input
stringlengths 50
13.9k
| output_program
stringlengths 5
655k
| output_answer
stringlengths 5
655k
| split
stringclasses 1
value | dataset
stringclasses 1
value |
---|---|---|---|---|
The Jones Trucking Company tracks the location of each of its trucks on a grid similar to an (x, y) plane. The home office is at the location (0, 0). Read the coordinates of truck A and the coordinates of truck B and determine which is closer to the office.
-----Input:-----
The first line of the data set for this problem is an integer representing the number of collections of data that follow. Each collection contains 4 integers: the x-coordinate and then the
y-coordinate of truck A followed by the x-coordinate and then the y-coordinate of truck B.
-----Output:-----
All letters are upper case.
The output is to be formatted exactly like that for the sample output given below.
-----Assumptions:-----
The x-coordinate is in the range –20 .. 20. The y-coordinate is in the range –20 .. 20.
-----Discussion:-----
The distance between point #1 with coordinates (x1, y1) and point #2 with coordinates (x2, y2) is:
-----Sample Input:-----
4
3 -2 -5 -3
0 6 1 2
-7 8 4 -1
3 3 -2 2
-----Sample Output:-----
A IS CLOSER
B IS CLOSER
B IS CLOSER
B IS CLOSER | t = int(input())
for x in range(t):
a = [int(x) for x in input().split()]
d1 = (a[0]**2 + a[1]**2)**0.5
d2 = (a[2] ** 2 + a[2] ** 2) ** 0.5
if d1 < d2:
print("A IS CLOSER")
else:
print("B IS CLOSER") | t = int(input())
for x in range(t):
a = [int(x) for x in input().split()]
d1 = (a[0]**2 + a[1]**2)**0.5
d2 = (a[2] ** 2 + a[2] ** 2) ** 0.5
if d1 < d2:
print("A IS CLOSER")
else:
print("B IS CLOSER") | train | APPS_structured |
A [Word Square](https://en.wikipedia.org/wiki/Word_square) is a set of words written out in a square grid, such that the same words can be read both horizontally and vertically. The number of words, equal to the number of letters in each word, is known as the *order* of the square.
For example, this is an *order* `5` square found in the ruins of Herculaneum:
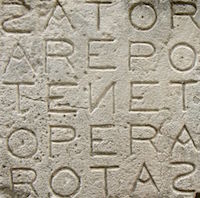
Given a string of various uppercase `letters`, check whether a *Word Square* can be formed from it.
Note that you should use each letter from `letters` the exact number of times it occurs in the string. If a *Word Square* can be formed, return `true`, otherwise return `false`.
__Example__
* For `letters = "SATORAREPOTENETOPERAROTAS"`, the output should be
`WordSquare(letters) = true`.
It is possible to form a *word square* in the example above.
* For `letters = "AAAAEEEENOOOOPPRRRRSSTTTT"`, (which is sorted form of `"SATORAREPOTENETOPERAROTAS"`), the output should also be
`WordSquare(letters) = true`.
* For `letters = "NOTSQUARE"`, the output should be
`WordSquare(letters) = false`.
__Input/Output__
* [input] string letters
A string of uppercase English letters.
Constraints: `3 ≤ letters.length ≤ 100`.
* [output] boolean
`true`, if a Word Square can be formed;
`false`, if a Word Square cannot be formed. | from collections import Counter
def word_square(ls):
n = int(len(ls)**0.5)
return n*n==len(ls) and sum(i%2 for i in list(Counter(ls).values())) <= n
| from collections import Counter
def word_square(ls):
n = int(len(ls)**0.5)
return n*n==len(ls) and sum(i%2 for i in list(Counter(ls).values())) <= n
| train | APPS_structured |
You are given two positive integers ```a``` and ```b```.
You can perform the following operations on ```a``` so as to obtain ```b``` :
```
(a-1)/2 (if (a-1) is divisible by 2)
a/2 (if a is divisible by 2)
a*2
```
```b``` will always be a power of 2.
You are to write a function ```operation(a,b)``` that efficiently returns the minimum number of operations required to transform ```a``` into ```b```.
For example :
```
operation(2,8) -> 2
2*2 = 4
4*2 = 8
operation(9,2) -> 2
(9-1)/2 = 4
4/2 = 2
operation(1024,1024) -> 0
``` | from math import log2
def operation(a, b):
if a in (0, b): return 0
c = 0
while a != b:
if a % 2 and a > 1: a = (a - 1) // 2
elif a < b and log2(a).is_integer(): a *= 2
elif a > b or not log2(a).is_integer(): a //= 2
c += 1
return c | from math import log2
def operation(a, b):
if a in (0, b): return 0
c = 0
while a != b:
if a % 2 and a > 1: a = (a - 1) // 2
elif a < b and log2(a).is_integer(): a *= 2
elif a > b or not log2(a).is_integer(): a //= 2
c += 1
return c | train | APPS_structured |
This is the simple version of [Fastest Code : Equal to 24](http://www.codewars.com/kata/574e890e296e412a0400149c).
## Task
A game I played when I was young: Draw 4 cards from playing cards, use ```+ - * / and ()``` to make the final results equal to 24.
You will coding in function ```equalTo24```. Function accept 4 parameters ```a b c d```(4 cards), value range is 1-13.
The result is a string such as ```"2*2*2*3"``` ,```(4+2)*(5-1)```; If it is not possible to calculate the 24, please return "It's not possible!"
All four cards are to be used, only use three or two cards are incorrect; Use a card twice or more is incorrect too.
You just need to return one correct solution, don't need to find out all the possibilities.
## Examples | class Calculator(object):
def evaluate(self, in_string):
what = str()
lst = []
count_par = 0;
index = 0
while(index < len(in_string)):
if in_string[index] == '(':
count_par +=1
flag_par = True
elif in_string[index] == ')':
count_par -=1
elif count_par == 0:
if in_string[index] in ['+', '-']:
break
elif in_string[index] in ['*', '/']:
if in_string[index+1] == '(':
break
elif in_string[index+1:].count('+') == 0 and in_string[index+1:].count('-')==0:
break
index += 1
if index == len(in_string):
lst.append(in_string)
else:
temp1 = in_string[0:index]
temp2 = in_string[index+1:]
what = in_string[index]
if temp1[0] == '(':
temp1 = temp1[1:-1]
if temp2[0] == '(':
temp2 = temp2[1:-1]
if what == '-' and in_string[index+1] != '(':
temp2 = temp2.replace('-','x')
temp2 = temp2.replace('+','-')
temp2 = temp2.replace('x','+')
lst.append(temp1)
lst.append(temp2)
if len(lst) == 1:
return float(lst[0])
else:
L = self.evaluate(lst[0])
R = self.evaluate(lst[1])
if what == '+':
return L + R
elif what == '-':
return L - R
elif what == '*':
return L*R
elif what == '/':
return L/R if R!=0 else 0
def index_numbers(a, b, c, d):
lst = [a,b,c,d]
for i in lst:
lst1 = lst[:]
lst1.remove(i)
for j in lst1:
lst2 = lst1[:]
lst2.remove(j)
for k in lst2:
lst3 = lst2[:]
lst3.remove(k)
for l in lst3:
yield [i,j,k,l]
def index_symbols():
lst = ['+', '-', '*', '/']
for i in lst:
for j in lst:
for k in lst:
yield [i,j,k]
CAL = Calculator()
def evaluate0(num, sym):
expr = num[0] + sym[0] + num[1] + sym[1] + num[2] + sym[2] + num[3]
#print(expr)
return CAL.evaluate(expr), expr
def evaluate1(num, sym):
expr = '(' + num[0] + sym[0] + num[1] + sym[1] + num[2] + ')' + sym[2] + num[3]
return CAL.evaluate(expr), expr
def evaluate2(num, sym):
expr = '(' + num[0] + sym[0] + num[1] + ')' + sym[1] + '(' + num[2] + sym[2] + num[3] + ')'
return CAL.evaluate(expr), expr
def evaluate3(num, sym):
expr = num[0] + sym[0] + '(' + num[1] + sym[1] + '(' + num[2] + sym[2] + num[3] + "))"
return CAL.evaluate(expr), expr
def evaluate4(num, sym):
expr = "((" +num[0] + sym[0] + num[1] + ')' + sym[1] + num[2] + ')' + sym[2] + num[3]
return CAL.evaluate(expr), expr
def equal_to_24(a,b,c,d):
for num in index_numbers(str(a), str(b), str(c), str(d)):
for sym in index_symbols():
val, text = evaluate0(num, sym)
if val == 24:
return text
val, text = evaluate1(num, sym)
if val == 24:
return text
val, text = evaluate2(num, sym)
if val == 24:
return text
val, text = evaluate3(num, sym)
if val == 24:
return text
val, text = evaluate4(num, sym)
if val == 24:
return text
return "It's not possible!"
| class Calculator(object):
def evaluate(self, in_string):
what = str()
lst = []
count_par = 0;
index = 0
while(index < len(in_string)):
if in_string[index] == '(':
count_par +=1
flag_par = True
elif in_string[index] == ')':
count_par -=1
elif count_par == 0:
if in_string[index] in ['+', '-']:
break
elif in_string[index] in ['*', '/']:
if in_string[index+1] == '(':
break
elif in_string[index+1:].count('+') == 0 and in_string[index+1:].count('-')==0:
break
index += 1
if index == len(in_string):
lst.append(in_string)
else:
temp1 = in_string[0:index]
temp2 = in_string[index+1:]
what = in_string[index]
if temp1[0] == '(':
temp1 = temp1[1:-1]
if temp2[0] == '(':
temp2 = temp2[1:-1]
if what == '-' and in_string[index+1] != '(':
temp2 = temp2.replace('-','x')
temp2 = temp2.replace('+','-')
temp2 = temp2.replace('x','+')
lst.append(temp1)
lst.append(temp2)
if len(lst) == 1:
return float(lst[0])
else:
L = self.evaluate(lst[0])
R = self.evaluate(lst[1])
if what == '+':
return L + R
elif what == '-':
return L - R
elif what == '*':
return L*R
elif what == '/':
return L/R if R!=0 else 0
def index_numbers(a, b, c, d):
lst = [a,b,c,d]
for i in lst:
lst1 = lst[:]
lst1.remove(i)
for j in lst1:
lst2 = lst1[:]
lst2.remove(j)
for k in lst2:
lst3 = lst2[:]
lst3.remove(k)
for l in lst3:
yield [i,j,k,l]
def index_symbols():
lst = ['+', '-', '*', '/']
for i in lst:
for j in lst:
for k in lst:
yield [i,j,k]
CAL = Calculator()
def evaluate0(num, sym):
expr = num[0] + sym[0] + num[1] + sym[1] + num[2] + sym[2] + num[3]
#print(expr)
return CAL.evaluate(expr), expr
def evaluate1(num, sym):
expr = '(' + num[0] + sym[0] + num[1] + sym[1] + num[2] + ')' + sym[2] + num[3]
return CAL.evaluate(expr), expr
def evaluate2(num, sym):
expr = '(' + num[0] + sym[0] + num[1] + ')' + sym[1] + '(' + num[2] + sym[2] + num[3] + ')'
return CAL.evaluate(expr), expr
def evaluate3(num, sym):
expr = num[0] + sym[0] + '(' + num[1] + sym[1] + '(' + num[2] + sym[2] + num[3] + "))"
return CAL.evaluate(expr), expr
def evaluate4(num, sym):
expr = "((" +num[0] + sym[0] + num[1] + ')' + sym[1] + num[2] + ')' + sym[2] + num[3]
return CAL.evaluate(expr), expr
def equal_to_24(a,b,c,d):
for num in index_numbers(str(a), str(b), str(c), str(d)):
for sym in index_symbols():
val, text = evaluate0(num, sym)
if val == 24:
return text
val, text = evaluate1(num, sym)
if val == 24:
return text
val, text = evaluate2(num, sym)
if val == 24:
return text
val, text = evaluate3(num, sym)
if val == 24:
return text
val, text = evaluate4(num, sym)
if val == 24:
return text
return "It's not possible!"
| train | APPS_structured |
John Smith knows that his son, Thomas Smith, is among the best students in his class and even in his school. After the students of the school took the exams in English, German, Math, and History, a table of results was formed.
There are $n$ students, each of them has a unique id (from $1$ to $n$). Thomas's id is $1$. Every student has four scores correspond to his or her English, German, Math, and History scores. The students are given in order of increasing of their ids.
In the table, the students will be sorted by decreasing the sum of their scores. So, a student with the largest sum will get the first place. If two or more students have the same sum, these students will be sorted by increasing their ids.
Please help John find out the rank of his son.
-----Input-----
The first line contains a single integer $n$ ($1 \le n \le 1000$) — the number of students.
Each of the next $n$ lines contains four integers $a_i$, $b_i$, $c_i$, and $d_i$ ($0\leq a_i, b_i, c_i, d_i\leq 100$) — the grades of the $i$-th student on English, German, Math, and History. The id of the $i$-th student is equal to $i$.
-----Output-----
Print the rank of Thomas Smith. Thomas's id is $1$.
-----Examples-----
Input
5
100 98 100 100
100 100 100 100
100 100 99 99
90 99 90 100
100 98 60 99
Output
2
Input
6
100 80 90 99
60 60 60 60
90 60 100 60
60 100 60 80
100 100 0 100
0 0 0 0
Output
1
-----Note-----
In the first sample, the students got total scores: $398$, $400$, $398$, $379$, and $357$. Among the $5$ students, Thomas and the third student have the second highest score, but Thomas has a smaller id, so his rank is $2$.
In the second sample, the students got total scores: $369$, $240$, $310$, $300$, $300$, and $0$. Among the $6$ students, Thomas got the highest score, so his rank is $1$. | USE_STDIO = False
if not USE_STDIO:
try: import mypc
except: pass
def main():
n, = list(map(int, input().split(' ')))
scores = []
for id in range(n):
scores.append((-sum(map(int, input().split(' '))), id))
scores.sort()
for rank in range(n):
if scores[rank][1] == 0:
print(rank + 1)
return
def __starting_point():
main()
__starting_point() | USE_STDIO = False
if not USE_STDIO:
try: import mypc
except: pass
def main():
n, = list(map(int, input().split(' ')))
scores = []
for id in range(n):
scores.append((-sum(map(int, input().split(' '))), id))
scores.sort()
for rank in range(n):
if scores[rank][1] == 0:
print(rank + 1)
return
def __starting_point():
main()
__starting_point() | train | APPS_structured |
Create a function that takes any sentence and redistributes the spaces (and adds additional spaces if needed) so that each word starts with a vowel. The letters should all be in the same order but every vowel in the sentence should be the start of a new word. The first word in the new sentence may start without a vowel. It should return a string in all lowercase with no punctuation (only alphanumeric characters).
EXAMPLES
'It is beautiful weather today!' becomes 'it isb e a ut if ulw e ath ert od ay'
'Coding is great' becomes 'c od ing isgr e at'
'my number is 0208-533-2325' becomes 'myn umb er is02085332325' | from re import sub
def vowel_start(st):
return sub(r'(?<=.)([aeiou])', r' \1', sub(r'[^a-z0-9]', '', st.lower()))
| from re import sub
def vowel_start(st):
return sub(r'(?<=.)([aeiou])', r' \1', sub(r'[^a-z0-9]', '', st.lower()))
| train | APPS_structured |
In this Kata, two players, Alice and Bob, are playing a palindrome game. Alice starts with `string1`, Bob starts with `string2`, and the board starts out as an empty string. Alice and Bob take turns; during a turn, a player selects a letter from his or her string, removes it from the string, and appends it to the board; if the board becomes a palindrome (of length >= 2), the player wins. Alice makes the first move. Since Bob has the disadvantage of playing second, then he wins automatically if letters run out or the board is never a palindrome. Note also that each player can see the other player's letters.
The problem will be presented as `solve(string1,string2)`. Return 1 if Alice wins and 2 it Bob wins.
For example:
```Haskell
solve("abc","baxy") = 2 -- There is no way for Alice to win. If she starts with 'a', Bob wins by playing 'a'. The same case with 'b'. If Alice starts with 'c', Bob still wins because a palindrome is not possible. Return 2.
solve("eyfjy","ooigvo") = 1 -- Alice plays 'y' and whatever Bob plays, Alice wins by playing another 'y'. Return 1.
solve("abc","xyz") = 2 -- No palindrome is possible, so Bob wins; return 2
solve("gzyqsczkctutjves","hpaqrfwkdntfwnvgs") = 1 -- If Alice plays 'g', Bob wins by playing 'g'. Alice must be clever. She starts with 'z'. She knows that since she has two 'z', the win is guaranteed. Note that she also has two 's'. But she cannot play that. Can you see why?
solve("rmevmtw","uavtyft") = 1 -- Alice wins by playing 'm'. Can you see why?
```
Palindrome lengths should be at least `2` characters. More examples in the test cases.
Good luck! | solve=lambda Q,S:1 if any(V in Q[1+F:] and V not in S for F,V in enumerate(Q)) else 2 | solve=lambda Q,S:1 if any(V in Q[1+F:] and V not in S for F,V in enumerate(Q)) else 2 | train | APPS_structured |
# Leaderboard climbers
In this kata you will be given a leaderboard of unique names for example:
```python
['John',
'Brian',
'Jim',
'Dave',
'Fred']
```
Then you will be given a list of strings for example:
```python
['Dave +1', 'Fred +4', 'Brian -1']
```
Then you sort the leaderboard.
The steps for our example would be:
```python
# Dave up 1
['John',
'Brian',
'Dave',
'Jim',
'Fred']
```
```python
# Fred up 4
['Fred',
'John',
'Brian',
'Dave',
'Jim']
```
```python
# Brian down 1
['Fred',
'John',
'Dave',
'Brian',
'Jim']
```
Then once you have done this you need to return the leaderboard.
All inputs will be valid. All strings in the second list will never ask to move a name up higher or lower than possible eg. `"John +3"` could not be added to the end of the second input list in the example above.
The strings in the second list will always be something in the leaderboard followed by a space and a `+` or `-` sign followed by a number. | def leaderboard_sort(leaderboard, changes):
for change in changes:
name, offset = change.split(' ')
pos = leaderboard.index(name)
del leaderboard[pos]
leaderboard.insert(pos - int(offset), name)
return leaderboard
| def leaderboard_sort(leaderboard, changes):
for change in changes:
name, offset = change.split(' ')
pos = leaderboard.index(name)
del leaderboard[pos]
leaderboard.insert(pos - int(offset), name)
return leaderboard
| train | APPS_structured |
Zombies zombies everywhere!!
In a parallel world of zombies, there are N zombies. There are infinite number of unused cars, each of same model only differentiated by the their colors. The cars are of K colors.
A zombie parent can give birth to any number of zombie-children (possibly zero), i.e. each zombie will have its parent except the head zombie which was born in the winters by combination of ice and fire.
Now, zombies are having great difficulties to commute to their offices without cars, so they decided to use the cars available. Every zombie will need only one car. Head zombie called a meeting regarding this, in which he will allow each zombie to select a car for him.
Out of all the cars, the head zombie chose one of cars for him. Now, he called his children to choose the cars for them. After that they called their children and so on till each of the zombie had a car. Head zombie knew that it won't be a good idea to allow children to have cars of same color as that of parent, as they might mistakenly use that. So, he enforced this rule during the selection of cars.
Professor James Moriarty is a criminal mastermind and has trapped Watson again in the zombie world. Sherlock somehow manages to go there and met the head zombie. Head zombie told Sherlock that they will let Watson free if and only if Sherlock manages to tell him the maximum number of ways in which the cars can be selected by N Zombies among all possible hierarchies. A hierarchy represents parent-child relationships among the N zombies. Since the answer may be large, output the answer modulo 109 + 7. Sherlock can not compute big numbers, so he confides you to solve this for him.
-----Input-----
The first line consists of a single integer T, the number of test-cases.
Each test case consists of two space-separated integers N and K, denoting number of zombies and the possible number of colors of the cars respectively.
-----Output-----
For each test-case, output a single line denoting the answer of the problem.
-----Constraints-----
- 1 ≤ T ≤ 100
- 1 ≤ N ≤ 10^9
- 1 ≤ K ≤ 10^9
-----Subtasks-----
Subtask #1 : (10 points)
- 1 ≤ T ≤ 20
- 1 ≤ N, K ≤ 10
Subtask 2 : (20 points)
- 1 ≤ T ≤ 10
- 1 ≤ N, K ≤ 10000
Subtask 3 : (70 points)
- 1 ≤ T ≤ 100
- 1 ≤ N, K ≤ 10^9
-----Example-----
Input
2
2 2
3 3
Output:
2
12
-----Explanation
In the first sample test case, there are 2 zombies. Let us name them Z1 and Z2. Let one hierarchy be one in which Z1 is parent of Z2. There are 2 colors, suppose red and blue. If Z1 takes red, then Z2 should take a blue. If Z1 takes blue, then Z2 should take red.
Note that one other possible hierarchy could be one in which Z2 is a parent of Z1. In that hierarchy also, number of possible ways of assigning cars is 2.
So there maximum number of possible ways is 2.
In the second example, we have 3 Zombies say Z1, Z2, Z3 and cars of 3 colors, suppose red, blue and green.
A hierarchy to maximize the number of possibilities is Z1 is the parent of Z2, Z2 is the parent of Z3.
Zombie Z1 can choose one of red, blue or green cars. Z2 can choose one of the remaining two colors (as its car's color can not be same as its parent car.). Z3 can also choose his car in two colors, (one of them could be color same as Z1, and other being the color which is not same as cars of both Z1 and Z2.). This way, there can be 12 different ways of selecting the cars.
-----
In the first sample test case, there are 2 zombies. Let us name them Z1 and Z2. Let one hierarchy be one in which Z1 is parent of Z2. There are 2 colors, suppose red and blue. If Z1 takes red, then Z2 should take a blue. If Z1 takes blue, then Z2 should take red.
Note that one other possible hierarchy could be one in which Z2 is a parent of Z1. In that hierarchy also, number of possible ways of assigning cars is 2.
So there maximum number of possible ways is 2.
In the second example, we have 3 Zombies say Z1, Z2, Z3 and cars of 3 colors, suppose red, blue and green.
A hierarchy to maximize the number of possibilities is Z1 is the parent of Z2, Z2 is the parent of Z3.
Zombie Z1 can choose one of red, blue or green cars. Z2 can choose one of the remaining two colors (as its car's color can not be same as its parent car.). Z3 can also choose his car in two colors, (one of them could be color same as Z1, and other being the color which is not same as cars of both Z1 and Z2.). This way, there can be 12 different ways of selecting the cars. | import math
MOD=1000000007
def fast_exp(base,exp):
res=1;
while(exp>0):
if(exp%2==1):
res=(res*base)%MOD
base=(base*base)%MOD
exp/=2;
return res
for t in range(int(input())):
pro=1
a,b=list(map(int,input().split()))
#for i in xrange(a):
pro*=b
pro*=fast_exp((b-1),(a-1))
print(pro%MOD)
| import math
MOD=1000000007
def fast_exp(base,exp):
res=1;
while(exp>0):
if(exp%2==1):
res=(res*base)%MOD
base=(base*base)%MOD
exp/=2;
return res
for t in range(int(input())):
pro=1
a,b=list(map(int,input().split()))
#for i in xrange(a):
pro*=b
pro*=fast_exp((b-1),(a-1))
print(pro%MOD)
| train | APPS_structured |
The objective is to write a method that takes two integer parameters and returns a single integer equal to the number of 1s in the binary representation of the greatest common divisor of the parameters.
Taken from Wikipedia:
"In mathematics, the greatest common divisor (gcd) of two or more integers, when at least one of them is not zero, is the largest positive integer that divides the numbers without a remainder. For example, the GCD of 8 and 12 is 4."
For example: the greatest common divisor of 300 and 45 is 15. The binary representation of 15 is 1111, so the correct output would be 4.
If both parameters are 0, the method should return 0.
The function must be able to handle negative input. | from fractions import gcd
def binary_gcd(x, y):
if not (x or y):
bin(max(x,y)).count('1')
return bin(gcd(x,y)).count('1')
| from fractions import gcd
def binary_gcd(x, y):
if not (x or y):
bin(max(x,y)).count('1')
return bin(gcd(x,y)).count('1')
| train | APPS_structured |
A number `n` is called `prime happy` if there is at least one prime less than `n` and the `sum of all primes less than n` is evenly divisible by `n`. Write `isPrimeHappy(n)` which returns `true` if `n` is `prime happy` else `false`. | is_prime_happy=lambda n:n>2and(2+sum(p*all(p%d for d in range(3,int(p**.5)+1,2))for p in range(3,n,2)))%n<1 | is_prime_happy=lambda n:n>2and(2+sum(p*all(p%d for d in range(3,int(p**.5)+1,2))for p in range(3,n,2)))%n<1 | train | APPS_structured |
For a string $S$ let the unique set of characters that occur in it one or more times be $C$. Consider a permutation of the elements of $C$ as $(c_1, c_2, c_3 ... )$. Let $f(c)$ be the number of times $c$ occurs in $S$.
If any such permutation of the elements of $C$ satisfies $f(c_i) = f(c_{i-1}) + f(c_{i-2})$ for all $i \ge 3$, the string is said to be a dynamic string.
Mr Bancroft is given the task to check if the string is dynamic, but he is busy playing with sandpaper. Would you help him in such a state?
Note that if the number of distinct characters in the string is less than 3, i.e. if $|C| < 3$, then the string is always dynamic.
-----Input:-----
- First line will contain $T$, number of testcases. Then the testcases follow.
- Each testcase contains of a single line of input, a string $S$.
-----Output:-----
For each testcase, output in a single line "Dynamic" if the given string is dynamic, otherwise print "Not". (Note that the judge is case sensitive)
-----Constraints-----
- $1 \leq T \leq 10$
- $1 \leq |S| \leq 10^5$
- $S$ contains only lower case alphabets: $a$, $b$, …, $z$
-----Sample Input:-----
3
aaaabccc
aabbcc
ppppmmnnoooopp
-----Sample Output:-----
Dynamic
Not
Dynamic
-----Explanation:-----
- Testase 1: For the given string, $C = \{a, b, c\}$ and $f(a)=4, f(b)=1, f(c)=3$. $f(a) = f(c) + f(b)$ so the permutation $(b, c, a)$ satisfies the requirement.
- Testcase 2: Here too $C = \{a, b, c\}$ but no permutation satisfies the requirement of a dynamic string.
- Testcase 3: Here $C = \{m, n, o, p\}$ and $(m, n, o, p)$ is a permutation that makes it a dynamic string. | # cook your dish here
from collections import Counter
def fib_str(s):
counts = Counter(s)
if len(counts) < 3:
return True
[x, y, z] = counts.most_common(3)
if x[1] == y[1] + z[1]:
return True
return False
def __starting_point():
test = int(input())
for _ in range(test):
s = input()
if fib_str(s):
print("Dynamic")
else:
print("Not")
__starting_point() | # cook your dish here
from collections import Counter
def fib_str(s):
counts = Counter(s)
if len(counts) < 3:
return True
[x, y, z] = counts.most_common(3)
if x[1] == y[1] + z[1]:
return True
return False
def __starting_point():
test = int(input())
for _ in range(test):
s = input()
if fib_str(s):
print("Dynamic")
else:
print("Not")
__starting_point() | train | APPS_structured |
The concept of "[smooth number](https://en.wikipedia.org/wiki/Smooth_number)" is applied to all those numbers whose prime factors are lesser than or equal to `7`: `60` is a smooth number (`2 * 2 * 3 * 5`), `111` is not (`3 * 37`).
More specifically, smooth numbers are classified by their highest prime factor and your are tasked with writing a `isSmooth`/`is_smooth` function that returns a string with this classification as it follows:
* 2-smooth numbers should be all defined as a `"power of 2"`, as they are merely that;
* 3-smooth numbers are to return a result of `"3-smooth"`;
* 5-smooth numbers will be labelled as `"Hamming number"`s (incidentally, you might appreciate [this nice kata on them](https://www.codewars.com/kata/hamming-numbers));
* 7-smooth numbers are classified as `"humble numbers"`s;
* for all the other numbers, just return `non-smooth`.
Examples:
```python
is_smooth(16) == "power of 2"
is_smooth(36) == "3-smooth"
is_smooth(60) == "Hamming number"
is_smooth(98) == "humble number"
is_smooth(111) == "non-smooth"
```
The provided input `n` is always going to be a positive number `> 1`. | def is_smooth(n):
i = 2
a = []
while i * i <= n:
if n % i:
i += 1
else:
n //= i
a.append(i)
if n > 1:
a.append(n)
m = max(a)
if m==2:
return "power of 2"
if m==3:
return "3-smooth"
if m == 5:
return "Hamming number"
if m == 7:
return "humble number"
else:
return "non-smooth"
| def is_smooth(n):
i = 2
a = []
while i * i <= n:
if n % i:
i += 1
else:
n //= i
a.append(i)
if n > 1:
a.append(n)
m = max(a)
if m==2:
return "power of 2"
if m==3:
return "3-smooth"
if m == 5:
return "Hamming number"
if m == 7:
return "humble number"
else:
return "non-smooth"
| train | APPS_structured |
A forest fire has been spotted at *fire*, a simple 2 element array with x, y coordinates.
The forest service has decided to send smoke jumpers in by plane and drop them in the forest.
The terrain is dangerous and surveyors have determined that there are three possible safe *dropzones*, an array of three simple arrays with x, y coordinates.
The plane is en route and time is of the essence. Your mission is to return a simple [x,y] array with the coordinates of the dropzone closest to the fire.
EDIT:
The airplane is leaving from the origin at 0,0. If your result returns two possible dropzones that are both an equal distance from the fire, choose the dropzone that is closest to 0,0.
If the two dropzones are both equal distance away from 0,0, then return the dropzone that is first in the given array.
For example, if you are given: fire = [1,1], possibleDZ = [0,1],[1,0],[2,2] . The answer is [0,1] because that is the first possible drop zone in the given array. | def dropzone(p, dropzones):
return min(dropzones,key=lambda d:(((d[0]-p[0])**2+(d[1]-p[1])**2)**0.5,(d[0]**2+d[1]**2)**0.5)) | def dropzone(p, dropzones):
return min(dropzones,key=lambda d:(((d[0]-p[0])**2+(d[1]-p[1])**2)**0.5,(d[0]**2+d[1]**2)**0.5)) | train | APPS_structured |
An Ironman Triathlon is one of a series of long-distance triathlon races organized by the World Triathlon Corporaion (WTC).
