c-java-python-beginner-friendly/)\n8. [Majority Element](https://leetcode.com/problems/majority-element/solutions/3676530/3-methods-beats-100-c-java-python-beginner-friendly/)\n9. [Remove Duplicates from Sorted Array](https://leetcode.com/problems/remove-duplicates-from-sorted-array/solutions/3676877/best-method-100-c-java-python-beginner-friendly/)\n10. **Practice them in a row for better understanding and please Upvote for more questions.**\n\n\n\n**If you found my solution helpful, I would greatly appreciate your upvote, as it would motivate me to continue sharing more solutions.**\n\n | 766 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
Simplest Python3 solution.!! | palindrome-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThis is the simplest solution.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nJust reverse the string and compare it.\n\n# Complexity\n- Time complexity:\nO(1)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isPalindrome(self, x: int) -> bool:\n x = str(x)\n rev = x[::-1]\n return x == rev\n``` | 2 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
[VIDEO] Visualization of Solution Using Modulo and Floor Division | palindrome-number | 0 | 1 | ERROR: type should be string, got "https://www.youtube.com/watch?v=OlTk8wM48ww\\n\\nThe idea here is that we don\\'t need to reverse all the digits, only <i>half</i> the digits. The first line checks some edge cases, and returns False immediately if the number is negative or ends with a 0 (with the exception of the number 0 itself). The loop then uses the modulo and floor division operators to reverse the digits and transfer them to the `half` variable.\\n\\nOnce the halfway point is reached, we return True if the two halves are equal to each other. If the number originally had an <i>odd</i> number of digits, then the two halves will be off by 1 digit, so we also remove that digit using floor division, then compare for equality.\\n# Code\\n```\\nclass Solution(object):\\n def isPalindrome(self, x):\\n if x < 0 or (x != 0 and x % 10 == 0):\\n return False\\n\\n half = 0\\n while x > half:\\n half = (half * 10) + (x % 10)\\n x = x // 10\\n\\n return x == half or x == half // 10\\n```\\n\\n# Alternate solution\\n```\\nclass Solution(object):\\n def isPalindrome(self, x):\\n x = str(x)\\n return x == x[::-1]\\n```\\nAlternate solution: turn the number into a string, reverse it, and see if they\\'re equal. This is the simplest solution, but the question does challenge us to solve it <i>without</i> turning the number into a string.\\n" | 11 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
Python 3 -> 1 solution is 89.20% faster. 2nd is 99.14% faster. Explanation added | palindrome-number | 0 | 1 | **Suggestions to make them better are always welcomed.**\n\n**Solution 1: 89.20% faster**\nThis is the easiest way to check if integer is palindrome. \n\nConvert the number to string and compare it with the reversed string.\n\nI wrote this working solution first and then found in the description that we need to solve this problem without converting the input to string. Then I wrote solution 2.\n```\ndef isPalindrome(self, x: int) -> bool:\n\tif x < 0:\n\t\treturn False\n\t\n\treturn str(x) == str(x)[::-1]\n```\n\nIf we don\'t want to convert the number to string, then recreate a new number in reverse order.\n```\ndef isPalindrome(self, x: int) -> bool:\n\tif x<0:\n\t\treturn False\n\n\tinputNum = x\n\tnewNum = 0\n\twhile x>0:\n\t\tnewNum = newNum * 10 + x%10\n\t\tx = x//10\n\treturn newNum == inputNum\n```\n\n**Solution 2: 99.14% faster.**\nI\'d recommend you to solve leetcode question 7 (reverse integer) to understand the logic behind this solution.\n\nPython3 int type has no lower or upper bounds. But if there are constraints given then we have to make sure that while reversing the integer we don\'t cross those constraints.\n\nSo, instead of reversing the whole integer, let\'s convert half of the integer and then check if it\'s palindrome.\nBut we don\'t know when is that half going to come. \n\nExample, if x = 15951, then let\'s create reverse of x in loop. Initially, x = 15951, revX = 0\n1. x = 1595, revX = 1\n2. x = 159, revX = 15\n3. x = 15, revX = 159\n\nWe see that revX > x after 3 loops and we crossed the half way in the integer bcoz it\'s an odd length integer.\nIf it\'s an even length integer, our loop stops exactly in the middle.\n\nNow we can compare x and revX, if even length, or x and revX//10 if odd length and return True if they match.\n\nThere\'s a difference between / and // division in Python3. Read it here on [stackoverflow](https://stackoverflow.com/questions/183853/what-is-the-difference-between-and-when-used-for-division).\n```\ndef isPalindrome(self, x: int) -> bool:\n\tif x < 0 or (x > 0 and x%10 == 0): # if x is negative, return False. if x is positive and last digit is 0, that also cannot form a palindrome, return False.\n\t\treturn False\n\t\n\tresult = 0\n\twhile x > result:\n\t\tresult = result * 10 + x % 10\n\t\tx = x // 10\n\t\t\n\treturn True if (x == result or x == result // 10) else False\n```\n\n **If you like the solutions, please upvote it for better reach to other people.** | 656 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
🔥🔥🔥Python Eazy to understand beat 95% and simple 🔥🔥🔥🔥🚀 | palindrome-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isPalindrome(self, x: int) -> bool:\n #Stringfy for to be able to reverse easily\n rev=str(x)\n # Comparsion and \n if rev == rev[::-1]:\n return True \n else:\n return False \n\n\n``` | 2 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
🚀C++ || Java || Python || Two Methods || Explained Intuition🚀 | palindrome-number | 1 | 1 | # Problem Description\n**Given** an integer x, return **true** if x is a **palindrome**, and **false** otherwise.\n\n**Palindrome** is a **word**, **phrase**, **number**, or **other sequence of characters** that **reads** the **same** **forward** and **backward**. In simpler terms, it\'s something that `remains unchanged when its order is reversed`.\n\n- Here are a few **examples** of palindromes:\n - Words :"racecar", "madam"\n - Numbers: 121, 1331, 12321\n\n- **Constraints:**\n - `-2e31 <= x <= 2e31 - 1`\n\n---\n# Intuition\n## First Approach (Convert to String)\nThe **first** approach is to **convert our number into a string** and there we can **access** any digit **freely** unlike the integer that we can\'t do that there.\nthen we can make a **mirror** around the middle of the string and **compare** the first char with last char, the second with the one before last and so on.\nif there is a **difference** then return false otherwise return true. \n\n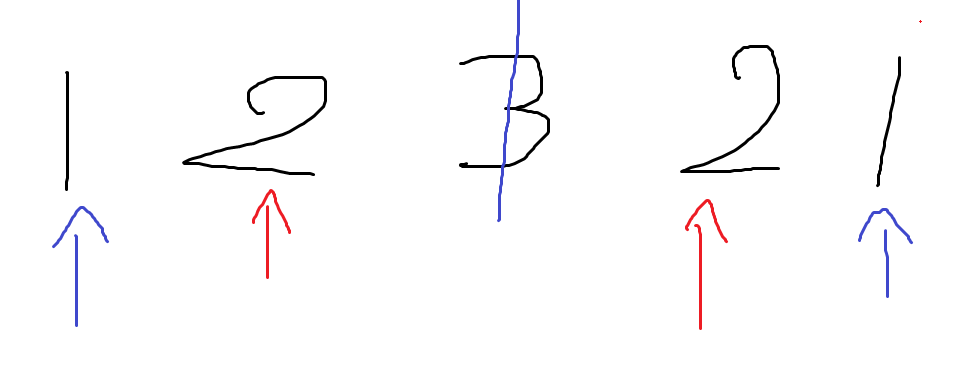\n\n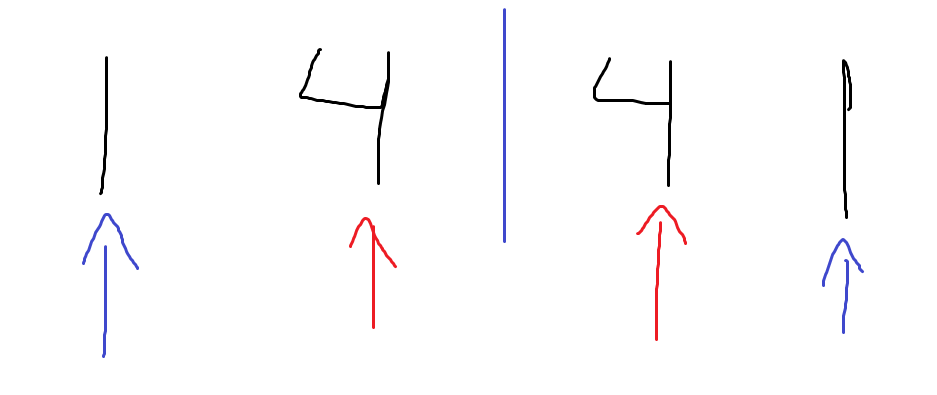\n\n\n## Second Approach (Extract Digits using %)\n\n**Modulo operation** is is a mathematical operation that returns the remainder when one number is divided by another.\n- 7 % 3 = 1\n- 9 % 5 = 4\n\nModulo operation is very helpful in our problem since it helps us to **extract the least digit in our number**.\nThe solution is **extract** the digit from the original number then **put it as least digit** in our new reversed number then **delete** that digit by dividing by 10.\n\n**Example : 121**\n```\nnumber = 121 , reversed = 0 \ndigit = 121 % 10 = 1 \nreversed = 0 * 10 + 1 = 1\nnumber = 121 / 10 = 12\n```\n```\nnumber = 12 , reversed = 1 \ndigit = 12 % 10 = 2 \nreversed = 1 * 10 + 2 = 2\nnumber = 12 / 10 = 1\n```\n```\nnumber = 1 , reversed = 12\ndigit = 1 % 10 = 1 \nreversed = 12 * 10 + 1 = 121\nnumber = 1 / 10 = 0\n```\nWe can see that reversed number **equal** to original number which means it is palindrome.\n\n\n---\n\n\n# Approach\n## First Approach (Convert to String)\n- **Convert** the integer x to a string representation.\n- Determine the **length** of the string.\n- Set up a for **loop** that iterates from the beginning of the string (index 0) to halfway through the string (length / 2).\n- Inside the loop, **compare** the character at the **current** position (number[i]) with the character at the **corresponding** position from the end of the string (number[length - i - 1]).\n- If the characters **do not match** (i.e., the number is not palindrome from the center outward), return **false** immediately, indicating that it\'s not a palindrome.\n- If the **loop** **completes** without finding any mismatches (all digits match), return **true**, indicating that the integer is a palindrome.\n\n# Complexity\n- **Time complexity:**$$O(N)$$\nSince we are iterating over all the digits.\n- **Space complexity:**$$O(N)$$\nSince we are storing the number as a string where `N` here is the number of the digits.\n---\n## Second Approach (Extract Digits using %)\n- Create **two long variables**, number and reversed, both initially set to the value of the input integer x. The number variable stores the **original** number, and reversed will store the **number in reverse order**.\n- Enter a while **loop** that continues as long as the value of x is greater than 0.\n- Inside the **loop**:\n - Calculate the **last digit of x** by taking the remainder of x when divided by 10 **(x % 10)**. This digit is stored in the digit variable.\n - **Update the reversed variable** by multiplying it by 10 (shifting digits left) and then adding the digit to it. This effectively builds the reversed number digit by digit.\n - **Remove the last digit** from x by dividing it by 10 (x /= 10).\n- After the loop completes, all digits of x have been processed and reversed in reversed.\n- Check if the number (the original input) is **equal** to the reversed (the reversed input). If they are equal, return **true**, indicating that the integer is a palindrome. If they are not equal, return **false**.\n\n# Complexity\n- **Time complexity:**$$O(N)$$\nSince we are iterating over all the digits.\n- **Space complexity:**$$O(1)$$\nWe only store two additional variables.\n\n# Code\n## First Approach (Convert to String)\n```C++ []\nclass Solution {\npublic:\n bool isPalindrome(int x) {\n string number = to_string(x) ;\n int length = number.size() ;\n for(int i = 0 ; i < length / 2 ; i ++){\n if(number[i] != number[length - i - 1])\n return false ;\n }\n return true;\n }\n};\n```\n```Java []\nclass Solution {\n public boolean isPalindrome(int x) {\n String number = Integer.toString(x);\n int length = number.length();\n for (int i = 0; i < length / 2; i++) {\n if (number.charAt(i) != number.charAt(length - i - 1)) {\n return false;\n }\n }\n return true;\n }\n}\n```\n```Python []\nclass Solution:\n def isPalindrome(self, x: int) -> bool:\n number = str(x)\n length = len(number)\n \n for i in range(length // 2):\n if number[i] != number[length - i - 1]:\n return False\n \n return True\n```\n## Second Approach (Extract Digits using %)\n```C++ []\nclass Solution {\npublic:\n bool isPalindrome(int x) {\n long long number = x, reversed = 0;\n while(x > 0){\n int digit = x % 10 ;\n reversed = (reversed * 10) + digit ; \n x /= 10 ;\n }\n return number == reversed ;\n }\n};\n```\n```Java []\nclass Solution {\n public boolean isPalindrome(int x) {\n long number = x;\n long reversed = 0;\n \n while (x > 0) {\n int digit = x % 10;\n reversed = (reversed * 10) + digit;\n x /= 10;\n }\n return number == reversed;\n }\n}\n```\n```Python []\nclass Solution:\n def isPalindrome(self, x: int) -> bool:\n number = x\n reversed_num = 0\n \n while x > 0:\n digit = x % 10\n reversed_num = (reversed_num * 10) + digit\n x //= 10\n \n return number == reversed_num\n```\n\n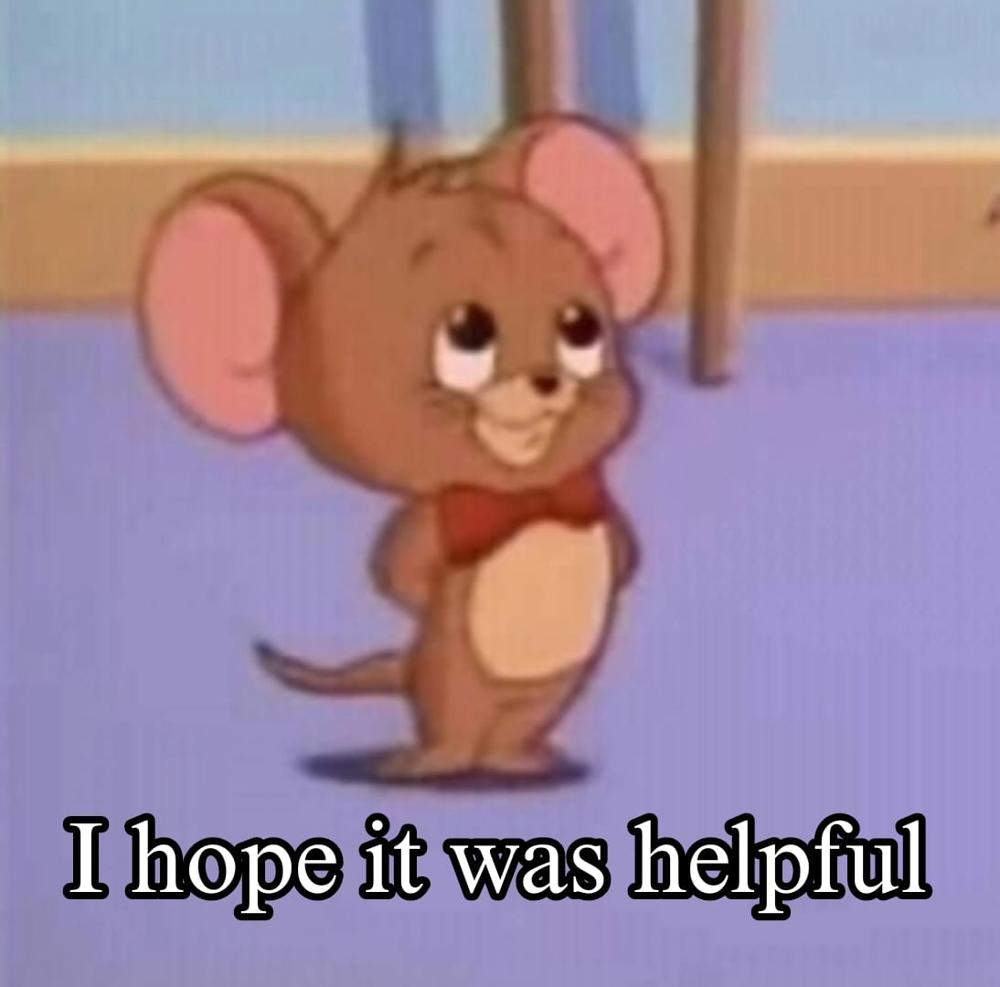\n\n\n\n | 16 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
✅ Beats 98.37% using Python 3 || Clean and Simply ✅ | palindrome-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. --> \nTo solve this problem, I considered the number of cases to account for and the potential subcases within each.\n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nI approached this problem by considering two cases: **positive** and **negative** integers. \n\nFor a **positive integer**, I converted it to a string and then compared it to its reversed form. To reverse the string, I used the join() method to convert the reversed list back into a string. If the two strings are the same then it enters the if statement and returns True, if not then it will return False by entering the else statement.\n\nFor a **negative integer**, I returned False in the else statement because a negative number cannot be a palindrome number.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n43 ms\nBeats 98.37% of users with Python3\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n16.06 MB\nBeats 95.31% of users with Python3\n# Code\n```\nclass Solution:\n def isPalindrome(self, x: int) -> bool:\n if x >= 0: \n if str(x) == \'\'.join(reversed(str(x))):\n return True\n else:\n return False\n\n\n\'\'\'\ncase 1: Positive integer\n - compare it with its reverse\n\ncase 2: Negative integer\n - always returns false\n\n\'\'\'\n``` | 0 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
this is it lol | palindrome-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isPalindrome(self, x: int) -> bool:\n \n return str(x) == str(x)[::-1]\n``` | 0 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
Amazing Awesome Solution | palindrome-number | 0 | 1 | Don\'t need False if there is True\n\n# Code\n```\nclass Solution:\n def isPalindrome(self, x: int) -> bool:\n\n if str(x) == str(x)[::-1]:\n return True\n``` | 1 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
✅DETAILED EXPLANATION | Beginner Friendly🔥🔥🔥 | PYTHON | | palindrome-number | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe given code is implementing a method isPalindrome in the Solution class. This method takes an integer x as input and checks whether it is a palindrome or not.\n\nHere\'s how the code works:\n\nThe method converts the integer x into a list of characters by first converting it to a string using str(x), and then using the list function to convert the string to a list. This is done for both a and b lists.\nThe variable a stores the list of characters representing the digits of x in the original order.\nThe variable b stores the list of characters representing the digits of x in the reversed order. This is achieved by using the reverse method on the b list.\nThe code then checks if the lists a and b are equal using the == operator.\nFinally, the method returns True if a is equal to b, indicating that the original number x is a palindrome. Otherwise, it returns False, indicating that x is not a palindrome.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nntuitively, the code converts the input number into two lists, a and b, where b is the reversed version of a. It then compares the two lists to check if they are equal. If they are equal, it means the original number is a palindrome.\n\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe time complexity of the code is O(d), where d is the number of digits in x. This is because the code converts x into a string and creates two lists, which take O(d) time complexity. The comparison of the two lists also takes O(d) time complexity.\n\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space complexity of the code is O(d) as well. This is because the code creates two lists, a and b, each containing d elements, where d is the number of digits in x.\n\n\n\n\n\n# Code\n```\nclass Solution:\n def isPalindrome(self, x: int) -> bool:\n a=list(str(x))\n b=list(str(x))\n b.reverse()\n return True if a==b else False\n``` | 2 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
Python Integer Palindrome Checker | palindrome-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe can convert the integer to a string and check if the string is a palindrome by comparing it with its reverse.\n\nWe can see from the examples that negative numbers are not palindromes because they start with - and when reversed the - sign will come at the end which is invalid. Therefore, we only have to check for positive numbers.\n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Convert the integer x to a string.\n2. Check if the string is equal to its reverse (using slicing).\n3. If the string is equal to its reverse, return True, indicating that x is a palindrome; otherwise, return False.\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isPalindrome(self, x: int) -> bool:\n return str(x) == str(x)[::-1]\n \n``` | 3 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
Python simple one line solution | palindrome-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIntution is to check whether the given integer is a palindrome\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nwhat i have done here is convert the integer into list and using slicing notation reverse matched\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\no(n) is the time complexity of this code\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nspace complexity of this code is also o(n)\n# Code\n```\nclass Solution:\n def isPalindrome(self, x: int) -> bool:\n return True if list(str(x))==list(str(x))[::-1] else False\n \n``` | 5 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
Palindrome without using convertation to str | palindrome-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe hint was not to use convertation x to string , so I used tricks of division. \n\n# Approach\nWe start from the latest digit of initial number and build reverted number. Then check is real number equals to reverted and returns the result. \n\n# Complexity\n- Time complexity: O(n) \n*where n is length of x , we just go through all numbers of initial x \n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution(object):\n def isPalindrome(self, x):\n if x < 0:\n return False\n reversed_number = 0\n number = x\n while x > 0:\n digit = x % 10\n x = x // 10\n reversed_number = reversed_number * 10 + digit\n\n return number == reversed_number\n``` | 11 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
Python code just visit the code | palindrome-number | 0 | 1 | # Approach\n1. first we have to check weather it is x < 0 then return false.\n2. then we have to save one temp memory as copy i used u can use your own variables. \n3. we use the while for loop to get result, for that take result with default value as 0.\n4. In while loop first we have to find reminder using (x%10),then we find result by using (result* + rem) in that we store the result values, then x//10 floor divition to get remaining values.\n5. After that in return copy == result it gives wheather condition is true or false.\n\n# Code\n```\nclass Solution:\n def isPalindrome(self, x: int) -> bool:\n if x < 0:\n return False\n copy = x\n result = 0 \n while x != 0:\n rem = x%10\n result = result*10 + rem\n x //= 10\n return copy == result\n\n``` | 2 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
Easy way to solve the Palindrome in Python3 | palindrome-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isPalindrome(self, x: int) -> bool:\n \n if str(x) == str(x)[::-1]:\n return True\n else:\n return False\n``` | 2 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
✅💡100% Acceptance Rate with ❣️Easy and Detailed Explanation - O(log10(x)) solution 💡😄 | palindrome-number | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nTo check if an integer is a palindrome, we reverse the integer and compare it with the original number. If they are the same, the number is a palindrome. However, we need to handle some special cases like negative numbers and numbers ending with zero.\n\n---\n# Do upvote if you like the solution and explanation \uD83D\uDCA1\uD83D\uDE04\n\n---\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. If the input number x is negative or ends with a zero (except when x is 0 itself), it cannot be a palindrome. In such cases, we return false.\n\n2. We initialize a variable reversed to 0. We use a while loop to reverse the second half of the integer x. In each iteration of the loop:\n\n3. Multiply reversed by 10 to shift its digits to the left.\nAdd the last digit of x to reversed (obtained using x % 10).\nDivide x by 10 to remove the last digit.\n- After the loop, we check if x is equal to reversed or if x is equal to reversed / 10. This step handles both even and odd-length palindromes. If the condition is true, we return true, indicating that x is a palindrome; otherwise, we return false.\n\n---\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe time complexity of this code is O(log10(x)), where log10(x) is the number of digits in x. In the worst case, the while loop runs for half of the digits in x, so the time complexity is proportional to the number of digits.\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space complexity of this code is O(1) because it uses a fixed amount of extra space regardless of the input x.\n\n---\n\n# \uD83D\uDCA1"If you have journeyed this far, I would kindly beseech you to consider upvoting this solution, thereby facilitating its reach to a wider audience."\u2763\uFE0F\uD83D\uDCA1\n\n---\n\n\n# Code\n```\nclass Solution {\npublic:\n bool isPalindrome(int x) {\n // If x is negative or ends with 0 but is not 0 itself, it cannot be a palindrome.\n if (x < 0 || (x % 10 == 0 && x != 0)) {\n return false;\n }\n\n int reversed = 0;\n while (x > reversed) {\n reversed = reversed * 10 + x % 10;\n x /= 10;\n }\n\n // When the length of the remaining number is odd, we can remove the middle digit.\n // For example, in the number 12321, we can remove the middle \'3\'.\n return x == reversed || x == reversed / 10;\n }\n};\n\n``` | 6 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
Python one-line solution with explanation | palindrome-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nString has method to reverse\n# Approach\n<!-- Describe your approach to solving the problem. -->\nwith reversing it is possible to write in one line\n\'[::]\' this means print all, and this \'[::-1]\' reverses, it is mostly used with list in python \n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isPalindrome(self, x: int) -> bool:\n return True if str(x)==str(x)[::-1] else False | 39 | Given an integer `x`, return `true` _if_ `x` _is a_ _**palindrome**__, and_ `false` _otherwise_.
