input
stringlengths 50
13.9k
| output_program
stringlengths 5
655k
| output_answer
stringlengths 5
655k
| split
stringclasses 1
value | dataset
stringclasses 1
value |
---|---|---|---|---|
Move every letter in the provided string forward 10 letters through the alphabet.
If it goes past 'z', start again at 'a'.
Input will be a string with length > 0. | def move_ten(st):
a = 'abcdefghijklmnopqrstuvwxyzabcdefghij'
return (''.join([a[a.find(i)+10] for i in st])) | def move_ten(st):
a = 'abcdefghijklmnopqrstuvwxyzabcdefghij'
return (''.join([a[a.find(i)+10] for i in st])) | train | APPS_structured |
Scheduling is how the processor decides which jobs(processes) get to use the processor and for how long. This can cause a lot of problems. Like a really long process taking the entire CPU and freezing all the other processes. One solution is Shortest Job First(SJF), which today you will be implementing.
SJF works by, well, letting the shortest jobs take the CPU first. If the jobs are the same size then it is First In First Out (FIFO). The idea is that the shorter jobs will finish quicker, so theoretically jobs won't get frozen because of large jobs. (In practice they're frozen because of small jobs).
You will be implementing:
```python
def SJF(jobs, index)
```
It takes in:
1. "jobs" a non-empty array of positive integers. They represent the clock-cycles(cc) needed to finish the job.
2. "index" a positive integer. That represents the job we're interested in.
SJF returns:
1. A positive integer representing the cc it takes to complete the job at index.
Here's an example:
```
SJF([3, 10, 20, 1, 2], 0)
at 0cc [3, 10, 20, 1, 2] jobs[3] starts
at 1cc [3, 10, 20, 0, 2] jobs[3] finishes, jobs[4] starts
at 3cc [3, 10, 20, 0, 0] jobs[4] finishes, jobs[0] starts
at 6cc [0, 10, 20, 0, 0] jobs[0] finishes
```
so:
```
SJF([3,10,20,1,2], 0) == 6
``` | from operator import itemgetter
def SJF(jobs, index):
total = 0
for i, duration in sorted(enumerate(jobs), key=itemgetter(1)):
total += duration
if i == index:
return total | from operator import itemgetter
def SJF(jobs, index):
total = 0
for i, duration in sorted(enumerate(jobs), key=itemgetter(1)):
total += duration
if i == index:
return total | train | APPS_structured |
DevOps legacy roasting!
Save the business from technological purgatory.
Convert IT to DevOps, modernize application workloads, take it all to the Cloud…….
You will receive a string of workloads represented by words….some legacy and some modern mixed in with complaints from the business….your job is to burn the legacy in a disco inferno and count the value of each roasting and the number of complaints resolved.
```
complaints (in this format, case-insensitive) -> "slow!", "expensive!", "manual!", "down!", "hostage!", "security!"
```
The value is based on real or perceived pain by the business and the expense of keeping it all running.
Pull the values from the list below...
```
1970s Disco Fever Architecture………
Sort of like a design from Saturday night Fever….
( . )
) ( )
. ' . ' . ' .
( , ) (. ) ( ', )
.' ) ( . ) , ( , ) ( .
). , ( . ( ) ( , ') .' ( , )
(_,) . ), ) _) _,') (, ) '. ) ,. (' )
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
Burn Baby Burn in a Disco Inferno
Legacy is identified by the following keywords:
1000 points - COBOL of any kind whatsoever
keyword => "COBOL"
500 points - non-object oriented architecture
keyword => "nonobject"
500 points - monolithic architecture
keyword => "monolithic"
100 points - fax system dependencies
keyword => "fax"
100 points - modem dependencies
keyword => "modem"
50 points - thick client dependencies
keyword => "thickclient"
50 points - tape drive dependencies
keyword => "tape"
50 points - floppy drive dependencies
keyword => "floppy"
50 points - workloads with DevOps Anti-patterns
keyword => "oldschoolIT"
The count is based on case-insensitive words!
Return a string in the following format
->'Burn baby burn disco inferno 2400 points earned in this roasting and 2 complaints resolved!'
If there are no complaints and no legacy from above return
->'These guys are already DevOps and in the Cloud and the business is happy!'
```
If you have any doubt COBOL should be burned.. just look at
this quote from Dijkstra.
The use of COBOL cripples the mind; its teaching should, therefore, be regarded as a criminal offense. Edsger Dijkstra
For more information on how to have a disco inferno
https://www.youtube.com/watch?v=A_sY2rjxq6M
Disclaimer - this should only be attempted by trained professionals and in accordance with local ordinances. EX: Disco may be outlawed in certain countries. | legacy={
'cobol':1000, 'nonobject':500, 'monolithic':500, 'fax':100,
'modem':100, 'thickclient':50, 'tape':50, 'floppy':50, 'oldschoolit':50
}
def roast_legacy(workloads):
comp=0
for w in ['slow!','expensive!','manual!','down!','hostage!','security!']:
comp+=workloads.lower().count(w)
s=0
for k,v in legacy.items():
s+=workloads.lower().count(k)*v
if comp==s==0:
return 'These guys are already DevOps and in the Cloud and the business is happy!'
return 'Burn baby burn disco inferno {} points earned in this roasting and {} complaints resolved!'.format(s,comp) | legacy={
'cobol':1000, 'nonobject':500, 'monolithic':500, 'fax':100,
'modem':100, 'thickclient':50, 'tape':50, 'floppy':50, 'oldschoolit':50
}
def roast_legacy(workloads):
comp=0
for w in ['slow!','expensive!','manual!','down!','hostage!','security!']:
comp+=workloads.lower().count(w)
s=0
for k,v in legacy.items():
s+=workloads.lower().count(k)*v
if comp==s==0:
return 'These guys are already DevOps and in the Cloud and the business is happy!'
return 'Burn baby burn disco inferno {} points earned in this roasting and {} complaints resolved!'.format(s,comp) | train | APPS_structured |
Given a chemical formula (given as a string), return the count of each atom.
An atomic element always starts with an uppercase character, then zero or more lowercase letters, representing the name.
1 or more digits representing the count of that element may follow if the count is greater than 1. If the count is 1, no digits will follow. For example, H2O and H2O2 are possible, but H1O2 is impossible.
Two formulas concatenated together produce another formula. For example, H2O2He3Mg4 is also a formula.
A formula placed in parentheses, and a count (optionally added) is also a formula. For example, (H2O2) and (H2O2)3 are formulas.
Given a formula, output the count of all elements as a string in the following form: the first name (in sorted order), followed by its count (if that count is more than 1), followed by the second name (in sorted order), followed by its count (if that count is more than 1), and so on.
Example 1:
Input:
formula = "H2O"
Output: "H2O"
Explanation:
The count of elements are {'H': 2, 'O': 1}.
Example 2:
Input:
formula = "Mg(OH)2"
Output: "H2MgO2"
Explanation:
The count of elements are {'H': 2, 'Mg': 1, 'O': 2}.
Example 3:
Input:
formula = "K4(ON(SO3)2)2"
Output: "K4N2O14S4"
Explanation:
The count of elements are {'K': 4, 'N': 2, 'O': 14, 'S': 4}.
Note:
All atom names consist of lowercase letters, except for the first character which is uppercase.
The length of formula will be in the range [1, 1000].
formula will only consist of letters, digits, and round parentheses, and is a valid formula as defined in the problem. | class Solution(object):
loc=0
lastloc=-1
f=''
def getNext(self,formular,locked=False):
stype=0 # 0:null, 1:numeric, 2/20: Elem, 3: parenthesis
ret=0
if self.loc==self.lastloc: return (0,0)
i=self.loc
while i <len(formular):
if stype in (0,1) and formular[i].isnumeric():
ret=int(formular[i])+ret*10
stype=1
elif stype==0 and formular[i].isupper():
stype=20
ret=formular[i]
elif stype in (20,2) and formular[i].islower():
stype=2
ret+=formular[i]
elif stype==0 and formular[i] in "()":
stype=3+"()".index(formular[i])
ret=formular[i]
else: break
i+=1
if not locked:
self.lastloc=self.loc
self.loc=i
return (stype,ret)
def countOfAtoms(self, formula):
stk=[]
cnt={}
r=''
self.loc=0
n=self.getNext(formula)
while n!=(0,0):
if n[0] in (2,3,20):
stk.append([n[1],1])
elif n[0]==1:
stk[-1][1]=n[1]
elif n[0]==4:
time=1
i=-1
if self.getNext(formula,True)[0]==1:
time=self.getNext(formula)[1]
while stk[i][0]!='(':
stk[i][1]*=time
i-=1
stk[i][0]='$'
n=self.getNext(formula)
while any(stk):
n=stk.pop()
if n[0]!='$':
cnt[n[0]]=cnt.get(n[0],0)+n[1]
for i in sorted(cnt.keys()):
r+="%s%d"%(i,cnt[i]) if cnt[i]>1 else i
return r | class Solution(object):
loc=0
lastloc=-1
f=''
def getNext(self,formular,locked=False):
stype=0 # 0:null, 1:numeric, 2/20: Elem, 3: parenthesis
ret=0
if self.loc==self.lastloc: return (0,0)
i=self.loc
while i <len(formular):
if stype in (0,1) and formular[i].isnumeric():
ret=int(formular[i])+ret*10
stype=1
elif stype==0 and formular[i].isupper():
stype=20
ret=formular[i]
elif stype in (20,2) and formular[i].islower():
stype=2
ret+=formular[i]
elif stype==0 and formular[i] in "()":
stype=3+"()".index(formular[i])
ret=formular[i]
else: break
i+=1
if not locked:
self.lastloc=self.loc
self.loc=i
return (stype,ret)
def countOfAtoms(self, formula):
stk=[]
cnt={}
r=''
self.loc=0
n=self.getNext(formula)
while n!=(0,0):
if n[0] in (2,3,20):
stk.append([n[1],1])
elif n[0]==1:
stk[-1][1]=n[1]
elif n[0]==4:
time=1
i=-1
if self.getNext(formula,True)[0]==1:
time=self.getNext(formula)[1]
while stk[i][0]!='(':
stk[i][1]*=time
i-=1
stk[i][0]='$'
n=self.getNext(formula)
while any(stk):
n=stk.pop()
if n[0]!='$':
cnt[n[0]]=cnt.get(n[0],0)+n[1]
for i in sorted(cnt.keys()):
r+="%s%d"%(i,cnt[i]) if cnt[i]>1 else i
return r | train | APPS_structured |
Return the Nth Even Number
The input will not be 0. | def nth_even(n):
return (n-1)*2+0 | def nth_even(n):
return (n-1)*2+0 | train | APPS_structured |
A despotic king decided that his kingdom needed to be rid of corruption and disparity. He called his prime minister and ordered that all corrupt citizens be put to death. Moreover, he wanted this done quickly.
The wily prime minister realised that investigating every citizen to decide who was corrupt and who was not was rather difficult. So he decided on the following plan: He ordered all the citizens to appear in the court one by one and declare their wealth.
The king does not sit in the court all the time (he has other important business to attend to - for instance, meet dignitaries from neighbouring kingdoms, spend time with his family …) Whenever the king walks into the court, the prime minister pulls out the richest man who has appeared before the court so far and is still alive and beheads him for being corrupt. Since the rich are more likely to be corrupt, he hopes to get rid of most of the corrupt and the king is happy as he sees his policy being implemented enthusiastically.
Suppose the wealth of the citizens trooping into the court is
1376518911241376518911241\; 3\; 7\; 6\; 5\; 18\; 9\; 11\; 2\; 4
and the king walked in three times: the first time after the first four persons have seen the minister, the second time after the first five persons have seen the minister and, finally after the first nine persons have seen the minister.
At the king's first visit the richest person to have met the minister has wealth $7$ and he would be beheaded. At the second visit, the wealth of the richest person who has met the minister and is still alive has wealth $6$ and so he would be beheaded. At the third visit the richest person to have met the minister who is still alive has wealth $18$ and so he would be beheaded.
You may assume that the input is such that whenever the king walks in, it is always possible to behead someone.
Your aim is to write a program that will enable the prime minister to identify the richest man to have met the minister and who is still alive quickly. You may assume that no two citizens have the same wealth.
-----Input:-----
The first line of the input consists of two numbers $N$ and $M$, where $N$ is the number of citizens in the kingdom and M is the number of visits to the court by the king.
The next $N+M$ lines describe the order in which the $N$ citizens' appearances are interleaved with the $M$ visits by the king. A citizen's visit is denoted by a positive integer, signifying his wealth. You may assume that no two citizens have the same wealth. A visit by the king is denoted by $-1$.
-----Output:-----
Your output should consist of $M$ lines, where the $i^{th}$ line contains the wealth of the citizen who is beheaded at the $i^{th}$ visit of the king.
-----Constraints:-----
- $1 \leq M \leq 10000$.
- $1 \leq N \leq 100000$.
- You may assume that in $50 \%$ of the inputs $1 \leq M \leq 1000$ and $1 \leq N \leq 8000$.
-----Sample Input-----
10 3
1
3
7
6
-1
5
-1
18
9
11
2
-1
4
-----Sample Output-----
7
6
18 | # cook your dish here
n,m=map(int,input().split())
t=n+m
l=[]
while t>0:
a=int(input())
if a!=-1:
l.append(a)
else:
l.sort()
print(l[-1])
l[-1]=0
t=t-1 | # cook your dish here
n,m=map(int,input().split())
t=n+m
l=[]
while t>0:
a=int(input())
if a!=-1:
l.append(a)
else:
l.sort()
print(l[-1])
l[-1]=0
t=t-1 | train | APPS_structured |
In this Kata you need to write the method SharedBits that returns true if 2 integers share at least two '1' bits. For simplicity assume that all numbers are positive
For example
int seven = 7; //0111
int ten = 10; //1010
int fifteen = 15; //1111
SharedBits(seven, ten); //false
SharedBits(seven, fifteen); //true
SharedBits(ten, fifteen); //true
- seven and ten share only a single '1' (at index 3)
- seven and fifteen share 3 bits (at indexes 1, 2, and 3)
- ten and fifteen share 2 bits (at indexes 0 and 2)
Hint: you can do this with just string manipulation, but binary operators will make your life much easier. | def shared_bits(a, b):
a = bin(a)[2:]
b = bin(b)[2:]
if len(a) > len(b):
b = b.zfill(len(a))
else:
a = a.zfill(len(b))
a = list(a)
b = list(b)
return [x+y for x,y in zip(a,b)].count('11')>1 | def shared_bits(a, b):
a = bin(a)[2:]
b = bin(b)[2:]
if len(a) > len(b):
b = b.zfill(len(a))
else:
a = a.zfill(len(b))
a = list(a)
b = list(b)
return [x+y for x,y in zip(a,b)].count('11')>1 | train | APPS_structured |
In Programmers Army Land, people have started preparation as sports day is scheduled next week.
You are given a task to form 1 team of $k$ consecutive players, from a list of sports player whose powers are given to you.
You want your team to win this championship, so you have to chose your $k$ team players optimally i.e. there must not be any other $k$ consecutive team players who have their total power greater than your team members total power.
-----Input:-----
- The first line of the input contains a single integer $T$. $T$ denoting the number of test cases. The description of $T$ test cases is as follows.
- The next line of the input contains 2 space separated integers $N$ and $K$. $N$ denotes the total number of players and $K$ denotes the number of players allowed in a team.
- The next line of the input contains $N$ space-separated integers $A1, A2, A3...An$ where $ith$ number denotes power of $ith$ player.
Note: power of players can also be negative
-----Output:-----
- For each test-case print the total power that your selected team have(each test case output must be printed on a new line).
-----Constraints:-----
- $1 \leq T \leq 10^3$
- $1 \leq N,K \leq 10^5$
- $-10^7 \leq A1, A2, A3...An \leq 10^7$
-----Sample Input:-----
1
5 3
1 2 3 4 5
-----Sample Output:-----
12 | def maxSum(arr, n, k):
res = 0
for i in range(k):
res += arr[i]
curr_sum = res
for i in range(k, n):
curr_sum += arr[i] - arr[i-k]
res = max(res, curr_sum)
return res
for i in range(int(input())):
n,k=map(int,input().split())
arr=list(map(int,input().split()))
print(maxSum(arr,n,k)) | def maxSum(arr, n, k):
res = 0
for i in range(k):
res += arr[i]
curr_sum = res
for i in range(k, n):
curr_sum += arr[i] - arr[i-k]
res = max(res, curr_sum)
return res
for i in range(int(input())):
n,k=map(int,input().split())
arr=list(map(int,input().split()))
print(maxSum(arr,n,k)) | train | APPS_structured |
This calculator takes values that could be written in a browsers route path as a single string. It then returns the result as a number (or an error message).
Route paths use the '/' symbol so this can't be in our calculator. Instead we are using the '$' symbol as our divide operator.
You will be passed a string of any length containing numbers and operators:
* '+' = add
* '-' = subtract
* '*' = multiply
* '$' = divide
Your task is to break the string down and calculate the expression using this order of operations. (division => multiplication => subtraction => addition)
If you are given an invalid input (i.e. any character except `.0123456789+-*$`) you should return the error message:`'400: Bad request'`
### Remember:
1. Operations are infinite
2. Order of operations is imperitive
3. No `eval` or equivalent functions
**Examples:**
```python
calculate('1+1') => '2'
calculate('10$2') => '5'
calculate('1.5*3') => '4.5'
calculate('5+5+5+5') => '20'
calculate('1000$2.5$5+5-5+6$6') =>'81'
calculate('10-9p') => '400: Bad request'
```
### Further Notes - Parameters outside of this challenge:
- Brackets e.g. 10*(1+2)
- Square root, log, % or any other operators
- Unary minus (10*-1)
- Bad mathematical input (10**$10 or 5+5$)
- You may have to deal with floats
If enjoy this and want something harder please see http://www.codewars.com/kata/evaluate-mathematical-expression/ for making a much more complicated calculator. This is a good kata leading up to that. | def operation(op1, op2, op):
if op == "*":
return op1 * op2
elif op == "+":
return op1 + op2
elif op == "-":
return op1 - op2
else:
return op1 / op2
def calculate(expression):
i, lst, num = 0, [], ""
if "." in expression:
if expression.replace(".", "").isdigit():
return float(expression)
while i < len(expression):
if not expression[i].isdigit():
if expression[i] in "+-*$":
lst.append(int(num))
lst.append(expression[i])
num = ""
else:
return "400: Bad request"
else:
num += expression[i]
i += 1
if expression[i-1].isdigit():
lst.append(int(num))
length = len(lst)
while(True):
if "*" in lst and "$" in lst:
index1, index2 = lst.index('*'), lst.index('$')
if index1 < index2 and index1-1 > -1 and index1+1 < length and (type(lst[index1-1]) == int or type(lst[index1-1]) == float) and (type(lst[index1+1]) == int or type(lst[index1+1]) == float):
lst[index1-1] = operation(lst.pop(index1-1), lst.pop(index1), lst[index1-1])
elif index2-1 > -1 and index2+1 < length and (type(lst[index2-1]) == int or type(lst[index2-1]) == float) and (type(lst[index2+1]) == int or type(lst[index2+1]) == float):
lst[index2-1] = operation(lst.pop(index2-1), lst.pop(index2), lst[index2-1])
else:
return "400: Bad request"
elif "*" in lst:
index = lst.index("*")
if index-1 > -1 and index+1 < length and (type(lst[index-1]) == int or type(lst[index-1]) == float) and (type(lst[index+1]) == int or type(lst[index+1]) == float):
lst[index-1] = operation(lst.pop(index-1), lst.pop(index), lst[index-1])
else:
return "400: Bad request"
elif "$" in lst:
index = lst.index("$")
if index-1 > -1 and index+1 < length and (type(lst[index-1]) == int or type(lst[index-1]) == float) and (type(lst[index+1]) == int or type(lst[index+1]) == float):
lst[index-1] = operation(lst.pop(index-1), lst.pop(index), lst[index-1])
else:
return "400: Bad request"
elif "+" in lst and "-" in lst:
index1, index2 = lst.index('+'), lst.index('-')
if index1 < index2 and index1-1 > -1 and index1+1 < length and (type(lst[index1-1]) == int or type(lst[index1-1]) == float) and (type(lst[index1+1]) == int or type(lst[index1+1]) == float):
lst[index1-1] = operation(lst.pop(index1-1), lst.pop(index1), lst[index1-1])
elif index2-1 > -1 and index2+1 < length and (type(lst[index2-1]) == int or type(lst[index2-1]) == float) and (type(lst[index2+1]) == int or type(lst[index2+1]) == float):
lst[index2-1] = operation(lst.pop(index2-1), lst.pop(index2), lst[index2-1])
else:
return "400: Bad request"
elif "+" in lst:
index = lst.index("+")
if index-1 > -1 and index+1 < length and (type(lst[index-1]) == int or type(lst[index-1]) == float) and (type(lst[index+1]) == int or type(lst[index+1]) == float):
lst[index-1] = operation(lst.pop(index-1), lst.pop(index), lst[index-1])
else:
return "400: Bad request"
elif "-" in lst:
index = lst.index("-")
if index-1 > -1 and index+1 < length and (type(lst[index-1]) == int or type(lst[index-1]) == float) and (type(lst[index+1]) == int or type(lst[index+1]) == float):
lst[index-1] = operation(lst.pop(index-1), lst.pop(index), lst[index-1])
else:
return "400: Bad request"
else:
break
return lst.pop() | def operation(op1, op2, op):
if op == "*":
return op1 * op2
elif op == "+":
return op1 + op2
elif op == "-":
return op1 - op2
else:
return op1 / op2
def calculate(expression):
i, lst, num = 0, [], ""
if "." in expression:
if expression.replace(".", "").isdigit():
return float(expression)
while i < len(expression):
if not expression[i].isdigit():
if expression[i] in "+-*$":
lst.append(int(num))
lst.append(expression[i])
num = ""
else:
return "400: Bad request"
else:
num += expression[i]
i += 1
if expression[i-1].isdigit():
lst.append(int(num))
length = len(lst)
while(True):
if "*" in lst and "$" in lst:
index1, index2 = lst.index('*'), lst.index('$')
if index1 < index2 and index1-1 > -1 and index1+1 < length and (type(lst[index1-1]) == int or type(lst[index1-1]) == float) and (type(lst[index1+1]) == int or type(lst[index1+1]) == float):
lst[index1-1] = operation(lst.pop(index1-1), lst.pop(index1), lst[index1-1])
elif index2-1 > -1 and index2+1 < length and (type(lst[index2-1]) == int or type(lst[index2-1]) == float) and (type(lst[index2+1]) == int or type(lst[index2+1]) == float):
lst[index2-1] = operation(lst.pop(index2-1), lst.pop(index2), lst[index2-1])
else:
return "400: Bad request"
elif "*" in lst:
index = lst.index("*")
if index-1 > -1 and index+1 < length and (type(lst[index-1]) == int or type(lst[index-1]) == float) and (type(lst[index+1]) == int or type(lst[index+1]) == float):
lst[index-1] = operation(lst.pop(index-1), lst.pop(index), lst[index-1])
else:
return "400: Bad request"
elif "$" in lst:
index = lst.index("$")
if index-1 > -1 and index+1 < length and (type(lst[index-1]) == int or type(lst[index-1]) == float) and (type(lst[index+1]) == int or type(lst[index+1]) == float):
lst[index-1] = operation(lst.pop(index-1), lst.pop(index), lst[index-1])
else:
return "400: Bad request"
elif "+" in lst and "-" in lst:
index1, index2 = lst.index('+'), lst.index('-')
if index1 < index2 and index1-1 > -1 and index1+1 < length and (type(lst[index1-1]) == int or type(lst[index1-1]) == float) and (type(lst[index1+1]) == int or type(lst[index1+1]) == float):
lst[index1-1] = operation(lst.pop(index1-1), lst.pop(index1), lst[index1-1])
elif index2-1 > -1 and index2+1 < length and (type(lst[index2-1]) == int or type(lst[index2-1]) == float) and (type(lst[index2+1]) == int or type(lst[index2+1]) == float):
lst[index2-1] = operation(lst.pop(index2-1), lst.pop(index2), lst[index2-1])
else:
return "400: Bad request"
elif "+" in lst:
index = lst.index("+")
if index-1 > -1 and index+1 < length and (type(lst[index-1]) == int or type(lst[index-1]) == float) and (type(lst[index+1]) == int or type(lst[index+1]) == float):
lst[index-1] = operation(lst.pop(index-1), lst.pop(index), lst[index-1])
else:
return "400: Bad request"
elif "-" in lst:
index = lst.index("-")
if index-1 > -1 and index+1 < length and (type(lst[index-1]) == int or type(lst[index-1]) == float) and (type(lst[index+1]) == int or type(lst[index+1]) == float):
lst[index-1] = operation(lst.pop(index-1), lst.pop(index), lst[index-1])
else:
return "400: Bad request"
else:
break
return lst.pop() | train | APPS_structured |
Converting a 24-hour time like "0830" or "2030" to a 12-hour time (like "8:30 am" or "8:30 pm") sounds easy enough, right? Well, let's see if you can do it!
You will have to define a function named "to12hourtime", and you will be given a four digit time string (in "hhmm" format) as input.
Your task is to return a 12-hour time string in the form of "h:mm am" or "h:mm pm". (Of course, the "h" part will be two digits if the hour is greater than 9.)
If you like this kata, try converting 12-hour time to 24-hour time:
https://www.codewars.com/kata/converting-12-hour-time-to-24-hour-time/train/python | def to12hourtime(timestring):
h = int(timestring[:2])
return '{}:{} {}m'.format(h % 12 or 12, timestring[2:], 'ap'[h > 11]) | def to12hourtime(timestring):
h = int(timestring[:2])
return '{}:{} {}m'.format(h % 12 or 12, timestring[2:], 'ap'[h > 11]) | train | APPS_structured |
The accounts of the "Fat to Fit Club (FFC)" association are supervised by John as a volunteered accountant.
The association is funded through financial donations from generous benefactors. John has a list of
the first `n` donations: `[14, 30, 5, 7, 9, 11, 15]`
He wants to know how much the next benefactor should give to the association so that the
average of the first `n + 1` donations should reach an average of `30`.
After doing the math he found `149`. He thinks that he made a mistake.
Could you help him?
if `dons = [14, 30, 5, 7, 9, 11, 15]` then `new_avg(dons, 30) --> 149`
The function `new_avg(arr, navg)` should return the expected donation
(rounded up to the next integer) that will permit to reach the average `navg`.
Should the last donation be a non positive number `(<= 0)` John wants us to throw (or raise) an error or
- return Nothing in Haskell
- return None in F#, Ocaml, Scala
- return `-1` in C, Fortran, Nim, PowerShell, Go, Prolog
- echo `ERROR` in Shell
- raise-argument-error in Racket
so that he clearly sees that his expectations are not great enough.
Notes:
- all donations and `navg` are numbers (integers or floats), `arr` can be empty.
- See examples below and "Test Samples" to see which return is to be done.
```
new_avg([14, 30, 5, 7, 9, 11, 15], 92) should return 645
new_avg([14, 30, 5, 7, 9, 11, 15], 2)
should raise an error (ValueError or invalid_argument or argument-error or DomainError)
or return `-1` or ERROR or Nothing or None depending on the language.
``` | def new_avg(arr, newavg):
extra = newavg*float(len(arr) + 1) - sum(arr)
if extra < 0:
raise ValueError
return int(extra + 0.999) | def new_avg(arr, newavg):
extra = newavg*float(len(arr) + 1) - sum(arr)
if extra < 0:
raise ValueError
return int(extra + 0.999) | train | APPS_structured |
You are given $N$ gears numbered $1$ through $N$. For each valid $i$, gear $i$ has $A_i$ teeth. In the beginning, no gear is connected to any other. Your task is to process $M$ queries and simulate the gears' mechanism. There are three types of queries:
- Type 1: Change the number of teeth of gear $X$ to $C$.
- Type 2: Connect two gears $X$ and $Y$.
- Type 3: Find the speed of rotation of gear $Y$ if gear $X$ rotates with speed $V$.
It is known that if gear $i$ is directly connected to gear $j$ and gear $i$ rotates with speed $V$, then gear $j$ will rotate with speed $-V A_i / A_j$, where the sign of rotation speed denotes the direction of rotation (so minus here denotes rotation in the opposite direction). You may also notice that gears can be blocked in some cases. This happens when some gear would have to rotate in different directions. If a gear is connected to any blocked gear, it is also blocked. For example, if three gears are connected to each other, this configuration can not rotate at all, and if we connect a fourth gear to these three, it will also be blocked and not rotate.
-----Input-----
- The first line of the input contains two space-separated integers $N$ and $M$.
- The second line contains $N$ space-separated integers $A_1, A_2, \dots, A_N$.
- The following $M$ lines describe queries. Each of these lines begins with an integer $T$ denoting the type of the current query.
- If $T = 1$, it is followed by a space and two space-separated integers $X$ and $C$.
- If $T = 2$, it is followed by a space and two space-separated integers $X$ and $Y$.
