Unnamed: 0
int64 0
0
| repo_id
stringlengths 5
186
| file_path
stringlengths 15
223
| content
stringlengths 1
32.8M
⌀ |
---|---|---|---|
0 | repos/glslang.zig/glslang | repos/glslang.zig/glslang/SPIRV/GLSL.std.450.h | /*
** Copyright (c) 2014-2016 The Khronos Group Inc.
**
** Permission is hereby granted, free of charge, to any person obtaining a copy
** of this software and/or associated documentation files (the "Materials"),
** to deal in the Materials without restriction, including without limitation
** the rights to use, copy, modify, merge, publish, distribute, sublicense,
** and/or sell copies of the Materials, and to permit persons to whom the
** Materials are furnished to do so, subject to the following conditions:
**
** The above copyright notice and this permission notice shall be included in
** all copies or substantial portions of the Materials.
**
** MODIFICATIONS TO THIS FILE MAY MEAN IT NO LONGER ACCURATELY REFLECTS KHRONOS
** STANDARDS. THE UNMODIFIED, NORMATIVE VERSIONS OF KHRONOS SPECIFICATIONS AND
** HEADER INFORMATION ARE LOCATED AT https://www.khronos.org/registry/
**
** THE MATERIALS ARE PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS
** OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
** FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
** THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
** LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
** FROM,OUT OF OR IN CONNECTION WITH THE MATERIALS OR THE USE OR OTHER DEALINGS
** IN THE MATERIALS.
*/
#ifndef GLSLstd450_H
#define GLSLstd450_H
static const int GLSLstd450Version = 100;
static const int GLSLstd450Revision = 1;
enum GLSLstd450 {
GLSLstd450Bad = 0, // Don't use
GLSLstd450Round = 1,
GLSLstd450RoundEven = 2,
GLSLstd450Trunc = 3,
GLSLstd450FAbs = 4,
GLSLstd450SAbs = 5,
GLSLstd450FSign = 6,
GLSLstd450SSign = 7,
GLSLstd450Floor = 8,
GLSLstd450Ceil = 9,
GLSLstd450Fract = 10,
GLSLstd450Radians = 11,
GLSLstd450Degrees = 12,
GLSLstd450Sin = 13,
GLSLstd450Cos = 14,
GLSLstd450Tan = 15,
GLSLstd450Asin = 16,
GLSLstd450Acos = 17,
GLSLstd450Atan = 18,
GLSLstd450Sinh = 19,
GLSLstd450Cosh = 20,
GLSLstd450Tanh = 21,
GLSLstd450Asinh = 22,
GLSLstd450Acosh = 23,
GLSLstd450Atanh = 24,
GLSLstd450Atan2 = 25,
GLSLstd450Pow = 26,
GLSLstd450Exp = 27,
GLSLstd450Log = 28,
GLSLstd450Exp2 = 29,
GLSLstd450Log2 = 30,
GLSLstd450Sqrt = 31,
GLSLstd450InverseSqrt = 32,
GLSLstd450Determinant = 33,
GLSLstd450MatrixInverse = 34,
GLSLstd450Modf = 35, // second operand needs an OpVariable to write to
GLSLstd450ModfStruct = 36, // no OpVariable operand
GLSLstd450FMin = 37,
GLSLstd450UMin = 38,
GLSLstd450SMin = 39,
GLSLstd450FMax = 40,
GLSLstd450UMax = 41,
GLSLstd450SMax = 42,
GLSLstd450FClamp = 43,
GLSLstd450UClamp = 44,
GLSLstd450SClamp = 45,
GLSLstd450FMix = 46,
GLSLstd450IMix = 47, // Reserved
GLSLstd450Step = 48,
GLSLstd450SmoothStep = 49,
GLSLstd450Fma = 50,
GLSLstd450Frexp = 51, // second operand needs an OpVariable to write to
GLSLstd450FrexpStruct = 52, // no OpVariable operand
GLSLstd450Ldexp = 53,
GLSLstd450PackSnorm4x8 = 54,
GLSLstd450PackUnorm4x8 = 55,
GLSLstd450PackSnorm2x16 = 56,
GLSLstd450PackUnorm2x16 = 57,
GLSLstd450PackHalf2x16 = 58,
GLSLstd450PackDouble2x32 = 59,
GLSLstd450UnpackSnorm2x16 = 60,
GLSLstd450UnpackUnorm2x16 = 61,
GLSLstd450UnpackHalf2x16 = 62,
GLSLstd450UnpackSnorm4x8 = 63,
GLSLstd450UnpackUnorm4x8 = 64,
GLSLstd450UnpackDouble2x32 = 65,
GLSLstd450Length = 66,
GLSLstd450Distance = 67,
GLSLstd450Cross = 68,
GLSLstd450Normalize = 69,
GLSLstd450FaceForward = 70,
GLSLstd450Reflect = 71,
GLSLstd450Refract = 72,
GLSLstd450FindILsb = 73,
GLSLstd450FindSMsb = 74,
GLSLstd450FindUMsb = 75,
GLSLstd450InterpolateAtCentroid = 76,
GLSLstd450InterpolateAtSample = 77,
GLSLstd450InterpolateAtOffset = 78,
GLSLstd450NMin = 79,
GLSLstd450NMax = 80,
GLSLstd450NClamp = 81,
GLSLstd450Count
};
#endif // #ifndef GLSLstd450_H
|
0 | repos/glslang.zig/glslang | repos/glslang.zig/glslang/SPIRV/bitutils.h | // Copyright (c) 2015-2016 The Khronos Group Inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
#ifndef LIBSPIRV_UTIL_BITUTILS_H_
#define LIBSPIRV_UTIL_BITUTILS_H_
#include <cstdint>
#include <cstring>
namespace spvutils {
// Performs a bitwise copy of source to the destination type Dest.
template <typename Dest, typename Src>
Dest BitwiseCast(Src source) {
Dest dest;
static_assert(sizeof(source) == sizeof(dest),
"BitwiseCast: Source and destination must have the same size");
std::memcpy(static_cast<void*>(&dest), &source, sizeof(dest));
return dest;
}
// SetBits<T, First, Num> returns an integer of type <T> with bits set
// for position <First> through <First + Num - 1>, counting from the least
// significant bit. In particular when Num == 0, no positions are set to 1.
// A static assert will be triggered if First + Num > sizeof(T) * 8, that is,
// a bit that will not fit in the underlying type is set.
template <typename T, size_t First = 0, size_t Num = 0>
struct SetBits {
static_assert(First < sizeof(T) * 8,
"Tried to set a bit that is shifted too far.");
const static T get = (T(1) << First) | SetBits<T, First + 1, Num - 1>::get;
};
template <typename T, size_t Last>
struct SetBits<T, Last, 0> {
const static T get = T(0);
};
// This is all compile-time so we can put our tests right here.
static_assert(SetBits<uint32_t, 0, 0>::get == uint32_t(0x00000000),
"SetBits failed");
static_assert(SetBits<uint32_t, 0, 1>::get == uint32_t(0x00000001),
"SetBits failed");
static_assert(SetBits<uint32_t, 31, 1>::get == uint32_t(0x80000000),
"SetBits failed");
static_assert(SetBits<uint32_t, 1, 2>::get == uint32_t(0x00000006),
"SetBits failed");
static_assert(SetBits<uint32_t, 30, 2>::get == uint32_t(0xc0000000),
"SetBits failed");
static_assert(SetBits<uint32_t, 0, 31>::get == uint32_t(0x7FFFFFFF),
"SetBits failed");
static_assert(SetBits<uint32_t, 0, 32>::get == uint32_t(0xFFFFFFFF),
"SetBits failed");
static_assert(SetBits<uint32_t, 16, 16>::get == uint32_t(0xFFFF0000),
"SetBits failed");
static_assert(SetBits<uint64_t, 0, 1>::get == uint64_t(0x0000000000000001LL),
"SetBits failed");
static_assert(SetBits<uint64_t, 63, 1>::get == uint64_t(0x8000000000000000LL),
"SetBits failed");
static_assert(SetBits<uint64_t, 62, 2>::get == uint64_t(0xc000000000000000LL),
"SetBits failed");
static_assert(SetBits<uint64_t, 31, 1>::get == uint64_t(0x0000000080000000LL),
"SetBits failed");
static_assert(SetBits<uint64_t, 16, 16>::get == uint64_t(0x00000000FFFF0000LL),
"SetBits failed");
} // namespace spvutils
#endif // LIBSPIRV_UTIL_BITUTILS_H_
|
0 | repos/glslang.zig/glslang | repos/glslang.zig/glslang/SPIRV/GLSL.ext.AMD.h | /*
** Copyright (c) 2014-2016 The Khronos Group Inc.
**
** Permission is hereby granted, free of charge, to any person obtaining a copy
** of this software and/or associated documentation files (the "Materials"),
** to deal in the Materials without restriction, including without limitation
** the rights to use, copy, modify, merge, publish, distribute, sublicense,
** and/or sell copies of the Materials, and to permit persons to whom the
** Materials are furnished to do so, subject to the following conditions:
**
** The above copyright notice and this permission notice shall be included in
** all copies or substantial portions of the Materials.
**
** MODIFICATIONS TO THIS FILE MAY MEAN IT NO LONGER ACCURATELY REFLECTS KHRONOS
** STANDARDS. THE UNMODIFIED, NORMATIVE VERSIONS OF KHRONOS SPECIFICATIONS AND
** HEADER INFORMATION ARE LOCATED AT https://www.khronos.org/registry/
**
** THE MATERIALS ARE PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS
** OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
** FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
** THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
** LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
** FROM,OUT OF OR IN CONNECTION WITH THE MATERIALS OR THE USE OR OTHER DEALINGS
** IN THE MATERIALS.
*/
#ifndef GLSLextAMD_H
#define GLSLextAMD_H
static const int GLSLextAMDVersion = 100;
static const int GLSLextAMDRevision = 7;
// SPV_AMD_shader_ballot
static const char* const E_SPV_AMD_shader_ballot = "SPV_AMD_shader_ballot";
enum ShaderBallotAMD {
ShaderBallotBadAMD = 0, // Don't use
SwizzleInvocationsAMD = 1,
SwizzleInvocationsMaskedAMD = 2,
WriteInvocationAMD = 3,
MbcntAMD = 4,
ShaderBallotCountAMD
};
// SPV_AMD_shader_trinary_minmax
static const char* const E_SPV_AMD_shader_trinary_minmax = "SPV_AMD_shader_trinary_minmax";
enum ShaderTrinaryMinMaxAMD {
ShaderTrinaryMinMaxBadAMD = 0, // Don't use
FMin3AMD = 1,
UMin3AMD = 2,
SMin3AMD = 3,
FMax3AMD = 4,
UMax3AMD = 5,
SMax3AMD = 6,
FMid3AMD = 7,
UMid3AMD = 8,
SMid3AMD = 9,
ShaderTrinaryMinMaxCountAMD
};
// SPV_AMD_shader_explicit_vertex_parameter
static const char* const E_SPV_AMD_shader_explicit_vertex_parameter = "SPV_AMD_shader_explicit_vertex_parameter";
enum ShaderExplicitVertexParameterAMD {
ShaderExplicitVertexParameterBadAMD = 0, // Don't use
InterpolateAtVertexAMD = 1,
ShaderExplicitVertexParameterCountAMD
};
// SPV_AMD_gcn_shader
static const char* const E_SPV_AMD_gcn_shader = "SPV_AMD_gcn_shader";
enum GcnShaderAMD {
GcnShaderBadAMD = 0, // Don't use
CubeFaceIndexAMD = 1,
CubeFaceCoordAMD = 2,
TimeAMD = 3,
GcnShaderCountAMD
};
// SPV_AMD_gpu_shader_half_float
static const char* const E_SPV_AMD_gpu_shader_half_float = "SPV_AMD_gpu_shader_half_float";
// SPV_AMD_texture_gather_bias_lod
static const char* const E_SPV_AMD_texture_gather_bias_lod = "SPV_AMD_texture_gather_bias_lod";
// SPV_AMD_gpu_shader_int16
static const char* const E_SPV_AMD_gpu_shader_int16 = "SPV_AMD_gpu_shader_int16";
// SPV_AMD_shader_image_load_store_lod
static const char* const E_SPV_AMD_shader_image_load_store_lod = "SPV_AMD_shader_image_load_store_lod";
// SPV_AMD_shader_fragment_mask
static const char* const E_SPV_AMD_shader_fragment_mask = "SPV_AMD_shader_fragment_mask";
// SPV_AMD_gpu_shader_half_float_fetch
static const char* const E_SPV_AMD_gpu_shader_half_float_fetch = "SPV_AMD_gpu_shader_half_float_fetch";
#endif // #ifndef GLSLextAMD_H
|
0 | repos/glslang.zig/glslang | repos/glslang.zig/glslang/SPIRV/SpvPostProcess.cpp | //
// Copyright (C) 2018 Google, Inc.
//
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
//
// Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
//
// Redistributions in binary form must reproduce the above
// copyright notice, this list of conditions and the following
// disclaimer in the documentation and/or other materials provided
// with the distribution.
//
// Neither the name of 3Dlabs Inc. Ltd. nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
// "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
// LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
// FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
// COPYRIGHT HOLDERS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
// INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
// BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
// LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
// CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
// LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
// ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
// POSSIBILITY OF SUCH DAMAGE.
//
// Post-processing for SPIR-V IR, in internal form, not standard binary form.
//
#include <cassert>
#include <cstdlib>
#include <unordered_map>
#include <unordered_set>
#include <algorithm>
#include "SpvBuilder.h"
#include "spirv.hpp"
namespace spv {
#include "GLSL.std.450.h"
#include "GLSL.ext.KHR.h"
#include "GLSL.ext.EXT.h"
#include "GLSL.ext.AMD.h"
#include "GLSL.ext.NV.h"
#include "GLSL.ext.ARM.h"
#include "GLSL.ext.QCOM.h"
}
namespace spv {
// Hook to visit each operand type and result type of an instruction.
// Will be called multiple times for one instruction, once for each typed
// operand and the result.
void Builder::postProcessType(const Instruction& inst, Id typeId)
{
// Characterize the type being questioned
Id basicTypeOp = getMostBasicTypeClass(typeId);
int width = 0;
if (basicTypeOp == OpTypeFloat || basicTypeOp == OpTypeInt)
width = getScalarTypeWidth(typeId);
// Do opcode-specific checks
switch (inst.getOpCode()) {
case OpLoad:
case OpStore:
if (basicTypeOp == OpTypeStruct) {
if (containsType(typeId, OpTypeInt, 8))
addCapability(CapabilityInt8);
if (containsType(typeId, OpTypeInt, 16))
addCapability(CapabilityInt16);
if (containsType(typeId, OpTypeFloat, 16))
addCapability(CapabilityFloat16);
} else {
StorageClass storageClass = getStorageClass(inst.getIdOperand(0));
if (width == 8) {
switch (storageClass) {
case StorageClassPhysicalStorageBufferEXT:
case StorageClassUniform:
case StorageClassStorageBuffer:
case StorageClassPushConstant:
break;
default:
addCapability(CapabilityInt8);
break;
}
} else if (width == 16) {
switch (storageClass) {
case StorageClassPhysicalStorageBufferEXT:
case StorageClassUniform:
case StorageClassStorageBuffer:
case StorageClassPushConstant:
case StorageClassInput:
case StorageClassOutput:
break;
default:
if (basicTypeOp == OpTypeInt)
addCapability(CapabilityInt16);
if (basicTypeOp == OpTypeFloat)
addCapability(CapabilityFloat16);
break;
}
}
}
break;
case OpCopyObject:
break;
case OpFConvert:
case OpSConvert:
case OpUConvert:
// Look for any 8/16-bit storage capabilities. If there are none, assume that
// the convert instruction requires the Float16/Int8/16 capability.
if (containsType(typeId, OpTypeFloat, 16) || containsType(typeId, OpTypeInt, 16)) {
bool foundStorage = false;
for (auto it = capabilities.begin(); it != capabilities.end(); ++it) {
spv::Capability cap = *it;
if (cap == spv::CapabilityStorageInputOutput16 ||
cap == spv::CapabilityStoragePushConstant16 ||
cap == spv::CapabilityStorageUniformBufferBlock16 ||
cap == spv::CapabilityStorageUniform16) {
foundStorage = true;
break;
}
}
if (!foundStorage) {
if (containsType(typeId, OpTypeFloat, 16))
addCapability(CapabilityFloat16);
if (containsType(typeId, OpTypeInt, 16))
addCapability(CapabilityInt16);
}
}
if (containsType(typeId, OpTypeInt, 8)) {
bool foundStorage = false;
for (auto it = capabilities.begin(); it != capabilities.end(); ++it) {
spv::Capability cap = *it;
if (cap == spv::CapabilityStoragePushConstant8 ||
cap == spv::CapabilityUniformAndStorageBuffer8BitAccess ||
cap == spv::CapabilityStorageBuffer8BitAccess) {
foundStorage = true;
break;
}
}
if (!foundStorage) {
addCapability(CapabilityInt8);
}
}
break;
case OpExtInst:
switch (inst.getImmediateOperand(1)) {
case GLSLstd450Frexp:
case GLSLstd450FrexpStruct:
if (getSpvVersion() < spv::Spv_1_3 && containsType(typeId, OpTypeInt, 16))
addExtension(spv::E_SPV_AMD_gpu_shader_int16);
break;
case GLSLstd450InterpolateAtCentroid:
case GLSLstd450InterpolateAtSample:
case GLSLstd450InterpolateAtOffset:
if (getSpvVersion() < spv::Spv_1_3 && containsType(typeId, OpTypeFloat, 16))
addExtension(spv::E_SPV_AMD_gpu_shader_half_float);
break;
default:
break;
}
break;
case OpAccessChain:
case OpPtrAccessChain:
if (isPointerType(typeId))
break;
if (basicTypeOp == OpTypeInt) {
if (width == 16)
addCapability(CapabilityInt16);
else if (width == 8)
addCapability(CapabilityInt8);
}
break;
default:
if (basicTypeOp == OpTypeInt) {
if (width == 16)
addCapability(CapabilityInt16);
else if (width == 8)
addCapability(CapabilityInt8);
else if (width == 64)
addCapability(CapabilityInt64);
} else if (basicTypeOp == OpTypeFloat) {
if (width == 16)
addCapability(CapabilityFloat16);
else if (width == 64)
addCapability(CapabilityFloat64);
}
break;
}
}
// Called for each instruction that resides in a block.
void Builder::postProcess(Instruction& inst)
{
// Add capabilities based simply on the opcode.
switch (inst.getOpCode()) {
case OpExtInst:
switch (inst.getImmediateOperand(1)) {
case GLSLstd450InterpolateAtCentroid:
case GLSLstd450InterpolateAtSample:
case GLSLstd450InterpolateAtOffset:
addCapability(CapabilityInterpolationFunction);
break;
default:
break;
}
break;
case OpDPdxFine:
case OpDPdyFine:
case OpFwidthFine:
case OpDPdxCoarse:
case OpDPdyCoarse:
case OpFwidthCoarse:
addCapability(CapabilityDerivativeControl);
break;
case OpImageQueryLod:
case OpImageQuerySize:
case OpImageQuerySizeLod:
case OpImageQuerySamples:
case OpImageQueryLevels:
addCapability(CapabilityImageQuery);
break;
case OpGroupNonUniformPartitionNV:
addExtension(E_SPV_NV_shader_subgroup_partitioned);
addCapability(CapabilityGroupNonUniformPartitionedNV);
break;
case OpLoad:
case OpStore:
{
// For any load/store to a PhysicalStorageBufferEXT, walk the accesschain
// index list to compute the misalignment. The pre-existing alignment value
// (set via Builder::AccessChain::alignment) only accounts for the base of
// the reference type and any scalar component selection in the accesschain,
// and this function computes the rest from the SPIR-V Offset decorations.
Instruction *accessChain = module.getInstruction(inst.getIdOperand(0));
if (accessChain->getOpCode() == OpAccessChain) {
Instruction *base = module.getInstruction(accessChain->getIdOperand(0));
// Get the type of the base of the access chain. It must be a pointer type.
Id typeId = base->getTypeId();
Instruction *type = module.getInstruction(typeId);
assert(type->getOpCode() == OpTypePointer);
if (type->getImmediateOperand(0) != StorageClassPhysicalStorageBufferEXT) {
break;
}
// Get the pointee type.
typeId = type->getIdOperand(1);
type = module.getInstruction(typeId);
// Walk the index list for the access chain. For each index, find any
// misalignment that can apply when accessing the member/element via
// Offset/ArrayStride/MatrixStride decorations, and bitwise OR them all
// together.
int alignment = 0;
for (int i = 1; i < accessChain->getNumOperands(); ++i) {
Instruction *idx = module.getInstruction(accessChain->getIdOperand(i));
if (type->getOpCode() == OpTypeStruct) {
assert(idx->getOpCode() == OpConstant);
unsigned int c = idx->getImmediateOperand(0);
const auto function = [&](const std::unique_ptr<Instruction>& decoration) {
if (decoration.get()->getOpCode() == OpMemberDecorate &&
decoration.get()->getIdOperand(0) == typeId &&
decoration.get()->getImmediateOperand(1) == c &&
(decoration.get()->getImmediateOperand(2) == DecorationOffset ||
decoration.get()->getImmediateOperand(2) == DecorationMatrixStride)) {
alignment |= decoration.get()->getImmediateOperand(3);
}
};
std::for_each(decorations.begin(), decorations.end(), function);
// get the next member type
typeId = type->getIdOperand(c);
type = module.getInstruction(typeId);
} else if (type->getOpCode() == OpTypeArray ||
type->getOpCode() == OpTypeRuntimeArray) {
const auto function = [&](const std::unique_ptr<Instruction>& decoration) {
if (decoration.get()->getOpCode() == OpDecorate &&
decoration.get()->getIdOperand(0) == typeId &&
decoration.get()->getImmediateOperand(1) == DecorationArrayStride) {
alignment |= decoration.get()->getImmediateOperand(2);
}
};
std::for_each(decorations.begin(), decorations.end(), function);
// Get the element type
typeId = type->getIdOperand(0);
type = module.getInstruction(typeId);
} else {
// Once we get to any non-aggregate type, we're done.
break;
}
}
assert(inst.getNumOperands() >= 3);
unsigned int memoryAccess = inst.getImmediateOperand((inst.getOpCode() == OpStore) ? 2 : 1);
assert(memoryAccess & MemoryAccessAlignedMask);
static_cast<void>(memoryAccess);
// Compute the index of the alignment operand.
int alignmentIdx = 2;
if (inst.getOpCode() == OpStore)
alignmentIdx++;
// Merge new and old (mis)alignment
alignment |= inst.getImmediateOperand(alignmentIdx);
// Pick the LSB
alignment = alignment & ~(alignment & (alignment-1));
// update the Aligned operand
inst.setImmediateOperand(alignmentIdx, alignment);
}
break;
}
default:
break;
}
// Checks based on type
if (inst.getTypeId() != NoType)
postProcessType(inst, inst.getTypeId());
for (int op = 0; op < inst.getNumOperands(); ++op) {
if (inst.isIdOperand(op)) {
// In blocks, these are always result ids, but we are relying on
// getTypeId() to return NoType for things like OpLabel.
if (getTypeId(inst.getIdOperand(op)) != NoType)
postProcessType(inst, getTypeId(inst.getIdOperand(op)));
}
}
}
// comment in header
void Builder::postProcessCFG()
{
// reachableBlocks is the set of blockss reached via control flow, or which are
// unreachable continue targert or unreachable merge.
std::unordered_set<const Block*> reachableBlocks;
std::unordered_map<Block*, Block*> headerForUnreachableContinue;
std::unordered_set<Block*> unreachableMerges;
std::unordered_set<Id> unreachableDefinitions;
// Collect IDs defined in unreachable blocks. For each function, label the
// reachable blocks first. Then for each unreachable block, collect the
// result IDs of the instructions in it.
for (auto fi = module.getFunctions().cbegin(); fi != module.getFunctions().cend(); fi++) {
Function* f = *fi;
Block* entry = f->getEntryBlock();
inReadableOrder(entry,
[&reachableBlocks, &unreachableMerges, &headerForUnreachableContinue]
(Block* b, ReachReason why, Block* header) {
reachableBlocks.insert(b);
if (why == ReachDeadContinue) headerForUnreachableContinue[b] = header;
if (why == ReachDeadMerge) unreachableMerges.insert(b);
});
for (auto bi = f->getBlocks().cbegin(); bi != f->getBlocks().cend(); bi++) {
Block* b = *bi;
if (unreachableMerges.count(b) != 0 || headerForUnreachableContinue.count(b) != 0) {
auto ii = b->getInstructions().cbegin();
++ii; // Keep potential decorations on the label.
for (; ii != b->getInstructions().cend(); ++ii)
unreachableDefinitions.insert(ii->get()->getResultId());
} else if (reachableBlocks.count(b) == 0) {
// The normal case for unreachable code. All definitions are considered dead.
for (auto ii = b->getInstructions().cbegin(); ii != b->getInstructions().cend(); ++ii)
unreachableDefinitions.insert(ii->get()->getResultId());
}
}
}
// Modify unreachable merge blocks and unreachable continue targets.
// Delete their contents.
for (auto mergeIter = unreachableMerges.begin(); mergeIter != unreachableMerges.end(); ++mergeIter) {
(*mergeIter)->rewriteAsCanonicalUnreachableMerge();
}
for (auto continueIter = headerForUnreachableContinue.begin();
continueIter != headerForUnreachableContinue.end();
++continueIter) {
Block* continue_target = continueIter->first;
Block* header = continueIter->second;
continue_target->rewriteAsCanonicalUnreachableContinue(header);
}
// Remove unneeded decorations, for unreachable instructions
decorations.erase(std::remove_if(decorations.begin(), decorations.end(),
[&unreachableDefinitions](std::unique_ptr<Instruction>& I) -> bool {
Id decoration_id = I.get()->getIdOperand(0);
return unreachableDefinitions.count(decoration_id) != 0;
}),
decorations.end());
}
// comment in header
void Builder::postProcessFeatures() {
// Add per-instruction capabilities, extensions, etc.,
// Look for any 8/16 bit type in physical storage buffer class, and set the
// appropriate capability. This happens in createSpvVariable for other storage
// classes, but there isn't always a variable for physical storage buffer.
for (int t = 0; t < (int)groupedTypes[OpTypePointer].size(); ++t) {
Instruction* type = groupedTypes[OpTypePointer][t];
if (type->getImmediateOperand(0) == (unsigned)StorageClassPhysicalStorageBufferEXT) {
if (containsType(type->getIdOperand(1), OpTypeInt, 8)) {
addIncorporatedExtension(spv::E_SPV_KHR_8bit_storage, spv::Spv_1_5);
addCapability(spv::CapabilityStorageBuffer8BitAccess);
}
if (containsType(type->getIdOperand(1), OpTypeInt, 16) ||
containsType(type->getIdOperand(1), OpTypeFloat, 16)) {
addIncorporatedExtension(spv::E_SPV_KHR_16bit_storage, spv::Spv_1_3);
addCapability(spv::CapabilityStorageBuffer16BitAccess);
}
}
}
// process all block-contained instructions
for (auto fi = module.getFunctions().cbegin(); fi != module.getFunctions().cend(); fi++) {
Function* f = *fi;
for (auto bi = f->getBlocks().cbegin(); bi != f->getBlocks().cend(); bi++) {
Block* b = *bi;
for (auto ii = b->getInstructions().cbegin(); ii != b->getInstructions().cend(); ii++)
postProcess(*ii->get());
// For all local variables that contain pointers to PhysicalStorageBufferEXT, check whether
// there is an existing restrict/aliased decoration. If we don't find one, add Aliased as the
// default.
for (auto vi = b->getLocalVariables().cbegin(); vi != b->getLocalVariables().cend(); vi++) {
const Instruction& inst = *vi->get();
Id resultId = inst.getResultId();
if (containsPhysicalStorageBufferOrArray(getDerefTypeId(resultId))) {
bool foundDecoration = false;
const auto function = [&](const std::unique_ptr<Instruction>& decoration) {
if (decoration.get()->getIdOperand(0) == resultId &&
decoration.get()->getOpCode() == OpDecorate &&
(decoration.get()->getImmediateOperand(1) == spv::DecorationAliasedPointerEXT ||
decoration.get()->getImmediateOperand(1) == spv::DecorationRestrictPointerEXT)) {
foundDecoration = true;
}
};
std::for_each(decorations.begin(), decorations.end(), function);
if (!foundDecoration) {
addDecoration(resultId, spv::DecorationAliasedPointerEXT);
}
}
}
}
}
// If any Vulkan memory model-specific functionality is used, update the
// OpMemoryModel to match.
if (capabilities.find(spv::CapabilityVulkanMemoryModelKHR) != capabilities.end()) {
memoryModel = spv::MemoryModelVulkanKHR;
addIncorporatedExtension(spv::E_SPV_KHR_vulkan_memory_model, spv::Spv_1_5);
}
// Add Aliased decoration if there's more than one Workgroup Block variable.
if (capabilities.find(spv::CapabilityWorkgroupMemoryExplicitLayoutKHR) != capabilities.end()) {
assert(entryPoints.size() == 1);
auto &ep = entryPoints[0];
std::vector<Id> workgroup_variables;
for (int i = 0; i < (int)ep->getNumOperands(); i++) {
if (!ep->isIdOperand(i))
continue;
const Id id = ep->getIdOperand(i);
const Instruction *instr = module.getInstruction(id);
if (instr->getOpCode() != spv::OpVariable)
continue;
if (instr->getImmediateOperand(0) == spv::StorageClassWorkgroup)
workgroup_variables.push_back(id);
}
if (workgroup_variables.size() > 1) {
for (size_t i = 0; i < workgroup_variables.size(); i++)
addDecoration(workgroup_variables[i], spv::DecorationAliased);
}
}
}
// SPIR-V requires that any instruction consuming the result of an OpSampledImage
// be in the same block as the OpSampledImage instruction. This pass goes finds
// uses of OpSampledImage where that is not the case and duplicates the
// OpSampledImage to be immediately before the instruction that consumes it.
// The old OpSampledImage is left in place, potentially with no users.
void Builder::postProcessSamplers()
{
// first, find all OpSampledImage instructions and store them in a map.
std::map<Id, Instruction*> sampledImageInstrs;
for (auto f: module.getFunctions()) {
for (auto b: f->getBlocks()) {
for (auto &i: b->getInstructions()) {
if (i->getOpCode() == spv::OpSampledImage) {
sampledImageInstrs[i->getResultId()] = i.get();
}
}
}
}
// next find all uses of the given ids and rewrite them if needed.
for (auto f: module.getFunctions()) {
for (auto b: f->getBlocks()) {
auto &instrs = b->getInstructions();
for (size_t idx = 0; idx < instrs.size(); idx++) {
Instruction *i = instrs[idx].get();
for (int opnum = 0; opnum < i->getNumOperands(); opnum++) {
// Is this operand of the current instruction the result of an OpSampledImage?
if (i->isIdOperand(opnum) &&
sampledImageInstrs.count(i->getIdOperand(opnum)))
{
Instruction *opSampImg = sampledImageInstrs[i->getIdOperand(opnum)];
if (i->getBlock() != opSampImg->getBlock()) {
Instruction *newInstr = new Instruction(getUniqueId(),
opSampImg->getTypeId(),
spv::OpSampledImage);
newInstr->addIdOperand(opSampImg->getIdOperand(0));
newInstr->addIdOperand(opSampImg->getIdOperand(1));
newInstr->setBlock(b);
// rewrite the user of the OpSampledImage to use the new instruction.
i->setIdOperand(opnum, newInstr->getResultId());
// insert the new OpSampledImage right before the current instruction.
instrs.insert(instrs.begin() + idx,
std::unique_ptr<Instruction>(newInstr));
idx++;
}
}
}
}
}
}
}
// comment in header
void Builder::postProcess(bool compileOnly)
{
// postProcessCFG needs an entrypoint to determine what is reachable, but if we are not creating an "executable" shader, we don't have an entrypoint
if (!compileOnly)
postProcessCFG();
postProcessFeatures();
postProcessSamplers();
}
}; // end spv namespace
|
0 | repos/glslang.zig/glslang | repos/glslang.zig/glslang/SPIRV/doc.cpp | //
// Copyright (C) 2014-2015 LunarG, Inc.
// Copyright (C) 2022-2024 Arm Limited.
// Modifications Copyright (C) 2020 Advanced Micro Devices, Inc. All rights reserved.
//
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
//
// Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
//
// Redistributions in binary form must reproduce the above
// copyright notice, this list of conditions and the following
// disclaimer in the documentation and/or other materials provided
// with the distribution.
//
// Neither the name of 3Dlabs Inc. Ltd. nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
// "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
// LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
// FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
// COPYRIGHT HOLDERS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
// INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
// BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
// LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
// CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
// LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
// ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
// POSSIBILITY OF SUCH DAMAGE.
//
// 1) Programmatically fill in instruction/operand information.
// This can be used for disassembly, printing documentation, etc.
//
// 2) Print documentation from this parameterization.
//
#include "doc.h"
#include <cstdio>
#include <cstring>
#include <algorithm>
#include <mutex>
namespace spv {
extern "C" {
// Include C-based headers that don't have a namespace
#include "GLSL.ext.KHR.h"
#include "GLSL.ext.EXT.h"
#include "GLSL.ext.AMD.h"
#include "GLSL.ext.NV.h"
#include "GLSL.ext.ARM.h"
#include "GLSL.ext.QCOM.h"
}
}
namespace spv {
//
// Whole set of functions that translate enumerants to their text strings for
// the specification (or their sanitized versions for auto-generating the
// spirv headers.
//
// Also, for masks the ceilings are declared next to these, to help keep them in sync.
// Ceilings should be
// - one more than the maximum value an enumerant takes on, for non-mask enumerants
// (for non-sparse enums, this is the number of enumerants)
// - the number of bits consumed by the set of masks
// (for non-sparse mask enums, this is the number of enumerants)
//
const char* SourceString(int source)
{
switch (source) {
case 0: return "Unknown";
case 1: return "ESSL";
case 2: return "GLSL";
case 3: return "OpenCL_C";
case 4: return "OpenCL_CPP";
case 5: return "HLSL";
default: return "Bad";
}
}
const char* ExecutionModelString(int model)
{
switch (model) {
case 0: return "Vertex";
case 1: return "TessellationControl";
case 2: return "TessellationEvaluation";
case 3: return "Geometry";
case 4: return "Fragment";
case 5: return "GLCompute";
case 6: return "Kernel";
case ExecutionModelTaskNV: return "TaskNV";
case ExecutionModelMeshNV: return "MeshNV";
case ExecutionModelTaskEXT: return "TaskEXT";
case ExecutionModelMeshEXT: return "MeshEXT";
default: return "Bad";
case ExecutionModelRayGenerationKHR: return "RayGenerationKHR";
case ExecutionModelIntersectionKHR: return "IntersectionKHR";
case ExecutionModelAnyHitKHR: return "AnyHitKHR";
case ExecutionModelClosestHitKHR: return "ClosestHitKHR";
case ExecutionModelMissKHR: return "MissKHR";
case ExecutionModelCallableKHR: return "CallableKHR";
}
}
const char* AddressingString(int addr)
{
switch (addr) {
case 0: return "Logical";
case 1: return "Physical32";
case 2: return "Physical64";
case AddressingModelPhysicalStorageBuffer64EXT: return "PhysicalStorageBuffer64EXT";
default: return "Bad";
}
}
const char* MemoryString(int mem)
{
switch (mem) {
case MemoryModelSimple: return "Simple";
case MemoryModelGLSL450: return "GLSL450";
case MemoryModelOpenCL: return "OpenCL";
case MemoryModelVulkanKHR: return "VulkanKHR";
default: return "Bad";
}
}
const int ExecutionModeCeiling = 40;
const char* ExecutionModeString(int mode)
{
switch (mode) {
case 0: return "Invocations";
case 1: return "SpacingEqual";
case 2: return "SpacingFractionalEven";
case 3: return "SpacingFractionalOdd";
case 4: return "VertexOrderCw";
case 5: return "VertexOrderCcw";
case 6: return "PixelCenterInteger";
case 7: return "OriginUpperLeft";
case 8: return "OriginLowerLeft";
case 9: return "EarlyFragmentTests";
case 10: return "PointMode";
case 11: return "Xfb";
case 12: return "DepthReplacing";
case 13: return "Bad";
case 14: return "DepthGreater";
case 15: return "DepthLess";
case 16: return "DepthUnchanged";
case 17: return "LocalSize";
case 18: return "LocalSizeHint";
case 19: return "InputPoints";
case 20: return "InputLines";
case 21: return "InputLinesAdjacency";
case 22: return "Triangles";
case 23: return "InputTrianglesAdjacency";
case 24: return "Quads";
case 25: return "Isolines";
case 26: return "OutputVertices";
case 27: return "OutputPoints";
case 28: return "OutputLineStrip";
case 29: return "OutputTriangleStrip";
case 30: return "VecTypeHint";
case 31: return "ContractionOff";
case 32: return "Bad";
case ExecutionModeInitializer: return "Initializer";
case ExecutionModeFinalizer: return "Finalizer";
case ExecutionModeSubgroupSize: return "SubgroupSize";
case ExecutionModeSubgroupsPerWorkgroup: return "SubgroupsPerWorkgroup";
case ExecutionModeSubgroupsPerWorkgroupId: return "SubgroupsPerWorkgroupId";
case ExecutionModeLocalSizeId: return "LocalSizeId";
case ExecutionModeLocalSizeHintId: return "LocalSizeHintId";
case ExecutionModePostDepthCoverage: return "PostDepthCoverage";
case ExecutionModeDenormPreserve: return "DenormPreserve";
case ExecutionModeDenormFlushToZero: return "DenormFlushToZero";
case ExecutionModeSignedZeroInfNanPreserve: return "SignedZeroInfNanPreserve";
case ExecutionModeRoundingModeRTE: return "RoundingModeRTE";
case ExecutionModeRoundingModeRTZ: return "RoundingModeRTZ";
case ExecutionModeEarlyAndLateFragmentTestsAMD: return "EarlyAndLateFragmentTestsAMD";
case ExecutionModeStencilRefUnchangedFrontAMD: return "StencilRefUnchangedFrontAMD";
case ExecutionModeStencilRefLessFrontAMD: return "StencilRefLessFrontAMD";
case ExecutionModeStencilRefGreaterBackAMD: return "StencilRefGreaterBackAMD";
case ExecutionModeStencilRefReplacingEXT: return "StencilRefReplacingEXT";
case ExecutionModeSubgroupUniformControlFlowKHR: return "SubgroupUniformControlFlow";
case ExecutionModeMaximallyReconvergesKHR: return "MaximallyReconverges";
case ExecutionModeOutputLinesNV: return "OutputLinesNV";
case ExecutionModeOutputPrimitivesNV: return "OutputPrimitivesNV";
case ExecutionModeOutputTrianglesNV: return "OutputTrianglesNV";
case ExecutionModeDerivativeGroupQuadsNV: return "DerivativeGroupQuadsNV";
case ExecutionModeDerivativeGroupLinearNV: return "DerivativeGroupLinearNV";
case ExecutionModePixelInterlockOrderedEXT: return "PixelInterlockOrderedEXT";
case ExecutionModePixelInterlockUnorderedEXT: return "PixelInterlockUnorderedEXT";
case ExecutionModeSampleInterlockOrderedEXT: return "SampleInterlockOrderedEXT";
case ExecutionModeSampleInterlockUnorderedEXT: return "SampleInterlockUnorderedEXT";
case ExecutionModeShadingRateInterlockOrderedEXT: return "ShadingRateInterlockOrderedEXT";
case ExecutionModeShadingRateInterlockUnorderedEXT: return "ShadingRateInterlockUnorderedEXT";
case ExecutionModeMaxWorkgroupSizeINTEL: return "MaxWorkgroupSizeINTEL";
case ExecutionModeMaxWorkDimINTEL: return "MaxWorkDimINTEL";
case ExecutionModeNoGlobalOffsetINTEL: return "NoGlobalOffsetINTEL";
case ExecutionModeNumSIMDWorkitemsINTEL: return "NumSIMDWorkitemsINTEL";
case ExecutionModeRequireFullQuadsKHR: return "RequireFullQuadsKHR";
case ExecutionModeQuadDerivativesKHR: return "QuadDerivativesKHR";
case ExecutionModeNonCoherentColorAttachmentReadEXT: return "NonCoherentColorAttachmentReadEXT";
case ExecutionModeNonCoherentDepthAttachmentReadEXT: return "NonCoherentDepthAttachmentReadEXT";
case ExecutionModeNonCoherentStencilAttachmentReadEXT: return "NonCoherentStencilAttachmentReadEXT";
case ExecutionModeCeiling:
default: return "Bad";
}
}
const char* StorageClassString(int StorageClass)
{
switch (StorageClass) {
case 0: return "UniformConstant";
case 1: return "Input";
case 2: return "Uniform";
case 3: return "Output";
case 4: return "Workgroup";
case 5: return "CrossWorkgroup";
case 6: return "Private";
case 7: return "Function";
case 8: return "Generic";
case 9: return "PushConstant";
case 10: return "AtomicCounter";
case 11: return "Image";
case 12: return "StorageBuffer";
case StorageClassRayPayloadKHR: return "RayPayloadKHR";
case StorageClassHitAttributeKHR: return "HitAttributeKHR";
case StorageClassIncomingRayPayloadKHR: return "IncomingRayPayloadKHR";
case StorageClassShaderRecordBufferKHR: return "ShaderRecordBufferKHR";
case StorageClassCallableDataKHR: return "CallableDataKHR";
case StorageClassIncomingCallableDataKHR: return "IncomingCallableDataKHR";
case StorageClassPhysicalStorageBufferEXT: return "PhysicalStorageBufferEXT";
case StorageClassTaskPayloadWorkgroupEXT: return "TaskPayloadWorkgroupEXT";
case StorageClassHitObjectAttributeNV: return "HitObjectAttributeNV";
case StorageClassTileImageEXT: return "TileImageEXT";
default: return "Bad";
}
}
const int DecorationCeiling = 45;
const char* DecorationString(int decoration)
{
switch (decoration) {
case 0: return "RelaxedPrecision";
case 1: return "SpecId";
case 2: return "Block";
case 3: return "BufferBlock";
case 4: return "RowMajor";
case 5: return "ColMajor";
case 6: return "ArrayStride";
case 7: return "MatrixStride";
case 8: return "GLSLShared";
case 9: return "GLSLPacked";
case 10: return "CPacked";
case 11: return "BuiltIn";
case 12: return "Bad";
case 13: return "NoPerspective";
case 14: return "Flat";
case 15: return "Patch";
case 16: return "Centroid";
case 17: return "Sample";
case 18: return "Invariant";
case 19: return "Restrict";
case 20: return "Aliased";
case 21: return "Volatile";
case 22: return "Constant";
case 23: return "Coherent";
case 24: return "NonWritable";
case 25: return "NonReadable";
case 26: return "Uniform";
case 27: return "Bad";
case 28: return "SaturatedConversion";
case 29: return "Stream";
case 30: return "Location";
case 31: return "Component";
case 32: return "Index";
case 33: return "Binding";
case 34: return "DescriptorSet";
case 35: return "Offset";
case 36: return "XfbBuffer";
case 37: return "XfbStride";
case 38: return "FuncParamAttr";
case 39: return "FP Rounding Mode";
case 40: return "FP Fast Math Mode";
case 41: return "Linkage Attributes";
case 42: return "NoContraction";
case 43: return "InputAttachmentIndex";
case 44: return "Alignment";
case DecorationCeiling:
default: return "Bad";
case DecorationWeightTextureQCOM: return "DecorationWeightTextureQCOM";
case DecorationBlockMatchTextureQCOM: return "DecorationBlockMatchTextureQCOM";
case DecorationBlockMatchSamplerQCOM: return "DecorationBlockMatchSamplerQCOM";
case DecorationExplicitInterpAMD: return "ExplicitInterpAMD";
case DecorationOverrideCoverageNV: return "OverrideCoverageNV";
case DecorationPassthroughNV: return "PassthroughNV";
case DecorationViewportRelativeNV: return "ViewportRelativeNV";
case DecorationSecondaryViewportRelativeNV: return "SecondaryViewportRelativeNV";
case DecorationPerPrimitiveNV: return "PerPrimitiveNV";
case DecorationPerViewNV: return "PerViewNV";
case DecorationPerTaskNV: return "PerTaskNV";
case DecorationPerVertexKHR: return "PerVertexKHR";
case DecorationNonUniformEXT: return "DecorationNonUniformEXT";
case DecorationHlslCounterBufferGOOGLE: return "DecorationHlslCounterBufferGOOGLE";
case DecorationHlslSemanticGOOGLE: return "DecorationHlslSemanticGOOGLE";
case DecorationRestrictPointerEXT: return "DecorationRestrictPointerEXT";
case DecorationAliasedPointerEXT: return "DecorationAliasedPointerEXT";
case DecorationHitObjectShaderRecordBufferNV: return "DecorationHitObjectShaderRecordBufferNV";
}
}
const char* BuiltInString(int builtIn)
{
switch (builtIn) {
case 0: return "Position";
case 1: return "PointSize";
case 2: return "Bad";
case 3: return "ClipDistance";
case 4: return "CullDistance";
case 5: return "VertexId";
case 6: return "InstanceId";
case 7: return "PrimitiveId";
case 8: return "InvocationId";
case 9: return "Layer";
case 10: return "ViewportIndex";
case 11: return "TessLevelOuter";
case 12: return "TessLevelInner";
case 13: return "TessCoord";
case 14: return "PatchVertices";
case 15: return "FragCoord";
case 16: return "PointCoord";
case 17: return "FrontFacing";
case 18: return "SampleId";
case 19: return "SamplePosition";
case 20: return "SampleMask";
case 21: return "Bad";
case 22: return "FragDepth";
case 23: return "HelperInvocation";
case 24: return "NumWorkgroups";
case 25: return "WorkgroupSize";
case 26: return "WorkgroupId";
case 27: return "LocalInvocationId";
case 28: return "GlobalInvocationId";
case 29: return "LocalInvocationIndex";
case 30: return "WorkDim";
case 31: return "GlobalSize";
case 32: return "EnqueuedWorkgroupSize";
case 33: return "GlobalOffset";
case 34: return "GlobalLinearId";
case 35: return "Bad";
case 36: return "SubgroupSize";
case 37: return "SubgroupMaxSize";
case 38: return "NumSubgroups";
case 39: return "NumEnqueuedSubgroups";
case 40: return "SubgroupId";
case 41: return "SubgroupLocalInvocationId";
case 42: return "VertexIndex"; // TBD: put next to VertexId?
case 43: return "InstanceIndex"; // TBD: put next to InstanceId?
case 4416: return "SubgroupEqMaskKHR";
case 4417: return "SubgroupGeMaskKHR";
case 4418: return "SubgroupGtMaskKHR";
case 4419: return "SubgroupLeMaskKHR";
case 4420: return "SubgroupLtMaskKHR";
case 4438: return "DeviceIndex";
case 4440: return "ViewIndex";
case 4424: return "BaseVertex";
case 4425: return "BaseInstance";
case 4426: return "DrawIndex";
case 4432: return "PrimitiveShadingRateKHR";
case 4444: return "ShadingRateKHR";
case 5014: return "FragStencilRefEXT";
case 4992: return "BaryCoordNoPerspAMD";
case 4993: return "BaryCoordNoPerspCentroidAMD";
case 4994: return "BaryCoordNoPerspSampleAMD";
case 4995: return "BaryCoordSmoothAMD";
case 4996: return "BaryCoordSmoothCentroidAMD";
case 4997: return "BaryCoordSmoothSampleAMD";
case 4998: return "BaryCoordPullModelAMD";
case BuiltInLaunchIdKHR: return "LaunchIdKHR";
case BuiltInLaunchSizeKHR: return "LaunchSizeKHR";
case BuiltInWorldRayOriginKHR: return "WorldRayOriginKHR";
case BuiltInWorldRayDirectionKHR: return "WorldRayDirectionKHR";
case BuiltInObjectRayOriginKHR: return "ObjectRayOriginKHR";
case BuiltInObjectRayDirectionKHR: return "ObjectRayDirectionKHR";
case BuiltInRayTminKHR: return "RayTminKHR";
case BuiltInRayTmaxKHR: return "RayTmaxKHR";
case BuiltInCullMaskKHR: return "CullMaskKHR";
case BuiltInHitTriangleVertexPositionsKHR: return "HitTriangleVertexPositionsKHR";
case BuiltInHitMicroTriangleVertexPositionsNV: return "HitMicroTriangleVertexPositionsNV";
case BuiltInHitMicroTriangleVertexBarycentricsNV: return "HitMicroTriangleVertexBarycentricsNV";
case BuiltInHitKindFrontFacingMicroTriangleNV: return "HitKindFrontFacingMicroTriangleNV";
case BuiltInHitKindBackFacingMicroTriangleNV: return "HitKindBackFacingMicroTriangleNV";
case BuiltInInstanceCustomIndexKHR: return "InstanceCustomIndexKHR";
case BuiltInRayGeometryIndexKHR: return "RayGeometryIndexKHR";
case BuiltInObjectToWorldKHR: return "ObjectToWorldKHR";
case BuiltInWorldToObjectKHR: return "WorldToObjectKHR";
case BuiltInHitTNV: return "HitTNV";
case BuiltInHitKindKHR: return "HitKindKHR";
case BuiltInIncomingRayFlagsKHR: return "IncomingRayFlagsKHR";
case BuiltInViewportMaskNV: return "ViewportMaskNV";
case BuiltInSecondaryPositionNV: return "SecondaryPositionNV";
case BuiltInSecondaryViewportMaskNV: return "SecondaryViewportMaskNV";
case BuiltInPositionPerViewNV: return "PositionPerViewNV";
case BuiltInViewportMaskPerViewNV: return "ViewportMaskPerViewNV";
// case BuiltInFragmentSizeNV: return "FragmentSizeNV"; // superseded by BuiltInFragSizeEXT
// case BuiltInInvocationsPerPixelNV: return "InvocationsPerPixelNV"; // superseded by BuiltInFragInvocationCountEXT
case BuiltInBaryCoordKHR: return "BaryCoordKHR";
case BuiltInBaryCoordNoPerspKHR: return "BaryCoordNoPerspKHR";
case BuiltInFragSizeEXT: return "FragSizeEXT";
case BuiltInFragInvocationCountEXT: return "FragInvocationCountEXT";
case 5264: return "FullyCoveredEXT";
case BuiltInTaskCountNV: return "TaskCountNV";
case BuiltInPrimitiveCountNV: return "PrimitiveCountNV";
case BuiltInPrimitiveIndicesNV: return "PrimitiveIndicesNV";
case BuiltInClipDistancePerViewNV: return "ClipDistancePerViewNV";
case BuiltInCullDistancePerViewNV: return "CullDistancePerViewNV";
case BuiltInLayerPerViewNV: return "LayerPerViewNV";
case BuiltInMeshViewCountNV: return "MeshViewCountNV";
case BuiltInMeshViewIndicesNV: return "MeshViewIndicesNV";
case BuiltInWarpsPerSMNV: return "WarpsPerSMNV";
case BuiltInSMCountNV: return "SMCountNV";
case BuiltInWarpIDNV: return "WarpIDNV";
case BuiltInSMIDNV: return "SMIDNV";
case BuiltInCurrentRayTimeNV: return "CurrentRayTimeNV";
case BuiltInPrimitivePointIndicesEXT: return "PrimitivePointIndicesEXT";
case BuiltInPrimitiveLineIndicesEXT: return "PrimitiveLineIndicesEXT";
case BuiltInPrimitiveTriangleIndicesEXT: return "PrimitiveTriangleIndicesEXT";
case BuiltInCullPrimitiveEXT: return "CullPrimitiveEXT";
case BuiltInCoreCountARM: return "CoreCountARM";
case BuiltInCoreIDARM: return "CoreIDARM";
case BuiltInCoreMaxIDARM: return "CoreMaxIDARM";
case BuiltInWarpIDARM: return "WarpIDARM";
case BuiltInWarpMaxIDARM: return "BuiltInWarpMaxIDARM";
default: return "Bad";
}
}
const char* DimensionString(int dim)
{
switch (dim) {
case 0: return "1D";
case 1: return "2D";
case 2: return "3D";
case 3: return "Cube";
case 4: return "Rect";
case 5: return "Buffer";
case 6: return "SubpassData";
case DimTileImageDataEXT: return "TileImageDataEXT";
default: return "Bad";
}
}
const char* SamplerAddressingModeString(int mode)
{
switch (mode) {
case 0: return "None";
case 1: return "ClampToEdge";
case 2: return "Clamp";
case 3: return "Repeat";
case 4: return "RepeatMirrored";
default: return "Bad";
}
}
const char* SamplerFilterModeString(int mode)
{
switch (mode) {
case 0: return "Nearest";
case 1: return "Linear";
default: return "Bad";
}
}
const char* ImageFormatString(int format)
{
switch (format) {
case 0: return "Unknown";
// ES/Desktop float
case 1: return "Rgba32f";
case 2: return "Rgba16f";
case 3: return "R32f";
case 4: return "Rgba8";
case 5: return "Rgba8Snorm";
// Desktop float
case 6: return "Rg32f";
case 7: return "Rg16f";
case 8: return "R11fG11fB10f";
case 9: return "R16f";
case 10: return "Rgba16";
case 11: return "Rgb10A2";
case 12: return "Rg16";
case 13: return "Rg8";
case 14: return "R16";
case 15: return "R8";
case 16: return "Rgba16Snorm";
case 17: return "Rg16Snorm";
case 18: return "Rg8Snorm";
case 19: return "R16Snorm";
case 20: return "R8Snorm";
// ES/Desktop int
case 21: return "Rgba32i";
case 22: return "Rgba16i";
case 23: return "Rgba8i";
case 24: return "R32i";
// Desktop int
case 25: return "Rg32i";
case 26: return "Rg16i";
case 27: return "Rg8i";
case 28: return "R16i";
case 29: return "R8i";
// ES/Desktop uint
case 30: return "Rgba32ui";
case 31: return "Rgba16ui";
case 32: return "Rgba8ui";
case 33: return "R32ui";
// Desktop uint
case 34: return "Rgb10a2ui";
case 35: return "Rg32ui";
case 36: return "Rg16ui";
case 37: return "Rg8ui";
case 38: return "R16ui";
case 39: return "R8ui";
case 40: return "R64ui";
case 41: return "R64i";
default:
return "Bad";
}
}
const char* ImageChannelOrderString(int format)
{
switch (format) {
case 0: return "R";
case 1: return "A";
case 2: return "RG";
case 3: return "RA";
case 4: return "RGB";
case 5: return "RGBA";
case 6: return "BGRA";
case 7: return "ARGB";
case 8: return "Intensity";
case 9: return "Luminance";
case 10: return "Rx";
case 11: return "RGx";
case 12: return "RGBx";
case 13: return "Depth";
case 14: return "DepthStencil";
case 15: return "sRGB";
case 16: return "sRGBx";
case 17: return "sRGBA";
case 18: return "sBGRA";
default:
return "Bad";
}
}
const char* ImageChannelDataTypeString(int type)
{
switch (type)
{
case 0: return "SnormInt8";
case 1: return "SnormInt16";
case 2: return "UnormInt8";
case 3: return "UnormInt16";
case 4: return "UnormShort565";
case 5: return "UnormShort555";
case 6: return "UnormInt101010";
case 7: return "SignedInt8";
case 8: return "SignedInt16";
case 9: return "SignedInt32";
case 10: return "UnsignedInt8";
case 11: return "UnsignedInt16";
case 12: return "UnsignedInt32";
case 13: return "HalfFloat";
case 14: return "Float";
case 15: return "UnormInt24";
case 16: return "UnormInt101010_2";
default:
return "Bad";
}
}
const int ImageOperandsCeiling = 14;
const char* ImageOperandsString(int format)
{
switch (format) {
case ImageOperandsBiasShift: return "Bias";
case ImageOperandsLodShift: return "Lod";
case ImageOperandsGradShift: return "Grad";
case ImageOperandsConstOffsetShift: return "ConstOffset";
case ImageOperandsOffsetShift: return "Offset";
case ImageOperandsConstOffsetsShift: return "ConstOffsets";
case ImageOperandsSampleShift: return "Sample";
case ImageOperandsMinLodShift: return "MinLod";
case ImageOperandsMakeTexelAvailableKHRShift: return "MakeTexelAvailableKHR";
case ImageOperandsMakeTexelVisibleKHRShift: return "MakeTexelVisibleKHR";
case ImageOperandsNonPrivateTexelKHRShift: return "NonPrivateTexelKHR";
case ImageOperandsVolatileTexelKHRShift: return "VolatileTexelKHR";
case ImageOperandsSignExtendShift: return "SignExtend";
case ImageOperandsZeroExtendShift: return "ZeroExtend";
case ImageOperandsCeiling:
default:
return "Bad";
}
}
const char* FPFastMathString(int mode)
{
switch (mode) {
case 0: return "NotNaN";
case 1: return "NotInf";
case 2: return "NSZ";
case 3: return "AllowRecip";
case 4: return "Fast";
default: return "Bad";
}
}
const char* FPRoundingModeString(int mode)
{
switch (mode) {
case 0: return "RTE";
case 1: return "RTZ";
case 2: return "RTP";
case 3: return "RTN";
default: return "Bad";
}
}
const char* LinkageTypeString(int type)
{
switch (type) {
case 0: return "Export";
case 1: return "Import";
default: return "Bad";
}
}
const char* FuncParamAttrString(int attr)
{
switch (attr) {
case 0: return "Zext";
case 1: return "Sext";
case 2: return "ByVal";
case 3: return "Sret";
case 4: return "NoAlias";
case 5: return "NoCapture";
case 6: return "NoWrite";
case 7: return "NoReadWrite";
default: return "Bad";
}
}
const char* AccessQualifierString(int attr)
{
switch (attr) {
case 0: return "ReadOnly";
case 1: return "WriteOnly";
case 2: return "ReadWrite";
default: return "Bad";
}
}
const int SelectControlCeiling = 2;
const char* SelectControlString(int cont)
{
switch (cont) {
case 0: return "Flatten";
case 1: return "DontFlatten";
case SelectControlCeiling:
default: return "Bad";
}
}
const int LoopControlCeiling = LoopControlPartialCountShift + 1;
const char* LoopControlString(int cont)
{
switch (cont) {
case LoopControlUnrollShift: return "Unroll";
case LoopControlDontUnrollShift: return "DontUnroll";
case LoopControlDependencyInfiniteShift: return "DependencyInfinite";
case LoopControlDependencyLengthShift: return "DependencyLength";
case LoopControlMinIterationsShift: return "MinIterations";
case LoopControlMaxIterationsShift: return "MaxIterations";
case LoopControlIterationMultipleShift: return "IterationMultiple";
case LoopControlPeelCountShift: return "PeelCount";
case LoopControlPartialCountShift: return "PartialCount";
case LoopControlCeiling:
default: return "Bad";
}
}
const int FunctionControlCeiling = 4;
const char* FunctionControlString(int cont)
{
switch (cont) {
case 0: return "Inline";
case 1: return "DontInline";
case 2: return "Pure";
case 3: return "Const";
case FunctionControlCeiling:
default: return "Bad";
}
}
const char* MemorySemanticsString(int mem)
{
// Note: No bits set (None) means "Relaxed"
switch (mem) {
case 0: return "Bad"; // Note: this is a placeholder for 'Consume'
case 1: return "Acquire";
case 2: return "Release";
case 3: return "AcquireRelease";
case 4: return "SequentiallyConsistent";
case 5: return "Bad"; // Note: reserved for future expansion
case 6: return "UniformMemory";
case 7: return "SubgroupMemory";
case 8: return "WorkgroupMemory";
case 9: return "CrossWorkgroupMemory";
case 10: return "AtomicCounterMemory";
case 11: return "ImageMemory";
default: return "Bad";
}
}
const int MemoryAccessCeiling = 6;
const char* MemoryAccessString(int mem)
{
switch (mem) {
case MemoryAccessVolatileShift: return "Volatile";
case MemoryAccessAlignedShift: return "Aligned";
case MemoryAccessNontemporalShift: return "Nontemporal";
case MemoryAccessMakePointerAvailableKHRShift: return "MakePointerAvailableKHR";
case MemoryAccessMakePointerVisibleKHRShift: return "MakePointerVisibleKHR";
case MemoryAccessNonPrivatePointerKHRShift: return "NonPrivatePointerKHR";
default: return "Bad";
}
}
const int CooperativeMatrixOperandsCeiling = 6;
const char* CooperativeMatrixOperandsString(int op)
{
switch (op) {
case CooperativeMatrixOperandsMatrixASignedComponentsKHRShift: return "ASignedComponentsKHR";
case CooperativeMatrixOperandsMatrixBSignedComponentsKHRShift: return "BSignedComponentsKHR";
case CooperativeMatrixOperandsMatrixCSignedComponentsKHRShift: return "CSignedComponentsKHR";
case CooperativeMatrixOperandsMatrixResultSignedComponentsKHRShift: return "ResultSignedComponentsKHR";
case CooperativeMatrixOperandsSaturatingAccumulationKHRShift: return "SaturatingAccumulationKHR";
default: return "Bad";
}
}
const char* ScopeString(int mem)
{
switch (mem) {
case 0: return "CrossDevice";
case 1: return "Device";
case 2: return "Workgroup";
case 3: return "Subgroup";
case 4: return "Invocation";
default: return "Bad";
}
}
const char* GroupOperationString(int gop)
{
switch (gop)
{
case GroupOperationReduce: return "Reduce";
case GroupOperationInclusiveScan: return "InclusiveScan";
case GroupOperationExclusiveScan: return "ExclusiveScan";
case GroupOperationClusteredReduce: return "ClusteredReduce";
case GroupOperationPartitionedReduceNV: return "PartitionedReduceNV";
case GroupOperationPartitionedInclusiveScanNV: return "PartitionedInclusiveScanNV";
case GroupOperationPartitionedExclusiveScanNV: return "PartitionedExclusiveScanNV";
default: return "Bad";
}
}
const char* KernelEnqueueFlagsString(int flag)
{
switch (flag)
{
case 0: return "NoWait";
case 1: return "WaitKernel";
case 2: return "WaitWorkGroup";
default: return "Bad";
}
}
const char* KernelProfilingInfoString(int info)
{
switch (info)
{
case 0: return "CmdExecTime";
default: return "Bad";
}
}
const char* CapabilityString(int info)
{
switch (info)
{
case 0: return "Matrix";
case 1: return "Shader";
case 2: return "Geometry";
case 3: return "Tessellation";
case 4: return "Addresses";
case 5: return "Linkage";
case 6: return "Kernel";
case 7: return "Vector16";
case 8: return "Float16Buffer";
case 9: return "Float16";
case 10: return "Float64";
case 11: return "Int64";
case 12: return "Int64Atomics";
case 13: return "ImageBasic";
case 14: return "ImageReadWrite";
case 15: return "ImageMipmap";
case 16: return "Bad";
case 17: return "Pipes";
case 18: return "Groups";
case 19: return "DeviceEnqueue";
case 20: return "LiteralSampler";
case 21: return "AtomicStorage";
case 22: return "Int16";
case 23: return "TessellationPointSize";
case 24: return "GeometryPointSize";
case 25: return "ImageGatherExtended";
case 26: return "Bad";
case 27: return "StorageImageMultisample";
case 28: return "UniformBufferArrayDynamicIndexing";
case 29: return "SampledImageArrayDynamicIndexing";
case 30: return "StorageBufferArrayDynamicIndexing";
case 31: return "StorageImageArrayDynamicIndexing";
case 32: return "ClipDistance";
case 33: return "CullDistance";
case 34: return "ImageCubeArray";
case 35: return "SampleRateShading";
case 36: return "ImageRect";
case 37: return "SampledRect";
case 38: return "GenericPointer";
case 39: return "Int8";
case 40: return "InputAttachment";
case 41: return "SparseResidency";
case 42: return "MinLod";
case 43: return "Sampled1D";
case 44: return "Image1D";
case 45: return "SampledCubeArray";
case 46: return "SampledBuffer";
case 47: return "ImageBuffer";
case 48: return "ImageMSArray";
case 49: return "StorageImageExtendedFormats";
case 50: return "ImageQuery";
case 51: return "DerivativeControl";
case 52: return "InterpolationFunction";
case 53: return "TransformFeedback";
case 54: return "GeometryStreams";
case 55: return "StorageImageReadWithoutFormat";
case 56: return "StorageImageWriteWithoutFormat";
case 57: return "MultiViewport";
case 61: return "GroupNonUniform";
case 62: return "GroupNonUniformVote";
case 63: return "GroupNonUniformArithmetic";
case 64: return "GroupNonUniformBallot";
case 65: return "GroupNonUniformShuffle";
case 66: return "GroupNonUniformShuffleRelative";
case 67: return "GroupNonUniformClustered";
case 68: return "GroupNonUniformQuad";
case CapabilitySubgroupBallotKHR: return "SubgroupBallotKHR";
case CapabilityDrawParameters: return "DrawParameters";
case CapabilitySubgroupVoteKHR: return "SubgroupVoteKHR";
case CapabilityGroupNonUniformRotateKHR: return "CapabilityGroupNonUniformRotateKHR";
case CapabilityStorageUniformBufferBlock16: return "StorageUniformBufferBlock16";
case CapabilityStorageUniform16: return "StorageUniform16";
case CapabilityStoragePushConstant16: return "StoragePushConstant16";
case CapabilityStorageInputOutput16: return "StorageInputOutput16";
case CapabilityStorageBuffer8BitAccess: return "StorageBuffer8BitAccess";
case CapabilityUniformAndStorageBuffer8BitAccess: return "UniformAndStorageBuffer8BitAccess";
case CapabilityStoragePushConstant8: return "StoragePushConstant8";
case CapabilityDeviceGroup: return "DeviceGroup";
case CapabilityMultiView: return "MultiView";
case CapabilityDenormPreserve: return "DenormPreserve";
case CapabilityDenormFlushToZero: return "DenormFlushToZero";
case CapabilitySignedZeroInfNanPreserve: return "SignedZeroInfNanPreserve";
case CapabilityRoundingModeRTE: return "RoundingModeRTE";
case CapabilityRoundingModeRTZ: return "RoundingModeRTZ";
case CapabilityStencilExportEXT: return "StencilExportEXT";
case CapabilityFloat16ImageAMD: return "Float16ImageAMD";
case CapabilityImageGatherBiasLodAMD: return "ImageGatherBiasLodAMD";
case CapabilityFragmentMaskAMD: return "FragmentMaskAMD";
case CapabilityImageReadWriteLodAMD: return "ImageReadWriteLodAMD";
case CapabilityAtomicStorageOps: return "AtomicStorageOps";
case CapabilitySampleMaskPostDepthCoverage: return "SampleMaskPostDepthCoverage";
case CapabilityGeometryShaderPassthroughNV: return "GeometryShaderPassthroughNV";
case CapabilityShaderViewportIndexLayerNV: return "ShaderViewportIndexLayerNV";
case CapabilityShaderViewportMaskNV: return "ShaderViewportMaskNV";
case CapabilityShaderStereoViewNV: return "ShaderStereoViewNV";
case CapabilityPerViewAttributesNV: return "PerViewAttributesNV";
case CapabilityGroupNonUniformPartitionedNV: return "GroupNonUniformPartitionedNV";
case CapabilityRayTracingNV: return "RayTracingNV";
case CapabilityRayTracingMotionBlurNV: return "RayTracingMotionBlurNV";
case CapabilityRayTracingKHR: return "RayTracingKHR";
case CapabilityRayCullMaskKHR: return "RayCullMaskKHR";
case CapabilityRayQueryKHR: return "RayQueryKHR";
case CapabilityRayTracingProvisionalKHR: return "RayTracingProvisionalKHR";
case CapabilityRayTraversalPrimitiveCullingKHR: return "RayTraversalPrimitiveCullingKHR";
case CapabilityRayTracingPositionFetchKHR: return "RayTracingPositionFetchKHR";
case CapabilityDisplacementMicromapNV: return "DisplacementMicromapNV";
case CapabilityRayTracingDisplacementMicromapNV: return "CapabilityRayTracingDisplacementMicromapNV";
case CapabilityRayQueryPositionFetchKHR: return "RayQueryPositionFetchKHR";
case CapabilityComputeDerivativeGroupQuadsNV: return "ComputeDerivativeGroupQuadsNV";
case CapabilityComputeDerivativeGroupLinearNV: return "ComputeDerivativeGroupLinearNV";
case CapabilityFragmentBarycentricKHR: return "FragmentBarycentricKHR";
case CapabilityMeshShadingNV: return "MeshShadingNV";
case CapabilityImageFootprintNV: return "ImageFootprintNV";
case CapabilityMeshShadingEXT: return "MeshShadingEXT";
// case CapabilityShadingRateNV: return "ShadingRateNV"; // superseded by FragmentDensityEXT
case CapabilitySampleMaskOverrideCoverageNV: return "SampleMaskOverrideCoverageNV";
case CapabilityFragmentDensityEXT: return "FragmentDensityEXT";
case CapabilityFragmentFullyCoveredEXT: return "FragmentFullyCoveredEXT";
case CapabilityShaderNonUniformEXT: return "ShaderNonUniformEXT";
case CapabilityRuntimeDescriptorArrayEXT: return "RuntimeDescriptorArrayEXT";
case CapabilityInputAttachmentArrayDynamicIndexingEXT: return "InputAttachmentArrayDynamicIndexingEXT";
case CapabilityUniformTexelBufferArrayDynamicIndexingEXT: return "UniformTexelBufferArrayDynamicIndexingEXT";
case CapabilityStorageTexelBufferArrayDynamicIndexingEXT: return "StorageTexelBufferArrayDynamicIndexingEXT";
case CapabilityUniformBufferArrayNonUniformIndexingEXT: return "UniformBufferArrayNonUniformIndexingEXT";
case CapabilitySampledImageArrayNonUniformIndexingEXT: return "SampledImageArrayNonUniformIndexingEXT";
case CapabilityStorageBufferArrayNonUniformIndexingEXT: return "StorageBufferArrayNonUniformIndexingEXT";
case CapabilityStorageImageArrayNonUniformIndexingEXT: return "StorageImageArrayNonUniformIndexingEXT";
case CapabilityInputAttachmentArrayNonUniformIndexingEXT: return "InputAttachmentArrayNonUniformIndexingEXT";
case CapabilityUniformTexelBufferArrayNonUniformIndexingEXT: return "UniformTexelBufferArrayNonUniformIndexingEXT";
case CapabilityStorageTexelBufferArrayNonUniformIndexingEXT: return "StorageTexelBufferArrayNonUniformIndexingEXT";
case CapabilityVulkanMemoryModelKHR: return "VulkanMemoryModelKHR";
case CapabilityVulkanMemoryModelDeviceScopeKHR: return "VulkanMemoryModelDeviceScopeKHR";
case CapabilityPhysicalStorageBufferAddressesEXT: return "PhysicalStorageBufferAddressesEXT";
case CapabilityVariablePointers: return "VariablePointers";
case CapabilityCooperativeMatrixNV: return "CooperativeMatrixNV";
case CapabilityCooperativeMatrixKHR: return "CooperativeMatrixKHR";
case CapabilityShaderSMBuiltinsNV: return "ShaderSMBuiltinsNV";
case CapabilityFragmentShaderSampleInterlockEXT: return "CapabilityFragmentShaderSampleInterlockEXT";
case CapabilityFragmentShaderPixelInterlockEXT: return "CapabilityFragmentShaderPixelInterlockEXT";
case CapabilityFragmentShaderShadingRateInterlockEXT: return "CapabilityFragmentShaderShadingRateInterlockEXT";
case CapabilityTileImageColorReadAccessEXT: return "TileImageColorReadAccessEXT";
case CapabilityTileImageDepthReadAccessEXT: return "TileImageDepthReadAccessEXT";
case CapabilityTileImageStencilReadAccessEXT: return "TileImageStencilReadAccessEXT";
case CapabilityCooperativeMatrixLayoutsARM: return "CooperativeMatrixLayoutsARM";
case CapabilityFragmentShadingRateKHR: return "FragmentShadingRateKHR";
case CapabilityDemoteToHelperInvocationEXT: return "DemoteToHelperInvocationEXT";
case CapabilityAtomicFloat16VectorNV: return "AtomicFloat16VectorNV";
case CapabilityShaderClockKHR: return "ShaderClockKHR";
case CapabilityQuadControlKHR: return "QuadControlKHR";
case CapabilityInt64ImageEXT: return "Int64ImageEXT";
case CapabilityIntegerFunctions2INTEL: return "CapabilityIntegerFunctions2INTEL";
case CapabilityExpectAssumeKHR: return "ExpectAssumeKHR";
case CapabilityAtomicFloat16AddEXT: return "AtomicFloat16AddEXT";
case CapabilityAtomicFloat32AddEXT: return "AtomicFloat32AddEXT";
case CapabilityAtomicFloat64AddEXT: return "AtomicFloat64AddEXT";
case CapabilityAtomicFloat16MinMaxEXT: return "AtomicFloat16MinMaxEXT";
case CapabilityAtomicFloat32MinMaxEXT: return "AtomicFloat32MinMaxEXT";
case CapabilityAtomicFloat64MinMaxEXT: return "AtomicFloat64MinMaxEXT";
case CapabilityWorkgroupMemoryExplicitLayoutKHR: return "CapabilityWorkgroupMemoryExplicitLayoutKHR";
case CapabilityWorkgroupMemoryExplicitLayout8BitAccessKHR: return "CapabilityWorkgroupMemoryExplicitLayout8BitAccessKHR";
case CapabilityWorkgroupMemoryExplicitLayout16BitAccessKHR: return "CapabilityWorkgroupMemoryExplicitLayout16BitAccessKHR";
case CapabilityCoreBuiltinsARM: return "CoreBuiltinsARM";
case CapabilityShaderInvocationReorderNV: return "ShaderInvocationReorderNV";
case CapabilityTextureSampleWeightedQCOM: return "TextureSampleWeightedQCOM";
case CapabilityTextureBoxFilterQCOM: return "TextureBoxFilterQCOM";
case CapabilityTextureBlockMatchQCOM: return "TextureBlockMatchQCOM";
case CapabilityTextureBlockMatch2QCOM: return "TextureBlockMatch2QCOM";
case CapabilityReplicatedCompositesEXT: return "CapabilityReplicatedCompositesEXT";
default: return "Bad";
}
}
const char* OpcodeString(int op)
{
switch (op) {
case 0: return "OpNop";
case 1: return "OpUndef";
case 2: return "OpSourceContinued";
case 3: return "OpSource";
case 4: return "OpSourceExtension";
case 5: return "OpName";
case 6: return "OpMemberName";
case 7: return "OpString";
case 8: return "OpLine";
case 9: return "Bad";
case 10: return "OpExtension";
case 11: return "OpExtInstImport";
case 12: return "OpExtInst";
case 13: return "Bad";
case 14: return "OpMemoryModel";
case 15: return "OpEntryPoint";
case 16: return "OpExecutionMode";
case 17: return "OpCapability";
case 18: return "Bad";
case 19: return "OpTypeVoid";
case 20: return "OpTypeBool";
case 21: return "OpTypeInt";
case 22: return "OpTypeFloat";
case 23: return "OpTypeVector";
case 24: return "OpTypeMatrix";
case 25: return "OpTypeImage";
case 26: return "OpTypeSampler";
case 27: return "OpTypeSampledImage";
case 28: return "OpTypeArray";
case 29: return "OpTypeRuntimeArray";
case 30: return "OpTypeStruct";
case 31: return "OpTypeOpaque";
case 32: return "OpTypePointer";
case 33: return "OpTypeFunction";
case 34: return "OpTypeEvent";
case 35: return "OpTypeDeviceEvent";
case 36: return "OpTypeReserveId";
case 37: return "OpTypeQueue";
case 38: return "OpTypePipe";
case 39: return "OpTypeForwardPointer";
case 40: return "Bad";
case 41: return "OpConstantTrue";
case 42: return "OpConstantFalse";
case 43: return "OpConstant";
case 44: return "OpConstantComposite";
case 45: return "OpConstantSampler";
case 46: return "OpConstantNull";
case 47: return "Bad";
case 48: return "OpSpecConstantTrue";
case 49: return "OpSpecConstantFalse";
case 50: return "OpSpecConstant";
case 51: return "OpSpecConstantComposite";
case 52: return "OpSpecConstantOp";
case 53: return "Bad";
case 54: return "OpFunction";
case 55: return "OpFunctionParameter";
case 56: return "OpFunctionEnd";
case 57: return "OpFunctionCall";
case 58: return "Bad";
case 59: return "OpVariable";
case 60: return "OpImageTexelPointer";
case 61: return "OpLoad";
case 62: return "OpStore";
case 63: return "OpCopyMemory";
case 64: return "OpCopyMemorySized";
case 65: return "OpAccessChain";
case 66: return "OpInBoundsAccessChain";
case 67: return "OpPtrAccessChain";
case 68: return "OpArrayLength";
case 69: return "OpGenericPtrMemSemantics";
case 70: return "OpInBoundsPtrAccessChain";
case 71: return "OpDecorate";
case 72: return "OpMemberDecorate";
case 73: return "OpDecorationGroup";
case 74: return "OpGroupDecorate";
case 75: return "OpGroupMemberDecorate";
case 76: return "Bad";
case 77: return "OpVectorExtractDynamic";
case 78: return "OpVectorInsertDynamic";
case 79: return "OpVectorShuffle";
case 80: return "OpCompositeConstruct";
case 81: return "OpCompositeExtract";
case 82: return "OpCompositeInsert";
case 83: return "OpCopyObject";
case 84: return "OpTranspose";
case OpCopyLogical: return "OpCopyLogical";
case 85: return "Bad";
case 86: return "OpSampledImage";
case 87: return "OpImageSampleImplicitLod";
case 88: return "OpImageSampleExplicitLod";
case 89: return "OpImageSampleDrefImplicitLod";
case 90: return "OpImageSampleDrefExplicitLod";
case 91: return "OpImageSampleProjImplicitLod";
case 92: return "OpImageSampleProjExplicitLod";
case 93: return "OpImageSampleProjDrefImplicitLod";
case 94: return "OpImageSampleProjDrefExplicitLod";
case 95: return "OpImageFetch";
case 96: return "OpImageGather";
case 97: return "OpImageDrefGather";
case 98: return "OpImageRead";
case 99: return "OpImageWrite";
case 100: return "OpImage";
case 101: return "OpImageQueryFormat";
case 102: return "OpImageQueryOrder";
case 103: return "OpImageQuerySizeLod";
case 104: return "OpImageQuerySize";
case 105: return "OpImageQueryLod";
case 106: return "OpImageQueryLevels";
case 107: return "OpImageQuerySamples";
case 108: return "Bad";
case 109: return "OpConvertFToU";
case 110: return "OpConvertFToS";
case 111: return "OpConvertSToF";
case 112: return "OpConvertUToF";
case 113: return "OpUConvert";
case 114: return "OpSConvert";
case 115: return "OpFConvert";
case 116: return "OpQuantizeToF16";
case 117: return "OpConvertPtrToU";
case 118: return "OpSatConvertSToU";
case 119: return "OpSatConvertUToS";
case 120: return "OpConvertUToPtr";
case 121: return "OpPtrCastToGeneric";
case 122: return "OpGenericCastToPtr";
case 123: return "OpGenericCastToPtrExplicit";
case 124: return "OpBitcast";
case 125: return "Bad";
case 126: return "OpSNegate";
case 127: return "OpFNegate";
case 128: return "OpIAdd";
case 129: return "OpFAdd";
case 130: return "OpISub";
case 131: return "OpFSub";
case 132: return "OpIMul";
case 133: return "OpFMul";
case 134: return "OpUDiv";
case 135: return "OpSDiv";
case 136: return "OpFDiv";
case 137: return "OpUMod";
case 138: return "OpSRem";
case 139: return "OpSMod";
case 140: return "OpFRem";
case 141: return "OpFMod";
case 142: return "OpVectorTimesScalar";
case 143: return "OpMatrixTimesScalar";
case 144: return "OpVectorTimesMatrix";
case 145: return "OpMatrixTimesVector";
case 146: return "OpMatrixTimesMatrix";
case 147: return "OpOuterProduct";
case 148: return "OpDot";
case 149: return "OpIAddCarry";
case 150: return "OpISubBorrow";
case 151: return "OpUMulExtended";
case 152: return "OpSMulExtended";
case 153: return "Bad";
case 154: return "OpAny";
case 155: return "OpAll";
case 156: return "OpIsNan";
case 157: return "OpIsInf";
case 158: return "OpIsFinite";
case 159: return "OpIsNormal";
case 160: return "OpSignBitSet";
case 161: return "OpLessOrGreater";
case 162: return "OpOrdered";
case 163: return "OpUnordered";
case 164: return "OpLogicalEqual";
case 165: return "OpLogicalNotEqual";
case 166: return "OpLogicalOr";
case 167: return "OpLogicalAnd";
case 168: return "OpLogicalNot";
case 169: return "OpSelect";
case 170: return "OpIEqual";
case 171: return "OpINotEqual";
case 172: return "OpUGreaterThan";
case 173: return "OpSGreaterThan";
case 174: return "OpUGreaterThanEqual";
case 175: return "OpSGreaterThanEqual";
case 176: return "OpULessThan";
case 177: return "OpSLessThan";
case 178: return "OpULessThanEqual";
case 179: return "OpSLessThanEqual";
case 180: return "OpFOrdEqual";
case 181: return "OpFUnordEqual";
case 182: return "OpFOrdNotEqual";
case 183: return "OpFUnordNotEqual";
case 184: return "OpFOrdLessThan";
case 185: return "OpFUnordLessThan";
case 186: return "OpFOrdGreaterThan";
case 187: return "OpFUnordGreaterThan";
case 188: return "OpFOrdLessThanEqual";
case 189: return "OpFUnordLessThanEqual";
case 190: return "OpFOrdGreaterThanEqual";
case 191: return "OpFUnordGreaterThanEqual";
case 192: return "Bad";
case 193: return "Bad";
case 194: return "OpShiftRightLogical";
case 195: return "OpShiftRightArithmetic";
case 196: return "OpShiftLeftLogical";
case 197: return "OpBitwiseOr";
case 198: return "OpBitwiseXor";
case 199: return "OpBitwiseAnd";
case 200: return "OpNot";
case 201: return "OpBitFieldInsert";
case 202: return "OpBitFieldSExtract";
case 203: return "OpBitFieldUExtract";
case 204: return "OpBitReverse";
case 205: return "OpBitCount";
case 206: return "Bad";
case 207: return "OpDPdx";
case 208: return "OpDPdy";
case 209: return "OpFwidth";
case 210: return "OpDPdxFine";
case 211: return "OpDPdyFine";
case 212: return "OpFwidthFine";
case 213: return "OpDPdxCoarse";
case 214: return "OpDPdyCoarse";
case 215: return "OpFwidthCoarse";
case 216: return "Bad";
case 217: return "Bad";
case 218: return "OpEmitVertex";
case 219: return "OpEndPrimitive";
case 220: return "OpEmitStreamVertex";
case 221: return "OpEndStreamPrimitive";
case 222: return "Bad";
case 223: return "Bad";
case 224: return "OpControlBarrier";
case 225: return "OpMemoryBarrier";
case 226: return "Bad";
case 227: return "OpAtomicLoad";
case 228: return "OpAtomicStore";
case 229: return "OpAtomicExchange";
case 230: return "OpAtomicCompareExchange";
case 231: return "OpAtomicCompareExchangeWeak";
case 232: return "OpAtomicIIncrement";
case 233: return "OpAtomicIDecrement";
case 234: return "OpAtomicIAdd";
case 235: return "OpAtomicISub";
case 236: return "OpAtomicSMin";
case 237: return "OpAtomicUMin";
case 238: return "OpAtomicSMax";
case 239: return "OpAtomicUMax";
case 240: return "OpAtomicAnd";
case 241: return "OpAtomicOr";
case 242: return "OpAtomicXor";
case 243: return "Bad";
case 244: return "Bad";
case 245: return "OpPhi";
case 246: return "OpLoopMerge";
case 247: return "OpSelectionMerge";
case 248: return "OpLabel";
case 249: return "OpBranch";
case 250: return "OpBranchConditional";
case 251: return "OpSwitch";
case 252: return "OpKill";
case 253: return "OpReturn";
case 254: return "OpReturnValue";
case 255: return "OpUnreachable";
case 256: return "OpLifetimeStart";
case 257: return "OpLifetimeStop";
case 258: return "Bad";
case 259: return "OpGroupAsyncCopy";
case 260: return "OpGroupWaitEvents";
case 261: return "OpGroupAll";
case 262: return "OpGroupAny";
case 263: return "OpGroupBroadcast";
case 264: return "OpGroupIAdd";
case 265: return "OpGroupFAdd";
case 266: return "OpGroupFMin";
case 267: return "OpGroupUMin";
case 268: return "OpGroupSMin";
case 269: return "OpGroupFMax";
case 270: return "OpGroupUMax";
case 271: return "OpGroupSMax";
case 272: return "Bad";
case 273: return "Bad";
case 274: return "OpReadPipe";
case 275: return "OpWritePipe";
case 276: return "OpReservedReadPipe";
case 277: return "OpReservedWritePipe";
case 278: return "OpReserveReadPipePackets";
case 279: return "OpReserveWritePipePackets";
case 280: return "OpCommitReadPipe";
case 281: return "OpCommitWritePipe";
case 282: return "OpIsValidReserveId";
case 283: return "OpGetNumPipePackets";
case 284: return "OpGetMaxPipePackets";
case 285: return "OpGroupReserveReadPipePackets";
case 286: return "OpGroupReserveWritePipePackets";
case 287: return "OpGroupCommitReadPipe";
case 288: return "OpGroupCommitWritePipe";
case 289: return "Bad";
case 290: return "Bad";
case 291: return "OpEnqueueMarker";
case 292: return "OpEnqueueKernel";
case 293: return "OpGetKernelNDrangeSubGroupCount";
case 294: return "OpGetKernelNDrangeMaxSubGroupSize";
case 295: return "OpGetKernelWorkGroupSize";
case 296: return "OpGetKernelPreferredWorkGroupSizeMultiple";
case 297: return "OpRetainEvent";
case 298: return "OpReleaseEvent";
case 299: return "OpCreateUserEvent";
case 300: return "OpIsValidEvent";
case 301: return "OpSetUserEventStatus";
case 302: return "OpCaptureEventProfilingInfo";
case 303: return "OpGetDefaultQueue";
case 304: return "OpBuildNDRange";
case 305: return "OpImageSparseSampleImplicitLod";
case 306: return "OpImageSparseSampleExplicitLod";
case 307: return "OpImageSparseSampleDrefImplicitLod";
case 308: return "OpImageSparseSampleDrefExplicitLod";
case 309: return "OpImageSparseSampleProjImplicitLod";
case 310: return "OpImageSparseSampleProjExplicitLod";
case 311: return "OpImageSparseSampleProjDrefImplicitLod";
case 312: return "OpImageSparseSampleProjDrefExplicitLod";
case 313: return "OpImageSparseFetch";
case 314: return "OpImageSparseGather";
case 315: return "OpImageSparseDrefGather";
case 316: return "OpImageSparseTexelsResident";
case 317: return "OpNoLine";
case 318: return "OpAtomicFlagTestAndSet";
case 319: return "OpAtomicFlagClear";
case 320: return "OpImageSparseRead";
case OpModuleProcessed: return "OpModuleProcessed";
case OpExecutionModeId: return "OpExecutionModeId";
case OpDecorateId: return "OpDecorateId";
case 333: return "OpGroupNonUniformElect";
case 334: return "OpGroupNonUniformAll";
case 335: return "OpGroupNonUniformAny";
case 336: return "OpGroupNonUniformAllEqual";
case 337: return "OpGroupNonUniformBroadcast";
case 338: return "OpGroupNonUniformBroadcastFirst";
case 339: return "OpGroupNonUniformBallot";
case 340: return "OpGroupNonUniformInverseBallot";
case 341: return "OpGroupNonUniformBallotBitExtract";
case 342: return "OpGroupNonUniformBallotBitCount";
case 343: return "OpGroupNonUniformBallotFindLSB";
case 344: return "OpGroupNonUniformBallotFindMSB";
case 345: return "OpGroupNonUniformShuffle";
case 346: return "OpGroupNonUniformShuffleXor";
case 347: return "OpGroupNonUniformShuffleUp";
case 348: return "OpGroupNonUniformShuffleDown";
case 349: return "OpGroupNonUniformIAdd";
case 350: return "OpGroupNonUniformFAdd";
case 351: return "OpGroupNonUniformIMul";
case 352: return "OpGroupNonUniformFMul";
case 353: return "OpGroupNonUniformSMin";
case 354: return "OpGroupNonUniformUMin";
case 355: return "OpGroupNonUniformFMin";
case 356: return "OpGroupNonUniformSMax";
case 357: return "OpGroupNonUniformUMax";
case 358: return "OpGroupNonUniformFMax";
case 359: return "OpGroupNonUniformBitwiseAnd";
case 360: return "OpGroupNonUniformBitwiseOr";
case 361: return "OpGroupNonUniformBitwiseXor";
case 362: return "OpGroupNonUniformLogicalAnd";
case 363: return "OpGroupNonUniformLogicalOr";
case 364: return "OpGroupNonUniformLogicalXor";
case 365: return "OpGroupNonUniformQuadBroadcast";
case 366: return "OpGroupNonUniformQuadSwap";
case OpTerminateInvocation: return "OpTerminateInvocation";
case 4421: return "OpSubgroupBallotKHR";
case 4422: return "OpSubgroupFirstInvocationKHR";
case 4428: return "OpSubgroupAllKHR";
case 4429: return "OpSubgroupAnyKHR";
case 4430: return "OpSubgroupAllEqualKHR";
case 4432: return "OpSubgroupReadInvocationKHR";
case 4433: return "OpExtInstWithForwardRefsKHR";
case OpGroupNonUniformQuadAllKHR: return "OpGroupNonUniformQuadAllKHR";
case OpGroupNonUniformQuadAnyKHR: return "OpGroupNonUniformQuadAnyKHR";
case OpAtomicFAddEXT: return "OpAtomicFAddEXT";
case OpAtomicFMinEXT: return "OpAtomicFMinEXT";
case OpAtomicFMaxEXT: return "OpAtomicFMaxEXT";
case OpAssumeTrueKHR: return "OpAssumeTrueKHR";
case OpExpectKHR: return "OpExpectKHR";
case 5000: return "OpGroupIAddNonUniformAMD";
case 5001: return "OpGroupFAddNonUniformAMD";
case 5002: return "OpGroupFMinNonUniformAMD";
case 5003: return "OpGroupUMinNonUniformAMD";
case 5004: return "OpGroupSMinNonUniformAMD";
case 5005: return "OpGroupFMaxNonUniformAMD";
case 5006: return "OpGroupUMaxNonUniformAMD";
case 5007: return "OpGroupSMaxNonUniformAMD";
case 5011: return "OpFragmentMaskFetchAMD";
case 5012: return "OpFragmentFetchAMD";
case OpReadClockKHR: return "OpReadClockKHR";
case OpDecorateStringGOOGLE: return "OpDecorateStringGOOGLE";
case OpMemberDecorateStringGOOGLE: return "OpMemberDecorateStringGOOGLE";
case OpReportIntersectionKHR: return "OpReportIntersectionKHR";
case OpIgnoreIntersectionNV: return "OpIgnoreIntersectionNV";
case OpIgnoreIntersectionKHR: return "OpIgnoreIntersectionKHR";
case OpTerminateRayNV: return "OpTerminateRayNV";
case OpTerminateRayKHR: return "OpTerminateRayKHR";
case OpTraceNV: return "OpTraceNV";
case OpTraceRayMotionNV: return "OpTraceRayMotionNV";
case OpTraceRayKHR: return "OpTraceRayKHR";
case OpTypeAccelerationStructureKHR: return "OpTypeAccelerationStructureKHR";
case OpExecuteCallableNV: return "OpExecuteCallableNV";
case OpExecuteCallableKHR: return "OpExecuteCallableKHR";
case OpConvertUToAccelerationStructureKHR: return "OpConvertUToAccelerationStructureKHR";
case OpGroupNonUniformPartitionNV: return "OpGroupNonUniformPartitionNV";
case OpImageSampleFootprintNV: return "OpImageSampleFootprintNV";
case OpWritePackedPrimitiveIndices4x8NV: return "OpWritePackedPrimitiveIndices4x8NV";
case OpEmitMeshTasksEXT: return "OpEmitMeshTasksEXT";
case OpSetMeshOutputsEXT: return "OpSetMeshOutputsEXT";
case OpGroupNonUniformRotateKHR: return "OpGroupNonUniformRotateKHR";
case OpTypeRayQueryKHR: return "OpTypeRayQueryKHR";
case OpRayQueryInitializeKHR: return "OpRayQueryInitializeKHR";
case OpRayQueryTerminateKHR: return "OpRayQueryTerminateKHR";
case OpRayQueryGenerateIntersectionKHR: return "OpRayQueryGenerateIntersectionKHR";
case OpRayQueryConfirmIntersectionKHR: return "OpRayQueryConfirmIntersectionKHR";
case OpRayQueryProceedKHR: return "OpRayQueryProceedKHR";
case OpRayQueryGetIntersectionTypeKHR: return "OpRayQueryGetIntersectionTypeKHR";
case OpRayQueryGetRayTMinKHR: return "OpRayQueryGetRayTMinKHR";
case OpRayQueryGetRayFlagsKHR: return "OpRayQueryGetRayFlagsKHR";
case OpRayQueryGetIntersectionTKHR: return "OpRayQueryGetIntersectionTKHR";
case OpRayQueryGetIntersectionInstanceCustomIndexKHR: return "OpRayQueryGetIntersectionInstanceCustomIndexKHR";
case OpRayQueryGetIntersectionInstanceIdKHR: return "OpRayQueryGetIntersectionInstanceIdKHR";
case OpRayQueryGetIntersectionInstanceShaderBindingTableRecordOffsetKHR: return "OpRayQueryGetIntersectionInstanceShaderBindingTableRecordOffsetKHR";
case OpRayQueryGetIntersectionGeometryIndexKHR: return "OpRayQueryGetIntersectionGeometryIndexKHR";
case OpRayQueryGetIntersectionPrimitiveIndexKHR: return "OpRayQueryGetIntersectionPrimitiveIndexKHR";
case OpRayQueryGetIntersectionBarycentricsKHR: return "OpRayQueryGetIntersectionBarycentricsKHR";
case OpRayQueryGetIntersectionFrontFaceKHR: return "OpRayQueryGetIntersectionFrontFaceKHR";
case OpRayQueryGetIntersectionCandidateAABBOpaqueKHR: return "OpRayQueryGetIntersectionCandidateAABBOpaqueKHR";
case OpRayQueryGetIntersectionObjectRayDirectionKHR: return "OpRayQueryGetIntersectionObjectRayDirectionKHR";
case OpRayQueryGetIntersectionObjectRayOriginKHR: return "OpRayQueryGetIntersectionObjectRayOriginKHR";
case OpRayQueryGetWorldRayDirectionKHR: return "OpRayQueryGetWorldRayDirectionKHR";
case OpRayQueryGetWorldRayOriginKHR: return "OpRayQueryGetWorldRayOriginKHR";
case OpRayQueryGetIntersectionObjectToWorldKHR: return "OpRayQueryGetIntersectionObjectToWorldKHR";
case OpRayQueryGetIntersectionWorldToObjectKHR: return "OpRayQueryGetIntersectionWorldToObjectKHR";
case OpRayQueryGetIntersectionTriangleVertexPositionsKHR: return "OpRayQueryGetIntersectionTriangleVertexPositionsKHR";
case OpTypeCooperativeMatrixNV: return "OpTypeCooperativeMatrixNV";
case OpCooperativeMatrixLoadNV: return "OpCooperativeMatrixLoadNV";
case OpCooperativeMatrixStoreNV: return "OpCooperativeMatrixStoreNV";
case OpCooperativeMatrixMulAddNV: return "OpCooperativeMatrixMulAddNV";
case OpCooperativeMatrixLengthNV: return "OpCooperativeMatrixLengthNV";
case OpTypeCooperativeMatrixKHR: return "OpTypeCooperativeMatrixKHR";
case OpCooperativeMatrixLoadKHR: return "OpCooperativeMatrixLoadKHR";
case OpCooperativeMatrixStoreKHR: return "OpCooperativeMatrixStoreKHR";
case OpCooperativeMatrixMulAddKHR: return "OpCooperativeMatrixMulAddKHR";
case OpCooperativeMatrixLengthKHR: return "OpCooperativeMatrixLengthKHR";
case OpDemoteToHelperInvocationEXT: return "OpDemoteToHelperInvocationEXT";
case OpIsHelperInvocationEXT: return "OpIsHelperInvocationEXT";
case OpBeginInvocationInterlockEXT: return "OpBeginInvocationInterlockEXT";
case OpEndInvocationInterlockEXT: return "OpEndInvocationInterlockEXT";
case OpTypeHitObjectNV: return "OpTypeHitObjectNV";
case OpHitObjectTraceRayNV: return "OpHitObjectTraceRayNV";
case OpHitObjectTraceRayMotionNV: return "OpHitObjectTraceRayMotionNV";
case OpHitObjectRecordHitNV: return "OpHitObjectRecordHitNV";
case OpHitObjectRecordHitMotionNV: return "OpHitObjectRecordHitMotionNV";
case OpHitObjectRecordHitWithIndexNV: return "OpHitObjectRecordHitWithIndexNV";
case OpHitObjectRecordHitWithIndexMotionNV: return "OpHitObjectRecordHitWithIndexMotionNV";
case OpHitObjectRecordMissNV: return "OpHitObjectRecordMissNV";
case OpHitObjectRecordMissMotionNV: return "OpHitObjectRecordMissMotionNV";
case OpHitObjectRecordEmptyNV: return "OpHitObjectRecordEmptyNV";
case OpHitObjectExecuteShaderNV: return "OpHitObjectExecuteShaderNV";
case OpReorderThreadWithHintNV: return "OpReorderThreadWithHintNV";
case OpReorderThreadWithHitObjectNV: return "OpReorderThreadWithHitObjectNV";
case OpHitObjectGetCurrentTimeNV: return "OpHitObjectGetCurrentTimeNV";
case OpHitObjectGetAttributesNV: return "OpHitObjectGetAttributesNV";
case OpHitObjectGetHitKindNV: return "OpHitObjectGetFrontFaceNV";
case OpHitObjectGetPrimitiveIndexNV: return "OpHitObjectGetPrimitiveIndexNV";
case OpHitObjectGetGeometryIndexNV: return "OpHitObjectGetGeometryIndexNV";
case OpHitObjectGetInstanceIdNV: return "OpHitObjectGetInstanceIdNV";
case OpHitObjectGetInstanceCustomIndexNV: return "OpHitObjectGetInstanceCustomIndexNV";
case OpHitObjectGetObjectRayDirectionNV: return "OpHitObjectGetObjectRayDirectionNV";
case OpHitObjectGetObjectRayOriginNV: return "OpHitObjectGetObjectRayOriginNV";
case OpHitObjectGetWorldRayDirectionNV: return "OpHitObjectGetWorldRayDirectionNV";
case OpHitObjectGetWorldRayOriginNV: return "OpHitObjectGetWorldRayOriginNV";
case OpHitObjectGetWorldToObjectNV: return "OpHitObjectGetWorldToObjectNV";
case OpHitObjectGetObjectToWorldNV: return "OpHitObjectGetObjectToWorldNV";
case OpHitObjectGetRayTMaxNV: return "OpHitObjectGetRayTMaxNV";
case OpHitObjectGetRayTMinNV: return "OpHitObjectGetRayTMinNV";
case OpHitObjectIsEmptyNV: return "OpHitObjectIsEmptyNV";
case OpHitObjectIsHitNV: return "OpHitObjectIsHitNV";
case OpHitObjectIsMissNV: return "OpHitObjectIsMissNV";
case OpHitObjectGetShaderBindingTableRecordIndexNV: return "OpHitObjectGetShaderBindingTableRecordIndexNV";
case OpHitObjectGetShaderRecordBufferHandleNV: return "OpHitObjectGetShaderRecordBufferHandleNV";
case OpFetchMicroTriangleVertexBarycentricNV: return "OpFetchMicroTriangleVertexBarycentricNV";
case OpFetchMicroTriangleVertexPositionNV: return "OpFetchMicroTriangleVertexPositionNV";
case OpColorAttachmentReadEXT: return "OpColorAttachmentReadEXT";
case OpDepthAttachmentReadEXT: return "OpDepthAttachmentReadEXT";
case OpStencilAttachmentReadEXT: return "OpStencilAttachmentReadEXT";
case OpImageSampleWeightedQCOM: return "OpImageSampleWeightedQCOM";
case OpImageBoxFilterQCOM: return "OpImageBoxFilterQCOM";
case OpImageBlockMatchSADQCOM: return "OpImageBlockMatchSADQCOM";
case OpImageBlockMatchSSDQCOM: return "OpImageBlockMatchSSDQCOM";
case OpImageBlockMatchWindowSSDQCOM: return "OpImageBlockMatchWindowSSDQCOM";
case OpImageBlockMatchWindowSADQCOM: return "OpImageBlockMatchWindowSADQCOM";
case OpImageBlockMatchGatherSSDQCOM: return "OpImageBlockMatchGatherSSDQCOM";
case OpImageBlockMatchGatherSADQCOM: return "OpImageBlockMatchGatherSADQCOM";
case OpConstantCompositeReplicateEXT: return "OpConstantCompositeReplicateEXT";
case OpSpecConstantCompositeReplicateEXT: return "OpSpecConstantCompositeReplicateEXT";
case OpCompositeConstructReplicateEXT: return "OpCompositeConstructReplicateEXT";
default:
return "Bad";
}
}
// The set of objects that hold all the instruction/operand
// parameterization information.
InstructionParameters InstructionDesc[OpCodeMask + 1];
OperandParameters ExecutionModeOperands[ExecutionModeCeiling];
OperandParameters DecorationOperands[DecorationCeiling];
EnumDefinition OperandClassParams[OperandCount];
EnumParameters ExecutionModeParams[ExecutionModeCeiling];
EnumParameters ImageOperandsParams[ImageOperandsCeiling];
EnumParameters DecorationParams[DecorationCeiling];
EnumParameters LoopControlParams[FunctionControlCeiling];
EnumParameters SelectionControlParams[SelectControlCeiling];
EnumParameters FunctionControlParams[FunctionControlCeiling];
EnumParameters MemoryAccessParams[MemoryAccessCeiling];
EnumParameters CooperativeMatrixOperandsParams[CooperativeMatrixOperandsCeiling];
// Set up all the parameterizing descriptions of the opcodes, operands, etc.
void Parameterize()
{
// only do this once.
static std::once_flag initialized;
std::call_once(initialized, [](){
// Exceptions to having a result <id> and a resulting type <id>.
// (Everything is initialized to have both).