**Example 1:**
**Input:** x = 121
**Output:** true
**Explanation:** 121 reads as 121 from left to right and from right to left.
**Example 2:**
**Input:** x = -121
**Output:** false
**Explanation:** From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
**Example 3:**
**Input:** x = 10
**Output:** false
**Explanation:** Reads 01 from right to left. Therefore it is not a palindrome.
**Constraints:**
* `-231 <= x <= 231 - 1`
**Follow up:** Could you solve it without converting the integer to a string? | Beware of overflow when you reverse the integer. |
Solution | regular-expression-matching | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n bool isMatch(string s, string p) {\n int n = s.length(), m = p.length();\n bool dp[n+1][m+1];\n memset(dp, false, sizeof(dp));\n dp[0][0] = true;\n \n for(int i=0; i<=n; i++){\n for(int j=1; j<=m; j++){\n if(p[j-1] == \'*\'){\n dp[i][j] = dp[i][j-2] || (i > 0 && (s[i-1] == p[j-2] || p[j-2] == \'.\') && dp[i-1][j]);\n }\n else{\n dp[i][j] = i > 0 && dp[i-1][j-1] && (s[i-1] == p[j-1] || p[j-1] == \'.\');\n }\n }\n }\n \n return dp[n][m];\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def isMatch(self, s: str, p: str) -> bool:\n i, j = len(s) - 1, len(p) - 1\n return self.backtrack({}, s, p, i, j)\n\n def backtrack(self, cache, s, p, i, j):\n key = (i, j)\n if key in cache:\n return cache[key]\n\n if i == -1 and j == -1:\n cache[key] = True\n return True\n\n if i != -1 and j == -1:\n cache[key] = False\n return cache[key]\n\n if i == -1 and p[j] == \'*\':\n k = j\n while k != -1 and p[k] == \'*\':\n k -= 2\n \n if k == -1:\n cache[key] = True\n return cache[key]\n \n cache[key] = False\n return cache[key]\n \n if i == -1 and p[j] != \'*\':\n cache[key] = False\n return cache[key]\n\n if p[j] == \'*\':\n if self.backtrack(cache, s, p, i, j - 2):\n cache[key] = True\n return cache[key]\n \n if p[j - 1] == s[i] or p[j - 1] == \'.\':\n if self.backtrack(cache, s, p, i - 1, j):\n cache[key] = True\n return cache[key]\n \n if p[j] == \'.\' or s[i] == p[j]:\n if self.backtrack(cache, s, p, i - 1, j - 1):\n cache[key] = True\n return cache[key]\n\n cache[key] = False\n return cache[key]\n```\n\n```Java []\nenum Result {\n TRUE, FALSE\n}\n\nclass Solution {\n Result[][] memo;\n\n public boolean isMatch(String text, String pattern) {\n memo = new Result[text.length() + 1][pattern.length() + 1];\n return dp(0, 0, text, pattern);\n }\n\n public boolean dp(int i, int j, String text, String pattern) {\n if (memo[i][j] != null) {\n return memo[i][j] == Result.TRUE;\n }\n boolean ans;\n if (j == pattern.length()){\n ans = i == text.length();\n } else{\n boolean first_match = (i < text.length() &&\n (pattern.charAt(j) == text.charAt(i) ||\n pattern.charAt(j) == \'.\'));\n\n if (j + 1 < pattern.length() && pattern.charAt(j+1) == \'*\'){\n ans = (dp(i, j+2, text, pattern) ||\n first_match && dp(i+1, j, text, pattern));\n } else {\n ans = first_match && dp(i+1, j+1, text, pattern);\n }\n }\n memo[i][j] = ans ? Result.TRUE : Result.FALSE;\n return ans;\n }\n}\n```\n | 461 | Given an input string `s` and a pattern `p`, implement regular expression matching with support for `'.'` and `'*'` where:
* `'.'` Matches any single character.
* `'*'` Matches zero or more of the preceding element.
The matching should cover the **entire** input string (not partial).
**Example 1:**
**Input:** s = "aa ", p = "a "
**Output:** false
**Explanation:** "a " does not match the entire string "aa ".
**Example 2:**
**Input:** s = "aa ", p = "a\* "
**Output:** true
**Explanation:** '\*' means zero or more of the preceding element, 'a'. Therefore, by repeating 'a' once, it becomes "aa ".
**Example 3:**
**Input:** s = "ab ", p = ".\* "
**Output:** true
**Explanation:** ".\* " means "zero or more (\*) of any character (.) ".
**Constraints:**
* `1 <= s.length <= 20`
* `1 <= p.length <= 20`
* `s` contains only lowercase English letters.
* `p` contains only lowercase English letters, `'.'`, and `'*'`.
* It is guaranteed for each appearance of the character `'*'`, there will be a previous valid character to match. | null |
Beats 99% Easy Solution | regular-expression-matching | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isMatch(self, s: str, p: str) -> bool:\n memo = {}\n\n def backtrack(i, j):\n if (i, j) in memo:\n return memo[(i, j)]\n\n if j == -1:\n result = i == -1\n elif i == -1:\n result = j > 0 and p[j] == \'*\' and backtrack(i, j - 2)\n elif p[j] == \'*\':\n result = (backtrack(i, j - 2) or\n (p[j - 1] == s[i] or p[j - 1] == \'.\') and backtrack(i - 1, j))\n else:\n result = (p[j] == \'.\' or s[i] == p[j]) and backtrack(i - 1, j - 1)\n\n memo[(i, j)] = result\n return result\n\n return backtrack(len(s) - 1, len(p) - 1)\n\n``` | 2 | Given an input string `s` and a pattern `p`, implement regular expression matching with support for `'.'` and `'*'` where:
* `'.'` Matches any single character.
* `'*'` Matches zero or more of the preceding element.
The matching should cover the **entire** input string (not partial).
**Example 1:**
**Input:** s = "aa ", p = "a "
**Output:** false
**Explanation:** "a " does not match the entire string "aa ".
**Example 2:**
**Input:** s = "aa ", p = "a\* "
**Output:** true
**Explanation:** '\*' means zero or more of the preceding element, 'a'. Therefore, by repeating 'a' once, it becomes "aa ".
**Example 3:**
**Input:** s = "ab ", p = ".\* "
**Output:** true
**Explanation:** ".\* " means "zero or more (\*) of any character (.) ".
**Constraints:**
* `1 <= s.length <= 20`
* `1 <= p.length <= 20`
* `s` contains only lowercase English letters.
* `p` contains only lowercase English letters, `'.'`, and `'*'`.
* It is guaranteed for each appearance of the character `'*'`, there will be a previous valid character to match. | null |
[ Beats 98% ] Regular Expression Matching using Dynamic Programming in Python | regular-expression-matching | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThis problem can be solved by using dynamic programming. We can define dp(i, j) as the boolean value indicating whether the substring s[i:] matches the pattern p[j:].\n# Approach\n<!-- Describe your approach to solving the problem. -->\nWe can solve the problem by defining a recursive function that tries to match s[i:] with p[j:] for all possible i and j indices. The function should return true if and only if it matches the entire string.\n\nTo avoid redundant computation, we can use memoization to store the previously computed results of dp(i, j) in a memo dictionary. The recursive function first checks if the result is already computed in the memo dictionary and returns it if so.\n\nIf j reaches the end of p, the function returns true if and only if i also reaches the end of s.\n\nIf the next character in p is followed by a \'\', the function can match zero or more occurrences of the preceding character. The function recursively tries two cases: either skip the preceding character and the \'\', or match the preceding character and recursively try to match the remaining part of s and p. The function returns true if either case succeeds.\n\nOtherwise, the function simply checks if the next character in p matches the next character in s or is a dot character (\'.\') that matches any character. If so, the function recursively tries to match the remaining part of s and p. The function returns true if both cases succeed.\n# Complexity\n- Time complexity: O(SP), where S is the length of the string s and P is the length of the pattern p. The function tries to match each character in s with each character in p at most once and uses memoization to avoid redundant computation.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(SP), where S is the length of the string s and P is the length of the pattern p. The function uses memoization to store the previously computed results of dp(i, j).\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isMatch(self, s: str, p: str) -> bool:\n memo = {}\n \n def dp(i: int, j: int) -> bool:\n if (i, j) in memo:\n return memo[(i, j)]\n \n if j == len(p):\n return i == len(s)\n \n first_match = i < len(s) and (p[j] == s[i] or p[j] == \'.\')\n \n if j + 1 < len(p) and p[j+1] == \'*\':\n ans = dp(i, j+2) or (first_match and dp(i+1, j))\n else:\n ans = first_match and dp(i+1, j+1)\n \n memo[(i, j)] = ans\n return ans\n \n return dp(0, 0)\n``` | 17 | Given an input string `s` and a pattern `p`, implement regular expression matching with support for `'.'` and `'*'` where:
* `'.'` Matches any single character.
* `'*'` Matches zero or more of the preceding element.
The matching should cover the **entire** input string (not partial).
**Example 1:**
**Input:** s = "aa ", p = "a "
**Output:** false
**Explanation:** "a " does not match the entire string "aa ".
**Example 2:**
**Input:** s = "aa ", p = "a\* "
**Output:** true
**Explanation:** '\*' means zero or more of the preceding element, 'a'. Therefore, by repeating 'a' once, it becomes "aa ".
**Example 3:**
**Input:** s = "ab ", p = ".\* "
**Output:** true
**Explanation:** ".\* " means "zero or more (\*) of any character (.) ".
**Constraints:**
* `1 <= s.length <= 20`
* `1 <= p.length <= 20`
* `s` contains only lowercase English letters.
* `p` contains only lowercase English letters, `'.'`, and `'*'`.
* It is guaranteed for each appearance of the character `'*'`, there will be a previous valid character to match. | null |
Python solution | regular-expression-matching | 0 | 1 | \n\n# Code\n```\nclass Solution:\n\n def func(self, i,j) :\n\n if self.dp[i][j] != -1 :\n return self.dp[i][j]\n\n if i==len(self.s) and j==len(self.p) :\n return True \n if i>=len(self.s):\n \n while(j<len(self.p)-1) :\n if self.p[j+1] == "*" :\n j += 2 \n else :\n return False \n if j >= len(self.p) : \n return True \n return False\n \n if j>=len(self.p) :\n return False\n \n a = False\n b = False\n \n if j<len(self.p)-1 :\n if self.p[j+1] == "*" :\n if self.s[i] == self.p[j] :\n a = self.func(i+1,j)\n elif self.p[j] == "." :\n a = self.func(i+1,j)\n b = self.func(i , j+2) \n elif self.p[j] == "." :\n a = self.func(i+1 , j+1) \n else :\n if self.p[j] == self.s[i] :\n a = self.func(i+1,j+1)\n else :\n return False\n elif self.p[j] == "." :\n a = self.func(i+1 , j+1) \n else :\n if self.p[j] == self.s[i] :\n a = self.func(i+1,j+1)\n else :\n return False \n self.dp[i][j] = a or b\n return a or b\n \n def isMatch(self, s: str, p: str) -> bool:\n self.s = s \n self.p = p \n self.dp = [[-1 for i in range(len(p)+1)] for j in range(len(s)+1)]\n return self.func(0,0)\n \n``` | 1 | Given an input string `s` and a pattern `p`, implement regular expression matching with support for `'.'` and `'*'` where:
* `'.'` Matches any single character.
* `'*'` Matches zero or more of the preceding element.
The matching should cover the **entire** input string (not partial).
**Example 1:**
**Input:** s = "aa ", p = "a "
**Output:** false
**Explanation:** "a " does not match the entire string "aa ".
**Example 2:**
**Input:** s = "aa ", p = "a\* "
**Output:** true
**Explanation:** '\*' means zero or more of the preceding element, 'a'. Therefore, by repeating 'a' once, it becomes "aa ".
**Example 3:**
**Input:** s = "ab ", p = ".\* "
**Output:** true
**Explanation:** ".\* " means "zero or more (\*) of any character (.) ".
**Constraints:**
* `1 <= s.length <= 20`
* `1 <= p.length <= 20`
* `s` contains only lowercase English letters.
* `p` contains only lowercase English letters, `'.'`, and `'*'`.
* It is guaranteed for each appearance of the character `'*'`, there will be a previous valid character to match. | null |
[0ms][1LINER][100%][Fastest Solution Explained] O(n) time complexity | O(n) space complexity | regular-expression-matching | 1 | 1 | (Note: This is part of a series of Leetcode solution explanations. If you like this solution or find it useful, ***please upvote*** this post.)\n***Take care brother, peace, love!***\n\n```\n```\n\nThe best result for the code below is ***0ms / 38.2MB*** (beats 92.04% / 24.00%).\n* *** Python ***\n\n```\n\n def isMatch(self, s: str, p: str) -> bool:\n s, p = \' \'+ s, \' \'+ p\n lenS, lenP = len(s), len(p)\n dp = [[0]*(lenP) for i in range(lenS)]\n dp[0][0] = 1\n\n for j in range(1, lenP):\n if p[j] == \'*\':\n dp[0][j] = dp[0][j-2]\n\n for i in range(1, lenS):\n for j in range(1, lenP):\n if p[j] in {s[i], \'.\'}:\n dp[i][j] = dp[i-1][j-1]\n elif p[j] == "*":\n dp[i][j] = dp[i][j-2] or int(dp[i-1][j] and p[j-1] in {s[i], \'.\'})\n\n return bool(dp[-1][-1])\n\n```\n\n```\n```\n\n```\n```\n***"We are Anonymous. We are legion. We do not forgive. We do not forget. Expect us. Open your eyes.." - \uD835\uDCD0\uD835\uDCF7\uD835\uDCF8\uD835\uDCF7\uD835\uDD02\uD835\uDCF6\uD835\uDCF8\uD835\uDCFE\uD835\uDCFC*** | 19 | Given an input string `s` and a pattern `p`, implement regular expression matching with support for `'.'` and `'*'` where:
* `'.'` Matches any single character.
* `'*'` Matches zero or more of the preceding element.
The matching should cover the **entire** input string (not partial).
**Example 1:**
**Input:** s = "aa ", p = "a "
**Output:** false
**Explanation:** "a " does not match the entire string "aa ".
**Example 2:**
**Input:** s = "aa ", p = "a\* "
**Output:** true
**Explanation:** '\*' means zero or more of the preceding element, 'a'. Therefore, by repeating 'a' once, it becomes "aa ".
**Example 3:**
**Input:** s = "ab ", p = ".\* "
**Output:** true
**Explanation:** ".\* " means "zero or more (\*) of any character (.) ".
**Constraints:**
* `1 <= s.length <= 20`
* `1 <= p.length <= 20`
* `s` contains only lowercase English letters.
* `p` contains only lowercase English letters, `'.'`, and `'*'`.