- If $T = 3$, it is followed by a space and three space-separated integers $X$, $Y$ and $V$.
-----Output-----
For each query of type 3, print a single line containing two integers separated by a slash '/' — the numerator and denominator of the rotation speed of the given gear expressed as an irreducible fraction (even if this speed is an integer), or $0$ if the gear does not rotate at all.
-----Constraints-----
- $1 \le N \le 10^5$
- $1 \le M \le 2\cdot 10^5$
- $6 \le A_i \le 10^6$ for each valid $i$
- $1 \le X, Y \le N$
- $1 \le C, V \le 10^6$
-----Subtasks-----
Subtask #1 (30 points):
- $N \le 2,000$
- $M \le 5,000$
Subtask #2 (70 points): original constraints
-----Example Input-----
4 10
6 8 10 13
3 1 2 2
2 1 2
3 1 2 3
2 2 3
1 1 7
3 1 3 10
2 3 1
3 1 3 2
2 1 4
3 1 4 6
-----Example Output-----
0
-9/4
7/1
0
0
-----Explanation-----
For the first query of type 3, there are no connections between gears, so the answer is $0$.
For the second query of type 3, we can calculate the rotation speed using the formula $-3\cdot\frac{6}{8} = \frac{-9}{4}$.
For the third query of type 3, we can use the formula twice, so the speed of the second gear is $-10\cdot\frac{7}{8} = -\frac{35}{4}$, and the speed of the third gear is $-(-\frac{35}{4})\frac{8}{10} = \frac{7}{1}$.
For the last query of type 3, all gears are blocked. | class Dsu:
def __init__(self, v, s):
self.par = s
self.v = v
self.dr = [1] * v
self.zero = [False] * v
self.speed = []
for i in range(v):
self.speed.append([])
self.speed[i].append(i)
def find(self, i):
# if parent[i] == -1:
# return i
# else: return self.find_parent(parent, parent[i])
if i != self.par[i][0]:
org = self.par[i][0]
self.par[i][0] = self.find(self.par[i][0])
if self.zero[i] or self.zero[self.par[i][0]] or self.zero[org]:
self.zero[i] = self.zero[self.par[i][0]] = self.zero[org] = True
if org != self.par[i][0]:
self.speed[self.par[i][0]].append(i)
return self.par[i][0]
def union(self, x, y):
# def union(self, parent, x, y):
# x_set = self.find_parent(parent, x)
# y_set = self.find_parent(parent, y)
# parent[x_set] = y_set
self.rx = self.find(x)
self.ry = self.find(y)
self.sign = -self.dr[x] * self.dr[y]
if self.rx != self.ry:
if (self.par[self.rx][1]<self.par[self.ry][1]):
mx=self.ry
mn=self.rx
if (self.par[self.rx][1]>self.par[self.ry][1]):
mx=self.rx
mn=self.ry
if self.par[self.rx][1] != self.par[self.ry][1]:
self.par[mn][0] = mx
if self.zero[mn] or self.zero[mx] or self.zero[x] or self.zero[y]:
self.zero[mn] = self.zero[mx] = self.zero[x] = self.zero[y] = True
else:
for i in range(len(self.speed[mn])):
self.dr[self.speed[mn][i]] *= self.sign
org = self.par[self.speed[mn][i]][0]
if org != mx:
self.par[self.speed[mn][i]][0] = mx
self.speed[mx].append(self.speed[mn][i])
self.speed[mx].append(mn)
else:
self.par[self.ry][0] = self.rx
self.par[self.rx][1] += 1
if self.zero[self.rx] or self.zero[self.ry] or self.zero[x] or self.zero[y]:
self.zero[self.rx] = self.zero[self.ry] = self.zero[x] = self.zero[y] = True
else:
for i in range(len(self.speed[self.ry])):
self.dr[self.speed[self.ry][i]] *= self.sign
org = self.par[self.speed[self.ry][i]][0]
if org != self.rx:
self.par[self.speed[self.ry][i]][0] = self.rx
self.speed[self.rx].append(self.speed[self.ry][i])
self.speed[self.rx].append(self.ry)
else:
return
def optwo(x, y, D):
if (D.find(x) == D.find(y) and D.dr[x] == D.dr[y]):
D.zero[x] = D.zero[y] = True
D.union(x, y)
def gcd(a, b):
if a == 0:
return b
else:
return gcd(b % a, a)
def opthree(x, y, v, D):
if (D.find(x) != D.find(y)) or (D.zero[D.par[y][0]]):
print(0)
else:
g = gcd(v * speed[x], speed[y])
flag=(D.dr[x] * D.dr[y])//abs(D.dr[x] * D.dr[y])
print(str(flag * v * speed[x] // g) + "/" + str(speed[y] // g))
n, M = map(int, input().split())
speed = list(map(int, input().split()))
s = []
for i in range(n):
s.append([i, 0])
D = Dsu(n, s)
for i in range(M):
T = list(map(int, input().split()))
if (T[0] == 1):
speed[T[1] - 1] = T[2]
elif (T[0] == 2):
optwo(T[1] - 1, T[2] - 1, D)
elif (T[0] == 3):
opthree(T[1] - 1, T[2] - 1, T[3], D)
| class Dsu:
def __init__(self, v, s):
self.par = s
self.v = v
self.dr = [1] * v
self.zero = [False] * v
self.speed = []
for i in range(v):
self.speed.append([])
self.speed[i].append(i)
def find(self, i):
# if parent[i] == -1:
# return i
# else: return self.find_parent(parent, parent[i])
if i != self.par[i][0]:
org = self.par[i][0]
self.par[i][0] = self.find(self.par[i][0])
if self.zero[i] or self.zero[self.par[i][0]] or self.zero[org]:
self.zero[i] = self.zero[self.par[i][0]] = self.zero[org] = True
if org != self.par[i][0]:
self.speed[self.par[i][0]].append(i)
return self.par[i][0]
def union(self, x, y):
# def union(self, parent, x, y):
# x_set = self.find_parent(parent, x)
# y_set = self.find_parent(parent, y)
# parent[x_set] = y_set
self.rx = self.find(x)
self.ry = self.find(y)
self.sign = -self.dr[x] * self.dr[y]
if self.rx != self.ry:
if (self.par[self.rx][1]<self.par[self.ry][1]):
mx=self.ry
mn=self.rx
if (self.par[self.rx][1]>self.par[self.ry][1]):
mx=self.rx
mn=self.ry
if self.par[self.rx][1] != self.par[self.ry][1]:
self.par[mn][0] = mx
if self.zero[mn] or self.zero[mx] or self.zero[x] or self.zero[y]:
self.zero[mn] = self.zero[mx] = self.zero[x] = self.zero[y] = True
else:
for i in range(len(self.speed[mn])):
self.dr[self.speed[mn][i]] *= self.sign
org = self.par[self.speed[mn][i]][0]
if org != mx:
self.par[self.speed[mn][i]][0] = mx
self.speed[mx].append(self.speed[mn][i])
self.speed[mx].append(mn)
else:
self.par[self.ry][0] = self.rx
self.par[self.rx][1] += 1
if self.zero[self.rx] or self.zero[self.ry] or self.zero[x] or self.zero[y]:
self.zero[self.rx] = self.zero[self.ry] = self.zero[x] = self.zero[y] = True
else:
for i in range(len(self.speed[self.ry])):
self.dr[self.speed[self.ry][i]] *= self.sign
org = self.par[self.speed[self.ry][i]][0]
if org != self.rx:
self.par[self.speed[self.ry][i]][0] = self.rx
self.speed[self.rx].append(self.speed[self.ry][i])
self.speed[self.rx].append(self.ry)
else:
return
def optwo(x, y, D):
if (D.find(x) == D.find(y) and D.dr[x] == D.dr[y]):
D.zero[x] = D.zero[y] = True
D.union(x, y)
def gcd(a, b):
if a == 0:
return b
else:
return gcd(b % a, a)
def opthree(x, y, v, D):
if (D.find(x) != D.find(y)) or (D.zero[D.par[y][0]]):
print(0)
else:
g = gcd(v * speed[x], speed[y])
flag=(D.dr[x] * D.dr[y])//abs(D.dr[x] * D.dr[y])
print(str(flag * v * speed[x] // g) + "/" + str(speed[y] // g))
n, M = map(int, input().split())
speed = list(map(int, input().split()))
s = []
for i in range(n):
s.append([i, 0])
D = Dsu(n, s)
for i in range(M):
T = list(map(int, input().split()))
if (T[0] == 1):
speed[T[1] - 1] = T[2]
elif (T[0] == 2):
optwo(T[1] - 1, T[2] - 1, D)
elif (T[0] == 3):
opthree(T[1] - 1, T[2] - 1, T[3], D)
| train | APPS_structured |
Write a function that reverses the bits in an integer.
For example, the number `417` is `110100001` in binary. Reversing the binary is `100001011` which is `267`.
You can assume that the number is not negative. | def reverse_bits(n):
listbin = [bin(n)]
listbin.sort(reverse = True)
pleasework = list(''.join(listbin))
comeonguy = ''.join(pleasework)
almostanswer = comeonguy[:1:-1]
answer = int(almostanswer,2)
return answer
#bin(almost)
pass | def reverse_bits(n):
listbin = [bin(n)]
listbin.sort(reverse = True)
pleasework = list(''.join(listbin))
comeonguy = ''.join(pleasework)
almostanswer = comeonguy[:1:-1]
answer = int(almostanswer,2)
return answer
#bin(almost)
pass | train | APPS_structured |
The sum of divisors of `6` is `12` and the sum of divisors of `28` is `56`. You will notice that `12/6 = 2` and `56/28 = 2`. We shall say that `(6,28)` is a pair with a ratio of `2`. Similarly, `(30,140)` is also a pair but with a ratio of `2.4`. These ratios are simply decimal representations of fractions.
`(6,28)` and `(30,140)` are the only pairs in which `every member of a pair is 0 <= n < 200`. The sum of the lowest members of each pair is `6 + 30 = 36`.
You will be given a `range(a,b)`, and your task is to group the numbers into pairs with the same ratios. You will return the sum of the lowest member of each pair in the range. If there are no pairs. return `nil` in Ruby, `0` in python. Upper limit is `2000`.
```Haskell
solve(0,200) = 36
```
Good luck!
if you like this Kata, please try:
[Simple division](https://www.codewars.com/kata/59ec2d112332430ce9000005)
[Sub-array division](https://www.codewars.com/kata/59eb64cba954273cd4000099) | from itertools import groupby
from operator import itemgetter
def solve(a,b):
def factors(n):
seen = set([1, n])
for a in range(2, 1 + int(n ** 0.5)):
b, m = divmod(n, a)
if m == 0:
if a in seen: break
seen.add(a)
seen.add(b)
return seen
s = 0
for k, g in groupby(sorted(((sum(factors(a)) / a, a) for a in range(a, b)), key = itemgetter(0)), key = itemgetter(0)):
gl = list(g)
if len(gl) > 1:
s += min(map(itemgetter(1), gl))
return s | from itertools import groupby
from operator import itemgetter
def solve(a,b):
def factors(n):
seen = set([1, n])
for a in range(2, 1 + int(n ** 0.5)):
b, m = divmod(n, a)
if m == 0:
if a in seen: break
seen.add(a)
seen.add(b)
return seen
s = 0
for k, g in groupby(sorted(((sum(factors(a)) / a, a) for a in range(a, b)), key = itemgetter(0)), key = itemgetter(0)):
gl = list(g)
if len(gl) > 1:
s += min(map(itemgetter(1), gl))
return s | train | APPS_structured |
Given an array A of length N, your task is to find the element which repeats in A maximum number of times as well as the corresponding count. In case of ties, choose the smaller element first.
-----Input-----
First line of input contains an integer T, denoting the number of test cases. Then follows description of T cases. Each case begins with a single integer N, the length of A. Then follow N space separated integers in next line. Assume that 1 <= T <= 100, 1 <= N <= 100 and for all i in [1..N] : 1 <= A[i] <= 10000
-----Output-----
For each test case, output two space separated integers V & C. V is the value which occurs maximum number of times and C is its count.
-----Example-----
Input:
2
5
1 2 3 2 5
6
1 2 2 1 1 2
Output:
2 2
1 3
Description:
In first case 2 occurs twice whereas all other elements occur only once.
In second case, both 1 and 2 occur 3 times but 1 is smaller than 2. | n=int(input())
while(n):
size=int(input())
a=[]
a=input().split()
i=0
#b=len(a)*[0]
while(i<len(a)):
a[i]=int(a[i])
#print 'hello'
i+=1
j=max(a)
freq=[]
freq=(j+1)*[0]
k=0
while(k<len(a)):
if(a[k]!=0):
freq[a[k]-1]+=1
k+=1
count=max(freq)
key=freq.index(count)+1
print(key,count)
n-=1
| n=int(input())
while(n):
size=int(input())
a=[]
a=input().split()
i=0
#b=len(a)*[0]
while(i<len(a)):
a[i]=int(a[i])
#print 'hello'
i+=1
j=max(a)
freq=[]
freq=(j+1)*[0]
k=0
while(k<len(a)):
if(a[k]!=0):
freq[a[k]-1]+=1
k+=1
count=max(freq)
key=freq.index(count)+1
print(key,count)
n-=1
| train | APPS_structured |
# Task
You are given n rectangular boxes, the ith box has the length lengthi, the width widthi and the height heighti.
Your task is to check if it is possible to pack all boxes into one so that inside each box there is no more than one another box (which, in turn, can contain at most one another box, and so on).
More formally, your task is to check whether there is such sequence of n different numbers pi (1 ≤ pi ≤ n) that for each 1 ≤ i < n the box number pi can be put into the box number pi+1.
A box can be put into another box if all sides of the first one are less than the respective ones of the second one. You can rotate each box as you wish, i.e. you can `swap` its length, width and height if necessary.
# Example
For `length = [1, 3, 2], width = [1, 3, 2] and height = [1, 3, 2]`, the output should be `true`;
For `length = [1, 1], width = [1, 1] and height = [1, 1],` the output should be `false`;
For `length = [3, 1, 2], width = [3, 1, 2] and height = [3, 2, 1]`, the output should be `false`.
# Input/Output
- `[input]` integer array `length`
Array of positive integers.
Constraints:
`1 ≤ length.length ≤ 100,`
`1 ≤ length[i] ≤ 2000.`
- `[input]` integer array `width`
Array of positive integers.
Constraints:
`width.length = length.length,`
`1 ≤ width[i] ≤ 2000.`
- `[input]` integer array `height`
Array of positive integers.
Constraints:
`height.length = length.length,`
`1 ≤ height[i] ≤ 2000.`
- `[output]` a boolean value
`true` if it is possible to put all boxes into one, `false` otherwise. | def boxes_packing(length, width, height):
L = list(map(sorted, zip(length, width, height)))
return all(all(x<y for x,y in zip(b1, b2)) or all(x>y for x,y in zip(b1, b2)) for i,b1 in enumerate(L) for b2 in L[i+1:]) | def boxes_packing(length, width, height):
L = list(map(sorted, zip(length, width, height)))
return all(all(x<y for x,y in zip(b1, b2)) or all(x>y for x,y in zip(b1, b2)) for i,b1 in enumerate(L) for b2 in L[i+1:]) | train | APPS_structured |
Yup!! The problem name reflects your task; just add a set of numbers. But you may feel yourselves condescended, to write a C/C++ program just to add a set of numbers. Such a problem will simply question your erudition. So, lets add some flavor of ingenuity to it. Addition operation requires cost now, and the cost is the summation of those two to be added. So,to add 1 and 10, you need a cost of 11. If you want to add 1, 2 and 3. There are several ways
```c++
1 + 2 = 3, cost = 3,
3 + 3 = 6, cost = 6,
Total = 9.
```
```c++
1 + 3 = 4, cost = 4,
2 + 4 = 6, cost = 6,
Total = 10.
```
```c++
2 + 3 = 5, cost = 5,
1 + 5 = 6, cost = 6,
Total = 11.
```
I hope you have understood already your mission, to add a set of integers so that the cost is minimal
# Your Task
Given a vector of integers, return the minimum total cost of addition. | from heapq import *
def add_all(lst):
heapify(lst)
res = 0
while len(lst) >= 2:
s = heappop(lst) + heappop(lst)
res += s
heappush(lst, s)
return res | from heapq import *
def add_all(lst):
heapify(lst)
res = 0
while len(lst) >= 2:
s = heappop(lst) + heappop(lst)
res += s
heappush(lst, s)
return res | train | APPS_structured |
Create a function which answers the question "Are you playing banjo?".
If your name starts with the letter "R" or lower case "r", you are playing banjo!
The function takes a name as its only argument, and returns one of the following strings:
```
name + " plays banjo"
name + " does not play banjo"
```
Names given are always valid strings. | def areYouPlayingBanjo(name):
return name + " plays banjo" if name[0].upper()== 'R' else name + ' does not play banjo' | def areYouPlayingBanjo(name):
return name + " plays banjo" if name[0].upper()== 'R' else name + ' does not play banjo' | train | APPS_structured |
# Task
The number is considered to be `unlucky` if it does not have digits `4` and `7` and is divisible by `13`. Please count all unlucky numbers not greater than `n`.
# Example
For `n = 20`, the result should be `2` (numbers `0 and 13`).
For `n = 100`, the result should be `7` (numbers `0, 13, 26, 39, 52, 65, and 91`)
# Input/Output
- `[input]` integer `n`
`1 ≤ n ≤ 10^8(10^6 in Python)`
- `[output]` an integer | def unlucky_number(n):
return sum(x % 13 == 0 and '4' not in str(x) and '7' not in str(x) for x in range(n+1)) | def unlucky_number(n):
return sum(x % 13 == 0 and '4' not in str(x) and '7' not in str(x) for x in range(n+1)) | train | APPS_structured |
The chef is trying to solve some series problems, Chef wants your help to code it. Chef has one number N. Help the chef to find N'th number in the series.
0, 1, 5, 14, 30, 55 …..
-----Input:-----
- First-line will contain $T$, the number of test cases. Then the test cases follow.
- Each test case contains a single line of input, one integer $N$.
-----Output:-----
For each test case, output as the pattern.
-----Constraints-----
- $1 \leq T \leq 10^4$
- $1 \leq N \leq 10^4$
-----Sample Input:-----
3
1
7
8
-----Sample Output:-----
0
91
140 | # your code goes here
for _ in range(int(input())):
n=int(input())
n=n-1
k=n*(n+1)*(2*n + 1)
print(int(k/6))
| # your code goes here
for _ in range(int(input())):
n=int(input())
n=n-1
k=n*(n+1)*(2*n + 1)
print(int(k/6))
| train | APPS_structured |
In this kata you will have to change every letter in a given string to the next letter in the alphabet. You will write a function `nextLetter` to do this. The function will take a single parameter `s` (string).
Examples:
```
"Hello" --> "Ifmmp"
"What is your name?" --> "Xibu jt zpvs obnf?"
"zoo" --> "app"
"zzZAaa" --> "aaABbb"
```
Note: spaces and special characters should remain the same. Capital letters should transfer in the same way but remain capitilized. | from string import ascii_lowercase as L, ascii_uppercase as U
def next_letter(s):
return s.translate(str.maketrans(L + U, L[1:] + L[0] + U[1:] + U[0])) | from string import ascii_lowercase as L, ascii_uppercase as U
def next_letter(s):
return s.translate(str.maketrans(L + U, L[1:] + L[0] + U[1:] + U[0])) | train | APPS_structured |
Young wilderness explorers set off to their first expedition led by senior explorer Russell. Explorers went into a forest, set up a camp and decided to split into groups to explore as much interesting locations as possible. Russell was trying to form groups, but ran into some difficulties...
Most of the young explorers are inexperienced, and sending them alone would be a mistake. Even Russell himself became senior explorer not long ago. Each of young explorers has a positive integer parameter $e_i$ — his inexperience. Russell decided that an explorer with inexperience $e$ can only join the group of $e$ or more people.
Now Russell needs to figure out how many groups he can organize. It's not necessary to include every explorer in one of the groups: some can stay in the camp. Russell is worried about this expedition, so he asked you to help him.
-----Input-----
The first line contains the number of independent test cases $T$($1 \leq T \leq 2 \cdot 10^5$). Next $2T$ lines contain description of test cases.
The first line of description of each test case contains the number of young explorers $N$ ($1 \leq N \leq 2 \cdot 10^5$).
The second line contains $N$ integers $e_1, e_2, \ldots, e_N$ ($1 \leq e_i \leq N$), where $e_i$ is the inexperience of the $i$-th explorer.
It's guaranteed that sum of all $N$ doesn't exceed $3 \cdot 10^5$.
-----Output-----
Print $T$ numbers, each number on a separate line.
In $i$-th line print the maximum number of groups Russell can form in $i$-th test case.
-----Example-----
Input
2
3
1 1 1
5
2 3 1 2 2
Output
3
2
-----Note-----
In the first example we can organize three groups. There will be only one explorer in each group. It's correct because inexperience of each explorer equals to $1$, so it's not less than the size of his group.
In the second example we can organize two groups. Explorers with inexperience $1$, $2$ and $3$ will form the first group, and the other two explorers with inexperience equal to $2$ will form the second group.
This solution is not unique. For example, we can form the first group using the three explorers with inexperience equal to $2$, and the second group using only one explorer with inexperience equal to $1$. In this case the young explorer with inexperience equal to $3$ will not be included in any group. | import sys
input=sys.stdin.readline
for _ in range(int(input())):
N=int(input())
e=list(map(int,input().split()))
e.sort()
ans=0
val=0
g=0
for i in range(0,N):
g+=1
val=e[i]
if g>=val:
ans+=1
g=0
val=0
print(ans)
| import sys
input=sys.stdin.readline
for _ in range(int(input())):
N=int(input())
e=list(map(int,input().split()))
e.sort()
ans=0
val=0
g=0
for i in range(0,N):
g+=1
val=e[i]
if g>=val:
ans+=1
g=0
val=0
print(ans)
| train | APPS_structured |
Zaikia has $N$ sticks of distinct positive lengths $A_1,A_2,\dots,A_N$. For no good reason at all, he wants to know if there is a triplet of sticks which when connected end-to-end will form a non-trivial triangle. Here non-trivial refers to a triangle with positive area.
Help Zaikia know if such a triplet exists or not. If such a triplet exists, help him find the lexicographically largest applicable triplet.Input
- The first line contains an integer $N$.
- The second line contains $N$ space-seperated integers $A_1,A_2,\dots,A_N$. Output
- In the first line print YES if a triplet exists or NO if it doesn't.
- If such a triplet exists, then in the second line print the lexicographically largest applicable triplet.Constraints
- $3 \leq N \leq {2}\times{10}^{5}$
- $1 \leq A_i \leq {10}^{9}$ for each valid $i$Sample Input 1
5
4 2 10 3 5
Sample Output 1
YES
5 4 3
Explanation 1
There are three unordered triplets of sticks which can be used to create a triangle:
- $4,2,3$
- $4,2,5$
- $4,3,5$
Arranging them in lexicographically largest fashion
- $4,3,2$
- $5,4,2$
- $5,4,3$
Here $5,4,3$ is the lexicographically largest so it is the triplet which dristiron wantsSample Input 2
5
1 2 4 8 16
Sample Output 2
NO
Explanation 2
There are no triplets of sticks here that can be used to create a triangle. | # cook your dish here
n=int(input())
arr=list(map(int,input().split()))
arr.sort()
a,b,c=0,0,0
temp=1
for i in range(n-2):
if(arr[i]+arr[i+1]>arr[i+2]):
a=arr[i+2]
b=arr[i+1]
c=arr[i]
temp=0
if(temp==1):
print('NO')
else:
print('YES')
print(a,b,c)
| # cook your dish here
n=int(input())
arr=list(map(int,input().split()))
arr.sort()
a,b,c=0,0,0
temp=1
for i in range(n-2):
if(arr[i]+arr[i+1]>arr[i+2]):
a=arr[i+2]
b=arr[i+1]
c=arr[i]
temp=0
if(temp==1):
print('NO')
else:
print('YES')
print(a,b,c)
| train | APPS_structured |
I've got a crazy mental illness.
I dislike numbers a lot. But it's a little complicated:
The number I'm afraid of depends on which day of the week it is...
This is a concrete description of my mental illness:
Monday --> 12
Tuesday --> numbers greater than 95
Wednesday --> 34
Thursday --> 0
Friday --> numbers divisible by 2
Saturday --> 56
Sunday --> 666 or -666
Write a function which takes a string (day of the week) and an integer (number to be tested) so it tells the doctor if I'm afraid or not. (return a boolean) | def am_I_afraid(day,num):
return {'Monday' : num == 12,
'Tuesday' : num > 95,
'Wednesday' : num == 34,
'Thursday' : not num,
'Friday' : not (num % 2),
'Saturday' : num == 56,
'Sunday' : num == 666 or num == -666
}[day] | def am_I_afraid(day,num):
return {'Monday' : num == 12,
'Tuesday' : num > 95,
'Wednesday' : num == 34,
'Thursday' : not num,
'Friday' : not (num % 2),
'Saturday' : num == 56,
'Sunday' : num == 666 or num == -666
}[day] | train | APPS_structured |
You are working at a lower league football stadium and you've been tasked with automating the scoreboard.
The referee will shout out the score, you have already set up the voice recognition module which turns the ref's voice into a string, but the spoken score needs to be converted into a pair for the scoreboard!
e.g. `"The score is four nil"` should return `[4,0]`
Either teams score has a range of 0-9, and the ref won't say the same string every time e.g.
"new score: two three"
"two two"
"Arsenal just conceded another goal, two nil"
Note:
```python
Please return an array
```
Please rate and enjoy! | def scoreboard(string):
scores = {'nil': 0, 'one': 1, 'two': 2, 'three': 3, 'four': 4, 'five': 5, 'six': 6, 'seven': 7, 'eight': 8, 'nine': 9}
return [scores[x] for x in string.split() if x in scores] | def scoreboard(string):
scores = {'nil': 0, 'one': 1, 'two': 2, 'three': 3, 'four': 4, 'five': 5, 'six': 6, 'seven': 7, 'eight': 8, 'nine': 9}
return [scores[x] for x in string.split() if x in scores] | train | APPS_structured |
# Definition
**_Extra perfect number_** *is the number that* **_first_** and **_last_** *bits* are **_set bits_**.
____
# Task
**_Given_** *a positive integer* `N` , **_Return_** the **_extra perfect numbers_** *in range from* `1` to `N` .
____
# Warm-up (Highly recommended)
# [Playing With Numbers Series](https://www.codewars.com/collections/playing-with-numbers)
___
# Notes
* **_Number_** *passed is always* **_Positive_** .
* **_Returned array/list_** should *contain the extra perfect numbers in ascending order* **from lowest to highest**
___
# Input >> Output Examples
```
extraPerfect(3) ==> return {1,3}
```
## **_Explanation_**:
# (1)10 =(1)2
**First** and **last** bits as **_set bits_**.
# (3)10 = (11)2
**First** and **last** bits as **_set bits_**.
___
```
extraPerfect(7) ==> return {1,3,5,7}
```
## **_Explanation_**:
# (5)10 = (101)2
**First** and **last** bits as **_set bits_**.
# (7)10 = (111)2
**First** and **last** bits as **_set bits_**.
___
___
___
# [Playing with Numbers Series](https://www.codewars.com/collections/playing-with-numbers)
# [Playing With Lists/Arrays Series](https://www.codewars.com/collections/playing-with-lists-slash-arrays)
# [For More Enjoyable Katas](http://www.codewars.com/users/MrZizoScream/authored)
___
## ALL translations are welcomed
## Enjoy Learning !!
# Zizou | def extra_perfect(n):
n = n if n % 2 != 0 else n-1
F = []
i = 0
while 2*i + 1 <= n:
F.append(2*i+1)
i += 1
return F | def extra_perfect(n):
n = n if n % 2 != 0 else n-1
F = []
i = 0
while 2*i + 1 <= n:
F.append(2*i+1)
i += 1
return F | train | APPS_structured |
At a lemonade stand, each lemonade costs $5.
Customers are standing in a queue to buy from you, and order one at a time (in the order specified by bills).
Each customer will only buy one lemonade and pay with either a $5, $10, or $20 bill. You must provide the correct change to each customer, so that the net transaction is that the customer pays $5.
Note that you don't have any change in hand at first.
Return true if and only if you can provide every customer with correct change.
Example 1:
Input: [5,5,5,10,20]
Output: true
Explanation:
From the first 3 customers, we collect three $5 bills in order.
From the fourth customer, we collect a $10 bill and give back a $5.
From the fifth customer, we give a $10 bill and a $5 bill.
Since all customers got correct change, we output true.
Example 2:
Input: [5,5,10]
Output: true
Example 3:
Input: [10,10]
Output: false
Example 4:
Input: [5,5,10,10,20]
Output: false
Explanation:
From the first two customers in order, we collect two $5 bills.
For the next two customers in order, we collect a $10 bill and give back a $5 bill.
For the last customer, we can't give change of $15 back because we only have two $10 bills.