It consists of a 2.4-mile swim, a 112-mile bicycle ride and a marathon (26.2-mile) (run, raced in that order and without a break. It hurts... trust me.
Your task is to take a distance that an athlete is through the race, and return one of the following:
If the distance is zero, return `'Starting Line... Good Luck!'`.
If the athlete will be swimming, return an object with `'Swim'` as the key, and the remaining race distance as the value.
If the athlete will be riding their bike, return an object with `'Bike'` as the key, and the remaining race distance as the value.
If the athlete will be running, and has more than 10 miles to go, return an object with `'Run'` as the key, and the remaining race distance as the value.
If the athlete has 10 miles or less to go, return return an object with `'Run'` as the key, and `'Nearly there!'` as the value.
Finally, if the athlete has completed te distance, return `"You're done! Stop running!"`.
All distance should be calculated to two decimal places. | def i_tri(s):
remain = 2.4 + 112 + 26.2 - s
if s == 0:
return 'Starting Line... Good Luck!'
elif s < 2.4:
return {'Swim': f'{remain:.2f} to go!'}
elif s < 2.4 + 112:
return {'Bike': f'{remain:.2f} to go!'}
elif remain > 10:
return {'Run': f'{remain:.2f} to go!'}
elif remain > 0:
return {'Run': 'Nearly there!'}
else:
return "You're done! Stop running!" | def i_tri(s):
remain = 2.4 + 112 + 26.2 - s
if s == 0:
return 'Starting Line... Good Luck!'
elif s < 2.4:
return {'Swim': f'{remain:.2f} to go!'}
elif s < 2.4 + 112:
return {'Bike': f'{remain:.2f} to go!'}
elif remain > 10:
return {'Run': f'{remain:.2f} to go!'}
elif remain > 0:
return {'Run': 'Nearly there!'}
else:
return "You're done! Stop running!" | train | APPS_structured |
The new Formula 1 season is about to begin and Chef has got the chance to work with the Formula 1 technical team.
Recently, the pre-season testing ended and the technical team found out that their timing system for qualifying was a little bit buggy. So, they asked Chef to fix it before the season begins.
His task is to write a program to find the starting lineup of the race by taking the timings of the drivers in qualifying.
(If you don’t know the rules that make the starting grid in Formula 1, consider that the driver with the least time will start at the front).
Note:
- Two or more drivers can have the same name.
- Every driver will have a distinct time.
-----Input:-----
- The first line of the input consists of a single integer $T$ denoting the number of test cases.
- First line of each test case consists of a single integer $N$ denoting the number of drivers to set a time.
- The following $2*N$ lines consists of the driver’s name $S$ in one line and its timing details $X$ (in milliseconds) in the next line.
-----Output:-----
- For each test case output the starting lineup of the race i.e., name of each driver in the order they will start the race. Print each name in a new line.
-----Constraints-----
- 1 <= $T$ <= 10
- 1 <= $N$ <= 105
- 1 <= $|S|$ <= 20
- 1 <= $X$ <= 109
-----Subtasks-----
Subtask #1 (20 points):
- 1 <= $N$ <= 100
Subtask #2 (80 points):
- Original Constraints
-----Sample Input:-----
2
3
Hamilton
75000
Vettel
76000
Bottas
75500
2
Leclerc
666666
Verstappen
666777
-----Sample Output:-----
Hamilton
Bottas
Vettel
Leclerc
Verstappen
-----EXPLANATION:-----
The drivers with the least time are ahead in the lineup. | # cook your dish here
tc = int(input())
for t in range(tc):
n = int(input())
tr=['']*n
nr=['']*n
ck=False
for i in range(n):
name = input()
tim = int(input())
if not ck:
ck=True
tr[0]=tim
nr[0]=name
else:
tr[i]=tim
nr[i]=name
for j in range(i+1):
key=tr[j]
kname=nr[j]
k=j-1
while k>=0 and tr[k]>key:
tr[k+1]=tr[k]
nr[k+1]=nr[k]
k = k - 1
tr[k+1]=key
nr[k+1]=kname
print(*nr,sep='\n') | # cook your dish here
tc = int(input())
for t in range(tc):
n = int(input())
tr=['']*n
nr=['']*n
ck=False
for i in range(n):
name = input()
tim = int(input())
if not ck:
ck=True
tr[0]=tim
nr[0]=name
else:
tr[i]=tim
nr[i]=name
for j in range(i+1):
key=tr[j]
kname=nr[j]
k=j-1
while k>=0 and tr[k]>key:
tr[k+1]=tr[k]
nr[k+1]=nr[k]
k = k - 1
tr[k+1]=key
nr[k+1]=kname
print(*nr,sep='\n') | train | APPS_structured |
We have an array of integers, nums, and an array of requests where requests[i] = [starti, endi]. The ith request asks for the sum of nums[starti] + nums[starti + 1] + ... + nums[endi - 1] + nums[endi]. Both starti and endi are 0-indexed.
Return the maximum total sum of all requests among all permutations of nums.
Since the answer may be too large, return it modulo 109 + 7.
Example 1:
Input: nums = [1,2,3,4,5], requests = [[1,3],[0,1]]
Output: 19
Explanation: One permutation of nums is [2,1,3,4,5] with the following result:
requests[0] -> nums[1] + nums[2] + nums[3] = 1 + 3 + 4 = 8
requests[1] -> nums[0] + nums[1] = 2 + 1 = 3
Total sum: 8 + 3 = 11.
A permutation with a higher total sum is [3,5,4,2,1] with the following result:
requests[0] -> nums[1] + nums[2] + nums[3] = 5 + 4 + 2 = 11
requests[1] -> nums[0] + nums[1] = 3 + 5 = 8
Total sum: 11 + 8 = 19, which is the best that you can do.
Example 2:
Input: nums = [1,2,3,4,5,6], requests = [[0,1]]
Output: 11
Explanation: A permutation with the max total sum is [6,5,4,3,2,1] with request sums [11].
Example 3:
Input: nums = [1,2,3,4,5,10], requests = [[0,2],[1,3],[1,1]]
Output: 47
Explanation: A permutation with the max total sum is [4,10,5,3,2,1] with request sums [19,18,10].
Constraints:
n == nums.length
1 <= n <= 105
0 <= nums[i] <= 105
1 <= requests.length <= 105
requests[i].length == 2
0 <= starti <= endi < n | class Solution:
def maxSumRangeQuery(self, nums: List[int], requests: List[List[int]]) -> int:
requestCount = defaultdict(int) #Number of request per index
#Create a min-heap of the start and end intervals.
begHeap = []
endHeap = []
for req in requests:
heapq.heappush(begHeap, req[0])
heapq.heappush(endHeap, req[1]+1)
poppedCount = 0 #Keeps track of how many intervals we have entered without exiting. (Currently active)
for i in range(len(nums)):
while begHeap and begHeap[0] <= i:
heapq.heappop(begHeap)
poppedCount += 1
while endHeap and endHeap[0] <= i:
heapq.heappop(endHeap)
poppedCount -= 1
continue
requestCount[i] = poppedCount
#Then we simply get the highest interval frequency and match with the highest nums
sortedRequest = sorted(list(requestCount.items()), key = lambda x: -x[1])
nums.sort(key = lambda x: -x)
out = 0
for n, s in zip(nums, sortedRequest):
out += (n*s[1])
return out % (pow(10, 9)+7)
| class Solution:
def maxSumRangeQuery(self, nums: List[int], requests: List[List[int]]) -> int:
requestCount = defaultdict(int) #Number of request per index
#Create a min-heap of the start and end intervals.
begHeap = []
endHeap = []
for req in requests:
heapq.heappush(begHeap, req[0])
heapq.heappush(endHeap, req[1]+1)
poppedCount = 0 #Keeps track of how many intervals we have entered without exiting. (Currently active)
for i in range(len(nums)):
while begHeap and begHeap[0] <= i:
heapq.heappop(begHeap)
poppedCount += 1
while endHeap and endHeap[0] <= i:
heapq.heappop(endHeap)
poppedCount -= 1
continue
requestCount[i] = poppedCount
#Then we simply get the highest interval frequency and match with the highest nums
sortedRequest = sorted(list(requestCount.items()), key = lambda x: -x[1])
nums.sort(key = lambda x: -x)
out = 0
for n, s in zip(nums, sortedRequest):
out += (n*s[1])
return out % (pow(10, 9)+7)
| train | APPS_structured |
Ronald's uncle left him 3 fertile chickens in his will. When life gives you chickens, you start a business selling chicken eggs which is exactly what Ronald decided to do.
A chicken lays 300 eggs in its first year. However, each chicken's egg production decreases by 20% every following year (rounded down) until when it dies (after laying its quota of eggs).
After his first successful year of business, Ronald decides to buy 3 more chickens at the start of each year.
Your Task:
For a given year, and life span of chicken span, calculate how many eggs Ronald's chickens will lay him that year, whereby year=1 is when Ronald first got his inheritance and span>0.
If year=0, make sure to return "No chickens yet!".
Note:
1. All chickens have the same life span regardless of when they are bought.
2. Let's assume all calculations are made at the end of the year so don't bother taking eggs laid per month into consideration.
3. Each chicken's egg production goes down by 20% each year, NOT the total number of eggs produced by each 'batch' of chickens. While this might appear to be the same thing, it doesn't once non-integers come into play so take care that this is reflected in your kata! | def egged(year, span):
eggs = lambda e,n: 0 if n==0 else e + eggs(int(e*.8), n-1)
return eggs(300,min(year,span)) * 3 if year else 'No chickens yet!' | def egged(year, span):
eggs = lambda e,n: 0 if n==0 else e + eggs(int(e*.8), n-1)
return eggs(300,min(year,span)) * 3 if year else 'No chickens yet!' | train | APPS_structured |
Design a stack which supports the following operations.
Implement the CustomStack class:
CustomStack(int maxSize) Initializes the object with maxSize which is the maximum number of elements in the stack or do nothing if the stack reached the maxSize.
void push(int x) Adds x to the top of the stack if the stack hasn't reached the maxSize.
int pop() Pops and returns the top of stack or -1 if the stack is empty.
void inc(int k, int val) Increments the bottom k elements of the stack by val. If there are less than k elements in the stack, just increment all the elements in the stack.
Example 1:
Input
["CustomStack","push","push","pop","push","push","push","increment","increment","pop","pop","pop","pop"]
[[3],[1],[2],[],[2],[3],[4],[5,100],[2,100],[],[],[],[]]
Output
[null,null,null,2,null,null,null,null,null,103,202,201,-1]
Explanation
CustomStack customStack = new CustomStack(3); // Stack is Empty []
customStack.push(1); // stack becomes [1]
customStack.push(2); // stack becomes [1, 2]
customStack.pop(); // return 2 --> Return top of the stack 2, stack becomes [1]
customStack.push(2); // stack becomes [1, 2]
customStack.push(3); // stack becomes [1, 2, 3]
customStack.push(4); // stack still [1, 2, 3], Don't add another elements as size is 4
customStack.increment(5, 100); // stack becomes [101, 102, 103]
customStack.increment(2, 100); // stack becomes [201, 202, 103]
customStack.pop(); // return 103 --> Return top of the stack 103, stack becomes [201, 202]
customStack.pop(); // return 202 --> Return top of the stack 102, stack becomes [201]
customStack.pop(); // return 201 --> Return top of the stack 101, stack becomes []
customStack.pop(); // return -1 --> Stack is empty return -1.
Constraints:
1 <= maxSize <= 1000
1 <= x <= 1000
1 <= k <= 1000
0 <= val <= 100
At most 1000 calls will be made to each method of increment, push and pop each separately. | class CustomStack:
def __init__(self, maxSize: int):
self._max_size = maxSize
self._len = 0
self._stack = []
def push(self, x: int) -> None:
if self._len < self._max_size:
self._stack.insert(0, x)
self._len += 1
def pop(self) -> int:
if self._len > 0:
self._len -= 1
ret = self._stack.pop(0)
else:
ret = -1
return ret
def increment(self, k: int, val: int) -> None:
i = 0
while(i < k and i < self._len):
self._stack[self._len - i -1] += val
i += 1
# Your CustomStack object will be instantiated and called as such:
# obj = CustomStack(maxSize)
# obj.push(x)
# param_2 = obj.pop()
# obj.increment(k,val)
| class CustomStack:
def __init__(self, maxSize: int):
self._max_size = maxSize
self._len = 0
self._stack = []
def push(self, x: int) -> None:
if self._len < self._max_size:
self._stack.insert(0, x)
self._len += 1
def pop(self) -> int:
if self._len > 0:
self._len -= 1
ret = self._stack.pop(0)
else:
ret = -1
return ret
def increment(self, k: int, val: int) -> None:
i = 0
while(i < k and i < self._len):
self._stack[self._len - i -1] += val
i += 1
# Your CustomStack object will be instantiated and called as such:
# obj = CustomStack(maxSize)
# obj.push(x)
# param_2 = obj.pop()
# obj.increment(k,val)
| train | APPS_structured |
Chef had an interesting dream last night. He dreamed of a new revolutionary chicken recipe. When he woke up today he tried very hard to reconstruct the ingredient list. But, he could only remember certain ingredients. To simplify the problem, the ingredient list can be represented by a string of lowercase characters 'a' - 'z'.
Chef can recall some characters of the ingredient list, all the others, he has forgotten. However, he is quite sure that the ingredient list was a palindrome.
You are given the ingredient list Chef dreamed last night. The forgotten characters are represented by a question mark ('?'). Count the number of ways Chef can replace the forgotten characters with characters 'a' - 'z' in such a way that resulting ingredient list is a palindrome.
-----Input-----
The first line of input contains a single integer T, the number of test cases. T lines follow, each containing a single non-empty string - the ingredient list as recalled by Chef. Whatever letters he couldn't recall are represented by a '?'.
-----Output-----
For each test case, output a single line containing the number of valid ways the ingredient list could be completed. Since the answers can be very large, output each answer modulo 10,000,009.
-----Example-----
Input:
5
?
??
ab?
a?c
aba
Output:
26
26
1
0
1
-----Constraints-----
1 ≤ T ≤ 20
1 ≤ sum of length of all input strings ≤ 1,000,000
Each input string contains only lowercase roman letters ('a' - 'z') or question marks. | for _ in range(int(input())):
s=list(input())
n=len(s)
an=1
mod=10000009
for i in range(n):
if s[i]=="?" and s[n-i-1]=="?":
an=(an*26)%mod
s[i]="."
s[n-i-1]="."
elif s[i]!="?" and s[n-i-1]!="?":
if s[i]!=s[n-i-1]:
an=0
break
print(an%mod)
| for _ in range(int(input())):
s=list(input())
n=len(s)
an=1
mod=10000009
for i in range(n):
if s[i]=="?" and s[n-i-1]=="?":
an=(an*26)%mod
s[i]="."
s[n-i-1]="."
elif s[i]!="?" and s[n-i-1]!="?":
if s[i]!=s[n-i-1]:
an=0
break
print(an%mod)
| train | APPS_structured |
An array is **circularly sorted** if the elements are sorted in ascending order, but displaced, or rotated, by any number of steps.
Complete the function/method that determines if the given array of integers is circularly sorted.
## Examples
These arrays are circularly sorted (`true`):
```
[2, 3, 4, 5, 0, 1] --> [0, 1] + [2, 3, 4, 5]
[4, 5, 6, 9, 1] --> [1] + [4, 5, 6, 9]
[10, 11, 6, 7, 9] --> [6, 7, 9] + [10, 11]
[1, 2, 3, 4, 5] --> [1, 2, 3, 4, 5]
[5, 7, 43, 987, -9, 0] --> [-9, 0] + [5, 7, 43, 987]
[1, 2, 3, 4, 1] --> [1] + [1, 2, 3, 4]
```
While these are not (`false`):
```
[4, 1, 2, 5]
[8, 7, 6, 5, 4, 3]
[6, 7, 4, 8]
[7, 6, 5, 4, 3, 2, 1]
``` | def circularly_sorted(arr):
flag= 0
for i in range(0, len(arr)):
prev = arr[i-1]
if prev > arr[i]:
flag +=1
if flag > 1:
return False
else:
return True | def circularly_sorted(arr):
flag= 0
for i in range(0, len(arr)):
prev = arr[i-1]
if prev > arr[i]:
flag +=1
if flag > 1:
return False
else:
return True | train | APPS_structured |
Write function alternateCase which switch every letter in string from upper to lower and from lower to upper.
E.g: Hello World -> hELLO wORLD | def alternateCase(s):
'''
Salvador Dali - The Persistence of Memory
;!>,!!!>
<! ;!!!`
!! `!!!.
!!`,!!!>
!! !!!!!
;!! !!!!!
`!! !!!!!
!! !!!!!> .
`!.`!!!!! ;<!`
!! `!!!! ,<!``
`!> !!!! ;<!`
<!! !!!!> ;!``
!!! `!!!! !!` ,c,
!!!> !!!!> ;`< ,cc$$cc .,r== $$c !
!!!! !!!!!!. ,<!` !!!!!>>>;;;;;;;;.`"?$$c MMMMMMM )MM ,= "$$.`
<!!> !!!!!!!!!!!!!>`` ,>```` ``````````!!!; ?$$c`MMMMMM.`MMMP== `$h
`!! ;!!!!!!````.,;;;``` JF !;;;,,,,; 3$$.`MMMMMb MMMnnnM $$h
;!! <!!!!.;;!!!``` JF.!!!!!!!!!>`$$h `MMMMM MMMMMMM $$$
;!!>`!!!!!!`` ?> !!!!!!!!!> $$$ MMMMM MMMMMMM $$$
<!! ;!!!!` `h !!!!!!!!!! $$$ MMMMMM MMMM" M $$$
`!>`!!!! b !!!!!!!!!! $$$ MMMMMM MMML,,`,$$$
,,,,,, ;! <!!!> ,,,,,,,,,,,,,,,, $ !!!!!!!!!! $$$ MMMMMM MMMMML J$$F
!!!!!! !! !!!! `!!!!!!!!!!!!!!` ; $ !!!!!!!!!! $$$ MMMMMP.MMMMMP $$$F
!!!!! ;!! !!!!> !!!!!!!!!!!!` ;` .`.`.`. ?.`!!!!!!!!! 3$$ MMMP `MMMMM>,$$P
!!!!` !!` !!!!> !!!!!!!!!!` ;!` `.`.`.`. `h !!!!!!!!! $$$ MMML MMPPP J$$`.
!!!! !!!;!!!!!`;!!!!!!!!` ;!` .`.`.`.`.` ?,`!!!!!!!! ?$$ MMMMM.,MM_"`,$$F .
!!!`;!!!.!!!!` <!!!!!!!` ;` .`.`.`.`.`.`. ? `!!!!!!!>`$$ MMMMMbdML ` $$$ .
``` !!!> !!!! ```````` ;! .`.`.`.`.`.`.`.` h `!!!!!!> $$ )MMMMMMMMM d$$` `.
!!` !!!``!!! <!!!!!!!> ` .`.`.`.`.`.`.`.`.` `?,``!!!!! ?$h 4MMMMMMP z$$` .`.
`` <!!! !!!> ```````` .`.`.`.`.`.`.`.`.`.`.` ?h.````..`$$ MMMMMM ,$$F `.`.
` !!!! <!!!.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. `"??$F". `$h.`MMMMM $$$`.`.`.
<!`! .!!!!> .`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. cccc `$$.`4MMP.3$F .`.`.
<!``! !!!!!> .`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. J$$$$$F . "$h." . 3$h .`.`.
!` ! !!!!!!> .`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. """" .`.`.`$$, 4 3$$ .`.`.
;! !!!!!!! `.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. ?$h J$F .`.`.
;` !!!!!!! `.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. "$$$$P` .`.`.
` <!!!!!!! `.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. . .`.`.`.`.
,` !!!!!!! .`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.
!! !!!!!!`,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,, `.`.`.`.
!! !!!!!! !!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` `.`.`.`.
!! <!!!!`;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!! ;! `.`.`.`.
!! ;!!!!>`!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` <!! `.`.`.`.
`,,!!!!``.;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` <!!! `.`.`.`.
`````.,;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` <!!!! `.`.`.`.
;!!!!!!!!!!!!!!!!!!!!!!!>>>````````````````````<!!!!!!!!!!!` !!!!!! `.`.`.`.
!!!!!!!!!!!!!!!!!!````_,,uunmnmnmdddPPPPPPbbbnmnyy,_`````! !!!!!!! `.`.`.`.
!!!!!!!!!``_``!``,nmMMPP"""`,.,ccccccr==pccccc,,..`""444n,.`<!!!!!! `.`.`.`.
!!!!!!!` ,dMM ,nMMP"",zcd$h.`$$$$$$$$L c $$$$$$$$$$??cc`4Mn <!!!!! `.`.`.`.
!!!!!! ,MMMP uMMP ,d$$$$$$$$cd$F ??$$$$$cd$$$$$$$$$F, ??h.`Mx !!!!! `.`.`.`.
!!!!!! MMMP uMM",F,c ".$$$$$$$P` ?$$$$$$$$$$$$$$$$C`,J$$.`M.`!!!! `.`.`.`.
!!!`` MMM 4MMM L`"=-z$$P".,,. ,c$$$$$$$$$$$$$$$$$$$$$$$ ML !!!! `.`.`.`.
!!!. `"" MMMM `$$hc$$$L,c,.,czc$$$$$$$$$$$$$$$$$$$$$$$$$$.4M `!!! `.`.`.`.
!!!!;;;.. `MMMb ?$$$$$$??""""?????????????????? ;.`$$$$$$$`JM`,!!! `.`.`.`.
!!!!!!!!!> 4MMMb."?$$$cc,.. .,,cccccccccccccccc,c`.$$$$$$$ MM <!!! `.`.`.`.
!!!!!!!!!! `MMMMMb,."??$$$$$$$$$$$$$$$$$$$$$$$$$$ $$$$?$$$ MM ;!!! `.`.`.`.
!!!!!!!!!! "4MMMMMMmn,."""???$$$$$$$$$$$$$$$$$$ $$$" ?$$ MP !!!! `.`.`.`.
!!!!!!!!!!!;. "4MMMMMMMMMMMmnmn,.`"""?????$$$$$$ ?$$ `CF.M> !!!! `.`.`.`.
!!!!!!!!!!!!!!;. `""44MMMMMMMMMMMMMMMMnnnn. ?$$$.<$$$h.$h MM !!!! `.`.`.`.
!!!!!!!!!!!!!!!!>.;. `""444MMMMMMMMMMMMMb $$$:<$$$$$$$ 4M <!!! `.`.`.`.
!!!!!!!!!!!!!!!!!!!!!;<;);>;. ..""""44MMMM J$` <$$$$$$h`Mb`!!! `.`.`.`.
!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!>; `MMM ?$. d$$$$$$$ MM.`!! `.`.`.`.
!!!!!!!!!!```!``` ..``<!!!!!!!!!!!!!!!; . MMM.<$$ <$$$$$$$$ 4Mb !! `.`.`.`.
!`````````.. ;MMMMnn.`!!!!!!!!!!!!!!! ; MMM J$$hJ$$$$$$$$h`MM !! `.`.`.`.
,xmdMMMbnmx,. MMMMMMMr !!!!!!!!!!!!` ;: MMM $$$$$$$$$$??$$ MM !! `.`.`.`.
P"`.,,,,c`""4MMn MMMMMMx !!!!!!!!!!!` <!: MMM $$$$$$$$L =c$F.MP !! `.`.`.`.
ub "P4MM")M,x,"4b,`44MMMP !!!!!!!!!! !!!: MMM ???$$$$$$"=,$ JM`;!! `.`.`.`.
ML,,nn4Mx`"MMMx.`ML ;,,,;<!!!!!!!!! ;!!!!: MMM.-=,$$$$$$hc$`.MP !!! `.`.`.`.
. MMM 4Mx`MMML MM,`<!!!!!!!!!!!! ;!!!!!` `MMb d$$$$$$$$` MM`,!!! `.`.`.`.
,d" MMn. .,nMMMM MMM `!!!!!!!!!!! ;!!!!!!> 4MMb `$$$$$$$`.dM`.!!!! `.`.`.`.
.`"M"_" MMMMMMMP,MMM ;!>>!!!!!!` <!!!!!!!!!. "MMb $$$$$$$ dM ;!!!!! `.`.`.`.
`nr;MMMMM"MMMP dMM` >>!(!)<<! <!!!!!!!!!!!. `MM.`$$$$$$ MM !!!!!! `.`.`.`.
",Jn,"". ,uMMP dMMP /)<;>><>` .!!!!!!!!!!!!!!; `Mb $$$$$F;MP !!!!!! `.`.`.`.
dPPM 4MMMMM" dMMP (->;)<><` ;!!!!!!!!!!!!!!!!. 4M $$$$$h M>.!!!!!! `.`.`.`.
=M uMMnMMM" uMMM" <!;/;->;` ;!!!!!!!!!!!!!!!!!; 4M.??"$$`,M <!!!!!! `.`.`.`.
JM )MMMM" uMMMM`,!>;`(>!>` <!!!!!!!!!!!!!!!!!!! 4M> -??`,M` !!!!!!! `.`.`.`.
MM `MP" xdMMMP <(;<:)!`)` <!!!!!!!!!!!!!!!!!!!! MMb - ,M`;!!!!!!!` `.`.`.`.
MP ,nMMMMP" (>:)/;<:! !!!!!!!!!!!!!!!!!!!!!! `MM.-= d`;!!!!!!!! .`.`.`.`.
,xndMMMMP" .;)`;:`>(;: !!!!!!!!!!!!!!!!!!!!!!!; 4MMnndM <!!!!!!! .`.`.`.`.
MMMMMP" .;(:`-;(.><(` ;!!!!!!!!!!!!!!!!!!!!!!!!!, 4MMMMP !!!!!!!> `.`.`.`.`.
P"" .,;<):(;/(\`>-)` ;!!!!!!!!!!!!!!!!!!!!!!!!!!!>.`"P" <!!!!!!! .`.`.`.`.`.
,<;),<-:><;,<- >;>` ;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!;;<!!!!!!!! .`.`.`.`.`.
)-/;`<:<;\->:(`;(` ;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` `.`.`.`.`.`.
:\;`<(.:>-;(;<>: <!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!! .`.`.`.`.`.`.
(.;>:`<;:<;-/)/ <!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!! .`.`.`.`.`.`.
;-;:>:.;`;(`;` ;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` `.`.`.`.`.`.`.
'''
return s.swapcase() | def alternateCase(s):
'''
Salvador Dali - The Persistence of Memory
;!>,!!!>
<! ;!!!`
!! `!!!.
!!`,!!!>
!! !!!!!
;!! !!!!!
`!! !!!!!
!! !!!!!> .
`!.`!!!!! ;<!`
!! `!!!! ,<!``
`!> !!!! ;<!`
<!! !!!!> ;!``
!!! `!!!! !!` ,c,
!!!> !!!!> ;`< ,cc$$cc .,r== $$c !
!!!! !!!!!!. ,<!` !!!!!>>>;;;;;;;;.`"?$$c MMMMMMM )MM ,= "$$.`
<!!> !!!!!!!!!!!!!>`` ,>```` ``````````!!!; ?$$c`MMMMMM.`MMMP== `$h
`!! ;!!!!!!````.,;;;``` JF !;;;,,,,; 3$$.`MMMMMb MMMnnnM $$h
;!! <!!!!.;;!!!``` JF.!!!!!!!!!>`$$h `MMMMM MMMMMMM $$$
;!!>`!!!!!!`` ?> !!!!!!!!!> $$$ MMMMM MMMMMMM $$$
<!! ;!!!!` `h !!!!!!!!!! $$$ MMMMMM MMMM" M $$$
`!>`!!!! b !!!!!!!!!! $$$ MMMMMM MMML,,`,$$$
,,,,,, ;! <!!!> ,,,,,,,,,,,,,,,, $ !!!!!!!!!! $$$ MMMMMM MMMMML J$$F
!!!!!! !! !!!! `!!!!!!!!!!!!!!` ; $ !!!!!!!!!! $$$ MMMMMP.MMMMMP $$$F
!!!!! ;!! !!!!> !!!!!!!!!!!!` ;` .`.`.`. ?.`!!!!!!!!! 3$$ MMMP `MMMMM>,$$P
!!!!` !!` !!!!> !!!!!!!!!!` ;!` `.`.`.`. `h !!!!!!!!! $$$ MMML MMPPP J$$`.
!!!! !!!;!!!!!`;!!!!!!!!` ;!` .`.`.`.`.` ?,`!!!!!!!! ?$$ MMMMM.,MM_"`,$$F .
!!!`;!!!.!!!!` <!!!!!!!` ;` .`.`.`.`.`.`. ? `!!!!!!!>`$$ MMMMMbdML ` $$$ .
``` !!!> !!!! ```````` ;! .`.`.`.`.`.`.`.` h `!!!!!!> $$ )MMMMMMMMM d$$` `.
!!` !!!``!!! <!!!!!!!> ` .`.`.`.`.`.`.`.`.` `?,``!!!!! ?$h 4MMMMMMP z$$` .`.
`` <!!! !!!> ```````` .`.`.`.`.`.`.`.`.`.`.` ?h.````..`$$ MMMMMM ,$$F `.`.
` !!!! <!!!.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. `"??$F". `$h.`MMMMM $$$`.`.`.
<!`! .!!!!> .`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. cccc `$$.`4MMP.3$F .`.`.
<!``! !!!!!> .`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. J$$$$$F . "$h." . 3$h .`.`.
!` ! !!!!!!> .`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. """" .`.`.`$$, 4 3$$ .`.`.
;! !!!!!!! `.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. ?$h J$F .`.`.
;` !!!!!!! `.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. "$$$$P` .`.`.
` <!!!!!!! `.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. . .`.`.`.`.
,` !!!!!!! .`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.
!! !!!!!!`,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,, `.`.`.`.
!! !!!!!! !!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` `.`.`.`.
!! <!!!!`;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!! ;! `.`.`.`.
!! ;!!!!>`!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` <!! `.`.`.`.