* It is guaranteed for each appearance of the character `'*'`, there will be a previous valid character to match. | null |
[Fastest Solution Explained][0ms][100%] O(n)time complexity O(n)space complexity | regular-expression-matching | 1 | 1 | \n(Note: This is part of a series of Leetcode solution explanations. If you like this solution or find it useful, ***please upvote*** this post.)\n***Take care brother, peace, love!***\n\n```\n```\n\nThe best result for the code below is ***0ms / 3.27MB*** (beats 99.04% / 90.42%).\n* *** Java ***\n\n```\n\npublic boolean isMatch(String s, String p) {\n\t\t/**\n\t\t * This solution is assuming s has no regular expressions.\n\t\t * \n\t\t * dp: res[i][j]=is s[0,...,i-1] matched with p[0,...,j-1];\n\t\t * \n\t\t * If p[j-1]!=\'*\', res[i][j] = res[i-1][j-1] &&\n\t\t * (s[i-1]==p[j-1]||p[j-1]==\'.\'). Otherwise, res[i][j] is true if\n\t\t * res[i][j-1] or res[i][j-2] or\n\t\t * res[i-1][j]&&(s[i-1]==p[j-2]||p[j-2]==\'.\'), and notice the third\n\t\t * \'or\' case includes the first \'or\'.\n\t\t * \n\t\t * \n\t\t * Boundaries: res[0][0]=true;//s=p="". res[i][0]=false, i>0.\n\t\t * res[0][j]=is p[0,...,j-1] empty, j>0, and so res[0][1]=false,\n\t\t * res[0][j]=p[j-1]==\'*\'&&res[0][j-2].\n\t\t * \n\t\t * O(n) space is enough to store a row of res.\n\t\t */\n\n\t\tint m = s.length(), n = p.length();\n\t\tboolean[] res = new boolean[n + 1];\n\t\tres[0] = true;\n\n\t\tint i, j;\n\t\tfor (j = 2; j <= n; j++)\n\t\t\tres[j] = res[j - 2] && p.charAt(j - 1) == \'*\';\n\n\t\tchar pc, sc, tc;\n\t\tboolean pre, cur; // pre=res[i - 1][j - 1], cur=res[i-1][j]\n\n\t\tfor (i = 1; i <= m; i++) {\n\t\t\tpre = res[0];\n\t\t\tres[0] = false;\n\t\t\tsc = s.charAt(i - 1);\n\n\t\t\tfor (j = 1; j <= n; j++) {\n\t\t\t\tcur = res[j];\n\t\t\t\tpc = p.charAt(j - 1);\n\t\t\t\tif (pc != \'*\')\n\t\t\t\t\tres[j] = pre && (sc == pc || pc == \'.\');\n\t\t\t\telse {\n\t\t\t\t\t// pc == \'*\' then it has a preceding char, i.e. j>1\n\t\t\t\t\ttc = p.charAt(j - 2);\n\t\t\t\t\tres[j] = res[j - 2] || (res[j] && (sc == tc || tc == \'.\'));\n\t\t\t\t}\n\t\t\t\tpre = cur;\n\t\t\t}\n\t\t}\n\n\t\treturn res[n];\n\t}\n\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***0ms / 10MB*** (beats 100.00% / 95.49%).\n* *** Python ***\n\n```\n\ncache = {}\ndef isMatch(self, s, p):\n if (s, p) in self.cache:\n return self.cache[(s, p)]\n if not p:\n return not s\n if p[-1] == \'*\':\n if self.isMatch(s, p[:-2]):\n self.cache[(s, p)] = True\n return True\n if s and (s[-1] == p[-2] or p[-2] == \'.\') and self.isMatch(s[:-1], p):\n self.cache[(s, p)] = True\n return True\n if s and (p[-1] == s[-1] or p[-1] == \'.\') and self.isMatch(s[:-1], p[:-1]):\n self.cache[(s, p)] = True\n return True\n self.cache[(s, p)] = False\n return False\n\n```\n\n```\ndef isMatch(self, s, p):\n dp = [[False] * (len(s) + 1) for _ in range(len(p) + 1)]\n dp[0][0] = True\n for i in range(1, len(p)):\n dp[i + 1][0] = dp[i - 1][0] and p[i] == \'*\'\n for i in range(len(p)):\n for j in range(len(s)):\n if p[i] == \'*\':\n dp[i + 1][j + 1] = dp[i - 1][j + 1] or dp[i][j + 1]\n if p[i - 1] == s[j] or p[i - 1] == \'.\':\n dp[i + 1][j + 1] |= dp[i + 1][j]\n else:\n dp[i + 1][j + 1] = dp[i][j] and (p[i] == s[j] or p[i] == \'.\')\n return dp[-1][-1]\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***0ms / 23.7MB*** (beats 59.24% / 60.42%).\n* *** C++ ***\n\n\nWe can solve the problem using recursion. We need the helper function to keep track of the current indices of the pattern and the string. The basic condition would be when pattern index reaches the end, then we will check if the string index has also reached the end or not.\n\nNow we will check if the pattern current index matches the string current index character, this would be true either when the characters are equal i.e. s[i]==p[j] or if the p[j]==\'.\' since \'.\' can be replaced by any character.\n\nIf the next pattern character is \'\' that means the current pattern character p[j] could occur 0 or infinite times. So, then there would be two possibility either we can take match the current pattern character with the string and move i by 1 or we can just take zero occurence of the current pattern character and move the pattern character by 2. We will apply the OR condition between these two conditions since if either of them matches then it solves our problem and if next pattern character is not \'\' , then we need to check if the current character matches or not and also move both string and pattern character by 1.\n\nThe time complexity of this brute force approach is O(3^(max(m,n)) and space complexity is O(max(m,n)) where m and n are the length of pattern and string respectively.\n\n```\nclass Solution {\npublic:\n bool isMatch(string s, string p) {\n return helper(s,p,0,0);\n }\n \n bool helper(string s, string p, int i, int j)\n {\n if(j==p.length())\n return i==s.length();\n bool first_match=(i<s.length() && (s[i]==p[j] || p[j]==\'.\' ));\n \n if(j+1<p.length() && p[j+1]==\'*\')\n {\n return (helper(s,p,i,j+2)|| (first_match && helper(s,p,i+1,j) ));\n }\n else\n {\n return (first_match && helper(s,p,i+1,j+1));\n }\n }\n};\n```\n\nWe are actually recomputing the solution for the same subproblems many times. So to avoid that we can initialize dp matrix with all values with being -1. Now if dp[i][j]>=0 then that means this has been already computed so we can return the results here only, thus, it saves time and we don\'t need to recompute that again. Notice that we are saving the results in dp[i][j] in the second last line and this result would always be positive either 0 or 1.\n\nThe time complexity is now O(mn) and space complexity is O(mn) where m and n are the length of pattern and string respectively.\n\n```\nclass Solution {\npublic:\n bool isMatch(string s, string p) {\n vector<vector<int>> dp(s.length()+1,vector<int>(p.length(),-1));\n return helper(s,p,0,0,dp);\n }\n \n bool helper(string s, string p, int i, int j,vector<vector<int>> &dp)\n {\n if(j==p.length())\n return i==s.length();\n if(dp[i][j]>=0)\n return dp[i][j];\n bool first_match=(i<s.length() && (s[i]==p[j] || p[j]==\'.\' ));\n bool ans=0;\n if(j+1<p.length() && p[j+1]==\'*\')\n {\n ans= (helper(s,p,i,j+2,dp)|| (first_match && helper(s,p,i+1,j,dp) ));\n }\n else\n {\n ans= (first_match && helper(s,p,i+1,j+1,dp));\n }\n dp[i][j]=ans;\n return ans;\n }\n};\n```\n\nBottom up solution\n\nWe can derive the bottom up solution from top down approach only. We will make the matrix of length (s.length()+1)* (p.length()+1) . dp[s.length()][p.length()]=1 since both have ended at that point.\n\n```\nclass Solution {\npublic:\n bool isMatch(string s, string p) {\n vector<vector<int>> dp(s.length()+1,vector<int>(p.length()+1,0));\n dp[s.length()][p.length()]=1;\n \n for(int i=s.length();i>=0;i--)\n {\n for(int j=p.length()-1;j>=0;j--)\n {\n bool first_match=(i<s.length() && (p[j]==s[i]|| p[j]==\'.\'));\n if(j+1<p.length() && p[j+1]==\'*\')\n {\n dp[i][j]=dp[i][j+2] || (first_match && dp[i+1][j]);\n }\n else\n {\n dp[i][j]=first_match && dp[i+1][j+1];\n }\n }\n }\n \n return dp[0][0];\n }\n};\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***0ms / 78MB*** (beats 100.00% / 100.00%).\n* *** JavaScript ***\n\n\n```\n\nfunction isMatch(s, p) {\n var lenS = s.length;\n var lenP = p.length;\n var map = {};\n\n return check(0, 0);\n\n function check(idxS, idxP) {\n if (map[idxS + \':\' + idxP] !== undefined) return map[idxS + \':\' + idxP];\n if (idxS > lenS) return false;\n if (idxS === lenS && idxP === lenP) return true;\n\n if (p[idxP] === \'.\' || p[idxP] === s[idxS]) {\n map[idxS + \':\' + idxP] = p[idxP + 1] === \'*\' ?\n check(idxS + 1, idxP) || check(idxS, idxP + 2) :\n check(idxS + 1, idxP + 1);\n } else {\n map[idxS + \':\' + idxP] = p[idxP + 1] === \'*\' ?\n check(idxS, idxP + 2) : false;\n }\n return map[idxS + \':\' + idxP];\n }\n}\n\n```\n\n```\nconst isMatch = (string, pattern) => {\n // early return when pattern is empty\n if (!pattern) {\n\t\t// returns true when string and pattern are empty\n\t\t// returns false when string contains chars with empty pattern\n return !string;\n }\n \n\t// check if the current char of the string and pattern match when the string has chars\n const hasFirstCharMatch = Boolean(string) && (pattern[0] === \'.\' || pattern[0] === string[0]);\n\n // track when the next character * is next in line in the pattern\n if (pattern[1] === \'*\') {\n // if next pattern match (after *) is fine with current string, then proceed with it (s, p+2). That\'s because the current pattern may be skipped.\n // otherwise check hasFirstCharMatch. That\'s because if we want to proceed with the current pattern, we must be sure that the current pattern char matches the char\n\t\t// If hasFirstCharMatch is true, then do the recursion with next char and current pattern (s+1, p). That\'s because current char matches the pattern char. \n return (\n isMatch(string, pattern.slice(2)) || \n (hasFirstCharMatch && isMatch(string.slice(1), pattern))\n );\n }\n \n // now we know for sure that we need to do 2 simple actions\n\t// check the current pattern and string chars\n\t// if so, then can proceed with next string and pattern chars (s+1, p+1)\n return hasFirstCharMatch ? isMatch(string.slice(1), pattern.slice(1)) : false;\n};\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***0ms / 15.23MB*** (beats 89.94% / 90.99%).\n* *** Python3 ***\n\n\nIf dp[i][j] == False, it means s[:i] doesn\'t match p[:j]\u2028If dp[i][j] == True, it means s[:i] matches p[:j]\u2028\'.\' Matches any single character.\u2028\'\' Matches zero or more of the preceding element.\u2028s = "aa"\u2028p = "a"\u2028Output: true\u2028Explanation: \'*\' means zero or more of the preceding element, \'a\'. Therefore, by repeating \'a\' once, it becomes "aa".\n\n```\ns = "aab"\np = "c*a*b"\n c * a * b\ndp = [ \n [True, False, True, False, True, False], \n\t a [False, False, False, True, True, False], \n\t a [False, False, False, False, True, False], \n\t b\t[False, False, False, False, False, True]] \n\t\n# Case 1 p[:j] == alphabet or \'.\'\n# Case 2: p[:j] is \'*\'\n\t# Case 2a: p[:j-1] doesn\'t need a \'*\' to match the substring s[:i]\n\t #1 p[:j-1] ==s[:i] dp[i][j] =d[i-1][j]\n\t\t 0 a * 0 a a \n 0 0\n a T ---> a\n a T a T\n\t\t#2 p[:j-1] == \'.\' dp[i][j]=dp[i][j-2]\n\t\t 0 a b *\n 0 \n a T T \n \n\t# Case 2b: p[:j] needs \'*\' to match the substring s[:i]\n```\n```\n # about *:\n case 1: 0 preceding element\n case 2: 1 or more preceding element\n\t\t\t the preceding element could be a \'.\' or an alpharbet.\n\t\t\t more proceding element is easy to handle once you use dynamic programming. \n ```\n ```\nclass Solution:\n def isMatch(self, s, p):\n n = len(s)\n m = len(p)\n dp = [[False for _ in range (m+1)] for _ in range (n+1)]\n dp[0][0] = True\n for c in range(1,m+1):\n if p[c-1] == \'*\' and c > 1:\n dp[0][c] = dp[0][c-2]\n for r in range(1,n+1):\n for c in range(1,m+1):\n if p[c-1] == s[r-1] or p[c-1] == \'.\':\n dp[r][c] = dp[r-1][c-1]\n elif c > 1 and p[c-1] == \'*\':\n if p[c-2] ==\'.\' or s[r-1]==p[c-2]:\n dp[r][c] =dp[r][c-2] or dp[r-1][c]\n else:\n dp[r][c] = dp[r][c-2]\n return dp[n][m]\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***0ms / 33.33MB*** (beats 99.00% / 60.12%).\n* *** Kotlin ***\n\n\n```\n\nclass Solution {\n fun isMatch(s: String, p: String): Boolean {\n return isMatchRecursive(s, p)\n }\n \n fun isMatchRecursive(s: String, p: String): Boolean {\n if (p == "") return s == ""\n \n if (p.length >= 2 && p[1] == \'*\' && s.length > 0 && (s[0] == p[0] || p[0] == \'.\')) {\n return isMatchRecursive(s.substring(1), p) || isMatchRecursive(s, p.substring(2))\n } else if (p.length >= 2 && p[1] == \'*\') {\n return isMatchRecursive(s, p.substring(2))\n }\n \n return if (s.length > 0) {\n if (s[0] == p[0] || p[0] == \'.\') {\n isMatchRecursive(s.substring(1), p.substring(1))\n } else {\n false\n }\n } else {\n false\n }\n }\n}\n\n```\n\n```\nclass Solution {\n fun isMatch(s: String, p: String): Boolean {\n return p.toRegex().matches(s)\n }\n}\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***0ms / 13.17MB*** (beats 79.34% / 99.92%).\n* *** Swift ***\n\n\n```\n\nclass Solution {\n func isMatch(_ s: String, _ p: String) -> Bool {\n \n var visit = [[Bool]]()\n let sLength = s.count, pCount = p.count\n \n for _ in 0...sLength + 1 {\n visit.append([Bool](repeating: false, count: pCount + 1))\n }\n \n visit[sLength][pCount] = true\n \n for i in stride(from: sLength, through: 0, by: -1) {\n for j in stride(from: pCount - 1, through: 0, by: -1) {\n \n let arrS = Array(s), arrP = Array(p)\n \n let first = i < sLength && (arrS[i] == arrP[j] || arrP[j] == ".")\n \n if j + 1 < pCount && arrP[j + 1] == "*" {\n visit[i][j] = visit[i][j + 2] || first && visit[i + 1][j]\n } else {\n visit[i][j] = first && visit[i + 1][j + 1]\n }\n }\n }\n return visit[0][0]\n }\n}\n\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***0ms / 62.07MB*** (beats 99.99% / 99.99%).\n* *** PHP ***\n\n\n```\nclass Solution {\n\n /**\n * @param String $s\n * @param String $p\n * @return Boolean\n */\n function isMatch($s, $p) {\n return preg_match("/^{$p}$/", $s);\n }\n}\n```\n\nHowever, that kinda defeats the point of the exercise, which is to show you can write a regex matcher. So here is my solution.\n```\nclass Solution {\n \n const WILDCARD = "*";\n const ANY_CHAR = ".";\n \n /**\n * @param String $string\n * @param String $pattern\n * @return Boolean\n */\n function isMatch($string, $pattern) \n {\n if (!$pattern) {\n return !$string;\n }\n \n $matchResult = $string && ($string[0] === $pattern[0] || self::ANY_CHAR === $pattern[0]);\n $greedyMatch = !empty($pattern[1]) && $pattern[1] == self::WILDCARD;\n\n if (!$matchResult && !$greedyMatch) {\n return false;\n }\n\n if ($greedyMatch) {\n return ($matchResult && $this->isMatch(substr($string, 1), $pattern)) || $this->isMatch($string, substr($pattern, 2));\n }\n \n return $matchResult && $this->isMatch(substr($string, 1), substr($pattern, 1));\n }\n}\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***0ms / 1.17MB*** (beats 99.64% / 99.92%).\n* *** C ***\n\n\n```\n\nbool match(char* s, char* p) {\n return (s[0] == p[0]) || (p[0] == \'.\' && s[0]);\n}\n\nbool isMatch(char * s, char * p){\n if (!p[0]) return !s[0];\n if (p[1] == \'*\') return isMatch(s, p+2) || match(s, p) && isMatch(s+1, p);\n if (p[0] == \'.\') return s[0] && isMatch(s+1, p+1);\n return match(s, p) && isMatch(s+1, p+1);\n}\n\n```\n\n```\n```\n\n```\n```\n\n***"Open your eyes. Expect us." - \uD835\uDCD0\uD835\uDCF7\uD835\uDCF8\uD835\uDCF7\uD835\uDD02\uD835\uDCF6\uD835\uDCF8\uD835\uDCFE\uD835\uDCFC***\n | 13 | Given an input string `s` and a pattern `p`, implement regular expression matching with support for `'.'` and `'*'` where:
* `'.'` Matches any single character.
* `'*'` Matches zero or more of the preceding element.
The matching should cover the **entire** input string (not partial).
**Example 1:**
**Input:** s = "aa ", p = "a "
**Output:** false
**Explanation:** "a " does not match the entire string "aa ".
**Example 2:**
**Input:** s = "aa ", p = "a\* "
**Output:** true
**Explanation:** '\*' means zero or more of the preceding element, 'a'. Therefore, by repeating 'a' once, it becomes "aa ".
**Example 3:**
**Input:** s = "ab ", p = ".\* "
**Output:** true
**Explanation:** ".\* " means "zero or more (\*) of any character (.) ".
**Constraints:**
* `1 <= s.length <= 20`
* `1 <= p.length <= 20`
* `s` contains only lowercase English letters.
* `p` contains only lowercase English letters, `'.'`, and `'*'`.
* It is guaranteed for each appearance of the character `'*'`, there will be a previous valid character to match. | null |
one-line python solution | regular-expression-matching | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isMatch(self, s: str, p: str) -> bool:\n return re.match(fr"^{p}$", s) is not None\n``` | 5 | Given an input string `s` and a pattern `p`, implement regular expression matching with support for `'.'` and `'*'` where:
* `'.'` Matches any single character.
* `'*'` Matches zero or more of the preceding element.
The matching should cover the **entire** input string (not partial).
**Example 1:**
**Input:** s = "aa ", p = "a "
**Output:** false
**Explanation:** "a " does not match the entire string "aa ".
**Example 2:**
**Input:** s = "aa ", p = "a\* "
**Output:** true
**Explanation:** '\*' means zero or more of the preceding element, 'a'. Therefore, by repeating 'a' once, it becomes "aa ".
**Example 3:**
**Input:** s = "ab ", p = ".\* "
**Output:** true
**Explanation:** ".\* " means "zero or more (\*) of any character (.) ".
**Constraints:**
* `1 <= s.length <= 20`
* `1 <= p.length <= 20`
* `s` contains only lowercase English letters.
* `p` contains only lowercase English letters, `'.'`, and `'*'`.
* It is guaranteed for each appearance of the character `'*'`, there will be a previous valid character to match. | null |
dp / python3 / time O(mn) / space O(mn) | regular-expression-matching | 0 | 1 | This problem can be solved using dynamic programming. Let dp[i][j] be a boolean indicating whether the first i characters of s match the first j characters of p. We can initialize dp[0][0] = True because an empty string matches an empty pattern.\n\nFor the first row dp[0][j], we need to consider two cases: if p[j-1] is \'*\', we can ignore it and look at dp[0][j-2] (the pattern without the \'*\' and its preceding character), or if p[j-2] matches the empty string, we can look at dp[0][j-1] (the pattern with only the \'*\' and its preceding character).\n\nFor each subsequent cell dp[i][j], we need to consider three cases: if p[j-1] is a regular character and matches s[i-1], then dp[i][j] = dp[i-1][j-1]. If p[j-1] is a \'*\', then we can either ignore the preceding character and look at dp[i][j-2] (the pattern without the \'*\' and its preceding character), or if the preceding character matches s[i-1], we can look at dp[i-1][j] (the pattern with the \'*\' and one more repetition of the preceding character), or if the preceding character does not match s[i-1], we can ignore both the preceding character and the \'*\' and look at dp[i][j-2]. If p[j-1] is a ., then dp[i][j] = dp[i-1][j-1].\n\nThe final answer is dp[m][n], where m and n are the lengths of s and p, respectively.\n\nThe time complexity of this algorithm is O(mn) and the space complexity is O(mn), where m and n are the lengths of s and p, respectively.\n\n# Code\n```\nclass Solution:\n def isMatch(self, s: str, p: str) -> bool:\n m, n = len(s), len(p)\n dp = [[False] * (n+1) for _ in range(m+1)]\n dp[0][0] = True\n for j in range(1, n+1):\n if p[j-1] == \'*\':\n dp[0][j] = dp[0][j-2]\n else:\n dp[0][j] = j > 1 and p[j-2] == \'*\' and dp[0][j-2]\n for i in range(1, m+1):\n for j in range(1, n+1):\n if p[j-1] == s[i-1] or p[j-1] == \'.\':\n dp[i][j] = dp[i-1][j-1]\n elif p[j-1] == \'*\':\n dp[i][j] = dp[i][j-2] or (p[j-2] == s[i-1] or p[j-2] == \'.\') and dp[i-1][j]\n else:\n dp[i][j] = False\n return dp[m][n]\n``` | 6 | Given an input string `s` and a pattern `p`, implement regular expression matching with support for `'.'` and `'*'` where:
* `'.'` Matches any single character.
* `'*'` Matches zero or more of the preceding element.
The matching should cover the **entire** input string (not partial).
**Example 1:**
**Input:** s = "aa ", p = "a "
**Output:** false
**Explanation:** "a " does not match the entire string "aa ".
**Example 2:**
**Input:** s = "aa ", p = "a\* "
**Output:** true
**Explanation:** '\*' means zero or more of the preceding element, 'a'. Therefore, by repeating 'a' once, it becomes "aa ".
**Example 3:**
**Input:** s = "ab ", p = ".\* "
**Output:** true
**Explanation:** ".\* " means "zero or more (\*) of any character (.) ".
**Constraints:**
* `1 <= s.length <= 20`
* `1 <= p.length <= 20`
* `s` contains only lowercase English letters.
* `p` contains only lowercase English letters, `'.'`, and `'*'`.