InstructionDesc[OpNop].setResultAndType(false, false);
InstructionDesc[OpSource].setResultAndType(false, false);
InstructionDesc[OpSourceContinued].setResultAndType(false, false);
InstructionDesc[OpSourceExtension].setResultAndType(false, false);
InstructionDesc[OpExtension].setResultAndType(false, false);
InstructionDesc[OpExtInstImport].setResultAndType(true, false);
InstructionDesc[OpCapability].setResultAndType(false, false);
InstructionDesc[OpMemoryModel].setResultAndType(false, false);
InstructionDesc[OpEntryPoint].setResultAndType(false, false);
InstructionDesc[OpExecutionMode].setResultAndType(false, false);
InstructionDesc[OpExecutionModeId].setResultAndType(false, false);
InstructionDesc[OpTypeVoid].setResultAndType(true, false);
InstructionDesc[OpTypeBool].setResultAndType(true, false);
InstructionDesc[OpTypeInt].setResultAndType(true, false);
InstructionDesc[OpTypeFloat].setResultAndType(true, false);
InstructionDesc[OpTypeVector].setResultAndType(true, false);
InstructionDesc[OpTypeMatrix].setResultAndType(true, false);
InstructionDesc[OpTypeImage].setResultAndType(true, false);
InstructionDesc[OpTypeSampler].setResultAndType(true, false);
InstructionDesc[OpTypeSampledImage].setResultAndType(true, false);
InstructionDesc[OpTypeArray].setResultAndType(true, false);
InstructionDesc[OpTypeRuntimeArray].setResultAndType(true, false);
InstructionDesc[OpTypeStruct].setResultAndType(true, false);
InstructionDesc[OpTypeOpaque].setResultAndType(true, false);
InstructionDesc[OpTypePointer].setResultAndType(true, false);
InstructionDesc[OpTypeForwardPointer].setResultAndType(false, false);
InstructionDesc[OpTypeFunction].setResultAndType(true, false);
InstructionDesc[OpTypeEvent].setResultAndType(true, false);
InstructionDesc[OpTypeDeviceEvent].setResultAndType(true, false);
InstructionDesc[OpTypeReserveId].setResultAndType(true, false);
InstructionDesc[OpTypeQueue].setResultAndType(true, false);
InstructionDesc[OpTypePipe].setResultAndType(true, false);
InstructionDesc[OpFunctionEnd].setResultAndType(false, false);
InstructionDesc[OpStore].setResultAndType(false, false);
InstructionDesc[OpImageWrite].setResultAndType(false, false);
InstructionDesc[OpDecorationGroup].setResultAndType(true, false);
InstructionDesc[OpDecorate].setResultAndType(false, false);
InstructionDesc[OpDecorateId].setResultAndType(false, false);
InstructionDesc[OpDecorateStringGOOGLE].setResultAndType(false, false);
InstructionDesc[OpMemberDecorate].setResultAndType(false, false);
InstructionDesc[OpMemberDecorateStringGOOGLE].setResultAndType(false, false);
InstructionDesc[OpGroupDecorate].setResultAndType(false, false);
InstructionDesc[OpGroupMemberDecorate].setResultAndType(false, false);
InstructionDesc[OpName].setResultAndType(false, false);
InstructionDesc[OpMemberName].setResultAndType(false, false);
InstructionDesc[OpString].setResultAndType(true, false);
InstructionDesc[OpLine].setResultAndType(false, false);
InstructionDesc[OpNoLine].setResultAndType(false, false);
InstructionDesc[OpCopyMemory].setResultAndType(false, false);
InstructionDesc[OpCopyMemorySized].setResultAndType(false, false);
InstructionDesc[OpEmitVertex].setResultAndType(false, false);
InstructionDesc[OpEndPrimitive].setResultAndType(false, false);
InstructionDesc[OpEmitStreamVertex].setResultAndType(false, false);
InstructionDesc[OpEndStreamPrimitive].setResultAndType(false, false);
InstructionDesc[OpControlBarrier].setResultAndType(false, false);
InstructionDesc[OpMemoryBarrier].setResultAndType(false, false);
InstructionDesc[OpAtomicStore].setResultAndType(false, false);
InstructionDesc[OpLoopMerge].setResultAndType(false, false);
InstructionDesc[OpSelectionMerge].setResultAndType(false, false);
InstructionDesc[OpLabel].setResultAndType(true, false);
InstructionDesc[OpBranch].setResultAndType(false, false);
InstructionDesc[OpBranchConditional].setResultAndType(false, false);
InstructionDesc[OpSwitch].setResultAndType(false, false);
InstructionDesc[OpKill].setResultAndType(false, false);
InstructionDesc[OpTerminateInvocation].setResultAndType(false, false);
InstructionDesc[OpReturn].setResultAndType(false, false);
InstructionDesc[OpReturnValue].setResultAndType(false, false);
InstructionDesc[OpUnreachable].setResultAndType(false, false);
InstructionDesc[OpLifetimeStart].setResultAndType(false, false);
InstructionDesc[OpLifetimeStop].setResultAndType(false, false);
InstructionDesc[OpCommitReadPipe].setResultAndType(false, false);
InstructionDesc[OpCommitWritePipe].setResultAndType(false, false);
InstructionDesc[OpGroupCommitWritePipe].setResultAndType(false, false);
InstructionDesc[OpGroupCommitReadPipe].setResultAndType(false, false);
InstructionDesc[OpCaptureEventProfilingInfo].setResultAndType(false, false);
InstructionDesc[OpSetUserEventStatus].setResultAndType(false, false);
InstructionDesc[OpRetainEvent].setResultAndType(false, false);
InstructionDesc[OpReleaseEvent].setResultAndType(false, false);
InstructionDesc[OpGroupWaitEvents].setResultAndType(false, false);
InstructionDesc[OpAtomicFlagClear].setResultAndType(false, false);
InstructionDesc[OpModuleProcessed].setResultAndType(false, false);
InstructionDesc[OpTypeCooperativeMatrixNV].setResultAndType(true, false);
InstructionDesc[OpCooperativeMatrixStoreNV].setResultAndType(false, false);
InstructionDesc[OpTypeCooperativeMatrixKHR].setResultAndType(true, false);
InstructionDesc[OpCooperativeMatrixStoreKHR].setResultAndType(false, false);
InstructionDesc[OpBeginInvocationInterlockEXT].setResultAndType(false, false);
InstructionDesc[OpEndInvocationInterlockEXT].setResultAndType(false, false);
InstructionDesc[OpAssumeTrueKHR].setResultAndType(false, false);
// Specific additional context-dependent operands
ExecutionModeOperands[ExecutionModeInvocations].push(OperandLiteralNumber, "'Number of <<Invocation,invocations>>'");
ExecutionModeOperands[ExecutionModeLocalSize].push(OperandLiteralNumber, "'x size'");
ExecutionModeOperands[ExecutionModeLocalSize].push(OperandLiteralNumber, "'y size'");
ExecutionModeOperands[ExecutionModeLocalSize].push(OperandLiteralNumber, "'z size'");
ExecutionModeOperands[ExecutionModeLocalSizeHint].push(OperandLiteralNumber, "'x size'");
ExecutionModeOperands[ExecutionModeLocalSizeHint].push(OperandLiteralNumber, "'y size'");
ExecutionModeOperands[ExecutionModeLocalSizeHint].push(OperandLiteralNumber, "'z size'");
ExecutionModeOperands[ExecutionModeOutputVertices].push(OperandLiteralNumber, "'Vertex count'");
ExecutionModeOperands[ExecutionModeVecTypeHint].push(OperandLiteralNumber, "'Vector type'");
DecorationOperands[DecorationStream].push(OperandLiteralNumber, "'Stream Number'");
DecorationOperands[DecorationLocation].push(OperandLiteralNumber, "'Location'");
DecorationOperands[DecorationComponent].push(OperandLiteralNumber, "'Component'");
DecorationOperands[DecorationIndex].push(OperandLiteralNumber, "'Index'");
DecorationOperands[DecorationBinding].push(OperandLiteralNumber, "'Binding Point'");
DecorationOperands[DecorationDescriptorSet].push(OperandLiteralNumber, "'Descriptor Set'");
DecorationOperands[DecorationOffset].push(OperandLiteralNumber, "'Byte Offset'");
DecorationOperands[DecorationXfbBuffer].push(OperandLiteralNumber, "'XFB Buffer Number'");
DecorationOperands[DecorationXfbStride].push(OperandLiteralNumber, "'XFB Stride'");
DecorationOperands[DecorationArrayStride].push(OperandLiteralNumber, "'Array Stride'");
DecorationOperands[DecorationMatrixStride].push(OperandLiteralNumber, "'Matrix Stride'");
DecorationOperands[DecorationBuiltIn].push(OperandLiteralNumber, "See <<BuiltIn,*BuiltIn*>>");
DecorationOperands[DecorationFPRoundingMode].push(OperandFPRoundingMode, "'Floating-Point Rounding Mode'");
DecorationOperands[DecorationFPFastMathMode].push(OperandFPFastMath, "'Fast-Math Mode'");
DecorationOperands[DecorationLinkageAttributes].push(OperandLiteralString, "'Name'");
DecorationOperands[DecorationLinkageAttributes].push(OperandLinkageType, "'Linkage Type'");
DecorationOperands[DecorationFuncParamAttr].push(OperandFuncParamAttr, "'Function Parameter Attribute'");
DecorationOperands[DecorationSpecId].push(OperandLiteralNumber, "'Specialization Constant ID'");
DecorationOperands[DecorationInputAttachmentIndex].push(OperandLiteralNumber, "'Attachment Index'");
DecorationOperands[DecorationAlignment].push(OperandLiteralNumber, "'Alignment'");
OperandClassParams[OperandSource].set(0, SourceString, nullptr);
OperandClassParams[OperandExecutionModel].set(0, ExecutionModelString, nullptr);
OperandClassParams[OperandAddressing].set(0, AddressingString, nullptr);
OperandClassParams[OperandMemory].set(0, MemoryString, nullptr);
OperandClassParams[OperandExecutionMode].set(ExecutionModeCeiling, ExecutionModeString, ExecutionModeParams);
OperandClassParams[OperandExecutionMode].setOperands(ExecutionModeOperands);
OperandClassParams[OperandStorage].set(0, StorageClassString, nullptr);
OperandClassParams[OperandDimensionality].set(0, DimensionString, nullptr);
OperandClassParams[OperandSamplerAddressingMode].set(0, SamplerAddressingModeString, nullptr);
OperandClassParams[OperandSamplerFilterMode].set(0, SamplerFilterModeString, nullptr);
OperandClassParams[OperandSamplerImageFormat].set(0, ImageFormatString, nullptr);
OperandClassParams[OperandImageChannelOrder].set(0, ImageChannelOrderString, nullptr);
OperandClassParams[OperandImageChannelDataType].set(0, ImageChannelDataTypeString, nullptr);
OperandClassParams[OperandImageOperands].set(ImageOperandsCeiling, ImageOperandsString, ImageOperandsParams, true);
OperandClassParams[OperandFPFastMath].set(0, FPFastMathString, nullptr, true);
OperandClassParams[OperandFPRoundingMode].set(0, FPRoundingModeString, nullptr);
OperandClassParams[OperandLinkageType].set(0, LinkageTypeString, nullptr);
OperandClassParams[OperandFuncParamAttr].set(0, FuncParamAttrString, nullptr);
OperandClassParams[OperandAccessQualifier].set(0, AccessQualifierString, nullptr);
OperandClassParams[OperandDecoration].set(DecorationCeiling, DecorationString, DecorationParams);
OperandClassParams[OperandDecoration].setOperands(DecorationOperands);
OperandClassParams[OperandBuiltIn].set(0, BuiltInString, nullptr);
OperandClassParams[OperandSelect].set(SelectControlCeiling, SelectControlString, SelectionControlParams, true);
OperandClassParams[OperandLoop].set(LoopControlCeiling, LoopControlString, LoopControlParams, true);
OperandClassParams[OperandFunction].set(FunctionControlCeiling, FunctionControlString, FunctionControlParams, true);
OperandClassParams[OperandMemorySemantics].set(0, MemorySemanticsString, nullptr, true);
OperandClassParams[OperandMemoryAccess].set(MemoryAccessCeiling, MemoryAccessString, MemoryAccessParams, true);
OperandClassParams[OperandScope].set(0, ScopeString, nullptr);
OperandClassParams[OperandGroupOperation].set(0, GroupOperationString, nullptr);
OperandClassParams[OperandKernelEnqueueFlags].set(0, KernelEnqueueFlagsString, nullptr);
OperandClassParams[OperandKernelProfilingInfo].set(0, KernelProfilingInfoString, nullptr, true);
OperandClassParams[OperandCapability].set(0, CapabilityString, nullptr);
OperandClassParams[OperandCooperativeMatrixOperands].set(CooperativeMatrixOperandsCeiling, CooperativeMatrixOperandsString, CooperativeMatrixOperandsParams, true);
OperandClassParams[OperandOpcode].set(OpCodeMask + 1, OpcodeString, nullptr);
// set name of operator, an initial set of <id> style operands, and the description
InstructionDesc[OpSource].operands.push(OperandSource, "");
InstructionDesc[OpSource].operands.push(OperandLiteralNumber, "'Version'");
InstructionDesc[OpSource].operands.push(OperandId, "'File'", true);
InstructionDesc[OpSource].operands.push(OperandLiteralString, "'Source'", true);
InstructionDesc[OpSourceContinued].operands.push(OperandLiteralString, "'Continued Source'");
InstructionDesc[OpSourceExtension].operands.push(OperandLiteralString, "'Extension'");
InstructionDesc[OpName].operands.push(OperandId, "'Target'");
InstructionDesc[OpName].operands.push(OperandLiteralString, "'Name'");
InstructionDesc[OpMemberName].operands.push(OperandId, "'Type'");
InstructionDesc[OpMemberName].operands.push(OperandLiteralNumber, "'Member'");
InstructionDesc[OpMemberName].operands.push(OperandLiteralString, "'Name'");
InstructionDesc[OpString].operands.push(OperandLiteralString, "'String'");
InstructionDesc[OpLine].operands.push(OperandId, "'File'");
InstructionDesc[OpLine].operands.push(OperandLiteralNumber, "'Line'");
InstructionDesc[OpLine].operands.push(OperandLiteralNumber, "'Column'");
InstructionDesc[OpExtension].operands.push(OperandLiteralString, "'Name'");
InstructionDesc[OpExtInstImport].operands.push(OperandLiteralString, "'Name'");
InstructionDesc[OpCapability].operands.push(OperandCapability, "'Capability'");
InstructionDesc[OpMemoryModel].operands.push(OperandAddressing, "");
InstructionDesc[OpMemoryModel].operands.push(OperandMemory, "");
InstructionDesc[OpEntryPoint].operands.push(OperandExecutionModel, "");
InstructionDesc[OpEntryPoint].operands.push(OperandId, "'Entry Point'");
InstructionDesc[OpEntryPoint].operands.push(OperandLiteralString, "'Name'");
InstructionDesc[OpEntryPoint].operands.push(OperandVariableIds, "'Interface'");
InstructionDesc[OpExecutionMode].operands.push(OperandId, "'Entry Point'");
InstructionDesc[OpExecutionMode].operands.push(OperandExecutionMode, "'Mode'");
InstructionDesc[OpExecutionMode].operands.push(OperandOptionalLiteral, "See <<Execution_Mode,Execution Mode>>");
InstructionDesc[OpExecutionModeId].operands.push(OperandId, "'Entry Point'");
InstructionDesc[OpExecutionModeId].operands.push(OperandExecutionMode, "'Mode'");
InstructionDesc[OpExecutionModeId].operands.push(OperandVariableIds, "See <<Execution_Mode,Execution Mode>>");
InstructionDesc[OpTypeInt].operands.push(OperandLiteralNumber, "'Width'");
InstructionDesc[OpTypeInt].operands.push(OperandLiteralNumber, "'Signedness'");
InstructionDesc[OpTypeFloat].operands.push(OperandLiteralNumber, "'Width'");
InstructionDesc[OpTypeVector].operands.push(OperandId, "'Component Type'");
InstructionDesc[OpTypeVector].operands.push(OperandLiteralNumber, "'Component Count'");
InstructionDesc[OpTypeMatrix].operands.push(OperandId, "'Column Type'");
InstructionDesc[OpTypeMatrix].operands.push(OperandLiteralNumber, "'Column Count'");
InstructionDesc[OpTypeImage].operands.push(OperandId, "'Sampled Type'");
InstructionDesc[OpTypeImage].operands.push(OperandDimensionality, "");
InstructionDesc[OpTypeImage].operands.push(OperandLiteralNumber, "'Depth'");
InstructionDesc[OpTypeImage].operands.push(OperandLiteralNumber, "'Arrayed'");
InstructionDesc[OpTypeImage].operands.push(OperandLiteralNumber, "'MS'");
InstructionDesc[OpTypeImage].operands.push(OperandLiteralNumber, "'Sampled'");
InstructionDesc[OpTypeImage].operands.push(OperandSamplerImageFormat, "");
InstructionDesc[OpTypeImage].operands.push(OperandAccessQualifier, "", true);
InstructionDesc[OpTypeSampledImage].operands.push(OperandId, "'Image Type'");
InstructionDesc[OpTypeArray].operands.push(OperandId, "'Element Type'");
InstructionDesc[OpTypeArray].operands.push(OperandId, "'Length'");
InstructionDesc[OpTypeRuntimeArray].operands.push(OperandId, "'Element Type'");
InstructionDesc[OpTypeStruct].operands.push(OperandVariableIds, "'Member 0 type', +\n'member 1 type', +\n...");
InstructionDesc[OpTypeOpaque].operands.push(OperandLiteralString, "The name of the opaque type.");
InstructionDesc[OpTypePointer].operands.push(OperandStorage, "");
InstructionDesc[OpTypePointer].operands.push(OperandId, "'Type'");
InstructionDesc[OpTypeForwardPointer].operands.push(OperandId, "'Pointer Type'");
InstructionDesc[OpTypeForwardPointer].operands.push(OperandStorage, "");
InstructionDesc[OpTypePipe].operands.push(OperandAccessQualifier, "'Qualifier'");
InstructionDesc[OpTypeFunction].operands.push(OperandId, "'Return Type'");
InstructionDesc[OpTypeFunction].operands.push(OperandVariableIds, "'Parameter 0 Type', +\n'Parameter 1 Type', +\n...");
InstructionDesc[OpConstant].operands.push(OperandVariableLiterals, "'Value'");
InstructionDesc[OpConstantComposite].operands.push(OperandVariableIds, "'Constituents'");
InstructionDesc[OpConstantSampler].operands.push(OperandSamplerAddressingMode, "");
InstructionDesc[OpConstantSampler].operands.push(OperandLiteralNumber, "'Param'");
InstructionDesc[OpConstantSampler].operands.push(OperandSamplerFilterMode, "");
InstructionDesc[OpSpecConstant].operands.push(OperandVariableLiterals, "'Value'");
InstructionDesc[OpSpecConstantComposite].operands.push(OperandVariableIds, "'Constituents'");
InstructionDesc[OpSpecConstantOp].operands.push(OperandLiteralNumber, "'Opcode'");
InstructionDesc[OpSpecConstantOp].operands.push(OperandVariableIds, "'Operands'");
InstructionDesc[OpVariable].operands.push(OperandStorage, "");
InstructionDesc[OpVariable].operands.push(OperandId, "'Initializer'", true);
InstructionDesc[OpFunction].operands.push(OperandFunction, "");
InstructionDesc[OpFunction].operands.push(OperandId, "'Function Type'");
InstructionDesc[OpFunctionCall].operands.push(OperandId, "'Function'");
InstructionDesc[OpFunctionCall].operands.push(OperandVariableIds, "'Argument 0', +\n'Argument 1', +\n...");
InstructionDesc[OpExtInst].operands.push(OperandId, "'Set'");
InstructionDesc[OpExtInst].operands.push(OperandLiteralNumber, "'Instruction'");
InstructionDesc[OpExtInst].operands.push(OperandVariableIds, "'Operand 1', +\n'Operand 2', +\n...");
InstructionDesc[OpExtInstWithForwardRefsKHR].operands.push(OperandId, "'Set'");
InstructionDesc[OpExtInstWithForwardRefsKHR].operands.push(OperandLiteralNumber, "'Instruction'");
InstructionDesc[OpExtInstWithForwardRefsKHR].operands.push(OperandVariableIds, "'Operand 1', +\n'Operand 2', +\n...");
InstructionDesc[OpLoad].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpLoad].operands.push(OperandMemoryAccess, "", true);
InstructionDesc[OpLoad].operands.push(OperandLiteralNumber, "", true);
InstructionDesc[OpLoad].operands.push(OperandId, "", true);
InstructionDesc[OpStore].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpStore].operands.push(OperandId, "'Object'");
InstructionDesc[OpStore].operands.push(OperandMemoryAccess, "", true);
InstructionDesc[OpStore].operands.push(OperandLiteralNumber, "", true);
InstructionDesc[OpStore].operands.push(OperandId, "", true);
InstructionDesc[OpPhi].operands.push(OperandVariableIds, "'Variable, Parent, ...'");
InstructionDesc[OpDecorate].operands.push(OperandId, "'Target'");
InstructionDesc[OpDecorate].operands.push(OperandDecoration, "");
InstructionDesc[OpDecorate].operands.push(OperandVariableLiterals, "See <<Decoration,'Decoration'>>.");
InstructionDesc[OpDecorateId].operands.push(OperandId, "'Target'");
InstructionDesc[OpDecorateId].operands.push(OperandDecoration, "");
InstructionDesc[OpDecorateId].operands.push(OperandVariableIds, "See <<Decoration,'Decoration'>>.");
InstructionDesc[OpDecorateStringGOOGLE].operands.push(OperandId, "'Target'");
InstructionDesc[OpDecorateStringGOOGLE].operands.push(OperandDecoration, "");
InstructionDesc[OpDecorateStringGOOGLE].operands.push(OperandVariableLiteralStrings, "'Literal Strings'");
InstructionDesc[OpMemberDecorate].operands.push(OperandId, "'Structure Type'");
InstructionDesc[OpMemberDecorate].operands.push(OperandLiteralNumber, "'Member'");
InstructionDesc[OpMemberDecorate].operands.push(OperandDecoration, "");
InstructionDesc[OpMemberDecorate].operands.push(OperandVariableLiterals, "See <<Decoration,'Decoration'>>.");
InstructionDesc[OpMemberDecorateStringGOOGLE].operands.push(OperandId, "'Structure Type'");
InstructionDesc[OpMemberDecorateStringGOOGLE].operands.push(OperandLiteralNumber, "'Member'");
InstructionDesc[OpMemberDecorateStringGOOGLE].operands.push(OperandDecoration, "");
InstructionDesc[OpMemberDecorateStringGOOGLE].operands.push(OperandVariableLiteralStrings, "'Literal Strings'");
InstructionDesc[OpGroupDecorate].operands.push(OperandId, "'Decoration Group'");
InstructionDesc[OpGroupDecorate].operands.push(OperandVariableIds, "'Targets'");
InstructionDesc[OpGroupMemberDecorate].operands.push(OperandId, "'Decoration Group'");
InstructionDesc[OpGroupMemberDecorate].operands.push(OperandVariableIdLiteral, "'Targets'");
InstructionDesc[OpVectorExtractDynamic].operands.push(OperandId, "'Vector'");
InstructionDesc[OpVectorExtractDynamic].operands.push(OperandId, "'Index'");
InstructionDesc[OpVectorInsertDynamic].operands.push(OperandId, "'Vector'");
InstructionDesc[OpVectorInsertDynamic].operands.push(OperandId, "'Component'");
InstructionDesc[OpVectorInsertDynamic].operands.push(OperandId, "'Index'");
InstructionDesc[OpVectorShuffle].operands.push(OperandId, "'Vector 1'");
InstructionDesc[OpVectorShuffle].operands.push(OperandId, "'Vector 2'");
InstructionDesc[OpVectorShuffle].operands.push(OperandVariableLiterals, "'Components'");
InstructionDesc[OpCompositeConstruct].operands.push(OperandVariableIds, "'Constituents'");
InstructionDesc[OpCompositeExtract].operands.push(OperandId, "'Composite'");
InstructionDesc[OpCompositeExtract].operands.push(OperandVariableLiterals, "'Indexes'");
InstructionDesc[OpCompositeInsert].operands.push(OperandId, "'Object'");
InstructionDesc[OpCompositeInsert].operands.push(OperandId, "'Composite'");
InstructionDesc[OpCompositeInsert].operands.push(OperandVariableLiterals, "'Indexes'");
InstructionDesc[OpCopyObject].operands.push(OperandId, "'Operand'");
InstructionDesc[OpCopyMemory].operands.push(OperandId, "'Target'");
InstructionDesc[OpCopyMemory].operands.push(OperandId, "'Source'");
InstructionDesc[OpCopyMemory].operands.push(OperandMemoryAccess, "", true);
InstructionDesc[OpCopyMemorySized].operands.push(OperandId, "'Target'");
InstructionDesc[OpCopyMemorySized].operands.push(OperandId, "'Source'");
InstructionDesc[OpCopyMemorySized].operands.push(OperandId, "'Size'");
InstructionDesc[OpCopyMemorySized].operands.push(OperandMemoryAccess, "", true);
InstructionDesc[OpSampledImage].operands.push(OperandId, "'Image'");
InstructionDesc[OpSampledImage].operands.push(OperandId, "'Sampler'");
InstructionDesc[OpImage].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageRead].operands.push(OperandId, "'Image'");
InstructionDesc[OpImageRead].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageRead].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageRead].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageWrite].operands.push(OperandId, "'Image'");
InstructionDesc[OpImageWrite].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageWrite].operands.push(OperandId, "'Texel'");
InstructionDesc[OpImageWrite].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageWrite].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSampleImplicitLod].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSampleImplicitLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSampleImplicitLod].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSampleImplicitLod].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSampleExplicitLod].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSampleExplicitLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSampleExplicitLod].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSampleExplicitLod].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSampleDrefImplicitLod].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSampleDrefImplicitLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSampleDrefImplicitLod].operands.push(OperandId, "'D~ref~'");
InstructionDesc[OpImageSampleDrefImplicitLod].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSampleDrefImplicitLod].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSampleDrefExplicitLod].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSampleDrefExplicitLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSampleDrefExplicitLod].operands.push(OperandId, "'D~ref~'");
InstructionDesc[OpImageSampleDrefExplicitLod].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSampleDrefExplicitLod].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSampleProjImplicitLod].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSampleProjImplicitLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSampleProjImplicitLod].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSampleProjImplicitLod].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSampleProjExplicitLod].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSampleProjExplicitLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSampleProjExplicitLod].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSampleProjExplicitLod].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSampleProjDrefImplicitLod].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSampleProjDrefImplicitLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSampleProjDrefImplicitLod].operands.push(OperandId, "'D~ref~'");
InstructionDesc[OpImageSampleProjDrefImplicitLod].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSampleProjDrefImplicitLod].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSampleProjDrefExplicitLod].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSampleProjDrefExplicitLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSampleProjDrefExplicitLod].operands.push(OperandId, "'D~ref~'");
InstructionDesc[OpImageSampleProjDrefExplicitLod].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSampleProjDrefExplicitLod].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageFetch].operands.push(OperandId, "'Image'");
InstructionDesc[OpImageFetch].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageFetch].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageFetch].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageGather].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageGather].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageGather].operands.push(OperandId, "'Component'");
InstructionDesc[OpImageGather].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageGather].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageDrefGather].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageDrefGather].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageDrefGather].operands.push(OperandId, "'D~ref~'");
InstructionDesc[OpImageDrefGather].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageDrefGather].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSparseSampleImplicitLod].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSparseSampleImplicitLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSparseSampleImplicitLod].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSparseSampleImplicitLod].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSparseSampleExplicitLod].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSparseSampleExplicitLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSparseSampleExplicitLod].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSparseSampleExplicitLod].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSparseSampleDrefImplicitLod].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSparseSampleDrefImplicitLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSparseSampleDrefImplicitLod].operands.push(OperandId, "'D~ref~'");
InstructionDesc[OpImageSparseSampleDrefImplicitLod].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSparseSampleDrefImplicitLod].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSparseSampleDrefExplicitLod].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSparseSampleDrefExplicitLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSparseSampleDrefExplicitLod].operands.push(OperandId, "'D~ref~'");
InstructionDesc[OpImageSparseSampleDrefExplicitLod].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSparseSampleDrefExplicitLod].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSparseSampleProjImplicitLod].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSparseSampleProjImplicitLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSparseSampleProjImplicitLod].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSparseSampleProjImplicitLod].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSparseSampleProjExplicitLod].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSparseSampleProjExplicitLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSparseSampleProjExplicitLod].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSparseSampleProjExplicitLod].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSparseSampleProjDrefImplicitLod].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSparseSampleProjDrefImplicitLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSparseSampleProjDrefImplicitLod].operands.push(OperandId, "'D~ref~'");
InstructionDesc[OpImageSparseSampleProjDrefImplicitLod].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSparseSampleProjDrefImplicitLod].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSparseSampleProjDrefExplicitLod].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSparseSampleProjDrefExplicitLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSparseSampleProjDrefExplicitLod].operands.push(OperandId, "'D~ref~'");
InstructionDesc[OpImageSparseSampleProjDrefExplicitLod].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSparseSampleProjDrefExplicitLod].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSparseFetch].operands.push(OperandId, "'Image'");
InstructionDesc[OpImageSparseFetch].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSparseFetch].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSparseFetch].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSparseGather].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSparseGather].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSparseGather].operands.push(OperandId, "'Component'");
InstructionDesc[OpImageSparseGather].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSparseGather].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSparseDrefGather].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSparseDrefGather].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSparseDrefGather].operands.push(OperandId, "'D~ref~'");
InstructionDesc[OpImageSparseDrefGather].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSparseDrefGather].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSparseRead].operands.push(OperandId, "'Image'");
InstructionDesc[OpImageSparseRead].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSparseRead].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSparseRead].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpImageSparseTexelsResident].operands.push(OperandId, "'Resident Code'");
InstructionDesc[OpImageQuerySizeLod].operands.push(OperandId, "'Image'");
InstructionDesc[OpImageQuerySizeLod].operands.push(OperandId, "'Level of Detail'");
InstructionDesc[OpImageQuerySize].operands.push(OperandId, "'Image'");
InstructionDesc[OpImageQueryLod].operands.push(OperandId, "'Image'");
InstructionDesc[OpImageQueryLod].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageQueryLevels].operands.push(OperandId, "'Image'");
InstructionDesc[OpImageQuerySamples].operands.push(OperandId, "'Image'");
InstructionDesc[OpImageQueryFormat].operands.push(OperandId, "'Image'");
InstructionDesc[OpImageQueryOrder].operands.push(OperandId, "'Image'");
InstructionDesc[OpAccessChain].operands.push(OperandId, "'Base'");
InstructionDesc[OpAccessChain].operands.push(OperandVariableIds, "'Indexes'");
InstructionDesc[OpInBoundsAccessChain].operands.push(OperandId, "'Base'");
InstructionDesc[OpInBoundsAccessChain].operands.push(OperandVariableIds, "'Indexes'");
InstructionDesc[OpPtrAccessChain].operands.push(OperandId, "'Base'");
InstructionDesc[OpPtrAccessChain].operands.push(OperandId, "'Element'");
InstructionDesc[OpPtrAccessChain].operands.push(OperandVariableIds, "'Indexes'");
InstructionDesc[OpInBoundsPtrAccessChain].operands.push(OperandId, "'Base'");
InstructionDesc[OpInBoundsPtrAccessChain].operands.push(OperandId, "'Element'");
InstructionDesc[OpInBoundsPtrAccessChain].operands.push(OperandVariableIds, "'Indexes'");
InstructionDesc[OpSNegate].operands.push(OperandId, "'Operand'");
InstructionDesc[OpFNegate].operands.push(OperandId, "'Operand'");
InstructionDesc[OpNot].operands.push(OperandId, "'Operand'");
InstructionDesc[OpAny].operands.push(OperandId, "'Vector'");
InstructionDesc[OpAll].operands.push(OperandId, "'Vector'");
InstructionDesc[OpConvertFToU].operands.push(OperandId, "'Float Value'");
InstructionDesc[OpConvertFToS].operands.push(OperandId, "'Float Value'");
InstructionDesc[OpConvertSToF].operands.push(OperandId, "'Signed Value'");
InstructionDesc[OpConvertUToF].operands.push(OperandId, "'Unsigned Value'");
InstructionDesc[OpUConvert].operands.push(OperandId, "'Unsigned Value'");
InstructionDesc[OpSConvert].operands.push(OperandId, "'Signed Value'");
InstructionDesc[OpFConvert].operands.push(OperandId, "'Float Value'");
InstructionDesc[OpSatConvertSToU].operands.push(OperandId, "'Signed Value'");
InstructionDesc[OpSatConvertUToS].operands.push(OperandId, "'Unsigned Value'");
InstructionDesc[OpConvertPtrToU].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpConvertUToPtr].operands.push(OperandId, "'Integer Value'");
InstructionDesc[OpPtrCastToGeneric].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpGenericCastToPtr].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpGenericCastToPtrExplicit].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpGenericCastToPtrExplicit].operands.push(OperandStorage, "'Storage'");
InstructionDesc[OpGenericPtrMemSemantics].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpBitcast].operands.push(OperandId, "'Operand'");
InstructionDesc[OpQuantizeToF16].operands.push(OperandId, "'Value'");
InstructionDesc[OpTranspose].operands.push(OperandId, "'Matrix'");
InstructionDesc[OpCopyLogical].operands.push(OperandId, "'Operand'");
InstructionDesc[OpIsNan].operands.push(OperandId, "'x'");
InstructionDesc[OpIsInf].operands.push(OperandId, "'x'");
InstructionDesc[OpIsFinite].operands.push(OperandId, "'x'");
InstructionDesc[OpIsNormal].operands.push(OperandId, "'x'");
InstructionDesc[OpSignBitSet].operands.push(OperandId, "'x'");
InstructionDesc[OpLessOrGreater].operands.push(OperandId, "'x'");
InstructionDesc[OpLessOrGreater].operands.push(OperandId, "'y'");
InstructionDesc[OpOrdered].operands.push(OperandId, "'x'");
InstructionDesc[OpOrdered].operands.push(OperandId, "'y'");
InstructionDesc[OpUnordered].operands.push(OperandId, "'x'");
InstructionDesc[OpUnordered].operands.push(OperandId, "'y'");
InstructionDesc[OpArrayLength].operands.push(OperandId, "'Structure'");
InstructionDesc[OpArrayLength].operands.push(OperandLiteralNumber, "'Array member'");
InstructionDesc[OpIAdd].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpIAdd].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFAdd].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFAdd].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpISub].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpISub].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFSub].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFSub].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpIMul].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpIMul].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFMul].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFMul].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpUDiv].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpUDiv].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpSDiv].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpSDiv].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFDiv].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFDiv].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpUMod].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpUMod].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpSRem].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpSRem].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpSMod].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpSMod].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFRem].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFRem].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFMod].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFMod].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpVectorTimesScalar].operands.push(OperandId, "'Vector'");
InstructionDesc[OpVectorTimesScalar].operands.push(OperandId, "'Scalar'");
InstructionDesc[OpMatrixTimesScalar].operands.push(OperandId, "'Matrix'");
InstructionDesc[OpMatrixTimesScalar].operands.push(OperandId, "'Scalar'");
InstructionDesc[OpVectorTimesMatrix].operands.push(OperandId, "'Vector'");
InstructionDesc[OpVectorTimesMatrix].operands.push(OperandId, "'Matrix'");
InstructionDesc[OpMatrixTimesVector].operands.push(OperandId, "'Matrix'");
InstructionDesc[OpMatrixTimesVector].operands.push(OperandId, "'Vector'");
InstructionDesc[OpMatrixTimesMatrix].operands.push(OperandId, "'LeftMatrix'");
InstructionDesc[OpMatrixTimesMatrix].operands.push(OperandId, "'RightMatrix'");
InstructionDesc[OpOuterProduct].operands.push(OperandId, "'Vector 1'");
InstructionDesc[OpOuterProduct].operands.push(OperandId, "'Vector 2'");
InstructionDesc[OpDot].operands.push(OperandId, "'Vector 1'");
InstructionDesc[OpDot].operands.push(OperandId, "'Vector 2'");
InstructionDesc[OpIAddCarry].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpIAddCarry].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpISubBorrow].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpISubBorrow].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpUMulExtended].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpUMulExtended].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpSMulExtended].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpSMulExtended].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpShiftRightLogical].operands.push(OperandId, "'Base'");
InstructionDesc[OpShiftRightLogical].operands.push(OperandId, "'Shift'");
InstructionDesc[OpShiftRightArithmetic].operands.push(OperandId, "'Base'");
InstructionDesc[OpShiftRightArithmetic].operands.push(OperandId, "'Shift'");
InstructionDesc[OpShiftLeftLogical].operands.push(OperandId, "'Base'");
InstructionDesc[OpShiftLeftLogical].operands.push(OperandId, "'Shift'");
InstructionDesc[OpLogicalOr].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpLogicalOr].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpLogicalAnd].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpLogicalAnd].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpLogicalEqual].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpLogicalEqual].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpLogicalNotEqual].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpLogicalNotEqual].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpLogicalNot].operands.push(OperandId, "'Operand'");
InstructionDesc[OpBitwiseOr].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpBitwiseOr].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpBitwiseXor].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpBitwiseXor].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpBitwiseAnd].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpBitwiseAnd].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpBitFieldInsert].operands.push(OperandId, "'Base'");
InstructionDesc[OpBitFieldInsert].operands.push(OperandId, "'Insert'");
InstructionDesc[OpBitFieldInsert].operands.push(OperandId, "'Offset'");
InstructionDesc[OpBitFieldInsert].operands.push(OperandId, "'Count'");
InstructionDesc[OpBitFieldSExtract].operands.push(OperandId, "'Base'");
InstructionDesc[OpBitFieldSExtract].operands.push(OperandId, "'Offset'");
InstructionDesc[OpBitFieldSExtract].operands.push(OperandId, "'Count'");
InstructionDesc[OpBitFieldUExtract].operands.push(OperandId, "'Base'");
InstructionDesc[OpBitFieldUExtract].operands.push(OperandId, "'Offset'");
InstructionDesc[OpBitFieldUExtract].operands.push(OperandId, "'Count'");
InstructionDesc[OpBitReverse].operands.push(OperandId, "'Base'");
InstructionDesc[OpBitCount].operands.push(OperandId, "'Base'");
InstructionDesc[OpSelect].operands.push(OperandId, "'Condition'");
InstructionDesc[OpSelect].operands.push(OperandId, "'Object 1'");
InstructionDesc[OpSelect].operands.push(OperandId, "'Object 2'");
InstructionDesc[OpIEqual].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpIEqual].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFOrdEqual].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFOrdEqual].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFUnordEqual].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFUnordEqual].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpINotEqual].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpINotEqual].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFOrdNotEqual].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFOrdNotEqual].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFUnordNotEqual].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFUnordNotEqual].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpULessThan].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpULessThan].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpSLessThan].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpSLessThan].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFOrdLessThan].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFOrdLessThan].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFUnordLessThan].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFUnordLessThan].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpUGreaterThan].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpUGreaterThan].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpSGreaterThan].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpSGreaterThan].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFOrdGreaterThan].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFOrdGreaterThan].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFUnordGreaterThan].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFUnordGreaterThan].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpULessThanEqual].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpULessThanEqual].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpSLessThanEqual].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpSLessThanEqual].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFOrdLessThanEqual].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFOrdLessThanEqual].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFUnordLessThanEqual].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFUnordLessThanEqual].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpUGreaterThanEqual].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpUGreaterThanEqual].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpSGreaterThanEqual].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpSGreaterThanEqual].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFOrdGreaterThanEqual].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFOrdGreaterThanEqual].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpFUnordGreaterThanEqual].operands.push(OperandId, "'Operand 1'");
InstructionDesc[OpFUnordGreaterThanEqual].operands.push(OperandId, "'Operand 2'");
InstructionDesc[OpDPdx].operands.push(OperandId, "'P'");
InstructionDesc[OpDPdy].operands.push(OperandId, "'P'");
InstructionDesc[OpFwidth].operands.push(OperandId, "'P'");
InstructionDesc[OpDPdxFine].operands.push(OperandId, "'P'");
InstructionDesc[OpDPdyFine].operands.push(OperandId, "'P'");
InstructionDesc[OpFwidthFine].operands.push(OperandId, "'P'");
InstructionDesc[OpDPdxCoarse].operands.push(OperandId, "'P'");
InstructionDesc[OpDPdyCoarse].operands.push(OperandId, "'P'");
InstructionDesc[OpFwidthCoarse].operands.push(OperandId, "'P'");
InstructionDesc[OpEmitStreamVertex].operands.push(OperandId, "'Stream'");
InstructionDesc[OpEndStreamPrimitive].operands.push(OperandId, "'Stream'");
InstructionDesc[OpControlBarrier].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpControlBarrier].operands.push(OperandScope, "'Memory'");
InstructionDesc[OpControlBarrier].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpMemoryBarrier].operands.push(OperandScope, "'Memory'");
InstructionDesc[OpMemoryBarrier].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpImageTexelPointer].operands.push(OperandId, "'Image'");
InstructionDesc[OpImageTexelPointer].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageTexelPointer].operands.push(OperandId, "'Sample'");
InstructionDesc[OpAtomicLoad].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicLoad].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicLoad].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicStore].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicStore].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicStore].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicStore].operands.push(OperandId, "'Value'");
InstructionDesc[OpAtomicExchange].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicExchange].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicExchange].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicExchange].operands.push(OperandId, "'Value'");
InstructionDesc[OpAtomicCompareExchange].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicCompareExchange].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicCompareExchange].operands.push(OperandMemorySemantics, "'Equal'");
InstructionDesc[OpAtomicCompareExchange].operands.push(OperandMemorySemantics, "'Unequal'");
InstructionDesc[OpAtomicCompareExchange].operands.push(OperandId, "'Value'");
InstructionDesc[OpAtomicCompareExchange].operands.push(OperandId, "'Comparator'");
InstructionDesc[OpAtomicCompareExchangeWeak].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicCompareExchangeWeak].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicCompareExchangeWeak].operands.push(OperandMemorySemantics, "'Equal'");
InstructionDesc[OpAtomicCompareExchangeWeak].operands.push(OperandMemorySemantics, "'Unequal'");
InstructionDesc[OpAtomicCompareExchangeWeak].operands.push(OperandId, "'Value'");
InstructionDesc[OpAtomicCompareExchangeWeak].operands.push(OperandId, "'Comparator'");
InstructionDesc[OpAtomicIIncrement].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicIIncrement].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicIIncrement].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicIDecrement].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicIDecrement].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicIDecrement].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicIAdd].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicIAdd].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicIAdd].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicIAdd].operands.push(OperandId, "'Value'");
InstructionDesc[OpAtomicFAddEXT].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicFAddEXT].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicFAddEXT].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicFAddEXT].operands.push(OperandId, "'Value'");
InstructionDesc[OpAssumeTrueKHR].operands.push(OperandId, "'Condition'");
InstructionDesc[OpExpectKHR].operands.push(OperandId, "'Value'");
InstructionDesc[OpExpectKHR].operands.push(OperandId, "'ExpectedValue'");
InstructionDesc[OpAtomicISub].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicISub].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicISub].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicISub].operands.push(OperandId, "'Value'");
InstructionDesc[OpAtomicUMin].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicUMin].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicUMin].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicUMin].operands.push(OperandId, "'Value'");
InstructionDesc[OpAtomicUMax].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicUMax].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicUMax].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicUMax].operands.push(OperandId, "'Value'");
InstructionDesc[OpAtomicSMin].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicSMin].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicSMin].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicSMin].operands.push(OperandId, "'Value'");
InstructionDesc[OpAtomicSMax].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicSMax].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicSMax].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicSMax].operands.push(OperandId, "'Value'");
InstructionDesc[OpAtomicFMinEXT].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicFMinEXT].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicFMinEXT].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicFMinEXT].operands.push(OperandId, "'Value'");
InstructionDesc[OpAtomicFMaxEXT].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicFMaxEXT].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicFMaxEXT].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicFMaxEXT].operands.push(OperandId, "'Value'");
InstructionDesc[OpAtomicAnd].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicAnd].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicAnd].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicAnd].operands.push(OperandId, "'Value'");
InstructionDesc[OpAtomicOr].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicOr].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicOr].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicOr].operands.push(OperandId, "'Value'");
InstructionDesc[OpAtomicXor].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicXor].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicXor].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicXor].operands.push(OperandId, "'Value'");
InstructionDesc[OpAtomicFlagTestAndSet].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicFlagTestAndSet].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicFlagTestAndSet].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpAtomicFlagClear].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpAtomicFlagClear].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpAtomicFlagClear].operands.push(OperandMemorySemantics, "'Semantics'");
InstructionDesc[OpLoopMerge].operands.push(OperandId, "'Merge Block'");
InstructionDesc[OpLoopMerge].operands.push(OperandId, "'Continue Target'");
InstructionDesc[OpLoopMerge].operands.push(OperandLoop, "");
InstructionDesc[OpLoopMerge].operands.push(OperandOptionalLiteral, "");
InstructionDesc[OpSelectionMerge].operands.push(OperandId, "'Merge Block'");
InstructionDesc[OpSelectionMerge].operands.push(OperandSelect, "");
InstructionDesc[OpBranch].operands.push(OperandId, "'Target Label'");
InstructionDesc[OpBranchConditional].operands.push(OperandId, "'Condition'");
InstructionDesc[OpBranchConditional].operands.push(OperandId, "'True Label'");
InstructionDesc[OpBranchConditional].operands.push(OperandId, "'False Label'");
InstructionDesc[OpBranchConditional].operands.push(OperandVariableLiterals, "'Branch weights'");
InstructionDesc[OpSwitch].operands.push(OperandId, "'Selector'");
InstructionDesc[OpSwitch].operands.push(OperandId, "'Default'");
InstructionDesc[OpSwitch].operands.push(OperandVariableLiteralId, "'Target'");
InstructionDesc[OpReturnValue].operands.push(OperandId, "'Value'");
InstructionDesc[OpLifetimeStart].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpLifetimeStart].operands.push(OperandLiteralNumber, "'Size'");
InstructionDesc[OpLifetimeStop].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpLifetimeStop].operands.push(OperandLiteralNumber, "'Size'");
InstructionDesc[OpGroupAsyncCopy].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupAsyncCopy].operands.push(OperandId, "'Destination'");
InstructionDesc[OpGroupAsyncCopy].operands.push(OperandId, "'Source'");
InstructionDesc[OpGroupAsyncCopy].operands.push(OperandId, "'Num Elements'");
InstructionDesc[OpGroupAsyncCopy].operands.push(OperandId, "'Stride'");
InstructionDesc[OpGroupAsyncCopy].operands.push(OperandId, "'Event'");
InstructionDesc[OpGroupWaitEvents].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupWaitEvents].operands.push(OperandId, "'Num Events'");
InstructionDesc[OpGroupWaitEvents].operands.push(OperandId, "'Events List'");
InstructionDesc[OpGroupAll].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupAll].operands.push(OperandId, "'Predicate'");
InstructionDesc[OpGroupAny].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupAny].operands.push(OperandId, "'Predicate'");
InstructionDesc[OpGroupBroadcast].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupBroadcast].operands.push(OperandId, "'Value'");
InstructionDesc[OpGroupBroadcast].operands.push(OperandId, "'LocalId'");
InstructionDesc[OpGroupIAdd].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupIAdd].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupIAdd].operands.push(OperandId, "'X'");
InstructionDesc[OpGroupFAdd].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupFAdd].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupFAdd].operands.push(OperandId, "'X'");
InstructionDesc[OpGroupUMin].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupUMin].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupUMin].operands.push(OperandId, "'X'");
InstructionDesc[OpGroupSMin].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupSMin].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupSMin].operands.push(OperandId, "X");
InstructionDesc[OpGroupFMin].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupFMin].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupFMin].operands.push(OperandId, "X");
InstructionDesc[OpGroupUMax].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupUMax].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupUMax].operands.push(OperandId, "X");
InstructionDesc[OpGroupSMax].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupSMax].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupSMax].operands.push(OperandId, "X");
InstructionDesc[OpGroupFMax].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupFMax].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupFMax].operands.push(OperandId, "X");
InstructionDesc[OpReadPipe].operands.push(OperandId, "'Pipe'");
InstructionDesc[OpReadPipe].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpReadPipe].operands.push(OperandId, "'Packet Size'");
InstructionDesc[OpReadPipe].operands.push(OperandId, "'Packet Alignment'");
InstructionDesc[OpWritePipe].operands.push(OperandId, "'Pipe'");
InstructionDesc[OpWritePipe].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpWritePipe].operands.push(OperandId, "'Packet Size'");
InstructionDesc[OpWritePipe].operands.push(OperandId, "'Packet Alignment'");
InstructionDesc[OpReservedReadPipe].operands.push(OperandId, "'Pipe'");
InstructionDesc[OpReservedReadPipe].operands.push(OperandId, "'Reserve Id'");
InstructionDesc[OpReservedReadPipe].operands.push(OperandId, "'Index'");
InstructionDesc[OpReservedReadPipe].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpReservedReadPipe].operands.push(OperandId, "'Packet Size'");
InstructionDesc[OpReservedReadPipe].operands.push(OperandId, "'Packet Alignment'");
InstructionDesc[OpReservedWritePipe].operands.push(OperandId, "'Pipe'");
InstructionDesc[OpReservedWritePipe].operands.push(OperandId, "'Reserve Id'");
InstructionDesc[OpReservedWritePipe].operands.push(OperandId, "'Index'");
InstructionDesc[OpReservedWritePipe].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpReservedWritePipe].operands.push(OperandId, "'Packet Size'");
InstructionDesc[OpReservedWritePipe].operands.push(OperandId, "'Packet Alignment'");
InstructionDesc[OpReserveReadPipePackets].operands.push(OperandId, "'Pipe'");
InstructionDesc[OpReserveReadPipePackets].operands.push(OperandId, "'Num Packets'");
InstructionDesc[OpReserveReadPipePackets].operands.push(OperandId, "'Packet Size'");
InstructionDesc[OpReserveReadPipePackets].operands.push(OperandId, "'Packet Alignment'");
InstructionDesc[OpReserveWritePipePackets].operands.push(OperandId, "'Pipe'");
InstructionDesc[OpReserveWritePipePackets].operands.push(OperandId, "'Num Packets'");
InstructionDesc[OpReserveWritePipePackets].operands.push(OperandId, "'Packet Size'");
InstructionDesc[OpReserveWritePipePackets].operands.push(OperandId, "'Packet Alignment'");
InstructionDesc[OpCommitReadPipe].operands.push(OperandId, "'Pipe'");
InstructionDesc[OpCommitReadPipe].operands.push(OperandId, "'Reserve Id'");
InstructionDesc[OpCommitReadPipe].operands.push(OperandId, "'Packet Size'");
InstructionDesc[OpCommitReadPipe].operands.push(OperandId, "'Packet Alignment'");
InstructionDesc[OpCommitWritePipe].operands.push(OperandId, "'Pipe'");
InstructionDesc[OpCommitWritePipe].operands.push(OperandId, "'Reserve Id'");
InstructionDesc[OpCommitWritePipe].operands.push(OperandId, "'Packet Size'");
InstructionDesc[OpCommitWritePipe].operands.push(OperandId, "'Packet Alignment'");
InstructionDesc[OpIsValidReserveId].operands.push(OperandId, "'Reserve Id'");
InstructionDesc[OpGetNumPipePackets].operands.push(OperandId, "'Pipe'");
InstructionDesc[OpGetNumPipePackets].operands.push(OperandId, "'Packet Size'");
InstructionDesc[OpGetNumPipePackets].operands.push(OperandId, "'Packet Alignment'");
InstructionDesc[OpGetMaxPipePackets].operands.push(OperandId, "'Pipe'");
InstructionDesc[OpGetMaxPipePackets].operands.push(OperandId, "'Packet Size'");
InstructionDesc[OpGetMaxPipePackets].operands.push(OperandId, "'Packet Alignment'");
InstructionDesc[OpGroupReserveReadPipePackets].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupReserveReadPipePackets].operands.push(OperandId, "'Pipe'");
InstructionDesc[OpGroupReserveReadPipePackets].operands.push(OperandId, "'Num Packets'");
InstructionDesc[OpGroupReserveReadPipePackets].operands.push(OperandId, "'Packet Size'");
InstructionDesc[OpGroupReserveReadPipePackets].operands.push(OperandId, "'Packet Alignment'");
InstructionDesc[OpGroupReserveWritePipePackets].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupReserveWritePipePackets].operands.push(OperandId, "'Pipe'");
InstructionDesc[OpGroupReserveWritePipePackets].operands.push(OperandId, "'Num Packets'");
InstructionDesc[OpGroupReserveWritePipePackets].operands.push(OperandId, "'Packet Size'");
InstructionDesc[OpGroupReserveWritePipePackets].operands.push(OperandId, "'Packet Alignment'");
InstructionDesc[OpGroupCommitReadPipe].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupCommitReadPipe].operands.push(OperandId, "'Pipe'");
InstructionDesc[OpGroupCommitReadPipe].operands.push(OperandId, "'Reserve Id'");
InstructionDesc[OpGroupCommitReadPipe].operands.push(OperandId, "'Packet Size'");
InstructionDesc[OpGroupCommitReadPipe].operands.push(OperandId, "'Packet Alignment'");
InstructionDesc[OpGroupCommitWritePipe].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupCommitWritePipe].operands.push(OperandId, "'Pipe'");
InstructionDesc[OpGroupCommitWritePipe].operands.push(OperandId, "'Reserve Id'");
InstructionDesc[OpGroupCommitWritePipe].operands.push(OperandId, "'Packet Size'");
InstructionDesc[OpGroupCommitWritePipe].operands.push(OperandId, "'Packet Alignment'");
InstructionDesc[OpBuildNDRange].operands.push(OperandId, "'GlobalWorkSize'");
InstructionDesc[OpBuildNDRange].operands.push(OperandId, "'LocalWorkSize'");
InstructionDesc[OpBuildNDRange].operands.push(OperandId, "'GlobalWorkOffset'");
InstructionDesc[OpCaptureEventProfilingInfo].operands.push(OperandId, "'Event'");
InstructionDesc[OpCaptureEventProfilingInfo].operands.push(OperandId, "'Profiling Info'");
InstructionDesc[OpCaptureEventProfilingInfo].operands.push(OperandId, "'Value'");
InstructionDesc[OpSetUserEventStatus].operands.push(OperandId, "'Event'");
InstructionDesc[OpSetUserEventStatus].operands.push(OperandId, "'Status'");
InstructionDesc[OpIsValidEvent].operands.push(OperandId, "'Event'");
InstructionDesc[OpRetainEvent].operands.push(OperandId, "'Event'");
InstructionDesc[OpReleaseEvent].operands.push(OperandId, "'Event'");
InstructionDesc[OpGetKernelWorkGroupSize].operands.push(OperandId, "'Invoke'");
InstructionDesc[OpGetKernelWorkGroupSize].operands.push(OperandId, "'Param'");
InstructionDesc[OpGetKernelWorkGroupSize].operands.push(OperandId, "'Param Size'");
InstructionDesc[OpGetKernelWorkGroupSize].operands.push(OperandId, "'Param Align'");
InstructionDesc[OpGetKernelPreferredWorkGroupSizeMultiple].operands.push(OperandId, "'Invoke'");
InstructionDesc[OpGetKernelPreferredWorkGroupSizeMultiple].operands.push(OperandId, "'Param'");
InstructionDesc[OpGetKernelPreferredWorkGroupSizeMultiple].operands.push(OperandId, "'Param Size'");
InstructionDesc[OpGetKernelPreferredWorkGroupSizeMultiple].operands.push(OperandId, "'Param Align'");
InstructionDesc[OpGetKernelNDrangeSubGroupCount].operands.push(OperandId, "'ND Range'");
InstructionDesc[OpGetKernelNDrangeSubGroupCount].operands.push(OperandId, "'Invoke'");
InstructionDesc[OpGetKernelNDrangeSubGroupCount].operands.push(OperandId, "'Param'");
InstructionDesc[OpGetKernelNDrangeSubGroupCount].operands.push(OperandId, "'Param Size'");
InstructionDesc[OpGetKernelNDrangeSubGroupCount].operands.push(OperandId, "'Param Align'");
InstructionDesc[OpGetKernelNDrangeMaxSubGroupSize].operands.push(OperandId, "'ND Range'");
InstructionDesc[OpGetKernelNDrangeMaxSubGroupSize].operands.push(OperandId, "'Invoke'");
InstructionDesc[OpGetKernelNDrangeMaxSubGroupSize].operands.push(OperandId, "'Param'");
InstructionDesc[OpGetKernelNDrangeMaxSubGroupSize].operands.push(OperandId, "'Param Size'");
InstructionDesc[OpGetKernelNDrangeMaxSubGroupSize].operands.push(OperandId, "'Param Align'");
InstructionDesc[OpEnqueueKernel].operands.push(OperandId, "'Queue'");
InstructionDesc[OpEnqueueKernel].operands.push(OperandId, "'Flags'");
InstructionDesc[OpEnqueueKernel].operands.push(OperandId, "'ND Range'");
InstructionDesc[OpEnqueueKernel].operands.push(OperandId, "'Num Events'");
InstructionDesc[OpEnqueueKernel].operands.push(OperandId, "'Wait Events'");
InstructionDesc[OpEnqueueKernel].operands.push(OperandId, "'Ret Event'");
InstructionDesc[OpEnqueueKernel].operands.push(OperandId, "'Invoke'");
InstructionDesc[OpEnqueueKernel].operands.push(OperandId, "'Param'");
InstructionDesc[OpEnqueueKernel].operands.push(OperandId, "'Param Size'");
InstructionDesc[OpEnqueueKernel].operands.push(OperandId, "'Param Align'");
InstructionDesc[OpEnqueueKernel].operands.push(OperandVariableIds, "'Local Size'");
InstructionDesc[OpEnqueueMarker].operands.push(OperandId, "'Queue'");
InstructionDesc[OpEnqueueMarker].operands.push(OperandId, "'Num Events'");
InstructionDesc[OpEnqueueMarker].operands.push(OperandId, "'Wait Events'");
InstructionDesc[OpEnqueueMarker].operands.push(OperandId, "'Ret Event'");
InstructionDesc[OpGroupNonUniformElect].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformAll].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformAll].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformAny].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformAny].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformAllEqual].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformAllEqual].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformBroadcast].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformBroadcast].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformBroadcast].operands.push(OperandId, "ID");
InstructionDesc[OpGroupNonUniformBroadcastFirst].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformBroadcastFirst].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformBallot].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformBallot].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformInverseBallot].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformInverseBallot].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformBallotBitExtract].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformBallotBitExtract].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformBallotBitExtract].operands.push(OperandId, "Bit");
InstructionDesc[OpGroupNonUniformBallotBitCount].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformBallotBitCount].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformBallotBitCount].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformBallotFindLSB].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformBallotFindLSB].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformBallotFindMSB].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformBallotFindMSB].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformShuffle].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformShuffle].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformShuffle].operands.push(OperandId, "'Id'");
InstructionDesc[OpGroupNonUniformShuffleXor].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformShuffleXor].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformShuffleXor].operands.push(OperandId, "Mask");
InstructionDesc[OpGroupNonUniformShuffleUp].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformShuffleUp].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformShuffleUp].operands.push(OperandId, "Offset");
InstructionDesc[OpGroupNonUniformShuffleDown].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformShuffleDown].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformShuffleDown].operands.push(OperandId, "Offset");
InstructionDesc[OpGroupNonUniformIAdd].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformIAdd].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformIAdd].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformIAdd].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpGroupNonUniformFAdd].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformFAdd].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformFAdd].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformFAdd].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpGroupNonUniformIMul].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformIMul].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformIMul].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformIMul].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpGroupNonUniformFMul].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformFMul].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformFMul].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformFMul].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpGroupNonUniformSMin].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformSMin].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformSMin].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformSMin].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpGroupNonUniformUMin].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformUMin].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformUMin].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformUMin].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpGroupNonUniformFMin].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformFMin].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformFMin].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformFMin].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpGroupNonUniformSMax].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformSMax].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformSMax].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformSMax].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpGroupNonUniformUMax].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformUMax].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformUMax].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformUMax].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpGroupNonUniformFMax].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformFMax].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformFMax].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformFMax].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpGroupNonUniformBitwiseAnd].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformBitwiseAnd].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformBitwiseAnd].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformBitwiseAnd].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpGroupNonUniformBitwiseOr].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformBitwiseOr].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformBitwiseOr].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformBitwiseOr].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpGroupNonUniformBitwiseXor].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformBitwiseXor].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformBitwiseXor].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformBitwiseXor].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpGroupNonUniformLogicalAnd].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformLogicalAnd].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformLogicalAnd].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformLogicalAnd].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpGroupNonUniformLogicalOr].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformLogicalOr].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformLogicalOr].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformLogicalOr].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpGroupNonUniformLogicalXor].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformLogicalXor].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupNonUniformLogicalXor].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformLogicalXor].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpGroupNonUniformQuadBroadcast].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformQuadBroadcast].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformQuadBroadcast].operands.push(OperandId, "'Id'");
InstructionDesc[OpGroupNonUniformQuadSwap].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformQuadSwap].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformQuadSwap].operands.push(OperandId, "'Direction'");
InstructionDesc[OpSubgroupBallotKHR].operands.push(OperandId, "'Predicate'");
InstructionDesc[OpSubgroupFirstInvocationKHR].operands.push(OperandId, "'Value'");
InstructionDesc[OpSubgroupAnyKHR].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpSubgroupAnyKHR].operands.push(OperandId, "'Predicate'");
InstructionDesc[OpSubgroupAllKHR].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpSubgroupAllKHR].operands.push(OperandId, "'Predicate'");
InstructionDesc[OpSubgroupAllEqualKHR].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpSubgroupAllEqualKHR].operands.push(OperandId, "'Predicate'");
InstructionDesc[OpGroupNonUniformRotateKHR].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupNonUniformRotateKHR].operands.push(OperandId, "'X'");
InstructionDesc[OpGroupNonUniformRotateKHR].operands.push(OperandId, "'Delta'");
InstructionDesc[OpGroupNonUniformRotateKHR].operands.push(OperandId, "'ClusterSize'", true);
InstructionDesc[OpSubgroupReadInvocationKHR].operands.push(OperandId, "'Value'");
InstructionDesc[OpSubgroupReadInvocationKHR].operands.push(OperandId, "'Index'");
InstructionDesc[OpModuleProcessed].operands.push(OperandLiteralString, "'process'");
InstructionDesc[OpGroupIAddNonUniformAMD].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupIAddNonUniformAMD].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupIAddNonUniformAMD].operands.push(OperandId, "'X'");
InstructionDesc[OpGroupFAddNonUniformAMD].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupFAddNonUniformAMD].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupFAddNonUniformAMD].operands.push(OperandId, "'X'");
InstructionDesc[OpGroupUMinNonUniformAMD].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupUMinNonUniformAMD].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupUMinNonUniformAMD].operands.push(OperandId, "'X'");
InstructionDesc[OpGroupSMinNonUniformAMD].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupSMinNonUniformAMD].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupSMinNonUniformAMD].operands.push(OperandId, "X");
InstructionDesc[OpGroupFMinNonUniformAMD].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupFMinNonUniformAMD].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupFMinNonUniformAMD].operands.push(OperandId, "X");
InstructionDesc[OpGroupUMaxNonUniformAMD].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupUMaxNonUniformAMD].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupUMaxNonUniformAMD].operands.push(OperandId, "X");
InstructionDesc[OpGroupSMaxNonUniformAMD].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupSMaxNonUniformAMD].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupSMaxNonUniformAMD].operands.push(OperandId, "X");
InstructionDesc[OpGroupFMaxNonUniformAMD].operands.push(OperandScope, "'Execution'");
InstructionDesc[OpGroupFMaxNonUniformAMD].operands.push(OperandGroupOperation, "'Operation'");
InstructionDesc[OpGroupFMaxNonUniformAMD].operands.push(OperandId, "X");
InstructionDesc[OpFragmentMaskFetchAMD].operands.push(OperandId, "'Image'");
InstructionDesc[OpFragmentMaskFetchAMD].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpFragmentFetchAMD].operands.push(OperandId, "'Image'");
InstructionDesc[OpFragmentFetchAMD].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpFragmentFetchAMD].operands.push(OperandId, "'Fragment Index'");
InstructionDesc[OpGroupNonUniformPartitionNV].operands.push(OperandId, "X");
InstructionDesc[OpGroupNonUniformQuadAllKHR].operands.push(OperandId, "'Predicate'");
InstructionDesc[OpGroupNonUniformQuadAnyKHR].operands.push(OperandId, "'Predicate'");
InstructionDesc[OpTypeAccelerationStructureKHR].setResultAndType(true, false);
InstructionDesc[OpTraceNV].operands.push(OperandId, "'Acceleration Structure'");
InstructionDesc[OpTraceNV].operands.push(OperandId, "'Ray Flags'");
InstructionDesc[OpTraceNV].operands.push(OperandId, "'Cull Mask'");
InstructionDesc[OpTraceNV].operands.push(OperandId, "'SBT Record Offset'");
InstructionDesc[OpTraceNV].operands.push(OperandId, "'SBT Record Stride'");
InstructionDesc[OpTraceNV].operands.push(OperandId, "'Miss Index'");
InstructionDesc[OpTraceNV].operands.push(OperandId, "'Ray Origin'");
InstructionDesc[OpTraceNV].operands.push(OperandId, "'TMin'");
InstructionDesc[OpTraceNV].operands.push(OperandId, "'Ray Direction'");
InstructionDesc[OpTraceNV].operands.push(OperandId, "'TMax'");
InstructionDesc[OpTraceNV].operands.push(OperandId, "'Payload'");
InstructionDesc[OpTraceNV].setResultAndType(false, false);
InstructionDesc[OpTraceRayMotionNV].operands.push(OperandId, "'Acceleration Structure'");
InstructionDesc[OpTraceRayMotionNV].operands.push(OperandId, "'Ray Flags'");
InstructionDesc[OpTraceRayMotionNV].operands.push(OperandId, "'Cull Mask'");
InstructionDesc[OpTraceRayMotionNV].operands.push(OperandId, "'SBT Record Offset'");
InstructionDesc[OpTraceRayMotionNV].operands.push(OperandId, "'SBT Record Stride'");
InstructionDesc[OpTraceRayMotionNV].operands.push(OperandId, "'Miss Index'");
InstructionDesc[OpTraceRayMotionNV].operands.push(OperandId, "'Ray Origin'");
InstructionDesc[OpTraceRayMotionNV].operands.push(OperandId, "'TMin'");
InstructionDesc[OpTraceRayMotionNV].operands.push(OperandId, "'Ray Direction'");
InstructionDesc[OpTraceRayMotionNV].operands.push(OperandId, "'TMax'");
InstructionDesc[OpTraceRayMotionNV].operands.push(OperandId, "'Time'");
InstructionDesc[OpTraceRayMotionNV].operands.push(OperandId, "'Payload'");
InstructionDesc[OpTraceRayMotionNV].setResultAndType(false, false);
InstructionDesc[OpTraceRayKHR].operands.push(OperandId, "'Acceleration Structure'");
InstructionDesc[OpTraceRayKHR].operands.push(OperandId, "'Ray Flags'");
InstructionDesc[OpTraceRayKHR].operands.push(OperandId, "'Cull Mask'");
InstructionDesc[OpTraceRayKHR].operands.push(OperandId, "'SBT Record Offset'");
InstructionDesc[OpTraceRayKHR].operands.push(OperandId, "'SBT Record Stride'");
InstructionDesc[OpTraceRayKHR].operands.push(OperandId, "'Miss Index'");
InstructionDesc[OpTraceRayKHR].operands.push(OperandId, "'Ray Origin'");
InstructionDesc[OpTraceRayKHR].operands.push(OperandId, "'TMin'");
InstructionDesc[OpTraceRayKHR].operands.push(OperandId, "'Ray Direction'");
InstructionDesc[OpTraceRayKHR].operands.push(OperandId, "'TMax'");
InstructionDesc[OpTraceRayKHR].operands.push(OperandId, "'Payload'");
InstructionDesc[OpTraceRayKHR].setResultAndType(false, false);
InstructionDesc[OpReportIntersectionKHR].operands.push(OperandId, "'Hit Parameter'");
InstructionDesc[OpReportIntersectionKHR].operands.push(OperandId, "'Hit Kind'");
InstructionDesc[OpIgnoreIntersectionNV].setResultAndType(false, false);
InstructionDesc[OpIgnoreIntersectionKHR].setResultAndType(false, false);
InstructionDesc[OpTerminateRayNV].setResultAndType(false, false);
InstructionDesc[OpTerminateRayKHR].setResultAndType(false, false);
InstructionDesc[OpExecuteCallableNV].operands.push(OperandId, "SBT Record Index");
InstructionDesc[OpExecuteCallableNV].operands.push(OperandId, "CallableData ID");
InstructionDesc[OpExecuteCallableNV].setResultAndType(false, false);
InstructionDesc[OpExecuteCallableKHR].operands.push(OperandId, "SBT Record Index");
InstructionDesc[OpExecuteCallableKHR].operands.push(OperandId, "CallableData");
InstructionDesc[OpExecuteCallableKHR].setResultAndType(false, false);
InstructionDesc[OpConvertUToAccelerationStructureKHR].operands.push(OperandId, "Value");
InstructionDesc[OpConvertUToAccelerationStructureKHR].setResultAndType(true, true);
// Ray Query
InstructionDesc[OpTypeAccelerationStructureKHR].setResultAndType(true, false);
InstructionDesc[OpTypeRayQueryKHR].setResultAndType(true, false);
InstructionDesc[OpRayQueryInitializeKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryInitializeKHR].operands.push(OperandId, "'AccelerationS'");
InstructionDesc[OpRayQueryInitializeKHR].operands.push(OperandId, "'RayFlags'");
InstructionDesc[OpRayQueryInitializeKHR].operands.push(OperandId, "'CullMask'");
InstructionDesc[OpRayQueryInitializeKHR].operands.push(OperandId, "'Origin'");
InstructionDesc[OpRayQueryInitializeKHR].operands.push(OperandId, "'Tmin'");
InstructionDesc[OpRayQueryInitializeKHR].operands.push(OperandId, "'Direction'");
InstructionDesc[OpRayQueryInitializeKHR].operands.push(OperandId, "'Tmax'");
InstructionDesc[OpRayQueryInitializeKHR].setResultAndType(false, false);
InstructionDesc[OpRayQueryTerminateKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryTerminateKHR].setResultAndType(false, false);
InstructionDesc[OpRayQueryGenerateIntersectionKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGenerateIntersectionKHR].operands.push(OperandId, "'THit'");
InstructionDesc[OpRayQueryGenerateIntersectionKHR].setResultAndType(false, false);
InstructionDesc[OpRayQueryConfirmIntersectionKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryConfirmIntersectionKHR].setResultAndType(false, false);
InstructionDesc[OpRayQueryProceedKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryProceedKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetIntersectionTypeKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetIntersectionTypeKHR].operands.push(OperandId, "'Committed'");
InstructionDesc[OpRayQueryGetIntersectionTypeKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetRayTMinKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetRayTMinKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetRayFlagsKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetRayFlagsKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetIntersectionTKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetIntersectionTKHR].operands.push(OperandId, "'Committed'");
InstructionDesc[OpRayQueryGetIntersectionTKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetIntersectionInstanceCustomIndexKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetIntersectionInstanceCustomIndexKHR].operands.push(OperandId, "'Committed'");
InstructionDesc[OpRayQueryGetIntersectionInstanceCustomIndexKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetIntersectionInstanceIdKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetIntersectionInstanceIdKHR].operands.push(OperandId, "'Committed'");
InstructionDesc[OpRayQueryGetIntersectionInstanceIdKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetIntersectionInstanceShaderBindingTableRecordOffsetKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetIntersectionInstanceShaderBindingTableRecordOffsetKHR].operands.push(OperandId, "'Committed'");
InstructionDesc[OpRayQueryGetIntersectionInstanceShaderBindingTableRecordOffsetKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetIntersectionGeometryIndexKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetIntersectionGeometryIndexKHR].operands.push(OperandId, "'Committed'");
InstructionDesc[OpRayQueryGetIntersectionGeometryIndexKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetIntersectionPrimitiveIndexKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetIntersectionPrimitiveIndexKHR].operands.push(OperandId, "'Committed'");
InstructionDesc[OpRayQueryGetIntersectionPrimitiveIndexKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetIntersectionBarycentricsKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetIntersectionBarycentricsKHR].operands.push(OperandId, "'Committed'");
InstructionDesc[OpRayQueryGetIntersectionBarycentricsKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetIntersectionFrontFaceKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetIntersectionFrontFaceKHR].operands.push(OperandId, "'Committed'");
InstructionDesc[OpRayQueryGetIntersectionFrontFaceKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetIntersectionCandidateAABBOpaqueKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetIntersectionCandidateAABBOpaqueKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetIntersectionObjectRayDirectionKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetIntersectionObjectRayDirectionKHR].operands.push(OperandId, "'Committed'");
InstructionDesc[OpRayQueryGetIntersectionObjectRayDirectionKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetIntersectionObjectRayOriginKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetIntersectionObjectRayOriginKHR].operands.push(OperandId, "'Committed'");
InstructionDesc[OpRayQueryGetIntersectionObjectRayOriginKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetWorldRayDirectionKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetWorldRayDirectionKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetWorldRayOriginKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetWorldRayOriginKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetIntersectionObjectToWorldKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetIntersectionObjectToWorldKHR].operands.push(OperandId, "'Committed'");
InstructionDesc[OpRayQueryGetIntersectionObjectToWorldKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetIntersectionWorldToObjectKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetIntersectionWorldToObjectKHR].operands.push(OperandId, "'Committed'");
InstructionDesc[OpRayQueryGetIntersectionWorldToObjectKHR].setResultAndType(true, true);
InstructionDesc[OpRayQueryGetIntersectionTriangleVertexPositionsKHR].operands.push(OperandId, "'RayQuery'");
InstructionDesc[OpRayQueryGetIntersectionTriangleVertexPositionsKHR].operands.push(OperandId, "'Committed'");
InstructionDesc[OpRayQueryGetIntersectionTriangleVertexPositionsKHR].setResultAndType(true, true);
InstructionDesc[OpImageSampleFootprintNV].operands.push(OperandId, "'Sampled Image'");
InstructionDesc[OpImageSampleFootprintNV].operands.push(OperandId, "'Coordinate'");
InstructionDesc[OpImageSampleFootprintNV].operands.push(OperandId, "'Granularity'");
InstructionDesc[OpImageSampleFootprintNV].operands.push(OperandId, "'Coarse'");
InstructionDesc[OpImageSampleFootprintNV].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSampleFootprintNV].operands.push(OperandVariableIds, "", true);
InstructionDesc[OpWritePackedPrimitiveIndices4x8NV].operands.push(OperandId, "'Index Offset'");
InstructionDesc[OpWritePackedPrimitiveIndices4x8NV].operands.push(OperandId, "'Packed Indices'");
InstructionDesc[OpEmitMeshTasksEXT].operands.push(OperandId, "'groupCountX'");
InstructionDesc[OpEmitMeshTasksEXT].operands.push(OperandId, "'groupCountY'");
InstructionDesc[OpEmitMeshTasksEXT].operands.push(OperandId, "'groupCountZ'");
InstructionDesc[OpEmitMeshTasksEXT].operands.push(OperandId, "'Payload'");
InstructionDesc[OpEmitMeshTasksEXT].setResultAndType(false, false);
InstructionDesc[OpSetMeshOutputsEXT].operands.push(OperandId, "'vertexCount'");
InstructionDesc[OpSetMeshOutputsEXT].operands.push(OperandId, "'primitiveCount'");
InstructionDesc[OpSetMeshOutputsEXT].setResultAndType(false, false);
InstructionDesc[OpTypeCooperativeMatrixNV].operands.push(OperandId, "'Component Type'");
InstructionDesc[OpTypeCooperativeMatrixNV].operands.push(OperandId, "'Scope'");
InstructionDesc[OpTypeCooperativeMatrixNV].operands.push(OperandId, "'Rows'");
InstructionDesc[OpTypeCooperativeMatrixNV].operands.push(OperandId, "'Columns'");
InstructionDesc[OpCooperativeMatrixLoadNV].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpCooperativeMatrixLoadNV].operands.push(OperandId, "'Stride'");
InstructionDesc[OpCooperativeMatrixLoadNV].operands.push(OperandId, "'Column Major'");
InstructionDesc[OpCooperativeMatrixLoadNV].operands.push(OperandMemoryAccess, "'Memory Access'");
InstructionDesc[OpCooperativeMatrixLoadNV].operands.push(OperandLiteralNumber, "", true);
InstructionDesc[OpCooperativeMatrixLoadNV].operands.push(OperandId, "", true);
InstructionDesc[OpCooperativeMatrixStoreNV].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpCooperativeMatrixStoreNV].operands.push(OperandId, "'Object'");
InstructionDesc[OpCooperativeMatrixStoreNV].operands.push(OperandId, "'Stride'");
InstructionDesc[OpCooperativeMatrixStoreNV].operands.push(OperandId, "'Column Major'");
InstructionDesc[OpCooperativeMatrixStoreNV].operands.push(OperandMemoryAccess, "'Memory Access'");
InstructionDesc[OpCooperativeMatrixStoreNV].operands.push(OperandLiteralNumber, "", true);
InstructionDesc[OpCooperativeMatrixStoreNV].operands.push(OperandId, "", true);
InstructionDesc[OpCooperativeMatrixMulAddNV].operands.push(OperandId, "'A'");
InstructionDesc[OpCooperativeMatrixMulAddNV].operands.push(OperandId, "'B'");
InstructionDesc[OpCooperativeMatrixMulAddNV].operands.push(OperandId, "'C'");
InstructionDesc[OpCooperativeMatrixLengthNV].operands.push(OperandId, "'Type'");
InstructionDesc[OpTypeCooperativeMatrixKHR].operands.push(OperandId, "'Component Type'");
InstructionDesc[OpTypeCooperativeMatrixKHR].operands.push(OperandId, "'Scope'");
InstructionDesc[OpTypeCooperativeMatrixKHR].operands.push(OperandId, "'Rows'");
InstructionDesc[OpTypeCooperativeMatrixKHR].operands.push(OperandId, "'Columns'");
InstructionDesc[OpTypeCooperativeMatrixKHR].operands.push(OperandId, "'Use'");
InstructionDesc[OpCooperativeMatrixLoadKHR].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpCooperativeMatrixLoadKHR].operands.push(OperandId, "'Memory Layout'");
InstructionDesc[OpCooperativeMatrixLoadKHR].operands.push(OperandId, "'Stride'");
InstructionDesc[OpCooperativeMatrixLoadKHR].operands.push(OperandMemoryAccess, "'Memory Access'");
InstructionDesc[OpCooperativeMatrixLoadKHR].operands.push(OperandLiteralNumber, "", true);
InstructionDesc[OpCooperativeMatrixLoadKHR].operands.push(OperandId, "", true);
InstructionDesc[OpCooperativeMatrixStoreKHR].operands.push(OperandId, "'Pointer'");
InstructionDesc[OpCooperativeMatrixStoreKHR].operands.push(OperandId, "'Object'");
InstructionDesc[OpCooperativeMatrixStoreKHR].operands.push(OperandId, "'Memory Layout'");
InstructionDesc[OpCooperativeMatrixStoreKHR].operands.push(OperandId, "'Stride'");
InstructionDesc[OpCooperativeMatrixStoreKHR].operands.push(OperandMemoryAccess, "'Memory Access'");
InstructionDesc[OpCooperativeMatrixStoreKHR].operands.push(OperandLiteralNumber, "", true);
InstructionDesc[OpCooperativeMatrixStoreKHR].operands.push(OperandId, "", true);
InstructionDesc[OpCooperativeMatrixMulAddKHR].operands.push(OperandId, "'A'");
InstructionDesc[OpCooperativeMatrixMulAddKHR].operands.push(OperandId, "'B'");
InstructionDesc[OpCooperativeMatrixMulAddKHR].operands.push(OperandId, "'C'");
InstructionDesc[OpCooperativeMatrixMulAddKHR].operands.push(OperandCooperativeMatrixOperands, "'Cooperative Matrix Operands'", true);
InstructionDesc[OpCooperativeMatrixLengthKHR].operands.push(OperandId, "'Type'");
InstructionDesc[OpDemoteToHelperInvocationEXT].setResultAndType(false, false);
InstructionDesc[OpReadClockKHR].operands.push(OperandScope, "'Scope'");
InstructionDesc[OpTypeHitObjectNV].setResultAndType(true, false);
InstructionDesc[OpHitObjectGetShaderRecordBufferHandleNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetShaderRecordBufferHandleNV].setResultAndType(true, true);
InstructionDesc[OpReorderThreadWithHintNV].operands.push(OperandId, "'Hint'");
InstructionDesc[OpReorderThreadWithHintNV].operands.push(OperandId, "'Bits'");
InstructionDesc[OpReorderThreadWithHintNV].setResultAndType(false, false);
InstructionDesc[OpReorderThreadWithHitObjectNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpReorderThreadWithHitObjectNV].operands.push(OperandId, "'Hint'");
InstructionDesc[OpReorderThreadWithHitObjectNV].operands.push(OperandId, "'Bits'");
InstructionDesc[OpReorderThreadWithHitObjectNV].setResultAndType(false, false);
InstructionDesc[OpHitObjectGetCurrentTimeNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetCurrentTimeNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectGetHitKindNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetHitKindNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectGetPrimitiveIndexNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetPrimitiveIndexNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectGetGeometryIndexNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetGeometryIndexNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectGetInstanceIdNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetInstanceIdNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectGetInstanceCustomIndexNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetInstanceCustomIndexNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectGetObjectRayDirectionNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetObjectRayDirectionNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectGetObjectRayOriginNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetObjectRayOriginNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectGetWorldRayDirectionNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetWorldRayDirectionNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectGetWorldRayOriginNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetWorldRayOriginNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectGetWorldToObjectNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetWorldToObjectNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectGetObjectToWorldNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetObjectToWorldNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectGetRayTMaxNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetRayTMaxNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectGetRayTMinNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetRayTMinNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectGetShaderBindingTableRecordIndexNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetShaderBindingTableRecordIndexNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectIsEmptyNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectIsEmptyNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectIsHitNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectIsHitNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectIsMissNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectIsMissNV].setResultAndType(true, true);
InstructionDesc[OpHitObjectGetAttributesNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectGetAttributesNV].operands.push(OperandId, "'HitObjectAttribute'");
InstructionDesc[OpHitObjectGetAttributesNV].setResultAndType(false, false);
InstructionDesc[OpHitObjectExecuteShaderNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectExecuteShaderNV].operands.push(OperandId, "'Payload'");
InstructionDesc[OpHitObjectExecuteShaderNV].setResultAndType(false, false);
InstructionDesc[OpHitObjectRecordHitNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectRecordHitNV].operands.push(OperandId, "'Acceleration Structure'");
InstructionDesc[OpHitObjectRecordHitNV].operands.push(OperandId, "'InstanceId'");
InstructionDesc[OpHitObjectRecordHitNV].operands.push(OperandId, "'PrimitiveId'");
InstructionDesc[OpHitObjectRecordHitNV].operands.push(OperandId, "'GeometryIndex'");
InstructionDesc[OpHitObjectRecordHitNV].operands.push(OperandId, "'HitKind'");
InstructionDesc[OpHitObjectRecordHitNV].operands.push(OperandId, "'SBT Record Offset'");
InstructionDesc[OpHitObjectRecordHitNV].operands.push(OperandId, "'SBT Record Stride'");
InstructionDesc[OpHitObjectRecordHitNV].operands.push(OperandId, "'Origin'");
InstructionDesc[OpHitObjectRecordHitNV].operands.push(OperandId, "'TMin'");
InstructionDesc[OpHitObjectRecordHitNV].operands.push(OperandId, "'Direction'");
InstructionDesc[OpHitObjectRecordHitNV].operands.push(OperandId, "'TMax'");
InstructionDesc[OpHitObjectRecordHitNV].operands.push(OperandId, "'HitObject Attribute'");
InstructionDesc[OpHitObjectRecordHitNV].setResultAndType(false, false);
InstructionDesc[OpHitObjectRecordHitMotionNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectRecordHitMotionNV].operands.push(OperandId, "'Acceleration Structure'");
InstructionDesc[OpHitObjectRecordHitMotionNV].operands.push(OperandId, "'InstanceId'");
InstructionDesc[OpHitObjectRecordHitMotionNV].operands.push(OperandId, "'PrimitiveId'");
InstructionDesc[OpHitObjectRecordHitMotionNV].operands.push(OperandId, "'GeometryIndex'");
InstructionDesc[OpHitObjectRecordHitMotionNV].operands.push(OperandId, "'HitKind'");
InstructionDesc[OpHitObjectRecordHitMotionNV].operands.push(OperandId, "'SBT Record Offset'");
InstructionDesc[OpHitObjectRecordHitMotionNV].operands.push(OperandId, "'SBT Record Stride'");
InstructionDesc[OpHitObjectRecordHitMotionNV].operands.push(OperandId, "'Origin'");
InstructionDesc[OpHitObjectRecordHitMotionNV].operands.push(OperandId, "'TMin'");
InstructionDesc[OpHitObjectRecordHitMotionNV].operands.push(OperandId, "'Direction'");
InstructionDesc[OpHitObjectRecordHitMotionNV].operands.push(OperandId, "'TMax'");
InstructionDesc[OpHitObjectRecordHitMotionNV].operands.push(OperandId, "'Current Time'");
InstructionDesc[OpHitObjectRecordHitMotionNV].operands.push(OperandId, "'HitObject Attribute'");
InstructionDesc[OpHitObjectRecordHitMotionNV].setResultAndType(false, false);
InstructionDesc[OpHitObjectRecordHitWithIndexNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectRecordHitWithIndexNV].operands.push(OperandId, "'Acceleration Structure'");
InstructionDesc[OpHitObjectRecordHitWithIndexNV].operands.push(OperandId, "'InstanceId'");
InstructionDesc[OpHitObjectRecordHitWithIndexNV].operands.push(OperandId, "'PrimitiveId'");
InstructionDesc[OpHitObjectRecordHitWithIndexNV].operands.push(OperandId, "'GeometryIndex'");
InstructionDesc[OpHitObjectRecordHitWithIndexNV].operands.push(OperandId, "'HitKind'");
InstructionDesc[OpHitObjectRecordHitWithIndexNV].operands.push(OperandId, "'SBT Record Index'");
InstructionDesc[OpHitObjectRecordHitWithIndexNV].operands.push(OperandId, "'Origin'");
InstructionDesc[OpHitObjectRecordHitWithIndexNV].operands.push(OperandId, "'TMin'");
InstructionDesc[OpHitObjectRecordHitWithIndexNV].operands.push(OperandId, "'Direction'");
InstructionDesc[OpHitObjectRecordHitWithIndexNV].operands.push(OperandId, "'TMax'");
InstructionDesc[OpHitObjectRecordHitWithIndexNV].operands.push(OperandId, "'HitObject Attribute'");
InstructionDesc[OpHitObjectRecordHitWithIndexNV].setResultAndType(false, false);
InstructionDesc[OpHitObjectRecordHitWithIndexMotionNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectRecordHitWithIndexMotionNV].operands.push(OperandId, "'Acceleration Structure'");
InstructionDesc[OpHitObjectRecordHitWithIndexMotionNV].operands.push(OperandId, "'InstanceId'");
InstructionDesc[OpHitObjectRecordHitWithIndexMotionNV].operands.push(OperandId, "'PrimitiveId'");
InstructionDesc[OpHitObjectRecordHitWithIndexMotionNV].operands.push(OperandId, "'GeometryIndex'");
InstructionDesc[OpHitObjectRecordHitWithIndexMotionNV].operands.push(OperandId, "'HitKind'");
InstructionDesc[OpHitObjectRecordHitWithIndexMotionNV].operands.push(OperandId, "'SBT Record Index'");
InstructionDesc[OpHitObjectRecordHitWithIndexMotionNV].operands.push(OperandId, "'Origin'");
InstructionDesc[OpHitObjectRecordHitWithIndexMotionNV].operands.push(OperandId, "'TMin'");
InstructionDesc[OpHitObjectRecordHitWithIndexMotionNV].operands.push(OperandId, "'Direction'");
InstructionDesc[OpHitObjectRecordHitWithIndexMotionNV].operands.push(OperandId, "'TMax'");
InstructionDesc[OpHitObjectRecordHitWithIndexMotionNV].operands.push(OperandId, "'Current Time'");
InstructionDesc[OpHitObjectRecordHitWithIndexMotionNV].operands.push(OperandId, "'HitObject Attribute'");
InstructionDesc[OpHitObjectRecordHitWithIndexMotionNV].setResultAndType(false, false);
InstructionDesc[OpHitObjectRecordMissNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectRecordMissNV].operands.push(OperandId, "'SBT Index'");
InstructionDesc[OpHitObjectRecordMissNV].operands.push(OperandId, "'Origin'");
InstructionDesc[OpHitObjectRecordMissNV].operands.push(OperandId, "'TMin'");
InstructionDesc[OpHitObjectRecordMissNV].operands.push(OperandId, "'Direction'");
InstructionDesc[OpHitObjectRecordMissNV].operands.push(OperandId, "'TMax'");
InstructionDesc[OpHitObjectRecordMissNV].setResultAndType(false, false);
InstructionDesc[OpHitObjectRecordMissMotionNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectRecordMissMotionNV].operands.push(OperandId, "'SBT Index'");
InstructionDesc[OpHitObjectRecordMissMotionNV].operands.push(OperandId, "'Origin'");
InstructionDesc[OpHitObjectRecordMissMotionNV].operands.push(OperandId, "'TMin'");
InstructionDesc[OpHitObjectRecordMissMotionNV].operands.push(OperandId, "'Direction'");
InstructionDesc[OpHitObjectRecordMissMotionNV].operands.push(OperandId, "'TMax'");
InstructionDesc[OpHitObjectRecordMissMotionNV].operands.push(OperandId, "'Current Time'");
InstructionDesc[OpHitObjectRecordMissMotionNV].setResultAndType(false, false);
InstructionDesc[OpHitObjectRecordEmptyNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectRecordEmptyNV].setResultAndType(false, false);
InstructionDesc[OpHitObjectTraceRayNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectTraceRayNV].operands.push(OperandId, "'Acceleration Structure'");
InstructionDesc[OpHitObjectTraceRayNV].operands.push(OperandId, "'RayFlags'");
InstructionDesc[OpHitObjectTraceRayNV].operands.push(OperandId, "'Cullmask'");
InstructionDesc[OpHitObjectTraceRayNV].operands.push(OperandId, "'SBT Record Offset'");
InstructionDesc[OpHitObjectTraceRayNV].operands.push(OperandId, "'SBT Record Stride'");
InstructionDesc[OpHitObjectTraceRayNV].operands.push(OperandId, "'Miss Index'");
InstructionDesc[OpHitObjectTraceRayNV].operands.push(OperandId, "'Origin'");
InstructionDesc[OpHitObjectTraceRayNV].operands.push(OperandId, "'TMin'");
InstructionDesc[OpHitObjectTraceRayNV].operands.push(OperandId, "'Direction'");
InstructionDesc[OpHitObjectTraceRayNV].operands.push(OperandId, "'TMax'");
InstructionDesc[OpHitObjectTraceRayNV].operands.push(OperandId, "'Payload'");
InstructionDesc[OpHitObjectTraceRayNV].setResultAndType(false, false);
InstructionDesc[OpHitObjectTraceRayMotionNV].operands.push(OperandId, "'HitObject'");
InstructionDesc[OpHitObjectTraceRayMotionNV].operands.push(OperandId, "'Acceleration Structure'");
InstructionDesc[OpHitObjectTraceRayMotionNV].operands.push(OperandId, "'RayFlags'");
InstructionDesc[OpHitObjectTraceRayMotionNV].operands.push(OperandId, "'Cullmask'");
InstructionDesc[OpHitObjectTraceRayMotionNV].operands.push(OperandId, "'SBT Record Offset'");
InstructionDesc[OpHitObjectTraceRayMotionNV].operands.push(OperandId, "'SBT Record Stride'");
InstructionDesc[OpHitObjectTraceRayMotionNV].operands.push(OperandId, "'Miss Index'");
InstructionDesc[OpHitObjectTraceRayMotionNV].operands.push(OperandId, "'Origin'");
InstructionDesc[OpHitObjectTraceRayMotionNV].operands.push(OperandId, "'TMin'");
InstructionDesc[OpHitObjectTraceRayMotionNV].operands.push(OperandId, "'Direction'");
InstructionDesc[OpHitObjectTraceRayMotionNV].operands.push(OperandId, "'TMax'");
InstructionDesc[OpHitObjectTraceRayMotionNV].operands.push(OperandId, "'Time'");
InstructionDesc[OpHitObjectTraceRayMotionNV].operands.push(OperandId, "'Payload'");
InstructionDesc[OpHitObjectTraceRayMotionNV].setResultAndType(false, false);
InstructionDesc[OpFetchMicroTriangleVertexBarycentricNV].operands.push(OperandId, "'Acceleration Structure'");
InstructionDesc[OpFetchMicroTriangleVertexBarycentricNV].operands.push(OperandId, "'Instance ID'");
InstructionDesc[OpFetchMicroTriangleVertexBarycentricNV].operands.push(OperandId, "'Geometry Index'");
InstructionDesc[OpFetchMicroTriangleVertexBarycentricNV].operands.push(OperandId, "'Primitive Index'");
InstructionDesc[OpFetchMicroTriangleVertexBarycentricNV].operands.push(OperandId, "'Barycentrics'");
InstructionDesc[OpFetchMicroTriangleVertexBarycentricNV].setResultAndType(true, true);
InstructionDesc[OpFetchMicroTriangleVertexPositionNV].operands.push(OperandId, "'Acceleration Structure'");
InstructionDesc[OpFetchMicroTriangleVertexPositionNV].operands.push(OperandId, "'Instance ID'");
InstructionDesc[OpFetchMicroTriangleVertexPositionNV].operands.push(OperandId, "'Geometry Index'");
InstructionDesc[OpFetchMicroTriangleVertexPositionNV].operands.push(OperandId, "'Primitive Index'");
InstructionDesc[OpFetchMicroTriangleVertexPositionNV].operands.push(OperandId, "'Barycentrics'");
InstructionDesc[OpFetchMicroTriangleVertexPositionNV].setResultAndType(true, true);
InstructionDesc[OpColorAttachmentReadEXT].operands.push(OperandId, "'Attachment'");
InstructionDesc[OpColorAttachmentReadEXT].operands.push(OperandId, "'Sample'", true);
InstructionDesc[OpStencilAttachmentReadEXT].operands.push(OperandId, "'Sample'", true);
InstructionDesc[OpDepthAttachmentReadEXT].operands.push(OperandId, "'Sample'", true);
InstructionDesc[OpImageSampleWeightedQCOM].operands.push(OperandId, "'source texture'");
InstructionDesc[OpImageSampleWeightedQCOM].operands.push(OperandId, "'texture coordinates'");
InstructionDesc[OpImageSampleWeightedQCOM].operands.push(OperandId, "'weights texture'");
InstructionDesc[OpImageSampleWeightedQCOM].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageSampleWeightedQCOM].setResultAndType(true, true);
InstructionDesc[OpImageBoxFilterQCOM].operands.push(OperandId, "'source texture'");
InstructionDesc[OpImageBoxFilterQCOM].operands.push(OperandId, "'texture coordinates'");
InstructionDesc[OpImageBoxFilterQCOM].operands.push(OperandId, "'box size'");
InstructionDesc[OpImageBoxFilterQCOM].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageBoxFilterQCOM].setResultAndType(true, true);
InstructionDesc[OpImageBlockMatchSADQCOM].operands.push(OperandId, "'target texture'");
InstructionDesc[OpImageBlockMatchSADQCOM].operands.push(OperandId, "'target coordinates'");
InstructionDesc[OpImageBlockMatchSADQCOM].operands.push(OperandId, "'reference texture'");
InstructionDesc[OpImageBlockMatchSADQCOM].operands.push(OperandId, "'reference coordinates'");
InstructionDesc[OpImageBlockMatchSADQCOM].operands.push(OperandId, "'block size'");
InstructionDesc[OpImageBlockMatchSADQCOM].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageBlockMatchSADQCOM].setResultAndType(true, true);
InstructionDesc[OpImageBlockMatchSSDQCOM].operands.push(OperandId, "'target texture'");
InstructionDesc[OpImageBlockMatchSSDQCOM].operands.push(OperandId, "'target coordinates'");
InstructionDesc[OpImageBlockMatchSSDQCOM].operands.push(OperandId, "'reference texture'");
InstructionDesc[OpImageBlockMatchSSDQCOM].operands.push(OperandId, "'reference coordinates'");
InstructionDesc[OpImageBlockMatchSSDQCOM].operands.push(OperandId, "'block size'");
InstructionDesc[OpImageBlockMatchSSDQCOM].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageBlockMatchSSDQCOM].setResultAndType(true, true);
InstructionDesc[OpImageBlockMatchWindowSSDQCOM].operands.push(OperandId, "'target texture'");
InstructionDesc[OpImageBlockMatchWindowSSDQCOM].operands.push(OperandId, "'target coordinates'");
InstructionDesc[OpImageBlockMatchWindowSSDQCOM].operands.push(OperandId, "'reference texture'");
InstructionDesc[OpImageBlockMatchWindowSSDQCOM].operands.push(OperandId, "'reference coordinates'");
InstructionDesc[OpImageBlockMatchWindowSSDQCOM].operands.push(OperandId, "'block size'");
InstructionDesc[OpImageBlockMatchWindowSSDQCOM].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageBlockMatchWindowSSDQCOM].setResultAndType(true, true);
InstructionDesc[OpImageBlockMatchWindowSADQCOM].operands.push(OperandId, "'target texture'");
InstructionDesc[OpImageBlockMatchWindowSADQCOM].operands.push(OperandId, "'target coordinates'");
InstructionDesc[OpImageBlockMatchWindowSADQCOM].operands.push(OperandId, "'reference texture'");
InstructionDesc[OpImageBlockMatchWindowSADQCOM].operands.push(OperandId, "'reference coordinates'");
InstructionDesc[OpImageBlockMatchWindowSADQCOM].operands.push(OperandId, "'block size'");
InstructionDesc[OpImageBlockMatchWindowSADQCOM].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageBlockMatchWindowSADQCOM].setResultAndType(true, true);
InstructionDesc[OpImageBlockMatchGatherSSDQCOM].operands.push(OperandId, "'target texture'");
InstructionDesc[OpImageBlockMatchGatherSSDQCOM].operands.push(OperandId, "'target coordinates'");
InstructionDesc[OpImageBlockMatchGatherSSDQCOM].operands.push(OperandId, "'reference texture'");
InstructionDesc[OpImageBlockMatchGatherSSDQCOM].operands.push(OperandId, "'reference coordinates'");
InstructionDesc[OpImageBlockMatchGatherSSDQCOM].operands.push(OperandId, "'block size'");
InstructionDesc[OpImageBlockMatchGatherSSDQCOM].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageBlockMatchGatherSSDQCOM].setResultAndType(true, true);
InstructionDesc[OpImageBlockMatchGatherSADQCOM].operands.push(OperandId, "'target texture'");
InstructionDesc[OpImageBlockMatchGatherSADQCOM].operands.push(OperandId, "'target coordinates'");
InstructionDesc[OpImageBlockMatchGatherSADQCOM].operands.push(OperandId, "'reference texture'");
InstructionDesc[OpImageBlockMatchGatherSADQCOM].operands.push(OperandId, "'reference coordinates'");
InstructionDesc[OpImageBlockMatchGatherSADQCOM].operands.push(OperandId, "'block size'");
InstructionDesc[OpImageBlockMatchGatherSADQCOM].operands.push(OperandImageOperands, "", true);
InstructionDesc[OpImageBlockMatchGatherSADQCOM].setResultAndType(true, true);
InstructionDesc[OpConstantCompositeReplicateEXT].operands.push(OperandId, "'Value'");
InstructionDesc[OpSpecConstantCompositeReplicateEXT].operands.push(OperandId, "'Value'");
InstructionDesc[OpCompositeConstructReplicateEXT].operands.push(OperandId, "'Value'");
});
}
}; // end spv namespace
|
0 | repos/glslang.zig/glslang | repos/glslang.zig/glslang/SPIRV/spirv.hpp | // Copyright (c) 2014-2024 The Khronos Group Inc.
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and/or associated documentation files (the "Materials"),
// to deal in the Materials without restriction, including without limitation
// the rights to use, copy, modify, merge, publish, distribute, sublicense,
// and/or sell copies of the Materials, and to permit persons to whom the
// Materials are furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Materials.
//
// MODIFICATIONS TO THIS FILE MAY MEAN IT NO LONGER ACCURATELY REFLECTS KHRONOS
// STANDARDS. THE UNMODIFIED, NORMATIVE VERSIONS OF KHRONOS SPECIFICATIONS AND
// HEADER INFORMATION ARE LOCATED AT https://www.khronos.org/registry/
//
// THE MATERIALS ARE PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS
// OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
// THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
// FROM,OUT OF OR IN CONNECTION WITH THE MATERIALS OR THE USE OR OTHER DEALINGS
// IN THE MATERIALS.
// This header is automatically generated by the same tool that creates
// the Binary Section of the SPIR-V specification.
// Enumeration tokens for SPIR-V, in various styles:
// C, C++, C++11, JSON, Lua, Python, C#, D, Beef
//
// - C will have tokens with a "Spv" prefix, e.g.: SpvSourceLanguageGLSL
// - C++ will have tokens in the "spv" name space, e.g.: spv::SourceLanguageGLSL
// - C++11 will use enum classes in the spv namespace, e.g.: spv::SourceLanguage::GLSL
// - Lua will use tables, e.g.: spv.SourceLanguage.GLSL
// - Python will use dictionaries, e.g.: spv['SourceLanguage']['GLSL']
// - C# will use enum classes in the Specification class located in the "Spv" namespace,
// e.g.: Spv.Specification.SourceLanguage.GLSL
// - D will have tokens under the "spv" module, e.g: spv.SourceLanguage.GLSL
// - Beef will use enum classes in the Specification class located in the "Spv" namespace,
// e.g.: Spv.Specification.SourceLanguage.GLSL
//
// Some tokens act like mask values, which can be OR'd together,
// while others are mutually exclusive. The mask-like ones have
// "Mask" in their name, and a parallel enum that has the shift
// amount (1 << x) for each corresponding enumerant.
#ifndef spirv_HPP
#define spirv_HPP
namespace spv {
typedef unsigned int Id;
#define SPV_VERSION 0x10600
#define SPV_REVISION 1
static const unsigned int MagicNumber = 0x07230203;
static const unsigned int Version = 0x00010600;
static const unsigned int Revision = 1;
static const unsigned int OpCodeMask = 0xffff;
static const unsigned int WordCountShift = 16;
enum SourceLanguage {
SourceLanguageUnknown = 0,
SourceLanguageESSL = 1,
SourceLanguageGLSL = 2,
SourceLanguageOpenCL_C = 3,
SourceLanguageOpenCL_CPP = 4,
SourceLanguageHLSL = 5,
SourceLanguageCPP_for_OpenCL = 6,
SourceLanguageSYCL = 7,
SourceLanguageMax = 0x7fffffff,
};
enum ExecutionModel {
ExecutionModelVertex = 0,
ExecutionModelTessellationControl = 1,
ExecutionModelTessellationEvaluation = 2,
ExecutionModelGeometry = 3,
ExecutionModelFragment = 4,
ExecutionModelGLCompute = 5,
ExecutionModelKernel = 6,
ExecutionModelTaskNV = 5267,
ExecutionModelMeshNV = 5268,
ExecutionModelRayGenerationKHR = 5313,
ExecutionModelRayGenerationNV = 5313,
ExecutionModelIntersectionKHR = 5314,
ExecutionModelIntersectionNV = 5314,
ExecutionModelAnyHitKHR = 5315,
ExecutionModelAnyHitNV = 5315,
ExecutionModelClosestHitKHR = 5316,
ExecutionModelClosestHitNV = 5316,
ExecutionModelMissKHR = 5317,
ExecutionModelMissNV = 5317,
ExecutionModelCallableKHR = 5318,
ExecutionModelCallableNV = 5318,
ExecutionModelTaskEXT = 5364,
ExecutionModelMeshEXT = 5365,
ExecutionModelMax = 0x7fffffff,
};
enum AddressingModel {
AddressingModelLogical = 0,
AddressingModelPhysical32 = 1,
AddressingModelPhysical64 = 2,
AddressingModelPhysicalStorageBuffer64 = 5348,
AddressingModelPhysicalStorageBuffer64EXT = 5348,
AddressingModelMax = 0x7fffffff,
};
enum MemoryModel {
MemoryModelSimple = 0,
MemoryModelGLSL450 = 1,
MemoryModelOpenCL = 2,
MemoryModelVulkan = 3,
MemoryModelVulkanKHR = 3,
MemoryModelMax = 0x7fffffff,
};
enum ExecutionMode {
ExecutionModeInvocations = 0,
ExecutionModeSpacingEqual = 1,
ExecutionModeSpacingFractionalEven = 2,
ExecutionModeSpacingFractionalOdd = 3,
ExecutionModeVertexOrderCw = 4,
ExecutionModeVertexOrderCcw = 5,
ExecutionModePixelCenterInteger = 6,
ExecutionModeOriginUpperLeft = 7,
ExecutionModeOriginLowerLeft = 8,
ExecutionModeEarlyFragmentTests = 9,
ExecutionModePointMode = 10,
ExecutionModeXfb = 11,
ExecutionModeDepthReplacing = 12,
ExecutionModeDepthGreater = 14,
ExecutionModeDepthLess = 15,
ExecutionModeDepthUnchanged = 16,
ExecutionModeLocalSize = 17,
ExecutionModeLocalSizeHint = 18,
ExecutionModeInputPoints = 19,
ExecutionModeInputLines = 20,
ExecutionModeInputLinesAdjacency = 21,
ExecutionModeTriangles = 22,
ExecutionModeInputTrianglesAdjacency = 23,
ExecutionModeQuads = 24,
ExecutionModeIsolines = 25,
ExecutionModeOutputVertices = 26,
ExecutionModeOutputPoints = 27,
ExecutionModeOutputLineStrip = 28,
ExecutionModeOutputTriangleStrip = 29,
ExecutionModeVecTypeHint = 30,
ExecutionModeContractionOff = 31,
ExecutionModeInitializer = 33,
ExecutionModeFinalizer = 34,
ExecutionModeSubgroupSize = 35,
ExecutionModeSubgroupsPerWorkgroup = 36,
ExecutionModeSubgroupsPerWorkgroupId = 37,
ExecutionModeLocalSizeId = 38,
ExecutionModeLocalSizeHintId = 39,
ExecutionModeNonCoherentColorAttachmentReadEXT = 4169,
ExecutionModeNonCoherentDepthAttachmentReadEXT = 4170,
ExecutionModeNonCoherentStencilAttachmentReadEXT = 4171,
ExecutionModeSubgroupUniformControlFlowKHR = 4421,
ExecutionModePostDepthCoverage = 4446,
ExecutionModeDenormPreserve = 4459,
ExecutionModeDenormFlushToZero = 4460,
ExecutionModeSignedZeroInfNanPreserve = 4461,
ExecutionModeRoundingModeRTE = 4462,
ExecutionModeRoundingModeRTZ = 4463,
ExecutionModeEarlyAndLateFragmentTestsAMD = 5017,
ExecutionModeStencilRefReplacingEXT = 5027,
ExecutionModeStencilRefUnchangedFrontAMD = 5079,
ExecutionModeStencilRefGreaterFrontAMD = 5080,
ExecutionModeStencilRefLessFrontAMD = 5081,
ExecutionModeStencilRefUnchangedBackAMD = 5082,
ExecutionModeStencilRefGreaterBackAMD = 5083,
ExecutionModeStencilRefLessBackAMD = 5084,
ExecutionModeQuadDerivativesKHR = 5088,
ExecutionModeRequireFullQuadsKHR = 5089,
ExecutionModeOutputLinesEXT = 5269,
ExecutionModeOutputLinesNV = 5269,
ExecutionModeOutputPrimitivesEXT = 5270,
ExecutionModeOutputPrimitivesNV = 5270,
ExecutionModeDerivativeGroupQuadsNV = 5289,
ExecutionModeDerivativeGroupLinearNV = 5290,
ExecutionModeOutputTrianglesEXT = 5298,
ExecutionModeOutputTrianglesNV = 5298,
ExecutionModePixelInterlockOrderedEXT = 5366,
ExecutionModePixelInterlockUnorderedEXT = 5367,
ExecutionModeSampleInterlockOrderedEXT = 5368,
ExecutionModeSampleInterlockUnorderedEXT = 5369,
ExecutionModeShadingRateInterlockOrderedEXT = 5370,
ExecutionModeShadingRateInterlockUnorderedEXT = 5371,
ExecutionModeSharedLocalMemorySizeINTEL = 5618,
ExecutionModeRoundingModeRTPINTEL = 5620,
ExecutionModeRoundingModeRTNINTEL = 5621,
ExecutionModeFloatingPointModeALTINTEL = 5622,
ExecutionModeFloatingPointModeIEEEINTEL = 5623,
ExecutionModeMaxWorkgroupSizeINTEL = 5893,
ExecutionModeMaxWorkDimINTEL = 5894,
ExecutionModeNoGlobalOffsetINTEL = 5895,
ExecutionModeNumSIMDWorkitemsINTEL = 5896,
ExecutionModeSchedulerTargetFmaxMhzINTEL = 5903,
ExecutionModeMaximallyReconvergesKHR = 6023,
ExecutionModeStreamingInterfaceINTEL = 6154,
ExecutionModeNamedBarrierCountINTEL = 6417,
ExecutionModeMax = 0x7fffffff,
};
enum StorageClass {
StorageClassUniformConstant = 0,
StorageClassInput = 1,
StorageClassUniform = 2,
StorageClassOutput = 3,
StorageClassWorkgroup = 4,
StorageClassCrossWorkgroup = 5,
StorageClassPrivate = 6,
StorageClassFunction = 7,
StorageClassGeneric = 8,
StorageClassPushConstant = 9,
StorageClassAtomicCounter = 10,
StorageClassImage = 11,
StorageClassStorageBuffer = 12,
StorageClassTileImageEXT = 4172,
StorageClassCallableDataKHR = 5328,
StorageClassCallableDataNV = 5328,
StorageClassIncomingCallableDataKHR = 5329,
StorageClassIncomingCallableDataNV = 5329,
StorageClassRayPayloadKHR = 5338,
StorageClassRayPayloadNV = 5338,
StorageClassHitAttributeKHR = 5339,
StorageClassHitAttributeNV = 5339,
StorageClassIncomingRayPayloadKHR = 5342,
StorageClassIncomingRayPayloadNV = 5342,
StorageClassShaderRecordBufferKHR = 5343,
StorageClassShaderRecordBufferNV = 5343,
StorageClassPhysicalStorageBuffer = 5349,
StorageClassPhysicalStorageBufferEXT = 5349,
StorageClassHitObjectAttributeNV = 5385,
StorageClassTaskPayloadWorkgroupEXT = 5402,
StorageClassCodeSectionINTEL = 5605,
StorageClassDeviceOnlyINTEL = 5936,
StorageClassHostOnlyINTEL = 5937,
StorageClassMax = 0x7fffffff,
};
enum Dim {
Dim1D = 0,
Dim2D = 1,
Dim3D = 2,
DimCube = 3,
DimRect = 4,
DimBuffer = 5,
DimSubpassData = 6,
DimTileImageDataEXT = 4173,
DimMax = 0x7fffffff,
};
enum SamplerAddressingMode {
SamplerAddressingModeNone = 0,
SamplerAddressingModeClampToEdge = 1,
SamplerAddressingModeClamp = 2,
SamplerAddressingModeRepeat = 3,
SamplerAddressingModeRepeatMirrored = 4,
SamplerAddressingModeMax = 0x7fffffff,
};
enum SamplerFilterMode {
SamplerFilterModeNearest = 0,
SamplerFilterModeLinear = 1,
SamplerFilterModeMax = 0x7fffffff,
};
enum ImageFormat {
ImageFormatUnknown = 0,
ImageFormatRgba32f = 1,
ImageFormatRgba16f = 2,
ImageFormatR32f = 3,
ImageFormatRgba8 = 4,
ImageFormatRgba8Snorm = 5,
ImageFormatRg32f = 6,
ImageFormatRg16f = 7,
ImageFormatR11fG11fB10f = 8,
ImageFormatR16f = 9,
ImageFormatRgba16 = 10,
ImageFormatRgb10A2 = 11,
ImageFormatRg16 = 12,
ImageFormatRg8 = 13,
ImageFormatR16 = 14,
ImageFormatR8 = 15,
ImageFormatRgba16Snorm = 16,
ImageFormatRg16Snorm = 17,
ImageFormatRg8Snorm = 18,
ImageFormatR16Snorm = 19,
ImageFormatR8Snorm = 20,
ImageFormatRgba32i = 21,
ImageFormatRgba16i = 22,
ImageFormatRgba8i = 23,
ImageFormatR32i = 24,
ImageFormatRg32i = 25,
ImageFormatRg16i = 26,
ImageFormatRg8i = 27,
ImageFormatR16i = 28,
ImageFormatR8i = 29,
ImageFormatRgba32ui = 30,
ImageFormatRgba16ui = 31,
ImageFormatRgba8ui = 32,
ImageFormatR32ui = 33,
ImageFormatRgb10a2ui = 34,
ImageFormatRg32ui = 35,
ImageFormatRg16ui = 36,
ImageFormatRg8ui = 37,
ImageFormatR16ui = 38,
ImageFormatR8ui = 39,
ImageFormatR64ui = 40,
ImageFormatR64i = 41,
ImageFormatMax = 0x7fffffff,
};
enum ImageChannelOrder {
ImageChannelOrderR = 0,
ImageChannelOrderA = 1,
ImageChannelOrderRG = 2,
ImageChannelOrderRA = 3,
ImageChannelOrderRGB = 4,
ImageChannelOrderRGBA = 5,
ImageChannelOrderBGRA = 6,
ImageChannelOrderARGB = 7,
ImageChannelOrderIntensity = 8,
ImageChannelOrderLuminance = 9,
ImageChannelOrderRx = 10,
ImageChannelOrderRGx = 11,
ImageChannelOrderRGBx = 12,
ImageChannelOrderDepth = 13,
ImageChannelOrderDepthStencil = 14,
ImageChannelOrdersRGB = 15,
ImageChannelOrdersRGBx = 16,
ImageChannelOrdersRGBA = 17,
ImageChannelOrdersBGRA = 18,
ImageChannelOrderABGR = 19,
ImageChannelOrderMax = 0x7fffffff,
};
enum ImageChannelDataType {
ImageChannelDataTypeSnormInt8 = 0,
ImageChannelDataTypeSnormInt16 = 1,
ImageChannelDataTypeUnormInt8 = 2,
ImageChannelDataTypeUnormInt16 = 3,
ImageChannelDataTypeUnormShort565 = 4,
ImageChannelDataTypeUnormShort555 = 5,
ImageChannelDataTypeUnormInt101010 = 6,
ImageChannelDataTypeSignedInt8 = 7,
ImageChannelDataTypeSignedInt16 = 8,
ImageChannelDataTypeSignedInt32 = 9,
ImageChannelDataTypeUnsignedInt8 = 10,
ImageChannelDataTypeUnsignedInt16 = 11,
ImageChannelDataTypeUnsignedInt32 = 12,
ImageChannelDataTypeHalfFloat = 13,
ImageChannelDataTypeFloat = 14,
ImageChannelDataTypeUnormInt24 = 15,
ImageChannelDataTypeUnormInt101010_2 = 16,
ImageChannelDataTypeMax = 0x7fffffff,
};
enum ImageOperandsShift {
ImageOperandsBiasShift = 0,
ImageOperandsLodShift = 1,
ImageOperandsGradShift = 2,
ImageOperandsConstOffsetShift = 3,
ImageOperandsOffsetShift = 4,
ImageOperandsConstOffsetsShift = 5,
ImageOperandsSampleShift = 6,
ImageOperandsMinLodShift = 7,
ImageOperandsMakeTexelAvailableShift = 8,
ImageOperandsMakeTexelAvailableKHRShift = 8,
ImageOperandsMakeTexelVisibleShift = 9,
ImageOperandsMakeTexelVisibleKHRShift = 9,
ImageOperandsNonPrivateTexelShift = 10,
ImageOperandsNonPrivateTexelKHRShift = 10,
ImageOperandsVolatileTexelShift = 11,
ImageOperandsVolatileTexelKHRShift = 11,
ImageOperandsSignExtendShift = 12,
ImageOperandsZeroExtendShift = 13,
ImageOperandsNontemporalShift = 14,
ImageOperandsOffsetsShift = 16,
ImageOperandsMax = 0x7fffffff,
};
enum ImageOperandsMask {
ImageOperandsMaskNone = 0,
ImageOperandsBiasMask = 0x00000001,
ImageOperandsLodMask = 0x00000002,
ImageOperandsGradMask = 0x00000004,
ImageOperandsConstOffsetMask = 0x00000008,
ImageOperandsOffsetMask = 0x00000010,
ImageOperandsConstOffsetsMask = 0x00000020,
ImageOperandsSampleMask = 0x00000040,
ImageOperandsMinLodMask = 0x00000080,
ImageOperandsMakeTexelAvailableMask = 0x00000100,
ImageOperandsMakeTexelAvailableKHRMask = 0x00000100,
ImageOperandsMakeTexelVisibleMask = 0x00000200,
ImageOperandsMakeTexelVisibleKHRMask = 0x00000200,
ImageOperandsNonPrivateTexelMask = 0x00000400,
ImageOperandsNonPrivateTexelKHRMask = 0x00000400,
ImageOperandsVolatileTexelMask = 0x00000800,
ImageOperandsVolatileTexelKHRMask = 0x00000800,
ImageOperandsSignExtendMask = 0x00001000,
ImageOperandsZeroExtendMask = 0x00002000,
ImageOperandsNontemporalMask = 0x00004000,
ImageOperandsOffsetsMask = 0x00010000,
};
enum FPFastMathModeShift {
FPFastMathModeNotNaNShift = 0,
FPFastMathModeNotInfShift = 1,
FPFastMathModeNSZShift = 2,
FPFastMathModeAllowRecipShift = 3,
FPFastMathModeFastShift = 4,
FPFastMathModeAllowContractFastINTELShift = 16,
FPFastMathModeAllowReassocINTELShift = 17,
FPFastMathModeMax = 0x7fffffff,
};
enum FPFastMathModeMask {
FPFastMathModeMaskNone = 0,
FPFastMathModeNotNaNMask = 0x00000001,
FPFastMathModeNotInfMask = 0x00000002,
FPFastMathModeNSZMask = 0x00000004,
FPFastMathModeAllowRecipMask = 0x00000008,
FPFastMathModeFastMask = 0x00000010,
FPFastMathModeAllowContractFastINTELMask = 0x00010000,
FPFastMathModeAllowReassocINTELMask = 0x00020000,
};
enum FPRoundingMode {
FPRoundingModeRTE = 0,
FPRoundingModeRTZ = 1,
FPRoundingModeRTP = 2,
FPRoundingModeRTN = 3,
FPRoundingModeMax = 0x7fffffff,
};
enum LinkageType {
LinkageTypeExport = 0,
LinkageTypeImport = 1,
LinkageTypeLinkOnceODR = 2,
LinkageTypeMax = 0x7fffffff,
};
enum AccessQualifier {
AccessQualifierReadOnly = 0,
AccessQualifierWriteOnly = 1,
AccessQualifierReadWrite = 2,
AccessQualifierMax = 0x7fffffff,
};
enum FunctionParameterAttribute {
FunctionParameterAttributeZext = 0,
FunctionParameterAttributeSext = 1,
FunctionParameterAttributeByVal = 2,
FunctionParameterAttributeSret = 3,
FunctionParameterAttributeNoAlias = 4,
FunctionParameterAttributeNoCapture = 5,
FunctionParameterAttributeNoWrite = 6,
FunctionParameterAttributeNoReadWrite = 7,
FunctionParameterAttributeRuntimeAlignedINTEL = 5940,
FunctionParameterAttributeMax = 0x7fffffff,
};
enum Decoration {
DecorationRelaxedPrecision = 0,
DecorationSpecId = 1,
DecorationBlock = 2,
DecorationBufferBlock = 3,
DecorationRowMajor = 4,
DecorationColMajor = 5,
DecorationArrayStride = 6,
DecorationMatrixStride = 7,
DecorationGLSLShared = 8,
DecorationGLSLPacked = 9,
DecorationCPacked = 10,
DecorationBuiltIn = 11,
DecorationNoPerspective = 13,
DecorationFlat = 14,
DecorationPatch = 15,
DecorationCentroid = 16,
DecorationSample = 17,
DecorationInvariant = 18,
DecorationRestrict = 19,
DecorationAliased = 20,
DecorationVolatile = 21,
DecorationConstant = 22,
DecorationCoherent = 23,
DecorationNonWritable = 24,
DecorationNonReadable = 25,
DecorationUniform = 26,
DecorationUniformId = 27,
DecorationSaturatedConversion = 28,
DecorationStream = 29,
DecorationLocation = 30,
DecorationComponent = 31,
DecorationIndex = 32,
DecorationBinding = 33,
DecorationDescriptorSet = 34,
DecorationOffset = 35,
DecorationXfbBuffer = 36,
DecorationXfbStride = 37,
DecorationFuncParamAttr = 38,
DecorationFPRoundingMode = 39,
DecorationFPFastMathMode = 40,
DecorationLinkageAttributes = 41,
DecorationNoContraction = 42,
DecorationInputAttachmentIndex = 43,
DecorationAlignment = 44,
DecorationMaxByteOffset = 45,
DecorationAlignmentId = 46,
DecorationMaxByteOffsetId = 47,
DecorationNoSignedWrap = 4469,
DecorationNoUnsignedWrap = 4470,
DecorationWeightTextureQCOM = 4487,
DecorationBlockMatchTextureQCOM = 4488,
DecorationBlockMatchSamplerQCOM = 4499,
DecorationExplicitInterpAMD = 4999,
DecorationOverrideCoverageNV = 5248,
DecorationPassthroughNV = 5250,
DecorationViewportRelativeNV = 5252,
DecorationSecondaryViewportRelativeNV = 5256,
DecorationPerPrimitiveEXT = 5271,
DecorationPerPrimitiveNV = 5271,
DecorationPerViewNV = 5272,
DecorationPerTaskNV = 5273,
DecorationPerVertexKHR = 5285,
DecorationPerVertexNV = 5285,
DecorationNonUniform = 5300,
DecorationNonUniformEXT = 5300,
DecorationRestrictPointer = 5355,
DecorationRestrictPointerEXT = 5355,
DecorationAliasedPointer = 5356,
DecorationAliasedPointerEXT = 5356,
DecorationHitObjectShaderRecordBufferNV = 5386,
DecorationBindlessSamplerNV = 5398,
DecorationBindlessImageNV = 5399,
DecorationBoundSamplerNV = 5400,
DecorationBoundImageNV = 5401,
DecorationSIMTCallINTEL = 5599,
DecorationReferencedIndirectlyINTEL = 5602,
DecorationClobberINTEL = 5607,
DecorationSideEffectsINTEL = 5608,
DecorationVectorComputeVariableINTEL = 5624,
DecorationFuncParamIOKindINTEL = 5625,
DecorationVectorComputeFunctionINTEL = 5626,
DecorationStackCallINTEL = 5627,
DecorationGlobalVariableOffsetINTEL = 5628,
DecorationCounterBuffer = 5634,
DecorationHlslCounterBufferGOOGLE = 5634,
DecorationHlslSemanticGOOGLE = 5635,
DecorationUserSemantic = 5635,
DecorationUserTypeGOOGLE = 5636,
DecorationFunctionRoundingModeINTEL = 5822,
DecorationFunctionDenormModeINTEL = 5823,
DecorationRegisterINTEL = 5825,
DecorationMemoryINTEL = 5826,
DecorationNumbanksINTEL = 5827,
DecorationBankwidthINTEL = 5828,
DecorationMaxPrivateCopiesINTEL = 5829,
DecorationSinglepumpINTEL = 5830,
DecorationDoublepumpINTEL = 5831,
DecorationMaxReplicatesINTEL = 5832,
DecorationSimpleDualPortINTEL = 5833,
DecorationMergeINTEL = 5834,
DecorationBankBitsINTEL = 5835,
DecorationForcePow2DepthINTEL = 5836,
DecorationBurstCoalesceINTEL = 5899,
DecorationCacheSizeINTEL = 5900,
DecorationDontStaticallyCoalesceINTEL = 5901,
DecorationPrefetchINTEL = 5902,
DecorationStallEnableINTEL = 5905,
DecorationFuseLoopsInFunctionINTEL = 5907,
DecorationMathOpDSPModeINTEL = 5909,
DecorationAliasScopeINTEL = 5914,
DecorationNoAliasINTEL = 5915,
DecorationInitiationIntervalINTEL = 5917,
DecorationMaxConcurrencyINTEL = 5918,
DecorationPipelineEnableINTEL = 5919,
DecorationBufferLocationINTEL = 5921,
DecorationIOPipeStorageINTEL = 5944,
DecorationFunctionFloatingPointModeINTEL = 6080,
DecorationSingleElementVectorINTEL = 6085,
DecorationVectorComputeCallableFunctionINTEL = 6087,
DecorationMediaBlockIOINTEL = 6140,
DecorationConduitKernelArgumentINTEL = 6175,
DecorationRegisterMapKernelArgumentINTEL = 6176,
DecorationMMHostInterfaceAddressWidthINTEL = 6177,
DecorationMMHostInterfaceDataWidthINTEL = 6178,
DecorationMMHostInterfaceLatencyINTEL = 6179,
DecorationMMHostInterfaceReadWriteModeINTEL = 6180,
DecorationMMHostInterfaceMaxBurstINTEL = 6181,
DecorationMMHostInterfaceWaitRequestINTEL = 6182,
DecorationStableKernelArgumentINTEL = 6183,
DecorationMax = 0x7fffffff,
};
enum BuiltIn {
BuiltInPosition = 0,
BuiltInPointSize = 1,
BuiltInClipDistance = 3,
BuiltInCullDistance = 4,
BuiltInVertexId = 5,
BuiltInInstanceId = 6,
BuiltInPrimitiveId = 7,
BuiltInInvocationId = 8,
BuiltInLayer = 9,
BuiltInViewportIndex = 10,
BuiltInTessLevelOuter = 11,
BuiltInTessLevelInner = 12,
BuiltInTessCoord = 13,
BuiltInPatchVertices = 14,
BuiltInFragCoord = 15,
BuiltInPointCoord = 16,
BuiltInFrontFacing = 17,
BuiltInSampleId = 18,
BuiltInSamplePosition = 19,
BuiltInSampleMask = 20,
BuiltInFragDepth = 22,
BuiltInHelperInvocation = 23,
BuiltInNumWorkgroups = 24,
BuiltInWorkgroupSize = 25,
BuiltInWorkgroupId = 26,
BuiltInLocalInvocationId = 27,
BuiltInGlobalInvocationId = 28,
BuiltInLocalInvocationIndex = 29,
BuiltInWorkDim = 30,
BuiltInGlobalSize = 31,
BuiltInEnqueuedWorkgroupSize = 32,
BuiltInGlobalOffset = 33,
BuiltInGlobalLinearId = 34,
BuiltInSubgroupSize = 36,
BuiltInSubgroupMaxSize = 37,
BuiltInNumSubgroups = 38,
BuiltInNumEnqueuedSubgroups = 39,
BuiltInSubgroupId = 40,
BuiltInSubgroupLocalInvocationId = 41,
BuiltInVertexIndex = 42,
BuiltInInstanceIndex = 43,
BuiltInCoreIDARM = 4160,
BuiltInCoreCountARM = 4161,
BuiltInCoreMaxIDARM = 4162,
BuiltInWarpIDARM = 4163,
BuiltInWarpMaxIDARM = 4164,
BuiltInSubgroupEqMask = 4416,
BuiltInSubgroupEqMaskKHR = 4416,
BuiltInSubgroupGeMask = 4417,
BuiltInSubgroupGeMaskKHR = 4417,
BuiltInSubgroupGtMask = 4418,
BuiltInSubgroupGtMaskKHR = 4418,
BuiltInSubgroupLeMask = 4419,
BuiltInSubgroupLeMaskKHR = 4419,
BuiltInSubgroupLtMask = 4420,
BuiltInSubgroupLtMaskKHR = 4420,
BuiltInBaseVertex = 4424,
BuiltInBaseInstance = 4425,
BuiltInDrawIndex = 4426,
BuiltInPrimitiveShadingRateKHR = 4432,
BuiltInDeviceIndex = 4438,
BuiltInViewIndex = 4440,
BuiltInShadingRateKHR = 4444,
BuiltInBaryCoordNoPerspAMD = 4992,
BuiltInBaryCoordNoPerspCentroidAMD = 4993,
BuiltInBaryCoordNoPerspSampleAMD = 4994,
BuiltInBaryCoordSmoothAMD = 4995,
BuiltInBaryCoordSmoothCentroidAMD = 4996,
BuiltInBaryCoordSmoothSampleAMD = 4997,
BuiltInBaryCoordPullModelAMD = 4998,
BuiltInFragStencilRefEXT = 5014,
BuiltInViewportMaskNV = 5253,
BuiltInSecondaryPositionNV = 5257,
BuiltInSecondaryViewportMaskNV = 5258,
BuiltInPositionPerViewNV = 5261,
BuiltInViewportMaskPerViewNV = 5262,
BuiltInFullyCoveredEXT = 5264,
BuiltInTaskCountNV = 5274,
BuiltInPrimitiveCountNV = 5275,
BuiltInPrimitiveIndicesNV = 5276,
BuiltInClipDistancePerViewNV = 5277,
BuiltInCullDistancePerViewNV = 5278,
BuiltInLayerPerViewNV = 5279,
BuiltInMeshViewCountNV = 5280,
BuiltInMeshViewIndicesNV = 5281,
BuiltInBaryCoordKHR = 5286,
BuiltInBaryCoordNV = 5286,
BuiltInBaryCoordNoPerspKHR = 5287,
BuiltInBaryCoordNoPerspNV = 5287,
BuiltInFragSizeEXT = 5292,
BuiltInFragmentSizeNV = 5292,
BuiltInFragInvocationCountEXT = 5293,
BuiltInInvocationsPerPixelNV = 5293,
BuiltInPrimitivePointIndicesEXT = 5294,
BuiltInPrimitiveLineIndicesEXT = 5295,
BuiltInPrimitiveTriangleIndicesEXT = 5296,
BuiltInCullPrimitiveEXT = 5299,
BuiltInLaunchIdKHR = 5319,
BuiltInLaunchIdNV = 5319,
BuiltInLaunchSizeKHR = 5320,
BuiltInLaunchSizeNV = 5320,
BuiltInWorldRayOriginKHR = 5321,
BuiltInWorldRayOriginNV = 5321,
BuiltInWorldRayDirectionKHR = 5322,
BuiltInWorldRayDirectionNV = 5322,
BuiltInObjectRayOriginKHR = 5323,
BuiltInObjectRayOriginNV = 5323,
BuiltInObjectRayDirectionKHR = 5324,
BuiltInObjectRayDirectionNV = 5324,
BuiltInRayTminKHR = 5325,
BuiltInRayTminNV = 5325,
BuiltInRayTmaxKHR = 5326,
BuiltInRayTmaxNV = 5326,
BuiltInInstanceCustomIndexKHR = 5327,
BuiltInInstanceCustomIndexNV = 5327,
BuiltInObjectToWorldKHR = 5330,
BuiltInObjectToWorldNV = 5330,
BuiltInWorldToObjectKHR = 5331,
BuiltInWorldToObjectNV = 5331,
BuiltInHitTNV = 5332,
BuiltInHitKindKHR = 5333,
BuiltInHitKindNV = 5333,
BuiltInCurrentRayTimeNV = 5334,
BuiltInHitTriangleVertexPositionsKHR = 5335,
BuiltInHitMicroTriangleVertexPositionsNV = 5337,
BuiltInHitMicroTriangleVertexBarycentricsNV = 5344,
BuiltInIncomingRayFlagsKHR = 5351,
BuiltInIncomingRayFlagsNV = 5351,
BuiltInRayGeometryIndexKHR = 5352,
BuiltInWarpsPerSMNV = 5374,
BuiltInSMCountNV = 5375,
BuiltInWarpIDNV = 5376,
BuiltInSMIDNV = 5377,
BuiltInHitKindFrontFacingMicroTriangleNV = 5405,
BuiltInHitKindBackFacingMicroTriangleNV = 5406,
BuiltInCullMaskKHR = 6021,
BuiltInMax = 0x7fffffff,
};
enum SelectionControlShift {
SelectionControlFlattenShift = 0,
SelectionControlDontFlattenShift = 1,
SelectionControlMax = 0x7fffffff,
};
enum SelectionControlMask {
SelectionControlMaskNone = 0,
SelectionControlFlattenMask = 0x00000001,
SelectionControlDontFlattenMask = 0x00000002,
};
enum LoopControlShift {
LoopControlUnrollShift = 0,
LoopControlDontUnrollShift = 1,
LoopControlDependencyInfiniteShift = 2,
LoopControlDependencyLengthShift = 3,
LoopControlMinIterationsShift = 4,
LoopControlMaxIterationsShift = 5,
LoopControlIterationMultipleShift = 6,
LoopControlPeelCountShift = 7,
LoopControlPartialCountShift = 8,
LoopControlInitiationIntervalINTELShift = 16,
LoopControlMaxConcurrencyINTELShift = 17,
LoopControlDependencyArrayINTELShift = 18,
LoopControlPipelineEnableINTELShift = 19,
LoopControlLoopCoalesceINTELShift = 20,
LoopControlMaxInterleavingINTELShift = 21,
LoopControlSpeculatedIterationsINTELShift = 22,
LoopControlNoFusionINTELShift = 23,
LoopControlLoopCountINTELShift = 24,
LoopControlMaxReinvocationDelayINTELShift = 25,
LoopControlMax = 0x7fffffff,
};
enum LoopControlMask {
LoopControlMaskNone = 0,
LoopControlUnrollMask = 0x00000001,
LoopControlDontUnrollMask = 0x00000002,
LoopControlDependencyInfiniteMask = 0x00000004,
LoopControlDependencyLengthMask = 0x00000008,
LoopControlMinIterationsMask = 0x00000010,
LoopControlMaxIterationsMask = 0x00000020,
LoopControlIterationMultipleMask = 0x00000040,
LoopControlPeelCountMask = 0x00000080,
LoopControlPartialCountMask = 0x00000100,
LoopControlInitiationIntervalINTELMask = 0x00010000,
LoopControlMaxConcurrencyINTELMask = 0x00020000,
LoopControlDependencyArrayINTELMask = 0x00040000,
LoopControlPipelineEnableINTELMask = 0x00080000,
LoopControlLoopCoalesceINTELMask = 0x00100000,
LoopControlMaxInterleavingINTELMask = 0x00200000,
LoopControlSpeculatedIterationsINTELMask = 0x00400000,
LoopControlNoFusionINTELMask = 0x00800000,
LoopControlLoopCountINTELMask = 0x01000000,
LoopControlMaxReinvocationDelayINTELMask = 0x02000000,
};
enum FunctionControlShift {
FunctionControlInlineShift = 0,
FunctionControlDontInlineShift = 1,
FunctionControlPureShift = 2,
FunctionControlConstShift = 3,
FunctionControlOptNoneINTELShift = 16,
FunctionControlMax = 0x7fffffff,
};
enum FunctionControlMask {
FunctionControlMaskNone = 0,
FunctionControlInlineMask = 0x00000001,
FunctionControlDontInlineMask = 0x00000002,
FunctionControlPureMask = 0x00000004,
FunctionControlConstMask = 0x00000008,
FunctionControlOptNoneINTELMask = 0x00010000,
};