`,,!!!!``.;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` <!!! `.`.`.`.
`````.,;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` <!!!! `.`.`.`.
;!!!!!!!!!!!!!!!!!!!!!!!>>>````````````````````<!!!!!!!!!!!` !!!!!! `.`.`.`.
!!!!!!!!!!!!!!!!!!````_,,uunmnmnmdddPPPPPPbbbnmnyy,_`````! !!!!!!! `.`.`.`.
!!!!!!!!!``_``!``,nmMMPP"""`,.,ccccccr==pccccc,,..`""444n,.`<!!!!!! `.`.`.`.
!!!!!!!` ,dMM ,nMMP"",zcd$h.`$$$$$$$$L c $$$$$$$$$$??cc`4Mn <!!!!! `.`.`.`.
!!!!!! ,MMMP uMMP ,d$$$$$$$$cd$F ??$$$$$cd$$$$$$$$$F, ??h.`Mx !!!!! `.`.`.`.
!!!!!! MMMP uMM",F,c ".$$$$$$$P` ?$$$$$$$$$$$$$$$$C`,J$$.`M.`!!!! `.`.`.`.
!!!`` MMM 4MMM L`"=-z$$P".,,. ,c$$$$$$$$$$$$$$$$$$$$$$$ ML !!!! `.`.`.`.
!!!. `"" MMMM `$$hc$$$L,c,.,czc$$$$$$$$$$$$$$$$$$$$$$$$$$.4M `!!! `.`.`.`.
!!!!;;;.. `MMMb ?$$$$$$??""""?????????????????? ;.`$$$$$$$`JM`,!!! `.`.`.`.
!!!!!!!!!> 4MMMb."?$$$cc,.. .,,cccccccccccccccc,c`.$$$$$$$ MM <!!! `.`.`.`.
!!!!!!!!!! `MMMMMb,."??$$$$$$$$$$$$$$$$$$$$$$$$$$ $$$$?$$$ MM ;!!! `.`.`.`.
!!!!!!!!!! "4MMMMMMmn,."""???$$$$$$$$$$$$$$$$$$ $$$" ?$$ MP !!!! `.`.`.`.
!!!!!!!!!!!;. "4MMMMMMMMMMMmnmn,.`"""?????$$$$$$ ?$$ `CF.M> !!!! `.`.`.`.
!!!!!!!!!!!!!!;. `""44MMMMMMMMMMMMMMMMnnnn. ?$$$.<$$$h.$h MM !!!! `.`.`.`.
!!!!!!!!!!!!!!!!>.;. `""444MMMMMMMMMMMMMb $$$:<$$$$$$$ 4M <!!! `.`.`.`.
!!!!!!!!!!!!!!!!!!!!!;<;);>;. ..""""44MMMM J$` <$$$$$$h`Mb`!!! `.`.`.`.
!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!>; `MMM ?$. d$$$$$$$ MM.`!! `.`.`.`.
!!!!!!!!!!```!``` ..``<!!!!!!!!!!!!!!!; . MMM.<$$ <$$$$$$$$ 4Mb !! `.`.`.`.
!`````````.. ;MMMMnn.`!!!!!!!!!!!!!!! ; MMM J$$hJ$$$$$$$$h`MM !! `.`.`.`.
,xmdMMMbnmx,. MMMMMMMr !!!!!!!!!!!!` ;: MMM $$$$$$$$$$??$$ MM !! `.`.`.`.
P"`.,,,,c`""4MMn MMMMMMx !!!!!!!!!!!` <!: MMM $$$$$$$$L =c$F.MP !! `.`.`.`.
ub "P4MM")M,x,"4b,`44MMMP !!!!!!!!!! !!!: MMM ???$$$$$$"=,$ JM`;!! `.`.`.`.
ML,,nn4Mx`"MMMx.`ML ;,,,;<!!!!!!!!! ;!!!!: MMM.-=,$$$$$$hc$`.MP !!! `.`.`.`.
. MMM 4Mx`MMML MM,`<!!!!!!!!!!!! ;!!!!!` `MMb d$$$$$$$$` MM`,!!! `.`.`.`.
,d" MMn. .,nMMMM MMM `!!!!!!!!!!! ;!!!!!!> 4MMb `$$$$$$$`.dM`.!!!! `.`.`.`.
.`"M"_" MMMMMMMP,MMM ;!>>!!!!!!` <!!!!!!!!!. "MMb $$$$$$$ dM ;!!!!! `.`.`.`.
`nr;MMMMM"MMMP dMM` >>!(!)<<! <!!!!!!!!!!!. `MM.`$$$$$$ MM !!!!!! `.`.`.`.
",Jn,"". ,uMMP dMMP /)<;>><>` .!!!!!!!!!!!!!!; `Mb $$$$$F;MP !!!!!! `.`.`.`.
dPPM 4MMMMM" dMMP (->;)<><` ;!!!!!!!!!!!!!!!!. 4M $$$$$h M>.!!!!!! `.`.`.`.
=M uMMnMMM" uMMM" <!;/;->;` ;!!!!!!!!!!!!!!!!!; 4M.??"$$`,M <!!!!!! `.`.`.`.
JM )MMMM" uMMMM`,!>;`(>!>` <!!!!!!!!!!!!!!!!!!! 4M> -??`,M` !!!!!!! `.`.`.`.
MM `MP" xdMMMP <(;<:)!`)` <!!!!!!!!!!!!!!!!!!!! MMb - ,M`;!!!!!!!` `.`.`.`.
MP ,nMMMMP" (>:)/;<:! !!!!!!!!!!!!!!!!!!!!!! `MM.-= d`;!!!!!!!! .`.`.`.`.
,xndMMMMP" .;)`;:`>(;: !!!!!!!!!!!!!!!!!!!!!!!; 4MMnndM <!!!!!!! .`.`.`.`.
MMMMMP" .;(:`-;(.><(` ;!!!!!!!!!!!!!!!!!!!!!!!!!, 4MMMMP !!!!!!!> `.`.`.`.`.
P"" .,;<):(;/(\`>-)` ;!!!!!!!!!!!!!!!!!!!!!!!!!!!>.`"P" <!!!!!!! .`.`.`.`.`.
,<;),<-:><;,<- >;>` ;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!;;<!!!!!!!! .`.`.`.`.`.
)-/;`<:<;\->:(`;(` ;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` `.`.`.`.`.`.
:\;`<(.:>-;(;<>: <!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!! .`.`.`.`.`.`.
(.;>:`<;:<;-/)/ <!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!! .`.`.`.`.`.`.
;-;:>:.;`;(`;` ;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` `.`.`.`.`.`.`.
'''
return s.swapcase() | train | APPS_structured |
#Sorting on planet Twisted-3-7
There is a planet... in a galaxy far far away. It is exactly like our planet, but it has one difference:
#The values of the digits 3 and 7 are twisted.
Our 3 means 7 on the planet Twisted-3-7. And 7 means 3.
Your task is to create a method, that can sort an array the way it would be sorted on Twisted-3-7.
7 Examples from a friend from Twisted-3-7:
```
[1,2,3,4,5,6,7,8,9] -> [1,2,7,4,5,6,3,8,9]
[12,13,14] -> [12,14,13]
[9,2,4,7,3] -> [2,7,4,3,9]
```
There is no need for a precheck. The array will always be not null and will always contain at least one number.
You should not modify the input array!
Have fun coding it and please don't forget to vote and rank this kata! :-)
I have also created other katas. Take a look if you enjoyed this kata! | def sort_twisted37(arr):
return sorted(arr, key=lambda x: int(str(x).translate(str.maketrans('37', '73')))) | def sort_twisted37(arr):
return sorted(arr, key=lambda x: int(str(x).translate(str.maketrans('37', '73')))) | train | APPS_structured |
Karlsson has recently discovered a huge stock of berry jam jars in the basement of the house. More specifically, there were $2n$ jars of strawberry and blueberry jam.
All the $2n$ jars are arranged in a row. The stairs to the basement are exactly in the middle of that row. So when Karlsson enters the basement, he sees exactly $n$ jars to his left and $n$ jars to his right.
For example, the basement might look like this: [Image]
Being the starightforward man he is, he immediately starts eating the jam. In one minute he chooses to empty either the first non-empty jar to his left or the first non-empty jar to his right.
Finally, Karlsson decided that at the end the amount of full strawberry and blueberry jam jars should become the same.
For example, this might be the result: [Image] He has eaten $1$ jar to his left and then $5$ jars to his right. There remained exactly $3$ full jars of both strawberry and blueberry jam.
Jars are numbered from $1$ to $2n$ from left to right, so Karlsson initially stands between jars $n$ and $n+1$.
What is the minimum number of jars Karlsson is required to empty so that an equal number of full strawberry and blueberry jam jars is left?
Your program should answer $t$ independent test cases.
-----Input-----
The first line contains one integer $t$ ($1 \le t \le 1000$) — the number of test cases.
The first line of each test case contains a single integer $n$ ($1 \le n \le 10^5$).
The second line of each test case contains $2n$ integers $a_1, a_2, \dots, a_{2n}$ ($1 \le a_i \le 2$) — $a_i=1$ means that the $i$-th jar from the left is a strawberry jam jar and $a_i=2$ means that it is a blueberry jam jar.
It is guaranteed that the sum of $n$ over all test cases does not exceed $10^5$.
-----Output-----
For each test case print the answer to it — the minimum number of jars Karlsson is required to empty so that an equal number of full strawberry and blueberry jam jars is left.
-----Example-----
Input
4
6
1 1 1 2 2 1 2 1 2 1 1 2
2
1 2 1 2
3
1 1 1 1 1 1
2
2 1 1 1
Output
6
0
6
2
-----Note-----
The picture from the statement describes the first test case.
In the second test case the number of strawberry and blueberry jam jars is already equal.
In the third test case Karlsson is required to eat all $6$ jars so that there remain $0$ jars of both jams.
In the fourth test case Karlsson can empty either the second and the third jars or the third and the fourth one. The both scenarios will leave $1$ jar of both jams. | q = int(input())
for rwier in range(q):
n = int(input())
l = list(map(int,input().split()))
j = l.count(1)
d = l.count(2)
pr = [0] * n
le = [0] * n
pr[0] = (1 if l[n] == 1 else -1)
le[0] = (1 if l[n-1] == 1 else -1)
for i in range(1, n):
pr[i] = pr[i-1] + (1 if l[n+i] == 1 else -1)
le[i] = le[i-1] + (1 if l[n-i-1] == 1 else -1)
#print(pr,le)
if j - d < 0:
for i in range(n):
pr[i] = -pr[i]
le[i] = -le[i]
ab = abs(j-d)
if ab == 0:
print(0)
else:
#suma = abs
najwp = [123456789] * (2*n+1)
najwl = [123456789] * (2*n+1)
le = [0] + le
pr = [0] + pr
for i in range(n+1):
if pr[i] >= 0 and najwp[pr[i]] == 123456789:
najwp[pr[i]] = i
if le[i] >= 0 and najwl[le[i]] == 123456789:
najwl[le[i]] = i
wyn = 41343443143
for i in range(ab+1):
if najwp[i] + najwl[ab-i] < wyn:
wyn = najwp[i] +najwl[ab-i]
print(wyn)
| q = int(input())
for rwier in range(q):
n = int(input())
l = list(map(int,input().split()))
j = l.count(1)
d = l.count(2)
pr = [0] * n
le = [0] * n
pr[0] = (1 if l[n] == 1 else -1)
le[0] = (1 if l[n-1] == 1 else -1)
for i in range(1, n):
pr[i] = pr[i-1] + (1 if l[n+i] == 1 else -1)
le[i] = le[i-1] + (1 if l[n-i-1] == 1 else -1)
#print(pr,le)
if j - d < 0:
for i in range(n):
pr[i] = -pr[i]
le[i] = -le[i]
ab = abs(j-d)
if ab == 0:
print(0)
else:
#suma = abs
najwp = [123456789] * (2*n+1)
najwl = [123456789] * (2*n+1)
le = [0] + le
pr = [0] + pr
for i in range(n+1):
if pr[i] >= 0 and najwp[pr[i]] == 123456789:
najwp[pr[i]] = i
if le[i] >= 0 and najwl[le[i]] == 123456789:
najwl[le[i]] = i
wyn = 41343443143
for i in range(ab+1):
if najwp[i] + najwl[ab-i] < wyn:
wyn = najwp[i] +najwl[ab-i]
print(wyn)
| train | APPS_structured |
Gorodetskiy is a university student. He is really good at math and really likes solving engaging math problems. In the last exam, his professor gave him really hard math problems to solve, but Gorodetskiy could not solve them and failed the exam, so the professor told him: "These problems are a piece of cake, you should know them from school!" Here is one of the problems from the exam - you can decide if it really was an easy problem or not.
You are given a positive integer $M$. Let's call a positive integer $A$ an interesting number if there is at least one integer $B$ ($A \le B$) such that $A \cdot B$ is divisible by $M$ and $\frac{A \cdot B}{M} = A+B$.
Find all interesting numbers. Let's denote the number of such integers by $K$; it is guaranteed that $K \le 3*10^5$.
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first and only line of each test case contains a single integer $M$.
-----Output-----
For each test case:
- First, print a line containing a single integer $K$.
- Then, print $K$ lines. For each valid $i$, the $i$-th of these lines should contain a single integer ― the $i$-th interesting number in increasing order.
-----Constraints-----
- $1 \le T \le 10$
- $1 \le M \le 10^{14}$
-----Subtasks-----
Subtask #1 (20 points): $1 \le M \le 100$
Subtask #2 (20 points): $1 \le M \le 10^7$
Subtask #3 (60 points): original constraints
-----Example Input-----
2
3
6
-----Example Output-----
2
4
6
5
7
8
9
10
12
-----Explanation-----
Example case 1: There are two interesting numbers, $4$ and $6$. For $A = 4$, we can choose $B = 12$ and for $A = 6$, we can choose $B = 6$. | T = int(input())
for t in range(T):
M = int(input())
A = []
for a in range(M+1, 2*M+1):
b = (a*M)/(a-M)
if float(a+b).is_integer() == True and b > 0:
A.append(a)
print(len(A))
for i in range(len(A)):
print(A[i])
| T = int(input())
for t in range(T):
M = int(input())
A = []
for a in range(M+1, 2*M+1):
b = (a*M)/(a-M)
if float(a+b).is_integer() == True and b > 0:
A.append(a)
print(len(A))
for i in range(len(A)):
print(A[i])
| train | APPS_structured |
*This kata is based on [Project Euler Problem 539](https://projecteuler.net/problem=539)*
##Object
Find the last number between 1 and `n` (inclusive) that survives the elimination process
####How It Works
Start with the first number on the left then remove every other number moving right until you reach the the end, then from the numbers remaining start with the first number on the right and remove every other number moving left, repeat the process alternating between left and right until only one number remains which you return as the "last man standing"
##Example
given an input of `9` our set of numbers is `1 2 3 4 5 6 7 8 9`
start by removing from the left 2 4 6 8
1 3 5 7 9
then from the right 2 6
4 8
then the left again 6
2
until we end with `6` as the last man standing
**Note:** due to the randomness of the tests it is possible that you will get unlucky and a few of the tests will be really large, so try submitting 2 or 3 times.
As allways any feedback would be much appreciated | def last_man_standing(n):
l=[]
l.extend(range(1,n+1))
def doer(t):
w = []
return t[1::2]
while len(l) > 1:
l = doer(l)
l.reverse()
return l[0] | def last_man_standing(n):
l=[]
l.extend(range(1,n+1))
def doer(t):
w = []
return t[1::2]
while len(l) > 1:
l = doer(l)
l.reverse()
return l[0] | train | APPS_structured |
Given a non-empty string s, you may delete at most one character. Judge whether you can make it a palindrome.
Example 1:
Input: "aba"
Output: True
Example 2:
Input: "abca"
Output: True
Explanation: You could delete the character 'c'.
Note:
The string will only contain lowercase characters a-z.
The maximum length of the string is 50000. | class Solution:
def validPalindrome(self, s):
"""
:type s: str
:rtype: bool
"""
if s == s[::-1]:
return True
i,j = 0, len(s)-1
while i < j:
if s[i] == s[j]:
i+=1
j-=1
else:
remove_i = s[:i] + s[i+1:]
remove_j = s[:j] + s[j+1:]
return remove_i == remove_i[::-1] or remove_j == remove_j[::-1] | class Solution:
def validPalindrome(self, s):
"""
:type s: str
:rtype: bool
"""
if s == s[::-1]:
return True
i,j = 0, len(s)-1
while i < j:
if s[i] == s[j]:
i+=1
j-=1
else:
remove_i = s[:i] + s[i+1:]
remove_j = s[:j] + s[j+1:]
return remove_i == remove_i[::-1] or remove_j == remove_j[::-1] | train | APPS_structured |
Given an equation, represented by words on left side and the result on right side.
You need to check if the equation is solvable under the following rules:
Each character is decoded as one digit (0 - 9).
Every pair of different characters they must map to different digits.
Each words[i] and result are decoded as one number without leading zeros.
Sum of numbers on left side (words) will equal to the number on right side (result).
Return True if the equation is solvable otherwise return False.
Example 1:
Input: words = ["SEND","MORE"], result = "MONEY"
Output: true
Explanation: Map 'S'-> 9, 'E'->5, 'N'->6, 'D'->7, 'M'->1, 'O'->0, 'R'->8, 'Y'->'2'
Such that: "SEND" + "MORE" = "MONEY" , 9567 + 1085 = 10652
Example 2:
Input: words = ["SIX","SEVEN","SEVEN"], result = "TWENTY"
Output: true
Explanation: Map 'S'-> 6, 'I'->5, 'X'->0, 'E'->8, 'V'->7, 'N'->2, 'T'->1, 'W'->'3', 'Y'->4
Such that: "SIX" + "SEVEN" + "SEVEN" = "TWENTY" , 650 + 68782 + 68782 = 138214
Example 3:
Input: words = ["THIS","IS","TOO"], result = "FUNNY"
Output: true
Example 4:
Input: words = ["LEET","CODE"], result = "POINT"
Output: false
Constraints:
2 <= words.length <= 5
1 <= words[i].length, result.length <= 7
words[i], result contains only upper case English letters.
Number of different characters used on the expression is at most 10. | class Solution:
def isSolvable(self, words: List[str], result: str) -> bool:
max_len = len(result)
if max(len(w) for w in words) > max_len:
return False
words = [word.rjust(max_len, '#') for word in words]
result = result.rjust(max_len, '#')
equations = list(zip(result, *words))
used_digits = set()
nonzero_chars = set(equations[0])
assignments = {'#':0}
def isSAT(eqn_index, carry):
if eqn_index<0:
return carry == 0
remaining_terms = []
for t in equations[eqn_index]:
if t not in assignments:
for guess in range(10):
if guess in used_digits:
continue
if guess == 0 and t in nonzero_chars:
continue
assignments[t] = guess
used_digits.add(guess)
if isSAT(eqn_index, carry):
return True
del assignments[t]
used_digits.remove(guess)
return False
s = sum(assignments[c] for c in equations[eqn_index][1:]) + carry
if s % 10 != assignments[equations[eqn_index][0]]:
return False
else:
return isSAT(eqn_index - 1, s // 10)
return isSAT(max_len-1, 0) | class Solution:
def isSolvable(self, words: List[str], result: str) -> bool:
max_len = len(result)
if max(len(w) for w in words) > max_len:
return False
words = [word.rjust(max_len, '#') for word in words]
result = result.rjust(max_len, '#')
equations = list(zip(result, *words))
used_digits = set()
nonzero_chars = set(equations[0])
assignments = {'#':0}
def isSAT(eqn_index, carry):
if eqn_index<0:
return carry == 0
remaining_terms = []
for t in equations[eqn_index]:
if t not in assignments:
for guess in range(10):
if guess in used_digits:
continue
if guess == 0 and t in nonzero_chars:
continue
assignments[t] = guess
used_digits.add(guess)
if isSAT(eqn_index, carry):
return True
del assignments[t]
used_digits.remove(guess)
return False
s = sum(assignments[c] for c in equations[eqn_index][1:]) + carry
if s % 10 != assignments[equations[eqn_index][0]]:
return False
else:
return isSAT(eqn_index - 1, s // 10)
return isSAT(max_len-1, 0) | train | APPS_structured |
Most of this problem is by the original author of [the harder kata](https://www.codewars.com/kata/556206664efbe6376700005c), I just made it simpler.
I read a book recently, titled "Things to Make and Do in the Fourth Dimension" by comedian and mathematician Matt Parker ( [Youtube](https://www.youtube.com/user/standupmaths) ), and in the first chapter of the book Matt talks about problems he likes to solve in his head to take his mind off the fact that he is in his dentist's chair, we've all been there!
The problem he talks about relates to polydivisible numbers, and I thought a kata should be written on the subject as it's quite interesting. (Well it's interesting to me, so there!)
### Polydivisib... huh what?
So what are they?
A polydivisible number is divisible in an unusual way. The first digit is cleanly divisible by `1`, the first two digits are cleanly divisible by `2`, the first three by `3`, and so on.
### Examples
Let's take the number `1232` as an example.
```
1 / 1 = 1 // Works
12 / 2 = 6 // Works
123 / 3 = 41 // Works
1232 / 4 = 308 // Works
```
`1232` is a polydivisible number.
However, let's take the number `123220` and see what happens.
```
1 /1 = 1 // Works
12 /2 = 6 // Works
123 /3 = 41 // Works
1232 /4 = 308 // Works
12322 /5 = 2464.4 // Doesn't work
123220 /6 = 220536.333... // Doesn't work
```
`123220` is not polydivisible.
### Your job: check if a number is polydivisible or not.
Return `true` if it is, and `false` if it isn't.
Note: All inputs will be valid numbers between `0` and `2^53-1 (9,007,199,254,740,991)` (inclusive).
Note: All single digit numbers (including `0`) are trivially polydivisible.
Note: Except for `0`, no numbers will start with `0`. | def polydivisible(x):
x = str(x)
return len([0 for i in range(1, len(x) + 1) if int(x[:i]) % i]) == 0 | def polydivisible(x):
x = str(x)
return len([0 for i in range(1, len(x) + 1) if int(x[:i]) % i]) == 0 | train | APPS_structured |
You are given an array $a$ of $n$ integers and an integer $s$. It is guaranteed that $n$ is odd.
In one operation you can either increase or decrease any single element by one. Calculate the minimum number of operations required to make the median of the array being equal to $s$.
The median of the array with odd length is the value of the element which is located on the middle position after the array is sorted. For example, the median of the array $6, 5, 8$ is equal to $6$, since if we sort this array we will get $5, 6, 8$, and $6$ is located on the middle position.
-----Input-----
The first line contains two integers $n$ and $s$ ($1\le n\le 2\cdot 10^5-1$, $1\le s\le 10^9$) — the length of the array and the required value of median.
The second line contains $n$ integers $a_1, a_2, \ldots, a_n$ ($1\le a_i \le 10^9$) — the elements of the array $a$.
It is guaranteed that $n$ is odd.
-----Output-----
In a single line output the minimum number of operations to make the median being equal to $s$.
-----Examples-----
Input
3 8
6 5 8
Output
2
Input
7 20
21 15 12 11 20 19 12
Output
6
-----Note-----
In the first sample, $6$ can be increased twice. The array will transform to $8, 5, 8$, which becomes $5, 8, 8$ after sorting, hence the median is equal to $8$.
In the second sample, $19$ can be increased once and $15$ can be increased five times. The array will become equal to $21, 20, 12, 11, 20, 20, 12$. If we sort this array we get $11, 12, 12, 20, 20, 20, 21$, this way the median is $20$. | c = 0
n, s = list(map(int, input().split()))
arr = sorted([int(i) for i in input().split()])
if arr[n//2] < s:
for i in range(n//2, n): c += (s - arr[i])*(arr[i] < s)
else:
for i in range(n//2+1): c -= (s - arr[i])*(arr[i] > s)
print(c)
| c = 0
n, s = list(map(int, input().split()))
arr = sorted([int(i) for i in input().split()])
if arr[n//2] < s:
for i in range(n//2, n): c += (s - arr[i])*(arr[i] < s)
else:
for i in range(n//2+1): c -= (s - arr[i])*(arr[i] > s)
print(c)
| train | APPS_structured |
Consider the infinite x$x$ axis. There are N$N$ impacts on this X-axis at integral points (X1$X_1$,X2$X_2$,....XN$X_N$) (all distinct) . An impact at a point X$X$i propagates such that at a point X$X$0, the effect of the impact is K|Xi−X0|$K^{|X_i - X_0|}$. Given the point X0$X_0$, N$N$ and K$K$. Assume the total impact on X0$X_0$ is M$M$, find if it is possible to do so.Note: You are not required to find the set X
Formally print "yes" if this is possible and "no" if not possible.
-----Input:-----
- First line will contain T$T$, number of testcases. Then the testcases follow.
- Each testcase contains of a single line of input, four integers N$N$,K$K$,M$M$,X$X$0
-----Output:-----
- The output of each test case is either "yes" or "no"
-----Constraints -----
- 1≤T≤1000$1\leq T \leq 1000$
- 1≤N≤100$1\leq N \leq 100$
- 1≤K≤1000$1\leq K \leq 1000$
- 1≤M≤1018$1\leq M \leq 10^{18}$
- −109≤X0≤109$-10^9 \leq X_0 \leq 10^9$
-----Sample Input:-----
2
4 3 10 10
2 3 10 10
-----Sample Output:-----
no
yes | # cook your dish here
T = int(input())
for i in range(T):
l = list(map(int, input().split()))
n, k, m, x = l[0], l[1], l[2], l[3]
if k == 1:
if n == m:
print("yes")
else:
print("no")
elif m % k > 1:
print("no")
elif k == 2:
stack = []
var = 0
while m != 0:
var += m % k
stack.append(m % k)
m //= k
if var > n:
print("no")
elif var == n:
print("yes")
else:
for p in range(100):
for q in range(2, len(stack)):
if stack[q - 1] == 0 and stack[q] >= 1:
stack[q-1] = 2
stack[q] -= 1
var += 1
if var == n:
print("yes")
if var < n:
print("no")
else:
temp = 0
rog = 1
while m != 0:
if m % k > 2:
rog = 0
print("no")
temp += m % k
m //= k
if rog:
if temp == n:
print("yes")
else:
print("no")
| # cook your dish here
T = int(input())
for i in range(T):
l = list(map(int, input().split()))
n, k, m, x = l[0], l[1], l[2], l[3]
if k == 1:
if n == m:
print("yes")
else:
print("no")
elif m % k > 1:
print("no")
elif k == 2:
stack = []
var = 0
while m != 0:
var += m % k
stack.append(m % k)
m //= k
if var > n:
print("no")
elif var == n:
print("yes")
else:
for p in range(100):
for q in range(2, len(stack)):
if stack[q - 1] == 0 and stack[q] >= 1:
stack[q-1] = 2
stack[q] -= 1
var += 1
if var == n:
print("yes")
if var < n:
print("no")
else:
temp = 0
rog = 1
while m != 0:
if m % k > 2:
rog = 0
print("no")
temp += m % k
m //= k
if rog:
if temp == n:
print("yes")
else:
print("no")
| train | APPS_structured |
Given an array of integers.
Return an array, where the first element is the count of positives numbers and the second element is sum of negative numbers.
If the input array is empty or null, return an empty array.
# Example
For input `[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, -11, -12, -13, -14, -15]`, you should return `[10, -65]`. | def count_positives_sum_negatives(arr):
if arr == []:
return []
pos = sum([i>0 for i in arr])
neg = sum(i for i in arr if i< 0)
return [pos,neg]
| def count_positives_sum_negatives(arr):
if arr == []:
return []
pos = sum([i>0 for i in arr])
neg = sum(i for i in arr if i< 0)
return [pos,neg]
| train | APPS_structured |
Given a binary array nums, you should delete one element from it.
Return the size of the longest non-empty subarray containing only 1's in the resulting array.
Return 0 if there is no such subarray.
Example 1:
Input: nums = [1,1,0,1]
Output: 3
Explanation: After deleting the number in position 2, [1,1,1] contains 3 numbers with value of 1's.
Example 2:
Input: nums = [0,1,1,1,0,1,1,0,1]
Output: 5
Explanation: After deleting the number in position 4, [0,1,1,1,1,1,0,1] longest subarray with value of 1's is [1,1,1,1,1].
Example 3:
Input: nums = [1,1,1]
Output: 2
Explanation: You must delete one element.
Example 4:
Input: nums = [1,1,0,0,1,1,1,0,1]
Output: 4
Example 5:
Input: nums = [0,0,0]
Output: 0
Constraints:
1 <= nums.length <= 10^5
nums[i] is either 0 or 1. | class Solution:
def longestSubarray(self, nums: List[int]) -> int:
k = 1
i = 0
for j in range(len(nums)):
if nums[j] == 0: k -= 1
if k < 0:
if nums[i] == 0: k += 1
i += 1
return j-i | class Solution:
def longestSubarray(self, nums: List[int]) -> int:
k = 1
i = 0
for j in range(len(nums)):
if nums[j] == 0: k -= 1
if k < 0:
if nums[i] == 0: k += 1
i += 1
return j-i | train | APPS_structured |
There are $n$ athletes in front of you. Athletes are numbered from $1$ to $n$ from left to right. You know the strength of each athlete — the athlete number $i$ has the strength $s_i$.
You want to split all athletes into two teams. Each team must have at least one athlete, and each athlete must be exactly in one team.
You want the strongest athlete from the first team to differ as little as possible from the weakest athlete from the second team. Formally, you want to split the athletes into two teams $A$ and $B$ so that the value $|\max(A) - \min(B)|$ is as small as possible, where $\max(A)$ is the maximum strength of an athlete from team $A$, and $\min(B)$ is the minimum strength of an athlete from team $B$.
For example, if $n=5$ and the strength of the athletes is $s=[3, 1, 2, 6, 4]$, then one of the possible split into teams is: first team: $A = [1, 2, 4]$, second team: $B = [3, 6]$.
In this case, the value $|\max(A) - \min(B)|$ will be equal to $|4-3|=1$. This example illustrates one of the ways of optimal split into two teams.
Print the minimum value $|\max(A) - \min(B)|$.