* It is guaranteed for each appearance of the character `'*'`, there will be a previous valid character to match. | null |
✅Best Method 🔥 || C++ || JAVA || PYTHON || Beginner Friendly🔥🔥🔥 | container-with-most-water | 1 | 1 | # Intuition:\nThe two-pointer technique starts with the widest container and moves the pointers inward based on the comparison of heights. \nIncreasing the width of the container can only lead to a larger area if the height of the new boundary is greater. By moving the pointers towards the center, we explore containers with the potential for greater areas.\n\n# Explanation:\n1. Initialize the variables:\n - `left` to represent the left pointer, starting at the beginning of the container (index 0).\n - `right` to represent the right pointer, starting at the end of the container (index `height.size() - 1`).\n - `maxArea` to keep track of the maximum area found, initially set to 0.\n\n2. Enter a loop using the condition `left < right`, which means the pointers have not crossed each other yet.\n\n3. Calculate the current area:\n - Use the `min` function to find the minimum height between the `left` and `right` pointers.\n - Multiply the minimum height by the width, which is the difference between the indices of the pointers: `(right - left)`.\n - Store this value in the `currentArea` variable.\n\n4. Update the maximum area:\n - Use the `max` function to compare the `currentArea` with the `maxArea`.\n - If the `currentArea` is greater than the `maxArea`, update `maxArea` with the `currentArea`.\n\n5. Move the pointers inward: (Explained in detail below)\n - Check if the height at the `left` pointer is smaller than the height at the `right` pointer.\n - If so, increment the `left` pointer, moving it towards the center of the container.\n - Otherwise, decrement the `right` pointer, also moving it towards the center.\n\n6. Repeat steps 3 to 5 until the pointers meet (`left >= right`), indicating that all possible containers have been explored.\n\n7. Return the `maxArea`, which represents the maximum area encountered among all the containers.\n\n# Update the maximum area:\nThe purpose of this condition is to determine which pointer to move inward, either the left pointer (`i`) or the right pointer (`j`), based on the comparison of heights at their respective positions.\n\nImagine you have two containers represented by the heights at the left and right pointers. The condition checks which container has a smaller height and moves the pointer corresponding to that container.\n\n1. If `height[i] > height[j]`:\n - This means that the height of the left container is greater than the height of the right container.\n - Moving the right pointer (`j`) would not increase the potential area because the height of the right container is the limiting factor.\n - So, to explore containers with the possibility of greater areas, we need to move the right pointer inward by decrementing `j`.\n\n2. If `height[i] <= height[j]`:\n - This means that the height of the left container is less than or equal to the height of the right container.\n - Moving the left pointer (`i`) would not increase the potential area because the height of the left container is the limiting factor.\n - So, to explore containers with the possibility of greater areas, we need to move the left pointer inward by incrementing `i`.\n\nBy making these pointer movements, we ensure that we are always exploring containers with the potential for larger areas. The approach is based on the observation that increasing the width of the container can only lead to a larger area if the height of the new boundary is greater.\nBy following this condition and moving the pointers accordingly, the algorithm explores all possible containers and finds the one with the maximum area.\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int maxArea(vector<int>& height) {\n int left = 0;\n int right = height.size() - 1;\n int maxArea = 0;\n\n while (left < right) {\n int currentArea = min(height[left], height[right]) * (right - left);\n maxArea = max(maxArea, currentArea);\n\n if (height[left] < height[right]) {\n left++;\n } else {\n right--;\n }\n }\n\n return maxArea;\n }\n};\n```\n```Java []\nclass Solution {\n public int maxArea(int[] height) {\n int left = 0;\n int right = height.length - 1;\n int maxArea = 0;\n\n while (left < right) {\n int currentArea = Math.min(height[left], height[right]) * (right - left);\n maxArea = Math.max(maxArea, currentArea);\n\n if (height[left] < height[right]) {\n left++;\n } else {\n right--;\n }\n }\n\n return maxArea;\n }\n}\n```\n```Python3 []\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n left = 0\n right = len(height) - 1\n maxArea = 0\n\n while left < right:\n currentArea = min(height[left], height[right]) * (right - left)\n maxArea = max(maxArea, currentArea)\n\n if height[left] < height[right]:\n left += 1\n else:\n right -= 1\n\n return maxArea\n```\n\n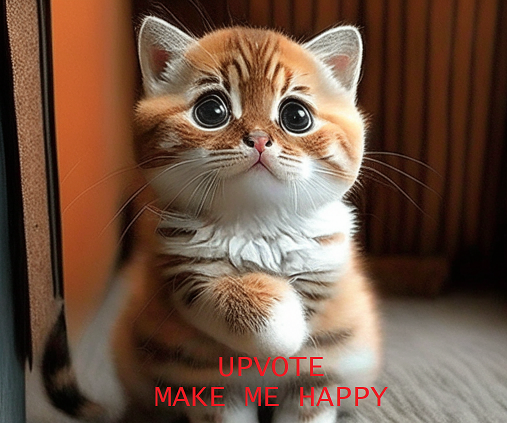\n\n\n**If you found my solution helpful, I would greatly appreciate your upvote, as it would motivate me to continue sharing more solutions.** | 627 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
✅Best Method 🔥 || C++ || JAVA || PYTHON || Beginner Friendly🔥🔥🔥 | container-with-most-water | 1 | 1 | # Intuition:\nThe two-pointer technique starts with the widest container and moves the pointers inward based on the comparison of heights. \nIncreasing the width of the container can only lead to a larger area if the height of the new boundary is greater. By moving the pointers towards the center, we explore containers with the potential for greater areas.\n\n# Explanation:\n1. Initialize the variables:\n - `left` to represent the left pointer, starting at the beginning of the container (index 0).\n - `right` to represent the right pointer, starting at the end of the container (index `height.size() - 1`).\n - `maxArea` to keep track of the maximum area found, initially set to 0.\n\n2. Enter a loop using the condition `left < right`, which means the pointers have not crossed each other yet.\n\n3. Calculate the current area:\n - Use the `min` function to find the minimum height between the `left` and `right` pointers.\n - Multiply the minimum height by the width, which is the difference between the indices of the pointers: `(right - left)`.\n - Store this value in the `currentArea` variable.\n\n4. Update the maximum area:\n - Use the `max` function to compare the `currentArea` with the `maxArea`.\n - If the `currentArea` is greater than the `maxArea`, update `maxArea` with the `currentArea`.\n\n5. Move the pointers inward: (Explained in detail below)\n - Check if the height at the `left` pointer is smaller than the height at the `right` pointer.\n - If so, increment the `left` pointer, moving it towards the center of the container.\n - Otherwise, decrement the `right` pointer, also moving it towards the center.\n\n6. Repeat steps 3 to 5 until the pointers meet (`left >= right`), indicating that all possible containers have been explored.\n\n7. Return the `maxArea`, which represents the maximum area encountered among all the containers.\n\n# Update the maximum area:\nThe purpose of this condition is to determine which pointer to move inward, either the left pointer (`i`) or the right pointer (`j`), based on the comparison of heights at their respective positions.\n\nImagine you have two containers represented by the heights at the left and right pointers. The condition checks which container has a smaller height and moves the pointer corresponding to that container.\n\n1. If `height[i] > height[j]`:\n - This means that the height of the left container is greater than the height of the right container.\n - Moving the right pointer (`j`) would not increase the potential area because the height of the right container is the limiting factor.\n - So, to explore containers with the possibility of greater areas, we need to move the right pointer inward by decrementing `j`.\n\n2. If `height[i] <= height[j]`:\n - This means that the height of the left container is less than or equal to the height of the right container.\n - Moving the left pointer (`i`) would not increase the potential area because the height of the left container is the limiting factor.\n - So, to explore containers with the possibility of greater areas, we need to move the left pointer inward by incrementing `i`.\n\nBy making these pointer movements, we ensure that we are always exploring containers with the potential for larger areas. The approach is based on the observation that increasing the width of the container can only lead to a larger area if the height of the new boundary is greater.\nBy following this condition and moving the pointers accordingly, the algorithm explores all possible containers and finds the one with the maximum area.\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int maxArea(vector<int>& height) {\n int left = 0;\n int right = height.size() - 1;\n int maxArea = 0;\n\n while (left < right) {\n int currentArea = min(height[left], height[right]) * (right - left);\n maxArea = max(maxArea, currentArea);\n\n if (height[left] < height[right]) {\n left++;\n } else {\n right--;\n }\n }\n\n return maxArea;\n }\n};\n```\n```Java []\nclass Solution {\n public int maxArea(int[] height) {\n int left = 0;\n int right = height.length - 1;\n int maxArea = 0;\n\n while (left < right) {\n int currentArea = Math.min(height[left], height[right]) * (right - left);\n maxArea = Math.max(maxArea, currentArea);\n\n if (height[left] < height[right]) {\n left++;\n } else {\n right--;\n }\n }\n\n return maxArea;\n }\n}\n```\n```Python3 []\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n left = 0\n right = len(height) - 1\n maxArea = 0\n\n while left < right:\n currentArea = min(height[left], height[right]) * (right - left)\n maxArea = max(maxArea, currentArea)\n\n if height[left] < height[right]:\n left += 1\n else:\n right -= 1\n\n return maxArea\n```\n\n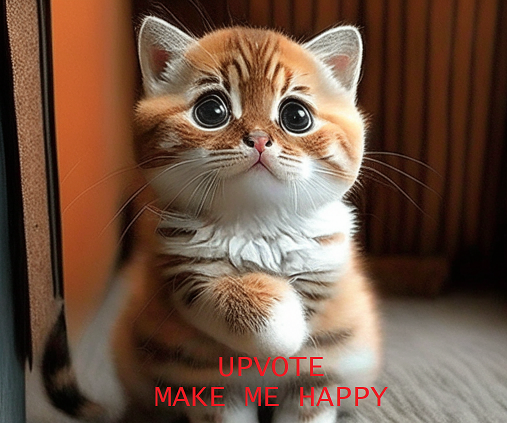\n\n\n**If you found my solution helpful, I would greatly appreciate your upvote, as it would motivate me to continue sharing more solutions.** | 627 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
🔥Beats 100% | 🔥O(n) Solution With Detailed Example💡| C++ | Java | Python | Javascript | C | Ruby | container-with-most-water | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem involves finding two lines in a set of vertical lines (represented by an array of heights) that can form a container to hold the maximum amount of water. The water\'s capacity is determined by the height of the shorter line and the distance between the two lines. We want to find the maximum capacity among all possible combinations of two lines.\n\n---\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. We use a two-pointer approach, starting with the left pointer at the leftmost edge of the array (left = 0) and the right pointer at the rightmost edge of the array (right = height.size() - 1).\n\n2. We initialize a variable maxWater to store the maximum water capacity, initially set to 0.\n\n3. We enter a while loop, which continues as long as the left pointer is less than the right pointer. This loop allows us to explore all possible combinations of two lines.\n\n4. Inside the loop, we calculate the width of the container by subtracting the positions of the two pointers: width = right - left.\n\n5. We calculate the height of the container by finding the minimum height between the two lines at positions height[left] and height[right]: h = min(height[left], height[right]).\n\n6. We calculate the water capacity of the current container by multiplying the width and height: water = width * h.\n\n7. We update the maxWater variable if the current container holds more water than the previous maximum: maxWater = max(maxWater, water).\n\n8. Finally, we adjust the pointers: if the height at the left pointer is smaller than the height at the right pointer (height[left] < height[right]), we move the left pointer to the right (left++); otherwise, we move the right pointer to the left (right--).\n\n9. We repeat steps 4-8 until the left pointer is less than the right pointer. Once the pointers meet, we have explored all possible combinations, and the maxWater variable contains the maximum water capacity.\n\n10. We return the maxWater value as the final result.\n\n---\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe time complexity of this algorithm is O(n), where n is the number of elements in the height array. This is because we explore all possible combinations of two lines once, and each operation inside the loop is performed in constant time.\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space complexity is O(1), which means the algorithm uses a constant amount of extra space regardless of the input size. We only use a few extra variables (left, right, maxWater, width, h, and water) that do not depend on the input size.\n\n---\n\n\n```C++ []\nclass Solution {\npublic:\n int maxArea(vector<int>& height) {\n int left = 0; // Left pointer starting from the leftmost edge\n int right = height.size() - 1; // Right pointer starting from the rightmost edge\n int maxWater = 0; // Initialize the maximum water capacity\n \n while (left < right) {\n // Calculate the width of the container\n int width = right - left;\n \n // Calculate the height of the container (the minimum height between the two lines)\n int h = min(height[left], height[right]);\n \n // Calculate the water capacity of the current container\n int water = width * h;\n \n // Update the maximum water capacity if the current container holds more water\n maxWater = max(maxWater, water);\n \n // Move the pointers towards each other\n if (height[left] < height[right]) {\n left++;\n } else {\n right--;\n }\n }\n \n return maxWater;\n }\n};\n```\n```Java []\nclass Solution {\n public int maxArea(int[] height) {\n int left = 0; // Left pointer starting from the leftmost edge\n int right = height.length - 1; // Right pointer starting from the rightmost edge\n int maxWater = 0; // Initialize the maximum water capacity\n \n while (left < right) {\n // Calculate the width of the container\n int width = right - left;\n \n // Calculate the height of the container (the minimum height between the two lines)\n int h = Math.min(height[left], height[right]);\n \n // Calculate the water capacity of the current container\n int water = width * h;\n \n // Update the maximum water capacity if the current container holds more water\n maxWater = Math.max(maxWater, water);\n \n // Move the pointers towards each other\n if (height[left] < height[right]) {\n left++;\n } else {\n right--;\n }\n }\n \n return maxWater;\n }\n}\n\n```\n```Python []\nclass Solution:\n def maxArea(self, height):\n left = 0 # Left pointer starting from the leftmost edge\n right = len(height) - 1 # Right pointer starting from the rightmost edge\n maxWater = 0 # Initialize the maximum water capacity\n \n while left < right:\n # Calculate the width of the container\n width = right - left\n \n # Calculate the height of the container (the minimum height between the two lines)\n h = min(height[left], height[right])\n \n # Calculate the water capacity of the current container\n water = width * h\n \n # Update the maximum water capacity if the current container holds more water\n maxWater = max(maxWater, water)\n \n # Move the pointers towards each other\n if height[left] < height[right]:\n left += 1\n else:\n right -= 1\n \n return maxWater\n\n```\n```Javascript []\nvar maxArea = function(height) {\n let left = 0; // Left pointer starting from the leftmost edge\n let right = height.length - 1; // Right pointer starting from the rightmost edge\n let maxWater = 0; // Initialize the maximum water capacity\n \n while (left < right) {\n // Calculate the width of the container\n let width = right - left;\n \n // Calculate the height of the container (the minimum height between the two lines)\n let h = Math.min(height[left], height[right]);\n \n // Calculate the water capacity of the current container\n let water = width * h;\n \n // Update the maximum water capacity if the current container holds more water\n maxWater = Math.max(maxWater, water);\n \n // Move the pointers towards each other\n if (height[left] < height[right]) {\n left++;\n } else {\n right--;\n }\n }\n \n return maxWater;\n};\n\n```\n```Ruby []\nclass Solution\n def max_area(height)\n left = 0 # Left pointer starting from the leftmost edge\n right = height.size - 1 # Right pointer starting from the rightmost edge\n max_water = 0 # Initialize the maximum water capacity\n \n while left < right\n # Calculate the width of the container\n width = right - left\n \n # Calculate the height of the container (the minimum height between the two lines)\n h = [height[left], height[right]].min\n \n # Calculate the water capacity of the current container\n water = width * h\n \n # Update the maximum water capacity if the current container holds more water\n max_water = [max_water, water].max\n \n # Move the pointers towards each other\n if height[left] < height[right]\n left += 1\n else\n right -= 1\n end\n end\n \n max_water\n end\nend\n\n```\n```C []\nint maxArea(int* height, int heightSize) {\n int left = 0; // Left pointer starting from the leftmost edge\n int right = heightSize - 1; // Right pointer starting from the rightmost edge\n int maxWater = 0; // Initialize the maximum water capacity\n\n while (left < right) {\n // Calculate the width of the container\n int width = right - left;\n\n // Calculate the height of the container (the minimum height between the two lines)\n int h = height[left] < height[right] ? height[left] : height[right];\n\n // Calculate the water capacity of the current container\n int water = width * h;\n\n // Update the maximum water capacity if the current container holds more water\n if (water > maxWater) {\n maxWater = water;\n }\n\n // Move the pointers towards each other\n if (height[left] < height[right]) {\n left++;\n } else {\n right--;\n }\n }\n\n return maxWater;\n}\n\n```\n\n# \uD83D\uDCA1If you come this far, then i would like to request you to please upvote this solution so that it could reach out to another one \u2763\uFE0F\uD83D\uDCA1\n\n\n\n | 73 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
🔥Beats 100% | 🔥O(n) Solution With Detailed Example💡| C++ | Java | Python | Javascript | C | Ruby | container-with-most-water | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem involves finding two lines in a set of vertical lines (represented by an array of heights) that can form a container to hold the maximum amount of water. The water\'s capacity is determined by the height of the shorter line and the distance between the two lines. We want to find the maximum capacity among all possible combinations of two lines.\n\n---\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. We use a two-pointer approach, starting with the left pointer at the leftmost edge of the array (left = 0) and the right pointer at the rightmost edge of the array (right = height.size() - 1).\n\n2. We initialize a variable maxWater to store the maximum water capacity, initially set to 0.\n\n3. We enter a while loop, which continues as long as the left pointer is less than the right pointer. This loop allows us to explore all possible combinations of two lines.\n\n4. Inside the loop, we calculate the width of the container by subtracting the positions of the two pointers: width = right - left.\n\n5. We calculate the height of the container by finding the minimum height between the two lines at positions height[left] and height[right]: h = min(height[left], height[right]).\n\n6. We calculate the water capacity of the current container by multiplying the width and height: water = width * h.\n\n7. We update the maxWater variable if the current container holds more water than the previous maximum: maxWater = max(maxWater, water).\n\n8. Finally, we adjust the pointers: if the height at the left pointer is smaller than the height at the right pointer (height[left] < height[right]), we move the left pointer to the right (left++); otherwise, we move the right pointer to the left (right--).\n\n9. We repeat steps 4-8 until the left pointer is less than the right pointer. Once the pointers meet, we have explored all possible combinations, and the maxWater variable contains the maximum water capacity.\n\n10. We return the maxWater value as the final result.\n\n---\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe time complexity of this algorithm is O(n), where n is the number of elements in the height array. This is because we explore all possible combinations of two lines once, and each operation inside the loop is performed in constant time.\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space complexity is O(1), which means the algorithm uses a constant amount of extra space regardless of the input size. We only use a few extra variables (left, right, maxWater, width, h, and water) that do not depend on the input size.\n\n---\n\n\n```C++ []\nclass Solution {\npublic:\n int maxArea(vector<int>& height) {\n int left = 0; // Left pointer starting from the leftmost edge\n int right = height.size() - 1; // Right pointer starting from the rightmost edge\n int maxWater = 0; // Initialize the maximum water capacity\n \n while (left < right) {\n // Calculate the width of the container\n int width = right - left;\n \n // Calculate the height of the container (the minimum height between the two lines)\n int h = min(height[left], height[right]);\n \n // Calculate the water capacity of the current container\n int water = width * h;\n \n // Update the maximum water capacity if the current container holds more water\n maxWater = max(maxWater, water);\n \n // Move the pointers towards each other\n if (height[left] < height[right]) {\n left++;\n } else {\n right--;\n }\n }\n \n return maxWater;\n }\n};\n```\n```Java []\nclass Solution {\n public int maxArea(int[] height) {\n int left = 0; // Left pointer starting from the leftmost edge\n int right = height.length - 1; // Right pointer starting from the rightmost edge\n int maxWater = 0; // Initialize the maximum water capacity\n \n while (left < right) {\n // Calculate the width of the container\n int width = right - left;\n \n // Calculate the height of the container (the minimum height between the two lines)\n int h = Math.min(height[left], height[right]);\n \n // Calculate the water capacity of the current container\n int water = width * h;\n \n // Update the maximum water capacity if the current container holds more water\n maxWater = Math.max(maxWater, water);\n \n // Move the pointers towards each other\n if (height[left] < height[right]) {\n left++;\n } else {\n right--;\n }\n }\n \n return maxWater;\n }\n}\n\n```\n```Python []\nclass Solution:\n def maxArea(self, height):\n left = 0 # Left pointer starting from the leftmost edge\n right = len(height) - 1 # Right pointer starting from the rightmost edge\n maxWater = 0 # Initialize the maximum water capacity\n \n while left < right:\n # Calculate the width of the container\n width = right - left\n \n # Calculate the height of the container (the minimum height between the two lines)\n h = min(height[left], height[right])\n \n # Calculate the water capacity of the current container\n water = width * h\n \n # Update the maximum water capacity if the current container holds more water\n maxWater = max(maxWater, water)\n \n # Move the pointers towards each other\n if height[left] < height[right]:\n left += 1\n else:\n right -= 1\n \n return maxWater\n\n```\n```Javascript []\nvar maxArea = function(height) {\n let left = 0; // Left pointer starting from the leftmost edge\n let right = height.length - 1; // Right pointer starting from the rightmost edge\n let maxWater = 0; // Initialize the maximum water capacity\n \n while (left < right) {\n // Calculate the width of the container\n let width = right - left;\n \n // Calculate the height of the container (the minimum height between the two lines)\n let h = Math.min(height[left], height[right]);\n \n // Calculate the water capacity of the current container\n let water = width * h;\n \n // Update the maximum water capacity if the current container holds more water\n maxWater = Math.max(maxWater, water);\n \n // Move the pointers towards each other\n if (height[left] < height[right]) {\n left++;\n } else {\n right--;\n }\n }\n \n return maxWater;\n};\n\n```\n```Ruby []\nclass Solution\n def max_area(height)\n left = 0 # Left pointer starting from the leftmost edge\n right = height.size - 1 # Right pointer starting from the rightmost edge\n max_water = 0 # Initialize the maximum water capacity\n \n while left < right\n # Calculate the width of the container\n width = right - left\n \n # Calculate the height of the container (the minimum height between the two lines)\n h = [height[left], height[right]].min\n \n # Calculate the water capacity of the current container\n water = width * h\n \n # Update the maximum water capacity if the current container holds more water\n max_water = [max_water, water].max\n \n # Move the pointers towards each other\n if height[left] < height[right]\n left += 1\n else\n right -= 1\n end\n end\n \n max_water\n end\nend\n\n```\n```C []\nint maxArea(int* height, int heightSize) {\n int left = 0; // Left pointer starting from the leftmost edge\n int right = heightSize - 1; // Right pointer starting from the rightmost edge\n int maxWater = 0; // Initialize the maximum water capacity\n\n while (left < right) {\n // Calculate the width of the container\n int width = right - left;\n\n // Calculate the height of the container (the minimum height between the two lines)\n int h = height[left] < height[right] ? height[left] : height[right];\n\n // Calculate the water capacity of the current container\n int water = width * h;\n\n // Update the maximum water capacity if the current container holds more water\n if (water > maxWater) {\n maxWater = water;\n }\n\n // Move the pointers towards each other\n if (height[left] < height[right]) {\n left++;\n } else {\n right--;\n }\n }\n\n return maxWater;\n}\n\n```\n\n# \uD83D\uDCA1If you come this far, then i would like to request you to please upvote this solution so that it could reach out to another one \u2763\uFE0F\uD83D\uDCA1\n\n\n\n | 73 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