enum MemorySemanticsShift {
MemorySemanticsAcquireShift = 1,
MemorySemanticsReleaseShift = 2,
MemorySemanticsAcquireReleaseShift = 3,
MemorySemanticsSequentiallyConsistentShift = 4,
MemorySemanticsUniformMemoryShift = 6,
MemorySemanticsSubgroupMemoryShift = 7,
MemorySemanticsWorkgroupMemoryShift = 8,
MemorySemanticsCrossWorkgroupMemoryShift = 9,
MemorySemanticsAtomicCounterMemoryShift = 10,
MemorySemanticsImageMemoryShift = 11,
MemorySemanticsOutputMemoryShift = 12,
MemorySemanticsOutputMemoryKHRShift = 12,
MemorySemanticsMakeAvailableShift = 13,
MemorySemanticsMakeAvailableKHRShift = 13,
MemorySemanticsMakeVisibleShift = 14,
MemorySemanticsMakeVisibleKHRShift = 14,
MemorySemanticsVolatileShift = 15,
MemorySemanticsMax = 0x7fffffff,
};
enum MemorySemanticsMask {
MemorySemanticsMaskNone = 0,
MemorySemanticsAcquireMask = 0x00000002,
MemorySemanticsReleaseMask = 0x00000004,
MemorySemanticsAcquireReleaseMask = 0x00000008,
MemorySemanticsSequentiallyConsistentMask = 0x00000010,
MemorySemanticsUniformMemoryMask = 0x00000040,
MemorySemanticsSubgroupMemoryMask = 0x00000080,
MemorySemanticsWorkgroupMemoryMask = 0x00000100,
MemorySemanticsCrossWorkgroupMemoryMask = 0x00000200,
MemorySemanticsAtomicCounterMemoryMask = 0x00000400,
MemorySemanticsImageMemoryMask = 0x00000800,
MemorySemanticsOutputMemoryMask = 0x00001000,
MemorySemanticsOutputMemoryKHRMask = 0x00001000,
MemorySemanticsMakeAvailableMask = 0x00002000,
MemorySemanticsMakeAvailableKHRMask = 0x00002000,
MemorySemanticsMakeVisibleMask = 0x00004000,
MemorySemanticsMakeVisibleKHRMask = 0x00004000,
MemorySemanticsVolatileMask = 0x00008000,
};
enum MemoryAccessShift {
MemoryAccessVolatileShift = 0,
MemoryAccessAlignedShift = 1,
MemoryAccessNontemporalShift = 2,
MemoryAccessMakePointerAvailableShift = 3,
MemoryAccessMakePointerAvailableKHRShift = 3,
MemoryAccessMakePointerVisibleShift = 4,
MemoryAccessMakePointerVisibleKHRShift = 4,
MemoryAccessNonPrivatePointerShift = 5,
MemoryAccessNonPrivatePointerKHRShift = 5,
MemoryAccessAliasScopeINTELMaskShift = 16,
MemoryAccessNoAliasINTELMaskShift = 17,
MemoryAccessMax = 0x7fffffff,
};
enum MemoryAccessMask {
MemoryAccessMaskNone = 0,
MemoryAccessVolatileMask = 0x00000001,
MemoryAccessAlignedMask = 0x00000002,
MemoryAccessNontemporalMask = 0x00000004,
MemoryAccessMakePointerAvailableMask = 0x00000008,
MemoryAccessMakePointerAvailableKHRMask = 0x00000008,
MemoryAccessMakePointerVisibleMask = 0x00000010,
MemoryAccessMakePointerVisibleKHRMask = 0x00000010,
MemoryAccessNonPrivatePointerMask = 0x00000020,
MemoryAccessNonPrivatePointerKHRMask = 0x00000020,
MemoryAccessAliasScopeINTELMaskMask = 0x00010000,
MemoryAccessNoAliasINTELMaskMask = 0x00020000,
};
enum Scope {
ScopeCrossDevice = 0,
ScopeDevice = 1,
ScopeWorkgroup = 2,
ScopeSubgroup = 3,
ScopeInvocation = 4,
ScopeQueueFamily = 5,
ScopeQueueFamilyKHR = 5,
ScopeShaderCallKHR = 6,
ScopeMax = 0x7fffffff,
};
enum GroupOperation {
GroupOperationReduce = 0,
GroupOperationInclusiveScan = 1,
GroupOperationExclusiveScan = 2,
GroupOperationClusteredReduce = 3,
GroupOperationPartitionedReduceNV = 6,
GroupOperationPartitionedInclusiveScanNV = 7,
GroupOperationPartitionedExclusiveScanNV = 8,
GroupOperationMax = 0x7fffffff,
};
enum KernelEnqueueFlags {
KernelEnqueueFlagsNoWait = 0,
KernelEnqueueFlagsWaitKernel = 1,
KernelEnqueueFlagsWaitWorkGroup = 2,
KernelEnqueueFlagsMax = 0x7fffffff,
};
enum KernelProfilingInfoShift {
KernelProfilingInfoCmdExecTimeShift = 0,
KernelProfilingInfoMax = 0x7fffffff,
};
enum KernelProfilingInfoMask {
KernelProfilingInfoMaskNone = 0,
KernelProfilingInfoCmdExecTimeMask = 0x00000001,
};
enum Capability {
CapabilityMatrix = 0,
CapabilityShader = 1,
CapabilityGeometry = 2,
CapabilityTessellation = 3,
CapabilityAddresses = 4,
CapabilityLinkage = 5,
CapabilityKernel = 6,
CapabilityVector16 = 7,
CapabilityFloat16Buffer = 8,
CapabilityFloat16 = 9,
CapabilityFloat64 = 10,
CapabilityInt64 = 11,
CapabilityInt64Atomics = 12,
CapabilityImageBasic = 13,
CapabilityImageReadWrite = 14,
CapabilityImageMipmap = 15,
CapabilityPipes = 17,
CapabilityGroups = 18,
CapabilityDeviceEnqueue = 19,
CapabilityLiteralSampler = 20,
CapabilityAtomicStorage = 21,
CapabilityInt16 = 22,
CapabilityTessellationPointSize = 23,
CapabilityGeometryPointSize = 24,
CapabilityImageGatherExtended = 25,
CapabilityStorageImageMultisample = 27,
CapabilityUniformBufferArrayDynamicIndexing = 28,
CapabilitySampledImageArrayDynamicIndexing = 29,
CapabilityStorageBufferArrayDynamicIndexing = 30,
CapabilityStorageImageArrayDynamicIndexing = 31,
CapabilityClipDistance = 32,
CapabilityCullDistance = 33,
CapabilityImageCubeArray = 34,
CapabilitySampleRateShading = 35,
CapabilityImageRect = 36,
CapabilitySampledRect = 37,
CapabilityGenericPointer = 38,
CapabilityInt8 = 39,
CapabilityInputAttachment = 40,
CapabilitySparseResidency = 41,
CapabilityMinLod = 42,
CapabilitySampled1D = 43,
CapabilityImage1D = 44,
CapabilitySampledCubeArray = 45,
CapabilitySampledBuffer = 46,
CapabilityImageBuffer = 47,
CapabilityImageMSArray = 48,
CapabilityStorageImageExtendedFormats = 49,
CapabilityImageQuery = 50,
CapabilityDerivativeControl = 51,
CapabilityInterpolationFunction = 52,
CapabilityTransformFeedback = 53,
CapabilityGeometryStreams = 54,
CapabilityStorageImageReadWithoutFormat = 55,
CapabilityStorageImageWriteWithoutFormat = 56,
CapabilityMultiViewport = 57,
CapabilitySubgroupDispatch = 58,
CapabilityNamedBarrier = 59,
CapabilityPipeStorage = 60,
CapabilityGroupNonUniform = 61,
CapabilityGroupNonUniformVote = 62,
CapabilityGroupNonUniformArithmetic = 63,
CapabilityGroupNonUniformBallot = 64,
CapabilityGroupNonUniformShuffle = 65,
CapabilityGroupNonUniformShuffleRelative = 66,
CapabilityGroupNonUniformClustered = 67,
CapabilityGroupNonUniformQuad = 68,
CapabilityShaderLayer = 69,
CapabilityShaderViewportIndex = 70,
CapabilityUniformDecoration = 71,
CapabilityCoreBuiltinsARM = 4165,
CapabilityTileImageColorReadAccessEXT = 4166,
CapabilityTileImageDepthReadAccessEXT = 4167,
CapabilityTileImageStencilReadAccessEXT = 4168,
CapabilityCooperativeMatrixLayoutsARM = 4201,
CapabilityFragmentShadingRateKHR = 4422,
CapabilitySubgroupBallotKHR = 4423,
CapabilityDrawParameters = 4427,
CapabilityWorkgroupMemoryExplicitLayoutKHR = 4428,
CapabilityWorkgroupMemoryExplicitLayout8BitAccessKHR = 4429,
CapabilityWorkgroupMemoryExplicitLayout16BitAccessKHR = 4430,
CapabilitySubgroupVoteKHR = 4431,
CapabilityStorageBuffer16BitAccess = 4433,
CapabilityStorageUniformBufferBlock16 = 4433,
CapabilityStorageUniform16 = 4434,
CapabilityUniformAndStorageBuffer16BitAccess = 4434,
CapabilityStoragePushConstant16 = 4435,
CapabilityStorageInputOutput16 = 4436,
CapabilityDeviceGroup = 4437,
CapabilityMultiView = 4439,
CapabilityVariablePointersStorageBuffer = 4441,
CapabilityVariablePointers = 4442,
CapabilityAtomicStorageOps = 4445,
CapabilitySampleMaskPostDepthCoverage = 4447,
CapabilityStorageBuffer8BitAccess = 4448,
CapabilityUniformAndStorageBuffer8BitAccess = 4449,
CapabilityStoragePushConstant8 = 4450,
CapabilityDenormPreserve = 4464,
CapabilityDenormFlushToZero = 4465,
CapabilitySignedZeroInfNanPreserve = 4466,
CapabilityRoundingModeRTE = 4467,
CapabilityRoundingModeRTZ = 4468,
CapabilityRayQueryProvisionalKHR = 4471,
CapabilityRayQueryKHR = 4472,
CapabilityRayTraversalPrimitiveCullingKHR = 4478,
CapabilityRayTracingKHR = 4479,
CapabilityTextureSampleWeightedQCOM = 4484,
CapabilityTextureBoxFilterQCOM = 4485,
CapabilityTextureBlockMatchQCOM = 4486,
CapabilityTextureBlockMatch2QCOM = 4498,
CapabilityFloat16ImageAMD = 5008,
CapabilityImageGatherBiasLodAMD = 5009,
CapabilityFragmentMaskAMD = 5010,
CapabilityStencilExportEXT = 5013,
CapabilityImageReadWriteLodAMD = 5015,
CapabilityInt64ImageEXT = 5016,
CapabilityShaderClockKHR = 5055,
CapabilityQuadControlKHR = 5087,
CapabilitySampleMaskOverrideCoverageNV = 5249,
CapabilityGeometryShaderPassthroughNV = 5251,
CapabilityShaderViewportIndexLayerEXT = 5254,
CapabilityShaderViewportIndexLayerNV = 5254,
CapabilityShaderViewportMaskNV = 5255,
CapabilityShaderStereoViewNV = 5259,
CapabilityPerViewAttributesNV = 5260,
CapabilityFragmentFullyCoveredEXT = 5265,
CapabilityMeshShadingNV = 5266,
CapabilityImageFootprintNV = 5282,
CapabilityMeshShadingEXT = 5283,
CapabilityFragmentBarycentricKHR = 5284,
CapabilityFragmentBarycentricNV = 5284,
CapabilityComputeDerivativeGroupQuadsNV = 5288,
CapabilityFragmentDensityEXT = 5291,
CapabilityShadingRateNV = 5291,
CapabilityGroupNonUniformPartitionedNV = 5297,
CapabilityShaderNonUniform = 5301,
CapabilityShaderNonUniformEXT = 5301,
CapabilityRuntimeDescriptorArray = 5302,
CapabilityRuntimeDescriptorArrayEXT = 5302,
CapabilityInputAttachmentArrayDynamicIndexing = 5303,
CapabilityInputAttachmentArrayDynamicIndexingEXT = 5303,
CapabilityUniformTexelBufferArrayDynamicIndexing = 5304,
CapabilityUniformTexelBufferArrayDynamicIndexingEXT = 5304,
CapabilityStorageTexelBufferArrayDynamicIndexing = 5305,
CapabilityStorageTexelBufferArrayDynamicIndexingEXT = 5305,
CapabilityUniformBufferArrayNonUniformIndexing = 5306,
CapabilityUniformBufferArrayNonUniformIndexingEXT = 5306,
CapabilitySampledImageArrayNonUniformIndexing = 5307,
CapabilitySampledImageArrayNonUniformIndexingEXT = 5307,
CapabilityStorageBufferArrayNonUniformIndexing = 5308,
CapabilityStorageBufferArrayNonUniformIndexingEXT = 5308,
CapabilityStorageImageArrayNonUniformIndexing = 5309,
CapabilityStorageImageArrayNonUniformIndexingEXT = 5309,
CapabilityInputAttachmentArrayNonUniformIndexing = 5310,
CapabilityInputAttachmentArrayNonUniformIndexingEXT = 5310,
CapabilityUniformTexelBufferArrayNonUniformIndexing = 5311,
CapabilityUniformTexelBufferArrayNonUniformIndexingEXT = 5311,
CapabilityStorageTexelBufferArrayNonUniformIndexing = 5312,
CapabilityStorageTexelBufferArrayNonUniformIndexingEXT = 5312,
CapabilityRayTracingPositionFetchKHR = 5336,
CapabilityRayTracingNV = 5340,
CapabilityRayTracingMotionBlurNV = 5341,
CapabilityVulkanMemoryModel = 5345,
CapabilityVulkanMemoryModelKHR = 5345,
CapabilityVulkanMemoryModelDeviceScope = 5346,
CapabilityVulkanMemoryModelDeviceScopeKHR = 5346,
CapabilityPhysicalStorageBufferAddresses = 5347,
CapabilityPhysicalStorageBufferAddressesEXT = 5347,
CapabilityComputeDerivativeGroupLinearNV = 5350,
CapabilityRayTracingProvisionalKHR = 5353,
CapabilityCooperativeMatrixNV = 5357,
CapabilityFragmentShaderSampleInterlockEXT = 5363,
CapabilityFragmentShaderShadingRateInterlockEXT = 5372,
CapabilityShaderSMBuiltinsNV = 5373,
CapabilityFragmentShaderPixelInterlockEXT = 5378,
CapabilityDemoteToHelperInvocation = 5379,
CapabilityDemoteToHelperInvocationEXT = 5379,
CapabilityDisplacementMicromapNV = 5380,
CapabilityRayTracingOpacityMicromapEXT = 5381,
CapabilityShaderInvocationReorderNV = 5383,
CapabilityBindlessTextureNV = 5390,
CapabilityRayQueryPositionFetchKHR = 5391,
CapabilityAtomicFloat16VectorNV = 5404,
CapabilityRayTracingDisplacementMicromapNV = 5409,
CapabilitySubgroupShuffleINTEL = 5568,
CapabilitySubgroupBufferBlockIOINTEL = 5569,
CapabilitySubgroupImageBlockIOINTEL = 5570,
CapabilitySubgroupImageMediaBlockIOINTEL = 5579,
CapabilityRoundToInfinityINTEL = 5582,
CapabilityFloatingPointModeINTEL = 5583,
CapabilityIntegerFunctions2INTEL = 5584,
CapabilityFunctionPointersINTEL = 5603,
CapabilityIndirectReferencesINTEL = 5604,
CapabilityAsmINTEL = 5606,
CapabilityAtomicFloat32MinMaxEXT = 5612,
CapabilityAtomicFloat64MinMaxEXT = 5613,
CapabilityAtomicFloat16MinMaxEXT = 5616,
CapabilityVectorComputeINTEL = 5617,
CapabilityVectorAnyINTEL = 5619,
CapabilityExpectAssumeKHR = 5629,
CapabilitySubgroupAvcMotionEstimationINTEL = 5696,
CapabilitySubgroupAvcMotionEstimationIntraINTEL = 5697,
CapabilitySubgroupAvcMotionEstimationChromaINTEL = 5698,
CapabilityVariableLengthArrayINTEL = 5817,
CapabilityFunctionFloatControlINTEL = 5821,
CapabilityFPGAMemoryAttributesINTEL = 5824,
CapabilityFPFastMathModeINTEL = 5837,
CapabilityArbitraryPrecisionIntegersINTEL = 5844,
CapabilityArbitraryPrecisionFloatingPointINTEL = 5845,
CapabilityUnstructuredLoopControlsINTEL = 5886,
CapabilityFPGALoopControlsINTEL = 5888,
CapabilityKernelAttributesINTEL = 5892,
CapabilityFPGAKernelAttributesINTEL = 5897,
CapabilityFPGAMemoryAccessesINTEL = 5898,
CapabilityFPGAClusterAttributesINTEL = 5904,
CapabilityLoopFuseINTEL = 5906,
CapabilityFPGADSPControlINTEL = 5908,
CapabilityMemoryAccessAliasingINTEL = 5910,
CapabilityFPGAInvocationPipeliningAttributesINTEL = 5916,
CapabilityFPGABufferLocationINTEL = 5920,
CapabilityArbitraryPrecisionFixedPointINTEL = 5922,
CapabilityUSMStorageClassesINTEL = 5935,
CapabilityRuntimeAlignedAttributeINTEL = 5939,
CapabilityIOPipesINTEL = 5943,
CapabilityBlockingPipesINTEL = 5945,
CapabilityFPGARegINTEL = 5948,
CapabilityDotProductInputAll = 6016,
CapabilityDotProductInputAllKHR = 6016,
CapabilityDotProductInput4x8Bit = 6017,
CapabilityDotProductInput4x8BitKHR = 6017,
CapabilityDotProductInput4x8BitPacked = 6018,
CapabilityDotProductInput4x8BitPackedKHR = 6018,
CapabilityDotProduct = 6019,
CapabilityDotProductKHR = 6019,
CapabilityRayCullMaskKHR = 6020,
CapabilityCooperativeMatrixKHR = 6022,
CapabilityReplicatedCompositesEXT = 6024,
CapabilityBitInstructions = 6025,
CapabilityGroupNonUniformRotateKHR = 6026,
CapabilityAtomicFloat32AddEXT = 6033,
CapabilityAtomicFloat64AddEXT = 6034,
CapabilityLongConstantCompositeINTEL = 6089,
CapabilityOptNoneINTEL = 6094,
CapabilityAtomicFloat16AddEXT = 6095,
CapabilityDebugInfoModuleINTEL = 6114,
CapabilitySplitBarrierINTEL = 6141,
CapabilityFPGAArgumentInterfacesINTEL = 6174,
CapabilityGroupUniformArithmeticKHR = 6400,
CapabilityMax = 0x7fffffff,
};
enum RayFlagsShift {
RayFlagsOpaqueKHRShift = 0,
RayFlagsNoOpaqueKHRShift = 1,
RayFlagsTerminateOnFirstHitKHRShift = 2,
RayFlagsSkipClosestHitShaderKHRShift = 3,
RayFlagsCullBackFacingTrianglesKHRShift = 4,
RayFlagsCullFrontFacingTrianglesKHRShift = 5,
RayFlagsCullOpaqueKHRShift = 6,
RayFlagsCullNoOpaqueKHRShift = 7,
RayFlagsSkipTrianglesKHRShift = 8,
RayFlagsSkipAABBsKHRShift = 9,
RayFlagsForceOpacityMicromap2StateEXTShift = 10,
RayFlagsMax = 0x7fffffff,
};
enum RayFlagsMask {
RayFlagsMaskNone = 0,
RayFlagsOpaqueKHRMask = 0x00000001,
RayFlagsNoOpaqueKHRMask = 0x00000002,
RayFlagsTerminateOnFirstHitKHRMask = 0x00000004,
RayFlagsSkipClosestHitShaderKHRMask = 0x00000008,
RayFlagsCullBackFacingTrianglesKHRMask = 0x00000010,
RayFlagsCullFrontFacingTrianglesKHRMask = 0x00000020,
RayFlagsCullOpaqueKHRMask = 0x00000040,
RayFlagsCullNoOpaqueKHRMask = 0x00000080,
RayFlagsSkipTrianglesKHRMask = 0x00000100,
RayFlagsSkipAABBsKHRMask = 0x00000200,
RayFlagsForceOpacityMicromap2StateEXTMask = 0x00000400,
};
enum RayQueryIntersection {
RayQueryIntersectionRayQueryCandidateIntersectionKHR = 0,
RayQueryIntersectionRayQueryCommittedIntersectionKHR = 1,
RayQueryIntersectionMax = 0x7fffffff,
};
enum RayQueryCommittedIntersectionType {
RayQueryCommittedIntersectionTypeRayQueryCommittedIntersectionNoneKHR = 0,
RayQueryCommittedIntersectionTypeRayQueryCommittedIntersectionTriangleKHR = 1,
RayQueryCommittedIntersectionTypeRayQueryCommittedIntersectionGeneratedKHR = 2,
RayQueryCommittedIntersectionTypeMax = 0x7fffffff,
};
enum RayQueryCandidateIntersectionType {
RayQueryCandidateIntersectionTypeRayQueryCandidateIntersectionTriangleKHR = 0,
RayQueryCandidateIntersectionTypeRayQueryCandidateIntersectionAABBKHR = 1,
RayQueryCandidateIntersectionTypeMax = 0x7fffffff,
};
enum FragmentShadingRateShift {
FragmentShadingRateVertical2PixelsShift = 0,
FragmentShadingRateVertical4PixelsShift = 1,
FragmentShadingRateHorizontal2PixelsShift = 2,
FragmentShadingRateHorizontal4PixelsShift = 3,
FragmentShadingRateMax = 0x7fffffff,
};
enum FragmentShadingRateMask {
FragmentShadingRateMaskNone = 0,
FragmentShadingRateVertical2PixelsMask = 0x00000001,
FragmentShadingRateVertical4PixelsMask = 0x00000002,
FragmentShadingRateHorizontal2PixelsMask = 0x00000004,
FragmentShadingRateHorizontal4PixelsMask = 0x00000008,
};
enum FPDenormMode {
FPDenormModePreserve = 0,
FPDenormModeFlushToZero = 1,
FPDenormModeMax = 0x7fffffff,
};
enum FPOperationMode {
FPOperationModeIEEE = 0,
FPOperationModeALT = 1,
FPOperationModeMax = 0x7fffffff,
};
enum QuantizationModes {
QuantizationModesTRN = 0,
QuantizationModesTRN_ZERO = 1,
QuantizationModesRND = 2,
QuantizationModesRND_ZERO = 3,
QuantizationModesRND_INF = 4,
QuantizationModesRND_MIN_INF = 5,
QuantizationModesRND_CONV = 6,
QuantizationModesRND_CONV_ODD = 7,
QuantizationModesMax = 0x7fffffff,
};
enum OverflowModes {
OverflowModesWRAP = 0,
OverflowModesSAT = 1,
OverflowModesSAT_ZERO = 2,
OverflowModesSAT_SYM = 3,
OverflowModesMax = 0x7fffffff,
};
enum PackedVectorFormat {
PackedVectorFormatPackedVectorFormat4x8Bit = 0,
PackedVectorFormatPackedVectorFormat4x8BitKHR = 0,
PackedVectorFormatMax = 0x7fffffff,
};
enum CooperativeMatrixOperandsShift {
CooperativeMatrixOperandsMatrixASignedComponentsKHRShift = 0,
CooperativeMatrixOperandsMatrixBSignedComponentsKHRShift = 1,
CooperativeMatrixOperandsMatrixCSignedComponentsKHRShift = 2,
CooperativeMatrixOperandsMatrixResultSignedComponentsKHRShift = 3,
CooperativeMatrixOperandsSaturatingAccumulationKHRShift = 4,
CooperativeMatrixOperandsMax = 0x7fffffff,
};
enum CooperativeMatrixOperandsMask {
CooperativeMatrixOperandsMaskNone = 0,
CooperativeMatrixOperandsMatrixASignedComponentsKHRMask = 0x00000001,
CooperativeMatrixOperandsMatrixBSignedComponentsKHRMask = 0x00000002,
CooperativeMatrixOperandsMatrixCSignedComponentsKHRMask = 0x00000004,
CooperativeMatrixOperandsMatrixResultSignedComponentsKHRMask = 0x00000008,
CooperativeMatrixOperandsSaturatingAccumulationKHRMask = 0x00000010,
};
enum CooperativeMatrixLayout {
CooperativeMatrixLayoutRowMajorKHR = 0,
CooperativeMatrixLayoutColumnMajorKHR = 1,
CooperativeMatrixLayoutRowBlockedInterleavedARM = 4202,
CooperativeMatrixLayoutColumnBlockedInterleavedARM = 4203,
CooperativeMatrixLayoutMax = 0x7fffffff,
};
enum CooperativeMatrixUse {
CooperativeMatrixUseMatrixAKHR = 0,
CooperativeMatrixUseMatrixBKHR = 1,
CooperativeMatrixUseMatrixAccumulatorKHR = 2,
CooperativeMatrixUseMax = 0x7fffffff,
};
enum Op {
OpNop = 0,
OpUndef = 1,
OpSourceContinued = 2,
OpSource = 3,
OpSourceExtension = 4,
OpName = 5,
OpMemberName = 6,
OpString = 7,
OpLine = 8,
OpExtension = 10,
OpExtInstImport = 11,
OpExtInst = 12,
OpMemoryModel = 14,
OpEntryPoint = 15,
OpExecutionMode = 16,
OpCapability = 17,
OpTypeVoid = 19,
OpTypeBool = 20,
OpTypeInt = 21,
OpTypeFloat = 22,
OpTypeVector = 23,
OpTypeMatrix = 24,
OpTypeImage = 25,
OpTypeSampler = 26,
OpTypeSampledImage = 27,
OpTypeArray = 28,
OpTypeRuntimeArray = 29,
OpTypeStruct = 30,
OpTypeOpaque = 31,
OpTypePointer = 32,
OpTypeFunction = 33,
OpTypeEvent = 34,
OpTypeDeviceEvent = 35,
OpTypeReserveId = 36,
OpTypeQueue = 37,
OpTypePipe = 38,
OpTypeForwardPointer = 39,
OpConstantTrue = 41,
OpConstantFalse = 42,
OpConstant = 43,
OpConstantComposite = 44,
OpConstantSampler = 45,
OpConstantNull = 46,
OpSpecConstantTrue = 48,
OpSpecConstantFalse = 49,
OpSpecConstant = 50,
OpSpecConstantComposite = 51,
OpSpecConstantOp = 52,
OpFunction = 54,
OpFunctionParameter = 55,
OpFunctionEnd = 56,
OpFunctionCall = 57,
OpVariable = 59,
OpImageTexelPointer = 60,
OpLoad = 61,
OpStore = 62,
OpCopyMemory = 63,
OpCopyMemorySized = 64,
OpAccessChain = 65,
OpInBoundsAccessChain = 66,
OpPtrAccessChain = 67,
OpArrayLength = 68,
OpGenericPtrMemSemantics = 69,
OpInBoundsPtrAccessChain = 70,
OpDecorate = 71,
OpMemberDecorate = 72,
OpDecorationGroup = 73,
OpGroupDecorate = 74,
OpGroupMemberDecorate = 75,
OpVectorExtractDynamic = 77,
OpVectorInsertDynamic = 78,
OpVectorShuffle = 79,
OpCompositeConstruct = 80,
OpCompositeExtract = 81,
OpCompositeInsert = 82,
OpCopyObject = 83,
OpTranspose = 84,
OpSampledImage = 86,
OpImageSampleImplicitLod = 87,
OpImageSampleExplicitLod = 88,
OpImageSampleDrefImplicitLod = 89,
OpImageSampleDrefExplicitLod = 90,
OpImageSampleProjImplicitLod = 91,
OpImageSampleProjExplicitLod = 92,
OpImageSampleProjDrefImplicitLod = 93,
OpImageSampleProjDrefExplicitLod = 94,
OpImageFetch = 95,
OpImageGather = 96,
OpImageDrefGather = 97,
OpImageRead = 98,
OpImageWrite = 99,
OpImage = 100,
OpImageQueryFormat = 101,
OpImageQueryOrder = 102,
OpImageQuerySizeLod = 103,
OpImageQuerySize = 104,
OpImageQueryLod = 105,
OpImageQueryLevels = 106,
OpImageQuerySamples = 107,
OpConvertFToU = 109,
OpConvertFToS = 110,
OpConvertSToF = 111,
OpConvertUToF = 112,
OpUConvert = 113,
OpSConvert = 114,
OpFConvert = 115,
OpQuantizeToF16 = 116,
OpConvertPtrToU = 117,
OpSatConvertSToU = 118,
OpSatConvertUToS = 119,
OpConvertUToPtr = 120,
OpPtrCastToGeneric = 121,
OpGenericCastToPtr = 122,
OpGenericCastToPtrExplicit = 123,
OpBitcast = 124,
OpSNegate = 126,
OpFNegate = 127,
OpIAdd = 128,
OpFAdd = 129,
OpISub = 130,
OpFSub = 131,
OpIMul = 132,
OpFMul = 133,
OpUDiv = 134,
OpSDiv = 135,
OpFDiv = 136,
OpUMod = 137,
OpSRem = 138,
OpSMod = 139,
OpFRem = 140,
OpFMod = 141,
OpVectorTimesScalar = 142,
OpMatrixTimesScalar = 143,
OpVectorTimesMatrix = 144,
OpMatrixTimesVector = 145,
OpMatrixTimesMatrix = 146,
OpOuterProduct = 147,
OpDot = 148,
OpIAddCarry = 149,
OpISubBorrow = 150,
OpUMulExtended = 151,
OpSMulExtended = 152,
OpAny = 154,
OpAll = 155,
OpIsNan = 156,
OpIsInf = 157,
OpIsFinite = 158,
OpIsNormal = 159,
OpSignBitSet = 160,
OpLessOrGreater = 161,
OpOrdered = 162,
OpUnordered = 163,
OpLogicalEqual = 164,
OpLogicalNotEqual = 165,
OpLogicalOr = 166,
OpLogicalAnd = 167,
OpLogicalNot = 168,
OpSelect = 169,
OpIEqual = 170,
OpINotEqual = 171,
OpUGreaterThan = 172,
OpSGreaterThan = 173,
OpUGreaterThanEqual = 174,
OpSGreaterThanEqual = 175,
OpULessThan = 176,
OpSLessThan = 177,
OpULessThanEqual = 178,
OpSLessThanEqual = 179,
OpFOrdEqual = 180,
OpFUnordEqual = 181,
OpFOrdNotEqual = 182,
OpFUnordNotEqual = 183,
OpFOrdLessThan = 184,
OpFUnordLessThan = 185,
OpFOrdGreaterThan = 186,
OpFUnordGreaterThan = 187,
OpFOrdLessThanEqual = 188,
OpFUnordLessThanEqual = 189,
OpFOrdGreaterThanEqual = 190,
OpFUnordGreaterThanEqual = 191,
OpShiftRightLogical = 194,
OpShiftRightArithmetic = 195,
OpShiftLeftLogical = 196,
OpBitwiseOr = 197,
OpBitwiseXor = 198,
OpBitwiseAnd = 199,
OpNot = 200,
OpBitFieldInsert = 201,
OpBitFieldSExtract = 202,
OpBitFieldUExtract = 203,
OpBitReverse = 204,
OpBitCount = 205,
OpDPdx = 207,
OpDPdy = 208,
OpFwidth = 209,
OpDPdxFine = 210,
OpDPdyFine = 211,
OpFwidthFine = 212,
OpDPdxCoarse = 213,
OpDPdyCoarse = 214,
OpFwidthCoarse = 215,
OpEmitVertex = 218,
OpEndPrimitive = 219,
OpEmitStreamVertex = 220,
OpEndStreamPrimitive = 221,
OpControlBarrier = 224,
OpMemoryBarrier = 225,
OpAtomicLoad = 227,
OpAtomicStore = 228,
OpAtomicExchange = 229,
OpAtomicCompareExchange = 230,
OpAtomicCompareExchangeWeak = 231,
OpAtomicIIncrement = 232,
OpAtomicIDecrement = 233,
OpAtomicIAdd = 234,
OpAtomicISub = 235,
OpAtomicSMin = 236,
OpAtomicUMin = 237,
OpAtomicSMax = 238,
OpAtomicUMax = 239,
OpAtomicAnd = 240,
OpAtomicOr = 241,
OpAtomicXor = 242,
OpPhi = 245,
OpLoopMerge = 246,
OpSelectionMerge = 247,
OpLabel = 248,
OpBranch = 249,
OpBranchConditional = 250,
OpSwitch = 251,
OpKill = 252,
OpReturn = 253,
OpReturnValue = 254,
OpUnreachable = 255,
OpLifetimeStart = 256,
OpLifetimeStop = 257,
OpGroupAsyncCopy = 259,
OpGroupWaitEvents = 260,
OpGroupAll = 261,
OpGroupAny = 262,
OpGroupBroadcast = 263,
OpGroupIAdd = 264,
OpGroupFAdd = 265,
OpGroupFMin = 266,
OpGroupUMin = 267,
OpGroupSMin = 268,
OpGroupFMax = 269,
OpGroupUMax = 270,
OpGroupSMax = 271,
OpReadPipe = 274,
OpWritePipe = 275,
OpReservedReadPipe = 276,
OpReservedWritePipe = 277,
OpReserveReadPipePackets = 278,
OpReserveWritePipePackets = 279,
OpCommitReadPipe = 280,
OpCommitWritePipe = 281,
OpIsValidReserveId = 282,
OpGetNumPipePackets = 283,
OpGetMaxPipePackets = 284,
OpGroupReserveReadPipePackets = 285,
OpGroupReserveWritePipePackets = 286,
OpGroupCommitReadPipe = 287,
OpGroupCommitWritePipe = 288,
OpEnqueueMarker = 291,
OpEnqueueKernel = 292,
OpGetKernelNDrangeSubGroupCount = 293,
OpGetKernelNDrangeMaxSubGroupSize = 294,
OpGetKernelWorkGroupSize = 295,
OpGetKernelPreferredWorkGroupSizeMultiple = 296,
OpRetainEvent = 297,
OpReleaseEvent = 298,
OpCreateUserEvent = 299,
OpIsValidEvent = 300,
OpSetUserEventStatus = 301,
OpCaptureEventProfilingInfo = 302,
OpGetDefaultQueue = 303,
OpBuildNDRange = 304,
OpImageSparseSampleImplicitLod = 305,
OpImageSparseSampleExplicitLod = 306,
OpImageSparseSampleDrefImplicitLod = 307,
OpImageSparseSampleDrefExplicitLod = 308,
OpImageSparseSampleProjImplicitLod = 309,
OpImageSparseSampleProjExplicitLod = 310,
OpImageSparseSampleProjDrefImplicitLod = 311,
OpImageSparseSampleProjDrefExplicitLod = 312,
OpImageSparseFetch = 313,
OpImageSparseGather = 314,
OpImageSparseDrefGather = 315,
OpImageSparseTexelsResident = 316,
OpNoLine = 317,
OpAtomicFlagTestAndSet = 318,
OpAtomicFlagClear = 319,
OpImageSparseRead = 320,
OpSizeOf = 321,
OpTypePipeStorage = 322,
OpConstantPipeStorage = 323,
OpCreatePipeFromPipeStorage = 324,
OpGetKernelLocalSizeForSubgroupCount = 325,
OpGetKernelMaxNumSubgroups = 326,
OpTypeNamedBarrier = 327,
OpNamedBarrierInitialize = 328,
OpMemoryNamedBarrier = 329,
OpModuleProcessed = 330,
OpExecutionModeId = 331,
OpDecorateId = 332,
OpGroupNonUniformElect = 333,
OpGroupNonUniformAll = 334,
OpGroupNonUniformAny = 335,
OpGroupNonUniformAllEqual = 336,
OpGroupNonUniformBroadcast = 337,
OpGroupNonUniformBroadcastFirst = 338,
OpGroupNonUniformBallot = 339,
OpGroupNonUniformInverseBallot = 340,
OpGroupNonUniformBallotBitExtract = 341,
OpGroupNonUniformBallotBitCount = 342,
OpGroupNonUniformBallotFindLSB = 343,
OpGroupNonUniformBallotFindMSB = 344,
OpGroupNonUniformShuffle = 345,
OpGroupNonUniformShuffleXor = 346,
OpGroupNonUniformShuffleUp = 347,
OpGroupNonUniformShuffleDown = 348,
OpGroupNonUniformIAdd = 349,
OpGroupNonUniformFAdd = 350,
OpGroupNonUniformIMul = 351,
OpGroupNonUniformFMul = 352,
OpGroupNonUniformSMin = 353,
OpGroupNonUniformUMin = 354,
OpGroupNonUniformFMin = 355,
OpGroupNonUniformSMax = 356,
OpGroupNonUniformUMax = 357,
OpGroupNonUniformFMax = 358,
OpGroupNonUniformBitwiseAnd = 359,
OpGroupNonUniformBitwiseOr = 360,
OpGroupNonUniformBitwiseXor = 361,
OpGroupNonUniformLogicalAnd = 362,
OpGroupNonUniformLogicalOr = 363,
OpGroupNonUniformLogicalXor = 364,
OpGroupNonUniformQuadBroadcast = 365,
OpGroupNonUniformQuadSwap = 366,
OpCopyLogical = 400,
OpPtrEqual = 401,
OpPtrNotEqual = 402,
OpPtrDiff = 403,
OpColorAttachmentReadEXT = 4160,
OpDepthAttachmentReadEXT = 4161,
OpStencilAttachmentReadEXT = 4162,
OpTerminateInvocation = 4416,
OpSubgroupBallotKHR = 4421,
OpSubgroupFirstInvocationKHR = 4422,
OpSubgroupAllKHR = 4428,
OpSubgroupAnyKHR = 4429,
OpSubgroupAllEqualKHR = 4430,
OpGroupNonUniformRotateKHR = 4431,
OpSubgroupReadInvocationKHR = 4432,
OpExtInstWithForwardRefsKHR = 4433,
OpTraceRayKHR = 4445,
OpExecuteCallableKHR = 4446,
OpConvertUToAccelerationStructureKHR = 4447,
OpIgnoreIntersectionKHR = 4448,
OpTerminateRayKHR = 4449,
OpSDot = 4450,
OpSDotKHR = 4450,
OpUDot = 4451,
OpUDotKHR = 4451,
OpSUDot = 4452,
OpSUDotKHR = 4452,
OpSDotAccSat = 4453,
OpSDotAccSatKHR = 4453,
OpUDotAccSat = 4454,
OpUDotAccSatKHR = 4454,
OpSUDotAccSat = 4455,
OpSUDotAccSatKHR = 4455,
OpTypeCooperativeMatrixKHR = 4456,
OpCooperativeMatrixLoadKHR = 4457,
OpCooperativeMatrixStoreKHR = 4458,
OpCooperativeMatrixMulAddKHR = 4459,
OpCooperativeMatrixLengthKHR = 4460,
OpConstantCompositeReplicateEXT = 4461,
OpSpecConstantCompositeReplicateEXT = 4462,
OpCompositeConstructReplicateEXT = 4463,
OpTypeRayQueryKHR = 4472,
OpRayQueryInitializeKHR = 4473,
OpRayQueryTerminateKHR = 4474,
OpRayQueryGenerateIntersectionKHR = 4475,
OpRayQueryConfirmIntersectionKHR = 4476,
OpRayQueryProceedKHR = 4477,
OpRayQueryGetIntersectionTypeKHR = 4479,
OpImageSampleWeightedQCOM = 4480,
OpImageBoxFilterQCOM = 4481,
OpImageBlockMatchSSDQCOM = 4482,
OpImageBlockMatchSADQCOM = 4483,
OpImageBlockMatchWindowSSDQCOM = 4500,
OpImageBlockMatchWindowSADQCOM = 4501,
OpImageBlockMatchGatherSSDQCOM = 4502,
OpImageBlockMatchGatherSADQCOM = 4503,
OpGroupIAddNonUniformAMD = 5000,
OpGroupFAddNonUniformAMD = 5001,
OpGroupFMinNonUniformAMD = 5002,
OpGroupUMinNonUniformAMD = 5003,
OpGroupSMinNonUniformAMD = 5004,
OpGroupFMaxNonUniformAMD = 5005,
OpGroupUMaxNonUniformAMD = 5006,
OpGroupSMaxNonUniformAMD = 5007,
OpFragmentMaskFetchAMD = 5011,
OpFragmentFetchAMD = 5012,
OpReadClockKHR = 5056,
OpGroupNonUniformQuadAllKHR = 5110,
OpGroupNonUniformQuadAnyKHR = 5111,
OpHitObjectRecordHitMotionNV = 5249,
OpHitObjectRecordHitWithIndexMotionNV = 5250,
OpHitObjectRecordMissMotionNV = 5251,
OpHitObjectGetWorldToObjectNV = 5252,
OpHitObjectGetObjectToWorldNV = 5253,
OpHitObjectGetObjectRayDirectionNV = 5254,
OpHitObjectGetObjectRayOriginNV = 5255,
OpHitObjectTraceRayMotionNV = 5256,
OpHitObjectGetShaderRecordBufferHandleNV = 5257,
OpHitObjectGetShaderBindingTableRecordIndexNV = 5258,
OpHitObjectRecordEmptyNV = 5259,
OpHitObjectTraceRayNV = 5260,
OpHitObjectRecordHitNV = 5261,
OpHitObjectRecordHitWithIndexNV = 5262,
OpHitObjectRecordMissNV = 5263,
OpHitObjectExecuteShaderNV = 5264,
OpHitObjectGetCurrentTimeNV = 5265,
OpHitObjectGetAttributesNV = 5266,
OpHitObjectGetHitKindNV = 5267,
OpHitObjectGetPrimitiveIndexNV = 5268,
OpHitObjectGetGeometryIndexNV = 5269,
OpHitObjectGetInstanceIdNV = 5270,
OpHitObjectGetInstanceCustomIndexNV = 5271,
OpHitObjectGetWorldRayDirectionNV = 5272,
OpHitObjectGetWorldRayOriginNV = 5273,
OpHitObjectGetRayTMaxNV = 5274,
OpHitObjectGetRayTMinNV = 5275,
OpHitObjectIsEmptyNV = 5276,
OpHitObjectIsHitNV = 5277,
OpHitObjectIsMissNV = 5278,
OpReorderThreadWithHitObjectNV = 5279,
OpReorderThreadWithHintNV = 5280,
OpTypeHitObjectNV = 5281,
OpImageSampleFootprintNV = 5283,
OpEmitMeshTasksEXT = 5294,
OpSetMeshOutputsEXT = 5295,
OpGroupNonUniformPartitionNV = 5296,
OpWritePackedPrimitiveIndices4x8NV = 5299,
OpFetchMicroTriangleVertexPositionNV = 5300,
OpFetchMicroTriangleVertexBarycentricNV = 5301,
OpReportIntersectionKHR = 5334,
OpReportIntersectionNV = 5334,
OpIgnoreIntersectionNV = 5335,
OpTerminateRayNV = 5336,
OpTraceNV = 5337,
OpTraceMotionNV = 5338,
OpTraceRayMotionNV = 5339,
OpRayQueryGetIntersectionTriangleVertexPositionsKHR = 5340,
OpTypeAccelerationStructureKHR = 5341,
OpTypeAccelerationStructureNV = 5341,
OpExecuteCallableNV = 5344,
OpTypeCooperativeMatrixNV = 5358,
OpCooperativeMatrixLoadNV = 5359,
OpCooperativeMatrixStoreNV = 5360,
OpCooperativeMatrixMulAddNV = 5361,
OpCooperativeMatrixLengthNV = 5362,
OpBeginInvocationInterlockEXT = 5364,
OpEndInvocationInterlockEXT = 5365,
OpDemoteToHelperInvocation = 5380,
OpDemoteToHelperInvocationEXT = 5380,
OpIsHelperInvocationEXT = 5381,
OpConvertUToImageNV = 5391,
OpConvertUToSamplerNV = 5392,
OpConvertImageToUNV = 5393,
OpConvertSamplerToUNV = 5394,
OpConvertUToSampledImageNV = 5395,
OpConvertSampledImageToUNV = 5396,
OpSamplerImageAddressingModeNV = 5397,
OpSubgroupShuffleINTEL = 5571,
OpSubgroupShuffleDownINTEL = 5572,
OpSubgroupShuffleUpINTEL = 5573,
OpSubgroupShuffleXorINTEL = 5574,
OpSubgroupBlockReadINTEL = 5575,
OpSubgroupBlockWriteINTEL = 5576,
OpSubgroupImageBlockReadINTEL = 5577,
OpSubgroupImageBlockWriteINTEL = 5578,
OpSubgroupImageMediaBlockReadINTEL = 5580,
OpSubgroupImageMediaBlockWriteINTEL = 5581,
OpUCountLeadingZerosINTEL = 5585,
OpUCountTrailingZerosINTEL = 5586,
OpAbsISubINTEL = 5587,
OpAbsUSubINTEL = 5588,
OpIAddSatINTEL = 5589,
OpUAddSatINTEL = 5590,
OpIAverageINTEL = 5591,
OpUAverageINTEL = 5592,
OpIAverageRoundedINTEL = 5593,
OpUAverageRoundedINTEL = 5594,
OpISubSatINTEL = 5595,
OpUSubSatINTEL = 5596,
OpIMul32x16INTEL = 5597,
OpUMul32x16INTEL = 5598,
OpConstantFunctionPointerINTEL = 5600,
OpFunctionPointerCallINTEL = 5601,
OpAsmTargetINTEL = 5609,
OpAsmINTEL = 5610,
OpAsmCallINTEL = 5611,
OpAtomicFMinEXT = 5614,
OpAtomicFMaxEXT = 5615,
OpAssumeTrueKHR = 5630,
OpExpectKHR = 5631,
OpDecorateString = 5632,
OpDecorateStringGOOGLE = 5632,
OpMemberDecorateString = 5633,
OpMemberDecorateStringGOOGLE = 5633,
OpVmeImageINTEL = 5699,
OpTypeVmeImageINTEL = 5700,
OpTypeAvcImePayloadINTEL = 5701,
OpTypeAvcRefPayloadINTEL = 5702,
OpTypeAvcSicPayloadINTEL = 5703,
OpTypeAvcMcePayloadINTEL = 5704,
OpTypeAvcMceResultINTEL = 5705,
OpTypeAvcImeResultINTEL = 5706,
OpTypeAvcImeResultSingleReferenceStreamoutINTEL = 5707,
OpTypeAvcImeResultDualReferenceStreamoutINTEL = 5708,
OpTypeAvcImeSingleReferenceStreaminINTEL = 5709,
OpTypeAvcImeDualReferenceStreaminINTEL = 5710,
OpTypeAvcRefResultINTEL = 5711,
OpTypeAvcSicResultINTEL = 5712,
OpSubgroupAvcMceGetDefaultInterBaseMultiReferencePenaltyINTEL = 5713,
OpSubgroupAvcMceSetInterBaseMultiReferencePenaltyINTEL = 5714,
OpSubgroupAvcMceGetDefaultInterShapePenaltyINTEL = 5715,
OpSubgroupAvcMceSetInterShapePenaltyINTEL = 5716,
OpSubgroupAvcMceGetDefaultInterDirectionPenaltyINTEL = 5717,
OpSubgroupAvcMceSetInterDirectionPenaltyINTEL = 5718,
OpSubgroupAvcMceGetDefaultIntraLumaShapePenaltyINTEL = 5719,
OpSubgroupAvcMceGetDefaultInterMotionVectorCostTableINTEL = 5720,
OpSubgroupAvcMceGetDefaultHighPenaltyCostTableINTEL = 5721,
OpSubgroupAvcMceGetDefaultMediumPenaltyCostTableINTEL = 5722,
OpSubgroupAvcMceGetDefaultLowPenaltyCostTableINTEL = 5723,
OpSubgroupAvcMceSetMotionVectorCostFunctionINTEL = 5724,
OpSubgroupAvcMceGetDefaultIntraLumaModePenaltyINTEL = 5725,
OpSubgroupAvcMceGetDefaultNonDcLumaIntraPenaltyINTEL = 5726,
OpSubgroupAvcMceGetDefaultIntraChromaModeBasePenaltyINTEL = 5727,
OpSubgroupAvcMceSetAcOnlyHaarINTEL = 5728,
OpSubgroupAvcMceSetSourceInterlacedFieldPolarityINTEL = 5729,
OpSubgroupAvcMceSetSingleReferenceInterlacedFieldPolarityINTEL = 5730,
OpSubgroupAvcMceSetDualReferenceInterlacedFieldPolaritiesINTEL = 5731,
OpSubgroupAvcMceConvertToImePayloadINTEL = 5732,
OpSubgroupAvcMceConvertToImeResultINTEL = 5733,
OpSubgroupAvcMceConvertToRefPayloadINTEL = 5734,
OpSubgroupAvcMceConvertToRefResultINTEL = 5735,
OpSubgroupAvcMceConvertToSicPayloadINTEL = 5736,
OpSubgroupAvcMceConvertToSicResultINTEL = 5737,
OpSubgroupAvcMceGetMotionVectorsINTEL = 5738,
OpSubgroupAvcMceGetInterDistortionsINTEL = 5739,
OpSubgroupAvcMceGetBestInterDistortionsINTEL = 5740,
OpSubgroupAvcMceGetInterMajorShapeINTEL = 5741,
OpSubgroupAvcMceGetInterMinorShapeINTEL = 5742,
OpSubgroupAvcMceGetInterDirectionsINTEL = 5743,
OpSubgroupAvcMceGetInterMotionVectorCountINTEL = 5744,
OpSubgroupAvcMceGetInterReferenceIdsINTEL = 5745,
OpSubgroupAvcMceGetInterReferenceInterlacedFieldPolaritiesINTEL = 5746,
OpSubgroupAvcImeInitializeINTEL = 5747,
OpSubgroupAvcImeSetSingleReferenceINTEL = 5748,
OpSubgroupAvcImeSetDualReferenceINTEL = 5749,
OpSubgroupAvcImeRefWindowSizeINTEL = 5750,
OpSubgroupAvcImeAdjustRefOffsetINTEL = 5751,
OpSubgroupAvcImeConvertToMcePayloadINTEL = 5752,
OpSubgroupAvcImeSetMaxMotionVectorCountINTEL = 5753,
OpSubgroupAvcImeSetUnidirectionalMixDisableINTEL = 5754,
OpSubgroupAvcImeSetEarlySearchTerminationThresholdINTEL = 5755,
OpSubgroupAvcImeSetWeightedSadINTEL = 5756,
OpSubgroupAvcImeEvaluateWithSingleReferenceINTEL = 5757,
OpSubgroupAvcImeEvaluateWithDualReferenceINTEL = 5758,
OpSubgroupAvcImeEvaluateWithSingleReferenceStreaminINTEL = 5759,
OpSubgroupAvcImeEvaluateWithDualReferenceStreaminINTEL = 5760,
OpSubgroupAvcImeEvaluateWithSingleReferenceStreamoutINTEL = 5761,
OpSubgroupAvcImeEvaluateWithDualReferenceStreamoutINTEL = 5762,
OpSubgroupAvcImeEvaluateWithSingleReferenceStreaminoutINTEL = 5763,
OpSubgroupAvcImeEvaluateWithDualReferenceStreaminoutINTEL = 5764,
OpSubgroupAvcImeConvertToMceResultINTEL = 5765,
OpSubgroupAvcImeGetSingleReferenceStreaminINTEL = 5766,
OpSubgroupAvcImeGetDualReferenceStreaminINTEL = 5767,
OpSubgroupAvcImeStripSingleReferenceStreamoutINTEL = 5768,
OpSubgroupAvcImeStripDualReferenceStreamoutINTEL = 5769,
OpSubgroupAvcImeGetStreamoutSingleReferenceMajorShapeMotionVectorsINTEL = 5770,
OpSubgroupAvcImeGetStreamoutSingleReferenceMajorShapeDistortionsINTEL = 5771,
OpSubgroupAvcImeGetStreamoutSingleReferenceMajorShapeReferenceIdsINTEL = 5772,
OpSubgroupAvcImeGetStreamoutDualReferenceMajorShapeMotionVectorsINTEL = 5773,
OpSubgroupAvcImeGetStreamoutDualReferenceMajorShapeDistortionsINTEL = 5774,
OpSubgroupAvcImeGetStreamoutDualReferenceMajorShapeReferenceIdsINTEL = 5775,
OpSubgroupAvcImeGetBorderReachedINTEL = 5776,
OpSubgroupAvcImeGetTruncatedSearchIndicationINTEL = 5777,
OpSubgroupAvcImeGetUnidirectionalEarlySearchTerminationINTEL = 5778,
OpSubgroupAvcImeGetWeightingPatternMinimumMotionVectorINTEL = 5779,
OpSubgroupAvcImeGetWeightingPatternMinimumDistortionINTEL = 5780,
OpSubgroupAvcFmeInitializeINTEL = 5781,
OpSubgroupAvcBmeInitializeINTEL = 5782,
OpSubgroupAvcRefConvertToMcePayloadINTEL = 5783,
OpSubgroupAvcRefSetBidirectionalMixDisableINTEL = 5784,
OpSubgroupAvcRefSetBilinearFilterEnableINTEL = 5785,
OpSubgroupAvcRefEvaluateWithSingleReferenceINTEL = 5786,
OpSubgroupAvcRefEvaluateWithDualReferenceINTEL = 5787,
OpSubgroupAvcRefEvaluateWithMultiReferenceINTEL = 5788,
OpSubgroupAvcRefEvaluateWithMultiReferenceInterlacedINTEL = 5789,
OpSubgroupAvcRefConvertToMceResultINTEL = 5790,
OpSubgroupAvcSicInitializeINTEL = 5791,
OpSubgroupAvcSicConfigureSkcINTEL = 5792,
OpSubgroupAvcSicConfigureIpeLumaINTEL = 5793,
OpSubgroupAvcSicConfigureIpeLumaChromaINTEL = 5794,
OpSubgroupAvcSicGetMotionVectorMaskINTEL = 5795,
OpSubgroupAvcSicConvertToMcePayloadINTEL = 5796,
OpSubgroupAvcSicSetIntraLumaShapePenaltyINTEL = 5797,
OpSubgroupAvcSicSetIntraLumaModeCostFunctionINTEL = 5798,
OpSubgroupAvcSicSetIntraChromaModeCostFunctionINTEL = 5799,
OpSubgroupAvcSicSetBilinearFilterEnableINTEL = 5800,
OpSubgroupAvcSicSetSkcForwardTransformEnableINTEL = 5801,
OpSubgroupAvcSicSetBlockBasedRawSkipSadINTEL = 5802,
OpSubgroupAvcSicEvaluateIpeINTEL = 5803,
OpSubgroupAvcSicEvaluateWithSingleReferenceINTEL = 5804,
OpSubgroupAvcSicEvaluateWithDualReferenceINTEL = 5805,
OpSubgroupAvcSicEvaluateWithMultiReferenceINTEL = 5806,
OpSubgroupAvcSicEvaluateWithMultiReferenceInterlacedINTEL = 5807,
OpSubgroupAvcSicConvertToMceResultINTEL = 5808,
OpSubgroupAvcSicGetIpeLumaShapeINTEL = 5809,
OpSubgroupAvcSicGetBestIpeLumaDistortionINTEL = 5810,
OpSubgroupAvcSicGetBestIpeChromaDistortionINTEL = 5811,
OpSubgroupAvcSicGetPackedIpeLumaModesINTEL = 5812,
OpSubgroupAvcSicGetIpeChromaModeINTEL = 5813,
OpSubgroupAvcSicGetPackedSkcLumaCountThresholdINTEL = 5814,
OpSubgroupAvcSicGetPackedSkcLumaSumThresholdINTEL = 5815,
OpSubgroupAvcSicGetInterRawSadsINTEL = 5816,
OpVariableLengthArrayINTEL = 5818,
OpSaveMemoryINTEL = 5819,
OpRestoreMemoryINTEL = 5820,
OpArbitraryFloatSinCosPiINTEL = 5840,
OpArbitraryFloatCastINTEL = 5841,
OpArbitraryFloatCastFromIntINTEL = 5842,
OpArbitraryFloatCastToIntINTEL = 5843,
OpArbitraryFloatAddINTEL = 5846,
OpArbitraryFloatSubINTEL = 5847,
OpArbitraryFloatMulINTEL = 5848,
OpArbitraryFloatDivINTEL = 5849,
OpArbitraryFloatGTINTEL = 5850,
OpArbitraryFloatGEINTEL = 5851,
OpArbitraryFloatLTINTEL = 5852,
OpArbitraryFloatLEINTEL = 5853,
OpArbitraryFloatEQINTEL = 5854,
OpArbitraryFloatRecipINTEL = 5855,
OpArbitraryFloatRSqrtINTEL = 5856,
OpArbitraryFloatCbrtINTEL = 5857,
OpArbitraryFloatHypotINTEL = 5858,
OpArbitraryFloatSqrtINTEL = 5859,
OpArbitraryFloatLogINTEL = 5860,
OpArbitraryFloatLog2INTEL = 5861,
OpArbitraryFloatLog10INTEL = 5862,
OpArbitraryFloatLog1pINTEL = 5863,
OpArbitraryFloatExpINTEL = 5864,
OpArbitraryFloatExp2INTEL = 5865,
OpArbitraryFloatExp10INTEL = 5866,
OpArbitraryFloatExpm1INTEL = 5867,
OpArbitraryFloatSinINTEL = 5868,
OpArbitraryFloatCosINTEL = 5869,
OpArbitraryFloatSinCosINTEL = 5870,
OpArbitraryFloatSinPiINTEL = 5871,
OpArbitraryFloatCosPiINTEL = 5872,
OpArbitraryFloatASinINTEL = 5873,
OpArbitraryFloatASinPiINTEL = 5874,
OpArbitraryFloatACosINTEL = 5875,
OpArbitraryFloatACosPiINTEL = 5876,
OpArbitraryFloatATanINTEL = 5877,
OpArbitraryFloatATanPiINTEL = 5878,
OpArbitraryFloatATan2INTEL = 5879,
OpArbitraryFloatPowINTEL = 5880,
OpArbitraryFloatPowRINTEL = 5881,
OpArbitraryFloatPowNINTEL = 5882,
OpLoopControlINTEL = 5887,
OpAliasDomainDeclINTEL = 5911,
OpAliasScopeDeclINTEL = 5912,
OpAliasScopeListDeclINTEL = 5913,
OpFixedSqrtINTEL = 5923,
OpFixedRecipINTEL = 5924,
OpFixedRsqrtINTEL = 5925,
OpFixedSinINTEL = 5926,
OpFixedCosINTEL = 5927,
OpFixedSinCosINTEL = 5928,
OpFixedSinPiINTEL = 5929,
OpFixedCosPiINTEL = 5930,
OpFixedSinCosPiINTEL = 5931,
OpFixedLogINTEL = 5932,
OpFixedExpINTEL = 5933,
OpPtrCastToCrossWorkgroupINTEL = 5934,
OpCrossWorkgroupCastToPtrINTEL = 5938,
OpReadPipeBlockingINTEL = 5946,
OpWritePipeBlockingINTEL = 5947,
OpFPGARegINTEL = 5949,
OpRayQueryGetRayTMinKHR = 6016,
OpRayQueryGetRayFlagsKHR = 6017,
OpRayQueryGetIntersectionTKHR = 6018,
OpRayQueryGetIntersectionInstanceCustomIndexKHR = 6019,
OpRayQueryGetIntersectionInstanceIdKHR = 6020,
OpRayQueryGetIntersectionInstanceShaderBindingTableRecordOffsetKHR = 6021,
OpRayQueryGetIntersectionGeometryIndexKHR = 6022,
OpRayQueryGetIntersectionPrimitiveIndexKHR = 6023,
OpRayQueryGetIntersectionBarycentricsKHR = 6024,
OpRayQueryGetIntersectionFrontFaceKHR = 6025,
OpRayQueryGetIntersectionCandidateAABBOpaqueKHR = 6026,
OpRayQueryGetIntersectionObjectRayDirectionKHR = 6027,
OpRayQueryGetIntersectionObjectRayOriginKHR = 6028,
OpRayQueryGetWorldRayDirectionKHR = 6029,
OpRayQueryGetWorldRayOriginKHR = 6030,
OpRayQueryGetIntersectionObjectToWorldKHR = 6031,
OpRayQueryGetIntersectionWorldToObjectKHR = 6032,
OpAtomicFAddEXT = 6035,
OpTypeBufferSurfaceINTEL = 6086,
OpTypeStructContinuedINTEL = 6090,
OpConstantCompositeContinuedINTEL = 6091,
OpSpecConstantCompositeContinuedINTEL = 6092,
OpControlBarrierArriveINTEL = 6142,
OpControlBarrierWaitINTEL = 6143,
OpGroupIMulKHR = 6401,
OpGroupFMulKHR = 6402,
OpGroupBitwiseAndKHR = 6403,
OpGroupBitwiseOrKHR = 6404,
OpGroupBitwiseXorKHR = 6405,
OpGroupLogicalAndKHR = 6406,
OpGroupLogicalOrKHR = 6407,
OpGroupLogicalXorKHR = 6408,
OpMax = 0x7fffffff,
};
#ifdef SPV_ENABLE_UTILITY_CODE
#ifndef __cplusplus
#include <stdbool.h>
#endif
inline void HasResultAndType(Op opcode, bool *hasResult, bool *hasResultType) {
*hasResult = *hasResultType = false;
switch (opcode) {
default: /* unknown opcode */ break;
case OpNop: *hasResult = false; *hasResultType = false; break;
case OpUndef: *hasResult = true; *hasResultType = true; break;
case OpSourceContinued: *hasResult = false; *hasResultType = false; break;
case OpSource: *hasResult = false; *hasResultType = false; break;
case OpSourceExtension: *hasResult = false; *hasResultType = false; break;
case OpName: *hasResult = false; *hasResultType = false; break;
case OpMemberName: *hasResult = false; *hasResultType = false; break;
case OpString: *hasResult = true; *hasResultType = false; break;
case OpLine: *hasResult = false; *hasResultType = false; break;
case OpExtension: *hasResult = false; *hasResultType = false; break;
case OpExtInstImport: *hasResult = true; *hasResultType = false; break;
case OpExtInst: *hasResult = true; *hasResultType = true; break;
case OpMemoryModel: *hasResult = false; *hasResultType = false; break;
case OpEntryPoint: *hasResult = false; *hasResultType = false; break;
case OpExecutionMode: *hasResult = false; *hasResultType = false; break;
case OpCapability: *hasResult = false; *hasResultType = false; break;
case OpTypeVoid: *hasResult = true; *hasResultType = false; break;
case OpTypeBool: *hasResult = true; *hasResultType = false; break;
case OpTypeInt: *hasResult = true; *hasResultType = false; break;
case OpTypeFloat: *hasResult = true; *hasResultType = false; break;
case OpTypeVector: *hasResult = true; *hasResultType = false; break;
case OpTypeMatrix: *hasResult = true; *hasResultType = false; break;
case OpTypeImage: *hasResult = true; *hasResultType = false; break;
case OpTypeSampler: *hasResult = true; *hasResultType = false; break;
case OpTypeSampledImage: *hasResult = true; *hasResultType = false; break;
case OpTypeArray: *hasResult = true; *hasResultType = false; break;
case OpTypeRuntimeArray: *hasResult = true; *hasResultType = false; break;
case OpTypeStruct: *hasResult = true; *hasResultType = false; break;
case OpTypeOpaque: *hasResult = true; *hasResultType = false; break;
case OpTypePointer: *hasResult = true; *hasResultType = false; break;
case OpTypeFunction: *hasResult = true; *hasResultType = false; break;
case OpTypeEvent: *hasResult = true; *hasResultType = false; break;
case OpTypeDeviceEvent: *hasResult = true; *hasResultType = false; break;
case OpTypeReserveId: *hasResult = true; *hasResultType = false; break;
case OpTypeQueue: *hasResult = true; *hasResultType = false; break;
case OpTypePipe: *hasResult = true; *hasResultType = false; break;
case OpTypeForwardPointer: *hasResult = false; *hasResultType = false; break;
case OpConstantTrue: *hasResult = true; *hasResultType = true; break;
case OpConstantFalse: *hasResult = true; *hasResultType = true; break;
case OpConstant: *hasResult = true; *hasResultType = true; break;
case OpConstantComposite: *hasResult = true; *hasResultType = true; break;
case OpConstantSampler: *hasResult = true; *hasResultType = true; break;
case OpConstantNull: *hasResult = true; *hasResultType = true; break;
case OpSpecConstantTrue: *hasResult = true; *hasResultType = true; break;
case OpSpecConstantFalse: *hasResult = true; *hasResultType = true; break;
case OpSpecConstant: *hasResult = true; *hasResultType = true; break;
case OpSpecConstantComposite: *hasResult = true; *hasResultType = true; break;
case OpSpecConstantOp: *hasResult = true; *hasResultType = true; break;
case OpFunction: *hasResult = true; *hasResultType = true; break;
case OpFunctionParameter: *hasResult = true; *hasResultType = true; break;
case OpFunctionEnd: *hasResult = false; *hasResultType = false; break;
case OpFunctionCall: *hasResult = true; *hasResultType = true; break;
case OpVariable: *hasResult = true; *hasResultType = true; break;
case OpImageTexelPointer: *hasResult = true; *hasResultType = true; break;
case OpLoad: *hasResult = true; *hasResultType = true; break;
case OpStore: *hasResult = false; *hasResultType = false; break;
case OpCopyMemory: *hasResult = false; *hasResultType = false; break;
case OpCopyMemorySized: *hasResult = false; *hasResultType = false; break;
case OpAccessChain: *hasResult = true; *hasResultType = true; break;
case OpInBoundsAccessChain: *hasResult = true; *hasResultType = true; break;
case OpPtrAccessChain: *hasResult = true; *hasResultType = true; break;
case OpArrayLength: *hasResult = true; *hasResultType = true; break;
case OpGenericPtrMemSemantics: *hasResult = true; *hasResultType = true; break;
case OpInBoundsPtrAccessChain: *hasResult = true; *hasResultType = true; break;
case OpDecorate: *hasResult = false; *hasResultType = false; break;
case OpMemberDecorate: *hasResult = false; *hasResultType = false; break;
case OpDecorationGroup: *hasResult = true; *hasResultType = false; break;
case OpGroupDecorate: *hasResult = false; *hasResultType = false; break;
case OpGroupMemberDecorate: *hasResult = false; *hasResultType = false; break;
case OpVectorExtractDynamic: *hasResult = true; *hasResultType = true; break;
case OpVectorInsertDynamic: *hasResult = true; *hasResultType = true; break;
case OpVectorShuffle: *hasResult = true; *hasResultType = true; break;
case OpCompositeConstruct: *hasResult = true; *hasResultType = true; break;
case OpCompositeExtract: *hasResult = true; *hasResultType = true; break;
case OpCompositeInsert: *hasResult = true; *hasResultType = true; break;
case OpCopyObject: *hasResult = true; *hasResultType = true; break;
case OpTranspose: *hasResult = true; *hasResultType = true; break;
case OpSampledImage: *hasResult = true; *hasResultType = true; break;
case OpImageSampleImplicitLod: *hasResult = true; *hasResultType = true; break;
case OpImageSampleExplicitLod: *hasResult = true; *hasResultType = true; break;
case OpImageSampleDrefImplicitLod: *hasResult = true; *hasResultType = true; break;
case OpImageSampleDrefExplicitLod: *hasResult = true; *hasResultType = true; break;
case OpImageSampleProjImplicitLod: *hasResult = true; *hasResultType = true; break;
case OpImageSampleProjExplicitLod: *hasResult = true; *hasResultType = true; break;
case OpImageSampleProjDrefImplicitLod: *hasResult = true; *hasResultType = true; break;
case OpImageSampleProjDrefExplicitLod: *hasResult = true; *hasResultType = true; break;
case OpImageFetch: *hasResult = true; *hasResultType = true; break;
case OpImageGather: *hasResult = true; *hasResultType = true; break;
case OpImageDrefGather: *hasResult = true; *hasResultType = true; break;
case OpImageRead: *hasResult = true; *hasResultType = true; break;
case OpImageWrite: *hasResult = false; *hasResultType = false; break;
case OpImage: *hasResult = true; *hasResultType = true; break;
case OpImageQueryFormat: *hasResult = true; *hasResultType = true; break;
case OpImageQueryOrder: *hasResult = true; *hasResultType = true; break;
case OpImageQuerySizeLod: *hasResult = true; *hasResultType = true; break;
case OpImageQuerySize: *hasResult = true; *hasResultType = true; break;
case OpImageQueryLod: *hasResult = true; *hasResultType = true; break;
case OpImageQueryLevels: *hasResult = true; *hasResultType = true; break;
case OpImageQuerySamples: *hasResult = true; *hasResultType = true; break;
case OpConvertFToU: *hasResult = true; *hasResultType = true; break;
case OpConvertFToS: *hasResult = true; *hasResultType = true; break;
case OpConvertSToF: *hasResult = true; *hasResultType = true; break;
case OpConvertUToF: *hasResult = true; *hasResultType = true; break;
case OpUConvert: *hasResult = true; *hasResultType = true; break;
case OpSConvert: *hasResult = true; *hasResultType = true; break;
case OpFConvert: *hasResult = true; *hasResultType = true; break;
case OpQuantizeToF16: *hasResult = true; *hasResultType = true; break;
case OpConvertPtrToU: *hasResult = true; *hasResultType = true; break;
case OpSatConvertSToU: *hasResult = true; *hasResultType = true; break;
case OpSatConvertUToS: *hasResult = true; *hasResultType = true; break;
case OpConvertUToPtr: *hasResult = true; *hasResultType = true; break;
case OpPtrCastToGeneric: *hasResult = true; *hasResultType = true; break;
case OpGenericCastToPtr: *hasResult = true; *hasResultType = true; break;
case OpGenericCastToPtrExplicit: *hasResult = true; *hasResultType = true; break;
case OpBitcast: *hasResult = true; *hasResultType = true; break;
case OpSNegate: *hasResult = true; *hasResultType = true; break;
case OpFNegate: *hasResult = true; *hasResultType = true; break;
case OpIAdd: *hasResult = true; *hasResultType = true; break;
case OpFAdd: *hasResult = true; *hasResultType = true; break;
case OpISub: *hasResult = true; *hasResultType = true; break;
case OpFSub: *hasResult = true; *hasResultType = true; break;
case OpIMul: *hasResult = true; *hasResultType = true; break;
case OpFMul: *hasResult = true; *hasResultType = true; break;
case OpUDiv: *hasResult = true; *hasResultType = true; break;
case OpSDiv: *hasResult = true; *hasResultType = true; break;
case OpFDiv: *hasResult = true; *hasResultType = true; break;
case OpUMod: *hasResult = true; *hasResultType = true; break;
case OpSRem: *hasResult = true; *hasResultType = true; break;
case OpSMod: *hasResult = true; *hasResultType = true; break;
case OpFRem: *hasResult = true; *hasResultType = true; break;
case OpFMod: *hasResult = true; *hasResultType = true; break;
case OpVectorTimesScalar: *hasResult = true; *hasResultType = true; break;
case OpMatrixTimesScalar: *hasResult = true; *hasResultType = true; break;
case OpVectorTimesMatrix: *hasResult = true; *hasResultType = true; break;
case OpMatrixTimesVector: *hasResult = true; *hasResultType = true; break;
case OpMatrixTimesMatrix: *hasResult = true; *hasResultType = true; break;
case OpOuterProduct: *hasResult = true; *hasResultType = true; break;
case OpDot: *hasResult = true; *hasResultType = true; break;
case OpIAddCarry: *hasResult = true; *hasResultType = true; break;
case OpISubBorrow: *hasResult = true; *hasResultType = true; break;
case OpUMulExtended: *hasResult = true; *hasResultType = true; break;
case OpSMulExtended: *hasResult = true; *hasResultType = true; break;
case OpAny: *hasResult = true; *hasResultType = true; break;
case OpAll: *hasResult = true; *hasResultType = true; break;
case OpIsNan: *hasResult = true; *hasResultType = true; break;
case OpIsInf: *hasResult = true; *hasResultType = true; break;
case OpIsFinite: *hasResult = true; *hasResultType = true; break;
case OpIsNormal: *hasResult = true; *hasResultType = true; break;
case OpSignBitSet: *hasResult = true; *hasResultType = true; break;
case OpLessOrGreater: *hasResult = true; *hasResultType = true; break;
case OpOrdered: *hasResult = true; *hasResultType = true; break;
case OpUnordered: *hasResult = true; *hasResultType = true; break;
case OpLogicalEqual: *hasResult = true; *hasResultType = true; break;
case OpLogicalNotEqual: *hasResult = true; *hasResultType = true; break;
case OpLogicalOr: *hasResult = true; *hasResultType = true; break;
case OpLogicalAnd: *hasResult = true; *hasResultType = true; break;
case OpLogicalNot: *hasResult = true; *hasResultType = true; break;
case OpSelect: *hasResult = true; *hasResultType = true; break;
case OpIEqual: *hasResult = true; *hasResultType = true; break;
case OpINotEqual: *hasResult = true; *hasResultType = true; break;
case OpUGreaterThan: *hasResult = true; *hasResultType = true; break;
case OpSGreaterThan: *hasResult = true; *hasResultType = true; break;
case OpUGreaterThanEqual: *hasResult = true; *hasResultType = true; break;
case OpSGreaterThanEqual: *hasResult = true; *hasResultType = true; break;
case OpULessThan: *hasResult = true; *hasResultType = true; break;
case OpSLessThan: *hasResult = true; *hasResultType = true; break;
case OpULessThanEqual: *hasResult = true; *hasResultType = true; break;
case OpSLessThanEqual: *hasResult = true; *hasResultType = true; break;
case OpFOrdEqual: *hasResult = true; *hasResultType = true; break;
case OpFUnordEqual: *hasResult = true; *hasResultType = true; break;
case OpFOrdNotEqual: *hasResult = true; *hasResultType = true; break;
case OpFUnordNotEqual: *hasResult = true; *hasResultType = true; break;
case OpFOrdLessThan: *hasResult = true; *hasResultType = true; break;
case OpFUnordLessThan: *hasResult = true; *hasResultType = true; break;
case OpFOrdGreaterThan: *hasResult = true; *hasResultType = true; break;
case OpFUnordGreaterThan: *hasResult = true; *hasResultType = true; break;
case OpFOrdLessThanEqual: *hasResult = true; *hasResultType = true; break;
case OpFUnordLessThanEqual: *hasResult = true; *hasResultType = true; break;
case OpFOrdGreaterThanEqual: *hasResult = true; *hasResultType = true; break;
case OpFUnordGreaterThanEqual: *hasResult = true; *hasResultType = true; break;
case OpShiftRightLogical: *hasResult = true; *hasResultType = true; break;
case OpShiftRightArithmetic: *hasResult = true; *hasResultType = true; break;
case OpShiftLeftLogical: *hasResult = true; *hasResultType = true; break;
case OpBitwiseOr: *hasResult = true; *hasResultType = true; break;
case OpBitwiseXor: *hasResult = true; *hasResultType = true; break;
case OpBitwiseAnd: *hasResult = true; *hasResultType = true; break;
case OpNot: *hasResult = true; *hasResultType = true; break;
case OpBitFieldInsert: *hasResult = true; *hasResultType = true; break;
case OpBitFieldSExtract: *hasResult = true; *hasResultType = true; break;
case OpBitFieldUExtract: *hasResult = true; *hasResultType = true; break;
case OpBitReverse: *hasResult = true; *hasResultType = true; break;
case OpBitCount: *hasResult = true; *hasResultType = true; break;
case OpDPdx: *hasResult = true; *hasResultType = true; break;
case OpDPdy: *hasResult = true; *hasResultType = true; break;
case OpFwidth: *hasResult = true; *hasResultType = true; break;
case OpDPdxFine: *hasResult = true; *hasResultType = true; break;
case OpDPdyFine: *hasResult = true; *hasResultType = true; break;
case OpFwidthFine: *hasResult = true; *hasResultType = true; break;
case OpDPdxCoarse: *hasResult = true; *hasResultType = true; break;
case OpDPdyCoarse: *hasResult = true; *hasResultType = true; break;
case OpFwidthCoarse: *hasResult = true; *hasResultType = true; break;
case OpEmitVertex: *hasResult = false; *hasResultType = false; break;
case OpEndPrimitive: *hasResult = false; *hasResultType = false; break;
case OpEmitStreamVertex: *hasResult = false; *hasResultType = false; break;
case OpEndStreamPrimitive: *hasResult = false; *hasResultType = false; break;
case OpControlBarrier: *hasResult = false; *hasResultType = false; break;
case OpMemoryBarrier: *hasResult = false; *hasResultType = false; break;
case OpAtomicLoad: *hasResult = true; *hasResultType = true; break;
case OpAtomicStore: *hasResult = false; *hasResultType = false; break;
case OpAtomicExchange: *hasResult = true; *hasResultType = true; break;
case OpAtomicCompareExchange: *hasResult = true; *hasResultType = true; break;
case OpAtomicCompareExchangeWeak: *hasResult = true; *hasResultType = true; break;
case OpAtomicIIncrement: *hasResult = true; *hasResultType = true; break;
case OpAtomicIDecrement: *hasResult = true; *hasResultType = true; break;
case OpAtomicIAdd: *hasResult = true; *hasResultType = true; break;
case OpAtomicISub: *hasResult = true; *hasResultType = true; break;
case OpAtomicSMin: *hasResult = true; *hasResultType = true; break;
case OpAtomicUMin: *hasResult = true; *hasResultType = true; break;
case OpAtomicSMax: *hasResult = true; *hasResultType = true; break;
case OpAtomicUMax: *hasResult = true; *hasResultType = true; break;
case OpAtomicAnd: *hasResult = true; *hasResultType = true; break;
case OpAtomicOr: *hasResult = true; *hasResultType = true; break;
case OpAtomicXor: *hasResult = true; *hasResultType = true; break;
case OpPhi: *hasResult = true; *hasResultType = true; break;
case OpLoopMerge: *hasResult = false; *hasResultType = false; break;
case OpSelectionMerge: *hasResult = false; *hasResultType = false; break;
case OpLabel: *hasResult = true; *hasResultType = false; break;
case OpBranch: *hasResult = false; *hasResultType = false; break;
case OpBranchConditional: *hasResult = false; *hasResultType = false; break;
case OpSwitch: *hasResult = false; *hasResultType = false; break;
case OpKill: *hasResult = false; *hasResultType = false; break;
case OpReturn: *hasResult = false; *hasResultType = false; break;
case OpReturnValue: *hasResult = false; *hasResultType = false; break;
case OpUnreachable: *hasResult = false; *hasResultType = false; break;
case OpLifetimeStart: *hasResult = false; *hasResultType = false; break;
case OpLifetimeStop: *hasResult = false; *hasResultType = false; break;
case OpGroupAsyncCopy: *hasResult = true; *hasResultType = true; break;
case OpGroupWaitEvents: *hasResult = false; *hasResultType = false; break;
case OpGroupAll: *hasResult = true; *hasResultType = true; break;
case OpGroupAny: *hasResult = true; *hasResultType = true; break;
case OpGroupBroadcast: *hasResult = true; *hasResultType = true; break;
case OpGroupIAdd: *hasResult = true; *hasResultType = true; break;
case OpGroupFAdd: *hasResult = true; *hasResultType = true; break;
case OpGroupFMin: *hasResult = true; *hasResultType = true; break;
case OpGroupUMin: *hasResult = true; *hasResultType = true; break;
case OpGroupSMin: *hasResult = true; *hasResultType = true; break;
case OpGroupFMax: *hasResult = true; *hasResultType = true; break;
case OpGroupUMax: *hasResult = true; *hasResultType = true; break;
case OpGroupSMax: *hasResult = true; *hasResultType = true; break;
case OpReadPipe: *hasResult = true; *hasResultType = true; break;
case OpWritePipe: *hasResult = true; *hasResultType = true; break;
case OpReservedReadPipe: *hasResult = true; *hasResultType = true; break;
case OpReservedWritePipe: *hasResult = true; *hasResultType = true; break;
case OpReserveReadPipePackets: *hasResult = true; *hasResultType = true; break;
case OpReserveWritePipePackets: *hasResult = true; *hasResultType = true; break;
case OpCommitReadPipe: *hasResult = false; *hasResultType = false; break;
case OpCommitWritePipe: *hasResult = false; *hasResultType = false; break;
case OpIsValidReserveId: *hasResult = true; *hasResultType = true; break;
case OpGetNumPipePackets: *hasResult = true; *hasResultType = true; break;
case OpGetMaxPipePackets: *hasResult = true; *hasResultType = true; break;
case OpGroupReserveReadPipePackets: *hasResult = true; *hasResultType = true; break;
case OpGroupReserveWritePipePackets: *hasResult = true; *hasResultType = true; break;
case OpGroupCommitReadPipe: *hasResult = false; *hasResultType = false; break;
case OpGroupCommitWritePipe: *hasResult = false; *hasResultType = false; break;
case OpEnqueueMarker: *hasResult = true; *hasResultType = true; break;
case OpEnqueueKernel: *hasResult = true; *hasResultType = true; break;
case OpGetKernelNDrangeSubGroupCount: *hasResult = true; *hasResultType = true; break;
case OpGetKernelNDrangeMaxSubGroupSize: *hasResult = true; *hasResultType = true; break;
case OpGetKernelWorkGroupSize: *hasResult = true; *hasResultType = true; break;
case OpGetKernelPreferredWorkGroupSizeMultiple: *hasResult = true; *hasResultType = true; break;
case OpRetainEvent: *hasResult = false; *hasResultType = false; break;
case OpReleaseEvent: *hasResult = false; *hasResultType = false; break;
case OpCreateUserEvent: *hasResult = true; *hasResultType = true; break;
case OpIsValidEvent: *hasResult = true; *hasResultType = true; break;
case OpSetUserEventStatus: *hasResult = false; *hasResultType = false; break;
case OpCaptureEventProfilingInfo: *hasResult = false; *hasResultType = false; break;
case OpGetDefaultQueue: *hasResult = true; *hasResultType = true; break;
case OpBuildNDRange: *hasResult = true; *hasResultType = true; break;
case OpImageSparseSampleImplicitLod: *hasResult = true; *hasResultType = true; break;
case OpImageSparseSampleExplicitLod: *hasResult = true; *hasResultType = true; break;
case OpImageSparseSampleDrefImplicitLod: *hasResult = true; *hasResultType = true; break;
case OpImageSparseSampleDrefExplicitLod: *hasResult = true; *hasResultType = true; break;
case OpImageSparseSampleProjImplicitLod: *hasResult = true; *hasResultType = true; break;
case OpImageSparseSampleProjExplicitLod: *hasResult = true; *hasResultType = true; break;
case OpImageSparseSampleProjDrefImplicitLod: *hasResult = true; *hasResultType = true; break;
case OpImageSparseSampleProjDrefExplicitLod: *hasResult = true; *hasResultType = true; break;
case OpImageSparseFetch: *hasResult = true; *hasResultType = true; break;
case OpImageSparseGather: *hasResult = true; *hasResultType = true; break;
case OpImageSparseDrefGather: *hasResult = true; *hasResultType = true; break;
case OpImageSparseTexelsResident: *hasResult = true; *hasResultType = true; break;
case OpNoLine: *hasResult = false; *hasResultType = false; break;
case OpAtomicFlagTestAndSet: *hasResult = true; *hasResultType = true; break;
case OpAtomicFlagClear: *hasResult = false; *hasResultType = false; break;
case OpImageSparseRead: *hasResult = true; *hasResultType = true; break;
case OpSizeOf: *hasResult = true; *hasResultType = true; break;
case OpTypePipeStorage: *hasResult = true; *hasResultType = false; break;
case OpConstantPipeStorage: *hasResult = true; *hasResultType = true; break;
case OpCreatePipeFromPipeStorage: *hasResult = true; *hasResultType = true; break;
case OpGetKernelLocalSizeForSubgroupCount: *hasResult = true; *hasResultType = true; break;
case OpGetKernelMaxNumSubgroups: *hasResult = true; *hasResultType = true; break;
case OpTypeNamedBarrier: *hasResult = true; *hasResultType = false; break;
case OpNamedBarrierInitialize: *hasResult = true; *hasResultType = true; break;
case OpMemoryNamedBarrier: *hasResult = false; *hasResultType = false; break;
case OpModuleProcessed: *hasResult = false; *hasResultType = false; break;
case OpExecutionModeId: *hasResult = false; *hasResultType = false; break;
case OpDecorateId: *hasResult = false; *hasResultType = false; break;
case OpGroupNonUniformElect: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformAll: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformAny: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformAllEqual: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformBroadcast: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformBroadcastFirst: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformBallot: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformInverseBallot: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformBallotBitExtract: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformBallotBitCount: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformBallotFindLSB: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformBallotFindMSB: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformShuffle: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformShuffleXor: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformShuffleUp: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformShuffleDown: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformIAdd: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformFAdd: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformIMul: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformFMul: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformSMin: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformUMin: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformFMin: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformSMax: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformUMax: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformFMax: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformBitwiseAnd: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformBitwiseOr: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformBitwiseXor: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformLogicalAnd: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformLogicalOr: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformLogicalXor: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformQuadBroadcast: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformQuadSwap: *hasResult = true; *hasResultType = true; break;
case OpCopyLogical: *hasResult = true; *hasResultType = true; break;
case OpPtrEqual: *hasResult = true; *hasResultType = true; break;
case OpPtrNotEqual: *hasResult = true; *hasResultType = true; break;
case OpPtrDiff: *hasResult = true; *hasResultType = true; break;
case OpExtInstWithForwardRefsKHR: *hasResult = true; *hasResultType = true; break;
case OpColorAttachmentReadEXT: *hasResult = true; *hasResultType = true; break;
case OpDepthAttachmentReadEXT: *hasResult = true; *hasResultType = true; break;
case OpStencilAttachmentReadEXT: *hasResult = true; *hasResultType = true; break;
case OpTerminateInvocation: *hasResult = false; *hasResultType = false; break;
case OpSubgroupBallotKHR: *hasResult = true; *hasResultType = true; break;
case OpSubgroupFirstInvocationKHR: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAllKHR: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAnyKHR: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAllEqualKHR: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformRotateKHR: *hasResult = true; *hasResultType = true; break;
case OpSubgroupReadInvocationKHR: *hasResult = true; *hasResultType = true; break;
case OpTraceRayKHR: *hasResult = false; *hasResultType = false; break;
case OpExecuteCallableKHR: *hasResult = false; *hasResultType = false; break;
case OpConvertUToAccelerationStructureKHR: *hasResult = true; *hasResultType = true; break;
case OpIgnoreIntersectionKHR: *hasResult = false; *hasResultType = false; break;
case OpTerminateRayKHR: *hasResult = false; *hasResultType = false; break;
case OpSDot: *hasResult = true; *hasResultType = true; break;
case OpUDot: *hasResult = true; *hasResultType = true; break;
case OpSUDot: *hasResult = true; *hasResultType = true; break;
case OpSDotAccSat: *hasResult = true; *hasResultType = true; break;
case OpUDotAccSat: *hasResult = true; *hasResultType = true; break;
case OpSUDotAccSat: *hasResult = true; *hasResultType = true; break;
case OpTypeCooperativeMatrixKHR: *hasResult = true; *hasResultType = false; break;
case OpCooperativeMatrixLoadKHR: *hasResult = true; *hasResultType = true; break;
case OpCooperativeMatrixStoreKHR: *hasResult = false; *hasResultType = false; break;
case OpCooperativeMatrixMulAddKHR: *hasResult = true; *hasResultType = true; break;
case OpCooperativeMatrixLengthKHR: *hasResult = true; *hasResultType = true; break;
case OpConstantCompositeReplicateEXT: *hasResult = true; *hasResultType = true; break;
case OpSpecConstantCompositeReplicateEXT: *hasResult = true; *hasResultType = true; break;
case OpCompositeConstructReplicateEXT: *hasResult = true; *hasResultType = true; break;
case OpTypeRayQueryKHR: *hasResult = true; *hasResultType = false; break;
case OpRayQueryInitializeKHR: *hasResult = false; *hasResultType = false; break;
case OpRayQueryTerminateKHR: *hasResult = false; *hasResultType = false; break;
case OpRayQueryGenerateIntersectionKHR: *hasResult = false; *hasResultType = false; break;
case OpRayQueryConfirmIntersectionKHR: *hasResult = false; *hasResultType = false; break;
case OpRayQueryProceedKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetIntersectionTypeKHR: *hasResult = true; *hasResultType = true; break;
case OpImageSampleWeightedQCOM: *hasResult = true; *hasResultType = true; break;
case OpImageBoxFilterQCOM: *hasResult = true; *hasResultType = true; break;
case OpImageBlockMatchSSDQCOM: *hasResult = true; *hasResultType = true; break;
case OpImageBlockMatchSADQCOM: *hasResult = true; *hasResultType = true; break;
case OpImageBlockMatchWindowSSDQCOM: *hasResult = true; *hasResultType = true; break;
case OpImageBlockMatchWindowSADQCOM: *hasResult = true; *hasResultType = true; break;
case OpImageBlockMatchGatherSSDQCOM: *hasResult = true; *hasResultType = true; break;
case OpImageBlockMatchGatherSADQCOM: *hasResult = true; *hasResultType = true; break;
case OpGroupIAddNonUniformAMD: *hasResult = true; *hasResultType = true; break;
case OpGroupFAddNonUniformAMD: *hasResult = true; *hasResultType = true; break;
case OpGroupFMinNonUniformAMD: *hasResult = true; *hasResultType = true; break;
case OpGroupUMinNonUniformAMD: *hasResult = true; *hasResultType = true; break;
case OpGroupSMinNonUniformAMD: *hasResult = true; *hasResultType = true; break;
case OpGroupFMaxNonUniformAMD: *hasResult = true; *hasResultType = true; break;
case OpGroupUMaxNonUniformAMD: *hasResult = true; *hasResultType = true; break;
case OpGroupSMaxNonUniformAMD: *hasResult = true; *hasResultType = true; break;
case OpFragmentMaskFetchAMD: *hasResult = true; *hasResultType = true; break;
case OpFragmentFetchAMD: *hasResult = true; *hasResultType = true; break;
case OpReadClockKHR: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformQuadAllKHR: *hasResult = true; *hasResultType = true; break;
case OpGroupNonUniformQuadAnyKHR: *hasResult = true; *hasResultType = true; break;
case OpHitObjectRecordHitMotionNV: *hasResult = false; *hasResultType = false; break;
case OpHitObjectRecordHitWithIndexMotionNV: *hasResult = false; *hasResultType = false; break;
case OpHitObjectRecordMissMotionNV: *hasResult = false; *hasResultType = false; break;
case OpHitObjectGetWorldToObjectNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectGetObjectToWorldNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectGetObjectRayDirectionNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectGetObjectRayOriginNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectTraceRayMotionNV: *hasResult = false; *hasResultType = false; break;
case OpHitObjectGetShaderRecordBufferHandleNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectGetShaderBindingTableRecordIndexNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectRecordEmptyNV: *hasResult = false; *hasResultType = false; break;
case OpHitObjectTraceRayNV: *hasResult = false; *hasResultType = false; break;
case OpHitObjectRecordHitNV: *hasResult = false; *hasResultType = false; break;
case OpHitObjectRecordHitWithIndexNV: *hasResult = false; *hasResultType = false; break;
case OpHitObjectRecordMissNV: *hasResult = false; *hasResultType = false; break;
case OpHitObjectExecuteShaderNV: *hasResult = false; *hasResultType = false; break;
case OpHitObjectGetCurrentTimeNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectGetAttributesNV: *hasResult = false; *hasResultType = false; break;
case OpHitObjectGetHitKindNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectGetPrimitiveIndexNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectGetGeometryIndexNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectGetInstanceIdNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectGetInstanceCustomIndexNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectGetWorldRayDirectionNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectGetWorldRayOriginNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectGetRayTMaxNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectGetRayTMinNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectIsEmptyNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectIsHitNV: *hasResult = true; *hasResultType = true; break;
case OpHitObjectIsMissNV: *hasResult = true; *hasResultType = true; break;
case OpReorderThreadWithHitObjectNV: *hasResult = false; *hasResultType = false; break;
case OpReorderThreadWithHintNV: *hasResult = false; *hasResultType = false; break;
case OpTypeHitObjectNV: *hasResult = true; *hasResultType = false; break;
case OpImageSampleFootprintNV: *hasResult = true; *hasResultType = true; break;
case OpEmitMeshTasksEXT: *hasResult = false; *hasResultType = false; break;
case OpSetMeshOutputsEXT: *hasResult = false; *hasResultType = false; break;
case OpGroupNonUniformPartitionNV: *hasResult = true; *hasResultType = true; break;
case OpWritePackedPrimitiveIndices4x8NV: *hasResult = false; *hasResultType = false; break;
case OpReportIntersectionNV: *hasResult = true; *hasResultType = true; break;
case OpIgnoreIntersectionNV: *hasResult = false; *hasResultType = false; break;
case OpTerminateRayNV: *hasResult = false; *hasResultType = false; break;
case OpTraceNV: *hasResult = false; *hasResultType = false; break;
case OpTraceMotionNV: *hasResult = false; *hasResultType = false; break;
case OpTraceRayMotionNV: *hasResult = false; *hasResultType = false; break;
case OpRayQueryGetIntersectionTriangleVertexPositionsKHR: *hasResult = true; *hasResultType = true; break;
case OpTypeAccelerationStructureNV: *hasResult = true; *hasResultType = false; break;
case OpExecuteCallableNV: *hasResult = false; *hasResultType = false; break;
case OpTypeCooperativeMatrixNV: *hasResult = true; *hasResultType = false; break;
case OpCooperativeMatrixLoadNV: *hasResult = true; *hasResultType = true; break;
case OpCooperativeMatrixStoreNV: *hasResult = false; *hasResultType = false; break;
case OpCooperativeMatrixMulAddNV: *hasResult = true; *hasResultType = true; break;
case OpCooperativeMatrixLengthNV: *hasResult = true; *hasResultType = true; break;
case OpBeginInvocationInterlockEXT: *hasResult = false; *hasResultType = false; break;
case OpEndInvocationInterlockEXT: *hasResult = false; *hasResultType = false; break;
case OpDemoteToHelperInvocation: *hasResult = false; *hasResultType = false; break;
case OpIsHelperInvocationEXT: *hasResult = true; *hasResultType = true; break;
case OpConvertUToImageNV: *hasResult = true; *hasResultType = true; break;
case OpConvertUToSamplerNV: *hasResult = true; *hasResultType = true; break;
case OpConvertImageToUNV: *hasResult = true; *hasResultType = true; break;
case OpConvertSamplerToUNV: *hasResult = true; *hasResultType = true; break;
case OpConvertUToSampledImageNV: *hasResult = true; *hasResultType = true; break;
case OpConvertSampledImageToUNV: *hasResult = true; *hasResultType = true; break;
case OpSamplerImageAddressingModeNV: *hasResult = false; *hasResultType = false; break;
case OpSubgroupShuffleINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupShuffleDownINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupShuffleUpINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupShuffleXorINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupBlockReadINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupBlockWriteINTEL: *hasResult = false; *hasResultType = false; break;
case OpSubgroupImageBlockReadINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupImageBlockWriteINTEL: *hasResult = false; *hasResultType = false; break;
case OpSubgroupImageMediaBlockReadINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupImageMediaBlockWriteINTEL: *hasResult = false; *hasResultType = false; break;
case OpUCountLeadingZerosINTEL: *hasResult = true; *hasResultType = true; break;
case OpUCountTrailingZerosINTEL: *hasResult = true; *hasResultType = true; break;
case OpAbsISubINTEL: *hasResult = true; *hasResultType = true; break;
case OpAbsUSubINTEL: *hasResult = true; *hasResultType = true; break;
case OpIAddSatINTEL: *hasResult = true; *hasResultType = true; break;
case OpUAddSatINTEL: *hasResult = true; *hasResultType = true; break;
case OpIAverageINTEL: *hasResult = true; *hasResultType = true; break;
case OpUAverageINTEL: *hasResult = true; *hasResultType = true; break;
case OpIAverageRoundedINTEL: *hasResult = true; *hasResultType = true; break;
case OpUAverageRoundedINTEL: *hasResult = true; *hasResultType = true; break;
case OpISubSatINTEL: *hasResult = true; *hasResultType = true; break;
case OpUSubSatINTEL: *hasResult = true; *hasResultType = true; break;
case OpIMul32x16INTEL: *hasResult = true; *hasResultType = true; break;
case OpUMul32x16INTEL: *hasResult = true; *hasResultType = true; break;
case OpConstantFunctionPointerINTEL: *hasResult = true; *hasResultType = true; break;
case OpFunctionPointerCallINTEL: *hasResult = true; *hasResultType = true; break;
case OpAsmTargetINTEL: *hasResult = true; *hasResultType = true; break;
case OpAsmINTEL: *hasResult = true; *hasResultType = true; break;
case OpAsmCallINTEL: *hasResult = true; *hasResultType = true; break;
case OpAtomicFMinEXT: *hasResult = true; *hasResultType = true; break;
case OpAtomicFMaxEXT: *hasResult = true; *hasResultType = true; break;
case OpAssumeTrueKHR: *hasResult = false; *hasResultType = false; break;
case OpExpectKHR: *hasResult = true; *hasResultType = true; break;
case OpDecorateString: *hasResult = false; *hasResultType = false; break;
case OpMemberDecorateString: *hasResult = false; *hasResultType = false; break;
case OpVmeImageINTEL: *hasResult = true; *hasResultType = true; break;
case OpTypeVmeImageINTEL: *hasResult = true; *hasResultType = false; break;
case OpTypeAvcImePayloadINTEL: *hasResult = true; *hasResultType = false; break;
case OpTypeAvcRefPayloadINTEL: *hasResult = true; *hasResultType = false; break;
case OpTypeAvcSicPayloadINTEL: *hasResult = true; *hasResultType = false; break;
case OpTypeAvcMcePayloadINTEL: *hasResult = true; *hasResultType = false; break;
case OpTypeAvcMceResultINTEL: *hasResult = true; *hasResultType = false; break;
case OpTypeAvcImeResultINTEL: *hasResult = true; *hasResultType = false; break;
case OpTypeAvcImeResultSingleReferenceStreamoutINTEL: *hasResult = true; *hasResultType = false; break;
case OpTypeAvcImeResultDualReferenceStreamoutINTEL: *hasResult = true; *hasResultType = false; break;
case OpTypeAvcImeSingleReferenceStreaminINTEL: *hasResult = true; *hasResultType = false; break;
case OpTypeAvcImeDualReferenceStreaminINTEL: *hasResult = true; *hasResultType = false; break;
case OpTypeAvcRefResultINTEL: *hasResult = true; *hasResultType = false; break;
case OpTypeAvcSicResultINTEL: *hasResult = true; *hasResultType = false; break;
case OpSubgroupAvcMceGetDefaultInterBaseMultiReferencePenaltyINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceSetInterBaseMultiReferencePenaltyINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetDefaultInterShapePenaltyINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceSetInterShapePenaltyINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetDefaultInterDirectionPenaltyINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceSetInterDirectionPenaltyINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetDefaultIntraLumaShapePenaltyINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetDefaultInterMotionVectorCostTableINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetDefaultHighPenaltyCostTableINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetDefaultMediumPenaltyCostTableINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetDefaultLowPenaltyCostTableINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceSetMotionVectorCostFunctionINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetDefaultIntraLumaModePenaltyINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetDefaultNonDcLumaIntraPenaltyINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetDefaultIntraChromaModeBasePenaltyINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceSetAcOnlyHaarINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceSetSourceInterlacedFieldPolarityINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceSetSingleReferenceInterlacedFieldPolarityINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceSetDualReferenceInterlacedFieldPolaritiesINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceConvertToImePayloadINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceConvertToImeResultINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceConvertToRefPayloadINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceConvertToRefResultINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceConvertToSicPayloadINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceConvertToSicResultINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetMotionVectorsINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetInterDistortionsINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetBestInterDistortionsINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetInterMajorShapeINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetInterMinorShapeINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetInterDirectionsINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetInterMotionVectorCountINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetInterReferenceIdsINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcMceGetInterReferenceInterlacedFieldPolaritiesINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeInitializeINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeSetSingleReferenceINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeSetDualReferenceINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeRefWindowSizeINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeAdjustRefOffsetINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeConvertToMcePayloadINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeSetMaxMotionVectorCountINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeSetUnidirectionalMixDisableINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeSetEarlySearchTerminationThresholdINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeSetWeightedSadINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeEvaluateWithSingleReferenceINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeEvaluateWithDualReferenceINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeEvaluateWithSingleReferenceStreaminINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeEvaluateWithDualReferenceStreaminINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeEvaluateWithSingleReferenceStreamoutINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeEvaluateWithDualReferenceStreamoutINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeEvaluateWithSingleReferenceStreaminoutINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeEvaluateWithDualReferenceStreaminoutINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeConvertToMceResultINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeGetSingleReferenceStreaminINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeGetDualReferenceStreaminINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeStripSingleReferenceStreamoutINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeStripDualReferenceStreamoutINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeGetStreamoutSingleReferenceMajorShapeMotionVectorsINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeGetStreamoutSingleReferenceMajorShapeDistortionsINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeGetStreamoutSingleReferenceMajorShapeReferenceIdsINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeGetStreamoutDualReferenceMajorShapeMotionVectorsINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeGetStreamoutDualReferenceMajorShapeDistortionsINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeGetStreamoutDualReferenceMajorShapeReferenceIdsINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeGetBorderReachedINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeGetTruncatedSearchIndicationINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeGetUnidirectionalEarlySearchTerminationINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeGetWeightingPatternMinimumMotionVectorINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcImeGetWeightingPatternMinimumDistortionINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcFmeInitializeINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcBmeInitializeINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcRefConvertToMcePayloadINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcRefSetBidirectionalMixDisableINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcRefSetBilinearFilterEnableINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcRefEvaluateWithSingleReferenceINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcRefEvaluateWithDualReferenceINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcRefEvaluateWithMultiReferenceINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcRefEvaluateWithMultiReferenceInterlacedINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcRefConvertToMceResultINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicInitializeINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicConfigureSkcINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicConfigureIpeLumaINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicConfigureIpeLumaChromaINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicGetMotionVectorMaskINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicConvertToMcePayloadINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicSetIntraLumaShapePenaltyINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicSetIntraLumaModeCostFunctionINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicSetIntraChromaModeCostFunctionINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicSetBilinearFilterEnableINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicSetSkcForwardTransformEnableINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicSetBlockBasedRawSkipSadINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicEvaluateIpeINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicEvaluateWithSingleReferenceINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicEvaluateWithDualReferenceINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicEvaluateWithMultiReferenceINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicEvaluateWithMultiReferenceInterlacedINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicConvertToMceResultINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicGetIpeLumaShapeINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicGetBestIpeLumaDistortionINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicGetBestIpeChromaDistortionINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicGetPackedIpeLumaModesINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicGetIpeChromaModeINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicGetPackedSkcLumaCountThresholdINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicGetPackedSkcLumaSumThresholdINTEL: *hasResult = true; *hasResultType = true; break;
case OpSubgroupAvcSicGetInterRawSadsINTEL: *hasResult = true; *hasResultType = true; break;
case OpVariableLengthArrayINTEL: *hasResult = true; *hasResultType = true; break;
case OpSaveMemoryINTEL: *hasResult = true; *hasResultType = true; break;
case OpRestoreMemoryINTEL: *hasResult = false; *hasResultType = false; break;
case OpArbitraryFloatSinCosPiINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatCastINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatCastFromIntINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatCastToIntINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatAddINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatSubINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatMulINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatDivINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatGTINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatGEINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatLTINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatLEINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatEQINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatRecipINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatRSqrtINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatCbrtINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatHypotINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatSqrtINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatLogINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatLog2INTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatLog10INTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatLog1pINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatExpINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatExp2INTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatExp10INTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatExpm1INTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatSinINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatCosINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatSinCosINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatSinPiINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatCosPiINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatASinINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatASinPiINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatACosINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatACosPiINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatATanINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatATanPiINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatATan2INTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatPowINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatPowRINTEL: *hasResult = true; *hasResultType = true; break;
case OpArbitraryFloatPowNINTEL: *hasResult = true; *hasResultType = true; break;
case OpLoopControlINTEL: *hasResult = false; *hasResultType = false; break;
case OpAliasDomainDeclINTEL: *hasResult = true; *hasResultType = false; break;
case OpAliasScopeDeclINTEL: *hasResult = true; *hasResultType = false; break;
case OpAliasScopeListDeclINTEL: *hasResult = true; *hasResultType = false; break;
case OpFixedSqrtINTEL: *hasResult = true; *hasResultType = true; break;
case OpFixedRecipINTEL: *hasResult = true; *hasResultType = true; break;
case OpFixedRsqrtINTEL: *hasResult = true; *hasResultType = true; break;
case OpFixedSinINTEL: *hasResult = true; *hasResultType = true; break;
case OpFixedCosINTEL: *hasResult = true; *hasResultType = true; break;
case OpFixedSinCosINTEL: *hasResult = true; *hasResultType = true; break;
case OpFixedSinPiINTEL: *hasResult = true; *hasResultType = true; break;
case OpFixedCosPiINTEL: *hasResult = true; *hasResultType = true; break;
case OpFixedSinCosPiINTEL: *hasResult = true; *hasResultType = true; break;
case OpFixedLogINTEL: *hasResult = true; *hasResultType = true; break;
case OpFixedExpINTEL: *hasResult = true; *hasResultType = true; break;
case OpPtrCastToCrossWorkgroupINTEL: *hasResult = true; *hasResultType = true; break;
case OpCrossWorkgroupCastToPtrINTEL: *hasResult = true; *hasResultType = true; break;
case OpReadPipeBlockingINTEL: *hasResult = true; *hasResultType = true; break;
case OpWritePipeBlockingINTEL: *hasResult = true; *hasResultType = true; break;
case OpFPGARegINTEL: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetRayTMinKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetRayFlagsKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetIntersectionTKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetIntersectionInstanceCustomIndexKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetIntersectionInstanceIdKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetIntersectionInstanceShaderBindingTableRecordOffsetKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetIntersectionGeometryIndexKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetIntersectionPrimitiveIndexKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetIntersectionBarycentricsKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetIntersectionFrontFaceKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetIntersectionCandidateAABBOpaqueKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetIntersectionObjectRayDirectionKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetIntersectionObjectRayOriginKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetWorldRayDirectionKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetWorldRayOriginKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetIntersectionObjectToWorldKHR: *hasResult = true; *hasResultType = true; break;
case OpRayQueryGetIntersectionWorldToObjectKHR: *hasResult = true; *hasResultType = true; break;
case OpAtomicFAddEXT: *hasResult = true; *hasResultType = true; break;
case OpTypeBufferSurfaceINTEL: *hasResult = true; *hasResultType = false; break;
case OpTypeStructContinuedINTEL: *hasResult = false; *hasResultType = false; break;
case OpConstantCompositeContinuedINTEL: *hasResult = false; *hasResultType = false; break;
case OpSpecConstantCompositeContinuedINTEL: *hasResult = false; *hasResultType = false; break;
case OpControlBarrierArriveINTEL: *hasResult = false; *hasResultType = false; break;
case OpControlBarrierWaitINTEL: *hasResult = false; *hasResultType = false; break;
case OpGroupIMulKHR: *hasResult = true; *hasResultType = true; break;
case OpGroupFMulKHR: *hasResult = true; *hasResultType = true; break;
case OpGroupBitwiseAndKHR: *hasResult = true; *hasResultType = true; break;
case OpGroupBitwiseOrKHR: *hasResult = true; *hasResultType = true; break;
case OpGroupBitwiseXorKHR: *hasResult = true; *hasResultType = true; break;
case OpGroupLogicalAndKHR: *hasResult = true; *hasResultType = true; break;
case OpGroupLogicalOrKHR: *hasResult = true; *hasResultType = true; break;
case OpGroupLogicalXorKHR: *hasResult = true; *hasResultType = true; break;
}
}
#endif /* SPV_ENABLE_UTILITY_CODE */
// Overload bitwise operators for mask bit combining
inline ImageOperandsMask operator|(ImageOperandsMask a, ImageOperandsMask b) { return ImageOperandsMask(unsigned(a) | unsigned(b)); }
inline ImageOperandsMask operator&(ImageOperandsMask a, ImageOperandsMask b) { return ImageOperandsMask(unsigned(a) & unsigned(b)); }
inline ImageOperandsMask operator^(ImageOperandsMask a, ImageOperandsMask b) { return ImageOperandsMask(unsigned(a) ^ unsigned(b)); }
inline ImageOperandsMask operator~(ImageOperandsMask a) { return ImageOperandsMask(~unsigned(a)); }
inline FPFastMathModeMask operator|(FPFastMathModeMask a, FPFastMathModeMask b) { return FPFastMathModeMask(unsigned(a) | unsigned(b)); }
inline FPFastMathModeMask operator&(FPFastMathModeMask a, FPFastMathModeMask b) { return FPFastMathModeMask(unsigned(a) & unsigned(b)); }
inline FPFastMathModeMask operator^(FPFastMathModeMask a, FPFastMathModeMask b) { return FPFastMathModeMask(unsigned(a) ^ unsigned(b)); }
inline FPFastMathModeMask operator~(FPFastMathModeMask a) { return FPFastMathModeMask(~unsigned(a)); }
inline SelectionControlMask operator|(SelectionControlMask a, SelectionControlMask b) { return SelectionControlMask(unsigned(a) | unsigned(b)); }
inline SelectionControlMask operator&(SelectionControlMask a, SelectionControlMask b) { return SelectionControlMask(unsigned(a) & unsigned(b)); }
inline SelectionControlMask operator^(SelectionControlMask a, SelectionControlMask b) { return SelectionControlMask(unsigned(a) ^ unsigned(b)); }
inline SelectionControlMask operator~(SelectionControlMask a) { return SelectionControlMask(~unsigned(a)); }
inline LoopControlMask operator|(LoopControlMask a, LoopControlMask b) { return LoopControlMask(unsigned(a) | unsigned(b)); }
inline LoopControlMask operator&(LoopControlMask a, LoopControlMask b) { return LoopControlMask(unsigned(a) & unsigned(b)); }
inline LoopControlMask operator^(LoopControlMask a, LoopControlMask b) { return LoopControlMask(unsigned(a) ^ unsigned(b)); }
inline LoopControlMask operator~(LoopControlMask a) { return LoopControlMask(~unsigned(a)); }
inline FunctionControlMask operator|(FunctionControlMask a, FunctionControlMask b) { return FunctionControlMask(unsigned(a) | unsigned(b)); }
inline FunctionControlMask operator&(FunctionControlMask a, FunctionControlMask b) { return FunctionControlMask(unsigned(a) & unsigned(b)); }
inline FunctionControlMask operator^(FunctionControlMask a, FunctionControlMask b) { return FunctionControlMask(unsigned(a) ^ unsigned(b)); }
inline FunctionControlMask operator~(FunctionControlMask a) { return FunctionControlMask(~unsigned(a)); }
inline MemorySemanticsMask operator|(MemorySemanticsMask a, MemorySemanticsMask b) { return MemorySemanticsMask(unsigned(a) | unsigned(b)); }
inline MemorySemanticsMask operator&(MemorySemanticsMask a, MemorySemanticsMask b) { return MemorySemanticsMask(unsigned(a) & unsigned(b)); }
inline MemorySemanticsMask operator^(MemorySemanticsMask a, MemorySemanticsMask b) { return MemorySemanticsMask(unsigned(a) ^ unsigned(b)); }
inline MemorySemanticsMask operator~(MemorySemanticsMask a) { return MemorySemanticsMask(~unsigned(a)); }
inline MemoryAccessMask operator|(MemoryAccessMask a, MemoryAccessMask b) { return MemoryAccessMask(unsigned(a) | unsigned(b)); }
inline MemoryAccessMask operator&(MemoryAccessMask a, MemoryAccessMask b) { return MemoryAccessMask(unsigned(a) & unsigned(b)); }
inline MemoryAccessMask operator^(MemoryAccessMask a, MemoryAccessMask b) { return MemoryAccessMask(unsigned(a) ^ unsigned(b)); }
inline MemoryAccessMask operator~(MemoryAccessMask a) { return MemoryAccessMask(~unsigned(a)); }
inline KernelProfilingInfoMask operator|(KernelProfilingInfoMask a, KernelProfilingInfoMask b) { return KernelProfilingInfoMask(unsigned(a) | unsigned(b)); }
inline KernelProfilingInfoMask operator&(KernelProfilingInfoMask a, KernelProfilingInfoMask b) { return KernelProfilingInfoMask(unsigned(a) & unsigned(b)); }
inline KernelProfilingInfoMask operator^(KernelProfilingInfoMask a, KernelProfilingInfoMask b) { return KernelProfilingInfoMask(unsigned(a) ^ unsigned(b)); }
inline KernelProfilingInfoMask operator~(KernelProfilingInfoMask a) { return KernelProfilingInfoMask(~unsigned(a)); }
inline RayFlagsMask operator|(RayFlagsMask a, RayFlagsMask b) { return RayFlagsMask(unsigned(a) | unsigned(b)); }
inline RayFlagsMask operator&(RayFlagsMask a, RayFlagsMask b) { return RayFlagsMask(unsigned(a) & unsigned(b)); }
inline RayFlagsMask operator^(RayFlagsMask a, RayFlagsMask b) { return RayFlagsMask(unsigned(a) ^ unsigned(b)); }
inline RayFlagsMask operator~(RayFlagsMask a) { return RayFlagsMask(~unsigned(a)); }
inline FragmentShadingRateMask operator|(FragmentShadingRateMask a, FragmentShadingRateMask b) { return FragmentShadingRateMask(unsigned(a) | unsigned(b)); }
inline FragmentShadingRateMask operator&(FragmentShadingRateMask a, FragmentShadingRateMask b) { return FragmentShadingRateMask(unsigned(a) & unsigned(b)); }
inline FragmentShadingRateMask operator^(FragmentShadingRateMask a, FragmentShadingRateMask b) { return FragmentShadingRateMask(unsigned(a) ^ unsigned(b)); }
inline FragmentShadingRateMask operator~(FragmentShadingRateMask a) { return FragmentShadingRateMask(~unsigned(a)); }
inline CooperativeMatrixOperandsMask operator|(CooperativeMatrixOperandsMask a, CooperativeMatrixOperandsMask b) { return CooperativeMatrixOperandsMask(unsigned(a) | unsigned(b)); }
inline CooperativeMatrixOperandsMask operator&(CooperativeMatrixOperandsMask a, CooperativeMatrixOperandsMask b) { return CooperativeMatrixOperandsMask(unsigned(a) & unsigned(b)); }
inline CooperativeMatrixOperandsMask operator^(CooperativeMatrixOperandsMask a, CooperativeMatrixOperandsMask b) { return CooperativeMatrixOperandsMask(unsigned(a) ^ unsigned(b)); }
inline CooperativeMatrixOperandsMask operator~(CooperativeMatrixOperandsMask a) { return CooperativeMatrixOperandsMask(~unsigned(a)); }
} // end namespace spv
#endif // #ifndef spirv_HPP
|
0 | repos/glslang.zig/glslang | repos/glslang.zig/glslang/SPIRV/Logger.cpp | //
// Copyright (C) 2016 Google, Inc.
//
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
//
// Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
//
// Redistributions in binary form must reproduce the above
// copyright notice, this list of conditions and the following
// disclaimer in the documentation and/or other materials provided
// with the distribution.
//
// Neither the name of Google Inc. nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
// "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
// LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
// FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
// COPYRIGHT HOLDERS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
// INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
// BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
// LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
// CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
// LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
// ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
// POSSIBILITY OF SUCH DAMAGE.
#include "Logger.h"
#include <algorithm>
#include <iterator>
#include <sstream>
namespace spv {
void SpvBuildLogger::tbdFunctionality(const std::string& f)
{
if (std::find(std::begin(tbdFeatures), std::end(tbdFeatures), f) == std::end(tbdFeatures))
tbdFeatures.push_back(f);
}
void SpvBuildLogger::missingFunctionality(const std::string& f)
{
if (std::find(std::begin(missingFeatures), std::end(missingFeatures), f) == std::end(missingFeatures))
missingFeatures.push_back(f);
}
std::string SpvBuildLogger::getAllMessages() const {
std::ostringstream messages;
for (auto it = tbdFeatures.cbegin(); it != tbdFeatures.cend(); ++it)
messages << "TBD functionality: " << *it << "\n";
for (auto it = missingFeatures.cbegin(); it != missingFeatures.cend(); ++it)
messages << "Missing functionality: " << *it << "\n";
for (auto it = warnings.cbegin(); it != warnings.cend(); ++it)
messages << "warning: " << *it << "\n";
for (auto it = errors.cbegin(); it != errors.cend(); ++it)
messages << "error: " << *it << "\n";
return messages.str();
}
} // end spv namespace
|
0 | repos/glslang.zig/glslang | repos/glslang.zig/glslang/SPIRV/disassemble.h | //
// Copyright (C) 2014-2015 LunarG, Inc.
//
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
//
// Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
//
// Redistributions in binary form must reproduce the above
// copyright notice, this list of conditions and the following
// disclaimer in the documentation and/or other materials provided
// with the distribution.
//
// Neither the name of 3Dlabs Inc. Ltd. nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
// "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
// LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
// FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
// COPYRIGHT HOLDERS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
// INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
// BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
// LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
// CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
// LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
// ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
// POSSIBILITY OF SUCH DAMAGE.
//
// Disassembler for SPIR-V.
//
#pragma once
#ifndef disassembler_H
#define disassembler_H
#include <iostream>
#include <vector>
namespace spv {
// disassemble with glslang custom disassembler
void Disassemble(std::ostream& out, const std::vector<unsigned int>&);
} // end namespace spv
#endif // disassembler_H
|
0 | repos/glslang.zig/glslang | repos/glslang.zig/glslang/SPIRV/SpvBuilder.cpp | //
// Copyright (C) 2014-2015 LunarG, Inc.
// Copyright (C) 2015-2018 Google, Inc.
// Modifications Copyright (C) 2020 Advanced Micro Devices, Inc. All rights reserved.
//
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
//
// Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
//
// Redistributions in binary form must reproduce the above
// copyright notice, this list of conditions and the following
// disclaimer in the documentation and/or other materials provided
// with the distribution.
//
// Neither the name of 3Dlabs Inc. Ltd. nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
// "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
// LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
// FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
// COPYRIGHT HOLDERS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
// INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
// BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
// LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
// CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
// LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
// ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
// POSSIBILITY OF SUCH DAMAGE.
//
// Helper for making SPIR-V IR. Generally, this is documented in the header
// SpvBuilder.h.
//
#include <cassert>
#include <cstdlib>
#include <unordered_set>
#include <algorithm>
#include "SpvBuilder.h"
#include "hex_float.h"
#ifndef _WIN32
#include <cstdio>
#endif
namespace spv {
Builder::Builder(unsigned int spvVersion, unsigned int magicNumber, SpvBuildLogger* buildLogger) :
spvVersion(spvVersion),
sourceLang(SourceLanguageUnknown),
sourceVersion(0),
addressModel(AddressingModelLogical),
memoryModel(MemoryModelGLSL450),
builderNumber(magicNumber),
buildPoint(nullptr),
uniqueId(0),
entryPointFunction(nullptr),
generatingOpCodeForSpecConst(false),
logger(buildLogger)
{
clearAccessChain();
}
Builder::~Builder()
{
}
Id Builder::import(const char* name)
{
Instruction* import = new Instruction(getUniqueId(), NoType, OpExtInstImport);
import->addStringOperand(name);
module.mapInstruction(import);
imports.push_back(std::unique_ptr<Instruction>(import));
return import->getResultId();
}
// For creating new groupedTypes (will return old type if the requested one was already made).
Id Builder::makeVoidType()
{
Instruction* type;
if (groupedTypes[OpTypeVoid].size() == 0) {
Id typeId = getUniqueId();
type = new Instruction(typeId, NoType, OpTypeVoid);
groupedTypes[OpTypeVoid].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
// Core OpTypeVoid used for debug void type
if (emitNonSemanticShaderDebugInfo)
debugId[typeId] = typeId;
} else
type = groupedTypes[OpTypeVoid].back();
return type->getResultId();
}
Id Builder::makeBoolType()
{
Instruction* type;
if (groupedTypes[OpTypeBool].size() == 0) {
type = new Instruction(getUniqueId(), NoType, OpTypeBool);
groupedTypes[OpTypeBool].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
if (emitNonSemanticShaderDebugInfo) {
auto const debugResultId = makeBoolDebugType(32);
debugId[type->getResultId()] = debugResultId;
}
} else
type = groupedTypes[OpTypeBool].back();
return type->getResultId();
}
Id Builder::makeSamplerType()
{
Instruction* type;
if (groupedTypes[OpTypeSampler].size() == 0) {
type = new Instruction(getUniqueId(), NoType, OpTypeSampler);
groupedTypes[OpTypeSampler].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
} else
type = groupedTypes[OpTypeSampler].back();
if (emitNonSemanticShaderDebugInfo)
{
auto const debugResultId = makeCompositeDebugType({}, "type.sampler", NonSemanticShaderDebugInfo100Structure, true);
debugId[type->getResultId()] = debugResultId;
}
return type->getResultId();
}
Id Builder::makePointer(StorageClass storageClass, Id pointee)
{
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedTypes[OpTypePointer].size(); ++t) {
type = groupedTypes[OpTypePointer][t];
if (type->getImmediateOperand(0) == (unsigned)storageClass &&
type->getIdOperand(1) == pointee)
return type->getResultId();
}
// not found, make it
type = new Instruction(getUniqueId(), NoType, OpTypePointer);
type->reserveOperands(2);
type->addImmediateOperand(storageClass);
type->addIdOperand(pointee);
groupedTypes[OpTypePointer].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
if (emitNonSemanticShaderDebugInfo) {
const Id debugResultId = makePointerDebugType(storageClass, pointee);
debugId[type->getResultId()] = debugResultId;
}
return type->getResultId();
}
Id Builder::makeForwardPointer(StorageClass storageClass)
{
// Caching/uniquifying doesn't work here, because we don't know the
// pointee type and there can be multiple forward pointers of the same
// storage type. Somebody higher up in the stack must keep track.
Instruction* type = new Instruction(getUniqueId(), NoType, OpTypeForwardPointer);
type->addImmediateOperand(storageClass);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
if (emitNonSemanticShaderDebugInfo) {
const Id debugResultId = makeForwardPointerDebugType(storageClass);
debugId[type->getResultId()] = debugResultId;
}
return type->getResultId();
}
Id Builder::makePointerFromForwardPointer(StorageClass storageClass, Id forwardPointerType, Id pointee)
{
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedTypes[OpTypePointer].size(); ++t) {
type = groupedTypes[OpTypePointer][t];
if (type->getImmediateOperand(0) == (unsigned)storageClass &&
type->getIdOperand(1) == pointee)
return type->getResultId();
}
type = new Instruction(forwardPointerType, NoType, OpTypePointer);
type->reserveOperands(2);
type->addImmediateOperand(storageClass);
type->addIdOperand(pointee);
groupedTypes[OpTypePointer].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
// If we are emitting nonsemantic debuginfo, we need to patch the debug pointer type
// that was emitted alongside the forward pointer, now that we have a pointee debug
// type for it to point to.
if (emitNonSemanticShaderDebugInfo) {
Instruction *debugForwardPointer = module.getInstruction(debugId[forwardPointerType]);
assert(debugId[pointee]);
debugForwardPointer->setIdOperand(2, debugId[pointee]);
}
return type->getResultId();
}
Id Builder::makeIntegerType(int width, bool hasSign)
{
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedTypes[OpTypeInt].size(); ++t) {
type = groupedTypes[OpTypeInt][t];
if (type->getImmediateOperand(0) == (unsigned)width &&
type->getImmediateOperand(1) == (hasSign ? 1u : 0u))
return type->getResultId();
}
// not found, make it
type = new Instruction(getUniqueId(), NoType, OpTypeInt);
type->reserveOperands(2);
type->addImmediateOperand(width);
type->addImmediateOperand(hasSign ? 1 : 0);
groupedTypes[OpTypeInt].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
// deal with capabilities
switch (width) {
case 8:
case 16:
// these are currently handled by storage-type declarations and post processing
break;
case 64:
addCapability(CapabilityInt64);
break;
default:
break;
}
if (emitNonSemanticShaderDebugInfo)
{
auto const debugResultId = makeIntegerDebugType(width, hasSign);
debugId[type->getResultId()] = debugResultId;
}
return type->getResultId();
}
Id Builder::makeFloatType(int width)
{
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedTypes[OpTypeFloat].size(); ++t) {
type = groupedTypes[OpTypeFloat][t];
if (type->getImmediateOperand(0) == (unsigned)width)
return type->getResultId();
}
// not found, make it
type = new Instruction(getUniqueId(), NoType, OpTypeFloat);
type->addImmediateOperand(width);
groupedTypes[OpTypeFloat].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
// deal with capabilities
switch (width) {
case 16:
// currently handled by storage-type declarations and post processing
break;
case 64:
addCapability(CapabilityFloat64);
break;
default:
break;
}
if (emitNonSemanticShaderDebugInfo)
{
auto const debugResultId = makeFloatDebugType(width);
debugId[type->getResultId()] = debugResultId;
}
return type->getResultId();
}
// Make a struct without checking for duplication.
// See makeStructResultType() for non-decorated structs
// needed as the result of some instructions, which does
// check for duplicates.
Id Builder::makeStructType(const std::vector<Id>& members, const char* name, bool const compilerGenerated)
{
// Don't look for previous one, because in the general case,
// structs can be duplicated except for decorations.
// not found, make it
Instruction* type = new Instruction(getUniqueId(), NoType, OpTypeStruct);
for (int op = 0; op < (int)members.size(); ++op)
type->addIdOperand(members[op]);
groupedTypes[OpTypeStruct].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
addName(type->getResultId(), name);
if (emitNonSemanticShaderDebugInfo && !compilerGenerated)
{
auto const debugResultId = makeCompositeDebugType(members, name, NonSemanticShaderDebugInfo100Structure);
debugId[type->getResultId()] = debugResultId;
}
return type->getResultId();
}
// Make a struct for the simple results of several instructions,
// checking for duplication.
Id Builder::makeStructResultType(Id type0, Id type1)
{
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedTypes[OpTypeStruct].size(); ++t) {
type = groupedTypes[OpTypeStruct][t];
if (type->getNumOperands() != 2)
continue;
if (type->getIdOperand(0) != type0 ||
type->getIdOperand(1) != type1)
continue;
return type->getResultId();
}
// not found, make it
std::vector<spv::Id> members;
members.push_back(type0);
members.push_back(type1);
return makeStructType(members, "ResType");
}
Id Builder::makeVectorType(Id component, int size)
{
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedTypes[OpTypeVector].size(); ++t) {
type = groupedTypes[OpTypeVector][t];
if (type->getIdOperand(0) == component &&
type->getImmediateOperand(1) == (unsigned)size)
return type->getResultId();
}
// not found, make it
type = new Instruction(getUniqueId(), NoType, OpTypeVector);
type->reserveOperands(2);
type->addIdOperand(component);
type->addImmediateOperand(size);
groupedTypes[OpTypeVector].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
if (emitNonSemanticShaderDebugInfo)
{
auto const debugResultId = makeVectorDebugType(component, size);
debugId[type->getResultId()] = debugResultId;
}
return type->getResultId();
}
Id Builder::makeMatrixType(Id component, int cols, int rows)
{
assert(cols <= maxMatrixSize && rows <= maxMatrixSize);
Id column = makeVectorType(component, rows);
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedTypes[OpTypeMatrix].size(); ++t) {
type = groupedTypes[OpTypeMatrix][t];
if (type->getIdOperand(0) == column &&
type->getImmediateOperand(1) == (unsigned)cols)
return type->getResultId();
}
// not found, make it
type = new Instruction(getUniqueId(), NoType, OpTypeMatrix);
type->reserveOperands(2);
type->addIdOperand(column);
type->addImmediateOperand(cols);
groupedTypes[OpTypeMatrix].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
if (emitNonSemanticShaderDebugInfo)
{
auto const debugResultId = makeMatrixDebugType(column, cols);
debugId[type->getResultId()] = debugResultId;
}
return type->getResultId();
}
Id Builder::makeCooperativeMatrixTypeKHR(Id component, Id scope, Id rows, Id cols, Id use)
{
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedTypes[OpTypeCooperativeMatrixKHR].size(); ++t) {
type = groupedTypes[OpTypeCooperativeMatrixKHR][t];
if (type->getIdOperand(0) == component &&
type->getIdOperand(1) == scope &&
type->getIdOperand(2) == rows &&
type->getIdOperand(3) == cols &&
type->getIdOperand(4) == use)
return type->getResultId();
}
// not found, make it
type = new Instruction(getUniqueId(), NoType, OpTypeCooperativeMatrixKHR);
type->reserveOperands(5);
type->addIdOperand(component);
type->addIdOperand(scope);
type->addIdOperand(rows);
type->addIdOperand(cols);
type->addIdOperand(use);
groupedTypes[OpTypeCooperativeMatrixKHR].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
return type->getResultId();
}
Id Builder::makeCooperativeMatrixTypeNV(Id component, Id scope, Id rows, Id cols)
{
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedTypes[OpTypeCooperativeMatrixNV].size(); ++t) {
type = groupedTypes[OpTypeCooperativeMatrixNV][t];
if (type->getIdOperand(0) == component && type->getIdOperand(1) == scope && type->getIdOperand(2) == rows &&
type->getIdOperand(3) == cols)
return type->getResultId();
}
// not found, make it
type = new Instruction(getUniqueId(), NoType, OpTypeCooperativeMatrixNV);
type->reserveOperands(4);
type->addIdOperand(component);
type->addIdOperand(scope);
type->addIdOperand(rows);
type->addIdOperand(cols);
groupedTypes[OpTypeCooperativeMatrixNV].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
return type->getResultId();
}
Id Builder::makeCooperativeMatrixTypeWithSameShape(Id component, Id otherType)
{
Instruction* instr = module.getInstruction(otherType);
if (instr->getOpCode() == OpTypeCooperativeMatrixNV) {
return makeCooperativeMatrixTypeNV(component, instr->getIdOperand(1), instr->getIdOperand(2), instr->getIdOperand(3));
} else {
assert(instr->getOpCode() == OpTypeCooperativeMatrixKHR);
return makeCooperativeMatrixTypeKHR(component, instr->getIdOperand(1), instr->getIdOperand(2), instr->getIdOperand(3), instr->getIdOperand(4));
}
}
Id Builder::makeGenericType(spv::Op opcode, std::vector<spv::IdImmediate>& operands)
{
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedTypes[opcode].size(); ++t) {
type = groupedTypes[opcode][t];
if (static_cast<size_t>(type->getNumOperands()) != operands.size())
continue; // Number mismatch, find next
bool match = true;
for (int op = 0; match && op < (int)operands.size(); ++op) {
match = (operands[op].isId ? type->getIdOperand(op) : type->getImmediateOperand(op)) == operands[op].word;
}
if (match)
return type->getResultId();
}
// not found, make it
type = new Instruction(getUniqueId(), NoType, opcode);
type->reserveOperands(operands.size());
for (size_t op = 0; op < operands.size(); ++op) {
if (operands[op].isId)
type->addIdOperand(operands[op].word);
else
type->addImmediateOperand(operands[op].word);
}
groupedTypes[opcode].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
return type->getResultId();
}
// TODO: performance: track arrays per stride
// If a stride is supplied (non-zero) make an array.
// If no stride (0), reuse previous array types.
// 'size' is an Id of a constant or specialization constant of the array size
Id Builder::makeArrayType(Id element, Id sizeId, int stride)
{
Instruction* type;
if (stride == 0) {
// try to find existing type
for (int t = 0; t < (int)groupedTypes[OpTypeArray].size(); ++t) {
type = groupedTypes[OpTypeArray][t];
if (type->getIdOperand(0) == element &&
type->getIdOperand(1) == sizeId)
return type->getResultId();
}
}
// not found, make it
type = new Instruction(getUniqueId(), NoType, OpTypeArray);
type->reserveOperands(2);
type->addIdOperand(element);
type->addIdOperand(sizeId);
groupedTypes[OpTypeArray].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
if (emitNonSemanticShaderDebugInfo)
{
auto const debugResultId = makeArrayDebugType(element, sizeId);
debugId[type->getResultId()] = debugResultId;
}
return type->getResultId();
}
Id Builder::makeRuntimeArray(Id element)
{
Instruction* type = new Instruction(getUniqueId(), NoType, OpTypeRuntimeArray);
type->addIdOperand(element);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
if (emitNonSemanticShaderDebugInfo)
{
auto const debugResultId = makeArrayDebugType(element, makeUintConstant(0));
debugId[type->getResultId()] = debugResultId;
}
return type->getResultId();
}
Id Builder::makeFunctionType(Id returnType, const std::vector<Id>& paramTypes)
{
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedTypes[OpTypeFunction].size(); ++t) {
type = groupedTypes[OpTypeFunction][t];
if (type->getIdOperand(0) != returnType || (int)paramTypes.size() != type->getNumOperands() - 1)
continue;
bool mismatch = false;
for (int p = 0; p < (int)paramTypes.size(); ++p) {
if (paramTypes[p] != type->getIdOperand(p + 1)) {
mismatch = true;
break;
}
}
if (! mismatch)
{
// If compiling HLSL, glslang will create a wrapper function around the entrypoint. Accordingly, a void(void)
// function type is created for the wrapper function. However, nonsemantic shader debug information is disabled
// while creating the HLSL wrapper. Consequently, if we encounter another void(void) function, we need to create
// the associated debug function type if it hasn't been created yet.
if(emitNonSemanticShaderDebugInfo && debugId[type->getResultId()] == 0) {
assert(sourceLang == spv::SourceLanguageHLSL);
assert(getTypeClass(returnType) == OpTypeVoid && paramTypes.size() == 0);
Id debugTypeId = makeDebugFunctionType(returnType, {});
debugId[type->getResultId()] = debugTypeId;
}
return type->getResultId();
}
}
// not found, make it
Id typeId = getUniqueId();
type = new Instruction(typeId, NoType, OpTypeFunction);
type->reserveOperands(paramTypes.size() + 1);
type->addIdOperand(returnType);
for (int p = 0; p < (int)paramTypes.size(); ++p)
type->addIdOperand(paramTypes[p]);
groupedTypes[OpTypeFunction].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
// make debug type and map it
if (emitNonSemanticShaderDebugInfo) {
Id debugTypeId = makeDebugFunctionType(returnType, paramTypes);
debugId[typeId] = debugTypeId;
}
return type->getResultId();
}
Id Builder::makeDebugFunctionType(Id returnType, const std::vector<Id>& paramTypes)
{
assert(debugId[returnType] != 0);
Id typeId = getUniqueId();
auto type = new Instruction(typeId, makeVoidType(), OpExtInst);
type->reserveOperands(paramTypes.size() + 4);
type->addIdOperand(nonSemanticShaderDebugInfo);
type->addImmediateOperand(NonSemanticShaderDebugInfo100DebugTypeFunction);
type->addIdOperand(makeUintConstant(NonSemanticShaderDebugInfo100FlagIsPublic));
type->addIdOperand(debugId[returnType]);
for (auto const paramType : paramTypes) {
if (isPointerType(paramType) || isArrayType(paramType)) {
type->addIdOperand(debugId[getContainedTypeId(paramType)]);
}
else {
type->addIdOperand(debugId[paramType]);
}
}
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
return typeId;
}
Id Builder::makeImageType(Id sampledType, Dim dim, bool depth, bool arrayed, bool ms, unsigned sampled,
ImageFormat format)
{
assert(sampled == 1 || sampled == 2);
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedTypes[OpTypeImage].size(); ++t) {
type = groupedTypes[OpTypeImage][t];
if (type->getIdOperand(0) == sampledType &&
type->getImmediateOperand(1) == (unsigned int)dim &&
type->getImmediateOperand(2) == ( depth ? 1u : 0u) &&
type->getImmediateOperand(3) == (arrayed ? 1u : 0u) &&
type->getImmediateOperand(4) == ( ms ? 1u : 0u) &&
type->getImmediateOperand(5) == sampled &&
type->getImmediateOperand(6) == (unsigned int)format)
return type->getResultId();
}
// not found, make it
type = new Instruction(getUniqueId(), NoType, OpTypeImage);
type->reserveOperands(7);
type->addIdOperand(sampledType);
type->addImmediateOperand( dim);
type->addImmediateOperand( depth ? 1 : 0);
type->addImmediateOperand(arrayed ? 1 : 0);
type->addImmediateOperand( ms ? 1 : 0);
type->addImmediateOperand(sampled);
type->addImmediateOperand((unsigned int)format);
groupedTypes[OpTypeImage].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
// deal with capabilities
switch (dim) {
case DimBuffer:
if (sampled == 1)
addCapability(CapabilitySampledBuffer);
else
addCapability(CapabilityImageBuffer);
break;
case Dim1D:
if (sampled == 1)
addCapability(CapabilitySampled1D);
else
addCapability(CapabilityImage1D);
break;
case DimCube:
if (arrayed) {
if (sampled == 1)
addCapability(CapabilitySampledCubeArray);
else
addCapability(CapabilityImageCubeArray);
}
break;
case DimRect:
if (sampled == 1)
addCapability(CapabilitySampledRect);
else
addCapability(CapabilityImageRect);
break;
case DimSubpassData:
addCapability(CapabilityInputAttachment);
break;
default:
break;
}
if (ms) {
if (sampled == 2) {
// Images used with subpass data are not storage
// images, so don't require the capability for them.
if (dim != Dim::DimSubpassData)
addCapability(CapabilityStorageImageMultisample);
if (arrayed)
addCapability(CapabilityImageMSArray);
}
}
if (emitNonSemanticShaderDebugInfo)
{
auto TypeName = [&dim]() -> char const* {
switch (dim) {
case Dim1D: return "type.1d.image";
case Dim2D: return "type.2d.image";
case Dim3D: return "type.3d.image";
case DimCube: return "type.cube.image";
default: return "type.image";
}
};
auto const debugResultId = makeCompositeDebugType({}, TypeName(), NonSemanticShaderDebugInfo100Class, true);
debugId[type->getResultId()] = debugResultId;
}
return type->getResultId();
}
Id Builder::makeSampledImageType(Id imageType)
{
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedTypes[OpTypeSampledImage].size(); ++t) {
type = groupedTypes[OpTypeSampledImage][t];
if (type->getIdOperand(0) == imageType)
return type->getResultId();
}
// not found, make it
type = new Instruction(getUniqueId(), NoType, OpTypeSampledImage);
type->addIdOperand(imageType);
groupedTypes[OpTypeSampledImage].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
if (emitNonSemanticShaderDebugInfo)
{
auto const debugResultId = makeCompositeDebugType({}, "type.sampled.image", NonSemanticShaderDebugInfo100Class, true);
debugId[type->getResultId()] = debugResultId;
}
return type->getResultId();
}
Id Builder::makeDebugInfoNone()
{
if (debugInfoNone != 0)
return debugInfoNone;
Instruction* inst = new Instruction(getUniqueId(), makeVoidType(), OpExtInst);
inst->reserveOperands(2);
inst->addIdOperand(nonSemanticShaderDebugInfo);
inst->addImmediateOperand(NonSemanticShaderDebugInfo100DebugInfoNone);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(inst));
module.mapInstruction(inst);
debugInfoNone = inst->getResultId();
return debugInfoNone;
}
Id Builder::makeBoolDebugType(int const size)
{
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypeBasic].size(); ++t) {
type = groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypeBasic][t];
if (type->getIdOperand(0) == getStringId("bool") &&
type->getIdOperand(1) == static_cast<unsigned int>(size) &&
type->getIdOperand(2) == NonSemanticShaderDebugInfo100Boolean)
return type->getResultId();
}
type = new Instruction(getUniqueId(), makeVoidType(), OpExtInst);
type->reserveOperands(6);
type->addIdOperand(nonSemanticShaderDebugInfo);
type->addImmediateOperand(NonSemanticShaderDebugInfo100DebugTypeBasic);
type->addIdOperand(getStringId("bool")); // name id
type->addIdOperand(makeUintConstant(size)); // size id
type->addIdOperand(makeUintConstant(NonSemanticShaderDebugInfo100Boolean)); // encoding id
type->addIdOperand(makeUintConstant(NonSemanticShaderDebugInfo100None)); // flags id
groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypeBasic].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
return type->getResultId();
}
Id Builder::makeIntegerDebugType(int const width, bool const hasSign)
{
const char* typeName = nullptr;
switch (width) {
case 8: typeName = hasSign ? "int8_t" : "uint8_t"; break;
case 16: typeName = hasSign ? "int16_t" : "uint16_t"; break;
case 64: typeName = hasSign ? "int64_t" : "uint64_t"; break;
default: typeName = hasSign ? "int" : "uint";
}
auto nameId = getStringId(typeName);
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypeBasic].size(); ++t) {
type = groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypeBasic][t];
if (type->getIdOperand(0) == nameId &&
type->getIdOperand(1) == static_cast<unsigned int>(width) &&
type->getIdOperand(2) == (hasSign ? NonSemanticShaderDebugInfo100Signed : NonSemanticShaderDebugInfo100Unsigned))
return type->getResultId();
}
// not found, make it
type = new Instruction(getUniqueId(), makeVoidType(), OpExtInst);
type->reserveOperands(6);
type->addIdOperand(nonSemanticShaderDebugInfo);
type->addImmediateOperand(NonSemanticShaderDebugInfo100DebugTypeBasic);
type->addIdOperand(nameId); // name id
type->addIdOperand(makeUintConstant(width)); // size id
if(hasSign == true) {
type->addIdOperand(makeUintConstant(NonSemanticShaderDebugInfo100Signed)); // encoding id
} else {
type->addIdOperand(makeUintConstant(NonSemanticShaderDebugInfo100Unsigned)); // encoding id
}
type->addIdOperand(makeUintConstant(NonSemanticShaderDebugInfo100None)); // flags id
groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypeBasic].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
return type->getResultId();
}
Id Builder::makeFloatDebugType(int const width)
{
const char* typeName = nullptr;
switch (width) {
case 16: typeName = "float16_t"; break;
case 64: typeName = "double"; break;
default: typeName = "float"; break;
}
auto nameId = getStringId(typeName);
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypeBasic].size(); ++t) {
type = groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypeBasic][t];
if (type->getIdOperand(0) == nameId &&
type->getIdOperand(1) == static_cast<unsigned int>(width) &&
type->getIdOperand(2) == NonSemanticShaderDebugInfo100Float)
return type->getResultId();
}
// not found, make it
type = new Instruction(getUniqueId(), makeVoidType(), OpExtInst);
type->reserveOperands(6);
type->addIdOperand(nonSemanticShaderDebugInfo);
type->addImmediateOperand(NonSemanticShaderDebugInfo100DebugTypeBasic);
type->addIdOperand(nameId); // name id
type->addIdOperand(makeUintConstant(width)); // size id
type->addIdOperand(makeUintConstant(NonSemanticShaderDebugInfo100Float)); // encoding id
type->addIdOperand(makeUintConstant(NonSemanticShaderDebugInfo100None)); // flags id
groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypeBasic].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
return type->getResultId();
}
Id Builder::makeSequentialDebugType(Id const baseType, Id const componentCount, NonSemanticShaderDebugInfo100Instructions const sequenceType)
{
assert(sequenceType == NonSemanticShaderDebugInfo100DebugTypeArray ||
sequenceType == NonSemanticShaderDebugInfo100DebugTypeVector);
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedDebugTypes[sequenceType].size(); ++t) {
type = groupedDebugTypes[sequenceType][t];
if (type->getIdOperand(0) == baseType &&
type->getIdOperand(1) == makeUintConstant(componentCount))
return type->getResultId();
}
// not found, make it
type = new Instruction(getUniqueId(), makeVoidType(), OpExtInst);
type->reserveOperands(4);
type->addIdOperand(nonSemanticShaderDebugInfo);
type->addImmediateOperand(sequenceType);
type->addIdOperand(debugId[baseType]); // base type
type->addIdOperand(componentCount); // component count
groupedDebugTypes[sequenceType].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
return type->getResultId();
}
Id Builder::makeArrayDebugType(Id const baseType, Id const componentCount)
{
return makeSequentialDebugType(baseType, componentCount, NonSemanticShaderDebugInfo100DebugTypeArray);
}
Id Builder::makeVectorDebugType(Id const baseType, int const componentCount)
{
return makeSequentialDebugType(baseType, makeUintConstant(componentCount), NonSemanticShaderDebugInfo100DebugTypeVector);
}
Id Builder::makeMatrixDebugType(Id const vectorType, int const vectorCount, bool columnMajor)
{
// try to find it
Instruction* type;
for (int t = 0; t < (int)groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypeMatrix].size(); ++t) {
type = groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypeMatrix][t];
if (type->getIdOperand(0) == vectorType &&
type->getIdOperand(1) == makeUintConstant(vectorCount))
return type->getResultId();
}
// not found, make it
type = new Instruction(getUniqueId(), makeVoidType(), OpExtInst);
type->reserveOperands(5);
type->addIdOperand(nonSemanticShaderDebugInfo);
type->addImmediateOperand(NonSemanticShaderDebugInfo100DebugTypeMatrix);
type->addIdOperand(debugId[vectorType]); // vector type id
type->addIdOperand(makeUintConstant(vectorCount)); // component count id
type->addIdOperand(makeBoolConstant(columnMajor)); // column-major id
groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypeMatrix].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
return type->getResultId();
}
Id Builder::makeMemberDebugType(Id const memberType, DebugTypeLoc const& debugTypeLoc)
{
assert(debugId[memberType] != 0);
Instruction* type = new Instruction(getUniqueId(), makeVoidType(), OpExtInst);
type->reserveOperands(10);
type->addIdOperand(nonSemanticShaderDebugInfo);
type->addImmediateOperand(NonSemanticShaderDebugInfo100DebugTypeMember);
type->addIdOperand(getStringId(debugTypeLoc.name)); // name id
type->addIdOperand(debugId[memberType]); // type id
type->addIdOperand(makeDebugSource(currentFileId)); // source id
type->addIdOperand(makeUintConstant(debugTypeLoc.line)); // line id TODO: currentLine is always zero
type->addIdOperand(makeUintConstant(debugTypeLoc.column)); // TODO: column id
type->addIdOperand(makeUintConstant(0)); // TODO: offset id
type->addIdOperand(makeUintConstant(0)); // TODO: size id
type->addIdOperand(makeUintConstant(NonSemanticShaderDebugInfo100FlagIsPublic)); // flags id
groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypeMember].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
return type->getResultId();
}
// Note: To represent a source language opaque type, this instruction must have no Members operands, Size operand must be
// DebugInfoNone, and Name must start with @ to avoid clashes with user defined names.