Since not every customer received correct change, the answer is false.
Note:
0 <= bills.length <= 10000
bills[i] will be either 5, 10, or 20. | class Solution:
def lemonadeChange(self, bills: List[int]) -> bool:
five, ten = 0, 0
for bill in bills:
if bill == 5:
five += 1
elif bill == 10:
five -= 1
ten += 1
# now last two cases handle if it is a 20
# if we have a ten give that and one five
elif ten > 0:
five -= 1
ten -= 1
# last option is to give 3 fives
else:
five -= 3
# check if five is under zero then return false
if five < 0:
return False
return True
| class Solution:
def lemonadeChange(self, bills: List[int]) -> bool:
five, ten = 0, 0
for bill in bills:
if bill == 5:
five += 1
elif bill == 10:
five -= 1
ten += 1
# now last two cases handle if it is a 20
# if we have a ten give that and one five
elif ten > 0:
five -= 1
ten -= 1
# last option is to give 3 fives
else:
five -= 3
# check if five is under zero then return false
if five < 0:
return False
return True
| train | APPS_structured |
Given a list of non negative integers, arrange them such that they form the largest number.
Example 1:
Input: [10,2]
Output: "210"
Example 2:
Input: [3,30,34,5,9]
Output: "9534330"
Note: The result may be very large, so you need to return a string instead of an integer. | class Solution:
def largestNumber(self, nums):
"""
:type nums: List[int]
:rtype: str
"""
s=self.sort(nums)
if s.startswith("0"):
return "0"
else:return s
def sort(self,nums):
if not nums:return ""
else:
pivot=nums[0]
left=[]
right=[]
for i in range(1,len(nums)):
if self.compare(nums[i],pivot):
left.append(nums[i])
else:
right.append(nums[i])
return self.sort(left)+str(pivot)+self.sort(right)
def compare(self,num1,num2):
num1,num2=str(num1),str(num2)
return int(num1+num2)>int(num2+num1) | class Solution:
def largestNumber(self, nums):
"""
:type nums: List[int]
:rtype: str
"""
s=self.sort(nums)
if s.startswith("0"):
return "0"
else:return s
def sort(self,nums):
if not nums:return ""
else:
pivot=nums[0]
left=[]
right=[]
for i in range(1,len(nums)):
if self.compare(nums[i],pivot):
left.append(nums[i])
else:
right.append(nums[i])
return self.sort(left)+str(pivot)+self.sort(right)
def compare(self,num1,num2):
num1,num2=str(num1),str(num2)
return int(num1+num2)>int(num2+num1) | train | APPS_structured |
###Story
Sometimes we are faced with problems when we have a big nested dictionary with which it's hard to work. Now, we need to solve this problem by writing a function that will flatten a given dictionary.
###Info
Python dictionaries are a convenient data type to store and process configurations. They allow you to store data by keys to create nested structures. You are given a dictionary where the keys are strings and the values are strings or dictionaries. The goal is flatten the dictionary, but save the structures in the keys. The result should be a dictionary without the nested dictionaries. The keys should contain paths that contain the parent keys from the original dictionary. The keys in the path are separated by a `/`. If a value is an empty dictionary, then it should be replaced by an empty string `""`.
###Examples
```python
{
"name": {
"first": "One",
"last": "Drone"
},
"job": "scout",
"recent": {},
"additional": {
"place": {
"zone": "1",
"cell": "2"
}
}
}
```
The result will be:
```python
{"name/first": "One", #one parent
"name/last": "Drone",
"job": "scout", #root key
"recent": "", #empty dict
"additional/place/zone": "1", #third level
"additional/place/cell": "2"}
```
***`Input: An original dictionary as a dict.`***
***`Output: The flattened dictionary as a dict.`***
***`Precondition:
Keys in a dictionary are non-empty strings.
Values in a dictionary are strings or dicts.
root_dictionary != {}`***
```python
flatten({"key": "value"}) == {"key": "value"}
flatten({"key": {"deeper": {"more": {"enough": "value"}}}}) == {"key/deeper/more/enough": "value"}
flatten({"empty": {}}) == {"empty": ""}
``` | def flatten(dictionary, prefix=[]):
res = {}
for key in dictionary.keys():
if isinstance(dictionary[key], dict) and dictionary[key]:
res.update(flatten(dictionary[key], prefix + [key]))
else:
res["/".join(prefix + [key])] = dictionary[key] or ''
return res | def flatten(dictionary, prefix=[]):
res = {}
for key in dictionary.keys():
if isinstance(dictionary[key], dict) and dictionary[key]:
res.update(flatten(dictionary[key], prefix + [key]))
else:
res["/".join(prefix + [key])] = dictionary[key] or ''
return res | train | APPS_structured |
Mitya has a rooted tree with $n$ vertices indexed from $1$ to $n$, where the root has index $1$. Each vertex $v$ initially had an integer number $a_v \ge 0$ written on it. For every vertex $v$ Mitya has computed $s_v$: the sum of all values written on the vertices on the path from vertex $v$ to the root, as well as $h_v$ — the depth of vertex $v$, which denotes the number of vertices on the path from vertex $v$ to the root. Clearly, $s_1=a_1$ and $h_1=1$.
Then Mitya erased all numbers $a_v$, and by accident he also erased all values $s_v$ for vertices with even depth (vertices with even $h_v$). Your task is to restore the values $a_v$ for every vertex, or determine that Mitya made a mistake. In case there are multiple ways to restore the values, you're required to find one which minimizes the total sum of values $a_v$ for all vertices in the tree.
-----Input-----
The first line contains one integer $n$ — the number of vertices in the tree ($2 \le n \le 10^5$). The following line contains integers $p_2$, $p_3$, ... $p_n$, where $p_i$ stands for the parent of vertex with index $i$ in the tree ($1 \le p_i < i$). The last line contains integer values $s_1$, $s_2$, ..., $s_n$ ($-1 \le s_v \le 10^9$), where erased values are replaced by $-1$.
-----Output-----
Output one integer — the minimum total sum of all values $a_v$ in the original tree, or $-1$ if such tree does not exist.
-----Examples-----
Input
5
1 1 1 1
1 -1 -1 -1 -1
Output
1
Input
5
1 2 3 1
1 -1 2 -1 -1
Output
2
Input
3
1 2
2 -1 1
Output
-1 | import sys
input=sys.stdin.readline
import collections
from collections import defaultdict
n=int(input())
par=[ int(i) for i in input().split() if i!='\n']
suma=[int(i) for i in input().split() if i!='\n']
graph=defaultdict(list)
for i in range(n-1):
graph[i+2].append(par[i])
graph[par[i]].append(i+2)
#print(graph)
weight=[0]*(n+1)
weight[1]=suma[0]
queue=collections.deque([1])
visited=set()
visited.add(1)
ok=True
while queue:
vertex=queue.popleft()
for child in graph[vertex]:
if child not in visited:
if suma[child-1]==-1:
mina=[]
if len(graph[child])==1:
mina=[0]
else:
for j in graph[child]:
if j!=vertex:
mina.append(suma[j-1]-suma[vertex-1])
ans=suma[vertex-1]+min(mina)
suma[child-1]=ans
if suma[child-1]<suma[vertex-1]:
ok=False
else:
weight[child]=(suma[child-1]-suma[vertex-1])
else:
if suma[child-1]<suma[vertex-1]:
ok=False
else:
weight[child]=(suma[child-1]-suma[vertex-1])
#print(child,vertex,weight,suma[child-1],suma[vertex-1])
queue.append(child)
visited.add(child)
#print(weight,ok,suma)
if ok==True:
print(sum(weight))
else:
print(-1)
| import sys
input=sys.stdin.readline
import collections
from collections import defaultdict
n=int(input())
par=[ int(i) for i in input().split() if i!='\n']
suma=[int(i) for i in input().split() if i!='\n']
graph=defaultdict(list)
for i in range(n-1):
graph[i+2].append(par[i])
graph[par[i]].append(i+2)
#print(graph)
weight=[0]*(n+1)
weight[1]=suma[0]
queue=collections.deque([1])
visited=set()
visited.add(1)
ok=True
while queue:
vertex=queue.popleft()
for child in graph[vertex]:
if child not in visited:
if suma[child-1]==-1:
mina=[]
if len(graph[child])==1:
mina=[0]
else:
for j in graph[child]:
if j!=vertex:
mina.append(suma[j-1]-suma[vertex-1])
ans=suma[vertex-1]+min(mina)
suma[child-1]=ans
if suma[child-1]<suma[vertex-1]:
ok=False
else:
weight[child]=(suma[child-1]-suma[vertex-1])
else:
if suma[child-1]<suma[vertex-1]:
ok=False
else:
weight[child]=(suma[child-1]-suma[vertex-1])
#print(child,vertex,weight,suma[child-1],suma[vertex-1])
queue.append(child)
visited.add(child)
#print(weight,ok,suma)
if ok==True:
print(sum(weight))
else:
print(-1)
| train | APPS_structured |
You are given a string $S$ and an integer $L$. A operation is described as :- "You are allowed to pick any substring from first $L$ charcaters of $S$, and place it at the end of the string $S$.
A string $A$ is a substring of an string $B$ if $A$ can be obtained from $B$ by deletion of several (possibly, zero or all) characters from the beginning and several (possibly, zero or all) elements from the end.
Find the lexographically smallest string after performing this opertaion any number of times (possibly zero).
For example $S$ = "codechef" and $L=4$. Then, we can take substring "ode" from S[0-3] and place it at end of the string $S$ = "cchefode".
-----Input:-----
- First line will contain $T$, number of testcases.
- Then each of the N lines contain an integer $L$ and a string $S$.
-----Output:-----
For each testcase, output in a single line answer lexographically smallest string.
-----Constraints-----
- $1 \leq T \leq 10^4$
- $2 \leq |S| \leq 10^3$
- $1 \leq L \leq N $
-----Sample Input:-----
2
1 rga
2 cab
-----Sample Output:-----
arg
abc
-----EXPLANATION:-----
In the first testcase:
substring 'r' is picked and placed at the end of the string. rga -> gar
Then performing same operation gives :- gar -> arg | def least_rotation(S: str) -> int:
"""Booth's algorithm."""
S += S # Concatenate string to it self to avoid modular arithmetic
f = [-1] * len(S) # Failure function
k = 0 # Least rotation of string found so far
for j in range(1, len(S)):
sj = S[j]
i = f[j - k - 1]
while i != -1 and sj != S[k + i + 1]:
if sj < S[k + i + 1]:
k = j - i - 1
i = f[i]
if sj != S[k + i + 1]: # if sj != S[k+i+1], then i == -1
if sj < S[k]: # k+i+1 = k
k = j
f[j - k] = -1
else:
f[j - k] = i + 1
return k
t=int(input())
for _ in range(t):
l,s=input().split()
if(l=='1'):
k=least_rotation(s)
ans=""
n=len(s)
s+=s
for i in range(k,k+n):
ans+=s[i]
print(ans)
else:
s=sorted(s)
print(''.join(s)) | def least_rotation(S: str) -> int:
"""Booth's algorithm."""
S += S # Concatenate string to it self to avoid modular arithmetic
f = [-1] * len(S) # Failure function
k = 0 # Least rotation of string found so far
for j in range(1, len(S)):
sj = S[j]
i = f[j - k - 1]
while i != -1 and sj != S[k + i + 1]:
if sj < S[k + i + 1]:
k = j - i - 1
i = f[i]
if sj != S[k + i + 1]: # if sj != S[k+i+1], then i == -1
if sj < S[k]: # k+i+1 = k
k = j
f[j - k] = -1
else:
f[j - k] = i + 1
return k
t=int(input())
for _ in range(t):
l,s=input().split()
if(l=='1'):
k=least_rotation(s)
ans=""
n=len(s)
s+=s
for i in range(k,k+n):
ans+=s[i]
print(ans)
else:
s=sorted(s)
print(''.join(s)) | train | APPS_structured |
Given two strings S and T, each of which represents a non-negative rational number, return True if and only if they represent the same number. The strings may use parentheses to denote the repeating part of the rational number.
In general a rational number can be represented using up to three parts: an integer part, a non-repeating part, and a repeating part. The number will be represented in one of the following three ways:
<IntegerPart> (e.g. 0, 12, 123)
<IntegerPart><.><NonRepeatingPart> (e.g. 0.5, 1., 2.12, 2.0001)
<IntegerPart><.><NonRepeatingPart><(><RepeatingPart><)> (e.g. 0.1(6), 0.9(9), 0.00(1212))
The repeating portion of a decimal expansion is conventionally denoted within a pair of round brackets. For example:
1 / 6 = 0.16666666... = 0.1(6) = 0.1666(6) = 0.166(66)
Both 0.1(6) or 0.1666(6) or 0.166(66) are correct representations of 1 / 6.
Example 1:
Input: S = "0.(52)", T = "0.5(25)"
Output: true
Explanation:
Because "0.(52)" represents 0.52525252..., and "0.5(25)" represents 0.52525252525..... , the strings represent the same number.
Example 2:
Input: S = "0.1666(6)", T = "0.166(66)"
Output: true
Example 3:
Input: S = "0.9(9)", T = "1."
Output: true
Explanation:
"0.9(9)" represents 0.999999999... repeated forever, which equals 1. [See this link for an explanation.]
"1." represents the number 1, which is formed correctly: (IntegerPart) = "1" and (NonRepeatingPart) = "".
Note:
Each part consists only of digits.
The <IntegerPart> will not begin with 2 or more zeros. (There is no other restriction on the digits of each part.)
1 <= <IntegerPart>.length <= 4
0 <= <NonRepeatingPart>.length <= 4
1 <= <RepeatingPart>.length <= 4 | import math
class Solution:
def isRationalEqual(self, S: str, T: str) -> bool:
if len(S) == 0 or len(T) == 0:
return False
def process(s):
if s[-1] == '.':
s = s[:-1]
stack, repeat_9 = [], False
for i, x in enumerate(s):
if x != ')':
stack.append(x)
else:
tmp = ''
while stack[-1] != '(':
tmp += stack.pop()
if len(tmp) == tmp.count('9'):
repeat_9 = True
stack.pop()
return ''.join(stack) + tmp[::-1] * (24 // len(tmp)), repeat_9
return ''.join(stack), repeat_9
x, y = process(S), process(T)
if x[0].count('.') == 0 or y[0].count('.') == 0:
return float(x[0]) == float(y[0])
l = max(len(x[0]), len(y[0]))
if x[0][:17] == y[0][:17]:
return True
if x[1] or y[1]:
m = min(len(x[0].split('.')[1]), len(y[0].split('.')[1]))
if round(float(x[0]), m) == round(float(y[0]), m):
return True
return False
| import math
class Solution:
def isRationalEqual(self, S: str, T: str) -> bool:
if len(S) == 0 or len(T) == 0:
return False
def process(s):
if s[-1] == '.':
s = s[:-1]
stack, repeat_9 = [], False
for i, x in enumerate(s):
if x != ')':
stack.append(x)
else:
tmp = ''
while stack[-1] != '(':
tmp += stack.pop()
if len(tmp) == tmp.count('9'):
repeat_9 = True
stack.pop()
return ''.join(stack) + tmp[::-1] * (24 // len(tmp)), repeat_9
return ''.join(stack), repeat_9
x, y = process(S), process(T)
if x[0].count('.') == 0 or y[0].count('.') == 0:
return float(x[0]) == float(y[0])
l = max(len(x[0]), len(y[0]))
if x[0][:17] == y[0][:17]:
return True
if x[1] or y[1]:
m = min(len(x[0].split('.')[1]), len(y[0].split('.')[1]))
if round(float(x[0]), m) == round(float(y[0]), m):
return True
return False
| train | APPS_structured |
Devu loves to play with his dear mouse Jerry. One day they play a game on 2 dimensional grid of dimensions n * n (n ≥ 2). Jerry is currently at coordinates (sx, sy) and wants to move to location (ex, ey) where cheese is placed by Devu. Also Devu is very cunning and has placed a bomb at location (bx, by). All these three locations are distinct.
In a single move, Jerry can go either up, down, left or right in the grid such that it never goes out of the grid. Also, it has to avoid the bomb. Find out minimum number of moves Jerry needs. It is guaranteed that it is always possible to do so.
-----Input-----
- The first line of the input contains an integer T denoting the number of test cases. The description of T test cases follows."
- The first line of each test case contains seven space separated integers n, sx, sy , ex, ey, bx, by.
-----Output-----
- For each test case, output a single line containing an integer corresponding to minimum number of moves Jerry needs.
-----Constraints-----
- 1 ≤ T ≤ 1000
- 2 ≤ n ≤ 20
- 1 ≤ sx, sy , ex, ey, bx, by ≤ n
- No two or more poitns in the three points are same.
-----Example-----
Input:
2
2 1 1 2 2 1 2
3 1 1 1 3 1 2
Output:
2
4
-----Explanation-----
Example case 1. ...
Jerry will move directly (1, 1) to (2, 1) and then to (2, 2) in total 2 moves.
Example case 2. ... | for t in range(eval(input())):
n, sx, sy, ex, ey, bx, by = list(map(int, input().strip().split()))
bomb = ((sx==ex or sy==ey)
and min(sx,ex) <= bx <= max(sx, ex)
and min(sy,ey) <= by <= max(sy, ey))
print(abs(sx-ex) + abs (sy-ey) + 2 * bomb) | for t in range(eval(input())):
n, sx, sy, ex, ey, bx, by = list(map(int, input().strip().split()))
bomb = ((sx==ex or sy==ey)
and min(sx,ex) <= bx <= max(sx, ex)
and min(sy,ey) <= by <= max(sy, ey))
print(abs(sx-ex) + abs (sy-ey) + 2 * bomb) | train | APPS_structured |
There are N pinholes on the xy-plane. The i-th pinhole is located at (x_i,y_i).
We will denote the Manhattan distance between the i-th and j-th pinholes as d(i,j)(=|x_i-x_j|+|y_i-y_j|).
You have a peculiar pair of compasses, called Manhattan Compass.
This instrument always points at two of the pinholes.
The two legs of the compass are indistinguishable, thus we do not distinguish the following two states: the state where the compass points at the p-th and q-th pinholes, and the state where it points at the q-th and p-th pinholes.
When the compass points at the p-th and q-th pinholes and d(p,q)=d(p,r), one of the legs can be moved so that the compass will point at the p-th and r-th pinholes.
Initially, the compass points at the a-th and b-th pinholes.
Find the number of the pairs of pinholes that can be pointed by the compass.
-----Constraints-----
- 2≦N≦10^5
- 1≦x_i, y_i≦10^9
- 1≦a < b≦N
- When i ≠ j, (x_i, y_i) ≠ (x_j, y_j)
- x_i and y_i are integers.
-----Input-----
The input is given from Standard Input in the following format:
N a b
x_1 y_1
:
x_N y_N
-----Output-----
Print the number of the pairs of pinholes that can be pointed by the compass.
-----Sample Input-----
5 1 2
1 1
4 3
6 1
5 5
4 8
-----Sample Output-----
4
Initially, the compass points at the first and second pinholes.
Since d(1,2) = d(1,3), the compass can be moved so that it will point at the first and third pinholes.
Since d(1,3) = d(3,4), the compass can also point at the third and fourth pinholes.
Since d(1,2) = d(2,5), the compass can also point at the second and fifth pinholes.
No other pairs of pinholes can be pointed by the compass, thus the answer is 4. | N, a, b = list(map(int, input().split())); a -= 1; b -= 1
P = []
Q = []
for i in range(N):
x, y = list(map(int, input().split()))
P.append((x-y, x+y, i))
Q.append((x+y, x-y, i))
d = max(abs(P[a][0] - P[b][0]), abs(P[a][1] - P[b][1]))
*parent, = list(range(N))
def root(x):
if x == parent[x]:
return x
y = parent[x] = root(parent[x])
return y
def unite(x, y):
px = root(x); py = root(y)
if px < py:
parent[py] = px
else:
parent[px] = py
C = [0]*N
D = [0]*N
def check(P0, i0, j0):
return abs(P0[i0][0] - P0[j0][0]) == abs(P0[i0][1] - P0[j0][1])
def solve(P0):
P = P0[:]
P.sort()
s = t = 0; prev = -1
for i in range(N):
x, y, i0 = P[i]
while t < N and P[t][0] < x-d or (P[t][0] == x-d and P[t][1] <= y+d): t += 1
while s < N and (P[s][0] < x-d or (P[s][0] == x-d and P[s][1] < y-d)): s += 1
if s < t:
j0 = P[s][2]
unite(i0, j0)
if check(P0, i0, j0):
D[i0] += 1
else:
C[i0] += 1
if s < t-1:
j0 = P[t-1][2]
if check(P0, i0, j0):
D[i0] += 1
C[i0] += t-s-2
else:
C[i0] += t-s-1
for j in range(max(prev, s), t-1):
unite(P[j][2], P[j+1][2])
prev = t-1
solve(P)
solve(Q)
S = T = 0
r = root(a)
for i in range(N):
if root(i) == r:
S += C[i]; T += D[i]
print((S + T//2))
| N, a, b = list(map(int, input().split())); a -= 1; b -= 1
P = []
Q = []
for i in range(N):
x, y = list(map(int, input().split()))
P.append((x-y, x+y, i))
Q.append((x+y, x-y, i))
d = max(abs(P[a][0] - P[b][0]), abs(P[a][1] - P[b][1]))
*parent, = list(range(N))
def root(x):
if x == parent[x]:
return x
y = parent[x] = root(parent[x])
return y
def unite(x, y):
px = root(x); py = root(y)
if px < py:
parent[py] = px
else:
parent[px] = py
C = [0]*N
D = [0]*N
def check(P0, i0, j0):
return abs(P0[i0][0] - P0[j0][0]) == abs(P0[i0][1] - P0[j0][1])
def solve(P0):
P = P0[:]
P.sort()
s = t = 0; prev = -1
for i in range(N):
x, y, i0 = P[i]
while t < N and P[t][0] < x-d or (P[t][0] == x-d and P[t][1] <= y+d): t += 1
while s < N and (P[s][0] < x-d or (P[s][0] == x-d and P[s][1] < y-d)): s += 1
if s < t:
j0 = P[s][2]
unite(i0, j0)
if check(P0, i0, j0):
D[i0] += 1
else:
C[i0] += 1
if s < t-1:
j0 = P[t-1][2]
if check(P0, i0, j0):
D[i0] += 1
C[i0] += t-s-2
else:
C[i0] += t-s-1
for j in range(max(prev, s), t-1):
unite(P[j][2], P[j+1][2])
prev = t-1
solve(P)
solve(Q)
S = T = 0
r = root(a)
for i in range(N):
if root(i) == r:
S += C[i]; T += D[i]
print((S + T//2))
| train | APPS_structured |
As a member of the editorial board of the prestigous scientific Journal _Proceedings of the National Academy of Sciences_, you've decided to go back and review how well old articles you've published stand up to modern publication best practices. Specifically, you'd like to re-evaluate old findings in light of recent literature about ["researcher degrees of freedom"](http://journals.sagepub.com/doi/full/10.1177/0956797611417632).
You want to categorize all the old articles into three groups: "Fine", "Needs review" and "Pants on fire".
In order to categorize them you've enlisted an army of unpaid grad students to review and give you two data points from each study: (1) the p-value behind the paper's primary conclusions, and (2) the number of recommended author requirements to limit researcher degrees of freedom the authors satisfied:
* Authors must decide the rule for terminating data collection before data collection begins and report this rule in the article.
* Authors must collect at least 20 observations per cell or else provide a compelling cost-of-data-collection justification.
* Authors must list all variables collected in a study.
* Authors must report all experimental conditions, including failed manipulations.
* If observations are eliminated, authors must also report what the statistical results are if those observations are included.
* If an analysis includes a covariate, authors must report the statistical results of the analysis without the covariate.
Your army of tenure-hungry grad students will give you the p-value as a float between `1.0` and `0.0` exclusive, and the number of author requirements satisfied as an integer from `0` through `6` inclusive.
You've decided to write a function, `categorize_study()` to automatically categorize each study based on these two inputs using the completely scientifically legitimate "bs-factor". The bs-factor for a particular paper is calculated as follows:
* bs-factor when the authors satisfy all six requirements is 1
* bs-factor when the authors satisfy only five requirements is 2
* bs-factor when the authors satisfy only four requirements is 4
* bs-factor when the authors satisfy only three requirements is 8...
Your function should multiply the p-value by the bs-factor and use that product to return one of the following strings:
* product is less than 0.05: "Fine"
* product is 0.05 to 0.15: "Needs review"
* product is 0.15 or higher: "Pants on fire"
You've also decided that all studies meeting _none_ of the author requirements that would have been categorized as "Fine" should instead be categorized as "Needs review".
For example:
`categorize_study(0.01, 3)` should return `"Needs review"` because the p-value times the bs-factor is `0.08`.
`categorize_study(0.04, 6)` should return `"Fine"` because the p-value times the bs-factor is only `0.04`.
`categorize_study(0.0001, 0)` should return `"Needs review"` even though the p-value times the bs-factor is only `0.0064`.
`categorize_study(0.012, 0)` should return `"Pants on fire"` because the p-value times the bs-factor is `0.768`. | def categorize_study(p_value, requirements):
study_value = p_value * (2**(6-requirements))
if study_value < 0.05 and requirements != 0:
return "Fine"
elif study_value < 0.05 and requirements == 0:
return "Needs review"
elif study_value > 0.05 and study_value < 0.15:
return "Needs review"
else:
return "Pants on fire"
| def categorize_study(p_value, requirements):
study_value = p_value * (2**(6-requirements))
if study_value < 0.05 and requirements != 0:
return "Fine"
elif study_value < 0.05 and requirements == 0:
return "Needs review"
elif study_value > 0.05 and study_value < 0.15:
return "Needs review"
else:
return "Pants on fire"
| train | APPS_structured |
Given a linked list, reverse the nodes of a linked list k at a time and return its modified list.
k is a positive integer and is less than or equal to the length of the linked list. If the number of nodes is not a multiple of k then left-out nodes in the end should remain as it is.
Example:
Given this linked list: 1->2->3->4->5
For k = 2, you should return: 2->1->4->3->5
For k = 3, you should return: 3->2->1->4->5
Note:
Only constant extra memory is allowed.
You may not alter the values in the list's nodes, only nodes itself may be changed. | # Definition for singly-linked list.
# class ListNode:
# def __init__(self, x):
# self.val = x
# self.next = None
class Solution:
def reverseKGroup(self, head, k):
"""
:type head: ListNode
:type k: int
:rtype: ListNode
"""
if head == None:
return None
if k == 1:
return head
res = []
while(head!=None):
res.append(head.val)
head = head.next
lens = len(res)
res1 = []
start = 0
while(start+k <= lens):
b = res[start:start+k]
c = list(reversed(b))
res1 += c
start += k
b = res[start:lens]
res1 += b
return res1
| # Definition for singly-linked list.
# class ListNode:
# def __init__(self, x):
# self.val = x
# self.next = None
class Solution:
def reverseKGroup(self, head, k):
"""
:type head: ListNode
:type k: int
:rtype: ListNode
"""
if head == None:
return None
if k == 1:
return head
res = []
while(head!=None):
res.append(head.val)
head = head.next
lens = len(res)
res1 = []
start = 0
while(start+k <= lens):
b = res[start:start+k]
c = list(reversed(b))
res1 += c
start += k
b = res[start:lens]
res1 += b
return res1
| train | APPS_structured |
The [Linear Congruential Generator (LCG)](https://en.wikipedia.org/wiki/Linear_congruential_generator) is one of the oldest pseudo random number generator functions.
The algorithm is as follows:
## Xn+1=(aXn + c) mod m
where:
* `a`/`A` is the multiplier (we'll be using `2`)
* `c`/`C` is the increment (we'll be using `3`)
* `m`/`M` is the modulus (we'll be using `10`)
X0 is the seed.
# Your task
Define a method `random`/`Random` in the class `LCG` that provides the next random number based on a seed. You never return the initial seed value.
Similar to [random](https://docs.python.org/3/library/random.html#random.random) return the result as a floating point number in the range `[0.0, 1.0)`
# Example
```python
# initialize the generator with seed = 5
LCG(5)
# first random number (seed = 5)
LCG.random() = 0.3 # (2 * 5 + 3) mod 10 = 3 --> return 0.3
# next random number (seed = 3)
LCG.random() = 0.9 # (2 * 3 + 3) mod 10 = 9 --> return 0.9
# next random number (seed = 9)
LCG.random() = 0.1
# next random number (seed = 1)
LCG.random() = 0.5
``` | class LCG(object):
a, c, m = 2, 3, 10
def __init__(self, seed):
self.current = seed
def random(self):
self.current = (self.a * self.current + self.c) % self.m
return self.current / self.m | class LCG(object):
a, c, m = 2, 3, 10
def __init__(self, seed):
self.current = seed
def random(self):
self.current = (self.a * self.current + self.c) % self.m
return self.current / self.m | train | APPS_structured |
“Jesse, you asked me if I was in the meth business, or the money business… Neither. I’m in the empire business.”