[C++] [Python] Brute Force Approach || Too Easy || Fully Explained | container-with-most-water | 0 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\nSuppose we have the following input vector:\n```\nvector<int> hit = {1, 8, 6, 2, 5, 4, 8, 3, 7};\n```\n\nNow, let\'s go through the function step by step:\n\n1. Initialize `mx` (maximum area) to INT_MIN (the minimum value that can be represented by the `int` data type).\n2. Initialize `st` (start) to 0 and `end` (end) to `hit.size() - 1`.\n3. Enter a `while` loop that continues as long as `st` is less than `end`.\n - In the first iteration:\n - Calculate the difference between `end` and `st` (indicated as `indDif`), which is 8.\n - Find the minimum value between `hit[st]` (which is 1) and `hit[end]` (which is 7), which is 1.\n - Calculate the area as `indDif * mn`, which is 8 * 1 = 8.\n - Since `mx` is INT_MIN, update `mx` to 8.\n - Compare `hit[st]` (which is 1) with `hit[end]` (which is 7), and since `hit[st]` is smaller, increment `st` to 1.\n - In the second iteration:\n - Calculate the difference between `end` and `st` (indicated as `indDif`), which is 7.\n - Find the minimum value between `hit[st]` (which is 8) and `hit[end]` (which is 7), which is 7.\n - Calculate the area as `indDif * mn`, which is 7 * 7 = 49.\n - Since `mx` (which was 8) is less than 49, update `mx` to 49.\n - Compare `hit[st]` (which is 8) with `hit[end]` (which is 7), and since `hit[end]` is smaller, decrement `end` to 7.\n - The loop continues with similar steps until `st` becomes greater than or equal to `end`.\n\n4. The loop ends when `st` becomes greater than or equal to `end`.\n5. The function returns `mx` as the result: 49. \n\n# Complexity\n- Time complexity:$$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n``` C++ []\nclass Solution {\npublic:\n int maxArea(vector<int>& hit) {\n int mx = INT_MIN;\n int st = 0; \n int end = hit.size() - 1;\n while(st < end){\n int indDif = end - st;\n int mn = min(hit[st], hit[end]);\n mx = max(mx, (indDif * mn));\n if(hit[st] < hit[end])\n st++;\n else\n end--;\n }\n return mx;\n }\n};\n```\n``` Python []\nclass Solution:\n def maxArea(self, hit: List[int]) -> int:\n mx = 0\n i = 0\n size = len(hit) - 1\n while i < size:\n dif = size - i\n mn = min(hit[i], hit[size])\n mx = max(mx, dif * mn)\n \n if hit[i] < hit[size]:\n i += 1\n else :\n size -= 1\n return mx\n``` | 1 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
[C++] [Python] Brute Force Approach || Too Easy || Fully Explained | container-with-most-water | 0 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\nSuppose we have the following input vector:\n```\nvector<int> hit = {1, 8, 6, 2, 5, 4, 8, 3, 7};\n```\n\nNow, let\'s go through the function step by step:\n\n1. Initialize `mx` (maximum area) to INT_MIN (the minimum value that can be represented by the `int` data type).\n2. Initialize `st` (start) to 0 and `end` (end) to `hit.size() - 1`.\n3. Enter a `while` loop that continues as long as `st` is less than `end`.\n - In the first iteration:\n - Calculate the difference between `end` and `st` (indicated as `indDif`), which is 8.\n - Find the minimum value between `hit[st]` (which is 1) and `hit[end]` (which is 7), which is 1.\n - Calculate the area as `indDif * mn`, which is 8 * 1 = 8.\n - Since `mx` is INT_MIN, update `mx` to 8.\n - Compare `hit[st]` (which is 1) with `hit[end]` (which is 7), and since `hit[st]` is smaller, increment `st` to 1.\n - In the second iteration:\n - Calculate the difference between `end` and `st` (indicated as `indDif`), which is 7.\n - Find the minimum value between `hit[st]` (which is 8) and `hit[end]` (which is 7), which is 7.\n - Calculate the area as `indDif * mn`, which is 7 * 7 = 49.\n - Since `mx` (which was 8) is less than 49, update `mx` to 49.\n - Compare `hit[st]` (which is 8) with `hit[end]` (which is 7), and since `hit[end]` is smaller, decrement `end` to 7.\n - The loop continues with similar steps until `st` becomes greater than or equal to `end`.\n\n4. The loop ends when `st` becomes greater than or equal to `end`.\n5. The function returns `mx` as the result: 49. \n\n# Complexity\n- Time complexity:$$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n``` C++ []\nclass Solution {\npublic:\n int maxArea(vector<int>& hit) {\n int mx = INT_MIN;\n int st = 0; \n int end = hit.size() - 1;\n while(st < end){\n int indDif = end - st;\n int mn = min(hit[st], hit[end]);\n mx = max(mx, (indDif * mn));\n if(hit[st] < hit[end])\n st++;\n else\n end--;\n }\n return mx;\n }\n};\n```\n``` Python []\nclass Solution:\n def maxArea(self, hit: List[int]) -> int:\n mx = 0\n i = 0\n size = len(hit) - 1\n while i < size:\n dif = size - i\n mn = min(hit[i], hit[size])\n mx = max(mx, dif * mn)\n \n if hit[i] < hit[size]:\n i += 1\n else :\n size -= 1\n return mx\n``` | 1 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
container-with-most-water with O(n) time complexity | container-with-most-water | 0 | 1 | \n# Approach\nBrute Force approach would be:\n Traversing through the array, considering each element of the array and calcualting the container area with the rest of the elements in the array would give the solution but results in O(n^2) time complexity, the code snippet would be:\n```\nCode block\narea_tracker=0\nfor i in range(len(height)):\n //running the above block results in O(n) time complexity\n for j in range(i+1, len(height)):\n //running this loop in association with the above block would result in O(n^2) time complexity.\n area = (j-i)*min(height[j],height[i])\n if area>area_tracker:\n area_tracker = area\nreturn area_tracker\n``` \nThe optimal solution can be using two pointer approach, i.e.,\n1) keeping left pointer at the left most index of the array\n2) keeping the right pointer at the right most index of the array\n\u2139\uFE0F as the focus is on maximum area to be covered, keep the pointer which is at the maximum value between the two and play with moving the pointer which has the least of the two elements the pointers are currently pointing to.\nThe code snippet will be:\n```\nl=0\nr = len(height)-1\nheight_tracker=0\nwhile l<=r:\n length = r-l\n h = min(height[l],height[r])\n if height_tracker<length*h:\n height_tracker=length*h\n if height[l]<=height[r]:\n l+=1\n else:\n r-=1\n\n```\nworst case scenario would result in O(n) time complexity.\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n l=0\n r = len(height)-1\n height_tracker=0\n while l<=r:\n length = r-l\n h = min(height[l],height[r])\n if height_tracker<length*h:\n height_tracker=length*h\n if height[l]<=height[r]:\n l+=1\n else:\n r-=1\n \n return height_tracker\n``` | 1 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
container-with-most-water with O(n) time complexity | container-with-most-water | 0 | 1 | \n# Approach\nBrute Force approach would be:\n Traversing through the array, considering each element of the array and calcualting the container area with the rest of the elements in the array would give the solution but results in O(n^2) time complexity, the code snippet would be:\n```\nCode block\narea_tracker=0\nfor i in range(len(height)):\n //running the above block results in O(n) time complexity\n for j in range(i+1, len(height)):\n //running this loop in association with the above block would result in O(n^2) time complexity.\n area = (j-i)*min(height[j],height[i])\n if area>area_tracker:\n area_tracker = area\nreturn area_tracker\n``` \nThe optimal solution can be using two pointer approach, i.e.,\n1) keeping left pointer at the left most index of the array\n2) keeping the right pointer at the right most index of the array\n\u2139\uFE0F as the focus is on maximum area to be covered, keep the pointer which is at the maximum value between the two and play with moving the pointer which has the least of the two elements the pointers are currently pointing to.\nThe code snippet will be:\n```\nl=0\nr = len(height)-1\nheight_tracker=0\nwhile l<=r:\n length = r-l\n h = min(height[l],height[r])\n if height_tracker<length*h:\n height_tracker=length*h\n if height[l]<=height[r]:\n l+=1\n else:\n r-=1\n\n```\nworst case scenario would result in O(n) time complexity.\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n l=0\n r = len(height)-1\n height_tracker=0\n while l<=r:\n length = r-l\n h = min(height[l],height[r])\n if height_tracker<length*h:\n height_tracker=length*h\n if height[l]<=height[r]:\n l+=1\n else:\n r-=1\n \n return height_tracker\n``` | 1 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
Container with most water | container-with-most-water | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n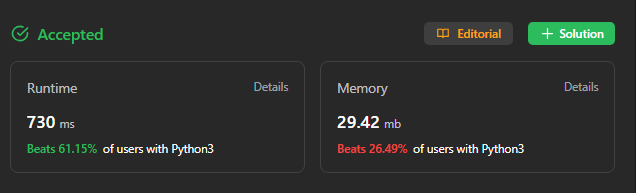\n\n# Code\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n left=0\n right=len(height)-1\n ans=0\n while left<right:\n min_=min(height[left],height[right])*(right-left)\n ans=max(ans,min_)\n if height[left]<height[right]:\n left+=1\n else:\n right-=1\n return ans\n``` | 1 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
Container with most water | container-with-most-water | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n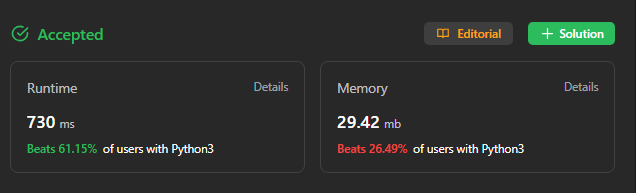\n\n# Code\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n left=0\n right=len(height)-1\n ans=0\n while left<right:\n min_=min(height[left],height[right])*(right-left)\n ans=max(ans,min_)\n if height[left]<height[right]:\n left+=1\n else:\n right-=1\n return ans\n``` | 1 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
Easy Python Solution : beats 91.96%: With GREAT explanation | container-with-most-water | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nLet us take the two edges as the container walls and calculate the water in them.\n\nSo now we change the smaller wall.THE reason for NOT Changing the bigger wall is that the amount of water would not increase no matter the size of the bigger wall hence we are considering the size of the smaller wall to calculate the amount of water needed.\n\nNOTE: If you can not understand my explanation please read the approach and then try to understand my intuition\n# Approach\n<!-- Describe your approach to solving the problem. -->\n->Take two variables ```(l,r)``` and assign the boundaries of the given list.\n\n->Now calculate the amount of water in them.The amount of water present is basically the distance between the walls multiplied by the smaller wall heigth\n\n->Now compare the heights of the walls in the positions and move the smaller wall towards the bigger wall.\n[The reason for this is given in the intuition .I recommend reading it if u have not read it]\n\n->continue this process till the left wall crosses the right wall.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n l,r=0,len(height)-1\n\n water=0\n while l<r:\n water=max(min(height[l],height[r])*(r-l),water)\n if height[l]<height[r]:\n l+=1\n else:\n r-=1\n \n return water\n\n\n\n``` | 4 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
Easy Python Solution : beats 91.96%: With GREAT explanation | container-with-most-water | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nLet us take the two edges as the container walls and calculate the water in them.\n\nSo now we change the smaller wall.THE reason for NOT Changing the bigger wall is that the amount of water would not increase no matter the size of the bigger wall hence we are considering the size of the smaller wall to calculate the amount of water needed.\n\nNOTE: If you can not understand my explanation please read the approach and then try to understand my intuition\n# Approach\n<!-- Describe your approach to solving the problem. -->\n->Take two variables ```(l,r)``` and assign the boundaries of the given list.\n\n->Now calculate the amount of water in them.The amount of water present is basically the distance between the walls multiplied by the smaller wall heigth\n\n->Now compare the heights of the walls in the positions and move the smaller wall towards the bigger wall.\n[The reason for this is given in the intuition .I recommend reading it if u have not read it]\n\n->continue this process till the left wall crosses the right wall.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n l,r=0,len(height)-1\n\n water=0\n while l<r:\n water=max(min(height[l],height[r])*(r-l),water)\n if height[l]<height[r]:\n l+=1\n else:\n r-=1\n \n return water\n\n\n\n``` | 4 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
[VIDEO] Visualization and Intuitive Proof of Greedy Solution | container-with-most-water | 0 | 1 | https://youtu.be/Kb20p6zy_14\n\nThe strategy is to start with the container of the <i>longest</i> width and move the sides inwards one by one to see if we can get a larger area by shortening the width but getting a taller height. But how do we know which side to move?\n\nThe key insight here is that moving the <i>longer</i> side inwards is completely unnecessary because the height of the water is bounded by the <i>shorter</i> side. In other words, we will never be able to get a greater area by moving the longer side inwards because the height will either stay the same or get shorter, and the width will keep decreasing.\n\nSo we can skip all those calculations and instead move the <i>shorter</i> side inwards. This way, we <i>might</i> get a taller height and a larger area. So at each step, we calculate the area then move the shorter side inwards. When the left and right sides meet, we are done and we can return the largest area calculated so far.\n\n# Code\n```\nclass Solution(object):\n def maxArea(self, height):\n max_area = 0\n l = 0\n r = len(height) - 1\n while l < r:\n area = (r - l) * min(height[r], height[l])\n max_area = max(max_area, area)\n if height[l] < height[r]:\n l += 1\n else:\n r -= 1\n return max_area\n``` | 6 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
[VIDEO] Visualization and Intuitive Proof of Greedy Solution | container-with-most-water | 0 | 1 | https://youtu.be/Kb20p6zy_14\n\nThe strategy is to start with the container of the <i>longest</i> width and move the sides inwards one by one to see if we can get a larger area by shortening the width but getting a taller height. But how do we know which side to move?\n\nThe key insight here is that moving the <i>longer</i> side inwards is completely unnecessary because the height of the water is bounded by the <i>shorter</i> side. In other words, we will never be able to get a greater area by moving the longer side inwards because the height will either stay the same or get shorter, and the width will keep decreasing.\n\nSo we can skip all those calculations and instead move the <i>shorter</i> side inwards. This way, we <i>might</i> get a taller height and a larger area. So at each step, we calculate the area then move the shorter side inwards. When the left and right sides meet, we are done and we can return the largest area calculated so far.\n\n# Code\n```\nclass Solution(object):\n def maxArea(self, height):\n max_area = 0\n l = 0\n r = len(height) - 1\n while l < r:\n area = (r - l) * min(height[r], height[l])\n max_area = max(max_area, area)\n if height[l] < height[r]:\n l += 1\n else:\n r -= 1\n return max_area\n``` | 6 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
[Python] - Clean & Simple Math Solution - Using Two Pointer | container-with-most-water | 0 | 1 | # Code\n```\nclass Solution:\n def maxArea(self, h: List[int]) -> int:\n n = len(h)\n\n l, r = 0, n - 1\n\n ans = 0\n while l <= r:\n ans = max(ans, (r - l) * min(h[l], h[r]))\n if h[l] < h[r]: l += 1\n else: r -= 1\n return ans\n``` | 3 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
[Python] - Clean & Simple Math Solution - Using Two Pointer | container-with-most-water | 0 | 1 | # Code\n```\nclass Solution:\n def maxArea(self, h: List[int]) -> int:\n n = len(h)\n\n l, r = 0, n - 1\n\n ans = 0\n while l <= r:\n ans = max(ans, (r - l) * min(h[l], h[r]))\n if h[l] < h[r]: l += 1\n else: r -= 1\n return ans\n``` | 3 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
2 pointers | container-with-most-water | 0 | 1 | \n# Approach\nUsing 2 pointers that are set to 0 and n-1 at first, we move them towards the middle to find the best container. We are starting at the end because then we get the best base of rectangle, then we move worse pointer (that one pointing at smaller wall) towards the middle \nto try finding. Loop ends when pointers have the same value.\n# Complexity\n- Time complexity:\nO(N)\n# Code\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n maxi = 0\n n = len(height)\n l = 0\n r = n-1\n while l < r:\n area = min(height[l],height[r]) * (r-l)\n if area > maxi:\n maxi = area\n if height[l] > height[r]:\n r -= 1\n else:\n l += 1\n return maxi\n``` | 1 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
2 pointers | container-with-most-water | 0 | 1 | \n# Approach\nUsing 2 pointers that are set to 0 and n-1 at first, we move them towards the middle to find the best container. We are starting at the end because then we get the best base of rectangle, then we move worse pointer (that one pointing at smaller wall) towards the middle \nto try finding. Loop ends when pointers have the same value.\n# Complexity\n- Time complexity:\nO(N)\n# Code\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n maxi = 0\n n = len(height)\n l = 0\n r = n-1\n while l < r:\n area = min(height[l],height[r]) * (r-l)\n if area > maxi:\n maxi = area\n if height[l] > height[r]:\n r -= 1\n else:\n l += 1\n return maxi\n``` | 1 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
Two Pointer Logic-->Python3 | container-with-most-water | 0 | 1 | \n\n# Two Pointer Logic : TC----->O(N)\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n left,right,answer=0,len(height)-1,0\n while left<=right:\n area=min(height[right],height[left])*(right-left)\n answer=max(answer,area)\n if height[right]>height[left]:\n left+=1\n else:\n right-=1\n return answer\n```\n# please upvote me it would encourage me alot\n | 63 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
Two Pointer Logic-->Python3 | container-with-most-water | 0 | 1 | \n\n# Two Pointer Logic : TC----->O(N)\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n left,right,answer=0,len(height)-1,0\n while left<=right:\n area=min(height[right],height[left])*(right-left)\n answer=max(answer,area)\n if height[right]>height[left]:\n left+=1\n else:\n right-=1\n return answer\n```\n# please upvote me it would encourage me alot\n | 63 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
✔️ [Python3] GREEDY TWO POINTERS ~(˘▾˘~), Explained | container-with-most-water | 0 | 1 | **UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.**\n\nFirst, we check the widest possible container starting from the first line to the last one. Next, we ask ourselves, how is it possible to form an even bigger container? Every time we narrow the container, the width becomes smaller so the only way to get a bigger area is to find higher lines. So why not just greedily shrink the container on the side that has a shorter line? Every time we shrink the container, we calculate the area and save the maximum.\n\nTime: **O(n)** \nSpace: **O(1)**\n\nRuntime: 819 ms, faster than **75.10%** of Python3 online submissions for Container With Most Water.\nMemory Usage: 27.4 MB, less than **58.32%** of Python3 online submissions for Container With Most Water.\n\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n l, r, area = 0, len(height) - 1, 0\n while l < r:\n area = max(area, (r - l) * min(height[l], height[r]))\n if height[l] < height[r]:\n\t\t\t\tl += 1\n else:\n\t\t\t\tr -= 1\n\t\t\t\t\n return area\n```\n\n**UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.** | 185 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
✔️ [Python3] GREEDY TWO POINTERS ~(˘▾˘~), Explained | container-with-most-water | 0 | 1 | **UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.**\n\nFirst, we check the widest possible container starting from the first line to the last one. Next, we ask ourselves, how is it possible to form an even bigger container? Every time we narrow the container, the width becomes smaller so the only way to get a bigger area is to find higher lines. So why not just greedily shrink the container on the side that has a shorter line? Every time we shrink the container, we calculate the area and save the maximum.\n\nTime: **O(n)** \nSpace: **O(1)**\n\nRuntime: 819 ms, faster than **75.10%** of Python3 online submissions for Container With Most Water.\nMemory Usage: 27.4 MB, less than **58.32%** of Python3 online submissions for Container With Most Water.\n\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n l, r, area = 0, len(height) - 1, 0\n while l < r:\n area = max(area, (r - l) * min(height[l], height[r]))\n if height[l] < height[r]:\n\t\t\t\tl += 1\n else:\n\t\t\t\tr -= 1\n\t\t\t\t\n return area\n```\n\n**UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.** | 185 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