Id Builder::makeCompositeDebugType(std::vector<Id> const& memberTypes, char const*const name,
NonSemanticShaderDebugInfo100DebugCompositeType const tag, bool const isOpaqueType)
{
// Create the debug member types.
std::vector<Id> memberDebugTypes;
for(auto const memberType : memberTypes) {
assert(debugTypeLocs.find(memberType) != debugTypeLocs.end());
// There _should_ be debug types for all the member types but currently buffer references
// do not have member debug info generated.
if (debugId[memberType])
memberDebugTypes.emplace_back(makeMemberDebugType(memberType, debugTypeLocs[memberType]));
// TODO: Need to rethink this method of passing location information.
// debugTypeLocs.erase(memberType);
}
// Create The structure debug type.
Instruction* type = new Instruction(getUniqueId(), makeVoidType(), OpExtInst);
type->reserveOperands(memberDebugTypes.size() + 11);
type->addIdOperand(nonSemanticShaderDebugInfo);
type->addImmediateOperand(NonSemanticShaderDebugInfo100DebugTypeComposite);
type->addIdOperand(getStringId(name)); // name id
type->addIdOperand(makeUintConstant(tag)); // tag id
type->addIdOperand(makeDebugSource(currentFileId)); // source id
type->addIdOperand(makeUintConstant(currentLine)); // line id TODO: currentLine always zero?
type->addIdOperand(makeUintConstant(0)); // TODO: column id
type->addIdOperand(makeDebugCompilationUnit()); // scope id
if(isOpaqueType == true) {
// Prepend '@' to opaque types.
type->addIdOperand(getStringId('@' + std::string(name))); // linkage name id
type->addIdOperand(makeDebugInfoNone()); // size id
} else {
type->addIdOperand(getStringId(name)); // linkage name id
type->addIdOperand(makeUintConstant(0)); // TODO: size id
}
type->addIdOperand(makeUintConstant(NonSemanticShaderDebugInfo100FlagIsPublic)); // flags id
assert(isOpaqueType == false || (isOpaqueType == true && memberDebugTypes.empty()));
for(auto const memberDebugType : memberDebugTypes) {
type->addIdOperand(memberDebugType);
}
groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypeComposite].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
return type->getResultId();
}
Id Builder::makePointerDebugType(StorageClass storageClass, Id const baseType)
{
const Id debugBaseType = debugId[baseType];
if (!debugBaseType) {
return makeDebugInfoNone();
}
const Id scID = makeUintConstant(storageClass);
for (Instruction* otherType : groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypePointer]) {
if (otherType->getIdOperand(2) == debugBaseType &&
otherType->getIdOperand(3) == scID) {
return otherType->getResultId();
}
}
Instruction* type = new Instruction(getUniqueId(), makeVoidType(), OpExtInst);
type->reserveOperands(5);
type->addIdOperand(nonSemanticShaderDebugInfo);
type->addImmediateOperand(NonSemanticShaderDebugInfo100DebugTypePointer);
type->addIdOperand(debugBaseType);
type->addIdOperand(scID);
type->addIdOperand(makeUintConstant(0));
groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypePointer].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
return type->getResultId();
}
// Emit a OpExtInstWithForwardRefsKHR nonsemantic instruction for a pointer debug type
// where we don't have the pointee yet. Since we don't have the pointee yet, it just
// points to itself and we rely on patching it later.
Id Builder::makeForwardPointerDebugType(StorageClass storageClass)
{
const Id scID = makeUintConstant(storageClass);
this->addExtension(spv::E_SPV_KHR_relaxed_extended_instruction);
Instruction *type = new Instruction(getUniqueId(), makeVoidType(), OpExtInstWithForwardRefsKHR);
type->addIdOperand(nonSemanticShaderDebugInfo);
type->addImmediateOperand(NonSemanticShaderDebugInfo100DebugTypePointer);
type->addIdOperand(type->getResultId());
type->addIdOperand(scID);
type->addIdOperand(makeUintConstant(0));
groupedDebugTypes[NonSemanticShaderDebugInfo100DebugTypePointer].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
return type->getResultId();
}
Id Builder::makeDebugSource(const Id fileName) {
if (debugSourceId.find(fileName) != debugSourceId.end())
return debugSourceId[fileName];
spv::Id resultId = getUniqueId();
Instruction* sourceInst = new Instruction(resultId, makeVoidType(), OpExtInst);
sourceInst->reserveOperands(3);
sourceInst->addIdOperand(nonSemanticShaderDebugInfo);
sourceInst->addImmediateOperand(NonSemanticShaderDebugInfo100DebugSource);
sourceInst->addIdOperand(fileName);
if (emitNonSemanticShaderDebugSource) {
spv::Id sourceId = 0;
if (fileName == mainFileId) {
sourceId = getStringId(sourceText);
} else {
auto incItr = includeFiles.find(fileName);
if (incItr != includeFiles.end()) {
sourceId = getStringId(*incItr->second);
}
}
// We omit the optional source text item if not available in glslang
if (sourceId != 0) {
sourceInst->addIdOperand(sourceId);
}
}
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(sourceInst));
module.mapInstruction(sourceInst);
debugSourceId[fileName] = resultId;
return resultId;
}
Id Builder::makeDebugCompilationUnit() {
if (nonSemanticShaderCompilationUnitId != 0)
return nonSemanticShaderCompilationUnitId;
spv::Id resultId = getUniqueId();
Instruction* sourceInst = new Instruction(resultId, makeVoidType(), OpExtInst);
sourceInst->reserveOperands(6);
sourceInst->addIdOperand(nonSemanticShaderDebugInfo);
sourceInst->addImmediateOperand(NonSemanticShaderDebugInfo100DebugCompilationUnit);
sourceInst->addIdOperand(makeUintConstant(1)); // TODO(greg-lunarg): Get rid of magic number
sourceInst->addIdOperand(makeUintConstant(4)); // TODO(greg-lunarg): Get rid of magic number
sourceInst->addIdOperand(makeDebugSource(mainFileId));
sourceInst->addIdOperand(makeUintConstant(sourceLang));
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(sourceInst));
module.mapInstruction(sourceInst);
nonSemanticShaderCompilationUnitId = resultId;
// We can reasonably assume that makeDebugCompilationUnit will be called before any of
// debug-scope stack. Function scopes and lexical scopes will occur afterward.
assert(currentDebugScopeId.empty());
currentDebugScopeId.push(nonSemanticShaderCompilationUnitId);
return resultId;
}
Id Builder::createDebugGlobalVariable(Id const type, char const*const name, Id const variable)
{
assert(type != 0);
Instruction* inst = new Instruction(getUniqueId(), makeVoidType(), OpExtInst);
inst->reserveOperands(11);
inst->addIdOperand(nonSemanticShaderDebugInfo);
inst->addImmediateOperand(NonSemanticShaderDebugInfo100DebugGlobalVariable);
inst->addIdOperand(getStringId(name)); // name id
inst->addIdOperand(type); // type id
inst->addIdOperand(makeDebugSource(currentFileId)); // source id
inst->addIdOperand(makeUintConstant(currentLine)); // line id TODO: currentLine always zero?
inst->addIdOperand(makeUintConstant(0)); // TODO: column id
inst->addIdOperand(makeDebugCompilationUnit()); // scope id
inst->addIdOperand(getStringId(name)); // linkage name id
inst->addIdOperand(variable); // variable id
inst->addIdOperand(makeUintConstant(NonSemanticShaderDebugInfo100FlagIsDefinition)); // flags id
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(inst));
module.mapInstruction(inst);
return inst->getResultId();
}
Id Builder::createDebugLocalVariable(Id type, char const*const name, size_t const argNumber)
{
assert(name != nullptr);
assert(!currentDebugScopeId.empty());
Instruction* inst = new Instruction(getUniqueId(), makeVoidType(), OpExtInst);
inst->reserveOperands(9);
inst->addIdOperand(nonSemanticShaderDebugInfo);
inst->addImmediateOperand(NonSemanticShaderDebugInfo100DebugLocalVariable);
inst->addIdOperand(getStringId(name)); // name id
inst->addIdOperand(type); // type id
inst->addIdOperand(makeDebugSource(currentFileId)); // source id
inst->addIdOperand(makeUintConstant(currentLine)); // line id
inst->addIdOperand(makeUintConstant(0)); // TODO: column id
inst->addIdOperand(currentDebugScopeId.top()); // scope id
inst->addIdOperand(makeUintConstant(NonSemanticShaderDebugInfo100FlagIsLocal)); // flags id
if(argNumber != 0) {
inst->addIdOperand(makeUintConstant(argNumber));
}
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(inst));
module.mapInstruction(inst);
return inst->getResultId();
}
Id Builder::makeDebugExpression()
{
if (debugExpression != 0)
return debugExpression;
Instruction* inst = new Instruction(getUniqueId(), makeVoidType(), OpExtInst);
inst->reserveOperands(2);
inst->addIdOperand(nonSemanticShaderDebugInfo);
inst->addImmediateOperand(NonSemanticShaderDebugInfo100DebugExpression);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(inst));
module.mapInstruction(inst);
debugExpression = inst->getResultId();
return debugExpression;
}
Id Builder::makeDebugDeclare(Id const debugLocalVariable, Id const pointer)
{
Instruction* inst = new Instruction(getUniqueId(), makeVoidType(), OpExtInst);
inst->reserveOperands(5);
inst->addIdOperand(nonSemanticShaderDebugInfo);
inst->addImmediateOperand(NonSemanticShaderDebugInfo100DebugDeclare);
inst->addIdOperand(debugLocalVariable); // debug local variable id
inst->addIdOperand(pointer); // pointer to local variable id
inst->addIdOperand(makeDebugExpression()); // expression id
addInstruction(std::unique_ptr<Instruction>(inst));
return inst->getResultId();
}
Id Builder::makeDebugValue(Id const debugLocalVariable, Id const value)
{
Instruction* inst = new Instruction(getUniqueId(), makeVoidType(), OpExtInst);
inst->reserveOperands(5);
inst->addIdOperand(nonSemanticShaderDebugInfo);
inst->addImmediateOperand(NonSemanticShaderDebugInfo100DebugValue);
inst->addIdOperand(debugLocalVariable); // debug local variable id
inst->addIdOperand(value); // value of local variable id
inst->addIdOperand(makeDebugExpression()); // expression id
addInstruction(std::unique_ptr<Instruction>(inst));
return inst->getResultId();
}
Id Builder::makeAccelerationStructureType()
{
Instruction *type;
if (groupedTypes[OpTypeAccelerationStructureKHR].size() == 0) {
type = new Instruction(getUniqueId(), NoType, OpTypeAccelerationStructureKHR);
groupedTypes[OpTypeAccelerationStructureKHR].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
if (emitNonSemanticShaderDebugInfo) {
spv::Id debugType = makeCompositeDebugType({}, "accelerationStructure", NonSemanticShaderDebugInfo100Structure, true);
debugId[type->getResultId()] = debugType;
}
} else {
type = groupedTypes[OpTypeAccelerationStructureKHR].back();
}
return type->getResultId();
}
Id Builder::makeRayQueryType()
{
Instruction *type;
if (groupedTypes[OpTypeRayQueryKHR].size() == 0) {
type = new Instruction(getUniqueId(), NoType, OpTypeRayQueryKHR);
groupedTypes[OpTypeRayQueryKHR].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
if (emitNonSemanticShaderDebugInfo) {
spv::Id debugType = makeCompositeDebugType({}, "rayQuery", NonSemanticShaderDebugInfo100Structure, true);
debugId[type->getResultId()] = debugType;
}
} else {
type = groupedTypes[OpTypeRayQueryKHR].back();
}
return type->getResultId();
}
Id Builder::makeHitObjectNVType()
{
Instruction *type;
if (groupedTypes[OpTypeHitObjectNV].size() == 0) {
type = new Instruction(getUniqueId(), NoType, OpTypeHitObjectNV);
groupedTypes[OpTypeHitObjectNV].push_back(type);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
} else {
type = groupedTypes[OpTypeHitObjectNV].back();
}
return type->getResultId();
}
Id Builder::getDerefTypeId(Id resultId) const
{
Id typeId = getTypeId(resultId);
assert(isPointerType(typeId));
return module.getInstruction(typeId)->getIdOperand(1);
}
Op Builder::getMostBasicTypeClass(Id typeId) const
{
Instruction* instr = module.getInstruction(typeId);
Op typeClass = instr->getOpCode();
switch (typeClass)
{
case OpTypeVector:
case OpTypeMatrix:
case OpTypeArray:
case OpTypeRuntimeArray:
return getMostBasicTypeClass(instr->getIdOperand(0));
case OpTypePointer:
return getMostBasicTypeClass(instr->getIdOperand(1));
default:
return typeClass;
}
}
unsigned int Builder::getNumTypeConstituents(Id typeId) const
{
Instruction* instr = module.getInstruction(typeId);
switch (instr->getOpCode())
{
case OpTypeBool:
case OpTypeInt:
case OpTypeFloat:
case OpTypePointer:
return 1;
case OpTypeVector:
case OpTypeMatrix:
return instr->getImmediateOperand(1);
case OpTypeArray:
{
Id lengthId = instr->getIdOperand(1);
return module.getInstruction(lengthId)->getImmediateOperand(0);
}
case OpTypeStruct:
return instr->getNumOperands();
case OpTypeCooperativeMatrixKHR:
case OpTypeCooperativeMatrixNV:
// has only one constituent when used with OpCompositeConstruct.
return 1;
default:
assert(0);
return 1;
}
}
// Return the lowest-level type of scalar that an homogeneous composite is made out of.
// Typically, this is just to find out if something is made out of ints or floats.
// However, it includes returning a structure, if say, it is an array of structure.
Id Builder::getScalarTypeId(Id typeId) const
{
Instruction* instr = module.getInstruction(typeId);
Op typeClass = instr->getOpCode();
switch (typeClass)
{
case OpTypeVoid:
case OpTypeBool:
case OpTypeInt:
case OpTypeFloat:
case OpTypeStruct:
return instr->getResultId();
case OpTypeVector:
case OpTypeMatrix:
case OpTypeArray:
case OpTypeRuntimeArray:
case OpTypePointer:
return getScalarTypeId(getContainedTypeId(typeId));
default:
assert(0);
return NoResult;
}
}
// Return the type of 'member' of a composite.
Id Builder::getContainedTypeId(Id typeId, int member) const
{
Instruction* instr = module.getInstruction(typeId);
Op typeClass = instr->getOpCode();
switch (typeClass)
{
case OpTypeVector:
case OpTypeMatrix:
case OpTypeArray:
case OpTypeRuntimeArray:
case OpTypeCooperativeMatrixKHR:
case OpTypeCooperativeMatrixNV:
return instr->getIdOperand(0);
case OpTypePointer:
return instr->getIdOperand(1);
case OpTypeStruct:
return instr->getIdOperand(member);
default:
assert(0);
return NoResult;
}
}
// Figure out the final resulting type of the access chain.
Id Builder::getResultingAccessChainType() const
{
assert(accessChain.base != NoResult);
Id typeId = getTypeId(accessChain.base);
assert(isPointerType(typeId));
typeId = getContainedTypeId(typeId);
for (int i = 0; i < (int)accessChain.indexChain.size(); ++i) {
if (isStructType(typeId)) {
assert(isConstantScalar(accessChain.indexChain[i]));
typeId = getContainedTypeId(typeId, getConstantScalar(accessChain.indexChain[i]));
} else
typeId = getContainedTypeId(typeId, accessChain.indexChain[i]);
}
return typeId;
}
// Return the immediately contained type of a given composite type.
Id Builder::getContainedTypeId(Id typeId) const
{
return getContainedTypeId(typeId, 0);
}
// Returns true if 'typeId' is or contains a scalar type declared with 'typeOp'
// of width 'width'. The 'width' is only consumed for int and float types.
// Returns false otherwise.
bool Builder::containsType(Id typeId, spv::Op typeOp, unsigned int width) const
{
const Instruction& instr = *module.getInstruction(typeId);
Op typeClass = instr.getOpCode();
switch (typeClass)
{
case OpTypeInt:
case OpTypeFloat:
return typeClass == typeOp && instr.getImmediateOperand(0) == width;
case OpTypeStruct:
for (int m = 0; m < instr.getNumOperands(); ++m) {
if (containsType(instr.getIdOperand(m), typeOp, width))
return true;
}
return false;
case OpTypePointer:
return false;
case OpTypeVector:
case OpTypeMatrix:
case OpTypeArray:
case OpTypeRuntimeArray:
return containsType(getContainedTypeId(typeId), typeOp, width);
default:
return typeClass == typeOp;
}
}
// return true if the type is a pointer to PhysicalStorageBufferEXT or an
// contains such a pointer. These require restrict/aliased decorations.
bool Builder::containsPhysicalStorageBufferOrArray(Id typeId) const
{
const Instruction& instr = *module.getInstruction(typeId);
Op typeClass = instr.getOpCode();
switch (typeClass)
{
case OpTypePointer:
return getTypeStorageClass(typeId) == StorageClassPhysicalStorageBufferEXT;
case OpTypeArray:
return containsPhysicalStorageBufferOrArray(getContainedTypeId(typeId));
case OpTypeStruct:
for (int m = 0; m < instr.getNumOperands(); ++m) {
if (containsPhysicalStorageBufferOrArray(instr.getIdOperand(m)))
return true;
}
return false;
default:
return false;
}
}
// See if a scalar constant of this type has already been created, so it
// can be reused rather than duplicated. (Required by the specification).
Id Builder::findScalarConstant(Op typeClass, Op opcode, Id typeId, unsigned value)
{
Instruction* constant;
for (int i = 0; i < (int)groupedConstants[typeClass].size(); ++i) {
constant = groupedConstants[typeClass][i];
if (constant->getOpCode() == opcode &&
constant->getTypeId() == typeId &&
constant->getImmediateOperand(0) == value)
return constant->getResultId();
}
return 0;
}
// Version of findScalarConstant (see above) for scalars that take two operands (e.g. a 'double' or 'int64').
Id Builder::findScalarConstant(Op typeClass, Op opcode, Id typeId, unsigned v1, unsigned v2)
{
Instruction* constant;
for (int i = 0; i < (int)groupedConstants[typeClass].size(); ++i) {
constant = groupedConstants[typeClass][i];
if (constant->getOpCode() == opcode &&
constant->getTypeId() == typeId &&
constant->getImmediateOperand(0) == v1 &&
constant->getImmediateOperand(1) == v2)
return constant->getResultId();
}
return 0;
}
// Return true if consuming 'opcode' means consuming a constant.
// "constant" here means after final transform to executable code,
// the value consumed will be a constant, so includes specialization.
bool Builder::isConstantOpCode(Op opcode) const
{
switch (opcode) {
case OpUndef:
case OpConstantTrue:
case OpConstantFalse:
case OpConstant:
case OpConstantComposite:
case OpConstantCompositeReplicateEXT:
case OpConstantSampler:
case OpConstantNull:
case OpSpecConstantTrue:
case OpSpecConstantFalse:
case OpSpecConstant:
case OpSpecConstantComposite:
case OpSpecConstantCompositeReplicateEXT:
case OpSpecConstantOp:
return true;
default:
return false;
}
}
// Return true if consuming 'opcode' means consuming a specialization constant.
bool Builder::isSpecConstantOpCode(Op opcode) const
{
switch (opcode) {
case OpSpecConstantTrue:
case OpSpecConstantFalse:
case OpSpecConstant:
case OpSpecConstantComposite:
case OpSpecConstantOp:
case OpSpecConstantCompositeReplicateEXT:
return true;
default:
return false;
}
}
Id Builder::makeNullConstant(Id typeId)
{
Instruction* constant;
// See if we already made it.
Id existing = NoResult;
for (int i = 0; i < (int)nullConstants.size(); ++i) {
constant = nullConstants[i];
if (constant->getTypeId() == typeId)
existing = constant->getResultId();
}
if (existing != NoResult)
return existing;
// Make it
Instruction* c = new Instruction(getUniqueId(), typeId, OpConstantNull);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(c));
nullConstants.push_back(c);
module.mapInstruction(c);
return c->getResultId();
}
Id Builder::makeBoolConstant(bool b, bool specConstant)
{
Id typeId = makeBoolType();
Instruction* constant;
Op opcode = specConstant ? (b ? OpSpecConstantTrue : OpSpecConstantFalse) : (b ? OpConstantTrue : OpConstantFalse);
// See if we already made it. Applies only to regular constants, because specialization constants
// must remain distinct for the purpose of applying a SpecId decoration.
if (! specConstant) {
Id existing = 0;
for (int i = 0; i < (int)groupedConstants[OpTypeBool].size(); ++i) {
constant = groupedConstants[OpTypeBool][i];
if (constant->getTypeId() == typeId && constant->getOpCode() == opcode)
existing = constant->getResultId();
}
if (existing)
return existing;
}
// Make it
Instruction* c = new Instruction(getUniqueId(), typeId, opcode);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(c));
groupedConstants[OpTypeBool].push_back(c);
module.mapInstruction(c);
return c->getResultId();
}
Id Builder::makeIntConstant(Id typeId, unsigned value, bool specConstant)
{
Op opcode = specConstant ? OpSpecConstant : OpConstant;
// See if we already made it. Applies only to regular constants, because specialization constants
// must remain distinct for the purpose of applying a SpecId decoration.
if (! specConstant) {
Id existing = findScalarConstant(OpTypeInt, opcode, typeId, value);
if (existing)
return existing;
}
Instruction* c = new Instruction(getUniqueId(), typeId, opcode);
c->addImmediateOperand(value);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(c));
groupedConstants[OpTypeInt].push_back(c);
module.mapInstruction(c);
return c->getResultId();
}
Id Builder::makeInt64Constant(Id typeId, unsigned long long value, bool specConstant)
{
Op opcode = specConstant ? OpSpecConstant : OpConstant;
unsigned op1 = value & 0xFFFFFFFF;
unsigned op2 = value >> 32;
// See if we already made it. Applies only to regular constants, because specialization constants
// must remain distinct for the purpose of applying a SpecId decoration.
if (! specConstant) {
Id existing = findScalarConstant(OpTypeInt, opcode, typeId, op1, op2);
if (existing)
return existing;
}
Instruction* c = new Instruction(getUniqueId(), typeId, opcode);
c->reserveOperands(2);
c->addImmediateOperand(op1);
c->addImmediateOperand(op2);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(c));
groupedConstants[OpTypeInt].push_back(c);
module.mapInstruction(c);
return c->getResultId();
}
Id Builder::makeFloatConstant(float f, bool specConstant)
{
Op opcode = specConstant ? OpSpecConstant : OpConstant;
Id typeId = makeFloatType(32);
union { float fl; unsigned int ui; } u;
u.fl = f;
unsigned value = u.ui;
// See if we already made it. Applies only to regular constants, because specialization constants
// must remain distinct for the purpose of applying a SpecId decoration.
if (! specConstant) {
Id existing = findScalarConstant(OpTypeFloat, opcode, typeId, value);
if (existing)
return existing;
}
Instruction* c = new Instruction(getUniqueId(), typeId, opcode);
c->addImmediateOperand(value);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(c));
groupedConstants[OpTypeFloat].push_back(c);
module.mapInstruction(c);
return c->getResultId();
}
Id Builder::makeDoubleConstant(double d, bool specConstant)
{
Op opcode = specConstant ? OpSpecConstant : OpConstant;
Id typeId = makeFloatType(64);
union { double db; unsigned long long ull; } u;
u.db = d;
unsigned long long value = u.ull;
unsigned op1 = value & 0xFFFFFFFF;
unsigned op2 = value >> 32;
// See if we already made it. Applies only to regular constants, because specialization constants
// must remain distinct for the purpose of applying a SpecId decoration.
if (! specConstant) {
Id existing = findScalarConstant(OpTypeFloat, opcode, typeId, op1, op2);
if (existing)
return existing;
}
Instruction* c = new Instruction(getUniqueId(), typeId, opcode);
c->reserveOperands(2);
c->addImmediateOperand(op1);
c->addImmediateOperand(op2);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(c));
groupedConstants[OpTypeFloat].push_back(c);
module.mapInstruction(c);
return c->getResultId();
}
Id Builder::makeFloat16Constant(float f16, bool specConstant)
{
Op opcode = specConstant ? OpSpecConstant : OpConstant;
Id typeId = makeFloatType(16);
spvutils::HexFloat<spvutils::FloatProxy<float>> fVal(f16);
spvutils::HexFloat<spvutils::FloatProxy<spvutils::Float16>> f16Val(0);
fVal.castTo(f16Val, spvutils::kRoundToZero);
unsigned value = f16Val.value().getAsFloat().get_value();
// See if we already made it. Applies only to regular constants, because specialization constants
// must remain distinct for the purpose of applying a SpecId decoration.
if (!specConstant) {
Id existing = findScalarConstant(OpTypeFloat, opcode, typeId, value);
if (existing)
return existing;
}
Instruction* c = new Instruction(getUniqueId(), typeId, opcode);
c->addImmediateOperand(value);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(c));
groupedConstants[OpTypeFloat].push_back(c);
module.mapInstruction(c);
return c->getResultId();
}
Id Builder::makeFpConstant(Id type, double d, bool specConstant)
{
const int width = getScalarTypeWidth(type);
assert(isFloatType(type));
switch (width) {
case 16:
return makeFloat16Constant((float)d, specConstant);
case 32:
return makeFloatConstant((float)d, specConstant);
case 64:
return makeDoubleConstant(d, specConstant);
default:
break;
}
assert(false);
return NoResult;
}
Id Builder::importNonSemanticShaderDebugInfoInstructions()
{
assert(emitNonSemanticShaderDebugInfo == true);
if(nonSemanticShaderDebugInfo == 0)
{
this->addExtension(spv::E_SPV_KHR_non_semantic_info);
nonSemanticShaderDebugInfo = this->import("NonSemantic.Shader.DebugInfo.100");
}
return nonSemanticShaderDebugInfo;
}
Id Builder::findCompositeConstant(Op typeClass, Id typeId, const std::vector<Id>& comps)
{
Instruction* constant = nullptr;
bool found = false;
for (int i = 0; i < (int)groupedConstants[typeClass].size(); ++i) {
constant = groupedConstants[typeClass][i];
if (constant->getTypeId() != typeId)
continue;
// same contents?
bool mismatch = false;
for (int op = 0; op < constant->getNumOperands(); ++op) {
if (constant->getIdOperand(op) != comps[op]) {
mismatch = true;
break;
}
}
if (! mismatch) {
found = true;
break;
}
}
return found ? constant->getResultId() : NoResult;
}
Id Builder::findStructConstant(Id typeId, const std::vector<Id>& comps)
{
Instruction* constant = nullptr;
bool found = false;
for (int i = 0; i < (int)groupedStructConstants[typeId].size(); ++i) {
constant = groupedStructConstants[typeId][i];
// same contents?
bool mismatch = false;
for (int op = 0; op < constant->getNumOperands(); ++op) {
if (constant->getIdOperand(op) != comps[op]) {
mismatch = true;
break;
}
}
if (! mismatch) {
found = true;
break;
}
}
return found ? constant->getResultId() : NoResult;
}
// Comments in header
Id Builder::makeCompositeConstant(Id typeId, const std::vector<Id>& members, bool specConstant)
{
assert(typeId);
Op typeClass = getTypeClass(typeId);
bool replicate = false;
size_t numMembers = members.size();
if (useReplicatedComposites) {
// use replicate if all members are the same
replicate = numMembers > 0 &&
std::equal(members.begin() + 1, members.end(), members.begin());
if (replicate) {
numMembers = 1;
addCapability(spv::CapabilityReplicatedCompositesEXT);
addExtension(spv::E_SPV_EXT_replicated_composites);
}
}
Op opcode = replicate ?
(specConstant ? OpSpecConstantCompositeReplicateEXT : OpConstantCompositeReplicateEXT) :
(specConstant ? OpSpecConstantComposite : OpConstantComposite);
switch (typeClass) {
case OpTypeVector:
case OpTypeArray:
case OpTypeMatrix:
case OpTypeCooperativeMatrixKHR:
case OpTypeCooperativeMatrixNV:
if (! specConstant) {
Id existing = findCompositeConstant(typeClass, typeId, members);
if (existing)
return existing;
}
break;
case OpTypeStruct:
if (! specConstant) {
Id existing = findStructConstant(typeId, members);
if (existing)
return existing;
}
break;
default:
assert(0);
return makeFloatConstant(0.0);
}
Instruction* c = new Instruction(getUniqueId(), typeId, opcode);
c->reserveOperands(members.size());
for (size_t op = 0; op < numMembers; ++op)
c->addIdOperand(members[op]);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(c));
if (typeClass == OpTypeStruct)
groupedStructConstants[typeId].push_back(c);
else
groupedConstants[typeClass].push_back(c);
module.mapInstruction(c);
return c->getResultId();
}
Instruction* Builder::addEntryPoint(ExecutionModel model, Function* function, const char* name)
{
Instruction* entryPoint = new Instruction(OpEntryPoint);
entryPoint->reserveOperands(3);
entryPoint->addImmediateOperand(model);
entryPoint->addIdOperand(function->getId());
entryPoint->addStringOperand(name);
entryPoints.push_back(std::unique_ptr<Instruction>(entryPoint));
return entryPoint;
}
// Currently relying on the fact that all 'value' of interest are small non-negative values.
void Builder::addExecutionMode(Function* entryPoint, ExecutionMode mode, int value1, int value2, int value3)
{
// entryPoint can be null if we are in compile-only mode
if (!entryPoint)
return;
Instruction* instr = new Instruction(OpExecutionMode);
instr->reserveOperands(3);
instr->addIdOperand(entryPoint->getId());
instr->addImmediateOperand(mode);
if (value1 >= 0)
instr->addImmediateOperand(value1);
if (value2 >= 0)
instr->addImmediateOperand(value2);
if (value3 >= 0)
instr->addImmediateOperand(value3);
executionModes.push_back(std::unique_ptr<Instruction>(instr));
}
void Builder::addExecutionMode(Function* entryPoint, ExecutionMode mode, const std::vector<unsigned>& literals)
{
// entryPoint can be null if we are in compile-only mode
if (!entryPoint)
return;
Instruction* instr = new Instruction(OpExecutionMode);
instr->reserveOperands(literals.size() + 2);
instr->addIdOperand(entryPoint->getId());
instr->addImmediateOperand(mode);
for (auto literal : literals)
instr->addImmediateOperand(literal);
executionModes.push_back(std::unique_ptr<Instruction>(instr));
}
void Builder::addExecutionModeId(Function* entryPoint, ExecutionMode mode, const std::vector<Id>& operandIds)
{
// entryPoint can be null if we are in compile-only mode
if (!entryPoint)
return;
Instruction* instr = new Instruction(OpExecutionModeId);
instr->reserveOperands(operandIds.size() + 2);
instr->addIdOperand(entryPoint->getId());
instr->addImmediateOperand(mode);
for (auto operandId : operandIds)
instr->addIdOperand(operandId);
executionModes.push_back(std::unique_ptr<Instruction>(instr));
}
void Builder::addName(Id id, const char* string)
{
Instruction* name = new Instruction(OpName);
name->reserveOperands(2);
name->addIdOperand(id);
name->addStringOperand(string);
names.push_back(std::unique_ptr<Instruction>(name));
}
void Builder::addMemberName(Id id, int memberNumber, const char* string)
{
Instruction* name = new Instruction(OpMemberName);
name->reserveOperands(3);
name->addIdOperand(id);
name->addImmediateOperand(memberNumber);
name->addStringOperand(string);
names.push_back(std::unique_ptr<Instruction>(name));
}
void Builder::addDecoration(Id id, Decoration decoration, int num)
{
if (decoration == spv::DecorationMax)
return;
Instruction* dec = new Instruction(OpDecorate);
dec->reserveOperands(2);
dec->addIdOperand(id);
dec->addImmediateOperand(decoration);
if (num >= 0)
dec->addImmediateOperand(num);
decorations.push_back(std::unique_ptr<Instruction>(dec));
}
void Builder::addDecoration(Id id, Decoration decoration, const char* s)
{
if (decoration == spv::DecorationMax)
return;
Instruction* dec = new Instruction(OpDecorateString);
dec->reserveOperands(3);
dec->addIdOperand(id);
dec->addImmediateOperand(decoration);
dec->addStringOperand(s);
decorations.push_back(std::unique_ptr<Instruction>(dec));
}
void Builder::addDecoration(Id id, Decoration decoration, const std::vector<unsigned>& literals)
{
if (decoration == spv::DecorationMax)
return;
Instruction* dec = new Instruction(OpDecorate);
dec->reserveOperands(literals.size() + 2);
dec->addIdOperand(id);
dec->addImmediateOperand(decoration);
for (auto literal : literals)
dec->addImmediateOperand(literal);
decorations.push_back(std::unique_ptr<Instruction>(dec));
}
void Builder::addDecoration(Id id, Decoration decoration, const std::vector<const char*>& strings)
{
if (decoration == spv::DecorationMax)
return;
Instruction* dec = new Instruction(OpDecorateString);
dec->reserveOperands(strings.size() + 2);
dec->addIdOperand(id);
dec->addImmediateOperand(decoration);
for (auto string : strings)
dec->addStringOperand(string);
decorations.push_back(std::unique_ptr<Instruction>(dec));
}
void Builder::addLinkageDecoration(Id id, const char* name, spv::LinkageType linkType) {
Instruction* dec = new Instruction(OpDecorate);
dec->reserveOperands(4);
dec->addIdOperand(id);
dec->addImmediateOperand(spv::DecorationLinkageAttributes);
dec->addStringOperand(name);
dec->addImmediateOperand(linkType);
decorations.push_back(std::unique_ptr<Instruction>(dec));
}
void Builder::addDecorationId(Id id, Decoration decoration, Id idDecoration)
{
if (decoration == spv::DecorationMax)
return;
Instruction* dec = new Instruction(OpDecorateId);
dec->reserveOperands(3);
dec->addIdOperand(id);
dec->addImmediateOperand(decoration);
dec->addIdOperand(idDecoration);
decorations.push_back(std::unique_ptr<Instruction>(dec));
}
void Builder::addDecorationId(Id id, Decoration decoration, const std::vector<Id>& operandIds)
{
if(decoration == spv::DecorationMax)
return;
Instruction* dec = new Instruction(OpDecorateId);
dec->reserveOperands(operandIds.size() + 2);
dec->addIdOperand(id);
dec->addImmediateOperand(decoration);
for (auto operandId : operandIds)
dec->addIdOperand(operandId);
decorations.push_back(std::unique_ptr<Instruction>(dec));
}
void Builder::addMemberDecoration(Id id, unsigned int member, Decoration decoration, int num)
{
if (decoration == spv::DecorationMax)
return;
Instruction* dec = new Instruction(OpMemberDecorate);
dec->reserveOperands(3);
dec->addIdOperand(id);
dec->addImmediateOperand(member);
dec->addImmediateOperand(decoration);
if (num >= 0)
dec->addImmediateOperand(num);
decorations.push_back(std::unique_ptr<Instruction>(dec));
}
void Builder::addMemberDecoration(Id id, unsigned int member, Decoration decoration, const char *s)
{
if (decoration == spv::DecorationMax)
return;
Instruction* dec = new Instruction(OpMemberDecorateStringGOOGLE);
dec->reserveOperands(4);
dec->addIdOperand(id);
dec->addImmediateOperand(member);
dec->addImmediateOperand(decoration);
dec->addStringOperand(s);
decorations.push_back(std::unique_ptr<Instruction>(dec));
}
void Builder::addMemberDecoration(Id id, unsigned int member, Decoration decoration, const std::vector<unsigned>& literals)
{
if (decoration == spv::DecorationMax)
return;
Instruction* dec = new Instruction(OpMemberDecorate);
dec->reserveOperands(literals.size() + 3);
dec->addIdOperand(id);
dec->addImmediateOperand(member);
dec->addImmediateOperand(decoration);
for (auto literal : literals)
dec->addImmediateOperand(literal);
decorations.push_back(std::unique_ptr<Instruction>(dec));
}
void Builder::addMemberDecoration(Id id, unsigned int member, Decoration decoration, const std::vector<const char*>& strings)
{
if (decoration == spv::DecorationMax)
return;
Instruction* dec = new Instruction(OpMemberDecorateString);
dec->reserveOperands(strings.size() + 3);
dec->addIdOperand(id);
dec->addImmediateOperand(member);
dec->addImmediateOperand(decoration);
for (auto string : strings)
dec->addStringOperand(string);
decorations.push_back(std::unique_ptr<Instruction>(dec));
}
void Builder::addInstruction(std::unique_ptr<Instruction> inst) {
// Optionally insert OpDebugScope
if (emitNonSemanticShaderDebugInfo && dirtyScopeTracker) {
if (buildPoint->updateDebugScope(currentDebugScopeId.top())) {
auto scopeInst = std::make_unique<Instruction>(getUniqueId(), makeVoidType(), OpExtInst);
scopeInst->reserveOperands(3);
scopeInst->addIdOperand(nonSemanticShaderDebugInfo);
scopeInst->addImmediateOperand(NonSemanticShaderDebugInfo100DebugScope);
scopeInst->addIdOperand(currentDebugScopeId.top());
buildPoint->addInstruction(std::move(scopeInst));
}
dirtyScopeTracker = false;
}
// Insert OpLine/OpDebugLine if the debug source location has changed
if (trackDebugInfo && dirtyLineTracker) {
if (buildPoint->updateDebugSourceLocation(currentLine, 0, currentFileId)) {
if (emitSpirvDebugInfo) {
auto lineInst = std::make_unique<Instruction>(OpLine);
lineInst->reserveOperands(3);
lineInst->addIdOperand(currentFileId);
lineInst->addImmediateOperand(currentLine);
lineInst->addImmediateOperand(0);
buildPoint->addInstruction(std::move(lineInst));
}
if (emitNonSemanticShaderDebugInfo) {
auto lineInst = std::make_unique<Instruction>(getUniqueId(), makeVoidType(), OpExtInst);
lineInst->reserveOperands(7);
lineInst->addIdOperand(nonSemanticShaderDebugInfo);
lineInst->addImmediateOperand(NonSemanticShaderDebugInfo100DebugLine);
lineInst->addIdOperand(makeDebugSource(currentFileId));
lineInst->addIdOperand(makeUintConstant(currentLine));
lineInst->addIdOperand(makeUintConstant(currentLine));
lineInst->addIdOperand(makeUintConstant(0));
lineInst->addIdOperand(makeUintConstant(0));
buildPoint->addInstruction(std::move(lineInst));
}
}
dirtyLineTracker = false;
}
buildPoint->addInstruction(std::move(inst));
}
// Comments in header
Function* Builder::makeEntryPoint(const char* entryPoint)
{
assert(! entryPointFunction);
auto const returnType = makeVoidType();
restoreNonSemanticShaderDebugInfo = emitNonSemanticShaderDebugInfo;
if(sourceLang == spv::SourceLanguageHLSL) {
emitNonSemanticShaderDebugInfo = false;
}
Block* entry = nullptr;
entryPointFunction = makeFunctionEntry(NoPrecision, returnType, entryPoint, LinkageTypeMax, {}, {}, &entry);
emitNonSemanticShaderDebugInfo = restoreNonSemanticShaderDebugInfo;
return entryPointFunction;
}
// Comments in header
Function* Builder::makeFunctionEntry(Decoration precision, Id returnType, const char* name, LinkageType linkType,
const std::vector<Id>& paramTypes,
const std::vector<std::vector<Decoration>>& decorations, Block** entry)
{
// Make the function and initial instructions in it
Id typeId = makeFunctionType(returnType, paramTypes);
Id firstParamId = paramTypes.size() == 0 ? 0 : getUniqueIds((int)paramTypes.size());
Id funcId = getUniqueId();
Function* function = new Function(funcId, returnType, typeId, firstParamId, linkType, name, module);
// Set up the precisions
setPrecision(function->getId(), precision);
function->setReturnPrecision(precision);
for (unsigned p = 0; p < (unsigned)decorations.size(); ++p) {
for (int d = 0; d < (int)decorations[p].size(); ++d) {
addDecoration(firstParamId + p, decorations[p][d]);
function->addParamPrecision(p, decorations[p][d]);
}
}
// reset last debug scope
if (emitNonSemanticShaderDebugInfo) {
dirtyScopeTracker = true;
}
// CFG
assert(entry != nullptr);
*entry = new Block(getUniqueId(), *function);
function->addBlock(*entry);
setBuildPoint(*entry);
if (name)
addName(function->getId(), name);
functions.push_back(std::unique_ptr<Function>(function));
return function;
}
void Builder::setupDebugFunctionEntry(Function* function, const char* name, int line, const std::vector<Id>& paramTypes,
const std::vector<char const*>& paramNames)
{
if (!emitNonSemanticShaderDebugInfo)
return;
currentLine = line;
Id nameId = getStringId(unmangleFunctionName(name));
Id funcTypeId = function->getFuncTypeId();
assert(debugId[funcTypeId] != 0);
Id funcId = function->getId();
assert(funcId != 0);
// Make the debug function instruction
Id debugFuncId = makeDebugFunction(function, nameId, funcTypeId);
debugId[funcId] = debugFuncId;
currentDebugScopeId.push(debugFuncId);
// DebugScope and DebugLine for parameter DebugDeclares
assert(paramTypes.size() == paramNames.size());
if ((int)paramTypes.size() > 0) {
Id firstParamId = function->getParamId(0);
for (size_t p = 0; p < paramTypes.size(); ++p) {
bool passByRef = false;
Id paramTypeId = paramTypes[p];
// For pointer-typed parameters, they are actually passed by reference and we need unwrap the pointer to get the actual parameter type.
if (isPointerType(paramTypeId) || isArrayType(paramTypeId)) {
passByRef = true;
paramTypeId = getContainedTypeId(paramTypeId);
}
auto const& paramName = paramNames[p];
auto const debugLocalVariableId = createDebugLocalVariable(debugId[paramTypeId], paramName, p + 1);
auto const paramId = static_cast<Id>(firstParamId + p);
debugId[paramId] = debugLocalVariableId;
if (passByRef) {
makeDebugDeclare(debugLocalVariableId, paramId);
} else {
makeDebugValue(debugLocalVariableId, paramId);
}
}
}
// Clear debug scope stack
if (emitNonSemanticShaderDebugInfo)
currentDebugScopeId.pop();
}
Id Builder::makeDebugFunction([[maybe_unused]] Function* function, Id nameId, Id funcTypeId)
{
assert(function != nullptr);
assert(nameId != 0);
assert(funcTypeId != 0);
assert(debugId[funcTypeId] != 0);
Id funcId = getUniqueId();
auto type = new Instruction(funcId, makeVoidType(), OpExtInst);
type->reserveOperands(11);
type->addIdOperand(nonSemanticShaderDebugInfo);
type->addImmediateOperand(NonSemanticShaderDebugInfo100DebugFunction);
type->addIdOperand(nameId);
type->addIdOperand(debugId[funcTypeId]);
type->addIdOperand(makeDebugSource(currentFileId)); // TODO: This points to file of definition instead of declaration
type->addIdOperand(makeUintConstant(currentLine)); // TODO: This points to line of definition instead of declaration
type->addIdOperand(makeUintConstant(0)); // column
type->addIdOperand(makeDebugCompilationUnit()); // scope
type->addIdOperand(nameId); // linkage name
type->addIdOperand(makeUintConstant(NonSemanticShaderDebugInfo100FlagIsPublic));
type->addIdOperand(makeUintConstant(currentLine));
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(type));
module.mapInstruction(type);
return funcId;
}
Id Builder::makeDebugLexicalBlock(uint32_t line) {
assert(!currentDebugScopeId.empty());
Id lexId = getUniqueId();
auto lex = new Instruction(lexId, makeVoidType(), OpExtInst);
lex->reserveOperands(6);
lex->addIdOperand(nonSemanticShaderDebugInfo);
lex->addImmediateOperand(NonSemanticShaderDebugInfo100DebugLexicalBlock);
lex->addIdOperand(makeDebugSource(currentFileId));
lex->addIdOperand(makeUintConstant(line));
lex->addIdOperand(makeUintConstant(0)); // column
lex->addIdOperand(currentDebugScopeId.top()); // scope
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(lex));
module.mapInstruction(lex);
return lexId;
}
std::string Builder::unmangleFunctionName(std::string const& name) const
{
assert(name.length() > 0);
if(name.rfind('(') != std::string::npos) {
return name.substr(0, name.rfind('('));
} else {
return name;
}
}
// Comments in header
void Builder::makeReturn(bool implicit, Id retVal)
{
if (retVal) {
Instruction* inst = new Instruction(NoResult, NoType, OpReturnValue);
inst->addIdOperand(retVal);
addInstruction(std::unique_ptr<Instruction>(inst));
} else
addInstruction(std::unique_ptr<Instruction>(new Instruction(NoResult, NoType, OpReturn)));
if (! implicit)
createAndSetNoPredecessorBlock("post-return");
}
// Comments in header
void Builder::enterLexicalBlock(uint32_t line)
{
// Generate new lexical scope debug instruction
Id lexId = makeDebugLexicalBlock(line);
currentDebugScopeId.push(lexId);
dirtyScopeTracker = true;
}
// Comments in header
void Builder::leaveLexicalBlock()
{
// Pop current scope from stack and clear current scope
currentDebugScopeId.pop();
dirtyScopeTracker = true;
}
// Comments in header
void Builder::enterFunction(Function const* function)
{
// Save and disable debugInfo for HLSL entry point function. It is a wrapper
// function with no user code in it.
restoreNonSemanticShaderDebugInfo = emitNonSemanticShaderDebugInfo;
if (sourceLang == spv::SourceLanguageHLSL && function == entryPointFunction) {
emitNonSemanticShaderDebugInfo = false;
}
if (emitNonSemanticShaderDebugInfo) {
// Initialize scope state
Id funcId = function->getFuncId();
currentDebugScopeId.push(debugId[funcId]);
// Create DebugFunctionDefinition
spv::Id resultId = getUniqueId();
Instruction* defInst = new Instruction(resultId, makeVoidType(), OpExtInst);
defInst->reserveOperands(4);
defInst->addIdOperand(nonSemanticShaderDebugInfo);
defInst->addImmediateOperand(NonSemanticShaderDebugInfo100DebugFunctionDefinition);
defInst->addIdOperand(debugId[funcId]);
defInst->addIdOperand(funcId);
addInstruction(std::unique_ptr<Instruction>(defInst));
}
if (auto linkType = function->getLinkType(); linkType != LinkageTypeMax) {
Id funcId = function->getFuncId();
addCapability(CapabilityLinkage);
addLinkageDecoration(funcId, function->getExportName(), linkType);
}
}
// Comments in header
void Builder::leaveFunction()
{
Block* block = buildPoint;
Function& function = buildPoint->getParent();
assert(block);
// If our function did not contain a return, add a return void now.
if (! block->isTerminated()) {
if (function.getReturnType() == makeVoidType())
makeReturn(true);
else {
makeReturn(true, createUndefined(function.getReturnType()));
}
}
// Clear function scope from debug scope stack
if (emitNonSemanticShaderDebugInfo)
currentDebugScopeId.pop();
emitNonSemanticShaderDebugInfo = restoreNonSemanticShaderDebugInfo;
}
// Comments in header
void Builder::makeStatementTerminator(spv::Op opcode, const char *name)
{
addInstruction(std::unique_ptr<Instruction>(new Instruction(opcode)));
createAndSetNoPredecessorBlock(name);
}
// Comments in header
void Builder::makeStatementTerminator(spv::Op opcode, const std::vector<Id>& operands, const char* name)
{
// It's assumed that the terminator instruction is always of void return type
// However in future if there is a need for non void return type, new helper
// methods can be created.
createNoResultOp(opcode, operands);
createAndSetNoPredecessorBlock(name);
}
// Comments in header
Id Builder::createVariable(Decoration precision, StorageClass storageClass, Id type, const char* name, Id initializer,
bool const compilerGenerated)
{
Id pointerType = makePointer(storageClass, type);
Instruction* inst = new Instruction(getUniqueId(), pointerType, OpVariable);
inst->addImmediateOperand(storageClass);
if (initializer != NoResult)
inst->addIdOperand(initializer);
switch (storageClass) {
case StorageClassFunction:
// Validation rules require the declaration in the entry block
buildPoint->getParent().addLocalVariable(std::unique_ptr<Instruction>(inst));
if (emitNonSemanticShaderDebugInfo && !compilerGenerated)
{
auto const debugLocalVariableId = createDebugLocalVariable(debugId[type], name);
debugId[inst->getResultId()] = debugLocalVariableId;
makeDebugDeclare(debugLocalVariableId, inst->getResultId());
}
break;
default:
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(inst));
module.mapInstruction(inst);
if (emitNonSemanticShaderDebugInfo)
{
auto const debugResultId = createDebugGlobalVariable(debugId[type], name, inst->getResultId());
debugId[inst->getResultId()] = debugResultId;
}
break;
}
if (name)
addName(inst->getResultId(), name);
setPrecision(inst->getResultId(), precision);
return inst->getResultId();
}
// Comments in header
Id Builder::createUndefined(Id type)
{
Instruction* inst = new Instruction(getUniqueId(), type, OpUndef);
addInstruction(std::unique_ptr<Instruction>(inst));
return inst->getResultId();
}
// av/vis/nonprivate are unnecessary and illegal for some storage classes.
spv::MemoryAccessMask Builder::sanitizeMemoryAccessForStorageClass(spv::MemoryAccessMask memoryAccess, StorageClass sc)
const
{
switch (sc) {
case spv::StorageClassUniform:
case spv::StorageClassWorkgroup:
case spv::StorageClassStorageBuffer:
case spv::StorageClassPhysicalStorageBufferEXT:
break;
default:
memoryAccess = spv::MemoryAccessMask(memoryAccess &
~(spv::MemoryAccessMakePointerAvailableKHRMask |
spv::MemoryAccessMakePointerVisibleKHRMask |
spv::MemoryAccessNonPrivatePointerKHRMask));
break;
}
return memoryAccess;
}
// Comments in header
void Builder::createStore(Id rValue, Id lValue, spv::MemoryAccessMask memoryAccess, spv::Scope scope,
unsigned int alignment)
{
Instruction* store = new Instruction(OpStore);
store->reserveOperands(2);
store->addIdOperand(lValue);
store->addIdOperand(rValue);
memoryAccess = sanitizeMemoryAccessForStorageClass(memoryAccess, getStorageClass(lValue));
if (memoryAccess != MemoryAccessMaskNone) {
store->addImmediateOperand(memoryAccess);
if (memoryAccess & spv::MemoryAccessAlignedMask) {
store->addImmediateOperand(alignment);
}
if (memoryAccess & spv::MemoryAccessMakePointerAvailableKHRMask) {
store->addIdOperand(makeUintConstant(scope));
}
}
addInstruction(std::unique_ptr<Instruction>(store));
}
// Comments in header
Id Builder::createLoad(Id lValue, spv::Decoration precision, spv::MemoryAccessMask memoryAccess,
spv::Scope scope, unsigned int alignment)
{
Instruction* load = new Instruction(getUniqueId(), getDerefTypeId(lValue), OpLoad);
load->addIdOperand(lValue);
memoryAccess = sanitizeMemoryAccessForStorageClass(memoryAccess, getStorageClass(lValue));
if (memoryAccess != MemoryAccessMaskNone) {
load->addImmediateOperand(memoryAccess);
if (memoryAccess & spv::MemoryAccessAlignedMask) {
load->addImmediateOperand(alignment);
}
if (memoryAccess & spv::MemoryAccessMakePointerVisibleKHRMask) {
load->addIdOperand(makeUintConstant(scope));
}
}
addInstruction(std::unique_ptr<Instruction>(load));
setPrecision(load->getResultId(), precision);
return load->getResultId();
}
// Comments in header
Id Builder::createAccessChain(StorageClass storageClass, Id base, const std::vector<Id>& offsets)
{
// Figure out the final resulting type.
Id typeId = getResultingAccessChainType();
typeId = makePointer(storageClass, typeId);
// Make the instruction
Instruction* chain = new Instruction(getUniqueId(), typeId, OpAccessChain);
chain->reserveOperands(offsets.size() + 1);
chain->addIdOperand(base);
for (int i = 0; i < (int)offsets.size(); ++i)
chain->addIdOperand(offsets[i]);
addInstruction(std::unique_ptr<Instruction>(chain));
return chain->getResultId();
}
Id Builder::createArrayLength(Id base, unsigned int member)
{
spv::Id intType = makeUintType(32);
Instruction* length = new Instruction(getUniqueId(), intType, OpArrayLength);
length->reserveOperands(2);
length->addIdOperand(base);
length->addImmediateOperand(member);
addInstruction(std::unique_ptr<Instruction>(length));
return length->getResultId();
}
Id Builder::createCooperativeMatrixLengthKHR(Id type)
{
spv::Id intType = makeUintType(32);
// Generate code for spec constants if in spec constant operation
// generation mode.
if (generatingOpCodeForSpecConst) {
return createSpecConstantOp(OpCooperativeMatrixLengthKHR, intType, std::vector<Id>(1, type), std::vector<Id>());
}
Instruction* length = new Instruction(getUniqueId(), intType, OpCooperativeMatrixLengthKHR);
length->addIdOperand(type);
addInstruction(std::unique_ptr<Instruction>(length));
return length->getResultId();
}
Id Builder::createCooperativeMatrixLengthNV(Id type)
{
spv::Id intType = makeUintType(32);
// Generate code for spec constants if in spec constant operation
// generation mode.
if (generatingOpCodeForSpecConst) {
return createSpecConstantOp(OpCooperativeMatrixLengthNV, intType, std::vector<Id>(1, type), std::vector<Id>());
}
Instruction* length = new Instruction(getUniqueId(), intType, OpCooperativeMatrixLengthNV);
length->addIdOperand(type);
addInstruction(std::unique_ptr<Instruction>(length));
return length->getResultId();
}
Id Builder::createCompositeExtract(Id composite, Id typeId, unsigned index)
{
// Generate code for spec constants if in spec constant operation
// generation mode.
if (generatingOpCodeForSpecConst) {
return createSpecConstantOp(OpCompositeExtract, typeId, std::vector<Id>(1, composite),
std::vector<Id>(1, index));
}
Instruction* extract = new Instruction(getUniqueId(), typeId, OpCompositeExtract);
extract->reserveOperands(2);
extract->addIdOperand(composite);
extract->addImmediateOperand(index);
addInstruction(std::unique_ptr<Instruction>(extract));
return extract->getResultId();
}
Id Builder::createCompositeExtract(Id composite, Id typeId, const std::vector<unsigned>& indexes)
{
// Generate code for spec constants if in spec constant operation
// generation mode.
if (generatingOpCodeForSpecConst) {
return createSpecConstantOp(OpCompositeExtract, typeId, std::vector<Id>(1, composite), indexes);
}
Instruction* extract = new Instruction(getUniqueId(), typeId, OpCompositeExtract);
extract->reserveOperands(indexes.size() + 1);
extract->addIdOperand(composite);
for (int i = 0; i < (int)indexes.size(); ++i)
extract->addImmediateOperand(indexes[i]);
addInstruction(std::unique_ptr<Instruction>(extract));
return extract->getResultId();
}
Id Builder::createCompositeInsert(Id object, Id composite, Id typeId, unsigned index)
{
Instruction* insert = new Instruction(getUniqueId(), typeId, OpCompositeInsert);
insert->reserveOperands(3);
insert->addIdOperand(object);
insert->addIdOperand(composite);
insert->addImmediateOperand(index);
addInstruction(std::unique_ptr<Instruction>(insert));
return insert->getResultId();
}
Id Builder::createCompositeInsert(Id object, Id composite, Id typeId, const std::vector<unsigned>& indexes)
{
Instruction* insert = new Instruction(getUniqueId(), typeId, OpCompositeInsert);
insert->reserveOperands(indexes.size() + 2);
insert->addIdOperand(object);
insert->addIdOperand(composite);
for (int i = 0; i < (int)indexes.size(); ++i)
insert->addImmediateOperand(indexes[i]);
addInstruction(std::unique_ptr<Instruction>(insert));
return insert->getResultId();
}
Id Builder::createVectorExtractDynamic(Id vector, Id typeId, Id componentIndex)
{
Instruction* extract = new Instruction(getUniqueId(), typeId, OpVectorExtractDynamic);
extract->reserveOperands(2);
extract->addIdOperand(vector);
extract->addIdOperand(componentIndex);
addInstruction(std::unique_ptr<Instruction>(extract));
return extract->getResultId();
}
Id Builder::createVectorInsertDynamic(Id vector, Id typeId, Id component, Id componentIndex)
{
Instruction* insert = new Instruction(getUniqueId(), typeId, OpVectorInsertDynamic);
insert->reserveOperands(3);
insert->addIdOperand(vector);
insert->addIdOperand(component);
insert->addIdOperand(componentIndex);
addInstruction(std::unique_ptr<Instruction>(insert));
return insert->getResultId();
}
// An opcode that has no operands, no result id, and no type
void Builder::createNoResultOp(Op opCode)
{
Instruction* op = new Instruction(opCode);
addInstruction(std::unique_ptr<Instruction>(op));
}
// An opcode that has one id operand, no result id, and no type
void Builder::createNoResultOp(Op opCode, Id operand)
{
Instruction* op = new Instruction(opCode);
op->addIdOperand(operand);
addInstruction(std::unique_ptr<Instruction>(op));
}
// An opcode that has one or more operands, no result id, and no type
void Builder::createNoResultOp(Op opCode, const std::vector<Id>& operands)
{
Instruction* op = new Instruction(opCode);
op->reserveOperands(operands.size());
for (auto id : operands) {
op->addIdOperand(id);
}
addInstruction(std::unique_ptr<Instruction>(op));
}
// An opcode that has multiple operands, no result id, and no type
void Builder::createNoResultOp(Op opCode, const std::vector<IdImmediate>& operands)
{
Instruction* op = new Instruction(opCode);
op->reserveOperands(operands.size());
for (auto it = operands.cbegin(); it != operands.cend(); ++it) {
if (it->isId)
op->addIdOperand(it->word);
else
op->addImmediateOperand(it->word);
}
addInstruction(std::unique_ptr<Instruction>(op));
}
void Builder::createControlBarrier(Scope execution, Scope memory, MemorySemanticsMask semantics)
{
Instruction* op = new Instruction(OpControlBarrier);
op->reserveOperands(3);
op->addIdOperand(makeUintConstant(execution));
op->addIdOperand(makeUintConstant(memory));
op->addIdOperand(makeUintConstant(semantics));
addInstruction(std::unique_ptr<Instruction>(op));
}
void Builder::createMemoryBarrier(unsigned executionScope, unsigned memorySemantics)
{
Instruction* op = new Instruction(OpMemoryBarrier);
op->reserveOperands(2);
op->addIdOperand(makeUintConstant(executionScope));
op->addIdOperand(makeUintConstant(memorySemantics));
addInstruction(std::unique_ptr<Instruction>(op));
}
// An opcode that has one operands, a result id, and a type
Id Builder::createUnaryOp(Op opCode, Id typeId, Id operand)
{
// Generate code for spec constants if in spec constant operation
// generation mode.
if (generatingOpCodeForSpecConst) {
return createSpecConstantOp(opCode, typeId, std::vector<Id>(1, operand), std::vector<Id>());
}
Instruction* op = new Instruction(getUniqueId(), typeId, opCode);
op->addIdOperand(operand);
addInstruction(std::unique_ptr<Instruction>(op));
return op->getResultId();
}
Id Builder::createBinOp(Op opCode, Id typeId, Id left, Id right)
{
// Generate code for spec constants if in spec constant operation
// generation mode.
if (generatingOpCodeForSpecConst) {
std::vector<Id> operands(2);
operands[0] = left; operands[1] = right;
return createSpecConstantOp(opCode, typeId, operands, std::vector<Id>());
}
Instruction* op = new Instruction(getUniqueId(), typeId, opCode);
op->reserveOperands(2);
op->addIdOperand(left);
op->addIdOperand(right);
addInstruction(std::unique_ptr<Instruction>(op));
return op->getResultId();
}
Id Builder::createTriOp(Op opCode, Id typeId, Id op1, Id op2, Id op3)
{
// Generate code for spec constants if in spec constant operation
// generation mode.
if (generatingOpCodeForSpecConst) {
std::vector<Id> operands(3);
operands[0] = op1;
operands[1] = op2;
operands[2] = op3;
return createSpecConstantOp(
opCode, typeId, operands, std::vector<Id>());
}
Instruction* op = new Instruction(getUniqueId(), typeId, opCode);
op->reserveOperands(3);
op->addIdOperand(op1);
op->addIdOperand(op2);
op->addIdOperand(op3);
addInstruction(std::unique_ptr<Instruction>(op));
return op->getResultId();
}
Id Builder::createOp(Op opCode, Id typeId, const std::vector<Id>& operands)
{
Instruction* op = new Instruction(getUniqueId(), typeId, opCode);
op->reserveOperands(operands.size());
for (auto id : operands)
op->addIdOperand(id);
addInstruction(std::unique_ptr<Instruction>(op));
return op->getResultId();
}
Id Builder::createOp(Op opCode, Id typeId, const std::vector<IdImmediate>& operands)
{
Instruction* op = new Instruction(getUniqueId(), typeId, opCode);
op->reserveOperands(operands.size());
for (auto it = operands.cbegin(); it != operands.cend(); ++it) {
if (it->isId)
op->addIdOperand(it->word);
else
op->addImmediateOperand(it->word);
}
addInstruction(std::unique_ptr<Instruction>(op));
return op->getResultId();
}
Id Builder::createSpecConstantOp(Op opCode, Id typeId, const std::vector<Id>& operands,
const std::vector<unsigned>& literals)
{
Instruction* op = new Instruction(getUniqueId(), typeId, OpSpecConstantOp);
op->reserveOperands(operands.size() + literals.size() + 1);
op->addImmediateOperand((unsigned) opCode);
for (auto it = operands.cbegin(); it != operands.cend(); ++it)
op->addIdOperand(*it);
for (auto it = literals.cbegin(); it != literals.cend(); ++it)
op->addImmediateOperand(*it);
module.mapInstruction(op);
constantsTypesGlobals.push_back(std::unique_ptr<Instruction>(op));
// OpSpecConstantOp's using 8 or 16 bit types require the associated capability
if (containsType(typeId, OpTypeInt, 8))
addCapability(CapabilityInt8);
if (containsType(typeId, OpTypeInt, 16))
addCapability(CapabilityInt16);
if (containsType(typeId, OpTypeFloat, 16))
addCapability(CapabilityFloat16);
return op->getResultId();
}
Id Builder::createFunctionCall(spv::Function* function, const std::vector<spv::Id>& args)
{
Instruction* op = new Instruction(getUniqueId(), function->getReturnType(), OpFunctionCall);
op->reserveOperands(args.size() + 1);
op->addIdOperand(function->getId());
for (int a = 0; a < (int)args.size(); ++a)
op->addIdOperand(args[a]);
addInstruction(std::unique_ptr<Instruction>(op));
return op->getResultId();
}
// Comments in header
Id Builder::createRvalueSwizzle(Decoration precision, Id typeId, Id source, const std::vector<unsigned>& channels)
{
if (channels.size() == 1)
return setPrecision(createCompositeExtract(source, typeId, channels.front()), precision);
if (generatingOpCodeForSpecConst) {
std::vector<Id> operands(2);
operands[0] = operands[1] = source;
return setPrecision(createSpecConstantOp(OpVectorShuffle, typeId, operands, channels), precision);
}
Instruction* swizzle = new Instruction(getUniqueId(), typeId, OpVectorShuffle);
assert(isVector(source));
swizzle->reserveOperands(channels.size() + 2);
swizzle->addIdOperand(source);
swizzle->addIdOperand(source);
for (int i = 0; i < (int)channels.size(); ++i)
swizzle->addImmediateOperand(channels[i]);
addInstruction(std::unique_ptr<Instruction>(swizzle));
return setPrecision(swizzle->getResultId(), precision);
}
// Comments in header
Id Builder::createLvalueSwizzle(Id typeId, Id target, Id source, const std::vector<unsigned>& channels)
{
if (channels.size() == 1 && getNumComponents(source) == 1)
return createCompositeInsert(source, target, typeId, channels.front());
Instruction* swizzle = new Instruction(getUniqueId(), typeId, OpVectorShuffle);
assert(isVector(target));
swizzle->reserveOperands(2);
swizzle->addIdOperand(target);
assert(getNumComponents(source) == channels.size());
assert(isVector(source));
swizzle->addIdOperand(source);
// Set up an identity shuffle from the base value to the result value
unsigned int components[4];
int numTargetComponents = getNumComponents(target);
for (int i = 0; i < numTargetComponents; ++i)
components[i] = i;
// Punch in the l-value swizzle
for (int i = 0; i < (int)channels.size(); ++i)
components[channels[i]] = numTargetComponents + i;
// finish the instruction with these components selectors
swizzle->reserveOperands(numTargetComponents);
for (int i = 0; i < numTargetComponents; ++i)
swizzle->addImmediateOperand(components[i]);
addInstruction(std::unique_ptr<Instruction>(swizzle));
return swizzle->getResultId();
}
// Comments in header
void Builder::promoteScalar(Decoration precision, Id& left, Id& right)
{
int direction = getNumComponents(right) - getNumComponents(left);
if (direction > 0)
left = smearScalar(precision, left, makeVectorType(getTypeId(left), getNumComponents(right)));
else if (direction < 0)
right = smearScalar(precision, right, makeVectorType(getTypeId(right), getNumComponents(left)));
return;
}
// Comments in header
Id Builder::smearScalar(Decoration precision, Id scalar, Id vectorType)
{
assert(getNumComponents(scalar) == 1);
assert(getTypeId(scalar) == getScalarTypeId(vectorType));
int numComponents = getNumTypeComponents(vectorType);
if (numComponents == 1)
return scalar;
Instruction* smear = nullptr;
if (generatingOpCodeForSpecConst) {
auto members = std::vector<spv::Id>(numComponents, scalar);
// Sometime even in spec-constant-op mode, the temporary vector created by
// promoting a scalar might not be a spec constant. This should depend on
// the scalar.
// e.g.:
// const vec2 spec_const_result = a_spec_const_vec2 + a_front_end_const_scalar;
// In such cases, the temporary vector created from a_front_end_const_scalar
// is not a spec constant vector, even though the binary operation node is marked
// as 'specConstant' and we are in spec-constant-op mode.
auto result_id = makeCompositeConstant(vectorType, members, isSpecConstant(scalar));
smear = module.getInstruction(result_id);
} else {
bool replicate = useReplicatedComposites && (numComponents > 0);
if (replicate) {
numComponents = 1;
addCapability(spv::CapabilityReplicatedCompositesEXT);
addExtension(spv::E_SPV_EXT_replicated_composites);
}
Op opcode = replicate ? OpCompositeConstructReplicateEXT : OpCompositeConstruct;
smear = new Instruction(getUniqueId(), vectorType, opcode);
smear->reserveOperands(numComponents);
for (int c = 0; c < numComponents; ++c)
smear->addIdOperand(scalar);
addInstruction(std::unique_ptr<Instruction>(smear));
}
return setPrecision(smear->getResultId(), precision);
}
// Comments in header
Id Builder::createBuiltinCall(Id resultType, Id builtins, int entryPoint, const std::vector<Id>& args)
{
Instruction* inst = new Instruction(getUniqueId(), resultType, OpExtInst);
inst->reserveOperands(args.size() + 2);
inst->addIdOperand(builtins);
inst->addImmediateOperand(entryPoint);
for (int arg = 0; arg < (int)args.size(); ++arg)
inst->addIdOperand(args[arg]);
addInstruction(std::unique_ptr<Instruction>(inst));
return inst->getResultId();
}
// Accept all parameters needed to create a texture instruction.
// Create the correct instruction based on the inputs, and make the call.
Id Builder::createTextureCall(Decoration precision, Id resultType, bool sparse, bool fetch, bool proj, bool gather,
bool noImplicitLod, const TextureParameters& parameters, ImageOperandsMask signExtensionMask)
{
std::vector<Id> texArgs;
//
// Set up the fixed arguments
//
bool explicitLod = false;
texArgs.push_back(parameters.sampler);
texArgs.push_back(parameters.coords);
if (parameters.Dref != NoResult)
texArgs.push_back(parameters.Dref);
if (parameters.component != NoResult)
texArgs.push_back(parameters.component);
if (parameters.granularity != NoResult)
texArgs.push_back(parameters.granularity);
if (parameters.coarse != NoResult)
texArgs.push_back(parameters.coarse);
//
// Set up the optional arguments
//
size_t optArgNum = texArgs.size(); // the position of the mask for the optional arguments, if any.
ImageOperandsMask mask = ImageOperandsMaskNone; // the mask operand
if (parameters.bias) {
mask = (ImageOperandsMask)(mask | ImageOperandsBiasMask);
texArgs.push_back(parameters.bias);
}
if (parameters.lod) {
mask = (ImageOperandsMask)(mask | ImageOperandsLodMask);
texArgs.push_back(parameters.lod);
explicitLod = true;
} else if (parameters.gradX) {
mask = (ImageOperandsMask)(mask | ImageOperandsGradMask);
texArgs.push_back(parameters.gradX);
texArgs.push_back(parameters.gradY);
explicitLod = true;
} else if (noImplicitLod && ! fetch && ! gather) {
// have to explicitly use lod of 0 if not allowed to have them be implicit, and
// we would otherwise be about to issue an implicit instruction
mask = (ImageOperandsMask)(mask | ImageOperandsLodMask);
texArgs.push_back(makeFloatConstant(0.0));
explicitLod = true;
}
if (parameters.offset) {
if (isConstant(parameters.offset))
mask = (ImageOperandsMask)(mask | ImageOperandsConstOffsetMask);
else {
addCapability(CapabilityImageGatherExtended);
mask = (ImageOperandsMask)(mask | ImageOperandsOffsetMask);
}
texArgs.push_back(parameters.offset);
}
if (parameters.offsets) {
addCapability(CapabilityImageGatherExtended);
mask = (ImageOperandsMask)(mask | ImageOperandsConstOffsetsMask);
texArgs.push_back(parameters.offsets);
}
if (parameters.sample) {
mask = (ImageOperandsMask)(mask | ImageOperandsSampleMask);
texArgs.push_back(parameters.sample);
}
if (parameters.lodClamp) {
// capability if this bit is used
addCapability(CapabilityMinLod);
mask = (ImageOperandsMask)(mask | ImageOperandsMinLodMask);
texArgs.push_back(parameters.lodClamp);
}
if (parameters.nonprivate) {
mask = mask | ImageOperandsNonPrivateTexelKHRMask;
}
if (parameters.volatil) {
mask = mask | ImageOperandsVolatileTexelKHRMask;
}
mask = mask | signExtensionMask;
// insert the operand for the mask, if any bits were set.
if (mask != ImageOperandsMaskNone)
texArgs.insert(texArgs.begin() + optArgNum, mask);
//
// Set up the instruction
//
Op opCode = OpNop; // All paths below need to set this
if (fetch) {
if (sparse)
opCode = OpImageSparseFetch;
else
opCode = OpImageFetch;
} else if (parameters.granularity && parameters.coarse) {
opCode = OpImageSampleFootprintNV;
} else if (gather) {
if (parameters.Dref)
if (sparse)
opCode = OpImageSparseDrefGather;
else
opCode = OpImageDrefGather;
else
if (sparse)
opCode = OpImageSparseGather;
else
opCode = OpImageGather;
} else if (explicitLod) {
if (parameters.Dref) {
if (proj)
if (sparse)
opCode = OpImageSparseSampleProjDrefExplicitLod;
else
opCode = OpImageSampleProjDrefExplicitLod;
else
if (sparse)
opCode = OpImageSparseSampleDrefExplicitLod;
else
opCode = OpImageSampleDrefExplicitLod;
} else {
if (proj)
if (sparse)
opCode = OpImageSparseSampleProjExplicitLod;
else
opCode = OpImageSampleProjExplicitLod;
else
if (sparse)
opCode = OpImageSparseSampleExplicitLod;
else
opCode = OpImageSampleExplicitLod;
}
} else {
if (parameters.Dref) {
if (proj)
if (sparse)
opCode = OpImageSparseSampleProjDrefImplicitLod;
else
opCode = OpImageSampleProjDrefImplicitLod;
else
if (sparse)
opCode = OpImageSparseSampleDrefImplicitLod;
else
opCode = OpImageSampleDrefImplicitLod;
} else {
if (proj)
if (sparse)
opCode = OpImageSparseSampleProjImplicitLod;
else
opCode = OpImageSampleProjImplicitLod;
else
if (sparse)
opCode = OpImageSparseSampleImplicitLod;
else
opCode = OpImageSampleImplicitLod;
}
}
// See if the result type is expecting a smeared result.
// This happens when a legacy shadow*() call is made, which
// gets a vec4 back instead of a float.
Id smearedType = resultType;
if (! isScalarType(resultType)) {
switch (opCode) {
case OpImageSampleDrefImplicitLod:
case OpImageSampleDrefExplicitLod:
case OpImageSampleProjDrefImplicitLod:
case OpImageSampleProjDrefExplicitLod:
resultType = getScalarTypeId(resultType);
break;
default:
break;
}
}
Id typeId0 = 0;
Id typeId1 = 0;
if (sparse) {
typeId0 = resultType;
typeId1 = getDerefTypeId(parameters.texelOut);
resultType = makeStructResultType(typeId0, typeId1);
}
// Build the SPIR-V instruction
Instruction* textureInst = new Instruction(getUniqueId(), resultType, opCode);
textureInst->reserveOperands(optArgNum + (texArgs.size() - (optArgNum + 1)));
for (size_t op = 0; op < optArgNum; ++op)
textureInst->addIdOperand(texArgs[op]);
if (optArgNum < texArgs.size())
textureInst->addImmediateOperand(texArgs[optArgNum]);
for (size_t op = optArgNum + 1; op < texArgs.size(); ++op)
textureInst->addIdOperand(texArgs[op]);
setPrecision(textureInst->getResultId(), precision);
addInstruction(std::unique_ptr<Instruction>(textureInst));
Id resultId = textureInst->getResultId();
if (sparse) {
// set capability
addCapability(CapabilitySparseResidency);
// Decode the return type that was a special structure
createStore(createCompositeExtract(resultId, typeId1, 1), parameters.texelOut);
resultId = createCompositeExtract(resultId, typeId0, 0);
setPrecision(resultId, precision);
} else {
// When a smear is needed, do it, as per what was computed
// above when resultType was changed to a scalar type.
if (resultType != smearedType)
resultId = smearScalar(precision, resultId, smearedType);
}
return resultId;
}
// Comments in header
Id Builder::createTextureQueryCall(Op opCode, const TextureParameters& parameters, bool isUnsignedResult)
{
// Figure out the result type
Id resultType = 0;
switch (opCode) {
case OpImageQuerySize:
case OpImageQuerySizeLod:
{
int numComponents = 0;
switch (getTypeDimensionality(getImageType(parameters.sampler))) {
case Dim1D:
case DimBuffer:
numComponents = 1;
break;
case Dim2D:
case DimCube:
case DimRect:
case DimSubpassData:
numComponents = 2;
break;
case Dim3D:
numComponents = 3;
break;
default:
assert(0);
break;
}
if (isArrayedImageType(getImageType(parameters.sampler)))
++numComponents;
Id intType = isUnsignedResult ? makeUintType(32) : makeIntType(32);
if (numComponents == 1)
resultType = intType;
else
resultType = makeVectorType(intType, numComponents);
break;
}
case OpImageQueryLod:
resultType = makeVectorType(getScalarTypeId(getTypeId(parameters.coords)), 2);
break;
case OpImageQueryLevels:
case OpImageQuerySamples:
resultType = isUnsignedResult ? makeUintType(32) : makeIntType(32);
break;
default:
assert(0);
break;
}
Instruction* query = new Instruction(getUniqueId(), resultType, opCode);
query->addIdOperand(parameters.sampler);
if (parameters.coords)
query->addIdOperand(parameters.coords);
if (parameters.lod)
query->addIdOperand(parameters.lod);
addInstruction(std::unique_ptr<Instruction>(query));
addCapability(CapabilityImageQuery);
return query->getResultId();
}
// External comments in header.
// Operates recursively to visit the composite's hierarchy.
Id Builder::createCompositeCompare(Decoration precision, Id value1, Id value2, bool equal)
{
Id boolType = makeBoolType();
Id valueType = getTypeId(value1);
Id resultId = NoResult;
int numConstituents = getNumTypeConstituents(valueType);
// Scalars and Vectors
if (isScalarType(valueType) || isVectorType(valueType)) {
assert(valueType == getTypeId(value2));
// These just need a single comparison, just have
// to figure out what it is.
Op op;
switch (getMostBasicTypeClass(valueType)) {
case OpTypeFloat:
op = equal ? OpFOrdEqual : OpFUnordNotEqual;
break;
case OpTypeInt:
default:
op = equal ? OpIEqual : OpINotEqual;
break;
case OpTypeBool:
op = equal ? OpLogicalEqual : OpLogicalNotEqual;
precision = NoPrecision;
break;
}
if (isScalarType(valueType)) {
// scalar
resultId = createBinOp(op, boolType, value1, value2);
} else {
// vector
resultId = createBinOp(op, makeVectorType(boolType, numConstituents), value1, value2);
setPrecision(resultId, precision);
// reduce vector compares...
resultId = createUnaryOp(equal ? OpAll : OpAny, boolType, resultId);
}
return setPrecision(resultId, precision);
}
// Only structs, arrays, and matrices should be left.
// They share in common the reduction operation across their constituents.
assert(isAggregateType(valueType) || isMatrixType(valueType));
// Compare each pair of constituents
for (int constituent = 0; constituent < numConstituents; ++constituent) {
std::vector<unsigned> indexes(1, constituent);
Id constituentType1 = getContainedTypeId(getTypeId(value1), constituent);
Id constituentType2 = getContainedTypeId(getTypeId(value2), constituent);
Id constituent1 = createCompositeExtract(value1, constituentType1, indexes);
Id constituent2 = createCompositeExtract(value2, constituentType2, indexes);
Id subResultId = createCompositeCompare(precision, constituent1, constituent2, equal);
if (constituent == 0)
resultId = subResultId;
else
resultId = setPrecision(createBinOp(equal ? OpLogicalAnd : OpLogicalOr, boolType, resultId, subResultId),
precision);
}
return resultId;
}
// OpCompositeConstruct
Id Builder::createCompositeConstruct(Id typeId, const std::vector<Id>& constituents)
{
assert(isAggregateType(typeId) || (getNumTypeConstituents(typeId) > 1 &&
getNumTypeConstituents(typeId) == constituents.size()));
if (generatingOpCodeForSpecConst) {
// Sometime, even in spec-constant-op mode, the constant composite to be
// constructed may not be a specialization constant.
// e.g.:
// const mat2 m2 = mat2(a_spec_const, a_front_end_const, another_front_end_const, third_front_end_const);
// The first column vector should be a spec constant one, as a_spec_const is a spec constant.
// The second column vector should NOT be spec constant, as it does not contain any spec constants.
// To handle such cases, we check the constituents of the constant vector to determine whether this
// vector should be created as a spec constant.
return makeCompositeConstant(typeId, constituents,
std::any_of(constituents.begin(), constituents.end(),
[&](spv::Id id) { return isSpecConstant(id); }));
}
bool replicate = false;
size_t numConstituents = constituents.size();
if (useReplicatedComposites) {
replicate = numConstituents > 0 &&
std::equal(constituents.begin() + 1, constituents.end(), constituents.begin());
}
if (replicate) {
numConstituents = 1;
addCapability(spv::CapabilityReplicatedCompositesEXT);
addExtension(spv::E_SPV_EXT_replicated_composites);
}
Op opcode = replicate ? OpCompositeConstructReplicateEXT : OpCompositeConstruct;
Instruction* op = new Instruction(getUniqueId(), typeId, opcode);
op->reserveOperands(constituents.size());
for (size_t c = 0; c < numConstituents; ++c)
op->addIdOperand(constituents[c]);
addInstruction(std::unique_ptr<Instruction>(op));
return op->getResultId();
}
// Vector or scalar constructor
Id Builder::createConstructor(Decoration precision, const std::vector<Id>& sources, Id resultTypeId)
{
Id result = NoResult;
unsigned int numTargetComponents = getNumTypeComponents(resultTypeId);
unsigned int targetComponent = 0;
// Special case: when calling a vector constructor with a single scalar
// argument, smear the scalar
if (sources.size() == 1 && isScalar(sources[0]) && numTargetComponents > 1)
return smearScalar(precision, sources[0], resultTypeId);
// Special case: 2 vectors of equal size
if (sources.size() == 1 && isVector(sources[0]) && numTargetComponents == getNumComponents(sources[0])) {
assert(resultTypeId == getTypeId(sources[0]));
return sources[0];
}
// accumulate the arguments for OpCompositeConstruct
std::vector<Id> constituents;
Id scalarTypeId = getScalarTypeId(resultTypeId);
// lambda to store the result of visiting an argument component
const auto latchResult = [&](Id comp) {
if (numTargetComponents > 1)
constituents.push_back(comp);
else
result = comp;
++targetComponent;
};
// lambda to visit a vector argument's components
const auto accumulateVectorConstituents = [&](Id sourceArg) {
unsigned int sourceSize = getNumComponents(sourceArg);
unsigned int sourcesToUse = sourceSize;
if (sourcesToUse + targetComponent > numTargetComponents)
sourcesToUse = numTargetComponents - targetComponent;
for (unsigned int s = 0; s < sourcesToUse; ++s) {
std::vector<unsigned> swiz;
swiz.push_back(s);
latchResult(createRvalueSwizzle(precision, scalarTypeId, sourceArg, swiz));
}
};
// lambda to visit a matrix argument's components
const auto accumulateMatrixConstituents = [&](Id sourceArg) {
unsigned int sourceSize = getNumColumns(sourceArg) * getNumRows(sourceArg);
unsigned int sourcesToUse = sourceSize;
if (sourcesToUse + targetComponent > numTargetComponents)
sourcesToUse = numTargetComponents - targetComponent;
unsigned int col = 0;
unsigned int row = 0;
for (unsigned int s = 0; s < sourcesToUse; ++s) {
if (row >= getNumRows(sourceArg)) {
row = 0;
col++;
}
std::vector<Id> indexes;
indexes.push_back(col);
indexes.push_back(row);
latchResult(createCompositeExtract(sourceArg, scalarTypeId, indexes));
row++;
}
};
// Go through the source arguments, each one could have either
// a single or multiple components to contribute.
for (unsigned int i = 0; i < sources.size(); ++i) {
if (isScalar(sources[i]) || isPointer(sources[i]))
latchResult(sources[i]);
else if (isVector(sources[i]))
accumulateVectorConstituents(sources[i]);
else if (isMatrix(sources[i]))
accumulateMatrixConstituents(sources[i]);
else
assert(0);
if (targetComponent >= numTargetComponents)
break;
}
// If the result is a vector, make it from the gathered constituents.
if (constituents.size() > 0) {
result = createCompositeConstruct(resultTypeId, constituents);
return setPrecision(result, precision);
} else {
// Precision was set when generating this component.
return result;
}
}
// Comments in header
Id Builder::createMatrixConstructor(Decoration precision, const std::vector<Id>& sources, Id resultTypeId)
{
Id componentTypeId = getScalarTypeId(resultTypeId);
unsigned int numCols = getTypeNumColumns(resultTypeId);
unsigned int numRows = getTypeNumRows(resultTypeId);
Instruction* instr = module.getInstruction(componentTypeId);
const unsigned bitCount = instr->getImmediateOperand(0);
// Optimize matrix constructed from a bigger matrix
if (isMatrix(sources[0]) && getNumColumns(sources[0]) >= numCols && getNumRows(sources[0]) >= numRows) {
// To truncate the matrix to a smaller number of rows/columns, we need to:
// 1. For each column, extract the column and truncate it to the required size using shuffle
// 2. Assemble the resulting matrix from all columns
Id matrix = sources[0];
Id columnTypeId = getContainedTypeId(resultTypeId);
Id sourceColumnTypeId = getContainedTypeId(getTypeId(matrix));
std::vector<unsigned> channels;
for (unsigned int row = 0; row < numRows; ++row)
channels.push_back(row);
std::vector<Id> matrixColumns;
for (unsigned int col = 0; col < numCols; ++col) {
std::vector<unsigned> indexes;
indexes.push_back(col);
Id colv = createCompositeExtract(matrix, sourceColumnTypeId, indexes);
setPrecision(colv, precision);
if (numRows != getNumRows(matrix)) {
matrixColumns.push_back(createRvalueSwizzle(precision, columnTypeId, colv, channels));
} else {
matrixColumns.push_back(colv);
}
}
return setPrecision(createCompositeConstruct(resultTypeId, matrixColumns), precision);
}
// Detect a matrix being constructed from a repeated vector of the correct size.
// Create the composite directly from it.
if (sources.size() == numCols && isVector(sources[0]) && getNumComponents(sources[0]) == numRows &&
std::equal(sources.begin() + 1, sources.end(), sources.begin())) {
return setPrecision(createCompositeConstruct(resultTypeId, sources), precision);
}
// Otherwise, will use a two step process
// 1. make a compile-time 2D array of values
// 2. construct a matrix from that array
// Step 1.
// initialize the array to the identity matrix
Id ids[maxMatrixSize][maxMatrixSize];
Id one = (bitCount == 64 ? makeDoubleConstant(1.0) : makeFloatConstant(1.0));
Id zero = (bitCount == 64 ? makeDoubleConstant(0.0) : makeFloatConstant(0.0));
for (int col = 0; col < 4; ++col) {
for (int row = 0; row < 4; ++row) {
if (col == row)
ids[col][row] = one;
else
ids[col][row] = zero;
}
}
// modify components as dictated by the arguments
if (sources.size() == 1 && isScalar(sources[0])) {
// a single scalar; resets the diagonals
for (int col = 0; col < 4; ++col)
ids[col][col] = sources[0];
} else if (isMatrix(sources[0])) {
// constructing from another matrix; copy over the parts that exist in both the argument and constructee
Id matrix = sources[0];
unsigned int minCols = std::min(numCols, getNumColumns(matrix));
unsigned int minRows = std::min(numRows, getNumRows(matrix));
for (unsigned int col = 0; col < minCols; ++col) {
std::vector<unsigned> indexes;
indexes.push_back(col);
for (unsigned int row = 0; row < minRows; ++row) {
indexes.push_back(row);
ids[col][row] = createCompositeExtract(matrix, componentTypeId, indexes);
indexes.pop_back();
setPrecision(ids[col][row], precision);
}
}
} else {
// fill in the matrix in column-major order with whatever argument components are available
unsigned int row = 0;
unsigned int col = 0;
for (unsigned int arg = 0; arg < sources.size() && col < numCols; ++arg) {
Id argComp = sources[arg];
for (unsigned int comp = 0; comp < getNumComponents(sources[arg]); ++comp) {
if (getNumComponents(sources[arg]) > 1) {
argComp = createCompositeExtract(sources[arg], componentTypeId, comp);
setPrecision(argComp, precision);
}
ids[col][row++] = argComp;
if (row == numRows) {
row = 0;
col++;
}
if (col == numCols) {
// If more components are provided than fit the matrix, discard the rest.
break;
}
}
}
}
// Step 2: Construct a matrix from that array.
// First make the column vectors, then make the matrix.
// make the column vectors
Id columnTypeId = getContainedTypeId(resultTypeId);
std::vector<Id> matrixColumns;
for (unsigned int col = 0; col < numCols; ++col) {
std::vector<Id> vectorComponents;
for (unsigned int row = 0; row < numRows; ++row)
vectorComponents.push_back(ids[col][row]);
Id column = createCompositeConstruct(columnTypeId, vectorComponents);
setPrecision(column, precision);
matrixColumns.push_back(column);
}
// make the matrix
return setPrecision(createCompositeConstruct(resultTypeId, matrixColumns), precision);
}
// Comments in header
Builder::If::If(Id cond, unsigned int ctrl, Builder& gb) :
builder(gb),
condition(cond),
control(ctrl),
elseBlock(nullptr)
{
function = &builder.getBuildPoint()->getParent();
// make the blocks, but only put the then-block into the function,
// the else-block and merge-block will be added later, in order, after
// earlier code is emitted
thenBlock = new Block(builder.getUniqueId(), *function);
mergeBlock = new Block(builder.getUniqueId(), *function);
// Save the current block, so that we can add in the flow control split when
// makeEndIf is called.
headerBlock = builder.getBuildPoint();
function->addBlock(thenBlock);
builder.setBuildPoint(thenBlock);
}
// Comments in header
void Builder::If::makeBeginElse()
{
// Close out the "then" by having it jump to the mergeBlock
builder.createBranch(mergeBlock);
// Make the first else block and add it to the function
elseBlock = new Block(builder.getUniqueId(), *function);
function->addBlock(elseBlock);
// Start building the else block
builder.setBuildPoint(elseBlock);
}
// Comments in header
void Builder::If::makeEndIf()
{
// jump to the merge block
builder.createBranch(mergeBlock);
// Go back to the headerBlock and make the flow control split
builder.setBuildPoint(headerBlock);
builder.createSelectionMerge(mergeBlock, control);
if (elseBlock)
builder.createConditionalBranch(condition, thenBlock, elseBlock);
else
builder.createConditionalBranch(condition, thenBlock, mergeBlock);
// add the merge block to the function
function->addBlock(mergeBlock);
builder.setBuildPoint(mergeBlock);
}
// Comments in header
void Builder::makeSwitch(Id selector, unsigned int control, int numSegments, const std::vector<int>& caseValues,
const std::vector<int>& valueIndexToSegment, int defaultSegment,
std::vector<Block*>& segmentBlocks)
{
Function& function = buildPoint->getParent();
// make all the blocks
for (int s = 0; s < numSegments; ++s)
segmentBlocks.push_back(new Block(getUniqueId(), function));
Block* mergeBlock = new Block(getUniqueId(), function);
// make and insert the switch's selection-merge instruction
createSelectionMerge(mergeBlock, control);
// make the switch instruction
Instruction* switchInst = new Instruction(NoResult, NoType, OpSwitch);
switchInst->reserveOperands((caseValues.size() * 2) + 2);
switchInst->addIdOperand(selector);
auto defaultOrMerge = (defaultSegment >= 0) ? segmentBlocks[defaultSegment] : mergeBlock;
switchInst->addIdOperand(defaultOrMerge->getId());
defaultOrMerge->addPredecessor(buildPoint);
for (int i = 0; i < (int)caseValues.size(); ++i) {
switchInst->addImmediateOperand(caseValues[i]);
switchInst->addIdOperand(segmentBlocks[valueIndexToSegment[i]]->getId());
segmentBlocks[valueIndexToSegment[i]]->addPredecessor(buildPoint);
}
addInstruction(std::unique_ptr<Instruction>(switchInst));
// push the merge block
switchMerges.push(mergeBlock);
}
// Comments in header
void Builder::addSwitchBreak()
{
// branch to the top of the merge block stack
createBranch(switchMerges.top());
createAndSetNoPredecessorBlock("post-switch-break");
}
// Comments in header
void Builder::nextSwitchSegment(std::vector<Block*>& segmentBlock, int nextSegment)
{
int lastSegment = nextSegment - 1;
if (lastSegment >= 0) {
// Close out previous segment by jumping, if necessary, to next segment
if (! buildPoint->isTerminated())
createBranch(segmentBlock[nextSegment]);
}
Block* block = segmentBlock[nextSegment];
block->getParent().addBlock(block);
setBuildPoint(block);
}
// Comments in header
void Builder::endSwitch(std::vector<Block*>& /*segmentBlock*/)
{
// Close out previous segment by jumping, if necessary, to next segment
if (! buildPoint->isTerminated())
addSwitchBreak();
switchMerges.top()->getParent().addBlock(switchMerges.top());
setBuildPoint(switchMerges.top());
switchMerges.pop();
}
Block& Builder::makeNewBlock()
{
Function& function = buildPoint->getParent();
auto block = new Block(getUniqueId(), function);
function.addBlock(block);
return *block;
}
Builder::LoopBlocks& Builder::makeNewLoop()
{
// This verbosity is needed to simultaneously get the same behavior
// everywhere (id's in the same order), have a syntax that works
// across lots of versions of C++, have no warnings from pedantic
// compilation modes, and leave the rest of the code alone.
Block& head = makeNewBlock();
Block& body = makeNewBlock();
Block& merge = makeNewBlock();
Block& continue_target = makeNewBlock();
LoopBlocks blocks(head, body, merge, continue_target);
loops.push(blocks);
return loops.top();
}
void Builder::createLoopContinue()
{
createBranch(&loops.top().continue_target);
// Set up a block for dead code.
createAndSetNoPredecessorBlock("post-loop-continue");
}
void Builder::createLoopExit()
{
createBranch(&loops.top().merge);
// Set up a block for dead code.
createAndSetNoPredecessorBlock("post-loop-break");
}
void Builder::closeLoop()
{
loops.pop();
}
void Builder::clearAccessChain()
{
accessChain.base = NoResult;
accessChain.indexChain.clear();
accessChain.instr = NoResult;
accessChain.swizzle.clear();
accessChain.component = NoResult;
accessChain.preSwizzleBaseType = NoType;
accessChain.isRValue = false;
accessChain.coherentFlags.clear();
accessChain.alignment = 0;
}
// Comments in header
void Builder::accessChainPushSwizzle(std::vector<unsigned>& swizzle, Id preSwizzleBaseType,
AccessChain::CoherentFlags coherentFlags, unsigned int alignment)
{
accessChain.coherentFlags |= coherentFlags;
accessChain.alignment |= alignment;
// swizzles can be stacked in GLSL, but simplified to a single
// one here; the base type doesn't change
if (accessChain.preSwizzleBaseType == NoType)
accessChain.preSwizzleBaseType = preSwizzleBaseType;
// if needed, propagate the swizzle for the current access chain
if (accessChain.swizzle.size() > 0) {
std::vector<unsigned> oldSwizzle = accessChain.swizzle;
accessChain.swizzle.resize(0);
for (unsigned int i = 0; i < swizzle.size(); ++i) {
assert(swizzle[i] < oldSwizzle.size());
accessChain.swizzle.push_back(oldSwizzle[swizzle[i]]);
}
} else
accessChain.swizzle = swizzle;
// determine if we need to track this swizzle anymore
simplifyAccessChainSwizzle();
}
// Comments in header
void Builder::accessChainStore(Id rvalue, Decoration nonUniform, spv::MemoryAccessMask memoryAccess, spv::Scope scope, unsigned int alignment)
{
assert(accessChain.isRValue == false);
transferAccessChainSwizzle(true);
// If a swizzle exists and is not full and is not dynamic, then the swizzle will be broken into individual stores.
if (accessChain.swizzle.size() > 0 &&
getNumTypeComponents(getResultingAccessChainType()) != accessChain.swizzle.size() &&
accessChain.component == NoResult) {
for (unsigned int i = 0; i < accessChain.swizzle.size(); ++i) {
accessChain.indexChain.push_back(makeUintConstant(accessChain.swizzle[i]));
accessChain.instr = NoResult;
Id base = collapseAccessChain();
addDecoration(base, nonUniform);
accessChain.indexChain.pop_back();
accessChain.instr = NoResult;
// dynamic component should be gone
assert(accessChain.component == NoResult);
Id source = createCompositeExtract(rvalue, getContainedTypeId(getTypeId(rvalue)), i);
// take LSB of alignment
alignment = alignment & ~(alignment & (alignment-1));
if (getStorageClass(base) == StorageClassPhysicalStorageBufferEXT) {
memoryAccess = (spv::MemoryAccessMask)(memoryAccess | spv::MemoryAccessAlignedMask);
}
createStore(source, base, memoryAccess, scope, alignment);
}
}
else {
Id base = collapseAccessChain();
addDecoration(base, nonUniform);
Id source = rvalue;
// dynamic component should be gone
assert(accessChain.component == NoResult);
// If swizzle still exists, it may be out-of-order, we must load the target vector,
// extract and insert elements to perform writeMask and/or swizzle.
if (accessChain.swizzle.size() > 0) {
Id tempBaseId = createLoad(base, spv::NoPrecision);
source = createLvalueSwizzle(getTypeId(tempBaseId), tempBaseId, source, accessChain.swizzle);
}
// take LSB of alignment
alignment = alignment & ~(alignment & (alignment-1));
if (getStorageClass(base) == StorageClassPhysicalStorageBufferEXT) {
memoryAccess = (spv::MemoryAccessMask)(memoryAccess | spv::MemoryAccessAlignedMask);
}
createStore(source, base, memoryAccess, scope, alignment);
}
}
// Comments in header
Id Builder::accessChainLoad(Decoration precision, Decoration l_nonUniform,
Decoration r_nonUniform, Id resultType, spv::MemoryAccessMask memoryAccess,
spv::Scope scope, unsigned int alignment)
{
Id id;
if (accessChain.isRValue) {
// transfer access chain, but try to stay in registers
transferAccessChainSwizzle(false);
if (accessChain.indexChain.size() > 0) {
Id swizzleBase = accessChain.preSwizzleBaseType != NoType ? accessChain.preSwizzleBaseType : resultType;
// if all the accesses are constants, we can use OpCompositeExtract
std::vector<unsigned> indexes;
bool constant = true;
for (int i = 0; i < (int)accessChain.indexChain.size(); ++i) {
if (isConstantScalar(accessChain.indexChain[i]))
indexes.push_back(getConstantScalar(accessChain.indexChain[i]));
else {
constant = false;
break;
}
}
if (constant) {
id = createCompositeExtract(accessChain.base, swizzleBase, indexes);
setPrecision(id, precision);
} else {
Id lValue = NoResult;
if (spvVersion >= Spv_1_4 && isValidInitializer(accessChain.base)) {
// make a new function variable for this r-value, using an initializer,
// and mark it as NonWritable so that downstream it can be detected as a lookup
// table
lValue = createVariable(NoPrecision, StorageClassFunction, getTypeId(accessChain.base),
"indexable", accessChain.base);
addDecoration(lValue, DecorationNonWritable);
} else {
lValue = createVariable(NoPrecision, StorageClassFunction, getTypeId(accessChain.base),
"indexable");
// store into it
createStore(accessChain.base, lValue);
}
// move base to the new variable
accessChain.base = lValue;
accessChain.isRValue = false;
// load through the access chain
id = createLoad(collapseAccessChain(), precision);
}
} else
id = accessChain.base; // no precision, it was set when this was defined
} else {
transferAccessChainSwizzle(true);
// take LSB of alignment
alignment = alignment & ~(alignment & (alignment-1));
if (getStorageClass(accessChain.base) == StorageClassPhysicalStorageBufferEXT) {
memoryAccess = (spv::MemoryAccessMask)(memoryAccess | spv::MemoryAccessAlignedMask);
}
// load through the access chain
id = collapseAccessChain();
// Apply nonuniform both to the access chain and the loaded value.
// Buffer accesses need the access chain decorated, and this is where
// loaded image types get decorated. TODO: This should maybe move to
// createImageTextureFunctionCall.
addDecoration(id, l_nonUniform);
id = createLoad(id, precision, memoryAccess, scope, alignment);
addDecoration(id, r_nonUniform);
}
// Done, unless there are swizzles to do
if (accessChain.swizzle.size() == 0 && accessChain.component == NoResult)
return id;
// Do remaining swizzling
// Do the basic swizzle
if (accessChain.swizzle.size() > 0) {
Id swizzledType = getScalarTypeId(getTypeId(id));
if (accessChain.swizzle.size() > 1)
swizzledType = makeVectorType(swizzledType, (int)accessChain.swizzle.size());
id = createRvalueSwizzle(precision, swizzledType, id, accessChain.swizzle);
}
// Do the dynamic component
if (accessChain.component != NoResult)
id = setPrecision(createVectorExtractDynamic(id, resultType, accessChain.component), precision);
addDecoration(id, r_nonUniform);
return id;
}
Id Builder::accessChainGetLValue()
{
assert(accessChain.isRValue == false);
transferAccessChainSwizzle(true);
Id lvalue = collapseAccessChain();
// If swizzle exists, it is out-of-order or not full, we must load the target vector,
// extract and insert elements to perform writeMask and/or swizzle. This does not
// go with getting a direct l-value pointer.
assert(accessChain.swizzle.size() == 0);
assert(accessChain.component == NoResult);
return lvalue;
}
// comment in header
Id Builder::accessChainGetInferredType()
{
// anything to operate on?
if (accessChain.base == NoResult)
return NoType;
Id type = getTypeId(accessChain.base);
// do initial dereference
if (! accessChain.isRValue)
type = getContainedTypeId(type);
// dereference each index
for (auto it = accessChain.indexChain.cbegin(); it != accessChain.indexChain.cend(); ++it) {
if (isStructType(type))
type = getContainedTypeId(type, getConstantScalar(*it));
else
type = getContainedTypeId(type);
}
// dereference swizzle
if (accessChain.swizzle.size() == 1)
type = getContainedTypeId(type);
else if (accessChain.swizzle.size() > 1)
type = makeVectorType(getContainedTypeId(type), (int)accessChain.swizzle.size());
// dereference component selection
if (accessChain.component)
type = getContainedTypeId(type);
return type;
}
void Builder::dump(std::vector<unsigned int>& out) const
{
// Header, before first instructions:
out.push_back(MagicNumber);
out.push_back(spvVersion);
out.push_back(builderNumber);
out.push_back(uniqueId + 1);
out.push_back(0);
// Capabilities
for (auto it = capabilities.cbegin(); it != capabilities.cend(); ++it) {
Instruction capInst(0, 0, OpCapability);
capInst.addImmediateOperand(*it);
capInst.dump(out);
}
for (auto it = extensions.cbegin(); it != extensions.cend(); ++it) {
Instruction extInst(0, 0, OpExtension);
extInst.addStringOperand(it->c_str());
extInst.dump(out);
}
dumpInstructions(out, imports);
Instruction memInst(0, 0, OpMemoryModel);
memInst.addImmediateOperand(addressModel);
memInst.addImmediateOperand(memoryModel);
memInst.dump(out);
// Instructions saved up while building:
dumpInstructions(out, entryPoints);
dumpInstructions(out, executionModes);
// Debug instructions
dumpInstructions(out, strings);
dumpSourceInstructions(out);
for (int e = 0; e < (int)sourceExtensions.size(); ++e) {
Instruction sourceExtInst(0, 0, OpSourceExtension);
sourceExtInst.addStringOperand(sourceExtensions[e]);
sourceExtInst.dump(out);
}
dumpInstructions(out, names);
dumpModuleProcesses(out);
// Annotation instructions
dumpInstructions(out, decorations);
dumpInstructions(out, constantsTypesGlobals);
dumpInstructions(out, externals);
// The functions
module.dump(out);
}
//
// Protected methods.
//
// Turn the described access chain in 'accessChain' into an instruction(s)
// computing its address. This *cannot* include complex swizzles, which must
// be handled after this is called.
//
// Can generate code.
Id Builder::collapseAccessChain()
{
assert(accessChain.isRValue == false);
// did we already emit an access chain for this?
if (accessChain.instr != NoResult)
return accessChain.instr;
// If we have a dynamic component, we can still transfer
// that into a final operand to the access chain. We need to remap the
// dynamic component through the swizzle to get a new dynamic component to
// update.
//
// This was not done in transferAccessChainSwizzle() because it might
// generate code.
remapDynamicSwizzle();
if (accessChain.component != NoResult) {
// transfer the dynamic component to the access chain
accessChain.indexChain.push_back(accessChain.component);
accessChain.component = NoResult;
}
// note that non-trivial swizzling is left pending
// do we have an access chain?
if (accessChain.indexChain.size() == 0)
return accessChain.base;
// emit the access chain
StorageClass storageClass = (StorageClass)module.getStorageClass(getTypeId(accessChain.base));
accessChain.instr = createAccessChain(storageClass, accessChain.base, accessChain.indexChain);
return accessChain.instr;
}
// For a dynamic component selection of a swizzle.
//
// Turn the swizzle and dynamic component into just a dynamic component.
//
// Generates code.
void Builder::remapDynamicSwizzle()
{
// do we have a swizzle to remap a dynamic component through?
if (accessChain.component != NoResult && accessChain.swizzle.size() > 1) {
// build a vector of the swizzle for the component to map into
std::vector<Id> components;
for (int c = 0; c < (int)accessChain.swizzle.size(); ++c)
components.push_back(makeUintConstant(accessChain.swizzle[c]));
Id mapType = makeVectorType(makeUintType(32), (int)accessChain.swizzle.size());
Id map = makeCompositeConstant(mapType, components);
// use it
accessChain.component = createVectorExtractDynamic(map, makeUintType(32), accessChain.component);
accessChain.swizzle.clear();
}
}
// clear out swizzle if it is redundant, that is reselecting the same components
// that would be present without the swizzle.
void Builder::simplifyAccessChainSwizzle()
{
// If the swizzle has fewer components than the vector, it is subsetting, and must stay
// to preserve that fact.
if (getNumTypeComponents(accessChain.preSwizzleBaseType) > accessChain.swizzle.size())
return;
// if components are out of order, it is a swizzle
for (unsigned int i = 0; i < accessChain.swizzle.size(); ++i) {
if (i != accessChain.swizzle[i])
return;
}
// otherwise, there is no need to track this swizzle
accessChain.swizzle.clear();
if (accessChain.component == NoResult)
accessChain.preSwizzleBaseType = NoType;
}
// To the extent any swizzling can become part of the chain
// of accesses instead of a post operation, make it so.
// If 'dynamic' is true, include transferring the dynamic component,
// otherwise, leave it pending.
//
// Does not generate code. just updates the access chain.
void Builder::transferAccessChainSwizzle(bool dynamic)
{
// non existent?
if (accessChain.swizzle.size() == 0 && accessChain.component == NoResult)
return;
// too complex?
// (this requires either a swizzle, or generating code for a dynamic component)
if (accessChain.swizzle.size() > 1)
return;
// single component, either in the swizzle and/or dynamic component
if (accessChain.swizzle.size() == 1) {
assert(accessChain.component == NoResult);
// handle static component selection
accessChain.indexChain.push_back(makeUintConstant(accessChain.swizzle.front()));
accessChain.swizzle.clear();
accessChain.preSwizzleBaseType = NoType;
} else if (dynamic && accessChain.component != NoResult) {
assert(accessChain.swizzle.size() == 0);
// handle dynamic component
accessChain.indexChain.push_back(accessChain.component);
accessChain.preSwizzleBaseType = NoType;
accessChain.component = NoResult;
}
}
// Utility method for creating a new block and setting the insert point to
// be in it. This is useful for flow-control operations that need a "dummy"
// block proceeding them (e.g. instructions after a discard, etc).
void Builder::createAndSetNoPredecessorBlock(const char* /*name*/)
{
Block* block = new Block(getUniqueId(), buildPoint->getParent());
block->setUnreachable();
buildPoint->getParent().addBlock(block);
setBuildPoint(block);
// if (name)
// addName(block->getId(), name);
}
// Comments in header
void Builder::createBranch(Block* block)
{
Instruction* branch = new Instruction(OpBranch);
branch->addIdOperand(block->getId());
addInstruction(std::unique_ptr<Instruction>(branch));
block->addPredecessor(buildPoint);
}
void Builder::createSelectionMerge(Block* mergeBlock, unsigned int control)
{
Instruction* merge = new Instruction(OpSelectionMerge);
merge->reserveOperands(2);
merge->addIdOperand(mergeBlock->getId());
merge->addImmediateOperand(control);
addInstruction(std::unique_ptr<Instruction>(merge));
}
void Builder::createLoopMerge(Block* mergeBlock, Block* continueBlock, unsigned int control,
const std::vector<unsigned int>& operands)
{
Instruction* merge = new Instruction(OpLoopMerge);
merge->reserveOperands(operands.size() + 3);
merge->addIdOperand(mergeBlock->getId());
merge->addIdOperand(continueBlock->getId());
merge->addImmediateOperand(control);
for (int op = 0; op < (int)operands.size(); ++op)
merge->addImmediateOperand(operands[op]);
addInstruction(std::unique_ptr<Instruction>(merge));
}
void Builder::createConditionalBranch(Id condition, Block* thenBlock, Block* elseBlock)
{
Instruction* branch = new Instruction(OpBranchConditional);
branch->reserveOperands(3);
branch->addIdOperand(condition);
branch->addIdOperand(thenBlock->getId());
branch->addIdOperand(elseBlock->getId());
addInstruction(std::unique_ptr<Instruction>(branch));
thenBlock->addPredecessor(buildPoint);
elseBlock->addPredecessor(buildPoint);
}
// OpSource
// [OpSourceContinued]
// ...
void Builder::dumpSourceInstructions(const spv::Id fileId, const std::string& text,
std::vector<unsigned int>& out) const
{
const int maxWordCount = 0xFFFF;
const int opSourceWordCount = 4;
const int nonNullBytesPerInstruction = 4 * (maxWordCount - opSourceWordCount) - 1;
if (sourceLang != SourceLanguageUnknown) {
// OpSource Language Version File Source
Instruction sourceInst(NoResult, NoType, OpSource);
sourceInst.reserveOperands(3);
sourceInst.addImmediateOperand(sourceLang);
sourceInst.addImmediateOperand(sourceVersion);
// File operand
if (fileId != NoResult) {
sourceInst.addIdOperand(fileId);
// Source operand
if (text.size() > 0) {
int nextByte = 0;
std::string subString;
while ((int)text.size() - nextByte > 0) {
subString = text.substr(nextByte, nonNullBytesPerInstruction);
if (nextByte == 0) {
// OpSource
sourceInst.addStringOperand(subString.c_str());
sourceInst.dump(out);
} else {
// OpSourcContinued
Instruction sourceContinuedInst(OpSourceContinued);
sourceContinuedInst.addStringOperand(subString.c_str());
sourceContinuedInst.dump(out);
}
nextByte += nonNullBytesPerInstruction;
}
} else
sourceInst.dump(out);
} else
sourceInst.dump(out);
}
}
// Dump an OpSource[Continued] sequence for the source and every include file
void Builder::dumpSourceInstructions(std::vector<unsigned int>& out) const
{
if (emitNonSemanticShaderDebugInfo) return;
dumpSourceInstructions(mainFileId, sourceText, out);
for (auto iItr = includeFiles.begin(); iItr != includeFiles.end(); ++iItr)
dumpSourceInstructions(iItr->first, *iItr->second, out);
}
void Builder::dumpInstructions(std::vector<unsigned int>& out,
const std::vector<std::unique_ptr<Instruction> >& instructions) const
{
for (int i = 0; i < (int)instructions.size(); ++i) {
instructions[i]->dump(out);
}
}
void Builder::dumpModuleProcesses(std::vector<unsigned int>& out) const
{
for (int i = 0; i < (int)moduleProcesses.size(); ++i) {
Instruction moduleProcessed(OpModuleProcessed);
moduleProcessed.addStringOperand(moduleProcesses[i]);
moduleProcessed.dump(out);
}
}
} // end spv namespace
|
0 | repos/glslang.zig/glslang | repos/glslang.zig/glslang/SPIRV/GlslangToSpv.cpp | //
// Copyright (C) 2014-2016 LunarG, Inc.
// Copyright (C) 2015-2020 Google, Inc.
// Copyright (C) 2017, 2022-2024 Arm Limited.
// Modifications Copyright (C) 2020 Advanced Micro Devices, Inc. All rights reserved.
//
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
//
// Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
//
// Redistributions in binary form must reproduce the above
// copyright notice, this list of conditions and the following
// disclaimer in the documentation and/or other materials provided
// with the distribution.
//
// Neither the name of 3Dlabs Inc. Ltd. nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
// "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
// LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
// FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
// COPYRIGHT HOLDERS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
// INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
// BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
// LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
// CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
// LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
// ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
// POSSIBILITY OF SUCH DAMAGE.
//
// Visit the nodes in the glslang intermediate tree representation to
// translate them to SPIR-V.
//
#include "spirv.hpp"
#include "GlslangToSpv.h"
#include "SpvBuilder.h"
#include "SpvTools.h"
namespace spv {
#include "GLSL.std.450.h"
#include "GLSL.ext.KHR.h"
#include "GLSL.ext.EXT.h"
#include "GLSL.ext.AMD.h"
#include "GLSL.ext.NV.h"
#include "GLSL.ext.ARM.h"
#include "GLSL.ext.QCOM.h"
#include "NonSemanticDebugPrintf.h"
}
// Glslang includes
#include "../glslang/MachineIndependent/localintermediate.h"
#include "../glslang/MachineIndependent/SymbolTable.h"
#include "../glslang/Include/Common.h"
// Build-time generated includes
#include "glslang/build_info.h"
#include <fstream>
#include <iomanip>
#include <list>
#include <map>
#include <optional>
#include <stack>
#include <string>
#include <vector>
namespace {
namespace {
class SpecConstantOpModeGuard {
public:
SpecConstantOpModeGuard(spv::Builder* builder)
: builder_(builder) {
previous_flag_ = builder->isInSpecConstCodeGenMode();
}
~SpecConstantOpModeGuard() {
previous_flag_ ? builder_->setToSpecConstCodeGenMode()
: builder_->setToNormalCodeGenMode();
}
void turnOnSpecConstantOpMode() {
builder_->setToSpecConstCodeGenMode();
}
private:
spv::Builder* builder_;
bool previous_flag_;
};
struct OpDecorations {
public:
OpDecorations(spv::Decoration precision, spv::Decoration noContraction, spv::Decoration nonUniform) :
precision(precision)
,
noContraction(noContraction),
nonUniform(nonUniform)
{ }
spv::Decoration precision;
void addNoContraction(spv::Builder& builder, spv::Id t) { builder.addDecoration(t, noContraction); }
void addNonUniform(spv::Builder& builder, spv::Id t) { builder.addDecoration(t, nonUniform); }
protected:
spv::Decoration noContraction;
spv::Decoration nonUniform;
};
} // namespace
//
// The main holder of information for translating glslang to SPIR-V.
//
// Derives from the AST walking base class.
//
class TGlslangToSpvTraverser : public glslang::TIntermTraverser {
public:
TGlslangToSpvTraverser(unsigned int spvVersion, const glslang::TIntermediate*, spv::SpvBuildLogger* logger,
glslang::SpvOptions& options);
virtual ~TGlslangToSpvTraverser() { }
bool visitAggregate(glslang::TVisit, glslang::TIntermAggregate*);
bool visitBinary(glslang::TVisit, glslang::TIntermBinary*);
void visitConstantUnion(glslang::TIntermConstantUnion*);
bool visitSelection(glslang::TVisit, glslang::TIntermSelection*);
bool visitSwitch(glslang::TVisit, glslang::TIntermSwitch*);
void visitSymbol(glslang::TIntermSymbol* symbol);
bool visitUnary(glslang::TVisit, glslang::TIntermUnary*);
bool visitLoop(glslang::TVisit, glslang::TIntermLoop*);
bool visitBranch(glslang::TVisit visit, glslang::TIntermBranch*);
void finishSpv(bool compileOnly);
void dumpSpv(std::vector<unsigned int>& out);
protected:
TGlslangToSpvTraverser(TGlslangToSpvTraverser&);
TGlslangToSpvTraverser& operator=(TGlslangToSpvTraverser&);
spv::Decoration TranslateInterpolationDecoration(const glslang::TQualifier& qualifier);
spv::Decoration TranslateAuxiliaryStorageDecoration(const glslang::TQualifier& qualifier);
spv::Decoration TranslateNonUniformDecoration(const glslang::TQualifier& qualifier);
spv::Decoration TranslateNonUniformDecoration(const spv::Builder::AccessChain::CoherentFlags& coherentFlags);
spv::Builder::AccessChain::CoherentFlags TranslateCoherent(const glslang::TType& type);
spv::MemoryAccessMask TranslateMemoryAccess(const spv::Builder::AccessChain::CoherentFlags &coherentFlags);
spv::ImageOperandsMask TranslateImageOperands(const spv::Builder::AccessChain::CoherentFlags &coherentFlags);
spv::Scope TranslateMemoryScope(const spv::Builder::AccessChain::CoherentFlags &coherentFlags);
spv::BuiltIn TranslateBuiltInDecoration(glslang::TBuiltInVariable, bool memberDeclaration);
spv::ImageFormat TranslateImageFormat(const glslang::TType& type);
spv::SelectionControlMask TranslateSelectionControl(const glslang::TIntermSelection&) const;
spv::SelectionControlMask TranslateSwitchControl(const glslang::TIntermSwitch&) const;
spv::LoopControlMask TranslateLoopControl(const glslang::TIntermLoop&, std::vector<unsigned int>& operands) const;
spv::StorageClass TranslateStorageClass(const glslang::TType&);
void TranslateLiterals(const glslang::TVector<const glslang::TIntermConstantUnion*>&, std::vector<unsigned>&) const;
void addIndirectionIndexCapabilities(const glslang::TType& baseType, const glslang::TType& indexType);
spv::Id createSpvVariable(const glslang::TIntermSymbol*, spv::Id forcedType);
spv::Id getSampledType(const glslang::TSampler&);
spv::Id getInvertedSwizzleType(const glslang::TIntermTyped&);
spv::Id createInvertedSwizzle(spv::Decoration precision, const glslang::TIntermTyped&, spv::Id parentResult);
void convertSwizzle(const glslang::TIntermAggregate&, std::vector<unsigned>& swizzle);
spv::Id convertGlslangToSpvType(const glslang::TType& type, bool forwardReferenceOnly = false);
spv::Id convertGlslangToSpvType(const glslang::TType& type, glslang::TLayoutPacking, const glslang::TQualifier&,
bool lastBufferBlockMember, bool forwardReferenceOnly = false);
void applySpirvDecorate(const glslang::TType& type, spv::Id id, std::optional<int> member);
bool filterMember(const glslang::TType& member);
spv::Id convertGlslangStructToSpvType(const glslang::TType&, const glslang::TTypeList* glslangStruct,
glslang::TLayoutPacking, const glslang::TQualifier&);
spv::LinkageType convertGlslangLinkageToSpv(glslang::TLinkType glslangLinkType);
void decorateStructType(const glslang::TType&, const glslang::TTypeList* glslangStruct, glslang::TLayoutPacking,
const glslang::TQualifier&, spv::Id, const std::vector<spv::Id>& spvMembers);
spv::Id makeArraySizeId(const glslang::TArraySizes&, int dim, bool allowZero = false);
spv::Id accessChainLoad(const glslang::TType& type);
void accessChainStore(const glslang::TType& type, spv::Id rvalue);
void multiTypeStore(const glslang::TType&, spv::Id rValue);
spv::Id convertLoadedBoolInUniformToUint(const glslang::TType& type, spv::Id nominalTypeId, spv::Id loadedId);
glslang::TLayoutPacking getExplicitLayout(const glslang::TType& type) const;
int getArrayStride(const glslang::TType& arrayType, glslang::TLayoutPacking, glslang::TLayoutMatrix);
int getMatrixStride(const glslang::TType& matrixType, glslang::TLayoutPacking, glslang::TLayoutMatrix);
void updateMemberOffset(const glslang::TType& structType, const glslang::TType& memberType, int& currentOffset,
int& nextOffset, glslang::TLayoutPacking, glslang::TLayoutMatrix);
void declareUseOfStructMember(const glslang::TTypeList& members, int glslangMember);
bool isShaderEntryPoint(const glslang::TIntermAggregate* node);
bool writableParam(glslang::TStorageQualifier) const;
bool originalParam(glslang::TStorageQualifier, const glslang::TType&, bool implicitThisParam);
void makeFunctions(const glslang::TIntermSequence&);
void makeGlobalInitializers(const glslang::TIntermSequence&);
void collectRayTracingLinkerObjects();
void visitFunctions(const glslang::TIntermSequence&);
void handleFunctionEntry(const glslang::TIntermAggregate* node);
void translateArguments(const glslang::TIntermAggregate& node, std::vector<spv::Id>& arguments,
spv::Builder::AccessChain::CoherentFlags &lvalueCoherentFlags);
void translateArguments(glslang::TIntermUnary& node, std::vector<spv::Id>& arguments);
spv::Id createImageTextureFunctionCall(glslang::TIntermOperator* node);
spv::Id handleUserFunctionCall(const glslang::TIntermAggregate*);
spv::Id createBinaryOperation(glslang::TOperator op, OpDecorations&, spv::Id typeId, spv::Id left, spv::Id right,
glslang::TBasicType typeProxy, bool reduceComparison = true);
spv::Id createBinaryMatrixOperation(spv::Op, OpDecorations&, spv::Id typeId, spv::Id left, spv::Id right);
spv::Id createUnaryOperation(glslang::TOperator op, OpDecorations&, spv::Id typeId, spv::Id operand,
glslang::TBasicType typeProxy,
const spv::Builder::AccessChain::CoherentFlags &lvalueCoherentFlags,
const glslang::TType &opType);
spv::Id createUnaryMatrixOperation(spv::Op op, OpDecorations&, spv::Id typeId, spv::Id operand,
glslang::TBasicType typeProxy);
spv::Id createConversion(glslang::TOperator op, OpDecorations&, spv::Id destTypeId, spv::Id operand,
glslang::TBasicType typeProxy);
spv::Id createIntWidthConversion(glslang::TOperator op, spv::Id operand, int vectorSize, spv::Id destType);
spv::Id makeSmearedConstant(spv::Id constant, int vectorSize);
spv::Id createAtomicOperation(glslang::TOperator op, spv::Decoration precision, spv::Id typeId,
std::vector<spv::Id>& operands, glslang::TBasicType typeProxy,
const spv::Builder::AccessChain::CoherentFlags &lvalueCoherentFlags,
const glslang::TType &opType);
spv::Id createInvocationsOperation(glslang::TOperator op, spv::Id typeId, std::vector<spv::Id>& operands,
glslang::TBasicType typeProxy);
spv::Id CreateInvocationsVectorOperation(spv::Op op, spv::GroupOperation groupOperation,
spv::Id typeId, std::vector<spv::Id>& operands);
spv::Id createSubgroupOperation(glslang::TOperator op, spv::Id typeId, std::vector<spv::Id>& operands,
glslang::TBasicType typeProxy);
spv::Id createMiscOperation(glslang::TOperator op, spv::Decoration precision, spv::Id typeId,
std::vector<spv::Id>& operands, glslang::TBasicType typeProxy);
spv::Id createNoArgOperation(glslang::TOperator op, spv::Decoration precision, spv::Id typeId);
spv::Id getSymbolId(const glslang::TIntermSymbol* node);
void addMeshNVDecoration(spv::Id id, int member, const glslang::TQualifier & qualifier);
bool hasQCOMImageProceessingDecoration(spv::Id id, spv::Decoration decor);
void addImageProcessingQCOMDecoration(spv::Id id, spv::Decoration decor);
void addImageProcessing2QCOMDecoration(spv::Id id, bool isForGather);
spv::Id createSpvConstant(const glslang::TIntermTyped&);
spv::Id createSpvConstantFromConstUnionArray(const glslang::TType& type, const glslang::TConstUnionArray&,
int& nextConst, bool specConstant);
bool isTrivialLeaf(const glslang::TIntermTyped* node);
bool isTrivial(const glslang::TIntermTyped* node);
spv::Id createShortCircuit(glslang::TOperator, glslang::TIntermTyped& left, glslang::TIntermTyped& right);
spv::Id getExtBuiltins(const char* name);
std::pair<spv::Id, spv::Id> getForcedType(glslang::TBuiltInVariable builtIn, const glslang::TType&);
spv::Id translateForcedType(spv::Id object);
spv::Id createCompositeConstruct(spv::Id typeId, std::vector<spv::Id> constituents);
glslang::SpvOptions& options;
spv::Function* shaderEntry;
spv::Function* currentFunction;
spv::Instruction* entryPoint;
int sequenceDepth;
spv::SpvBuildLogger* logger;
// There is a 1:1 mapping between a spv builder and a module; this is thread safe
spv::Builder builder;
bool inEntryPoint;
bool entryPointTerminated;
bool linkageOnly; // true when visiting the set of objects in the AST present only for
// establishing interface, whether or not they were statically used
std::set<spv::Id> iOSet; // all input/output variables from either static use or declaration of interface
const glslang::TIntermediate* glslangIntermediate;
bool nanMinMaxClamp; // true if use NMin/NMax/NClamp instead of FMin/FMax/FClamp
spv::Id stdBuiltins;
spv::Id nonSemanticDebugPrintf;
std::unordered_map<std::string, spv::Id> extBuiltinMap;
std::unordered_map<long long, spv::Id> symbolValues;
std::unordered_map<uint32_t, spv::Id> builtInVariableIds;
std::unordered_set<long long> rValueParameters; // set of formal function parameters passed as rValues,
// rather than a pointer
std::unordered_map<std::string, spv::Function*> functionMap;
std::unordered_map<const glslang::TTypeList*, spv::Id> structMap[glslang::ElpCount][glslang::ElmCount];
// for mapping glslang block indices to spv indices (e.g., due to hidden members):
std::unordered_map<long long, std::vector<int>> memberRemapper;
// for mapping glslang symbol struct to symbol Id
std::unordered_map<const glslang::TTypeList*, long long> glslangTypeToIdMap;
std::stack<bool> breakForLoop; // false means break for switch
std::unordered_map<std::string, const glslang::TIntermSymbol*> counterOriginator;
// Map pointee types for EbtReference to their forward pointers
std::map<const glslang::TType *, spv::Id> forwardPointers;
// Type forcing, for when SPIR-V wants a different type than the AST,
// requiring local translation to and from SPIR-V type on every access.