-----Input-----
The first line contains an integer $t$ ($1 \le t \le 1000$) — the number of test cases in the input. Then $t$ test cases follow.
Each test case consists of two lines.
The first line contains positive integer $n$ ($2 \le n \le 50$) — number of athletes.
The second line contains $n$ positive integers $s_1, s_2, \ldots, s_n$ ($1 \le s_i \le 1000$), where $s_i$ — is the strength of the $i$-th athlete. Please note that $s$ values may not be distinct.
-----Output-----
For each test case print one integer — the minimum value of $|\max(A) - \min(B)|$ with the optimal split of all athletes into two teams. Each of the athletes must be a member of exactly one of the two teams.
-----Example-----
Input
5
5
3 1 2 6 4
6
2 1 3 2 4 3
4
7 9 3 1
2
1 1000
3
100 150 200
Output
1
0
2
999
50
-----Note-----
The first test case was explained in the statement. In the second test case, one of the optimal splits is $A=[2, 1]$, $B=[3, 2, 4, 3]$, so the answer is $|2-2|=0$. | q = int(input())
for i in range(q):
n = int(input())
l = list(map(int,input().split()))
l.sort()
wynik = 23472983749823739
for i in range(1,n):
wynik = min(wynik, l[i]-l[i-1])
print(wynik) | q = int(input())
for i in range(q):
n = int(input())
l = list(map(int,input().split()))
l.sort()
wynik = 23472983749823739
for i in range(1,n):
wynik = min(wynik, l[i]-l[i-1])
print(wynik) | train | APPS_structured |
##Task:
You have to write a function **pattern** which creates the following pattern upto n number of rows. *If the Argument is 0 or a Negative Integer then it should return "" i.e. empty string.*
##Examples:
pattern(4):
1234
234
34
4
pattern(6):
123456
23456
3456
456
56
6
```Note: There are no blank spaces```
```Hint: Use \n in string to jump to next line``` | def pattern(n):
return '\n'.join( ''.join(str(j) for j in range(i,n+1)) for i in range(1,n+1) ) | def pattern(n):
return '\n'.join( ''.join(str(j) for j in range(i,n+1)) for i in range(1,n+1) ) | train | APPS_structured |
### Description
As hex values can include letters `A` through to `F`, certain English words can be spelled out, such as `CAFE`, `BEEF`, or `FACADE`.
This vocabulary can be extended by using numbers to represent other letters, such as `5EAF00D`, or `DEC0DE5`.
Given a string, your task is to return the decimal sum of all words in the string that can be interpreted as such hex values.
### Example
Working with the string `BAG OF BEES`:
* `BAG` ==> `0` as it is not a valid hex value
* `OF` ==> `0F` ==> `15`
* `BEES` ==> `BEE5` ==> `48869`
So `hex_word_sum('BAG OF BEES')` returns the sum of these, `48884`.
### Notes
* Inputs are all uppercase and contain no punctuation
* `0` can be substituted for `O`
* `5` can be substituted for `S` | import re
def hex_word_sum(s):
s = s.translate(str.maketrans('OS','05'))
return sum( int(x,16) for x in re.findall(r'\b[\dA-F]+\b', s)) | import re
def hex_word_sum(s):
s = s.translate(str.maketrans('OS','05'))
return sum( int(x,16) for x in re.findall(r'\b[\dA-F]+\b', s)) | train | APPS_structured |
Write a function that merges two sorted arrays into a single one. The arrays only contain integers. Also, the final outcome must be sorted and not have any duplicate. | def merge_arrays(first, second):
merge = sorted(set(first + second))
return merge | def merge_arrays(first, second):
merge = sorted(set(first + second))
return merge | train | APPS_structured |
## Task
Create a RomanNumerals class that can convert a roman numeral to and from an integer value. It should follow the API demonstrated in the examples below. Multiple roman numeral values will be tested for each helper method.
Modern Roman numerals are written by expressing each digit separately starting with the left most digit and skipping any digit with a value of zero. In Roman numerals 1990 is rendered: 1000=M, 900=CM, 90=XC; resulting in MCMXC. 2008 is written as 2000=MM, 8=VIII; or MMVIII. 1666 uses each Roman symbol in descending order: MDCLXVI.
## Examples
```python
RomanNumerals.to_roman(1000) # should return 'M'
RomanNumerals.from_roman('M') # should return 1000
```
## Help
| Symbol | Value |
|----------------|
| I | 1 |
| V | 5 |
| X | 10 |
| L | 50 |
| C | 100 |
| D | 500 |
| M | 1000 | | from itertools import groupby
"""A RomanNumerals helper object"""
class RomanNumerals(object):
letters = [('M',1000), ('CM',900), ('D',500), ('CD',400), ('C',100), ('XC',90),
('L',50), ('XL',40), ('X',10), ('IX',9), ('V',5), ('IV',4), ('I',1)]
@classmethod
def to_roman(cls, val):
rom = []
for l, v in cls.letters:
m = val // v
rom += m*[l]
val -= m*v
return ''.join(rom)
@classmethod
def from_roman(cls, rom):
cumu = 0
for l, v in cls.letters:
while rom[:len(l)] == l:
rom = rom[len(l):]
cumu += v
if not rom: break
return cumu
| from itertools import groupby
"""A RomanNumerals helper object"""
class RomanNumerals(object):
letters = [('M',1000), ('CM',900), ('D',500), ('CD',400), ('C',100), ('XC',90),
('L',50), ('XL',40), ('X',10), ('IX',9), ('V',5), ('IV',4), ('I',1)]
@classmethod
def to_roman(cls, val):
rom = []
for l, v in cls.letters:
m = val // v
rom += m*[l]
val -= m*v
return ''.join(rom)
@classmethod
def from_roman(cls, rom):
cumu = 0
for l, v in cls.letters:
while rom[:len(l)] == l:
rom = rom[len(l):]
cumu += v
if not rom: break
return cumu
| train | APPS_structured |
# Background
My pet bridge-maker ants are marching across a terrain from left to right.
If they encounter a gap, the first one stops and then next one climbs over him, then the next, and the next, until a bridge is formed across the gap.
What clever little things they are!
Now all the other ants can walk over the ant-bridge.
When the last ant is across, the ant-bridge dismantles itself similar to how it was constructed.
This process repeats as many times as necessary (there may be more than one gap to cross) until all the ants reach the right hand side.
# Kata Task
My little ants are marching across the terrain from left-to-right in the order ```A``` then ```B``` then ```C```...
What order do they exit on the right hand side?
# Notes
* ```-``` = solid ground
* ```.``` = a gap
* The number of ants may differ but there are always enough ants to bridge the gaps
* The terrain never starts or ends with a gap
* Ants cannot pass other ants except by going over ant-bridges
* If there is ever ambiguity which ant should move, then the ant at the **back** moves first
# Example
## Input
* ants = ````GFEDCBA````
* terrain = ```------------...-----------```
## Output
* result = ```EDCBAGF```
## Details
Ants moving left to right.
GFEDCBA
------------...-----------
The first one arrives at a gap.
GFEDCB A
------------...-----------
They start to bridge over the gap...
GFED ABC
------------...-----------
...until the ant-bridge is completed!
GF ABCDE
------------...-----------
And then the remaining ants can walk across the bridge.
F
G ABCDE
------------...-----------
And when no more ants need to cross...
ABCDE GF
------------...-----------
... the bridge dismantles itself one ant at a time....
CDE BAGF
------------...-----------
...until all ants get to the other side
EDCBAGF
------------...----------- | import re
def ant_bridge(ants, terrain):
nGap = sum( 2 + len(gap) - (free == '-') for free,gap in re.findall(r'(-+)(\.+)', '-'+terrain) ) % len(ants)
return ants[-nGap:] + ants[:-nGap] | import re
def ant_bridge(ants, terrain):
nGap = sum( 2 + len(gap) - (free == '-') for free,gap in re.findall(r'(-+)(\.+)', '-'+terrain) ) % len(ants)
return ants[-nGap:] + ants[:-nGap] | train | APPS_structured |
Tuzik and Vanya are playing the following game.
They have an N × M board and a chip to indicate the current game position. The chip can be placed on any one cell of the game board, each of which is uniquely identified by an ordered pair of positive integers (r, c), where 1 ≤ r ≤ N is the row number and 1 ≤ c ≤ M is the column number.
Initially, the chip is placed at the cell identified as (1, 1). For his move, a player can move it either 1, 2 or 3 cells up, or 1 or 2 cells to the right. The player who can not move the chip loses. In other words, a player suffers defeat if the chip is placed in the cell (N, M) at the start of his turn.
Tuzik starts the game. You have to determine who will win the game if both players play optimally.
-----Input-----
The first line contains an integer T denoting the number of tests. Each of the following T lines contain two integers N and M.
-----Output-----
For each test output "Tuzik" or "Vanya" on a separate line, indicating who will win the game.
-----Constraints-----
- 1 ≤ T ≤ 100
- 1 ≤ N, M ≤ 106
-----Example-----
Input:
2
4 4
2 2
Output:
Tuzik
Vanya
-----Explanation-----Test case 1: On his first turn Tuzik moves chip 3 cells up to the cell (4, 1). On his turn Vanya can move chip only right to the cell (4, 2) or to the cell (4, 3) and after that Tuzik moves it to (4, 4) and finishes the game.
Test case 2: On his first Turn Tuzik can move chip to the cell (2, 1) or (1, 2), but on his next turn Vanya moves it to (2, 2) and wins the game. | cases = int(input())
for _ in range(cases):
rows, cols = map(int, input().split())
if (cols - 1) % 3 == 0 and (rows - 1) % 4 == 0: print('Vanya')
elif (cols - 1) % 3 != 0 and (rows - 1) % 4 == 0: print('Tuzik')
elif (cols - 1) % 3 == 0 and (rows - 1) % 4 != 0: print('Tuzik')
else:
if (cols - 1) % 3 == 1 and (rows - 1) % 4 == 1: print('Vanya')
elif (cols - 1) % 3 == 2 and (rows - 1) % 4 == 2: print('Vanya')
else: print('Tuzik') | cases = int(input())
for _ in range(cases):
rows, cols = map(int, input().split())
if (cols - 1) % 3 == 0 and (rows - 1) % 4 == 0: print('Vanya')
elif (cols - 1) % 3 != 0 and (rows - 1) % 4 == 0: print('Tuzik')
elif (cols - 1) % 3 == 0 and (rows - 1) % 4 != 0: print('Tuzik')
else:
if (cols - 1) % 3 == 1 and (rows - 1) % 4 == 1: print('Vanya')
elif (cols - 1) % 3 == 2 and (rows - 1) % 4 == 2: print('Vanya')
else: print('Tuzik') | train | APPS_structured |
You know combinations: for example,
if you take 5 cards from a 52 cards deck you have 2,598,960 different combinations.
In mathematics the number of x combinations you can take from a set of n elements
is called the binomial coefficient of n and x, or more often `n choose x`.
The formula to compute `m = n choose x` is: `m = n! / (x! * (n - x)!)`
where ! is the factorial operator.
You are a renowned poster designer and painter. You are asked to provide 6 posters
all having the same design each in 2 colors. Posters must all have a different color combination and you have the choice of 4 colors: red, blue, yellow, green.
How many colors can you choose for each poster?
The answer is two since `4 choose 2 = 6`. The combinations will be:
{red, blue}, {red, yellow}, {red, green}, {blue, yellow}, {blue, green}, {yellow, green}.
Now same question but you have 35 posters to provide and 7 colors available. How many colors for each poster?
If you take combinations `7 choose 2` you will get 21 with the above formula.
But 21 schemes aren't enough for 35 posters. If you take `7 choose 5` combinations you will get 21 too.
Fortunately if you take `7 choose 3` or `7 choose 4` combinations you get 35 and so each poster will have a different combination of
3 colors or 5 colors. You will take 3 colors because it's less expensive.
Hence the problem is:
knowing `m` (number of posters to design),
knowing `n` (total number of available colors),
let us
search `x` (number of colors for each poster so that each poster has a unique combination of colors and the number of combinations is exactly the same as the number of posters).
In other words we must find **x** such as `n choose x = m (1)` for a given m and a given n;
`m >= 0 and n > 0`. If many x are solutions give as result the smallest x.
It can happen that when m is given at random there are no x satisfying `equation (1)` then
return -1.
Examples:
```
checkchoose(6, 4) --> 2
checkchoose(4, 4) --> 1
checkchoose(4, 2) --> -1
checkchoose(35, 7) --> 3
checkchoose(36, 7) --> -1
a = 47129212243960
checkchoose(a, 50) --> 20
checkchoose(a + 1, 50) --> -1
``` | from math import factorial as fact
def checkchoose(m, n):
for k in range(0, (n)//2 + 1):
if fact(n) // (fact(n-k) * fact(k)) == m:
return k
return -1 | from math import factorial as fact
def checkchoose(m, n):
for k in range(0, (n)//2 + 1):
if fact(n) // (fact(n-k) * fact(k)) == m:
return k
return -1 | train | APPS_structured |
Consider all binary strings of length $m$ ($1 \le m \le 60$). A binary string is a string that consists of the characters 0 and 1 only. For example, 0110 is a binary string, and 012aba is not. Obviously, there are exactly $2^m$ such strings in total.
The string $s$ is lexicographically smaller than the string $t$ (both have the same length $m$) if in the first position $i$ from the left in which they differ, we have $s[i] < t[i]$. This is exactly the way strings are compared in dictionaries and in most modern programming languages when comparing them in a standard way. For example, the string 01011 is lexicographically smaller than the string 01100, because the first two characters are the same, and the third character in the first string is less than that in the second.
We remove from this set $n$ ($1 \le n \le \min(2^m-1, 100)$) distinct binary strings $a_1, a_2, \ldots, a_n$, each of length $m$. Thus, the set will have $k=2^m-n$ strings. Sort all strings of the resulting set in lexicographical ascending order (as in the dictionary).
We number all the strings after sorting from $0$ to $k-1$. Print the string whose index is $\lfloor \frac{k-1}{2} \rfloor$ (such an element is called median), where $\lfloor x \rfloor$ is the rounding of the number down to the nearest integer.
For example, if $n=3$, $m=3$ and $a=[$010, 111, 001$]$, then after removing the strings $a_i$ and sorting, the result will take the form: $[$000, 011, 100, 101, 110$]$. Thus, the desired median is 100.
-----Input-----
The first line contains an integer $t$ ($1 \le t \le 1000$) — the number of test cases. Then, $t$ test cases follow.
The first line of each test case contains integers $n$ ($1 \le n \le \min(2^m-1, 100)$) and $m$ ($1 \le m \le 60$), where $n$ is the number of strings to remove, and $m$ is the length of binary strings. The next $n$ lines contain $a_1, a_2, \ldots, a_n$ — distinct binary strings of length $m$.
The total length of all given binary strings in all test cases in one test does not exceed $10^5$.
-----Output-----
Print $t$ answers to the test cases. For each test case, print a string of length $m$ — the median of the sorted sequence of remaining strings in the corresponding test case.
-----Example-----
Input
5
3 3
010
001
111
4 3
000
111
100
011
1 1
1
1 1
0
3 2
00
01
10
Output
100
010
0
1
11
-----Note-----
The first test case is explained in the statement.
In the second test case, the result after removing strings and sorting is $[$001, 010, 101, 110$]$. Therefore, the desired median is 010. | def count_smaller(value, banlist):
total = value
for v in banlist:
if v < value:
total -= 1
return total
cases = int(input())
for case in range(cases):
n, m = map(int, input().split())
banned = []
for i in range(n):
banned.append(int(input(), 2))
desindex = ((1 << m) - n - 1) // 2
candid = desindex
while (count_smaller(candid, banned) < desindex) or (candid in banned):
candid += 1
print(bin(candid)[2:].zfill(m)) | def count_smaller(value, banlist):
total = value
for v in banlist:
if v < value:
total -= 1
return total
cases = int(input())
for case in range(cases):
n, m = map(int, input().split())
banned = []
for i in range(n):
banned.append(int(input(), 2))
desindex = ((1 << m) - n - 1) // 2
candid = desindex
while (count_smaller(candid, banned) < desindex) or (candid in banned):
candid += 1
print(bin(candid)[2:].zfill(m)) | train | APPS_structured |
Note : This question carries $150$ $points$
There is an outcry in Middle Earth, as the greatest war between Orgs of Dark Lord Sauron and Frodo Baggins is about to begin. To end the war, Frodo decides to destroy the ring in the volcano of Mordor. There are many ships that lead Frodo to Mordor, and he is confused about which one he should board. Given two-ship numbers $M$ and $N$, Frodo has to solve a problem to find the ship which he should board.
Find the number of pairs (x, y), such that $1<=x<=M$ and $1<=y<=N$, for which $x*y + x+ y = string(x)+string(y)$ is true.
Also, calculate the number of distinct x satisfying the given condition. The number of pairs and the number of distinct x will help select Frodo the boat he should board. Help Frodo defeat Sauron.
-----Input :-----
- First line contains $T$ as number of test cases
- Each test case contains two integers $M$ and $N$
-----Output :-----
- For each test case, print two integers - the number of such pairs (x,y) and the number of distinct x
-----Constraints :-----
- 1 ≤ T ≤ 5000
- 1 ≤ M, N ≤ 10^9
-----Sample Input :-----
1
1 9
-----Sample Output :-----
1 1
-----Explanation :-----
For test case two M=1 and N=9 Pair (1,9) satisfies the above condition 1*9+1+9= “19” and only x=1 satisfies the equation. |
def lordoftherings(m, n):
if n<9 :return 0, 0
digits = len(str(n))
mul = '9'*digits
if int(mul) > n: mul = digits-1
else: mul = digits
return (m*mul)%1000000007, m
for _ in range(int(input())):
m, n = map(int, input().split())
x,y = lordoftherings(m, n)
print(x, y) |
def lordoftherings(m, n):
if n<9 :return 0, 0
digits = len(str(n))
mul = '9'*digits
if int(mul) > n: mul = digits-1
else: mul = digits
return (m*mul)%1000000007, m
for _ in range(int(input())):
m, n = map(int, input().split())
x,y = lordoftherings(m, n)
print(x, y) | train | APPS_structured |
Section numbers are strings of dot-separated integers. The highest level sections (chapters) are numbered 1, 2, 3, etc. Second level sections are numbered 1.1, 1.2, 1.3, 2.1, 2.2, 2.3, etc. Next level sections are numbered 1.1.1, 1.1.2, 1.1.2, 1.2.1, 1.2.2, erc. There is no bound on the number of sections a document may have, nor is there any bound on the number of levels.
A section of a certain level may appear directly inside a section several levels higher without the levels between. For example, section 1.0.1 may appear directly under section 1, without there being any level 2 section. Section 1.1 comes after section 1.0.1. Sections with trailing ".0" are considered to be the same as the section with the trailing ".0" truncated. Thus, section 1.0 is the same as section 1, and section 1.2.0.0 is the same as section 1.2.
```if:python
Write a function `compare(section1, section2)` that returns `-1`, `0`, or `1` depending on whether `section1` is before, same as, or after `section2` respectively.
```
```if:javascript
Write a function `cmp(section1, section2)` that returns `-1`, `0`, or `1` depending on whether `section1` is before, same as, or after `section2` respectively.
```
```if:haskell
Write a function `cmp section1 section2` that returns `LT`, `EQ` or `GT` depending on whether `section1` is before, same as, or after `section2` respectively.
``` | from itertools import zip_longest as z
compare=lambda s1,s2:next(([1,-1][int(i)<int(j)] for i,j in z(s1.split('.'),s2.split('.'),fillvalue='0') if int(i)!=int(j)),0) | from itertools import zip_longest as z
compare=lambda s1,s2:next(([1,-1][int(i)<int(j)] for i,j in z(s1.split('.'),s2.split('.'),fillvalue='0') if int(i)!=int(j)),0) | train | APPS_structured |
There are $5$ cities in the country.
The map of the country is given below.
The tour starts from the red city.
Each road is associated with a character.
Initially, there is an empty string.
Every time a road has been travelled the character associated gets appended to the string.
At the green city either the string can be printed or the tour can be continued.
In the problem, you are given a string tell whether it is possible to print the string while following the rules of the country?
-----Input:-----
- First line will contain $T$, number of testcases. Then the testcases follow.
- Each testcase contains a single line of input, a string $ s $. The string consists only of $0's$ and $1's$.
-----Output:-----
For each testcase, output "YES" or "NO" depending on the input.
-----Constraints-----
-
$1 \leq T \leq 10000$
-
$1 \leq length of each string \leq 10000$
-
$ 1 \leq Summation length \leq 10^5$
-----Sample Input:-----
1
100
-----Sample Output:-----
NO
-----EXPLANATION:----- | # cook your dish here
for e in range(int(input())):
s = input()
if len(s) >= 4:
if(s[-4::] == '1000'):
print('YES')
else:
print('NO')
else:
print('NO') | # cook your dish here
for e in range(int(input())):
s = input()
if len(s) >= 4:
if(s[-4::] == '1000'):
print('YES')
else:
print('NO')
else:
print('NO') | train | APPS_structured |
This kata is inspired on the problem #50 of the Project Euler.
The prime ``` 41``` is the result of the sum of many consecutive primes.
In fact, ``` 2 + 3 + 5 + 7 + 11 + 13 = 41 , (6 addens) ```
Furthermore, the prime ``` 41``` is the prime below ``` 100 (val_max)``` that has the longest chain of consecutive prime addens.
The prime with longest chain of addens for ```val_max = 500``` is ```499``` with ```17``` addens.
In fact:
```3+5+7+11+13+17+19+23+29+31+37+41+43+47+53+59+61= 499```
Find the function ```prime_maxlength_chain()```(primeMaxlengthChain() javascript), that receives an argument ```val_max```, the upper limit, all the found primes should be less than ```val_max``` and outputs this found prime.
Let's see some cases:
```python
prime_maxlength_chain(100) == [41]
prime_maxlength_chain(500) == [499]
```
If we have more than one prime with these features, the function should output an array with the found primes sorted.
```python
prime_maxlength_chain(499) == [379, 491]
```
Random Tests for `val_max` (`valMax`)
```
100 ≤ val_max ≤ 500.000
```
Enjoy it! | limit = 500000
sieve = [0]*2+list(range(2,limit))
for i in range(2,limit):
for j in range(i*i,limit,i):
sieve[j]=0
primes = [i for i in sieve if i]
def prime_maxlength_chain(n):
req = [i for i in primes if i < n]
P = set(req)
D = {}
found = []
for i, j in enumerate(req):
D[j] = [0, 0]
for k in list(D):
o, p = D[k]
if o in P : found.append([o, p])
if o + j > n:
del D[k]
continue
D[k][0] += j
D[k][1] += 1
m = max(found, key=lambda x: x[1])[1]
return [i[0] for i in found if i[1] == m] | limit = 500000
sieve = [0]*2+list(range(2,limit))
for i in range(2,limit):
for j in range(i*i,limit,i):
sieve[j]=0
primes = [i for i in sieve if i]
def prime_maxlength_chain(n):
req = [i for i in primes if i < n]
P = set(req)
D = {}
found = []
for i, j in enumerate(req):
D[j] = [0, 0]
for k in list(D):
o, p = D[k]
if o in P : found.append([o, p])
if o + j > n:
del D[k]
continue
D[k][0] += j
D[k][1] += 1
m = max(found, key=lambda x: x[1])[1]
return [i[0] for i in found if i[1] == m] | train | APPS_structured |
Finish the solution so that it sorts the passed in array of numbers. If the function passes in an empty array or null/nil value then it should return an empty array.
For example:
```python
solution([1,2,3,10,5]) # should return [1,2,3,5,10]
solution(None) # should return []
```
```Hakell
sortNumbers [1, 2, 10, 50, 5] = Just [1, 2, 5, 10, 50]
sortNumbers [] = Nothing
``` | import pandas as pd
def solution(nums):
df = pd.DataFrame()
df['S']=nums
answer = list(df['S'].sort_values().values)
print (list(answer))
return answer | import pandas as pd
def solution(nums):
df = pd.DataFrame()
df['S']=nums
answer = list(df['S'].sort_values().values)
print (list(answer))
return answer | train | APPS_structured |
---
# Hint
This Kata is an extension of the earlier ones in this series. Completing those first will make this task easier.
# Background
My TV remote control has arrow buttons and an `OK` button.
I can use these to move a "cursor" on a logical screen keyboard to type words...
# Keyboard
The screen "keyboard" layouts look like this
#tvkb {
width : 400px;
border: 5px solid gray; border-collapse: collapse;
}
#tvkb td {
color : orange;
background-color : black;
text-align : center;
border: 3px solid gray; border-collapse: collapse;
}
#legend {
width : 400px;
border: 1px solid gray; border-collapse: collapse;
}
#legend td {
text-align : center;
border: 1px solid gray; border-collapse: collapse;
}
Keypad Mode 1 = alpha-numeric (lowercase)
Keypad Mode 3 = symbols
abcde123
fghij456
klmno789
pqrst.@0
uvwxyz_/
aA#SP
^~?!'"()
-:;+&%*=
<>€£$¥¤\
[]{},.@§
#¿¡_/
aA#SP
* `aA#` is the SHIFT key. Pressing this key cycles through THREE keypad modes.
* **Mode 1** = alpha-numeric keypad with lowercase alpha (as depicted above)
* **Mode 2** = alpha-numeric keypad with UPPERCASE alpha
* **Mode 3** = symbolic keypad (as depicted above)
* `SP` is the space character
* The other (solid fill) keys in the bottom row have no function
## Special Symbols
For your convenience, here are Unicode values for the less obvious symbols of the **Mode 3** keypad
¡ = U-00A1£ = U-00A3¤ = U-00A4¥ = U-00A5
§ = U-00A7¿ = U-00BF€ = U-20AC
# Kata task
How many button presses on my remote are required to type the given `words`?
## Notes
* The cursor always starts on the letter `a` (top left)
* The inital keypad layout is **Mode 1**
* Remember to also press `OK` to "accept" each letter
* Take the shortest route from one letter to the next
* The cursor wraps, so as it moves off one edge it will reappear on the opposite edge
* Although the blank keys have no function, you may navigate through them if you want to
* Spaces may occur anywhere in the `words` string
* Do not press the SHIFT key until you need to. For example, with the word `e.Z`, the SHIFT change happens **after** the `.` is pressed (not before). In other words, do not try to optimize total key presses by pressing SHIFT early.
```if:c,cpp
## C/C++ warriors
The standard I/O libraries choke on wide characters beyond the value 255. This kata includes the Euro € (U-20AC). So the function `ws2utf8()` has been preloaded for converting wchar_t strings to UTF-8 for printing.
```
# Example
words = `Too Easy?`
* T => `a`-`aA#`-OK-`U`-`V`-`W`-`X`-`Y`-`T`-OK = 9
* o => `T`-`Y`-`X`-`W`-`V`-`U`-`aA#`-OK-OK-`a`-`b`-`c`-`d`-`e`-`j`-`o`-OK = 16
* o => `o`-OK = 1
* space => `o`-`n`-`m`-`l`-`q`-`v`-`SP`-OK = 7
* E => `SP`-`aA#`-OK-`A`-`3`-`2`-`1`-`-E`-OK = 8
* a => `E`-`1`-`2`-`3`-`A`-`aA`-OK-OK-`a`-OK = 9
* s => `a`-`b`-`c`-`d`-`i`-`n`-`s`-OK = 7
* y => `s`-`x`-`y`-OK = 3
* ? => `y`-`x`-`w`-`v`-`u`-`aA#`-OK-OK-`^`-`~`-`?`-OK = 11
Answer = 9 + 16 + 1 + 7 + 8 + 9 + 7 + 3 + 11 = 71
*Good Luck!
DM.*
Series
* TV Remote
* TV Remote (shift and space)
* TV Remote (wrap)
* TV Remote (symbols) | from typing import Tuple
KEYBOARDS = [
[
'abcde123',
'fghij456',
'klmno789',
'pqrst.@0',
'uvwxyz_/',
],
[
'ABCDE123',
'FGHIJ456',
'KLMNO789',
'PQRST.@0',
'UVWXYZ_/',
],
[
'^~?!\'\"()',
'-:;+&%*=',
'<>€£$¥¤\\',
'[]{},.@§',
'#¿¡ _/',
],
]
Position = Tuple[int, int]
SPACE = 5, 1
SWITCH_MODE = 5, 0
def get_pos(mode: int, c: str) -> Position:
if c == ' ':
return SPACE
for i, chars in enumerate(KEYBOARDS[mode]):
if c in chars:
return i, chars.index(c)
def get_diff(a: Position, b: Position) -> int:
(x1, y1), (x2, y2) = a, b
x, y = abs(x1 - x2), abs(y1 - y2)
return min(x, 6 - x) + min(y, 8 - y)
def mode_switch(mode: int, c: str, pos: Position) -> Tuple[int, int, Position]:
if c == ' ' or c in ''.join(KEYBOARDS[mode]):
return mode, 0, pos
switches = get_diff(pos, SWITCH_MODE)
while c not in ''.join(KEYBOARDS[mode]):
switches += 1
mode = (mode + 1) % len(KEYBOARDS)
return mode, switches, SWITCH_MODE
def tv_remote(words: str):
total = mode = 0
pos = 0, 0
for w in words:
mode, switches, pos = mode_switch(mode, w, pos)
new_pos = get_pos(mode, w)
total += switches + get_diff(pos, new_pos) + 1
pos = new_pos
return total | from typing import Tuple
KEYBOARDS = [
[
'abcde123',
'fghij456',
'klmno789',
'pqrst.@0',
'uvwxyz_/',
],
[
'ABCDE123',
'FGHIJ456',
'KLMNO789',
'PQRST.@0',
'UVWXYZ_/',
],
[
'^~?!\'\"()',
'-:;+&%*=',
'<>€£$¥¤\\',
'[]{},.@§',
'#¿¡ _/',
],
]
Position = Tuple[int, int]
SPACE = 5, 1
SWITCH_MODE = 5, 0
def get_pos(mode: int, c: str) -> Position:
if c == ' ':
return SPACE
for i, chars in enumerate(KEYBOARDS[mode]):
if c in chars:
return i, chars.index(c)
def get_diff(a: Position, b: Position) -> int:
(x1, y1), (x2, y2) = a, b
x, y = abs(x1 - x2), abs(y1 - y2)
return min(x, 6 - x) + min(y, 8 - y)
def mode_switch(mode: int, c: str, pos: Position) -> Tuple[int, int, Position]:
if c == ' ' or c in ''.join(KEYBOARDS[mode]):
return mode, 0, pos
switches = get_diff(pos, SWITCH_MODE)
while c not in ''.join(KEYBOARDS[mode]):
switches += 1
mode = (mode + 1) % len(KEYBOARDS)
return mode, switches, SWITCH_MODE
def tv_remote(words: str):
total = mode = 0
pos = 0, 0
for w in words:
mode, switches, pos = mode_switch(mode, w, pos)
new_pos = get_pos(mode, w)
total += switches + get_diff(pos, new_pos) + 1
pos = new_pos
return total | train | APPS_structured |
Suppose an array sorted in ascending order is rotated at some pivot unknown to you beforehand.