[Python] Constructive and Intuitive Solution in Linear Time, The Best | container-with-most-water | 0 | 1 | # Intuition\nWe would like to arrive at a constructive solution that is easily seen as correct (not missing any options), while running in linear time. This is different from the regular approach of moving left or right index one at a time, which requires an elaborate proof to use (correctness is not intuitive).\n\n# Approach\nLet\'s look for containers with **left line higher or equal in height to the right line**. This means that for each possible right line, we need to find the left-most line that\'s at least as high as this right line. So we only need to build a list of left lines with ascending height, going left to right, while any line that is not higher than the current maximum is a possible right line. Thus, each line is either a new left line to add to the ascending heights array, or a right line, which we use to binary search for the first possible left line and update the maximum area \u2014 see the the first code snippet.\n\nNow, we note that if right line indexes are considered right-to-left instead of left-to-right as previously (while still constructing the ascending left indexes as before), we can avoid binary search altogether by looking for right indexes of ascending height, stopping whenever the assumption of right line not being higher than the left is violated.\n\nThis new approach is functionally equivalent to the first one, except that we ignore possible right lines to the left of the point where left and right indexes meet. However, note that right index advancement stops when right line is momentarily higher than the left line, which is also used to update the maximum area. Therefore, those missed right lines are guaranteed to result in a lesser area.\n\nNote also that the number of area computations may be much less than $$n$$, unlike in the canonical solution. The Best!\n\n# Complexity\n- Time complexity: $$O(n)$$; number of actual area computations can be $$\\ll n$$\n- Space complexity: $$O(1)$$, if two different traversal functions are used instead of reversing the input list\n\n# Code \u2014 $$O(n \\log n)$$ time complexity\n```\nclass Solution:\n def maxArea(self, height: list[int]) -> int:\n area_left_higher = self.max_area_when_left_higher(height)\n\n height.reverse()\n area_right_higher = self.max_area_when_left_higher(height)\n\n return max(area_left_higher, area_right_higher)\n\n def max_area_when_left_higher(self, height: list[int]) -> int:\n max_area = 0\n\n ascending_indexes, ascending_heights = [], []\n ascending_height_max = -1\n\n for right_index, right_height in enumerate(height):\n if right_height > ascending_height_max:\n ascending_height_max = right_height\n ascending_indexes.append(right_index)\n ascending_heights.append(right_height)\n else:\n left_index = ascending_indexes[bisect.bisect_left(ascending_heights, right_height)]\n max_area = max(max_area, (right_index - left_index) * right_height)\n\n return max_area\n```\n\n# Improved Code - $$O(n)$$ time complexity\n```\ndef max_area_when_left_higher(self, height: list[int]) -> int:\n max_area = 0\n left_index, right_index = 0, len(height) - 1\n\n while left_index < right_index:\n area = (right_index - left_index) * min(height[left_index], height[right_index])\n max_area = max(max_area, area)\n\n if right_index - left_index == 1:\n break\n\n if height[left_index] < height[right_index]:\n left_height = height[left_index]\n while left_index < right_index and left_height >= height[left_index]:\n left_index += 1\n\n else:\n while left_index < right_index and height[left_index] >= height[right_index]:\n right_index -= 1\n\n return max_area\n```\n | 1 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
[Python] Constructive and Intuitive Solution in Linear Time, The Best | container-with-most-water | 0 | 1 | # Intuition\nWe would like to arrive at a constructive solution that is easily seen as correct (not missing any options), while running in linear time. This is different from the regular approach of moving left or right index one at a time, which requires an elaborate proof to use (correctness is not intuitive).\n\n# Approach\nLet\'s look for containers with **left line higher or equal in height to the right line**. This means that for each possible right line, we need to find the left-most line that\'s at least as high as this right line. So we only need to build a list of left lines with ascending height, going left to right, while any line that is not higher than the current maximum is a possible right line. Thus, each line is either a new left line to add to the ascending heights array, or a right line, which we use to binary search for the first possible left line and update the maximum area \u2014 see the the first code snippet.\n\nNow, we note that if right line indexes are considered right-to-left instead of left-to-right as previously (while still constructing the ascending left indexes as before), we can avoid binary search altogether by looking for right indexes of ascending height, stopping whenever the assumption of right line not being higher than the left is violated.\n\nThis new approach is functionally equivalent to the first one, except that we ignore possible right lines to the left of the point where left and right indexes meet. However, note that right index advancement stops when right line is momentarily higher than the left line, which is also used to update the maximum area. Therefore, those missed right lines are guaranteed to result in a lesser area.\n\nNote also that the number of area computations may be much less than $$n$$, unlike in the canonical solution. The Best!\n\n# Complexity\n- Time complexity: $$O(n)$$; number of actual area computations can be $$\\ll n$$\n- Space complexity: $$O(1)$$, if two different traversal functions are used instead of reversing the input list\n\n# Code \u2014 $$O(n \\log n)$$ time complexity\n```\nclass Solution:\n def maxArea(self, height: list[int]) -> int:\n area_left_higher = self.max_area_when_left_higher(height)\n\n height.reverse()\n area_right_higher = self.max_area_when_left_higher(height)\n\n return max(area_left_higher, area_right_higher)\n\n def max_area_when_left_higher(self, height: list[int]) -> int:\n max_area = 0\n\n ascending_indexes, ascending_heights = [], []\n ascending_height_max = -1\n\n for right_index, right_height in enumerate(height):\n if right_height > ascending_height_max:\n ascending_height_max = right_height\n ascending_indexes.append(right_index)\n ascending_heights.append(right_height)\n else:\n left_index = ascending_indexes[bisect.bisect_left(ascending_heights, right_height)]\n max_area = max(max_area, (right_index - left_index) * right_height)\n\n return max_area\n```\n\n# Improved Code - $$O(n)$$ time complexity\n```\ndef max_area_when_left_higher(self, height: list[int]) -> int:\n max_area = 0\n left_index, right_index = 0, len(height) - 1\n\n while left_index < right_index:\n area = (right_index - left_index) * min(height[left_index], height[right_index])\n max_area = max(max_area, area)\n\n if right_index - left_index == 1:\n break\n\n if height[left_index] < height[right_index]:\n left_height = height[left_index]\n while left_index < right_index and left_height >= height[left_index]:\n left_index += 1\n\n else:\n while left_index < right_index and height[left_index] >= height[right_index]:\n right_index -= 1\n\n return max_area\n```\n | 1 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
✅Worst method 🔥|| Python3 || Beginner Un-Friendly || Abysmal unreadable one liner. 🔥Beats 26% 🔥 | container-with-most-water | 0 | 1 | # Intuition\nAn esoteric solution to a reasonable interview question!\n\n# Approach\nSolve normally using two pointer approach. Purposefully obfuscate to one line.\n\nUses the exec() function from Python to encode a solution into a one-line string with escape codes.\n\n\\- Please, do not do this in an interview.\n\n# Complexity\n- Time complexity:\nO(n) maybe? Underlying function is still two pointer.\n\n- Space complexity:\nexec() statement makes this abhorrent.\n\n# Code\n```\nclass Solution:\n def maxArea(self, h: List[int]) -> int:\n global w, g; w, g = 0, h; exec(\'l, r, w = 0, len(g)-1, 0\\nwhile l < r:\\n\\tw = max(w, min(g[l], g[r]) * (r-l))\\n\\tif g[l] >= g[r]: r -=1\\n\\telse: l +=1\', globals()); return w\n``` | 13 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
✅Worst method 🔥|| Python3 || Beginner Un-Friendly || Abysmal unreadable one liner. 🔥Beats 26% 🔥 | container-with-most-water | 0 | 1 | # Intuition\nAn esoteric solution to a reasonable interview question!\n\n# Approach\nSolve normally using two pointer approach. Purposefully obfuscate to one line.\n\nUses the exec() function from Python to encode a solution into a one-line string with escape codes.\n\n\\- Please, do not do this in an interview.\n\n# Complexity\n- Time complexity:\nO(n) maybe? Underlying function is still two pointer.\n\n- Space complexity:\nexec() statement makes this abhorrent.\n\n# Code\n```\nclass Solution:\n def maxArea(self, h: List[int]) -> int:\n global w, g; w, g = 0, h; exec(\'l, r, w = 0, len(g)-1, 0\\nwhile l < r:\\n\\tw = max(w, min(g[l], g[r]) * (r-l))\\n\\tif g[l] >= g[r]: r -=1\\n\\telse: l +=1\', globals()); return w\n``` | 13 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
Easy solution Omegalul | container-with-most-water | 0 | 1 | # Intuition\n2 pointers, left and right, see all possibilities, and move whnichever is smaller\n# Code\n```\nclass Solution:\n def maxArea(self, li: List[int]) -> int:\n sussyballs = 0\n p1 = 0\n p2 = len(li) - 1\n\n while p1 < p2:\n girth = p2 - p1\n length = min(li[p1], li[p2])\n sussyballs = max(sussyballs, girth * length)\n\n if li[p1] < li[p2]:\n p1 += 1\n else:\n p2 -= 1\n\n return sussyballs\n``` | 0 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
Easy solution Omegalul | container-with-most-water | 0 | 1 | # Intuition\n2 pointers, left and right, see all possibilities, and move whnichever is smaller\n# Code\n```\nclass Solution:\n def maxArea(self, li: List[int]) -> int:\n sussyballs = 0\n p1 = 0\n p2 = len(li) - 1\n\n while p1 < p2:\n girth = p2 - p1\n length = min(li[p1], li[p2])\n sussyballs = max(sussyballs, girth * length)\n\n if li[p1] < li[p2]:\n p1 += 1\n else:\n p2 -= 1\n\n return sussyballs\n``` | 0 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
Alternative O(N) solution | container-with-most-water | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe need to have a sorted (max to min) heights and their corresponding indices.\nOnce we have these values, we can iterate from the max heights to min heights, where the min heights will be the limiting height for any possible position pairs iterated before.\nThus, we only need to maintain the min and max positions, and calculate a pseudo area: (max-min) * current_height.\nWe only need to go through the sorted heights once to obtain the max area.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n- We first use a dictionary ("record") to store the height as keys and corresponding positions as list of values.\n- We sort the key of the dictornary from highest to lowest ("sorted_h").\n- We process the dictionary items using keys from "sorted_h" from left to right. Every time, we find a min position and max position, and use (max-min) * current_height to calculate an area that potentially be the max area.\n\n\n\n# Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(N)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n record = {}\n for i in range(len(height)): # store all height in dict with pos list (if repeat)\n h = height[i]\n if h not in record:\n record[h] = []\n record[h].append(i)\n sorted_h = sorted(record.keys()), reverse=True)\n\n # process the first one\n items = record[sorted_h[0]]\n if len(items) > 1:\n min_pos = min(items)\n max_pos = max(items)\n max_area = (max_pos - min_pos) * sorted_h[0]\n else:\n min_pos = items[0]\n max_pos = items[0]\n max_area = 0\n\n # process subsequent items\n for i in sorted_h[1:]:\n items = record[i]\n min_pos = min(items+[min_pos])\n max_pos = max(items+[max_pos])\n area = (max_pos - min_pos) * i\n max_area = max(area, max_area)\n\n return max_area\n\n\n\n\n\n\n\n\n \n \n \n``` | 0 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
Alternative O(N) solution | container-with-most-water | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe need to have a sorted (max to min) heights and their corresponding indices.\nOnce we have these values, we can iterate from the max heights to min heights, where the min heights will be the limiting height for any possible position pairs iterated before.\nThus, we only need to maintain the min and max positions, and calculate a pseudo area: (max-min) * current_height.\nWe only need to go through the sorted heights once to obtain the max area.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n- We first use a dictionary ("record") to store the height as keys and corresponding positions as list of values.\n- We sort the key of the dictornary from highest to lowest ("sorted_h").\n- We process the dictionary items using keys from "sorted_h" from left to right. Every time, we find a min position and max position, and use (max-min) * current_height to calculate an area that potentially be the max area.\n\n\n\n# Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(N)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n record = {}\n for i in range(len(height)): # store all height in dict with pos list (if repeat)\n h = height[i]\n if h not in record:\n record[h] = []\n record[h].append(i)\n sorted_h = sorted(record.keys()), reverse=True)\n\n # process the first one\n items = record[sorted_h[0]]\n if len(items) > 1:\n min_pos = min(items)\n max_pos = max(items)\n max_area = (max_pos - min_pos) * sorted_h[0]\n else:\n min_pos = items[0]\n max_pos = items[0]\n max_area = 0\n\n # process subsequent items\n for i in sorted_h[1:]:\n items = record[i]\n min_pos = min(items+[min_pos])\n max_pos = max(items+[max_pos])\n area = (max_pos - min_pos) * i\n max_area = max(area, max_area)\n\n return max_area\n\n\n\n\n\n\n\n\n \n \n \n``` | 0 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
Python3 | container-with-most-water | 0 | 1 | # Complexity\n- Time complexity: O(n)\n\n# Code\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n left, right = 0, len(height) - 1 # Initialize two pointers, left and right, pointing to the start and end of the height list respectively.\n out = 0 # Initialize the maximum area to 0.\n\n while left < right: # Continue the loop until the pointers meet or cross each other.\n area = min(height[left], height[right]) * (right - left) # Calculate the area of the container formed by the current left and right boundaries.\n out = max(out, area) # Update the maximum area if the current area is greater.\n\n if height[left] < height[right]: # If the height at the left pointer is smaller than the height at the right pointer:\n left += 1 # Move the left pointer to the right.\n else: # If the height at the left pointer is greater than or equal to the height at the right pointer:\n right -= 1 # Move the right pointer to the left.\n\n return out # Return the maximum area of water that can be contained.\n\n``` | 1 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
Python3 | container-with-most-water | 0 | 1 | # Complexity\n- Time complexity: O(n)\n\n# Code\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n left, right = 0, len(height) - 1 # Initialize two pointers, left and right, pointing to the start and end of the height list respectively.\n out = 0 # Initialize the maximum area to 0.\n\n while left < right: # Continue the loop until the pointers meet or cross each other.\n area = min(height[left], height[right]) * (right - left) # Calculate the area of the container formed by the current left and right boundaries.\n out = max(out, area) # Update the maximum area if the current area is greater.\n\n if height[left] < height[right]: # If the height at the left pointer is smaller than the height at the right pointer:\n left += 1 # Move the left pointer to the right.\n else: # If the height at the left pointer is greater than or equal to the height at the right pointer:\n right -= 1 # Move the right pointer to the left.\n\n return out # Return the maximum area of water that can be contained.\n\n``` | 1 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
Beats 99.72% of code | container-with-most-water | 0 | 1 | # Intuition\nUsing two-pointer is the best approach to solve this problem.\n\n\n# Complexity\n- Time complexity:\n- Big(N)\n\n- Space complexity:\n- Big(1)\n\n# Code\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n l = 0\n size = len(height) - 1\n r = size\n maxAmount = 0\n while l < r:\n temp = height[r] * size if height[l] > height[r] else height[l] * size\n \n if height[l] < height[r]:\n l += 1\n else:\n r -= 1\n\n if temp > maxAmount:\n maxAmount = temp\n size -= 1\n \n return maxAmount\n```\n\n# Please Upvote if you find it useful | 1 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
Beats 99.72% of code | container-with-most-water | 0 | 1 | # Intuition\nUsing two-pointer is the best approach to solve this problem.\n\n\n# Complexity\n- Time complexity:\n- Big(N)\n\n- Space complexity:\n- Big(1)\n\n# Code\n```\nclass Solution:\n def maxArea(self, height: List[int]) -> int:\n l = 0\n size = len(height) - 1\n r = size\n maxAmount = 0\n while l < r:\n temp = height[r] * size if height[l] > height[r] else height[l] * size\n \n if height[l] < height[r]:\n l += 1\n else:\n r -= 1\n\n if temp > maxAmount:\n maxAmount = temp\n size -= 1\n \n return maxAmount\n```\n\n# Please Upvote if you find it useful | 1 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
✅C++ Solution | | Python | | Java | | Easy Understanding | | Two-Pointer Approach | container-with-most-water | 1 | 1 | \n# Approach\n<!-- Describe your approach to solving the problem. -->\nFor maximinzing the area , breadth should be maximum , so thats why we start our "j" pointer from the last and "i" pointer to the start .\n\n# Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ --> \n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# C++\n```\nclass Solution {\npublic:\n int maxArea(vector<int>& v) {\n\n int i = 0;\n int n = v.size();\n int j = n - 1;\n int area = 0;\n\n while(j > i){\n area = max(area, min(v[i],v[j]) * abs(i - j));\n if(v[i] < v[j]) i++;\n else j--;\n }\n\n return area;\n \n }\n};\n```\n\n# Python \n```\nclass Solution:\n def maxArea(self, v):\n i = 0\n n = len(v)\n j = n - 1\n area = 0\n while j > i:\n area = max(area, min(v[i], v[j]) * abs(i - j))\n if v[i] < v[j]:\n i += 1\n else:\n j -= 1\n return area\n\n```\n\n# Java\n```\nclass Solution {\n public int maxArea(int[] arr) {\n final int N = arr.length;\n int max = 0 , area, i=0, j=N-1;\n while(j>i){\n area = arr[i] < arr[j] ? arr[i] : arr[j];\n area = (j - i) * area;\n if(max < area) max = area;\n if(arr[i] < arr[j]) i++ ;\n else j--;\n }\n return max;\n }\n}\n\n```\n# Tom need a UPVOTE : |\n\n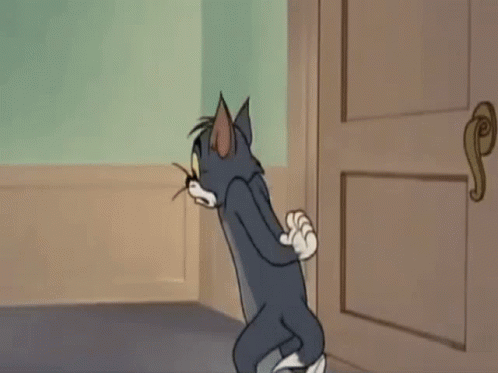\n | 35 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
✅C++ Solution | | Python | | Java | | Easy Understanding | | Two-Pointer Approach | container-with-most-water | 1 | 1 | \n# Approach\n<!-- Describe your approach to solving the problem. -->\nFor maximinzing the area , breadth should be maximum , so thats why we start our "j" pointer from the last and "i" pointer to the start .\n\n# Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ --> \n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# C++\n```\nclass Solution {\npublic:\n int maxArea(vector<int>& v) {\n\n int i = 0;\n int n = v.size();\n int j = n - 1;\n int area = 0;\n\n while(j > i){\n area = max(area, min(v[i],v[j]) * abs(i - j));\n if(v[i] < v[j]) i++;\n else j--;\n }\n\n return area;\n \n }\n};\n```\n\n# Python \n```\nclass Solution:\n def maxArea(self, v):\n i = 0\n n = len(v)\n j = n - 1\n area = 0\n while j > i:\n area = max(area, min(v[i], v[j]) * abs(i - j))\n if v[i] < v[j]:\n i += 1\n else:\n j -= 1\n return area\n\n```\n\n# Java\n```\nclass Solution {\n public int maxArea(int[] arr) {\n final int N = arr.length;\n int max = 0 , area, i=0, j=N-1;\n while(j>i){\n area = arr[i] < arr[j] ? arr[i] : arr[j];\n area = (j - i) * area;\n if(max < area) max = area;\n if(arr[i] < arr[j]) i++ ;\n else j--;\n }\n return max;\n }\n}\n\n```\n# Tom need a UPVOTE : |\n\n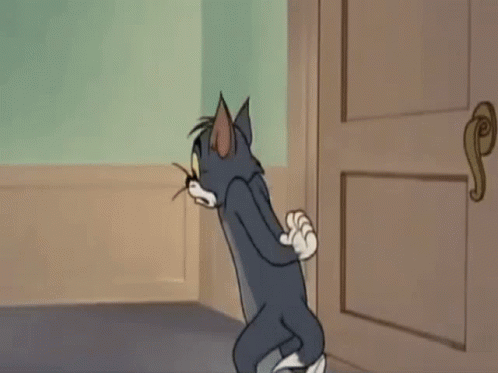\n | 35 | You are given an integer array `height` of length `n`. There are `n` vertical lines drawn such that the two endpoints of the `ith` line are `(i, 0)` and `(i, height[i])`.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return _the maximum amount of water a container can store_.
**Notice** that you may not slant the container.
**Example 1:**
**Input:** height = \[1,8,6,2,5,4,8,3,7\]
**Output:** 49
**Explanation:** The above vertical lines are represented by array \[1,8,6,2,5,4,8,3,7\]. In this case, the max area of water (blue section) the container can contain is 49.
**Example 2:**
**Input:** height = \[1,1\]
**Output:** 1
**Constraints:**
* `n == height.length`
* `2 <= n <= 105`
* `0 <= height[i] <= 104` | The aim is to maximize the area formed between the vertical lines. The area of any container is calculated using the shorter line as length and the distance between the lines as the width of the rectangle.
Area = length of shorter vertical line * distance between lines
We can definitely get the maximum width container as the outermost lines have the maximum distance between them. However, this container might not be the maximum in size as one of the vertical lines of this container could be really short. Start with the maximum width container and go to a shorter width container if there is a vertical line longer than the current containers shorter line. This way we are compromising on the width but we are looking forward to a longer length container. |
Simple Python solution with minimal lines O(n) | integer-to-roman | 0 | 1 | # Intuition\nIf the number isn\'t in the table of roman mappings, we need to find the nearest roman below it and then subtract it, then find the mapping for the rest of the number all over again. How?\n\n# Approach\nAfter creating a mapping of the requirement constraints, make a list of keys sorted reverse. Iterate down the key list til you find the nearest key lower than the number. Then take the quotient (number // key) and add the value, (quotient) times. Then make the number the remainder (number % key) and keep going until the number reaches 0.\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution:\n def intToRoman(self, num: int) -> str:\n romans = {\n 1: \'I\',\n 4: \'IV\',\n 5: \'V\',\n 9: \'IX\',\n 10: \'X\',\n 40: \'XL\',\n 50: \'L\',\n 90: \'XC\',\n 100: \'C\',\n 400: \'CD\',\n 500: \'D\',\n 900: \'CM\',\n 1000: \'M\'\n }\n \n sortedKeys = [1000, 900, 500, 400, 100, 90, 50, 40, 10, 9, 5, 4, 1]\n result = ""\n while num > 0:\n print(num)\n for key in sortedKeys:\n if num >= key:\n quo = num // key\n result += romans[key] * quo\n num = num % key\n \n return result\n\n``` | 1 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two one's added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given an integer, convert it to a roman numeral.
**Example 1:**
**Input:** num = 3
**Output:** "III "
**Explanation:** 3 is represented as 3 ones.
**Example 2:**
**Input:** num = 58
**Output:** "LVIII "
**Explanation:** L = 50, V = 5, III = 3.
**Example 3:**
**Input:** num = 1994
**Output:** "MCMXCIV "
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= num <= 3999` | null |
✔️ Python's Simple and Easy to Understand Solution | 99% Faster 🔥 | integer-to-roman | 0 | 1 | **\uD83D\uDD3C IF YOU FIND THIS POST HELPFUL PLEASE UPVOTE \uD83D\uDC4D**\n\nVisit this blog to learn Python tips and techniques and to find a Leetcode solution with an explanation: https://www.python-techs.com/\n\n**Solution:**\n```\nclass Solution:\n def intToRoman(self, num: int) -> str:\n # Creating Dictionary for Lookup\n num_map = {\n 1: "I",\n 5: "V", 4: "IV",\n 10: "X", 9: "IX",\n 50: "L", 40: "XL",\n 100: "C", 90: "XC",\n 500: "D", 400: "CD",\n 1000: "M", 900: "CM",\n }\n \n # Result Variable\n r = \'\'\n \n \n for n in [1000, 900, 500, 400, 100, 90, 50, 40, 10, 9, 5, 4, 1]:\n # If n in list then add the roman value to result variable\n while n <= num:\n r += num_map[n]\n num-=n\n return r\n```\n**Thank you for reading! \uD83D\uDE04 Comment if you have any questions or feedback.** | 170 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two one's added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given an integer, convert it to a roman numeral.