// Maps <builtin-variable-id -> AST-required-type-id>
std::unordered_map<spv::Id, spv::Id> forceType;
// Used by Task shader while generating opearnds for OpEmitMeshTasksEXT
spv::Id taskPayloadID;
// Used later for generating OpTraceKHR/OpExecuteCallableKHR/OpHitObjectRecordHit*/OpHitObjectGetShaderBindingTableData
std::unordered_map<unsigned int, glslang::TIntermSymbol *> locationToSymbol[4];
std::unordered_map<spv::Id, std::vector<spv::Decoration> > idToQCOMDecorations;
};
//
// Helper functions for translating glslang representations to SPIR-V enumerants.
//
// Translate glslang profile to SPIR-V source language.
spv::SourceLanguage TranslateSourceLanguage(glslang::EShSource source, EProfile profile)
{
switch (source) {
case glslang::EShSourceGlsl:
switch (profile) {
case ENoProfile:
case ECoreProfile:
case ECompatibilityProfile:
return spv::SourceLanguageGLSL;
case EEsProfile:
return spv::SourceLanguageESSL;
default:
return spv::SourceLanguageUnknown;
}
case glslang::EShSourceHlsl:
return spv::SourceLanguageHLSL;
default:
return spv::SourceLanguageUnknown;
}
}
// Translate glslang language (stage) to SPIR-V execution model.
spv::ExecutionModel TranslateExecutionModel(EShLanguage stage, bool isMeshShaderEXT = false)
{
switch (stage) {
case EShLangVertex: return spv::ExecutionModelVertex;
case EShLangFragment: return spv::ExecutionModelFragment;
case EShLangCompute: return spv::ExecutionModelGLCompute;
case EShLangTessControl: return spv::ExecutionModelTessellationControl;
case EShLangTessEvaluation: return spv::ExecutionModelTessellationEvaluation;
case EShLangGeometry: return spv::ExecutionModelGeometry;
case EShLangRayGen: return spv::ExecutionModelRayGenerationKHR;
case EShLangIntersect: return spv::ExecutionModelIntersectionKHR;
case EShLangAnyHit: return spv::ExecutionModelAnyHitKHR;
case EShLangClosestHit: return spv::ExecutionModelClosestHitKHR;
case EShLangMiss: return spv::ExecutionModelMissKHR;
case EShLangCallable: return spv::ExecutionModelCallableKHR;
case EShLangTask: return (isMeshShaderEXT)? spv::ExecutionModelTaskEXT : spv::ExecutionModelTaskNV;
case EShLangMesh: return (isMeshShaderEXT)? spv::ExecutionModelMeshEXT: spv::ExecutionModelMeshNV;
default:
assert(0);
return spv::ExecutionModelFragment;
}
}
// Translate glslang sampler type to SPIR-V dimensionality.
spv::Dim TranslateDimensionality(const glslang::TSampler& sampler)
{
switch (sampler.dim) {
case glslang::Esd1D: return spv::Dim1D;
case glslang::Esd2D: return spv::Dim2D;
case glslang::Esd3D: return spv::Dim3D;
case glslang::EsdCube: return spv::DimCube;
case glslang::EsdRect: return spv::DimRect;
case glslang::EsdBuffer: return spv::DimBuffer;
case glslang::EsdSubpass: return spv::DimSubpassData;
case glslang::EsdAttachmentEXT: return spv::DimTileImageDataEXT;
default:
assert(0);
return spv::Dim2D;
}
}
// Translate glslang precision to SPIR-V precision decorations.
spv::Decoration TranslatePrecisionDecoration(glslang::TPrecisionQualifier glslangPrecision)
{
switch (glslangPrecision) {
case glslang::EpqLow: return spv::DecorationRelaxedPrecision;
case glslang::EpqMedium: return spv::DecorationRelaxedPrecision;
default:
return spv::NoPrecision;
}
}
// Translate glslang type to SPIR-V precision decorations.
spv::Decoration TranslatePrecisionDecoration(const glslang::TType& type)
{
return TranslatePrecisionDecoration(type.getQualifier().precision);
}
// Translate glslang type to SPIR-V block decorations.
spv::Decoration TranslateBlockDecoration(const glslang::TStorageQualifier storage, bool useStorageBuffer)
{
switch (storage) {
case glslang::EvqUniform: return spv::DecorationBlock;
case glslang::EvqBuffer: return useStorageBuffer ? spv::DecorationBlock : spv::DecorationBufferBlock;
case glslang::EvqVaryingIn: return spv::DecorationBlock;
case glslang::EvqVaryingOut: return spv::DecorationBlock;
case glslang::EvqShared: return spv::DecorationBlock;
case glslang::EvqPayload: return spv::DecorationBlock;
case glslang::EvqPayloadIn: return spv::DecorationBlock;
case glslang::EvqHitAttr: return spv::DecorationBlock;
case glslang::EvqCallableData: return spv::DecorationBlock;
case glslang::EvqCallableDataIn: return spv::DecorationBlock;
case glslang::EvqHitObjectAttrNV: return spv::DecorationBlock;
default:
assert(0);
break;
}
return spv::DecorationMax;
}
// Translate glslang type to SPIR-V memory decorations.
void TranslateMemoryDecoration(const glslang::TQualifier& qualifier, std::vector<spv::Decoration>& memory,
bool useVulkanMemoryModel)
{
if (!useVulkanMemoryModel) {
if (qualifier.isVolatile()) {
memory.push_back(spv::DecorationVolatile);
memory.push_back(spv::DecorationCoherent);
} else if (qualifier.isCoherent()) {
memory.push_back(spv::DecorationCoherent);
}
}
if (qualifier.isRestrict())
memory.push_back(spv::DecorationRestrict);
if (qualifier.isReadOnly())
memory.push_back(spv::DecorationNonWritable);
if (qualifier.isWriteOnly())
memory.push_back(spv::DecorationNonReadable);
}
// Translate glslang type to SPIR-V layout decorations.
spv::Decoration TranslateLayoutDecoration(const glslang::TType& type, glslang::TLayoutMatrix matrixLayout)
{
if (type.isMatrix()) {
switch (matrixLayout) {
case glslang::ElmRowMajor:
return spv::DecorationRowMajor;
case glslang::ElmColumnMajor:
return spv::DecorationColMajor;
default:
// opaque layouts don't need a majorness
return spv::DecorationMax;
}
} else {
switch (type.getBasicType()) {
default:
return spv::DecorationMax;
break;
case glslang::EbtBlock:
switch (type.getQualifier().storage) {
case glslang::EvqShared:
case glslang::EvqUniform:
case glslang::EvqBuffer:
switch (type.getQualifier().layoutPacking) {
case glslang::ElpShared: return spv::DecorationGLSLShared;
case glslang::ElpPacked: return spv::DecorationGLSLPacked;
default:
return spv::DecorationMax;
}
case glslang::EvqVaryingIn:
case glslang::EvqVaryingOut:
if (type.getQualifier().isTaskMemory()) {
switch (type.getQualifier().layoutPacking) {
case glslang::ElpShared: return spv::DecorationGLSLShared;
case glslang::ElpPacked: return spv::DecorationGLSLPacked;
default: break;
}
} else {
assert(type.getQualifier().layoutPacking == glslang::ElpNone);
}
return spv::DecorationMax;
case glslang::EvqPayload:
case glslang::EvqPayloadIn:
case glslang::EvqHitAttr:
case glslang::EvqCallableData:
case glslang::EvqCallableDataIn:
case glslang::EvqHitObjectAttrNV:
return spv::DecorationMax;
default:
assert(0);
return spv::DecorationMax;
}
}
}
}
// Translate glslang type to SPIR-V interpolation decorations.
// Returns spv::DecorationMax when no decoration
// should be applied.
spv::Decoration TGlslangToSpvTraverser::TranslateInterpolationDecoration(const glslang::TQualifier& qualifier)
{
if (qualifier.smooth)
// Smooth decoration doesn't exist in SPIR-V 1.0
return spv::DecorationMax;
else if (qualifier.isNonPerspective())
return spv::DecorationNoPerspective;
else if (qualifier.flat)
return spv::DecorationFlat;
else if (qualifier.isExplicitInterpolation()) {
builder.addExtension(spv::E_SPV_AMD_shader_explicit_vertex_parameter);
return spv::DecorationExplicitInterpAMD;
}
else
return spv::DecorationMax;
}
// Translate glslang type to SPIR-V auxiliary storage decorations.
// Returns spv::DecorationMax when no decoration
// should be applied.
spv::Decoration TGlslangToSpvTraverser::TranslateAuxiliaryStorageDecoration(const glslang::TQualifier& qualifier)
{
if (qualifier.centroid)
return spv::DecorationCentroid;
else if (qualifier.patch)
return spv::DecorationPatch;
else if (qualifier.sample) {
builder.addCapability(spv::CapabilitySampleRateShading);
return spv::DecorationSample;
}
return spv::DecorationMax;
}
// If glslang type is invariant, return SPIR-V invariant decoration.
spv::Decoration TranslateInvariantDecoration(const glslang::TQualifier& qualifier)
{
if (qualifier.invariant)
return spv::DecorationInvariant;
else
return spv::DecorationMax;
}
// If glslang type is noContraction, return SPIR-V NoContraction decoration.
spv::Decoration TranslateNoContractionDecoration(const glslang::TQualifier& qualifier)
{
if (qualifier.isNoContraction())
return spv::DecorationNoContraction;
else
return spv::DecorationMax;
}
// If glslang type is nonUniform, return SPIR-V NonUniform decoration.
spv::Decoration TGlslangToSpvTraverser::TranslateNonUniformDecoration(const glslang::TQualifier& qualifier)
{
if (qualifier.isNonUniform()) {
builder.addIncorporatedExtension("SPV_EXT_descriptor_indexing", spv::Spv_1_5);
builder.addCapability(spv::CapabilityShaderNonUniformEXT);
return spv::DecorationNonUniformEXT;
} else
return spv::DecorationMax;
}
// If lvalue flags contains nonUniform, return SPIR-V NonUniform decoration.
spv::Decoration TGlslangToSpvTraverser::TranslateNonUniformDecoration(
const spv::Builder::AccessChain::CoherentFlags& coherentFlags)
{
if (coherentFlags.isNonUniform()) {
builder.addIncorporatedExtension("SPV_EXT_descriptor_indexing", spv::Spv_1_5);
builder.addCapability(spv::CapabilityShaderNonUniformEXT);
return spv::DecorationNonUniformEXT;
} else
return spv::DecorationMax;
}
spv::MemoryAccessMask TGlslangToSpvTraverser::TranslateMemoryAccess(
const spv::Builder::AccessChain::CoherentFlags &coherentFlags)
{
spv::MemoryAccessMask mask = spv::MemoryAccessMaskNone;
if (!glslangIntermediate->usingVulkanMemoryModel() || coherentFlags.isImage)
return mask;
if (coherentFlags.isVolatile() || coherentFlags.anyCoherent()) {
mask = mask | spv::MemoryAccessMakePointerAvailableKHRMask |
spv::MemoryAccessMakePointerVisibleKHRMask;
}
if (coherentFlags.nonprivate) {
mask = mask | spv::MemoryAccessNonPrivatePointerKHRMask;
}
if (coherentFlags.volatil) {
mask = mask | spv::MemoryAccessVolatileMask;
}
if (mask != spv::MemoryAccessMaskNone) {
builder.addCapability(spv::CapabilityVulkanMemoryModelKHR);
}
return mask;
}
spv::ImageOperandsMask TGlslangToSpvTraverser::TranslateImageOperands(
const spv::Builder::AccessChain::CoherentFlags &coherentFlags)
{
spv::ImageOperandsMask mask = spv::ImageOperandsMaskNone;
if (!glslangIntermediate->usingVulkanMemoryModel())
return mask;
if (coherentFlags.volatil ||
coherentFlags.anyCoherent()) {
mask = mask | spv::ImageOperandsMakeTexelAvailableKHRMask |
spv::ImageOperandsMakeTexelVisibleKHRMask;
}
if (coherentFlags.nonprivate) {
mask = mask | spv::ImageOperandsNonPrivateTexelKHRMask;
}
if (coherentFlags.volatil) {
mask = mask | spv::ImageOperandsVolatileTexelKHRMask;
}
if (mask != spv::ImageOperandsMaskNone) {
builder.addCapability(spv::CapabilityVulkanMemoryModelKHR);
}
return mask;
}
spv::Builder::AccessChain::CoherentFlags TGlslangToSpvTraverser::TranslateCoherent(const glslang::TType& type)
{
spv::Builder::AccessChain::CoherentFlags flags = {};
flags.coherent = type.getQualifier().coherent;
flags.devicecoherent = type.getQualifier().devicecoherent;
flags.queuefamilycoherent = type.getQualifier().queuefamilycoherent;
// shared variables are implicitly workgroupcoherent in GLSL.
flags.workgroupcoherent = type.getQualifier().workgroupcoherent ||
type.getQualifier().storage == glslang::EvqShared;
flags.subgroupcoherent = type.getQualifier().subgroupcoherent;
flags.shadercallcoherent = type.getQualifier().shadercallcoherent;
flags.volatil = type.getQualifier().volatil;
// *coherent variables are implicitly nonprivate in GLSL
flags.nonprivate = type.getQualifier().nonprivate ||
flags.anyCoherent() ||
flags.volatil;
flags.isImage = type.getBasicType() == glslang::EbtSampler;
flags.nonUniform = type.getQualifier().nonUniform;
return flags;
}
spv::Scope TGlslangToSpvTraverser::TranslateMemoryScope(
const spv::Builder::AccessChain::CoherentFlags &coherentFlags)
{
spv::Scope scope = spv::ScopeMax;
if (coherentFlags.volatil || coherentFlags.coherent) {
// coherent defaults to Device scope in the old model, QueueFamilyKHR scope in the new model
scope = glslangIntermediate->usingVulkanMemoryModel() ? spv::ScopeQueueFamilyKHR : spv::ScopeDevice;
} else if (coherentFlags.devicecoherent) {
scope = spv::ScopeDevice;
} else if (coherentFlags.queuefamilycoherent) {
scope = spv::ScopeQueueFamilyKHR;
} else if (coherentFlags.workgroupcoherent) {
scope = spv::ScopeWorkgroup;
} else if (coherentFlags.subgroupcoherent) {
scope = spv::ScopeSubgroup;
} else if (coherentFlags.shadercallcoherent) {
scope = spv::ScopeShaderCallKHR;
}
if (glslangIntermediate->usingVulkanMemoryModel() && scope == spv::ScopeDevice) {
builder.addCapability(spv::CapabilityVulkanMemoryModelDeviceScopeKHR);
}
return scope;
}
// Translate a glslang built-in variable to a SPIR-V built in decoration. Also generate
// associated capabilities when required. For some built-in variables, a capability
// is generated only when using the variable in an executable instruction, but not when
// just declaring a struct member variable with it. This is true for PointSize,
// ClipDistance, and CullDistance.
spv::BuiltIn TGlslangToSpvTraverser::TranslateBuiltInDecoration(glslang::TBuiltInVariable builtIn,
bool memberDeclaration)
{
switch (builtIn) {
case glslang::EbvPointSize:
// Defer adding the capability until the built-in is actually used.
if (! memberDeclaration) {
switch (glslangIntermediate->getStage()) {
case EShLangGeometry:
builder.addCapability(spv::CapabilityGeometryPointSize);
break;
case EShLangTessControl:
case EShLangTessEvaluation:
builder.addCapability(spv::CapabilityTessellationPointSize);
break;
default:
break;
}
}
return spv::BuiltInPointSize;
case glslang::EbvPosition: return spv::BuiltInPosition;
case glslang::EbvVertexId: return spv::BuiltInVertexId;
case glslang::EbvInstanceId: return spv::BuiltInInstanceId;
case glslang::EbvVertexIndex: return spv::BuiltInVertexIndex;
case glslang::EbvInstanceIndex: return spv::BuiltInInstanceIndex;
case glslang::EbvFragCoord: return spv::BuiltInFragCoord;
case glslang::EbvPointCoord: return spv::BuiltInPointCoord;
case glslang::EbvFace: return spv::BuiltInFrontFacing;
case glslang::EbvFragDepth: return spv::BuiltInFragDepth;
case glslang::EbvNumWorkGroups: return spv::BuiltInNumWorkgroups;
case glslang::EbvWorkGroupSize: return spv::BuiltInWorkgroupSize;
case glslang::EbvWorkGroupId: return spv::BuiltInWorkgroupId;
case glslang::EbvLocalInvocationId: return spv::BuiltInLocalInvocationId;
case glslang::EbvLocalInvocationIndex: return spv::BuiltInLocalInvocationIndex;
case glslang::EbvGlobalInvocationId: return spv::BuiltInGlobalInvocationId;
// These *Distance capabilities logically belong here, but if the member is declared and
// then never used, consumers of SPIR-V prefer the capability not be declared.
// They are now generated when used, rather than here when declared.
// Potentially, the specification should be more clear what the minimum
// use needed is to trigger the capability.
//
case glslang::EbvClipDistance:
if (!memberDeclaration)
builder.addCapability(spv::CapabilityClipDistance);
return spv::BuiltInClipDistance;
case glslang::EbvCullDistance:
if (!memberDeclaration)
builder.addCapability(spv::CapabilityCullDistance);
return spv::BuiltInCullDistance;
case glslang::EbvViewportIndex:
if (glslangIntermediate->getStage() == EShLangGeometry ||
glslangIntermediate->getStage() == EShLangFragment) {
builder.addCapability(spv::CapabilityMultiViewport);
}
if (glslangIntermediate->getStage() == EShLangVertex ||
glslangIntermediate->getStage() == EShLangTessControl ||
glslangIntermediate->getStage() == EShLangTessEvaluation) {
if (builder.getSpvVersion() < spv::Spv_1_5) {
builder.addIncorporatedExtension(spv::E_SPV_EXT_shader_viewport_index_layer, spv::Spv_1_5);
builder.addCapability(spv::CapabilityShaderViewportIndexLayerEXT);
}
else
builder.addCapability(spv::CapabilityShaderViewportIndex);
}
return spv::BuiltInViewportIndex;
case glslang::EbvSampleId:
builder.addCapability(spv::CapabilitySampleRateShading);
return spv::BuiltInSampleId;
case glslang::EbvSamplePosition:
builder.addCapability(spv::CapabilitySampleRateShading);
return spv::BuiltInSamplePosition;
case glslang::EbvSampleMask:
return spv::BuiltInSampleMask;
case glslang::EbvLayer:
if (glslangIntermediate->getStage() == EShLangMesh) {
return spv::BuiltInLayer;
}
if (glslangIntermediate->getStage() == EShLangGeometry ||
glslangIntermediate->getStage() == EShLangFragment) {
builder.addCapability(spv::CapabilityGeometry);
}
if (glslangIntermediate->getStage() == EShLangVertex ||
glslangIntermediate->getStage() == EShLangTessControl ||
glslangIntermediate->getStage() == EShLangTessEvaluation) {
if (builder.getSpvVersion() < spv::Spv_1_5) {
builder.addIncorporatedExtension(spv::E_SPV_EXT_shader_viewport_index_layer, spv::Spv_1_5);
builder.addCapability(spv::CapabilityShaderViewportIndexLayerEXT);
} else
builder.addCapability(spv::CapabilityShaderLayer);
}
return spv::BuiltInLayer;
case glslang::EbvBaseVertex:
builder.addIncorporatedExtension(spv::E_SPV_KHR_shader_draw_parameters, spv::Spv_1_3);
builder.addCapability(spv::CapabilityDrawParameters);
return spv::BuiltInBaseVertex;
case glslang::EbvBaseInstance:
builder.addIncorporatedExtension(spv::E_SPV_KHR_shader_draw_parameters, spv::Spv_1_3);
builder.addCapability(spv::CapabilityDrawParameters);
return spv::BuiltInBaseInstance;
case glslang::EbvDrawId:
builder.addIncorporatedExtension(spv::E_SPV_KHR_shader_draw_parameters, spv::Spv_1_3);
builder.addCapability(spv::CapabilityDrawParameters);
return spv::BuiltInDrawIndex;
case glslang::EbvPrimitiveId:
if (glslangIntermediate->getStage() == EShLangFragment)
builder.addCapability(spv::CapabilityGeometry);
return spv::BuiltInPrimitiveId;
case glslang::EbvFragStencilRef:
builder.addExtension(spv::E_SPV_EXT_shader_stencil_export);
builder.addCapability(spv::CapabilityStencilExportEXT);
return spv::BuiltInFragStencilRefEXT;
case glslang::EbvShadingRateKHR:
builder.addExtension(spv::E_SPV_KHR_fragment_shading_rate);
builder.addCapability(spv::CapabilityFragmentShadingRateKHR);
return spv::BuiltInShadingRateKHR;
case glslang::EbvPrimitiveShadingRateKHR:
builder.addExtension(spv::E_SPV_KHR_fragment_shading_rate);
builder.addCapability(spv::CapabilityFragmentShadingRateKHR);
return spv::BuiltInPrimitiveShadingRateKHR;
case glslang::EbvInvocationId: return spv::BuiltInInvocationId;
case glslang::EbvTessLevelInner: return spv::BuiltInTessLevelInner;
case glslang::EbvTessLevelOuter: return spv::BuiltInTessLevelOuter;
case glslang::EbvTessCoord: return spv::BuiltInTessCoord;
case glslang::EbvPatchVertices: return spv::BuiltInPatchVertices;
case glslang::EbvHelperInvocation: return spv::BuiltInHelperInvocation;
case glslang::EbvSubGroupSize:
builder.addExtension(spv::E_SPV_KHR_shader_ballot);
builder.addCapability(spv::CapabilitySubgroupBallotKHR);
return spv::BuiltInSubgroupSize;
case glslang::EbvSubGroupInvocation:
builder.addExtension(spv::E_SPV_KHR_shader_ballot);
builder.addCapability(spv::CapabilitySubgroupBallotKHR);
return spv::BuiltInSubgroupLocalInvocationId;
case glslang::EbvSubGroupEqMask:
builder.addExtension(spv::E_SPV_KHR_shader_ballot);
builder.addCapability(spv::CapabilitySubgroupBallotKHR);
return spv::BuiltInSubgroupEqMask;
case glslang::EbvSubGroupGeMask:
builder.addExtension(spv::E_SPV_KHR_shader_ballot);
builder.addCapability(spv::CapabilitySubgroupBallotKHR);
return spv::BuiltInSubgroupGeMask;
case glslang::EbvSubGroupGtMask:
builder.addExtension(spv::E_SPV_KHR_shader_ballot);
builder.addCapability(spv::CapabilitySubgroupBallotKHR);
return spv::BuiltInSubgroupGtMask;
case glslang::EbvSubGroupLeMask:
builder.addExtension(spv::E_SPV_KHR_shader_ballot);
builder.addCapability(spv::CapabilitySubgroupBallotKHR);
return spv::BuiltInSubgroupLeMask;
case glslang::EbvSubGroupLtMask:
builder.addExtension(spv::E_SPV_KHR_shader_ballot);
builder.addCapability(spv::CapabilitySubgroupBallotKHR);
return spv::BuiltInSubgroupLtMask;
case glslang::EbvNumSubgroups:
builder.addCapability(spv::CapabilityGroupNonUniform);
return spv::BuiltInNumSubgroups;
case glslang::EbvSubgroupID:
builder.addCapability(spv::CapabilityGroupNonUniform);
return spv::BuiltInSubgroupId;
case glslang::EbvSubgroupSize2:
builder.addCapability(spv::CapabilityGroupNonUniform);
return spv::BuiltInSubgroupSize;
case glslang::EbvSubgroupInvocation2:
builder.addCapability(spv::CapabilityGroupNonUniform);
return spv::BuiltInSubgroupLocalInvocationId;
case glslang::EbvSubgroupEqMask2:
builder.addCapability(spv::CapabilityGroupNonUniform);
builder.addCapability(spv::CapabilityGroupNonUniformBallot);
return spv::BuiltInSubgroupEqMask;
case glslang::EbvSubgroupGeMask2:
builder.addCapability(spv::CapabilityGroupNonUniform);
builder.addCapability(spv::CapabilityGroupNonUniformBallot);
return spv::BuiltInSubgroupGeMask;
case glslang::EbvSubgroupGtMask2:
builder.addCapability(spv::CapabilityGroupNonUniform);
builder.addCapability(spv::CapabilityGroupNonUniformBallot);
return spv::BuiltInSubgroupGtMask;
case glslang::EbvSubgroupLeMask2:
builder.addCapability(spv::CapabilityGroupNonUniform);
builder.addCapability(spv::CapabilityGroupNonUniformBallot);
return spv::BuiltInSubgroupLeMask;
case glslang::EbvSubgroupLtMask2:
builder.addCapability(spv::CapabilityGroupNonUniform);
builder.addCapability(spv::CapabilityGroupNonUniformBallot);
return spv::BuiltInSubgroupLtMask;
case glslang::EbvBaryCoordNoPersp:
builder.addExtension(spv::E_SPV_AMD_shader_explicit_vertex_parameter);
return spv::BuiltInBaryCoordNoPerspAMD;
case glslang::EbvBaryCoordNoPerspCentroid:
builder.addExtension(spv::E_SPV_AMD_shader_explicit_vertex_parameter);
return spv::BuiltInBaryCoordNoPerspCentroidAMD;
case glslang::EbvBaryCoordNoPerspSample:
builder.addExtension(spv::E_SPV_AMD_shader_explicit_vertex_parameter);
return spv::BuiltInBaryCoordNoPerspSampleAMD;
case glslang::EbvBaryCoordSmooth:
builder.addExtension(spv::E_SPV_AMD_shader_explicit_vertex_parameter);
return spv::BuiltInBaryCoordSmoothAMD;
case glslang::EbvBaryCoordSmoothCentroid:
builder.addExtension(spv::E_SPV_AMD_shader_explicit_vertex_parameter);
return spv::BuiltInBaryCoordSmoothCentroidAMD;
case glslang::EbvBaryCoordSmoothSample:
builder.addExtension(spv::E_SPV_AMD_shader_explicit_vertex_parameter);
return spv::BuiltInBaryCoordSmoothSampleAMD;
case glslang::EbvBaryCoordPullModel:
builder.addExtension(spv::E_SPV_AMD_shader_explicit_vertex_parameter);
return spv::BuiltInBaryCoordPullModelAMD;
case glslang::EbvDeviceIndex:
builder.addIncorporatedExtension(spv::E_SPV_KHR_device_group, spv::Spv_1_3);
builder.addCapability(spv::CapabilityDeviceGroup);
return spv::BuiltInDeviceIndex;
case glslang::EbvViewIndex:
builder.addIncorporatedExtension(spv::E_SPV_KHR_multiview, spv::Spv_1_3);
builder.addCapability(spv::CapabilityMultiView);
return spv::BuiltInViewIndex;
case glslang::EbvFragSizeEXT:
builder.addExtension(spv::E_SPV_EXT_fragment_invocation_density);
builder.addCapability(spv::CapabilityFragmentDensityEXT);
return spv::BuiltInFragSizeEXT;
case glslang::EbvFragInvocationCountEXT:
builder.addExtension(spv::E_SPV_EXT_fragment_invocation_density);
builder.addCapability(spv::CapabilityFragmentDensityEXT);
return spv::BuiltInFragInvocationCountEXT;
case glslang::EbvViewportMaskNV:
if (!memberDeclaration) {
builder.addExtension(spv::E_SPV_NV_viewport_array2);
builder.addCapability(spv::CapabilityShaderViewportMaskNV);
}
return spv::BuiltInViewportMaskNV;
case glslang::EbvSecondaryPositionNV:
if (!memberDeclaration) {
builder.addExtension(spv::E_SPV_NV_stereo_view_rendering);
builder.addCapability(spv::CapabilityShaderStereoViewNV);
}
return spv::BuiltInSecondaryPositionNV;
case glslang::EbvSecondaryViewportMaskNV:
if (!memberDeclaration) {
builder.addExtension(spv::E_SPV_NV_stereo_view_rendering);
builder.addCapability(spv::CapabilityShaderStereoViewNV);
}
return spv::BuiltInSecondaryViewportMaskNV;
case glslang::EbvPositionPerViewNV:
if (!memberDeclaration) {
builder.addExtension(spv::E_SPV_NVX_multiview_per_view_attributes);
builder.addCapability(spv::CapabilityPerViewAttributesNV);
}
return spv::BuiltInPositionPerViewNV;
case glslang::EbvViewportMaskPerViewNV:
if (!memberDeclaration) {
builder.addExtension(spv::E_SPV_NVX_multiview_per_view_attributes);
builder.addCapability(spv::CapabilityPerViewAttributesNV);
}
return spv::BuiltInViewportMaskPerViewNV;
case glslang::EbvFragFullyCoveredNV:
builder.addExtension(spv::E_SPV_EXT_fragment_fully_covered);
builder.addCapability(spv::CapabilityFragmentFullyCoveredEXT);
return spv::BuiltInFullyCoveredEXT;
case glslang::EbvFragmentSizeNV:
builder.addExtension(spv::E_SPV_NV_shading_rate);
builder.addCapability(spv::CapabilityShadingRateNV);
return spv::BuiltInFragmentSizeNV;
case glslang::EbvInvocationsPerPixelNV:
builder.addExtension(spv::E_SPV_NV_shading_rate);
builder.addCapability(spv::CapabilityShadingRateNV);
return spv::BuiltInInvocationsPerPixelNV;
// ray tracing
case glslang::EbvLaunchId:
return spv::BuiltInLaunchIdKHR;
case glslang::EbvLaunchSize:
return spv::BuiltInLaunchSizeKHR;
case glslang::EbvWorldRayOrigin:
return spv::BuiltInWorldRayOriginKHR;
case glslang::EbvWorldRayDirection:
return spv::BuiltInWorldRayDirectionKHR;
case glslang::EbvObjectRayOrigin:
return spv::BuiltInObjectRayOriginKHR;
case glslang::EbvObjectRayDirection:
return spv::BuiltInObjectRayDirectionKHR;
case glslang::EbvRayTmin:
return spv::BuiltInRayTminKHR;
case glslang::EbvRayTmax:
return spv::BuiltInRayTmaxKHR;
case glslang::EbvCullMask:
return spv::BuiltInCullMaskKHR;
case glslang::EbvPositionFetch:
return spv::BuiltInHitTriangleVertexPositionsKHR;
case glslang::EbvInstanceCustomIndex:
return spv::BuiltInInstanceCustomIndexKHR;
case glslang::EbvHitKind:
return spv::BuiltInHitKindKHR;
case glslang::EbvObjectToWorld:
case glslang::EbvObjectToWorld3x4:
return spv::BuiltInObjectToWorldKHR;
case glslang::EbvWorldToObject:
case glslang::EbvWorldToObject3x4:
return spv::BuiltInWorldToObjectKHR;
case glslang::EbvIncomingRayFlags:
return spv::BuiltInIncomingRayFlagsKHR;
case glslang::EbvGeometryIndex:
return spv::BuiltInRayGeometryIndexKHR;
case glslang::EbvCurrentRayTimeNV:
builder.addExtension(spv::E_SPV_NV_ray_tracing_motion_blur);
builder.addCapability(spv::CapabilityRayTracingMotionBlurNV);
return spv::BuiltInCurrentRayTimeNV;
case glslang::EbvMicroTrianglePositionNV:
builder.addCapability(spv::CapabilityRayTracingDisplacementMicromapNV);
builder.addExtension("SPV_NV_displacement_micromap");
return spv::BuiltInHitMicroTriangleVertexPositionsNV;
case glslang::EbvMicroTriangleBaryNV:
builder.addCapability(spv::CapabilityRayTracingDisplacementMicromapNV);
builder.addExtension("SPV_NV_displacement_micromap");
return spv::BuiltInHitMicroTriangleVertexBarycentricsNV;
case glslang::EbvHitKindFrontFacingMicroTriangleNV:
builder.addCapability(spv::CapabilityRayTracingDisplacementMicromapNV);
builder.addExtension("SPV_NV_displacement_micromap");
return spv::BuiltInHitKindFrontFacingMicroTriangleNV;
case glslang::EbvHitKindBackFacingMicroTriangleNV:
builder.addCapability(spv::CapabilityRayTracingDisplacementMicromapNV);
builder.addExtension("SPV_NV_displacement_micromap");
return spv::BuiltInHitKindBackFacingMicroTriangleNV;
// barycentrics
case glslang::EbvBaryCoordNV:
builder.addExtension(spv::E_SPV_NV_fragment_shader_barycentric);
builder.addCapability(spv::CapabilityFragmentBarycentricNV);
return spv::BuiltInBaryCoordNV;
case glslang::EbvBaryCoordNoPerspNV:
builder.addExtension(spv::E_SPV_NV_fragment_shader_barycentric);
builder.addCapability(spv::CapabilityFragmentBarycentricNV);
return spv::BuiltInBaryCoordNoPerspNV;
case glslang::EbvBaryCoordEXT:
builder.addExtension(spv::E_SPV_KHR_fragment_shader_barycentric);
builder.addCapability(spv::CapabilityFragmentBarycentricKHR);
return spv::BuiltInBaryCoordKHR;
case glslang::EbvBaryCoordNoPerspEXT:
builder.addExtension(spv::E_SPV_KHR_fragment_shader_barycentric);
builder.addCapability(spv::CapabilityFragmentBarycentricKHR);
return spv::BuiltInBaryCoordNoPerspKHR;
// mesh shaders
case glslang::EbvTaskCountNV:
return spv::BuiltInTaskCountNV;
case glslang::EbvPrimitiveCountNV:
return spv::BuiltInPrimitiveCountNV;
case glslang::EbvPrimitiveIndicesNV:
return spv::BuiltInPrimitiveIndicesNV;
case glslang::EbvClipDistancePerViewNV:
return spv::BuiltInClipDistancePerViewNV;
case glslang::EbvCullDistancePerViewNV:
return spv::BuiltInCullDistancePerViewNV;
case glslang::EbvLayerPerViewNV:
return spv::BuiltInLayerPerViewNV;
case glslang::EbvMeshViewCountNV:
return spv::BuiltInMeshViewCountNV;
case glslang::EbvMeshViewIndicesNV:
return spv::BuiltInMeshViewIndicesNV;
// SPV_EXT_mesh_shader
case glslang::EbvPrimitivePointIndicesEXT:
return spv::BuiltInPrimitivePointIndicesEXT;
case glslang::EbvPrimitiveLineIndicesEXT:
return spv::BuiltInPrimitiveLineIndicesEXT;
case glslang::EbvPrimitiveTriangleIndicesEXT:
return spv::BuiltInPrimitiveTriangleIndicesEXT;
case glslang::EbvCullPrimitiveEXT:
return spv::BuiltInCullPrimitiveEXT;
// sm builtins
case glslang::EbvWarpsPerSM:
builder.addExtension(spv::E_SPV_NV_shader_sm_builtins);
builder.addCapability(spv::CapabilityShaderSMBuiltinsNV);
return spv::BuiltInWarpsPerSMNV;
case glslang::EbvSMCount:
builder.addExtension(spv::E_SPV_NV_shader_sm_builtins);
builder.addCapability(spv::CapabilityShaderSMBuiltinsNV);
return spv::BuiltInSMCountNV;
case glslang::EbvWarpID:
builder.addExtension(spv::E_SPV_NV_shader_sm_builtins);
builder.addCapability(spv::CapabilityShaderSMBuiltinsNV);
return spv::BuiltInWarpIDNV;
case glslang::EbvSMID:
builder.addExtension(spv::E_SPV_NV_shader_sm_builtins);
builder.addCapability(spv::CapabilityShaderSMBuiltinsNV);
return spv::BuiltInSMIDNV;
// ARM builtins
case glslang::EbvCoreCountARM:
builder.addExtension(spv::E_SPV_ARM_core_builtins);
builder.addCapability(spv::CapabilityCoreBuiltinsARM);
return spv::BuiltInCoreCountARM;
case glslang::EbvCoreIDARM:
builder.addExtension(spv::E_SPV_ARM_core_builtins);
builder.addCapability(spv::CapabilityCoreBuiltinsARM);
return spv::BuiltInCoreIDARM;
case glslang::EbvCoreMaxIDARM:
builder.addExtension(spv::E_SPV_ARM_core_builtins);
builder.addCapability(spv::CapabilityCoreBuiltinsARM);
return spv::BuiltInCoreMaxIDARM;
case glslang::EbvWarpIDARM:
builder.addExtension(spv::E_SPV_ARM_core_builtins);
builder.addCapability(spv::CapabilityCoreBuiltinsARM);
return spv::BuiltInWarpIDARM;
case glslang::EbvWarpMaxIDARM:
builder.addExtension(spv::E_SPV_ARM_core_builtins);
builder.addCapability(spv::CapabilityCoreBuiltinsARM);
return spv::BuiltInWarpMaxIDARM;
default:
return spv::BuiltInMax;
}
}
// Translate glslang image layout format to SPIR-V image format.
spv::ImageFormat TGlslangToSpvTraverser::TranslateImageFormat(const glslang::TType& type)
{
assert(type.getBasicType() == glslang::EbtSampler);
// Check for capabilities
switch (type.getQualifier().getFormat()) {
case glslang::ElfRg32f:
case glslang::ElfRg16f:
case glslang::ElfR11fG11fB10f:
case glslang::ElfR16f:
case glslang::ElfRgba16:
case glslang::ElfRgb10A2:
case glslang::ElfRg16:
case glslang::ElfRg8:
case glslang::ElfR16:
case glslang::ElfR8:
case glslang::ElfRgba16Snorm:
case glslang::ElfRg16Snorm:
case glslang::ElfRg8Snorm:
case glslang::ElfR16Snorm:
case glslang::ElfR8Snorm:
case glslang::ElfRg32i:
case glslang::ElfRg16i:
case glslang::ElfRg8i:
case glslang::ElfR16i:
case glslang::ElfR8i:
case glslang::ElfRgb10a2ui:
case glslang::ElfRg32ui:
case glslang::ElfRg16ui:
case glslang::ElfRg8ui:
case glslang::ElfR16ui:
case glslang::ElfR8ui:
builder.addCapability(spv::CapabilityStorageImageExtendedFormats);
break;
case glslang::ElfR64ui:
case glslang::ElfR64i:
builder.addExtension(spv::E_SPV_EXT_shader_image_int64);
builder.addCapability(spv::CapabilityInt64ImageEXT);
break;
default:
break;
}
// do the translation
switch (type.getQualifier().getFormat()) {
case glslang::ElfNone: return spv::ImageFormatUnknown;
case glslang::ElfRgba32f: return spv::ImageFormatRgba32f;
case glslang::ElfRgba16f: return spv::ImageFormatRgba16f;
case glslang::ElfR32f: return spv::ImageFormatR32f;
case glslang::ElfRgba8: return spv::ImageFormatRgba8;
case glslang::ElfRgba8Snorm: return spv::ImageFormatRgba8Snorm;
case glslang::ElfRg32f: return spv::ImageFormatRg32f;
case glslang::ElfRg16f: return spv::ImageFormatRg16f;
case glslang::ElfR11fG11fB10f: return spv::ImageFormatR11fG11fB10f;
case glslang::ElfR16f: return spv::ImageFormatR16f;
case glslang::ElfRgba16: return spv::ImageFormatRgba16;
case glslang::ElfRgb10A2: return spv::ImageFormatRgb10A2;
case glslang::ElfRg16: return spv::ImageFormatRg16;
case glslang::ElfRg8: return spv::ImageFormatRg8;
case glslang::ElfR16: return spv::ImageFormatR16;
case glslang::ElfR8: return spv::ImageFormatR8;
case glslang::ElfRgba16Snorm: return spv::ImageFormatRgba16Snorm;
case glslang::ElfRg16Snorm: return spv::ImageFormatRg16Snorm;
case glslang::ElfRg8Snorm: return spv::ImageFormatRg8Snorm;
case glslang::ElfR16Snorm: return spv::ImageFormatR16Snorm;
case glslang::ElfR8Snorm: return spv::ImageFormatR8Snorm;
case glslang::ElfRgba32i: return spv::ImageFormatRgba32i;
case glslang::ElfRgba16i: return spv::ImageFormatRgba16i;
case glslang::ElfRgba8i: return spv::ImageFormatRgba8i;
case glslang::ElfR32i: return spv::ImageFormatR32i;
case glslang::ElfRg32i: return spv::ImageFormatRg32i;
case glslang::ElfRg16i: return spv::ImageFormatRg16i;
case glslang::ElfRg8i: return spv::ImageFormatRg8i;
case glslang::ElfR16i: return spv::ImageFormatR16i;
case glslang::ElfR8i: return spv::ImageFormatR8i;
case glslang::ElfRgba32ui: return spv::ImageFormatRgba32ui;
case glslang::ElfRgba16ui: return spv::ImageFormatRgba16ui;
case glslang::ElfRgba8ui: return spv::ImageFormatRgba8ui;
case glslang::ElfR32ui: return spv::ImageFormatR32ui;
case glslang::ElfRg32ui: return spv::ImageFormatRg32ui;
case glslang::ElfRg16ui: return spv::ImageFormatRg16ui;
case glslang::ElfRgb10a2ui: return spv::ImageFormatRgb10a2ui;
case glslang::ElfRg8ui: return spv::ImageFormatRg8ui;
case glslang::ElfR16ui: return spv::ImageFormatR16ui;
case glslang::ElfR8ui: return spv::ImageFormatR8ui;
case glslang::ElfR64ui: return spv::ImageFormatR64ui;
case glslang::ElfR64i: return spv::ImageFormatR64i;
default: return spv::ImageFormatMax;
}
}
spv::SelectionControlMask TGlslangToSpvTraverser::TranslateSelectionControl(
const glslang::TIntermSelection& selectionNode) const
{
if (selectionNode.getFlatten())
return spv::SelectionControlFlattenMask;
if (selectionNode.getDontFlatten())
return spv::SelectionControlDontFlattenMask;
return spv::SelectionControlMaskNone;
}
spv::SelectionControlMask TGlslangToSpvTraverser::TranslateSwitchControl(const glslang::TIntermSwitch& switchNode)
const
{
if (switchNode.getFlatten())
return spv::SelectionControlFlattenMask;
if (switchNode.getDontFlatten())
return spv::SelectionControlDontFlattenMask;
return spv::SelectionControlMaskNone;
}
// return a non-0 dependency if the dependency argument must be set
spv::LoopControlMask TGlslangToSpvTraverser::TranslateLoopControl(const glslang::TIntermLoop& loopNode,
std::vector<unsigned int>& operands) const
{
spv::LoopControlMask control = spv::LoopControlMaskNone;
if (loopNode.getDontUnroll())
control = control | spv::LoopControlDontUnrollMask;
if (loopNode.getUnroll())
control = control | spv::LoopControlUnrollMask;
if (unsigned(loopNode.getLoopDependency()) == glslang::TIntermLoop::dependencyInfinite)
control = control | spv::LoopControlDependencyInfiniteMask;
else if (loopNode.getLoopDependency() > 0) {
control = control | spv::LoopControlDependencyLengthMask;
operands.push_back((unsigned int)loopNode.getLoopDependency());
}
if (glslangIntermediate->getSpv().spv >= glslang::EShTargetSpv_1_4) {
if (loopNode.getMinIterations() > 0) {
control = control | spv::LoopControlMinIterationsMask;
operands.push_back(loopNode.getMinIterations());
}
if (loopNode.getMaxIterations() < glslang::TIntermLoop::iterationsInfinite) {
control = control | spv::LoopControlMaxIterationsMask;
operands.push_back(loopNode.getMaxIterations());
}
if (loopNode.getIterationMultiple() > 1) {
control = control | spv::LoopControlIterationMultipleMask;
operands.push_back(loopNode.getIterationMultiple());
}
if (loopNode.getPeelCount() > 0) {
control = control | spv::LoopControlPeelCountMask;
operands.push_back(loopNode.getPeelCount());
}
if (loopNode.getPartialCount() > 0) {
control = control | spv::LoopControlPartialCountMask;
operands.push_back(loopNode.getPartialCount());
}
}
return control;
}
// Translate glslang type to SPIR-V storage class.
spv::StorageClass TGlslangToSpvTraverser::TranslateStorageClass(const glslang::TType& type)
{
if (type.getBasicType() == glslang::EbtRayQuery || type.getBasicType() == glslang::EbtHitObjectNV)
return spv::StorageClassPrivate;
if (type.getQualifier().isSpirvByReference()) {
if (type.getQualifier().isParamInput() || type.getQualifier().isParamOutput())
return spv::StorageClassFunction;
}
if (type.getQualifier().isPipeInput())
return spv::StorageClassInput;
if (type.getQualifier().isPipeOutput())
return spv::StorageClassOutput;
if (type.getQualifier().storage == glslang::EvqTileImageEXT || type.isAttachmentEXT()) {
builder.addExtension(spv::E_SPV_EXT_shader_tile_image);
builder.addCapability(spv::CapabilityTileImageColorReadAccessEXT);
return spv::StorageClassTileImageEXT;
}
if (glslangIntermediate->getSource() != glslang::EShSourceHlsl ||
type.getQualifier().storage == glslang::EvqUniform) {
if (type.isAtomic())
return spv::StorageClassAtomicCounter;
if (type.containsOpaque() && !glslangIntermediate->getBindlessMode())
return spv::StorageClassUniformConstant;
}
if (type.getQualifier().isUniformOrBuffer() &&
type.getQualifier().isShaderRecord()) {
return spv::StorageClassShaderRecordBufferKHR;
}
if (glslangIntermediate->usingStorageBuffer() && type.getQualifier().storage == glslang::EvqBuffer) {
builder.addIncorporatedExtension(spv::E_SPV_KHR_storage_buffer_storage_class, spv::Spv_1_3);
return spv::StorageClassStorageBuffer;
}
if (type.getQualifier().isUniformOrBuffer()) {
if (type.getQualifier().isPushConstant())
return spv::StorageClassPushConstant;
if (type.getBasicType() == glslang::EbtBlock)
return spv::StorageClassUniform;
return spv::StorageClassUniformConstant;
}
if (type.getQualifier().storage == glslang::EvqShared && type.getBasicType() == glslang::EbtBlock) {
builder.addExtension(spv::E_SPV_KHR_workgroup_memory_explicit_layout);
builder.addCapability(spv::CapabilityWorkgroupMemoryExplicitLayoutKHR);
return spv::StorageClassWorkgroup;
}
switch (type.getQualifier().storage) {
case glslang::EvqGlobal: return spv::StorageClassPrivate;
case glslang::EvqConstReadOnly: return spv::StorageClassFunction;
case glslang::EvqTemporary: return spv::StorageClassFunction;
case glslang::EvqShared: return spv::StorageClassWorkgroup;
case glslang::EvqPayload: return spv::StorageClassRayPayloadKHR;
case glslang::EvqPayloadIn: return spv::StorageClassIncomingRayPayloadKHR;
case glslang::EvqHitAttr: return spv::StorageClassHitAttributeKHR;
case glslang::EvqCallableData: return spv::StorageClassCallableDataKHR;
case glslang::EvqCallableDataIn: return spv::StorageClassIncomingCallableDataKHR;
case glslang::EvqtaskPayloadSharedEXT : return spv::StorageClassTaskPayloadWorkgroupEXT;
case glslang::EvqHitObjectAttrNV: return spv::StorageClassHitObjectAttributeNV;
case glslang::EvqSpirvStorageClass: return static_cast<spv::StorageClass>(type.getQualifier().spirvStorageClass);
default:
assert(0);
break;
}
return spv::StorageClassFunction;
}
// Translate glslang constants to SPIR-V literals
void TGlslangToSpvTraverser::TranslateLiterals(const glslang::TVector<const glslang::TIntermConstantUnion*>& constants,
std::vector<unsigned>& literals) const
{
for (auto constant : constants) {
if (constant->getBasicType() == glslang::EbtFloat) {
float floatValue = static_cast<float>(constant->getConstArray()[0].getDConst());
unsigned literal;
static_assert(sizeof(literal) == sizeof(floatValue), "sizeof(unsigned) != sizeof(float)");
memcpy(&literal, &floatValue, sizeof(literal));
literals.push_back(literal);
} else if (constant->getBasicType() == glslang::EbtInt) {
unsigned literal = constant->getConstArray()[0].getIConst();
literals.push_back(literal);
} else if (constant->getBasicType() == glslang::EbtUint) {
unsigned literal = constant->getConstArray()[0].getUConst();
literals.push_back(literal);
} else if (constant->getBasicType() == glslang::EbtBool) {
unsigned literal = constant->getConstArray()[0].getBConst();
literals.push_back(literal);
} else if (constant->getBasicType() == glslang::EbtString) {
auto str = constant->getConstArray()[0].getSConst()->c_str();
unsigned literal = 0;
char* literalPtr = reinterpret_cast<char*>(&literal);
unsigned charCount = 0;
char ch = 0;
do {
ch = *(str++);
*(literalPtr++) = ch;
++charCount;
if (charCount == 4) {
literals.push_back(literal);
literalPtr = reinterpret_cast<char*>(&literal);
charCount = 0;
}
} while (ch != 0);
// Partial literal is padded with 0
if (charCount > 0) {
for (; charCount < 4; ++charCount)
*(literalPtr++) = 0;
literals.push_back(literal);
}
} else
assert(0); // Unexpected type
}
}
// Add capabilities pertaining to how an array is indexed.
void TGlslangToSpvTraverser::addIndirectionIndexCapabilities(const glslang::TType& baseType,
const glslang::TType& indexType)
{
if (indexType.getQualifier().isNonUniform()) {
// deal with an asserted non-uniform index
// SPV_EXT_descriptor_indexing already added in TranslateNonUniformDecoration
if (baseType.getBasicType() == glslang::EbtSampler) {
if (baseType.getQualifier().hasAttachment())
builder.addCapability(spv::CapabilityInputAttachmentArrayNonUniformIndexingEXT);
else if (baseType.isImage() && baseType.getSampler().isBuffer())
builder.addCapability(spv::CapabilityStorageTexelBufferArrayNonUniformIndexingEXT);
else if (baseType.isTexture() && baseType.getSampler().isBuffer())
builder.addCapability(spv::CapabilityUniformTexelBufferArrayNonUniformIndexingEXT);
else if (baseType.isImage())
builder.addCapability(spv::CapabilityStorageImageArrayNonUniformIndexingEXT);
else if (baseType.isTexture())
builder.addCapability(spv::CapabilitySampledImageArrayNonUniformIndexingEXT);
} else if (baseType.getBasicType() == glslang::EbtBlock) {
if (baseType.getQualifier().storage == glslang::EvqBuffer)
builder.addCapability(spv::CapabilityStorageBufferArrayNonUniformIndexingEXT);
else if (baseType.getQualifier().storage == glslang::EvqUniform)
builder.addCapability(spv::CapabilityUniformBufferArrayNonUniformIndexingEXT);
}
} else {
// assume a dynamically uniform index
if (baseType.getBasicType() == glslang::EbtSampler) {
if (baseType.getQualifier().hasAttachment()) {
builder.addIncorporatedExtension("SPV_EXT_descriptor_indexing", spv::Spv_1_5);
builder.addCapability(spv::CapabilityInputAttachmentArrayDynamicIndexingEXT);
} else if (baseType.isImage() && baseType.getSampler().isBuffer()) {
builder.addIncorporatedExtension("SPV_EXT_descriptor_indexing", spv::Spv_1_5);
builder.addCapability(spv::CapabilityStorageTexelBufferArrayDynamicIndexingEXT);
} else if (baseType.isTexture() && baseType.getSampler().isBuffer()) {
builder.addIncorporatedExtension("SPV_EXT_descriptor_indexing", spv::Spv_1_5);
builder.addCapability(spv::CapabilityUniformTexelBufferArrayDynamicIndexingEXT);
}
}
}
}
// Return whether or not the given type is something that should be tied to a
// descriptor set.
bool IsDescriptorResource(const glslang::TType& type)
{
// uniform and buffer blocks are included, unless it is a push_constant
if (type.getBasicType() == glslang::EbtBlock)
return type.getQualifier().isUniformOrBuffer() &&
! type.getQualifier().isShaderRecord() &&
! type.getQualifier().isPushConstant();
// non block...
// basically samplerXXX/subpass/sampler/texture are all included
// if they are the global-scope-class, not the function parameter
// (or local, if they ever exist) class.
if (type.getBasicType() == glslang::EbtSampler ||
type.getBasicType() == glslang::EbtAccStruct)
return type.getQualifier().isUniformOrBuffer();
// None of the above.
return false;
}
void InheritQualifiers(glslang::TQualifier& child, const glslang::TQualifier& parent)
{
if (child.layoutMatrix == glslang::ElmNone)
child.layoutMatrix = parent.layoutMatrix;
if (parent.invariant)
child.invariant = true;
if (parent.flat)
child.flat = true;
if (parent.centroid)
child.centroid = true;
if (parent.nopersp)
child.nopersp = true;
if (parent.explicitInterp)
child.explicitInterp = true;
if (parent.perPrimitiveNV)
child.perPrimitiveNV = true;
if (parent.perViewNV)
child.perViewNV = true;
if (parent.perTaskNV)
child.perTaskNV = true;
if (parent.storage == glslang::EvqtaskPayloadSharedEXT)
child.storage = glslang::EvqtaskPayloadSharedEXT;
if (parent.patch)
child.patch = true;
if (parent.sample)
child.sample = true;
if (parent.coherent)
child.coherent = true;
if (parent.devicecoherent)
child.devicecoherent = true;
if (parent.queuefamilycoherent)
child.queuefamilycoherent = true;
if (parent.workgroupcoherent)
child.workgroupcoherent = true;
if (parent.subgroupcoherent)
child.subgroupcoherent = true;
if (parent.shadercallcoherent)
child.shadercallcoherent = true;
if (parent.nonprivate)
child.nonprivate = true;
if (parent.volatil)
child.volatil = true;
if (parent.restrict)
child.restrict = true;
if (parent.readonly)
child.readonly = true;
if (parent.writeonly)
child.writeonly = true;
if (parent.nonUniform)
child.nonUniform = true;
}
bool HasNonLayoutQualifiers(const glslang::TType& type, const glslang::TQualifier& qualifier)
{
// This should list qualifiers that simultaneous satisfy:
// - struct members might inherit from a struct declaration
// (note that non-block structs don't explicitly inherit,
// only implicitly, meaning no decoration involved)
// - affect decorations on the struct members
// (note smooth does not, and expecting something like volatile
// to effect the whole object)
// - are not part of the offset/st430/etc or row/column-major layout
return qualifier.invariant || (qualifier.hasLocation() && type.getBasicType() == glslang::EbtBlock);
}
//
// Implement the TGlslangToSpvTraverser class.
//
TGlslangToSpvTraverser::TGlslangToSpvTraverser(unsigned int spvVersion,
const glslang::TIntermediate* glslangIntermediate,
spv::SpvBuildLogger* buildLogger, glslang::SpvOptions& options) :
TIntermTraverser(true, false, true),
options(options),
shaderEntry(nullptr), currentFunction(nullptr),
sequenceDepth(0), logger(buildLogger),
builder(spvVersion, (glslang::GetKhronosToolId() << 16) | glslang::GetSpirvGeneratorVersion(), logger),
inEntryPoint(false), entryPointTerminated(false), linkageOnly(false),
glslangIntermediate(glslangIntermediate),
nanMinMaxClamp(glslangIntermediate->getNanMinMaxClamp()),
nonSemanticDebugPrintf(0),
taskPayloadID(0)
{
bool isMeshShaderExt = (glslangIntermediate->getRequestedExtensions().find(glslang::E_GL_EXT_mesh_shader) !=
glslangIntermediate->getRequestedExtensions().end());
spv::ExecutionModel executionModel = TranslateExecutionModel(glslangIntermediate->getStage(), isMeshShaderExt);
builder.clearAccessChain();
builder.setSource(TranslateSourceLanguage(glslangIntermediate->getSource(), glslangIntermediate->getProfile()),
glslangIntermediate->getVersion());
if (options.emitNonSemanticShaderDebugSource)
this->options.emitNonSemanticShaderDebugInfo = true;
if (options.emitNonSemanticShaderDebugInfo)
this->options.generateDebugInfo = true;
if (this->options.generateDebugInfo) {
if (this->options.emitNonSemanticShaderDebugInfo) {
builder.setEmitNonSemanticShaderDebugInfo(this->options.emitNonSemanticShaderDebugSource);
}
else {
builder.setEmitSpirvDebugInfo();
}
builder.setDebugSourceFile(glslangIntermediate->getSourceFile());
// Set the source shader's text. If for SPV version 1.0, include
// a preamble in comments stating the OpModuleProcessed instructions.
// Otherwise, emit those as actual instructions.
std::string text;
const std::vector<std::string>& processes = glslangIntermediate->getProcesses();
for (int p = 0; p < (int)processes.size(); ++p) {
if (glslangIntermediate->getSpv().spv < glslang::EShTargetSpv_1_1) {
text.append("// OpModuleProcessed ");
text.append(processes[p]);
text.append("\n");
} else
builder.addModuleProcessed(processes[p]);
}
if (glslangIntermediate->getSpv().spv < glslang::EShTargetSpv_1_1 && (int)processes.size() > 0)
text.append("#line 1\n");
text.append(glslangIntermediate->getSourceText());
builder.setSourceText(text);
// Pass name and text for all included files
const std::map<std::string, std::string>& include_txt = glslangIntermediate->getIncludeText();
for (auto iItr = include_txt.begin(); iItr != include_txt.end(); ++iItr)
builder.addInclude(iItr->first, iItr->second);
}
builder.setUseReplicatedComposites(glslangIntermediate->usingReplicatedComposites());
stdBuiltins = builder.import("GLSL.std.450");
spv::AddressingModel addressingModel = spv::AddressingModelLogical;
spv::MemoryModel memoryModel = spv::MemoryModelGLSL450;
if (glslangIntermediate->usingPhysicalStorageBuffer()) {
addressingModel = spv::AddressingModelPhysicalStorageBuffer64EXT;
builder.addIncorporatedExtension(spv::E_SPV_KHR_physical_storage_buffer, spv::Spv_1_5);
builder.addCapability(spv::CapabilityPhysicalStorageBufferAddressesEXT);
}
if (glslangIntermediate->usingVulkanMemoryModel()) {
memoryModel = spv::MemoryModelVulkanKHR;
builder.addCapability(spv::CapabilityVulkanMemoryModelKHR);
builder.addIncorporatedExtension(spv::E_SPV_KHR_vulkan_memory_model, spv::Spv_1_5);
}
builder.setMemoryModel(addressingModel, memoryModel);
if (glslangIntermediate->usingVariablePointers()) {
builder.addCapability(spv::CapabilityVariablePointers);
}
// If not linking, there is no entry point
if (!options.compileOnly) {
shaderEntry = builder.makeEntryPoint(glslangIntermediate->getEntryPointName().c_str());
entryPoint =
builder.addEntryPoint(executionModel, shaderEntry, glslangIntermediate->getEntryPointName().c_str());
}
// Add the source extensions
const auto& sourceExtensions = glslangIntermediate->getRequestedExtensions();
for (auto it = sourceExtensions.begin(); it != sourceExtensions.end(); ++it)
builder.addSourceExtension(it->c_str());
// Add the top-level modes for this shader.
if (glslangIntermediate->getXfbMode()) {
builder.addCapability(spv::CapabilityTransformFeedback);
builder.addExecutionMode(shaderEntry, spv::ExecutionModeXfb);
}
if (glslangIntermediate->getLayoutPrimitiveCulling()) {
builder.addCapability(spv::CapabilityRayTraversalPrimitiveCullingKHR);
}
if (glslangIntermediate->getSubgroupUniformControlFlow()) {
builder.addExtension(spv::E_SPV_KHR_subgroup_uniform_control_flow);
builder.addExecutionMode(shaderEntry, spv::ExecutionModeSubgroupUniformControlFlowKHR);
}
if (glslangIntermediate->getMaximallyReconverges()) {
builder.addExtension(spv::E_SPV_KHR_maximal_reconvergence);
builder.addExecutionMode(shaderEntry, spv::ExecutionModeMaximallyReconvergesKHR);
}
if (glslangIntermediate->getQuadDerivMode())
{
builder.addCapability(spv::CapabilityQuadControlKHR);
builder.addExtension(spv::E_SPV_KHR_quad_control);
builder.addExecutionMode(shaderEntry, spv::ExecutionModeQuadDerivativesKHR);
}
if (glslangIntermediate->getReqFullQuadsMode())
{
builder.addCapability(spv::CapabilityQuadControlKHR);
builder.addExtension(spv::E_SPV_KHR_quad_control);
builder.addExecutionMode(shaderEntry, spv::ExecutionModeRequireFullQuadsKHR);
}
unsigned int mode;
switch (glslangIntermediate->getStage()) {
case EShLangVertex:
builder.addCapability(spv::CapabilityShader);
break;
case EShLangFragment:
builder.addCapability(spv::CapabilityShader);
if (glslangIntermediate->getPixelCenterInteger())
builder.addExecutionMode(shaderEntry, spv::ExecutionModePixelCenterInteger);
if (glslangIntermediate->getOriginUpperLeft())
builder.addExecutionMode(shaderEntry, spv::ExecutionModeOriginUpperLeft);
else
builder.addExecutionMode(shaderEntry, spv::ExecutionModeOriginLowerLeft);
if (glslangIntermediate->getEarlyFragmentTests())
builder.addExecutionMode(shaderEntry, spv::ExecutionModeEarlyFragmentTests);
if (glslangIntermediate->getEarlyAndLateFragmentTestsAMD())
{
builder.addExecutionMode(shaderEntry, spv::ExecutionModeEarlyAndLateFragmentTestsAMD);
builder.addExtension(spv::E_SPV_AMD_shader_early_and_late_fragment_tests);
}
if (glslangIntermediate->getPostDepthCoverage()) {
builder.addCapability(spv::CapabilitySampleMaskPostDepthCoverage);
builder.addExecutionMode(shaderEntry, spv::ExecutionModePostDepthCoverage);
builder.addExtension(spv::E_SPV_KHR_post_depth_coverage);
}
if (glslangIntermediate->getNonCoherentColorAttachmentReadEXT()) {
builder.addCapability(spv::CapabilityTileImageColorReadAccessEXT);
builder.addExecutionMode(shaderEntry, spv::ExecutionModeNonCoherentColorAttachmentReadEXT);
builder.addExtension(spv::E_SPV_EXT_shader_tile_image);
}
if (glslangIntermediate->getNonCoherentDepthAttachmentReadEXT()) {
builder.addCapability(spv::CapabilityTileImageDepthReadAccessEXT);
builder.addExecutionMode(shaderEntry, spv::ExecutionModeNonCoherentDepthAttachmentReadEXT);
builder.addExtension(spv::E_SPV_EXT_shader_tile_image);
}
if (glslangIntermediate->getNonCoherentStencilAttachmentReadEXT()) {
builder.addCapability(spv::CapabilityTileImageStencilReadAccessEXT);
builder.addExecutionMode(shaderEntry, spv::ExecutionModeNonCoherentStencilAttachmentReadEXT);
builder.addExtension(spv::E_SPV_EXT_shader_tile_image);
}
if (glslangIntermediate->isDepthReplacing())
builder.addExecutionMode(shaderEntry, spv::ExecutionModeDepthReplacing);
if (glslangIntermediate->isStencilReplacing())
builder.addExecutionMode(shaderEntry, spv::ExecutionModeStencilRefReplacingEXT);
switch(glslangIntermediate->getDepth()) {
case glslang::EldGreater: mode = spv::ExecutionModeDepthGreater; break;
case glslang::EldLess: mode = spv::ExecutionModeDepthLess; break;
case glslang::EldUnchanged: mode = spv::ExecutionModeDepthUnchanged; break;
default: mode = spv::ExecutionModeMax; break;
}
if (mode != spv::ExecutionModeMax)
builder.addExecutionMode(shaderEntry, (spv::ExecutionMode)mode);
switch (glslangIntermediate->getStencil()) {
case glslang::ElsRefUnchangedFrontAMD: mode = spv::ExecutionModeStencilRefUnchangedFrontAMD; break;
case glslang::ElsRefGreaterFrontAMD: mode = spv::ExecutionModeStencilRefGreaterFrontAMD; break;
case glslang::ElsRefLessFrontAMD: mode = spv::ExecutionModeStencilRefLessFrontAMD; break;
case glslang::ElsRefUnchangedBackAMD: mode = spv::ExecutionModeStencilRefUnchangedBackAMD; break;
case glslang::ElsRefGreaterBackAMD: mode = spv::ExecutionModeStencilRefGreaterBackAMD; break;
case glslang::ElsRefLessBackAMD: mode = spv::ExecutionModeStencilRefLessBackAMD; break;
default: mode = spv::ExecutionModeMax; break;
}
if (mode != spv::ExecutionModeMax)
builder.addExecutionMode(shaderEntry, (spv::ExecutionMode)mode);
switch (glslangIntermediate->getInterlockOrdering()) {
case glslang::EioPixelInterlockOrdered: mode = spv::ExecutionModePixelInterlockOrderedEXT;
break;
case glslang::EioPixelInterlockUnordered: mode = spv::ExecutionModePixelInterlockUnorderedEXT;
break;
case glslang::EioSampleInterlockOrdered: mode = spv::ExecutionModeSampleInterlockOrderedEXT;
break;
case glslang::EioSampleInterlockUnordered: mode = spv::ExecutionModeSampleInterlockUnorderedEXT;
break;
case glslang::EioShadingRateInterlockOrdered: mode = spv::ExecutionModeShadingRateInterlockOrderedEXT;
break;
case glslang::EioShadingRateInterlockUnordered: mode = spv::ExecutionModeShadingRateInterlockUnorderedEXT;
break;
default: mode = spv::ExecutionModeMax;
break;
}
if (mode != spv::ExecutionModeMax) {
builder.addExecutionMode(shaderEntry, (spv::ExecutionMode)mode);
if (mode == spv::ExecutionModeShadingRateInterlockOrderedEXT ||
mode == spv::ExecutionModeShadingRateInterlockUnorderedEXT) {
builder.addCapability(spv::CapabilityFragmentShaderShadingRateInterlockEXT);
} else if (mode == spv::ExecutionModePixelInterlockOrderedEXT ||
mode == spv::ExecutionModePixelInterlockUnorderedEXT) {
builder.addCapability(spv::CapabilityFragmentShaderPixelInterlockEXT);
} else {
builder.addCapability(spv::CapabilityFragmentShaderSampleInterlockEXT);
}
builder.addExtension(spv::E_SPV_EXT_fragment_shader_interlock);
}
break;
case EShLangCompute: {
builder.addCapability(spv::CapabilityShader);
bool needSizeId = false;
for (int dim = 0; dim < 3; ++dim) {
if ((glslangIntermediate->getLocalSizeSpecId(dim) != glslang::TQualifier::layoutNotSet)) {
needSizeId = true;
break;
}
}
if (glslangIntermediate->getSpv().spv >= glslang::EShTargetSpv_1_6 && needSizeId) {
std::vector<spv::Id> dimConstId;
for (int dim = 0; dim < 3; ++dim) {
bool specConst = (glslangIntermediate->getLocalSizeSpecId(dim) != glslang::TQualifier::layoutNotSet);
dimConstId.push_back(builder.makeUintConstant(glslangIntermediate->getLocalSize(dim), specConst));
if (specConst) {
builder.addDecoration(dimConstId.back(), spv::DecorationSpecId,
glslangIntermediate->getLocalSizeSpecId(dim));
needSizeId = true;
}
}
builder.addExecutionModeId(shaderEntry, spv::ExecutionModeLocalSizeId, dimConstId);
} else {
builder.addExecutionMode(shaderEntry, spv::ExecutionModeLocalSize, glslangIntermediate->getLocalSize(0),
glslangIntermediate->getLocalSize(1),
glslangIntermediate->getLocalSize(2));
}
if (glslangIntermediate->getLayoutDerivativeModeNone() == glslang::LayoutDerivativeGroupQuads) {
builder.addCapability(spv::CapabilityComputeDerivativeGroupQuadsNV);
builder.addExecutionMode(shaderEntry, spv::ExecutionModeDerivativeGroupQuadsNV);
builder.addExtension(spv::E_SPV_NV_compute_shader_derivatives);
} else if (glslangIntermediate->getLayoutDerivativeModeNone() == glslang::LayoutDerivativeGroupLinear) {
builder.addCapability(spv::CapabilityComputeDerivativeGroupLinearNV);
builder.addExecutionMode(shaderEntry, spv::ExecutionModeDerivativeGroupLinearNV);
builder.addExtension(spv::E_SPV_NV_compute_shader_derivatives);
}
break;
}
case EShLangTessEvaluation:
case EShLangTessControl:
builder.addCapability(spv::CapabilityTessellation);
glslang::TLayoutGeometry primitive;
if (glslangIntermediate->getStage() == EShLangTessControl) {
builder.addExecutionMode(shaderEntry, spv::ExecutionModeOutputVertices,
glslangIntermediate->getVertices());
primitive = glslangIntermediate->getOutputPrimitive();
} else {
primitive = glslangIntermediate->getInputPrimitive();
}
switch (primitive) {
case glslang::ElgTriangles: mode = spv::ExecutionModeTriangles; break;
case glslang::ElgQuads: mode = spv::ExecutionModeQuads; break;
case glslang::ElgIsolines: mode = spv::ExecutionModeIsolines; break;
default: mode = spv::ExecutionModeMax; break;
}
if (mode != spv::ExecutionModeMax)
builder.addExecutionMode(shaderEntry, (spv::ExecutionMode)mode);
switch (glslangIntermediate->getVertexSpacing()) {
case glslang::EvsEqual: mode = spv::ExecutionModeSpacingEqual; break;
case glslang::EvsFractionalEven: mode = spv::ExecutionModeSpacingFractionalEven; break;
case glslang::EvsFractionalOdd: mode = spv::ExecutionModeSpacingFractionalOdd; break;
default: mode = spv::ExecutionModeMax; break;
}
if (mode != spv::ExecutionModeMax)
builder.addExecutionMode(shaderEntry, (spv::ExecutionMode)mode);
switch (glslangIntermediate->getVertexOrder()) {
case glslang::EvoCw: mode = spv::ExecutionModeVertexOrderCw; break;
case glslang::EvoCcw: mode = spv::ExecutionModeVertexOrderCcw; break;
default: mode = spv::ExecutionModeMax; break;
}
if (mode != spv::ExecutionModeMax)
builder.addExecutionMode(shaderEntry, (spv::ExecutionMode)mode);
if (glslangIntermediate->getPointMode())
builder.addExecutionMode(shaderEntry, spv::ExecutionModePointMode);
break;
case EShLangGeometry:
builder.addCapability(spv::CapabilityGeometry);
switch (glslangIntermediate->getInputPrimitive()) {
case glslang::ElgPoints: mode = spv::ExecutionModeInputPoints; break;
case glslang::ElgLines: mode = spv::ExecutionModeInputLines; break;
case glslang::ElgLinesAdjacency: mode = spv::ExecutionModeInputLinesAdjacency; break;
case glslang::ElgTriangles: mode = spv::ExecutionModeTriangles; break;
case glslang::ElgTrianglesAdjacency: mode = spv::ExecutionModeInputTrianglesAdjacency; break;
default: mode = spv::ExecutionModeMax; break;
}
if (mode != spv::ExecutionModeMax)
builder.addExecutionMode(shaderEntry, (spv::ExecutionMode)mode);
builder.addExecutionMode(shaderEntry, spv::ExecutionModeInvocations, glslangIntermediate->getInvocations());
switch (glslangIntermediate->getOutputPrimitive()) {
case glslang::ElgPoints: mode = spv::ExecutionModeOutputPoints; break;
case glslang::ElgLineStrip: mode = spv::ExecutionModeOutputLineStrip; break;
case glslang::ElgTriangleStrip: mode = spv::ExecutionModeOutputTriangleStrip; break;
default: mode = spv::ExecutionModeMax; break;
}
if (mode != spv::ExecutionModeMax)
builder.addExecutionMode(shaderEntry, (spv::ExecutionMode)mode);
builder.addExecutionMode(shaderEntry, spv::ExecutionModeOutputVertices, glslangIntermediate->getVertices());
break;
case EShLangRayGen:
case EShLangIntersect:
case EShLangAnyHit:
case EShLangClosestHit:
case EShLangMiss:
case EShLangCallable:
{
auto& extensions = glslangIntermediate->getRequestedExtensions();
if (extensions.find("GL_NV_ray_tracing") == extensions.end()) {
builder.addCapability(spv::CapabilityRayTracingKHR);
builder.addExtension("SPV_KHR_ray_tracing");
}
else {
builder.addCapability(spv::CapabilityRayTracingNV);
builder.addExtension("SPV_NV_ray_tracing");
}
if (glslangIntermediate->getStage() != EShLangRayGen && glslangIntermediate->getStage() != EShLangCallable) {
if (extensions.find("GL_EXT_ray_cull_mask") != extensions.end()) {
builder.addCapability(spv::CapabilityRayCullMaskKHR);
builder.addExtension("SPV_KHR_ray_cull_mask");
}
if (extensions.find("GL_EXT_ray_tracing_position_fetch") != extensions.end()) {
builder.addCapability(spv::CapabilityRayTracingPositionFetchKHR);
builder.addExtension("SPV_KHR_ray_tracing_position_fetch");
}
}
break;
}
case EShLangTask:
case EShLangMesh:
if(isMeshShaderExt) {
builder.addCapability(spv::CapabilityMeshShadingEXT);
builder.addExtension(spv::E_SPV_EXT_mesh_shader);
} else {
builder.addCapability(spv::CapabilityMeshShadingNV);
builder.addExtension(spv::E_SPV_NV_mesh_shader);
}
if (glslangIntermediate->getSpv().spv >= glslang::EShTargetSpv_1_6) {
std::vector<spv::Id> dimConstId;
for (int dim = 0; dim < 3; ++dim) {
bool specConst = (glslangIntermediate->getLocalSizeSpecId(dim) != glslang::TQualifier::layoutNotSet);
dimConstId.push_back(builder.makeUintConstant(glslangIntermediate->getLocalSize(dim), specConst));
if (specConst) {
builder.addDecoration(dimConstId.back(), spv::DecorationSpecId,
glslangIntermediate->getLocalSizeSpecId(dim));
}
}
builder.addExecutionModeId(shaderEntry, spv::ExecutionModeLocalSizeId, dimConstId);
} else {
builder.addExecutionMode(shaderEntry, spv::ExecutionModeLocalSize, glslangIntermediate->getLocalSize(0),
glslangIntermediate->getLocalSize(1),
glslangIntermediate->getLocalSize(2));
}
if (glslangIntermediate->getStage() == EShLangMesh) {
builder.addExecutionMode(shaderEntry, spv::ExecutionModeOutputVertices,
glslangIntermediate->getVertices());
builder.addExecutionMode(shaderEntry, spv::ExecutionModeOutputPrimitivesNV,
glslangIntermediate->getPrimitives());
switch (glslangIntermediate->getOutputPrimitive()) {
case glslang::ElgPoints: mode = spv::ExecutionModeOutputPoints; break;
case glslang::ElgLines: mode = spv::ExecutionModeOutputLinesNV; break;
case glslang::ElgTriangles: mode = spv::ExecutionModeOutputTrianglesNV; break;
default: mode = spv::ExecutionModeMax; break;
}
if (mode != spv::ExecutionModeMax)
builder.addExecutionMode(shaderEntry, (spv::ExecutionMode)mode);
}
break;
default:
break;
}
//
// Add SPIR-V requirements (GL_EXT_spirv_intrinsics)
//
if (glslangIntermediate->hasSpirvRequirement()) {
const glslang::TSpirvRequirement& spirvRequirement = glslangIntermediate->getSpirvRequirement();
// Add SPIR-V extension requirement
for (auto& extension : spirvRequirement.extensions)
builder.addExtension(extension.c_str());
// Add SPIR-V capability requirement
for (auto capability : spirvRequirement.capabilities)
builder.addCapability(static_cast<spv::Capability>(capability));
}
//
// Add SPIR-V execution mode qualifiers (GL_EXT_spirv_intrinsics)
//
if (glslangIntermediate->hasSpirvExecutionMode()) {
const glslang::TSpirvExecutionMode spirvExecutionMode = glslangIntermediate->getSpirvExecutionMode();
// Add spirv_execution_mode
for (auto& mode : spirvExecutionMode.modes) {
if (!mode.second.empty()) {
std::vector<unsigned> literals;
TranslateLiterals(mode.second, literals);
builder.addExecutionMode(shaderEntry, static_cast<spv::ExecutionMode>(mode.first), literals);
} else
builder.addExecutionMode(shaderEntry, static_cast<spv::ExecutionMode>(mode.first));
}
// Add spirv_execution_mode_id
for (auto& modeId : spirvExecutionMode.modeIds) {
std::vector<spv::Id> operandIds;
assert(!modeId.second.empty());
for (auto extraOperand : modeId.second) {
if (extraOperand->getType().getQualifier().isSpecConstant())
operandIds.push_back(getSymbolId(extraOperand->getAsSymbolNode()));
else
operandIds.push_back(createSpvConstant(*extraOperand));
}
builder.addExecutionModeId(shaderEntry, static_cast<spv::ExecutionMode>(modeId.first), operandIds);
}
}
}
// Finish creating SPV, after the traversal is complete.
void TGlslangToSpvTraverser::finishSpv(bool compileOnly)
{
// If not linking, an entry point is not expected
if (!compileOnly) {
// Finish the entry point function
if (!entryPointTerminated) {
builder.setBuildPoint(shaderEntry->getLastBlock());
builder.leaveFunction();
}
// finish off the entry-point SPV instruction by adding the Input/Output <id>
entryPoint->reserveOperands(iOSet.size());
for (auto id : iOSet)
entryPoint->addIdOperand(id);
}
// Add capabilities, extensions, remove unneeded decorations, etc.,
// based on the resulting SPIR-V.
// Note: WebGPU code generation must have the opportunity to aggressively
// prune unreachable merge blocks and continue targets.
builder.postProcess(compileOnly);
}
// Write the SPV into 'out'.
void TGlslangToSpvTraverser::dumpSpv(std::vector<unsigned int>& out)
{
builder.dump(out);
}
//
// Implement the traversal functions.
//
// Return true from interior nodes to have the external traversal
// continue on to children. Return false if children were
// already processed.
//
//
// Symbols can turn into
// - uniform/input reads
// - output writes
// - complex lvalue base setups: foo.bar[3].... , where we see foo and start up an access chain
// - something simple that degenerates into the last bullet
//
void TGlslangToSpvTraverser::visitSymbol(glslang::TIntermSymbol* symbol)
{
// We update the line information even though no code might be generated here
// This is helpful to yield correct lines for control flow instructions
if (!linkageOnly) {
builder.setDebugSourceLocation(symbol->getLoc().line, symbol->getLoc().getFilename());
}
SpecConstantOpModeGuard spec_constant_op_mode_setter(&builder);
if (symbol->getType().isStruct())
glslangTypeToIdMap[symbol->getType().getStruct()] = symbol->getId();
if (symbol->getType().getQualifier().isSpecConstant())
spec_constant_op_mode_setter.turnOnSpecConstantOpMode();
#ifdef ENABLE_HLSL
// Skip symbol handling if it is string-typed
if (symbol->getBasicType() == glslang::EbtString)
return;
#endif
// getSymbolId() will set up all the IO decorations on the first call.
// Formal function parameters were mapped during makeFunctions().
spv::Id id = getSymbolId(symbol);
if (symbol->getType().getQualifier().isTaskPayload())
taskPayloadID = id; // cache the taskPayloadID to be used it as operand for OpEmitMeshTasksEXT
if (builder.isPointer(id)) {
if (!symbol->getType().getQualifier().isParamInput() &&
!symbol->getType().getQualifier().isParamOutput()) {
// Include all "static use" and "linkage only" interface variables on the OpEntryPoint instruction
// Consider adding to the OpEntryPoint interface list.
// Only looking at structures if they have at least one member.
if (!symbol->getType().isStruct() || symbol->getType().getStruct()->size() > 0) {
spv::StorageClass sc = builder.getStorageClass(id);
// Before SPIR-V 1.4, we only want to include Input and Output.
// Starting with SPIR-V 1.4, we want all globals.
if ((glslangIntermediate->getSpv().spv >= glslang::EShTargetSpv_1_4 && builder.isGlobalVariable(id)) ||
(sc == spv::StorageClassInput || sc == spv::StorageClassOutput)) {
iOSet.insert(id);
}
}
}
// If the SPIR-V type is required to be different than the AST type
// (for ex SubgroupMasks or 3x4 ObjectToWorld/WorldToObject matrices),
// translate now from the SPIR-V type to the AST type, for the consuming
// operation.
// Note this turns it from an l-value to an r-value.
// Currently, all symbols needing this are inputs; avoid the map lookup when non-input.
if (symbol->getType().getQualifier().storage == glslang::EvqVaryingIn)
id = translateForcedType(id);
}
// Only process non-linkage-only nodes for generating actual static uses
if (! linkageOnly || symbol->getQualifier().isSpecConstant()) {
// Prepare to generate code for the access
// L-value chains will be computed left to right. We're on the symbol now,
// which is the left-most part of the access chain, so now is "clear" time,
// followed by setting the base.
builder.clearAccessChain();
// For now, we consider all user variables as being in memory, so they are pointers,
// except for
// A) R-Value arguments to a function, which are an intermediate object.
// See comments in handleUserFunctionCall().
// B) Specialization constants (normal constants don't even come in as a variable),
// These are also pure R-values.
// C) R-Values from type translation, see above call to translateForcedType()
glslang::TQualifier qualifier = symbol->getQualifier();
if (qualifier.isSpecConstant() || rValueParameters.find(symbol->getId()) != rValueParameters.end() ||
!builder.isPointerType(builder.getTypeId(id)))
builder.setAccessChainRValue(id);
else
builder.setAccessChainLValue(id);
}
#ifdef ENABLE_HLSL
// Process linkage-only nodes for any special additional interface work.
if (linkageOnly) {
if (glslangIntermediate->getHlslFunctionality1()) {
// Map implicit counter buffers to their originating buffers, which should have been
// seen by now, given earlier pruning of unused counters, and preservation of order
// of declaration.
if (symbol->getType().getQualifier().isUniformOrBuffer()) {
if (!glslangIntermediate->hasCounterBufferName(symbol->getName())) {
// Save possible originating buffers for counter buffers, keyed by
// making the potential counter-buffer name.
std::string keyName = symbol->getName().c_str();
keyName = glslangIntermediate->addCounterBufferName(keyName);
counterOriginator[keyName] = symbol;
} else {
// Handle a counter buffer, by finding the saved originating buffer.
std::string keyName = symbol->getName().c_str();
auto it = counterOriginator.find(keyName);
if (it != counterOriginator.end()) {
id = getSymbolId(it->second);
if (id != spv::NoResult) {
spv::Id counterId = getSymbolId(symbol);
if (counterId != spv::NoResult) {
builder.addExtension("SPV_GOOGLE_hlsl_functionality1");
builder.addDecorationId(id, spv::DecorationHlslCounterBufferGOOGLE, counterId);
}
}
}
}
}
}
}
#endif
}
bool TGlslangToSpvTraverser::visitBinary(glslang::TVisit /* visit */, glslang::TIntermBinary* node)
{
builder.setDebugSourceLocation(node->getLoc().line, node->getLoc().getFilename());
if (node->getLeft()->getAsSymbolNode() != nullptr && node->getLeft()->getType().isStruct()) {
glslangTypeToIdMap[node->getLeft()->getType().getStruct()] = node->getLeft()->getAsSymbolNode()->getId();
}
if (node->getRight()->getAsSymbolNode() != nullptr && node->getRight()->getType().isStruct()) {
glslangTypeToIdMap[node->getRight()->getType().getStruct()] = node->getRight()->getAsSymbolNode()->getId();
}
SpecConstantOpModeGuard spec_constant_op_mode_setter(&builder);
if (node->getType().getQualifier().isSpecConstant())
spec_constant_op_mode_setter.turnOnSpecConstantOpMode();
// First, handle special cases
switch (node->getOp()) {
case glslang::EOpAssign:
case glslang::EOpAddAssign:
case glslang::EOpSubAssign:
case glslang::EOpMulAssign:
case glslang::EOpVectorTimesMatrixAssign:
case glslang::EOpVectorTimesScalarAssign:
case glslang::EOpMatrixTimesScalarAssign:
case glslang::EOpMatrixTimesMatrixAssign:
case glslang::EOpDivAssign:
case glslang::EOpModAssign:
case glslang::EOpAndAssign:
case glslang::EOpInclusiveOrAssign:
case glslang::EOpExclusiveOrAssign:
case glslang::EOpLeftShiftAssign:
case glslang::EOpRightShiftAssign:
// A bin-op assign "a += b" means the same thing as "a = a + b"
// where a is evaluated before b. For a simple assignment, GLSL
// says to evaluate the left before the right. So, always, left
// node then right node.
{
// get the left l-value, save it away
builder.clearAccessChain();
node->getLeft()->traverse(this);
spv::Builder::AccessChain lValue = builder.getAccessChain();
// evaluate the right
builder.clearAccessChain();
node->getRight()->traverse(this);
spv::Id rValue = accessChainLoad(node->getRight()->getType());
// reset line number for assignment
builder.setDebugSourceLocation(node->getLoc().line, node->getLoc().getFilename());
if (node->getOp() != glslang::EOpAssign) {
// the left is also an r-value
builder.setAccessChain(lValue);
spv::Id leftRValue = accessChainLoad(node->getLeft()->getType());
// do the operation
spv::Builder::AccessChain::CoherentFlags coherentFlags = TranslateCoherent(node->getLeft()->getType());
coherentFlags |= TranslateCoherent(node->getRight()->getType());
OpDecorations decorations = { TranslatePrecisionDecoration(node->getOperationPrecision()),
TranslateNoContractionDecoration(node->getType().getQualifier()),
TranslateNonUniformDecoration(coherentFlags) };
rValue = createBinaryOperation(node->getOp(), decorations,
convertGlslangToSpvType(node->getType()), leftRValue, rValue,
node->getType().getBasicType());
// these all need their counterparts in createBinaryOperation()
assert(rValue != spv::NoResult);
}
// store the result
builder.setAccessChain(lValue);
multiTypeStore(node->getLeft()->getType(), rValue);
// assignments are expressions having an rValue after they are evaluated...
builder.clearAccessChain();
builder.setAccessChainRValue(rValue);
}
return false;
case glslang::EOpIndexDirect:
case glslang::EOpIndexDirectStruct:
{
// Structure, array, matrix, or vector indirection with statically known index.
// Get the left part of the access chain.
node->getLeft()->traverse(this);
// Add the next element in the chain
const int glslangIndex = node->getRight()->getAsConstantUnion()->getConstArray()[0].getIConst();
if (! node->getLeft()->getType().isArray() &&
node->getLeft()->getType().isVector() &&
node->getOp() == glslang::EOpIndexDirect) {
// Swizzle is uniform so propagate uniform into access chain
spv::Builder::AccessChain::CoherentFlags coherentFlags = TranslateCoherent(node->getLeft()->getType());
coherentFlags.nonUniform = 0;
// This is essentially a hard-coded vector swizzle of size 1,
// so short circuit the access-chain stuff with a swizzle.
std::vector<unsigned> swizzle;
swizzle.push_back(glslangIndex);
int dummySize;
builder.accessChainPushSwizzle(swizzle, convertGlslangToSpvType(node->getLeft()->getType()),
coherentFlags,
glslangIntermediate->getBaseAlignmentScalar(
node->getLeft()->getType(), dummySize));
} else {
// Load through a block reference is performed with a dot operator that
// is mapped to EOpIndexDirectStruct. When we get to the actual reference,
// do a load and reset the access chain.
if (node->getLeft()->isReference() &&
!node->getLeft()->getType().isArray() &&
node->getOp() == glslang::EOpIndexDirectStruct)
{
spv::Id left = accessChainLoad(node->getLeft()->getType());
builder.clearAccessChain();
builder.setAccessChainLValue(left);
}
int spvIndex = glslangIndex;
if (node->getLeft()->getBasicType() == glslang::EbtBlock &&
node->getOp() == glslang::EOpIndexDirectStruct)
{
// This may be, e.g., an anonymous block-member selection, which generally need
// index remapping due to hidden members in anonymous blocks.
long long glslangId = glslangTypeToIdMap[node->getLeft()->getType().getStruct()];
if (memberRemapper.find(glslangId) != memberRemapper.end()) {
std::vector<int>& remapper = memberRemapper[glslangId];
assert(remapper.size() > 0);
spvIndex = remapper[glslangIndex];
}
}
// Struct reference propagates uniform lvalue
spv::Builder::AccessChain::CoherentFlags coherentFlags =
TranslateCoherent(node->getLeft()->getType());
coherentFlags.nonUniform = 0;
// normal case for indexing array or structure or block
builder.accessChainPush(builder.makeIntConstant(spvIndex),
coherentFlags,
node->getLeft()->getType().getBufferReferenceAlignment());
// Add capabilities here for accessing PointSize and clip/cull distance.
// We have deferred generation of associated capabilities until now.
if (node->getLeft()->getType().isStruct() && ! node->getLeft()->getType().isArray())
declareUseOfStructMember(*(node->getLeft()->getType().getStruct()), glslangIndex);
}
}
return false;
case glslang::EOpIndexIndirect:
{
// Array, matrix, or vector indirection with variable index.
// Will use native SPIR-V access-chain for and array indirection;
// matrices are arrays of vectors, so will also work for a matrix.
// Will use the access chain's 'component' for variable index into a vector.
// This adapter is building access chains left to right.
// Set up the access chain to the left.
node->getLeft()->traverse(this);
// save it so that computing the right side doesn't trash it
spv::Builder::AccessChain partial = builder.getAccessChain();
// compute the next index in the chain
builder.clearAccessChain();
node->getRight()->traverse(this);
spv::Id index = accessChainLoad(node->getRight()->getType());
addIndirectionIndexCapabilities(node->getLeft()->getType(), node->getRight()->getType());
// restore the saved access chain
builder.setAccessChain(partial);
// Only if index is nonUniform should we propagate nonUniform into access chain
spv::Builder::AccessChain::CoherentFlags index_flags = TranslateCoherent(node->getRight()->getType());
spv::Builder::AccessChain::CoherentFlags coherent_flags = TranslateCoherent(node->getLeft()->getType());
coherent_flags.nonUniform = index_flags.nonUniform;
if (! node->getLeft()->getType().isArray() && node->getLeft()->getType().isVector()) {
int dummySize;
builder.accessChainPushComponent(
index, convertGlslangToSpvType(node->getLeft()->getType()), coherent_flags,
glslangIntermediate->getBaseAlignmentScalar(node->getLeft()->getType(),
dummySize));
} else
builder.accessChainPush(index, coherent_flags,
node->getLeft()->getType().getBufferReferenceAlignment());
}
return false;
case glslang::EOpVectorSwizzle:
{
node->getLeft()->traverse(this);
std::vector<unsigned> swizzle;
convertSwizzle(*node->getRight()->getAsAggregate(), swizzle);
int dummySize;
builder.accessChainPushSwizzle(swizzle, convertGlslangToSpvType(node->getLeft()->getType()),
TranslateCoherent(node->getLeft()->getType()),
glslangIntermediate->getBaseAlignmentScalar(node->getLeft()->getType(),
dummySize));
}
return false;
case glslang::EOpMatrixSwizzle:
logger->missingFunctionality("matrix swizzle");
return true;
case glslang::EOpLogicalOr:
case glslang::EOpLogicalAnd:
{
// These may require short circuiting, but can sometimes be done as straight
// binary operations. The right operand must be short circuited if it has
// side effects, and should probably be if it is complex.
if (isTrivial(node->getRight()->getAsTyped()))
break; // handle below as a normal binary operation
// otherwise, we need to do dynamic short circuiting on the right operand
spv::Id result = createShortCircuit(node->getOp(), *node->getLeft()->getAsTyped(),
*node->getRight()->getAsTyped());
builder.clearAccessChain();
builder.setAccessChainRValue(result);
}
return false;
default:
break;
}
// Assume generic binary op...
// get right operand
builder.clearAccessChain();
node->getLeft()->traverse(this);
spv::Id left = accessChainLoad(node->getLeft()->getType());
// get left operand
builder.clearAccessChain();
node->getRight()->traverse(this);
spv::Id right = accessChainLoad(node->getRight()->getType());
// get result
OpDecorations decorations = { TranslatePrecisionDecoration(node->getOperationPrecision()),
TranslateNoContractionDecoration(node->getType().getQualifier()),
TranslateNonUniformDecoration(node->getType().getQualifier()) };
spv::Id result = createBinaryOperation(node->getOp(), decorations,
convertGlslangToSpvType(node->getType()), left, right,
node->getLeft()->getType().getBasicType());
builder.clearAccessChain();
if (! result) {
logger->missingFunctionality("unknown glslang binary operation");
return true; // pick up a child as the place-holder result
} else {
builder.setAccessChainRValue(result);
return false;
}
}
spv::Id TGlslangToSpvTraverser::convertLoadedBoolInUniformToUint(const glslang::TType& type,
spv::Id nominalTypeId,
spv::Id loadedId)
{
if (builder.isScalarType(nominalTypeId)) {
// Conversion for bool
spv::Id boolType = builder.makeBoolType();
if (nominalTypeId != boolType)
return builder.createBinOp(spv::OpINotEqual, boolType, loadedId, builder.makeUintConstant(0));
} else if (builder.isVectorType(nominalTypeId)) {
// Conversion for bvec
int vecSize = builder.getNumTypeComponents(nominalTypeId);
spv::Id bvecType = builder.makeVectorType(builder.makeBoolType(), vecSize);
if (nominalTypeId != bvecType)
loadedId = builder.createBinOp(spv::OpINotEqual, bvecType, loadedId,
makeSmearedConstant(builder.makeUintConstant(0), vecSize));
} else if (builder.isArrayType(nominalTypeId)) {
// Conversion for bool array
spv::Id boolArrayTypeId = convertGlslangToSpvType(type);
if (nominalTypeId != boolArrayTypeId)
{
// Use OpCopyLogical from SPIR-V 1.4 if available.
if (glslangIntermediate->getSpv().spv >= glslang::EShTargetSpv_1_4)
return builder.createUnaryOp(spv::OpCopyLogical, boolArrayTypeId, loadedId);
glslang::TType glslangElementType(type, 0);
spv::Id elementNominalTypeId = builder.getContainedTypeId(nominalTypeId);
std::vector<spv::Id> constituents;
for (int index = 0; index < type.getOuterArraySize(); ++index) {
// get the element
spv::Id elementValue = builder.createCompositeExtract(loadedId, elementNominalTypeId, index);
// recursively convert it
spv::Id elementConvertedValue = convertLoadedBoolInUniformToUint(glslangElementType, elementNominalTypeId, elementValue);
constituents.push_back(elementConvertedValue);
}
return builder.createCompositeConstruct(boolArrayTypeId, constituents);
}
}
return loadedId;
}
// Figure out what, if any, type changes are needed when accessing a specific built-in.
// Returns <the type SPIR-V requires for declarion, the type to translate to on use>.
// Also see comment for 'forceType', regarding tracking SPIR-V-required types.
std::pair<spv::Id, spv::Id> TGlslangToSpvTraverser::getForcedType(glslang::TBuiltInVariable glslangBuiltIn,
const glslang::TType& glslangType)
{
switch(glslangBuiltIn)
{
case glslang::EbvSubGroupEqMask:
case glslang::EbvSubGroupGeMask:
case glslang::EbvSubGroupGtMask:
case glslang::EbvSubGroupLeMask:
case glslang::EbvSubGroupLtMask: {
// these require changing a 64-bit scaler -> a vector of 32-bit components
if (glslangType.isVector())
break;
spv::Id ivec4_type = builder.makeVectorType(builder.makeUintType(32), 4);
spv::Id uint64_type = builder.makeUintType(64);
std::pair<spv::Id, spv::Id> ret(ivec4_type, uint64_type);
return ret;
}
// There are no SPIR-V builtins defined for these and map onto original non-transposed
// builtins. During visitBinary we insert a transpose
case glslang::EbvWorldToObject3x4:
case glslang::EbvObjectToWorld3x4: {
spv::Id mat43 = builder.makeMatrixType(builder.makeFloatType(32), 4, 3);
spv::Id mat34 = builder.makeMatrixType(builder.makeFloatType(32), 3, 4);
std::pair<spv::Id, spv::Id> ret(mat43, mat34);
return ret;
}
default:
break;
}
std::pair<spv::Id, spv::Id> ret(spv::NoType, spv::NoType);
return ret;
}
// For an object previously identified (see getForcedType() and forceType)
// as needing type translations, do the translation needed for a load, turning
// an L-value into in R-value.
spv::Id TGlslangToSpvTraverser::translateForcedType(spv::Id object)
{
const auto forceIt = forceType.find(object);
if (forceIt == forceType.end())
return object;
spv::Id desiredTypeId = forceIt->second;
spv::Id objectTypeId = builder.getTypeId(object);
assert(builder.isPointerType(objectTypeId));
objectTypeId = builder.getContainedTypeId(objectTypeId);
if (builder.isVectorType(objectTypeId) &&
builder.getScalarTypeWidth(builder.getContainedTypeId(objectTypeId)) == 32) {
if (builder.getScalarTypeWidth(desiredTypeId) == 64) {
// handle 32-bit v.xy* -> 64-bit
builder.clearAccessChain();
builder.setAccessChainLValue(object);
object = builder.accessChainLoad(spv::NoPrecision, spv::DecorationMax, spv::DecorationMax, objectTypeId);
std::vector<spv::Id> components;
components.push_back(builder.createCompositeExtract(object, builder.getContainedTypeId(objectTypeId), 0));
components.push_back(builder.createCompositeExtract(object, builder.getContainedTypeId(objectTypeId), 1));
spv::Id vecType = builder.makeVectorType(builder.getContainedTypeId(objectTypeId), 2);
return builder.createUnaryOp(spv::OpBitcast, desiredTypeId,
builder.createCompositeConstruct(vecType, components));
} else {
logger->missingFunctionality("forcing 32-bit vector type to non 64-bit scalar");
}
} else if (builder.isMatrixType(objectTypeId)) {
// There are no SPIR-V builtins defined for 3x4 variants of ObjectToWorld/WorldToObject
// and we insert a transpose after loading the original non-transposed builtins
builder.clearAccessChain();
builder.setAccessChainLValue(object);
object = builder.accessChainLoad(spv::NoPrecision, spv::DecorationMax, spv::DecorationMax, objectTypeId);
return builder.createUnaryOp(spv::OpTranspose, desiredTypeId, object);
} else {
logger->missingFunctionality("forcing non 32-bit vector type");
}
return object;
}
bool TGlslangToSpvTraverser::visitUnary(glslang::TVisit /* visit */, glslang::TIntermUnary* node)
{
builder.setDebugSourceLocation(node->getLoc().line, node->getLoc().getFilename());
SpecConstantOpModeGuard spec_constant_op_mode_setter(&builder);
if (node->getType().getQualifier().isSpecConstant())
spec_constant_op_mode_setter.turnOnSpecConstantOpMode();
spv::Id result = spv::NoResult;
// try texturing first
result = createImageTextureFunctionCall(node);
if (result != spv::NoResult) {
builder.clearAccessChain();
builder.setAccessChainRValue(result);
return false; // done with this node
}
// Non-texturing.
if (node->getOp() == glslang::EOpArrayLength) {
// Quite special; won't want to evaluate the operand.
// Currently, the front-end does not allow .length() on an array until it is sized,
// except for the last block membeor of an SSBO.
// TODO: If this changes, link-time sized arrays might show up here, and need their
// size extracted.
// Normal .length() would have been constant folded by the front-end.
// So, this has to be block.lastMember.length().
// SPV wants "block" and member number as the operands, go get them.
spv::Id length;
if (node->getOperand()->getType().isCoopMat()) {
spv::Id typeId = convertGlslangToSpvType(node->getOperand()->getType());
assert(builder.isCooperativeMatrixType(typeId));
if (node->getOperand()->getType().isCoopMatKHR()) {
length = builder.createCooperativeMatrixLengthKHR(typeId);
} else {
spec_constant_op_mode_setter.turnOnSpecConstantOpMode();
length = builder.createCooperativeMatrixLengthNV(typeId);
}
} else {
glslang::TIntermTyped* block = node->getOperand()->getAsBinaryNode()->getLeft();
block->traverse(this);
unsigned int member = node->getOperand()->getAsBinaryNode()->getRight()->getAsConstantUnion()
->getConstArray()[0].getUConst();
length = builder.createArrayLength(builder.accessChainGetLValue(), member);
}
// GLSL semantics say the result of .length() is an int, while SPIR-V says
// signedness must be 0. So, convert from SPIR-V unsigned back to GLSL's
// AST expectation of a signed result.
if (glslangIntermediate->getSource() == glslang::EShSourceGlsl) {
if (builder.isInSpecConstCodeGenMode()) {
length = builder.createBinOp(spv::OpIAdd, builder.makeIntType(32), length, builder.makeIntConstant(0));
} else {
length = builder.createUnaryOp(spv::OpBitcast, builder.makeIntType(32), length);
}
}
builder.clearAccessChain();
builder.setAccessChainRValue(length);
return false;
}
// Force variable declaration - Debug Mode Only
if (node->getOp() == glslang::EOpDeclare) {
builder.clearAccessChain();
node->getOperand()->traverse(this);
builder.clearAccessChain();
return false;
}
// Start by evaluating the operand
// Does it need a swizzle inversion? If so, evaluation is inverted;
// operate first on the swizzle base, then apply the swizzle.
spv::Id invertedType = spv::NoType;
auto resultType = [&invertedType, &node, this](){ return invertedType != spv::NoType ?
invertedType : convertGlslangToSpvType(node->getType()); };
if (node->getOp() == glslang::EOpInterpolateAtCentroid)
invertedType = getInvertedSwizzleType(*node->getOperand());
builder.clearAccessChain();
TIntermNode *operandNode;
if (invertedType != spv::NoType)
operandNode = node->getOperand()->getAsBinaryNode()->getLeft();
else
operandNode = node->getOperand();
operandNode->traverse(this);
spv::Id operand = spv::NoResult;
spv::Builder::AccessChain::CoherentFlags lvalueCoherentFlags;
const auto hitObjectOpsWithLvalue = [](glslang::TOperator op) {
switch(op) {
case glslang::EOpReorderThreadNV:
case glslang::EOpHitObjectGetCurrentTimeNV:
case glslang::EOpHitObjectGetHitKindNV:
case glslang::EOpHitObjectGetPrimitiveIndexNV:
case glslang::EOpHitObjectGetGeometryIndexNV:
case glslang::EOpHitObjectGetInstanceIdNV:
case glslang::EOpHitObjectGetInstanceCustomIndexNV:
case glslang::EOpHitObjectGetObjectRayDirectionNV:
case glslang::EOpHitObjectGetObjectRayOriginNV:
case glslang::EOpHitObjectGetWorldRayDirectionNV:
case glslang::EOpHitObjectGetWorldRayOriginNV:
case glslang::EOpHitObjectGetWorldToObjectNV:
case glslang::EOpHitObjectGetObjectToWorldNV:
case glslang::EOpHitObjectGetRayTMaxNV:
case glslang::EOpHitObjectGetRayTMinNV:
case glslang::EOpHitObjectIsEmptyNV:
case glslang::EOpHitObjectIsHitNV:
case glslang::EOpHitObjectIsMissNV:
case glslang::EOpHitObjectRecordEmptyNV:
case glslang::EOpHitObjectGetShaderBindingTableRecordIndexNV:
case glslang::EOpHitObjectGetShaderRecordBufferHandleNV:
return true;
default:
return false;
}
};
if (node->getOp() == glslang::EOpAtomicCounterIncrement ||
node->getOp() == glslang::EOpAtomicCounterDecrement ||
node->getOp() == glslang::EOpAtomicCounter ||
(node->getOp() == glslang::EOpInterpolateAtCentroid &&
glslangIntermediate->getSource() != glslang::EShSourceHlsl) ||
node->getOp() == glslang::EOpRayQueryProceed ||
node->getOp() == glslang::EOpRayQueryGetRayTMin ||
node->getOp() == glslang::EOpRayQueryGetRayFlags ||
node->getOp() == glslang::EOpRayQueryGetWorldRayOrigin ||
node->getOp() == glslang::EOpRayQueryGetWorldRayDirection ||
node->getOp() == glslang::EOpRayQueryGetIntersectionCandidateAABBOpaque ||
node->getOp() == glslang::EOpRayQueryTerminate ||
node->getOp() == glslang::EOpRayQueryConfirmIntersection ||
(node->getOp() == glslang::EOpSpirvInst && operandNode->getAsTyped()->getQualifier().isSpirvByReference()) ||
hitObjectOpsWithLvalue(node->getOp())) {
operand = builder.accessChainGetLValue(); // Special case l-value operands
lvalueCoherentFlags = builder.getAccessChain().coherentFlags;
lvalueCoherentFlags |= TranslateCoherent(operandNode->getAsTyped()->getType());
} else if (operandNode->getAsTyped()->getQualifier().isSpirvLiteral()) {
// Will be translated to a literal value, make a placeholder here
operand = spv::NoResult;
} else {
operand = accessChainLoad(node->getOperand()->getType());
}
OpDecorations decorations = { TranslatePrecisionDecoration(node->getOperationPrecision()),
TranslateNoContractionDecoration(node->getType().getQualifier()),
TranslateNonUniformDecoration(node->getType().getQualifier()) };
// it could be a conversion
if (! result)
result = createConversion(node->getOp(), decorations, resultType(), operand,
node->getOperand()->getBasicType());
// if not, then possibly an operation
if (! result)
result = createUnaryOperation(node->getOp(), decorations, resultType(), operand,
node->getOperand()->getBasicType(), lvalueCoherentFlags, node->getType());
// it could be attached to a SPIR-V intruction
if (!result) {
if (node->getOp() == glslang::EOpSpirvInst) {
const auto& spirvInst = node->getSpirvInstruction();
if (spirvInst.set == "") {
spv::IdImmediate idImmOp = {true, operand};
if (operandNode->getAsTyped()->getQualifier().isSpirvLiteral()) {
// Translate the constant to a literal value
std::vector<unsigned> literals;
glslang::TVector<const glslang::TIntermConstantUnion*> constants;
constants.push_back(operandNode->getAsConstantUnion());
TranslateLiterals(constants, literals);
idImmOp = {false, literals[0]};
}
if (node->getBasicType() == glslang::EbtVoid)
builder.createNoResultOp(static_cast<spv::Op>(spirvInst.id), {idImmOp});
else
result = builder.createOp(static_cast<spv::Op>(spirvInst.id), resultType(), {idImmOp});
} else {
result = builder.createBuiltinCall(
resultType(), spirvInst.set == "GLSL.std.450" ? stdBuiltins : getExtBuiltins(spirvInst.set.c_str()),
spirvInst.id, {operand});
}
if (node->getBasicType() == glslang::EbtVoid)
return false; // done with this node
}
}
if (result) {
if (invertedType) {
result = createInvertedSwizzle(decorations.precision, *node->getOperand(), result);
decorations.addNonUniform(builder, result);
}
builder.clearAccessChain();
builder.setAccessChainRValue(result);
return false; // done with this node
}
// it must be a special case, check...
switch (node->getOp()) {
case glslang::EOpPostIncrement:
case glslang::EOpPostDecrement:
case glslang::EOpPreIncrement:
case glslang::EOpPreDecrement:
{
// we need the integer value "1" or the floating point "1.0" to add/subtract
spv::Id one = 0;
if (node->getBasicType() == glslang::EbtFloat)
one = builder.makeFloatConstant(1.0F);
else if (node->getBasicType() == glslang::EbtDouble)
one = builder.makeDoubleConstant(1.0);
else if (node->getBasicType() == glslang::EbtFloat16)
one = builder.makeFloat16Constant(1.0F);
else if (node->getBasicType() == glslang::EbtInt8 || node->getBasicType() == glslang::EbtUint8)
one = builder.makeInt8Constant(1);
else if (node->getBasicType() == glslang::EbtInt16 || node->getBasicType() == glslang::EbtUint16)
one = builder.makeInt16Constant(1);
else if (node->getBasicType() == glslang::EbtInt64 || node->getBasicType() == glslang::EbtUint64)
one = builder.makeInt64Constant(1);
else
one = builder.makeIntConstant(1);
glslang::TOperator op;
if (node->getOp() == glslang::EOpPreIncrement ||
node->getOp() == glslang::EOpPostIncrement)
op = glslang::EOpAdd;
else
op = glslang::EOpSub;
spv::Id result = createBinaryOperation(op, decorations,
convertGlslangToSpvType(node->getType()), operand, one,
node->getType().getBasicType());
assert(result != spv::NoResult);
// The result of operation is always stored, but conditionally the
// consumed result. The consumed result is always an r-value.
builder.accessChainStore(result,
TranslateNonUniformDecoration(builder.getAccessChain().coherentFlags));
builder.clearAccessChain();
if (node->getOp() == glslang::EOpPreIncrement ||
node->getOp() == glslang::EOpPreDecrement)
builder.setAccessChainRValue(result);
else
builder.setAccessChainRValue(operand);
}
return false;
case glslang::EOpAssumeEXT:
builder.addCapability(spv::CapabilityExpectAssumeKHR);
builder.addExtension(spv::E_SPV_KHR_expect_assume);
builder.createNoResultOp(spv::OpAssumeTrueKHR, operand);
return false;
case glslang::EOpEmitStreamVertex:
builder.createNoResultOp(spv::OpEmitStreamVertex, operand);
return false;
case glslang::EOpEndStreamPrimitive:
builder.createNoResultOp(spv::OpEndStreamPrimitive, operand);
return false;
case glslang::EOpRayQueryTerminate:
builder.createNoResultOp(spv::OpRayQueryTerminateKHR, operand);
return false;
case glslang::EOpRayQueryConfirmIntersection:
builder.createNoResultOp(spv::OpRayQueryConfirmIntersectionKHR, operand);
return false;
case glslang::EOpReorderThreadNV:
builder.createNoResultOp(spv::OpReorderThreadWithHitObjectNV, operand);
return false;
case glslang::EOpHitObjectRecordEmptyNV:
builder.createNoResultOp(spv::OpHitObjectRecordEmptyNV, operand);
return false;
default:
logger->missingFunctionality("unknown glslang unary");
return true; // pick up operand as placeholder result
}
}
// Construct a composite object, recursively copying members if their types don't match
spv::Id TGlslangToSpvTraverser::createCompositeConstruct(spv::Id resultTypeId, std::vector<spv::Id> constituents)
{
for (int c = 0; c < (int)constituents.size(); ++c) {
spv::Id& constituent = constituents[c];
spv::Id lType = builder.getContainedTypeId(resultTypeId, c);
spv::Id rType = builder.getTypeId(constituent);
if (lType != rType) {
if (glslangIntermediate->getSpv().spv >= glslang::EShTargetSpv_1_4) {
constituent = builder.createUnaryOp(spv::OpCopyLogical, lType, constituent);
} else if (builder.isStructType(rType)) {
std::vector<spv::Id> rTypeConstituents;
int numrTypeConstituents = builder.getNumTypeConstituents(rType);
for (int i = 0; i < numrTypeConstituents; ++i) {
rTypeConstituents.push_back(builder.createCompositeExtract(constituent,
builder.getContainedTypeId(rType, i), i));
}
constituents[c] = createCompositeConstruct(lType, rTypeConstituents);
} else {
assert(builder.isArrayType(rType));
std::vector<spv::Id> rTypeConstituents;
int numrTypeConstituents = builder.getNumTypeConstituents(rType);
spv::Id elementRType = builder.getContainedTypeId(rType);
for (int i = 0; i < numrTypeConstituents; ++i) {
rTypeConstituents.push_back(builder.createCompositeExtract(constituent, elementRType, i));
}
constituents[c] = createCompositeConstruct(lType, rTypeConstituents);
}
}
}
return builder.createCompositeConstruct(resultTypeId, constituents);
}
bool TGlslangToSpvTraverser::visitAggregate(glslang::TVisit visit, glslang::TIntermAggregate* node)
{
SpecConstantOpModeGuard spec_constant_op_mode_setter(&builder);
if (node->getType().getQualifier().isSpecConstant())
spec_constant_op_mode_setter.turnOnSpecConstantOpMode();
spv::Id result = spv::NoResult;
spv::Id invertedType = spv::NoType; // to use to override the natural type of the node
std::vector<spv::Builder::AccessChain> complexLvalues; // for holding swizzling l-values too complex for
// SPIR-V, for an out parameter
std::vector<spv::Id> temporaryLvalues; // temporaries to pass, as proxies for complexLValues
auto resultType = [&invertedType, &node, this](){ return invertedType != spv::NoType ?
invertedType :
convertGlslangToSpvType(node->getType()); };
// try texturing
result = createImageTextureFunctionCall(node);
if (result != spv::NoResult) {
builder.clearAccessChain();
builder.setAccessChainRValue(result);
return false;
} else if (node->getOp() == glslang::EOpImageStore ||
node->getOp() == glslang::EOpImageStoreLod ||
node->getOp() == glslang::EOpImageAtomicStore) {
// "imageStore" is a special case, which has no result
return false;
}
glslang::TOperator binOp = glslang::EOpNull;
bool reduceComparison = true;
bool isMatrix = false;
bool noReturnValue = false;
bool atomic = false;
spv::Builder::AccessChain::CoherentFlags lvalueCoherentFlags;
assert(node->getOp());
spv::Decoration precision = TranslatePrecisionDecoration(node->getOperationPrecision());
switch (node->getOp()) {
case glslang::EOpScope:
case glslang::EOpSequence:
{
if (visit == glslang::EvPreVisit) {
++sequenceDepth;
if (sequenceDepth == 1) {
// If this is the parent node of all the functions, we want to see them
// early, so all call points have actual SPIR-V functions to reference.
// In all cases, still let the traverser visit the children for us.
makeFunctions(node->getAsAggregate()->getSequence());
// Global initializers is specific to the shader entry point, which does not exist in compile-only mode
if (!options.compileOnly) {
// Also, we want all globals initializers to go into the beginning of the entry point, before
// anything else gets there, so visit out of order, doing them all now.
makeGlobalInitializers(node->getAsAggregate()->getSequence());
}
//Pre process linker objects for ray tracing stages
if (glslangIntermediate->isRayTracingStage())
collectRayTracingLinkerObjects();
// Initializers are done, don't want to visit again, but functions and link objects need to be processed,
// so do them manually.
visitFunctions(node->getAsAggregate()->getSequence());
return false;
} else {
if (node->getOp() == glslang::EOpScope)
builder.enterLexicalBlock(0);
}
} else {
if (sequenceDepth > 1 && node->getOp() == glslang::EOpScope)
builder.leaveLexicalBlock();
--sequenceDepth;
}
return true;
}
case glslang::EOpLinkerObjects:
{
if (visit == glslang::EvPreVisit)
linkageOnly = true;
else
linkageOnly = false;
return true;
}
case glslang::EOpComma:
{
// processing from left to right naturally leaves the right-most
// lying around in the access chain
glslang::TIntermSequence& glslangOperands = node->getSequence();
for (int i = 0; i < (int)glslangOperands.size(); ++i)
glslangOperands[i]->traverse(this);
return false;
}
case glslang::EOpFunction:
if (visit == glslang::EvPreVisit) {
if (options.generateDebugInfo) {
builder.setDebugSourceLocation(node->getLoc().line, node->getLoc().getFilename());
}
if (isShaderEntryPoint(node)) {
inEntryPoint = true;
builder.setBuildPoint(shaderEntry->getLastBlock());
builder.enterFunction(shaderEntry);
currentFunction = shaderEntry;
} else {
handleFunctionEntry(node);
}
if (options.generateDebugInfo && !options.emitNonSemanticShaderDebugInfo) {
const auto& loc = node->getLoc();
const char* sourceFileName = loc.getFilename();
spv::Id sourceFileId = sourceFileName ? builder.getStringId(sourceFileName) : builder.getMainFileId();
currentFunction->setDebugLineInfo(sourceFileId, loc.line, loc.column);
}
} else {
if (inEntryPoint)
entryPointTerminated = true;
builder.leaveFunction();
inEntryPoint = false;
}
return true;
case glslang::EOpParameters:
// Parameters will have been consumed by EOpFunction processing, but not
// the body, so we still visited the function node's children, making this
// child redundant.
return false;
case glslang::EOpFunctionCall:
{
builder.setDebugSourceLocation(node->getLoc().line, node->getLoc().getFilename());
if (node->isUserDefined())
result = handleUserFunctionCall(node);
if (result) {
builder.clearAccessChain();
builder.setAccessChainRValue(result);
} else
logger->missingFunctionality("missing user function; linker needs to catch that");
return false;
}
case glslang::EOpConstructMat2x2:
case glslang::EOpConstructMat2x3:
case glslang::EOpConstructMat2x4:
case glslang::EOpConstructMat3x2:
case glslang::EOpConstructMat3x3:
case glslang::EOpConstructMat3x4:
case glslang::EOpConstructMat4x2:
case glslang::EOpConstructMat4x3:
case glslang::EOpConstructMat4x4:
case glslang::EOpConstructDMat2x2:
case glslang::EOpConstructDMat2x3:
case glslang::EOpConstructDMat2x4:
case glslang::EOpConstructDMat3x2:
case glslang::EOpConstructDMat3x3:
case glslang::EOpConstructDMat3x4:
case glslang::EOpConstructDMat4x2:
case glslang::EOpConstructDMat4x3:
case glslang::EOpConstructDMat4x4:
case glslang::EOpConstructIMat2x2:
case glslang::EOpConstructIMat2x3:
case glslang::EOpConstructIMat2x4:
case glslang::EOpConstructIMat3x2:
case glslang::EOpConstructIMat3x3:
case glslang::EOpConstructIMat3x4:
case glslang::EOpConstructIMat4x2:
case glslang::EOpConstructIMat4x3:
case glslang::EOpConstructIMat4x4:
case glslang::EOpConstructUMat2x2:
case glslang::EOpConstructUMat2x3:
case glslang::EOpConstructUMat2x4:
case glslang::EOpConstructUMat3x2:
case glslang::EOpConstructUMat3x3:
case glslang::EOpConstructUMat3x4:
case glslang::EOpConstructUMat4x2:
case glslang::EOpConstructUMat4x3:
case glslang::EOpConstructUMat4x4:
case glslang::EOpConstructBMat2x2:
case glslang::EOpConstructBMat2x3:
case glslang::EOpConstructBMat2x4:
case glslang::EOpConstructBMat3x2:
case glslang::EOpConstructBMat3x3:
case glslang::EOpConstructBMat3x4:
case glslang::EOpConstructBMat4x2:
case glslang::EOpConstructBMat4x3:
case glslang::EOpConstructBMat4x4:
case glslang::EOpConstructF16Mat2x2:
case glslang::EOpConstructF16Mat2x3:
case glslang::EOpConstructF16Mat2x4:
case glslang::EOpConstructF16Mat3x2:
case glslang::EOpConstructF16Mat3x3:
case glslang::EOpConstructF16Mat3x4:
case glslang::EOpConstructF16Mat4x2:
case glslang::EOpConstructF16Mat4x3:
case glslang::EOpConstructF16Mat4x4:
isMatrix = true;
[[fallthrough]];
case glslang::EOpConstructFloat:
case glslang::EOpConstructVec2:
case glslang::EOpConstructVec3:
case glslang::EOpConstructVec4:
case glslang::EOpConstructDouble:
case glslang::EOpConstructDVec2:
case glslang::EOpConstructDVec3:
case glslang::EOpConstructDVec4:
case glslang::EOpConstructFloat16:
case glslang::EOpConstructF16Vec2:
case glslang::EOpConstructF16Vec3:
case glslang::EOpConstructF16Vec4:
case glslang::EOpConstructBool:
case glslang::EOpConstructBVec2:
case glslang::EOpConstructBVec3:
case glslang::EOpConstructBVec4:
case glslang::EOpConstructInt8:
case glslang::EOpConstructI8Vec2:
case glslang::EOpConstructI8Vec3:
case glslang::EOpConstructI8Vec4:
case glslang::EOpConstructUint8:
case glslang::EOpConstructU8Vec2:
case glslang::EOpConstructU8Vec3:
case glslang::EOpConstructU8Vec4:
case glslang::EOpConstructInt16:
case glslang::EOpConstructI16Vec2:
case glslang::EOpConstructI16Vec3:
case glslang::EOpConstructI16Vec4:
case glslang::EOpConstructUint16:
case glslang::EOpConstructU16Vec2:
case glslang::EOpConstructU16Vec3:
case glslang::EOpConstructU16Vec4:
case glslang::EOpConstructInt:
case glslang::EOpConstructIVec2:
case glslang::EOpConstructIVec3:
case glslang::EOpConstructIVec4:
case glslang::EOpConstructUint:
case glslang::EOpConstructUVec2:
case glslang::EOpConstructUVec3:
case glslang::EOpConstructUVec4:
case glslang::EOpConstructInt64:
case glslang::EOpConstructI64Vec2:
case glslang::EOpConstructI64Vec3:
case glslang::EOpConstructI64Vec4:
case glslang::EOpConstructUint64:
case glslang::EOpConstructU64Vec2:
case glslang::EOpConstructU64Vec3:
case glslang::EOpConstructU64Vec4:
case glslang::EOpConstructStruct:
case glslang::EOpConstructTextureSampler:
case glslang::EOpConstructReference:
case glslang::EOpConstructCooperativeMatrixNV:
case glslang::EOpConstructCooperativeMatrixKHR:
{
builder.setDebugSourceLocation(node->getLoc().line, node->getLoc().getFilename());
std::vector<spv::Id> arguments;
translateArguments(*node, arguments, lvalueCoherentFlags);
spv::Id constructed;
if (node->getOp() == glslang::EOpConstructTextureSampler) {
const glslang::TType& texType = node->getSequence()[0]->getAsTyped()->getType();
if (glslangIntermediate->getSpv().spv >= glslang::EShTargetSpv_1_6 &&
texType.getSampler().isBuffer()) {
// SamplerBuffer is not supported in spirv1.6 so
// `samplerBuffer(textureBuffer, sampler)` is a no-op
// and textureBuffer is the result going forward
constructed = arguments[0];
} else
constructed = builder.createOp(spv::OpSampledImage, resultType(), arguments);
} else if (node->getOp() == glslang::EOpConstructStruct ||
node->getOp() == glslang::EOpConstructCooperativeMatrixNV ||
node->getOp() == glslang::EOpConstructCooperativeMatrixKHR ||
node->getType().isArray()) {
std::vector<spv::Id> constituents;
for (int c = 0; c < (int)arguments.size(); ++c)
constituents.push_back(arguments[c]);
constructed = createCompositeConstruct(resultType(), constituents);
} else if (isMatrix)
constructed = builder.createMatrixConstructor(precision, arguments, resultType());
else
constructed = builder.createConstructor(precision, arguments, resultType());
if (node->getType().getQualifier().isNonUniform()) {
builder.addDecoration(constructed, spv::DecorationNonUniformEXT);
}
builder.clearAccessChain();
builder.setAccessChainRValue(constructed);
return false;
}
// These six are component-wise compares with component-wise results.