Walter’s sold his stack in Gray Matter Technologies, a company which he deserved half a credit, for peanuts. Now this company is worth a billion dollar company. Walter wants to get it's shares to have his Empire Business back and he founds an opportunity.
There are $N$ persons having shares $A_1, A_2, A_3, … A_N$ in this company. Walter can buy these shares with their minimum Sold Values.
Sold Values of a person's share $ i $ $(1 \leq i \leq N) $ with another person's share $ j $ $ (1 \leq j \leq N) $ is equal to $ A_j+|i-j| $. So, a person's share can have $ N $ possible sold values and Walter has to find minimum sold value among them for each person.
Since Walter has to run his meth business also he asks you to find minimum sold value for each person.
-----Input:-----
- First line will contain $T$, number of test cases. Then the testcases follow.
- The First line of each test case contains a integer $N$.
- The Second line of each test case contains $N$ space integers namely $A_1,A_2,…A_N$.
-----Output:-----
For each test case, output in single line $N$ space integers denoting minimum sold value for each person.
-----Constraints-----
- $1 \leq T \leq 10^5$
- $1 \leq N \leq 2*10^6 $
- $1 \leq A_i \leq 10^9 $
Sum of $N$ over all test cases will not exceed $2*10^6$.
-----Sample Input:-----
2
5
6 5 5 5 2
5
1 2 3 4 5
-----Sample Output:-----
6 5 4 3 2
1 2 3 4 5
-----Explanation-----
For first case:
- Sold value for index $1$: $6,6,7,8,6$
- Sold value for index $2$: $7,5,6,7,5$
- Sold value for index $3$: $8,6,5,6,4$
- Sold value for index $4$: $9,7,6,5,3$
- Sold value for index $5$: $10,8,7,6,2$
Minimum sold value for each index will be $6,5,4,3,2$. | t = int(input())
for _ in range(t):
n = int(input())
arr = list(map(int, input().split()))
arr_modified = [-1 for _ in range(n)]
for i in range(n):
arr_modified[i] = arr[i] + i
# calculate minRights
minRights = [arr_modified[-1] for _ in range(n)]
for i in range(n-2, -1, -1):
minRights[i] = min(arr_modified[i], minRights[i+1])
# sold values
minLeft = float('inf')
soldValues = [-1 for _ in range(n)]
for i in range(n):
soldValues[i] = min(minLeft, minRights[i] - i)
minLeft = min(minLeft + 1, arr[i] + 1)
print(' '.join(str(x) for x in soldValues)) | t = int(input())
for _ in range(t):
n = int(input())
arr = list(map(int, input().split()))
arr_modified = [-1 for _ in range(n)]
for i in range(n):
arr_modified[i] = arr[i] + i
# calculate minRights
minRights = [arr_modified[-1] for _ in range(n)]
for i in range(n-2, -1, -1):
minRights[i] = min(arr_modified[i], minRights[i+1])
# sold values
minLeft = float('inf')
soldValues = [-1 for _ in range(n)]
for i in range(n):
soldValues[i] = min(minLeft, minRights[i] - i)
minLeft = min(minLeft + 1, arr[i] + 1)
print(' '.join(str(x) for x in soldValues)) | train | APPS_structured |
## Task
Generate a sorted list of all possible IP addresses in a network.
For a subnet that is not a valid IPv4 network return `None`.
## Examples
```
ipsubnet2list("192.168.1.0/31") == ["192.168.1.0", "192.168.1.1"]
ipsubnet2list("213.256.46.160/28") == None
``` | from ipaddress import IPv4Network, AddressValueError
def ipsubnet2list(subnet):
try: return list(map(str, IPv4Network(subnet).hosts()))
except AddressValueError: return None | from ipaddress import IPv4Network, AddressValueError
def ipsubnet2list(subnet):
try: return list(map(str, IPv4Network(subnet).hosts()))
except AddressValueError: return None | train | APPS_structured |
This kata is part of the collection [Mary's Puzzle Books](https://www.codewars.com/collections/marys-puzzle-books).
# Zero Terminated Sum
Mary has another puzzle book, and it's up to you to help her out! This book is filled with zero-terminated substrings, and you have to find the substring with the largest sum of its digits. For example, one puzzle looks like this:
```
"72102450111111090"
```
Here, there are 4 different substrings: `721`, `245`, `111111`, and `9`. The sums of their digits are `10`, `11`, `6`, and `9` respectively. Therefore, the substring with the largest sum of its digits is `245`, and its sum is `11`.
Write a function `largest_sum` which takes a string and returns the maximum of the sums of the substrings. In the example above, your function should return `11`.
### Notes:
- A substring can have length 0. For example, `123004560` has three substrings, and the middle one has length 0.
- All inputs will be valid strings of digits, and the last digit will always be `0`. | def largest_sum(s):
return max(sum(map(int, list(n))) for n in s.split('0'))
| def largest_sum(s):
return max(sum(map(int, list(n))) for n in s.split('0'))
| train | APPS_structured |
This is the easy version of the problem. The difference between the versions is the constraint on $n$ and the required number of operations. You can make hacks only if all versions of the problem are solved.
There are two binary strings $a$ and $b$ of length $n$ (a binary string is a string consisting of symbols $0$ and $1$). In an operation, you select a prefix of $a$, and simultaneously invert the bits in the prefix ($0$ changes to $1$ and $1$ changes to $0$) and reverse the order of the bits in the prefix.
For example, if $a=001011$ and you select the prefix of length $3$, it becomes $011011$. Then if you select the entire string, it becomes $001001$.
Your task is to transform the string $a$ into $b$ in at most $3n$ operations. It can be proved that it is always possible.
-----Input-----
The first line contains a single integer $t$ ($1\le t\le 1000$) — the number of test cases. Next $3t$ lines contain descriptions of test cases.
The first line of each test case contains a single integer $n$ ($1\le n\le 1000$) — the length of the binary strings.
The next two lines contain two binary strings $a$ and $b$ of length $n$.
It is guaranteed that the sum of $n$ across all test cases does not exceed $1000$.
-----Output-----
For each test case, output an integer $k$ ($0\le k\le 3n$), followed by $k$ integers $p_1,\ldots,p_k$ ($1\le p_i\le n$). Here $k$ is the number of operations you use and $p_i$ is the length of the prefix you flip in the $i$-th operation.
-----Example-----
Input
5
2
01
10
5
01011
11100
2
01
01
10
0110011011
1000110100
1
0
1
Output
3 1 2 1
6 5 2 5 3 1 2
0
9 4 1 2 10 4 1 2 1 5
1 1
-----Note-----
In the first test case, we have $01\to 11\to 00\to 10$.
In the second test case, we have $01011\to 00101\to 11101\to 01000\to 10100\to 00100\to 11100$.
In the third test case, the strings are already the same. Another solution is to flip the prefix of length $2$, which will leave $a$ unchanged. | import sys
input = sys.stdin.readline
from collections import deque
t=int(input())
for tests in range(t):
n=int(input())
a=input().strip()
b=input().strip()
Q=deque(a)
L=[]
while Q:
L.append(Q.popleft())
if Q:
L.append(Q.pop())
ANS=[]
for i in range(n):
if i%2==0:
if L[i]==b[-1-i]:
ANS.append(1)
else:
if L[i]!=b[-1-i]:
ANS.append(1)
ANS.append(n-i)
print(len(ANS),*ANS)
| import sys
input = sys.stdin.readline
from collections import deque
t=int(input())
for tests in range(t):
n=int(input())
a=input().strip()
b=input().strip()
Q=deque(a)
L=[]
while Q:
L.append(Q.popleft())
if Q:
L.append(Q.pop())
ANS=[]
for i in range(n):
if i%2==0:
if L[i]==b[-1-i]:
ANS.append(1)
else:
if L[i]!=b[-1-i]:
ANS.append(1)
ANS.append(n-i)
print(len(ANS),*ANS)
| train | APPS_structured |
Complete the function that takes two integers (`a, b`, where `a < b`) and return an array of all integers between the input parameters, **including** them.
For example:
```
a = 1
b = 4
--> [1, 2, 3, 4]
``` | def between(a,b):
s = [a]
while s[-1] < b:
t=s[-1]+1
s.append(t)
return s
| def between(a,b):
s = [a]
while s[-1] < b:
t=s[-1]+1
s.append(t)
return s
| train | APPS_structured |
Say you have an array for which the ith element is the price of a given stock on day i.
Design an algorithm to find the maximum profit. You may complete at most two transactions.
Note: You may not engage in multiple transactions at the same time (i.e., you must sell the stock before you buy again).
Example 1:
Input: [3,3,5,0,0,3,1,4]
Output: 6
Explanation: Buy on day 4 (price = 0) and sell on day 6 (price = 3), profit = 3-0 = 3.
Then buy on day 7 (price = 1) and sell on day 8 (price = 4), profit = 4-1 = 3.
Example 2:
Input: [1,2,3,4,5]
Output: 4
Explanation: Buy on day 1 (price = 1) and sell on day 5 (price = 5), profit = 5-1 = 4.
Note that you cannot buy on day 1, buy on day 2 and sell them later, as you are
engaging multiple transactions at the same time. You must sell before buying again.
Example 3:
Input: [7,6,4,3,1]
Output: 0
Explanation: In this case, no transaction is done, i.e. max profit = 0. | class Solution:
def maxProfit(self, prices):
"""
:type prices: List[int]
:rtype: int
"""
if not prices:
return 0
sell_date = [0] * len(prices)
min_price = prices[0]
for i in range(1, len(prices)):
sell_date[i] = max(sell_date[i - 1], prices[i] - min_price)
min_price = min(prices[i], min_price)
max_price, profit = prices[-1], 0
for i in range(len(prices) - 1, 0, -1):
profit = max(profit, max_price - prices[i] + sell_date[i])
max_price = max(max_price, prices[i])
return profit | class Solution:
def maxProfit(self, prices):
"""
:type prices: List[int]
:rtype: int
"""
if not prices:
return 0
sell_date = [0] * len(prices)
min_price = prices[0]
for i in range(1, len(prices)):
sell_date[i] = max(sell_date[i - 1], prices[i] - min_price)
min_price = min(prices[i], min_price)
max_price, profit = prices[-1], 0
for i in range(len(prices) - 1, 0, -1):
profit = max(profit, max_price - prices[i] + sell_date[i])
max_price = max(max_price, prices[i])
return profit | train | APPS_structured |
Write a function with the signature shown below:
```python
def is_int_array(arr):
return True
```
* returns `true / True` if every element in an array is an integer or a float with no decimals.
* returns `true / True` if array is empty.
* returns `false / False` for every other input. | def is_int_array(arr):
a = []
if arr == None or arr == "":
return False
for i in arr:
if type(i) == int:
a.append(i)
else:
if type(i) == float and i.is_integer():
a.append(i)
if len(arr) == len(a):
return True
return False | def is_int_array(arr):
a = []
if arr == None or arr == "":
return False
for i in arr:
if type(i) == int:
a.append(i)
else:
if type(i) == float and i.is_integer():
a.append(i)
if len(arr) == len(a):
return True
return False | train | APPS_structured |
Little Egor is a huge movie fan. He likes watching different kinds of movies: from drama movies to comedy movies, from teen movies to horror movies. He is planning to visit cinema this weekend, but he's not sure which movie he should watch.
There are n movies to watch during this weekend. Each movie can be characterized by two integers Li and Ri, denoting the length and the rating of the corresponding movie. Egor wants to watch exactly one movie with the maximal value of Li × Ri. If there are several such movies, he would pick a one with the maximal Ri among them. If there is still a tie, he would pick the one with the minimal index among them.
Your task is to help Egor to pick a movie to watch during this weekend.
-----Input-----
The first line of the input contains an integer T denoting the number of test cases.
The first line of the test case description contains an integer n.
The second line of the test case description contains n integers L1, L2, ...,Ln. The following line contains n integers R1, R2, ..., Rn.
-----Output-----
For each test case, output a single integer i denoting the index of the movie that Egor should watch during this weekend. Note that we follow 1-based indexing.
-----Constraints-----
- 1 ≤ T ≤ 5
- 1 ≤ n ≤ 100
- 1 ≤ Li, Ri ≤ 100
-----Example-----
Input:
2
2
1 2
2 1
4
2 1 4 1
2 4 1 4
Output:
1
2
-----Explanation-----
In the first example case, both films have the same value of L × R, but the first film has a better rating.
In the second example case, the second and the fourth movies are equally good, but the second movie has a smaller index. | t = int(input())
for e in range(t):
n = int(input())
l_str = input()
r_str = input()
l_str = l_str.strip().split(" ")
r_str = r_str.strip().split(" ")
lr_str = []
for x in range(n):
lr_str.append(int(l_str[x]) * int(r_str[x]))
lr_max = 0
maxIndex = 0
for x in range(n):
if int(lr_str[x]) > lr_max:
lr_max = lr_str[x]
maxIndex = x
max_r = 0
for x in range(n):
if int(lr_str[x]) == lr_max:
if int(r_str[x]) > max_r:
max_r = int(r_str[x])
maxIndex = x
max_i = 0
for x in range(n):
if int(lr_str[x]) == lr_max:
if int(r_str[x]) == max_r:
print(x + 1)
break | t = int(input())
for e in range(t):
n = int(input())
l_str = input()
r_str = input()
l_str = l_str.strip().split(" ")
r_str = r_str.strip().split(" ")
lr_str = []
for x in range(n):
lr_str.append(int(l_str[x]) * int(r_str[x]))
lr_max = 0
maxIndex = 0
for x in range(n):
if int(lr_str[x]) > lr_max:
lr_max = lr_str[x]
maxIndex = x
max_r = 0
for x in range(n):
if int(lr_str[x]) == lr_max:
if int(r_str[x]) > max_r:
max_r = int(r_str[x])
maxIndex = x
max_i = 0
for x in range(n):
if int(lr_str[x]) == lr_max:
if int(r_str[x]) == max_r:
print(x + 1)
break | train | APPS_structured |
In this problem the input will consist of a number of lines of English text consisting of the letters of the English alphabet, the punctuation marks ' (apostrophe), . (full stop), , (comma), ; (semicolon), :(colon) and white space characters (blank, newline). Your task is print the words in the text in reverse order without any punctuation marks.
For example consider the following candidate for the input text:
$ $
This is a sample piece of text to illustrate this
problem. If you are smart you will solve this right.
$ $
The corresponding output would read as:
$ $
right this solve will you smart are you If problem
this illustrate to text of piece sample a is This
$ $
That is, the lines are printed in reverse order and in each line the words are printed in reverse order.
-----Input:-----
The first line of input contains a single integer $N$, indicating the number of lines in the input. This is followed by $N$ lines of input text.
-----Output:-----
$N$ lines of output text containing the input lines in reverse order and where each line contains the words in reverse order as illustrated above.
-----Constraints:-----
- $1 \leq N \leq 10000$.
- There are at most $80$ characters in each line
-----Sample input-----
2
This is a sample piece of text to illustrate this
problem. If you are smart you will solve this right.
-----Sample output-----
right this solve will you smart are you If problem
this illustrate to text of piece sample a is This | n=int(input())
ans=[]
for x in range(n):
a=input()
a=(' '.join(a.split()[::-1]))
# print(a)
punctuation=".,:;'"
b=""
for i in a:
if i not in punctuation:
b+=i
ans.append(b)
n=(ans[::-1])
for i in n:
print(i) | n=int(input())
ans=[]
for x in range(n):
a=input()
a=(' '.join(a.split()[::-1]))
# print(a)
punctuation=".,:;'"
b=""
for i in a:
if i not in punctuation:
b+=i
ans.append(b)
n=(ans[::-1])
for i in n:
print(i) | train | APPS_structured |
Bertown is a city with $n$ buildings in a straight line.
The city's security service discovered that some buildings were mined. A map was compiled, which is a string of length $n$, where the $i$-th character is "1" if there is a mine under the building number $i$ and "0" otherwise.
Bertown's best sapper knows how to activate mines so that the buildings above them are not damaged. When a mine under the building numbered $x$ is activated, it explodes and activates two adjacent mines under the buildings numbered $x-1$ and $x+1$ (if there were no mines under the building, then nothing happens). Thus, it is enough to activate any one mine on a continuous segment of mines to activate all the mines of this segment. For manual activation of one mine, the sapper takes $a$ coins. He can repeat this operation as many times as you want.
Also, a sapper can place a mine under a building if it wasn't there. For such an operation, he takes $b$ coins. He can also repeat this operation as many times as you want.
The sapper can carry out operations in any order.
You want to blow up all the mines in the city to make it safe. Find the minimum number of coins that the sapper will have to pay so that after his actions there are no mines left in the city.
-----Input-----
The first line contains one positive integer $t$ ($1 \le t \le 10^5$) — the number of test cases. Then $t$ test cases follow.
Each test case begins with a line containing two integers $a$ and $b$ ($1 \le a, b \le 1000$) — the cost of activating and placing one mine, respectively.
The next line contains a map of mines in the city — a string consisting of zeros and ones.
The sum of the string lengths for all test cases does not exceed $10^5$.
-----Output-----
For each test case, output one integer — the minimum number of coins that the sapper will have to pay.
-----Example-----
Input
2
1 1
01000010
5 1
01101110
Output
2
6
-----Note-----
In the second test case, if we place a mine under the fourth building and then activate it, then all mines on the field are activated. The cost of such operations is six, $b=1$ coin for placing a mine and $a=5$ coins for activating. | import math
import sys
def chek(m, b, c, li):
for i in range(li):
if m[i] + b[i] > c:
return False
return True
# 113759
input = lambda: sys.stdin.readline().rstrip()
f = int(input())
for _ in range(f):
a, b = list(map(int, input().split()))
s = input()
mas = []
c = 1
k = len(s)
cur = 1
while c != k:
if s[c] == s[c - 1]:
cur += 1
else:
if len(mas) != 0:
mas.append(cur)
cur = 1
else:
if s[c] == "0":
mas.append(cur)
cur = 1
else:
cur = 1
c += 1
if s[c - 1] == "1":
mas.append(cur)
ans = 0
for i in range(len(mas)):
if i % 2 == 0:
ans += a
else:
if a > b * mas[i]:
ans += b * mas[i]
ans -= a
print(ans)
| import math
import sys
def chek(m, b, c, li):
for i in range(li):
if m[i] + b[i] > c:
return False
return True
# 113759
input = lambda: sys.stdin.readline().rstrip()
f = int(input())
for _ in range(f):
a, b = list(map(int, input().split()))
s = input()
mas = []
c = 1
k = len(s)
cur = 1
while c != k:
if s[c] == s[c - 1]:
cur += 1
else:
if len(mas) != 0:
mas.append(cur)
cur = 1
else:
if s[c] == "0":
mas.append(cur)
cur = 1
else:
cur = 1
c += 1
if s[c - 1] == "1":
mas.append(cur)
ans = 0
for i in range(len(mas)):
if i % 2 == 0:
ans += a
else:
if a > b * mas[i]:
ans += b * mas[i]
ans -= a
print(ans)
| train | APPS_structured |
Accepts a string from the user and print the reverse string as the output without using any built-in function.
-----Input:-----
Each testcase contains of a single line of input, a string.
-----Output:-----
For each testcase, output in a single line answer, the reverse string.
-----Sample Input:-----
1
Tracy
-----Sample Output:-----
ycarT | # cook your dish here
t = int(input())
for i in range(t):
s = input()
print(s[::-1]) | # cook your dish here
t = int(input())
for i in range(t):
s = input()
print(s[::-1]) | train | APPS_structured |
We are stacking blocks to form a pyramid. Each block has a color which is a one letter string, like `'Z'`.
For every block of color `C` we place not in the bottom row, we are placing it on top of a left block of color `A` and right block of color `B`. We are allowed to place the block there only if `(A, B, C)` is an allowed triple.
We start with a bottom row of bottom, represented as a single string. We also start with a list of allowed triples allowed. Each allowed triple is represented as a string of length 3.
Return true if we can build the pyramid all the way to the top, otherwise false.
Example 1:
Input: bottom = "XYZ", allowed = ["XYD", "YZE", "DEA", "FFF"]
Output: true
Explanation:
We can stack the pyramid like this:
A
/ \
D E
/ \ / \
X Y Z
This works because ('X', 'Y', 'D'), ('Y', 'Z', 'E'), and ('D', 'E', 'A') are allowed triples.
Example 2:
Input: bottom = "XXYX", allowed = ["XXX", "XXY", "XYX", "XYY", "YXZ"]
Output: false
Explanation:
We can't stack the pyramid to the top.
Note that there could be allowed triples (A, B, C) and (A, B, D) with C != D.
Note:
bottom will be a string with length in range [2, 8].
allowed will have length in range [0, 200].
Letters in all strings will be chosen from the set {'A', 'B', 'C', 'D', 'E', 'F', 'G'}. | class Solution:
def pourWater(self, heights, total, index):
"""
:type heights: List[int]
:type V: int
:type K: int
:rtype: List[int]
"""
if not heights or total <= 0 or index < 0 or index >= len(heights):
return heights
for _ in range(total):
left = -1
for i in range(index-1, -1, -1):
if heights[i] > heights[i+1]:
break
if heights[i] < heights[i+1]:
left = i
if left != -1:
heights[left] += 1
continue
right = -1
for j in range(index+1, len(heights)):
if heights[j] > heights[j-1]:
break
if heights[j] < heights[j-1]:
right = j
if right != -1:
heights[right] += 1
continue
heights[index] += 1
return heights
| class Solution:
def pourWater(self, heights, total, index):
"""
:type heights: List[int]
:type V: int
:type K: int
:rtype: List[int]
"""
if not heights or total <= 0 or index < 0 or index >= len(heights):
return heights
for _ in range(total):
left = -1
for i in range(index-1, -1, -1):
if heights[i] > heights[i+1]:
break
if heights[i] < heights[i+1]:
left = i
if left != -1:
heights[left] += 1
continue
right = -1
for j in range(index+1, len(heights)):
if heights[j] > heights[j-1]:
break
if heights[j] < heights[j-1]:
right = j
if right != -1:
heights[right] += 1
continue
heights[index] += 1
return heights
| train | APPS_structured |
In this Kata, you will be given a number in form of a string and an integer `k` and your task is to insert `k` commas into the string and determine which of the partitions is the largest.
```
For example:
solve('1234',1) = 234 because ('1','234') or ('12','34') or ('123','4').
solve('1234',2) = 34 because ('1','2','34') or ('1','23','4') or ('12','3','4').
solve('1234',3) = 4
solve('2020',1) = 202
```
More examples in test cases. Good luck!
Please also try [Simple remove duplicates](https://www.codewars.com/kata/5ba38ba180824a86850000f7) | def solve(st,k):
return max([int(st[i:len(st)-k+i]) for i in range(0, k+1)]) | def solve(st,k):
return max([int(st[i:len(st)-k+i]) for i in range(0, k+1)]) | train | APPS_structured |
Your task is to build a model^(1) which can predict y-coordinate.
You can pass tests if predicted y-coordinates are inside error margin.
You will receive train set which should be used to build a model.
After you build a model tests will call function ```predict``` and pass x to it.
Error is going to be calculated with RMSE.
Blocked libraries: sklearn, pandas, tensorflow, numpy, scipy
Explanation
[1] A mining model is created by applying an algorithm to data, but it is more than an algorithm or a metadata container: it is a set of data, statistics, and patterns that can be applied to new data to generate predictions and make inferences about relationships. | class Datamining:
def __init__(self, train_set):
x_mean = lambda train_set: sum( [i[0] for i in train_set] ) / len( [i[0] for i in train_set] )
y_mean = lambda train_set: sum( [i[1] for i in train_set] ) / len( [i[1] for i in train_set] )
f = lambda x_mean, y_mean, train_set: sum( (x - x_mean) * (y - y_mean) for x, y in train_set )
g = lambda x_mean, train_set: sum( (x - x_mean) ** 2 for x in [i[0] for i in train_set] )
self.a = f( x_mean(train_set), y_mean (train_set), train_set ) / g ( x_mean (train_set), train_set )
self.b = y_mean(train_set) - self.a * x_mean(train_set)
def predict(self, x): return x * self.a + self.b | class Datamining:
def __init__(self, train_set):
x_mean = lambda train_set: sum( [i[0] for i in train_set] ) / len( [i[0] for i in train_set] )
y_mean = lambda train_set: sum( [i[1] for i in train_set] ) / len( [i[1] for i in train_set] )
f = lambda x_mean, y_mean, train_set: sum( (x - x_mean) * (y - y_mean) for x, y in train_set )
g = lambda x_mean, train_set: sum( (x - x_mean) ** 2 for x in [i[0] for i in train_set] )
self.a = f( x_mean(train_set), y_mean (train_set), train_set ) / g ( x_mean (train_set), train_set )
self.b = y_mean(train_set) - self.a * x_mean(train_set)
def predict(self, x): return x * self.a + self.b | train | APPS_structured |
In this Kata, you will be given an integer array and your task is to return the sum of elements occupying prime-numbered indices.
~~~if-not:fortran
The first element of the array is at index `0`.
~~~
~~~if:fortran
The first element of an array is at index `1`.
~~~
Good luck!
If you like this Kata, try:
[Dominant primes](https://www.codewars.com/kata/59ce11ea9f0cbc8a390000ed). It takes this idea a step further.
[Consonant value](https://www.codewars.com/kata/59c633e7dcc4053512000073) | def total(arr):
fin_arr = []
for i in range(2, len(arr)):
d = 2
while i % d != 0:
d += 1
if d == i:
fin_arr.append(arr[i])
n = sum(fin_arr)
return n | def total(arr):
fin_arr = []
for i in range(2, len(arr)):
d = 2
while i % d != 0:
d += 1
if d == i:
fin_arr.append(arr[i])
n = sum(fin_arr)
return n | train | APPS_structured |
# A History Lesson
The Pony Express was a mail service operating in the US in 1859-60.
It reduced the time for messages to travel between the Atlantic and Pacific coasts to about 10 days, before it was made obsolete by the [transcontinental telegraph](https://en.wikipedia.org/wiki/First_transcontinental_telegraph).
# How it worked
There were a number of *stations*, where:
* The rider switched to a fresh horse and carried on, or
* The mail bag was handed over to the next rider
# Kata Task
`stations` is a list/array of distances (miles) from one station to the next along the Pony Express route.
Implement the `riders` method/function, to return how many riders are necessary to get the mail from one end to the other.
**NOTE:** Each rider travels as far as he can, but never more than 100 miles.
---
*Good Luck.
DM.*
---
See also
* The Pony Express
* The Pony Express (missing rider) | def riders(stations):
count = 1
distance = 0
for station in stations:
if distance + station > 100:
count += 1
distance = 0
distance += station
return count | def riders(stations):
count = 1
distance = 0
for station in stations:
if distance + station > 100:
count += 1
distance = 0
distance += station
return count | train | APPS_structured |
Greg has a weighed directed graph, consisting of n vertices. In this graph any pair of distinct vertices has an edge between them in both directions. Greg loves playing with the graph and now he has invented a new game: The game consists of n steps. On the i-th step Greg removes vertex number x_{i} from the graph. As Greg removes a vertex, he also removes all the edges that go in and out of this vertex. Before executing each step, Greg wants to know the sum of lengths of the shortest paths between all pairs of the remaining vertices. The shortest path can go through any remaining vertex. In other words, if we assume that d(i, v, u) is the shortest path between vertices v and u in the graph that formed before deleting vertex x_{i}, then Greg wants to know the value of the following sum: $\sum_{v, u, v \neq u} d(i, v, u)$.
Help Greg, print the value of the required sum before each step.
-----Input-----
The first line contains integer n (1 ≤ n ≤ 500) — the number of vertices in the graph.
Next n lines contain n integers each — the graph adjacency matrix: the j-th number in the i-th line a_{ij} (1 ≤ a_{ij} ≤ 10^5, a_{ii} = 0) represents the weight of the edge that goes from vertex i to vertex j.
The next line contains n distinct integers: x_1, x_2, ..., x_{n} (1 ≤ x_{i} ≤ n) — the vertices that Greg deletes.
-----Output-----
Print n integers — the i-th number equals the required sum before the i-th step.
Please, do not use the %lld specifier to read or write 64-bit integers in C++. It is preferred to use the cin, cout streams of the %I64d specifier.