**Example 1:**
**Input:** num = 3
**Output:** "III "
**Explanation:** 3 is represented as 3 ones.
**Example 2:**
**Input:** num = 58
**Output:** "LVIII "
**Explanation:** L = 50, V = 5, III = 3.
**Example 3:**
**Input:** num = 1994
**Output:** "MCMXCIV "
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= num <= 3999` | null |
brutish but efficient | integer-to-roman | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def intToRoman(self, num: int) -> str:\n # 6 instances of substraction\n # IV, IX\n # XL, XC\n # CD, CM\n roman_numeral = ""\n thousands = num // 1000\n roman_numeral += "M"*thousands\n\n hundreds = (num % 1000) // 100\n if hundreds == 9:\n roman_numeral += "CM"\n elif hundreds == 4:\n roman_numeral += "CD"\n elif hundreds == 5:\n roman_numeral += "D"\n elif hundreds > 5:\n roman_numeral += "D"\n roman_numeral += (hundreds - 5) * "C"\n else:\n roman_numeral += "C"*hundreds\n\n tens = (num % 100) // 10\n if tens == 9:\n roman_numeral += "XC"\n elif tens == 4:\n roman_numeral += "XL"\n elif tens == 5:\n roman_numeral += "L"\n elif tens > 5:\n roman_numeral += "L"\n roman_numeral += (tens - 5) * "X"\n else:\n roman_numeral += "X"*tens\n\n ones = (num % 10)\n if ones == 9:\n roman_numeral += "IX"\n elif ones == 4:\n roman_numeral += "IV"\n elif ones == 5:\n roman_numeral += "V"\n elif ones > 5:\n roman_numeral += "V"\n roman_numeral += (ones - 5) * "I"\n else:\n roman_numeral += "I"*ones\n\n return roman_numeral\n``` | 3 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two one's added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given an integer, convert it to a roman numeral.
**Example 1:**
**Input:** num = 3
**Output:** "III "
**Explanation:** 3 is represented as 3 ones.
**Example 2:**
**Input:** num = 58
**Output:** "LVIII "
**Explanation:** L = 50, V = 5, III = 3.
**Example 3:**
**Input:** num = 1994
**Output:** "MCMXCIV "
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= num <= 3999` | null |
probably brute force but works :3 | integer-to-roman | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def intToRoman(self, num: int) -> str:\n tous = num//1000\n huns = (num-(1000*tous))//100\n tens = (num-((tous*1000)+(huns*100)))//10\n ones = (num-((tous*1000)+(huns*100)+(tens*10)))//1\n th = tous * "M"\n if huns < 4:\n hu = huns * "C"\n elif huns == 4:\n hu = "CD"\n elif huns == 5:\n hu = "D"\n elif huns < 9:\n hu = "D"+((huns-5)*"C")\n elif huns == 9:\n hu = "CM"\n\n if tens < 4:\n te = tens * "X"\n elif tens == 4:\n te = "XL"\n elif tens == 5:\n te = "L"\n elif tens < 9:\n te = "L"+((tens-5)*"X")\n elif tens == 9:\n te = "XC"\n \n if ones < 4:\n on = ones * "I"\n elif ones == 4:\n on = "IV"\n elif ones == 5:\n on = "V"\n elif ones < 9:\n on = "V"+((ones-5)*"I")\n elif ones == 9:\n on = "IX"\n \n return th+hu+te+on\n``` | 3 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two one's added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given an integer, convert it to a roman numeral.
**Example 1:**
**Input:** num = 3
**Output:** "III "
**Explanation:** 3 is represented as 3 ones.
**Example 2:**
**Input:** num = 58
**Output:** "LVIII "
**Explanation:** L = 50, V = 5, III = 3.
**Example 3:**
**Input:** num = 1994
**Output:** "MCMXCIV "
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= num <= 3999` | null |
Dict change Python solution | integer-to-roman | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def intToRoman(self, data: int) -> str:\n A = {1: \'I\', 4: \'IV\', 5: \'V\', 9: \'IX\'}\n roman = \'\'\n d = [int(i) for i in list(str(data).rjust(4, \'0\'))]\n while sum(d):\n while d[-1]:\n if d[-1] in A:\n roman = A[d[-1]] + roman\n d[-1] -= d[-1]\n else:\n roman = A[1] + roman\n d[-1] -= 1\n d = d[:-1]\n if len(d)==3:\n A = {1: \'X\', 4: \'XL\', 5: \'L\', 9: \'XC\'}\n elif len(d)==2:\n A = {1: \'C\', 4: \'CD\', 5: \'D\', 9: \'CM\'}\n elif len(d)==1:\n A = {1: \'M\'}\n return roman\n``` | 1 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two one's added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given an integer, convert it to a roman numeral.
**Example 1:**
**Input:** num = 3
**Output:** "III "
**Explanation:** 3 is represented as 3 ones.
**Example 2:**
**Input:** num = 58
**Output:** "LVIII "
**Explanation:** L = 50, V = 5, III = 3.
**Example 3:**
**Input:** num = 1994
**Output:** "MCMXCIV "
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= num <= 3999` | null |
Awesome Python Solution | integer-to-roman | 0 | 1 | \n\n# Python Solution\n```\nclass Solution:\n def intToRoman(self, num: int) -> str:\n M=["","M","MM","MMM"]\n C=["","C","CC","CCC","CD","D","DC","DCC","DCCC","CM"]\n X=["","X","XX","XXX","XL","L","LX","LXX","LXXX","XC"]\n I=["","I","II","III","IV","V","VI","VII","VIII","IX"]\n return M[num//1000]+C[num%1000//100]+X[num%1000%100//10]+I[num%1000%100%10]\n```\n# please upvote me it would encourage me alot\n | 37 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two one's added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given an integer, convert it to a roman numeral.
**Example 1:**
**Input:** num = 3
**Output:** "III "
**Explanation:** 3 is represented as 3 ones.
**Example 2:**
**Input:** num = 58
**Output:** "LVIII "
**Explanation:** L = 50, V = 5, III = 3.
**Example 3:**
**Input:** num = 1994
**Output:** "MCMXCIV "
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= num <= 3999` | null |
Beats 99.55% / Brute force / Easy to understand / Beginner code | integer-to-roman | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n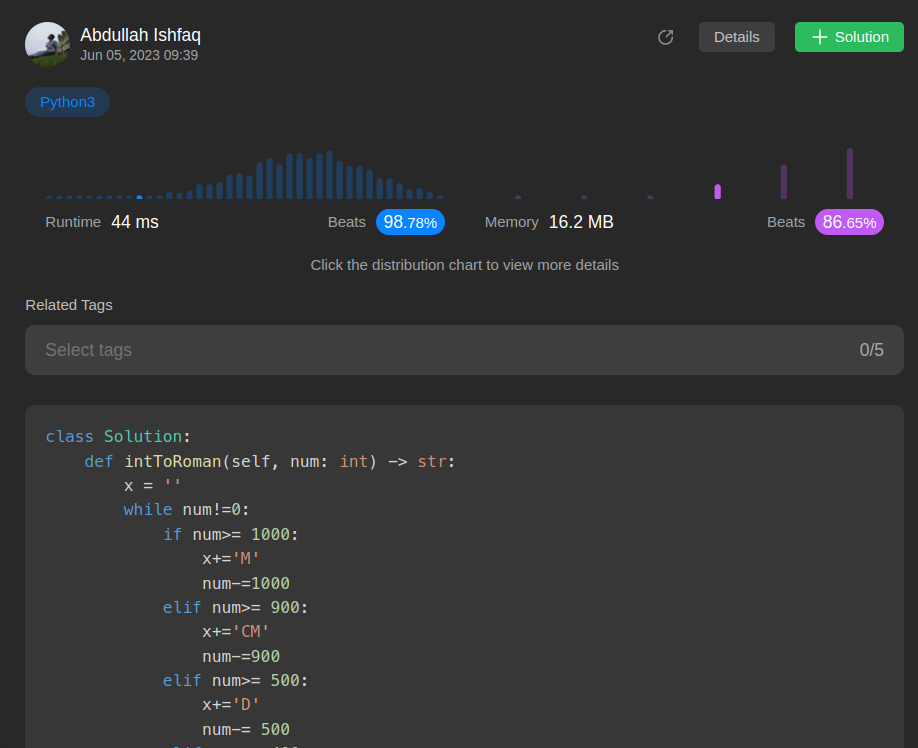\n\n# Code\n```\nclass Solution:\n def intToRoman(self, num: int) -> str:\n x = \'\'\n while num!=0:\n if num>= 1000:\n x+=\'M\'\n num-=1000\n elif num>= 900:\n x+=\'CM\'\n num-=900\n elif num>= 500:\n x+=\'D\'\n num-= 500\n elif num>= 400: \n x+=\'CD\' \n num-= 400\n elif num>= 100:\n x+=\'C\'\n num-=100\n elif num>= 90:\n x+=\'XC\' \n num-=90\n elif num>= 50:\n x+=\'L\' \n num-=50\n elif num>= 40:\n x+=\'XL\' \n num-=40\n elif num>= 10:\n x+=\'X\' \n num-=10\n elif num>= 9:\n x+=\'IX\' \n num-=9\n elif num>= 5:\n x+=\'V\' \n num-=5\n elif num>= 4:\n x+=\'IV\' \n num-=4\n else:\n x+=\'I\'\n num-=1\n return x\n``` | 8 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two one's added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given an integer, convert it to a roman numeral.
**Example 1:**
**Input:** num = 3
**Output:** "III "
**Explanation:** 3 is represented as 3 ones.
**Example 2:**
**Input:** num = 58
**Output:** "LVIII "
**Explanation:** L = 50, V = 5, III = 3.
**Example 3:**
**Input:** num = 1994
**Output:** "MCMXCIV "
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= num <= 3999` | null |
Eas Sol | integer-to-roman | 0 | 1 | \n```\nvalue = [1000,900,500,400,100,90,50,40,10,9,5,4,1]\nroman = ["M","CM","D","CD","C","XC","L","XL","X","IX","V","IV","I"]\n\nclass Solution:\n def intToRoman(self, N: int) -> str:\n ans = ""\n for i in range(13):\n while N >= value[i]:\n ans += roman[i]\n N -= value[i]\n return ans\n\n``` | 1 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two one's added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given an integer, convert it to a roman numeral.
**Example 1:**
**Input:** num = 3
**Output:** "III "
**Explanation:** 3 is represented as 3 ones.
**Example 2:**
**Input:** num = 58
**Output:** "LVIII "
**Explanation:** L = 50, V = 5, III = 3.
**Example 3:**
**Input:** num = 1994
**Output:** "MCMXCIV "
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= num <= 3999` | null |
Take this simple sol with python | integer-to-roman | 0 | 1 | # Code\n```\nclass Solution:\n def intToRoman(self, num: int) -> str:\n roman_mapping = {\n 1000: \'M\',\n 900: \'CM\',\n 500: \'D\',\n 400: \'CD\',\n 100: \'C\',\n 90: \'XC\',\n 50: \'L\',\n 40: \'XL\',\n 10: \'X\',\n 9: \'IX\',\n 5: \'V\',\n 4: \'IV\',\n 1: \'I\'\n }\n result = ""\n for value, symbol in roman_mapping.items():\n while num >= value:\n result += symbol\n num -= value\n return result\n\n``` | 6 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two one's added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given an integer, convert it to a roman numeral.
**Example 1:**
**Input:** num = 3
**Output:** "III "
**Explanation:** 3 is represented as 3 ones.
**Example 2:**
**Input:** num = 58
**Output:** "LVIII "
**Explanation:** L = 50, V = 5, III = 3.
**Example 3:**
**Input:** num = 1994
**Output:** "MCMXCIV "
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= num <= 3999` | null |
Fastest Python solution using mapping | integer-to-roman | 0 | 1 | ```\nclass Solution:\n def intToRoman(self, num: int) -> str:\n i=1\n dic={1:\'I\',5:\'V\',10:\'X\',50:\'L\',100:\'C\',500:\'D\',1000:\'M\'}\n s=""\n while num!=0:\n y=num%pow(10,i)//pow(10,i-1)\n if y==5:\n s=dic[y*pow(10,i-1)]+s\n elif y==1:\n s=dic[y*pow(10,i-1)]+s\n elif y==4:\n s=dic[1*pow(10,i-1)]+dic[5*pow(10,i-1)]+s\n elif y==9:\n s=dic[1*pow(10,i-1)]+dic[1*pow(10,i)]+s\n elif y<4:\n s=dic[pow(10,i-1)]*y+s\n elif y>5 and y<9:\n y-=5\n s=dic[5*pow(10,i-1)]+dic[pow(10,i-1)]*y+s\n num=num//pow(10,i)\n num*=pow(10,i)\n i+=1\n return s\n``` | 18 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two one's added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given an integer, convert it to a roman numeral.
**Example 1:**
**Input:** num = 3
**Output:** "III "
**Explanation:** 3 is represented as 3 ones.
**Example 2:**
**Input:** num = 58
**Output:** "LVIII "
**Explanation:** L = 50, V = 5, III = 3.
**Example 3:**
**Input:** num = 1994
**Output:** "MCMXCIV "
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= num <= 3999` | null |
✅Best Method || C++ || JAVA || PYTHON || Beginner Friendly🔥🔥🔥 | roman-to-integer | 1 | 1 | **Certainly! Let\'s break down the code and provide a clear intuition and explanation, using the examples "IX" and "XI" to demonstrate its functionality.**\n\n# Intuition:\nThe key intuition lies in the fact that in Roman numerals, when a smaller value appears before a larger value, it represents subtraction, while when a smaller value appears after or equal to a larger value, it represents addition.\n\n# Explanation:\n\n1. The unordered map `m` is created and initialized with mappings between Roman numeral characters and their corresponding integer values. For example, \'I\' is mapped to 1, \'V\' to 5, \'X\' to 10, and so on.\n2. The variable `ans` is initialized to 0. This variable will accumulate the final integer value of the Roman numeral string.\n3. The for loop iterates over each character in the input string `s`.\n **For the example "IX":**\n \n - When `i` is 0, the current character `s[i]` is \'I\'. Since there is a next character (\'X\'), and the value of \'I\' (1) is less than the value of \'X\' (10), the condition `m[s[i]] < m[s[i+1]]` is true. In this case, we subtract the value of the current character from `ans`.\n \n `ans -= m[s[i]];`\n `ans -= m[\'I\'];`\n `ans -= 1;`\n `ans` becomes -1.\n \n - When `i` is 1, the current character `s[i]` is \'X\'. This is the last character in the string, so there is no next character to compare. Since there is no next character, we don\'t need to evaluate the condition. In this case, we add the value of the current character to `ans`.\n \n `ans += m[s[i]];`\n `ans += m[\'X\'];`\n `ans += 10;`\n `ans` becomes 9.\n \n **For the example "XI":**\n \n - When `i` is 0, the current character `s[i]` is \'X\'. Since there is a next character (\'I\'), and the value of \'X\' (10) is greater than the value of \'I\' (1), the condition `m[s[i]] < m[s[i+1]]` is false. In this case, we add the value of the current character to `ans`.\n \n `ans += m[s[i]];`\n `ans += m[\'X\'];`\n `ans += 10;`\n `ans` becomes 10.\n \n - When `i` is 1, the current character `s[i]` is \'I\'. This is the last character in the string, so there is no next character to compare. Since there is no next character, we don\'t need to evaluate the condition. In this case, we add the value of the current character to `ans`.\n \n `ans += m[s[i]];`\n `ans += m[\'I\'];`\n `ans += 1;`\n `ans` becomes 11.\n\n4. After the for loop, the accumulated value in `ans` represents the integer conversion of the Roman numeral string, and it is returned as the result.\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int romanToInt(string s) {\n unordered_map<char, int> m;\n \n m[\'I\'] = 1;\n m[\'V\'] = 5;\n m[\'X\'] = 10;\n m[\'L\'] = 50;\n m[\'C\'] = 100;\n m[\'D\'] = 500;\n m[\'M\'] = 1000;\n \n int ans = 0;\n \n for(int i = 0; i < s.length(); i++){\n if(m[s[i]] < m[s[i+1]]){\n ans -= m[s[i]];\n }\n else{\n ans += m[s[i]];\n }\n }\n return ans;\n }\n};\n```\n```Java []\nclass Solution {\n public int romanToInt(String s) {\n Map<Character, Integer> m = new HashMap<>();\n \n m.put(\'I\', 1);\n m.put(\'V\', 5);\n m.put(\'X\', 10);\n m.put(\'L\', 50);\n m.put(\'C\', 100);\n m.put(\'D\', 500);\n m.put(\'M\', 1000);\n \n int ans = 0;\n \n for (int i = 0; i < s.length(); i++) {\n if (i < s.length() - 1 && m.get(s.charAt(i)) < m.get(s.charAt(i + 1))) {\n ans -= m.get(s.charAt(i));\n } else {\n ans += m.get(s.charAt(i));\n }\n }\n \n return ans;\n }\n}\n\n```\n```Python3 []\nclass Solution:\n def romanToInt(self, s: str) -> int:\n m = {\n \'I\': 1,\n \'V\': 5,\n \'X\': 10,\n \'L\': 50,\n \'C\': 100,\n \'D\': 500,\n \'M\': 1000\n }\n \n ans = 0\n \n for i in range(len(s)):\n if i < len(s) - 1 and m[s[i]] < m[s[i+1]]:\n ans -= m[s[i]]\n else:\n ans += m[s[i]]\n \n return ans\n```\n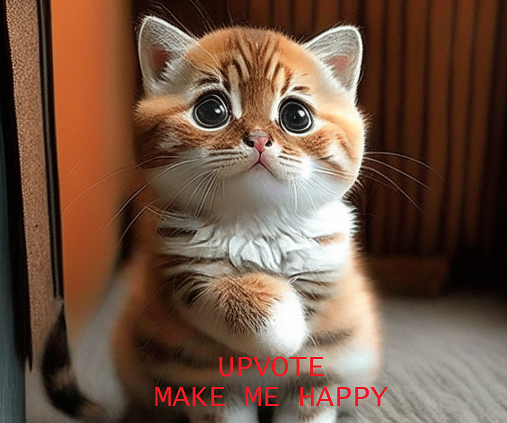\n\n**If you are a beginner solve these problems which makes concepts clear for future coding:**\n1. [Two Sum](https://leetcode.com/problems/two-sum/solutions/3619262/3-method-s-c-java-python-beginner-friendly/)\n2. [Roman to Integer](https://leetcode.com/problems/roman-to-integer/solutions/3651672/best-method-c-java-python-beginner-friendly/)\n3. [Palindrome Number](https://leetcode.com/problems/palindrome-number/solutions/3651712/2-method-s-c-java-python-beginner-friendly/)\n4. [Maximum Subarray](https://leetcode.com/problems/maximum-subarray/solutions/3666304/beats-100-c-java-python-beginner-friendly/)\n5. [Remove Element](https://leetcode.com/problems/remove-element/solutions/3670940/best-100-c-java-python-beginner-friendly/)\n6. [Contains Duplicate](https://leetcode.com/problems/contains-duplicate/solutions/3672475/4-method-s-c-java-python-beginner-friendly/)\n7. [Add Two Numbers](https://leetcode.com/problems/add-two-numbers/solutions/3675747/beats-100-c-java-python-beginner-friendly/)\n8. [Majority Element](https://leetcode.com/problems/majority-element/solutions/3676530/3-methods-beats-100-c-java-python-beginner-friendly/)\n9. [Remove Duplicates from Sorted Array](https://leetcode.com/problems/remove-duplicates-from-sorted-array/solutions/3676877/best-method-100-c-java-python-beginner-friendly/)\n10. **Practice them in a row for better understanding and please Upvote for more questions.**\n\n\n**If you found my solution helpful, I would greatly appreciate your upvote, as it would motivate me to continue sharing more solutions.**\n\n | 1,369 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two ones added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given a roman numeral, convert it to an integer.
**Example 1:**
**Input:** s = "III "
**Output:** 3
**Explanation:** III = 3.
**Example 2:**
**Input:** s = "LVIII "
**Output:** 58
**Explanation:** L = 50, V= 5, III = 3.
**Example 3:**
**Input:** s = "MCMXCIV "
**Output:** 1994
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= s.length <= 15`
* `s` contains only the characters `('I', 'V', 'X', 'L', 'C', 'D', 'M')`.
* It is **guaranteed** that `s` is a valid roman numeral in the range `[1, 3999]`. | Problem is simpler to solve by working the string from back to front and using a map. |
Hash Table Concept Python3 | roman-to-integer | 0 | 1 | \n# Hash Table in python\n```\nclass Solution:\n def romanToInt(self, s: str) -> int:\n roman={"I":1,"V":5,"X":10,"L":50,"C":100,"D":500,"M":1000}\n number=0\n for i in range(len(s)-1):\n if roman[s[i]] < roman[s[(i+1)]]:\n number-=roman[s[i]]\n else:\n number+=roman[s[i]]\n return number+roman[s[-1]]\n\n\n```\n# please upvote me it would encourage me alot\n | 323 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two ones added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given a roman numeral, convert it to an integer.
**Example 1:**
**Input:** s = "III "
**Output:** 3
**Explanation:** III = 3.
**Example 2:**
**Input:** s = "LVIII "
**Output:** 58
**Explanation:** L = 50, V= 5, III = 3.
**Example 3:**
**Input:** s = "MCMXCIV "
**Output:** 1994
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= s.length <= 15`
* `s` contains only the characters `('I', 'V', 'X', 'L', 'C', 'D', 'M')`.