(i.e., [0,1,2,4,5,6,7] might become [4,5,6,7,0,1,2]).
Find the minimum element.
The array may contain duplicates.
Example 1:
Input: [1,3,5]
Output: 1
Example 2:
Input: [2,2,2,0,1]
Output: 0
Note:
This is a follow up problem to Find Minimum in Rotated Sorted Array.
Would allow duplicates affect the run-time complexity? How and why? | class Solution:
def findMin(self, nums):
"""
:type nums: List[int]
:rtype: int
"""
start = 0
end = len(nums) - 1
while start < end:
if start < len(nums) - 1 and nums[start] == nums[start + 1]:
start += 1
continue
if nums[end] == nums[end - 1]:
end -= 1
continue
mid = start + (end - start) // 2
if nums[mid] > nums[end]:
start = mid + 1
else:
end = mid
return nums[start]
| class Solution:
def findMin(self, nums):
"""
:type nums: List[int]
:rtype: int
"""
start = 0
end = len(nums) - 1
while start < end:
if start < len(nums) - 1 and nums[start] == nums[start + 1]:
start += 1
continue
if nums[end] == nums[end - 1]:
end -= 1
continue
mid = start + (end - start) // 2
if nums[mid] > nums[end]:
start = mid + 1
else:
end = mid
return nums[start]
| train | APPS_structured |
You are given a sequence a1, a2, ..., aN. Find the smallest possible value of ai + aj, where 1 ≤ i < j ≤ N.
-----Input-----
The first line of the input contains an integer T denoting the number of test cases. The description of T test cases follows.
The first line of each description consists of a single integer N.
The second line of each description contains N space separated integers - a1, a2, ..., aN respectively.
-----Output-----
For each test case, output a single line containing a single integer - the smallest possible sum for the corresponding test case.
-----Constraints-----
- T = 105, N = 2 : 13 points.
- T = 105, 2 ≤ N ≤ 10 : 16 points.
- T = 1000, 2 ≤ N ≤ 100 : 31 points.
- T = 10, 2 ≤ N ≤ 105 : 40 points.
- 1 ≤ ai ≤ 106
-----Example-----
Input:
1
4
5 1 3 4
Output:
4
-----Explanation-----
Here we pick a2 and a3. Their sum equals to 1 + 3 = 4. | n=int(input())
for i in range(n):
t = int(input())
l=list(map(int,input().split()))
l.sort()
print(l[0]+l[1]) | n=int(input())
for i in range(n):
t = int(input())
l=list(map(int,input().split()))
l.sort()
print(l[0]+l[1]) | train | APPS_structured |
Kate constantly finds herself in some kind of a maze. Help her to find a way out!.
For a given maze and Kate's position find if there is a way out. Your function should return True or False.
Each maze is defined as a list of strings, where each char stays for a single maze "cell". ' ' (space) can be stepped on, '#' means wall and 'k' stays for Kate's starting position. Note that the maze may not always be square or even rectangular.
Kate can move left, up, right or down only.
There should be only one Kate in a maze. In any other case you have to throw an exception.
Example input
=============
```
['# ##',
'# k#',
'####']
```
Example output
==============
True
Example input
=============
```
['####'.
'# k#',
'####']
```
Example output
==============
False | #Approach: A breadth first search from the maze starting position; at each step see if
# we have solved the maze (which is defined as walking out of the boundary of the maze)
from copy import deepcopy
import queue
class MazeSymbols(object):
START_TILE = 'k'
WALL_TILE = '#'
WALKABLE_TILE = ' '
#---end class
class MazeStatus(object):
FOUND_EXIT = 1
NO_EXIT_EXISTS = 2
CONTINUE_SEARCH = 3
MAZE_VALID = 4
MAZE_ERROR_NO_START = 5
MAZE_ERROR_DUPLICATE_START = 6
#---end class
#Maze solver must deal with non-valid mazes, which constitute: No Start Position provided,
# or multiple start positions found
class MazeSolver(object):
def __init__(self, mazeBoard):
self.mazeBoard = deepcopy(mazeBoard)
self.numRows = len( self.mazeBoard )
self.numCols = len( self.mazeBoard[0] )
validStatus, startRow, startCol = self.__validateMaze__(self.mazeBoard)
if validStatus == MazeStatus.MAZE_VALID:
self.validMaze = True
else:
self.validMaze = False
self.startRow = startRow
self.startCol = startCol
#---end constructor
def isValidMaze(self):
return self.validMaze
#---end function
def attemptSolve(self):
nR = self.numRows
nC = self.numCols
hasVisited = [ [ False for _ in range(nC) ] for _ in range(nR) ]
mazeWalkResult = self.__walkMaze__( self.mazeBoard, hasVisited,
nR, nC, self.startRow, self.startCol)
return mazeWalkResult
#---end function
def __validateMaze__(self, mazeBoard):
startPos = self.__findStartPosition__(mazeBoard)
if not startPos:
return MazeStatus.MAZE_ERROR_NO_START, -1, -1
elif len(startPos)>=2:
return MazeStatus.MAZE_ERROR_DUPLICATE_START, -1, -1
else:
startRow = startPos[0][0]
startCol = startPos[0][1]
return MazeStatus.MAZE_VALID, startRow, startCol
#---end function
#Find every possible start position in the maze
def __findStartPosition__(self, mazeBoard):
startPos = []
for i in range(self.numRows):
for j in range(self.numCols):
if self.mazeBoard[i][j] == MazeSymbols.START_TILE:
startPos.append( [i, j])
return startPos #Board does not have a start position
#---end function
#part of the logic to find all connected shapes
def __inImage__(self, i,j, nR, nC):
if 0<=i and i<nR and 0<=j and j<nC:
return True
else:
return False
#-----end function
#part of the logic to find all connected shapes
# A desired cell is one that is a ship symbol and has not been visited yet
def __isDesiredCell__(self, img, hasVisited, i, j):
if img[i][j] == MazeSymbols.WALKABLE_TILE and not hasVisited[i][j]:
return True
else:
return False
#---end function
#part of the logic to find all connected shapes
def __markCellAsVisited__(self, hasVisited, i, j):
hasVisited[i][j] = True
#end function
def __foundExit__(self, curR, curC, nR, nC):
upStep = curR - 1
downStep = curR + 1
leftStep = curC - 1
rightStep = curC + 1
if not self.__inImage__(upStep, curC, nR, nC):
return True
elif not self.__inImage__(downStep, curC, nR, nC):
return True
elif not self.__inImage__(curR, leftStep, nR, nC):
return True
elif not self.__inImage__(curR, rightStep, nR, nC):
return True
else:
return False
#---end function
#Note: This function is never called at the start of the maze
def __foundDuplicateStartPos__( self, img, curR, curC):
if img[curR][curC] == MazeSymbols.START_TILE:
return True
else:
return False
#---end function
#Check if we have found a duplicae start point, or we can exit the maze
def __checkMazeStatus__(self, img, curR, curC, nR, nC):
if self.__foundExit__(curR, curC, nR, nC):
return MazeStatus.FOUND_EXIT
elif self.__foundDuplicateStartPos__( img, curR, curC):
return MazeStatus.MAZE_ERROR_DUPLICATE_START
else:
return MazeStatus.CONTINUE_SEARCH
#---end function
#We search only on the four cardinal directions for the Maze
#X is up and down, y is left and right
def __walkMaze__(self, img, hasVisited, nR, nC, iRoot, jRoot):
#dx and dy specify the directions we are going to search dx=0 dy=1 means
#search north (one cell above), dx=1 dy=-1 is one to right and one down
di = [0, 0, 1, -1]
dj = [1, -1, 0, 0]
numSearchDirs = len(di) #I do not like literal values
#x,y are lists that are going to hold the nodes conneced to the provided root (i,j)
iConnected, jConnected = [], []
q = queue.Queue()
#Begining the start of or breadth first search, starting at the provided root.
# Note that the root is always the starting location, and therefore should not
# be cheked for duplicate start position (but should be checked if we can exit)
q.put((iRoot, jRoot))
self.__markCellAsVisited__(hasVisited, iRoot, jRoot)
if self.__foundExit__(iRoot, jRoot, nR, nC):
return MazeStatus.FOUND_EXIT
#Now we search for all connections along the allowed (four) directions
while q.empty() == False:
u, v = q.get()
iConnected.append(u)
jConnected.append(v)
#from the current position (u,v), search in the four cardinal directions
for s in range(numSearchDirs):
iNew = u + di[s]
jNew = v + dj[s]
#if we're in the image and found a desired cell, add it to the queue
if self.__inImage__(iNew, jNew, nR, nC) and \
self.__isDesiredCell__(img, hasVisited, iNew, jNew):
self.__markCellAsVisited__(hasVisited, iNew, jNew)
q.put((iNew, jNew))
currentStatus = self.__checkMazeStatus__(img, iNew, jNew, nR, nC)
if currentStatus != MazeStatus.CONTINUE_SEARCH:
return currentStatus
#Should we get to this point, there does not exist a way to an exit of the maze
# from the starting location (path to exit: list(zip(xConnected, yConnected)))
return MazeStatus.NO_EXIT_EXISTS
#---end function
#---end class
#True: Maze solvable
#False: Maze has no exit
#Error Thrown: Either no start provided, or more than one start position found
def has_exit(maze):
m = MazeSolver(maze)
if not m.isValidMaze():
raise ValueError('Maze Not Valid')
else:
resultSolve = m.attemptSolve()
if resultSolve == MazeStatus.FOUND_EXIT:
return True
elif resultSolve == MazeStatus.NO_EXIT_EXISTS:
return False
#---end function
| #Approach: A breadth first search from the maze starting position; at each step see if
# we have solved the maze (which is defined as walking out of the boundary of the maze)
from copy import deepcopy
import queue
class MazeSymbols(object):
START_TILE = 'k'
WALL_TILE = '#'
WALKABLE_TILE = ' '
#---end class
class MazeStatus(object):
FOUND_EXIT = 1
NO_EXIT_EXISTS = 2
CONTINUE_SEARCH = 3
MAZE_VALID = 4
MAZE_ERROR_NO_START = 5
MAZE_ERROR_DUPLICATE_START = 6
#---end class
#Maze solver must deal with non-valid mazes, which constitute: No Start Position provided,
# or multiple start positions found
class MazeSolver(object):
def __init__(self, mazeBoard):
self.mazeBoard = deepcopy(mazeBoard)
self.numRows = len( self.mazeBoard )
self.numCols = len( self.mazeBoard[0] )
validStatus, startRow, startCol = self.__validateMaze__(self.mazeBoard)
if validStatus == MazeStatus.MAZE_VALID:
self.validMaze = True
else:
self.validMaze = False
self.startRow = startRow
self.startCol = startCol
#---end constructor
def isValidMaze(self):
return self.validMaze
#---end function
def attemptSolve(self):
nR = self.numRows
nC = self.numCols
hasVisited = [ [ False for _ in range(nC) ] for _ in range(nR) ]
mazeWalkResult = self.__walkMaze__( self.mazeBoard, hasVisited,
nR, nC, self.startRow, self.startCol)
return mazeWalkResult
#---end function
def __validateMaze__(self, mazeBoard):
startPos = self.__findStartPosition__(mazeBoard)
if not startPos:
return MazeStatus.MAZE_ERROR_NO_START, -1, -1
elif len(startPos)>=2:
return MazeStatus.MAZE_ERROR_DUPLICATE_START, -1, -1
else:
startRow = startPos[0][0]
startCol = startPos[0][1]
return MazeStatus.MAZE_VALID, startRow, startCol
#---end function
#Find every possible start position in the maze
def __findStartPosition__(self, mazeBoard):
startPos = []
for i in range(self.numRows):
for j in range(self.numCols):
if self.mazeBoard[i][j] == MazeSymbols.START_TILE:
startPos.append( [i, j])
return startPos #Board does not have a start position
#---end function
#part of the logic to find all connected shapes
def __inImage__(self, i,j, nR, nC):
if 0<=i and i<nR and 0<=j and j<nC:
return True
else:
return False
#-----end function
#part of the logic to find all connected shapes
# A desired cell is one that is a ship symbol and has not been visited yet
def __isDesiredCell__(self, img, hasVisited, i, j):
if img[i][j] == MazeSymbols.WALKABLE_TILE and not hasVisited[i][j]:
return True
else:
return False
#---end function
#part of the logic to find all connected shapes
def __markCellAsVisited__(self, hasVisited, i, j):
hasVisited[i][j] = True
#end function
def __foundExit__(self, curR, curC, nR, nC):
upStep = curR - 1
downStep = curR + 1
leftStep = curC - 1
rightStep = curC + 1
if not self.__inImage__(upStep, curC, nR, nC):
return True
elif not self.__inImage__(downStep, curC, nR, nC):
return True
elif not self.__inImage__(curR, leftStep, nR, nC):
return True
elif not self.__inImage__(curR, rightStep, nR, nC):
return True
else:
return False
#---end function
#Note: This function is never called at the start of the maze
def __foundDuplicateStartPos__( self, img, curR, curC):
if img[curR][curC] == MazeSymbols.START_TILE:
return True
else:
return False
#---end function
#Check if we have found a duplicae start point, or we can exit the maze
def __checkMazeStatus__(self, img, curR, curC, nR, nC):
if self.__foundExit__(curR, curC, nR, nC):
return MazeStatus.FOUND_EXIT
elif self.__foundDuplicateStartPos__( img, curR, curC):
return MazeStatus.MAZE_ERROR_DUPLICATE_START
else:
return MazeStatus.CONTINUE_SEARCH
#---end function
#We search only on the four cardinal directions for the Maze
#X is up and down, y is left and right
def __walkMaze__(self, img, hasVisited, nR, nC, iRoot, jRoot):
#dx and dy specify the directions we are going to search dx=0 dy=1 means
#search north (one cell above), dx=1 dy=-1 is one to right and one down
di = [0, 0, 1, -1]
dj = [1, -1, 0, 0]
numSearchDirs = len(di) #I do not like literal values
#x,y are lists that are going to hold the nodes conneced to the provided root (i,j)
iConnected, jConnected = [], []
q = queue.Queue()
#Begining the start of or breadth first search, starting at the provided root.
# Note that the root is always the starting location, and therefore should not
# be cheked for duplicate start position (but should be checked if we can exit)
q.put((iRoot, jRoot))
self.__markCellAsVisited__(hasVisited, iRoot, jRoot)
if self.__foundExit__(iRoot, jRoot, nR, nC):
return MazeStatus.FOUND_EXIT
#Now we search for all connections along the allowed (four) directions
while q.empty() == False:
u, v = q.get()
iConnected.append(u)
jConnected.append(v)
#from the current position (u,v), search in the four cardinal directions
for s in range(numSearchDirs):
iNew = u + di[s]
jNew = v + dj[s]
#if we're in the image and found a desired cell, add it to the queue
if self.__inImage__(iNew, jNew, nR, nC) and \
self.__isDesiredCell__(img, hasVisited, iNew, jNew):
self.__markCellAsVisited__(hasVisited, iNew, jNew)
q.put((iNew, jNew))
currentStatus = self.__checkMazeStatus__(img, iNew, jNew, nR, nC)
if currentStatus != MazeStatus.CONTINUE_SEARCH:
return currentStatus
#Should we get to this point, there does not exist a way to an exit of the maze
# from the starting location (path to exit: list(zip(xConnected, yConnected)))
return MazeStatus.NO_EXIT_EXISTS
#---end function
#---end class
#True: Maze solvable
#False: Maze has no exit
#Error Thrown: Either no start provided, or more than one start position found
def has_exit(maze):
m = MazeSolver(maze)
if not m.isValidMaze():
raise ValueError('Maze Not Valid')
else:
resultSolve = m.attemptSolve()
if resultSolve == MazeStatus.FOUND_EXIT:
return True
elif resultSolve == MazeStatus.NO_EXIT_EXISTS:
return False
#---end function
| train | APPS_structured |
Related to MrZizoScream's Product Array kata. You might want to solve that one first :)
```if:javascript
**Note:** Node 10 has now been enabled, and you can now use its BigInt capabilities if you wish, though your resulting array must still contain strings (e.g. "99999999999", not 9999999999n)
Pre-node 10: You will need to use the BigNumber.js library! Please use `.toFixed(0)` or `.toPrecision()` to round instead of `.toString(10)`, as the latter is _very_ slow
```
This is an adaptation of a problem I came across on LeetCode.
Given an array of numbers, your task is to return a new array where each index (`new_array[i]`) is equal to the product of the original array, except for the number at that index (`array[i]`).
**Two things to keep in mind:**
* Zeroes will be making their way into some of the arrays you are given
* O(n^2) solutions will not pass.
Examples:
**Note**: All inputs will be valid arrays of nonzero length.
Have fun! Please upvote if you enjoyed :) | import itertools
import operator
def product_sans_n(l):
r=[]
p=list(itertools.accumulate(l,operator.mul))[-1]
for i in range(len(l)):
try:
r.append(p//l[i])
except:
s=1
for j in range(len(l)):
if i!=j:
s*=l[j]
r.append(s)
return r | import itertools
import operator
def product_sans_n(l):
r=[]
p=list(itertools.accumulate(l,operator.mul))[-1]
for i in range(len(l)):
try:
r.append(p//l[i])
except:
s=1
for j in range(len(l)):
if i!=j:
s*=l[j]
r.append(s)
return r | train | APPS_structured |
### Task:
You have to write a function `pattern` which returns the following Pattern(See Examples) upto (2n-1) rows, where n is parameter.
* Note:`Returning` the pattern is not the same as `Printing` the pattern.
#### Parameters:
pattern( n );
^
|
Term upto which
Basic Pattern(this)
should be
created
#### Rules/Note:
* If `n < 1` then it should return "" i.e. empty string.
* `The length of each line is same`, and is equal to the length of longest line in the pattern i.e (2n-1).
* Range of Parameters (for the sake of CW Compiler) :
+ `n ∈ (-∞,100]`
### Examples:
* pattern(5):
1 1
2 2
3 3
4 4
5
4 4
3 3
2 2
1 1
* pattern(10):
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0
9 9
8 8
7 7
6 6
5 5
4 4
3 3
2 2
1 1
* pattern(15):
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0 0
1 1
2 2
3 3
4 4
5
4 4
3 3
2 2
1 1
0 0
9 9
8 8
7 7
6 6
5 5
4 4
3 3
2 2
1 1
[List of all my katas]("http://www.codewars.com/users/curious_db97/authored") | get_r = lambda y: str((y + 1) % 10)
row = lambda n, i: ' ' * i + get_r(i) + ' ' * max(0, 2 * n - 3 - i * 2) + (get_r(i) if i != n - 1 else '') + ' ' * i
def pattern(n):
if n < 1: return ''
top = [row(n, x) for x in range(n - 1)]
return '\n'.join(top + [row(n, n - 1)] + list(reversed(top))) | get_r = lambda y: str((y + 1) % 10)
row = lambda n, i: ' ' * i + get_r(i) + ' ' * max(0, 2 * n - 3 - i * 2) + (get_r(i) if i != n - 1 else '') + ' ' * i
def pattern(n):
if n < 1: return ''
top = [row(n, x) for x in range(n - 1)]
return '\n'.join(top + [row(n, n - 1)] + list(reversed(top))) | train | APPS_structured |
# Description:
Remove the minimum number of exclamation marks from the start/end of each word in the sentence to make their amount equal on both sides.
### Notes:
* Words are separated with spaces
* Each word will include at least 1 letter
* There will be no exclamation marks in the middle of a word
___
## Examples
```
remove("Hi!") === "Hi"
remove("!Hi! Hi!") === "!Hi! Hi"
remove("!!Hi! !Hi!!") === "!Hi! !Hi!"
remove("!!!!Hi!! !!!!Hi !Hi!!!") === "!!Hi!! Hi !Hi!"
``` | import re
def remove(stg):
return " ".join(re.sub(r"(!*)([^!]+)(!*)", equalize, word) for word in stg.split())
def equalize(match):
before, word, after = match.groups()
shortest = min(before, after, key=len)
return f"{shortest}{word}{shortest}" | import re
def remove(stg):
return " ".join(re.sub(r"(!*)([^!]+)(!*)", equalize, word) for word in stg.split())
def equalize(match):
before, word, after = match.groups()
shortest = min(before, after, key=len)
return f"{shortest}{word}{shortest}" | train | APPS_structured |
In this kata, we want to discover a small property of numbers.
We say that a number is a **dd** number if it contains d occurences of a digit d, (d is in [1,9]).
## Examples
* 664444309 is a **dd** number, as it contains 4 occurences of the number 4
* 30313, 122 are **dd** numbers as they respectively contain 3 occurences of the number 3, and (1 occurence of the number 1 AND 2 occurences of the number 2)
* 123109, 0, 56542 are not **dd** numbers
## Task
Your task is to implement a function called `is_dd` (`isDd` in javascript) that takes a **positive** number (type depends on the language) and returns a boolean corresponding to whether the number is a **dd** number or not. | from collections import Counter
def is_dd(n):
return any(value==count for value, count in Counter(int(x) for x in str(n)).items()) | from collections import Counter
def is_dd(n):
return any(value==count for value, count in Counter(int(x) for x in str(n)).items()) | train | APPS_structured |
S and T are strings composed of lowercase letters. In S, no letter occurs more than once.
S was sorted in some custom order previously. We want to permute the characters of T so that they match the order that S was sorted. More specifically, if x occurs before y in S, then x should occur before y in the returned string.
Return any permutation of T (as a string) that satisfies this property.
Example :
Input:
S = "cba"
T = "abcd"
Output: "cbad"
Explanation:
"a", "b", "c" appear in S, so the order of "a", "b", "c" should be "c", "b", and "a".
Since "d" does not appear in S, it can be at any position in T. "dcba", "cdba", "cbda" are also valid outputs.
Note:
S has length at most 26, and no character is repeated in S.
T has length at most 200.
S and T consist of lowercase letters only. | # Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution:
def splitBST(self, root, V):
"""
:type root: TreeNode
:type V: int
:rtype: List[TreeNode]
"""
if not root:
return [None, None]
if root.val == V:
a = root.right
root.right = None
return [root, a]
elif root.val<V:
small, large = self.splitBST(root.right, V)
root.right = small
return [root, large]
else:
small, large = self.splitBST(root.left, V)
root.left = large
return [root, small] | # Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution:
def splitBST(self, root, V):
"""
:type root: TreeNode
:type V: int
:rtype: List[TreeNode]
"""
if not root:
return [None, None]
if root.val == V:
a = root.right
root.right = None
return [root, a]
elif root.val<V:
small, large = self.splitBST(root.right, V)
root.right = small
return [root, large]
else:
small, large = self.splitBST(root.left, V)
root.left = large
return [root, small] | train | APPS_structured |
A beautiful sequence is defined as a sequence that do not have any repeating elements in it.
You will be given any random sequence of integers, and you have to tell whether it is a beautiful sequence or not.
-----Input:-----
- The first line of the input contains a single integer $T$. $T$ denoting the number of test cases. The description of $T$ test cases is as follows.
- The next line of the input contains a single integer $N$. $N$ denotes the total number of elements in the sequence.
- The next line of the input contains $N$ space-separated integers $A1, A2, A3...An$ denoting the sequence.
-----Output:-----
- Print "prekrasnyy"(without quotes) if the given sequence is a beautiful sequence, else print "ne krasivo"(without quotes)
Note: each test case output must be printed on new line
-----Constraints:-----
- $1 \leq T \leq 10^2$
- $1 \leq N \leq 10^3$
- $1 \leq A1, A2, A3...An \leq 10^5$
-----Sample Input:-----
2
4
1 2 3 4
6
1 2 3 5 1 4
-----Sample Output:-----
prekrasnyy
ne krasivo
-----Explanation:-----
-
As 1st sequence do not have any elements repeating, hence it is a beautiful sequence
-
As in 2nd sequence the element 1 is repeated twice, hence it is not a beautiful sequence | for _ in range(int(input())): n = int(input());arr = list(map(int , input().split()));print("prekrasnyy") if(len(arr) == len(set(arr))) else print("ne krasivo") | for _ in range(int(input())): n = int(input());arr = list(map(int , input().split()));print("prekrasnyy") if(len(arr) == len(set(arr))) else print("ne krasivo") | train | APPS_structured |
Given 2 integers n and start. Your task is return any permutation p of (0,1,2.....,2^n -1) such that :
p[0] = start
p[i] and p[i+1] differ by only one bit in their binary representation.
p[0] and p[2^n -1] must also differ by only one bit in their binary representation.
Example 1:
Input: n = 2, start = 3
Output: [3,2,0,1]
Explanation: The binary representation of the permutation is (11,10,00,01).
All the adjacent element differ by one bit. Another valid permutation is [3,1,0,2]
Example 2:
Input: n = 3, start = 2
Output: [2,6,7,5,4,0,1,3]
Explanation: The binary representation of the permutation is (010,110,111,101,100,000,001,011).
Constraints:
1 <= n <= 16
0 <= start < 2 ^ n | class Solution:
def circularPermutation(self, n: int, start: int) -> List[int]:
return self.solve(start, n)
def solve(self, num, length):
if length==1:
if num==1:
return [1, 0]
return [0, 1]
firstnum=1
if num<2**(length-1):
firstnum=0
res=self.solve(num-firstnum*2**(length-1), length-1)
ans=[]
for i in res:
ans.append(firstnum*2**(length-1)+i)
res.reverse()
for i in res:
ans.append((1-firstnum)*2**(length-1)+i)
return ans | class Solution:
def circularPermutation(self, n: int, start: int) -> List[int]:
return self.solve(start, n)
def solve(self, num, length):
if length==1:
if num==1:
return [1, 0]
return [0, 1]
firstnum=1
if num<2**(length-1):
firstnum=0
res=self.solve(num-firstnum*2**(length-1), length-1)
ans=[]
for i in res:
ans.append(firstnum*2**(length-1)+i)
res.reverse()
for i in res:
ans.append((1-firstnum)*2**(length-1)+i)
return ans | train | APPS_structured |
You have a string $s$ consisting of $n$ characters. Each character is either 0 or 1.
You can perform operations on the string. Each operation consists of two steps: select an integer $i$ from $1$ to the length of the string $s$, then delete the character $s_i$ (the string length gets reduced by $1$, the indices of characters to the right of the deleted one also get reduced by $1$); if the string $s$ is not empty, delete the maximum length prefix consisting of the same characters (the indices of the remaining characters and the string length get reduced by the length of the deleted prefix).
Note that both steps are mandatory in each operation, and their order cannot be changed.
For example, if you have a string $s =$ 111010, the first operation can be one of the following: select $i = 1$: we'll get 111010 $\rightarrow$ 11010 $\rightarrow$ 010; select $i = 2$: we'll get 111010 $\rightarrow$ 11010 $\rightarrow$ 010; select $i = 3$: we'll get 111010 $\rightarrow$ 11010 $\rightarrow$ 010; select $i = 4$: we'll get 111010 $\rightarrow$ 11110 $\rightarrow$ 0; select $i = 5$: we'll get 111010 $\rightarrow$ 11100 $\rightarrow$ 00; select $i = 6$: we'll get 111010 $\rightarrow$ 11101 $\rightarrow$ 01.
You finish performing operations when the string $s$ becomes empty. What is the maximum number of operations you can perform?
-----Input-----
The first line contains a single integer $t$ ($1 \le t \le 1000$) — the number of test cases.
The first line of each test case contains a single integer $n$ ($1 \le n \le 2 \cdot 10^5$) — the length of the string $s$.
The second line contains string $s$ of $n$ characters. Each character is either 0 or 1.
It's guaranteed that the total sum of $n$ over test cases doesn't exceed $2 \cdot 10^5$.
-----Output-----
For each test case, print a single integer — the maximum number of operations you can perform.
-----Example-----
Input
5
6
111010
1
0
1
1
2
11
6
101010
Output
3
1
1
1
3
-----Note-----
In the first test case, you can, for example, select $i = 2$ and get string 010 after the first operation. After that, you can select $i = 3$ and get string 1. Finally, you can only select $i = 1$ and get empty string. | from itertools import groupby
def main():
N = int(input())
S = input()
C = [len(list(x[1])) for x in groupby(S)]
M = len(C)
dup_idx = []
for i, c in enumerate(C):
if c > 1:
dup_idx.append(i)
dup_idx.reverse()
curr = 0
while dup_idx:
i = dup_idx[-1]
if i < curr:
dup_idx.pop()
continue
C[i] -= 1
if C[i] == 1:
dup_idx.pop()
curr += 1
ans = curr + (M-curr+1)//2
print(ans)
def __starting_point():
for __ in [0]*int(input()):
main()
__starting_point() | from itertools import groupby
def main():
N = int(input())
S = input()
C = [len(list(x[1])) for x in groupby(S)]
M = len(C)
dup_idx = []
for i, c in enumerate(C):
if c > 1:
dup_idx.append(i)
dup_idx.reverse()
curr = 0
while dup_idx:
i = dup_idx[-1]
if i < curr:
dup_idx.pop()
continue
C[i] -= 1
if C[i] == 1:
dup_idx.pop()
curr += 1
ans = curr + (M-curr+1)//2
print(ans)
def __starting_point():
for __ in [0]*int(input()):
main()
__starting_point() | train | APPS_structured |
In India, every individual is charged with income tax on the total income each year. This tax is applied to specific ranges of income, which are called income tax slabs. The slabs of income tax keep changing from year to year. This fiscal year (2020-21), the tax slabs and their respective tax rates are as follows:Total income (in rupees)Tax rateup to Rs. 250,0000%from Rs. 250,001 to Rs. 500,0005%from Rs. 500,001 to Rs. 750,00010%from Rs. 750,001 to Rs. 1,000,00015%from Rs. 1,000,001 to Rs. 1,250,00020%from Rs. 1,250,001 to Rs. 1,500,00025%above Rs. 1,500,00030%
See the sample explanation for details on how the income tax is calculated.