-----Examples-----
Input
1
0
1
Output
0
Input
2
0 5
4 0
1 2
Output
9 0
Input
4
0 3 1 1
6 0 400 1
2 4 0 1
1 1 1 0
4 1 2 3
Output
17 23 404 0 | import sys
from array import array # noqa: F401
n = int(input())
matrix = [array('i', list(map(int, input().split()))) for _ in range(n)]
aa = tuple([int(x) - 1 for x in input().split()])
ans = [''] * n
for i in range(n-1, -1, -1):
x = aa[i]
for a in range(n):
for b in range(n):
if matrix[a][b] > matrix[a][x] + matrix[x][b]:
matrix[a][b] = matrix[a][x] + matrix[x][b]
val, overflow = 0, 0
for a in aa[i:]:
for b in aa[i:]:
val += matrix[a][b]
if val > 10**9:
overflow += 1
val -= 10**9
ans[i] = str(10**9 * overflow + val)
print(' '.join(ans))
| import sys
from array import array # noqa: F401
n = int(input())
matrix = [array('i', list(map(int, input().split()))) for _ in range(n)]
aa = tuple([int(x) - 1 for x in input().split()])
ans = [''] * n
for i in range(n-1, -1, -1):
x = aa[i]
for a in range(n):
for b in range(n):
if matrix[a][b] > matrix[a][x] + matrix[x][b]:
matrix[a][b] = matrix[a][x] + matrix[x][b]
val, overflow = 0, 0
for a in aa[i:]:
for b in aa[i:]:
val += matrix[a][b]
if val > 10**9:
overflow += 1
val -= 10**9
ans[i] = str(10**9 * overflow + val)
print(' '.join(ans))
| train | APPS_structured |
Implement a function which multiplies two numbers. | def multiply (num1, num2):
return num1 * num2
print(multiply(3, 4)) | def multiply (num1, num2):
return num1 * num2
print(multiply(3, 4)) | train | APPS_structured |
# Task
`N` candles are placed in a row, some of them are initially lit. For each candle from the 1st to the Nth the following algorithm is applied: if the observed candle is lit then states of this candle and all candles before it are changed to the opposite. Which candles will remain lit after applying the algorithm to all candles in the order they are placed in the line?
# Example
For `a = [1, 1, 1, 1, 1]`, the output should be `[0, 1, 0, 1, 0].`
Check out the image below for better understanding:
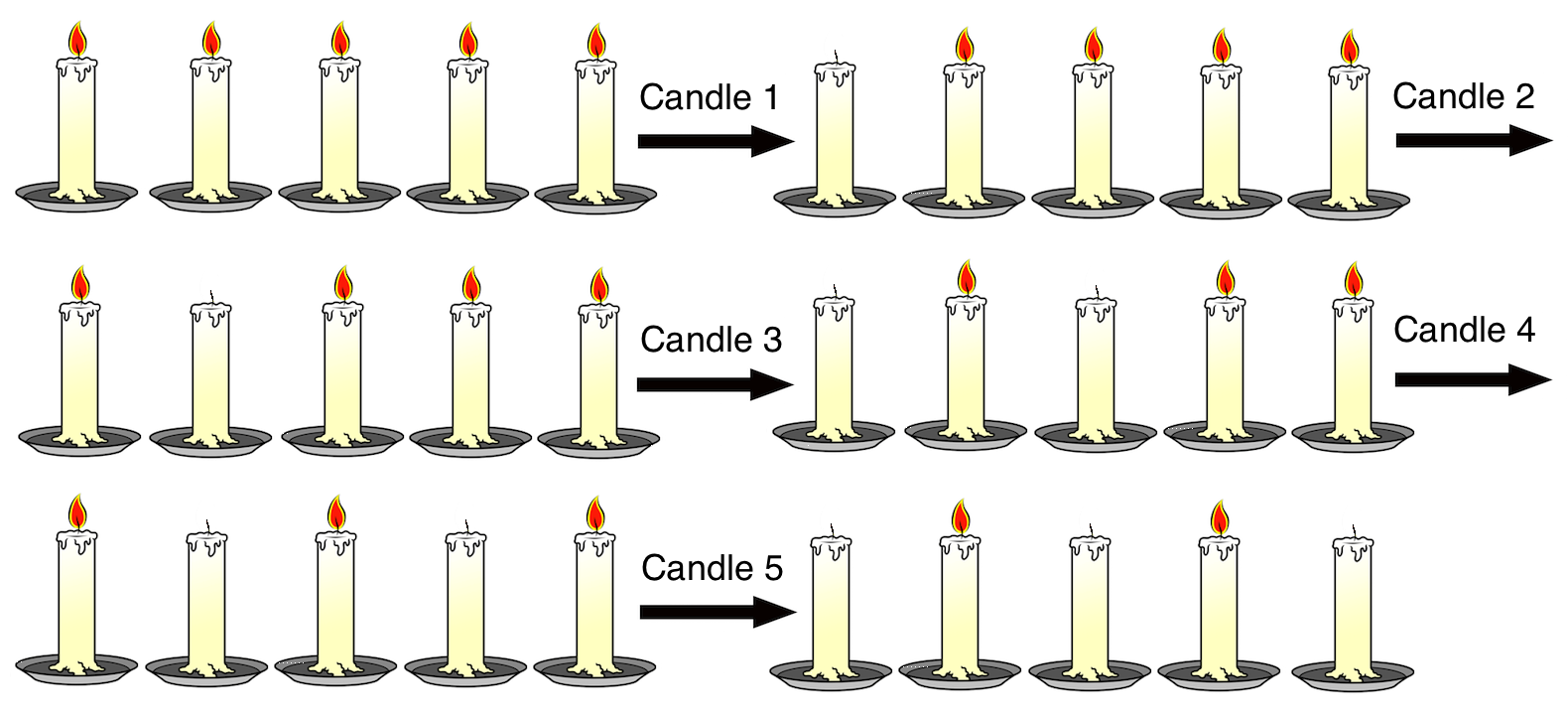
For `a = [0, 0]`, the output should be `[0, 0].`
The candles are not initially lit, so their states are not altered by the algorithm.
# Input/Output
- `[input]` integer array `a`
Initial situation - array of zeros and ones of length N, 1 means that the corresponding candle is lit.
Constraints: `2 ≤ a.length ≤ 5000.`
- `[output]` an integer array
Situation after applying the algorithm - array in the same format as input with the same length. | def switch_lights(a):
n = sum(a)
for i, x in enumerate(a):
if n % 2:
a[i] ^= 1
n -= x
return a | def switch_lights(a):
n = sum(a)
for i, x in enumerate(a):
if n % 2:
a[i] ^= 1
n -= x
return a | train | APPS_structured |
### The problem
How many zeroes are at the **end** of the [factorial](https://en.wikipedia.org/wiki/Factorial) of `10`? 10! = 3628800, i.e. there are `2` zeroes.
16! (or 0x10!) in [hexadecimal](https://en.wikipedia.org/wiki/Hexadecimal) would be 0x130777758000, which has `3` zeroes.
### Scalability
Unfortunately, machine integer numbers has not enough precision for larger values. Floating point numbers drop the tail we need. We can fall back to arbitrary-precision ones - built-ins or from a library, but calculating the full product isn't an efficient way to find just the _tail_ of a factorial. Calculating `100'000!` in compiled language takes around 10 seconds. `1'000'000!` would be around 10 minutes, even using efficient [Karatsuba algorithm](https://en.wikipedia.org/wiki/Karatsuba_algorithm)
### Your task
is to write a function, which will find the number of zeroes at the end of `(number)` factorial in arbitrary [radix](https://en.wikipedia.org/wiki/Radix) = `base` for larger numbers.
- `base` is an integer from 2 to 256
- `number` is an integer from 1 to 1'000'000
**Note** Second argument: number is always declared, passed and displayed as a regular _decimal_ number. If you see a test described as `42! in base 20` it's 4210 **not** 4220 = 8210. | def zeroes (b, n):
d1={} #for decomposition of base into power of primefactors
i=2
while b>1:
while b%i==0:
try:
d1[i]+=1
except KeyError:
d1[i]=1
b//=i
i+=1
d2={} #for total powers of the primefactors in the number
for i in d1:
s=0
r=i
while r<=n:
s+=n//r
r*=i
d2[i]=s
return min(list(d2[i]//d1[i] for i in d1))
| def zeroes (b, n):
d1={} #for decomposition of base into power of primefactors
i=2
while b>1:
while b%i==0:
try:
d1[i]+=1
except KeyError:
d1[i]=1
b//=i
i+=1
d2={} #for total powers of the primefactors in the number
for i in d1:
s=0
r=i
while r<=n:
s+=n//r
r*=i
d2[i]=s
return min(list(d2[i]//d1[i] for i in d1))
| train | APPS_structured |
Write a function that accepts two parameters, i) a string (containing a list of words) and ii) an integer (n). The function should alphabetize the list based on the nth letter of each word.
The letters should be compared case-insensitive. If both letters are the same, order them normally (lexicographically), again, case-insensitive.
example:
```javascript
function sortIt('bid, zag', 2) //=> 'zag, bid'
```
```ruby
function sortIt('bid, zag', 2) //=> 'zag, bid'
```
```python
function sortIt('bid, zag', 2) #=> 'zag, bid'
```
The length of all words provided in the list will be >= n. The format will be "x, x, x". In Haskell you'll get a list of `String`s instead. | def sort_it(s, n):
return ', '.join(sorted(s.split(', '), key=lambda s: s[n-1]))
| def sort_it(s, n):
return ', '.join(sorted(s.split(', '), key=lambda s: s[n-1]))
| train | APPS_structured |
A core idea of several left-wing ideologies is that the wealthiest should *support* the poorest, no matter what and that is exactly what you are called to do using this kata (which, on a side note, was born out of the necessity to redistribute the width of `div`s into a given container).
You will be given two parameters, `population` and `minimum`: your goal is to give to each one according to his own needs (which we assume to be equal to `minimum` for everyone, no matter what), taking from the richest (bigger numbers) first.
For example, assuming a population `[2,3,5,15,75]` and `5` as a minimum, the expected result should be `[5,5,5,15,70]`. Let's punish those filthy capitalists, as we all know that being rich has to be somehow a fault and a shame!
If you happen to have few people as the richest, just take from the ones with the lowest index (the closest to the left, in few words) in the array first, on a 1:1 based heroic proletarian redistribution, until everyone is satisfied.
To clarify this rule, assuming a population `[2,3,5,45,45]` and `5` as `minimum`, the expected result should be `[5,5,5,42,43]`.
If you want to see it in steps, consider removing `minimum` from every member of the population, then iteratively (or recursively) adding 1 to the poorest while removing 1 from the richest. Pick the element most at left if more elements exist with the same level of minimal poverty, as they are certainly even more aligned with the party will than other poor people; similarly, it is ok to take from the richest one on the left first, so they can learn their lesson and be more kind, possibly giving more *gifts* to the inspectors of the State!
In steps:
```
[ 2, 3, 5,45,45] becomes
[-3,-2, 0,40,40] that then becomes
[-2,-2, 0,39,40] that then becomes
[-1,-2, 0,39,39] that then becomes
[-1,-1, 0,38,39] that then becomes
[ 0,-1, 0,38,38] that then becomes
[ 0, 0, 0,37,38] that then finally becomes (adding the minimum again, as no value is no longer under the poverty threshold
[ 5, 5, 5,42,43]
```
If giving `minimum` is unfeasable with the current resources (as it often comes to be the case in socialist communities...), for example if the above starting population had set a goal of giving anyone at least `30`, just return an empty array `[]`. | def socialist_distribution(population, minimum):
if sum(population) < minimum * len(population):
return []
population = population[:]
for _ in range(sum(max(minimum - p, 0) for p in population)):
population[min(range(len(population)), key=population.__getitem__)] += 1
population[max(range(len(population)), key=population.__getitem__)] -= 1
return population | def socialist_distribution(population, minimum):
if sum(population) < minimum * len(population):
return []
population = population[:]
for _ in range(sum(max(minimum - p, 0) for p in population)):
population[min(range(len(population)), key=population.__getitem__)] += 1
population[max(range(len(population)), key=population.__getitem__)] -= 1
return population | train | APPS_structured |
We have two types of tiles: a 2x1 domino shape, and an "L" tromino shape. These shapes may be rotated.
XX <- domino
XX <- "L" tromino
X
Given N, how many ways are there to tile a 2 x N board? Return your answer modulo 10^9 + 7.
(In a tiling, every square must be covered by a tile. Two tilings are different if and only if there are two 4-directionally adjacent cells on the board such that exactly one of the tilings has both squares occupied by a tile.)
Example:
Input: 3
Output: 5
Explanation:
The five different ways are listed below, different letters indicates different tiles:
XYZ XXZ XYY XXY XYY
XYZ YYZ XZZ XYY XXY
Note:
N will be in range [1, 1000]. | class Solution:
def isIdealPermutation(self, A):
"""
:type A: List[int]
:rtype: bool
"""
return all(abs(v-i)<=1 for i,v in enumerate(A)) #有意思 其中的原理更有意思 | class Solution:
def isIdealPermutation(self, A):
"""
:type A: List[int]
:rtype: bool
"""
return all(abs(v-i)<=1 for i,v in enumerate(A)) #有意思 其中的原理更有意思 | train | APPS_structured |
The AKS algorithm for testing whether a number is prime is a polynomial-time test based on the following theorem:
A number p is prime if and only if all the coefficients of the polynomial expansion of `(x − 1)^p − (x^p − 1)` are divisible by `p`.
For example, trying `p = 3`:
(x − 1)^3 − (x^3 − 1) = (x^3 − 3x^2 + 3x − 1) − (x^3 − 1) = − 3x^2 + 3x
And all the coefficients are divisible by 3, so 3 is prime.
Your task is to code the test function, wich will be given an integer and should return true or false based on the above theorem. You should efficiently calculate every coefficient one by one and stop when a coefficient is not divisible by p to avoid pointless calculations. The easiest way to calculate coefficients is to take advantage of binomial coefficients: http://en.wikipedia.org/wiki/Binomial_coefficient and pascal triangle: http://en.wikipedia.org/wiki/Pascal%27s_triangle .
You should also take advantage of the symmetry of binomial coefficients. You can look at these kata: http://www.codewars.com/kata/pascals-triangle and http://www.codewars.com/kata/pascals-triangle-number-2
The kata here only use the simple theorem. The general AKS test is way more complex. The simple approach is a good exercie but impractical. The general AKS test will be the subject of another kata as it is one of the best performing primality test algorithms.
The main problem of this algorithm is the big numbers emerging from binomial coefficients in the polynomial expansion. As usual Javascript reach its limit on big integer very fast. (This simple algorithm is far less performing than a trivial algorithm here). You can compare the results with those of this kata: http://www.codewars.com/kata/lucas-lehmer-test-for-mersenne-primes Here, javascript can barely treat numbers bigger than 50, python can treat M13 but not M17. | def aks_test(n) :
if (n <= 1) :
return False
if (n <= 3) :
return True
if (n % 2 == 0 or n % 3 == 0) :
return False
i = 5
while(i * i <= n) :
if (n % i == 0 or n % (i + 2) == 0) :
return False
i = i + 6
return True | def aks_test(n) :
if (n <= 1) :
return False
if (n <= 3) :
return True
if (n % 2 == 0 or n % 3 == 0) :
return False
i = 5
while(i * i <= n) :
if (n % i == 0 or n % (i + 2) == 0) :
return False
i = i + 6
return True | train | APPS_structured |
You are given a set Y of n distinct positive integers y_1, y_2, ..., y_{n}.
Set X of n distinct positive integers x_1, x_2, ..., x_{n} is said to generate set Y if one can transform X to Y by applying some number of the following two operation to integers in X: Take any integer x_{i} and multiply it by two, i.e. replace x_{i} with 2·x_{i}. Take any integer x_{i}, multiply it by two and add one, i.e. replace x_{i} with 2·x_{i} + 1.
Note that integers in X are not required to be distinct after each operation.
Two sets of distinct integers X and Y are equal if they are equal as sets. In other words, if we write elements of the sets in the array in the increasing order, these arrays would be equal.
Note, that any set of integers (or its permutation) generates itself.
You are given a set Y and have to find a set X that generates Y and the maximum element of X is mininum possible.
-----Input-----
The first line of the input contains a single integer n (1 ≤ n ≤ 50 000) — the number of elements in Y.
The second line contains n integers y_1, ..., y_{n} (1 ≤ y_{i} ≤ 10^9), that are guaranteed to be distinct.
-----Output-----
Print n integers — set of distinct integers that generate Y and the maximum element of which is minimum possible. If there are several such sets, print any of them.
-----Examples-----
Input
5
1 2 3 4 5
Output
4 5 2 3 1
Input
6
15 14 3 13 1 12
Output
12 13 14 7 3 1
Input
6
9 7 13 17 5 11
Output
4 5 2 6 3 1 | def main():
from heapq import heapify, heapreplace
input()
s = set(map(int, input().split()))
xx = [-x for x in s]
heapify(xx)
while True:
x = -xx[0]
while x != 1:
x //= 2
if x not in s:
s.add(x)
heapreplace(xx, -x)
break
else:
break
print(' '.join(str(-x) for x in xx))
def __starting_point():
main()
__starting_point() | def main():
from heapq import heapify, heapreplace
input()
s = set(map(int, input().split()))
xx = [-x for x in s]
heapify(xx)
while True:
x = -xx[0]
while x != 1:
x //= 2
if x not in s:
s.add(x)
heapreplace(xx, -x)
break
else:
break
print(' '.join(str(-x) for x in xx))
def __starting_point():
main()
__starting_point() | train | APPS_structured |
The Fibonacci sequence $F_0, F_1, \ldots$ is a special infinite sequence of non-negative integers, where $F_0 = 0$, $F_1 = 1$ and for each integer $n \ge 2$, $F_n = F_{n-1} + F_{n-2}$.
Consider the sequence $D$ of the last decimal digits of the first $N$ Fibonacci numbers, i.e. $D = (F_0 \% 10, F_1 \% 10, \ldots, F_{N-1} \% 10)$. Now, you should perform the following process:
- Let $D = (D_1, D_2, \ldots, D_l)$.
- If $l = 1$, the process ends.
- Create a new sequence $E = (D_2, D_4, \ldots, D_{2 \lfloor l/2 \rfloor})$. In other words, $E$ is the sequence created by removing all odd-indexed elements from $D$.
- Change $D$ to $E$.
When this process terminates, the sequence $D$ contains only one number. You have to find this number.
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first and only line of each test case contains a single integer $N$.
-----Output-----
For each test case, print a single line containing one integer ― the last remaining number.
-----Constraints-----
- $1 \le T \le 10^5$
- $1 \le N \le 10^{18}$
-----Subtasks-----
Subtask #1 (20 points):
- $1 \le T \le 10^5$
- $1 \le N \le 10^7$
Subtask #2 (80 points): original constraints
-----Example Input-----
1
9
-----Example Output-----
3
-----Explanation-----
Example case 1: The first $N$ Fibonacci numbers are $(0, 1, 1, 2, 3, 5, 8, 13, 21)$. The sequence $D$ is $(0, 1, 1, 2, 3, 5, 8, 3, 1) \rightarrow (1, 2, 5, 3) \rightarrow (2, 3) \rightarrow (3)$. | import math
def r(n):
cycle = 60
index = (1 << int(math.floor(math.log2(n)))) % cycle
cur = 0
next = 1
for i in range(1, index):
nextNext = (cur + next) % 10
cur = next
next = nextNext
return cur
t = int(input())
for i in range(t):
c = int(input())
print(r(c)) | import math
def r(n):
cycle = 60
index = (1 << int(math.floor(math.log2(n)))) % cycle
cur = 0
next = 1
for i in range(1, index):
nextNext = (cur + next) % 10
cur = next
next = nextNext
return cur
t = int(input())
for i in range(t):
c = int(input())
print(r(c)) | train | APPS_structured |
Given an array of integers nums and a positive integer k, find whether it's possible to divide this array into sets of k consecutive numbers
Return True if its possible otherwise return False.
Example 1:
Input: nums = [1,2,3,3,4,4,5,6], k = 4
Output: true
Explanation: Array can be divided into [1,2,3,4] and [3,4,5,6].
Example 2:
Input: nums = [3,2,1,2,3,4,3,4,5,9,10,11], k = 3
Output: true
Explanation: Array can be divided into [1,2,3] , [2,3,4] , [3,4,5] and [9,10,11].
Example 3:
Input: nums = [3,3,2,2,1,1], k = 3
Output: true
Example 4:
Input: nums = [1,2,3,4], k = 3
Output: false
Explanation: Each array should be divided in subarrays of size 3.
Constraints:
1 <= nums.length <= 10^5
1 <= nums[i] <= 10^9
1 <= k <= nums.length | from collections import Counter
class Solution:
def isPossibleDivide(self, nums: List[int], k: int) -> bool:
d = Counter(nums)
nums.sort()
for x in nums:
if x in d:
for y in range(x, x + k):
if y in d:
d[y] -= 1
if d[y] == 0:
del d[y]
else:
return False
return True
| from collections import Counter
class Solution:
def isPossibleDivide(self, nums: List[int], k: int) -> bool:
d = Counter(nums)
nums.sort()
for x in nums:
if x in d:
for y in range(x, x + k):
if y in d:
d[y] -= 1
if d[y] == 0:
del d[y]
else:
return False
return True
| train | APPS_structured |
# Grasshopper - Function syntax debugging
A student was working on a function and made some syntax mistakes while coding. Help them find their mistakes and fix them. | def main(verb,noun):
return (verb + noun)
print((main('take ', 'item')))
| def main(verb,noun):
return (verb + noun)
print((main('take ', 'item')))
| train | APPS_structured |
We guessed a permutation $p$ consisting of $n$ integers. The permutation of length $n$ is the array of length $n$ where each element from $1$ to $n$ appears exactly once. This permutation is a secret for you.
For each position $r$ from $2$ to $n$ we chose some other index $l$ ($l < r$) and gave you the segment $p_l, p_{l + 1}, \dots, p_r$ in sorted order (i.e. we rearranged the elements of this segment in a way that the elements of this segment are sorted). Thus, you are given exactly $n-1$ segments of the initial permutation but elements inside each segment are sorted. The segments are given to you in random order.
For example, if the secret permutation is $p=[3, 1, 4, 6, 2, 5]$ then the possible given set of segments can be: $[2, 5, 6]$ $[4, 6]$ $[1, 3, 4]$ $[1, 3]$ $[1, 2, 4, 6]$
Your task is to find any suitable permutation (i.e. any permutation corresponding to the given input data). It is guaranteed that the input data corresponds to some permutation (i.e. such permutation exists).
You have to answer $t$ independent test cases.
-----Input-----
The first line of the input contains one integer $t$ ($1 \le t \le 100$) — the number of test cases. Then $t$ test cases follow.
The first line of the test case contains one integer $n$ ($2 \le n \le 200$) — the length of the permutation.
The next $n-1$ lines describe given segments.
The $i$-th line contains the description of the $i$-th segment. The line starts with the integer $k_i$ ($2 \le k_i \le n$) — the length of the $i$-th segment. Then $k_i$ integers follow. All integers in a line are distinct, sorted in ascending order, between $1$ and $n$, inclusive.
It is guaranteed that the required $p$ exists for each test case.
It is also guaranteed that the sum of $n$ over all test cases does not exceed $200$ ($\sum n \le 200$).
-----Output-----
For each test case, print the answer: $n$ integers $p_1, p_2, \dots, p_n$ ($1 \le p_i \le n$, all $p_i$ should be distinct) — any suitable permutation (i.e. any permutation corresponding to the test case input).
-----Example-----
Input
5
6
3 2 5 6
2 4 6
3 1 3 4
2 1 3
4 1 2 4 6
5
2 2 3
2 1 2
2 1 4
2 4 5
7
3 1 2 6
4 1 3 5 6
2 1 2
3 4 5 7
6 1 2 3 4 5 6
3 1 3 6
2
2 1 2
5
2 2 5
3 2 3 5
4 2 3 4 5
5 1 2 3 4 5
Output
3 1 4 6 2 5
3 2 1 4 5
2 1 6 3 5 4 7
1 2
2 5 3 4 1 | import sys
sys.setrecursionlimit(10 ** 6)
int1 = lambda x: int(x) - 1
p2D = lambda x: print(*x, sep="\n")
def II(): return int(sys.stdin.readline())
def MI(): return map(int, sys.stdin.readline().split())
def LI(): return list(map(int, sys.stdin.readline().split()))
def LI1(): return list(map(int1, sys.stdin.readline().split()))
def LLI(rows_number): return [LI() for _ in range(rows_number)]
def SI(): return sys.stdin.readline()[:-1]
def main():
def ok():
if len(ans)<n:return False
for r,si in enumerate(sii,2):
s=ss[si]
cur=set(ans[r-len(s):r])
if s!=cur:return False
return True
for _ in range(II()):
n=II()
ee=[[n**2]*n for _ in range(n)]
for ei in range(n):ee[ei][ei]=0
ss=[set(LI()[1:]) for _ in range(n-1)]
for a0 in range(1,n+1):
used=set([a0])
ans=[a0]
sii=[]
for _ in range(n-1):
nxt=[]
for si,s in enumerate(ss):
cur=s-used
if len(cur)==1:
for a in cur:nxt.append(a)
sii.append(si)
if len(nxt)!=1:break
ans.append(nxt[0])
used.add(nxt[0])
if ok():break
print(*ans)
main() | import sys
sys.setrecursionlimit(10 ** 6)
int1 = lambda x: int(x) - 1
p2D = lambda x: print(*x, sep="\n")
def II(): return int(sys.stdin.readline())
def MI(): return map(int, sys.stdin.readline().split())
def LI(): return list(map(int, sys.stdin.readline().split()))
def LI1(): return list(map(int1, sys.stdin.readline().split()))
def LLI(rows_number): return [LI() for _ in range(rows_number)]
def SI(): return sys.stdin.readline()[:-1]
def main():
def ok():
if len(ans)<n:return False
for r,si in enumerate(sii,2):
s=ss[si]
cur=set(ans[r-len(s):r])
if s!=cur:return False
return True
for _ in range(II()):
n=II()
ee=[[n**2]*n for _ in range(n)]
for ei in range(n):ee[ei][ei]=0
ss=[set(LI()[1:]) for _ in range(n-1)]
for a0 in range(1,n+1):
used=set([a0])
ans=[a0]
sii=[]
for _ in range(n-1):
nxt=[]
for si,s in enumerate(ss):
cur=s-used
if len(cur)==1:
for a in cur:nxt.append(a)
sii.append(si)
if len(nxt)!=1:break
ans.append(nxt[0])
used.add(nxt[0])
if ok():break
print(*ans)
main() | train | APPS_structured |
A mad scientist Dr.Jubal has made a competitive programming task. Try to solve it!
You are given integers $n,k$. Construct a grid $A$ with size $n \times n$ consisting of integers $0$ and $1$. The very important condition should be satisfied: the sum of all elements in the grid is exactly $k$. In other words, the number of $1$ in the grid is equal to $k$.
Let's define: $A_{i,j}$ as the integer in the $i$-th row and the $j$-th column. $R_i = A_{i,1}+A_{i,2}+...+A_{i,n}$ (for all $1 \le i \le n$). $C_j = A_{1,j}+A_{2,j}+...+A_{n,j}$ (for all $1 \le j \le n$). In other words, $R_i$ are row sums and $C_j$ are column sums of the grid $A$. For the grid $A$ let's define the value $f(A) = (\max(R)-\min(R))^2 + (\max(C)-\min(C))^2$ (here for an integer sequence $X$ we define $\max(X)$ as the maximum value in $X$ and $\min(X)$ as the minimum value in $X$).
Find any grid $A$, which satisfies the following condition. Among such grids find any, for which the value $f(A)$ is the minimum possible. Among such tables, you can find any.
-----Input-----
The input consists of multiple test cases. The first line contains a single integer $t$ ($1 \le t \le 100$) — the number of test cases. Next $t$ lines contain descriptions of test cases.
For each test case the only line contains two integers $n$, $k$ $(1 \le n \le 300, 0 \le k \le n^2)$.
It is guaranteed that the sum of $n^2$ for all test cases does not exceed $10^5$.
-----Output-----
For each test case, firstly print the minimum possible value of $f(A)$ among all tables, for which the condition is satisfied.
After that, print $n$ lines contain $n$ characters each. The $j$-th character in the $i$-th line should be equal to $A_{i,j}$.
If there are multiple answers you can print any.
-----Example-----
Input
4
2 2
3 8
1 0
4 16
Output
0
10
01
2
111
111
101
0
0
0
1111
1111
1111
1111
-----Note-----
In the first test case, the sum of all elements in the grid is equal to $2$, so the condition is satisfied. $R_1 = 1, R_2 = 1$ and $C_1 = 1, C_2 = 1$. Then, $f(A) = (1-1)^2 + (1-1)^2 = 0$, which is the minimum possible value of $f(A)$.