* It is **guaranteed** that `s` is a valid roman numeral in the range `[1, 3999]`. | Problem is simpler to solve by working the string from back to front and using a map. |
Clean Python, beats 99.78%. | roman-to-integer | 0 | 1 | The Romans would most likely be angered by how it butchers their numeric system. Sorry guys.\n\n```Python\nclass Solution:\n def romanToInt(self, s: str) -> int:\n translations = {\n "I": 1,\n "V": 5,\n "X": 10,\n "L": 50,\n "C": 100,\n "D": 500,\n "M": 1000\n }\n number = 0\n s = s.replace("IV", "IIII").replace("IX", "VIIII")\n s = s.replace("XL", "XXXX").replace("XC", "LXXXX")\n s = s.replace("CD", "CCCC").replace("CM", "DCCCC")\n for char in s:\n number += translations[char]\n return number\n``` | 1,920 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two ones added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given a roman numeral, convert it to an integer.
**Example 1:**
**Input:** s = "III "
**Output:** 3
**Explanation:** III = 3.
**Example 2:**
**Input:** s = "LVIII "
**Output:** 58
**Explanation:** L = 50, V= 5, III = 3.
**Example 3:**
**Input:** s = "MCMXCIV "
**Output:** 1994
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= s.length <= 15`
* `s` contains only the characters `('I', 'V', 'X', 'L', 'C', 'D', 'M')`.
* It is **guaranteed** that `s` is a valid roman numeral in the range `[1, 3999]`. | Problem is simpler to solve by working the string from back to front and using a map. |
Simple solution with Hashing in Python3 | roman-to-integer | 0 | 1 | # Intuition\nHere we have `s` as **Roman Integer** and our goal is to convert it into **decimal**.\n\n# Approach\nSimply use **HashTable** to map all the **possible** combinations.\nIn many cases such combs as `\'I\'`, `\'IV\'` etc. are **valid**, we should check for **the largest**.\n\n# Complexity\n- Time complexity: **O(N)**\n\n- Space complexity: **O(1)**\n\n# Code\n```\nclass Solution:\n def romanToInt(self, s: str) -> int:\n cache = {\n "I": 1,\n "V": 5,\n "X": 10,\n "L": 50,\n "C": 100,\n "D": 500,\n "M": 1000,\n \'IV\': 4,\n \'IX\': 9,\n \'XL\': 40,\n \'XC\': 90,\n \'CD\': 400,\n \'CM\': 900\n }\n ans = 0\n cur = \'\'\n\n for token in s:\n cur += token\n\n if cur not in cache:\n ans += cache[cur[:-1]]\n cur = token\n\n if cur in cache:\n ans += cache[cur]\n\n return ans\n``` | 1 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two ones added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given a roman numeral, convert it to an integer.
**Example 1:**
**Input:** s = "III "
**Output:** 3
**Explanation:** III = 3.
**Example 2:**
**Input:** s = "LVIII "
**Output:** 58
**Explanation:** L = 50, V= 5, III = 3.
**Example 3:**
**Input:** s = "MCMXCIV "
**Output:** 1994
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= s.length <= 15`
* `s` contains only the characters `('I', 'V', 'X', 'L', 'C', 'D', 'M')`.
* It is **guaranteed** that `s` is a valid roman numeral in the range `[1, 3999]`. | Problem is simpler to solve by working the string from back to front and using a map. |
Mastering IF ELIF in Python | roman-to-integer | 0 | 1 | # SOlution in Python\n```\nclass Solution:\n def romanToInt(self, s: str) -> int:\n s += " "\n answer = 0\n for i in range(len(s)):\n if s[i] == \'I\':\n if s[i+1] in {\'V\', \'X\'}: \n answer -= 1\n else:\n answer += 1\n elif s[i] == \'X\':\n if s[i+1] in {\'L\', \'C\'}: \n answer -= 10\n else:\n answer += 10\n elif s[i] == \'C\':\n if s[i+1] in {\'D\', \'M\'}: \n answer -= 100\n else:\n answer += 100\n elif s[i] == \'V\':\n answer += 5\n elif s[i] == \'L\':\n answer += 50\n elif s[i] == \'D\':\n answer += 500\n elif s[i] == \'M\':\n answer += 1000\n return answer\n``` | 1 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two ones added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given a roman numeral, convert it to an integer.
**Example 1:**
**Input:** s = "III "
**Output:** 3
**Explanation:** III = 3.
**Example 2:**
**Input:** s = "LVIII "
**Output:** 58
**Explanation:** L = 50, V= 5, III = 3.
**Example 3:**
**Input:** s = "MCMXCIV "
**Output:** 1994
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= s.length <= 15`
* `s` contains only the characters `('I', 'V', 'X', 'L', 'C', 'D', 'M')`.
* It is **guaranteed** that `s` is a valid roman numeral in the range `[1, 3999]`. | Problem is simpler to solve by working the string from back to front and using a map. |
Python + functools.reduce + operator | roman-to-integer | 0 | 1 | # Intuition\n\nUsing `reduce` function from module `functools`\n\n# Complexity\n- Time complexity: O(n)\n\n- Space complexity: O(n)\n# Code\n```\nimport functools as fn\nimport operator as op\n\nclass Solution:\n lookup_table = {\n "I": 1,\n "V": 5,\n "X": 10,\n "L": 50,\n "C": 100,\n "D": 500,\n "M": 1000,\n }\n ops = {\n False: op.add,\n True: op.sub\n }\n def romanToInt(self, s: str) -> int:\n _, result = fn.reduce(\n lambda state, c: \n (lambda num, prev_num, result:\n (num, self.ops[prev_num > num](result, num))\n )(self.lookup_table[c], *state),\n s[::-1],\n (0, 0) # prev_num, result\n )\n return result\n``` | 1 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two ones added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given a roman numeral, convert it to an integer.
**Example 1:**
**Input:** s = "III "
**Output:** 3
**Explanation:** III = 3.
**Example 2:**
**Input:** s = "LVIII "
**Output:** 58
**Explanation:** L = 50, V= 5, III = 3.
**Example 3:**
**Input:** s = "MCMXCIV "
**Output:** 1994
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= s.length <= 15`
* `s` contains only the characters `('I', 'V', 'X', 'L', 'C', 'D', 'M')`.
* It is **guaranteed** that `s` is a valid roman numeral in the range `[1, 3999]`. | Problem is simpler to solve by working the string from back to front and using a map. |
🔥 Python || Easily Understood ✅ || Faster than 98% || Less than 76% || O(n) | roman-to-integer | 0 | 1 | Code:\n```\nclass Solution:\n def romanToInt(self, s: str) -> int:\n roman_to_integer = {\n \'I\': 1,\n \'V\': 5,\n \'X\': 10,\n \'L\': 50,\n \'C\': 100,\n \'D\': 500,\n \'M\': 1000,\n }\n s = s.replace("IV", "IIII").replace("IX", "VIIII").replace("XL", "XXXX").replace("XC", "LXXXX").replace("CD", "CCCC").replace("CM", "DCCCC")\n return sum(map(lambda x: roman_to_integer[x], s))\n```\n**Time Complexity**: `O(n)`\n**Space Complexity**: `O(1)`\n<br/>\n | 402 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two ones added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given a roman numeral, convert it to an integer.
**Example 1:**
**Input:** s = "III "
**Output:** 3
**Explanation:** III = 3.
**Example 2:**
**Input:** s = "LVIII "
**Output:** 58
**Explanation:** L = 50, V= 5, III = 3.
**Example 3:**
**Input:** s = "MCMXCIV "
**Output:** 1994
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= s.length <= 15`
* `s` contains only the characters `('I', 'V', 'X', 'L', 'C', 'D', 'M')`.
* It is **guaranteed** that `s` is a valid roman numeral in the range `[1, 3999]`. | Problem is simpler to solve by working the string from back to front and using a map. |
Easy-to-understand Solution for Python | roman-to-integer | 0 | 1 | Explanation:\n\n1- class Solution:: Defines a class named "Solution."\n\n2- def romanToInt(self, s: str) -> int:: Defines a function within the class that takes a string of Roman numerals (s) and returns an integer. The function is a method of the class (self refers to the instance of the class).\n\n3- roman_list = {\'I\': 1, \'V\': 5, \'X\': 10, \'L\': 50, \'C\': 100, \'D\': 500, \'M\': 1000}: Creates a dictionary (roman_list) that maps each Roman numeral to its corresponding integer value.\n\n4- num = 0: Initializes a variable (num) to store the resulting integer, starting with a value of zero.\n\n5- for i in range(len(s)):: Iterates through each character in the input string \'s.\'\n\n6- Inside the loop, it checks whether the current Roman numeral is greater than the one before it. If true, it subtracts twice the value of the previous numeral to account for the special case (e.g., IV or IX). Otherwise, it adds the value of the current numeral to the result.\n\n7- Finally, it returns the calculated integer value.\n# Code\n```\nclass Solution:\n def romanToInt(self, s: str) -> int:\n # Define a dictionary to map Roman numerals to their corresponding values\n roman_list = {\'I\': 1, \'V\': 5, \'X\': 10, \'L\': 50, \'C\': 100, \'D\': 500, \'M\': 1000}\n \n # Initialize a variable to store the resulting integer\n num = 0\n \n # Iterate through each character in the input string \'s\'\n for i in range(len(s)):\n # Check if the current Roman numeral is greater than the one before it\n if i > 0 and roman_list[s[i]] > roman_list[s[i - 1]]:\n # If true, subtract twice the value of the previous numeral\n num += roman_list[s[i]] - 2 * roman_list[s[i - 1]]\n else:\n # Otherwise, add the value of the current numeral to the result\n num += roman_list[s[i]]\n\n # Return the final integer result\n return num\n\n``` | 2 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two ones added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given a roman numeral, convert it to an integer.
**Example 1:**
**Input:** s = "III "
**Output:** 3
**Explanation:** III = 3.
**Example 2:**
**Input:** s = "LVIII "
**Output:** 58
**Explanation:** L = 50, V= 5, III = 3.
**Example 3:**
**Input:** s = "MCMXCIV "
**Output:** 1994
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= s.length <= 15`
* `s` contains only the characters `('I', 'V', 'X', 'L', 'C', 'D', 'M')`.
* It is **guaranteed** that `s` is a valid roman numeral in the range `[1, 3999]`. | Problem is simpler to solve by working the string from back to front and using a map. |
[VIDEO] Visualization of Two Different Approaches | roman-to-integer | 0 | 1 | https://www.youtube.com/watch?v=tsmrUi5M1JU\n\n<b>Method 1:</b>\nThis solution takes the approach incorporating the general logic of roman numerals into the algorithm. We first create a dictionary that maps each roman numeral to the corresponding integer. We also create a total variable set to 0.\n\nWe then loop over each numeral and check if the one <i>after</i> it is bigger or smaller. If it\'s bigger, we can just add it to our total. If it\'s smaller, that means we have to <i>subtract</i> it from our total instead.\n\nThe loop stops at the second to last numeral and returns the total + the last numeral (since the last numeral always has to be added)\n\n```\nclass Solution(object):\n def romanToInt(self, s):\n roman = {\n "I": 1,\n "V": 5,\n "X": 10,\n "L": 50,\n "C": 100,\n "D": 500,\n "M": 1000\n }\n total = 0\n for i in range(len(s) - 1):\n if roman[s[i]] < roman[s[i+1]]:\n total -= roman[s[i]]\n else:\n total += roman[s[i]]\n return total + roman[s[-1]]\n```\n\n<b>Method 2:</b>\nThis solution takes the approach of saying "The logic of roman numerals is too complicated. Let\'s make it easier by rewriting the numeral so that we only have to <i>add</i> and not worry about subtraction.\n\nIt starts off the same way by creating a dictionary and a total variable. It then performs substring replacement for each of the 6 possible cases that subtraction can be used and replaces it with a version that can just be added together. For example, IV is converted to IIII, so that all the digits can be added up to 4. Then we just loop through the string and add up the total.\n```\n roman = {\n "I": 1,\n "V": 5,\n "X": 10,\n "L": 50,\n "C": 100,\n "D": 500,\n "M": 1000\n }\n total = 0\n s = s.replace("IV", "IIII").replace("IX", "VIIII")\n s = s.replace("XL", "XXXX").replace("XC", "LXXXX")\n s = s.replace("CD", "CCCC").replace("CM", "DCCCC")\n for symbol in s:\n total += roman[symbol]\n return total\n``` | 12 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two ones added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given a roman numeral, convert it to an integer.
**Example 1:**
**Input:** s = "III "
**Output:** 3
**Explanation:** III = 3.
**Example 2:**
**Input:** s = "LVIII "
**Output:** 58
**Explanation:** L = 50, V= 5, III = 3.
**Example 3:**
**Input:** s = "MCMXCIV "
**Output:** 1994
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= s.length <= 15`
* `s` contains only the characters `('I', 'V', 'X', 'L', 'C', 'D', 'M')`.
* It is **guaranteed** that `s` is a valid roman numeral in the range `[1, 3999]`. | Problem is simpler to solve by working the string from back to front and using a map. |
✅🔥Beats 99.99% || 📈Easy solution with O(n) Approach ✔| C++ | Python | Java | Javascript | roman-to-integer | 1 | 1 | # \u2705Do upvote if you like the solution and explanation please \uD83D\uDE80\n\n# \u2705Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe intuition behind this code is to iterate through the Roman numeral string from right to left, converting each symbol to its corresponding integer value. If the current symbol has a smaller value than the symbol to its right, we subtract its value from the result; otherwise, we add its value to the result. By processing the string from right to left, we can handle cases where subtraction is required (e.g., IV for 4) effectively.\n\n---\n\n# \u2705Approach for code \n<!-- Describe your approach to solving the problem. -->\n1. Create an unordered map (romanValues) to store the integer values corresponding to each Roman numeral symbol (\'I\', \'V\', \'X\', \'L\', \'C\', \'D\', \'M\').\n\n2. Initialize a variable result to 0 to store the accumulated integer value.\n\n3. Iterate through the input string s from right to left (starting from the last character).\n\n4. For each character at index i, get its integer value (currValue) from the romanValues map.\n\n5. Check if the current symbol has a smaller value than the symbol to its right (i.e., currValue < romanValues[s[i + 1]]) using the condition i < s.length() - 1. If true, subtract currValue from the result; otherwise, add it to the result.\n\n6. Update the result accordingly for each symbol as you iterate through the string.\n\n7. Finally, return the accumulated result as the integer equivalent of the Roman numeral.\n\n---\n\n# \u2705Complexity\n## 1. Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe time complexity of this code is O(n), where \'n\' is the length of the input string s. This is because we iterate through the entire string once from right to left.\n## 2. Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space complexity is O(1) because the size of the romanValues map is fixed and does not depend on the input size. We use a constant amount of additional space to store the result and loop variables, so the space complexity is constant.\n\n---\n\n# \uD83D\uDCA1"If you have made it this far, I would like to kindly request that you upvote this solution to ensure its reach extends to others as well."\u2763\uFE0F\uD83D\uDCA1\n\n---\n\n# \u2705Code\n```C++ []\nclass Solution {\npublic:\n int romanToInt(string s) {\n unordered_map<char, int> romanValues = {\n {\'I\', 1},\n {\'V\', 5},\n {\'X\', 10},\n {\'L\', 50},\n {\'C\', 100},\n {\'D\', 500},\n {\'M\', 1000}\n };\n\n int result = 0;\n\n for (int i = s.length() - 1; i >= 0; i--) {\n int currValue = romanValues[s[i]];\n\n if (i < s.length() - 1 && currValue < romanValues[s[i + 1]]) {\n result -= currValue;\n } else {\n result += currValue;\n }\n }\n\n return result;\n }\n};\n\n```\n```Python []\nclass Solution:\n def romanToInt(self, s: str) -> int:\n romanValues = {\n \'I\': 1,\n \'V\': 5,\n \'X\': 10,\n \'L\': 50,\n \'C\': 100,\n \'D\': 500,\n \'M\': 1000\n }\n\n result = 0\n\n for i in range(len(s) - 1, -1, -1):\n currValue = romanValues[s[i]]\n\n if i < len(s) - 1 and currValue < romanValues[s[i + 1]]:\n result -= currValue\n else:\n result += currValue\n\n return result\n\n```\n```Java []\nimport java.util.HashMap;\nimport java.util.Map;\n\nclass Solution {\n public int romanToInt(String s) {\n Map<Character, Integer> romanValues = new HashMap<>();\n romanValues.put(\'I\', 1);\n romanValues.put(\'V\', 5);\n romanValues.put(\'X\', 10);\n romanValues.put(\'L\', 50);\n romanValues.put(\'C\', 100);\n romanValues.put(\'D\', 500);\n romanValues.put(\'M\', 1000);\n\n int result = 0;\n\n for (int i = s.length() - 1; i >= 0; i--) {\n int currValue = romanValues.get(s.charAt(i));\n\n if (i < s.length() - 1 && currValue < romanValues.get(s.charAt(i + 1))) {\n result -= currValue;\n } else {\n result += currValue;\n }\n }\n\n return result;\n }\n}\n\n```\n```Javascript []\nvar romanToInt = function(s) {\n const romanValues = {\n \'I\': 1,\n \'V\': 5,\n \'X\': 10,\n \'L\': 50,\n \'C\': 100,\n \'D\': 500,\n \'M\': 1000\n };\n\n let result = 0;\n\n for (let i = s.length - 1; i >= 0; i--) {\n const currValue = romanValues[s[i]];\n\n if (i < s.length - 1 && currValue < romanValues[s[i + 1]]) {\n result -= currValue;\n } else {\n result += currValue;\n }\n }\n\n return result;\n};\n\n```\n | 25 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two ones added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given a roman numeral, convert it to an integer.
**Example 1:**
**Input:** s = "III "
**Output:** 3
**Explanation:** III = 3.
**Example 2:**
**Input:** s = "LVIII "
**Output:** 58
**Explanation:** L = 50, V= 5, III = 3.
**Example 3:**
**Input:** s = "MCMXCIV "
**Output:** 1994
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= s.length <= 15`
* `s` contains only the characters `('I', 'V', 'X', 'L', 'C', 'D', 'M')`.
* It is **guaranteed** that `s` is a valid roman numeral in the range `[1, 3999]`. | Problem is simpler to solve by working the string from back to front and using a map. |
[python] simple solution !! complexity -O(n) | roman-to-integer | 0 | 1 | https://github.com/parasdwi/Practice/blob/main/Roman_to_Integer.py | 1 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two ones added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given a roman numeral, convert it to an integer.
**Example 1:**
**Input:** s = "III "
**Output:** 3
**Explanation:** III = 3.
**Example 2:**
**Input:** s = "LVIII "
**Output:** 58
**Explanation:** L = 50, V= 5, III = 3.
**Example 3:**
**Input:** s = "MCMXCIV "
**Output:** 1994
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= s.length <= 15`
* `s` contains only the characters `('I', 'V', 'X', 'L', 'C', 'D', 'M')`.
* It is **guaranteed** that `s` is a valid roman numeral in the range `[1, 3999]`. | Problem is simpler to solve by working the string from back to front and using a map. |
👾C#, Java, Python3,JavaScript Solution (Easy-Understanding) | roman-to-integer | 1 | 1 | ### C#,Java,Python3,JavaScript different solution with explanation\n**\u2B50[https://zyrastory.com/en/coding-en/leetcode-en/leetcode-13-roman-to-integer-solution-and-explanation-en/](https://zyrastory.com/en/coding-en/leetcode-en/leetcode-13-roman-to-integer-solution-and-explanation-en/)\u2B50**\n\n**\uD83E\uDDE1See next question solution - [Zyrastory-Longest Common Prefix](https://zyrastory.com/en/coding-en/leetcode-en/leetcode-14-longest-common-prefix-solution-and-explanation-en/)**\n\n\n#### **Example : C# Code ( You can also find an easier solution in the post )**\n```\npublic class Solution {\n private readonly Dictionary<char, int> dict = new Dictionary<char, int>{{\'I\',1},{\'V\',5},{\'X\',10},{\'L\',50},{\'C\',100},{\'D\',500},{\'M\',1000}};\n \n public int RomanToInt(string s) {\n \n char[] ch = s.ToCharArray();\n \n int result = 0;\n int intVal,nextIntVal;\n \n for(int i = 0; i <ch.Length ; i++){\n intVal = dict[ch[i]];\n \n if(i != ch.Length-1)\n {\n nextIntVal = dict[ch[i+1]];\n \n if(nextIntVal>intVal){\n intVal = nextIntVal-intVal;\n i = i+1;\n }\n }\n result = result + intVal;\n }\n return result;\n }\n}\n```\n**\u2B06To see other languages please click the link above\u2B06**\n\nIf you got any problem about the explanation or you need other programming language solution, please feel free to let me know (leave comment or messenger me).\n\n**Thanks!** | 25 | Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
**Symbol** **Value**
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, `2` is written as `II` in Roman numeral, just two ones added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
* `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
* `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
* `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.
Given a roman numeral, convert it to an integer.
**Example 1:**
**Input:** s = "III "
**Output:** 3
**Explanation:** III = 3.
**Example 2:**
**Input:** s = "LVIII "
**Output:** 58
**Explanation:** L = 50, V= 5, III = 3.
**Example 3:**
**Input:** s = "MCMXCIV "
**Output:** 1994
**Explanation:** M = 1000, CM = 900, XC = 90 and IV = 4.
**Constraints:**
* `1 <= s.length <= 15`
* `s` contains only the characters `('I', 'V', 'X', 'L', 'C', 'D', 'M')`.
* It is **guaranteed** that `s` is a valid roman numeral in the range `[1, 3999]`. | Problem is simpler to solve by working the string from back to front and using a map. |