You are given Chef's total income: $N$ rupees (Rs.). Find his net income. The net income is calculated by subtracting the total tax (also called tax reduction) from the total income. Note that you do not need to worry about any other kind of tax reductions, only the one described above.
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first and only line of each test case contains a single integer $N$.
-----Output-----
For each test case, print a single line containing one integer — Chef's net income.
-----Constraints-----
- $1 \le T \le 10^3$
- $0 \le N \le 10^7$
- $N$ is a multiple of $100$
-----Example Input-----
2
600000
250000
-----Example Output-----
577500
250000
-----Explanation-----
Example case 1: We know that the total income is Rs. $6$ lakh ($1$ lakh rupees = $10^5$ rupees). The total tax for each slab is calculated as follows:
- Up to $2.5$ lakh, the tax is Rs. $0$, since the tax rate is $0$ percent.
- From above Rs. $2.5$ lakh to Rs. $5$ lakh, the tax rate is $5$ percent. Therefore, this tax is $0.05 \cdot (500,000-250,000)$, which is Rs. $12,500$.
- From above Rs. $5$ lakh to Rs. $6$ lakh, the tax rate is $10$ percent. Therefore, this tax is $0.10 \cdot (600,000-500,000)$, which is Rs. $10,000$.
- Summing them up, we get that the total tax on Chef's whole income is Rs. $22,500$. Since the net income is the total income minus the tax reduction, it is Rs. $600,000$ minus Rs. $22,500$, which is Rs. $577,500$.
Example case 2: For income up to Rs. $2.5$ lakh, we have no tax, so the net income is the same as the total income: Rs. $2.5$ lakh. | for _ in range(int(input())):
n=int(input())
tax=0
if n<=250000:
tax = n*0
elif n>250000 and n<=500000:
tax = (n-250000)*0.05
elif n>500000 and n<=750000:
tax = ((n-500000)*0.10)+12500
elif n>750000 and n<=1000000:
tax = ((n-750000)*0.15)+37500
elif n>1000000 and n<=1250000:
tax = ((n-1000000)*0.20)+75000
elif n>1250000 and n<=1500000:
tax = ((n-1250000)*0.25)+125000
elif n>1500000:
tax = ((n-1500000)*0.30)+187500
print(int(n-tax)) | for _ in range(int(input())):
n=int(input())
tax=0
if n<=250000:
tax = n*0
elif n>250000 and n<=500000:
tax = (n-250000)*0.05
elif n>500000 and n<=750000:
tax = ((n-500000)*0.10)+12500
elif n>750000 and n<=1000000:
tax = ((n-750000)*0.15)+37500
elif n>1000000 and n<=1250000:
tax = ((n-1000000)*0.20)+75000
elif n>1250000 and n<=1500000:
tax = ((n-1250000)*0.25)+125000
elif n>1500000:
tax = ((n-1500000)*0.30)+187500
print(int(n-tax)) | train | APPS_structured |
You are given a prime number $p$, $n$ integers $a_1, a_2, \ldots, a_n$, and an integer $k$.
Find the number of pairs of indexes $(i, j)$ ($1 \le i < j \le n$) for which $(a_i + a_j)(a_i^2 + a_j^2) \equiv k \bmod p$.
-----Input-----
The first line contains integers $n, p, k$ ($2 \le n \le 3 \cdot 10^5$, $2 \le p \le 10^9$, $0 \le k \le p-1$). $p$ is guaranteed to be prime.
The second line contains $n$ integers $a_1, a_2, \ldots, a_n$ ($0 \le a_i \le p-1$). It is guaranteed that all elements are different.
-----Output-----
Output a single integer — answer to the problem.
-----Examples-----
Input
3 3 0
0 1 2
Output
1
Input
6 7 2
1 2 3 4 5 6
Output
3
-----Note-----
In the first example:
$(0+1)(0^2 + 1^2) = 1 \equiv 1 \bmod 3$.
$(0+2)(0^2 + 2^2) = 8 \equiv 2 \bmod 3$.
$(1+2)(1^2 + 2^2) = 15 \equiv 0 \bmod 3$.
So only $1$ pair satisfies the condition.
In the second example, there are $3$ such pairs: $(1, 5)$, $(2, 3)$, $(4, 6)$. | from sys import stdin
n,p,k=list(map(int,stdin.readline().strip().split()))
s=list(map(int,stdin.readline().strip().split()))
st=set()
d=dict()
for i in s:
x=(i**4-i*k)%p
if x in st:
d[x]+=1
else:
st.add(x)
d[x]=1
s.sort()
ans=0
for i in s:
x=(i**4-i*k)%p
if x in st:
ans+=(d[x]-1)*d[x]//2
d[x]=0
print(ans)
| from sys import stdin
n,p,k=list(map(int,stdin.readline().strip().split()))
s=list(map(int,stdin.readline().strip().split()))
st=set()
d=dict()
for i in s:
x=(i**4-i*k)%p
if x in st:
d[x]+=1
else:
st.add(x)
d[x]=1
s.sort()
ans=0
for i in s:
x=(i**4-i*k)%p
if x in st:
ans+=(d[x]-1)*d[x]//2
d[x]=0
print(ans)
| train | APPS_structured |
< PREVIOUS KATA
NEXT KATA >
###Task:
You have to write a function `pattern` which returns the following Pattern(See Examples) upto (3n-2) rows, where n is parameter.
* Note:`Returning` the pattern is not the same as `Printing` the pattern.
####Rules/Note:
* The pattern should be created using only unit digits.
* If `n < 1` then it should return "" i.e. empty string.
* `The length of each line is same`, and is equal to the length of longest line in the pattern i.e. `length = (3n-2)`.
* Range of Parameters (for the sake of CW Compiler) :
+ `n ∈ (-∞,50]`
###Examples:
+ pattern(5) :
11111
22222
33333
44444
1234555554321
1234555554321
1234555554321
1234555554321
1234555554321
44444
33333
22222
11111
+ pattern(11):
11111111111
22222222222
33333333333
44444444444
55555555555
66666666666
77777777777
88888888888
99999999999
00000000000
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
1234567890111111111110987654321
00000000000
99999999999
88888888888
77777777777
66666666666
55555555555
44444444444
33333333333
22222222222
11111111111
>>>LIST OF ALL MY KATAS<<< | def pattern(n):
lines = []
for p in range(1,n):
lines.append((str(p%10)*n).center(3*n-2))
for p in range(1,n+1):
lines.append(''.join([str(m%10) for m in range(1,n)] + [str(n%10) for m in range(n)] + [str(m%10) for m in range(1,n)[::-1]]))
for p in range(n-1,0,-1):
lines.append((str(p%10)*n).center(3*n-2))
return '\n'.join(lines) | def pattern(n):
lines = []
for p in range(1,n):
lines.append((str(p%10)*n).center(3*n-2))
for p in range(1,n+1):
lines.append(''.join([str(m%10) for m in range(1,n)] + [str(n%10) for m in range(n)] + [str(m%10) for m in range(1,n)[::-1]]))
for p in range(n-1,0,-1):
lines.append((str(p%10)*n).center(3*n-2))
return '\n'.join(lines) | train | APPS_structured |
Some integral numbers are odd. All are more odd, or less odd, than others.
Even numbers satisfy `n = 2m` ( with `m` also integral ) and we will ( completely arbitrarily ) think of odd numbers as `n = 2m + 1`.
Now, some odd numbers can be more odd than others: when for some `n`, `m` is more odd than for another's. Recursively. :]
Even numbers are always less odd than odd numbers, but they also can be more, or less, odd than other even numbers, by the same mechanism.
# Task
Given a _non-empty_ finite list of _unique_ integral ( not necessarily non-negative ) numbers, determine the number that is _odder than the rest_.
Given the constraints, there will always be exactly one such number.
# Examples
```python
oddest([1,2]) => 1
oddest([1,3]) => 3
oddest([1,5]) => 5
```
# Hint
Do you _really_ want one? Point or tap here. | from functools import cmp_to_key
def comp(x, y):
rx, ry = x&1, y&1
while rx == ry:
x, y = (x - rx) >> 1, (y - ry) >> 1
rx, ry = x&1, y&1
return rx > ry
def oddest(a):
return max(a, key=cmp_to_key(comp)) | from functools import cmp_to_key
def comp(x, y):
rx, ry = x&1, y&1
while rx == ry:
x, y = (x - rx) >> 1, (y - ry) >> 1
rx, ry = x&1, y&1
return rx > ry
def oddest(a):
return max(a, key=cmp_to_key(comp)) | train | APPS_structured |
In genetics, a sequence’s motif is a nucleotides (or amino-acid) sequence pattern. Sequence motifs have a biological significance. For more information you can take a look [here](https://en.wikipedia.org/wiki/Sequence_motif).
For this kata you need to complete the function `motif_locator`. This function receives 2 arguments - a sequence and a motif. Both arguments are strings.
You should return an array that contains all the start positions of the motif (in order). A sequence may contain 0 or more repetitions of the given motif. Note that the number of the first position is 1, not 0.
**Some examples:**
- For the `sequence` "ACGTGGGGACTAGGGG" and the `motif` "GGGG" the result should be [5, 13].
- For the `sequence` "ACCGTACCAAGGGACC" and the `motif` "AAT" the result should be []
- For the `sequence` "GGG" and the motif "GG" the result should be [1, 2]
**Note**: You can take a look to my others bio-info kata [here](http://www.codewars.com/users/nbeck/authored) | import re
def motif_locator(sequence, motif):
return [m.start() + 1 for m in re.finditer('{}(?={})'.format(motif[0], motif[1:]), sequence)] | import re
def motif_locator(sequence, motif):
return [m.start() + 1 for m in re.finditer('{}(?={})'.format(motif[0], motif[1:]), sequence)] | train | APPS_structured |
In this simple exercise, you will create a program that will take two lists of integers, ```a``` and ```b```. Each list will consist of 3 positive integers above 0, representing the dimensions of cuboids ```a``` and ```b```. You must find the difference of the cuboids' volumes regardless of which is bigger.
For example, if the parameters passed are ```([2, 2, 3], [5, 4, 1])```, the volume of ```a``` is 12 and the volume of ```b``` is 20. Therefore, the function should return ```8```.
Your function will be tested with pre-made examples as well as random ones.
**If you can, try writing it in one line of code.** | def find_difference(a, b):
total_a = 1
total_b = 1
for i in a:
total_a *= i
for c in b:
total_b *= c
return abs(total_a - total_b) | def find_difference(a, b):
total_a = 1
total_b = 1
for i in a:
total_a *= i
for c in b:
total_b *= c
return abs(total_a - total_b) | train | APPS_structured |
# Introduction:
Reversi is a game usually played by 2 people on a 8x8 board.
Here we're only going to consider a single 8x1 row.
Players take turns placing pieces, which are black on one side and white on the
other, onto the board with their colour facing up. If one or more of the
opponents pieces are sandwiched by the piece just played and another piece of
the current player's colour, the opponents pieces are flipped to the
current players colour.
Note that the flipping stops when the first piece of the player's colour is reached.
# Task:
Your task is to take an array of moves and convert this into a string
representing the state of the board after all those moves have been played.
# Input:
The input to your function will be an array of moves.
Moves are represented by integers from 0 to 7 corresponding to the 8 squares on the board.
Black plays first, and black and white alternate turns.
Input is guaranteed to be valid. (No duplicates, all moves in range, but array may be empty)
# Output:
8 character long string representing the final state of the board.
Use '*' for black and 'O' for white and '.' for empty.
# Examples:
```python
reversi_row([]) # '........'
reversi_row([3]) # '...*....'
reversi_row([3,4]) # '...*O...'
reversi_row([3,4,5]) # '...***..'
``` | def reversi_row(moves):
stones = [set(), set()]
for i, p in enumerate(moves):
curr, oppn = stones[i%2], stones[(i+1)%2]
curr.add(p)
l, r = p-1, p+1
while l in oppn: l-=1
while r in oppn: r+=1
flip = set(range(l+1 if l in curr else p+1, r if r in curr else p))
oppn -= flip
curr |= flip
return ''.join('*' if i in stones[0] else 'O' if i in stones[1] else '.' for i in range(8)) | def reversi_row(moves):
stones = [set(), set()]
for i, p in enumerate(moves):
curr, oppn = stones[i%2], stones[(i+1)%2]
curr.add(p)
l, r = p-1, p+1
while l in oppn: l-=1
while r in oppn: r+=1
flip = set(range(l+1 if l in curr else p+1, r if r in curr else p))
oppn -= flip
curr |= flip
return ''.join('*' if i in stones[0] else 'O' if i in stones[1] else '.' for i in range(8)) | train | APPS_structured |
There are two lists of different length. The first one consists of keys, the second one consists of values. Write a function ```createDict(keys, values)``` that returns a dictionary created from keys and values. If there are not enough values, the rest of keys should have a ```None``` value. If there not enough keys, just ignore the rest of values.
Example 1:
```python
keys = ['a', 'b', 'c', 'd']
values = [1, 2, 3]
createDict(keys, values) # returns {'a': 1, 'b': 2, 'c': 3, 'd': None}
```
Example 2:
```python
keys = ['a', 'b', 'c']
values = [1, 2, 3, 4]
createDict(keys, values) # returns {'a': 1, 'b': 2, 'c': 3}
``` | def createDict(keys, values):
return dict(zip(keys, values + [None]*(len(keys) - len(values)))) | def createDict(keys, values):
return dict(zip(keys, values + [None]*(len(keys) - len(values)))) | train | APPS_structured |
Given an array of integers arr. Return the number of sub-arrays with odd sum.
As the answer may grow large, the answer must be computed modulo 10^9 + 7.
Example 1:
Input: arr = [1,3,5]
Output: 4
Explanation: All sub-arrays are [[1],[1,3],[1,3,5],[3],[3,5],[5]]
All sub-arrays sum are [1,4,9,3,8,5].
Odd sums are [1,9,3,5] so the answer is 4.
Example 2:
Input: arr = [2,4,6]
Output: 0
Explanation: All sub-arrays are [[2],[2,4],[2,4,6],[4],[4,6],[6]]
All sub-arrays sum are [2,6,12,4,10,6].
All sub-arrays have even sum and the answer is 0.
Example 3:
Input: arr = [1,2,3,4,5,6,7]
Output: 16
Example 4:
Input: arr = [100,100,99,99]
Output: 4
Example 5:
Input: arr = [7]
Output: 1
Constraints:
1 <= arr.length <= 10^5
1 <= arr[i] <= 100 | class Solution:
def numOfSubarrays(self, arr: List[int]) -> int:
dp = [[0, 0]]
ans = 0
for i in arr:
if i % 2 == 0:
dp.append([dp[-1][0] + 1, dp[-1][1]])
else:
dp.append([dp[-1][1], dp[-1][0] + 1])
ans += dp[-1][1]
return int(ans % (1e9 + 7)) | class Solution:
def numOfSubarrays(self, arr: List[int]) -> int:
dp = [[0, 0]]
ans = 0
for i in arr:
if i % 2 == 0:
dp.append([dp[-1][0] + 1, dp[-1][1]])
else:
dp.append([dp[-1][1], dp[-1][0] + 1])
ans += dp[-1][1]
return int(ans % (1e9 + 7)) | train | APPS_structured |
One day, Chef prepared D brand new dishes. He named the i-th dish by a string Si. After the cooking, he decided to categorize each of these D dishes as special or not.
A dish Si is called special if it's name (i.e. the string Si) can be represented in the form of a double string by removing at most one (possibly zero) character from it's name from any position.
A string is called a double string if it can be represented as a concatenation of two identical, non-empty strings.
e.g. "abab" is a double string as it can be represented as "ab" + "ab" where + operation denotes concatenation.
Similarly, "aa", "abcabc" are double strings whereas "a", "abba", "abc" are not.
-----Input-----
- First line of the input contains an integer D denoting the number of dishes prepared by Chef on that day.
- Each of the next D lines will contain description of a dish.
- The i-th line contains the name of i-th dish Si.
-----Output-----
For each of the D dishes, print a single line containing "YES" or "NO" (without quotes) denoting whether the dish can be called as a special or not.
-----Constraints-----
- 1 ≤ D ≤ 106
- 1 ≤ |Si| ≤ 106.
- Each character of string Si will be lower case English alphabet (i.e. from 'a' to 'z').
-----Subtasks-----
Subtask #1 : (20 points)
- Sum of |Si| in an input file doesn't exceed 2 * 103
Subtask 2 : (80 points)
- Sum of |Si| in an input file doesn't exceed 2 * 106
-----Example-----
Input:
3
aba
abac
abcd
Output:
YES
NO
NO
-----Explanation-----
Example case 1.
We can remove the character at position 1 (0-based index) to get "aa" which is a double string. Hence, it is a special dish.
Example case 2.
It is not possible to remove the character at any of the position to get the double string. Hence, it is not a special dish. | import sys
n = int(input())
for i in range(n):
s = input()
m = len(s)
if (m == 1):
sys.stdout.write('NO\n')
elif (m % 2 != 0):
#check left half
i = 0
j = int(m/2)+1
flag = False
Flag = True
while (j < m):
#print(i,j)
if (s[i] != s[j]):
if (not flag):
flag = True
i += 1
else:
Flag = False
break
else:
i += 1
j += 1
if (not Flag):
#check right half
i = 0
j = int(m/2)
flag = False
Flag = True
while (j < m):
#print(i,j)
if (s[i] != s[j]):
if (not flag):
flag = True
j += 1
else:
Flag = False
break
else:
i += 1
j += 1
if (Flag):
sys.stdout.write('YES\n')
else:
sys.stdout.write('NO\n')
else:
sys.stdout.write('YES\n')
else:
if (s[:int(m/2)] == s[int(m/2):]):
sys.stdout.write('YES\n')
else:
sys.stdout.write('NO\n') | import sys
n = int(input())
for i in range(n):
s = input()
m = len(s)
if (m == 1):
sys.stdout.write('NO\n')
elif (m % 2 != 0):
#check left half
i = 0
j = int(m/2)+1
flag = False
Flag = True
while (j < m):
#print(i,j)
if (s[i] != s[j]):
if (not flag):
flag = True
i += 1
else:
Flag = False
break
else:
i += 1
j += 1
if (not Flag):
#check right half
i = 0
j = int(m/2)
flag = False
Flag = True
while (j < m):
#print(i,j)
if (s[i] != s[j]):
if (not flag):
flag = True
j += 1
else:
Flag = False
break
else:
i += 1
j += 1
if (Flag):
sys.stdout.write('YES\n')
else:
sys.stdout.write('NO\n')
else:
sys.stdout.write('YES\n')
else:
if (s[:int(m/2)] == s[int(m/2):]):
sys.stdout.write('YES\n')
else:
sys.stdout.write('NO\n') | train | APPS_structured |
In computing, there are two primary byte order formats: big-endian and little-endian. Big-endian is used primarily for networking (e.g., IP addresses are transmitted in big-endian) whereas little-endian is used mainly by computers with microprocessors.
Here is an example (using 32-bit integers in hex format):
Little-Endian: 00 6D F4 C9 = 7,206,089
Big-Endian: C9 F4 6D 00 = 3,388,239,104
Your job is to write a function that switches the byte order of a given integer. The function should take an integer n for the first argument, and the bit-size of the integer for the second argument. The bit size must be a power of 2 greater than or equal to 8. Your function should return a None value if the integer is negative, if the specified bit size is not a power of 2 that is 8 or larger, or if the integer is larger than the specified bit size can handle. In this kata, assume that all integers are unsigned (non-negative) and that all input arguments are integers (no floats, strings, None/nil values, etc.). Remember that you will need to account for padding of null (00) bytes.
Hint: bitwise operators are very helpful! :) | # Switch the endianness of integer n
# Assume 64-bit unsigned integers
import re
import math
def switch_endian(n, bits):
number_in_hex = hex(n)
length = len(number_in_hex)-2
result = int(bits/4)
x = math.log(bits, 2).is_integer()
if x == False:
return None
if length > result:
return None
if length <= result:
diff = result-length
number_in_hex = number_in_hex.replace('0x','0'*diff)
res_list = re.findall('[\da-z]{2}[^\da-z]*', number_in_hex)
res_list = res_list[::-1]
number_in_hex = ''.join(res_list)
return(int('0x'+number_in_hex, 0))
| # Switch the endianness of integer n
# Assume 64-bit unsigned integers
import re
import math
def switch_endian(n, bits):
number_in_hex = hex(n)
length = len(number_in_hex)-2
result = int(bits/4)
x = math.log(bits, 2).is_integer()
if x == False:
return None
if length > result:
return None
if length <= result:
diff = result-length
number_in_hex = number_in_hex.replace('0x','0'*diff)
res_list = re.findall('[\da-z]{2}[^\da-z]*', number_in_hex)
res_list = res_list[::-1]
number_in_hex = ''.join(res_list)
return(int('0x'+number_in_hex, 0))
| train | APPS_structured |
Roger recently built a circular race track with length K$K$. After hosting a few races, he realised that people do not come there to see the race itself, they come to see racers crash into each other (what's wrong with our generation…). After this realisation, Roger decided to hold a different kind of "races": he hired N$N$ racers (numbered 1$1$ through N$N$) whose task is to crash into each other.
At the beginning, for each valid i$i$, the i$i$-th racer is Xi$X_i$ metres away from the starting point of the track (measured in the clockwise direction) and driving in the direction Di$D_i$ (clockwise or counterclockwise). All racers move with the constant speed 1$1$ metre per second. The lengths of cars are negligible, but the track is only wide enough for one car, so whenever two cars have the same position along the track, they crash into each other and the direction of movement of each of these cars changes (from clockwise to counterclockwise and vice versa). The cars do not change the directions of their movement otherwise.
Since crashes reduce the lifetime of the racing cars, Roger sometimes wonders how many crashes happen. You should answer Q$Q$ queries. In each query, you are given an integer T$T$ and you should find the number of crashes that happen until T$T$ seconds have passed (inclusive).
-----Input-----
- The first line of the input contains three space-separated integers N$N$, Q$Q$ and K$K$.
- N$N$ lines follow. For each i$i$ (1≤i≤N$1 \le i \le N$), the i$i$-th of these lines contains two space-separated integers Di$D_i$ and Xi$X_i$, where Di=1$D_i = 1$ and Di=2$D_i = 2$ denote the clockwise and counterclockwise directions respectively.
- Each of the next Q$Q$ lines contain a single integer T$T$ describing a query.
-----Output-----
For each query, print a single line containing one integer — the number of crashes.
-----Constraints-----
- 1≤N≤105$1 \le N \le 10^5$
- 1≤Q≤1,000$1 \le Q \le 1,000$
- 1≤K≤1012$1 \le K \le 10^{12}$
- 1≤Di≤2$1 \le D_i \le 2$ for each valid i$i$
- 0≤Xi≤K−1$0 \le X_i \le K - 1$ for each valid i$i$
- X1,X2,…,XN$X_1, X_2, \ldots, X_N$ are pairwise distinct
- 0≤T≤1012$0 \le T \le 10^{12}$
-----Example Input-----
5 3 11
1 3
1 10
2 4
2 7
2 0
3
8
100
-----Example Output-----
4
10
110 | import numpy as np
from numba import njit
i8 = np.int64
@njit
def solve(a, b, t, K, N):
t1 = t // K
d = t % K * 2
# b が a から a + d の位置にあれば衝突する
x = 0
y = 0
ans = 0
for c in a:
while b[x] < c:
x += 1
while b[y] <= c + d:
y += 1
ans += y - x
ans += t1 * len(a) * (N - len(a)) * 2
return ans
def set_ini(DX, K):
a = DX[1][DX[0] == 1]
a = np.sort(a)
b = DX[1][DX[0] == 2]
b = np.sort(b)
b = np.hstack((b, b + K, b + 2 * K, [3 * K]))
return a, b
def main():
f = open('/dev/stdin', 'rb')
vin = np.fromstring(f.read(), i8, sep=' ')
N, Q, K = vin[0:3]
head = 3
DX = vin[head:head + 2*N].reshape(-1, 2).T
a, b = set_ini(DX, K)
head += 2 * N
T = vin[head: head + Q]
for t in T:
print(solve(a, b, t, K, N))
def __starting_point():
main()
__starting_point() | import numpy as np
from numba import njit
i8 = np.int64
@njit
def solve(a, b, t, K, N):
t1 = t // K
d = t % K * 2
# b が a から a + d の位置にあれば衝突する
x = 0
y = 0
ans = 0
for c in a:
while b[x] < c:
x += 1
while b[y] <= c + d:
y += 1
ans += y - x
ans += t1 * len(a) * (N - len(a)) * 2
return ans
def set_ini(DX, K):
a = DX[1][DX[0] == 1]
a = np.sort(a)
b = DX[1][DX[0] == 2]
b = np.sort(b)
b = np.hstack((b, b + K, b + 2 * K, [3 * K]))
return a, b
def main():
f = open('/dev/stdin', 'rb')
vin = np.fromstring(f.read(), i8, sep=' ')
N, Q, K = vin[0:3]
head = 3
DX = vin[head:head + 2*N].reshape(-1, 2).T
a, b = set_ini(DX, K)
head += 2 * N
T = vin[head: head + Q]
for t in T:
print(solve(a, b, t, K, N))
def __starting_point():
main()
__starting_point() | train | APPS_structured |
Introduction
The GADERYPOLUKI is a simple substitution cypher used in scouting to encrypt messages. The encryption is based on short, easy to remember key. The key is written as paired letters, which are in the cipher simple replacement.
The most frequently used key is "GA-DE-RY-PO-LU-KI".
```
G => A
g => a
a => g
A => G
D => E
etc.
```
The letters, which are not on the list of substitutes, stays in the encrypted text without changes.
Other keys often used by Scouts:
```
PO-LI-TY-KA-RE-NU
KA-CE-MI-NU-TO-WY
KO-NI-EC-MA-TU-RY
ZA-RE-WY-BU-HO-KI
BA-WO-LE-TY-KI-JU
RE-GU-LA-MI-NO-WY
```
Task
Your task is to help scouts to encrypt and decrypt thier messages.
Write the `Encode` and `Decode` functions.
Input/Output
The function should have two parameters.
The `message` input string consists of lowercase and uperrcase characters and whitespace character.
The `key` input string consists of only lowercase characters.
The substitution has to be case-sensitive.
Example
# GADERYPOLUKI collection
GADERYPOLUKI cypher vol 1
GADERYPOLUKI cypher vol 2
GADERYPOLUKI cypher vol 3 - Missing Key
GADERYPOLUKI cypher vol 4 - Missing key madness | def encode(message, key):
x, y = key[::2] + key[::2].upper(), key[1::2] + key[1::2].upper()
return message.translate(str.maketrans(x + y, y + x))
decode = encode | def encode(message, key):
x, y = key[::2] + key[::2].upper(), key[1::2] + key[1::2].upper()
return message.translate(str.maketrans(x + y, y + x))
decode = encode | train | APPS_structured |
Bob is preparing to pass IQ test. The most frequent task in this test is `to find out which one of the given numbers differs from the others`. Bob observed that one number usually differs from the others in **evenness**. Help Bob — to check his answers, he needs a program that among the given numbers finds one that is different in evenness, and return a position of this number.
`!` Keep in mind that your task is to help Bob solve a `real IQ test`, which means indexes of the elements start from `1 (not 0)`
##Examples :
```python
iq_test("2 4 7 8 10") => 3 // Third number is odd, while the rest of the numbers are even
iq_test("1 2 1 1") => 2 // Second number is even, while the rest of the numbers are odd
``` | def iq_test(n):
n = [int(i)%2 for i in n.split()]
if n.count(0)>1:
return n.index(1)+1
else:
return n.index(0)+1
| def iq_test(n):
n = [int(i)%2 for i in n.split()]
if n.count(0)>1:
return n.index(1)+1
else:
return n.index(0)+1
| train | APPS_structured |
Chefina is always interested to play with string. But due to exam pressure she has no time to solve a string problem. She wants your help. Can you help her to solve that problem?
You are given a string. You have to find out the $Wonder$ $Sum$ of the string. $Wonder$ $Sum$ of a string is defined as the sum of the value of each character of the string.
The value of each character means:
- If the string is started with "a" , then the value of each character of the string is like "a"=100, "b"=101, "c"="102" ………"z"=125.
- If the string is started with "z" , then the value of each character of the string is like "a"=2600, "b"=2601, "c"="2602" ………"z"=2625.
Since even the $Wonder$ $Sum$ can be large, output $Wonder$ $Sum$ modulo ($10^9 + 7$).
-----Input:-----
- First line will contain $T$, number of testcases. Then the testcases follow.
- Each testcase contains of a single line of input, a string $S$ with lower case alphabet
only.
-----Output:-----
For each testcase, output in a single line integer i.e. $Wonder$ $Sum$ modulo ($10^9 + 7$).
-----Constraints-----
- $1 \leq T \leq 1000$
- $1 \leq |S| \leq 10^5$
-----Sample Input:-----
$2$$cab$
$sdef$
-----Sample Output:-----
$903$
$7630$
-----EXPLANATION:-----
i) For the first test case, since the string is started with "$c$", so output is ($302$+$300$+$301$)=$903$
ii)For the second test case, since the string is started with "$s$", so output is ($1918$+$1903$+$1904$+$1905$)=$7630$ | from sys import stdin
MODULO = 1000000007
for testcase in range(int(stdin.readline().strip())) :
s = stdin.readline().strip()
s0 = (ord(s[0]) - 96)*100
wsum = sum([ord(ch)-97 for ch in s]) + s0*len(s)
print(wsum%MODULO)
| from sys import stdin
MODULO = 1000000007
for testcase in range(int(stdin.readline().strip())) :
s = stdin.readline().strip()
s0 = (ord(s[0]) - 96)*100
wsum = sum([ord(ch)-97 for ch in s]) + s0*len(s)
print(wsum%MODULO)
| train | APPS_structured |
# Task
Given an integer array `arr`. Your task is to remove one element, maximize the product of elements.