In the second test case, the sum of all elements in the grid is equal to $8$, so the condition is satisfied. $R_1 = 3, R_2 = 3, R_3 = 2$ and $C_1 = 3, C_2 = 2, C_3 = 3$. Then, $f(A) = (3-2)^2 + (3-2)^2 = 2$. It can be proven, that it is the minimum possible value of $f(A)$. | t=int(input())
for _ in range(t):
n,k=map(int,input().split())
a=k//n
rem=k%n
grid=[]
for i in range(n):
grid.append([])
for j in range(n):
grid[-1].append('0')
for i in range(n):
for j in range(i,i+a):
grid[i][j%n]='1'
if i<rem:
grid[i][(i+a)%n]='1'
ans=0
r=[]
for i in range(n):
p=0
for j in range(n):
if grid[i][j]=='1':
p+=1
r.append(p)
c=[]
for i in range(n):
p=0
for j in range(n):
if grid[j][i]=='1':
p+=1
c.append(p)
print((max(r)-min(r))**2+(max(c)-min(c))**2)
for i in range(n):
ans=''.join(grid[i])
print(ans) | t=int(input())
for _ in range(t):
n,k=map(int,input().split())
a=k//n
rem=k%n
grid=[]
for i in range(n):
grid.append([])
for j in range(n):
grid[-1].append('0')
for i in range(n):
for j in range(i,i+a):
grid[i][j%n]='1'
if i<rem:
grid[i][(i+a)%n]='1'
ans=0
r=[]
for i in range(n):
p=0
for j in range(n):
if grid[i][j]=='1':
p+=1
r.append(p)
c=[]
for i in range(n):
p=0
for j in range(n):
if grid[j][i]=='1':
p+=1
c.append(p)
print((max(r)-min(r))**2+(max(c)-min(c))**2)
for i in range(n):
ans=''.join(grid[i])
print(ans) | train | APPS_structured |
Chef's friend Alex runs a movie theatre. Due to the increasing number of platforms for watching movies online, his business is not running well. As a friend, Alex asked Chef to help him maximise his profits. Since Chef is a busy person, he needs your help to support his friend Alex.
Alex's theatre has four showtimes: 12 PM, 3 PM, 6 PM and 9 PM. He has four movies which he would like to play ― let's call them A, B, C and D. Each of these movies must be played exactly once and all four must be played at different showtimes. For each showtime, the price of a ticket must be one of the following: Rs 25, Rs 50, Rs 75 or Rs 100. The prices of tickets for different showtimes must also be different.
Through his app, Alex receives various requests from his customers. Each request has the form "I want to watch this movie at this showtime". Let's assume that the number of people who come to watch a movie at a given showtime is the same as the number of requests for that movie at that showtime.
It is not necessary to accommodate everyone's requests ― Alex just wants to earn the maximum amount of money. There is no restriction on the capacity of the theatre. However, for each movie that is not watched by anyone, Alex would suffer a loss of Rs 100 (deducted from the profit).
You are given $N$ requests Alex received during one day. Find the maximum amount of money he can earn on that day by choosing when to play which movies and with which prices.
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first line of each test case contains a single integer $N$.
- $N$ lines follow. Each of these lines contains a character $m$, followed by a space and an integer $t$, describing a request to see the movie $m$ at the showtime $t$.
-----Output-----
For each test case, print a single line containing one integer ― the maximum profit Alex can earn (possibly negative).
Finally, print a line containing one integer ― the total profit over all test cases, i.e. over $T$ days.
-----Constraints-----
- $1 \le T \le 150$
- $0 \le N \le 100$
- $m$ is 'A', 'B', 'C' or 'D'
- $t$ is $12$, $3$, $6$ or $9$
-----Subtasks-----
Subtask #1 (30 points): it is possible to fulfill all requests
Subtask #2 (70 points): original constraints
-----Example Input-----
5
12
A 3
B 12
C 6
A 9
B 12
C 12
D 3
B 9
D 3
B 12
B 9
C 6
7
A 9
A 9
B 6
C 3
D 12
A 9
B 6
2
A 9
B 6
1
D 12
0
-----Example Output-----
575
525
-25
-200
-400
475
-----Explanation-----
Example case 1: The following table shows the number of people that want to watch the movies at the given showtimes: 12 3 6 9 A 0 1 0 1 B 3 0 0 2 C 1 0 2 0 D 0 2 0 0
The maximum number of requests was sent for movie B at 12 PM. Therefore, we play this movie at this time and the tickets cost Rs 100. Next, we play movie D at 3 PM with ticket price Rs 75 and movie C at 6 PM with ticket price Rs 50. Finally, we have a slot for 9 PM and the only movie we can play at that time now is movie A, with ticket price Rs 25. The total profit is $3 \cdot 100 + 2 \cdot 75 + 2 \cdot 50 + 1 \cdot 25 = 300 + 150 + 100 + 25 = 575$. Since each movie was watched by at least one person, there is no additional loss.
Example case 2: Just like above, we show the requests in a table: 12 3 6 9 A 0 0 0 3 B 0 0 2 0 C 0 1 0 0 D 1 0 0 0
The optimal solution is to play movie A at 9 PM, movie B at 6 PM, movie C at 3 PM and movie D at 12 PM, with decreasing ticket prices in this order. The profit is $3 \cdot 100 + 2 \cdot 75 + 1 \cdot 50 + 1 \cdot 25 = 300+150+50+25 = 525$.
Example case 3: Again, we show the requests in a table: 12 3 6 9 A 0 0 0 1 B 0 0 1 0 C 0 0 0 0 D 0 0 0 0
The optimal solution is to play movie A at 9 PM with ticket price Rs 100, movie B at 6 PM with ticket price Rs 75 and the remaining two movies in any order at 12 PM and 3 PM ― either way, there will be nobody watching them. We earn $1 \cdot 100 + 1 \cdot 75 = 175$, but we have to deduct Rs 200, so the resulting profit is $175 - 200 = -25$.
Example case 4: The optimal solution is to play movie D at 12 PM; the other three movies go unattended. We have to deduct Rs 300, so the profit is $1 \cdot 100 - 300 = -200$.
Example case 5: Since there are no requests for any movie at any time, all movies go unattended and Alex just suffers a loss of Rs 400.
The total profit for all 5 days is $575+525-25-200-400 = 475$. | # cook your dish here
from sys import stdin,stdout
I1=lambda:int(stdin.readline())
T=0
for _ in range(I1()):
m=I1()
li2=[12,3,6,9]
d={'A':[0,0,0,0],'B':[0,0,0,0],'C':[0,0,0,0],'D':[0,0,0,0]}
for i in range(m):
x,t=list(map(str,stdin.readline().split()))
t=int(t)
d[x][li2.index(t)]+=1
#print(d)
n=4
ans=[]
cost=[100,75,50,25]
for i in range(n):
li1=[]
li1.append(d['A'][i])
for j in range(n):
if j!=i:
li1.append(d['B'][j])
for k in range(n):
if k!=i and k!=j:
li1.append(d['C'][k])
for l in range(n):
if l!=i and l!=j and l!=k:
li1.append(d['D'][l])
c=0
z=0
for s in sorted(li1,reverse=True):
if s==0:
c-=100
else:
c+=s*cost[z]
z+=1
if c!=0:
ans.append(c)
li1.pop()
li1.pop()
li1.pop()
li1.pop()
#print(ans)
a=max(ans)
print(a)
T+=a
print(T)
| # cook your dish here
from sys import stdin,stdout
I1=lambda:int(stdin.readline())
T=0
for _ in range(I1()):
m=I1()
li2=[12,3,6,9]
d={'A':[0,0,0,0],'B':[0,0,0,0],'C':[0,0,0,0],'D':[0,0,0,0]}
for i in range(m):
x,t=list(map(str,stdin.readline().split()))
t=int(t)
d[x][li2.index(t)]+=1
#print(d)
n=4
ans=[]
cost=[100,75,50,25]
for i in range(n):
li1=[]
li1.append(d['A'][i])
for j in range(n):
if j!=i:
li1.append(d['B'][j])
for k in range(n):
if k!=i and k!=j:
li1.append(d['C'][k])
for l in range(n):
if l!=i and l!=j and l!=k:
li1.append(d['D'][l])
c=0
z=0
for s in sorted(li1,reverse=True):
if s==0:
c-=100
else:
c+=s*cost[z]
z+=1
if c!=0:
ans.append(c)
li1.pop()
li1.pop()
li1.pop()
li1.pop()
#print(ans)
a=max(ans)
print(a)
T+=a
print(T)
| train | APPS_structured |
# A History Lesson
Tetris is a puzzle video game originally designed and programmed by Soviet Russian software engineer Alexey Pajitnov. The first playable version was completed on June 6, 1984. Pajitnov derived its name from combining the Greek numerical prefix tetra- (the falling pieces contain 4 segments) and tennis, Pajitnov's favorite sport.
# About scoring system
The scoring formula is built on the idea that more difficult line clears should be awarded more points. For example, a single line clear is worth `40` points, clearing four lines at once (known as a Tetris) is worth `1200`.
A level multiplier is also used. The game starts at level `0`. The level increases every ten lines you clear. Note that after increasing the level, the total number of cleared lines is not reset.
For our task you can use this table:
.demo {
width:70%;
border:1px solid #C0C0C0;
border-collapse:collapse;
padding:5px;
}
.demo th {
border:1px solid #C0C0C0;
padding:5px;
}
.demo td {
border:1px solid #C0C0C0;
padding:5px;
}
Level
Points for 1 line
Points for 2 lines
Points for 3 lines
Points for 4 lines
0
40
100
300
1200
1
80
200
600
2400
2
120
300
900
3600
3
160
400
1200
4800
...
7
320
800
2400
9600
...
For level n you must determine the formula by yourself using given examples from the table.
# Task
Calculate the final score of the game using original Nintendo scoring system
# Input
Array with cleaned lines.
Example: `[4, 2, 2, 3, 3, 4, 2]`
Input will always be valid: array of random length (from `0` to `5000`) with numbers from `0` to `4`.
# Ouput
Calculated final score.
`def get_score(arr) -> int: return 0`
# Example
```python
get_score([4, 2, 2, 3, 3, 4, 2]); # returns 4900
```
Step 1: `+1200` points for 4 lines (current level `0`). Score: `0+1200=1200`;\
Step 2: `+100` for 2 lines. Score: `1200+100=1300`;\
Step 3: `+100`. Score: `1300+100=1400`;\
Step 4: `+300` for 3 lines (current level still `0`). Score: `1400+300=1700`.\
Total number of cleaned lines 11 (`4 + 2 + 2 + 3`), so level goes up to `1` (level ups each 10 lines);\
Step 5: `+600` for 3 lines (current level `1`). Score: `1700+600=2300`;\
Step 6: `+2400`. Score: `2300+2400=4700`;\
Step 7: `+200`. Total score: `4700+200=4900` points.
# Other
If you like the idea: leave feedback, and there will be more katas in the Tetris series.
* 7 kyuTetris Series #1 — Scoring System
* 6 kyuTetris Series #2 — Primitive Gameplay
* 6 kyuTetris Series #3 — Adding Rotation (TBA)
* 5 kyuTetris Series #4 — New Block Types (TBA)
* 4 kyuTetris Series #5 — Complex Block Types (TBA?) | def get_score(arr) -> int:
return sum([0, 40, 100, 300, 1200][n] * (1 + (sum(arr[:i]) // 10)) for i, n in enumerate(arr)) | def get_score(arr) -> int:
return sum([0, 40, 100, 300, 1200][n] * (1 + (sum(arr[:i]) // 10)) for i, n in enumerate(arr)) | train | APPS_structured |
You have a total of n coins that you want to form in a staircase shape, where every k-th row must have exactly k coins.
Given n, find the total number of full staircase rows that can be formed.
n is a non-negative integer and fits within the range of a 32-bit signed integer.
Example 1:
n = 5
The coins can form the following rows:
¤
¤ ¤
¤ ¤
Because the 3rd row is incomplete, we return 2.
Example 2:
n = 8
The coins can form the following rows:
¤
¤ ¤
¤ ¤ ¤
¤ ¤
Because the 4th row is incomplete, we return 3. | class Solution:
def arrangeCoins(self, n):
"""
:type n: int
:rtype: int
"""
return int((1+8*n)**0.5 - 1) // 2 | class Solution:
def arrangeCoins(self, n):
"""
:type n: int
:rtype: int
"""
return int((1+8*n)**0.5 - 1) // 2 | train | APPS_structured |
Given an array of strings arr. String s is a concatenation of a sub-sequence of arr which have unique characters.
Return the maximum possible length of s.
Example 1:
Input: arr = ["un","iq","ue"]
Output: 4
Explanation: All possible concatenations are "","un","iq","ue","uniq" and "ique".
Maximum length is 4.
Example 2:
Input: arr = ["cha","r","act","ers"]
Output: 6
Explanation: Possible solutions are "chaers" and "acters".
Example 3:
Input: arr = ["abcdefghijklmnopqrstuvwxyz"]
Output: 26
Constraints:
1 <= arr.length <= 16
1 <= arr[i].length <= 26
arr[i] contains only lower case English letters. | class Solution:
def maxLength(self, arr: List[str]) -> int:
res = 0
def dfs(n, s, l, cur):
nonlocal res
if n == l:
if len(set(cur)) == len(cur):
res = max(res, len(cur))
return
for i in range(s, len(arr)):
new_cur = cur + arr[i]
if len(set(cur)) != len(cur):
return
dfs(n, i+1, l+1, new_cur)
for i in range(len(arr)+1):
dfs(i, 0, 0, '')
return res
| class Solution:
def maxLength(self, arr: List[str]) -> int:
res = 0
def dfs(n, s, l, cur):
nonlocal res
if n == l:
if len(set(cur)) == len(cur):
res = max(res, len(cur))
return
for i in range(s, len(arr)):
new_cur = cur + arr[i]
if len(set(cur)) != len(cur):
return
dfs(n, i+1, l+1, new_cur)
for i in range(len(arr)+1):
dfs(i, 0, 0, '')
return res
| train | APPS_structured |
In order to establish dominance amongst his friends, Chef has decided that he will only walk in large steps of length exactly $K$ feet. However, this has presented many problems in Chef’s life because there are certain distances that he cannot traverse. Eg. If his step length is $5$ feet, he cannot travel a distance of $12$ feet. Chef has a strict travel plan that he follows on most days, but now he is worried that some of those distances may become impossible to travel. Given $N$ distances, tell Chef which ones he cannot travel.
-----Input:-----
- The first line will contain a single integer $T$, the number of test cases.
- The first line of each test case will contain two space separated integers - $N$, the number of distances, and $K$, Chef’s step length.
- The second line of each test case will contain $N$ space separated integers, the $i^{th}$ of which represents $D_i$, the distance of the $i^{th}$ path.
-----Output:-----
For each testcase, output a string consisting of $N$ characters. The $i^{th}$ character should be $1$ if the distance is traversable, and $0$ if not.
-----Constraints-----
- $1 \leq T \leq 1000$
- $1 \leq N \leq 1000$
- $1 \leq K \leq 10^9$
- $1 \leq D_i \leq 10^9$
-----Subtasks-----
- 100 points : No additional constraints.
-----Sample Input:-----
1
5 3
12 13 18 20 27216
-----Sample Output:-----
10101
-----Explanation:-----
The first distance can be traversed in $4$ steps.
The second distance cannot be traversed.
The third distance can be traversed in $6$ steps.
The fourth distance cannot be traversed.
The fifth distance can be traversed in $9072$ steps. | t=int(input())
for i in range(0,t):
n,k=map(int,input().split())
a1,*a=map(int,input().split())
a.insert(0,a1)
j=0
while j<n:
if a[j]%k==0:
print(1,end="")
else:
print(0,end="")
j+=1
print("") | t=int(input())
for i in range(0,t):
n,k=map(int,input().split())
a1,*a=map(int,input().split())
a.insert(0,a1)
j=0
while j<n:
if a[j]%k==0:
print(1,end="")
else:
print(0,end="")
j+=1
print("") | train | APPS_structured |
In this Kata, we will check if a string contains consecutive letters as they appear in the English alphabet and if each letter occurs only once.
```Haskell
Rules are: (1) the letters are adjacent in the English alphabet, and (2) each letter occurs only once.
For example:
solve("abc") = True, because it contains a,b,c
solve("abd") = False, because a, b, d are not consecutive/adjacent in the alphabet, and c is missing.
solve("dabc") = True, because it contains a, b, c, d
solve("abbc") = False, because b does not occur once.
solve("v") = True
```
All inputs will be lowercase letters.
More examples in test cases. Good luck! | def solve(s):
return len(s) == ord(max(s)) - ord(min(s)) + 1 | def solve(s):
return len(s) == ord(max(s)) - ord(min(s)) + 1 | train | APPS_structured |
>When no more interesting kata can be resolved, I just choose to create the new kata, to solve their own, to enjoy the process --myjinxin2015 said
# Description:
Give you two arrays `arr1` and `arr2`. They have the same length(length>=2). The elements of two arrays always be integer.
Sort `arr1` according to the ascending order of arr2; Sort `arr2` according to the ascending order of arr1. Description is not easy to understand, for example:
```
arr1=[5,4,3,2,1],
arr2=[6,5,7,8,9]
```
Let us try to sorting arr1.
First, we need know the ascending order of arr2:
```
[5,6,7,8,9]
```
We can see, after sort arr2 to ascending order, some elements' index are changed:
```
unsort arr2 ascending order arr2
[6,5,7,8,9]---> [5,6,7,8,9]
index0(6) ---> index1
index1(5) ---> index0
index2(7) index2(no change)
index3(8) index3(no change)
index4(9) index4(no change)
```
So, wo need according to these changes to sorting arr1:
```
unsort arr1 sorted arr1
[5,4,3,2,1]---> [4,5,3,2,1]
index0(5) ---> index1
index1(4) ---> index0
index2(3) index2(no change)
index3(2) index3(no change)
index4(1) index4(no change)
So: sorted arr1= [4,5,3,2,1]
```
And then, we sorting arr2 with the same process:
```
unsort arr1 ascending order arr1
[5,4,3,2,1]---> [1,2,3,4,5]
index0(5) ---> index4
index1(4) ---> index3
index2(3) index2(no change)
index3(2) ---> index1
index4(1) ---> index0
unsort arr2 sorted arr2
[6,5,7,8,9]---> [9,8,7,5,6]
index0(6) ---> index4
index1(5) ---> index3
index2(7) index2(no change)
index3(8) ---> index1
index4(9) ---> index0
So: sorted arr2= [9,8,7,5,6]
```
Finally, returns the sorted arrays by a 2D array: `[sorted arr1, sorted arr2]`
Note: In ascending order sorting process(not the final sort), if some elements have same value, sort them according to their index; You can modify the original array, but I advise you not to do that. ;-)
```if:haskell
Note: In Haskell, you are expected to return a tuple of arrays, and good luck trying to modifiy the original array. :]
```
# Some Examples and explain
```
sortArrays([5,4,3,2,1],[6,5,7,8,9]) should return
[[4,5,3,2,1],[9,8,7,5,6]]
sortArrays([2,1,3,4,5],[5,6,7,8,9]) should return
[[2,1,3,4,5],[6,5,7,8,9]]
sortArrays([5,6,9,2,6,5],[3,6,7,4,8,1]) should return
[[5,5,2,6,9,6],[4,3,1,6,8,7]]
``` | import numpy as np
def sort_two_arrays(arr1, arr2):
idx2 = np.argsort(arr2, kind='mergesort')
idx1 = np.argsort(arr1, kind='mergesort')
return [[arr1[i] for i in idx2], [arr2[i] for i in idx1]] | import numpy as np
def sort_two_arrays(arr1, arr2):
idx2 = np.argsort(arr2, kind='mergesort')
idx1 = np.argsort(arr1, kind='mergesort')
return [[arr1[i] for i in idx2], [arr2[i] for i in idx1]] | train | APPS_structured |
# Task
Given a string `str`, find the shortest possible string which can be achieved by adding characters to the end of initial string to make it a palindrome.
# Example
For `str = "abcdc"`, the output should be `"abcdcba"`.
# Input/Output
- `[input]` string `str`
A string consisting of lowercase latin letters.
Constraints: `3 ≤ str.length ≤ 10`.
- `[output]` a string | def build_palindrome(s):
if s == s[::-1]:
return s
else:
for i in range(1,len(s)):
if s + s[:i][::-1] == (s + s[:i][::-1])[::-1]:
return s + s[:i][::-1]
| def build_palindrome(s):
if s == s[::-1]:
return s
else:
for i in range(1,len(s)):
if s + s[:i][::-1] == (s + s[:i][::-1])[::-1]:
return s + s[:i][::-1]
| train | APPS_structured |
Given a wooden stick of length n units. The stick is labelled from 0 to n. For example, a stick of length 6 is labelled as follows:
Given an integer array cuts where cuts[i] denotes a position you should perform a cut at.
You should perform the cuts in order, you can change the order of the cuts as you wish.
The cost of one cut is the length of the stick to be cut, the total cost is the sum of costs of all cuts. When you cut a stick, it will be split into two smaller sticks (i.e. the sum of their lengths is the length of the stick before the cut). Please refer to the first example for a better explanation.
Return the minimum total cost of the cuts.
Example 1:
Input: n = 7, cuts = [1,3,4,5]
Output: 16
Explanation: Using cuts order = [1, 3, 4, 5] as in the input leads to the following scenario:
The first cut is done to a rod of length 7 so the cost is 7. The second cut is done to a rod of length 6 (i.e. the second part of the first cut), the third is done to a rod of length 4 and the last cut is to a rod of length 3. The total cost is 7 + 6 + 4 + 3 = 20.
Rearranging the cuts to be [3, 5, 1, 4] for example will lead to a scenario with total cost = 16 (as shown in the example photo 7 + 4 + 3 + 2 = 16).
Example 2:
Input: n = 9, cuts = [5,6,1,4,2]
Output: 22
Explanation: If you try the given cuts ordering the cost will be 25.
There are much ordering with total cost <= 25, for example, the order [4, 6, 5, 2, 1] has total cost = 22 which is the minimum possible.
Constraints:
2 <= n <= 10^6
1 <= cuts.length <= min(n - 1, 100)
1 <= cuts[i] <= n - 1
All the integers in cuts array are distinct. | from math import inf
class Solution:
def minCost(self, n: int, cuts: List[int]) -> int:
def recurse(l,r):
# if r<=l:
# return inf
if self.dp.get((l,r),-1)!=-1:
return self.dp[(l,r)]
mnCut=inf
flag=1
for i in range(len(cuts)):
if l<cuts[i]<r:
mnCut=min(mnCut,recurse(l,cuts[i])+recurse(cuts[i],r))
flag=0
if flag==0:
self.dp[(l,r)]=mnCut+r-l
else:
self.dp[(l,r)]=0
return self.dp[(l,r)]
self.dp={}
cuts.sort()
mn=recurse(0,n)
# print(self.dp)
return mn | from math import inf
class Solution:
def minCost(self, n: int, cuts: List[int]) -> int:
def recurse(l,r):
# if r<=l:
# return inf
if self.dp.get((l,r),-1)!=-1:
return self.dp[(l,r)]
mnCut=inf
flag=1
for i in range(len(cuts)):
if l<cuts[i]<r:
mnCut=min(mnCut,recurse(l,cuts[i])+recurse(cuts[i],r))
flag=0
if flag==0:
self.dp[(l,r)]=mnCut+r-l
else:
self.dp[(l,r)]=0
return self.dp[(l,r)]
self.dp={}
cuts.sort()
mn=recurse(0,n)
# print(self.dp)
return mn | train | APPS_structured |
Chef is multi-talented. He has developed a cure for coronavirus called COVAC-19. Now that everyone in the world is infected, it is time to distribute it throughout the world efficiently to wipe out coronavirus from the Earth. Chef just cooks the cure, you are his distribution manager.
In the world, there are $N$ countries (numbered $1$ through $N$) with populations $a_1, a_2, \ldots, a_N$. Each cure can be used to cure one infected person once. Due to lockdown rules, you may only deliver cures to one country per day, but you may choose that country arbitrarily and independently on each day. Days are numbered by positive integers. On day $1$, Chef has $x$ cures ready. On each subsequent day, Chef can supply twice the number of cures that were delivered (i.e. people that were cured) on the previous day. Chef cannot supply leftovers from the previous or any earlier day, as the cures expire in a day. The number of cures delivered to some country on some day cannot exceed the number of infected people it currently has, either.
However, coronavirus is not giving up so easily. It can infect a cured person that comes in contact with an infected person again ― formally, it means that the number of infected people in a country doubles at the end of each day, i.e. after the cures for this day are used (obviously up to the population of that country).
Find the minimum number of days needed to make the world corona-free.
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first line of each test case contains two space-separated integers $N$ and $x$.
- The second line contains $N$ space-separated integers $a_1, a_2, \ldots, a_N$.
-----Output-----
For each test case, print a single line containing one integer ― the minimum number of days.
-----Constraints-----
- $1 \le T \le 10^3$
- $1 \le N \le 10^5$
- $1 \le a_i \le 10^9$ for each valid $i$
- $1 \le x \le 10^9$
- the sum of $N$ over all test cases does not exceed $10^6$
-----Subtasks-----
Subtask #1 (20 points): $a_1 = a_2 = \ldots = a_N$
Subtask #2 (80 points): original constraints
-----Example Input-----
3
5 5
1 2 3 4 5
5 1
40 30 20 10 50
3 10
20 1 110
-----Example Output-----
5
9
6 | # cook your dish here
# cook your dish here
from sys import stdin, stdout
import math
from itertools import permutations, combinations,permutations
from collections import defaultdict
from collections import Counter
from bisect import bisect_left
import sys
from queue import PriorityQueue
import operator as op
from functools import reduce
mod = 1000000007
def L():
return list(map(int, stdin.readline().split()))
def In():
return list(map(int, stdin.readline().split()))
def I():
return int(stdin.readline())
def printIn(ob):
return stdout.write(str(ob) + '\n')
def powerLL(n, p):
result = 1
while (p):
if (p & 1):
result = result * n % mod
p = int(p / 2)
n = n * n % mod
return result
def ncr(n, r):
r = min(r, n - r)
numer = reduce(op.mul, list(range(n, n - r, -1)), 1)
denom = reduce(op.mul, list(range(1, r + 1)), 1)
return numer // denom
# --------------------------------------
def myCode():
n, x = In()
lst = L()
count = 0
lst.sort()
for i in lst:
if (x>=i):
count+=1
x = max(x,2*i)
else:
while x<i:
count+=1
x = 2*x
count+=1
x = 2*i
print(count)
def main():
for t in range(I()):
myCode()
def __starting_point():
main()
__starting_point() | # cook your dish here
# cook your dish here
from sys import stdin, stdout
import math
from itertools import permutations, combinations,permutations
from collections import defaultdict
from collections import Counter
from bisect import bisect_left
import sys
from queue import PriorityQueue
import operator as op
from functools import reduce
mod = 1000000007
def L():
return list(map(int, stdin.readline().split()))
def In():
return list(map(int, stdin.readline().split()))
def I():
return int(stdin.readline())
def printIn(ob):
return stdout.write(str(ob) + '\n')
def powerLL(n, p):
result = 1
while (p):
if (p & 1):
result = result * n % mod
p = int(p / 2)
n = n * n % mod
return result
def ncr(n, r):
r = min(r, n - r)
numer = reduce(op.mul, list(range(n, n - r, -1)), 1)
denom = reduce(op.mul, list(range(1, r + 1)), 1)
return numer // denom
# --------------------------------------
def myCode():
n, x = In()
lst = L()
count = 0
lst.sort()
for i in lst:
if (x>=i):
count+=1
x = max(x,2*i)
else:
while x<i:
count+=1
x = 2*x
count+=1
x = 2*i
print(count)
def main():
for t in range(I()):
myCode()
def __starting_point():
main()
__starting_point() | train | APPS_structured |
# Task
Smartphones software security has become a growing concern related to mobile telephony. It is particularly important as it relates to the security of available personal information.
For this reason, Ahmed decided to encrypt phone numbers of contacts in such a way that nobody can decrypt them. At first he tried encryption algorithms very complex, but the decryption process is tedious, especially when he needed to dial a speed dial.
He eventually found the algorithm following: instead of writing the number itself, Ahmed multiplied by 10, then adds the result to the original number.
For example, if the phone number is `123`, after the transformation, it becomes `1353`. Ahmed truncates the result (from the left), so it has as many digits as the original phone number. In this example Ahmed wrote `353` instead of `123` in his smart phone.
Ahmed needs a program to recover the original phone number from number stored on his phone. The program return "impossible" if the initial number can not be calculated.
Note: There is no left leading zero in either the input or the output; Input `s` is given by string format, because it may be very huge ;-)
# Example
For `s="353"`, the result should be `"123"`
```
1230
+ 123
.......
= 1353
truncates the result to 3 digit -->"353"
So the initial number is "123"
```
For `s="123456"`, the result should be `"738496"`
```
7384960
+ 738496
.........
= 8123456
truncates the result to 6 digit -->"123456"
So the initial number is "738496"
```
For `s="4334"`, the result should be `"impossible"`
Because no such a number can be encrypted to `"4334"`.
# Input/Output
- `[input]` string `s`
string presentation of n with `1 <= n <= 10^100`
- `[output]` a string
The original phone number before encryption, or `"impossible"` if the initial number can not be calculated. | def decrypt(s):
for i in range(1, 100):
n = int(str(i) + s)
if n % 11 == 0 and len(str(n//11)) == len(s):
return str(n // 11)
return 'impossible' | def decrypt(s):
for i in range(1, 100):
n = int(str(i) + s)
if n % 11 == 0 and len(str(n//11)) == len(s):
return str(n // 11)
return 'impossible' | train | APPS_structured |
Define a function that takes in two non-negative integers `$a$` and `$b$` and returns the last decimal digit of `$a^b$`. Note that `$a$` and `$b$` may be very large!