// Forward on to createBinaryOperation(), requesting a vector result.
case glslang::EOpLessThan:
case glslang::EOpGreaterThan:
case glslang::EOpLessThanEqual:
case glslang::EOpGreaterThanEqual:
case glslang::EOpVectorEqual:
case glslang::EOpVectorNotEqual:
{
// Map the operation to a binary
binOp = node->getOp();
reduceComparison = false;
switch (node->getOp()) {
case glslang::EOpVectorEqual: binOp = glslang::EOpVectorEqual; break;
case glslang::EOpVectorNotEqual: binOp = glslang::EOpVectorNotEqual; break;
default: binOp = node->getOp(); break;
}
break;
}
case glslang::EOpMul:
// component-wise matrix multiply
binOp = glslang::EOpMul;
break;
case glslang::EOpOuterProduct:
// two vectors multiplied to make a matrix
binOp = glslang::EOpOuterProduct;
break;
case glslang::EOpDot:
{
// for scalar dot product, use multiply
glslang::TIntermSequence& glslangOperands = node->getSequence();
if (glslangOperands[0]->getAsTyped()->getVectorSize() == 1)
binOp = glslang::EOpMul;
break;
}
case glslang::EOpMod:
// when an aggregate, this is the floating-point mod built-in function,
// which can be emitted by the one in createBinaryOperation()
binOp = glslang::EOpMod;
break;
case glslang::EOpEmitVertex:
case glslang::EOpEndPrimitive:
case glslang::EOpBarrier:
case glslang::EOpMemoryBarrier:
case glslang::EOpMemoryBarrierAtomicCounter:
case glslang::EOpMemoryBarrierBuffer:
case glslang::EOpMemoryBarrierImage:
case glslang::EOpMemoryBarrierShared:
case glslang::EOpGroupMemoryBarrier:
case glslang::EOpDeviceMemoryBarrier:
case glslang::EOpAllMemoryBarrierWithGroupSync:
case glslang::EOpDeviceMemoryBarrierWithGroupSync:
case glslang::EOpWorkgroupMemoryBarrier:
case glslang::EOpWorkgroupMemoryBarrierWithGroupSync:
case glslang::EOpSubgroupBarrier:
case glslang::EOpSubgroupMemoryBarrier:
case glslang::EOpSubgroupMemoryBarrierBuffer:
case glslang::EOpSubgroupMemoryBarrierImage:
case glslang::EOpSubgroupMemoryBarrierShared:
noReturnValue = true;
// These all have 0 operands and will naturally finish up in the code below for 0 operands
break;
case glslang::EOpAtomicAdd:
case glslang::EOpAtomicSubtract:
case glslang::EOpAtomicMin:
case glslang::EOpAtomicMax:
case glslang::EOpAtomicAnd:
case glslang::EOpAtomicOr:
case glslang::EOpAtomicXor:
case glslang::EOpAtomicExchange:
case glslang::EOpAtomicCompSwap:
atomic = true;
break;
case glslang::EOpAtomicStore:
noReturnValue = true;
[[fallthrough]];
case glslang::EOpAtomicLoad:
atomic = true;
break;
case glslang::EOpAtomicCounterAdd:
case glslang::EOpAtomicCounterSubtract:
case glslang::EOpAtomicCounterMin:
case glslang::EOpAtomicCounterMax:
case glslang::EOpAtomicCounterAnd:
case glslang::EOpAtomicCounterOr:
case glslang::EOpAtomicCounterXor:
case glslang::EOpAtomicCounterExchange:
case glslang::EOpAtomicCounterCompSwap:
builder.addExtension("SPV_KHR_shader_atomic_counter_ops");
builder.addCapability(spv::CapabilityAtomicStorageOps);
atomic = true;
break;
case glslang::EOpAbsDifference:
case glslang::EOpAddSaturate:
case glslang::EOpSubSaturate:
case glslang::EOpAverage:
case glslang::EOpAverageRounded:
case glslang::EOpMul32x16:
builder.addCapability(spv::CapabilityIntegerFunctions2INTEL);
builder.addExtension("SPV_INTEL_shader_integer_functions2");
binOp = node->getOp();
break;
case glslang::EOpExpectEXT:
builder.addCapability(spv::CapabilityExpectAssumeKHR);
builder.addExtension(spv::E_SPV_KHR_expect_assume);
binOp = node->getOp();
break;
case glslang::EOpIgnoreIntersectionNV:
case glslang::EOpTerminateRayNV:
case glslang::EOpTraceNV:
case glslang::EOpTraceRayMotionNV:
case glslang::EOpTraceKHR:
case glslang::EOpExecuteCallableNV:
case glslang::EOpExecuteCallableKHR:
case glslang::EOpWritePackedPrimitiveIndices4x8NV:
case glslang::EOpEmitMeshTasksEXT:
case glslang::EOpSetMeshOutputsEXT:
noReturnValue = true;
break;
case glslang::EOpRayQueryInitialize:
case glslang::EOpRayQueryTerminate:
case glslang::EOpRayQueryGenerateIntersection:
case glslang::EOpRayQueryConfirmIntersection:
builder.addExtension("SPV_KHR_ray_query");
builder.addCapability(spv::CapabilityRayQueryKHR);
noReturnValue = true;
break;
case glslang::EOpRayQueryProceed:
case glslang::EOpRayQueryGetIntersectionType:
case glslang::EOpRayQueryGetRayTMin:
case glslang::EOpRayQueryGetRayFlags:
case glslang::EOpRayQueryGetIntersectionT:
case glslang::EOpRayQueryGetIntersectionInstanceCustomIndex:
case glslang::EOpRayQueryGetIntersectionInstanceId:
case glslang::EOpRayQueryGetIntersectionInstanceShaderBindingTableRecordOffset:
case glslang::EOpRayQueryGetIntersectionGeometryIndex:
case glslang::EOpRayQueryGetIntersectionPrimitiveIndex:
case glslang::EOpRayQueryGetIntersectionBarycentrics:
case glslang::EOpRayQueryGetIntersectionFrontFace:
case glslang::EOpRayQueryGetIntersectionCandidateAABBOpaque:
case glslang::EOpRayQueryGetIntersectionObjectRayDirection:
case glslang::EOpRayQueryGetIntersectionObjectRayOrigin:
case glslang::EOpRayQueryGetWorldRayDirection:
case glslang::EOpRayQueryGetWorldRayOrigin:
case glslang::EOpRayQueryGetIntersectionObjectToWorld:
case glslang::EOpRayQueryGetIntersectionWorldToObject:
builder.addExtension("SPV_KHR_ray_query");
builder.addCapability(spv::CapabilityRayQueryKHR);
break;
case glslang::EOpCooperativeMatrixLoad:
case glslang::EOpCooperativeMatrixStore:
case glslang::EOpCooperativeMatrixLoadNV:
case glslang::EOpCooperativeMatrixStoreNV:
noReturnValue = true;
break;
case glslang::EOpBeginInvocationInterlock:
case glslang::EOpEndInvocationInterlock:
builder.addExtension(spv::E_SPV_EXT_fragment_shader_interlock);
noReturnValue = true;
break;
case glslang::EOpHitObjectTraceRayNV:
case glslang::EOpHitObjectTraceRayMotionNV:
case glslang::EOpHitObjectGetAttributesNV:
case glslang::EOpHitObjectExecuteShaderNV:
case glslang::EOpHitObjectRecordEmptyNV:
case glslang::EOpHitObjectRecordMissNV:
case glslang::EOpHitObjectRecordMissMotionNV:
case glslang::EOpHitObjectRecordHitNV:
case glslang::EOpHitObjectRecordHitMotionNV:
case glslang::EOpHitObjectRecordHitWithIndexNV:
case glslang::EOpHitObjectRecordHitWithIndexMotionNV:
case glslang::EOpReorderThreadNV:
noReturnValue = true;
[[fallthrough]];
case glslang::EOpHitObjectIsEmptyNV:
case glslang::EOpHitObjectIsMissNV:
case glslang::EOpHitObjectIsHitNV:
case glslang::EOpHitObjectGetRayTMinNV:
case glslang::EOpHitObjectGetRayTMaxNV:
case glslang::EOpHitObjectGetObjectRayOriginNV:
case glslang::EOpHitObjectGetObjectRayDirectionNV:
case glslang::EOpHitObjectGetWorldRayOriginNV:
case glslang::EOpHitObjectGetWorldRayDirectionNV:
case glslang::EOpHitObjectGetObjectToWorldNV:
case glslang::EOpHitObjectGetWorldToObjectNV:
case glslang::EOpHitObjectGetInstanceCustomIndexNV:
case glslang::EOpHitObjectGetInstanceIdNV:
case glslang::EOpHitObjectGetGeometryIndexNV:
case glslang::EOpHitObjectGetPrimitiveIndexNV:
case glslang::EOpHitObjectGetHitKindNV:
case glslang::EOpHitObjectGetCurrentTimeNV:
case glslang::EOpHitObjectGetShaderBindingTableRecordIndexNV:
case glslang::EOpHitObjectGetShaderRecordBufferHandleNV:
builder.addExtension(spv::E_SPV_NV_shader_invocation_reorder);
builder.addCapability(spv::CapabilityShaderInvocationReorderNV);
break;
case glslang::EOpRayQueryGetIntersectionTriangleVertexPositionsEXT:
builder.addExtension(spv::E_SPV_KHR_ray_tracing_position_fetch);
builder.addCapability(spv::CapabilityRayQueryPositionFetchKHR);
noReturnValue = true;
break;
case glslang::EOpImageSampleWeightedQCOM:
builder.addCapability(spv::CapabilityTextureSampleWeightedQCOM);
builder.addExtension(spv::E_SPV_QCOM_image_processing);
break;
case glslang::EOpImageBoxFilterQCOM:
builder.addCapability(spv::CapabilityTextureBoxFilterQCOM);
builder.addExtension(spv::E_SPV_QCOM_image_processing);
break;
case glslang::EOpImageBlockMatchSADQCOM:
case glslang::EOpImageBlockMatchSSDQCOM:
builder.addCapability(spv::CapabilityTextureBlockMatchQCOM);
builder.addExtension(spv::E_SPV_QCOM_image_processing);
break;
case glslang::EOpImageBlockMatchWindowSSDQCOM:
case glslang::EOpImageBlockMatchWindowSADQCOM:
builder.addCapability(spv::CapabilityTextureBlockMatchQCOM);
builder.addExtension(spv::E_SPV_QCOM_image_processing);
builder.addCapability(spv::CapabilityTextureBlockMatch2QCOM);
builder.addExtension(spv::E_SPV_QCOM_image_processing2);
break;
case glslang::EOpImageBlockMatchGatherSSDQCOM:
case glslang::EOpImageBlockMatchGatherSADQCOM:
builder.addCapability(spv::CapabilityTextureBlockMatchQCOM);
builder.addExtension(spv::E_SPV_QCOM_image_processing);
builder.addCapability(spv::CapabilityTextureBlockMatch2QCOM);
builder.addExtension(spv::E_SPV_QCOM_image_processing2);
break;
case glslang::EOpFetchMicroTriangleVertexPositionNV:
case glslang::EOpFetchMicroTriangleVertexBarycentricNV:
builder.addExtension(spv::E_SPV_NV_displacement_micromap);
builder.addCapability(spv::CapabilityDisplacementMicromapNV);
break;
case glslang::EOpDebugPrintf:
noReturnValue = true;
break;
default:
break;
}
//
// See if it maps to a regular operation.
//
if (binOp != glslang::EOpNull) {
glslang::TIntermTyped* left = node->getSequence()[0]->getAsTyped();
glslang::TIntermTyped* right = node->getSequence()[1]->getAsTyped();
assert(left && right);
builder.clearAccessChain();
left->traverse(this);
spv::Id leftId = accessChainLoad(left->getType());
builder.clearAccessChain();
right->traverse(this);
spv::Id rightId = accessChainLoad(right->getType());
builder.setDebugSourceLocation(node->getLoc().line, node->getLoc().getFilename());
OpDecorations decorations = { precision,
TranslateNoContractionDecoration(node->getType().getQualifier()),
TranslateNonUniformDecoration(node->getType().getQualifier()) };
result = createBinaryOperation(binOp, decorations,
resultType(), leftId, rightId,
left->getType().getBasicType(), reduceComparison);
// code above should only make binOp that exists in createBinaryOperation
assert(result != spv::NoResult);
builder.clearAccessChain();
builder.setAccessChainRValue(result);
return false;
}
//
// Create the list of operands.
//
glslang::TIntermSequence& glslangOperands = node->getSequence();
std::vector<spv::Id> operands;
std::vector<spv::IdImmediate> memoryAccessOperands;
for (int arg = 0; arg < (int)glslangOperands.size(); ++arg) {
// special case l-value operands; there are just a few
bool lvalue = false;
switch (node->getOp()) {
case glslang::EOpModf:
if (arg == 1)
lvalue = true;
break;
case glslang::EOpHitObjectRecordHitNV:
case glslang::EOpHitObjectRecordHitMotionNV:
case glslang::EOpHitObjectRecordHitWithIndexNV:
case glslang::EOpHitObjectRecordHitWithIndexMotionNV:
case glslang::EOpHitObjectTraceRayNV:
case glslang::EOpHitObjectTraceRayMotionNV:
case glslang::EOpHitObjectExecuteShaderNV:
case glslang::EOpHitObjectRecordMissNV:
case glslang::EOpHitObjectRecordMissMotionNV:
case glslang::EOpHitObjectGetAttributesNV:
if (arg == 0)
lvalue = true;
break;
case glslang::EOpRayQueryInitialize:
case glslang::EOpRayQueryTerminate:
case glslang::EOpRayQueryConfirmIntersection:
case glslang::EOpRayQueryProceed:
case glslang::EOpRayQueryGenerateIntersection:
case glslang::EOpRayQueryGetIntersectionType:
case glslang::EOpRayQueryGetIntersectionT:
case glslang::EOpRayQueryGetIntersectionInstanceCustomIndex:
case glslang::EOpRayQueryGetIntersectionInstanceId:
case glslang::EOpRayQueryGetIntersectionInstanceShaderBindingTableRecordOffset:
case glslang::EOpRayQueryGetIntersectionGeometryIndex:
case glslang::EOpRayQueryGetIntersectionPrimitiveIndex:
case glslang::EOpRayQueryGetIntersectionBarycentrics:
case glslang::EOpRayQueryGetIntersectionFrontFace:
case glslang::EOpRayQueryGetIntersectionObjectRayDirection:
case glslang::EOpRayQueryGetIntersectionObjectRayOrigin:
case glslang::EOpRayQueryGetIntersectionObjectToWorld:
case glslang::EOpRayQueryGetIntersectionWorldToObject:
if (arg == 0)
lvalue = true;
break;
case glslang::EOpAtomicAdd:
case glslang::EOpAtomicSubtract:
case glslang::EOpAtomicMin:
case glslang::EOpAtomicMax:
case glslang::EOpAtomicAnd:
case glslang::EOpAtomicOr:
case glslang::EOpAtomicXor:
case glslang::EOpAtomicExchange:
case glslang::EOpAtomicCompSwap:
if (arg == 0)
lvalue = true;
break;
case glslang::EOpFrexp:
if (arg == 1)
lvalue = true;
break;
case glslang::EOpInterpolateAtSample:
case glslang::EOpInterpolateAtOffset:
case glslang::EOpInterpolateAtVertex:
if (arg == 0) {
// If GLSL, use the address of the interpolant argument.
// If HLSL, use an internal version of OpInterolates that takes
// the rvalue of the interpolant. A fixup pass in spirv-opt
// legalization will remove the OpLoad and convert to an lvalue.
// Had to do this because legalization will only propagate a
// builtin into an rvalue.
lvalue = glslangIntermediate->getSource() != glslang::EShSourceHlsl;
// Does it need a swizzle inversion? If so, evaluation is inverted;
// operate first on the swizzle base, then apply the swizzle.
// That is, we transform
//
// interpolate(v.zy) -> interpolate(v).zy
//
if (glslangOperands[0]->getAsOperator() &&
glslangOperands[0]->getAsOperator()->getOp() == glslang::EOpVectorSwizzle)
invertedType = convertGlslangToSpvType(
glslangOperands[0]->getAsBinaryNode()->getLeft()->getType());
}
break;
case glslang::EOpAtomicLoad:
case glslang::EOpAtomicStore:
case glslang::EOpAtomicCounterAdd:
case glslang::EOpAtomicCounterSubtract:
case glslang::EOpAtomicCounterMin:
case glslang::EOpAtomicCounterMax:
case glslang::EOpAtomicCounterAnd:
case glslang::EOpAtomicCounterOr:
case glslang::EOpAtomicCounterXor:
case glslang::EOpAtomicCounterExchange:
case glslang::EOpAtomicCounterCompSwap:
if (arg == 0)
lvalue = true;
break;
case glslang::EOpAddCarry:
case glslang::EOpSubBorrow:
if (arg == 2)
lvalue = true;
break;
case glslang::EOpUMulExtended:
case glslang::EOpIMulExtended:
if (arg >= 2)
lvalue = true;
break;
case glslang::EOpCooperativeMatrixLoad:
case glslang::EOpCooperativeMatrixLoadNV:
if (arg == 0 || arg == 1)
lvalue = true;
break;
case glslang::EOpCooperativeMatrixStore:
case glslang::EOpCooperativeMatrixStoreNV:
if (arg == 1)
lvalue = true;
break;
case glslang::EOpSpirvInst:
if (glslangOperands[arg]->getAsTyped()->getQualifier().isSpirvByReference())
lvalue = true;
break;
case glslang::EOpReorderThreadNV:
//Three variants of reorderThreadNV, two of them use hitObjectNV
if (arg == 0 && glslangOperands.size() != 2)
lvalue = true;
break;
case glslang::EOpRayQueryGetIntersectionTriangleVertexPositionsEXT:
if (arg == 0 || arg == 2)
lvalue = true;
break;
default:
break;
}
builder.clearAccessChain();
if (invertedType != spv::NoType && arg == 0)
glslangOperands[0]->getAsBinaryNode()->getLeft()->traverse(this);
else
glslangOperands[arg]->traverse(this);
if (node->getOp() == glslang::EOpCooperativeMatrixLoad ||
node->getOp() == glslang::EOpCooperativeMatrixStore ||
node->getOp() == glslang::EOpCooperativeMatrixLoadNV ||
node->getOp() == glslang::EOpCooperativeMatrixStoreNV) {
if (arg == 1) {
// fold "element" parameter into the access chain
spv::Builder::AccessChain save = builder.getAccessChain();
builder.clearAccessChain();
glslangOperands[2]->traverse(this);
spv::Id elementId = accessChainLoad(glslangOperands[2]->getAsTyped()->getType());
builder.setAccessChain(save);
// Point to the first element of the array.
builder.accessChainPush(elementId,
TranslateCoherent(glslangOperands[arg]->getAsTyped()->getType()),
glslangOperands[arg]->getAsTyped()->getType().getBufferReferenceAlignment());
spv::Builder::AccessChain::CoherentFlags coherentFlags = builder.getAccessChain().coherentFlags;
unsigned int alignment = builder.getAccessChain().alignment;
int memoryAccess = TranslateMemoryAccess(coherentFlags);
if (node->getOp() == glslang::EOpCooperativeMatrixLoad ||
node->getOp() == glslang::EOpCooperativeMatrixLoadNV)
memoryAccess &= ~spv::MemoryAccessMakePointerAvailableKHRMask;
if (node->getOp() == glslang::EOpCooperativeMatrixStore ||
node->getOp() == glslang::EOpCooperativeMatrixStoreNV)
memoryAccess &= ~spv::MemoryAccessMakePointerVisibleKHRMask;
if (builder.getStorageClass(builder.getAccessChain().base) ==
spv::StorageClassPhysicalStorageBufferEXT) {
memoryAccess = (spv::MemoryAccessMask)(memoryAccess | spv::MemoryAccessAlignedMask);
}
memoryAccessOperands.push_back(spv::IdImmediate(false, memoryAccess));
if (memoryAccess & spv::MemoryAccessAlignedMask) {
memoryAccessOperands.push_back(spv::IdImmediate(false, alignment));
}
if (memoryAccess &
(spv::MemoryAccessMakePointerAvailableKHRMask | spv::MemoryAccessMakePointerVisibleKHRMask)) {
memoryAccessOperands.push_back(spv::IdImmediate(true,
builder.makeUintConstant(TranslateMemoryScope(coherentFlags))));
}
} else if (arg == 2) {
continue;
}
}
// for l-values, pass the address, for r-values, pass the value
if (lvalue) {
if (invertedType == spv::NoType && !builder.isSpvLvalue()) {
// SPIR-V cannot represent an l-value containing a swizzle that doesn't
// reduce to a simple access chain. So, we need a temporary vector to
// receive the result, and must later swizzle that into the original
// l-value.
complexLvalues.push_back(builder.getAccessChain());
temporaryLvalues.push_back(builder.createVariable(
spv::NoPrecision, spv::StorageClassFunction,
builder.accessChainGetInferredType(), "swizzleTemp"));
operands.push_back(temporaryLvalues.back());
} else {
operands.push_back(builder.accessChainGetLValue());
}
lvalueCoherentFlags = builder.getAccessChain().coherentFlags;
lvalueCoherentFlags |= TranslateCoherent(glslangOperands[arg]->getAsTyped()->getType());
} else {
builder.setDebugSourceLocation(node->getLoc().line, node->getLoc().getFilename());
glslang::TOperator glslangOp = node->getOp();
if (arg == 1 &&
(glslangOp == glslang::EOpRayQueryGetIntersectionType ||
glslangOp == glslang::EOpRayQueryGetIntersectionT ||
glslangOp == glslang::EOpRayQueryGetIntersectionInstanceCustomIndex ||
glslangOp == glslang::EOpRayQueryGetIntersectionInstanceId ||
glslangOp == glslang::EOpRayQueryGetIntersectionInstanceShaderBindingTableRecordOffset ||
glslangOp == glslang::EOpRayQueryGetIntersectionGeometryIndex ||
glslangOp == glslang::EOpRayQueryGetIntersectionPrimitiveIndex ||
glslangOp == glslang::EOpRayQueryGetIntersectionBarycentrics ||
glslangOp == glslang::EOpRayQueryGetIntersectionFrontFace ||
glslangOp == glslang::EOpRayQueryGetIntersectionObjectRayDirection ||
glslangOp == glslang::EOpRayQueryGetIntersectionObjectRayOrigin ||
glslangOp == glslang::EOpRayQueryGetIntersectionObjectToWorld ||
glslangOp == glslang::EOpRayQueryGetIntersectionWorldToObject ||
glslangOp == glslang::EOpRayQueryGetIntersectionTriangleVertexPositionsEXT
)) {
bool cond = glslangOperands[arg]->getAsConstantUnion()->getConstArray()[0].getBConst();
operands.push_back(builder.makeIntConstant(cond ? 1 : 0));
} else if ((arg == 10 && glslangOp == glslang::EOpTraceKHR) ||
(arg == 11 && glslangOp == glslang::EOpTraceRayMotionNV) ||
(arg == 1 && glslangOp == glslang::EOpExecuteCallableKHR) ||
(arg == 1 && glslangOp == glslang::EOpHitObjectExecuteShaderNV) ||
(arg == 11 && glslangOp == glslang::EOpHitObjectTraceRayNV) ||
(arg == 12 && glslangOp == glslang::EOpHitObjectTraceRayMotionNV)) {
const int set = glslangOp == glslang::EOpExecuteCallableKHR ? 1 : 0;
const int location = glslangOperands[arg]->getAsConstantUnion()->getConstArray()[0].getUConst();
auto itNode = locationToSymbol[set].find(location);
visitSymbol(itNode->second);
spv::Id symId = getSymbolId(itNode->second);
operands.push_back(symId);
} else if ((arg == 12 && glslangOp == glslang::EOpHitObjectRecordHitNV) ||
(arg == 13 && glslangOp == glslang::EOpHitObjectRecordHitMotionNV) ||
(arg == 11 && glslangOp == glslang::EOpHitObjectRecordHitWithIndexNV) ||
(arg == 12 && glslangOp == glslang::EOpHitObjectRecordHitWithIndexMotionNV) ||
(arg == 1 && glslangOp == glslang::EOpHitObjectGetAttributesNV)) {
const int location = glslangOperands[arg]->getAsConstantUnion()->getConstArray()[0].getUConst();
const int set = 2;
auto itNode = locationToSymbol[set].find(location);
visitSymbol(itNode->second);
spv::Id symId = getSymbolId(itNode->second);
operands.push_back(symId);
} else if (glslangOperands[arg]->getAsTyped()->getQualifier().isSpirvLiteral()) {
// Will be translated to a literal value, make a placeholder here
operands.push_back(spv::NoResult);
} else {
operands.push_back(accessChainLoad(glslangOperands[arg]->getAsTyped()->getType()));
}
}
}
builder.setDebugSourceLocation(node->getLoc().line, node->getLoc().getFilename());
if (node->getOp() == glslang::EOpCooperativeMatrixLoad ||
node->getOp() == glslang::EOpCooperativeMatrixLoadNV) {
std::vector<spv::IdImmediate> idImmOps;
idImmOps.push_back(spv::IdImmediate(true, operands[1])); // buf
if (node->getOp() == glslang::EOpCooperativeMatrixLoad) {
idImmOps.push_back(spv::IdImmediate(true, operands[3])); // matrixLayout
auto layout = builder.getConstantScalar(operands[3]);
if (layout == spv::CooperativeMatrixLayoutRowBlockedInterleavedARM ||
layout == spv::CooperativeMatrixLayoutColumnBlockedInterleavedARM) {
builder.addExtension(spv::E_SPV_ARM_cooperative_matrix_layouts);
builder.addCapability(spv::CapabilityCooperativeMatrixLayoutsARM);
}
idImmOps.push_back(spv::IdImmediate(true, operands[2])); // stride
} else {
idImmOps.push_back(spv::IdImmediate(true, operands[2])); // stride
idImmOps.push_back(spv::IdImmediate(true, operands[3])); // colMajor
}
idImmOps.insert(idImmOps.end(), memoryAccessOperands.begin(), memoryAccessOperands.end());
// get the pointee type
spv::Id typeId = builder.getContainedTypeId(builder.getTypeId(operands[0]));
assert(builder.isCooperativeMatrixType(typeId));
// do the op
spv::Id result = node->getOp() == glslang::EOpCooperativeMatrixLoad
? builder.createOp(spv::OpCooperativeMatrixLoadKHR, typeId, idImmOps)
: builder.createOp(spv::OpCooperativeMatrixLoadNV, typeId, idImmOps);
// store the result to the pointer (out param 'm')
builder.createStore(result, operands[0]);
result = 0;
} else if (node->getOp() == glslang::EOpCooperativeMatrixStore ||
node->getOp() == glslang::EOpCooperativeMatrixStoreNV) {
std::vector<spv::IdImmediate> idImmOps;
idImmOps.push_back(spv::IdImmediate(true, operands[1])); // buf
idImmOps.push_back(spv::IdImmediate(true, operands[0])); // object
if (node->getOp() == glslang::EOpCooperativeMatrixStore) {
idImmOps.push_back(spv::IdImmediate(true, operands[3])); // matrixLayout
auto layout = builder.getConstantScalar(operands[3]);
if (layout == spv::CooperativeMatrixLayoutRowBlockedInterleavedARM ||
layout == spv::CooperativeMatrixLayoutColumnBlockedInterleavedARM) {
builder.addExtension(spv::E_SPV_ARM_cooperative_matrix_layouts);
builder.addCapability(spv::CapabilityCooperativeMatrixLayoutsARM);
}
idImmOps.push_back(spv::IdImmediate(true, operands[2])); // stride
} else {
idImmOps.push_back(spv::IdImmediate(true, operands[2])); // stride
idImmOps.push_back(spv::IdImmediate(true, operands[3])); // colMajor
}
idImmOps.insert(idImmOps.end(), memoryAccessOperands.begin(), memoryAccessOperands.end());
if (node->getOp() == glslang::EOpCooperativeMatrixStore)
builder.createNoResultOp(spv::OpCooperativeMatrixStoreKHR, idImmOps);
else
builder.createNoResultOp(spv::OpCooperativeMatrixStoreNV, idImmOps);
result = 0;
} else if (node->getOp() == glslang::EOpRayQueryGetIntersectionTriangleVertexPositionsEXT) {
std::vector<spv::IdImmediate> idImmOps;
idImmOps.push_back(spv::IdImmediate(true, operands[0])); // q
idImmOps.push_back(spv::IdImmediate(true, operands[1])); // committed
spv::Id typeId = builder.makeArrayType(builder.makeVectorType(builder.makeFloatType(32), 3),
builder.makeUintConstant(3), 0);
// do the op
spv::Op spvOp = spv::OpRayQueryGetIntersectionTriangleVertexPositionsKHR;
spv::Id result = builder.createOp(spvOp, typeId, idImmOps);
// store the result to the pointer (out param 'm')
builder.createStore(result, operands[2]);
result = 0;
} else if (node->getOp() == glslang::EOpCooperativeMatrixMulAdd) {
uint32_t matrixOperands = 0;
// If the optional operand is present, initialize matrixOperands to that value.
if (glslangOperands.size() == 4 && glslangOperands[3]->getAsConstantUnion()) {
matrixOperands = glslangOperands[3]->getAsConstantUnion()->getConstArray()[0].getIConst();
}
// Determine Cooperative Matrix Operands bits from the signedness of the types.
if (isTypeSignedInt(glslangOperands[0]->getAsTyped()->getBasicType()))
matrixOperands |= spv::CooperativeMatrixOperandsMatrixASignedComponentsKHRMask;
if (isTypeSignedInt(glslangOperands[1]->getAsTyped()->getBasicType()))
matrixOperands |= spv::CooperativeMatrixOperandsMatrixBSignedComponentsKHRMask;
if (isTypeSignedInt(glslangOperands[2]->getAsTyped()->getBasicType()))
matrixOperands |= spv::CooperativeMatrixOperandsMatrixCSignedComponentsKHRMask;
if (isTypeSignedInt(node->getBasicType()))
matrixOperands |= spv::CooperativeMatrixOperandsMatrixResultSignedComponentsKHRMask;
std::vector<spv::IdImmediate> idImmOps;
idImmOps.push_back(spv::IdImmediate(true, operands[0]));
idImmOps.push_back(spv::IdImmediate(true, operands[1]));
idImmOps.push_back(spv::IdImmediate(true, operands[2]));
if (matrixOperands != 0)
idImmOps.push_back(spv::IdImmediate(false, matrixOperands));
result = builder.createOp(spv::OpCooperativeMatrixMulAddKHR, resultType(), idImmOps);
} else if (atomic) {
// Handle all atomics
glslang::TBasicType typeProxy = (node->getOp() == glslang::EOpAtomicStore)
? node->getSequence()[0]->getAsTyped()->getBasicType() : node->getBasicType();
result = createAtomicOperation(node->getOp(), precision, resultType(), operands, typeProxy,
lvalueCoherentFlags, node->getType());
} else if (node->getOp() == glslang::EOpSpirvInst) {
const auto& spirvInst = node->getSpirvInstruction();
if (spirvInst.set == "") {
std::vector<spv::IdImmediate> idImmOps;
for (unsigned int i = 0; i < glslangOperands.size(); ++i) {
if (glslangOperands[i]->getAsTyped()->getQualifier().isSpirvLiteral()) {
// Translate the constant to a literal value
std::vector<unsigned> literals;
glslang::TVector<const glslang::TIntermConstantUnion*> constants;
constants.push_back(glslangOperands[i]->getAsConstantUnion());
TranslateLiterals(constants, literals);
idImmOps.push_back({false, literals[0]});
} else
idImmOps.push_back({true, operands[i]});
}
if (node->getBasicType() == glslang::EbtVoid)
builder.createNoResultOp(static_cast<spv::Op>(spirvInst.id), idImmOps);
else
result = builder.createOp(static_cast<spv::Op>(spirvInst.id), resultType(), idImmOps);
} else {
result = builder.createBuiltinCall(
resultType(), spirvInst.set == "GLSL.std.450" ? stdBuiltins : getExtBuiltins(spirvInst.set.c_str()),
spirvInst.id, operands);
}
noReturnValue = node->getBasicType() == glslang::EbtVoid;
} else if (node->getOp() == glslang::EOpDebugPrintf) {
if (!nonSemanticDebugPrintf) {
nonSemanticDebugPrintf = builder.import("NonSemantic.DebugPrintf");
}
result = builder.createBuiltinCall(builder.makeVoidType(), nonSemanticDebugPrintf, spv::NonSemanticDebugPrintfDebugPrintf, operands);
builder.addExtension(spv::E_SPV_KHR_non_semantic_info);
} else {
// Pass through to generic operations.
switch (glslangOperands.size()) {
case 0:
result = createNoArgOperation(node->getOp(), precision, resultType());
break;
case 1:
{
OpDecorations decorations = { precision,
TranslateNoContractionDecoration(node->getType().getQualifier()),
TranslateNonUniformDecoration(node->getType().getQualifier()) };
result = createUnaryOperation(
node->getOp(), decorations,
resultType(), operands.front(),
glslangOperands[0]->getAsTyped()->getBasicType(), lvalueCoherentFlags, node->getType());
}
break;
default:
result = createMiscOperation(node->getOp(), precision, resultType(), operands, node->getBasicType());
break;
}
if (invertedType != spv::NoResult)
result = createInvertedSwizzle(precision, *glslangOperands[0]->getAsBinaryNode(), result);
for (unsigned int i = 0; i < temporaryLvalues.size(); ++i) {
builder.setAccessChain(complexLvalues[i]);
builder.accessChainStore(builder.createLoad(temporaryLvalues[i], spv::NoPrecision),
TranslateNonUniformDecoration(complexLvalues[i].coherentFlags));
}
}
if (noReturnValue)
return false;
if (! result) {
logger->missingFunctionality("unknown glslang aggregate");
return true; // pick up a child as a placeholder operand
} else {
builder.clearAccessChain();
builder.setAccessChainRValue(result);
return false;
}
}
// This path handles both if-then-else and ?:
// The if-then-else has a node type of void, while
// ?: has either a void or a non-void node type
//
// Leaving the result, when not void:
// GLSL only has r-values as the result of a :?, but
// if we have an l-value, that can be more efficient if it will
// become the base of a complex r-value expression, because the
// next layer copies r-values into memory to use the access-chain mechanism
bool TGlslangToSpvTraverser::visitSelection(glslang::TVisit /* visit */, glslang::TIntermSelection* node)
{
// see if OpSelect can handle it
const auto isOpSelectable = [&]() {
if (node->getBasicType() == glslang::EbtVoid)
return false;
// OpSelect can do all other types starting with SPV 1.4
if (glslangIntermediate->getSpv().spv < glslang::EShTargetSpv_1_4) {
// pre-1.4, only scalars and vectors can be handled
if ((!node->getType().isScalar() && !node->getType().isVector()))
return false;
}
return true;
};
// See if it simple and safe, or required, to execute both sides.
// Crucially, side effects must be either semantically required or avoided,
// and there are performance trade-offs.
// Return true if required or a good idea (and safe) to execute both sides,
// false otherwise.
const auto bothSidesPolicy = [&]() -> bool {
// do we have both sides?
if (node->getTrueBlock() == nullptr ||
node->getFalseBlock() == nullptr)
return false;
// required? (unless we write additional code to look for side effects
// and make performance trade-offs if none are present)
if (!node->getShortCircuit())
return true;
// if not required to execute both, decide based on performance/practicality...
if (!isOpSelectable())
return false;
assert(node->getType() == node->getTrueBlock() ->getAsTyped()->getType() &&
node->getType() == node->getFalseBlock()->getAsTyped()->getType());
// return true if a single operand to ? : is okay for OpSelect
const auto operandOkay = [](glslang::TIntermTyped* node) {
return node->getAsSymbolNode() || node->getType().getQualifier().isConstant();
};
return operandOkay(node->getTrueBlock() ->getAsTyped()) &&
operandOkay(node->getFalseBlock()->getAsTyped());
};
spv::Id result = spv::NoResult; // upcoming result selecting between trueValue and falseValue
// emit the condition before doing anything with selection
node->getCondition()->traverse(this);
spv::Id condition = accessChainLoad(node->getCondition()->getType());
// Find a way of executing both sides and selecting the right result.
const auto executeBothSides = [&]() -> void {
// execute both sides
spv::Id resultType = convertGlslangToSpvType(node->getType());
node->getTrueBlock()->traverse(this);
spv::Id trueValue = accessChainLoad(node->getTrueBlock()->getAsTyped()->getType());
node->getFalseBlock()->traverse(this);
spv::Id falseValue = accessChainLoad(node->getFalseBlock()->getAsTyped()->getType());
builder.setDebugSourceLocation(node->getLoc().line, node->getLoc().getFilename());
// done if void
if (node->getBasicType() == glslang::EbtVoid)
return;
// emit code to select between trueValue and falseValue
// see if OpSelect can handle the result type, and that the SPIR-V types
// of the inputs match the result type.
if (isOpSelectable()) {
// Emit OpSelect for this selection.
// smear condition to vector, if necessary (AST is always scalar)
// Before 1.4, smear like for mix(), starting with 1.4, keep it scalar
if (glslangIntermediate->getSpv().spv < glslang::EShTargetSpv_1_4 && builder.isVector(trueValue)) {
condition = builder.smearScalar(spv::NoPrecision, condition,
builder.makeVectorType(builder.makeBoolType(),
builder.getNumComponents(trueValue)));
}
// If the types do not match, it is because of mismatched decorations on aggregates.
// Since isOpSelectable only lets us get here for SPIR-V >= 1.4, we can use OpCopyObject
// to get matching types.
if (builder.getTypeId(trueValue) != resultType) {
trueValue = builder.createUnaryOp(spv::OpCopyLogical, resultType, trueValue);
}
if (builder.getTypeId(falseValue) != resultType) {
falseValue = builder.createUnaryOp(spv::OpCopyLogical, resultType, falseValue);
}
// OpSelect
result = builder.createTriOp(spv::OpSelect, resultType, condition, trueValue, falseValue);
builder.clearAccessChain();
builder.setAccessChainRValue(result);
} else {
// We need control flow to select the result.
// TODO: Once SPIR-V OpSelect allows arbitrary types, eliminate this path.
result = builder.createVariable(TranslatePrecisionDecoration(node->getType()),
spv::StorageClassFunction, resultType);
// Selection control:
const spv::SelectionControlMask control = TranslateSelectionControl(*node);
// make an "if" based on the value created by the condition
spv::Builder::If ifBuilder(condition, control, builder);
// emit the "then" statement
builder.clearAccessChain();
builder.setAccessChainLValue(result);
multiTypeStore(node->getType(), trueValue);
ifBuilder.makeBeginElse();
// emit the "else" statement
builder.clearAccessChain();
builder.setAccessChainLValue(result);
multiTypeStore(node->getType(), falseValue);
// finish off the control flow
ifBuilder.makeEndIf();
builder.clearAccessChain();
builder.setAccessChainLValue(result);
}
};
// Execute the one side needed, as per the condition
const auto executeOneSide = [&]() {
// Always emit control flow.
if (node->getBasicType() != glslang::EbtVoid) {
result = builder.createVariable(TranslatePrecisionDecoration(node->getType()), spv::StorageClassFunction,
convertGlslangToSpvType(node->getType()));
}
// Selection control:
const spv::SelectionControlMask control = TranslateSelectionControl(*node);
// make an "if" based on the value created by the condition
spv::Builder::If ifBuilder(condition, control, builder);
// emit the "then" statement
if (node->getTrueBlock() != nullptr) {
node->getTrueBlock()->traverse(this);
if (result != spv::NoResult) {
spv::Id load = accessChainLoad(node->getTrueBlock()->getAsTyped()->getType());
builder.clearAccessChain();
builder.setAccessChainLValue(result);
multiTypeStore(node->getType(), load);
}
}
if (node->getFalseBlock() != nullptr) {
ifBuilder.makeBeginElse();
// emit the "else" statement
node->getFalseBlock()->traverse(this);
if (result != spv::NoResult) {
spv::Id load = accessChainLoad(node->getFalseBlock()->getAsTyped()->getType());
builder.clearAccessChain();
builder.setAccessChainLValue(result);
multiTypeStore(node->getType(), load);
}
}
// finish off the control flow
ifBuilder.makeEndIf();
if (result != spv::NoResult) {
builder.clearAccessChain();
builder.setAccessChainLValue(result);
}
};
// Try for OpSelect (or a requirement to execute both sides)
if (bothSidesPolicy()) {
SpecConstantOpModeGuard spec_constant_op_mode_setter(&builder);
if (node->getType().getQualifier().isSpecConstant())
spec_constant_op_mode_setter.turnOnSpecConstantOpMode();
executeBothSides();
} else
executeOneSide();
return false;
}
bool TGlslangToSpvTraverser::visitSwitch(glslang::TVisit /* visit */, glslang::TIntermSwitch* node)
{
// emit and get the condition before doing anything with switch
node->getCondition()->traverse(this);
spv::Id selector = accessChainLoad(node->getCondition()->getAsTyped()->getType());
// Selection control:
const spv::SelectionControlMask control = TranslateSwitchControl(*node);
// browse the children to sort out code segments
int defaultSegment = -1;
std::vector<TIntermNode*> codeSegments;
glslang::TIntermSequence& sequence = node->getBody()->getSequence();
std::vector<int> caseValues;
std::vector<int> valueIndexToSegment(sequence.size()); // note: probably not all are used, it is an overestimate
for (glslang::TIntermSequence::iterator c = sequence.begin(); c != sequence.end(); ++c) {
TIntermNode* child = *c;
if (child->getAsBranchNode() && child->getAsBranchNode()->getFlowOp() == glslang::EOpDefault)
defaultSegment = (int)codeSegments.size();
else if (child->getAsBranchNode() && child->getAsBranchNode()->getFlowOp() == glslang::EOpCase) {
valueIndexToSegment[caseValues.size()] = (int)codeSegments.size();
caseValues.push_back(child->getAsBranchNode()->getExpression()->getAsConstantUnion()
->getConstArray()[0].getIConst());
} else
codeSegments.push_back(child);
}
// handle the case where the last code segment is missing, due to no code
// statements between the last case and the end of the switch statement
if ((caseValues.size() && (int)codeSegments.size() == valueIndexToSegment[caseValues.size() - 1]) ||
(int)codeSegments.size() == defaultSegment)
codeSegments.push_back(nullptr);
// make the switch statement
std::vector<spv::Block*> segmentBlocks; // returned, as the blocks allocated in the call
builder.makeSwitch(selector, control, (int)codeSegments.size(), caseValues, valueIndexToSegment, defaultSegment,
segmentBlocks);
// emit all the code in the segments
breakForLoop.push(false);
for (unsigned int s = 0; s < codeSegments.size(); ++s) {
builder.nextSwitchSegment(segmentBlocks, s);
if (codeSegments[s])
codeSegments[s]->traverse(this);
else
builder.addSwitchBreak();
}
breakForLoop.pop();
builder.endSwitch(segmentBlocks);
return false;
}
void TGlslangToSpvTraverser::visitConstantUnion(glslang::TIntermConstantUnion* node)
{
if (node->getQualifier().isSpirvLiteral())
return; // Translated to a literal value, skip further processing
int nextConst = 0;
spv::Id constant = createSpvConstantFromConstUnionArray(node->getType(), node->getConstArray(), nextConst, false);
builder.clearAccessChain();
builder.setAccessChainRValue(constant);
}
bool TGlslangToSpvTraverser::visitLoop(glslang::TVisit /* visit */, glslang::TIntermLoop* node)
{
auto blocks = builder.makeNewLoop();
builder.createBranch(&blocks.head);
// Loop control:
std::vector<unsigned int> operands;
const spv::LoopControlMask control = TranslateLoopControl(*node, operands);
// Spec requires back edges to target header blocks, and every header block
// must dominate its merge block. Make a header block first to ensure these
// conditions are met. By definition, it will contain OpLoopMerge, followed
// by a block-ending branch. But we don't want to put any other body/test
// instructions in it, since the body/test may have arbitrary instructions,
// including merges of its own.
builder.setBuildPoint(&blocks.head);
builder.setDebugSourceLocation(node->getLoc().line, node->getLoc().getFilename());
builder.createLoopMerge(&blocks.merge, &blocks.continue_target, control, operands);
if (node->testFirst() && node->getTest()) {
spv::Block& test = builder.makeNewBlock();
builder.createBranch(&test);
builder.setBuildPoint(&test);
node->getTest()->traverse(this);
spv::Id condition = accessChainLoad(node->getTest()->getType());
builder.createConditionalBranch(condition, &blocks.body, &blocks.merge);
builder.setBuildPoint(&blocks.body);
breakForLoop.push(true);
if (node->getBody())
node->getBody()->traverse(this);
builder.createBranch(&blocks.continue_target);
breakForLoop.pop();
builder.setBuildPoint(&blocks.continue_target);
if (node->getTerminal())
node->getTerminal()->traverse(this);
builder.createBranch(&blocks.head);
} else {
builder.setDebugSourceLocation(node->getLoc().line, node->getLoc().getFilename());
builder.createBranch(&blocks.body);
breakForLoop.push(true);
builder.setBuildPoint(&blocks.body);
if (node->getBody())
node->getBody()->traverse(this);
builder.createBranch(&blocks.continue_target);
breakForLoop.pop();
builder.setBuildPoint(&blocks.continue_target);
if (node->getTerminal())
node->getTerminal()->traverse(this);
if (node->getTest()) {
node->getTest()->traverse(this);
spv::Id condition =
accessChainLoad(node->getTest()->getType());
builder.createConditionalBranch(condition, &blocks.head, &blocks.merge);
} else {
// TODO: unless there was a break/return/discard instruction
// somewhere in the body, this is an infinite loop, so we should
// issue a warning.
builder.createBranch(&blocks.head);
}
}
builder.setBuildPoint(&blocks.merge);
builder.closeLoop();
return false;
}
bool TGlslangToSpvTraverser::visitBranch(glslang::TVisit /* visit */, glslang::TIntermBranch* node)
{
if (node->getExpression())
node->getExpression()->traverse(this);
builder.setDebugSourceLocation(node->getLoc().line, node->getLoc().getFilename());
switch (node->getFlowOp()) {
case glslang::EOpKill:
if (glslangIntermediate->getSpv().spv >= glslang::EShTargetSpv_1_6) {
if (glslangIntermediate->getSource() == glslang::EShSourceHlsl) {
builder.addCapability(spv::CapabilityDemoteToHelperInvocation);
builder.createNoResultOp(spv::OpDemoteToHelperInvocationEXT);
} else {
builder.makeStatementTerminator(spv::OpTerminateInvocation, "post-terminate-invocation");
}
} else {
builder.makeStatementTerminator(spv::OpKill, "post-discard");
}
break;
case glslang::EOpTerminateInvocation:
builder.addExtension(spv::E_SPV_KHR_terminate_invocation);
builder.makeStatementTerminator(spv::OpTerminateInvocation, "post-terminate-invocation");
break;
case glslang::EOpBreak:
if (breakForLoop.top())
builder.createLoopExit();
else
builder.addSwitchBreak();
break;
case glslang::EOpContinue:
builder.createLoopContinue();
break;
case glslang::EOpReturn:
if (node->getExpression() != nullptr) {
const glslang::TType& glslangReturnType = node->getExpression()->getType();
spv::Id returnId = accessChainLoad(glslangReturnType);
if (builder.getTypeId(returnId) != currentFunction->getReturnType() ||
TranslatePrecisionDecoration(glslangReturnType) != currentFunction->getReturnPrecision()) {
builder.clearAccessChain();
spv::Id copyId = builder.createVariable(currentFunction->getReturnPrecision(),
spv::StorageClassFunction, currentFunction->getReturnType());
builder.setAccessChainLValue(copyId);
multiTypeStore(glslangReturnType, returnId);
returnId = builder.createLoad(copyId, currentFunction->getReturnPrecision());
}
builder.makeReturn(false, returnId);
} else
builder.makeReturn(false);
builder.clearAccessChain();
break;
case glslang::EOpDemote:
builder.createNoResultOp(spv::OpDemoteToHelperInvocationEXT);
builder.addExtension(spv::E_SPV_EXT_demote_to_helper_invocation);
builder.addCapability(spv::CapabilityDemoteToHelperInvocationEXT);
break;
case glslang::EOpTerminateRayKHR:
builder.makeStatementTerminator(spv::OpTerminateRayKHR, "post-terminateRayKHR");
break;
case glslang::EOpIgnoreIntersectionKHR:
builder.makeStatementTerminator(spv::OpIgnoreIntersectionKHR, "post-ignoreIntersectionKHR");
break;
default:
assert(0);
break;
}
return false;
}
spv::Id TGlslangToSpvTraverser::createSpvVariable(const glslang::TIntermSymbol* node, spv::Id forcedType)
{
// First, steer off constants, which are not SPIR-V variables, but
// can still have a mapping to a SPIR-V Id.
// This includes specialization constants.
if (node->getQualifier().isConstant()) {
spv::Id result = createSpvConstant(*node);
if (result != spv::NoResult)
return result;
}
// Now, handle actual variables
spv::StorageClass storageClass = TranslateStorageClass(node->getType());
spv::Id spvType = forcedType == spv::NoType ? convertGlslangToSpvType(node->getType())
: forcedType;
const bool contains16BitType = node->getType().contains16BitFloat() ||
node->getType().contains16BitInt();
if (contains16BitType) {
switch (storageClass) {
case spv::StorageClassInput:
case spv::StorageClassOutput:
builder.addIncorporatedExtension(spv::E_SPV_KHR_16bit_storage, spv::Spv_1_3);
builder.addCapability(spv::CapabilityStorageInputOutput16);
break;
case spv::StorageClassUniform:
builder.addIncorporatedExtension(spv::E_SPV_KHR_16bit_storage, spv::Spv_1_3);
if (node->getType().getQualifier().storage == glslang::EvqBuffer)
builder.addCapability(spv::CapabilityStorageUniformBufferBlock16);
else
builder.addCapability(spv::CapabilityStorageUniform16);
break;
case spv::StorageClassPushConstant:
builder.addIncorporatedExtension(spv::E_SPV_KHR_16bit_storage, spv::Spv_1_3);
builder.addCapability(spv::CapabilityStoragePushConstant16);
break;
case spv::StorageClassStorageBuffer:
case spv::StorageClassPhysicalStorageBufferEXT:
builder.addIncorporatedExtension(spv::E_SPV_KHR_16bit_storage, spv::Spv_1_3);
builder.addCapability(spv::CapabilityStorageUniformBufferBlock16);
break;
default:
if (storageClass == spv::StorageClassWorkgroup &&
node->getType().getBasicType() == glslang::EbtBlock) {
builder.addCapability(spv::CapabilityWorkgroupMemoryExplicitLayout16BitAccessKHR);
break;
}
if (node->getType().contains16BitFloat())
builder.addCapability(spv::CapabilityFloat16);
if (node->getType().contains16BitInt())
builder.addCapability(spv::CapabilityInt16);
break;
}
}
if (node->getType().contains8BitInt()) {
if (storageClass == spv::StorageClassPushConstant) {
builder.addIncorporatedExtension(spv::E_SPV_KHR_8bit_storage, spv::Spv_1_5);
builder.addCapability(spv::CapabilityStoragePushConstant8);
} else if (storageClass == spv::StorageClassUniform) {
builder.addIncorporatedExtension(spv::E_SPV_KHR_8bit_storage, spv::Spv_1_5);
builder.addCapability(spv::CapabilityUniformAndStorageBuffer8BitAccess);
} else if (storageClass == spv::StorageClassStorageBuffer) {
builder.addIncorporatedExtension(spv::E_SPV_KHR_8bit_storage, spv::Spv_1_5);
builder.addCapability(spv::CapabilityStorageBuffer8BitAccess);
} else if (storageClass == spv::StorageClassWorkgroup &&
node->getType().getBasicType() == glslang::EbtBlock) {
builder.addCapability(spv::CapabilityWorkgroupMemoryExplicitLayout8BitAccessKHR);
} else {
builder.addCapability(spv::CapabilityInt8);
}
}
const char* name = node->getName().c_str();
if (glslang::IsAnonymous(name))
name = "";
spv::Id initializer = spv::NoResult;
if (node->getType().getQualifier().storage == glslang::EvqUniform && !node->getConstArray().empty()) {
int nextConst = 0;
initializer = createSpvConstantFromConstUnionArray(node->getType(),
node->getConstArray(),
nextConst,
false /* specConst */);
} else if (node->getType().getQualifier().isNullInit()) {
initializer = builder.makeNullConstant(spvType);
}
return builder.createVariable(spv::NoPrecision, storageClass, spvType, name, initializer, false);
}
// Return type Id of the sampled type.
spv::Id TGlslangToSpvTraverser::getSampledType(const glslang::TSampler& sampler)
{
switch (sampler.type) {
case glslang::EbtInt: return builder.makeIntType(32);
case glslang::EbtUint: return builder.makeUintType(32);
case glslang::EbtFloat: return builder.makeFloatType(32);
case glslang::EbtFloat16:
builder.addExtension(spv::E_SPV_AMD_gpu_shader_half_float_fetch);
builder.addCapability(spv::CapabilityFloat16ImageAMD);
return builder.makeFloatType(16);
case glslang::EbtInt64:
builder.addExtension(spv::E_SPV_EXT_shader_image_int64);
builder.addCapability(spv::CapabilityInt64ImageEXT);
return builder.makeIntType(64);
case glslang::EbtUint64:
builder.addExtension(spv::E_SPV_EXT_shader_image_int64);
builder.addCapability(spv::CapabilityInt64ImageEXT);
return builder.makeUintType(64);
default:
assert(0);
return builder.makeFloatType(32);
}
}
// If node is a swizzle operation, return the type that should be used if
// the swizzle base is first consumed by another operation, before the swizzle
// is applied.
spv::Id TGlslangToSpvTraverser::getInvertedSwizzleType(const glslang::TIntermTyped& node)
{
if (node.getAsOperator() &&
node.getAsOperator()->getOp() == glslang::EOpVectorSwizzle)
return convertGlslangToSpvType(node.getAsBinaryNode()->getLeft()->getType());
else
return spv::NoType;
}
// When inverting a swizzle with a parent op, this function
// will apply the swizzle operation to a completed parent operation.
spv::Id TGlslangToSpvTraverser::createInvertedSwizzle(spv::Decoration precision, const glslang::TIntermTyped& node,
spv::Id parentResult)
{
std::vector<unsigned> swizzle;
convertSwizzle(*node.getAsBinaryNode()->getRight()->getAsAggregate(), swizzle);
return builder.createRvalueSwizzle(precision, convertGlslangToSpvType(node.getType()), parentResult, swizzle);
}
// Convert a glslang AST swizzle node to a swizzle vector for building SPIR-V.
void TGlslangToSpvTraverser::convertSwizzle(const glslang::TIntermAggregate& node, std::vector<unsigned>& swizzle)
{
const glslang::TIntermSequence& swizzleSequence = node.getSequence();
for (int i = 0; i < (int)swizzleSequence.size(); ++i)
swizzle.push_back(swizzleSequence[i]->getAsConstantUnion()->getConstArray()[0].getIConst());
}
// Convert from a glslang type to an SPV type, by calling into a
// recursive version of this function. This establishes the inherited
// layout state rooted from the top-level type.
spv::Id TGlslangToSpvTraverser::convertGlslangToSpvType(const glslang::TType& type, bool forwardReferenceOnly)
{
return convertGlslangToSpvType(type, getExplicitLayout(type), type.getQualifier(), false, forwardReferenceOnly);
}
spv::LinkageType TGlslangToSpvTraverser::convertGlslangLinkageToSpv(glslang::TLinkType linkType)
{
switch (linkType) {
case glslang::ELinkExport:
return spv::LinkageTypeExport;
default:
return spv::LinkageTypeMax;
}
}
// Do full recursive conversion of an arbitrary glslang type to a SPIR-V Id.
// explicitLayout can be kept the same throughout the hierarchical recursive walk.
// Mutually recursive with convertGlslangStructToSpvType().
spv::Id TGlslangToSpvTraverser::convertGlslangToSpvType(const glslang::TType& type,
glslang::TLayoutPacking explicitLayout, const glslang::TQualifier& qualifier,
bool lastBufferBlockMember, bool forwardReferenceOnly)
{
spv::Id spvType = spv::NoResult;
switch (type.getBasicType()) {
case glslang::EbtVoid:
spvType = builder.makeVoidType();
assert (! type.isArray());
break;
case glslang::EbtBool:
// "transparent" bool doesn't exist in SPIR-V. The GLSL convention is
// a 32-bit int where non-0 means true.
if (explicitLayout != glslang::ElpNone)
spvType = builder.makeUintType(32);
else
spvType = builder.makeBoolType();
break;
case glslang::EbtInt:
spvType = builder.makeIntType(32);
break;
case glslang::EbtUint:
spvType = builder.makeUintType(32);
break;
case glslang::EbtFloat:
spvType = builder.makeFloatType(32);
break;
case glslang::EbtDouble:
spvType = builder.makeFloatType(64);
break;
case glslang::EbtFloat16:
spvType = builder.makeFloatType(16);
break;
case glslang::EbtInt8:
spvType = builder.makeIntType(8);
break;
case glslang::EbtUint8:
spvType = builder.makeUintType(8);
break;
case glslang::EbtInt16:
spvType = builder.makeIntType(16);
break;
case glslang::EbtUint16:
spvType = builder.makeUintType(16);
break;
case glslang::EbtInt64:
spvType = builder.makeIntType(64);
break;
case glslang::EbtUint64:
spvType = builder.makeUintType(64);
break;
case glslang::EbtAtomicUint:
builder.addCapability(spv::CapabilityAtomicStorage);
spvType = builder.makeUintType(32);
break;
case glslang::EbtAccStruct:
switch (glslangIntermediate->getStage()) {
case EShLangRayGen:
case EShLangIntersect:
case EShLangAnyHit:
case EShLangClosestHit:
case EShLangMiss:
case EShLangCallable:
// these all should have the RayTracingNV/KHR capability already
break;
default:
{
auto& extensions = glslangIntermediate->getRequestedExtensions();
if (extensions.find("GL_EXT_ray_query") != extensions.end()) {
builder.addExtension(spv::E_SPV_KHR_ray_query);
builder.addCapability(spv::CapabilityRayQueryKHR);
}
}
break;
}
spvType = builder.makeAccelerationStructureType();
break;
case glslang::EbtRayQuery:
{
auto& extensions = glslangIntermediate->getRequestedExtensions();
if (extensions.find("GL_EXT_ray_query") != extensions.end()) {
builder.addExtension(spv::E_SPV_KHR_ray_query);
builder.addCapability(spv::CapabilityRayQueryKHR);
}
spvType = builder.makeRayQueryType();
}
break;
case glslang::EbtReference:
{
// Make the forward pointer, then recurse to convert the structure type, then
// patch up the forward pointer with a real pointer type.
if (forwardPointers.find(type.getReferentType()) == forwardPointers.end()) {
spv::Id forwardId = builder.makeForwardPointer(spv::StorageClassPhysicalStorageBufferEXT);
forwardPointers[type.getReferentType()] = forwardId;
}
spvType = forwardPointers[type.getReferentType()];
if (!forwardReferenceOnly) {
spv::Id referentType = convertGlslangToSpvType(*type.getReferentType());
builder.makePointerFromForwardPointer(spv::StorageClassPhysicalStorageBufferEXT,
forwardPointers[type.getReferentType()],
referentType);
}
}
break;
case glslang::EbtSampler:
{
const glslang::TSampler& sampler = type.getSampler();
if (sampler.isPureSampler()) {
spvType = builder.makeSamplerType();
} else {
// an image is present, make its type
spvType = builder.makeImageType(getSampledType(sampler), TranslateDimensionality(sampler),
sampler.isShadow(), sampler.isArrayed(), sampler.isMultiSample(),
sampler.isImageClass() ? 2 : 1, TranslateImageFormat(type));
if (sampler.isCombined() &&
(!sampler.isBuffer() || glslangIntermediate->getSpv().spv < glslang::EShTargetSpv_1_6)) {
// Already has both image and sampler, make the combined type. Only combine sampler to
// buffer if before SPIR-V 1.6.
spvType = builder.makeSampledImageType(spvType);
}
}
}
break;
case glslang::EbtStruct:
case glslang::EbtBlock:
{
// If we've seen this struct type, return it
const glslang::TTypeList* glslangMembers = type.getStruct();
// Try to share structs for different layouts, but not yet for other
// kinds of qualification (primarily not yet including interpolant qualification).
if (! HasNonLayoutQualifiers(type, qualifier))
spvType = structMap[explicitLayout][qualifier.layoutMatrix][glslangMembers];
if (spvType != spv::NoResult)
break;
// else, we haven't seen it...
if (type.getBasicType() == glslang::EbtBlock)
memberRemapper[glslangTypeToIdMap[glslangMembers]].resize(glslangMembers->size());
spvType = convertGlslangStructToSpvType(type, glslangMembers, explicitLayout, qualifier);
}
break;
case glslang::EbtString:
// no type used for OpString
return 0;
case glslang::EbtHitObjectNV: {
builder.addExtension(spv::E_SPV_NV_shader_invocation_reorder);
builder.addCapability(spv::CapabilityShaderInvocationReorderNV);
spvType = builder.makeHitObjectNVType();
}
break;
case glslang::EbtSpirvType: {
// GL_EXT_spirv_intrinsics
const auto& spirvType = type.getSpirvType();
const auto& spirvInst = spirvType.spirvInst;
std::vector<spv::IdImmediate> operands;
for (const auto& typeParam : spirvType.typeParams) {
if (typeParam.getAsConstant() != nullptr) {
// Constant expression
auto constant = typeParam.getAsConstant();
if (constant->isLiteral()) {
if (constant->getBasicType() == glslang::EbtFloat) {
float floatValue = static_cast<float>(constant->getConstArray()[0].getDConst());
unsigned literal;
static_assert(sizeof(literal) == sizeof(floatValue), "sizeof(unsigned) != sizeof(float)");
memcpy(&literal, &floatValue, sizeof(literal));
operands.push_back({false, literal});
} else if (constant->getBasicType() == glslang::EbtInt) {
unsigned literal = constant->getConstArray()[0].getIConst();
operands.push_back({false, literal});
} else if (constant->getBasicType() == glslang::EbtUint) {
unsigned literal = constant->getConstArray()[0].getUConst();
operands.push_back({false, literal});
} else if (constant->getBasicType() == glslang::EbtBool) {
unsigned literal = constant->getConstArray()[0].getBConst();
operands.push_back({false, literal});
} else if (constant->getBasicType() == glslang::EbtString) {
auto str = constant->getConstArray()[0].getSConst()->c_str();
unsigned literal = 0;
char* literalPtr = reinterpret_cast<char*>(&literal);
unsigned charCount = 0;
char ch = 0;
do {
ch = *(str++);
*(literalPtr++) = ch;
++charCount;
if (charCount == 4) {
operands.push_back({false, literal});
literalPtr = reinterpret_cast<char*>(&literal);
charCount = 0;
}
} while (ch != 0);
// Partial literal is padded with 0
if (charCount > 0) {
for (; charCount < 4; ++charCount)
*(literalPtr++) = 0;
operands.push_back({false, literal});
}
} else
assert(0); // Unexpected type
} else
operands.push_back({true, createSpvConstant(*constant)});
} else {
// Type specifier
assert(typeParam.getAsType() != nullptr);
operands.push_back({true, convertGlslangToSpvType(*typeParam.getAsType())});
}
}
assert(spirvInst.set == ""); // Currently, couldn't be extended instructions.
spvType = builder.makeGenericType(static_cast<spv::Op>(spirvInst.id), operands);
break;
}
default:
assert(0);
break;
}
if (type.isMatrix())
spvType = builder.makeMatrixType(spvType, type.getMatrixCols(), type.getMatrixRows());
else {
// If this variable has a vector element count greater than 1, create a SPIR-V vector
if (type.getVectorSize() > 1)
spvType = builder.makeVectorType(spvType, type.getVectorSize());
}
if (type.isCoopMatNV()) {
builder.addCapability(spv::CapabilityCooperativeMatrixNV);
builder.addExtension(spv::E_SPV_NV_cooperative_matrix);
if (type.getBasicType() == glslang::EbtFloat16)
builder.addCapability(spv::CapabilityFloat16);
if (type.getBasicType() == glslang::EbtUint8 ||
type.getBasicType() == glslang::EbtInt8) {
builder.addCapability(spv::CapabilityInt8);
}
spv::Id scope = makeArraySizeId(*type.getTypeParameters()->arraySizes, 1);
spv::Id rows = makeArraySizeId(*type.getTypeParameters()->arraySizes, 2);
spv::Id cols = makeArraySizeId(*type.getTypeParameters()->arraySizes, 3);
spvType = builder.makeCooperativeMatrixTypeNV(spvType, scope, rows, cols);
}
if (type.isCoopMatKHR()) {
builder.addCapability(spv::CapabilityCooperativeMatrixKHR);
builder.addExtension(spv::E_SPV_KHR_cooperative_matrix);
if (type.getBasicType() == glslang::EbtFloat16)
builder.addCapability(spv::CapabilityFloat16);
if (type.getBasicType() == glslang::EbtUint8 || type.getBasicType() == glslang::EbtInt8) {
builder.addCapability(spv::CapabilityInt8);
}
spv::Id scope = makeArraySizeId(*type.getTypeParameters()->arraySizes, 0);
spv::Id rows = makeArraySizeId(*type.getTypeParameters()->arraySizes, 1);
spv::Id cols = makeArraySizeId(*type.getTypeParameters()->arraySizes, 2);
spv::Id use = builder.makeUintConstant(type.getCoopMatKHRuse());
spvType = builder.makeCooperativeMatrixTypeKHR(spvType, scope, rows, cols, use);
}
if (type.isArray()) {
int stride = 0; // keep this 0 unless doing an explicit layout; 0 will mean no decoration, no stride
// Do all but the outer dimension
if (type.getArraySizes()->getNumDims() > 1) {
// We need to decorate array strides for types needing explicit layout, except blocks.
if (explicitLayout != glslang::ElpNone && type.getBasicType() != glslang::EbtBlock) {
// Use a dummy glslang type for querying internal strides of
// arrays of arrays, but using just a one-dimensional array.
glslang::TType simpleArrayType(type, 0); // deference type of the array
while (simpleArrayType.getArraySizes()->getNumDims() > 1)
simpleArrayType.getArraySizes()->dereference();
// Will compute the higher-order strides here, rather than making a whole
// pile of types and doing repetitive recursion on their contents.
stride = getArrayStride(simpleArrayType, explicitLayout, qualifier.layoutMatrix);
}
// make the arrays
for (int dim = type.getArraySizes()->getNumDims() - 1; dim > 0; --dim) {
spvType = builder.makeArrayType(spvType, makeArraySizeId(*type.getArraySizes(), dim), stride);
if (stride > 0)
builder.addDecoration(spvType, spv::DecorationArrayStride, stride);
stride *= type.getArraySizes()->getDimSize(dim);
}
} else {
// single-dimensional array, and don't yet have stride
// We need to decorate array strides for types needing explicit layout, except blocks.
if (explicitLayout != glslang::ElpNone && type.getBasicType() != glslang::EbtBlock)
stride = getArrayStride(type, explicitLayout, qualifier.layoutMatrix);
}
// Do the outer dimension, which might not be known for a runtime-sized array.
// (Unsized arrays that survive through linking will be runtime-sized arrays)
if (type.isSizedArray())
spvType = builder.makeArrayType(spvType, makeArraySizeId(*type.getArraySizes(), 0), stride);
else {
if (!lastBufferBlockMember) {
builder.addIncorporatedExtension("SPV_EXT_descriptor_indexing", spv::Spv_1_5);
builder.addCapability(spv::CapabilityRuntimeDescriptorArrayEXT);
}
spvType = builder.makeRuntimeArray(spvType);
}
if (stride > 0)
builder.addDecoration(spvType, spv::DecorationArrayStride, stride);
}
return spvType;
}
// Apply SPIR-V decorations to the SPIR-V object (provided by SPIR-V ID). If member index is provided, the
// decorations are applied to this member.
void TGlslangToSpvTraverser::applySpirvDecorate(const glslang::TType& type, spv::Id id, std::optional<int> member)
{
assert(type.getQualifier().hasSpirvDecorate());
const glslang::TSpirvDecorate& spirvDecorate = type.getQualifier().getSpirvDecorate();
// Add spirv_decorate
for (auto& decorate : spirvDecorate.decorates) {
if (!decorate.second.empty()) {
std::vector<unsigned> literals;
TranslateLiterals(decorate.second, literals);
if (member.has_value())
builder.addMemberDecoration(id, *member, static_cast<spv::Decoration>(decorate.first), literals);
else
builder.addDecoration(id, static_cast<spv::Decoration>(decorate.first), literals);
} else {
if (member.has_value())
builder.addMemberDecoration(id, *member, static_cast<spv::Decoration>(decorate.first));
else
builder.addDecoration(id, static_cast<spv::Decoration>(decorate.first));
}
}
// Add spirv_decorate_id
if (member.has_value()) {
// spirv_decorate_id not applied to members
assert(spirvDecorate.decorateIds.empty());
} else {
for (auto& decorateId : spirvDecorate.decorateIds) {
std::vector<spv::Id> operandIds;
assert(!decorateId.second.empty());
for (auto extraOperand : decorateId.second) {
if (extraOperand->getQualifier().isFrontEndConstant())
operandIds.push_back(createSpvConstant(*extraOperand));
else
operandIds.push_back(getSymbolId(extraOperand->getAsSymbolNode()));
}
builder.addDecorationId(id, static_cast<spv::Decoration>(decorateId.first), operandIds);
}
}
// Add spirv_decorate_string
for (auto& decorateString : spirvDecorate.decorateStrings) {
std::vector<const char*> strings;
assert(!decorateString.second.empty());
for (auto extraOperand : decorateString.second) {
const char* string = extraOperand->getConstArray()[0].getSConst()->c_str();
strings.push_back(string);
}
if (member.has_value())
builder.addMemberDecoration(id, *member, static_cast<spv::Decoration>(decorateString.first), strings);
else
builder.addDecoration(id, static_cast<spv::Decoration>(decorateString.first), strings);
}
}
// TODO: this functionality should exist at a higher level, in creating the AST
//
// Identify interface members that don't have their required extension turned on.
//
bool TGlslangToSpvTraverser::filterMember(const glslang::TType& member)
{
auto& extensions = glslangIntermediate->getRequestedExtensions();
if (member.getFieldName() == "gl_SecondaryViewportMaskNV" &&
extensions.find("GL_NV_stereo_view_rendering") == extensions.end())
return true;
if (member.getFieldName() == "gl_SecondaryPositionNV" &&
extensions.find("GL_NV_stereo_view_rendering") == extensions.end())
return true;
if (glslangIntermediate->getStage() == EShLangMesh) {
if (member.getFieldName() == "gl_PrimitiveShadingRateEXT" &&
extensions.find("GL_EXT_fragment_shading_rate") == extensions.end())
return true;
}
if (glslangIntermediate->getStage() != EShLangMesh) {
if (member.getFieldName() == "gl_ViewportMask" &&
extensions.find("GL_NV_viewport_array2") == extensions.end())
return true;
if (member.getFieldName() == "gl_PositionPerViewNV" &&
extensions.find("GL_NVX_multiview_per_view_attributes") == extensions.end())
return true;
if (member.getFieldName() == "gl_ViewportMaskPerViewNV" &&
extensions.find("GL_NVX_multiview_per_view_attributes") == extensions.end())
return true;
}
return false;
};
// Do full recursive conversion of a glslang structure (or block) type to a SPIR-V Id.
// explicitLayout can be kept the same throughout the hierarchical recursive walk.
// Mutually recursive with convertGlslangToSpvType().
spv::Id TGlslangToSpvTraverser::convertGlslangStructToSpvType(const glslang::TType& type,
const glslang::TTypeList* glslangMembers,
glslang::TLayoutPacking explicitLayout,
const glslang::TQualifier& qualifier)
{
// Create a vector of struct types for SPIR-V to consume
std::vector<spv::Id> spvMembers;
int memberDelta = 0; // how much the member's index changes from glslang to SPIR-V, normally 0,
// except sometimes for blocks
std::vector<std::pair<glslang::TType*, glslang::TQualifier> > deferredForwardPointers;
for (int i = 0; i < (int)glslangMembers->size(); i++) {
auto& glslangMember = (*glslangMembers)[i];
if (glslangMember.type->hiddenMember()) {
++memberDelta;
if (type.getBasicType() == glslang::EbtBlock)
memberRemapper[glslangTypeToIdMap[glslangMembers]][i] = -1;
} else {
if (type.getBasicType() == glslang::EbtBlock) {
if (filterMember(*glslangMember.type)) {
memberDelta++;
memberRemapper[glslangTypeToIdMap[glslangMembers]][i] = -1;
continue;
}
memberRemapper[glslangTypeToIdMap[glslangMembers]][i] = i - memberDelta;
}
// modify just this child's view of the qualifier
glslang::TQualifier memberQualifier = glslangMember.type->getQualifier();
InheritQualifiers(memberQualifier, qualifier);
// manually inherit location
if (! memberQualifier.hasLocation() && qualifier.hasLocation())
memberQualifier.layoutLocation = qualifier.layoutLocation;
// recurse
bool lastBufferBlockMember = qualifier.storage == glslang::EvqBuffer &&
i == (int)glslangMembers->size() - 1;
// Make forward pointers for any pointer members.
if (glslangMember.type->isReference() &&
forwardPointers.find(glslangMember.type->getReferentType()) == forwardPointers.end()) {
deferredForwardPointers.push_back(std::make_pair(glslangMember.type, memberQualifier));
}
// Create the member type.
auto const spvMember = convertGlslangToSpvType(*glslangMember.type, explicitLayout, memberQualifier, lastBufferBlockMember,
glslangMember.type->isReference());
spvMembers.push_back(spvMember);
// Update the builder with the type's location so that we can create debug types for the structure members.
// There doesn't exist a "clean" entry point for this information to be passed along to the builder so, for now,
// it is stored in the builder and consumed during the construction of composite debug types.
// TODO: This probably warrants further investigation. This approach was decided to be the least ugly of the
// quick and dirty approaches that were tried.
// Advantages of this approach:
// + Relatively clean. No direct calls into debug type system.
// + Handles nested recursive structures.
// Disadvantages of this approach:
// + Not as clean as desired. Traverser queries/sets persistent state. This is fragile.
// + Table lookup during creation of composite debug types. This really shouldn't be necessary.
if(options.emitNonSemanticShaderDebugInfo) {
builder.debugTypeLocs[spvMember].name = glslangMember.type->getFieldName().c_str();
builder.debugTypeLocs[spvMember].line = glslangMember.loc.line;
builder.debugTypeLocs[spvMember].column = glslangMember.loc.column;
}
}
}
// Make the SPIR-V type
spv::Id spvType = builder.makeStructType(spvMembers, type.getTypeName().c_str(), false);
if (! HasNonLayoutQualifiers(type, qualifier))
structMap[explicitLayout][qualifier.layoutMatrix][glslangMembers] = spvType;
// Decorate it
decorateStructType(type, glslangMembers, explicitLayout, qualifier, spvType, spvMembers);
for (int i = 0; i < (int)deferredForwardPointers.size(); ++i) {
auto it = deferredForwardPointers[i];
convertGlslangToSpvType(*it.first, explicitLayout, it.second, false);
}
return spvType;
}
void TGlslangToSpvTraverser::decorateStructType(const glslang::TType& type,
const glslang::TTypeList* glslangMembers,
glslang::TLayoutPacking explicitLayout,
const glslang::TQualifier& qualifier,
spv::Id spvType,
const std::vector<spv::Id>& spvMembers)
{
// Name and decorate the non-hidden members
int offset = -1;
bool memberLocationInvalid = type.isArrayOfArrays() ||
(type.isArray() && (type.getQualifier().isArrayedIo(glslangIntermediate->getStage()) == false));
for (int i = 0; i < (int)glslangMembers->size(); i++) {
glslang::TType& glslangMember = *(*glslangMembers)[i].type;
int member = i;
if (type.getBasicType() == glslang::EbtBlock) {
member = memberRemapper[glslangTypeToIdMap[glslangMembers]][i];
if (filterMember(glslangMember))
continue;
}
// modify just this child's view of the qualifier
glslang::TQualifier memberQualifier = glslangMember.getQualifier();
InheritQualifiers(memberQualifier, qualifier);
// using -1 above to indicate a hidden member
if (member < 0)
continue;
builder.addMemberName(spvType, member, glslangMember.getFieldName().c_str());
builder.addMemberDecoration(spvType, member,
TranslateLayoutDecoration(glslangMember, memberQualifier.layoutMatrix));
builder.addMemberDecoration(spvType, member, TranslatePrecisionDecoration(glslangMember));
// Add interpolation and auxiliary storage decorations only to
// top-level members of Input and Output storage classes
if (type.getQualifier().storage == glslang::EvqVaryingIn ||
type.getQualifier().storage == glslang::EvqVaryingOut) {
if (type.getBasicType() == glslang::EbtBlock ||
glslangIntermediate->getSource() == glslang::EShSourceHlsl) {
builder.addMemberDecoration(spvType, member, TranslateInterpolationDecoration(memberQualifier));
builder.addMemberDecoration(spvType, member, TranslateAuxiliaryStorageDecoration(memberQualifier));
addMeshNVDecoration(spvType, member, memberQualifier);
}
}
builder.addMemberDecoration(spvType, member, TranslateInvariantDecoration(memberQualifier));
if (type.getBasicType() == glslang::EbtBlock &&
qualifier.storage == glslang::EvqBuffer) {
// Add memory decorations only to top-level members of shader storage block
std::vector<spv::Decoration> memory;
TranslateMemoryDecoration(memberQualifier, memory, glslangIntermediate->usingVulkanMemoryModel());
for (unsigned int i = 0; i < memory.size(); ++i)
builder.addMemberDecoration(spvType, member, memory[i]);
}
// Location assignment was already completed correctly by the front end,
// just track whether a member needs to be decorated.
// Ignore member locations if the container is an array, as that's
// ill-specified and decisions have been made to not allow this.
if (!memberLocationInvalid && memberQualifier.hasLocation())
builder.addMemberDecoration(spvType, member, spv::DecorationLocation, memberQualifier.layoutLocation);
// component, XFB, others
if (glslangMember.getQualifier().hasComponent())
builder.addMemberDecoration(spvType, member, spv::DecorationComponent,
glslangMember.getQualifier().layoutComponent);
if (glslangMember.getQualifier().hasXfbOffset())
builder.addMemberDecoration(spvType, member, spv::DecorationOffset,
glslangMember.getQualifier().layoutXfbOffset);
else if (explicitLayout != glslang::ElpNone) {
// figure out what to do with offset, which is accumulating
int nextOffset;
updateMemberOffset(type, glslangMember, offset, nextOffset, explicitLayout, memberQualifier.layoutMatrix);
if (offset >= 0)
builder.addMemberDecoration(spvType, member, spv::DecorationOffset, offset);
offset = nextOffset;
}
if (glslangMember.isMatrix() && explicitLayout != glslang::ElpNone)
builder.addMemberDecoration(spvType, member, spv::DecorationMatrixStride,
getMatrixStride(glslangMember, explicitLayout, memberQualifier.layoutMatrix));
// built-in variable decorations
spv::BuiltIn builtIn = TranslateBuiltInDecoration(glslangMember.getQualifier().builtIn, true);
if (builtIn != spv::BuiltInMax)
builder.addMemberDecoration(spvType, member, spv::DecorationBuiltIn, (int)builtIn);
// nonuniform
builder.addMemberDecoration(spvType, member, TranslateNonUniformDecoration(glslangMember.getQualifier()));
if (glslangIntermediate->getHlslFunctionality1() && memberQualifier.semanticName != nullptr) {
builder.addExtension("SPV_GOOGLE_hlsl_functionality1");
builder.addMemberDecoration(spvType, member, (spv::Decoration)spv::DecorationHlslSemanticGOOGLE,
memberQualifier.semanticName);
}
if (builtIn == spv::BuiltInLayer) {
// SPV_NV_viewport_array2 extension
if (glslangMember.getQualifier().layoutViewportRelative){
builder.addMemberDecoration(spvType, member, (spv::Decoration)spv::DecorationViewportRelativeNV);
builder.addCapability(spv::CapabilityShaderViewportMaskNV);
builder.addExtension(spv::E_SPV_NV_viewport_array2);
}
if (glslangMember.getQualifier().layoutSecondaryViewportRelativeOffset != -2048){
builder.addMemberDecoration(spvType, member,
(spv::Decoration)spv::DecorationSecondaryViewportRelativeNV,
glslangMember.getQualifier().layoutSecondaryViewportRelativeOffset);
builder.addCapability(spv::CapabilityShaderStereoViewNV);
builder.addExtension(spv::E_SPV_NV_stereo_view_rendering);
}
}
if (glslangMember.getQualifier().layoutPassthrough) {
builder.addMemberDecoration(spvType, member, (spv::Decoration)spv::DecorationPassthroughNV);
builder.addCapability(spv::CapabilityGeometryShaderPassthroughNV);
builder.addExtension(spv::E_SPV_NV_geometry_shader_passthrough);
}
// Add SPIR-V decorations (GL_EXT_spirv_intrinsics)
if (glslangMember.getQualifier().hasSpirvDecorate())
applySpirvDecorate(glslangMember, spvType, member);
}
// Decorate the structure
builder.addDecoration(spvType, TranslateLayoutDecoration(type, qualifier.layoutMatrix));
const auto basicType = type.getBasicType();
const auto typeStorageQualifier = type.getQualifier().storage;
if (basicType == glslang::EbtBlock) {
builder.addDecoration(spvType, TranslateBlockDecoration(typeStorageQualifier, glslangIntermediate->usingStorageBuffer()));
} else if (basicType == glslang::EbtStruct && glslangIntermediate->getSpv().vulkan > 0) {
const auto hasRuntimeArray = !spvMembers.empty() && builder.getOpCode(spvMembers.back()) == spv::OpTypeRuntimeArray;
if (hasRuntimeArray) {
builder.addDecoration(spvType, TranslateBlockDecoration(typeStorageQualifier, glslangIntermediate->usingStorageBuffer()));
}
}
if (qualifier.hasHitObjectShaderRecordNV())
builder.addDecoration(spvType, spv::DecorationHitObjectShaderRecordBufferNV);
}
// Turn the expression forming the array size into an id.
// This is not quite trivial, because of specialization constants.
// Sometimes, a raw constant is turned into an Id, and sometimes
// a specialization constant expression is.
spv::Id TGlslangToSpvTraverser::makeArraySizeId(const glslang::TArraySizes& arraySizes, int dim, bool allowZero)
{
// First, see if this is sized with a node, meaning a specialization constant:
glslang::TIntermTyped* specNode = arraySizes.getDimNode(dim);
if (specNode != nullptr) {
builder.clearAccessChain();
SpecConstantOpModeGuard spec_constant_op_mode_setter(&builder);
spec_constant_op_mode_setter.turnOnSpecConstantOpMode();
specNode->traverse(this);
return accessChainLoad(specNode->getAsTyped()->getType());
}
// Otherwise, need a compile-time (front end) size, get it:
int size = arraySizes.getDimSize(dim);
if (!allowZero)
assert(size > 0);
return builder.makeUintConstant(size);
}
// Wrap the builder's accessChainLoad to:
// - localize handling of RelaxedPrecision
// - use the SPIR-V inferred type instead of another conversion of the glslang type
// (avoids unnecessary work and possible type punning for structures)
// - do conversion of concrete to abstract type
spv::Id TGlslangToSpvTraverser::accessChainLoad(const glslang::TType& type)
{
spv::Id nominalTypeId = builder.accessChainGetInferredType();
spv::Builder::AccessChain::CoherentFlags coherentFlags = builder.getAccessChain().coherentFlags;
coherentFlags |= TranslateCoherent(type);
spv::MemoryAccessMask accessMask = spv::MemoryAccessMask(TranslateMemoryAccess(coherentFlags) & ~spv::MemoryAccessMakePointerAvailableKHRMask);
// If the value being loaded is HelperInvocation, SPIR-V 1.6 is being generated (so that
// SPV_EXT_demote_to_helper_invocation is in core) and the memory model is in use, add
// the Volatile MemoryAccess semantic.
if (type.getQualifier().builtIn == glslang::EbvHelperInvocation &&
glslangIntermediate->usingVulkanMemoryModel() &&
glslangIntermediate->getSpv().spv >= glslang::EShTargetSpv_1_6) {
accessMask = spv::MemoryAccessMask(accessMask | spv::MemoryAccessVolatileMask);
}
unsigned int alignment = builder.getAccessChain().alignment;
alignment |= type.getBufferReferenceAlignment();
spv::Id loadedId = builder.accessChainLoad(TranslatePrecisionDecoration(type),
TranslateNonUniformDecoration(builder.getAccessChain().coherentFlags),
TranslateNonUniformDecoration(type.getQualifier()),
nominalTypeId,
accessMask,
TranslateMemoryScope(coherentFlags),
alignment);
// Need to convert to abstract types when necessary
if (type.getBasicType() == glslang::EbtBool) {
loadedId = convertLoadedBoolInUniformToUint(type, nominalTypeId, loadedId);
}
return loadedId;
}
// Wrap the builder's accessChainStore to:
// - do conversion of concrete to abstract type
//
// Implicitly uses the existing builder.accessChain as the storage target.
void TGlslangToSpvTraverser::accessChainStore(const glslang::TType& type, spv::Id rvalue)
{
// Need to convert to abstract types when necessary
if (type.getBasicType() == glslang::EbtBool) {
spv::Id nominalTypeId = builder.accessChainGetInferredType();
if (builder.isScalarType(nominalTypeId)) {
// Conversion for bool
spv::Id boolType = builder.makeBoolType();
if (nominalTypeId != boolType) {
// keep these outside arguments, for determinant order-of-evaluation
spv::Id one = builder.makeUintConstant(1);
spv::Id zero = builder.makeUintConstant(0);
rvalue = builder.createTriOp(spv::OpSelect, nominalTypeId, rvalue, one, zero);
} else if (builder.getTypeId(rvalue) != boolType)
rvalue = builder.createBinOp(spv::OpINotEqual, boolType, rvalue, builder.makeUintConstant(0));
} else if (builder.isVectorType(nominalTypeId)) {
// Conversion for bvec
int vecSize = builder.getNumTypeComponents(nominalTypeId);
spv::Id bvecType = builder.makeVectorType(builder.makeBoolType(), vecSize);
if (nominalTypeId != bvecType) {
// keep these outside arguments, for determinant order-of-evaluation
spv::Id one = makeSmearedConstant(builder.makeUintConstant(1), vecSize);
spv::Id zero = makeSmearedConstant(builder.makeUintConstant(0), vecSize);
rvalue = builder.createTriOp(spv::OpSelect, nominalTypeId, rvalue, one, zero);
} else if (builder.getTypeId(rvalue) != bvecType)
rvalue = builder.createBinOp(spv::OpINotEqual, bvecType, rvalue,
makeSmearedConstant(builder.makeUintConstant(0), vecSize));
}
}
spv::Builder::AccessChain::CoherentFlags coherentFlags = builder.getAccessChain().coherentFlags;
coherentFlags |= TranslateCoherent(type);
unsigned int alignment = builder.getAccessChain().alignment;
alignment |= type.getBufferReferenceAlignment();
builder.accessChainStore(rvalue, TranslateNonUniformDecoration(builder.getAccessChain().coherentFlags),
spv::MemoryAccessMask(TranslateMemoryAccess(coherentFlags) &
~spv::MemoryAccessMakePointerVisibleKHRMask),
TranslateMemoryScope(coherentFlags), alignment);
}
// For storing when types match at the glslang level, but not might match at the
// SPIR-V level.
//
// This especially happens when a single glslang type expands to multiple
// SPIR-V types, like a struct that is used in a member-undecorated way as well
// as in a member-decorated way.
//
// NOTE: This function can handle any store request; if it's not special it
// simplifies to a simple OpStore.
//
// Implicitly uses the existing builder.accessChain as the storage target.
void TGlslangToSpvTraverser::multiTypeStore(const glslang::TType& type, spv::Id rValue)
{
// we only do the complex path here if it's an aggregate
if (! type.isStruct() && ! type.isArray()) {
accessChainStore(type, rValue);
return;
}
// and, it has to be a case of type aliasing
spv::Id rType = builder.getTypeId(rValue);
spv::Id lValue = builder.accessChainGetLValue();
spv::Id lType = builder.getContainedTypeId(builder.getTypeId(lValue));
if (lType == rType) {
accessChainStore(type, rValue);
return;
}
// Recursively (as needed) copy an aggregate type to a different aggregate type,
// where the two types were the same type in GLSL. This requires member
// by member copy, recursively.
// SPIR-V 1.4 added an instruction to do help do this.
if (glslangIntermediate->getSpv().spv >= glslang::EShTargetSpv_1_4) {
// However, bool in uniform space is changed to int, so
// OpCopyLogical does not work for that.
// TODO: It would be more robust to do a full recursive verification of the types satisfying SPIR-V rules.
bool rBool = builder.containsType(builder.getTypeId(rValue), spv::OpTypeBool, 0);
bool lBool = builder.containsType(lType, spv::OpTypeBool, 0);
if (lBool == rBool) {
spv::Id logicalCopy = builder.createUnaryOp(spv::OpCopyLogical, lType, rValue);
accessChainStore(type, logicalCopy);
return;
}
}
// If an array, copy element by element.
if (type.isArray()) {
glslang::TType glslangElementType(type, 0);
spv::Id elementRType = builder.getContainedTypeId(rType);
for (int index = 0; index < type.getOuterArraySize(); ++index) {
// get the source member
spv::Id elementRValue = builder.createCompositeExtract(rValue, elementRType, index);
// set up the target storage
builder.clearAccessChain();
builder.setAccessChainLValue(lValue);
builder.accessChainPush(builder.makeIntConstant(index), TranslateCoherent(type),
type.getBufferReferenceAlignment());
// store the member
multiTypeStore(glslangElementType, elementRValue);
}
} else {
assert(type.isStruct());
// loop over structure members
const glslang::TTypeList& members = *type.getStruct();
for (int m = 0; m < (int)members.size(); ++m) {
const glslang::TType& glslangMemberType = *members[m].type;
// get the source member
spv::Id memberRType = builder.getContainedTypeId(rType, m);
spv::Id memberRValue = builder.createCompositeExtract(rValue, memberRType, m);
// set up the target storage
builder.clearAccessChain();
builder.setAccessChainLValue(lValue);
builder.accessChainPush(builder.makeIntConstant(m), TranslateCoherent(type),
type.getBufferReferenceAlignment());
// store the member
multiTypeStore(glslangMemberType, memberRValue);
}
}
}
// Decide whether or not this type should be
// decorated with offsets and strides, and if so
// whether std140 or std430 rules should be applied.
glslang::TLayoutPacking TGlslangToSpvTraverser::getExplicitLayout(const glslang::TType& type) const
{
// has to be a block
if (type.getBasicType() != glslang::EbtBlock)
return glslang::ElpNone;
// has to be a uniform or buffer block or task in/out blocks
if (type.getQualifier().storage != glslang::EvqUniform &&
type.getQualifier().storage != glslang::EvqBuffer &&
type.getQualifier().storage != glslang::EvqShared &&
!type.getQualifier().isTaskMemory())
return glslang::ElpNone;
// return the layout to use
switch (type.getQualifier().layoutPacking) {
case glslang::ElpStd140:
case glslang::ElpStd430:
case glslang::ElpScalar:
return type.getQualifier().layoutPacking;
default:
return glslang::ElpNone;
}
}
// Given an array type, returns the integer stride required for that array
int TGlslangToSpvTraverser::getArrayStride(const glslang::TType& arrayType, glslang::TLayoutPacking explicitLayout,
glslang::TLayoutMatrix matrixLayout)
{
int size;
int stride;
glslangIntermediate->getMemberAlignment(arrayType, size, stride, explicitLayout,
matrixLayout == glslang::ElmRowMajor);
return stride;
}
// Given a matrix type, or array (of array) of matrixes type, returns the integer stride required for that matrix
// when used as a member of an interface block
int TGlslangToSpvTraverser::getMatrixStride(const glslang::TType& matrixType, glslang::TLayoutPacking explicitLayout,
glslang::TLayoutMatrix matrixLayout)
{
glslang::TType elementType;
elementType.shallowCopy(matrixType);
elementType.clearArraySizes();
int size;
int stride;
glslangIntermediate->getMemberAlignment(elementType, size, stride, explicitLayout,
matrixLayout == glslang::ElmRowMajor);
return stride;
}
// Given a member type of a struct, realign the current offset for it, and compute
// the next (not yet aligned) offset for the next member, which will get aligned
// on the next call.
// 'currentOffset' should be passed in already initialized, ready to modify, and reflecting
// the migration of data from nextOffset -> currentOffset. It should be -1 on the first call.
// -1 means a non-forced member offset (no decoration needed).
void TGlslangToSpvTraverser::updateMemberOffset(const glslang::TType& structType, const glslang::TType& memberType,
int& currentOffset, int& nextOffset, glslang::TLayoutPacking explicitLayout, glslang::TLayoutMatrix matrixLayout)
{
// this will get a positive value when deemed necessary
nextOffset = -1;
// override anything in currentOffset with user-set offset
if (memberType.getQualifier().hasOffset())
currentOffset = memberType.getQualifier().layoutOffset;
// It could be that current linker usage in glslang updated all the layoutOffset,
// in which case the following code does not matter. But, that's not quite right
// once cross-compilation unit GLSL validation is done, as the original user
// settings are needed in layoutOffset, and then the following will come into play.
if (explicitLayout == glslang::ElpNone) {
if (! memberType.getQualifier().hasOffset())
currentOffset = -1;
return;
}
// Getting this far means we need explicit offsets
if (currentOffset < 0)
currentOffset = 0;
// Now, currentOffset is valid (either 0, or from a previous nextOffset),
// but possibly not yet correctly aligned.
int memberSize;
int dummyStride;
int memberAlignment = glslangIntermediate->getMemberAlignment(memberType, memberSize, dummyStride, explicitLayout,
matrixLayout == glslang::ElmRowMajor);
bool isVectorLike = memberType.isVector();
if (memberType.isMatrix()) {
if (matrixLayout == glslang::ElmRowMajor)
isVectorLike = memberType.getMatrixRows() == 1;
else
isVectorLike = memberType.getMatrixCols() == 1;
}
// Adjust alignment for HLSL rules
// TODO: make this consistent in early phases of code:
// adjusting this late means inconsistencies with earlier code, which for reflection is an issue
// Until reflection is brought in sync with these adjustments, don't apply to $Global,
// which is the most likely to rely on reflection, and least likely to rely implicit layouts
if (glslangIntermediate->usingHlslOffsets() &&
! memberType.isStruct() && structType.getTypeName().compare("$Global") != 0) {
int componentSize;
int componentAlignment = glslangIntermediate->getBaseAlignmentScalar(memberType, componentSize);
if (! memberType.isArray() && isVectorLike && componentAlignment <= 4)
memberAlignment = componentAlignment;
// Don't add unnecessary padding after this member
if (memberType.isMatrix()) {
if (matrixLayout == glslang::ElmRowMajor)
memberSize -= componentSize * (4 - memberType.getMatrixCols());
else
memberSize -= componentSize * (4 - memberType.getMatrixRows());
} else if (memberType.isArray())
memberSize -= componentSize * (4 - memberType.getVectorSize());
}
// Bump up to member alignment
glslang::RoundToPow2(currentOffset, memberAlignment);
// Bump up to vec4 if there is a bad straddle
if (explicitLayout != glslang::ElpScalar && glslangIntermediate->improperStraddle(memberType, memberSize,
currentOffset, isVectorLike))
glslang::RoundToPow2(currentOffset, 16);
nextOffset = currentOffset + memberSize;
}
void TGlslangToSpvTraverser::declareUseOfStructMember(const glslang::TTypeList& members, int glslangMember)
{
const glslang::TBuiltInVariable glslangBuiltIn = members[glslangMember].type->getQualifier().builtIn;
switch (glslangBuiltIn)
{
case glslang::EbvPointSize:
case glslang::EbvClipDistance:
case glslang::EbvCullDistance:
case glslang::EbvViewportMaskNV:
case glslang::EbvSecondaryPositionNV:
case glslang::EbvSecondaryViewportMaskNV:
case glslang::EbvPositionPerViewNV:
case glslang::EbvViewportMaskPerViewNV:
case glslang::EbvTaskCountNV:
case glslang::EbvPrimitiveCountNV:
case glslang::EbvPrimitiveIndicesNV:
case glslang::EbvClipDistancePerViewNV:
case glslang::EbvCullDistancePerViewNV:
case glslang::EbvLayerPerViewNV:
case glslang::EbvMeshViewCountNV:
case glslang::EbvMeshViewIndicesNV:
// Generate the associated capability. Delegate to TranslateBuiltInDecoration.
// Alternately, we could just call this for any glslang built-in, since the
// capability already guards against duplicates.
TranslateBuiltInDecoration(glslangBuiltIn, false);
break;
default:
// Capabilities were already generated when the struct was declared.
break;
}
}
bool TGlslangToSpvTraverser::isShaderEntryPoint(const glslang::TIntermAggregate* node)
{
return node->getName().compare(glslangIntermediate->getEntryPointMangledName().c_str()) == 0;
}
// Does parameter need a place to keep writes, separate from the original?
// Assumes called after originalParam(), which filters out block/buffer/opaque-based
// qualifiers such that we should have only in/out/inout/constreadonly here.
bool TGlslangToSpvTraverser::writableParam(glslang::TStorageQualifier qualifier) const
{
assert(qualifier == glslang::EvqIn ||
qualifier == glslang::EvqOut ||
qualifier == glslang::EvqInOut ||
qualifier == glslang::EvqUniform ||
qualifier == glslang::EvqConstReadOnly);
return qualifier != glslang::EvqConstReadOnly &&
qualifier != glslang::EvqUniform;
}
// Is parameter pass-by-original?
bool TGlslangToSpvTraverser::originalParam(glslang::TStorageQualifier qualifier, const glslang::TType& paramType,
bool implicitThisParam)
{
if (implicitThisParam) // implicit this
return true;
if (glslangIntermediate->getSource() == glslang::EShSourceHlsl)
return paramType.getBasicType() == glslang::EbtBlock;
return (paramType.containsOpaque() && !glslangIntermediate->getBindlessMode()) || // sampler, etc.
paramType.getQualifier().isSpirvByReference() || // spirv_by_reference
(paramType.getBasicType() == glslang::EbtBlock && qualifier == glslang::EvqBuffer); // SSBO
}
// Make all the functions, skeletally, without actually visiting their bodies.
void TGlslangToSpvTraverser::makeFunctions(const glslang::TIntermSequence& glslFunctions)
{
const auto getParamDecorations = [&](std::vector<spv::Decoration>& decorations, const glslang::TType& type,
bool useVulkanMemoryModel) {
spv::Decoration paramPrecision = TranslatePrecisionDecoration(type);
if (paramPrecision != spv::NoPrecision)
decorations.push_back(paramPrecision);
TranslateMemoryDecoration(type.getQualifier(), decorations, useVulkanMemoryModel);
if (type.isReference()) {
// Original and non-writable params pass the pointer directly and
// use restrict/aliased, others are stored to a pointer in Function
// memory and use RestrictPointer/AliasedPointer.
if (originalParam(type.getQualifier().storage, type, false) ||
!writableParam(type.getQualifier().storage)) {
// TranslateMemoryDecoration added Restrict decoration already.
if (!type.getQualifier().isRestrict()) {
decorations.push_back(spv::DecorationAliased);
}
} else {
decorations.push_back(type.getQualifier().isRestrict() ? spv::DecorationRestrictPointerEXT :
spv::DecorationAliasedPointerEXT);
}
}
};
for (int f = 0; f < (int)glslFunctions.size(); ++f) {
glslang::TIntermAggregate* glslFunction = glslFunctions[f]->getAsAggregate();
if (! glslFunction || glslFunction->getOp() != glslang::EOpFunction)
continue;
if (isShaderEntryPoint(glslFunction)) {
if (glslangIntermediate->getSource() != glslang::EShSourceHlsl) {
builder.setupDebugFunctionEntry(shaderEntry, glslangIntermediate->getEntryPointMangledName().c_str(),
glslFunction->getLoc().line,
std::vector<spv::Id>(), // main function has no param
std::vector<char const*>());
}
continue;
}
// We're on a user function. Set up the basic interface for the function now,
// so that it's available to call. Translating the body will happen later.
//
// Typically (except for a "const in" parameter), an address will be passed to the
// function. What it is an address of varies:
//
// - "in" parameters not marked as "const" can be written to without modifying the calling
// argument so that write needs to be to a copy, hence the address of a copy works.
//
// - "const in" parameters can just be the r-value, as no writes need occur.
//
// - "out" and "inout" arguments can't be done as pointers to the calling argument, because
// GLSL has copy-in/copy-out semantics. They can be handled though with a pointer to a copy.
std::vector<spv::Id> paramTypes;
std::vector<char const*> paramNames;
std::vector<std::vector<spv::Decoration>> paramDecorations; // list of decorations per parameter
glslang::TIntermSequence& parameters = glslFunction->getSequence()[0]->getAsAggregate()->getSequence();
#ifdef ENABLE_HLSL
bool implicitThis = (int)parameters.size() > 0 && parameters[0]->getAsSymbolNode()->getName() ==
glslangIntermediate->implicitThisName;
#else
bool implicitThis = false;
#endif
paramDecorations.resize(parameters.size());
for (int p = 0; p < (int)parameters.size(); ++p) {
const glslang::TType& paramType = parameters[p]->getAsTyped()->getType();
spv::Id typeId = convertGlslangToSpvType(paramType);
if (originalParam(paramType.getQualifier().storage, paramType, implicitThis && p == 0))
typeId = builder.makePointer(TranslateStorageClass(paramType), typeId);
else if (writableParam(paramType.getQualifier().storage))
typeId = builder.makePointer(spv::StorageClassFunction, typeId);
else
rValueParameters.insert(parameters[p]->getAsSymbolNode()->getId());
getParamDecorations(paramDecorations[p], paramType, glslangIntermediate->usingVulkanMemoryModel());
paramTypes.push_back(typeId);
}
for (auto const parameter:parameters) {
paramNames.push_back(parameter->getAsSymbolNode()->getName().c_str());
}
spv::Block* functionBlock;
spv::Function* function = builder.makeFunctionEntry(
TranslatePrecisionDecoration(glslFunction->getType()), convertGlslangToSpvType(glslFunction->getType()),
glslFunction->getName().c_str(), convertGlslangLinkageToSpv(glslFunction->getLinkType()), paramTypes,
paramDecorations, &functionBlock);
builder.setupDebugFunctionEntry(function, glslFunction->getName().c_str(), glslFunction->getLoc().line,
paramTypes, paramNames);
if (implicitThis)
function->setImplicitThis();
// Track function to emit/call later
functionMap[glslFunction->getName().c_str()] = function;
// Set the parameter id's
for (int p = 0; p < (int)parameters.size(); ++p) {
symbolValues[parameters[p]->getAsSymbolNode()->getId()] = function->getParamId(p);
// give a name too
builder.addName(function->getParamId(p), parameters[p]->getAsSymbolNode()->getName().c_str());
const glslang::TType& paramType = parameters[p]->getAsTyped()->getType();
if (paramType.contains8BitInt())
builder.addCapability(spv::CapabilityInt8);
if (paramType.contains16BitInt())
builder.addCapability(spv::CapabilityInt16);
if (paramType.contains16BitFloat())
builder.addCapability(spv::CapabilityFloat16);
}
}
}
// Process all the initializers, while skipping the functions and link objects
void TGlslangToSpvTraverser::makeGlobalInitializers(const glslang::TIntermSequence& initializers)
{
builder.setBuildPoint(shaderEntry->getLastBlock());
for (int i = 0; i < (int)initializers.size(); ++i) {
glslang::TIntermAggregate* initializer = initializers[i]->getAsAggregate();
if (initializer && initializer->getOp() != glslang::EOpFunction && initializer->getOp() !=
glslang::EOpLinkerObjects) {
// We're on a top-level node that's not a function. Treat as an initializer, whose
// code goes into the beginning of the entry point.
initializer->traverse(this);
}
}
}
// Walk over all linker objects to create a map for payload and callable data linker objects
// and their location to be used during codegen for OpTraceKHR and OpExecuteCallableKHR
// This is done here since it is possible that these linker objects are not be referenced in the AST
void TGlslangToSpvTraverser::collectRayTracingLinkerObjects()
{
glslang::TIntermAggregate* linkerObjects = glslangIntermediate->findLinkerObjects();
for (auto& objSeq : linkerObjects->getSequence()) {
auto objNode = objSeq->getAsSymbolNode();
if (objNode != nullptr) {
if (objNode->getQualifier().hasLocation()) {
unsigned int location = objNode->getQualifier().layoutLocation;
auto st = objNode->getQualifier().storage;
int set;
switch (st)
{
case glslang::EvqPayload:
case glslang::EvqPayloadIn:
set = 0;
break;
case glslang::EvqCallableData:
case glslang::EvqCallableDataIn:
set = 1;
break;
case glslang::EvqHitObjectAttrNV:
set = 2;
break;
default:
set = -1;
}
if (set != -1)
locationToSymbol[set].insert(std::make_pair(location, objNode));
}
}
}
}
// Process all the functions, while skipping initializers.
void TGlslangToSpvTraverser::visitFunctions(const glslang::TIntermSequence& glslFunctions)
{
for (int f = 0; f < (int)glslFunctions.size(); ++f) {
glslang::TIntermAggregate* node = glslFunctions[f]->getAsAggregate();
if (node && (node->getOp() == glslang::EOpFunction || node->getOp() == glslang::EOpLinkerObjects))
node->traverse(this);
}
}
void TGlslangToSpvTraverser::handleFunctionEntry(const glslang::TIntermAggregate* node)
{
// SPIR-V functions should already be in the functionMap from the prepass
// that called makeFunctions().
currentFunction = functionMap[node->getName().c_str()];
spv::Block* functionBlock = currentFunction->getEntryBlock();
builder.setBuildPoint(functionBlock);
builder.enterFunction(currentFunction);
}
void TGlslangToSpvTraverser::translateArguments(const glslang::TIntermAggregate& node, std::vector<spv::Id>& arguments,
spv::Builder::AccessChain::CoherentFlags &lvalueCoherentFlags)
{
const glslang::TIntermSequence& glslangArguments = node.getSequence();
glslang::TSampler sampler = {};
bool cubeCompare = false;
bool f16ShadowCompare = false;
if (node.isTexture() || node.isImage()) {
sampler = glslangArguments[0]->getAsTyped()->getType().getSampler();
cubeCompare = sampler.dim == glslang::EsdCube && sampler.arrayed && sampler.shadow;
f16ShadowCompare = sampler.shadow &&
glslangArguments[1]->getAsTyped()->getType().getBasicType() == glslang::EbtFloat16;
}
for (int i = 0; i < (int)glslangArguments.size(); ++i) {
builder.clearAccessChain();
glslangArguments[i]->traverse(this);
// Special case l-value operands
bool lvalue = false;
switch (node.getOp()) {
case glslang::EOpImageAtomicAdd:
case glslang::EOpImageAtomicMin:
case glslang::EOpImageAtomicMax:
case glslang::EOpImageAtomicAnd:
case glslang::EOpImageAtomicOr:
case glslang::EOpImageAtomicXor:
case glslang::EOpImageAtomicExchange:
case glslang::EOpImageAtomicCompSwap:
case glslang::EOpImageAtomicLoad:
case glslang::EOpImageAtomicStore:
if (i == 0)
lvalue = true;
break;
case glslang::EOpSparseImageLoad:
if ((sampler.ms && i == 3) || (! sampler.ms && i == 2))
lvalue = true;
break;
case glslang::EOpSparseTexture:
if (((cubeCompare || f16ShadowCompare) && i == 3) || (! (cubeCompare || f16ShadowCompare) && i == 2))
lvalue = true;
break;
case glslang::EOpSparseTextureClamp:
if (((cubeCompare || f16ShadowCompare) && i == 4) || (! (cubeCompare || f16ShadowCompare) && i == 3))
lvalue = true;
break;
case glslang::EOpSparseTextureLod:
case glslang::EOpSparseTextureOffset:
if ((f16ShadowCompare && i == 4) || (! f16ShadowCompare && i == 3))
lvalue = true;
break;
case glslang::EOpSparseTextureFetch:
if ((sampler.dim != glslang::EsdRect && i == 3) || (sampler.dim == glslang::EsdRect && i == 2))
lvalue = true;
break;
case glslang::EOpSparseTextureFetchOffset:
if ((sampler.dim != glslang::EsdRect && i == 4) || (sampler.dim == glslang::EsdRect && i == 3))
lvalue = true;
break;
case glslang::EOpSparseTextureLodOffset:
case glslang::EOpSparseTextureGrad:
case glslang::EOpSparseTextureOffsetClamp:
if ((f16ShadowCompare && i == 5) || (! f16ShadowCompare && i == 4))
lvalue = true;
break;
case glslang::EOpSparseTextureGradOffset:
case glslang::EOpSparseTextureGradClamp:
if ((f16ShadowCompare && i == 6) || (! f16ShadowCompare && i == 5))
lvalue = true;
break;
case glslang::EOpSparseTextureGradOffsetClamp:
if ((f16ShadowCompare && i == 7) || (! f16ShadowCompare && i == 6))
lvalue = true;
break;
case glslang::EOpSparseTextureGather:
if ((sampler.shadow && i == 3) || (! sampler.shadow && i == 2))
lvalue = true;
break;
case glslang::EOpSparseTextureGatherOffset:
case glslang::EOpSparseTextureGatherOffsets:
if ((sampler.shadow && i == 4) || (! sampler.shadow && i == 3))
lvalue = true;
break;
case glslang::EOpSparseTextureGatherLod:
if (i == 3)
lvalue = true;
break;
case glslang::EOpSparseTextureGatherLodOffset:
case glslang::EOpSparseTextureGatherLodOffsets:
if (i == 4)
lvalue = true;
break;
case glslang::EOpSparseImageLoadLod:
if (i == 3)
lvalue = true;
break;
case glslang::EOpImageSampleFootprintNV:
if (i == 4)
lvalue = true;
break;
case glslang::EOpImageSampleFootprintClampNV:
case glslang::EOpImageSampleFootprintLodNV:
if (i == 5)
lvalue = true;
break;
case glslang::EOpImageSampleFootprintGradNV:
if (i == 6)
lvalue = true;
break;
case glslang::EOpImageSampleFootprintGradClampNV:
if (i == 7)
lvalue = true;
break;
case glslang::EOpRayQueryGetIntersectionTriangleVertexPositionsEXT:
if (i == 2)
lvalue = true;
break;
default:
break;
}
if (lvalue) {
spv::Id lvalue_id = builder.accessChainGetLValue();
arguments.push_back(lvalue_id);
lvalueCoherentFlags = builder.getAccessChain().coherentFlags;
builder.addDecoration(lvalue_id, TranslateNonUniformDecoration(lvalueCoherentFlags));
lvalueCoherentFlags |= TranslateCoherent(glslangArguments[i]->getAsTyped()->getType());
} else {
if (i > 0 &&
glslangArguments[i]->getAsSymbolNode() && glslangArguments[i-1]->getAsSymbolNode() &&
glslangArguments[i]->getAsSymbolNode()->getId() == glslangArguments[i-1]->getAsSymbolNode()->getId()) {
// Reuse the id if possible
arguments.push_back(arguments[i-1]);
} else {
arguments.push_back(accessChainLoad(glslangArguments[i]->getAsTyped()->getType()));
}
}
}
}
void TGlslangToSpvTraverser::translateArguments(glslang::TIntermUnary& node, std::vector<spv::Id>& arguments)
{
builder.clearAccessChain();
node.getOperand()->traverse(this);
arguments.push_back(accessChainLoad(node.getOperand()->getType()));
}
spv::Id TGlslangToSpvTraverser::createImageTextureFunctionCall(glslang::TIntermOperator* node)
{
if (! node->isImage() && ! node->isTexture())
return spv::NoResult;
builder.setDebugSourceLocation(node->getLoc().line, node->getLoc().getFilename());
// Process a GLSL texturing op (will be SPV image)
const glslang::TType &imageType = node->getAsAggregate()
? node->getAsAggregate()->getSequence()[0]->getAsTyped()->getType()
: node->getAsUnaryNode()->getOperand()->getAsTyped()->getType();
const glslang::TSampler sampler = imageType.getSampler();
bool f16ShadowCompare = (sampler.shadow && node->getAsAggregate())
? node->getAsAggregate()->getSequence()[1]->getAsTyped()->getType().getBasicType() == glslang::EbtFloat16
: false;
const auto signExtensionMask = [&]() {
if (builder.getSpvVersion() >= spv::Spv_1_4) {
if (sampler.type == glslang::EbtUint)
return spv::ImageOperandsZeroExtendMask;
else if (sampler.type == glslang::EbtInt)
return spv::ImageOperandsSignExtendMask;
}
return spv::ImageOperandsMaskNone;
};
spv::Builder::AccessChain::CoherentFlags lvalueCoherentFlags;
std::vector<spv::Id> arguments;
if (node->getAsAggregate())
translateArguments(*node->getAsAggregate(), arguments, lvalueCoherentFlags);
else
translateArguments(*node->getAsUnaryNode(), arguments);
spv::Decoration precision = TranslatePrecisionDecoration(node->getType());
spv::Builder::TextureParameters params = { };
params.sampler = arguments[0];
glslang::TCrackedTextureOp cracked;
node->crackTexture(sampler, cracked);
const bool isUnsignedResult = node->getType().getBasicType() == glslang::EbtUint;
if (builder.isSampledImage(params.sampler) &&
((cracked.query && node->getOp() != glslang::EOpTextureQueryLod) || cracked.fragMask || cracked.fetch)) {
params.sampler = builder.createUnaryOp(spv::OpImage, builder.getImageType(params.sampler), params.sampler);
if (imageType.getQualifier().isNonUniform()) {
builder.addDecoration(params.sampler, spv::DecorationNonUniformEXT);
}
}
// Check for queries
if (cracked.query) {
switch (node->getOp()) {
case glslang::EOpImageQuerySize:
case glslang::EOpTextureQuerySize:
if (arguments.size() > 1) {
params.lod = arguments[1];
return builder.createTextureQueryCall(spv::OpImageQuerySizeLod, params, isUnsignedResult);
} else
return builder.createTextureQueryCall(spv::OpImageQuerySize, params, isUnsignedResult);
case glslang::EOpImageQuerySamples:
case glslang::EOpTextureQuerySamples:
return builder.createTextureQueryCall(spv::OpImageQuerySamples, params, isUnsignedResult);
case glslang::EOpTextureQueryLod:
params.coords = arguments[1];
return builder.createTextureQueryCall(spv::OpImageQueryLod, params, isUnsignedResult);
case glslang::EOpTextureQueryLevels:
return builder.createTextureQueryCall(spv::OpImageQueryLevels, params, isUnsignedResult);
case glslang::EOpSparseTexelsResident:
return builder.createUnaryOp(spv::OpImageSparseTexelsResident, builder.makeBoolType(), arguments[0]);
default:
assert(0);
break;
}
}
int components = node->getType().getVectorSize();
if (node->getOp() == glslang::EOpImageLoad ||
node->getOp() == glslang::EOpImageLoadLod ||
node->getOp() == glslang::EOpTextureFetch ||
node->getOp() == glslang::EOpTextureFetchOffset) {
// These must produce 4 components, per SPIR-V spec. We'll add a conversion constructor if needed.
// This will only happen through the HLSL path for operator[], so we do not have to handle e.g.
// the EOpTexture/Proj/Lod/etc family. It would be harmless to do so, but would need more logic
// here around e.g. which ones return scalars or other types.
components = 4;
}
glslang::TType returnType(node->getType().getBasicType(), glslang::EvqTemporary, components);
auto resultType = [&returnType,this]{ return convertGlslangToSpvType(returnType); };
// Check for image functions other than queries
if (node->isImage()) {
std::vector<spv::IdImmediate> operands;
auto opIt = arguments.begin();
spv::IdImmediate image = { true, *(opIt++) };
operands.push_back(image);
// Handle subpass operations
// TODO: GLSL should change to have the "MS" only on the type rather than the
// built-in function.
if (cracked.subpass) {
// add on the (0,0) coordinate
spv::Id zero = builder.makeIntConstant(0);
std::vector<spv::Id> comps;
comps.push_back(zero);
comps.push_back(zero);
spv::IdImmediate coord = { true,
builder.makeCompositeConstant(builder.makeVectorType(builder.makeIntType(32), 2), comps) };
operands.push_back(coord);
spv::IdImmediate imageOperands = { false, spv::ImageOperandsMaskNone };
imageOperands.word = imageOperands.word | signExtensionMask();
if (sampler.isMultiSample()) {
imageOperands.word = imageOperands.word | spv::ImageOperandsSampleMask;
}
if (imageOperands.word != spv::ImageOperandsMaskNone) {
operands.push_back(imageOperands);
if (sampler.isMultiSample()) {
spv::IdImmediate imageOperand = { true, *(opIt++) };
operands.push_back(imageOperand);
}
}
spv::Id result = builder.createOp(spv::OpImageRead, resultType(), operands);
builder.setPrecision(result, precision);
return result;
}
if (cracked.attachmentEXT) {
if (opIt != arguments.end()) {
spv::IdImmediate sample = { true, *opIt };
operands.push_back(sample);
}
spv::Id result = builder.createOp(spv::OpColorAttachmentReadEXT, resultType(), operands);
builder.addExtension(spv::E_SPV_EXT_shader_tile_image);
builder.setPrecision(result, precision);
return result;
}
spv::IdImmediate coord = { true, *(opIt++) };
operands.push_back(coord);
if (node->getOp() == glslang::EOpImageLoad || node->getOp() == glslang::EOpImageLoadLod) {
spv::ImageOperandsMask mask = spv::ImageOperandsMaskNone;
if (sampler.isMultiSample()) {
mask = mask | spv::ImageOperandsSampleMask;
}
if (cracked.lod) {
builder.addExtension(spv::E_SPV_AMD_shader_image_load_store_lod);
builder.addCapability(spv::CapabilityImageReadWriteLodAMD);
mask = mask | spv::ImageOperandsLodMask;
}
mask = mask | TranslateImageOperands(TranslateCoherent(imageType));
mask = (spv::ImageOperandsMask)(mask & ~spv::ImageOperandsMakeTexelAvailableKHRMask);
mask = mask | signExtensionMask();
if (mask != spv::ImageOperandsMaskNone) {
spv::IdImmediate imageOperands = { false, (unsigned int)mask };
operands.push_back(imageOperands);
}
if (mask & spv::ImageOperandsSampleMask) {
spv::IdImmediate imageOperand = { true, *opIt++ };
operands.push_back(imageOperand);
}
if (mask & spv::ImageOperandsLodMask) {
spv::IdImmediate imageOperand = { true, *opIt++ };
operands.push_back(imageOperand);
}
if (mask & spv::ImageOperandsMakeTexelVisibleKHRMask) {
spv::IdImmediate imageOperand = { true,
builder.makeUintConstant(TranslateMemoryScope(TranslateCoherent(imageType))) };
operands.push_back(imageOperand);
}
if (builder.getImageTypeFormat(builder.getImageType(operands.front().word)) == spv::ImageFormatUnknown)
builder.addCapability(spv::CapabilityStorageImageReadWithoutFormat);
std::vector<spv::Id> result(1, builder.createOp(spv::OpImageRead, resultType(), operands));
builder.setPrecision(result[0], precision);
// If needed, add a conversion constructor to the proper size.
if (components != node->getType().getVectorSize())
result[0] = builder.createConstructor(precision, result, convertGlslangToSpvType(node->getType()));
return result[0];
} else if (node->getOp() == glslang::EOpImageStore || node->getOp() == glslang::EOpImageStoreLod) {
// Push the texel value before the operands
if (sampler.isMultiSample() || cracked.lod) {
spv::IdImmediate texel = { true, *(opIt + 1) };
operands.push_back(texel);
} else {
spv::IdImmediate texel = { true, *opIt };
operands.push_back(texel);
}
spv::ImageOperandsMask mask = spv::ImageOperandsMaskNone;
if (sampler.isMultiSample()) {
mask = mask | spv::ImageOperandsSampleMask;
}
if (cracked.lod) {
builder.addExtension(spv::E_SPV_AMD_shader_image_load_store_lod);
builder.addCapability(spv::CapabilityImageReadWriteLodAMD);
mask = mask | spv::ImageOperandsLodMask;
}
mask = mask | TranslateImageOperands(TranslateCoherent(imageType));
mask = (spv::ImageOperandsMask)(mask & ~spv::ImageOperandsMakeTexelVisibleKHRMask);
mask = mask | signExtensionMask();
if (mask != spv::ImageOperandsMaskNone) {
spv::IdImmediate imageOperands = { false, (unsigned int)mask };
operands.push_back(imageOperands);
}
if (mask & spv::ImageOperandsSampleMask) {
spv::IdImmediate imageOperand = { true, *opIt++ };
operands.push_back(imageOperand);
}
if (mask & spv::ImageOperandsLodMask) {
spv::IdImmediate imageOperand = { true, *opIt++ };
operands.push_back(imageOperand);
}
if (mask & spv::ImageOperandsMakeTexelAvailableKHRMask) {
spv::IdImmediate imageOperand = { true,
builder.makeUintConstant(TranslateMemoryScope(TranslateCoherent(imageType))) };
operands.push_back(imageOperand);
}
builder.createNoResultOp(spv::OpImageWrite, operands);
if (builder.getImageTypeFormat(builder.getImageType(operands.front().word)) == spv::ImageFormatUnknown)
builder.addCapability(spv::CapabilityStorageImageWriteWithoutFormat);
return spv::NoResult;
} else if (node->getOp() == glslang::EOpSparseImageLoad ||
node->getOp() == glslang::EOpSparseImageLoadLod) {
builder.addCapability(spv::CapabilitySparseResidency);
if (builder.getImageTypeFormat(builder.getImageType(operands.front().word)) == spv::ImageFormatUnknown)
builder.addCapability(spv::CapabilityStorageImageReadWithoutFormat);
spv::ImageOperandsMask mask = spv::ImageOperandsMaskNone;
if (sampler.isMultiSample()) {
mask = mask | spv::ImageOperandsSampleMask;
}
if (cracked.lod) {
builder.addExtension(spv::E_SPV_AMD_shader_image_load_store_lod);
builder.addCapability(spv::CapabilityImageReadWriteLodAMD);
mask = mask | spv::ImageOperandsLodMask;
}
mask = mask | TranslateImageOperands(TranslateCoherent(imageType));
mask = (spv::ImageOperandsMask)(mask & ~spv::ImageOperandsMakeTexelAvailableKHRMask);
mask = mask | signExtensionMask();
if (mask != spv::ImageOperandsMaskNone) {
spv::IdImmediate imageOperands = { false, (unsigned int)mask };
operands.push_back(imageOperands);
}
if (mask & spv::ImageOperandsSampleMask) {
spv::IdImmediate imageOperand = { true, *opIt++ };
operands.push_back(imageOperand);
}
if (mask & spv::ImageOperandsLodMask) {
spv::IdImmediate imageOperand = { true, *opIt++ };
operands.push_back(imageOperand);
}
if (mask & spv::ImageOperandsMakeTexelVisibleKHRMask) {
spv::IdImmediate imageOperand = { true, builder.makeUintConstant(TranslateMemoryScope(
TranslateCoherent(imageType))) };
operands.push_back(imageOperand);
}
// Create the return type that was a special structure
spv::Id texelOut = *opIt;
spv::Id typeId0 = resultType();
spv::Id typeId1 = builder.getDerefTypeId(texelOut);
spv::Id resultTypeId = builder.makeStructResultType(typeId0, typeId1);
spv::Id resultId = builder.createOp(spv::OpImageSparseRead, resultTypeId, operands);
// Decode the return type
builder.createStore(builder.createCompositeExtract(resultId, typeId1, 1), texelOut);
return builder.createCompositeExtract(resultId, typeId0, 0);
} else {
// Process image atomic operations
// GLSL "IMAGE_PARAMS" will involve in constructing an image texel pointer and this pointer,
// as the first source operand, is required by SPIR-V atomic operations.