The result is the element which should be removed. If more than one valid results exist, return the smallest one.
# Input/Output
`[input]` integer array `arr`
non-empty unsorted integer array. It contains positive integer, negative integer or zero.
`3 ≤ arr.length ≤ 15`
`-10 ≤ arr[i] ≤ 10`
`[output]` an integer
The element that should be removed.
# Example
For `arr = [1, 2, 3]`, the output should be `1`.
For `arr = [-1, 2, -3]`, the output should be `2`.
For `arr = [-1, -2, -3]`, the output should be `-1`.
For `arr = [-1, -2, -3, -4]`, the output should be `-4`. | from operator import mul
from functools import reduce
def maximum_product(arr):
prod_dct = { x: reduce(mul, arr[:i]+arr[i+1:], 1) for i,x in enumerate(arr)}
return max(arr, key=lambda x:(prod_dct[x],-x)) | from operator import mul
from functools import reduce
def maximum_product(arr):
prod_dct = { x: reduce(mul, arr[:i]+arr[i+1:], 1) for i,x in enumerate(arr)}
return max(arr, key=lambda x:(prod_dct[x],-x)) | train | APPS_structured |
This problem is actually a subproblem of problem G from the same contest.
There are $n$ candies in a candy box. The type of the $i$-th candy is $a_i$ ($1 \le a_i \le n$).
You have to prepare a gift using some of these candies with the following restriction: the numbers of candies of each type presented in a gift should be all distinct (i. e. for example, a gift having two candies of type $1$ and two candies of type $2$ is bad).
It is possible that multiple types of candies are completely absent from the gift. It is also possible that not all candies of some types will be taken to a gift.
Your task is to find out the maximum possible size of the single gift you can prepare using the candies you have.
You have to answer $q$ independent queries.
If you are Python programmer, consider using PyPy instead of Python when you submit your code.
-----Input-----
The first line of the input contains one integer $q$ ($1 \le q \le 2 \cdot 10^5$) — the number of queries. Each query is represented by two lines.
The first line of each query contains one integer $n$ ($1 \le n \le 2 \cdot 10^5$) — the number of candies.
The second line of each query contains $n$ integers $a_1, a_2, \dots, a_n$ ($1 \le a_i \le n$), where $a_i$ is the type of the $i$-th candy in the box.
It is guaranteed that the sum of $n$ over all queries does not exceed $2 \cdot 10^5$.
-----Output-----
For each query print one integer — the maximum possible size of the single gift you can compose using candies you got in this query with the restriction described in the problem statement.
-----Example-----
Input
3
8
1 4 8 4 5 6 3 8
16
2 1 3 3 4 3 4 4 1 3 2 2 2 4 1 1
9
2 2 4 4 4 7 7 7 7
Output
3
10
9
-----Note-----
In the first query, you can prepare a gift with two candies of type $8$ and one candy of type $5$, totalling to $3$ candies.
Note that this is not the only possible solution — taking two candies of type $4$ and one candy of type $6$ is also valid. | q = int(input())
for _ in range(q):
n = int(input())
a = sorted(list(map(int, input().split())))
s = []
last = a[0]
count = 1
for i in range(1, n):
if a[i] != last:
s.append(count)
last = a[i]
count = 0
count += 1
s.append(count)
s.sort()
answer = s[-1]
for i in range(len(s) - 2, -1, -1):
if s[i] >= s[i + 1]:
s[i] = s[i + 1] - 1
if not s[i]:
break
answer += s[i]
print(answer)
| q = int(input())
for _ in range(q):
n = int(input())
a = sorted(list(map(int, input().split())))
s = []
last = a[0]
count = 1
for i in range(1, n):
if a[i] != last:
s.append(count)
last = a[i]
count = 0
count += 1
s.append(count)
s.sort()
answer = s[-1]
for i in range(len(s) - 2, -1, -1):
if s[i] >= s[i + 1]:
s[i] = s[i + 1] - 1
if not s[i]:
break
answer += s[i]
print(answer)
| train | APPS_structured |
Given a list of integers values, your job is to return the sum of the values; however, if the same integer value appears multiple times in the list, you can only count it once in your sum.
For example:
```python
[ 1, 2, 3] ==> 6
[ 1, 3, 8, 1, 8] ==> 12
[ -1, -1, 5, 2, -7] ==> -1
[] ==> None
```
Good Luck! | def unique_sum(lst):
return sum(set(lst)) if len(lst) > 0 else None | def unique_sum(lst):
return sum(set(lst)) if len(lst) > 0 else None | train | APPS_structured |
Write a function which converts the input string to uppercase.
~~~if:bf
For BF all inputs end with \0, all inputs are lowercases and there is no space between.
~~~ | def make_upper_case(s):
alphabet = {}
counter = 65
for i in range(97, 123):
alphabet[chr(i)] = chr(counter)
counter = counter + 1
sum = ""
for i in s:
for j in alphabet:
if i == j:
sum = sum + alphabet[j]
i = ""
break
sum = sum + i
return sum | def make_upper_case(s):
alphabet = {}
counter = 65
for i in range(97, 123):
alphabet[chr(i)] = chr(counter)
counter = counter + 1
sum = ""
for i in s:
for j in alphabet:
if i == j:
sum = sum + alphabet[j]
i = ""
break
sum = sum + i
return sum | train | APPS_structured |
Write a function that determines whether a string is a valid guess in a Boggle board, as per the rules of Boggle. A Boggle board is a 2D array of individual characters, e.g.:
```python
[ ["I","L","A","W"],
["B","N","G","E"],
["I","U","A","O"],
["A","S","R","L"] ]
```
Valid guesses are strings which can be formed by connecting adjacent cells (horizontally, vertically, or diagonally) without re-using any previously used cells.
For example, in the above board "BINGO", "LINGO", and "ILNBIA" would all be valid guesses, while "BUNGIE", "BINS", and "SINUS" would not.
Your function should take two arguments (a 2D array and a string) and return true or false depending on whether the string is found in the array as per Boggle rules.
Test cases will provide various array and string sizes (squared arrays up to 150x150 and strings up to 150 uppercase letters). You do not have to check whether the string is a real word or not, only if it's a valid guess. | def find_word(board, word, cursor = None):
if not word:
return True
workboard = [row[:] for row in board]
sy, sx, ey, ex = 0, 0, len(board), len(board[0])
if cursor:
cx, cy = cursor
workboard[cy][cx] = "-"
sy = max(sy, cy - 1)
sx = max(sx, cx - 1)
ey = min(ey, cy + 2)
ex = min(ex, cx + 2)
for y, row in enumerate(workboard[:ey][sy:]):
for x, cell in enumerate( row[:ex][sx:]):
if cell == word[0] and find_word(workboard, word[1:], (x + sx, y + sy)):
return True
return False | def find_word(board, word, cursor = None):
if not word:
return True
workboard = [row[:] for row in board]
sy, sx, ey, ex = 0, 0, len(board), len(board[0])
if cursor:
cx, cy = cursor
workboard[cy][cx] = "-"
sy = max(sy, cy - 1)
sx = max(sx, cx - 1)
ey = min(ey, cy + 2)
ex = min(ex, cx + 2)
for y, row in enumerate(workboard[:ey][sy:]):
for x, cell in enumerate( row[:ex][sx:]):
if cell == word[0] and find_word(workboard, word[1:], (x + sx, y + sy)):
return True
return False | train | APPS_structured |
In an infinite array with two rows, the numbers in the top row are denoted
`. . . , A[−2], A[−1], A[0], A[1], A[2], . . .`
and the numbers in the bottom row are denoted
`. . . , B[−2], B[−1], B[0], B[1], B[2], . . .`
For each integer `k`, the entry `A[k]` is directly above
the entry `B[k]` in the array, as shown:
...|A[-2]|A[-1]|A[0]|A[1]|A[2]|...
...|B[-2]|B[-1]|B[0]|B[1]|B[2]|...
For each integer `k`, `A[k]` is the average of the entry to its left, the entry to its right,
and the entry below it; similarly, each entry `B[k]` is the average of the entry to its
left, the entry to its right, and the entry above it.
Given `A[0], A[1], A[2] and A[3]`, determine the value of `A[n]`. (Where range of n is -1000 Inputs and Outputs in BigInt!**
Adapted from 2018 Euclid Mathematics Contest.
https://www.cemc.uwaterloo.ca/contests/past_contests/2018/2018EuclidContest.pdf | def find_a(array, n):
if 0 <= n <= 3: return array[n]
a0 = array[0]
a1 = array[1]
a2 = array[2]
a3 = array[3]
b1 = 3*a1 - a0 - a2
b2 = 3*a2 - a1 - a3
b3 = 3*b2 - b1 - a2
b0 = 3*b1 - b2 - a1
if n > 3:
for k in range(4, n+1, 1):
an = 3*a3 - a2 - b3
bn = 3*b3 - b2 - a3
a2, a3 = a3, an
b2, b3 = b3, bn
else:
for k in range(-1, n-1, -1):
an = 3*a0 - a1 - b0
bn = 3*b0 - b1 - a0
a0, a1 = an, a0
b0, b1 = bn, b0
return an | def find_a(array, n):
if 0 <= n <= 3: return array[n]
a0 = array[0]
a1 = array[1]
a2 = array[2]
a3 = array[3]
b1 = 3*a1 - a0 - a2
b2 = 3*a2 - a1 - a3
b3 = 3*b2 - b1 - a2
b0 = 3*b1 - b2 - a1
if n > 3:
for k in range(4, n+1, 1):
an = 3*a3 - a2 - b3
bn = 3*b3 - b2 - a3
a2, a3 = a3, an
b2, b3 = b3, bn
else:
for k in range(-1, n-1, -1):
an = 3*a0 - a1 - b0
bn = 3*b0 - b1 - a0
a0, a1 = an, a0
b0, b1 = bn, b0
return an | train | APPS_structured |
Given an array of integers arr and two integers k and threshold.
Return the number of sub-arrays of size k and average greater than or equal to threshold.
Example 1:
Input: arr = [2,2,2,2,5,5,5,8], k = 3, threshold = 4
Output: 3
Explanation: Sub-arrays [2,5,5],[5,5,5] and [5,5,8] have averages 4, 5 and 6 respectively. All other sub-arrays of size 3 have averages less than 4 (the threshold).
Example 2:
Input: arr = [1,1,1,1,1], k = 1, threshold = 0
Output: 5
Example 3:
Input: arr = [11,13,17,23,29,31,7,5,2,3], k = 3, threshold = 5
Output: 6
Explanation: The first 6 sub-arrays of size 3 have averages greater than 5. Note that averages are not integers.
Example 4:
Input: arr = [7,7,7,7,7,7,7], k = 7, threshold = 7
Output: 1
Example 5:
Input: arr = [4,4,4,4], k = 4, threshold = 1
Output: 1
Constraints:
1 <= arr.length <= 10^5
1 <= arr[i] <= 10^4
1 <= k <= arr.length
0 <= threshold <= 10^4 | class Solution:
def numOfSubarrays(self, arr: List[int], k: int, threshold: int) -> int:
ans = 0
total = 0
threshold = k*threshold
if len(arr) < k:
return 0
else:
#check the first k numbers
for i in range(k):
total += arr[i]
if total >= threshold:
ans += 1
#slide window to the right 1
i = k
while i < len(arr):
total += arr[i] - arr[i-k]
if total >= threshold:
ans += 1
i += 1
return ans | class Solution:
def numOfSubarrays(self, arr: List[int], k: int, threshold: int) -> int:
ans = 0
total = 0
threshold = k*threshold
if len(arr) < k:
return 0
else:
#check the first k numbers
for i in range(k):
total += arr[i]
if total >= threshold:
ans += 1
#slide window to the right 1
i = k
while i < len(arr):
total += arr[i] - arr[i-k]
if total >= threshold:
ans += 1
i += 1
return ans | train | APPS_structured |
You are given an undirected unweighted graph consisting of $n$ vertices and $m$ edges (which represents the map of Bertown) and the array of prices $p$ of length $m$. It is guaranteed that there is a path between each pair of vertices (districts).
Mike has planned a trip from the vertex (district) $a$ to the vertex (district) $b$ and then from the vertex (district) $b$ to the vertex (district) $c$. He can visit the same district twice or more. But there is one issue: authorities of the city want to set a price for using the road so if someone goes along the road then he should pay the price corresponding to this road (he pays each time he goes along the road). The list of prices that will be used $p$ is ready and they just want to distribute it between all roads in the town in such a way that each price from the array corresponds to exactly one road.
You are a good friend of Mike (and suddenly a mayor of Bertown) and want to help him to make his trip as cheap as possible. So, your task is to distribute prices between roads in such a way that if Mike chooses the optimal path then the price of the trip is the minimum possible. Note that you cannot rearrange prices after the start of the trip.
You have to answer $t$ independent test cases.
-----Input-----
The first line of the input contains one integer $t$ ($1 \le t \le 10^4$) — the number of test cases. Then $t$ test cases follow.
The first line of the test case contains five integers $n, m, a, b$ and $c$ ($2 \le n \le 2 \cdot 10^5$, $n-1 \le m \le min(\frac{n(n-1)}{2}, 2 \cdot 10^5)$, $1 \le a, b, c \le n$) — the number of vertices, the number of edges and districts in Mike's trip.
The second line of the test case contains $m$ integers $p_1, p_2, \dots, p_m$ ($1 \le p_i \le 10^9$), where $p_i$ is the $i$-th price from the array.
The following $m$ lines of the test case denote edges: edge $i$ is represented by a pair of integers $v_i$, $u_i$ ($1 \le v_i, u_i \le n$, $u_i \ne v_i$), which are the indices of vertices connected by the edge. There are no loops or multiple edges in the given graph, i. e. for each pair ($v_i, u_i$) there are no other pairs ($v_i, u_i$) or ($u_i, v_i$) in the array of edges, and for each pair $(v_i, u_i)$ the condition $v_i \ne u_i$ is satisfied. It is guaranteed that the given graph is connected.
It is guaranteed that the sum of $n$ (as well as the sum of $m$) does not exceed $2 \cdot 10^5$ ($\sum n \le 2 \cdot 10^5$, $\sum m \le 2 \cdot 10^5$).
-----Output-----
For each test case, print the answer — the minimum possible price of Mike's trip if you distribute prices between edges optimally.
-----Example-----
Input
2
4 3 2 3 4
1 2 3
1 2
1 3
1 4
7 9 1 5 7
2 10 4 8 5 6 7 3 3
1 2
1 3
1 4
3 2
3 5
4 2
5 6
1 7
6 7
Output
7
12
-----Note-----
One of the possible solution to the first test case of the example:
[Image]
One of the possible solution to the second test case of the example:
[Image] | from collections import deque
import sys
input = sys.stdin.readline
inf = int(1e9)
for i in range(int(input())):
n, m, a, b, c = list(map(int, input().split()))
a -= 1
b -= 1
c -= 1
cost = list(map(int, input().split()))
g = [[] for _ in range(n)]
for i in range(m):
a2, b2 = list(map(int, input().split()))
g[a2 - 1].append(b2 - 1)
g[b2 - 1].append(a2 - 1)
def bfs(st):
Q = deque(maxlen=n)
lv = [-1] * n
Q.append(st)
lv[st] = 0
while Q:
cur = Q.popleft()
for to in g[cur]:
if lv[to] == -1:
lv[to] = lv[cur] + 1
Q.append(to)
return lv
lvA = bfs(a)
lvB = bfs(b)
lvC = bfs(c)
# print(lvA, lvB, lvC)
ans = int(1e18)
cost.sort()
cost.insert(0, 0)
for i in range(1, len(cost)):
cost[i] += cost[i - 1]
# print(cost)
for i in range(n):
d1 = lvB[i]
d2 = lvA[i] + lvC[i]
if d1 + d2 >= len(cost):
continue
# print(f'i={i}, d1={d1}, d2={d2}')
ans = min(ans, cost[d1] * 2 + cost[d1 + d2] - cost[d1])
print(ans)
| from collections import deque
import sys
input = sys.stdin.readline
inf = int(1e9)
for i in range(int(input())):
n, m, a, b, c = list(map(int, input().split()))
a -= 1
b -= 1
c -= 1
cost = list(map(int, input().split()))
g = [[] for _ in range(n)]
for i in range(m):
a2, b2 = list(map(int, input().split()))
g[a2 - 1].append(b2 - 1)
g[b2 - 1].append(a2 - 1)
def bfs(st):
Q = deque(maxlen=n)
lv = [-1] * n
Q.append(st)
lv[st] = 0
while Q:
cur = Q.popleft()
for to in g[cur]:
if lv[to] == -1:
lv[to] = lv[cur] + 1
Q.append(to)
return lv
lvA = bfs(a)
lvB = bfs(b)
lvC = bfs(c)
# print(lvA, lvB, lvC)
ans = int(1e18)
cost.sort()
cost.insert(0, 0)
for i in range(1, len(cost)):
cost[i] += cost[i - 1]
# print(cost)
for i in range(n):
d1 = lvB[i]
d2 = lvA[i] + lvC[i]
if d1 + d2 >= len(cost):
continue
# print(f'i={i}, d1={d1}, d2={d2}')
ans = min(ans, cost[d1] * 2 + cost[d1 + d2] - cost[d1])
print(ans)
| train | APPS_structured |
Your task is to write a function that takes two or more objects and returns a new object which combines all the input objects.
All input object properties will have only numeric values. Objects are combined together so that the values of matching keys are added together.
An example:
```python
objA = { 'a': 10, 'b': 20, 'c': 30 }
objB = { 'a': 3, 'c': 6, 'd': 3 }
combine(objA, objB) # Returns { a: 13, b: 20, c: 36, d: 3 }
```
The combine function should be a good citizen, so should not mutate the input objects. | def combine(*bs):
c = {}
for b in bs:
for k, v in list(b.items()):
c[k] = v + c.get(k, 0)
return c
| def combine(*bs):
c = {}
for b in bs:
for k, v in list(b.items()):
c[k] = v + c.get(k, 0)
return c
| train | APPS_structured |
One of Timofey's birthday presents is a colourbook in a shape of an infinite plane. On the plane n rectangles with sides parallel to coordinate axes are situated. All sides of the rectangles have odd length. Rectangles cannot intersect, but they can touch each other.
Help Timofey to color his rectangles in 4 different colors in such a way that every two rectangles touching each other by side would have different color, or determine that it is impossible.
Two rectangles intersect if their intersection has positive area. Two rectangles touch by sides if there is a pair of sides such that their intersection has non-zero length [Image] The picture corresponds to the first example
-----Input-----
The first line contains single integer n (1 ≤ n ≤ 5·10^5) — the number of rectangles.
n lines follow. The i-th of these lines contains four integers x_1, y_1, x_2 and y_2 ( - 10^9 ≤ x_1 < x_2 ≤ 10^9, - 10^9 ≤ y_1 < y_2 ≤ 10^9), that means that points (x_1, y_1) and (x_2, y_2) are the coordinates of two opposite corners of the i-th rectangle.
It is guaranteed, that all sides of the rectangles have odd lengths and rectangles don't intersect each other.
-----Output-----
Print "NO" in the only line if it is impossible to color the rectangles in 4 different colors in such a way that every two rectangles touching each other by side would have different color.
Otherwise, print "YES" in the first line. Then print n lines, in the i-th of them print single integer c_{i} (1 ≤ c_{i} ≤ 4) — the color of i-th rectangle.
-----Example-----
Input
8
0 0 5 3
2 -1 5 0
-3 -4 2 -1
-1 -1 2 0
-3 0 0 5
5 2 10 3
7 -3 10 2
4 -2 7 -1
Output
YES
1
2
2
3
2
2
4
1 | n = int(input())
print('YES')
for _ in range(n):
x, y, *z = list(map(int, input().split()))
print((x & 1) * 2 + (y & 1) + 1) | n = int(input())
print('YES')
for _ in range(n):
x, y, *z = list(map(int, input().split()))
print((x & 1) * 2 + (y & 1) + 1) | train | APPS_structured |
The bustling town of Siruseri has just one sports stadium. There are a number of schools, colleges, sports associations, etc. that use this stadium as the venue for their sports events.
Anyone interested in using the stadium has to apply to the Manager of the stadium indicating both the starting date (a positive integer $S$) and the length of the sporting event in days (a positive integer $D$) they plan to organise. Since these requests could overlap it may not be possible to satisfy everyone. Also, there should be at least one gap day between any two approved events, so that the stadium can be cleaned.
It is the job of the Manager to decide who gets to use the stadium and who does not. The Manager, being a genial man, would like to keep as many organisations happy as possible and hence would like to allocate the stadium so that maximum number of events are held.
Suppose, for example, the Manager receives the following 4 requests:
$ $
Event No. Starting Date Length
1 2 5
2 9 7
3 15 6
4 9 3
$ $
He would allot the stadium to events $1$, $4$ and $3$. Event $1$ begins on day $2$ and ends on day $6$, event $4$ begins on day $9$ and ends on day $11$ and event $3$ begins on day $15$ and ends on day $20$. You can verify that it is not possible to schedule all the $4$ events (since events $2$ and $3$ overlap and only one of them can get to use the stadium).
Your task is to help the manager find the best possible allotment (i.e., the maximum number of events that can use the stadium).
-----Input:-----
The first line of the input will contain a single integer $N$ indicating the number of events for which the Manager has received a request. Lines $2,3,...,N+1$ describe the requirements of the $N$ events. Line $i+1$ contains two integer $S_i$ and $D_i$ indicating the starting date and the duration of event $i$.
-----Output:-----
Your output must consist of a single line containing a single integer $M$, indicating the maximum possible number of events that can use the stadium.
-----Constraints:-----
- $1 \leq N \leq 100000$.
- $1 \leq S_i \leq 1000000$.
- $1 \leq D_i \leq 1000$.
- $50 \%$ of test cases will also satisfy $1 \leq N \leq 10000$.
-----Sample input:-----
4
2 5
9 7
15 6
9 3
-----Sample output:-----
3 | arr=[]
for _ in range(int(input())) :
start,end=[int(x) for x in input().split()]
arr.append((start,start+end))
arr.sort()
ans=1
check=arr[0][1]
for i in range(1,len(arr)) :
if arr[i][0] > check :
ans+=1
check = arr[i][1]
print(ans)
| arr=[]
for _ in range(int(input())) :
start,end=[int(x) for x in input().split()]
arr.append((start,start+end))
arr.sort()
ans=1
check=arr[0][1]
for i in range(1,len(arr)) :
if arr[i][0] > check :
ans+=1
check = arr[i][1]
print(ans)
| train | APPS_structured |
Given an integer n, add a dot (".") as the thousands separator and return it in string format.
Example 1:
Input: n = 987
Output: "987"
Example 2:
Input: n = 1234
Output: "1.234"
Example 3:
Input: n = 123456789
Output: "123.456.789"
Example 4:
Input: n = 0
Output: "0"
Constraints:
0 <= n < 2^31 | class Solution:
def thousandSeparator(self, n: int) -> str:
n = list(str(n))
if len(n) > 3:
for i in range(len(n)-3,0,-3):
n.insert(i, '.')
return ''.join(n)
| class Solution:
def thousandSeparator(self, n: int) -> str:
n = list(str(n))
if len(n) > 3:
for i in range(len(n)-3,0,-3):
n.insert(i, '.')
return ''.join(n)
| train | APPS_structured |
Given an array nums and an integer target.
Return the maximum number of non-empty non-overlapping subarrays such that the sum of values in each subarray is equal to target.
Example 1:
Input: nums = [1,1,1,1,1], target = 2
Output: 2
Explanation: There are 2 non-overlapping subarrays [1,1,1,1,1] with sum equals to target(2).
Example 2:
Input: nums = [-1,3,5,1,4,2,-9], target = 6
Output: 2
Explanation: There are 3 subarrays with sum equal to 6.
([5,1], [4,2], [3,5,1,4,2,-9]) but only the first 2 are non-overlapping.
Example 3:
Input: nums = [-2,6,6,3,5,4,1,2,8], target = 10
Output: 3
Example 4:
Input: nums = [0,0,0], target = 0
Output: 3
Constraints:
1 <= nums.length <= 10^5
-10^4 <= nums[i] <= 10^4
0 <= target <= 10^6 | class Solution:
def maxNonOverlapping(self, nums: List[int], target: int) -> int:
ln = len(nums)
ret = 0
sums = {0 : 0}
dp = [0] * (ln+1)
s = 0
for i in range(ln):
s += nums[i]
if s - target in sums:
idx = sums[s-target]
dp[i+1] = 1 + dp[idx]
dp[i+1] = max(dp[i], dp[i+1])
sums[s] = i+1
return dp[ln]
pass
| class Solution:
def maxNonOverlapping(self, nums: List[int], target: int) -> int:
ln = len(nums)
ret = 0
sums = {0 : 0}
dp = [0] * (ln+1)
s = 0
for i in range(ln):
s += nums[i]
if s - target in sums:
idx = sums[s-target]
dp[i+1] = 1 + dp[idx]
dp[i+1] = max(dp[i], dp[i+1])
sums[s] = i+1
return dp[ln]
pass
| train | APPS_structured |
You are given an array $a$ of length $n$, which initially is a permutation of numbers from $1$ to $n$. In one operation, you can choose an index $i$ ($1 \leq i < n$) such that $a_i < a_{i + 1}$, and remove either $a_i$ or $a_{i + 1}$ from the array (after the removal, the remaining parts are concatenated).
For example, if you have the array $[1, 3, 2]$, you can choose $i = 1$ (since $a_1 = 1 < a_2 = 3$), then either remove $a_1$ which gives the new array $[3, 2]$, or remove $a_2$ which gives the new array $[1, 2]$.
Is it possible to make the length of this array equal to $1$ with these operations?
-----Input-----
The first line contains a single integer $t$ ($1 \leq t \leq 2 \cdot 10^4$) — the number of test cases. The description of the test cases follows.
The first line of each test case contains a single integer $n$ ($2 \leq n \leq 3 \cdot 10^5$) — the length of the array.
The second line of each test case contains $n$ integers $a_1$, $a_2$, ..., $a_n$ ($1 \leq a_i \leq n$, $a_i$ are pairwise distinct) — elements of the array.
It is guaranteed that the sum of $n$ over all test cases doesn't exceed $3 \cdot 10^5$.
-----Output-----
For each test case, output on a single line the word "YES" if it is possible to reduce the array to a single element using the aforementioned operation, or "NO" if it is impossible to do so.
-----Example-----
Input
4
3
1 2 3
4
3 1 2 4
3
2 3 1
6
2 4 6 1 3 5
Output
YES
YES
NO
YES
-----Note-----
For the first two test cases and the fourth test case, we can operate as follow (the bolded elements are the pair chosen for that operation):
$[\text{1}, \textbf{2}, \textbf{3}] \rightarrow [\textbf{1}, \textbf{2}] \rightarrow [\text{1}]$
$[\text{3}, \textbf{1}, \textbf{2}, \text{4}] \rightarrow [\text{3}, \textbf{1}, \textbf{4}] \rightarrow [\textbf{3}, \textbf{4}] \rightarrow [\text{4}]$
$[\textbf{2}, \textbf{4}, \text{6}, \text{1}, \text{3}, \text{5}] \rightarrow [\textbf{4}, \textbf{6}, \text{1}, \text{3}, \text{5}] \rightarrow [\text{4}, \text{1}, \textbf{3}, \textbf{5}] \rightarrow [\text{4}, \textbf{1}, \textbf{5}] \rightarrow [\textbf{4}, \textbf{5}] \rightarrow [\text{4}]$ | def solve():
n = int(input())
a = list(map(int, input().split()))
q = []
for i in a:
while len(q) >= 2 and ((q[-2] < q[-1] and q[-1] > i) or (q[-2] > q[-1] and q[-1] < i)):
q.pop(-1)
q.append(i)
for i in range(len(q) - 1):
if q[i] > q[i + 1]:
print('NO')
return
print('YES')
t = int(input())
for _ in range(t):
solve() | def solve():
n = int(input())
a = list(map(int, input().split()))
q = []
for i in a:
while len(q) >= 2 and ((q[-2] < q[-1] and q[-1] > i) or (q[-2] > q[-1] and q[-1] < i)):
q.pop(-1)
q.append(i)
for i in range(len(q) - 1):
if q[i] > q[i + 1]:
print('NO')
return
print('YES')
t = int(input())
for _ in range(t):
solve() | train | APPS_structured |
Taru likes reading. Every month he gets a copy of the magazine "BIT". The magazine contains information about the latest advancements in technology. Taru
reads the book at night and writes the page number to which he has read on a piece of paper so that he can continue from there the next day. But sometimes
the page number is not printed or is so dull that it is unreadable. To make matters worse Taru's brother who is really naughty tears of some of the pages of
the Magazine and throws them in the dustbin. He remembers the number of leaves he had torn but he does not remember which page numbers got removed. When Taru
finds this out he is furious and wants to beat him up. His brother apologizes, and says he won't ever do this again. But Taru did not want to be easy on him
and he says "I will leave you only if you help me find the answer to this. I will tell you how many pages (Printed sides) were there in the Magazine plus the
pages on which the page numbers were not printed. You already know the number of leaves you tore (T). Can you tell me the expected sum of the page numbers
left in the Magazine?" Taru's brother replied "huh!! This is a coding problem". Please help Taru's brother.
Note: The magazine is like a standard book with all odd page numbers in front and the successive even page number on its back. If the book contains 6 pages,
Page number 1 and Page number 2 are front and back respectively. Tearing a leaf removes both the front and back page numbers.
-----Input-----
The first line contains the number of test cases t. 3t lines follow. The first line of each test case contains the number of pages (printed sides) in the
book. The second line's first integer is F, F integers follow which tell us the numbers of the page numbers not printed. The third line contains a single integer telling us the number of leaves Taru's brother tore.
-----Output-----
Output one real number correct up to 4 decimal digits which is equal to the expected sum of the page numbers left in the book.
-----Constraints-----
Number of printed Sides<=2000. All other values abide by the number of printed sides.