For example, the last decimal digit of `$9^7$` is `$9$`, since `$9^7 = 4782969$`. The last decimal digit of `$({2^{200}})^{2^{300}}$`, which has over `$10^{92}$` decimal digits, is `$6$`. Also, please take `$0^0$` to be `$1$`.
You may assume that the input will always be valid.
## Examples
```python
last_digit(4, 1) # returns 4
last_digit(4, 2) # returns 6
last_digit(9, 7) # returns 9
last_digit(10, 10 ** 10) # returns 0
last_digit(2 ** 200, 2 ** 300) # returns 6
```
___
## Remarks
### JavaScript, C++, R, PureScript
Since these languages don't have native arbitrarily large integers, your arguments are going to be strings representing non-negative integers instead. | E = [
[0, 0, 0, 0], [1, 1, 1, 1],
[6, 2, 4, 8], [1, 3, 9, 7],
[6, 4, 6, 4], [5, 5, 5, 5],
[6, 6, 6, 6], [1, 7, 9, 3],
[6, 8, 4, 2], [1, 9, 1, 9]
]
def last_digit(n1, n2):
if n2 == 0: return 1
return E[n1 % 10][n2 % 4]
| E = [
[0, 0, 0, 0], [1, 1, 1, 1],
[6, 2, 4, 8], [1, 3, 9, 7],
[6, 4, 6, 4], [5, 5, 5, 5],
[6, 6, 6, 6], [1, 7, 9, 3],
[6, 8, 4, 2], [1, 9, 1, 9]
]
def last_digit(n1, n2):
if n2 == 0: return 1
return E[n1 % 10][n2 % 4]
| train | APPS_structured |
## Task
You are at position [0, 0] in maze NxN and you can **only** move in one of the four cardinal directions (i.e. North, East, South, West). Return `true` if you can reach position [N-1, N-1] or `false` otherwise.
Empty positions are marked `.`. Walls are marked `W`. Start and exit positions are empty in all test cases.
## Path Finder Series:
- [#1: can you reach the exit?](https://www.codewars.com/kata/5765870e190b1472ec0022a2)
- [#2: shortest path](https://www.codewars.com/kata/57658bfa28ed87ecfa00058a)
- [#3: the Alpinist](https://www.codewars.com/kata/576986639772456f6f00030c)
- [#4: where are you?](https://www.codewars.com/kata/5a0573c446d8435b8e00009f)
- [#5: there's someone here](https://www.codewars.com/kata/5a05969cba2a14e541000129) | def path_finder(maze):
maze = maze.split('\n')
mazeD = { (x,y):1 for x, e in enumerate(maze) for y, _ in enumerate(e) if _ == '.' }
exit, DMap, stack = (len(maze)-1, len(maze[0])-1), {}, [(0, 0)]
while stack:
x, y = stack.pop()
if not DMap.get((x,y)):
DMap[(x,y)] = 1
for xc, yc in ((x, y-1), (x, y+1), (x-1, y), (x+1, y)):
if mazeD.get((xc,yc)):
stack.append((xc, yc))
return DMap.get(exit, 0) | def path_finder(maze):
maze = maze.split('\n')
mazeD = { (x,y):1 for x, e in enumerate(maze) for y, _ in enumerate(e) if _ == '.' }
exit, DMap, stack = (len(maze)-1, len(maze[0])-1), {}, [(0, 0)]
while stack:
x, y = stack.pop()
if not DMap.get((x,y)):
DMap[(x,y)] = 1
for xc, yc in ((x, y-1), (x, y+1), (x-1, y), (x+1, y)):
if mazeD.get((xc,yc)):
stack.append((xc, yc))
return DMap.get(exit, 0) | train | APPS_structured |
Given a mathematical equation that has `*,+,-,/`, reverse it as follows:
```Haskell
solve("100*b/y") = "y/b*100"
solve("a+b-c/d*30") = "30*d/c-b+a"
```
More examples in test cases.
Good luck!
Please also try:
[Simple time difference](https://www.codewars.com/kata/5b76a34ff71e5de9db0000f2)
[Simple remove duplicates](https://www.codewars.com/kata/5ba38ba180824a86850000f7) | def solve(eq):
#
# implemantation takes advantage of abscence of spaces
#
leq = (eq.replace('*', ' * ')
.replace('/', ' / ')
.replace('+', ' + ')
.replace('-', ' - ')
.split(' '))
out = ''.join(leq[::-1])
return out
| def solve(eq):
#
# implemantation takes advantage of abscence of spaces
#
leq = (eq.replace('*', ' * ')
.replace('/', ' / ')
.replace('+', ' + ')
.replace('-', ' - ')
.split(' '))
out = ''.join(leq[::-1])
return out
| train | APPS_structured |
Raj is a math pro and number theory expert. One day, he met his age-old friend Chef. Chef claimed to be better at number theory than Raj, so Raj gave him some fuzzy problems to solve. In one of those problems, he gave Chef a 3$3$-tuple of non-negative integers (a0,b0,c0)$(a_0, b_0, c_0)$ and told Chef to convert it to another tuple (x,y,z)$(x, y, z)$.
Chef may perform the following operations any number of times (including zero) on his current tuple (a,b,c)$(a, b, c)$, in any order:
- Choose one element of this tuple, i.e. a$a$, b$b$ or c$c$. Either add 1$1$ to that element or subtract 1$1$ from it. The cost of this operation is 1$1$.
- Merge: Change the tuple to (a−1,b−1,c+1)$(a-1, b-1, c+1)$, (a−1,b+1,c−1)$(a-1, b+1, c-1)$ or (a+1,b−1,c−1)$(a+1, b-1, c-1)$, i.e. add 1$1$ to one element and subtract 1$1$ from the other two. The cost of this operation is 0$0$.
- Split: Change the tuple to (a−1,b+1,c+1)$(a-1, b+1, c+1)$, (a+1,b−1,c+1)$(a+1, b-1, c+1)$ or (a+1,b+1,c−1)$(a+1, b+1, c-1)$, i.e. subtract 1$1$ from one element and add 1$1$ to the other two. The cost of this operation is also 0$0$.
After each operation, all elements of Chef's tuple must be non-negative. It is not allowed to perform an operation that would make one or more elements of this tuple negative.
Can you help Chef find the minimum cost of converting the tuple (a0,b0,c0)$(a_0, b_0, c_0)$ to the tuple (x,y,z)$(x, y, z)$? It can be easily proved that it is always possible to convert any tuple of non-negative integers to any other.
-----Input-----
- The first line of the input contains a single integer T$T$ denoting the number of test cases. The description of T$T$ test cases follows.
- The first and only line of each test case contains six space-separated integers a0$a_0$, b0$b_0$, c0$c_0$, x$x$, y$y$ and z$z$.
-----Output-----
For each test case, print a single line containing one integer ― the minimum cost.
-----Constraints-----
- 1≤T≤105$1 \le T \le 10^5$
- 0≤a0,b0,c0,x,y,z≤1018$0 \le a_0, b_0, c_0, x, y, z \le 10^{18}$
-----Subtasks-----
Subtask #1 (20 points): 0≤a0,b0,c0,x,y,z≤100$0 \le a_0, b_0, c_0, x, y, z \le 100$
Subtask #2 (80 points): original constraints
-----Example Input-----
2
1 1 1 2 2 2
1 2 3 2 4 2
-----Example Output-----
0
1
-----Explanation-----
Example case 1: The tuple (1,1,1)$(1, 1, 1)$ can be converted to (2,2,2)$(2, 2, 2)$ using only three Split operations, with cost 0$0$: (1,1,1)→(2,0,2)→(1,1,3)→(2,2,2)$(1, 1, 1) \rightarrow (2, 0, 2) \rightarrow (1, 1, 3) \rightarrow (2, 2, 2)$.
Example case 2: We can use one addition operation and one Split operation: (1,2,3)→(1,3,3)→(2,4,2)$(1, 2, 3) \rightarrow (1, 3, 3) \rightarrow (2, 4, 2)$. | def ch(a,b,c,d,e,f):
d1=abs(a-d)
d2=abs(b-e)
d3=abs(c-f)
if(a+b+c==0 or d+e+f==0):
if(d1%2==d2%2 and d2%2==d3%2):
print(2)
else:
print(1)
else:
case=d1%2+d2%2+d3%2
if(case%3==0):
print(0)
else:
print(1)
t=int(input())
while(t):
t-=1
a,b,c,d,e,f=list(map(int,input().split()))
if(a==d and b==e and c==f):
print(0)
else:
ch(a,b,c,d,e,f)
| def ch(a,b,c,d,e,f):
d1=abs(a-d)
d2=abs(b-e)
d3=abs(c-f)
if(a+b+c==0 or d+e+f==0):
if(d1%2==d2%2 and d2%2==d3%2):
print(2)
else:
print(1)
else:
case=d1%2+d2%2+d3%2
if(case%3==0):
print(0)
else:
print(1)
t=int(input())
while(t):
t-=1
a,b,c,d,e,f=list(map(int,input().split()))
if(a==d and b==e and c==f):
print(0)
else:
ch(a,b,c,d,e,f)
| train | APPS_structured |
Write a function to split a string and convert it into an array of words. For example:
```python
"Robin Singh" ==> ["Robin", "Singh"]
"I love arrays they are my favorite" ==> ["I", "love", "arrays", "they", "are", "my", "favorite"]
``` | #input - string
#output - list/array of words
#edge cases - no input given, integer or other data type given
#assumptions - I need to take a string and return a list of the words in the string.
#sample data - ("Robin Singh"), ["Robin", "Singh"]
#do I need to create a separate array? no
#I can just use string split function
#function string_to_array(string)
def string_to_array(s):
return s.split(' ')
| #input - string
#output - list/array of words
#edge cases - no input given, integer or other data type given
#assumptions - I need to take a string and return a list of the words in the string.
#sample data - ("Robin Singh"), ["Robin", "Singh"]
#do I need to create a separate array? no
#I can just use string split function
#function string_to_array(string)
def string_to_array(s):
return s.split(' ')
| train | APPS_structured |
It's been a tough week at work and you are stuggling to get out of bed in the morning.
While waiting at the bus stop you realise that if you could time your arrival to the nearest minute you could get valuable extra minutes in bed.
There is a bus that goes to your office every 15 minute, the first bus is at `06:00`, and the last bus is at `00:00`.
Given that it takes 5 minutes to walk from your front door to the bus stop, implement a function that when given the curent time will tell you much time is left, before you must leave to catch the next bus.
## Examples
```
"05:00" => 55
"10:00" => 10
"12:10" => 0
"12:11" => 14
```
### Notes
1. Return the number of minutes till the next bus
2. Input will be formatted as `HH:MM` (24-hour clock)
3. The input time might be after the buses have stopped running, i.e. after `00:00` | def bus_timer(time):
c = time.split(":")
z = int(c[1]) + 5
t = 0
if int(c[0]) == 23 and int(c[1]) >= 55:
c[0] = 0
c[1] = (int(c[1]) + 5) - 60
if int(c[1]) == 0:
t = 0
elif int(c[1]) > 0:
t = (((5 - int(c[0]))*60) + (60 - int(c[1])))
elif int(c[0]) == 5 and int(c[1]) >= 55:
c[0] = 6
c[1] = (int(c[1]) + 5) - 60
if int(c[1]) == 0:
t = 0
elif int(c[1]) > 0:
t = 15 - int(c[1])
elif int(c[0]) < 6 :
if int(c[1]) == 0:
t = ((6 - int(c[0]))*60) - 5
elif int(c[1]) > 0:
t = (((5 - int(c[0]))*60) + (60 - int(c[1]))) - 5
else:
if z > 60:
z = z - 60
if z < 15:
t = 15 - z
elif z > 15 and z < 30:
t = 30 - z
elif z > 30 and z < 45:
t = 45 - z
elif z > 45:
t = 60 - z
return t | def bus_timer(time):
c = time.split(":")
z = int(c[1]) + 5
t = 0
if int(c[0]) == 23 and int(c[1]) >= 55:
c[0] = 0
c[1] = (int(c[1]) + 5) - 60
if int(c[1]) == 0:
t = 0
elif int(c[1]) > 0:
t = (((5 - int(c[0]))*60) + (60 - int(c[1])))
elif int(c[0]) == 5 and int(c[1]) >= 55:
c[0] = 6
c[1] = (int(c[1]) + 5) - 60
if int(c[1]) == 0:
t = 0
elif int(c[1]) > 0:
t = 15 - int(c[1])
elif int(c[0]) < 6 :
if int(c[1]) == 0:
t = ((6 - int(c[0]))*60) - 5
elif int(c[1]) > 0:
t = (((5 - int(c[0]))*60) + (60 - int(c[1]))) - 5
else:
if z > 60:
z = z - 60
if z < 15:
t = 15 - z
elif z > 15 and z < 30:
t = 30 - z
elif z > 30 and z < 45:
t = 45 - z
elif z > 45:
t = 60 - z
return t | train | APPS_structured |
You are given an array $a$ consisting of $n$ integers.
In one move, you can choose some index $i$ ($1 \le i \le n - 2$) and shift the segment $[a_i, a_{i + 1}, a_{i + 2}]$ cyclically to the right (i.e. replace the segment $[a_i, a_{i + 1}, a_{i + 2}]$ with $[a_{i + 2}, a_i, a_{i + 1}]$).
Your task is to sort the initial array by no more than $n^2$ such operations or say that it is impossible to do that.
You have to answer $t$ independent test cases.
-----Input-----
The first line of the input contains one integer $t$ ($1 \le t \le 100$) — the number of test cases. Then $t$ test cases follow.
The first line of the test case contains one integer $n$ ($3 \le n \le 500$) — the length of $a$. The second line of the test case contains $n$ integers $a_1, a_2, \dots, a_n$ ($1 \le a_i \le 500$), where $a_i$ is the $i$-th element $a$.
It is guaranteed that the sum of $n$ does not exceed $500$.
-----Output-----
For each test case, print the answer: -1 on the only line if it is impossible to sort the given array using operations described in the problem statement, or the number of operations $ans$ on the first line and $ans$ integers $idx_1, idx_2, \dots, idx_{ans}$ ($1 \le idx_i \le n - 2$), where $idx_i$ is the index of left border of the segment for the $i$-th operation. You should print indices in order of performing operations.
-----Example-----
Input
5
5
1 2 3 4 5
5
5 4 3 2 1
8
8 4 5 2 3 6 7 3
7
5 2 1 6 4 7 3
6
1 2 3 3 6 4
Output
0
6
3 1 3 2 2 3
13
2 1 1 6 4 2 4 3 3 4 4 6 6
-1
4
3 3 4 4 | for _ in range(int(input())):
n,l,out = int(input()),list(map(lambda x: int(x)- 1, input().split())),[];ll = sorted([(l[i], i) for i in range(n)]);swap = (-1,-1);newl = [0]*n
for i in range(n - 1):
if ll[i][0] == ll[i + 1][0]:swap = (ll[i][1],ll[i+1][1])
for i in range(n):newl[ll[i][1]] = i
l,swapN = newl,0;works,done = True,False
for i in range(n):
for j in range(i + 1, n):
if l[i] > l[j]:swapN += 1
if swapN & 1:l[swap[0]],l[swap[1]] = l[swap[1]],l[swap[0]]
def shift(i):out.append(i + 1);l[i],l[i+1],l[i+2] = l[i+2],l[i],l[i+1]
while not done:
for i in range(n):
if l[i] != i:break
else:done = True
if done:break
for find in range(i + 1, n):
if l[find] == i:break
while find - i >= 2:find -= 2;shift(find)
if find - i == 1:
if find <= n - 2:shift(find - 1);shift(find - 1)
else:works = False;break
if works:print(len(out));print(' '.join(map(str,out)))
else:print(-1) | for _ in range(int(input())):
n,l,out = int(input()),list(map(lambda x: int(x)- 1, input().split())),[];ll = sorted([(l[i], i) for i in range(n)]);swap = (-1,-1);newl = [0]*n
for i in range(n - 1):
if ll[i][0] == ll[i + 1][0]:swap = (ll[i][1],ll[i+1][1])
for i in range(n):newl[ll[i][1]] = i
l,swapN = newl,0;works,done = True,False
for i in range(n):
for j in range(i + 1, n):
if l[i] > l[j]:swapN += 1
if swapN & 1:l[swap[0]],l[swap[1]] = l[swap[1]],l[swap[0]]
def shift(i):out.append(i + 1);l[i],l[i+1],l[i+2] = l[i+2],l[i],l[i+1]
while not done:
for i in range(n):
if l[i] != i:break
else:done = True
if done:break
for find in range(i + 1, n):
if l[find] == i:break
while find - i >= 2:find -= 2;shift(find)
if find - i == 1:
if find <= n - 2:shift(find - 1);shift(find - 1)
else:works = False;break
if works:print(len(out));print(' '.join(map(str,out)))
else:print(-1) | train | APPS_structured |
In ACM-ICPC contests, there are usually three people in a team. For each person in the team, you know their scores in three skills - hard work, intelligence and persistence.
You want to check whether it is possible to order these people (assign them numbers from 1 to 3) in such a way that for each 1 ≤ i ≤ 2, i+1-th person is stricly better than the i-th person.
A person x is said to be better than another person y if x doesn't score less than y in any of the skills and scores more than y in at least one skill.
Determine whether such an ordering exists.
-----Input-----
The first line fo the input contains an integer T denoting the number of test cases.
Each test consists of three lines. Each of these lines contains three space separated integers s1, s2 and s3 denoting the scores of one member of the team in each of the three skills, in the given order.
-----Output-----
For each test case, output a single line containing "yes" if such an ordering exists or "no" if doesn't exist (without quotes).
-----Constraints-----
- 1 ≤ T ≤ 1000
- 1 ≤ s1, s2, s3 ≤ 100
-----Example-----
Input
3
1 2 3
2 3 4
2 3 5
1 2 3
2 3 4
2 3 4
5 6 5
1 2 3
2 3 4
Output
yes
no
yes
-----Explanation-----
Test Case 1: We can order them as (3, 2, 1). Person 3 is better than Person 2 because his scores in the first two skills are not lesser than Person 2's. And in skill 3, Person 3 scores higher. Similarly, Person 2 is better than Person 1. He scores more than Person 1 in every skill, in fact. | t=int(input())
for i in range(t):
list1=[]
for i in range(3):
list1.append(list(map(int,input().split())))
min1=min(list1[0][0],list1[1][0],list1[2][0])
min2=min(list1[0][1],list1[1][1],list1[2][1])
min3=min(list1[0][2],list1[1][2],list1[2][2])
max1=max(list1[0][0],list1[1][0],list1[2][0])
max2=max(list1[0][1],list1[1][1],list1[2][1])
max3=max(list1[0][2],list1[1][2],list1[2][2])
list2=[]
list3=[]
list2=[min1,min2,min3]
list3=[max1,max2,max3]
if((list2 not in list1) or (list3 not in list1)):
print("no")
elif((list2 in list1) and (list3 in list1)):
list1.remove(list2)
list1.remove(list3)
if(list1[0]!=list2 and list1[0]!=list3):
print("yes")
else:
print("no") | t=int(input())
for i in range(t):
list1=[]
for i in range(3):
list1.append(list(map(int,input().split())))
min1=min(list1[0][0],list1[1][0],list1[2][0])
min2=min(list1[0][1],list1[1][1],list1[2][1])
min3=min(list1[0][2],list1[1][2],list1[2][2])
max1=max(list1[0][0],list1[1][0],list1[2][0])
max2=max(list1[0][1],list1[1][1],list1[2][1])
max3=max(list1[0][2],list1[1][2],list1[2][2])
list2=[]
list3=[]
list2=[min1,min2,min3]
list3=[max1,max2,max3]
if((list2 not in list1) or (list3 not in list1)):
print("no")
elif((list2 in list1) and (list3 in list1)):
list1.remove(list2)
list1.remove(list3)
if(list1[0]!=list2 and list1[0]!=list3):
print("yes")
else:
print("no") | train | APPS_structured |
Given a binary tree, return the postorder traversal of its nodes' values.
Example:
Input: [1,null,2,3]
1
\
2
/
3
Output: [3,2,1]
Follow up: Recursive solution is trivial, could you do it iteratively? | # Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution:
def postorderTraversal(self, root):
"""
:type root: TreeNode
:rtype: List[int]
"""
path = []
def postOrder(root):
if not root:
return
postOrder(root.left)
postOrder(root.right)
path.append(root.val)
postOrder(root)
return path | # Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution:
def postorderTraversal(self, root):
"""
:type root: TreeNode
:rtype: List[int]
"""
path = []
def postOrder(root):
if not root:
return
postOrder(root.left)
postOrder(root.right)
path.append(root.val)
postOrder(root)
return path | train | APPS_structured |
I need to save some money to buy a gift. I think I can do something like that:
First week (W0) I save nothing on Sunday, 1 on Monday, 2 on Tuesday... 6 on Saturday,
second week (W1) 2 on Monday... 7 on Saturday and so on according to the table below where the days are numbered from 0 to 6.
Can you tell me how much I will have for my gift on Saturday evening after I have saved 12? (Your function finance(6) should return 168 which is the sum of the savings in the table).
Imagine now that we live on planet XY140Z-n where the days of the week are numbered from 0 to n (integer n > 0) and where
I save from week number 0 to week number n included (in the table below n = 6).
How much money would I have at the end of my financing plan on planet XY140Z-n?
-- |Su|Mo|Tu|We|Th|Fr|Sa|
--|--|--|--|--|--|--|--|
W6 | | | | | | |12|
W5 | | | | | |10|11|
W4 | | | | |8 |9 |10|
W3 | | | |6 |7 |8 |9 |
W2 | | |4 |5 |6 |7 |8 |
W1 | |2 |3 |4 |5 |6 |7 |
W0 |0 |1 |2 |3 |4 |5 |6 |
#Example:
```
finance(5) --> 105
finance(6) --> 168
finance(7) --> 252
finance(5000) --> 62537505000
```
#Hint:
try to avoid nested loops | def finance(n):
week = [sum(range(2*i, n+i+1)) for i in range(n+1)]
return sum(week) | def finance(n):
week = [sum(range(2*i, n+i+1)) for i in range(n+1)]
return sum(week) | train | APPS_structured |
# # Task:
* #### Complete the pattern, using the special character ```■ □```
* #### In this kata, we draw some histogram of the sound performance of ups and downs.
# # Rules:
- parameter ```waves``` The value of sound waves, an array of number, all number in array >=0.
- return a string, ```■``` represents the sound waves, and ```□``` represents the blank part, draw the histogram from bottom to top.
# # Example:
```
draw([1,2,3,4])
□□□■
□□■■
□■■■
■■■■
draw([1,2,3,3,2,1])
□□■■□□
□■■■■□
■■■■■■
draw([1,2,3,3,2,1,1,2,3,4,5,6,7])
□□□□□□□□□□□□■
□□□□□□□□□□□■■
□□□□□□□□□□■■■
□□□□□□□□□■■■■
□□■■□□□□■■■■■
□■■■■□□■■■■■■
■■■■■■■■■■■■■
draw([5,3,1,2,4,6,5,4,2,3,5,2,1])
□□□□□■□□□□□□□
■□□□□■■□□□■□□
■□□□■■■■□□■□□
■■□□■■■■□■■□□
■■□■■■■■■■■■□
■■■■■■■■■■■■■
draw([1,0,1,0,1,0,1,0])
■□■□■□■□
``` | def draw(waves):
m = max(waves)
return "\n".join("".join("□" if n < (m - row) else "■" for n in waves) for row in range(m))
| def draw(waves):
m = max(waves)
return "\n".join("".join("□" if n < (m - row) else "■" for n in waves) for row in range(m))
| train | APPS_structured |
Write a function that replaces 'two', 'too' and 'to' with the number '2'. Even if the sound is found mid word (like in octopus) or not in lowercase grandma still thinks that should be replaced with a 2. Bless her.
```text
'I love to text' becomes 'I love 2 text'
'see you tomorrow' becomes 'see you 2morrow'
'look at that octopus' becomes 'look at that oc2pus'
```
Note that 'too' should become '2', not '2o' | import re
def textin(str):
return re.sub('(?i)t[wo]?o','2',str) | import re
def textin(str):
return re.sub('(?i)t[wo]?o','2',str) | train | APPS_structured |
The cat wants to lay down on the table, but the problem is that we don't know where it is in the room!
You'll get in input:
- the cat coordinates as a list of length 2, with the row on the map and the column on the map.
- the map of the room as a list of lists where every element can be 0 if empty or 1 if is the table (there can be only one 1 in the room / lists will never be empty).
# Task:
You'll need to return the route to the table, beginning from the cat's starting coordinates, as a String. The route must be a sequence of letters U, D, R, L that means Up, Down, Right, Left. The order of the letters in the output isn't important.
# Outputs:
* The route for the cat to reach the table, as a string
* If there isn't a table in the room you must return "NoTable"
* if the cat is outside of the room you must return "NoCat" (this output has the precedence on the above one).
* If the cat is alredy on the table, you can return an empty String.
# Example:
```
cat = [0,1]
room =[[0,0,0], [0,0,0], [0,0,1]]
The route will be "RDD", or "DRD" or "DDR"
``` | def put_the_cat_on_the_table(cat, room):
if cat[0] < 0 or cat[0] >= len(room) or cat[1] < 0 or cat[1] >= len(room[0]):
return "NoCat"
tableCoord = [-1, -1]
for row in range(len(room)):
for col in range(len(room[row])):
if room[row][col]==1:
tableCoord[0] = row
tableCoord[1] = col
if tableCoord == [-1, -1]:
return "NoTable"
if cat == tableCoord:
return ""
move = [0, 0]
move[0] = tableCoord[0] - cat[0]
move[1] = tableCoord[1] - cat[1]
retStr = ""
while move != [0, 0]:
if move[0] > 0:
retStr += "D"
move[0] -= 1
if move[0] < 0:
retStr += "U"
move[0] += 1
if move[1] > 0:
retStr += "R"
move[1] -= 1
if move[1] < 0:
retStr += "L"
move[1] += 1
return retStr | def put_the_cat_on_the_table(cat, room):
if cat[0] < 0 or cat[0] >= len(room) or cat[1] < 0 or cat[1] >= len(room[0]):
return "NoCat"
tableCoord = [-1, -1]
for row in range(len(room)):
for col in range(len(room[row])):
if room[row][col]==1:
tableCoord[0] = row
tableCoord[1] = col
if tableCoord == [-1, -1]:
return "NoTable"
if cat == tableCoord:
return ""
move = [0, 0]
move[0] = tableCoord[0] - cat[0]
move[1] = tableCoord[1] - cat[1]
retStr = ""
while move != [0, 0]:
if move[0] > 0:
retStr += "D"
move[0] -= 1
if move[0] < 0:
retStr += "U"
move[0] += 1
if move[1] > 0:
retStr += "R"
move[1] -= 1
if move[1] < 0:
retStr += "L"
move[1] += 1
return retStr | train | APPS_structured |
You will be given m strings. For each of those strings, you need to count the total number of appearances of that string as substrings in all possible strings of length n containing only lower case English letters.
A string may appear in a string multiple times. Also, these appearances may overlap. All these must be counted separately. For example, aa appears thrice in the string aaacaa: aaacaa, aaacaa and aaacaa.
-----Input-----
- The first line contains one integer, T, the number of test cases. The description of each test case follows:
- The first line of each test case will contain two integers n and m.
- The ith of the next m lines will have one string in each line. All the strings will consist only of lower case English letters.
-----Output-----
- For each test case, print "Case x:" (without quotes. x is the test case number, 1-indexed) in the first line.
- Then print m lines. The ith line should contain the number of appearances of the ith string in all possible strings of length n. As the numbers can be very large, print the answers modulo 109+7.
-----Constraints-----
- 1 ≤ T ≤ 100
- 1 ≤ n ≤ 100000
- 1 ≤ m ≤ 1000
- 1 ≤ Length of every string in input
- 1 ≤ Total length of all strings in one test case ≤ 5 * 105
- 1 ≤ Total length of all strings in one test file ≤ 5 * 106
-----Example-----
Input:
3
2 1
aa
2 1
d
12 3
cdmn
qweewef
qs
Output:
Case 1:
1
Case 2:
52
Case 3:
443568031
71288256
41317270
-----Explanation:-----
Testcase 1: aa is the only string of length 2 which contains aa as a substring. And it occurs only once. Hence the answer is 1. | t=int(input())
def power(x, y) :
res = 1 # Initialize result
# Update x if it is more
# than or equal to p
x = x % mod
while (y > 0) :
# If y is odd, multiply
# x with result
if ((y & 1) == 1) :
res = (res * x) % mod
# y must be even now
y = y >> 1 # y = y/2
x = (x * x) % mod
return res
# Driver Code
for tc in range(t):
n,m1=input().split()
n,m1=int(n),int(m1)
print("Case "+str(tc+1)+":")
for i in range(m1):
s=input()
k=len(s)
mod=10**9+7
m=n-k
if k>n:
print(0)
else:
#print((n-k+1)*(26**(n-k)))%(10**9+7)
print(((m+1)*power(26, m))%mod)
| t=int(input())
def power(x, y) :
res = 1 # Initialize result
# Update x if it is more
# than or equal to p
x = x % mod
while (y > 0) :
# If y is odd, multiply
# x with result
if ((y & 1) == 1) :
res = (res * x) % mod
# y must be even now
y = y >> 1 # y = y/2
x = (x * x) % mod
return res
# Driver Code
for tc in range(t):
n,m1=input().split()
n,m1=int(n),int(m1)
print("Case "+str(tc+1)+":")
for i in range(m1):
s=input()
k=len(s)
mod=10**9+7
m=n-k
if k>n:
print(0)
else:
#print((n-k+1)*(26**(n-k)))%(10**9+7)
print(((m+1)*power(26, m))%mod)
| train | APPS_structured |
The internet is a very confounding place for some adults. Tom has just joined an online forum and is trying to fit in with all the teens and tweens. It seems like they're speaking in another language! Help Tom fit in by translating his well-formatted English into n00b language.