// For non-MS, the sample value should be 0
spv::IdImmediate sample = { true, sampler.isMultiSample() ? *(opIt++) : builder.makeUintConstant(0) };
operands.push_back(sample);
spv::Id resultTypeId;
glslang::TBasicType typeProxy = node->getBasicType();
// imageAtomicStore has a void return type so base the pointer type on
// the type of the value operand.
if (node->getOp() == glslang::EOpImageAtomicStore) {
resultTypeId = builder.makePointer(spv::StorageClassImage, builder.getTypeId(*opIt));
typeProxy = node->getAsAggregate()->getSequence()[0]->getAsTyped()->getType().getSampler().type;
} else {
resultTypeId = builder.makePointer(spv::StorageClassImage, resultType());
}
spv::Id pointer = builder.createOp(spv::OpImageTexelPointer, resultTypeId, operands);
if (imageType.getQualifier().nonUniform) {
builder.addDecoration(pointer, spv::DecorationNonUniformEXT);
}
std::vector<spv::Id> operands;
operands.push_back(pointer);
for (; opIt != arguments.end(); ++opIt)
operands.push_back(*opIt);
return createAtomicOperation(node->getOp(), precision, resultType(), operands, typeProxy,
lvalueCoherentFlags, node->getType());
}
}
// Check for fragment mask functions other than queries
if (cracked.fragMask) {
assert(sampler.ms);
auto opIt = arguments.begin();
std::vector<spv::Id> operands;
operands.push_back(params.sampler);
++opIt;
if (sampler.isSubpass()) {
// add on the (0,0) coordinate
spv::Id zero = builder.makeIntConstant(0);
std::vector<spv::Id> comps;
comps.push_back(zero);
comps.push_back(zero);
operands.push_back(builder.makeCompositeConstant(
builder.makeVectorType(builder.makeIntType(32), 2), comps));
}
for (; opIt != arguments.end(); ++opIt)
operands.push_back(*opIt);
spv::Op fragMaskOp = spv::OpNop;
if (node->getOp() == glslang::EOpFragmentMaskFetch)
fragMaskOp = spv::OpFragmentMaskFetchAMD;
else if (node->getOp() == glslang::EOpFragmentFetch)
fragMaskOp = spv::OpFragmentFetchAMD;
builder.addExtension(spv::E_SPV_AMD_shader_fragment_mask);
builder.addCapability(spv::CapabilityFragmentMaskAMD);
return builder.createOp(fragMaskOp, resultType(), operands);
}
// Check for texture functions other than queries
bool sparse = node->isSparseTexture();
bool imageFootprint = node->isImageFootprint();
bool cubeCompare = sampler.dim == glslang::EsdCube && sampler.isArrayed() && sampler.isShadow();
// check for bias argument
bool bias = false;
if (! cracked.lod && ! cracked.grad && ! cracked.fetch && ! cubeCompare) {
int nonBiasArgCount = 2;
if (cracked.gather)
++nonBiasArgCount; // comp argument should be present when bias argument is present
if (f16ShadowCompare)
++nonBiasArgCount;
if (cracked.offset)
++nonBiasArgCount;
else if (cracked.offsets)
++nonBiasArgCount;
if (cracked.grad)
nonBiasArgCount += 2;
if (cracked.lodClamp)
++nonBiasArgCount;
if (sparse)
++nonBiasArgCount;
if (imageFootprint)
//Following three extra arguments
// int granularity, bool coarse, out gl_TextureFootprint2DNV footprint
nonBiasArgCount += 3;
if ((int)arguments.size() > nonBiasArgCount)
bias = true;
}
if (cracked.gather) {
const auto& sourceExtensions = glslangIntermediate->getRequestedExtensions();
if (bias || cracked.lod ||
sourceExtensions.find(glslang::E_GL_AMD_texture_gather_bias_lod) != sourceExtensions.end()) {
builder.addExtension(spv::E_SPV_AMD_texture_gather_bias_lod);
builder.addCapability(spv::CapabilityImageGatherBiasLodAMD);
}
}
// set the rest of the arguments
params.coords = arguments[1];
int extraArgs = 0;
bool noImplicitLod = false;
// sort out where Dref is coming from
if (cubeCompare || f16ShadowCompare) {
params.Dref = arguments[2];
++extraArgs;
} else if (sampler.shadow && cracked.gather) {
params.Dref = arguments[2];
++extraArgs;
} else if (sampler.shadow) {
std::vector<spv::Id> indexes;
int dRefComp;
if (cracked.proj)
dRefComp = 2; // "The resulting 3rd component of P in the shadow forms is used as Dref"
else
dRefComp = builder.getNumComponents(params.coords) - 1;
indexes.push_back(dRefComp);
params.Dref = builder.createCompositeExtract(params.coords,
builder.getScalarTypeId(builder.getTypeId(params.coords)), indexes);
}
// lod
if (cracked.lod) {
params.lod = arguments[2 + extraArgs];
++extraArgs;
} else if (glslangIntermediate->getStage() != EShLangFragment &&
!(glslangIntermediate->getStage() == EShLangCompute &&
glslangIntermediate->hasLayoutDerivativeModeNone())) {
// we need to invent the default lod for an explicit lod instruction for a non-fragment stage
noImplicitLod = true;
}
// multisample
if (sampler.isMultiSample()) {
params.sample = arguments[2 + extraArgs]; // For MS, "sample" should be specified
++extraArgs;
}
// gradient
if (cracked.grad) {
params.gradX = arguments[2 + extraArgs];
params.gradY = arguments[3 + extraArgs];
extraArgs += 2;
}
// offset and offsets
if (cracked.offset) {
params.offset = arguments[2 + extraArgs];
++extraArgs;
} else if (cracked.offsets) {
params.offsets = arguments[2 + extraArgs];
++extraArgs;
}
// lod clamp
if (cracked.lodClamp) {
params.lodClamp = arguments[2 + extraArgs];
++extraArgs;
}
// sparse
if (sparse) {
params.texelOut = arguments[2 + extraArgs];
++extraArgs;
}
// gather component
if (cracked.gather && ! sampler.shadow) {
// default component is 0, if missing, otherwise an argument
if (2 + extraArgs < (int)arguments.size()) {
params.component = arguments[2 + extraArgs];
++extraArgs;
} else
params.component = builder.makeIntConstant(0);
}
spv::Id resultStruct = spv::NoResult;
if (imageFootprint) {
//Following three extra arguments
// int granularity, bool coarse, out gl_TextureFootprint2DNV footprint
params.granularity = arguments[2 + extraArgs];
params.coarse = arguments[3 + extraArgs];
resultStruct = arguments[4 + extraArgs];
extraArgs += 3;
}
// bias
if (bias) {
params.bias = arguments[2 + extraArgs];
++extraArgs;
}
if (imageFootprint) {
builder.addExtension(spv::E_SPV_NV_shader_image_footprint);
builder.addCapability(spv::CapabilityImageFootprintNV);
//resultStructType(OpenGL type) contains 5 elements:
//struct gl_TextureFootprint2DNV {
// uvec2 anchor;
// uvec2 offset;
// uvec2 mask;
// uint lod;
// uint granularity;
//};
//or
//struct gl_TextureFootprint3DNV {
// uvec3 anchor;
// uvec3 offset;
// uvec2 mask;
// uint lod;
// uint granularity;
//};
spv::Id resultStructType = builder.getContainedTypeId(builder.getTypeId(resultStruct));
assert(builder.isStructType(resultStructType));
//resType (SPIR-V type) contains 6 elements:
//Member 0 must be a Boolean type scalar(LOD),
//Member 1 must be a vector of integer type, whose Signedness operand is 0(anchor),
//Member 2 must be a vector of integer type, whose Signedness operand is 0(offset),
//Member 3 must be a vector of integer type, whose Signedness operand is 0(mask),
//Member 4 must be a scalar of integer type, whose Signedness operand is 0(lod),
//Member 5 must be a scalar of integer type, whose Signedness operand is 0(granularity).
std::vector<spv::Id> members;
members.push_back(resultType());
for (int i = 0; i < 5; i++) {
members.push_back(builder.getContainedTypeId(resultStructType, i));
}
spv::Id resType = builder.makeStructType(members, "ResType");
//call ImageFootprintNV
spv::Id res = builder.createTextureCall(precision, resType, sparse, cracked.fetch, cracked.proj,
cracked.gather, noImplicitLod, params, signExtensionMask());
//copy resType (SPIR-V type) to resultStructType(OpenGL type)
for (int i = 0; i < 5; i++) {
builder.clearAccessChain();
builder.setAccessChainLValue(resultStruct);
//Accessing to a struct we created, no coherent flag is set
spv::Builder::AccessChain::CoherentFlags flags;
flags.clear();
builder.accessChainPush(builder.makeIntConstant(i), flags, 0);
builder.accessChainStore(builder.createCompositeExtract(res, builder.getContainedTypeId(resType, i+1),
i+1), TranslateNonUniformDecoration(imageType.getQualifier()));
}
return builder.createCompositeExtract(res, resultType(), 0);
}
// projective component (might not to move)
// GLSL: "The texture coordinates consumed from P, not including the last component of P,
// are divided by the last component of P."
// SPIR-V: "... (u [, v] [, w], q)... It may be a vector larger than needed, but all
// unused components will appear after all used components."
if (cracked.proj) {
int projSourceComp = builder.getNumComponents(params.coords) - 1;
int projTargetComp;
switch (sampler.dim) {
case glslang::Esd1D: projTargetComp = 1; break;
case glslang::Esd2D: projTargetComp = 2; break;
case glslang::EsdRect: projTargetComp = 2; break;
default: projTargetComp = projSourceComp; break;
}
// copy the projective coordinate if we have to
if (projTargetComp != projSourceComp) {
spv::Id projComp = builder.createCompositeExtract(params.coords,
builder.getScalarTypeId(builder.getTypeId(params.coords)), projSourceComp);
params.coords = builder.createCompositeInsert(projComp, params.coords,
builder.getTypeId(params.coords), projTargetComp);
}
}
// nonprivate
if (imageType.getQualifier().nonprivate) {
params.nonprivate = true;
}
// volatile
if (imageType.getQualifier().volatil) {
params.volatil = true;
}
std::vector<spv::Id> result( 1,
builder.createTextureCall(precision, resultType(), sparse, cracked.fetch, cracked.proj, cracked.gather,
noImplicitLod, params, signExtensionMask())
);
if (components != node->getType().getVectorSize())
result[0] = builder.createConstructor(precision, result, convertGlslangToSpvType(node->getType()));
return result[0];
}
spv::Id TGlslangToSpvTraverser::handleUserFunctionCall(const glslang::TIntermAggregate* node)
{
// Grab the function's pointer from the previously created function
spv::Function* function = functionMap[node->getName().c_str()];
if (! function)
return 0;
const glslang::TIntermSequence& glslangArgs = node->getSequence();
const glslang::TQualifierList& qualifiers = node->getQualifierList();
// See comments in makeFunctions() for details about the semantics for parameter passing.
//
// These imply we need a four step process:
// 1. Evaluate the arguments
// 2. Allocate and make copies of in, out, and inout arguments
// 3. Make the call
// 4. Copy back the results
// 1. Evaluate the arguments and their types
std::vector<spv::Builder::AccessChain> lValues;
std::vector<spv::Id> rValues;
std::vector<const glslang::TType*> argTypes;
for (int a = 0; a < (int)glslangArgs.size(); ++a) {
argTypes.push_back(&glslangArgs[a]->getAsTyped()->getType());
// build l-value
builder.clearAccessChain();
glslangArgs[a]->traverse(this);
// keep outputs and pass-by-originals as l-values, evaluate others as r-values
if (originalParam(qualifiers[a], *argTypes[a], function->hasImplicitThis() && a == 0) ||
writableParam(qualifiers[a])) {
// save l-value
lValues.push_back(builder.getAccessChain());
} else {
// process r-value
rValues.push_back(accessChainLoad(*argTypes.back()));
}
}
// 2. Allocate space for anything needing a copy, and if it's "in" or "inout"
// copy the original into that space.
//
// Also, build up the list of actual arguments to pass in for the call
int lValueCount = 0;
int rValueCount = 0;
std::vector<spv::Id> spvArgs;
for (int a = 0; a < (int)glslangArgs.size(); ++a) {
spv::Id arg;
if (originalParam(qualifiers[a], *argTypes[a], function->hasImplicitThis() && a == 0)) {
builder.setAccessChain(lValues[lValueCount]);
arg = builder.accessChainGetLValue();
++lValueCount;
} else if (writableParam(qualifiers[a])) {
// need space to hold the copy
arg = builder.createVariable(function->getParamPrecision(a), spv::StorageClassFunction,
builder.getContainedTypeId(function->getParamType(a)), "param");
if (qualifiers[a] == glslang::EvqIn || qualifiers[a] == glslang::EvqInOut) {
// need to copy the input into output space
builder.setAccessChain(lValues[lValueCount]);
spv::Id copy = accessChainLoad(*argTypes[a]);
builder.clearAccessChain();
builder.setAccessChainLValue(arg);
multiTypeStore(*argTypes[a], copy);
}
++lValueCount;
} else {
// process r-value, which involves a copy for a type mismatch
if (function->getParamType(a) != builder.getTypeId(rValues[rValueCount]) ||
TranslatePrecisionDecoration(*argTypes[a]) != function->getParamPrecision(a))
{
spv::Id argCopy = builder.createVariable(function->getParamPrecision(a), spv::StorageClassFunction, function->getParamType(a), "arg");
builder.clearAccessChain();
builder.setAccessChainLValue(argCopy);
multiTypeStore(*argTypes[a], rValues[rValueCount]);
arg = builder.createLoad(argCopy, function->getParamPrecision(a));
} else
arg = rValues[rValueCount];
++rValueCount;
}
spvArgs.push_back(arg);
}
// 3. Make the call.
spv::Id result = builder.createFunctionCall(function, spvArgs);
builder.setPrecision(result, TranslatePrecisionDecoration(node->getType()));
builder.addDecoration(result, TranslateNonUniformDecoration(node->getType().getQualifier()));
// 4. Copy back out an "out" arguments.
lValueCount = 0;
for (int a = 0; a < (int)glslangArgs.size(); ++a) {
if (originalParam(qualifiers[a], *argTypes[a], function->hasImplicitThis() && a == 0))
++lValueCount;
else if (writableParam(qualifiers[a])) {
if (qualifiers[a] == glslang::EvqOut || qualifiers[a] == glslang::EvqInOut) {
spv::Id copy = builder.createLoad(spvArgs[a], spv::NoPrecision);
builder.addDecoration(copy, TranslateNonUniformDecoration(argTypes[a]->getQualifier()));
builder.setAccessChain(lValues[lValueCount]);
multiTypeStore(*argTypes[a], copy);
}
++lValueCount;
}
}
return result;
}
// Translate AST operation to SPV operation, already having SPV-based operands/types.
spv::Id TGlslangToSpvTraverser::createBinaryOperation(glslang::TOperator op, OpDecorations& decorations,
spv::Id typeId, spv::Id left, spv::Id right,
glslang::TBasicType typeProxy, bool reduceComparison)
{
bool isUnsigned = isTypeUnsignedInt(typeProxy);
bool isFloat = isTypeFloat(typeProxy);
bool isBool = typeProxy == glslang::EbtBool;
spv::Op binOp = spv::OpNop;
bool needMatchingVectors = true; // for non-matrix ops, would a scalar need to smear to match a vector?
bool comparison = false;
switch (op) {
case glslang::EOpAdd:
case glslang::EOpAddAssign:
if (isFloat)
binOp = spv::OpFAdd;
else
binOp = spv::OpIAdd;
break;
case glslang::EOpSub:
case glslang::EOpSubAssign:
if (isFloat)
binOp = spv::OpFSub;
else
binOp = spv::OpISub;
break;
case glslang::EOpMul:
case glslang::EOpMulAssign:
if (isFloat)
binOp = spv::OpFMul;
else
binOp = spv::OpIMul;
break;
case glslang::EOpVectorTimesScalar:
case glslang::EOpVectorTimesScalarAssign:
if (isFloat && (builder.isVector(left) || builder.isVector(right))) {
if (builder.isVector(right))
std::swap(left, right);
assert(builder.isScalar(right));
needMatchingVectors = false;
binOp = spv::OpVectorTimesScalar;
} else if (isFloat)
binOp = spv::OpFMul;
else
binOp = spv::OpIMul;
break;
case glslang::EOpVectorTimesMatrix:
case glslang::EOpVectorTimesMatrixAssign:
binOp = spv::OpVectorTimesMatrix;
break;
case glslang::EOpMatrixTimesVector:
binOp = spv::OpMatrixTimesVector;
break;
case glslang::EOpMatrixTimesScalar:
case glslang::EOpMatrixTimesScalarAssign:
binOp = spv::OpMatrixTimesScalar;
break;
case glslang::EOpMatrixTimesMatrix:
case glslang::EOpMatrixTimesMatrixAssign:
binOp = spv::OpMatrixTimesMatrix;
break;
case glslang::EOpOuterProduct:
binOp = spv::OpOuterProduct;
needMatchingVectors = false;
break;
case glslang::EOpDiv:
case glslang::EOpDivAssign:
if (isFloat)
binOp = spv::OpFDiv;
else if (isUnsigned)
binOp = spv::OpUDiv;
else
binOp = spv::OpSDiv;
break;
case glslang::EOpMod:
case glslang::EOpModAssign:
if (isFloat)
binOp = spv::OpFMod;
else if (isUnsigned)
binOp = spv::OpUMod;
else
binOp = spv::OpSMod;
break;
case glslang::EOpRightShift:
case glslang::EOpRightShiftAssign:
if (isUnsigned)
binOp = spv::OpShiftRightLogical;
else
binOp = spv::OpShiftRightArithmetic;
break;
case glslang::EOpLeftShift:
case glslang::EOpLeftShiftAssign:
binOp = spv::OpShiftLeftLogical;
break;
case glslang::EOpAnd:
case glslang::EOpAndAssign:
binOp = spv::OpBitwiseAnd;
break;
case glslang::EOpLogicalAnd:
needMatchingVectors = false;
binOp = spv::OpLogicalAnd;
break;
case glslang::EOpInclusiveOr:
case glslang::EOpInclusiveOrAssign:
binOp = spv::OpBitwiseOr;
break;
case glslang::EOpLogicalOr:
needMatchingVectors = false;
binOp = spv::OpLogicalOr;
break;
case glslang::EOpExclusiveOr:
case glslang::EOpExclusiveOrAssign:
binOp = spv::OpBitwiseXor;
break;
case glslang::EOpLogicalXor:
needMatchingVectors = false;
binOp = spv::OpLogicalNotEqual;
break;
case glslang::EOpAbsDifference:
binOp = isUnsigned ? spv::OpAbsUSubINTEL : spv::OpAbsISubINTEL;
break;
case glslang::EOpAddSaturate:
binOp = isUnsigned ? spv::OpUAddSatINTEL : spv::OpIAddSatINTEL;
break;
case glslang::EOpSubSaturate:
binOp = isUnsigned ? spv::OpUSubSatINTEL : spv::OpISubSatINTEL;
break;
case glslang::EOpAverage:
binOp = isUnsigned ? spv::OpUAverageINTEL : spv::OpIAverageINTEL;
break;
case glslang::EOpAverageRounded:
binOp = isUnsigned ? spv::OpUAverageRoundedINTEL : spv::OpIAverageRoundedINTEL;
break;
case glslang::EOpMul32x16:
binOp = isUnsigned ? spv::OpUMul32x16INTEL : spv::OpIMul32x16INTEL;
break;
case glslang::EOpExpectEXT:
binOp = spv::OpExpectKHR;
break;
case glslang::EOpLessThan:
case glslang::EOpGreaterThan:
case glslang::EOpLessThanEqual:
case glslang::EOpGreaterThanEqual:
case glslang::EOpEqual:
case glslang::EOpNotEqual:
case glslang::EOpVectorEqual:
case glslang::EOpVectorNotEqual:
comparison = true;
break;
default:
break;
}
// handle mapped binary operations (should be non-comparison)
if (binOp != spv::OpNop) {
assert(comparison == false);
if (builder.isMatrix(left) || builder.isMatrix(right) ||
builder.isCooperativeMatrix(left) || builder.isCooperativeMatrix(right))
return createBinaryMatrixOperation(binOp, decorations, typeId, left, right);
// No matrix involved; make both operands be the same number of components, if needed
if (needMatchingVectors)
builder.promoteScalar(decorations.precision, left, right);
spv::Id result = builder.createBinOp(binOp, typeId, left, right);
decorations.addNoContraction(builder, result);
decorations.addNonUniform(builder, result);
return builder.setPrecision(result, decorations.precision);
}
if (! comparison)
return 0;
// Handle comparison instructions
if (reduceComparison && (op == glslang::EOpEqual || op == glslang::EOpNotEqual)
&& (builder.isVector(left) || builder.isMatrix(left) || builder.isAggregate(left))) {
spv::Id result = builder.createCompositeCompare(decorations.precision, left, right, op == glslang::EOpEqual);
decorations.addNonUniform(builder, result);
return result;
}
switch (op) {
case glslang::EOpLessThan:
if (isFloat)
binOp = spv::OpFOrdLessThan;
else if (isUnsigned)
binOp = spv::OpULessThan;
else
binOp = spv::OpSLessThan;
break;
case glslang::EOpGreaterThan:
if (isFloat)
binOp = spv::OpFOrdGreaterThan;
else if (isUnsigned)
binOp = spv::OpUGreaterThan;
else
binOp = spv::OpSGreaterThan;
break;
case glslang::EOpLessThanEqual:
if (isFloat)
binOp = spv::OpFOrdLessThanEqual;
else if (isUnsigned)
binOp = spv::OpULessThanEqual;
else
binOp = spv::OpSLessThanEqual;
break;
case glslang::EOpGreaterThanEqual:
if (isFloat)
binOp = spv::OpFOrdGreaterThanEqual;
else if (isUnsigned)
binOp = spv::OpUGreaterThanEqual;
else
binOp = spv::OpSGreaterThanEqual;
break;
case glslang::EOpEqual:
case glslang::EOpVectorEqual:
if (isFloat)
binOp = spv::OpFOrdEqual;
else if (isBool)
binOp = spv::OpLogicalEqual;
else
binOp = spv::OpIEqual;
break;
case glslang::EOpNotEqual:
case glslang::EOpVectorNotEqual:
if (isFloat)
binOp = spv::OpFUnordNotEqual;
else if (isBool)
binOp = spv::OpLogicalNotEqual;
else
binOp = spv::OpINotEqual;
break;
default:
break;
}
if (binOp != spv::OpNop) {
spv::Id result = builder.createBinOp(binOp, typeId, left, right);
decorations.addNoContraction(builder, result);
decorations.addNonUniform(builder, result);
return builder.setPrecision(result, decorations.precision);
}
return 0;
}
//
// Translate AST matrix operation to SPV operation, already having SPV-based operands/types.
// These can be any of:
//
// matrix * scalar
// scalar * matrix
// matrix * matrix linear algebraic
// matrix * vector
// vector * matrix
// matrix * matrix componentwise
// matrix op matrix op in {+, -, /}
// matrix op scalar op in {+, -, /}
// scalar op matrix op in {+, -, /}
//
spv::Id TGlslangToSpvTraverser::createBinaryMatrixOperation(spv::Op op, OpDecorations& decorations, spv::Id typeId,
spv::Id left, spv::Id right)
{
bool firstClass = true;
// First, handle first-class matrix operations (* and matrix/scalar)
switch (op) {
case spv::OpFDiv:
if (builder.isMatrix(left) && builder.isScalar(right)) {
// turn matrix / scalar into a multiply...
spv::Id resultType = builder.getTypeId(right);
right = builder.createBinOp(spv::OpFDiv, resultType, builder.makeFpConstant(resultType, 1.0), right);
op = spv::OpMatrixTimesScalar;
} else
firstClass = false;
break;
case spv::OpMatrixTimesScalar:
if (builder.isMatrix(right) || builder.isCooperativeMatrix(right))
std::swap(left, right);
assert(builder.isScalar(right));
break;
case spv::OpVectorTimesMatrix:
assert(builder.isVector(left));
assert(builder.isMatrix(right));
break;
case spv::OpMatrixTimesVector:
assert(builder.isMatrix(left));
assert(builder.isVector(right));
break;
case spv::OpMatrixTimesMatrix:
assert(builder.isMatrix(left));
assert(builder.isMatrix(right));
break;
default:
firstClass = false;
break;
}
if (builder.isCooperativeMatrix(left) || builder.isCooperativeMatrix(right))
firstClass = true;
if (firstClass) {
spv::Id result = builder.createBinOp(op, typeId, left, right);
decorations.addNoContraction(builder, result);
decorations.addNonUniform(builder, result);
return builder.setPrecision(result, decorations.precision);
}
// Handle component-wise +, -, *, %, and / for all combinations of type.
// The result type of all of them is the same type as the (a) matrix operand.
// The algorithm is to:
// - break the matrix(es) into vectors
// - smear any scalar to a vector
// - do vector operations
// - make a matrix out the vector results
switch (op) {
case spv::OpFAdd:
case spv::OpFSub:
case spv::OpFDiv:
case spv::OpFMod:
case spv::OpFMul:
{
// one time set up...
bool leftMat = builder.isMatrix(left);
bool rightMat = builder.isMatrix(right);
unsigned int numCols = leftMat ? builder.getNumColumns(left) : builder.getNumColumns(right);
int numRows = leftMat ? builder.getNumRows(left) : builder.getNumRows(right);
spv::Id scalarType = builder.getScalarTypeId(typeId);
spv::Id vecType = builder.makeVectorType(scalarType, numRows);
std::vector<spv::Id> results;
spv::Id smearVec = spv::NoResult;
if (builder.isScalar(left))
smearVec = builder.smearScalar(decorations.precision, left, vecType);
else if (builder.isScalar(right))
smearVec = builder.smearScalar(decorations.precision, right, vecType);
// do each vector op
for (unsigned int c = 0; c < numCols; ++c) {
std::vector<unsigned int> indexes;
indexes.push_back(c);
spv::Id leftVec = leftMat ? builder.createCompositeExtract( left, vecType, indexes) : smearVec;
spv::Id rightVec = rightMat ? builder.createCompositeExtract(right, vecType, indexes) : smearVec;
spv::Id result = builder.createBinOp(op, vecType, leftVec, rightVec);
decorations.addNoContraction(builder, result);
decorations.addNonUniform(builder, result);
results.push_back(builder.setPrecision(result, decorations.precision));
}
// put the pieces together
spv::Id result = builder.setPrecision(builder.createCompositeConstruct(typeId, results), decorations.precision);
decorations.addNonUniform(builder, result);
return result;
}
default:
assert(0);
return spv::NoResult;
}
}
spv::Id TGlslangToSpvTraverser::createUnaryOperation(glslang::TOperator op, OpDecorations& decorations, spv::Id typeId,
spv::Id operand, glslang::TBasicType typeProxy, const spv::Builder::AccessChain::CoherentFlags &lvalueCoherentFlags,
const glslang::TType &opType)
{
spv::Op unaryOp = spv::OpNop;
int extBuiltins = -1;
int libCall = -1;
bool isUnsigned = isTypeUnsignedInt(typeProxy);
bool isFloat = isTypeFloat(typeProxy);
switch (op) {
case glslang::EOpNegative:
if (isFloat) {
unaryOp = spv::OpFNegate;
if (builder.isMatrixType(typeId))
return createUnaryMatrixOperation(unaryOp, decorations, typeId, operand, typeProxy);
} else
unaryOp = spv::OpSNegate;
break;
case glslang::EOpLogicalNot:
case glslang::EOpVectorLogicalNot:
unaryOp = spv::OpLogicalNot;
break;
case glslang::EOpBitwiseNot:
unaryOp = spv::OpNot;
break;
case glslang::EOpDeterminant:
libCall = spv::GLSLstd450Determinant;
break;
case glslang::EOpMatrixInverse:
libCall = spv::GLSLstd450MatrixInverse;
break;
case glslang::EOpTranspose:
unaryOp = spv::OpTranspose;
break;
case glslang::EOpRadians:
libCall = spv::GLSLstd450Radians;
break;
case glslang::EOpDegrees:
libCall = spv::GLSLstd450Degrees;
break;
case glslang::EOpSin:
libCall = spv::GLSLstd450Sin;
break;
case glslang::EOpCos:
libCall = spv::GLSLstd450Cos;
break;
case glslang::EOpTan:
libCall = spv::GLSLstd450Tan;
break;
case glslang::EOpAcos:
libCall = spv::GLSLstd450Acos;
break;
case glslang::EOpAsin:
libCall = spv::GLSLstd450Asin;
break;
case glslang::EOpAtan:
libCall = spv::GLSLstd450Atan;
break;
case glslang::EOpAcosh:
libCall = spv::GLSLstd450Acosh;
break;
case glslang::EOpAsinh:
libCall = spv::GLSLstd450Asinh;
break;
case glslang::EOpAtanh:
libCall = spv::GLSLstd450Atanh;
break;
case glslang::EOpTanh:
libCall = spv::GLSLstd450Tanh;
break;
case glslang::EOpCosh:
libCall = spv::GLSLstd450Cosh;
break;
case glslang::EOpSinh:
libCall = spv::GLSLstd450Sinh;
break;
case glslang::EOpLength:
libCall = spv::GLSLstd450Length;
break;
case glslang::EOpNormalize:
libCall = spv::GLSLstd450Normalize;
break;
case glslang::EOpExp:
libCall = spv::GLSLstd450Exp;
break;
case glslang::EOpLog:
libCall = spv::GLSLstd450Log;
break;
case glslang::EOpExp2:
libCall = spv::GLSLstd450Exp2;
break;
case glslang::EOpLog2:
libCall = spv::GLSLstd450Log2;
break;
case glslang::EOpSqrt:
libCall = spv::GLSLstd450Sqrt;
break;
case glslang::EOpInverseSqrt:
libCall = spv::GLSLstd450InverseSqrt;
break;
case glslang::EOpFloor:
libCall = spv::GLSLstd450Floor;
break;
case glslang::EOpTrunc:
libCall = spv::GLSLstd450Trunc;
break;
case glslang::EOpRound:
libCall = spv::GLSLstd450Round;
break;
case glslang::EOpRoundEven:
libCall = spv::GLSLstd450RoundEven;
break;
case glslang::EOpCeil:
libCall = spv::GLSLstd450Ceil;
break;
case glslang::EOpFract:
libCall = spv::GLSLstd450Fract;
break;
case glslang::EOpIsNan:
unaryOp = spv::OpIsNan;
break;
case glslang::EOpIsInf:
unaryOp = spv::OpIsInf;
break;
case glslang::EOpIsFinite:
unaryOp = spv::OpIsFinite;
break;
case glslang::EOpFloatBitsToInt:
case glslang::EOpFloatBitsToUint:
case glslang::EOpIntBitsToFloat:
case glslang::EOpUintBitsToFloat:
case glslang::EOpDoubleBitsToInt64:
case glslang::EOpDoubleBitsToUint64:
case glslang::EOpInt64BitsToDouble:
case glslang::EOpUint64BitsToDouble:
case glslang::EOpFloat16BitsToInt16:
case glslang::EOpFloat16BitsToUint16:
case glslang::EOpInt16BitsToFloat16:
case glslang::EOpUint16BitsToFloat16:
unaryOp = spv::OpBitcast;
break;
case glslang::EOpPackSnorm2x16:
libCall = spv::GLSLstd450PackSnorm2x16;
break;
case glslang::EOpUnpackSnorm2x16:
libCall = spv::GLSLstd450UnpackSnorm2x16;
break;
case glslang::EOpPackUnorm2x16:
libCall = spv::GLSLstd450PackUnorm2x16;
break;
case glslang::EOpUnpackUnorm2x16:
libCall = spv::GLSLstd450UnpackUnorm2x16;
break;
case glslang::EOpPackHalf2x16:
libCall = spv::GLSLstd450PackHalf2x16;
break;
case glslang::EOpUnpackHalf2x16:
libCall = spv::GLSLstd450UnpackHalf2x16;
break;
case glslang::EOpPackSnorm4x8:
libCall = spv::GLSLstd450PackSnorm4x8;
break;
case glslang::EOpUnpackSnorm4x8:
libCall = spv::GLSLstd450UnpackSnorm4x8;
break;
case glslang::EOpPackUnorm4x8:
libCall = spv::GLSLstd450PackUnorm4x8;
break;
case glslang::EOpUnpackUnorm4x8:
libCall = spv::GLSLstd450UnpackUnorm4x8;
break;
case glslang::EOpPackDouble2x32:
libCall = spv::GLSLstd450PackDouble2x32;
break;
case glslang::EOpUnpackDouble2x32:
libCall = spv::GLSLstd450UnpackDouble2x32;
break;
case glslang::EOpPackInt2x32:
case glslang::EOpUnpackInt2x32:
case glslang::EOpPackUint2x32:
case glslang::EOpUnpackUint2x32:
case glslang::EOpPack16:
case glslang::EOpPack32:
case glslang::EOpPack64:
case glslang::EOpUnpack32:
case glslang::EOpUnpack16:
case glslang::EOpUnpack8:
case glslang::EOpPackInt2x16:
case glslang::EOpUnpackInt2x16:
case glslang::EOpPackUint2x16:
case glslang::EOpUnpackUint2x16:
case glslang::EOpPackInt4x16:
case glslang::EOpUnpackInt4x16:
case glslang::EOpPackUint4x16:
case glslang::EOpUnpackUint4x16:
case glslang::EOpPackFloat2x16:
case glslang::EOpUnpackFloat2x16:
unaryOp = spv::OpBitcast;
break;
case glslang::EOpDPdx:
unaryOp = spv::OpDPdx;
break;
case glslang::EOpDPdy:
unaryOp = spv::OpDPdy;
break;
case glslang::EOpFwidth:
unaryOp = spv::OpFwidth;
break;
case glslang::EOpAny:
unaryOp = spv::OpAny;
break;
case glslang::EOpAll:
unaryOp = spv::OpAll;
break;
case glslang::EOpAbs:
if (isFloat)
libCall = spv::GLSLstd450FAbs;
else
libCall = spv::GLSLstd450SAbs;
break;
case glslang::EOpSign:
if (isFloat)
libCall = spv::GLSLstd450FSign;
else
libCall = spv::GLSLstd450SSign;
break;
case glslang::EOpDPdxFine:
unaryOp = spv::OpDPdxFine;
break;
case glslang::EOpDPdyFine:
unaryOp = spv::OpDPdyFine;
break;
case glslang::EOpFwidthFine:
unaryOp = spv::OpFwidthFine;
break;
case glslang::EOpDPdxCoarse:
unaryOp = spv::OpDPdxCoarse;
break;
case glslang::EOpDPdyCoarse:
unaryOp = spv::OpDPdyCoarse;
break;
case glslang::EOpFwidthCoarse:
unaryOp = spv::OpFwidthCoarse;
break;
case glslang::EOpRayQueryProceed:
unaryOp = spv::OpRayQueryProceedKHR;
break;
case glslang::EOpRayQueryGetRayTMin:
unaryOp = spv::OpRayQueryGetRayTMinKHR;
break;
case glslang::EOpRayQueryGetRayFlags:
unaryOp = spv::OpRayQueryGetRayFlagsKHR;
break;
case glslang::EOpRayQueryGetWorldRayOrigin:
unaryOp = spv::OpRayQueryGetWorldRayOriginKHR;
break;
case glslang::EOpRayQueryGetWorldRayDirection:
unaryOp = spv::OpRayQueryGetWorldRayDirectionKHR;
break;
case glslang::EOpRayQueryGetIntersectionCandidateAABBOpaque:
unaryOp = spv::OpRayQueryGetIntersectionCandidateAABBOpaqueKHR;
break;
case glslang::EOpInterpolateAtCentroid:
if (typeProxy == glslang::EbtFloat16)
builder.addExtension(spv::E_SPV_AMD_gpu_shader_half_float);
libCall = spv::GLSLstd450InterpolateAtCentroid;
break;
case glslang::EOpAtomicCounterIncrement:
case glslang::EOpAtomicCounterDecrement:
case glslang::EOpAtomicCounter:
{
// Handle all of the atomics in one place, in createAtomicOperation()
std::vector<spv::Id> operands;
operands.push_back(operand);
return createAtomicOperation(op, decorations.precision, typeId, operands, typeProxy, lvalueCoherentFlags, opType);
}
case glslang::EOpBitFieldReverse:
unaryOp = spv::OpBitReverse;
break;
case glslang::EOpBitCount:
unaryOp = spv::OpBitCount;
break;
case glslang::EOpFindLSB:
libCall = spv::GLSLstd450FindILsb;
break;
case glslang::EOpFindMSB:
if (isUnsigned)
libCall = spv::GLSLstd450FindUMsb;
else
libCall = spv::GLSLstd450FindSMsb;
break;
case glslang::EOpCountLeadingZeros:
builder.addCapability(spv::CapabilityIntegerFunctions2INTEL);
builder.addExtension("SPV_INTEL_shader_integer_functions2");
unaryOp = spv::OpUCountLeadingZerosINTEL;
break;
case glslang::EOpCountTrailingZeros:
builder.addCapability(spv::CapabilityIntegerFunctions2INTEL);
builder.addExtension("SPV_INTEL_shader_integer_functions2");
unaryOp = spv::OpUCountTrailingZerosINTEL;
break;
case glslang::EOpBallot:
case glslang::EOpReadFirstInvocation:
case glslang::EOpAnyInvocation:
case glslang::EOpAllInvocations:
case glslang::EOpAllInvocationsEqual:
case glslang::EOpMinInvocations:
case glslang::EOpMaxInvocations:
case glslang::EOpAddInvocations:
case glslang::EOpMinInvocationsNonUniform:
case glslang::EOpMaxInvocationsNonUniform:
case glslang::EOpAddInvocationsNonUniform:
case glslang::EOpMinInvocationsInclusiveScan:
case glslang::EOpMaxInvocationsInclusiveScan:
case glslang::EOpAddInvocationsInclusiveScan:
case glslang::EOpMinInvocationsInclusiveScanNonUniform:
case glslang::EOpMaxInvocationsInclusiveScanNonUniform:
case glslang::EOpAddInvocationsInclusiveScanNonUniform:
case glslang::EOpMinInvocationsExclusiveScan:
case glslang::EOpMaxInvocationsExclusiveScan:
case glslang::EOpAddInvocationsExclusiveScan:
case glslang::EOpMinInvocationsExclusiveScanNonUniform:
case glslang::EOpMaxInvocationsExclusiveScanNonUniform:
case glslang::EOpAddInvocationsExclusiveScanNonUniform:
{
std::vector<spv::Id> operands;
operands.push_back(operand);
return createInvocationsOperation(op, typeId, operands, typeProxy);
}
case glslang::EOpSubgroupAll:
case glslang::EOpSubgroupAny:
case glslang::EOpSubgroupAllEqual:
case glslang::EOpSubgroupBroadcastFirst:
case glslang::EOpSubgroupBallot:
case glslang::EOpSubgroupInverseBallot:
case glslang::EOpSubgroupBallotBitCount:
case glslang::EOpSubgroupBallotInclusiveBitCount:
case glslang::EOpSubgroupBallotExclusiveBitCount:
case glslang::EOpSubgroupBallotFindLSB:
case glslang::EOpSubgroupBallotFindMSB:
case glslang::EOpSubgroupAdd:
case glslang::EOpSubgroupMul:
case glslang::EOpSubgroupMin:
case glslang::EOpSubgroupMax:
case glslang::EOpSubgroupAnd:
case glslang::EOpSubgroupOr:
case glslang::EOpSubgroupXor:
case glslang::EOpSubgroupInclusiveAdd:
case glslang::EOpSubgroupInclusiveMul:
case glslang::EOpSubgroupInclusiveMin:
case glslang::EOpSubgroupInclusiveMax:
case glslang::EOpSubgroupInclusiveAnd:
case glslang::EOpSubgroupInclusiveOr:
case glslang::EOpSubgroupInclusiveXor:
case glslang::EOpSubgroupExclusiveAdd:
case glslang::EOpSubgroupExclusiveMul:
case glslang::EOpSubgroupExclusiveMin:
case glslang::EOpSubgroupExclusiveMax:
case glslang::EOpSubgroupExclusiveAnd:
case glslang::EOpSubgroupExclusiveOr:
case glslang::EOpSubgroupExclusiveXor:
case glslang::EOpSubgroupQuadSwapHorizontal:
case glslang::EOpSubgroupQuadSwapVertical:
case glslang::EOpSubgroupQuadSwapDiagonal:
case glslang::EOpSubgroupQuadAll:
case glslang::EOpSubgroupQuadAny: {
std::vector<spv::Id> operands;
operands.push_back(operand);
return createSubgroupOperation(op, typeId, operands, typeProxy);
}
case glslang::EOpMbcnt:
extBuiltins = getExtBuiltins(spv::E_SPV_AMD_shader_ballot);
libCall = spv::MbcntAMD;
break;
case glslang::EOpCubeFaceIndex:
extBuiltins = getExtBuiltins(spv::E_SPV_AMD_gcn_shader);
libCall = spv::CubeFaceIndexAMD;
break;
case glslang::EOpCubeFaceCoord:
extBuiltins = getExtBuiltins(spv::E_SPV_AMD_gcn_shader);
libCall = spv::CubeFaceCoordAMD;
break;
case glslang::EOpSubgroupPartition:
unaryOp = spv::OpGroupNonUniformPartitionNV;
break;
case glslang::EOpConstructReference:
unaryOp = spv::OpBitcast;
break;
case glslang::EOpConvUint64ToAccStruct:
case glslang::EOpConvUvec2ToAccStruct:
unaryOp = spv::OpConvertUToAccelerationStructureKHR;
break;
case glslang::EOpHitObjectIsEmptyNV:
unaryOp = spv::OpHitObjectIsEmptyNV;
break;
case glslang::EOpHitObjectIsMissNV:
unaryOp = spv::OpHitObjectIsMissNV;
break;
case glslang::EOpHitObjectIsHitNV:
unaryOp = spv::OpHitObjectIsHitNV;
break;
case glslang::EOpHitObjectGetObjectRayOriginNV:
unaryOp = spv::OpHitObjectGetObjectRayOriginNV;
break;
case glslang::EOpHitObjectGetObjectRayDirectionNV:
unaryOp = spv::OpHitObjectGetObjectRayDirectionNV;
break;
case glslang::EOpHitObjectGetWorldRayOriginNV:
unaryOp = spv::OpHitObjectGetWorldRayOriginNV;
break;
case glslang::EOpHitObjectGetWorldRayDirectionNV:
unaryOp = spv::OpHitObjectGetWorldRayDirectionNV;
break;
case glslang::EOpHitObjectGetObjectToWorldNV:
unaryOp = spv::OpHitObjectGetObjectToWorldNV;
break;
case glslang::EOpHitObjectGetWorldToObjectNV:
unaryOp = spv::OpHitObjectGetWorldToObjectNV;
break;
case glslang::EOpHitObjectGetRayTMinNV:
unaryOp = spv::OpHitObjectGetRayTMinNV;
break;
case glslang::EOpHitObjectGetRayTMaxNV:
unaryOp = spv::OpHitObjectGetRayTMaxNV;
break;
case glslang::EOpHitObjectGetPrimitiveIndexNV:
unaryOp = spv::OpHitObjectGetPrimitiveIndexNV;
break;
case glslang::EOpHitObjectGetInstanceIdNV:
unaryOp = spv::OpHitObjectGetInstanceIdNV;
break;
case glslang::EOpHitObjectGetInstanceCustomIndexNV:
unaryOp = spv::OpHitObjectGetInstanceCustomIndexNV;
break;
case glslang::EOpHitObjectGetGeometryIndexNV:
unaryOp = spv::OpHitObjectGetGeometryIndexNV;
break;
case glslang::EOpHitObjectGetHitKindNV:
unaryOp = spv::OpHitObjectGetHitKindNV;
break;
case glslang::EOpHitObjectGetCurrentTimeNV:
unaryOp = spv::OpHitObjectGetCurrentTimeNV;
break;
case glslang::EOpHitObjectGetShaderBindingTableRecordIndexNV:
unaryOp = spv::OpHitObjectGetShaderBindingTableRecordIndexNV;
break;
case glslang::EOpHitObjectGetShaderRecordBufferHandleNV:
unaryOp = spv::OpHitObjectGetShaderRecordBufferHandleNV;
break;
case glslang::EOpFetchMicroTriangleVertexPositionNV:
unaryOp = spv::OpFetchMicroTriangleVertexPositionNV;
break;
case glslang::EOpFetchMicroTriangleVertexBarycentricNV:
unaryOp = spv::OpFetchMicroTriangleVertexBarycentricNV;
break;
case glslang::EOpCopyObject:
unaryOp = spv::OpCopyObject;
break;
case glslang::EOpDepthAttachmentReadEXT:
builder.addExtension(spv::E_SPV_EXT_shader_tile_image);
builder.addCapability(spv::CapabilityTileImageDepthReadAccessEXT);
unaryOp = spv::OpDepthAttachmentReadEXT;
decorations.precision = spv::NoPrecision;
break;
case glslang::EOpStencilAttachmentReadEXT:
builder.addExtension(spv::E_SPV_EXT_shader_tile_image);
builder.addCapability(spv::CapabilityTileImageStencilReadAccessEXT);
unaryOp = spv::OpStencilAttachmentReadEXT;
decorations.precision = spv::DecorationRelaxedPrecision;
break;
default:
return 0;
}
spv::Id id;
if (libCall >= 0) {
std::vector<spv::Id> args;
args.push_back(operand);
id = builder.createBuiltinCall(typeId, extBuiltins >= 0 ? extBuiltins : stdBuiltins, libCall, args);
} else {
id = builder.createUnaryOp(unaryOp, typeId, operand);
}
decorations.addNoContraction(builder, id);
decorations.addNonUniform(builder, id);
return builder.setPrecision(id, decorations.precision);
}
// Create a unary operation on a matrix
spv::Id TGlslangToSpvTraverser::createUnaryMatrixOperation(spv::Op op, OpDecorations& decorations, spv::Id typeId,
spv::Id operand, glslang::TBasicType /* typeProxy */)
{
// Handle unary operations vector by vector.
// The result type is the same type as the original type.
// The algorithm is to:
// - break the matrix into vectors
// - apply the operation to each vector
// - make a matrix out the vector results
// get the types sorted out
int numCols = builder.getNumColumns(operand);
int numRows = builder.getNumRows(operand);
spv::Id srcVecType = builder.makeVectorType(builder.getScalarTypeId(builder.getTypeId(operand)), numRows);
spv::Id destVecType = builder.makeVectorType(builder.getScalarTypeId(typeId), numRows);
std::vector<spv::Id> results;
// do each vector op
for (int c = 0; c < numCols; ++c) {
std::vector<unsigned int> indexes;
indexes.push_back(c);
spv::Id srcVec = builder.createCompositeExtract(operand, srcVecType, indexes);
spv::Id destVec = builder.createUnaryOp(op, destVecType, srcVec);
decorations.addNoContraction(builder, destVec);
decorations.addNonUniform(builder, destVec);
results.push_back(builder.setPrecision(destVec, decorations.precision));
}
// put the pieces together
spv::Id result = builder.setPrecision(builder.createCompositeConstruct(typeId, results), decorations.precision);
decorations.addNonUniform(builder, result);
return result;
}
// For converting integers where both the bitwidth and the signedness could
// change, but only do the width change here. The caller is still responsible
// for the signedness conversion.
// destType is the final type that will be converted to, but this function
// may only be doing part of that conversion.
spv::Id TGlslangToSpvTraverser::createIntWidthConversion(glslang::TOperator op, spv::Id operand, int vectorSize, spv::Id destType)
{
// Get the result type width, based on the type to convert to.
int width = 32;
switch(op) {
case glslang::EOpConvInt16ToUint8:
case glslang::EOpConvIntToUint8:
case glslang::EOpConvInt64ToUint8:
case glslang::EOpConvUint16ToInt8:
case glslang::EOpConvUintToInt8:
case glslang::EOpConvUint64ToInt8:
width = 8;
break;
case glslang::EOpConvInt8ToUint16:
case glslang::EOpConvIntToUint16:
case glslang::EOpConvInt64ToUint16:
case glslang::EOpConvUint8ToInt16:
case glslang::EOpConvUintToInt16:
case glslang::EOpConvUint64ToInt16:
width = 16;
break;
case glslang::EOpConvInt8ToUint:
case glslang::EOpConvInt16ToUint:
case glslang::EOpConvInt64ToUint:
case glslang::EOpConvUint8ToInt:
case glslang::EOpConvUint16ToInt:
case glslang::EOpConvUint64ToInt:
width = 32;
break;
case glslang::EOpConvInt8ToUint64:
case glslang::EOpConvInt16ToUint64:
case glslang::EOpConvIntToUint64:
case glslang::EOpConvUint8ToInt64:
case glslang::EOpConvUint16ToInt64:
case glslang::EOpConvUintToInt64:
width = 64;
break;
default:
assert(false && "Default missing");
break;
}
// Get the conversion operation and result type,
// based on the target width, but the source type.
spv::Id type = spv::NoType;
spv::Op convOp = spv::OpNop;
switch(op) {
case glslang::EOpConvInt8ToUint16:
case glslang::EOpConvInt8ToUint:
case glslang::EOpConvInt8ToUint64:
case glslang::EOpConvInt16ToUint8:
case glslang::EOpConvInt16ToUint:
case glslang::EOpConvInt16ToUint64:
case glslang::EOpConvIntToUint8:
case glslang::EOpConvIntToUint16:
case glslang::EOpConvIntToUint64:
case glslang::EOpConvInt64ToUint8:
case glslang::EOpConvInt64ToUint16:
case glslang::EOpConvInt64ToUint:
convOp = spv::OpSConvert;
type = builder.makeIntType(width);
break;
default:
convOp = spv::OpUConvert;
type = builder.makeUintType(width);
break;
}
if (vectorSize > 0)
type = builder.makeVectorType(type, vectorSize);
else if (builder.getOpCode(destType) == spv::OpTypeCooperativeMatrixKHR ||
builder.getOpCode(destType) == spv::OpTypeCooperativeMatrixNV) {
type = builder.makeCooperativeMatrixTypeWithSameShape(type, destType);
}
return builder.createUnaryOp(convOp, type, operand);
}
spv::Id TGlslangToSpvTraverser::createConversion(glslang::TOperator op, OpDecorations& decorations, spv::Id destType,
spv::Id operand, glslang::TBasicType typeProxy)
{
spv::Op convOp = spv::OpNop;
spv::Id zero = 0;
spv::Id one = 0;
int vectorSize = builder.isVectorType(destType) ? builder.getNumTypeComponents(destType) : 0;
switch (op) {
case glslang::EOpConvIntToBool:
case glslang::EOpConvUintToBool:
zero = builder.makeUintConstant(0);
zero = makeSmearedConstant(zero, vectorSize);
return builder.createBinOp(spv::OpINotEqual, destType, operand, zero);
case glslang::EOpConvFloatToBool:
zero = builder.makeFloatConstant(0.0F);
zero = makeSmearedConstant(zero, vectorSize);
return builder.createBinOp(spv::OpFUnordNotEqual, destType, operand, zero);
case glslang::EOpConvBoolToFloat:
convOp = spv::OpSelect;
zero = builder.makeFloatConstant(0.0F);
one = builder.makeFloatConstant(1.0F);
break;
case glslang::EOpConvBoolToInt:
case glslang::EOpConvBoolToInt64:
if (op == glslang::EOpConvBoolToInt64) {
zero = builder.makeInt64Constant(0);
one = builder.makeInt64Constant(1);
} else {
zero = builder.makeIntConstant(0);
one = builder.makeIntConstant(1);
}
convOp = spv::OpSelect;
break;
case glslang::EOpConvBoolToUint:
case glslang::EOpConvBoolToUint64:
if (op == glslang::EOpConvBoolToUint64) {
zero = builder.makeUint64Constant(0);
one = builder.makeUint64Constant(1);
} else {
zero = builder.makeUintConstant(0);
one = builder.makeUintConstant(1);
}
convOp = spv::OpSelect;
break;
case glslang::EOpConvInt8ToFloat16:
case glslang::EOpConvInt8ToFloat:
case glslang::EOpConvInt8ToDouble:
case glslang::EOpConvInt16ToFloat16:
case glslang::EOpConvInt16ToFloat:
case glslang::EOpConvInt16ToDouble:
case glslang::EOpConvIntToFloat16:
case glslang::EOpConvIntToFloat:
case glslang::EOpConvIntToDouble:
case glslang::EOpConvInt64ToFloat:
case glslang::EOpConvInt64ToDouble:
case glslang::EOpConvInt64ToFloat16:
convOp = spv::OpConvertSToF;
break;
case glslang::EOpConvUint8ToFloat16:
case glslang::EOpConvUint8ToFloat:
case glslang::EOpConvUint8ToDouble:
case glslang::EOpConvUint16ToFloat16:
case glslang::EOpConvUint16ToFloat:
case glslang::EOpConvUint16ToDouble:
case glslang::EOpConvUintToFloat16:
case glslang::EOpConvUintToFloat:
case glslang::EOpConvUintToDouble:
case glslang::EOpConvUint64ToFloat:
case glslang::EOpConvUint64ToDouble:
case glslang::EOpConvUint64ToFloat16:
convOp = spv::OpConvertUToF;
break;
case glslang::EOpConvFloat16ToInt8:
case glslang::EOpConvFloatToInt8:
case glslang::EOpConvDoubleToInt8:
case glslang::EOpConvFloat16ToInt16:
case glslang::EOpConvFloatToInt16:
case glslang::EOpConvDoubleToInt16:
case glslang::EOpConvFloat16ToInt:
case glslang::EOpConvFloatToInt:
case glslang::EOpConvDoubleToInt:
case glslang::EOpConvFloat16ToInt64:
case glslang::EOpConvFloatToInt64:
case glslang::EOpConvDoubleToInt64:
convOp = spv::OpConvertFToS;
break;
case glslang::EOpConvUint8ToInt8:
case glslang::EOpConvInt8ToUint8:
case glslang::EOpConvUint16ToInt16:
case glslang::EOpConvInt16ToUint16:
case glslang::EOpConvUintToInt:
case glslang::EOpConvIntToUint:
case glslang::EOpConvUint64ToInt64:
case glslang::EOpConvInt64ToUint64:
if (builder.isInSpecConstCodeGenMode()) {
// Build zero scalar or vector for OpIAdd.
if(op == glslang::EOpConvUint8ToInt8 || op == glslang::EOpConvInt8ToUint8) {
zero = builder.makeUint8Constant(0);
} else if (op == glslang::EOpConvUint16ToInt16 || op == glslang::EOpConvInt16ToUint16) {
zero = builder.makeUint16Constant(0);
} else if (op == glslang::EOpConvUint64ToInt64 || op == glslang::EOpConvInt64ToUint64) {
zero = builder.makeUint64Constant(0);
} else {
zero = builder.makeUintConstant(0);
}
zero = makeSmearedConstant(zero, vectorSize);
// Use OpIAdd, instead of OpBitcast to do the conversion when
// generating for OpSpecConstantOp instruction.
return builder.createBinOp(spv::OpIAdd, destType, operand, zero);
}
// For normal run-time conversion instruction, use OpBitcast.
convOp = spv::OpBitcast;
break;
case glslang::EOpConvFloat16ToUint8:
case glslang::EOpConvFloatToUint8:
case glslang::EOpConvDoubleToUint8:
case glslang::EOpConvFloat16ToUint16:
case glslang::EOpConvFloatToUint16:
case glslang::EOpConvDoubleToUint16:
case glslang::EOpConvFloat16ToUint:
case glslang::EOpConvFloatToUint:
case glslang::EOpConvDoubleToUint:
case glslang::EOpConvFloatToUint64:
case glslang::EOpConvDoubleToUint64:
case glslang::EOpConvFloat16ToUint64:
convOp = spv::OpConvertFToU;
break;
case glslang::EOpConvInt8ToBool:
case glslang::EOpConvUint8ToBool:
zero = builder.makeUint8Constant(0);
zero = makeSmearedConstant(zero, vectorSize);
return builder.createBinOp(spv::OpINotEqual, destType, operand, zero);
case glslang::EOpConvInt16ToBool:
case glslang::EOpConvUint16ToBool:
zero = builder.makeUint16Constant(0);
zero = makeSmearedConstant(zero, vectorSize);
return builder.createBinOp(spv::OpINotEqual, destType, operand, zero);
case glslang::EOpConvInt64ToBool:
case glslang::EOpConvUint64ToBool:
zero = builder.makeUint64Constant(0);
zero = makeSmearedConstant(zero, vectorSize);
return builder.createBinOp(spv::OpINotEqual, destType, operand, zero);
case glslang::EOpConvDoubleToBool:
zero = builder.makeDoubleConstant(0.0);
zero = makeSmearedConstant(zero, vectorSize);
return builder.createBinOp(spv::OpFUnordNotEqual, destType, operand, zero);
case glslang::EOpConvFloat16ToBool:
zero = builder.makeFloat16Constant(0.0F);
zero = makeSmearedConstant(zero, vectorSize);
return builder.createBinOp(spv::OpFUnordNotEqual, destType, operand, zero);
case glslang::EOpConvBoolToDouble:
convOp = spv::OpSelect;
zero = builder.makeDoubleConstant(0.0);
one = builder.makeDoubleConstant(1.0);
break;
case glslang::EOpConvBoolToFloat16:
convOp = spv::OpSelect;
zero = builder.makeFloat16Constant(0.0F);
one = builder.makeFloat16Constant(1.0F);
break;
case glslang::EOpConvBoolToInt8:
zero = builder.makeInt8Constant(0);
one = builder.makeInt8Constant(1);
convOp = spv::OpSelect;
break;
case glslang::EOpConvBoolToUint8:
zero = builder.makeUint8Constant(0);
one = builder.makeUint8Constant(1);
convOp = spv::OpSelect;
break;
case glslang::EOpConvBoolToInt16:
zero = builder.makeInt16Constant(0);
one = builder.makeInt16Constant(1);
convOp = spv::OpSelect;
break;
case glslang::EOpConvBoolToUint16:
zero = builder.makeUint16Constant(0);
one = builder.makeUint16Constant(1);
convOp = spv::OpSelect;
break;
case glslang::EOpConvDoubleToFloat:
case glslang::EOpConvFloatToDouble:
case glslang::EOpConvDoubleToFloat16:
case glslang::EOpConvFloat16ToDouble:
case glslang::EOpConvFloatToFloat16:
case glslang::EOpConvFloat16ToFloat:
convOp = spv::OpFConvert;
if (builder.isMatrixType(destType))
return createUnaryMatrixOperation(convOp, decorations, destType, operand, typeProxy);
break;
case glslang::EOpConvInt8ToInt16:
case glslang::EOpConvInt8ToInt:
case glslang::EOpConvInt8ToInt64:
case glslang::EOpConvInt16ToInt8:
case glslang::EOpConvInt16ToInt:
case glslang::EOpConvInt16ToInt64:
case glslang::EOpConvIntToInt8:
case glslang::EOpConvIntToInt16:
case glslang::EOpConvIntToInt64:
case glslang::EOpConvInt64ToInt8:
case glslang::EOpConvInt64ToInt16:
case glslang::EOpConvInt64ToInt:
convOp = spv::OpSConvert;
break;
case glslang::EOpConvUint8ToUint16:
case glslang::EOpConvUint8ToUint:
case glslang::EOpConvUint8ToUint64:
case glslang::EOpConvUint16ToUint8:
case glslang::EOpConvUint16ToUint:
case glslang::EOpConvUint16ToUint64:
case glslang::EOpConvUintToUint8:
case glslang::EOpConvUintToUint16:
case glslang::EOpConvUintToUint64:
case glslang::EOpConvUint64ToUint8:
case glslang::EOpConvUint64ToUint16:
case glslang::EOpConvUint64ToUint:
convOp = spv::OpUConvert;
break;
case glslang::EOpConvInt8ToUint16:
case glslang::EOpConvInt8ToUint:
case glslang::EOpConvInt8ToUint64:
case glslang::EOpConvInt16ToUint8:
case glslang::EOpConvInt16ToUint:
case glslang::EOpConvInt16ToUint64:
case glslang::EOpConvIntToUint8:
case glslang::EOpConvIntToUint16:
case glslang::EOpConvIntToUint64:
case glslang::EOpConvInt64ToUint8:
case glslang::EOpConvInt64ToUint16:
case glslang::EOpConvInt64ToUint:
case glslang::EOpConvUint8ToInt16:
case glslang::EOpConvUint8ToInt:
case glslang::EOpConvUint8ToInt64:
case glslang::EOpConvUint16ToInt8:
case glslang::EOpConvUint16ToInt:
case glslang::EOpConvUint16ToInt64:
case glslang::EOpConvUintToInt8:
case glslang::EOpConvUintToInt16:
case glslang::EOpConvUintToInt64:
case glslang::EOpConvUint64ToInt8:
case glslang::EOpConvUint64ToInt16:
case glslang::EOpConvUint64ToInt:
// OpSConvert/OpUConvert + OpBitCast
operand = createIntWidthConversion(op, operand, vectorSize, destType);
if (builder.isInSpecConstCodeGenMode()) {
// Build zero scalar or vector for OpIAdd.
switch(op) {
case glslang::EOpConvInt16ToUint8:
case glslang::EOpConvIntToUint8:
case glslang::EOpConvInt64ToUint8:
case glslang::EOpConvUint16ToInt8:
case glslang::EOpConvUintToInt8:
case glslang::EOpConvUint64ToInt8:
zero = builder.makeUint8Constant(0);
break;
case glslang::EOpConvInt8ToUint16:
case glslang::EOpConvIntToUint16:
case glslang::EOpConvInt64ToUint16:
case glslang::EOpConvUint8ToInt16:
case glslang::EOpConvUintToInt16:
case glslang::EOpConvUint64ToInt16:
zero = builder.makeUint16Constant(0);
break;
case glslang::EOpConvInt8ToUint:
case glslang::EOpConvInt16ToUint:
case glslang::EOpConvInt64ToUint:
case glslang::EOpConvUint8ToInt:
case glslang::EOpConvUint16ToInt:
case glslang::EOpConvUint64ToInt:
zero = builder.makeUintConstant(0);
break;
case glslang::EOpConvInt8ToUint64:
case glslang::EOpConvInt16ToUint64:
case glslang::EOpConvIntToUint64:
case glslang::EOpConvUint8ToInt64:
case glslang::EOpConvUint16ToInt64:
case glslang::EOpConvUintToInt64:
zero = builder.makeUint64Constant(0);
break;
default:
assert(false && "Default missing");
break;
}
zero = makeSmearedConstant(zero, vectorSize);
// Use OpIAdd, instead of OpBitcast to do the conversion when
// generating for OpSpecConstantOp instruction.
return builder.createBinOp(spv::OpIAdd, destType, operand, zero);
}
// For normal run-time conversion instruction, use OpBitcast.
convOp = spv::OpBitcast;
break;
case glslang::EOpConvUint64ToPtr:
convOp = spv::OpConvertUToPtr;
break;
case glslang::EOpConvPtrToUint64:
convOp = spv::OpConvertPtrToU;
break;
case glslang::EOpConvPtrToUvec2:
case glslang::EOpConvUvec2ToPtr:
convOp = spv::OpBitcast;
break;
default:
break;
}
spv::Id result = 0;
if (convOp == spv::OpNop)
return result;
if (convOp == spv::OpSelect) {
zero = makeSmearedConstant(zero, vectorSize);
one = makeSmearedConstant(one, vectorSize);
result = builder.createTriOp(convOp, destType, operand, one, zero);
} else
result = builder.createUnaryOp(convOp, destType, operand);
result = builder.setPrecision(result, decorations.precision);
decorations.addNonUniform(builder, result);
return result;
}
spv::Id TGlslangToSpvTraverser::makeSmearedConstant(spv::Id constant, int vectorSize)
{
if (vectorSize == 0)
return constant;
spv::Id vectorTypeId = builder.makeVectorType(builder.getTypeId(constant), vectorSize);
std::vector<spv::Id> components;
for (int c = 0; c < vectorSize; ++c)
components.push_back(constant);
return builder.makeCompositeConstant(vectorTypeId, components);
}
// For glslang ops that map to SPV atomic opCodes
spv::Id TGlslangToSpvTraverser::createAtomicOperation(glslang::TOperator op, spv::Decoration /*precision*/,
spv::Id typeId, std::vector<spv::Id>& operands, glslang::TBasicType typeProxy,
const spv::Builder::AccessChain::CoherentFlags &lvalueCoherentFlags, const glslang::TType &opType)
{
spv::Op opCode = spv::OpNop;
switch (op) {
case glslang::EOpAtomicAdd:
case glslang::EOpImageAtomicAdd:
case glslang::EOpAtomicCounterAdd:
opCode = spv::OpAtomicIAdd;
if (typeProxy == glslang::EbtFloat16 || typeProxy == glslang::EbtFloat || typeProxy == glslang::EbtDouble) {
opCode = spv::OpAtomicFAddEXT;
if (typeProxy == glslang::EbtFloat16 &&
(opType.getVectorSize() == 2 || opType.getVectorSize() == 4)) {
builder.addExtension(spv::E_SPV_NV_shader_atomic_fp16_vector);
builder.addCapability(spv::CapabilityAtomicFloat16VectorNV);
} else {
builder.addExtension(spv::E_SPV_EXT_shader_atomic_float_add);
if (typeProxy == glslang::EbtFloat16) {
builder.addExtension(spv::E_SPV_EXT_shader_atomic_float16_add);
builder.addCapability(spv::CapabilityAtomicFloat16AddEXT);
} else if (typeProxy == glslang::EbtFloat) {
builder.addCapability(spv::CapabilityAtomicFloat32AddEXT);
} else {
builder.addCapability(spv::CapabilityAtomicFloat64AddEXT);
}
}
}
break;
case glslang::EOpAtomicSubtract:
case glslang::EOpAtomicCounterSubtract:
opCode = spv::OpAtomicISub;
break;
case glslang::EOpAtomicMin:
case glslang::EOpImageAtomicMin:
case glslang::EOpAtomicCounterMin:
if (typeProxy == glslang::EbtFloat16 || typeProxy == glslang::EbtFloat || typeProxy == glslang::EbtDouble) {
opCode = spv::OpAtomicFMinEXT;
if (typeProxy == glslang::EbtFloat16 &&
(opType.getVectorSize() == 2 || opType.getVectorSize() == 4)) {
builder.addExtension(spv::E_SPV_NV_shader_atomic_fp16_vector);
builder.addCapability(spv::CapabilityAtomicFloat16VectorNV);
} else {
builder.addExtension(spv::E_SPV_EXT_shader_atomic_float_min_max);
if (typeProxy == glslang::EbtFloat16)
builder.addCapability(spv::CapabilityAtomicFloat16MinMaxEXT);
else if (typeProxy == glslang::EbtFloat)
builder.addCapability(spv::CapabilityAtomicFloat32MinMaxEXT);
else
builder.addCapability(spv::CapabilityAtomicFloat64MinMaxEXT);
}
} else if (typeProxy == glslang::EbtUint || typeProxy == glslang::EbtUint64) {
opCode = spv::OpAtomicUMin;
} else {
opCode = spv::OpAtomicSMin;
}
break;
case glslang::EOpAtomicMax:
case glslang::EOpImageAtomicMax:
case glslang::EOpAtomicCounterMax:
if (typeProxy == glslang::EbtFloat16 || typeProxy == glslang::EbtFloat || typeProxy == glslang::EbtDouble) {
opCode = spv::OpAtomicFMaxEXT;
if (typeProxy == glslang::EbtFloat16 &&
(opType.getVectorSize() == 2 || opType.getVectorSize() == 4)) {
builder.addExtension(spv::E_SPV_NV_shader_atomic_fp16_vector);
builder.addCapability(spv::CapabilityAtomicFloat16VectorNV);
} else {
builder.addExtension(spv::E_SPV_EXT_shader_atomic_float_min_max);
if (typeProxy == glslang::EbtFloat16)
builder.addCapability(spv::CapabilityAtomicFloat16MinMaxEXT);
else if (typeProxy == glslang::EbtFloat)
builder.addCapability(spv::CapabilityAtomicFloat32MinMaxEXT);
else
builder.addCapability(spv::CapabilityAtomicFloat64MinMaxEXT);
}
} else if (typeProxy == glslang::EbtUint || typeProxy == glslang::EbtUint64) {
opCode = spv::OpAtomicUMax;
} else {
opCode = spv::OpAtomicSMax;
}
break;
case glslang::EOpAtomicAnd:
case glslang::EOpImageAtomicAnd:
case glslang::EOpAtomicCounterAnd:
opCode = spv::OpAtomicAnd;
break;
case glslang::EOpAtomicOr:
case glslang::EOpImageAtomicOr:
case glslang::EOpAtomicCounterOr:
opCode = spv::OpAtomicOr;
break;
case glslang::EOpAtomicXor:
case glslang::EOpImageAtomicXor:
case glslang::EOpAtomicCounterXor:
opCode = spv::OpAtomicXor;
break;
case glslang::EOpAtomicExchange:
case glslang::EOpImageAtomicExchange:
case glslang::EOpAtomicCounterExchange:
if ((typeProxy == glslang::EbtFloat16) &&
(opType.getVectorSize() == 2 || opType.getVectorSize() == 4)) {
builder.addExtension(spv::E_SPV_NV_shader_atomic_fp16_vector);
builder.addCapability(spv::CapabilityAtomicFloat16VectorNV);
}
opCode = spv::OpAtomicExchange;
break;
case glslang::EOpAtomicCompSwap:
case glslang::EOpImageAtomicCompSwap:
case glslang::EOpAtomicCounterCompSwap:
opCode = spv::OpAtomicCompareExchange;
break;
case glslang::EOpAtomicCounterIncrement:
opCode = spv::OpAtomicIIncrement;
break;
case glslang::EOpAtomicCounterDecrement:
opCode = spv::OpAtomicIDecrement;
break;
case glslang::EOpAtomicCounter:
case glslang::EOpImageAtomicLoad:
case glslang::EOpAtomicLoad:
opCode = spv::OpAtomicLoad;
break;
case glslang::EOpAtomicStore:
case glslang::EOpImageAtomicStore:
opCode = spv::OpAtomicStore;
break;
default:
assert(0);
break;
}
if (typeProxy == glslang::EbtInt64 || typeProxy == glslang::EbtUint64)
builder.addCapability(spv::CapabilityInt64Atomics);
// Sort out the operands
// - mapping from glslang -> SPV
// - there are extra SPV operands that are optional in glslang
// - compare-exchange swaps the value and comparator
// - compare-exchange has an extra memory semantics
// - EOpAtomicCounterDecrement needs a post decrement
spv::Id pointerId = 0, compareId = 0, valueId = 0;
// scope defaults to Device in the old model, QueueFamilyKHR in the new model
spv::Id scopeId;
if (glslangIntermediate->usingVulkanMemoryModel()) {
scopeId = builder.makeUintConstant(spv::ScopeQueueFamilyKHR);
} else {
scopeId = builder.makeUintConstant(spv::ScopeDevice);
}
// semantics default to relaxed
spv::Id semanticsId = builder.makeUintConstant(lvalueCoherentFlags.isVolatile() &&
glslangIntermediate->usingVulkanMemoryModel() ?
spv::MemorySemanticsVolatileMask :
spv::MemorySemanticsMaskNone);
spv::Id semanticsId2 = semanticsId;
pointerId = operands[0];
if (opCode == spv::OpAtomicIIncrement || opCode == spv::OpAtomicIDecrement) {
// no additional operands
} else if (opCode == spv::OpAtomicCompareExchange) {
compareId = operands[1];
valueId = operands[2];
if (operands.size() > 3) {
scopeId = operands[3];
semanticsId = builder.makeUintConstant(
builder.getConstantScalar(operands[4]) | builder.getConstantScalar(operands[5]));
semanticsId2 = builder.makeUintConstant(
builder.getConstantScalar(operands[6]) | builder.getConstantScalar(operands[7]));
}
} else if (opCode == spv::OpAtomicLoad) {
if (operands.size() > 1) {
scopeId = operands[1];
semanticsId = builder.makeUintConstant(
builder.getConstantScalar(operands[2]) | builder.getConstantScalar(operands[3]));
}
} else {
// atomic store or RMW
valueId = operands[1];
if (operands.size() > 2) {
scopeId = operands[2];
semanticsId = builder.makeUintConstant
(builder.getConstantScalar(operands[3]) | builder.getConstantScalar(operands[4]));
}
}
// Check for capabilities
unsigned semanticsImmediate = builder.getConstantScalar(semanticsId) | builder.getConstantScalar(semanticsId2);
if (semanticsImmediate & (spv::MemorySemanticsMakeAvailableKHRMask |
spv::MemorySemanticsMakeVisibleKHRMask |
spv::MemorySemanticsOutputMemoryKHRMask |
spv::MemorySemanticsVolatileMask)) {
builder.addCapability(spv::CapabilityVulkanMemoryModelKHR);
}
if (builder.getConstantScalar(scopeId) == spv::ScopeQueueFamily) {
builder.addCapability(spv::CapabilityVulkanMemoryModelKHR);
}
if (glslangIntermediate->usingVulkanMemoryModel() && builder.getConstantScalar(scopeId) == spv::ScopeDevice) {
builder.addCapability(spv::CapabilityVulkanMemoryModelDeviceScopeKHR);
}
std::vector<spv::Id> spvAtomicOperands; // hold the spv operands
spvAtomicOperands.reserve(6);
spvAtomicOperands.push_back(pointerId);
spvAtomicOperands.push_back(scopeId);
spvAtomicOperands.push_back(semanticsId);
if (opCode == spv::OpAtomicCompareExchange) {
spvAtomicOperands.push_back(semanticsId2);
spvAtomicOperands.push_back(valueId);
spvAtomicOperands.push_back(compareId);
} else if (opCode != spv::OpAtomicLoad && opCode != spv::OpAtomicIIncrement && opCode != spv::OpAtomicIDecrement) {
spvAtomicOperands.push_back(valueId);
}
if (opCode == spv::OpAtomicStore) {
builder.createNoResultOp(opCode, spvAtomicOperands);
return 0;
} else {
spv::Id resultId = builder.createOp(opCode, typeId, spvAtomicOperands);
// GLSL and HLSL atomic-counter decrement return post-decrement value,
// while SPIR-V returns pre-decrement value. Translate between these semantics.
if (op == glslang::EOpAtomicCounterDecrement)
resultId = builder.createBinOp(spv::OpISub, typeId, resultId, builder.makeIntConstant(1));
return resultId;
}
}
// Create group invocation operations.
spv::Id TGlslangToSpvTraverser::createInvocationsOperation(glslang::TOperator op, spv::Id typeId,
std::vector<spv::Id>& operands, glslang::TBasicType typeProxy)
{
bool isUnsigned = isTypeUnsignedInt(typeProxy);
bool isFloat = isTypeFloat(typeProxy);
spv::Op opCode = spv::OpNop;
std::vector<spv::IdImmediate> spvGroupOperands;
spv::GroupOperation groupOperation = spv::GroupOperationMax;
if (op == glslang::EOpBallot || op == glslang::EOpReadFirstInvocation ||
op == glslang::EOpReadInvocation) {
builder.addExtension(spv::E_SPV_KHR_shader_ballot);
builder.addCapability(spv::CapabilitySubgroupBallotKHR);
} else if (op == glslang::EOpAnyInvocation ||
op == glslang::EOpAllInvocations ||
op == glslang::EOpAllInvocationsEqual) {
builder.addExtension(spv::E_SPV_KHR_subgroup_vote);
builder.addCapability(spv::CapabilitySubgroupVoteKHR);
} else {
builder.addCapability(spv::CapabilityGroups);
if (op == glslang::EOpMinInvocationsNonUniform ||
op == glslang::EOpMaxInvocationsNonUniform ||
op == glslang::EOpAddInvocationsNonUniform ||
op == glslang::EOpMinInvocationsInclusiveScanNonUniform ||
op == glslang::EOpMaxInvocationsInclusiveScanNonUniform ||
op == glslang::EOpAddInvocationsInclusiveScanNonUniform ||
op == glslang::EOpMinInvocationsExclusiveScanNonUniform ||
op == glslang::EOpMaxInvocationsExclusiveScanNonUniform ||
op == glslang::EOpAddInvocationsExclusiveScanNonUniform)
builder.addExtension(spv::E_SPV_AMD_shader_ballot);
switch (op) {
case glslang::EOpMinInvocations:
case glslang::EOpMaxInvocations:
case glslang::EOpAddInvocations:
case glslang::EOpMinInvocationsNonUniform:
case glslang::EOpMaxInvocationsNonUniform:
case glslang::EOpAddInvocationsNonUniform:
groupOperation = spv::GroupOperationReduce;
break;
case glslang::EOpMinInvocationsInclusiveScan:
case glslang::EOpMaxInvocationsInclusiveScan:
case glslang::EOpAddInvocationsInclusiveScan:
case glslang::EOpMinInvocationsInclusiveScanNonUniform:
case glslang::EOpMaxInvocationsInclusiveScanNonUniform:
case glslang::EOpAddInvocationsInclusiveScanNonUniform:
groupOperation = spv::GroupOperationInclusiveScan;
break;
case glslang::EOpMinInvocationsExclusiveScan:
case glslang::EOpMaxInvocationsExclusiveScan:
case glslang::EOpAddInvocationsExclusiveScan:
case glslang::EOpMinInvocationsExclusiveScanNonUniform:
case glslang::EOpMaxInvocationsExclusiveScanNonUniform:
case glslang::EOpAddInvocationsExclusiveScanNonUniform:
groupOperation = spv::GroupOperationExclusiveScan;
break;
default:
break;
}
spv::IdImmediate scope = { true, builder.makeUintConstant(spv::ScopeSubgroup) };
spvGroupOperands.push_back(scope);
if (groupOperation != spv::GroupOperationMax) {
spv::IdImmediate groupOp = { false, (unsigned)groupOperation };
spvGroupOperands.push_back(groupOp);
}
}
for (auto opIt = operands.begin(); opIt != operands.end(); ++opIt) {
spv::IdImmediate op = { true, *opIt };
spvGroupOperands.push_back(op);
}
switch (op) {
case glslang::EOpAnyInvocation:
opCode = spv::OpSubgroupAnyKHR;
break;
case glslang::EOpAllInvocations:
opCode = spv::OpSubgroupAllKHR;
break;
case glslang::EOpAllInvocationsEqual:
opCode = spv::OpSubgroupAllEqualKHR;
break;
case glslang::EOpReadInvocation:
opCode = spv::OpSubgroupReadInvocationKHR;
if (builder.isVectorType(typeId))
return CreateInvocationsVectorOperation(opCode, groupOperation, typeId, operands);
break;
case glslang::EOpReadFirstInvocation:
opCode = spv::OpSubgroupFirstInvocationKHR;
if (builder.isVectorType(typeId))
return CreateInvocationsVectorOperation(opCode, groupOperation, typeId, operands);
break;
case glslang::EOpBallot:
{
// NOTE: According to the spec, the result type of "OpSubgroupBallotKHR" must be a 4 component vector of 32
// bit integer types. The GLSL built-in function "ballotARB()" assumes the maximum number of invocations in
// a subgroup is 64. Thus, we have to convert uvec4.xy to uint64_t as follow:
//
// result = Bitcast(SubgroupBallotKHR(Predicate).xy)
//
spv::Id uintType = builder.makeUintType(32);
spv::Id uvec4Type = builder.makeVectorType(uintType, 4);
spv::Id result = builder.createOp(spv::OpSubgroupBallotKHR, uvec4Type, spvGroupOperands);
std::vector<spv::Id> components;
components.push_back(builder.createCompositeExtract(result, uintType, 0));
components.push_back(builder.createCompositeExtract(result, uintType, 1));
spv::Id uvec2Type = builder.makeVectorType(uintType, 2);
return builder.createUnaryOp(spv::OpBitcast, typeId,
builder.createCompositeConstruct(uvec2Type, components));
}
case glslang::EOpMinInvocations:
case glslang::EOpMaxInvocations:
case glslang::EOpAddInvocations:
case glslang::EOpMinInvocationsInclusiveScan:
case glslang::EOpMaxInvocationsInclusiveScan:
case glslang::EOpAddInvocationsInclusiveScan:
case glslang::EOpMinInvocationsExclusiveScan:
case glslang::EOpMaxInvocationsExclusiveScan:
case glslang::EOpAddInvocationsExclusiveScan:
if (op == glslang::EOpMinInvocations ||
op == glslang::EOpMinInvocationsInclusiveScan ||
op == glslang::EOpMinInvocationsExclusiveScan) {
if (isFloat)
opCode = spv::OpGroupFMin;
else {
if (isUnsigned)
opCode = spv::OpGroupUMin;
else
opCode = spv::OpGroupSMin;
}
} else if (op == glslang::EOpMaxInvocations ||
op == glslang::EOpMaxInvocationsInclusiveScan ||
op == glslang::EOpMaxInvocationsExclusiveScan) {
if (isFloat)
opCode = spv::OpGroupFMax;
else {
if (isUnsigned)
opCode = spv::OpGroupUMax;
else
opCode = spv::OpGroupSMax;
}
} else {
if (isFloat)
opCode = spv::OpGroupFAdd;
else
opCode = spv::OpGroupIAdd;
}
if (builder.isVectorType(typeId))
return CreateInvocationsVectorOperation(opCode, groupOperation, typeId, operands);
break;
case glslang::EOpMinInvocationsNonUniform:
case glslang::EOpMaxInvocationsNonUniform:
case glslang::EOpAddInvocationsNonUniform:
case glslang::EOpMinInvocationsInclusiveScanNonUniform:
case glslang::EOpMaxInvocationsInclusiveScanNonUniform:
case glslang::EOpAddInvocationsInclusiveScanNonUniform:
case glslang::EOpMinInvocationsExclusiveScanNonUniform:
case glslang::EOpMaxInvocationsExclusiveScanNonUniform:
case glslang::EOpAddInvocationsExclusiveScanNonUniform:
if (op == glslang::EOpMinInvocationsNonUniform ||
op == glslang::EOpMinInvocationsInclusiveScanNonUniform ||
op == glslang::EOpMinInvocationsExclusiveScanNonUniform) {
if (isFloat)
opCode = spv::OpGroupFMinNonUniformAMD;
else {
if (isUnsigned)
opCode = spv::OpGroupUMinNonUniformAMD;
else
opCode = spv::OpGroupSMinNonUniformAMD;
}
}
else if (op == glslang::EOpMaxInvocationsNonUniform ||
op == glslang::EOpMaxInvocationsInclusiveScanNonUniform ||
op == glslang::EOpMaxInvocationsExclusiveScanNonUniform) {
if (isFloat)
opCode = spv::OpGroupFMaxNonUniformAMD;
else {
if (isUnsigned)
opCode = spv::OpGroupUMaxNonUniformAMD;
else
opCode = spv::OpGroupSMaxNonUniformAMD;
}
}
else {
if (isFloat)
opCode = spv::OpGroupFAddNonUniformAMD;
else
opCode = spv::OpGroupIAddNonUniformAMD;
}
if (builder.isVectorType(typeId))
return CreateInvocationsVectorOperation(opCode, groupOperation, typeId, operands);
break;
default:
logger->missingFunctionality("invocation operation");
return spv::NoResult;
}
assert(opCode != spv::OpNop);
return builder.createOp(opCode, typeId, spvGroupOperands);
}
// Create group invocation operations on a vector
spv::Id TGlslangToSpvTraverser::CreateInvocationsVectorOperation(spv::Op op, spv::GroupOperation groupOperation,
spv::Id typeId, std::vector<spv::Id>& operands)
{
assert(op == spv::OpGroupFMin || op == spv::OpGroupUMin || op == spv::OpGroupSMin ||
op == spv::OpGroupFMax || op == spv::OpGroupUMax || op == spv::OpGroupSMax ||
op == spv::OpGroupFAdd || op == spv::OpGroupIAdd || op == spv::OpGroupBroadcast ||
op == spv::OpSubgroupReadInvocationKHR || op == spv::OpSubgroupFirstInvocationKHR ||
op == spv::OpGroupFMinNonUniformAMD || op == spv::OpGroupUMinNonUniformAMD ||
op == spv::OpGroupSMinNonUniformAMD ||
op == spv::OpGroupFMaxNonUniformAMD || op == spv::OpGroupUMaxNonUniformAMD ||
op == spv::OpGroupSMaxNonUniformAMD ||
op == spv::OpGroupFAddNonUniformAMD || op == spv::OpGroupIAddNonUniformAMD);
// Handle group invocation operations scalar by scalar.
// The result type is the same type as the original type.
// The algorithm is to:
// - break the vector into scalars
// - apply the operation to each scalar
// - make a vector out the scalar results
// get the types sorted out
int numComponents = builder.getNumComponents(operands[0]);
spv::Id scalarType = builder.getScalarTypeId(builder.getTypeId(operands[0]));
std::vector<spv::Id> results;
// do each scalar op
for (int comp = 0; comp < numComponents; ++comp) {
std::vector<unsigned int> indexes;
indexes.push_back(comp);
spv::IdImmediate scalar = { true, builder.createCompositeExtract(operands[0], scalarType, indexes) };
std::vector<spv::IdImmediate> spvGroupOperands;
if (op == spv::OpSubgroupReadInvocationKHR) {
spvGroupOperands.push_back(scalar);
spv::IdImmediate operand = { true, operands[1] };
spvGroupOperands.push_back(operand);
} else if (op == spv::OpSubgroupFirstInvocationKHR) {
spvGroupOperands.push_back(scalar);
} else if (op == spv::OpGroupBroadcast) {
spv::IdImmediate scope = { true, builder.makeUintConstant(spv::ScopeSubgroup) };
spvGroupOperands.push_back(scope);
spvGroupOperands.push_back(scalar);
spv::IdImmediate operand = { true, operands[1] };
spvGroupOperands.push_back(operand);
} else {
spv::IdImmediate scope = { true, builder.makeUintConstant(spv::ScopeSubgroup) };
spvGroupOperands.push_back(scope);
spv::IdImmediate groupOp = { false, (unsigned)groupOperation };
spvGroupOperands.push_back(groupOp);
spvGroupOperands.push_back(scalar);
}
results.push_back(builder.createOp(op, scalarType, spvGroupOperands));
}
// put the pieces together
return builder.createCompositeConstruct(typeId, results);
}
// Create subgroup invocation operations.
spv::Id TGlslangToSpvTraverser::createSubgroupOperation(glslang::TOperator op, spv::Id typeId,
std::vector<spv::Id>& operands, glslang::TBasicType typeProxy)
{
// Add the required capabilities.
switch (op) {
case glslang::EOpSubgroupElect:
builder.addCapability(spv::CapabilityGroupNonUniform);
break;
case glslang::EOpSubgroupQuadAll:
case glslang::EOpSubgroupQuadAny:
builder.addExtension(spv::E_SPV_KHR_quad_control);
builder.addCapability(spv::CapabilityQuadControlKHR);
[[fallthrough]];
case glslang::EOpSubgroupAll:
case glslang::EOpSubgroupAny:
case glslang::EOpSubgroupAllEqual:
builder.addCapability(spv::CapabilityGroupNonUniform);
builder.addCapability(spv::CapabilityGroupNonUniformVote);
break;
case glslang::EOpSubgroupBroadcast:
case glslang::EOpSubgroupBroadcastFirst:
case glslang::EOpSubgroupBallot:
case glslang::EOpSubgroupInverseBallot:
case glslang::EOpSubgroupBallotBitExtract:
case glslang::EOpSubgroupBallotBitCount:
case glslang::EOpSubgroupBallotInclusiveBitCount:
case glslang::EOpSubgroupBallotExclusiveBitCount:
case glslang::EOpSubgroupBallotFindLSB:
case glslang::EOpSubgroupBallotFindMSB:
builder.addCapability(spv::CapabilityGroupNonUniform);
builder.addCapability(spv::CapabilityGroupNonUniformBallot);
break;
case glslang::EOpSubgroupRotate:
case glslang::EOpSubgroupClusteredRotate:
builder.addExtension(spv::E_SPV_KHR_subgroup_rotate);
builder.addCapability(spv::CapabilityGroupNonUniformRotateKHR);
break;
case glslang::EOpSubgroupShuffle:
case glslang::EOpSubgroupShuffleXor:
builder.addCapability(spv::CapabilityGroupNonUniform);
builder.addCapability(spv::CapabilityGroupNonUniformShuffle);
break;
case glslang::EOpSubgroupShuffleUp:
case glslang::EOpSubgroupShuffleDown:
builder.addCapability(spv::CapabilityGroupNonUniform);
builder.addCapability(spv::CapabilityGroupNonUniformShuffleRelative);
break;
case glslang::EOpSubgroupAdd:
case glslang::EOpSubgroupMul:
case glslang::EOpSubgroupMin:
case glslang::EOpSubgroupMax:
case glslang::EOpSubgroupAnd:
case glslang::EOpSubgroupOr:
case glslang::EOpSubgroupXor:
case glslang::EOpSubgroupInclusiveAdd:
case glslang::EOpSubgroupInclusiveMul:
case glslang::EOpSubgroupInclusiveMin:
case glslang::EOpSubgroupInclusiveMax:
case glslang::EOpSubgroupInclusiveAnd:
case glslang::EOpSubgroupInclusiveOr:
case glslang::EOpSubgroupInclusiveXor:
case glslang::EOpSubgroupExclusiveAdd:
case glslang::EOpSubgroupExclusiveMul:
case glslang::EOpSubgroupExclusiveMin:
case glslang::EOpSubgroupExclusiveMax:
case glslang::EOpSubgroupExclusiveAnd:
case glslang::EOpSubgroupExclusiveOr:
case glslang::EOpSubgroupExclusiveXor:
builder.addCapability(spv::CapabilityGroupNonUniform);
builder.addCapability(spv::CapabilityGroupNonUniformArithmetic);
break;
case glslang::EOpSubgroupClusteredAdd:
case glslang::EOpSubgroupClusteredMul:
case glslang::EOpSubgroupClusteredMin:
case glslang::EOpSubgroupClusteredMax:
case glslang::EOpSubgroupClusteredAnd:
case glslang::EOpSubgroupClusteredOr:
case glslang::EOpSubgroupClusteredXor:
builder.addCapability(spv::CapabilityGroupNonUniform);
builder.addCapability(spv::CapabilityGroupNonUniformClustered);
break;
case glslang::EOpSubgroupQuadBroadcast:
case glslang::EOpSubgroupQuadSwapHorizontal:
case glslang::EOpSubgroupQuadSwapVertical:
case glslang::EOpSubgroupQuadSwapDiagonal:
builder.addCapability(spv::CapabilityGroupNonUniform);
builder.addCapability(spv::CapabilityGroupNonUniformQuad);
break;
case glslang::EOpSubgroupPartitionedAdd:
case glslang::EOpSubgroupPartitionedMul:
case glslang::EOpSubgroupPartitionedMin:
case glslang::EOpSubgroupPartitionedMax:
case glslang::EOpSubgroupPartitionedAnd:
case glslang::EOpSubgroupPartitionedOr:
case glslang::EOpSubgroupPartitionedXor:
case glslang::EOpSubgroupPartitionedInclusiveAdd:
case glslang::EOpSubgroupPartitionedInclusiveMul:
case glslang::EOpSubgroupPartitionedInclusiveMin:
case glslang::EOpSubgroupPartitionedInclusiveMax:
case glslang::EOpSubgroupPartitionedInclusiveAnd:
case glslang::EOpSubgroupPartitionedInclusiveOr:
case glslang::EOpSubgroupPartitionedInclusiveXor:
case glslang::EOpSubgroupPartitionedExclusiveAdd:
case glslang::EOpSubgroupPartitionedExclusiveMul:
case glslang::EOpSubgroupPartitionedExclusiveMin:
case glslang::EOpSubgroupPartitionedExclusiveMax:
case glslang::EOpSubgroupPartitionedExclusiveAnd:
case glslang::EOpSubgroupPartitionedExclusiveOr:
case glslang::EOpSubgroupPartitionedExclusiveXor:
builder.addExtension(spv::E_SPV_NV_shader_subgroup_partitioned);
builder.addCapability(spv::CapabilityGroupNonUniformPartitionedNV);
break;
default: assert(0 && "Unhandled subgroup operation!");
}
const bool isUnsigned = isTypeUnsignedInt(typeProxy);
const bool isFloat = isTypeFloat(typeProxy);
const bool isBool = typeProxy == glslang::EbtBool;
spv::Op opCode = spv::OpNop;
// Figure out which opcode to use.
switch (op) {
case glslang::EOpSubgroupElect: opCode = spv::OpGroupNonUniformElect; break;
case glslang::EOpSubgroupQuadAll: opCode = spv::OpGroupNonUniformQuadAllKHR; break;
case glslang::EOpSubgroupAll: opCode = spv::OpGroupNonUniformAll; break;
case glslang::EOpSubgroupQuadAny: opCode = spv::OpGroupNonUniformQuadAnyKHR; break;
case glslang::EOpSubgroupAny: opCode = spv::OpGroupNonUniformAny; break;
case glslang::EOpSubgroupAllEqual: opCode = spv::OpGroupNonUniformAllEqual; break;
case glslang::EOpSubgroupBroadcast: opCode = spv::OpGroupNonUniformBroadcast; break;
case glslang::EOpSubgroupBroadcastFirst: opCode = spv::OpGroupNonUniformBroadcastFirst; break;
case glslang::EOpSubgroupBallot: opCode = spv::OpGroupNonUniformBallot; break;
case glslang::EOpSubgroupInverseBallot: opCode = spv::OpGroupNonUniformInverseBallot; break;
case glslang::EOpSubgroupBallotBitExtract: opCode = spv::OpGroupNonUniformBallotBitExtract; break;
case glslang::EOpSubgroupBallotBitCount:
case glslang::EOpSubgroupBallotInclusiveBitCount:
case glslang::EOpSubgroupBallotExclusiveBitCount: opCode = spv::OpGroupNonUniformBallotBitCount; break;
case glslang::EOpSubgroupBallotFindLSB: opCode = spv::OpGroupNonUniformBallotFindLSB; break;
case glslang::EOpSubgroupBallotFindMSB: opCode = spv::OpGroupNonUniformBallotFindMSB; break;
case glslang::EOpSubgroupShuffle: opCode = spv::OpGroupNonUniformShuffle; break;
case glslang::EOpSubgroupShuffleXor: opCode = spv::OpGroupNonUniformShuffleXor; break;
case glslang::EOpSubgroupShuffleUp: opCode = spv::OpGroupNonUniformShuffleUp; break;
case glslang::EOpSubgroupShuffleDown: opCode = spv::OpGroupNonUniformShuffleDown; break;
case glslang::EOpSubgroupRotate:
case glslang::EOpSubgroupClusteredRotate: opCode = spv::OpGroupNonUniformRotateKHR; break;
case glslang::EOpSubgroupAdd:
case glslang::EOpSubgroupInclusiveAdd:
case glslang::EOpSubgroupExclusiveAdd:
case glslang::EOpSubgroupClusteredAdd:
case glslang::EOpSubgroupPartitionedAdd:
case glslang::EOpSubgroupPartitionedInclusiveAdd:
case glslang::EOpSubgroupPartitionedExclusiveAdd:
if (isFloat) {
opCode = spv::OpGroupNonUniformFAdd;
} else {
opCode = spv::OpGroupNonUniformIAdd;
}
break;
case glslang::EOpSubgroupMul:
case glslang::EOpSubgroupInclusiveMul:
case glslang::EOpSubgroupExclusiveMul:
case glslang::EOpSubgroupClusteredMul:
case glslang::EOpSubgroupPartitionedMul:
case glslang::EOpSubgroupPartitionedInclusiveMul:
case glslang::EOpSubgroupPartitionedExclusiveMul:
if (isFloat) {
opCode = spv::OpGroupNonUniformFMul;
} else {
opCode = spv::OpGroupNonUniformIMul;
}
break;
case glslang::EOpSubgroupMin:
case glslang::EOpSubgroupInclusiveMin:
case glslang::EOpSubgroupExclusiveMin:
case glslang::EOpSubgroupClusteredMin:
case glslang::EOpSubgroupPartitionedMin:
case glslang::EOpSubgroupPartitionedInclusiveMin:
case glslang::EOpSubgroupPartitionedExclusiveMin:
if (isFloat) {
opCode = spv::OpGroupNonUniformFMin;
} else if (isUnsigned) {
opCode = spv::OpGroupNonUniformUMin;
} else {
opCode = spv::OpGroupNonUniformSMin;
}
break;
case glslang::EOpSubgroupMax:
case glslang::EOpSubgroupInclusiveMax:
case glslang::EOpSubgroupExclusiveMax:
case glslang::EOpSubgroupClusteredMax:
case glslang::EOpSubgroupPartitionedMax:
case glslang::EOpSubgroupPartitionedInclusiveMax:
case glslang::EOpSubgroupPartitionedExclusiveMax:
if (isFloat) {
opCode = spv::OpGroupNonUniformFMax;
} else if (isUnsigned) {
opCode = spv::OpGroupNonUniformUMax;
} else {
opCode = spv::OpGroupNonUniformSMax;
}
break;
case glslang::EOpSubgroupAnd:
case glslang::EOpSubgroupInclusiveAnd:
case glslang::EOpSubgroupExclusiveAnd:
case glslang::EOpSubgroupClusteredAnd:
case glslang::EOpSubgroupPartitionedAnd:
case glslang::EOpSubgroupPartitionedInclusiveAnd:
case glslang::EOpSubgroupPartitionedExclusiveAnd:
if (isBool) {
opCode = spv::OpGroupNonUniformLogicalAnd;
} else {
opCode = spv::OpGroupNonUniformBitwiseAnd;
}
break;
case glslang::EOpSubgroupOr:
case glslang::EOpSubgroupInclusiveOr:
case glslang::EOpSubgroupExclusiveOr:
case glslang::EOpSubgroupClusteredOr:
case glslang::EOpSubgroupPartitionedOr:
case glslang::EOpSubgroupPartitionedInclusiveOr:
case glslang::EOpSubgroupPartitionedExclusiveOr:
if (isBool) {
opCode = spv::OpGroupNonUniformLogicalOr;
} else {
opCode = spv::OpGroupNonUniformBitwiseOr;
}
break;
case glslang::EOpSubgroupXor:
case glslang::EOpSubgroupInclusiveXor:
case glslang::EOpSubgroupExclusiveXor:
case glslang::EOpSubgroupClusteredXor:
case glslang::EOpSubgroupPartitionedXor:
case glslang::EOpSubgroupPartitionedInclusiveXor:
case glslang::EOpSubgroupPartitionedExclusiveXor:
if (isBool) {
opCode = spv::OpGroupNonUniformLogicalXor;
} else {
opCode = spv::OpGroupNonUniformBitwiseXor;
}
break;
case glslang::EOpSubgroupQuadBroadcast: opCode = spv::OpGroupNonUniformQuadBroadcast; break;
case glslang::EOpSubgroupQuadSwapHorizontal:
case glslang::EOpSubgroupQuadSwapVertical:
case glslang::EOpSubgroupQuadSwapDiagonal: opCode = spv::OpGroupNonUniformQuadSwap; break;
default: assert(0 && "Unhandled subgroup operation!");
}
// get the right Group Operation
spv::GroupOperation groupOperation = spv::GroupOperationMax;
switch (op) {
default:
break;
case glslang::EOpSubgroupBallotBitCount:
case glslang::EOpSubgroupAdd:
case glslang::EOpSubgroupMul:
case glslang::EOpSubgroupMin:
case glslang::EOpSubgroupMax:
case glslang::EOpSubgroupAnd:
case glslang::EOpSubgroupOr:
case glslang::EOpSubgroupXor:
groupOperation = spv::GroupOperationReduce;
break;
case glslang::EOpSubgroupBallotInclusiveBitCount:
case glslang::EOpSubgroupInclusiveAdd:
case glslang::EOpSubgroupInclusiveMul:
case glslang::EOpSubgroupInclusiveMin:
case glslang::EOpSubgroupInclusiveMax:
case glslang::EOpSubgroupInclusiveAnd:
case glslang::EOpSubgroupInclusiveOr:
case glslang::EOpSubgroupInclusiveXor:
groupOperation = spv::GroupOperationInclusiveScan;
break;
case glslang::EOpSubgroupBallotExclusiveBitCount:
case glslang::EOpSubgroupExclusiveAdd:
case glslang::EOpSubgroupExclusiveMul:
case glslang::EOpSubgroupExclusiveMin:
case glslang::EOpSubgroupExclusiveMax:
case glslang::EOpSubgroupExclusiveAnd:
case glslang::EOpSubgroupExclusiveOr:
case glslang::EOpSubgroupExclusiveXor:
groupOperation = spv::GroupOperationExclusiveScan;
break;
case glslang::EOpSubgroupClusteredAdd:
case glslang::EOpSubgroupClusteredMul:
case glslang::EOpSubgroupClusteredMin:
case glslang::EOpSubgroupClusteredMax:
case glslang::EOpSubgroupClusteredAnd:
case glslang::EOpSubgroupClusteredOr:
case glslang::EOpSubgroupClusteredXor:
groupOperation = spv::GroupOperationClusteredReduce;
break;
case glslang::EOpSubgroupPartitionedAdd:
case glslang::EOpSubgroupPartitionedMul:
case glslang::EOpSubgroupPartitionedMin:
case glslang::EOpSubgroupPartitionedMax:
case glslang::EOpSubgroupPartitionedAnd:
case glslang::EOpSubgroupPartitionedOr:
case glslang::EOpSubgroupPartitionedXor:
groupOperation = spv::GroupOperationPartitionedReduceNV;
break;
case glslang::EOpSubgroupPartitionedInclusiveAdd:
case glslang::EOpSubgroupPartitionedInclusiveMul:
case glslang::EOpSubgroupPartitionedInclusiveMin:
case glslang::EOpSubgroupPartitionedInclusiveMax:
case glslang::EOpSubgroupPartitionedInclusiveAnd:
case glslang::EOpSubgroupPartitionedInclusiveOr:
case glslang::EOpSubgroupPartitionedInclusiveXor:
groupOperation = spv::GroupOperationPartitionedInclusiveScanNV;
break;
case glslang::EOpSubgroupPartitionedExclusiveAdd:
case glslang::EOpSubgroupPartitionedExclusiveMul:
case glslang::EOpSubgroupPartitionedExclusiveMin:
case glslang::EOpSubgroupPartitionedExclusiveMax:
case glslang::EOpSubgroupPartitionedExclusiveAnd:
case glslang::EOpSubgroupPartitionedExclusiveOr:
case glslang::EOpSubgroupPartitionedExclusiveXor:
groupOperation = spv::GroupOperationPartitionedExclusiveScanNV;
break;
}
// build the instruction
std::vector<spv::IdImmediate> spvGroupOperands;
// Every operation begins with the Execution Scope operand.
spv::IdImmediate executionScope = { true, builder.makeUintConstant(spv::ScopeSubgroup) };
// All other ops need the execution scope. Quad Control Ops don't need scope, it's always Quad.
if (opCode != spv::OpGroupNonUniformQuadAllKHR && opCode != spv::OpGroupNonUniformQuadAnyKHR) {
spvGroupOperands.push_back(executionScope);
}
// Next, for all operations that use a Group Operation, push that as an operand.
if (groupOperation != spv::GroupOperationMax) {
spv::IdImmediate groupOperand = { false, (unsigned)groupOperation };
spvGroupOperands.push_back(groupOperand);
}
// Push back the operands next.
for (auto opIt = operands.cbegin(); opIt != operands.cend(); ++opIt) {
spv::IdImmediate operand = { true, *opIt };
spvGroupOperands.push_back(operand);
}
// Some opcodes have additional operands.
spv::Id directionId = spv::NoResult;
switch (op) {
default: break;
case glslang::EOpSubgroupQuadSwapHorizontal: directionId = builder.makeUintConstant(0); break;
case glslang::EOpSubgroupQuadSwapVertical: directionId = builder.makeUintConstant(1); break;
case glslang::EOpSubgroupQuadSwapDiagonal: directionId = builder.makeUintConstant(2); break;
}
if (directionId != spv::NoResult) {
spv::IdImmediate direction = { true, directionId };
spvGroupOperands.push_back(direction);
}
return builder.createOp(opCode, typeId, spvGroupOperands);
}
spv::Id TGlslangToSpvTraverser::createMiscOperation(glslang::TOperator op, spv::Decoration precision,
spv::Id typeId, std::vector<spv::Id>& operands, glslang::TBasicType typeProxy)
{
bool isUnsigned = isTypeUnsignedInt(typeProxy);
bool isFloat = isTypeFloat(typeProxy);
spv::Op opCode = spv::OpNop;
int extBuiltins = -1;
int libCall = -1;
size_t consumedOperands = operands.size();
spv::Id typeId0 = 0;
if (consumedOperands > 0)
typeId0 = builder.getTypeId(operands[0]);
spv::Id typeId1 = 0;
if (consumedOperands > 1)
typeId1 = builder.getTypeId(operands[1]);
spv::Id frexpIntType = 0;
switch (op) {
case glslang::EOpMin:
if (isFloat)
libCall = nanMinMaxClamp ? spv::GLSLstd450NMin : spv::GLSLstd450FMin;
else if (isUnsigned)
libCall = spv::GLSLstd450UMin;
else
libCall = spv::GLSLstd450SMin;
builder.promoteScalar(precision, operands.front(), operands.back());
break;
case glslang::EOpModf:
libCall = spv::GLSLstd450Modf;
break;
case glslang::EOpMax:
if (isFloat)
libCall = nanMinMaxClamp ? spv::GLSLstd450NMax : spv::GLSLstd450FMax;
else if (isUnsigned)
libCall = spv::GLSLstd450UMax;
else
libCall = spv::GLSLstd450SMax;
builder.promoteScalar(precision, operands.front(), operands.back());
break;
case glslang::EOpPow:
libCall = spv::GLSLstd450Pow;
break;
case glslang::EOpDot:
opCode = spv::OpDot;
break;
case glslang::EOpAtan:
libCall = spv::GLSLstd450Atan2;
break;
case glslang::EOpClamp:
if (isFloat)
libCall = nanMinMaxClamp ? spv::GLSLstd450NClamp : spv::GLSLstd450FClamp;
else if (isUnsigned)
libCall = spv::GLSLstd450UClamp;
else
libCall = spv::GLSLstd450SClamp;
builder.promoteScalar(precision, operands.front(), operands[1]);
builder.promoteScalar(precision, operands.front(), operands[2]);
break;
case glslang::EOpMix:
if (! builder.isBoolType(builder.getScalarTypeId(builder.getTypeId(operands.back())))) {
assert(isFloat);
libCall = spv::GLSLstd450FMix;
} else {
opCode = spv::OpSelect;
std::swap(operands.front(), operands.back());
}
builder.promoteScalar(precision, operands.front(), operands.back());
break;
case glslang::EOpStep:
libCall = spv::GLSLstd450Step;
builder.promoteScalar(precision, operands.front(), operands.back());
break;
case glslang::EOpSmoothStep:
libCall = spv::GLSLstd450SmoothStep;
builder.promoteScalar(precision, operands[0], operands[2]);
builder.promoteScalar(precision, operands[1], operands[2]);
break;
case glslang::EOpDistance:
libCall = spv::GLSLstd450Distance;
break;
case glslang::EOpCross:
libCall = spv::GLSLstd450Cross;
break;
case glslang::EOpFaceForward:
libCall = spv::GLSLstd450FaceForward;
break;
case glslang::EOpReflect:
libCall = spv::GLSLstd450Reflect;
break;
case glslang::EOpRefract:
libCall = spv::GLSLstd450Refract;
break;
case glslang::EOpBarrier:
{
// This is for the extended controlBarrier function, with four operands.
// The unextended barrier() goes through createNoArgOperation.
assert(operands.size() == 4);
unsigned int executionScope = builder.getConstantScalar(operands[0]);
unsigned int memoryScope = builder.getConstantScalar(operands[1]);
unsigned int semantics = builder.getConstantScalar(operands[2]) | builder.getConstantScalar(operands[3]);
builder.createControlBarrier((spv::Scope)executionScope, (spv::Scope)memoryScope,
(spv::MemorySemanticsMask)semantics);
if (semantics & (spv::MemorySemanticsMakeAvailableKHRMask |
spv::MemorySemanticsMakeVisibleKHRMask |
spv::MemorySemanticsOutputMemoryKHRMask |
spv::MemorySemanticsVolatileMask)) {
builder.addCapability(spv::CapabilityVulkanMemoryModelKHR);
}
if (glslangIntermediate->usingVulkanMemoryModel() && (executionScope == spv::ScopeDevice ||
memoryScope == spv::ScopeDevice)) {
builder.addCapability(spv::CapabilityVulkanMemoryModelDeviceScopeKHR);
}
return 0;
}
break;
case glslang::EOpMemoryBarrier:
{
// This is for the extended memoryBarrier function, with three operands.
// The unextended memoryBarrier() goes through createNoArgOperation.
assert(operands.size() == 3);
unsigned int memoryScope = builder.getConstantScalar(operands[0]);
unsigned int semantics = builder.getConstantScalar(operands[1]) | builder.getConstantScalar(operands[2]);
builder.createMemoryBarrier((spv::Scope)memoryScope, (spv::MemorySemanticsMask)semantics);
if (semantics & (spv::MemorySemanticsMakeAvailableKHRMask |
spv::MemorySemanticsMakeVisibleKHRMask |
spv::MemorySemanticsOutputMemoryKHRMask |
spv::MemorySemanticsVolatileMask)) {
builder.addCapability(spv::CapabilityVulkanMemoryModelKHR);
}
if (glslangIntermediate->usingVulkanMemoryModel() && memoryScope == spv::ScopeDevice) {
builder.addCapability(spv::CapabilityVulkanMemoryModelDeviceScopeKHR);
}
return 0;
}
break;
case glslang::EOpInterpolateAtSample:
if (typeProxy == glslang::EbtFloat16)
builder.addExtension(spv::E_SPV_AMD_gpu_shader_half_float);
libCall = spv::GLSLstd450InterpolateAtSample;
break;
case glslang::EOpInterpolateAtOffset:
if (typeProxy == glslang::EbtFloat16)
builder.addExtension(spv::E_SPV_AMD_gpu_shader_half_float);
libCall = spv::GLSLstd450InterpolateAtOffset;
break;
case glslang::EOpAddCarry:
opCode = spv::OpIAddCarry;
typeId = builder.makeStructResultType(typeId0, typeId0);
consumedOperands = 2;
break;
case glslang::EOpSubBorrow:
opCode = spv::OpISubBorrow;
typeId = builder.makeStructResultType(typeId0, typeId0);
consumedOperands = 2;
break;
case glslang::EOpUMulExtended:
opCode = spv::OpUMulExtended;
typeId = builder.makeStructResultType(typeId0, typeId0);
consumedOperands = 2;
break;
case glslang::EOpIMulExtended:
opCode = spv::OpSMulExtended;
typeId = builder.makeStructResultType(typeId0, typeId0);
consumedOperands = 2;
break;
case glslang::EOpBitfieldExtract:
if (isUnsigned)
opCode = spv::OpBitFieldUExtract;
else
opCode = spv::OpBitFieldSExtract;
break;
case glslang::EOpBitfieldInsert:
opCode = spv::OpBitFieldInsert;
break;
case glslang::EOpFma:
libCall = spv::GLSLstd450Fma;
break;
case glslang::EOpFrexp:
{
libCall = spv::GLSLstd450FrexpStruct;
assert(builder.isPointerType(typeId1));
typeId1 = builder.getContainedTypeId(typeId1);
int width = builder.getScalarTypeWidth(typeId1);
if (width == 16)
// Using 16-bit exp operand, enable extension SPV_AMD_gpu_shader_int16
builder.addExtension(spv::E_SPV_AMD_gpu_shader_int16);
if (builder.getNumComponents(operands[0]) == 1)
frexpIntType = builder.makeIntegerType(width, true);
else
frexpIntType = builder.makeVectorType(builder.makeIntegerType(width, true),
builder.getNumComponents(operands[0]));
typeId = builder.makeStructResultType(typeId0, frexpIntType);
consumedOperands = 1;
}
break;
case glslang::EOpLdexp:
libCall = spv::GLSLstd450Ldexp;
break;
case glslang::EOpReadInvocation:
return createInvocationsOperation(op, typeId, operands, typeProxy);
case glslang::EOpSubgroupBroadcast:
case glslang::EOpSubgroupBallotBitExtract:
case glslang::EOpSubgroupShuffle:
case glslang::EOpSubgroupShuffleXor:
case glslang::EOpSubgroupShuffleUp:
case glslang::EOpSubgroupShuffleDown:
case glslang::EOpSubgroupRotate:
case glslang::EOpSubgroupClusteredRotate:
case glslang::EOpSubgroupClusteredAdd:
case glslang::EOpSubgroupClusteredMul:
case glslang::EOpSubgroupClusteredMin:
case glslang::EOpSubgroupClusteredMax:
case glslang::EOpSubgroupClusteredAnd:
case glslang::EOpSubgroupClusteredOr:
case glslang::EOpSubgroupClusteredXor:
case glslang::EOpSubgroupQuadBroadcast:
case glslang::EOpSubgroupPartitionedAdd:
case glslang::EOpSubgroupPartitionedMul:
case glslang::EOpSubgroupPartitionedMin:
case glslang::EOpSubgroupPartitionedMax:
case glslang::EOpSubgroupPartitionedAnd:
case glslang::EOpSubgroupPartitionedOr:
case glslang::EOpSubgroupPartitionedXor:
case glslang::EOpSubgroupPartitionedInclusiveAdd:
case glslang::EOpSubgroupPartitionedInclusiveMul:
case glslang::EOpSubgroupPartitionedInclusiveMin:
case glslang::EOpSubgroupPartitionedInclusiveMax:
case glslang::EOpSubgroupPartitionedInclusiveAnd:
case glslang::EOpSubgroupPartitionedInclusiveOr:
case glslang::EOpSubgroupPartitionedInclusiveXor:
case glslang::EOpSubgroupPartitionedExclusiveAdd:
case glslang::EOpSubgroupPartitionedExclusiveMul:
case glslang::EOpSubgroupPartitionedExclusiveMin:
case glslang::EOpSubgroupPartitionedExclusiveMax:
case glslang::EOpSubgroupPartitionedExclusiveAnd:
case glslang::EOpSubgroupPartitionedExclusiveOr:
case glslang::EOpSubgroupPartitionedExclusiveXor:
return createSubgroupOperation(op, typeId, operands, typeProxy);
case glslang::EOpSwizzleInvocations:
extBuiltins = getExtBuiltins(spv::E_SPV_AMD_shader_ballot);
libCall = spv::SwizzleInvocationsAMD;
break;
case glslang::EOpSwizzleInvocationsMasked:
extBuiltins = getExtBuiltins(spv::E_SPV_AMD_shader_ballot);
libCall = spv::SwizzleInvocationsMaskedAMD;
break;
case glslang::EOpWriteInvocation:
extBuiltins = getExtBuiltins(spv::E_SPV_AMD_shader_ballot);
libCall = spv::WriteInvocationAMD;
break;
case glslang::EOpMin3:
extBuiltins = getExtBuiltins(spv::E_SPV_AMD_shader_trinary_minmax);
if (isFloat)
libCall = spv::FMin3AMD;
else {
if (isUnsigned)
libCall = spv::UMin3AMD;
else
libCall = spv::SMin3AMD;
}
break;
case glslang::EOpMax3:
extBuiltins = getExtBuiltins(spv::E_SPV_AMD_shader_trinary_minmax);
if (isFloat)
libCall = spv::FMax3AMD;
else {
if (isUnsigned)
libCall = spv::UMax3AMD;
else
libCall = spv::SMax3AMD;
}
break;
case glslang::EOpMid3:
extBuiltins = getExtBuiltins(spv::E_SPV_AMD_shader_trinary_minmax);
if (isFloat)
libCall = spv::FMid3AMD;
else {
if (isUnsigned)
libCall = spv::UMid3AMD;
else
libCall = spv::SMid3AMD;
}
break;
case glslang::EOpInterpolateAtVertex:
if (typeProxy == glslang::EbtFloat16)
builder.addExtension(spv::E_SPV_AMD_gpu_shader_half_float);
extBuiltins = getExtBuiltins(spv::E_SPV_AMD_shader_explicit_vertex_parameter);
libCall = spv::InterpolateAtVertexAMD;
break;
case glslang::EOpReportIntersection:
typeId = builder.makeBoolType();
opCode = spv::OpReportIntersectionKHR;
break;
case glslang::EOpTraceNV:
builder.createNoResultOp(spv::OpTraceNV, operands);
return 0;
case glslang::EOpTraceRayMotionNV:
builder.addExtension(spv::E_SPV_NV_ray_tracing_motion_blur);
builder.addCapability(spv::CapabilityRayTracingMotionBlurNV);
builder.createNoResultOp(spv::OpTraceRayMotionNV, operands);
return 0;
case glslang::EOpTraceKHR:
builder.createNoResultOp(spv::OpTraceRayKHR, operands);
return 0;
case glslang::EOpExecuteCallableNV:
builder.createNoResultOp(spv::OpExecuteCallableNV, operands);
return 0;
case glslang::EOpExecuteCallableKHR:
builder.createNoResultOp(spv::OpExecuteCallableKHR, operands);
return 0;
case glslang::EOpRayQueryInitialize:
builder.createNoResultOp(spv::OpRayQueryInitializeKHR, operands);
return 0;
case glslang::EOpRayQueryTerminate:
builder.createNoResultOp(spv::OpRayQueryTerminateKHR, operands);
return 0;
case glslang::EOpRayQueryGenerateIntersection:
builder.createNoResultOp(spv::OpRayQueryGenerateIntersectionKHR, operands);
return 0;
case glslang::EOpRayQueryConfirmIntersection:
builder.createNoResultOp(spv::OpRayQueryConfirmIntersectionKHR, operands);
return 0;
case glslang::EOpRayQueryProceed:
typeId = builder.makeBoolType();
opCode = spv::OpRayQueryProceedKHR;
break;
case glslang::EOpRayQueryGetIntersectionType:
typeId = builder.makeUintType(32);
opCode = spv::OpRayQueryGetIntersectionTypeKHR;
break;
case glslang::EOpRayQueryGetRayTMin:
typeId = builder.makeFloatType(32);
opCode = spv::OpRayQueryGetRayTMinKHR;
break;
case glslang::EOpRayQueryGetRayFlags:
typeId = builder.makeIntType(32);
opCode = spv::OpRayQueryGetRayFlagsKHR;
break;
case glslang::EOpRayQueryGetIntersectionT:
typeId = builder.makeFloatType(32);
opCode = spv::OpRayQueryGetIntersectionTKHR;
break;
case glslang::EOpRayQueryGetIntersectionInstanceCustomIndex:
typeId = builder.makeIntType(32);
opCode = spv::OpRayQueryGetIntersectionInstanceCustomIndexKHR;
break;
case glslang::EOpRayQueryGetIntersectionInstanceId:
typeId = builder.makeIntType(32);
opCode = spv::OpRayQueryGetIntersectionInstanceIdKHR;
break;
case glslang::EOpRayQueryGetIntersectionInstanceShaderBindingTableRecordOffset:
typeId = builder.makeUintType(32);
opCode = spv::OpRayQueryGetIntersectionInstanceShaderBindingTableRecordOffsetKHR;
break;
case glslang::EOpRayQueryGetIntersectionGeometryIndex:
typeId = builder.makeIntType(32);
opCode = spv::OpRayQueryGetIntersectionGeometryIndexKHR;
break;
case glslang::EOpRayQueryGetIntersectionPrimitiveIndex:
typeId = builder.makeIntType(32);
opCode = spv::OpRayQueryGetIntersectionPrimitiveIndexKHR;
break;
case glslang::EOpRayQueryGetIntersectionBarycentrics:
typeId = builder.makeVectorType(builder.makeFloatType(32), 2);
opCode = spv::OpRayQueryGetIntersectionBarycentricsKHR;
break;
case glslang::EOpRayQueryGetIntersectionFrontFace:
typeId = builder.makeBoolType();
opCode = spv::OpRayQueryGetIntersectionFrontFaceKHR;
break;
case glslang::EOpRayQueryGetIntersectionCandidateAABBOpaque:
typeId = builder.makeBoolType();
opCode = spv::OpRayQueryGetIntersectionCandidateAABBOpaqueKHR;
break;
case glslang::EOpRayQueryGetIntersectionObjectRayDirection:
typeId = builder.makeVectorType(builder.makeFloatType(32), 3);
opCode = spv::OpRayQueryGetIntersectionObjectRayDirectionKHR;
break;
case glslang::EOpRayQueryGetIntersectionObjectRayOrigin:
typeId = builder.makeVectorType(builder.makeFloatType(32), 3);
opCode = spv::OpRayQueryGetIntersectionObjectRayOriginKHR;
break;
case glslang::EOpRayQueryGetWorldRayDirection:
typeId = builder.makeVectorType(builder.makeFloatType(32), 3);
opCode = spv::OpRayQueryGetWorldRayDirectionKHR;
break;
case glslang::EOpRayQueryGetWorldRayOrigin:
typeId = builder.makeVectorType(builder.makeFloatType(32), 3);
opCode = spv::OpRayQueryGetWorldRayOriginKHR;
break;
case glslang::EOpRayQueryGetIntersectionObjectToWorld:
typeId = builder.makeMatrixType(builder.makeFloatType(32), 4, 3);
opCode = spv::OpRayQueryGetIntersectionObjectToWorldKHR;
break;
case glslang::EOpRayQueryGetIntersectionWorldToObject:
typeId = builder.makeMatrixType(builder.makeFloatType(32), 4, 3);
opCode = spv::OpRayQueryGetIntersectionWorldToObjectKHR;
break;
case glslang::EOpWritePackedPrimitiveIndices4x8NV:
builder.createNoResultOp(spv::OpWritePackedPrimitiveIndices4x8NV, operands);
return 0;
case glslang::EOpEmitMeshTasksEXT:
if (taskPayloadID)
operands.push_back(taskPayloadID);
// As per SPV_EXT_mesh_shader make it a terminating instruction in the current block
builder.makeStatementTerminator(spv::OpEmitMeshTasksEXT, operands, "post-OpEmitMeshTasksEXT");
return 0;
case glslang::EOpSetMeshOutputsEXT:
builder.createNoResultOp(spv::OpSetMeshOutputsEXT, operands);
return 0;
case glslang::EOpCooperativeMatrixMulAddNV:
opCode = spv::OpCooperativeMatrixMulAddNV;
break;
case glslang::EOpHitObjectTraceRayNV:
builder.createNoResultOp(spv::OpHitObjectTraceRayNV, operands);
return 0;
case glslang::EOpHitObjectTraceRayMotionNV:
builder.createNoResultOp(spv::OpHitObjectTraceRayMotionNV, operands);
return 0;
case glslang::EOpHitObjectRecordHitNV:
builder.createNoResultOp(spv::OpHitObjectRecordHitNV, operands);
return 0;
case glslang::EOpHitObjectRecordHitMotionNV:
builder.createNoResultOp(spv::OpHitObjectRecordHitMotionNV, operands);
return 0;
case glslang::EOpHitObjectRecordHitWithIndexNV:
builder.createNoResultOp(spv::OpHitObjectRecordHitWithIndexNV, operands);
return 0;
case glslang::EOpHitObjectRecordHitWithIndexMotionNV:
builder.createNoResultOp(spv::OpHitObjectRecordHitWithIndexMotionNV, operands);
return 0;
case glslang::EOpHitObjectRecordMissNV:
builder.createNoResultOp(spv::OpHitObjectRecordMissNV, operands);
return 0;
case glslang::EOpHitObjectRecordMissMotionNV:
builder.createNoResultOp(spv::OpHitObjectRecordMissMotionNV, operands);
return 0;
case glslang::EOpHitObjectExecuteShaderNV:
builder.createNoResultOp(spv::OpHitObjectExecuteShaderNV, operands);
return 0;
case glslang::EOpHitObjectIsEmptyNV:
typeId = builder.makeBoolType();
opCode = spv::OpHitObjectIsEmptyNV;
break;
case glslang::EOpHitObjectIsMissNV:
typeId = builder.makeBoolType();
opCode = spv::OpHitObjectIsMissNV;
break;
case glslang::EOpHitObjectIsHitNV:
typeId = builder.makeBoolType();
opCode = spv::OpHitObjectIsHitNV;
break;
case glslang::EOpHitObjectGetRayTMinNV:
typeId = builder.makeFloatType(32);
opCode = spv::OpHitObjectGetRayTMinNV;
break;
case glslang::EOpHitObjectGetRayTMaxNV:
typeId = builder.makeFloatType(32);
opCode = spv::OpHitObjectGetRayTMaxNV;
break;
case glslang::EOpHitObjectGetObjectRayOriginNV:
typeId = builder.makeVectorType(builder.makeFloatType(32), 3);
opCode = spv::OpHitObjectGetObjectRayOriginNV;
break;
case glslang::EOpHitObjectGetObjectRayDirectionNV:
typeId = builder.makeVectorType(builder.makeFloatType(32), 3);
opCode = spv::OpHitObjectGetObjectRayDirectionNV;
break;
case glslang::EOpHitObjectGetWorldRayOriginNV:
typeId = builder.makeVectorType(builder.makeFloatType(32), 3);
opCode = spv::OpHitObjectGetWorldRayOriginNV;
break;
case glslang::EOpHitObjectGetWorldRayDirectionNV:
typeId = builder.makeVectorType(builder.makeFloatType(32), 3);
opCode = spv::OpHitObjectGetWorldRayDirectionNV;
break;
case glslang::EOpHitObjectGetWorldToObjectNV:
typeId = builder.makeMatrixType(builder.makeFloatType(32), 4, 3);
opCode = spv::OpHitObjectGetWorldToObjectNV;
break;
case glslang::EOpHitObjectGetObjectToWorldNV:
typeId = builder.makeMatrixType(builder.makeFloatType(32), 4, 3);
opCode = spv::OpHitObjectGetObjectToWorldNV;
break;
case glslang::EOpHitObjectGetInstanceCustomIndexNV:
typeId = builder.makeIntegerType(32, 1);
opCode = spv::OpHitObjectGetInstanceCustomIndexNV;
break;
case glslang::EOpHitObjectGetInstanceIdNV:
typeId = builder.makeIntegerType(32, 1);
opCode = spv::OpHitObjectGetInstanceIdNV;
break;
case glslang::EOpHitObjectGetGeometryIndexNV:
typeId = builder.makeIntegerType(32, 1);
opCode = spv::OpHitObjectGetGeometryIndexNV;
break;
case glslang::EOpHitObjectGetPrimitiveIndexNV:
typeId = builder.makeIntegerType(32, 1);
opCode = spv::OpHitObjectGetPrimitiveIndexNV;
break;
case glslang::EOpHitObjectGetHitKindNV:
typeId = builder.makeIntegerType(32, 0);
opCode = spv::OpHitObjectGetHitKindNV;
break;
case glslang::EOpHitObjectGetCurrentTimeNV:
typeId = builder.makeFloatType(32);
opCode = spv::OpHitObjectGetCurrentTimeNV;
break;
case glslang::EOpHitObjectGetShaderBindingTableRecordIndexNV:
typeId = builder.makeIntegerType(32, 0);
opCode = spv::OpHitObjectGetShaderBindingTableRecordIndexNV;
return 0;
case glslang::EOpHitObjectGetAttributesNV:
builder.createNoResultOp(spv::OpHitObjectGetAttributesNV, operands);
return 0;
case glslang::EOpHitObjectGetShaderRecordBufferHandleNV:
typeId = builder.makeVectorType(builder.makeUintType(32), 2);
opCode = spv::OpHitObjectGetShaderRecordBufferHandleNV;
break;
case glslang::EOpReorderThreadNV: {
if (operands.size() == 2) {
builder.createNoResultOp(spv::OpReorderThreadWithHintNV, operands);
} else {
builder.createNoResultOp(spv::OpReorderThreadWithHitObjectNV, operands);
}
return 0;
}
case glslang::EOpImageSampleWeightedQCOM:
typeId = builder.makeVectorType(builder.makeFloatType(32), 4);
opCode = spv::OpImageSampleWeightedQCOM;
addImageProcessingQCOMDecoration(operands[2], spv::DecorationWeightTextureQCOM);
break;
case glslang::EOpImageBoxFilterQCOM:
typeId = builder.makeVectorType(builder.makeFloatType(32), 4);
opCode = spv::OpImageBoxFilterQCOM;
break;
case glslang::EOpImageBlockMatchSADQCOM:
typeId = builder.makeVectorType(builder.makeFloatType(32), 4);
opCode = spv::OpImageBlockMatchSADQCOM;
addImageProcessingQCOMDecoration(operands[0], spv::DecorationBlockMatchTextureQCOM);
addImageProcessingQCOMDecoration(operands[2], spv::DecorationBlockMatchTextureQCOM);
break;
case glslang::EOpImageBlockMatchSSDQCOM:
typeId = builder.makeVectorType(builder.makeFloatType(32), 4);
opCode = spv::OpImageBlockMatchSSDQCOM;
addImageProcessingQCOMDecoration(operands[0], spv::DecorationBlockMatchTextureQCOM);
addImageProcessingQCOMDecoration(operands[2], spv::DecorationBlockMatchTextureQCOM);
break;
case glslang::EOpFetchMicroTriangleVertexBarycentricNV:
typeId = builder.makeVectorType(builder.makeFloatType(32), 2);
opCode = spv::OpFetchMicroTriangleVertexBarycentricNV;
break;
case glslang::EOpFetchMicroTriangleVertexPositionNV:
typeId = builder.makeVectorType(builder.makeFloatType(32), 3);
opCode = spv::OpFetchMicroTriangleVertexPositionNV;
break;
case glslang::EOpImageBlockMatchWindowSSDQCOM:
typeId = builder.makeVectorType(builder.makeFloatType(32), 4);
opCode = spv::OpImageBlockMatchWindowSSDQCOM;
addImageProcessing2QCOMDecoration(operands[0], false);
addImageProcessing2QCOMDecoration(operands[2], false);
break;
case glslang::EOpImageBlockMatchWindowSADQCOM:
typeId = builder.makeVectorType(builder.makeFloatType(32), 4);
opCode = spv::OpImageBlockMatchWindowSADQCOM;
addImageProcessing2QCOMDecoration(operands[0], false);
addImageProcessing2QCOMDecoration(operands[2], false);
break;
case glslang::EOpImageBlockMatchGatherSSDQCOM:
typeId = builder.makeVectorType(builder.makeFloatType(32), 4);
opCode = spv::OpImageBlockMatchGatherSSDQCOM;
addImageProcessing2QCOMDecoration(operands[0], true);
addImageProcessing2QCOMDecoration(operands[2], true);
break;
case glslang::EOpImageBlockMatchGatherSADQCOM:
typeId = builder.makeVectorType(builder.makeFloatType(32), 4);
opCode = spv::OpImageBlockMatchGatherSADQCOM;
addImageProcessing2QCOMDecoration(operands[0], true);
addImageProcessing2QCOMDecoration(operands[2], true);
break;
default:
return 0;
}
spv::Id id = 0;
if (libCall >= 0) {
// Use an extended instruction from the standard library.