-----Example-----
Input:
2
10
2 1 2
2
10
1 8
0
Output:
31.2000
47.0000 | import sys
rl=sys.stdin.readline
T=int(rl())
for t in range(T):
P=int(rl())
T=(P+1)//2
F=list(map(int,rl().split()))[1:]
numtorn=int(rl())
t=sum(range(1,P+1))-sum(F)
K=T-numtorn
print('%.4f' % (t*K/float(T)))
| import sys
rl=sys.stdin.readline
T=int(rl())
for t in range(T):
P=int(rl())
T=(P+1)//2
F=list(map(int,rl().split()))[1:]
numtorn=int(rl())
t=sum(range(1,P+1))-sum(F)
K=T-numtorn
print('%.4f' % (t*K/float(T)))
| train | APPS_structured |
## Your Story
"A *piano* in the home meant something." - *Fried Green Tomatoes at the Whistle Stop Cafe*
You've just realized a childhood dream by getting a beautiful and beautiful-sounding upright piano from a friend who was leaving the country. You immediately started doing things like playing "Heart and Soul" over and over again, using one finger to pick out any melody that came into your head, requesting some sheet music books from the library, signing up for some MOOCs like Developing Your Musicianship, and wondering if you will think of any good ideas for writing piano-related katas and apps.
Now you're doing an exercise where you play the very first (leftmost, lowest in pitch) key on the 88-key keyboard, which (as shown below) is white, with the little finger on your left hand, then the second key, which is black, with the ring finger on your left hand, then the third key, which is white, with the middle finger on your left hand, then the fourth key, also white, with your left index finger, and then the fifth key, which is black, with your left thumb. Then you play the sixth key, which is white, with your right thumb, and continue on playing the seventh, eighth, ninth, and tenth keys with the other four fingers of your right hand. Then for the eleventh key you go back to your left little finger, and so on. Once you get to the rightmost/highest, 88th, key, you start all over again with your left little finger on the first key. Your thought is that this will help you to learn to move smoothly and with uniform pressure on the keys from each finger to the next and back and forth between hands.
You're not saying the names of the notes while you're doing this, but instead just counting each key press out loud (not starting again at 1 after 88, but continuing on to 89 and so forth) to try to keep a steady rhythm going and to see how far you can get before messing up. You move gracefully and with flourishes, and between screwups you hear, see, and feel that you are part of some great repeating progression between low and high notes and black and white keys.
## Your Function
The function you are going to write is not actually going to help you with your piano playing, but just explore one of the patterns you're experiencing: Given the number you stopped on, was it on a black key or a white key? For example, in the description of your piano exercise above, if you stopped at 5, your left thumb would be on the fifth key of the piano, which is black. Or if you stopped at 92, you would have gone all the way from keys 1 to 88 and then wrapped around, so that you would be on the fourth key, which is white.
Your function will receive an integer between 1 and 10000 (maybe you think that in principle it would be cool to count up to, say, a billion, but considering how many years it would take it is just not possible) and return the string "black" or "white" -- here are a few more examples:
```
1 "white"
12 "black"
42 "white"
100 "black"
2017 "white"
```
Have fun! And if you enjoy this kata, check out the sequel: Piano Kata, Part 2 | keyboard = [("white", "black")[int(n)] for n in "010010100101"]
def black_or_white_key(key_press_count):
return keyboard[(key_press_count - 1) % 88 % 12]
| keyboard = [("white", "black")[int(n)] for n in "010010100101"]
def black_or_white_key(key_press_count):
return keyboard[(key_press_count - 1) % 88 % 12]
| train | APPS_structured |
Given a string of integers, return the number of odd-numbered substrings that can be formed.
For example, in the case of `"1341"`, they are `1, 1, 3, 13, 41, 341, 1341`, a total of `7` numbers.
`solve("1341") = 7`. See test cases for more examples.
Good luck!
If you like substring Katas, please try
[Longest vowel chain](https://www.codewars.com/kata/59c5f4e9d751df43cf000035)
[Alphabet symmetry](https://www.codewars.com/kata/59d9ff9f7905dfeed50000b0) | def solve(s):
return sum(i for i, x in enumerate(s, 1) if x in '13579') | def solve(s):
return sum(i for i, x in enumerate(s, 1) if x in '13579') | train | APPS_structured |
You are the principal of the Cake school in chefland and today is your birthday. You want to treat each of the children with a small cupcake which is made by you. But there is a problem, You don't know how many students are present today.
The students have gathered of the morning assembly in $R$ rows and $C$ columns. Now you have to calculate how many cakes you have to make such that each child gets a cupcake.
-----Input:-----
- First-line will contain $T$, the number of test cases. Then the test cases follow.
- Each test case contains a single line of input, two integers $R$ and $C$.
-----Output:-----
For each test case, output number of cupcakes you have to make.
-----Constraints-----
- $1 \leq T \leq 1000$
- $2 \leq R,C \leq 10^6$
-----Sample Input:-----
1
5 10
-----Sample Output:-----
50 | # cook your dish here
for i in range(int(input())):
r,c = list(map(int,input().split()))
print(r*c)
| # cook your dish here
for i in range(int(input())):
r,c = list(map(int,input().split()))
print(r*c)
| train | APPS_structured |
Have you heard about Megamind? Megamind and Metro Man are two aliens who came to earth. Megamind wanted to destroy the earth, while Metro Man wanted to stop him and protect mankind. After a lot of fighting, Megamind finally threw Metro Man up into the sky. Metro Man was defeated and was never seen again. Megamind wanted to be a super villain. He believed that the difference between a villain and a super villain is nothing but presentation. Megamind became bored, as he had nobody or nothing to fight against since Metro Man was gone. So, he wanted to create another hero against whom he would fight for recreation. But accidentally, another villain named Hal Stewart was created in the process, who also wanted to destroy the earth. Also, at some point Megamind had fallen in love with a pretty girl named Roxanne Ritchi. This changed him into a new man. Now he wants to stop Hal Stewart for the sake of his love. So, the ultimate fight starts now.
* Megamind has unlimited supply of guns named Magic-48. Each of these guns has `shots` rounds of magic spells.
* Megamind has perfect aim. If he shoots a magic spell it will definitely hit Hal Stewart. Once hit, it decreases the energy level of Hal Stewart by `dps` units.
* However, since there are exactly `shots` rounds of magic spells in each of these guns, he may need to swap an old gun with a fully loaded one. This takes some time. Let’s call it swapping period.
* Since Hal Stewart is a mutant, he has regeneration power. His energy level increases by `regen` unit during a swapping period.
* Hal Stewart will be defeated immediately once his energy level becomes zero or negative.
* Hal Stewart initially has the energy level of `hp` and Megamind has a fully loaded gun in his hand.
* Given the values of `hp`, `dps`, `shots` and `regen`, find the minimum number of times Megamind needs to shoot to defeat Hal Stewart. If it is not possible to defeat him, return `-1` instead.
# Example
Suppose, `hp` = 13, `dps` = 4, `shots` = 3 and `regen` = 1. There are 3 rounds of spells in the gun. Megamind shoots all of them. Hal Stewart’s energy level decreases by 12 units, and thus his energy level becomes 1. Since Megamind’s gun is now empty, he will get a new gun and thus it’s a swapping period. At this time, Hal Stewart’s energy level will increase by 1 unit and will become 2. However, when Megamind shoots the next spell, Hal’s energy level will drop by 4 units and will become −2, thus defeating him. So it takes 4 shoots in total to defeat Hal Stewart. However, in this same example if Hal’s regeneration power was 50 instead of 1, it would have been impossible to defeat Hal. | from math import ceil
def mega_mind(hp, dps, shots, regen):
print(hp,dps,shots,regen)
if hp>dps*shots and regen>=dps*shots: return -1
if hp<=dps*shots:
return ceil(hp/dps)
a=ceil((hp-dps*shots)/(-regen+dps*shots))
return a*shots + ceil((hp+a*regen-a*dps*shots)/dps) | from math import ceil
def mega_mind(hp, dps, shots, regen):
print(hp,dps,shots,regen)
if hp>dps*shots and regen>=dps*shots: return -1
if hp<=dps*shots:
return ceil(hp/dps)
a=ceil((hp-dps*shots)/(-regen+dps*shots))
return a*shots + ceil((hp+a*regen-a*dps*shots)/dps) | train | APPS_structured |
There are a total of n courses you have to take, labeled from 0 to n-1.
Some courses may have prerequisites, for example to take course 0 you have to first take course 1, which is expressed as a pair: [0,1]
Given the total number of courses and a list of prerequisite pairs, return the ordering of courses you should take to finish all courses.
There may be multiple correct orders, you just need to return one of them. If it is impossible to finish all courses, return an empty array.
Example 1:
Input: 2, [[1,0]]
Output: [0,1]
Explanation: There are a total of 2 courses to take. To take course 1 you should have finished
course 0. So the correct course order is [0,1] .
Example 2:
Input: 4, [[1,0],[2,0],[3,1],[3,2]]
Output: [0,1,2,3] or [0,2,1,3]
Explanation: There are a total of 4 courses to take. To take course 3 you should have finished both
courses 1 and 2. Both courses 1 and 2 should be taken after you finished course 0.
So one correct course order is [0,1,2,3]. Another correct ordering is [0,2,1,3] .
Note:
The input prerequisites is a graph represented by a list of edges, not adjacency matrices. Read more about how a graph is represented.
You may assume that there are no duplicate edges in the input prerequisites. | class Solution:
def findOrder(self, numCourses, prerequisites):
"""
:type numCourses: int
:type prerequisites: List[List[int]]
:rtype: List[int]
"""
nums = [[] for _ in range(numCourses)]
du = [0] * numCourses
for end, start in prerequisites:
nums[start].append(end)
du[end] += 1
result = []
def dfs(idx):
du[idx] -= 1
result.append(idx)
for i in nums[idx]:
du[i] -= 1
if du[i] == 0:
dfs(i)
for i in range(numCourses):
if du[i] == 0:
dfs(i)
if len(result) == numCourses:
return result
return []
| class Solution:
def findOrder(self, numCourses, prerequisites):
"""
:type numCourses: int
:type prerequisites: List[List[int]]
:rtype: List[int]
"""
nums = [[] for _ in range(numCourses)]
du = [0] * numCourses
for end, start in prerequisites:
nums[start].append(end)
du[end] += 1
result = []
def dfs(idx):
du[idx] -= 1
result.append(idx)
for i in nums[idx]:
du[i] -= 1
if du[i] == 0:
dfs(i)
for i in range(numCourses):
if du[i] == 0:
dfs(i)
if len(result) == numCourses:
return result
return []
| train | APPS_structured |
Chef just come up with a very good idea for his business. He needs to hire two group of software engineers. Each group of engineers will work on completely different things and people from different groups don't want to disturb (and even hear) each other. Chef has just rented a whole floor for his purposes in business center "Cooking Plaza". The floor is a rectangle with dimensions N over M meters. For simplicity of description the floor's structure, let's imagine that it is split into imaginary squares of size 1x1 called "cells".
The whole floor is split into rooms (not necessarily rectangular). There are some not noise-resistant walls between some of the cells. Two adjacent cells belong to the same room if they don't have the wall between them. Cells are considered adjacent if and only if they share an edge. Also, we say that relation "belong to the same room" is transitive. In other words we say that if cells A and B belong to the same room and B and C belong to the same room then A and C belong to the same room.
So we end up having a partition of the floor into rooms. It also means, that each point on the floor belongs to some room.
Chef have to distribute the rooms between engineers of two groups. Engineers from the different groups cannot seat in the same room. If engineers from a different groups seat in adjacent rooms, the walls these rooms share have to be noise-resistant. The cost of having one meter of wall isolated is K per month. Due to various reasons Chef has to pay an additional cost for support of each of the room (e.g. cleaning costs money as well). Interesting to know that support cost for a particular room may differ depending on engineers of which group seat in this room.
Chef doesn't know the number of people he needs in each group of engineers so he wants to minimize the money he needs to pay for all the floor rent and support. He will see how it goes and then redistribute the floor or find another floor to rent or whatever. Either way, you don't need to care about this.
Please pay attention to the restriction that all the rooms should be occupied by engineers of one of the teams. Also, it might happen that all the rooms will be assigned to the same team and this is completely okay.
-----Input-----
The first line of the input contains three integers N, M, W, K and R, where N and M denote size of the floor, W denote number of one-meter-length walls, K denote cost of having one-meter-length wall be noise-resistant and R denote the number of rooms in which floor is partitioned.
Next W lines contain four integers each X1, Y1, X2, Y2. This means that cells with coordinates X1, Y1 and X2, Y2 have a wall between them. It's guaranteed that this cells share an edge.
Next R lines will contain four space separated integers each X, Y, C1, C2. This should be treated as support cost per month in a room that contain cell X, Y is C1 for first group of engineers and C2 for second group of engineers. It's guaranteed that all of cells among these R cells belong to different rooms. All coordinates are indexed starting from 1.
-----Output-----
Output a single integer - sum of the rent and support costs per month.
-----Constraints-----
- 1 ≤ N, M ≤ 1000
- 1 ≤ W ≤ min(2*N*M, 150000)
- 1 ≤ X1 ≤ N
- 1 ≤ Y1 ≤ M
- 1 ≤ X2 ≤ N
- 1 ≤ Y2 ≤ M
- 1 ≤ K ≤ 10000
- 1 ≤ C1, C2 ≤ 10000
- Limitations on R is described in subtasks section.
-----Subtasks-----
- Subtask #1 [30 points]: 1 ≤ R ≤ 50
- Subtask #2 [70 points]: 1 ≤ R ≤ 500
-----Example-----
Input:
2 4 5 5 3
1 2 1 3
1 2 2 2
1 3 2 3
1 4 2 4
2 1 2 2
1 1 30 12
1 3 10 15
2 3 11 22
Output:
48
-----Explanation-----
Here's the scheme of the floor
The correct assignment is the following.
- The blue color denotes assignment to the first team. Total cost for renting two rooms for this team is 11 + 10 = 21.
- The red color denotes assignment to the second team. Total cost for renting the only room for the team is 12.
- There are 3 meters of walls between them, resulting in isolating cost of 15.
The grand total is 21 + 12 + 15 = 48 | import sys
def findRoom(x,y,i):
R = [(x,y)]
GRID[x][y] = i
for n in R:
GRID[n[0]][n[1]] = i
if n[0]>0 and GRID[n[0]-1][n[1]]==0 and H[n[0]-1][n[1]]:
GRID[n[0]-1][n[1]] = i
R.append((n[0]-1,n[1]))
if n[0]<N-1 and GRID[n[0]+1][n[1]]==0 and H[n[0]][n[1]]:
GRID[n[0]+1][n[1]] = i
R.append((n[0]+1,n[1]))
if n[1]>0 and GRID[n[0]][n[1]-1]==0 and V[n[0]][n[1]-1]:
GRID[n[0]][n[1]-1] = i
R.append((n[0],n[1]-1))
if n[1]<M-1 and GRID[n[0]][n[1]+1]==0 and V[n[0]][n[1]]:
GRID[n[0]][n[1]+1] = i
R.append((n[0],n[1]+1))
def roomPrice(r):
wall_price_0 = wall_price_1 = 0
for i in range(R):
if C[i][r] and T[i] != 1:
wall_price_0 += C[i][r]*K
else:
wall_price_1 += C[i][r]*K
return [wall_price_0 + Rooms[r][0], wall_price_1 + Rooms[r][1]]
def total_price():
price = 0
for r in range(R):
for i in range(r):
if C[i][r] and T[i] != T[r]:
price += C[i][r]*K
price += Rooms[r][T[r]-1]
return price
def solve(r):
if r==R:
return 0
wall_price_0 = 0
wall_price_1 = 0
for i in range(r):
if C[i][r] and T[i] != 1:
wall_price_0 += C[i][r]*K
else:
wall_price_1 += C[i][r]*K
if T[r]!=0:
return [wall_price_0,wall_price_1][T[r]-1]+Rooms[r][T[r]-1]+solve(r+1)
T[r] = 1
result = solve(r+1)+wall_price_0+Rooms[r][0]
T[r] = 2
result = min(solve(r+1)+wall_price_1+Rooms[r][1], result)
T[r] = 0
return result
f = sys.stdin
N,M,W,K,R = list(map(int, f.readline().split(' ')))
T = [0] * R
GRID = list(map(list,[[0]*M]*N))
H = list(map(list,[[1]*M]*N))
V = list(map(list,[[1]*M]*N))
Walls = []
for _ in range(W):
x0,y0,x1,y1 = list(map(int, f.readline().split(' ')))
x0 -= 1
x1 -= 1
y0 -= 1
y1 -= 1
if x0==x1:
V[x0][y0] = 0
else:
H[x0][y0] = 0
Walls.append([x0,y0,x1,y1])
Rooms = []
for i in range(R):
x,y,t1,t2 = list(map(int, f.readline().split(' ')))
findRoom(x-1,y-1,i+1)
Rooms.append([t1,t2])
C = list(map(list,[[0]*R]*R))
for w in Walls:
r1 = GRID[w[0]][w[1]]-1
r2 = GRID[w[2]][w[3]]-1
C[r1][r2] += 1
C[r2][r1] += 1
Stable = [False]*R
for r in range(R):
walls_max_price = sum(C[r])*K
if walls_max_price<=abs(Rooms[r][0]-Rooms[r][1]):
# If we choose the cheaper team, no matter what the next rooms are the walls we not overprice it.
T[r] = 1+(Rooms[r][0]>Rooms[r][1])
Stable[r] = True
def try_teams():
for r in range(R):
if not Stable[r]:
T[r] = 1+(r&1)
change = True
while change:
change = False
for r in range(R):
price = roomPrice(r)
if price[T[r]-1]>price[2-T[r]]:
T[r] = 3-T[r]
change = True
print(total_price())
#try_teams()
print(solve(0))
| import sys
def findRoom(x,y,i):
R = [(x,y)]
GRID[x][y] = i
for n in R:
GRID[n[0]][n[1]] = i
if n[0]>0 and GRID[n[0]-1][n[1]]==0 and H[n[0]-1][n[1]]:
GRID[n[0]-1][n[1]] = i
R.append((n[0]-1,n[1]))
if n[0]<N-1 and GRID[n[0]+1][n[1]]==0 and H[n[0]][n[1]]:
GRID[n[0]+1][n[1]] = i
R.append((n[0]+1,n[1]))
if n[1]>0 and GRID[n[0]][n[1]-1]==0 and V[n[0]][n[1]-1]:
GRID[n[0]][n[1]-1] = i
R.append((n[0],n[1]-1))
if n[1]<M-1 and GRID[n[0]][n[1]+1]==0 and V[n[0]][n[1]]:
GRID[n[0]][n[1]+1] = i
R.append((n[0],n[1]+1))
def roomPrice(r):
wall_price_0 = wall_price_1 = 0
for i in range(R):
if C[i][r] and T[i] != 1:
wall_price_0 += C[i][r]*K
else:
wall_price_1 += C[i][r]*K
return [wall_price_0 + Rooms[r][0], wall_price_1 + Rooms[r][1]]
def total_price():
price = 0
for r in range(R):
for i in range(r):
if C[i][r] and T[i] != T[r]:
price += C[i][r]*K
price += Rooms[r][T[r]-1]
return price
def solve(r):
if r==R:
return 0
wall_price_0 = 0
wall_price_1 = 0
for i in range(r):
if C[i][r] and T[i] != 1:
wall_price_0 += C[i][r]*K
else:
wall_price_1 += C[i][r]*K
if T[r]!=0:
return [wall_price_0,wall_price_1][T[r]-1]+Rooms[r][T[r]-1]+solve(r+1)
T[r] = 1
result = solve(r+1)+wall_price_0+Rooms[r][0]
T[r] = 2
result = min(solve(r+1)+wall_price_1+Rooms[r][1], result)
T[r] = 0
return result
f = sys.stdin
N,M,W,K,R = list(map(int, f.readline().split(' ')))
T = [0] * R
GRID = list(map(list,[[0]*M]*N))
H = list(map(list,[[1]*M]*N))
V = list(map(list,[[1]*M]*N))
Walls = []
for _ in range(W):
x0,y0,x1,y1 = list(map(int, f.readline().split(' ')))
x0 -= 1
x1 -= 1
y0 -= 1
y1 -= 1
if x0==x1:
V[x0][y0] = 0
else:
H[x0][y0] = 0
Walls.append([x0,y0,x1,y1])
Rooms = []
for i in range(R):
x,y,t1,t2 = list(map(int, f.readline().split(' ')))
findRoom(x-1,y-1,i+1)
Rooms.append([t1,t2])
C = list(map(list,[[0]*R]*R))
for w in Walls:
r1 = GRID[w[0]][w[1]]-1
r2 = GRID[w[2]][w[3]]-1
C[r1][r2] += 1
C[r2][r1] += 1
Stable = [False]*R
for r in range(R):
walls_max_price = sum(C[r])*K
if walls_max_price<=abs(Rooms[r][0]-Rooms[r][1]):
# If we choose the cheaper team, no matter what the next rooms are the walls we not overprice it.
T[r] = 1+(Rooms[r][0]>Rooms[r][1])
Stable[r] = True
def try_teams():
for r in range(R):
if not Stable[r]:
T[r] = 1+(r&1)
change = True
while change:
change = False
for r in range(R):
price = roomPrice(r)
if price[T[r]-1]>price[2-T[r]]:
T[r] = 3-T[r]
change = True
print(total_price())
#try_teams()
print(solve(0))
| train | APPS_structured |
Because my other two parts of the serie were pretty well received I decided to do another part.
Puzzle Tiles
You will get two Integer n (width) and m (height) and your task is to draw following pattern. Each line is seperated with '\n'.
Both integers are equal or greater than 1. No need to check for invalid parameters.
There are no whitespaces at the end of each line.
e.g.:
\_( )\_\_ \_( )\_\_ \_( )\_\_ \_( )\_\_
\_| \_| \_| \_| \_|
(\_ \_ (\_ \_ (\_ \_ (\_ \_ (\_
|\_\_( )\_|\_\_( )\_|\_\_( )\_|\_\_( )\_|
|\_ |\_ |\_ |\_ |\_
puzzleTiles(4,3)=> \_) \_ \_) \_ \_) \_ \_) \_ \_)
|\_\_( )\_|\_\_( )\_|\_\_( )\_|\_\_( )\_|
\_| \_| \_| \_| \_|
(\_ \_ (\_ \_ (\_ \_ (\_ \_ (\_
|\_\_( )\_|\_\_( )\_|\_\_( )\_|\_\_( )\_|
For more informations take a look in the test cases!
Serie: ASCII Fun
ASCII Fun #1: X-Shape
ASCII Fun #2: Funny Dots
ASCII Fun #3: Puzzle Tiles
ASCII Fun #4: Build a pyramid | def puzzle_tiles(width, height):
r=[]
r.append(' '+' _( )__'*width)
for i in range(height):
if i%2==0:
r.append(' _'+'| _'*width+'|')
r.append('(_ _ '*width+'(_')
else:
r.append(' '+'|_ '*width+'|_')
r.append(' _)'+' _ _)'*width)
r.append(' '+'|__( )_'*width+'|')
return '\n'.join(r) | def puzzle_tiles(width, height):
r=[]
r.append(' '+' _( )__'*width)
for i in range(height):
if i%2==0:
r.append(' _'+'| _'*width+'|')
r.append('(_ _ '*width+'(_')
else:
r.append(' '+'|_ '*width+'|_')
r.append(' _)'+' _ _)'*width)
r.append(' '+'|__( )_'*width+'|')
return '\n'.join(r) | train | APPS_structured |
=====Function Descriptions=====
floor
The tool floor returns the floor of the input element-wise.
The floor of x is the largest integer i where i≤x.
import numpy
my_array = numpy.array([1.1, 2.2, 3.3, 4.4, 5.5, 6.6, 7.7, 8.8, 9.9])
print numpy.floor(my_array) #[ 1. 2. 3. 4. 5. 6. 7. 8. 9.]
ceil
The tool ceil returns the ceiling of the input element-wise.
The ceiling of x is the smallest integer i where i≥x.
import numpy
my_array = numpy.array([1.1, 2.2, 3.3, 4.4, 5.5, 6.6, 7.7, 8.8, 9.9])
print numpy.ceil(my_array) #[ 2. 3. 4. 5. 6. 7. 8. 9. 10.]
rint
The rint tool rounds to the nearest integer of input element-wise.
import numpy
my_array = numpy.array([1.1, 2.2, 3.3, 4.4, 5.5, 6.6, 7.7, 8.8, 9.9])
print numpy.rint(my_array) #[ 1. 2. 3. 4. 6. 7. 8. 9. 10.]
=====Problem Statement=====
You are given a 1-D array, A. Your task is to print the floor, ceil and rint of all the elements of A.
=====Input Format=====
A single line of input containing the space separated elements of array A.
=====Output Format=====
On the first line, print the floor of A.
On the second line, print the ceil of A.
On the third line, print the rint of A. | import numpy
np_ar = numpy.array(list(map(float,input().split())),float)
print((numpy.floor(np_ar)))
print((numpy.ceil(np_ar)))
print((numpy.rint(np_ar)))
| import numpy
np_ar = numpy.array(list(map(float,input().split())),float)
print((numpy.floor(np_ar)))
print((numpy.ceil(np_ar)))
print((numpy.rint(np_ar)))
| train | APPS_structured |
# Description
Given a number `n`, you should find a set of numbers for which the sum equals `n`. This set must consist exclusively of values that are a power of `2` (eg: `2^0 => 1, 2^1 => 2, 2^2 => 4, ...`).
The function `powers` takes a single parameter, the number `n`, and should return an array of unique numbers.
## Criteria
The function will always receive a valid input: any positive integer between `1` and the max integer value for your language (eg: for JavaScript this would be `9007199254740991` otherwise known as `Number.MAX_SAFE_INTEGER`).
The function should return an array of numbers that are a **power of 2** (`2^x = y`).
Each member of the returned array should be **unique**. (eg: the valid answer for `powers(2)` is `[2]`, not `[1, 1]`)
Members should be sorted in **ascending order** (small -> large). (eg: the valid answer for `powers(6)` is `[2, 4]`, not `[4, 2]`) | pows = [2**i for i in range(63, -1, -1)]
def powers(n):
result = []
for p in pows:
if n >= p:
result.append(p)
n -= p
return result[::-1] | pows = [2**i for i in range(63, -1, -1)]
def powers(n):
result = []
for p in pows:
if n >= p:
result.append(p)
n -= p
return result[::-1] | train | APPS_structured |
### Sudoku Background
Sudoku is a game played on a 9x9 grid. The goal of the game is to fill all cells of the grid with digits from 1 to 9, so that each column, each row, and each of the nine 3x3 sub-grids (also known as blocks) contain all of the digits from 1 to 9.
(More info at: http://en.wikipedia.org/wiki/Sudoku)
### Sudoku Solution Validator
Write a function `validSolution`/`ValidateSolution`/`valid_solution()` that accepts a 2D array representing a Sudoku board, and returns true if it is a valid solution, or false otherwise. The cells of the sudoku board may also contain 0's, which will represent empty cells. Boards containing one or more zeroes are considered to be invalid solutions.
The board is always 9 cells by 9 cells, and every cell only contains integers from 0 to 9.
### Examples
```
validSolution([
[5, 3, 4, 6, 7, 8, 9, 1, 2],
[6, 7, 2, 1, 9, 5, 3, 4, 8],
[1, 9, 8, 3, 4, 2, 5, 6, 7],
[8, 5, 9, 7, 6, 1, 4, 2, 3],
[4, 2, 6, 8, 5, 3, 7, 9, 1],
[7, 1, 3, 9, 2, 4, 8, 5, 6],
[9, 6, 1, 5, 3, 7, 2, 8, 4],
[2, 8, 7, 4, 1, 9, 6, 3, 5],
[3, 4, 5, 2, 8, 6, 1, 7, 9]
]); // => true
```
```
validSolution([
[5, 3, 4, 6, 7, 8, 9, 1, 2],
[6, 7, 2, 1, 9, 0, 3, 4, 8],
[1, 0, 0, 3, 4, 2, 5, 6, 0],
[8, 5, 9, 7, 6, 1, 0, 2, 0],
[4, 2, 6, 8, 5, 3, 7, 9, 1],
[7, 1, 3, 9, 2, 4, 8, 5, 6],
[9, 0, 1, 5, 3, 7, 2, 1, 4],
[2, 8, 7, 4, 1, 9, 6, 3, 5],
[3, 0, 0, 4, 8, 1, 1, 7, 9]
]); // => false
``` | correct = [1, 2, 3, 4, 5, 6, 7, 8, 9]
def validSolution(board):
# check rows
for row in board:
if sorted(row) != correct:
return False
# check columns
for column in zip(*board):
if sorted(column) != correct:
return False
# check regions
for i in range(3):
for j in range(3):
region = []
for line in board[i*3:(i+1)*3]:
region += line[j*3:(j+1)*3]
if sorted(region) != correct:
return False
# if everything correct
return True | correct = [1, 2, 3, 4, 5, 6, 7, 8, 9]
def validSolution(board):
# check rows
for row in board:
if sorted(row) != correct:
return False
# check columns
for column in zip(*board):
if sorted(column) != correct:
return False
# check regions
for i in range(3):
for j in range(3):
region = []
for line in board[i*3:(i+1)*3]:
region += line[j*3:(j+1)*3]
if sorted(region) != correct:
return False
# if everything correct
return True | train | APPS_structured |
An Arithmetic Progression is defined as one in which there is a constant difference between the consecutive terms of a given series of numbers. You are provided with consecutive elements of an Arithmetic Progression. There is however one hitch: exactly one term from the original series is missing from the set of numbers which have been given to you. The rest of the given series is the same as the original AP. Find the missing term.
You have to write a function that receives a list, list size will always be at least 3 numbers. The missing term will never be the first or last one.
## Example
```python
find_missing([1, 3, 5, 9, 11]) == 7
```
PS: This is a sample question of the facebook engineer challenge on interviewstreet.
I found it quite fun to solve on paper using math, derive the algo that way.
Have fun! | def find_missing(sequence):
return (sequence[-1] + sequence[0]) * (len(sequence) + 1) / 2 - sum(sequence)
| def find_missing(sequence):
return (sequence[-1] + sequence[0]) * (len(sequence) + 1) / 2 - sum(sequence)
| train | APPS_structured |