The following rules should be observed:
- "to" and "too" should be replaced by the number 2, even if they are only part of a word (E.g. today = 2day)
- Likewise, "for" and "fore" should be replaced by the number 4
- Any remaining double o's should be replaced with zeros (E.g. noob = n00b)
- "be", "are", "you", "please", "people", "really", "have", and "know" should be changed to "b", "r", "u", "plz", "ppl", "rly", "haz", and "no" respectively (even if they are only part of the word)
- When replacing words, always maintain case of the first letter unless another rule forces the word to all caps.
- The letter "s" should always be replaced by a "z", maintaining case
- "LOL" must be added to the beginning of any input string starting with a "w" or "W"
- "OMG" must be added to the beginning (after LOL, if applicable,) of a string 32 characters^(1) or longer
- All evenly numbered words^(2) must be in ALL CAPS (Example: ```Cake is very delicious.``` becomes ```Cake IZ very DELICIOUZ```)
- If the input string starts with "h" or "H", the entire output string should be in ALL CAPS
- Periods ( . ), commas ( , ), and apostrophes ( ' ) are to be removed
- ^(3)A question mark ( ? ) should have more question marks added to it, equal to the number of words^(2) in the sentence (Example: ```Are you a foo?``` has 4 words, so it would be converted to ```r U a F00????```)
- ^(3)Similarly, exclamation points ( ! ) should be replaced by a series of alternating exclamation points and the number 1, equal to the number of words^(2) in the sentence (Example: ```You are a foo!``` becomes ```u R a F00!1!1```)
^(1) Characters should be counted After: any word conversions, adding additional words, and removing punctuation. Excluding: All punctuation and any 1's added after exclamation marks ( ! ). Character count includes spaces.
^(2) For the sake of this kata, "words" are simply a space-delimited substring, regardless of its characters. Since the output may have a different number of words than the input, words should be counted based on the output string.
Example: ```whoa, you are my 123 <3``` becomes ```LOL WHOA u R my 123 <3``` = 7 words
^(3)The incoming string will be punctuated properly, so punctuation does not need to be validated. | def remove_punc(words):
if "'" in words:
temp_2 = words.partition("'")
words = temp_2[0] + temp_2[-1]
words = remove_punc(words)
if "." in words:
temp_2 = words.partition(".")
words = temp_2[0] + temp_2[-1]
words = remove_punc(words)
if "," in words:
temp_2 = words.partition(",")
words = temp_2[0] + temp_2[-1]
words = remove_punc(words)
return words
def transw(words, old, new):
temp_words = words.lower().replace(old, " "*len(old))
result = ""
threshold = 0
for i in range(0, len(temp_words)):
if temp_words[i] != " ":
result = result + words[i]
else:
threshold += 1
if threshold == len(old):
result = result + new
threshold = 0
return result
def n00bify(text):
temp = text.split()
result = ""
for i in temp:
i = transw(i, 'too', '2')
i = transw(i, 'to', '2')
i = transw(i, 'fore','4')
i = transw(i, 'for', '4')
i = transw(i, 'oo', '00')
i = transw(i, 'be', 'b')
i = transw(i, 'you', 'u')
i = transw(i, 'are', 'r')
i = transw(i, 'please', 'plz')
i = transw(i, 'people', 'ppl')
i = transw(i, 'really', 'rly')
i = transw(i, 'have', 'haz')
i = transw(i, 'know', 'no')
i = transw(i, 's', 'z')
i = remove_punc(i)
if len(result) == 0:
result = result + i
else:
result = result + " " + i
if result[0] == "w" or result[0] == "W":
result = "LOL" + " " + result
if result[0] == "h" or result[0] == "H":
result = result.upper()
t_result = result.split()
count = 0
for m in t_result:
for n in m:
if n == "!":
break
elif n.isalnum():
count += 1
count = count + len(t_result) - 1
if count >= 32:
if t_result[0] == "LOL":
t_result = ["OMG"] + t_result
t_result[0] = "LOL"
t_result[1] = "OMG"
else:
t_result = ["OMG"] + t_result
for k in range(0, len(t_result)):
if "?" in t_result[k]:
t_result[k] = t_result[k][:t_result[k].index("?")] + "?"*(len(t_result)-1) + t_result[k][t_result[k].index("?"):]
if "!" in t_result[k]:
add_in = ""
for l in range(0, len(t_result)):
if l % 2 == 0:
add_in = add_in + "!"
else:
add_in = add_in + "1"
t_result[k] = t_result[k][:t_result[k].index("!")] + add_in + t_result[k][t_result[k].index("!")+1:]
for i in range(0, len(t_result)):
if i % 2 != 0:
t_result[i] = t_result[i].upper()
return " ".join(t_result) | def remove_punc(words):
if "'" in words:
temp_2 = words.partition("'")
words = temp_2[0] + temp_2[-1]
words = remove_punc(words)
if "." in words:
temp_2 = words.partition(".")
words = temp_2[0] + temp_2[-1]
words = remove_punc(words)
if "," in words:
temp_2 = words.partition(",")
words = temp_2[0] + temp_2[-1]
words = remove_punc(words)
return words
def transw(words, old, new):
temp_words = words.lower().replace(old, " "*len(old))
result = ""
threshold = 0
for i in range(0, len(temp_words)):
if temp_words[i] != " ":
result = result + words[i]
else:
threshold += 1
if threshold == len(old):
result = result + new
threshold = 0
return result
def n00bify(text):
temp = text.split()
result = ""
for i in temp:
i = transw(i, 'too', '2')
i = transw(i, 'to', '2')
i = transw(i, 'fore','4')
i = transw(i, 'for', '4')
i = transw(i, 'oo', '00')
i = transw(i, 'be', 'b')
i = transw(i, 'you', 'u')
i = transw(i, 'are', 'r')
i = transw(i, 'please', 'plz')
i = transw(i, 'people', 'ppl')
i = transw(i, 'really', 'rly')
i = transw(i, 'have', 'haz')
i = transw(i, 'know', 'no')
i = transw(i, 's', 'z')
i = remove_punc(i)
if len(result) == 0:
result = result + i
else:
result = result + " " + i
if result[0] == "w" or result[0] == "W":
result = "LOL" + " " + result
if result[0] == "h" or result[0] == "H":
result = result.upper()
t_result = result.split()
count = 0
for m in t_result:
for n in m:
if n == "!":
break
elif n.isalnum():
count += 1
count = count + len(t_result) - 1
if count >= 32:
if t_result[0] == "LOL":
t_result = ["OMG"] + t_result
t_result[0] = "LOL"
t_result[1] = "OMG"
else:
t_result = ["OMG"] + t_result
for k in range(0, len(t_result)):
if "?" in t_result[k]:
t_result[k] = t_result[k][:t_result[k].index("?")] + "?"*(len(t_result)-1) + t_result[k][t_result[k].index("?"):]
if "!" in t_result[k]:
add_in = ""
for l in range(0, len(t_result)):
if l % 2 == 0:
add_in = add_in + "!"
else:
add_in = add_in + "1"
t_result[k] = t_result[k][:t_result[k].index("!")] + add_in + t_result[k][t_result[k].index("!")+1:]
for i in range(0, len(t_result)):
if i % 2 != 0:
t_result[i] = t_result[i].upper()
return " ".join(t_result) | train | APPS_structured |
The central dogma of molecular biology is that DNA is transcribed into RNA, which is then tranlsated into protein. RNA, like DNA, is a long strand of nucleic acids held together by a sugar backbone (ribose in this case). Each segment of three bases is called a codon. Molecular machines called ribosomes translate the RNA codons into amino acid chains, called polypeptides which are then folded into a protein.
Protein sequences are easily visualized in much the same way that DNA and RNA are, as large strings of letters. An important thing to note is that the 'Stop' codons do not encode for a specific amino acid. Their only function is to stop translation of the protein, as such they are not incorporated into the polypeptide chain. 'Stop' codons should not be in the final protein sequence. To save a you a lot of unnecessary (and boring) typing the keys and values for your amino acid dictionary are provided.
Given a string of RNA, create a funciton which translates the RNA into its protein sequence. Note: the test cases will always produce a valid string.
```python
protein('UGCGAUGAAUGGGCUCGCUCC') returns 'CDEWARS'
```
Included as test cases is a real world example! The last example test case encodes for a protein called green fluorescent protein; once spliced into the genome of another organism, proteins like GFP allow biologists to visualize cellular processes!
Amino Acid Dictionary
----------------------
```python
# Phenylalanine
'UUC':'F', 'UUU':'F',
# Leucine
'UUA':'L', 'UUG':'L', 'CUU':'L', 'CUC':'L','CUA':'L','CUG':'L',
# Isoleucine
'AUU':'I', 'AUC':'I', 'AUA':'I',
# Methionine
'AUG':'M',
# Valine
'GUU':'V', 'GUC':'V', 'GUA':'V', 'GUG':'V',
# Serine
'UCU':'S', 'UCC':'S', 'UCA':'S', 'UCG':'S', 'AGU':'S', 'AGC':'S',
# Proline
'CCU':'P', 'CCC':'P', 'CCA':'P', 'CCG':'P',
# Threonine
'ACU':'T', 'ACC':'T', 'ACA':'T', 'ACG':'T',
# Alanine
'GCU':'A', 'GCC':'A', 'GCA':'A', 'GCG':'A',
# Tyrosine
'UAU':'Y', 'UAC':'Y',
# Histidine
'CAU':'H', 'CAC':'H',
# Glutamine
'CAA':'Q', 'CAG':'Q',
# Asparagine
'AAU':'N', 'AAC':'N',
# Lysine
'AAA':'K', 'AAG':'K',
# Aspartic Acid
'GAU':'D', 'GAC':'D',
# Glutamic Acid
'GAA':'E', 'GAG':'E',
# Cystine
'UGU':'C', 'UGC':'C',
# Tryptophan
'UGG':'W',
# Arginine
'CGU':'R', 'CGC':'R', 'CGA':'R', 'CGG':'R', 'AGA':'R', 'AGG':'R',
# Glycine
'GGU':'G', 'GGC':'G', 'GGA':'G', 'GGG':'G',
# Stop codon
'UAA':'Stop', 'UGA':'Stop', 'UAG':'Stop'
``` | rnaDict = '''Phenylalanine (F): UUU, UUC
Leucine (L): UUA, UUG, CUU, CUC, CUA, CUG
Isoleucine (I): AUU, AUC, AUA
Methionine (M): AUG
Valine (V): GUU, GUC, GUA, GUG
Serine (S): UCU, UCC, UCA, UCG, AGU, AGC
Proline (P): CCU, CCC, CCA, CCG
Threonine (T): ACU, ACC, ACA, ACG
Alanine(A): GCU, GCC, GCA, GCG
Tyrosine (Y): UAU, UAC
Histidine (H): CAU, CAC
Glutamine (Q): CAA, CAG
Asparagine (N): AAU, AAC
Lysine (K): AAA, AAG
Aspartic Acid (D): GAU, GAC
Glutamic Acid (E): GAA, GAG
Cysteine (C): UGU, UGC
Tryptophan (W): UGG
Artinine (R): CGU, CGC, CGA, CGG, AGA, AGG
Glycine (G): GGU, GGC, GGA, GGG
Stop Codon ('Stop'): UGA, UAA, UAG'''
import re
def protein(rna):
transDict = {}
for line in rnaDict.split('\n'):
for section in line[line.index(':')+1:].replace(' ','').split(','):
transDict[section] = re.findall(r'\(+\'?(\w+)',line)[0]
codec = ''
while len(rna) > 0:
if transDict[rna[:3]] == 'Stop':
pass
else:
codec += transDict[rna[:3]]
rna = rna[3:]
return codec | rnaDict = '''Phenylalanine (F): UUU, UUC
Leucine (L): UUA, UUG, CUU, CUC, CUA, CUG
Isoleucine (I): AUU, AUC, AUA
Methionine (M): AUG
Valine (V): GUU, GUC, GUA, GUG
Serine (S): UCU, UCC, UCA, UCG, AGU, AGC
Proline (P): CCU, CCC, CCA, CCG
Threonine (T): ACU, ACC, ACA, ACG
Alanine(A): GCU, GCC, GCA, GCG
Tyrosine (Y): UAU, UAC
Histidine (H): CAU, CAC
Glutamine (Q): CAA, CAG
Asparagine (N): AAU, AAC
Lysine (K): AAA, AAG
Aspartic Acid (D): GAU, GAC
Glutamic Acid (E): GAA, GAG
Cysteine (C): UGU, UGC
Tryptophan (W): UGG
Artinine (R): CGU, CGC, CGA, CGG, AGA, AGG
Glycine (G): GGU, GGC, GGA, GGG
Stop Codon ('Stop'): UGA, UAA, UAG'''
import re
def protein(rna):
transDict = {}
for line in rnaDict.split('\n'):
for section in line[line.index(':')+1:].replace(' ','').split(','):
transDict[section] = re.findall(r'\(+\'?(\w+)',line)[0]
codec = ''
while len(rna) > 0:
if transDict[rna[:3]] == 'Stop':
pass
else:
codec += transDict[rna[:3]]
rna = rna[3:]
return codec | train | APPS_structured |
You are given an input string.
For each symbol in the string if it's the first character occurrence, replace it with a '1', else replace it with the amount of times you've already seen it...
But will your code be **performant enough**?
___
## Examples:
```
input = "Hello, World!"
result = "1112111121311"
input = "aaaaaaaaaaaa"
result = "123456789101112"
```
There might be some non-ascii characters in the string.
~~~if:java
Note: there will be no int domain overflow (character occurrences will be less than 2 billion).
~~~
~~~if:c
(this does not apply to the C language translation)
~~~ | def numericals(s):
history = {}
result = []
for char in s:
if char not in history:
history[char] = 1
else:
history[char] += 1
result.append(history[char])
return "".join(map(str, result))
| def numericals(s):
history = {}
result = []
for char in s:
if char not in history:
history[char] = 1
else:
history[char] += 1
result.append(history[char])
return "".join(map(str, result))
| train | APPS_structured |
The goal of this kata is to implement [trie](https://en.wikipedia.org/wiki/Trie) (or prefix tree) using dictionaries (aka hash maps or hash tables), where:
1. the dictionary keys are the prefixes
2. the value of a leaf node is `None` in Python, `nil` in Ruby and `null` in Groovy, JavaScript and Java.
3. the value for empty input is `{}` in Python, Ruby, Javascript and Java (empty map) and `[:]` in Groovy.
**Examples:**
```python
>>> build_trie()
{}
>>> build_trie("")
{}
>>> build_trie("trie")
{'t': {'tr': {'tri': {'trie': None}}}}
>>> build_trie("tree")
{'t': {'tr': {'tre': {'tree': None}}}}
>>> build_trie("A","to", "tea", "ted", "ten", "i", "in", "inn")
{'A': None, 't': {'to': None, 'te': {'tea': None, 'ted': None, 'ten': None}}, 'i': {'in': {'inn': None}}}
>>> build_trie("true", "trust")
{'t': {'tr': {'tru': {'true': None, 'trus': {'trust': None}}}}}
```
Happy coding! :) |
def build_trie(*words):
trie={}
k=''
def recurse(trie,wd,k):
for l in wd:
k=k+l
if k in trie:
if trie[k]==None and len(wd)>1:
trie[k]={}
recurse(trie[k],wd[1:],k)
else:
trie[k]={} if len(wd)>1 else None
recurse(trie[k],wd[1:],k)
return trie
[recurse(trie,word,'') for word in words]
return trie
|
def build_trie(*words):
trie={}
k=''
def recurse(trie,wd,k):
for l in wd:
k=k+l
if k in trie:
if trie[k]==None and len(wd)>1:
trie[k]={}
recurse(trie[k],wd[1:],k)
else:
trie[k]={} if len(wd)>1 else None
recurse(trie[k],wd[1:],k)
return trie
[recurse(trie,word,'') for word in words]
return trie
| train | APPS_structured |
Find the sum of the odd numbers within an array, after cubing the initial integers. The function should return `undefined`/`None`/`nil`/`NULL` if any of the values aren't numbers.
~~~if:java,csharp
Note: There are ONLY integers in the JAVA and C# versions of this Kata.
~~~
~~~if:python
Note: Booleans should not be considered as numbers.
~~~ | def cube_odd(arr):
return sum(x ** 3 for x in arr if x % 2) if all(isinstance(x, int) and not isinstance(x, bool) for x in arr) else None
| def cube_odd(arr):
return sum(x ** 3 for x in arr if x % 2) if all(isinstance(x, int) and not isinstance(x, bool) for x in arr) else None
| train | APPS_structured |
The chef is trying to decode some pattern problems, Chef wants your help to code it. Chef has one number K to form a new pattern. Help the chef to code this pattern problem.
-----Input:-----
- First-line will contain $T$, the number of test cases. Then the test cases follow.
- Each test case contains a single line of input, one integer $K$.
-----Output:-----
For each test case, output as the pattern.
-----Constraints-----
- $1 \leq T \leq 100$
- $1 \leq K \leq 100$
-----Sample Input:-----
4
1
2
3
4
-----Sample Output:-----
0
01
10
010
101
010
0101
1010
0101
1010
-----EXPLANATION:-----
No need, else pattern can be decode easily. | t = int(input())
for i in range(t):
k = int(input())
ini = [j % 2 for j in range(0, k)]
for j in range(0, k):
print(''.join([str((x + j) % 2) for x in ini])) | t = int(input())
for i in range(t):
k = int(input())
ini = [j % 2 for j in range(0, k)]
for j in range(0, k):
print(''.join([str((x + j) % 2) for x in ini])) | train | APPS_structured |
Design a Skiplist without using any built-in libraries.
A Skiplist is a data structure that takes O(log(n)) time to add, erase and search. Comparing with treap and red-black tree which has the same function and performance, the code length of Skiplist can be comparatively short and the idea behind Skiplists are just simple linked lists.
For example: we have a Skiplist containing [30,40,50,60,70,90] and we want to add 80 and 45 into it. The Skiplist works this way:
Artyom Kalinin [CC BY-SA 3.0], via Wikimedia Commons
You can see there are many layers in the Skiplist. Each layer is a sorted linked list. With the help of the top layers, add , erase and search can be faster than O(n). It can be proven that the average time complexity for each operation is O(log(n)) and space complexity is O(n).
To be specific, your design should include these functions:
bool search(int target) : Return whether the target exists in the Skiplist or not.
void add(int num): Insert a value into the SkipList.
bool erase(int num): Remove a value in the Skiplist. If num does not exist in the Skiplist, do nothing and return false. If there exists multiple num values, removing any one of them is fine.
See more about Skiplist : https://en.wikipedia.org/wiki/Skip_list
Note that duplicates may exist in the Skiplist, your code needs to handle this situation.
Example:
Skiplist skiplist = new Skiplist();
skiplist.add(1);
skiplist.add(2);
skiplist.add(3);
skiplist.search(0); // return false.
skiplist.add(4);
skiplist.search(1); // return true.
skiplist.erase(0); // return false, 0 is not in skiplist.
skiplist.erase(1); // return true.
skiplist.search(1); // return false, 1 has already been erased.
Constraints:
0 <= num, target <= 20000
At most 50000 calls will be made to search, add, and erase. | class Node(object):
def __init__(self, val):
self.val = val
self.cnt = 1
self.right = None
self.down = None
import random
class Skiplist:
def __init__(self):
self.root = Node(float('-inf'))
p = self.root
for _ in range(31):
p.down = Node(float('-inf'))
p = p.down
self.root.right = Node(float('inf'))
uplevel = self.root
clevel = self.root.down
while clevel:
clevel.right = Node(float('inf'))
uplevel.right.down = clevel.right
uplevel = clevel
clevel = clevel.down
def search(self, target: int) -> bool:
p = self.root
while p:
if p.val == target:
return p.cnt > 0
elif p.right.val <= target:
p = p.right
else:
p = p.down
return False
def add(self, num: int) -> None:
p = self.root
path = []
while p:
if p.val == num:
while p:
p.cnt += 1
p = p.down
return
elif p.right.val <= num:
p = p.right
else:
path.append(p)
p = p.down
node = Node(num)
node.right = path[-1].right
path[-1].right = node
path.pop(-1)
while path and random.random() < 0.5:
last_level = path.pop(-1)
new_node = Node(num)
new_node.right = last_level.right
last_level.right = new_node
new_node.down = node
node = new_node
def erase(self, target: int) -> bool:
p = self.root
while p:
if p.val == target:
if p.cnt > 0:
while p:
p.cnt -= 1
p = p.down
return True
else:
return False
elif p.right.val <= target:
p = p.right
else:
p = p.down
return False
# Your Skiplist object will be instantiated and called as such:
# obj = Skiplist()
# param_1 = obj.search(target)
# obj.add(num)
# param_3 = obj.erase(num)
| class Node(object):
def __init__(self, val):
self.val = val
self.cnt = 1
self.right = None
self.down = None
import random
class Skiplist:
def __init__(self):
self.root = Node(float('-inf'))
p = self.root
for _ in range(31):
p.down = Node(float('-inf'))
p = p.down
self.root.right = Node(float('inf'))
uplevel = self.root
clevel = self.root.down
while clevel:
clevel.right = Node(float('inf'))
uplevel.right.down = clevel.right
uplevel = clevel
clevel = clevel.down
def search(self, target: int) -> bool:
p = self.root
while p:
if p.val == target:
return p.cnt > 0
elif p.right.val <= target:
p = p.right
else:
p = p.down
return False
def add(self, num: int) -> None:
p = self.root
path = []
while p:
if p.val == num:
while p:
p.cnt += 1
p = p.down
return
elif p.right.val <= num:
p = p.right
else:
path.append(p)
p = p.down
node = Node(num)
node.right = path[-1].right
path[-1].right = node
path.pop(-1)
while path and random.random() < 0.5:
last_level = path.pop(-1)
new_node = Node(num)
new_node.right = last_level.right
last_level.right = new_node
new_node.down = node
node = new_node
def erase(self, target: int) -> bool:
p = self.root
while p:
if p.val == target:
if p.cnt > 0:
while p:
p.cnt -= 1
p = p.down
return True
else:
return False
elif p.right.val <= target:
p = p.right
else:
p = p.down
return False
# Your Skiplist object will be instantiated and called as such:
# obj = Skiplist()
# param_1 = obj.search(target)
# obj.add(num)
# param_3 = obj.erase(num)
| train | APPS_structured |
Ishank lives in a country in which there are N$N$ cities and N−1$N-1$ roads. All the cities are connected via these roads. Each city has been assigned a unique number from 1 to N$N$. The country can be assumed as a tree, with nodes representing the cities and edges representing the roads. The tree is rooted at 1.Every Time, when a traveler through a road, he will either gain some amount or has to pay some amount.
Abhineet is a traveler and wishes to travel to various cities in this country. There's a law in the country for travelers, according to which, when a traveler moves from the city A$A$ to city B$B$, where city A$A$ and B$B$ are connected by a road then the traveler is either paid or has to pay the amount of money equal to profit or loss respectively. When he moves from A$A$ to B$B$, he hires a special kind of vehicle which can reverse its direction at most once. Reversing the direction means earlier the vehicle is going towards the root, then away from the root or vice versa. Abhineet is analyzing his trip and therefore gave Q$Q$ queries to his friend, Ishank, a great coder. In every query, he gives two cities A$A$ and B$B$. Ishank has to calculate the maximum amount he can gain (if he cannot gain, then the minimum amount he will lose) if he goes from the city A$A$ to city B$B$.
-----Input:-----
-The first line of the input contains a two space-separated integers N and Q.
-The next N-1 line contains 3 space-separated integers Xi and Yi and Zi denoting that cities Xi and Yi are connected by a road which gives profit Zi (Negative Zi represents loss).
-The next Q contains 2 space-separated integers A and B denoting two cities.
-----Output:-----
Print a single line corresponding to each query — the maximum amount he can gain (if he cannot gain, then the minimum amount he will lose with negative sign) if he goes from city A to city B.
-----Constraints-----
- 2≤N≤105$2 \leq N \leq 10^5$
- 1≤Q≤105$1 \leq Q \leq 10^5$
- 1≤Xi,Yi,A,B≤N$1 \leq Xi, Yi, A, B \leq N$
- abs(Zi)≤109$ abs(Zi) \leq 10^9$
-----Sample Input:-----
9 5
1 2 8
1 3 -9
2 4 1
2 5 -6
3 6 7
3 7 6
6 8 3
6 9 4
1 2
2 7
4 3
3 2
8 9
-----Sample Output:-----
10
5
0
-1
21
-----EXPLANATION:-----
In the first query, he goes from 1 to 2, 2 to 4, takes a turn and go to 2. Therefore profit=8+1+1=10. | # cook your dish here
try:
X=list(map(int, input().split()))
except:
X=[0,0]
ch=[]
chnew=[]
par={}
par[1]=0
for i in range(X[0]+1):
ch.append([])
chnew.append([])
for i in range(X[0]-1):
Y=list(map(int, input().split()))
#par[Y[1]]=[Y[0],Y[2]]
ch[Y[0]].append([Y[1],Y[2]])
ch[Y[1]].append([Y[0],Y[2]])
tre=[1]
while(len(tre)):
cr=tre[-1]
tre=tre[:-1]
for i in ch[cr]:
chnew[cr].append(i)
par[i[0]]=[cr,i[1]]
tre.append(i[0])
for j in ch[i[0]]:
if(j[0]==cr):
ch[i[0]].remove(j)
break
ch=chnew
def goup(par,nd):
if(nd==1):
return 0
else:
p=par[nd]
ans=p[1]+goup(par,p[0])
return (max([ans,0]))
def godown(ch,nd):
ans=0
for i in ch[nd]:
ans=max([(i[1]+godown(ch,i[0])),ans])
return(ans)
for i in range(X[1]):
Z=list(map(int,input().split()))
r=Z[0]
s=Z[1]
nans=0
while(r!=s):
if(r>s):
nans=nans+par[r][1]
r=par[r][0]
else:
nans=nans+par[s][1]
s=par[s][0]
if((r==Z[0]) or (r==Z[1])):
if(Z[0]<Z[1]):
nans=nans+2*max(goup(par,Z[0]),godown(ch,Z[1]))
else:
nans=nans+2*max(goup(par,Z[1]),godown(ch,Z[0]))
else:
nans=nans+2*goup(par,r)
print(nans) | # cook your dish here
try:
X=list(map(int, input().split()))
except:
X=[0,0]
ch=[]
chnew=[]
par={}
par[1]=0
for i in range(X[0]+1):
ch.append([])
chnew.append([])
for i in range(X[0]-1):
Y=list(map(int, input().split()))
#par[Y[1]]=[Y[0],Y[2]]
ch[Y[0]].append([Y[1],Y[2]])
ch[Y[1]].append([Y[0],Y[2]])
tre=[1]
while(len(tre)):
cr=tre[-1]
tre=tre[:-1]
for i in ch[cr]:
chnew[cr].append(i)
par[i[0]]=[cr,i[1]]
tre.append(i[0])
for j in ch[i[0]]:
if(j[0]==cr):
ch[i[0]].remove(j)
break
ch=chnew
def goup(par,nd):
if(nd==1):
return 0
else:
p=par[nd]
ans=p[1]+goup(par,p[0])
return (max([ans,0]))
def godown(ch,nd):
ans=0
for i in ch[nd]:
ans=max([(i[1]+godown(ch,i[0])),ans])
return(ans)
for i in range(X[1]):
Z=list(map(int,input().split()))
r=Z[0]
s=Z[1]
nans=0
while(r!=s):
if(r>s):
nans=nans+par[r][1]
r=par[r][0]
else:
nans=nans+par[s][1]
s=par[s][0]
if((r==Z[0]) or (r==Z[1])):
if(Z[0]<Z[1]):
nans=nans+2*max(goup(par,Z[0]),godown(ch,Z[1]))
else:
nans=nans+2*max(goup(par,Z[1]),godown(ch,Z[0]))
else:
nans=nans+2*goup(par,r)
print(nans) | train | APPS_structured |