// Construct the call arguments, without modifying the original operands vector.
// We might need the remaining arguments, e.g. in the EOpFrexp case.
std::vector<spv::Id> callArguments(operands.begin(), operands.begin() + consumedOperands);
id = builder.createBuiltinCall(typeId, extBuiltins >= 0 ? extBuiltins : stdBuiltins, libCall, callArguments);
} else if (opCode == spv::OpDot && !isFloat) {
// int dot(int, int)
// NOTE: never called for scalar/vector1, this is turned into simple mul before this can be reached
const int componentCount = builder.getNumComponents(operands[0]);
spv::Id mulOp = builder.createBinOp(spv::OpIMul, builder.getTypeId(operands[0]), operands[0], operands[1]);
builder.setPrecision(mulOp, precision);
id = builder.createCompositeExtract(mulOp, typeId, 0);
for (int i = 1; i < componentCount; ++i) {
builder.setPrecision(id, precision);
id = builder.createBinOp(spv::OpIAdd, typeId, id, builder.createCompositeExtract(mulOp, typeId, i));
}
} else {
switch (consumedOperands) {
case 0:
// should all be handled by visitAggregate and createNoArgOperation
assert(0);
return 0;
case 1:
// should all be handled by createUnaryOperation
assert(0);
return 0;
case 2:
id = builder.createBinOp(opCode, typeId, operands[0], operands[1]);
break;
default:
// anything 3 or over doesn't have l-value operands, so all should be consumed
assert(consumedOperands == operands.size());
id = builder.createOp(opCode, typeId, operands);
break;
}
}
// Decode the return types that were structures
switch (op) {
case glslang::EOpAddCarry:
case glslang::EOpSubBorrow:
builder.createStore(builder.createCompositeExtract(id, typeId0, 1), operands[2]);
id = builder.createCompositeExtract(id, typeId0, 0);
break;
case glslang::EOpUMulExtended:
case glslang::EOpIMulExtended:
builder.createStore(builder.createCompositeExtract(id, typeId0, 0), operands[3]);
builder.createStore(builder.createCompositeExtract(id, typeId0, 1), operands[2]);
break;
case glslang::EOpFrexp:
{
assert(operands.size() == 2);
if (builder.isFloatType(builder.getScalarTypeId(typeId1))) {
// "exp" is floating-point type (from HLSL intrinsic)
spv::Id member1 = builder.createCompositeExtract(id, frexpIntType, 1);
member1 = builder.createUnaryOp(spv::OpConvertSToF, typeId1, member1);
builder.createStore(member1, operands[1]);
} else
// "exp" is integer type (from GLSL built-in function)
builder.createStore(builder.createCompositeExtract(id, frexpIntType, 1), operands[1]);
id = builder.createCompositeExtract(id, typeId0, 0);
}
break;
default:
break;
}
return builder.setPrecision(id, precision);
}
// Intrinsics with no arguments (or no return value, and no precision).
spv::Id TGlslangToSpvTraverser::createNoArgOperation(glslang::TOperator op, spv::Decoration precision, spv::Id typeId)
{
// GLSL memory barriers use queuefamily scope in new model, device scope in old model
spv::Scope memoryBarrierScope = glslangIntermediate->usingVulkanMemoryModel() ?
spv::ScopeQueueFamilyKHR : spv::ScopeDevice;
switch (op) {
case glslang::EOpBarrier:
if (glslangIntermediate->getStage() == EShLangTessControl) {
if (glslangIntermediate->usingVulkanMemoryModel()) {
builder.createControlBarrier(spv::ScopeWorkgroup, spv::ScopeWorkgroup,
spv::MemorySemanticsOutputMemoryKHRMask |
spv::MemorySemanticsAcquireReleaseMask);
builder.addCapability(spv::CapabilityVulkanMemoryModelKHR);
} else {
builder.createControlBarrier(spv::ScopeWorkgroup, spv::ScopeInvocation, spv::MemorySemanticsMaskNone);
}
} else {
builder.createControlBarrier(spv::ScopeWorkgroup, spv::ScopeWorkgroup,
spv::MemorySemanticsWorkgroupMemoryMask |
spv::MemorySemanticsAcquireReleaseMask);
}
return 0;
case glslang::EOpMemoryBarrier:
builder.createMemoryBarrier(memoryBarrierScope, spv::MemorySemanticsAllMemory |
spv::MemorySemanticsAcquireReleaseMask);
return 0;
case glslang::EOpMemoryBarrierBuffer:
builder.createMemoryBarrier(memoryBarrierScope, spv::MemorySemanticsUniformMemoryMask |
spv::MemorySemanticsAcquireReleaseMask);
return 0;
case glslang::EOpMemoryBarrierShared:
builder.createMemoryBarrier(memoryBarrierScope, spv::MemorySemanticsWorkgroupMemoryMask |
spv::MemorySemanticsAcquireReleaseMask);
return 0;
case glslang::EOpGroupMemoryBarrier:
builder.createMemoryBarrier(spv::ScopeWorkgroup, spv::MemorySemanticsAllMemory |
spv::MemorySemanticsAcquireReleaseMask);
return 0;
case glslang::EOpMemoryBarrierAtomicCounter:
builder.createMemoryBarrier(memoryBarrierScope, spv::MemorySemanticsAtomicCounterMemoryMask |
spv::MemorySemanticsAcquireReleaseMask);
return 0;
case glslang::EOpMemoryBarrierImage:
builder.createMemoryBarrier(memoryBarrierScope, spv::MemorySemanticsImageMemoryMask |
spv::MemorySemanticsAcquireReleaseMask);
return 0;
case glslang::EOpAllMemoryBarrierWithGroupSync:
builder.createControlBarrier(spv::ScopeWorkgroup, spv::ScopeDevice,
spv::MemorySemanticsAllMemory |
spv::MemorySemanticsAcquireReleaseMask);
return 0;
case glslang::EOpDeviceMemoryBarrier:
builder.createMemoryBarrier(spv::ScopeDevice, spv::MemorySemanticsUniformMemoryMask |
spv::MemorySemanticsImageMemoryMask |
spv::MemorySemanticsAcquireReleaseMask);
return 0;
case glslang::EOpDeviceMemoryBarrierWithGroupSync:
builder.createControlBarrier(spv::ScopeWorkgroup, spv::ScopeDevice, spv::MemorySemanticsUniformMemoryMask |
spv::MemorySemanticsImageMemoryMask |
spv::MemorySemanticsAcquireReleaseMask);
return 0;
case glslang::EOpWorkgroupMemoryBarrier:
builder.createMemoryBarrier(spv::ScopeWorkgroup, spv::MemorySemanticsWorkgroupMemoryMask |
spv::MemorySemanticsAcquireReleaseMask);
return 0;
case glslang::EOpWorkgroupMemoryBarrierWithGroupSync:
builder.createControlBarrier(spv::ScopeWorkgroup, spv::ScopeWorkgroup,
spv::MemorySemanticsWorkgroupMemoryMask |
spv::MemorySemanticsAcquireReleaseMask);
return 0;
case glslang::EOpSubgroupBarrier:
builder.createControlBarrier(spv::ScopeSubgroup, spv::ScopeSubgroup, spv::MemorySemanticsAllMemory |
spv::MemorySemanticsAcquireReleaseMask);
return spv::NoResult;
case glslang::EOpSubgroupMemoryBarrier:
builder.createMemoryBarrier(spv::ScopeSubgroup, spv::MemorySemanticsAllMemory |
spv::MemorySemanticsAcquireReleaseMask);
return spv::NoResult;
case glslang::EOpSubgroupMemoryBarrierBuffer:
builder.createMemoryBarrier(spv::ScopeSubgroup, spv::MemorySemanticsUniformMemoryMask |
spv::MemorySemanticsAcquireReleaseMask);
return spv::NoResult;
case glslang::EOpSubgroupMemoryBarrierImage:
builder.createMemoryBarrier(spv::ScopeSubgroup, spv::MemorySemanticsImageMemoryMask |
spv::MemorySemanticsAcquireReleaseMask);
return spv::NoResult;
case glslang::EOpSubgroupMemoryBarrierShared:
builder.createMemoryBarrier(spv::ScopeSubgroup, spv::MemorySemanticsWorkgroupMemoryMask |
spv::MemorySemanticsAcquireReleaseMask);
return spv::NoResult;
case glslang::EOpEmitVertex:
builder.createNoResultOp(spv::OpEmitVertex);
return 0;
case glslang::EOpEndPrimitive:
builder.createNoResultOp(spv::OpEndPrimitive);
return 0;
case glslang::EOpSubgroupElect: {
std::vector<spv::Id> operands;
return createSubgroupOperation(op, typeId, operands, glslang::EbtVoid);
}
case glslang::EOpTime:
{
std::vector<spv::Id> args; // Dummy arguments
spv::Id id = builder.createBuiltinCall(typeId, getExtBuiltins(spv::E_SPV_AMD_gcn_shader), spv::TimeAMD, args);
return builder.setPrecision(id, precision);
}
case glslang::EOpIgnoreIntersectionNV:
builder.createNoResultOp(spv::OpIgnoreIntersectionNV);
return 0;
case glslang::EOpTerminateRayNV:
builder.createNoResultOp(spv::OpTerminateRayNV);
return 0;
case glslang::EOpRayQueryInitialize:
builder.createNoResultOp(spv::OpRayQueryInitializeKHR);
return 0;
case glslang::EOpRayQueryTerminate:
builder.createNoResultOp(spv::OpRayQueryTerminateKHR);
return 0;
case glslang::EOpRayQueryGenerateIntersection:
builder.createNoResultOp(spv::OpRayQueryGenerateIntersectionKHR);
return 0;
case glslang::EOpRayQueryConfirmIntersection:
builder.createNoResultOp(spv::OpRayQueryConfirmIntersectionKHR);
return 0;
case glslang::EOpBeginInvocationInterlock:
builder.createNoResultOp(spv::OpBeginInvocationInterlockEXT);
return 0;
case glslang::EOpEndInvocationInterlock:
builder.createNoResultOp(spv::OpEndInvocationInterlockEXT);
return 0;
case glslang::EOpIsHelperInvocation:
{
std::vector<spv::Id> args; // Dummy arguments
builder.addExtension(spv::E_SPV_EXT_demote_to_helper_invocation);
builder.addCapability(spv::CapabilityDemoteToHelperInvocationEXT);
return builder.createOp(spv::OpIsHelperInvocationEXT, typeId, args);
}
case glslang::EOpReadClockSubgroupKHR: {
std::vector<spv::Id> args;
args.push_back(builder.makeUintConstant(spv::ScopeSubgroup));
builder.addExtension(spv::E_SPV_KHR_shader_clock);
builder.addCapability(spv::CapabilityShaderClockKHR);
return builder.createOp(spv::OpReadClockKHR, typeId, args);
}
case glslang::EOpReadClockDeviceKHR: {
std::vector<spv::Id> args;
args.push_back(builder.makeUintConstant(spv::ScopeDevice));
builder.addExtension(spv::E_SPV_KHR_shader_clock);
builder.addCapability(spv::CapabilityShaderClockKHR);
return builder.createOp(spv::OpReadClockKHR, typeId, args);
}
case glslang::EOpStencilAttachmentReadEXT:
case glslang::EOpDepthAttachmentReadEXT:
{
builder.addExtension(spv::E_SPV_EXT_shader_tile_image);
spv::Decoration precision;
spv::Op spv_op;
if (op == glslang::EOpStencilAttachmentReadEXT)
{
precision = spv::DecorationRelaxedPrecision;
spv_op = spv::OpStencilAttachmentReadEXT;
builder.addCapability(spv::CapabilityTileImageStencilReadAccessEXT);
}
else
{
precision = spv::NoPrecision;
spv_op = spv::OpDepthAttachmentReadEXT;
builder.addCapability(spv::CapabilityTileImageDepthReadAccessEXT);
}
std::vector<spv::Id> args; // Dummy args
spv::Id result = builder.createOp(spv_op, typeId, args);
return builder.setPrecision(result, precision);
}
default:
break;
}
logger->missingFunctionality("unknown operation with no arguments");
return 0;
}
spv::Id TGlslangToSpvTraverser::getSymbolId(const glslang::TIntermSymbol* symbol)
{
auto iter = symbolValues.find(symbol->getId());
spv::Id id;
if (symbolValues.end() != iter) {
id = iter->second;
return id;
}
// it was not found, create it
spv::BuiltIn builtIn = TranslateBuiltInDecoration(symbol->getQualifier().builtIn, false);
auto forcedType = getForcedType(symbol->getQualifier().builtIn, symbol->getType());
// There are pairs of symbols that map to the same SPIR-V built-in:
// gl_ObjectToWorldEXT and gl_ObjectToWorld3x4EXT, and gl_WorldToObjectEXT
// and gl_WorldToObject3x4EXT. SPIR-V forbids having two OpVariables
// with the same BuiltIn in the same storage class, so we must re-use one.
const bool mayNeedToReuseBuiltIn =
builtIn == spv::BuiltInObjectToWorldKHR ||
builtIn == spv::BuiltInWorldToObjectKHR;
if (mayNeedToReuseBuiltIn) {
auto iter = builtInVariableIds.find(uint32_t(builtIn));
if (builtInVariableIds.end() != iter) {
id = iter->second;
symbolValues[symbol->getId()] = id;
if (forcedType.second != spv::NoType)
forceType[id] = forcedType.second;
return id;
}
}
id = createSpvVariable(symbol, forcedType.first);
if (mayNeedToReuseBuiltIn) {
builtInVariableIds.insert({uint32_t(builtIn), id});
}
symbolValues[symbol->getId()] = id;
if (forcedType.second != spv::NoType)
forceType[id] = forcedType.second;
if (symbol->getBasicType() != glslang::EbtBlock) {
builder.addDecoration(id, TranslatePrecisionDecoration(symbol->getType()));
builder.addDecoration(id, TranslateInterpolationDecoration(symbol->getType().getQualifier()));
builder.addDecoration(id, TranslateAuxiliaryStorageDecoration(symbol->getType().getQualifier()));
addMeshNVDecoration(id, /*member*/ -1, symbol->getType().getQualifier());
if (symbol->getQualifier().hasComponent())
builder.addDecoration(id, spv::DecorationComponent, symbol->getQualifier().layoutComponent);
if (symbol->getQualifier().hasIndex())
builder.addDecoration(id, spv::DecorationIndex, symbol->getQualifier().layoutIndex);
if (symbol->getType().getQualifier().hasSpecConstantId())
builder.addDecoration(id, spv::DecorationSpecId, symbol->getType().getQualifier().layoutSpecConstantId);
// atomic counters use this:
if (symbol->getQualifier().hasOffset())
builder.addDecoration(id, spv::DecorationOffset, symbol->getQualifier().layoutOffset);
}
if (symbol->getQualifier().hasLocation()) {
if (!(glslangIntermediate->isRayTracingStage() &&
(glslangIntermediate->IsRequestedExtension(glslang::E_GL_EXT_ray_tracing) ||
glslangIntermediate->IsRequestedExtension(glslang::E_GL_NV_shader_invocation_reorder))
&& (builder.getStorageClass(id) == spv::StorageClassRayPayloadKHR ||
builder.getStorageClass(id) == spv::StorageClassIncomingRayPayloadKHR ||
builder.getStorageClass(id) == spv::StorageClassCallableDataKHR ||
builder.getStorageClass(id) == spv::StorageClassIncomingCallableDataKHR ||
builder.getStorageClass(id) == spv::StorageClassHitObjectAttributeNV))) {
// Location values are used to link TraceRayKHR/ExecuteCallableKHR/HitObjectGetAttributesNV
// to corresponding variables but are not valid in SPIRV since they are supported only
// for Input/Output Storage classes.
builder.addDecoration(id, spv::DecorationLocation, symbol->getQualifier().layoutLocation);
}
}
builder.addDecoration(id, TranslateInvariantDecoration(symbol->getType().getQualifier()));
if (symbol->getQualifier().hasStream() && glslangIntermediate->isMultiStream()) {
builder.addCapability(spv::CapabilityGeometryStreams);
builder.addDecoration(id, spv::DecorationStream, symbol->getQualifier().layoutStream);
}
if (symbol->getQualifier().hasSet())
builder.addDecoration(id, spv::DecorationDescriptorSet, symbol->getQualifier().layoutSet);
else if (IsDescriptorResource(symbol->getType())) {
// default to 0
builder.addDecoration(id, spv::DecorationDescriptorSet, 0);
}
if (symbol->getQualifier().hasBinding())
builder.addDecoration(id, spv::DecorationBinding, symbol->getQualifier().layoutBinding);
else if (IsDescriptorResource(symbol->getType())) {
// default to 0
builder.addDecoration(id, spv::DecorationBinding, 0);
}
if (symbol->getQualifier().hasAttachment())
builder.addDecoration(id, spv::DecorationInputAttachmentIndex, symbol->getQualifier().layoutAttachment);
if (glslangIntermediate->getXfbMode()) {
builder.addCapability(spv::CapabilityTransformFeedback);
if (symbol->getQualifier().hasXfbBuffer()) {
builder.addDecoration(id, spv::DecorationXfbBuffer, symbol->getQualifier().layoutXfbBuffer);
unsigned stride = glslangIntermediate->getXfbStride(symbol->getQualifier().layoutXfbBuffer);
if (stride != glslang::TQualifier::layoutXfbStrideEnd)
builder.addDecoration(id, spv::DecorationXfbStride, stride);
}
if (symbol->getQualifier().hasXfbOffset())
builder.addDecoration(id, spv::DecorationOffset, symbol->getQualifier().layoutXfbOffset);
}
// add built-in variable decoration
if (builtIn != spv::BuiltInMax) {
// WorkgroupSize deprecated in spirv1.6
if (glslangIntermediate->getSpv().spv < glslang::EShTargetSpv_1_6 ||
builtIn != spv::BuiltInWorkgroupSize)
builder.addDecoration(id, spv::DecorationBuiltIn, (int)builtIn);
}
// Add volatile decoration to HelperInvocation for spirv1.6 and beyond
if (builtIn == spv::BuiltInHelperInvocation &&
!glslangIntermediate->usingVulkanMemoryModel() &&
glslangIntermediate->getSpv().spv >= glslang::EShTargetSpv_1_6) {
builder.addDecoration(id, spv::DecorationVolatile);
}
// Subgroup builtins which have input storage class are volatile for ray tracing stages.
if (symbol->getType().isImage() || symbol->getQualifier().isPipeInput()) {
std::vector<spv::Decoration> memory;
TranslateMemoryDecoration(symbol->getType().getQualifier(), memory,
glslangIntermediate->usingVulkanMemoryModel());
for (unsigned int i = 0; i < memory.size(); ++i)
builder.addDecoration(id, memory[i]);
}
if (builtIn == spv::BuiltInSampleMask) {
spv::Decoration decoration;
// GL_NV_sample_mask_override_coverage extension
if (glslangIntermediate->getLayoutOverrideCoverage())
decoration = (spv::Decoration)spv::DecorationOverrideCoverageNV;
else
decoration = (spv::Decoration)spv::DecorationMax;
builder.addDecoration(id, decoration);
if (decoration != spv::DecorationMax) {
builder.addCapability(spv::CapabilitySampleMaskOverrideCoverageNV);
builder.addExtension(spv::E_SPV_NV_sample_mask_override_coverage);
}
}
else if (builtIn == spv::BuiltInLayer) {
// SPV_NV_viewport_array2 extension
if (symbol->getQualifier().layoutViewportRelative) {
builder.addDecoration(id, (spv::Decoration)spv::DecorationViewportRelativeNV);
builder.addCapability(spv::CapabilityShaderViewportMaskNV);
builder.addExtension(spv::E_SPV_NV_viewport_array2);
}
if (symbol->getQualifier().layoutSecondaryViewportRelativeOffset != -2048) {
builder.addDecoration(id, (spv::Decoration)spv::DecorationSecondaryViewportRelativeNV,
symbol->getQualifier().layoutSecondaryViewportRelativeOffset);
builder.addCapability(spv::CapabilityShaderStereoViewNV);
builder.addExtension(spv::E_SPV_NV_stereo_view_rendering);
}
}
if (symbol->getQualifier().layoutPassthrough) {
builder.addDecoration(id, spv::DecorationPassthroughNV);
builder.addCapability(spv::CapabilityGeometryShaderPassthroughNV);
builder.addExtension(spv::E_SPV_NV_geometry_shader_passthrough);
}
if (symbol->getQualifier().pervertexNV) {
builder.addDecoration(id, spv::DecorationPerVertexNV);
builder.addCapability(spv::CapabilityFragmentBarycentricNV);
builder.addExtension(spv::E_SPV_NV_fragment_shader_barycentric);
}
if (symbol->getQualifier().pervertexEXT) {
builder.addDecoration(id, spv::DecorationPerVertexKHR);
builder.addCapability(spv::CapabilityFragmentBarycentricKHR);
builder.addExtension(spv::E_SPV_KHR_fragment_shader_barycentric);
}
if (glslangIntermediate->getHlslFunctionality1() && symbol->getType().getQualifier().semanticName != nullptr) {
builder.addExtension("SPV_GOOGLE_hlsl_functionality1");
builder.addDecoration(id, (spv::Decoration)spv::DecorationHlslSemanticGOOGLE,
symbol->getType().getQualifier().semanticName);
}
if (symbol->isReference()) {
builder.addDecoration(id, symbol->getType().getQualifier().restrict ?
spv::DecorationRestrictPointerEXT : spv::DecorationAliasedPointerEXT);
}
// Add SPIR-V decorations (GL_EXT_spirv_intrinsics)
if (symbol->getType().getQualifier().hasSpirvDecorate())
applySpirvDecorate(symbol->getType(), id, {});
return id;
}
// add per-primitive, per-view. per-task decorations to a struct member (member >= 0) or an object
void TGlslangToSpvTraverser::addMeshNVDecoration(spv::Id id, int member, const glslang::TQualifier& qualifier)
{
bool isMeshShaderExt = (glslangIntermediate->getRequestedExtensions().find(glslang::E_GL_EXT_mesh_shader) !=
glslangIntermediate->getRequestedExtensions().end());
if (member >= 0) {
if (qualifier.perPrimitiveNV) {
// Need to add capability/extension for fragment shader.
// Mesh shader already adds this by default.
if (glslangIntermediate->getStage() == EShLangFragment) {
if(isMeshShaderExt) {
builder.addCapability(spv::CapabilityMeshShadingEXT);
builder.addExtension(spv::E_SPV_EXT_mesh_shader);
} else {
builder.addCapability(spv::CapabilityMeshShadingNV);
builder.addExtension(spv::E_SPV_NV_mesh_shader);
}
}
builder.addMemberDecoration(id, (unsigned)member, spv::DecorationPerPrimitiveNV);
}
if (qualifier.perViewNV)
builder.addMemberDecoration(id, (unsigned)member, spv::DecorationPerViewNV);
if (qualifier.perTaskNV)
builder.addMemberDecoration(id, (unsigned)member, spv::DecorationPerTaskNV);
} else {
if (qualifier.perPrimitiveNV) {
// Need to add capability/extension for fragment shader.
// Mesh shader already adds this by default.
if (glslangIntermediate->getStage() == EShLangFragment) {
if(isMeshShaderExt) {
builder.addCapability(spv::CapabilityMeshShadingEXT);
builder.addExtension(spv::E_SPV_EXT_mesh_shader);
} else {
builder.addCapability(spv::CapabilityMeshShadingNV);
builder.addExtension(spv::E_SPV_NV_mesh_shader);
}
}
builder.addDecoration(id, spv::DecorationPerPrimitiveNV);
}
if (qualifier.perViewNV)
builder.addDecoration(id, spv::DecorationPerViewNV);
if (qualifier.perTaskNV)
builder.addDecoration(id, spv::DecorationPerTaskNV);
}
}
bool TGlslangToSpvTraverser::hasQCOMImageProceessingDecoration(spv::Id id, spv::Decoration decor)
{
std::vector<spv::Decoration> &decoVec = idToQCOMDecorations[id];
for ( auto d : decoVec ) {
if ( d == decor )
return true;
}
return false;
}
void TGlslangToSpvTraverser::addImageProcessingQCOMDecoration(spv::Id id, spv::Decoration decor)
{
spv::Op opc = builder.getOpCode(id);
if (opc == spv::OpSampledImage) {
id = builder.getIdOperand(id, 0);
opc = builder.getOpCode(id);
}
if (opc == spv::OpLoad) {
spv::Id texid = builder.getIdOperand(id, 0);
if (!hasQCOMImageProceessingDecoration(texid, decor)) {//
builder.addDecoration(texid, decor);
idToQCOMDecorations[texid].push_back(decor);
}
}
}
void TGlslangToSpvTraverser::addImageProcessing2QCOMDecoration(spv::Id id, bool isForGather)
{
if (isForGather) {
return addImageProcessingQCOMDecoration(id, spv::DecorationBlockMatchTextureQCOM);
}
auto addDecor =
[this](spv::Id id, spv::Decoration decor) {
spv::Id tsopc = this->builder.getOpCode(id);
if (tsopc == spv::OpLoad) {
spv::Id tsid = this->builder.getIdOperand(id, 0);
if (this->glslangIntermediate->getSpv().spv >= glslang::EShTargetSpv_1_4) {
assert(iOSet.count(tsid) > 0);
}
if (!hasQCOMImageProceessingDecoration(tsid, decor)) {
this->builder.addDecoration(tsid, decor);
idToQCOMDecorations[tsid].push_back(decor);
}
}
};
spv::Id opc = builder.getOpCode(id);
bool isInterfaceObject = (opc != spv::OpSampledImage);
if (!isInterfaceObject) {
addDecor(builder.getIdOperand(id, 0), spv::DecorationBlockMatchTextureQCOM);
addDecor(builder.getIdOperand(id, 1), spv::DecorationBlockMatchSamplerQCOM);
} else {
addDecor(id, spv::DecorationBlockMatchTextureQCOM);
addDecor(id, spv::DecorationBlockMatchSamplerQCOM);
}
}
// Make a full tree of instructions to build a SPIR-V specialization constant,
// or regular constant if possible.
//
// TBD: this is not yet done, nor verified to be the best design, it does do the leaf symbols though
//
// Recursively walk the nodes. The nodes form a tree whose leaves are
// regular constants, which themselves are trees that createSpvConstant()
// recursively walks. So, this function walks the "top" of the tree:
// - emit specialization constant-building instructions for specConstant
// - when running into a non-spec-constant, switch to createSpvConstant()
spv::Id TGlslangToSpvTraverser::createSpvConstant(const glslang::TIntermTyped& node)
{
assert(node.getQualifier().isConstant());
// Handle front-end constants first (non-specialization constants).
if (! node.getQualifier().specConstant) {
// hand off to the non-spec-constant path
assert(node.getAsConstantUnion() != nullptr || node.getAsSymbolNode() != nullptr);
int nextConst = 0;
return createSpvConstantFromConstUnionArray(node.getType(), node.getAsConstantUnion() ?
node.getAsConstantUnion()->getConstArray() : node.getAsSymbolNode()->getConstArray(),
nextConst, false);
}
// We now know we have a specialization constant to build
// Extra capabilities may be needed.
if (node.getType().contains8BitInt())
builder.addCapability(spv::CapabilityInt8);
if (node.getType().contains16BitFloat())
builder.addCapability(spv::CapabilityFloat16);
if (node.getType().contains16BitInt())
builder.addCapability(spv::CapabilityInt16);
if (node.getType().contains64BitInt())
builder.addCapability(spv::CapabilityInt64);
if (node.getType().containsDouble())
builder.addCapability(spv::CapabilityFloat64);
// gl_WorkGroupSize is a special case until the front-end handles hierarchical specialization constants,
// even then, it's specialization ids are handled by special case syntax in GLSL: layout(local_size_x = ...
if (node.getType().getQualifier().builtIn == glslang::EbvWorkGroupSize) {
std::vector<spv::Id> dimConstId;
for (int dim = 0; dim < 3; ++dim) {
bool specConst = (glslangIntermediate->getLocalSizeSpecId(dim) != glslang::TQualifier::layoutNotSet);
dimConstId.push_back(builder.makeUintConstant(glslangIntermediate->getLocalSize(dim), specConst));
if (specConst) {
builder.addDecoration(dimConstId.back(), spv::DecorationSpecId,
glslangIntermediate->getLocalSizeSpecId(dim));
}
}
return builder.makeCompositeConstant(builder.makeVectorType(builder.makeUintType(32), 3), dimConstId, true);
}
// An AST node labelled as specialization constant should be a symbol node.
// Its initializer should either be a sub tree with constant nodes, or a constant union array.
if (auto* sn = node.getAsSymbolNode()) {
spv::Id result;
if (auto* sub_tree = sn->getConstSubtree()) {
// Traverse the constant constructor sub tree like generating normal run-time instructions.
// During the AST traversal, if the node is marked as 'specConstant', SpecConstantOpModeGuard
// will set the builder into spec constant op instruction generating mode.
sub_tree->traverse(this);
result = accessChainLoad(sub_tree->getType());
} else if (auto* const_union_array = &sn->getConstArray()) {
int nextConst = 0;
result = createSpvConstantFromConstUnionArray(sn->getType(), *const_union_array, nextConst, true);
} else {
logger->missingFunctionality("Invalid initializer for spec onstant.");
return spv::NoResult;
}
builder.addName(result, sn->getName().c_str());
return result;
}
// Neither a front-end constant node, nor a specialization constant node with constant union array or
// constant sub tree as initializer.
logger->missingFunctionality("Neither a front-end constant nor a spec constant.");
return spv::NoResult;
}
// Use 'consts' as the flattened glslang source of scalar constants to recursively
// build the aggregate SPIR-V constant.
//
// If there are not enough elements present in 'consts', 0 will be substituted;
// an empty 'consts' can be used to create a fully zeroed SPIR-V constant.
//
spv::Id TGlslangToSpvTraverser::createSpvConstantFromConstUnionArray(const glslang::TType& glslangType,
const glslang::TConstUnionArray& consts, int& nextConst, bool specConstant)
{
// vector of constants for SPIR-V
std::vector<spv::Id> spvConsts;
// Type is used for struct and array constants
spv::Id typeId = convertGlslangToSpvType(glslangType);
if (glslangType.isArray()) {
glslang::TType elementType(glslangType, 0);
for (int i = 0; i < glslangType.getOuterArraySize(); ++i)
spvConsts.push_back(createSpvConstantFromConstUnionArray(elementType, consts, nextConst, false));
} else if (glslangType.isMatrix()) {
glslang::TType vectorType(glslangType, 0);
for (int col = 0; col < glslangType.getMatrixCols(); ++col)
spvConsts.push_back(createSpvConstantFromConstUnionArray(vectorType, consts, nextConst, false));
} else if (glslangType.isCoopMat()) {
glslang::TType componentType(glslangType.getBasicType());
spvConsts.push_back(createSpvConstantFromConstUnionArray(componentType, consts, nextConst, false));
} else if (glslangType.isStruct()) {
glslang::TVector<glslang::TTypeLoc>::const_iterator iter;
for (iter = glslangType.getStruct()->begin(); iter != glslangType.getStruct()->end(); ++iter)
spvConsts.push_back(createSpvConstantFromConstUnionArray(*iter->type, consts, nextConst, false));
} else if (glslangType.getVectorSize() > 1) {
for (unsigned int i = 0; i < (unsigned int)glslangType.getVectorSize(); ++i) {
bool zero = nextConst >= consts.size();
switch (glslangType.getBasicType()) {
case glslang::EbtInt:
spvConsts.push_back(builder.makeIntConstant(zero ? 0 : consts[nextConst].getIConst()));
break;
case glslang::EbtUint:
spvConsts.push_back(builder.makeUintConstant(zero ? 0 : consts[nextConst].getUConst()));
break;
case glslang::EbtFloat:
spvConsts.push_back(builder.makeFloatConstant(zero ? 0.0F : (float)consts[nextConst].getDConst()));
break;
case glslang::EbtBool:
spvConsts.push_back(builder.makeBoolConstant(zero ? false : consts[nextConst].getBConst()));
break;
case glslang::EbtInt8:
builder.addCapability(spv::CapabilityInt8);
spvConsts.push_back(builder.makeInt8Constant(zero ? 0 : consts[nextConst].getI8Const()));
break;
case glslang::EbtUint8:
builder.addCapability(spv::CapabilityInt8);
spvConsts.push_back(builder.makeUint8Constant(zero ? 0 : consts[nextConst].getU8Const()));
break;
case glslang::EbtInt16:
builder.addCapability(spv::CapabilityInt16);
spvConsts.push_back(builder.makeInt16Constant(zero ? 0 : consts[nextConst].getI16Const()));
break;
case glslang::EbtUint16:
builder.addCapability(spv::CapabilityInt16);
spvConsts.push_back(builder.makeUint16Constant(zero ? 0 : consts[nextConst].getU16Const()));
break;
case glslang::EbtInt64:
spvConsts.push_back(builder.makeInt64Constant(zero ? 0 : consts[nextConst].getI64Const()));
break;
case glslang::EbtUint64:
spvConsts.push_back(builder.makeUint64Constant(zero ? 0 : consts[nextConst].getU64Const()));
break;
case glslang::EbtDouble:
spvConsts.push_back(builder.makeDoubleConstant(zero ? 0.0 : consts[nextConst].getDConst()));
break;
case glslang::EbtFloat16:
builder.addCapability(spv::CapabilityFloat16);
spvConsts.push_back(builder.makeFloat16Constant(zero ? 0.0F : (float)consts[nextConst].getDConst()));
break;
default:
assert(0);
break;
}
++nextConst;
}
} else {
// we have a non-aggregate (scalar) constant
bool zero = nextConst >= consts.size();
spv::Id scalar = 0;
switch (glslangType.getBasicType()) {
case glslang::EbtInt:
scalar = builder.makeIntConstant(zero ? 0 : consts[nextConst].getIConst(), specConstant);
break;
case glslang::EbtUint:
scalar = builder.makeUintConstant(zero ? 0 : consts[nextConst].getUConst(), specConstant);
break;
case glslang::EbtFloat:
scalar = builder.makeFloatConstant(zero ? 0.0F : (float)consts[nextConst].getDConst(), specConstant);
break;
case glslang::EbtBool:
scalar = builder.makeBoolConstant(zero ? false : consts[nextConst].getBConst(), specConstant);
break;
case glslang::EbtInt8:
builder.addCapability(spv::CapabilityInt8);
scalar = builder.makeInt8Constant(zero ? 0 : consts[nextConst].getI8Const(), specConstant);
break;
case glslang::EbtUint8:
builder.addCapability(spv::CapabilityInt8);
scalar = builder.makeUint8Constant(zero ? 0 : consts[nextConst].getU8Const(), specConstant);
break;
case glslang::EbtInt16:
builder.addCapability(spv::CapabilityInt16);
scalar = builder.makeInt16Constant(zero ? 0 : consts[nextConst].getI16Const(), specConstant);
break;
case glslang::EbtUint16:
builder.addCapability(spv::CapabilityInt16);
scalar = builder.makeUint16Constant(zero ? 0 : consts[nextConst].getU16Const(), specConstant);
break;
case glslang::EbtInt64:
scalar = builder.makeInt64Constant(zero ? 0 : consts[nextConst].getI64Const(), specConstant);
break;
case glslang::EbtUint64:
scalar = builder.makeUint64Constant(zero ? 0 : consts[nextConst].getU64Const(), specConstant);
break;
case glslang::EbtDouble:
scalar = builder.makeDoubleConstant(zero ? 0.0 : consts[nextConst].getDConst(), specConstant);
break;
case glslang::EbtFloat16:
builder.addCapability(spv::CapabilityFloat16);
scalar = builder.makeFloat16Constant(zero ? 0.0F : (float)consts[nextConst].getDConst(), specConstant);
break;
case glslang::EbtReference:
scalar = builder.makeUint64Constant(zero ? 0 : consts[nextConst].getU64Const(), specConstant);
scalar = builder.createUnaryOp(spv::OpBitcast, typeId, scalar);
break;
case glslang::EbtString:
scalar = builder.getStringId(consts[nextConst].getSConst()->c_str());
break;
default:
assert(0);
break;
}
++nextConst;
return scalar;
}
return builder.makeCompositeConstant(typeId, spvConsts);
}
// Return true if the node is a constant or symbol whose reading has no
// non-trivial observable cost or effect.
bool TGlslangToSpvTraverser::isTrivialLeaf(const glslang::TIntermTyped* node)
{
// don't know what this is
if (node == nullptr)
return false;
// a constant is safe
if (node->getAsConstantUnion() != nullptr)
return true;
// not a symbol means non-trivial
if (node->getAsSymbolNode() == nullptr)
return false;
// a symbol, depends on what's being read
switch (node->getType().getQualifier().storage) {
case glslang::EvqTemporary:
case glslang::EvqGlobal:
case glslang::EvqIn:
case glslang::EvqInOut:
case glslang::EvqConst:
case glslang::EvqConstReadOnly:
case glslang::EvqUniform:
return true;
default:
return false;
}
}
// A node is trivial if it is a single operation with no side effects.
// HLSL (and/or vectors) are always trivial, as it does not short circuit.
// Otherwise, error on the side of saying non-trivial.
// Return true if trivial.
bool TGlslangToSpvTraverser::isTrivial(const glslang::TIntermTyped* node)
{
if (node == nullptr)
return false;
// count non scalars as trivial, as well as anything coming from HLSL
if (! node->getType().isScalarOrVec1() || glslangIntermediate->getSource() == glslang::EShSourceHlsl)
return true;
// symbols and constants are trivial
if (isTrivialLeaf(node))
return true;
// otherwise, it needs to be a simple operation or one or two leaf nodes
// not a simple operation
const glslang::TIntermBinary* binaryNode = node->getAsBinaryNode();
const glslang::TIntermUnary* unaryNode = node->getAsUnaryNode();
if (binaryNode == nullptr && unaryNode == nullptr)
return false;
// not on leaf nodes
if (binaryNode && (! isTrivialLeaf(binaryNode->getLeft()) || ! isTrivialLeaf(binaryNode->getRight())))
return false;
if (unaryNode && ! isTrivialLeaf(unaryNode->getOperand())) {
return false;
}
switch (node->getAsOperator()->getOp()) {
case glslang::EOpLogicalNot:
case glslang::EOpConvIntToBool:
case glslang::EOpConvUintToBool:
case glslang::EOpConvFloatToBool:
case glslang::EOpConvDoubleToBool:
case glslang::EOpEqual:
case glslang::EOpNotEqual:
case glslang::EOpLessThan:
case glslang::EOpGreaterThan:
case glslang::EOpLessThanEqual:
case glslang::EOpGreaterThanEqual:
case glslang::EOpIndexDirect:
case glslang::EOpIndexDirectStruct:
case glslang::EOpLogicalXor:
case glslang::EOpAny:
case glslang::EOpAll:
return true;
default:
return false;
}
}
// Emit short-circuiting code, where 'right' is never evaluated unless
// the left side is true (for &&) or false (for ||).
spv::Id TGlslangToSpvTraverser::createShortCircuit(glslang::TOperator op, glslang::TIntermTyped& left,
glslang::TIntermTyped& right)
{
spv::Id boolTypeId = builder.makeBoolType();
// emit left operand
builder.clearAccessChain();
left.traverse(this);
spv::Id leftId = accessChainLoad(left.getType());
// Operands to accumulate OpPhi operands
std::vector<spv::Id> phiOperands;
phiOperands.reserve(4);
// accumulate left operand's phi information
phiOperands.push_back(leftId);
phiOperands.push_back(builder.getBuildPoint()->getId());
// Make the two kinds of operation symmetric with a "!"
// || => emit "if (! left) result = right"
// && => emit "if ( left) result = right"
//
// TODO: this runtime "not" for || could be avoided by adding functionality
// to 'builder' to have an "else" without an "then"
if (op == glslang::EOpLogicalOr)
leftId = builder.createUnaryOp(spv::OpLogicalNot, boolTypeId, leftId);
// make an "if" based on the left value
spv::Builder::If ifBuilder(leftId, spv::SelectionControlMaskNone, builder);
// emit right operand as the "then" part of the "if"
builder.clearAccessChain();
right.traverse(this);
spv::Id rightId = accessChainLoad(right.getType());
// accumulate left operand's phi information
phiOperands.push_back(rightId);
phiOperands.push_back(builder.getBuildPoint()->getId());
// finish the "if"
ifBuilder.makeEndIf();
// phi together the two results
return builder.createOp(spv::OpPhi, boolTypeId, phiOperands);
}
// Return type Id of the imported set of extended instructions corresponds to the name.
// Import this set if it has not been imported yet.
spv::Id TGlslangToSpvTraverser::getExtBuiltins(const char* name)
{
if (extBuiltinMap.find(name) != extBuiltinMap.end())
return extBuiltinMap[name];
else {
spv::Id extBuiltins = builder.import(name);
extBuiltinMap[name] = extBuiltins;
return extBuiltins;
}
}
}; // end anonymous namespace
namespace glslang {
void GetSpirvVersion(std::string& version)
{
const int bufSize = 100;
char buf[bufSize];
snprintf(buf, bufSize, "0x%08x, Revision %d", spv::Version, spv::Revision);
version = buf;
}
// For low-order part of the generator's magic number. Bump up
// when there is a change in the style (e.g., if SSA form changes,
// or a different instruction sequence to do something gets used).
int GetSpirvGeneratorVersion()
{
// return 1; // start
// return 2; // EOpAtomicCounterDecrement gets a post decrement, to map between GLSL -> SPIR-V
// return 3; // change/correct barrier-instruction operands, to match memory model group decisions
// return 4; // some deeper access chains: for dynamic vector component, and local Boolean component
// return 5; // make OpArrayLength result type be an int with signedness of 0
// return 6; // revert version 5 change, which makes a different (new) kind of incorrect code,
// versions 4 and 6 each generate OpArrayLength as it has long been done
// return 7; // GLSL volatile keyword maps to both SPIR-V decorations Volatile and Coherent
// return 8; // switch to new dead block eliminator; use OpUnreachable
// return 9; // don't include opaque function parameters in OpEntryPoint global's operand list
// return 10; // Generate OpFUnordNotEqual for != comparisons
return 11; // Make OpEmitMeshTasksEXT a terminal instruction
}
// Write SPIR-V out to a binary file
bool OutputSpvBin(const std::vector<unsigned int>& spirv, const char* baseName)
{
std::ofstream out;
out.open(baseName, std::ios::binary | std::ios::out);
if (out.fail()) {
printf("ERROR: Failed to open file: %s\n", baseName);
return false;
}
for (int i = 0; i < (int)spirv.size(); ++i) {
unsigned int word = spirv[i];
out.write((const char*)&word, 4);
}
out.close();
return true;
}
// Write SPIR-V out to a text file with 32-bit hexadecimal words
bool OutputSpvHex(const std::vector<unsigned int>& spirv, const char* baseName, const char* varName)
{
std::ofstream out;
out.open(baseName, std::ios::binary | std::ios::out);
if (out.fail()) {
printf("ERROR: Failed to open file: %s\n", baseName);
return false;
}
out << "\t// " <<
GetSpirvGeneratorVersion() <<
GLSLANG_VERSION_MAJOR << "." << GLSLANG_VERSION_MINOR << "." << GLSLANG_VERSION_PATCH <<
GLSLANG_VERSION_FLAVOR << std::endl;
if (varName != nullptr) {
out << "\t #pragma once" << std::endl;
out << "const uint32_t " << varName << "[] = {" << std::endl;
}
const int WORDS_PER_LINE = 8;
for (int i = 0; i < (int)spirv.size(); i += WORDS_PER_LINE) {
out << "\t";
for (int j = 0; j < WORDS_PER_LINE && i + j < (int)spirv.size(); ++j) {
const unsigned int word = spirv[i + j];
out << "0x" << std::hex << std::setw(8) << std::setfill('0') << word;
if (i + j + 1 < (int)spirv.size()) {
out << ",";
}
}
out << std::endl;
}
if (varName != nullptr) {
out << "};";
out << std::endl;
}
out.close();
return true;
}
//
// Set up the glslang traversal
//
void GlslangToSpv(const TIntermediate& intermediate, std::vector<unsigned int>& spirv, SpvOptions* options)
{
spv::SpvBuildLogger logger;
GlslangToSpv(intermediate, spirv, &logger, options);
}
void GlslangToSpv(const TIntermediate& intermediate, std::vector<unsigned int>& spirv,
spv::SpvBuildLogger* logger, SpvOptions* options)
{
TIntermNode* root = intermediate.getTreeRoot();
if (root == nullptr)
return;
SpvOptions defaultOptions;
if (options == nullptr)
options = &defaultOptions;
GetThreadPoolAllocator().push();
TGlslangToSpvTraverser it(intermediate.getSpv().spv, &intermediate, logger, *options);
root->traverse(&it);
it.finishSpv(options->compileOnly);
it.dumpSpv(spirv);
#if ENABLE_OPT
// If from HLSL, run spirv-opt to "legalize" the SPIR-V for Vulkan
// eg. forward and remove memory writes of opaque types.
bool prelegalization = intermediate.getSource() == EShSourceHlsl;
if ((prelegalization || options->optimizeSize) && !options->disableOptimizer) {
SpirvToolsTransform(intermediate, spirv, logger, options);
prelegalization = false;
}
else if (options->stripDebugInfo) {
// Strip debug info even if optimization is disabled.
SpirvToolsStripDebugInfo(intermediate, spirv, logger);
}
if (options->validate)
SpirvToolsValidate(intermediate, spirv, logger, prelegalization);
if (options->disassemble)
SpirvToolsDisassemble(std::cout, spirv);
#endif
GetThreadPoolAllocator().pop();
}
}; // end namespace glslang
|
0 | repos/glslang.zig/glslang | repos/glslang.zig/glslang/SPIRV/hex_float.h | // Copyright (c) 2015-2016 The Khronos Group Inc.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
#ifndef LIBSPIRV_UTIL_HEX_FLOAT_H_
#define LIBSPIRV_UTIL_HEX_FLOAT_H_
#include <cassert>
#include <cctype>
#include <cmath>
#include <cstdint>
#include <iomanip>
#include <limits>
#include <sstream>
#include "bitutils.h"
namespace spvutils {
class Float16 {
public:
Float16(uint16_t v) : val(v) {}
Float16() {}
static bool isNan(const Float16& val) {
return ((val.val & 0x7C00) == 0x7C00) && ((val.val & 0x3FF) != 0);
}
// Returns true if the given value is any kind of infinity.
static bool isInfinity(const Float16& val) {
return ((val.val & 0x7C00) == 0x7C00) && ((val.val & 0x3FF) == 0);
}
Float16(const Float16& other) { val = other.val; }
uint16_t get_value() const { return val; }
// Returns the maximum normal value.
static Float16 max() { return Float16(0x7bff); }
// Returns the lowest normal value.
static Float16 lowest() { return Float16(0xfbff); }
private:
uint16_t val;
};
// To specialize this type, you must override uint_type to define
// an unsigned integer that can fit your floating point type.
// You must also add a isNan function that returns true if
// a value is Nan.
template <typename T>
struct FloatProxyTraits {
typedef void uint_type;
};
template <>
struct FloatProxyTraits<float> {
typedef uint32_t uint_type;
static bool isNan(float f) { return std::isnan(f); }
// Returns true if the given value is any kind of infinity.
static bool isInfinity(float f) { return std::isinf(f); }
// Returns the maximum normal value.
static float max() { return std::numeric_limits<float>::max(); }
// Returns the lowest normal value.
static float lowest() { return std::numeric_limits<float>::lowest(); }
};
template <>
struct FloatProxyTraits<double> {
typedef uint64_t uint_type;
static bool isNan(double f) { return std::isnan(f); }
// Returns true if the given value is any kind of infinity.
static bool isInfinity(double f) { return std::isinf(f); }
// Returns the maximum normal value.
static double max() { return std::numeric_limits<double>::max(); }
// Returns the lowest normal value.
static double lowest() { return std::numeric_limits<double>::lowest(); }
};
template <>
struct FloatProxyTraits<Float16> {
typedef uint16_t uint_type;
static bool isNan(Float16 f) { return Float16::isNan(f); }
// Returns true if the given value is any kind of infinity.
static bool isInfinity(Float16 f) { return Float16::isInfinity(f); }
// Returns the maximum normal value.
static Float16 max() { return Float16::max(); }
// Returns the lowest normal value.
static Float16 lowest() { return Float16::lowest(); }
};
// Since copying a floating point number (especially if it is NaN)
// does not guarantee that bits are preserved, this class lets us
// store the type and use it as a float when necessary.
template <typename T>
class FloatProxy {
public:
typedef typename FloatProxyTraits<T>::uint_type uint_type;
// Since this is to act similar to the normal floats,
// do not initialize the data by default.
FloatProxy() {}
// Intentionally non-explicit. This is a proxy type so
// implicit conversions allow us to use it more transparently.
FloatProxy(T val) { data_ = BitwiseCast<uint_type>(val); }
// Intentionally non-explicit. This is a proxy type so
// implicit conversions allow us to use it more transparently.
FloatProxy(uint_type val) { data_ = val; }
// This is helpful to have and is guaranteed not to stomp bits.
FloatProxy<T> operator-() const {
return static_cast<uint_type>(data_ ^
(uint_type(0x1) << (sizeof(T) * 8 - 1)));
}
// Returns the data as a floating point value.
T getAsFloat() const { return BitwiseCast<T>(data_); }
// Returns the raw data.
uint_type data() const { return data_; }
// Returns true if the value represents any type of NaN.
bool isNan() { return FloatProxyTraits<T>::isNan(getAsFloat()); }
// Returns true if the value represents any type of infinity.
bool isInfinity() { return FloatProxyTraits<T>::isInfinity(getAsFloat()); }
// Returns the maximum normal value.
static FloatProxy<T> max() {
return FloatProxy<T>(FloatProxyTraits<T>::max());
}
// Returns the lowest normal value.
static FloatProxy<T> lowest() {
return FloatProxy<T>(FloatProxyTraits<T>::lowest());
}
private:
uint_type data_;
};
template <typename T>
bool operator==(const FloatProxy<T>& first, const FloatProxy<T>& second) {
return first.data() == second.data();
}
// Reads a FloatProxy value as a normal float from a stream.
template <typename T>
std::istream& operator>>(std::istream& is, FloatProxy<T>& value) {
T float_val;
is >> float_val;
value = FloatProxy<T>(float_val);
return is;
}
// This is an example traits. It is not meant to be used in practice, but will
// be the default for any non-specialized type.
template <typename T>
struct HexFloatTraits {
// Integer type that can store this hex-float.
typedef void uint_type;
// Signed integer type that can store this hex-float.
typedef void int_type;
// The numerical type that this HexFloat represents.
typedef void underlying_type;
// The type needed to construct the underlying type.
typedef void native_type;
// The number of bits that are actually relevant in the uint_type.
// This allows us to deal with, for example, 24-bit values in a 32-bit
// integer.
static const uint32_t num_used_bits = 0;
// Number of bits that represent the exponent.
static const uint32_t num_exponent_bits = 0;
// Number of bits that represent the fractional part.
static const uint32_t num_fraction_bits = 0;
// The bias of the exponent. (How much we need to subtract from the stored
// value to get the correct value.)
static const uint32_t exponent_bias = 0;
};
// Traits for IEEE float.
// 1 sign bit, 8 exponent bits, 23 fractional bits.
template <>
struct HexFloatTraits<FloatProxy<float>> {
typedef uint32_t uint_type;
typedef int32_t int_type;
typedef FloatProxy<float> underlying_type;
typedef float native_type;
static const uint_type num_used_bits = 32;
static const uint_type num_exponent_bits = 8;
static const uint_type num_fraction_bits = 23;
static const uint_type exponent_bias = 127;
};
// Traits for IEEE double.
// 1 sign bit, 11 exponent bits, 52 fractional bits.
template <>
struct HexFloatTraits<FloatProxy<double>> {
typedef uint64_t uint_type;
typedef int64_t int_type;
typedef FloatProxy<double> underlying_type;
typedef double native_type;
static const uint_type num_used_bits = 64;
static const uint_type num_exponent_bits = 11;
static const uint_type num_fraction_bits = 52;
static const uint_type exponent_bias = 1023;
};
// Traits for IEEE half.
// 1 sign bit, 5 exponent bits, 10 fractional bits.
template <>
struct HexFloatTraits<FloatProxy<Float16>> {
typedef uint16_t uint_type;
typedef int16_t int_type;
typedef uint16_t underlying_type;
typedef uint16_t native_type;
static const uint_type num_used_bits = 16;
static const uint_type num_exponent_bits = 5;
static const uint_type num_fraction_bits = 10;
static const uint_type exponent_bias = 15;
};
enum round_direction {
kRoundToZero,
kRoundToNearestEven,
kRoundToPositiveInfinity,
kRoundToNegativeInfinity
};
// Template class that houses a floating pointer number.
// It exposes a number of constants based on the provided traits to
// assist in interpreting the bits of the value.
template <typename T, typename Traits = HexFloatTraits<T>>
class HexFloat {
public:
typedef typename Traits::uint_type uint_type;
typedef typename Traits::int_type int_type;
typedef typename Traits::underlying_type underlying_type;
typedef typename Traits::native_type native_type;
explicit HexFloat(T f) : value_(f) {}
T value() const { return value_; }
void set_value(T f) { value_ = f; }
// These are all written like this because it is convenient to have
// compile-time constants for all of these values.
// Pass-through values to save typing.
static const uint32_t num_used_bits = Traits::num_used_bits;
static const uint32_t exponent_bias = Traits::exponent_bias;
static const uint32_t num_exponent_bits = Traits::num_exponent_bits;
static const uint32_t num_fraction_bits = Traits::num_fraction_bits;
// Number of bits to shift left to set the highest relevant bit.
static const uint32_t top_bit_left_shift = num_used_bits - 1;
// How many nibbles (hex characters) the fractional part takes up.
static const uint32_t fraction_nibbles = (num_fraction_bits + 3) / 4;
// If the fractional part does not fit evenly into a hex character (4-bits)
// then we have to left-shift to get rid of leading 0s. This is the amount
// we have to shift (might be 0).
static const uint32_t num_overflow_bits =
fraction_nibbles * 4 - num_fraction_bits;
// The representation of the fraction, not the actual bits. This
// includes the leading bit that is usually implicit.
static const uint_type fraction_represent_mask =
spvutils::SetBits<uint_type, 0,
num_fraction_bits + num_overflow_bits>::get;
// The topmost bit in the nibble-aligned fraction.
static const uint_type fraction_top_bit =
uint_type(1) << (num_fraction_bits + num_overflow_bits - 1);
// The least significant bit in the exponent, which is also the bit
// immediately to the left of the significand.
static const uint_type first_exponent_bit = uint_type(1)
<< (num_fraction_bits);
// The mask for the encoded fraction. It does not include the
// implicit bit.
static const uint_type fraction_encode_mask =
spvutils::SetBits<uint_type, 0, num_fraction_bits>::get;
// The bit that is used as a sign.
static const uint_type sign_mask = uint_type(1) << top_bit_left_shift;
// The bits that represent the exponent.
static const uint_type exponent_mask =
spvutils::SetBits<uint_type, num_fraction_bits, num_exponent_bits>::get;
// How far left the exponent is shifted.
static const uint32_t exponent_left_shift = num_fraction_bits;
// How far from the right edge the fraction is shifted.
static const uint32_t fraction_right_shift =
static_cast<uint32_t>(sizeof(uint_type) * 8) - num_fraction_bits;
// The maximum representable unbiased exponent.
static const int_type max_exponent =
(exponent_mask >> num_fraction_bits) - exponent_bias;
// The minimum representable exponent for normalized numbers.
static const int_type min_exponent = -static_cast<int_type>(exponent_bias);
// Returns the bits associated with the value.
uint_type getBits() const { return spvutils::BitwiseCast<uint_type>(value_); }
// Returns the bits associated with the value, without the leading sign bit.
uint_type getUnsignedBits() const {
return static_cast<uint_type>(spvutils::BitwiseCast<uint_type>(value_) &
~sign_mask);
}
// Returns the bits associated with the exponent, shifted to start at the
// lsb of the type.
const uint_type getExponentBits() const {
return static_cast<uint_type>((getBits() & exponent_mask) >>
num_fraction_bits);
}
// Returns the exponent in unbiased form. This is the exponent in the
// human-friendly form.
const int_type getUnbiasedExponent() const {
return static_cast<int_type>(getExponentBits() - exponent_bias);
}
// Returns just the significand bits from the value.
const uint_type getSignificandBits() const {
return getBits() & fraction_encode_mask;
}
// If the number was normalized, returns the unbiased exponent.
// If the number was denormal, normalize the exponent first.
const int_type getUnbiasedNormalizedExponent() const {
if ((getBits() & ~sign_mask) == 0) { // special case if everything is 0
return 0;
}
int_type exp = getUnbiasedExponent();
if (exp == min_exponent) { // We are in denorm land.
uint_type significand_bits = getSignificandBits();
while ((significand_bits & (first_exponent_bit >> 1)) == 0) {
significand_bits = static_cast<uint_type>(significand_bits << 1);
exp = static_cast<int_type>(exp - 1);
}
significand_bits &= fraction_encode_mask;
}
return exp;
}
// Returns the signficand after it has been normalized.
const uint_type getNormalizedSignificand() const {
int_type unbiased_exponent = getUnbiasedNormalizedExponent();
uint_type significand = getSignificandBits();
for (int_type i = unbiased_exponent; i <= min_exponent; ++i) {
significand = static_cast<uint_type>(significand << 1);
}
significand &= fraction_encode_mask;
return significand;
}
// Returns true if this number represents a negative value.
bool isNegative() const { return (getBits() & sign_mask) != 0; }
// Sets this HexFloat from the individual components.
// Note this assumes EVERY significand is normalized, and has an implicit
// leading one. This means that the only way that this method will set 0,
// is if you set a number so denormalized that it underflows.
// Do not use this method with raw bits extracted from a subnormal number,
// since subnormals do not have an implicit leading 1 in the significand.
// The significand is also expected to be in the
// lowest-most num_fraction_bits of the uint_type.
// The exponent is expected to be unbiased, meaning an exponent of
// 0 actually means 0.
// If underflow_round_up is set, then on underflow, if a number is non-0
// and would underflow, we round up to the smallest denorm.
void setFromSignUnbiasedExponentAndNormalizedSignificand(
bool negative, int_type exponent, uint_type significand,
bool round_denorm_up) {
bool significand_is_zero = significand == 0;
if (exponent <= min_exponent) {
// If this was denormalized, then we have to shift the bit on, meaning
// the significand is not zero.
significand_is_zero = false;
significand |= first_exponent_bit;
significand = static_cast<uint_type>(significand >> 1);
}
while (exponent < min_exponent) {
significand = static_cast<uint_type>(significand >> 1);
++exponent;
}
if (exponent == min_exponent) {
if (significand == 0 && !significand_is_zero && round_denorm_up) {
significand = static_cast<uint_type>(0x1);
}
}
uint_type new_value = 0;
if (negative) {
new_value = static_cast<uint_type>(new_value | sign_mask);
}
exponent = static_cast<int_type>(exponent + exponent_bias);
assert(exponent >= 0);
// put it all together
exponent = static_cast<uint_type>((exponent << exponent_left_shift) &
exponent_mask);
significand = static_cast<uint_type>(significand & fraction_encode_mask);
new_value = static_cast<uint_type>(new_value | (exponent | significand));
value_ = BitwiseCast<T>(new_value);
}
// Increments the significand of this number by the given amount.
// If this would spill the significand into the implicit bit,
// carry is set to true and the significand is shifted to fit into
// the correct location, otherwise carry is set to false.
// All significands and to_increment are assumed to be within the bounds
// for a valid significand.
static uint_type incrementSignificand(uint_type significand,
uint_type to_increment, bool* carry) {
significand = static_cast<uint_type>(significand + to_increment);
*carry = false;
if (significand & first_exponent_bit) {
*carry = true;
// The implicit 1-bit will have carried, so we should zero-out the
// top bit and shift back.
significand = static_cast<uint_type>(significand & ~first_exponent_bit);
significand = static_cast<uint_type>(significand >> 1);
}
return significand;
}
// These exist because MSVC throws warnings on negative right-shifts
// even if they are not going to be executed. Eg:
// constant_number < 0? 0: constant_number
// These convert the negative left-shifts into right shifts.
template <typename int_type>
uint_type negatable_left_shift(int_type N, uint_type val)
{
if(N >= 0)
return val << N;
return val >> -N;
}
template <typename int_type>
uint_type negatable_right_shift(int_type N, uint_type val)
{
if(N >= 0)
return val >> N;
return val << -N;
}
// Returns the significand, rounded to fit in a significand in
// other_T. This is shifted so that the most significant
// bit of the rounded number lines up with the most significant bit
// of the returned significand.
template <typename other_T>
typename other_T::uint_type getRoundedNormalizedSignificand(
round_direction dir, bool* carry_bit) {
typedef typename other_T::uint_type other_uint_type;
static const int_type num_throwaway_bits =
static_cast<int_type>(num_fraction_bits) -
static_cast<int_type>(other_T::num_fraction_bits);
static const uint_type last_significant_bit =
(num_throwaway_bits < 0)
? 0
: negatable_left_shift(num_throwaway_bits, 1u);
static const uint_type first_rounded_bit =
(num_throwaway_bits < 1)
? 0
: negatable_left_shift(num_throwaway_bits - 1, 1u);
static const uint_type throwaway_mask_bits =
num_throwaway_bits > 0 ? num_throwaway_bits : 0;
static const uint_type throwaway_mask =
spvutils::SetBits<uint_type, 0, throwaway_mask_bits>::get;
*carry_bit = false;
other_uint_type out_val = 0;
uint_type significand = getNormalizedSignificand();
// If we are up-casting, then we just have to shift to the right location.
if (num_throwaway_bits <= 0) {
out_val = static_cast<other_uint_type>(significand);
uint_type shift_amount = static_cast<uint_type>(-num_throwaway_bits);
out_val = static_cast<other_uint_type>(out_val << shift_amount);
return out_val;
}
// If every non-representable bit is 0, then we don't have any casting to
// do.
if ((significand & throwaway_mask) == 0) {
return static_cast<other_uint_type>(
negatable_right_shift(num_throwaway_bits, significand));
}
bool round_away_from_zero = false;
// We actually have to narrow the significand here, so we have to follow the
// rounding rules.
switch (dir) {
case kRoundToZero:
break;
case kRoundToPositiveInfinity:
round_away_from_zero = !isNegative();
break;
case kRoundToNegativeInfinity:
round_away_from_zero = isNegative();
break;
case kRoundToNearestEven:
// Have to round down, round bit is 0
if ((first_rounded_bit & significand) == 0) {
break;
}
if (((significand & throwaway_mask) & ~first_rounded_bit) != 0) {
// If any subsequent bit of the rounded portion is non-0 then we round
// up.
round_away_from_zero = true;
break;
}
// We are exactly half-way between 2 numbers, pick even.
if ((significand & last_significant_bit) != 0) {
// 1 for our last bit, round up.
round_away_from_zero = true;
break;
}
break;
}
if (round_away_from_zero) {
return static_cast<other_uint_type>(
negatable_right_shift(num_throwaway_bits, incrementSignificand(
significand, last_significant_bit, carry_bit)));
} else {
return static_cast<other_uint_type>(
negatable_right_shift(num_throwaway_bits, significand));
}
}
// Casts this value to another HexFloat. If the cast is widening,
// then round_dir is ignored. If the cast is narrowing, then
// the result is rounded in the direction specified.
// This number will retain Nan and Inf values.
// It will also saturate to Inf if the number overflows, and
// underflow to (0 or min depending on rounding) if the number underflows.
template <typename other_T>
void castTo(other_T& other, round_direction round_dir) {
other = other_T(static_cast<typename other_T::native_type>(0));
bool negate = isNegative();
if (getUnsignedBits() == 0) {
if (negate) {
other.set_value(-other.value());
}
return;
}
uint_type significand = getSignificandBits();
bool carried = false;
typename other_T::uint_type rounded_significand =
getRoundedNormalizedSignificand<other_T>(round_dir, &carried);
int_type exponent = getUnbiasedExponent();
if (exponent == min_exponent) {
// If we are denormal, normalize the exponent, so that we can encode
// easily.
exponent = static_cast<int_type>(exponent + 1);
for (uint_type check_bit = first_exponent_bit >> 1; check_bit != 0;
check_bit = static_cast<uint_type>(check_bit >> 1)) {
exponent = static_cast<int_type>(exponent - 1);
if (check_bit & significand) break;
}
}
bool is_nan =
(getBits() & exponent_mask) == exponent_mask && significand != 0;
bool is_inf =
!is_nan &&
((exponent + carried) > static_cast<int_type>(other_T::exponent_bias) ||
(significand == 0 && (getBits() & exponent_mask) == exponent_mask));
// If we are Nan or Inf we should pass that through.
if (is_inf) {
other.set_value(BitwiseCast<typename other_T::underlying_type>(
static_cast<typename other_T::uint_type>(
(negate ? other_T::sign_mask : 0) | other_T::exponent_mask)));
return;
}
if (is_nan) {
typename other_T::uint_type shifted_significand;
shifted_significand = static_cast<typename other_T::uint_type>(
negatable_left_shift(
static_cast<int_type>(other_T::num_fraction_bits) -
static_cast<int_type>(num_fraction_bits), significand));
// We are some sort of Nan. We try to keep the bit-pattern of the Nan
// as close as possible. If we had to shift off bits so we are 0, then we
// just set the last bit.
other.set_value(BitwiseCast<typename other_T::underlying_type>(
static_cast<typename other_T::uint_type>(
(negate ? other_T::sign_mask : 0) | other_T::exponent_mask |
(shifted_significand == 0 ? 0x1 : shifted_significand))));
return;
}
bool round_underflow_up =
isNegative() ? round_dir == kRoundToNegativeInfinity
: round_dir == kRoundToPositiveInfinity;
typedef typename other_T::int_type other_int_type;
// setFromSignUnbiasedExponentAndNormalizedSignificand will
// zero out any underflowing value (but retain the sign).
other.setFromSignUnbiasedExponentAndNormalizedSignificand(
negate, static_cast<other_int_type>(exponent), rounded_significand,
round_underflow_up);
return;
}
private:
T value_;
static_assert(num_used_bits ==
Traits::num_exponent_bits + Traits::num_fraction_bits + 1,
"The number of bits do not fit");
static_assert(sizeof(T) == sizeof(uint_type), "The type sizes do not match");
};
// Returns 4 bits represented by the hex character.
inline uint8_t get_nibble_from_character(int character) {
const char* dec = "0123456789";
const char* lower = "abcdef";
const char* upper = "ABCDEF";
const char* p = nullptr;
if ((p = strchr(dec, character))) {
return static_cast<uint8_t>(p - dec);
} else if ((p = strchr(lower, character))) {
return static_cast<uint8_t>(p - lower + 0xa);
} else if ((p = strchr(upper, character))) {
return static_cast<uint8_t>(p - upper + 0xa);
}
assert(false && "This was called with a non-hex character");
return 0;
}
// Outputs the given HexFloat to the stream.
template <typename T, typename Traits>
std::ostream& operator<<(std::ostream& os, const HexFloat<T, Traits>& value) {
typedef HexFloat<T, Traits> HF;
typedef typename HF::uint_type uint_type;
typedef typename HF::int_type int_type;
static_assert(HF::num_used_bits != 0,
"num_used_bits must be non-zero for a valid float");
static_assert(HF::num_exponent_bits != 0,
"num_exponent_bits must be non-zero for a valid float");
static_assert(HF::num_fraction_bits != 0,
"num_fractin_bits must be non-zero for a valid float");
const uint_type bits = spvutils::BitwiseCast<uint_type>(value.value());
const char* const sign = (bits & HF::sign_mask) ? "-" : "";
const uint_type exponent = static_cast<uint_type>(
(bits & HF::exponent_mask) >> HF::num_fraction_bits);
uint_type fraction = static_cast<uint_type>((bits & HF::fraction_encode_mask)
<< HF::num_overflow_bits);
const bool is_zero = exponent == 0 && fraction == 0;
const bool is_denorm = exponent == 0 && !is_zero;
// exponent contains the biased exponent we have to convert it back into
// the normal range.
int_type int_exponent = static_cast<int_type>(exponent - HF::exponent_bias);
// If the number is all zeros, then we actually have to NOT shift the
// exponent.
int_exponent = is_zero ? 0 : int_exponent;
// If we are denorm, then start shifting, and decreasing the exponent until
// our leading bit is 1.
if (is_denorm) {
while ((fraction & HF::fraction_top_bit) == 0) {
fraction = static_cast<uint_type>(fraction << 1);
int_exponent = static_cast<int_type>(int_exponent - 1);
}
// Since this is denormalized, we have to consume the leading 1 since it
// will end up being implicit.
fraction = static_cast<uint_type>(fraction << 1); // eat the leading 1
fraction &= HF::fraction_represent_mask;
}
uint_type fraction_nibbles = HF::fraction_nibbles;
// We do not have to display any trailing 0s, since this represents the
// fractional part.
while (fraction_nibbles > 0 && (fraction & 0xF) == 0) {
// Shift off any trailing values;
fraction = static_cast<uint_type>(fraction >> 4);
--fraction_nibbles;
}
const auto saved_flags = os.flags();
const auto saved_fill = os.fill();
os << sign << "0x" << (is_zero ? '0' : '1');
if (fraction_nibbles) {
// Make sure to keep the leading 0s in place, since this is the fractional
// part.
os << "." << std::setw(static_cast<int>(fraction_nibbles))
<< std::setfill('0') << std::hex << fraction;
}
os << "p" << std::dec << (int_exponent >= 0 ? "+" : "") << int_exponent;
os.flags(saved_flags);
os.fill(saved_fill);
return os;
}
// Returns true if negate_value is true and the next character on the
// input stream is a plus or minus sign. In that case we also set the fail bit
// on the stream and set the value to the zero value for its type.
template <typename T, typename Traits>
inline bool RejectParseDueToLeadingSign(std::istream& is, bool negate_value,
HexFloat<T, Traits>& value) {
if (negate_value) {
auto next_char = is.peek();
if (next_char == '-' || next_char == '+') {
// Fail the parse. Emulate standard behaviour by setting the value to
// the zero value, and set the fail bit on the stream.
value = HexFloat<T, Traits>(typename HexFloat<T, Traits>::uint_type(0));
is.setstate(std::ios_base::failbit);
return true;
}
}
return false;
}
// Parses a floating point number from the given stream and stores it into the
// value parameter.
// If negate_value is true then the number may not have a leading minus or
// plus, and if it successfully parses, then the number is negated before
// being stored into the value parameter.
// If the value cannot be correctly parsed or overflows the target floating
// point type, then set the fail bit on the stream.
// TODO(dneto): Promise C++11 standard behavior in how the value is set in
// the error case, but only after all target platforms implement it correctly.
// In particular, the Microsoft C++ runtime appears to be out of spec.
template <typename T, typename Traits>
inline std::istream& ParseNormalFloat(std::istream& is, bool negate_value,
HexFloat<T, Traits>& value) {
if (RejectParseDueToLeadingSign(is, negate_value, value)) {
return is;
}
T val;
is >> val;
if (negate_value) {
val = -val;
}
value.set_value(val);
// In the failure case, map -0.0 to 0.0.
if (is.fail() && value.getUnsignedBits() == 0u) {
value = HexFloat<T, Traits>(typename HexFloat<T, Traits>::uint_type(0));
}
if (val.isInfinity()) {
// Fail the parse. Emulate standard behaviour by setting the value to
// the closest normal value, and set the fail bit on the stream.
value.set_value((value.isNegative() || negate_value) ? T::lowest()
: T::max());
is.setstate(std::ios_base::failbit);
}
return is;
}
// Specialization of ParseNormalFloat for FloatProxy<Float16> values.
// This will parse the float as it were a 32-bit floating point number,
// and then round it down to fit into a Float16 value.
// The number is rounded towards zero.
// If negate_value is true then the number may not have a leading minus or
// plus, and if it successfully parses, then the number is negated before
// being stored into the value parameter.
// If the value cannot be correctly parsed or overflows the target floating
// point type, then set the fail bit on the stream.
// TODO(dneto): Promise C++11 standard behavior in how the value is set in
// the error case, but only after all target platforms implement it correctly.
// In particular, the Microsoft C++ runtime appears to be out of spec.
template <>
inline std::istream&
ParseNormalFloat<FloatProxy<Float16>, HexFloatTraits<FloatProxy<Float16>>>(
std::istream& is, bool negate_value,
HexFloat<FloatProxy<Float16>, HexFloatTraits<FloatProxy<Float16>>>& value) {
// First parse as a 32-bit float.
HexFloat<FloatProxy<float>> float_val(0.0f);
ParseNormalFloat(is, negate_value, float_val);
// Then convert to 16-bit float, saturating at infinities, and
// rounding toward zero.
float_val.castTo(value, kRoundToZero);
// Overflow on 16-bit behaves the same as for 32- and 64-bit: set the
// fail bit and set the lowest or highest value.
if (Float16::isInfinity(value.value().getAsFloat())) {
value.set_value(value.isNegative() ? Float16::lowest() : Float16::max());
is.setstate(std::ios_base::failbit);
}
return is;
}
// Reads a HexFloat from the given stream.
// If the float is not encoded as a hex-float then it will be parsed
// as a regular float.
// This may fail if your stream does not support at least one unget.
// Nan values can be encoded with "0x1.<not zero>p+exponent_bias".
// This would normally overflow a float and round to
// infinity but this special pattern is the exact representation for a NaN,
// and therefore is actually encoded as the correct NaN. To encode inf,
// either 0x0p+exponent_bias can be specified or any exponent greater than
// exponent_bias.
// Examples using IEEE 32-bit float encoding.
// 0x1.0p+128 (+inf)
// -0x1.0p-128 (-inf)
//
// 0x1.1p+128 (+Nan)
// -0x1.1p+128 (-Nan)
//
// 0x1p+129 (+inf)
// -0x1p+129 (-inf)
template <typename T, typename Traits>
std::istream& operator>>(std::istream& is, HexFloat<T, Traits>& value) {
using HF = HexFloat<T, Traits>;
using uint_type = typename HF::uint_type;
using int_type = typename HF::int_type;
value.set_value(static_cast<typename HF::native_type>(0.f));
if (is.flags() & std::ios::skipws) {
// If the user wants to skip whitespace , then we should obey that.
while (std::isspace(is.peek())) {
is.get();
}
}
auto next_char = is.peek();
bool negate_value = false;
if (next_char != '-' && next_char != '0') {
return ParseNormalFloat(is, negate_value, value);
}
if (next_char == '-') {
negate_value = true;
is.get();
next_char = is.peek();
}
if (next_char == '0') {
is.get(); // We may have to unget this.
auto maybe_hex_start = is.peek();
if (maybe_hex_start != 'x' && maybe_hex_start != 'X') {
is.unget();
return ParseNormalFloat(is, negate_value, value);
} else {
is.get(); // Throw away the 'x';
}
} else {
return ParseNormalFloat(is, negate_value, value);
}
// This "looks" like a hex-float so treat it as one.
bool seen_p = false;
bool seen_dot = false;
uint_type fraction_index = 0;
uint_type fraction = 0;
int_type exponent = HF::exponent_bias;
// Strip off leading zeros so we don't have to special-case them later.
while ((next_char = is.peek()) == '0') {
is.get();
}
bool is_denorm =
true; // Assume denorm "representation" until we hear otherwise.
// NB: This does not mean the value is actually denorm,
// it just means that it was written 0.
bool bits_written = false; // Stays false until we write a bit.
while (!seen_p && !seen_dot) {
// Handle characters that are left of the fractional part.
if (next_char == '.') {
seen_dot = true;
} else if (next_char == 'p') {
seen_p = true;
} else if (::isxdigit(next_char)) {
// We know this is not denormalized since we have stripped all leading
// zeroes and we are not a ".".
is_denorm = false;
int number = get_nibble_from_character(next_char);
for (int i = 0; i < 4; ++i, number <<= 1) {
uint_type write_bit = (number & 0x8) ? 0x1 : 0x0;
if (bits_written) {
// If we are here the bits represented belong in the fractional
// part of the float, and we have to adjust the exponent accordingly.
fraction = static_cast<uint_type>(
fraction |
static_cast<uint_type>(
write_bit << (HF::top_bit_left_shift - fraction_index++)));
exponent = static_cast<int_type>(exponent + 1);
}
bits_written |= write_bit != 0;
}
} else {
// We have not found our exponent yet, so we have to fail.
is.setstate(std::ios::failbit);
return is;
}
is.get();
next_char = is.peek();
}
bits_written = false;
while (seen_dot && !seen_p) {
// Handle only fractional parts now.
if (next_char == 'p') {
seen_p = true;
} else if (::isxdigit(next_char)) {
int number = get_nibble_from_character(next_char);
for (int i = 0; i < 4; ++i, number <<= 1) {
uint_type write_bit = (number & 0x8) ? 0x01 : 0x00;
bits_written |= write_bit != 0;
if (is_denorm && !bits_written) {
// Handle modifying the exponent here this way we can handle
// an arbitrary number of hex values without overflowing our
// integer.
exponent = static_cast<int_type>(exponent - 1);
} else {
fraction = static_cast<uint_type>(
fraction |
static_cast<uint_type>(
write_bit << (HF::top_bit_left_shift - fraction_index++)));
}
}
} else {
// We still have not found our 'p' exponent yet, so this is not a valid
// hex-float.
is.setstate(std::ios::failbit);
return is;
}
is.get();
next_char = is.peek();
}
bool seen_sign = false;
int8_t exponent_sign = 1;
int_type written_exponent = 0;
while (true) {
if ((next_char == '-' || next_char == '+')) {
if (seen_sign) {
is.setstate(std::ios::failbit);
return is;
}
seen_sign = true;
exponent_sign = (next_char == '-') ? -1 : 1;
} else if (::isdigit(next_char)) {
// Hex-floats express their exponent as decimal.
written_exponent = static_cast<int_type>(written_exponent * 10);
written_exponent =
static_cast<int_type>(written_exponent + (next_char - '0'));
} else {
break;
}
is.get();
next_char = is.peek();
}
written_exponent = static_cast<int_type>(written_exponent * exponent_sign);
exponent = static_cast<int_type>(exponent + written_exponent);
bool is_zero = is_denorm && (fraction == 0);
if (is_denorm && !is_zero) {
fraction = static_cast<uint_type>(fraction << 1);
exponent = static_cast<int_type>(exponent - 1);
} else if (is_zero) {
exponent = 0;
}
if (exponent <= 0 && !is_zero) {
fraction = static_cast<uint_type>(fraction >> 1);
fraction |= static_cast<uint_type>(1) << HF::top_bit_left_shift;
}
fraction = (fraction >> HF::fraction_right_shift) & HF::fraction_encode_mask;
const int_type max_exponent =
SetBits<uint_type, 0, HF::num_exponent_bits>::get;
// Handle actual denorm numbers
while (exponent < 0 && !is_zero) {
fraction = static_cast<uint_type>(fraction >> 1);
exponent = static_cast<int_type>(exponent + 1);
fraction &= HF::fraction_encode_mask;
if (fraction == 0) {
// We have underflowed our fraction. We should clamp to zero.
is_zero = true;
exponent = 0;
}
}
// We have overflowed so we should be inf/-inf.
if (exponent > max_exponent) {
exponent = max_exponent;
fraction = 0;
}
uint_type output_bits = static_cast<uint_type>(
static_cast<uint_type>(negate_value ? 1 : 0) << HF::top_bit_left_shift);
output_bits |= fraction;
uint_type shifted_exponent = static_cast<uint_type>(
static_cast<uint_type>(exponent << HF::exponent_left_shift) &
HF::exponent_mask);
output_bits |= shifted_exponent;
T output_float = spvutils::BitwiseCast<T>(output_bits);
value.set_value(output_float);
return is;
}
// Writes a FloatProxy value to a stream.
// Zero and normal numbers are printed in the usual notation, but with
// enough digits to fully reproduce the value. Other values (subnormal,
// NaN, and infinity) are printed as a hex float.
template <typename T>
std::ostream& operator<<(std::ostream& os, const FloatProxy<T>& value) {
auto float_val = value.getAsFloat();
switch (std::fpclassify(float_val)) {
case FP_ZERO:
case FP_NORMAL: {
auto saved_precision = os.precision();
os.precision(std::numeric_limits<T>::digits10);
os << float_val;
os.precision(saved_precision);
} break;
default:
os << HexFloat<FloatProxy<T>>(value);
break;
}
return os;
}
template <>
inline std::ostream& operator<<<Float16>(std::ostream& os,
const FloatProxy<Float16>& value) {
os << HexFloat<FloatProxy<Float16>>(value);
return os;
}
}
#endif // LIBSPIRV_UTIL_HEX_FLOAT_H_
|
0 | repos/glslang.zig/glslang | repos/glslang.zig/glslang/SPIRV/GlslangToSpv.h | //
// Copyright (C) 2014 LunarG, Inc.
// Copyright (C) 2015-2018 Google, Inc.
//
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
//
// Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
//
// Redistributions in binary form must reproduce the above
// copyright notice, this list of conditions and the following
// disclaimer in the documentation and/or other materials provided
// with the distribution.
//
// Neither the name of 3Dlabs Inc. Ltd. nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
// "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
// LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
// FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
// COPYRIGHT HOLDERS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
// INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
// BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
// LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
// CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
// LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
// ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
// POSSIBILITY OF SUCH DAMAGE.
#pragma once
#include <string>
#include <vector>
#include "Logger.h"
namespace glslang {
class TIntermediate;
struct SpvOptions {
bool generateDebugInfo {false};
bool stripDebugInfo {false};
bool disableOptimizer {true};
bool optimizeSize {false};
bool disassemble {false};
bool validate {false};
bool emitNonSemanticShaderDebugInfo {false};
bool emitNonSemanticShaderDebugSource{ false };
bool compileOnly{false};
};
void GetSpirvVersion(std::string&);
int GetSpirvGeneratorVersion();
void GlslangToSpv(const glslang::TIntermediate& intermediate, std::vector<unsigned int>& spirv,
SpvOptions* options = nullptr);
void GlslangToSpv(const glslang::TIntermediate& intermediate, std::vector<unsigned int>& spirv,
spv::SpvBuildLogger* logger, SpvOptions* options = nullptr);
bool OutputSpvBin(const std::vector<unsigned int>& spirv, const char* baseName);
bool OutputSpvHex(const std::vector<unsigned int>& spirv, const char* baseName, const char* varName);
}
|
0 | repos/glslang.zig/glslang | repos/glslang.zig/glslang/SPIRV/SpvTools.h | //
// Copyright (C) 2014-2016 LunarG, Inc.
// Copyright (C) 2018 Google, Inc.
//
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
//
// Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
//
// Redistributions in binary form must reproduce the above
// copyright notice, this list of conditions and the following
// disclaimer in the documentation and/or other materials provided
// with the distribution.
//
// Neither the name of 3Dlabs Inc. Ltd. nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
// "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
// LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
// FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
// COPYRIGHT HOLDERS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
// INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
// BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
// LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
// CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
// LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
// ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
// POSSIBILITY OF SUCH DAMAGE.
//
// Call into SPIRV-Tools to disassemble, validate, and optimize.
//
#pragma once
#ifndef GLSLANG_SPV_TOOLS_H
#define GLSLANG_SPV_TOOLS_H
#if ENABLE_OPT
#include <vector>
#include <ostream>
#include "spirv-tools/libspirv.h"
#endif
#include "glslang/MachineIndependent/localintermediate.h"
#include "GlslangToSpv.h"
#include "Logger.h"
namespace glslang {
#if ENABLE_OPT
// Translate glslang's view of target versioning to what SPIRV-Tools uses.
spv_target_env MapToSpirvToolsEnv(const SpvVersion& spvVersion, spv::SpvBuildLogger* logger);
// Use the SPIRV-Tools disassembler to print SPIR-V using a SPV_ENV_UNIVERSAL_1_3 environment.
void SpirvToolsDisassemble(std::ostream& out, const std::vector<unsigned int>& spirv);
// Use the SPIRV-Tools disassembler to print SPIR-V with a provided SPIR-V environment.
void SpirvToolsDisassemble(std::ostream& out, const std::vector<unsigned int>& spirv,
spv_target_env requested_context);
// Apply the SPIRV-Tools validator to generated SPIR-V.
void SpirvToolsValidate(const glslang::TIntermediate& intermediate, std::vector<unsigned int>& spirv,
spv::SpvBuildLogger*, bool prelegalization);
// Apply the SPIRV-Tools optimizer to generated SPIR-V. HLSL SPIR-V is legalized in the process.
void SpirvToolsTransform(const glslang::TIntermediate& intermediate, std::vector<unsigned int>& spirv,
spv::SpvBuildLogger*, const SpvOptions*);
// Apply the SPIRV-Tools EliminateDeadInputComponents pass to generated SPIR-V. Put result in |spirv|.
void SpirvToolsEliminateDeadInputComponents(spv_target_env target_env, std::vector<unsigned int>& spirv,
spv::SpvBuildLogger*);
// Apply the SPIRV-Tools AnalyzeDeadOutputStores pass to generated SPIR-V. Put result in |live_locs|.
// Return true if the result is valid.
bool SpirvToolsAnalyzeDeadOutputStores(spv_target_env target_env, std::vector<unsigned int>& spirv,
std::unordered_set<uint32_t>* live_locs,
std::unordered_set<uint32_t>* live_builtins, spv::SpvBuildLogger*);
// Apply the SPIRV-Tools EliminateDeadOutputStores and AggressiveDeadCodeElimination passes to generated SPIR-V using
// |live_locs|. Put result in |spirv|.
void SpirvToolsEliminateDeadOutputStores(spv_target_env target_env, std::vector<unsigned int>& spirv,
std::unordered_set<uint32_t>* live_locs,
std::unordered_set<uint32_t>* live_builtins, spv::SpvBuildLogger*);
// Apply the SPIRV-Tools optimizer to strip debug info from SPIR-V. This is implicitly done by
// SpirvToolsTransform if spvOptions->stripDebugInfo is set, but can be called separately if
// optimization is disabled.
void SpirvToolsStripDebugInfo(const glslang::TIntermediate& intermediate,
std::vector<unsigned int>& spirv, spv::SpvBuildLogger*);
#endif
} // end namespace glslang
#endif // GLSLANG_SPV_TOOLS_H
|
0 | repos/glslang.zig/glslang | repos/glslang.zig/glslang/SPIRV/Logger.h | //
// Copyright (C) 2016 Google, Inc.
//
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
//
// Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
//
// Redistributions in binary form must reproduce the above
// copyright notice, this list of conditions and the following
// disclaimer in the documentation and/or other materials provided
// with the distribution.
//
// Neither the name of Google Inc. nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
// "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
// LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
// FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
// COPYRIGHT HOLDERS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
// INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
// BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
// LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
// CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
// LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
// ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
// POSSIBILITY OF SUCH DAMAGE.
#ifndef GLSLANG_SPIRV_LOGGER_H
#define GLSLANG_SPIRV_LOGGER_H
#include <string>
#include <vector>
namespace spv {
// A class for holding all SPIR-V build status messages, including
// missing/TBD functionalities, warnings, and errors.
class SpvBuildLogger {
public:
SpvBuildLogger() {}
// Registers a TBD functionality.
void tbdFunctionality(const std::string& f);
// Registers a missing functionality.
void missingFunctionality(const std::string& f);
// Logs a warning.
void warning(const std::string& w) { warnings.push_back(w); }
// Logs an error.
void error(const std::string& e) { errors.push_back(e); }
// Returns all messages accumulated in the order of:
// TBD functionalities, missing functionalities, warnings, errors.
std::string getAllMessages() const;
private:
SpvBuildLogger(const SpvBuildLogger&);
std::vector<std::string> tbdFeatures;
std::vector<std::string> missingFeatures;
std::vector<std::string> warnings;
std::vector<std::string> errors;
};
} // end spv namespace
#endif // GLSLANG_SPIRV_LOGGER_H
|
0 | repos/glslang.zig/glslang | repos/glslang.zig/glslang/SPIRV/GLSL.ext.NV.h | /*
** Copyright (c) 2014-2017 The Khronos Group Inc.
**
** Permission is hereby granted, free of charge, to any person obtaining a copy
** of this software and/or associated documentation files (the "Materials"),
** to deal in the Materials without restriction, including without limitation
** the rights to use, copy, modify, merge, publish, distribute, sublicense,
** and/or sell copies of the Materials, and to permit persons to whom the
** Materials are furnished to do so, subject to the following conditions:
**
** The above copyright notice and this permission notice shall be included in
** all copies or substantial portions of the Materials.
**
** MODIFICATIONS TO THIS FILE MAY MEAN IT NO LONGER ACCURATELY REFLECTS KHRONOS
** STANDARDS. THE UNMODIFIED, NORMATIVE VERSIONS OF KHRONOS SPECIFICATIONS AND
** HEADER INFORMATION ARE LOCATED AT https://www.khronos.org/registry/
**
** THE MATERIALS ARE PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS
** OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
** FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
** THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
** LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
** FROM,OUT OF OR IN CONNECTION WITH THE MATERIALS OR THE USE OR OTHER DEALINGS
** IN THE MATERIALS.
*/
#ifndef GLSLextNV_H
#define GLSLextNV_H
enum BuiltIn;
enum Decoration;
enum Op;
enum Capability;
static const int GLSLextNVVersion = 100;
static const int GLSLextNVRevision = 11;
//SPV_NV_sample_mask_override_coverage
const char* const E_SPV_NV_sample_mask_override_coverage = "SPV_NV_sample_mask_override_coverage";
//SPV_NV_geometry_shader_passthrough
const char* const E_SPV_NV_geometry_shader_passthrough = "SPV_NV_geometry_shader_passthrough";
//SPV_NV_viewport_array2
const char* const E_SPV_NV_viewport_array2 = "SPV_NV_viewport_array2";
const char* const E_ARB_shader_viewport_layer_array = "SPV_ARB_shader_viewport_layer_array";
//SPV_NV_stereo_view_rendering
const char* const E_SPV_NV_stereo_view_rendering = "SPV_NV_stereo_view_rendering";
//SPV_NVX_multiview_per_view_attributes
const char* const E_SPV_NVX_multiview_per_view_attributes = "SPV_NVX_multiview_per_view_attributes";
//SPV_NV_shader_subgroup_partitioned
const char* const E_SPV_NV_shader_subgroup_partitioned = "SPV_NV_shader_subgroup_partitioned";
//SPV_NV_fragment_shader_barycentric
const char* const E_SPV_NV_fragment_shader_barycentric = "SPV_NV_fragment_shader_barycentric";
//SPV_NV_compute_shader_derivatives
const char* const E_SPV_NV_compute_shader_derivatives = "SPV_NV_compute_shader_derivatives";
//SPV_NV_shader_image_footprint
const char* const E_SPV_NV_shader_image_footprint = "SPV_NV_shader_image_footprint";
//SPV_NV_mesh_shader
const char* const E_SPV_NV_mesh_shader = "SPV_NV_mesh_shader";
//SPV_NV_raytracing
const char* const E_SPV_NV_ray_tracing = "SPV_NV_ray_tracing";
//SPV_NV_ray_tracing_motion_blur
const char* const E_SPV_NV_ray_tracing_motion_blur = "SPV_NV_ray_tracing_motion_blur";
//SPV_NV_shading_rate
const char* const E_SPV_NV_shading_rate = "SPV_NV_shading_rate";
//SPV_NV_cooperative_matrix
const char* const E_SPV_NV_cooperative_matrix = "SPV_NV_cooperative_matrix";
//SPV_NV_shader_sm_builtins
const char* const E_SPV_NV_shader_sm_builtins = "SPV_NV_shader_sm_builtins";
//SPV_NV_shader_execution_reorder
const char* const E_SPV_NV_shader_invocation_reorder = "SPV_NV_shader_invocation_reorder";
//SPV_NV_displacement_micromap
const char* const E_SPV_NV_displacement_micromap = "SPV_NV_displacement_micromap";
//SPV_NV_shader_atomic_fp16_vector
const char* const E_SPV_NV_shader_atomic_fp16_vector = "SPV_NV_shader_atomic_fp16_vector";
#endif // #ifndef GLSLextNV_H
|
0 | repos/glslang.zig/glslang | repos/glslang.zig/glslang/SPIRV/SpvTools.cpp | //
// Copyright (C) 2014-2016 LunarG, Inc.
// Copyright (C) 2018-2020 Google, Inc.
//
// All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
//
// Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
//
// Redistributions in binary form must reproduce the above
// copyright notice, this list of conditions and the following
// disclaimer in the documentation and/or other materials provided
// with the distribution.
//
// Neither the name of 3Dlabs Inc. Ltd. nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
// "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
// LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
// FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
// COPYRIGHT HOLDERS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
// INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
// BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
// LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
// CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
// LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
// ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
// POSSIBILITY OF SUCH DAMAGE.
//
// Call into SPIRV-Tools to disassemble, validate, and optimize.
//
#if ENABLE_OPT
#include <cstdio>
#include <iostream>
#include "SpvTools.h"
#include "spirv-tools/optimizer.hpp"
namespace glslang {
// Translate glslang's view of target versioning to what SPIRV-Tools uses.
spv_target_env MapToSpirvToolsEnv(const SpvVersion& spvVersion, spv::SpvBuildLogger* logger)
{
switch (spvVersion.vulkan) {
case glslang::EShTargetVulkan_1_0:
return spv_target_env::SPV_ENV_VULKAN_1_0;
case glslang::EShTargetVulkan_1_1:
switch (spvVersion.spv) {
case EShTargetSpv_1_0:
case EShTargetSpv_1_1:
case EShTargetSpv_1_2:
case EShTargetSpv_1_3:
return spv_target_env::SPV_ENV_VULKAN_1_1;
case EShTargetSpv_1_4:
return spv_target_env::SPV_ENV_VULKAN_1_1_SPIRV_1_4;
default:
logger->missingFunctionality("Target version for SPIRV-Tools validator");
return spv_target_env::SPV_ENV_VULKAN_1_1;
}
case glslang::EShTargetVulkan_1_2:
return spv_target_env::SPV_ENV_VULKAN_1_2;
case glslang::EShTargetVulkan_1_3:
return spv_target_env::SPV_ENV_VULKAN_1_3;
default:
break;
}
if (spvVersion.openGl > 0)
return spv_target_env::SPV_ENV_OPENGL_4_5;
logger->missingFunctionality("Target version for SPIRV-Tools validator");
return spv_target_env::SPV_ENV_UNIVERSAL_1_0;
}
// Callback passed to spvtools::Optimizer::SetMessageConsumer
void OptimizerMesssageConsumer(spv_message_level_t level, const char *source,
const spv_position_t &position, const char *message)
{
auto &out = std::cerr;
switch (level)
{
case SPV_MSG_FATAL:
case SPV_MSG_INTERNAL_ERROR:
case SPV_MSG_ERROR:
out << "error: ";
break;
case SPV_MSG_WARNING:
out << "warning: ";
break;
case SPV_MSG_INFO:
case SPV_MSG_DEBUG:
out << "info: ";
break;
default:
break;
}
if (source)
{
out << source << ":";
}
out << position.line << ":" << position.column << ":" << position.index << ":";
if (message)
{
out << " " << message;
}
out << std::endl;
}
// Use the SPIRV-Tools disassembler to print SPIR-V using a SPV_ENV_UNIVERSAL_1_3 environment.
void SpirvToolsDisassemble(std::ostream& out, const std::vector<unsigned int>& spirv)
{
SpirvToolsDisassemble(out, spirv, spv_target_env::SPV_ENV_UNIVERSAL_1_3);
}
// Use the SPIRV-Tools disassembler to print SPIR-V with a provided SPIR-V environment.
void SpirvToolsDisassemble(std::ostream& out, const std::vector<unsigned int>& spirv,
spv_target_env requested_context)
{
// disassemble
spv_context context = spvContextCreate(requested_context);
spv_text text;
spv_diagnostic diagnostic = nullptr;
spvBinaryToText(context, spirv.data(), spirv.size(),
SPV_BINARY_TO_TEXT_OPTION_FRIENDLY_NAMES | SPV_BINARY_TO_TEXT_OPTION_INDENT,
&text, &diagnostic);
// dump
if (diagnostic == nullptr)
out << text->str;
else
spvDiagnosticPrint(diagnostic);
// teardown
spvDiagnosticDestroy(diagnostic);
spvContextDestroy(context);
}
// Apply the SPIRV-Tools validator to generated SPIR-V.
void SpirvToolsValidate(const glslang::TIntermediate& intermediate, std::vector<unsigned int>& spirv,
spv::SpvBuildLogger* logger, bool prelegalization)
{
// validate
spv_context context = spvContextCreate(MapToSpirvToolsEnv(intermediate.getSpv(), logger));
spv_const_binary_t binary = { spirv.data(), spirv.size() };
spv_diagnostic diagnostic = nullptr;
spv_validator_options options = spvValidatorOptionsCreate();
spvValidatorOptionsSetRelaxBlockLayout(options, intermediate.usingHlslOffsets());
spvValidatorOptionsSetBeforeHlslLegalization(options, prelegalization);
spvValidatorOptionsSetScalarBlockLayout(options, intermediate.usingScalarBlockLayout());
spvValidatorOptionsSetWorkgroupScalarBlockLayout(options, intermediate.usingScalarBlockLayout());
spvValidateWithOptions(context, options, &binary, &diagnostic);
// report
if (diagnostic != nullptr) {
logger->error("SPIRV-Tools Validation Errors");
logger->error(diagnostic->error);
}
// tear down
spvValidatorOptionsDestroy(options);
spvDiagnosticDestroy(diagnostic);
spvContextDestroy(context);
}
// Apply the SPIRV-Tools optimizer to generated SPIR-V. HLSL SPIR-V is legalized in the process.
void SpirvToolsTransform(const glslang::TIntermediate& intermediate, std::vector<unsigned int>& spirv,
spv::SpvBuildLogger* logger, const SpvOptions* options)
{
spv_target_env target_env = MapToSpirvToolsEnv(intermediate.getSpv(), logger);
spvtools::Optimizer optimizer(target_env);
optimizer.SetMessageConsumer(OptimizerMesssageConsumer);
// If debug (specifically source line info) is being generated, propagate
// line information into all SPIR-V instructions. This avoids loss of
// information when instructions are deleted or moved. Later, remove
// redundant information to minimize final SPRIR-V size.
if (options->stripDebugInfo) {
optimizer.RegisterPass(spvtools::CreateStripDebugInfoPass());
}
optimizer.RegisterPass(spvtools::CreateWrapOpKillPass());
optimizer.RegisterPass(spvtools::CreateDeadBranchElimPass());
optimizer.RegisterPass(spvtools::CreateMergeReturnPass());
optimizer.RegisterPass(spvtools::CreateInlineExhaustivePass());
optimizer.RegisterPass(spvtools::CreateEliminateDeadFunctionsPass());
optimizer.RegisterPass(spvtools::CreateScalarReplacementPass());
optimizer.RegisterPass(spvtools::CreateLocalAccessChainConvertPass());
optimizer.RegisterPass(spvtools::CreateLocalSingleBlockLoadStoreElimPass());
optimizer.RegisterPass(spvtools::CreateLocalSingleStoreElimPass());
optimizer.RegisterPass(spvtools::CreateSimplificationPass());
optimizer.RegisterPass(spvtools::CreateAggressiveDCEPass());
optimizer.RegisterPass(spvtools::CreateVectorDCEPass());
optimizer.RegisterPass(spvtools::CreateDeadInsertElimPass());
optimizer.RegisterPass(spvtools::CreateAggressiveDCEPass());
optimizer.RegisterPass(spvtools::CreateDeadBranchElimPass());
optimizer.RegisterPass(spvtools::CreateBlockMergePass());
optimizer.RegisterPass(spvtools::CreateLocalMultiStoreElimPass());
optimizer.RegisterPass(spvtools::CreateIfConversionPass());
optimizer.RegisterPass(spvtools::CreateSimplificationPass());
optimizer.RegisterPass(spvtools::CreateAggressiveDCEPass());
optimizer.RegisterPass(spvtools::CreateVectorDCEPass());
optimizer.RegisterPass(spvtools::CreateDeadInsertElimPass());
optimizer.RegisterPass(spvtools::CreateInterpolateFixupPass());
if (options->optimizeSize) {
optimizer.RegisterPass(spvtools::CreateRedundancyEliminationPass());
optimizer.RegisterPass(spvtools::CreateEliminateDeadInputComponentsSafePass());
}
optimizer.RegisterPass(spvtools::CreateAggressiveDCEPass());
optimizer.RegisterPass(spvtools::CreateCFGCleanupPass());
spvtools::OptimizerOptions spvOptOptions;
optimizer.SetTargetEnv(MapToSpirvToolsEnv(intermediate.getSpv(), logger));
spvOptOptions.set_run_validator(false); // The validator may run as a separate step later on
optimizer.Run(spirv.data(), spirv.size(), &spirv, spvOptOptions);
}
bool SpirvToolsAnalyzeDeadOutputStores(spv_target_env target_env, std::vector<unsigned int>& spirv,
std::unordered_set<uint32_t>* live_locs,
std::unordered_set<uint32_t>* live_builtins,
spv::SpvBuildLogger*)
{
spvtools::Optimizer optimizer(target_env);
optimizer.SetMessageConsumer(OptimizerMesssageConsumer);
optimizer.RegisterPass(spvtools::CreateAnalyzeLiveInputPass(live_locs, live_builtins));
spvtools::OptimizerOptions spvOptOptions;
optimizer.SetTargetEnv(target_env);
spvOptOptions.set_run_validator(false);
return optimizer.Run(spirv.data(), spirv.size(), &spirv, spvOptOptions);
}
void SpirvToolsEliminateDeadOutputStores(spv_target_env target_env, std::vector<unsigned int>& spirv,
std::unordered_set<uint32_t>* live_locs,
std::unordered_set<uint32_t>* live_builtins,
spv::SpvBuildLogger*)
{
spvtools::Optimizer optimizer(target_env);
optimizer.SetMessageConsumer(OptimizerMesssageConsumer);
optimizer.RegisterPass(spvtools::CreateEliminateDeadOutputStoresPass(live_locs, live_builtins));
optimizer.RegisterPass(spvtools::CreateAggressiveDCEPass(false, true));
optimizer.RegisterPass(spvtools::CreateEliminateDeadOutputComponentsPass());
optimizer.RegisterPass(spvtools::CreateAggressiveDCEPass(false, true));
spvtools::OptimizerOptions spvOptOptions;
optimizer.SetTargetEnv(target_env);
spvOptOptions.set_run_validator(false);
optimizer.Run(spirv.data(), spirv.size(), &spirv, spvOptOptions);
}
void SpirvToolsEliminateDeadInputComponents(spv_target_env target_env, std::vector<unsigned int>& spirv,
spv::SpvBuildLogger*)
{
spvtools::Optimizer optimizer(target_env);
optimizer.SetMessageConsumer(OptimizerMesssageConsumer);
optimizer.RegisterPass(spvtools::CreateEliminateDeadInputComponentsPass());
optimizer.RegisterPass(spvtools::CreateAggressiveDCEPass());
spvtools::OptimizerOptions spvOptOptions;
optimizer.SetTargetEnv(target_env);
spvOptOptions.set_run_validator(false);
optimizer.Run(spirv.data(), spirv.size(), &spirv, spvOptOptions);
}
// Apply the SPIRV-Tools optimizer to strip debug info from SPIR-V. This is implicitly done by
// SpirvToolsTransform if spvOptions->stripDebugInfo is set, but can be called separately if
// optimization is disabled.
void SpirvToolsStripDebugInfo(const glslang::TIntermediate& intermediate,
std::vector<unsigned int>& spirv, spv::SpvBuildLogger* logger)
{
spv_target_env target_env = MapToSpirvToolsEnv(intermediate.getSpv(), logger);
spvtools::Optimizer optimizer(target_env);
optimizer.SetMessageConsumer(OptimizerMesssageConsumer);
optimizer.RegisterPass(spvtools::CreateStripDebugInfoPass());
spvtools::OptimizerOptions spvOptOptions;
optimizer.SetTargetEnv(MapToSpirvToolsEnv(intermediate.getSpv(), logger));
spvOptOptions.set_run_validator(false); // The validator may run as a separate step later on
optimizer.Run(spirv.data(), spirv.size(), &spirv, spvOptOptions);
}
}; // end namespace glslang
#endif
|
0 | repos/glslang.zig/glslang/SPIRV | repos/glslang.zig/glslang/SPIRV/CInterface/spirv_c_interface.cpp | /**
This code is based on the glslang_c_interface implementation by Viktor Latypov
**/
/**
BSD 2-Clause License
Copyright (c) 2019, Viktor Latypov
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
1. Redistributions of source code must retain the above copyright notice, this
list of conditions and the following disclaimer.
2. Redistributions in binary form must reproduce the above copyright notice,
this list of conditions and the following disclaimer in the documentation
and/or other materials provided with the distribution.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
**/
#include "glslang/Include/glslang_c_interface.h"
#include "SPIRV/GlslangToSpv.h"
#include "SPIRV/Logger.h"
#include "SPIRV/SpvTools.h"
static_assert(sizeof(glslang_spv_options_t) == sizeof(glslang::SpvOptions), "");
typedef struct glslang_program_s {
glslang::TProgram* program;
std::vector<unsigned int> spirv;
std::string loggerMessages;
} glslang_program_t;
static EShLanguage c_shader_stage(glslang_stage_t stage)
{
switch (stage) {
case GLSLANG_STAGE_VERTEX:
return EShLangVertex;
case GLSLANG_STAGE_TESSCONTROL:
return EShLangTessControl;
case GLSLANG_STAGE_TESSEVALUATION:
return EShLangTessEvaluation;
case GLSLANG_STAGE_GEOMETRY:
return EShLangGeometry;
case GLSLANG_STAGE_FRAGMENT:
return EShLangFragment;
case GLSLANG_STAGE_COMPUTE:
return EShLangCompute;
case GLSLANG_STAGE_RAYGEN:
return EShLangRayGen;
case GLSLANG_STAGE_INTERSECT:
return EShLangIntersect;
case GLSLANG_STAGE_ANYHIT:
return EShLangAnyHit;
case GLSLANG_STAGE_CLOSESTHIT:
return EShLangClosestHit;
case GLSLANG_STAGE_MISS:
return EShLangMiss;
case GLSLANG_STAGE_CALLABLE:
return EShLangCallable;
case GLSLANG_STAGE_TASK:
return EShLangTask;
case GLSLANG_STAGE_MESH:
return EShLangMesh;
default:
break;
}
return EShLangCount;
}
GLSLANG_EXPORT void glslang_program_SPIRV_generate(glslang_program_t* program, glslang_stage_t stage)
{
glslang_spv_options_t spv_options {};
spv_options.disable_optimizer = true;
spv_options.validate = true;
glslang_program_SPIRV_generate_with_options(program, stage, &spv_options);
}
GLSLANG_EXPORT void glslang_program_SPIRV_generate_with_options(glslang_program_t* program, glslang_stage_t stage, glslang_spv_options_t* spv_options) {
spv::SpvBuildLogger logger;
const glslang::TIntermediate* intermediate = program->program->getIntermediate(c_shader_stage(stage));
program->spirv.clear();
glslang::GlslangToSpv(*intermediate, program->spirv, &logger, reinterpret_cast<glslang::SpvOptions*>(spv_options));
program->loggerMessages = logger.getAllMessages();
}
GLSLANG_EXPORT size_t glslang_program_SPIRV_get_size(glslang_program_t* program) { return program->spirv.size(); }
GLSLANG_EXPORT void glslang_program_SPIRV_get(glslang_program_t* program, unsigned int* out)
{
memcpy(out, program->spirv.data(), program->spirv.size() * sizeof(unsigned int));
}
GLSLANG_EXPORT unsigned int* glslang_program_SPIRV_get_ptr(glslang_program_t* program)
{
return program->spirv.data();
}
GLSLANG_EXPORT const char* glslang_program_SPIRV_get_messages(glslang_program_t* program)
{
return program->loggerMessages.empty() ? nullptr : program->loggerMessages.c_str();
}
|
0 | repos | repos/tempora/build.zig.zon | .{
.name = "tempora",
.version = "0.1.3",
.minimum_zig_version = "0.12.0",
.paths = .{
"src",
"build.zig",
"build.zig.zon",
},
.dependencies = .{},
}
|
0 | repos | repos/tempora/build.zig | const std = @import("std");
pub fn build(b: *std.Build) void {
const target = b.standardTargetOptions(.{});
const optimize = b.standardOptimizeOption(.{});
const module = b.addModule("tempora", .{
.root_source_file = b.path("src/tempora.zig"),
});
const update_tzdb_exe = b.addExecutable(.{
.name = "update_tzdb",
.target = b.host,
.optimize = .ReleaseSafe,
.root_source_file = b.path("tools/update_tzdb.zig"),
});
update_tzdb_exe.root_module.addImport("tempora", module);
const update_tzdb = b.addRunArtifact(update_tzdb_exe);
_ = b.step("update_tzdb", "Rebuild tzdb data from the current host's /usr/share/zoneinfo/").dependOn(&update_tzdb.step);
b.installArtifact(update_tzdb_exe);
const dump_exe = b.addExecutable(.{
.name = "dump",
.target = target,
.optimize = optimize,
.root_source_file = b.path("tools/dump.zig"),
});
dump_exe.root_module.addImport("tempora", module);
b.installArtifact(dump_exe);
const run_all_tests = b.step("test", "Run all tests");
const tests = b.addTest(.{
.root_source_file = b.path("src/tempora.zig"),
.target = target,
.optimize = optimize,
});
const run_tests = b.addRunArtifact(tests);
run_all_tests.dependOn(&run_tests.step);
const parser_tests = b.addTest(.{
.root_source_file = b.path("test/parse_tzif.zig"),
.target = target,
.optimize = optimize,
});
parser_tests.root_module.addImport("tempora", module);
const run_parser_tests = b.addRunArtifact(parser_tests);
run_all_tests.dependOn(&run_parser_tests.step);
}
|
0 | repos | repos/tempora/readme.md | # Tempora
### Simple Zig Dates/Times/Timezones
## Features
* Efficient storage (32b `Time`, 32b `Date`, 64b `Date_Time`)
* Composition and decomposition (year, ordinal day/week, month, day, weekday, hour, minute, second, ms)
* Add/subtract days/hours/minutes/seconds/ms
* Advance to the next weekday/day/ordinal day
* Convert to/from unix timestamps
* Embedded IANA timezone database and modified version of [zig-tzif](https://github.com/leroycep/zig-tzif) (adds about 200k to binary size when used)
* Query current timezone on both unix and Windows systems
* Moment.js style formatting and parsing (through `std.fmt`)
## Limitations
* It's not possible to store most "out of bounds" dates/times (e.g. Jan 32).
* Localized month and weekday names are not supported; only English.
* Non-Gregorian calendars are not supported.
* Date/time values directly correspond to timestamps, so accurate durations that take leap seconds into account are not possible (but leap seconds are being abolished in 2035 anyway).
* I am certain that there are more optimized algorithms for timestamp <--> calendar conversions (but performance should be fine for all but the most demanding use cases).
|
0 | repos/tempora | repos/tempora/src/month.zig | pub const Month = enum (u4) {
january = 1,
february = 2,
march = 3,
april = 4,
may = 5,
june = 6,
july = 7,
august = 8,
september = 9,
october = 10,
november = 11,
december = 12,
pub fn from_number(month: i32) Month {
return @enumFromInt(month);
}
pub const From_String_Options = struct {
trim: []const u8 = formatting.default_from_string_trim,
allow_short: bool = true,
allow_long: bool = true,
allow_numeric: bool = true,
};
pub fn from_string(m: []const u8, options: From_String_Options) !Month {
const trimmed = if (options.trim.len > 0) std.mem.trim(u8, m, options.trim) else m;
if (options.allow_short and trimmed.len == 3 or options.allow_long and trimmed.len >= 3) {
inline for (std.meta.fields(Month)) |month| {
if (std.ascii.eqlIgnoreCase(trimmed[0..3], month.name[0..3])) {
if (options.allow_short and trimmed.len == 3) {
return @enumFromInt(month.value);
}
if (options.allow_long and std.ascii.eqlIgnoreCase(trimmed[3..], month.name[3..])) {
return @enumFromInt(month.value);
}
}
}
}
if (options.allow_numeric) {
const numeric = std.fmt.parseInt(u4, trimmed, 10) catch return error.InvalidFormat;
if (numeric >= 1 and numeric <= 12) {
return @enumFromInt(numeric);
}
}
return error.InvalidFormat;
}
pub fn as_number(self: Month) i32 {
return @intFromEnum(self);
}
pub fn as_unsigned(self: Month) u32 {
return @intFromEnum(self);
}
pub fn days(self: Month, year: Year) u16 {
const base = self.days_assume_non_leap_year();
if (self == .february and year.is_leap()) return base + 1;
return base;
}
pub fn days_from_yi(self: Month, yi: Year.Info) u16 {
const base = self.days_assume_non_leap_year();
if (self == .february and yi.is_leap) return base + 1;
return base;
}
pub fn days_assume_non_leap_year(self: Month) u16 {
if (self == .february) return 28;
const month: u16 = @intCast(self.as_number());
if (month < 8) {
return 30 + (month & 1);
} else {
return 30 + ((month + 1) & 1);
}
}
pub fn starting_date(self: Month, year: Year) Date {
return Date.from_ymd(year, self, .first);
}
pub fn name(self: Month) []const u8 {
return switch (self) {
.january => "January",
.february => "February",
.march => "March",
.april => "April",
.may => "May",
.june => "June",
.july => "July",
.august => "August",
.september => "September",
.october => "October",
.november => "November",
.december => "December",
};
}
pub fn short_name(self: Month) []const u8 {
return self.name()[0..3];
}
};
test "Month" {
try expectEqual(.january, try Month.from_string("Jan", .{}));
try expectError(error.InvalidFormat, Month.from_string("Jan", .{ .allow_short = false }));
try expectEqual(.february, try Month.from_string("february", .{}));
try expectEqual(.february, try Month.from_string("february", .{ .allow_short = false }));
try expectError(error.InvalidFormat, Month.from_string("febru", .{}));
try expectEqual(.may, try Month.from_string("may", .{}));
try expectEqual(.october, try Month.from_string("OCT", .{}));
try expectEqual(.november, try Month.from_string("NOVembER", .{}));
try expectEqual(.december, try Month.from_string(" 12 ", .{ .allow_short = false, .allow_long = false }));
try expectEqual(.september, try Month.from_string("___9", .{ .allow_long = false }));
try expectEqual(error.InvalidFormat, Month.from_string("___9", .{ .allow_numeric = false }));
try expectError(error.InvalidFormat, Month.from_string("00", .{}));
try expectError(error.InvalidFormat, Month.from_string("13", .{}));
try expectEqual(31, Month.from_number(1).days_assume_non_leap_year());
try expectEqual(28, Month.from_number(2).days_assume_non_leap_year());
try expectEqual(31, Month.from_number(3).days_assume_non_leap_year());
try expectEqual(30, Month.from_number(4).days_assume_non_leap_year());
try expectEqual(31, Month.from_number(5).days_assume_non_leap_year());
try expectEqual(30, Month.from_number(6).days_assume_non_leap_year());
try expectEqual(31, Month.from_number(7).days_assume_non_leap_year());
try expectEqual(31, Month.from_number(8).days_assume_non_leap_year());
try expectEqual(30, Month.from_number(9).days_assume_non_leap_year());
try expectEqual(31, Month.from_number(10).days_assume_non_leap_year());
try expectEqual(30, Month.from_number(11).days_assume_non_leap_year());
try expectEqual(31, Month.from_number(12).days_assume_non_leap_year());
try expectEqual(31, Month.from_number(1).days(Year.from_number(2020)));
try expectEqual(29, Month.from_number(2).days(Year.from_number(2020)));
try expectEqual(31, Month.from_number(3).days(Year.from_number(2020)));
try expectEqual(30, Month.from_number(4).days(Year.from_number(2020)));
try expectEqual(31, Month.from_number(5).days(Year.from_number(2020)));
try expectEqual(30, Month.from_number(6).days(Year.from_number(2020)));
try expectEqual(31, Month.from_number(7).days(Year.from_number(2020)));
try expectEqual(31, Month.from_number(8).days(Year.from_number(2020)));
try expectEqual(30, Month.from_number(9).days(Year.from_number(2020)));
try expectEqual(31, Month.from_number(10).days(Year.from_number(2020)));
try expectEqual(30, Month.from_number(11).days(Year.from_number(2020)));
try expectEqual(31, Month.from_number(12).days(Year.from_number(2020)));
}
const expectError = std.testing.expectError;
const expectEqual = std.testing.expectEqual;
const Date = @import("date.zig").Date;
const Year = @import("year.zig").Year;
const formatting = @import("formatting.zig");
const std = @import("std");
|
0 | repos/tempora | repos/tempora/src/week_day.zig | pub const Week_Day = enum(u3) {
sunday = 1,
monday = 2,
tuesday = 3,
wednesday = 4,
thursday = 5,
friday = 6,
saturday = 7,
pub fn from_number(d: i32) Week_Day {
return @enumFromInt(d);
}
pub const From_String_Options = struct {
trim: []const u8 = formatting.default_from_string_trim,
allow_long: bool = true,
allow_short: bool = true,
allow_numeric: bool = false,
};
pub fn from_string(d: []const u8, options: From_String_Options) !Week_Day {
const trimmed = if (options.trim.len > 0) std.mem.trim(u8, d, options.trim) else d;
if (options.allow_short and trimmed.len >= 2 or options.allow_long and trimmed.len >= 3) {
inline for (std.meta.fields(Week_Day)) |day| {
if (std.ascii.eqlIgnoreCase(trimmed[0..2], day.name[0..2])) {
if (options.allow_short) {
if (trimmed.len == 2) {
return @enumFromInt(day.value);
} else if (trimmed.len == 3 and trimmed[2] == day.name[2]) {
return @enumFromInt(day.value);
}
}
if (options.allow_long and std.ascii.eqlIgnoreCase(trimmed[2..], day.name[2..])) {
return @enumFromInt(day.value);
}
}
}
}
if (options.allow_numeric) {
const numeric = std.fmt.parseInt(u3, trimmed, 10) catch return error.InvalidFormat;
if (numeric >= 1 and numeric <= 7) {
return @enumFromInt(numeric);
}
}
return error.InvalidFormat;
}
pub fn as_number(self: Week_Day) i32 {
return @intFromEnum(self);
}
pub fn as_unsigned(self: Week_Day) u32 {
return @intFromEnum(self);
}
pub fn name(self: Week_Day) []const u8 {
return switch (self) {
.sunday => "Sunday",
.monday => "Monday",
.tuesday => "Tuesday",
.wednesday => "Wednesday",
.thursday => "Thursday",
.friday => "Friday",
.saturday => "Saturday",
};
}
pub fn short_name(self: Week_Day) []const u8 {
return self.name()[0..3];
}
};
test "Week_Day" {
try expectEqual(.monday, try Week_Day.from_string("Mon", .{}));
try expectError(error.InvalidFormat, Week_Day.from_string("Tues", .{}));
try expectEqual(.wednesday, try Week_Day.from_string("wednesday", .{}));
try expectError(error.InvalidFormat, Week_Day.from_string("7", .{}));
try expectEqual(.saturday, try Week_Day.from_string("7", .{ .allow_numeric = true }));
}
const expectError = std.testing.expectError;
const expectEqual = std.testing.expectEqual;
const formatting = @import("formatting.zig");
const std = @import("std");
|
0 | repos/tempora | repos/tempora/src/ordinal_day.zig |
pub const Ordinal_Day = enum (u9) {
first = 1,
_,
pub fn from_number(day: i32) Ordinal_Day {
std.debug.assert(day >= 1);
std.debug.assert(day <= 366);
return @enumFromInt(day);
}
pub fn from_ymd(y: Year, m: Month, d: Day) Ordinal_Day {
const day_of_month: i32 = d.as_number();
return Ordinal_Day.from_number(switch (m) {
.january => day_of_month,
.february => Month.january.days_assume_non_leap_year() + day_of_month,
inline else => |comptime_month| blk: {
comptime var base = 0;
inline for (1..comptime comptime_month.as_number()) |prev_month| {
base += comptime Month.from_number(prev_month).days_assume_non_leap_year();
}
break :blk base + day_of_month + if (y.is_leap()) @as(u1, 1) else 0;
},
});
}
pub fn from_yimd(yi: Year.Info, m: Month, d: Day) Ordinal_Day {
const day_of_month: i32 = d.as_number();
return Ordinal_Day.from_number(switch (m) {
.january => day_of_month,
.february => Month.january.days_assume_non_leap_year() + day_of_month,
inline else => |comptime_month| blk: {
comptime var base = 0;
inline for (1..comptime comptime_month.as_number()) |prev_month| {
base += comptime Month.from_number(prev_month).days_assume_non_leap_year();
}
break :blk base + day_of_month + if (yi.is_leap) @as(u1, 1) else 0;
},
});
}
pub fn from_md_assume_non_leap_year(m: Month, d: Day) Ordinal_Day {
const day_of_month: i32 = d.as_number();
return Ordinal_Day.from_number(switch (m) {
.january => day_of_month,
.february => Month.january.days_assume_non_leap_year() + day_of_month,
inline else => |comptime_month| blk: {
comptime var base = 0;
inline for (1..comptime_month.as_number()) |prev_month| {
base += comptime Month.from_number(prev_month).days_assume_non_leap_year();
}
break :blk base + day_of_month;
},
});
}
pub fn as_number(self: Ordinal_Day) i32 {
return @intFromEnum(self);
}
pub fn as_unsigned(self: Ordinal_Day) u32 {
return @intFromEnum(self);
}
pub fn date_from_year(self: Ordinal_Day, year: Year) Date {
return year.starting_date().plus_days(self.as_number() - 1);
}
pub fn date_from_yi(self: Ordinal_Day, yi: Year.Info) Date {
return yi.starting_date.plus_days(self.as_number() - 1);
}
pub fn ordinal_week(self: Ordinal_Day) Ordinal_Week {
return Ordinal_Week.from_number(@intCast((self.as_unsigned() - 1) / 7 + 1));
}
pub fn is_before(self: Ordinal_Day, other: Ordinal_Day) bool {
return @intFromEnum(self) < @intFromEnum(other);
}
pub fn is_after(self: Ordinal_Day, other: Ordinal_Day) bool {
return @intFromEnum(self) < @intFromEnum(other);
}
};
const Date = @import("date.zig").Date;
const Year = @import("year.zig").Year;
const Month = @import("month.zig").Month;
const Day = @import("day.zig").Day;
const Ordinal_Week = @import("ordinal_week.zig").Ordinal_Week;
const std = @import("std");
|
0 | repos/tempora | repos/tempora/src/day.zig | pub const Day = enum(u5) {
first = 1,
_,
pub fn from_number(d: i32) Day {
std.debug.assert(d >= 1);
std.debug.assert(d <= 31);
return @enumFromInt(d);
}
pub fn as_number(self: Day) i32 {
return @intFromEnum(self);
}
pub fn as_unsigned(self: Day) u32 {
return @intFromEnum(self);
}
};
const std = @import("std");
|
0 | repos/tempora | repos/tempora/src/year.zig | pub const Year = enum (i32) {
epoch = Date.epoch_year,
_,
pub fn from_number(y: i32) Year {
return @enumFromInt(y);
}
pub fn as_number(self: Year) i32 {
return @intFromEnum(self);
}
pub fn as_unsigned(self: Year) u32 {
return @intCast(@intFromEnum(self));
}
pub const From_String_Options = struct {
trim: []const u8 = formatting.default_from_string_trim,
allow_two_digit_year: bool = true,
allow_non_two_digit_year: bool = true,
};
pub fn from_string(y: []const u8, options: From_String_Options) !Year {
const trimmed = if (options.trim.len > 0) std.mem.trim(u8, y, options.trim) else y;
var numeric = std.fmt.parseInt(i32, trimmed, 10) catch return error.InvalidFormat;
if (options.allow_two_digit_year and trimmed.len == 2) {
if (numeric < 50) {
numeric += 2000;
} else {
numeric += 1900;
}
return from_number(numeric);
}
if (options.allow_non_two_digit_year) {
return from_number(numeric);
}
return error.InvalidFormat;
}
pub fn is_leap(self: Year) bool {
const y = self.as_number();
if ((y & 3) != 0) return false;
if (@mod(y, 100) != 0) return true;
if (@mod(y, 400) == 0) return true;
return false;
}
pub fn starting_date(self: Year) Date {
const year_offset = @intFromEnum(self) - Date.epoch_year;
var leap_years: i32 = 0;
if (year_offset < 0) {
const century_offset: i32 = @divTrunc(year_offset, 100);
leap_years = ((year_offset + 3) >> 2) - century_offset + ((century_offset + 3) >> 2);
} else if (year_offset > 0) {
// If 'year' is a leap year, the leap day doesn't happen until february, which is after
// the start of the year. So we won't actually "pass" it until the next year. To account
// for this we can just subtract 1 from the year offset when it's positive and add 1 to
// leap_years, since epoch_year is a leap year.
const modified_year_offset: i32 = year_offset - 1;
const century_offset: i32 = @divTrunc(modified_year_offset, 100);
leap_years = (modified_year_offset >> 2) - century_offset + (century_offset >> 2) + 1;
}
return @enumFromInt(year_offset * 365 + leap_years);
}
pub const Info = Year_Info;
pub fn info(self: Year) Info {
return .{
.year = self.as_number(),
.starting_date = self.starting_date(),
.is_leap = self.is_leap(),
};
}
};
pub const Year_Info = struct {
year: i32,
starting_date: Date,
is_leap: bool,
};
test "Year" {
try expectEqual(2000, Year.epoch.as_number());
try expectEqual(2000, Year.from_number(2000).as_number());
try expectEqual(2020, Year.from_number(2020).as_number());
try expectEqual(1999, Year.from_number(1999).as_number());
try expectEqual(1970, Year.from_number(1970).as_number());
try expectEqual(Year.from_number(2024), try Year.from_string("2024", .{}));
try expectError(error.InvalidFormat, Year.from_string("2024", .{ .allow_non_two_digit_year = false }));
try expectEqual(Year.from_number(2024), try Year.from_string("'24", .{}));
try expectEqual(Year.from_number(24), try Year.from_string("'24", .{ .allow_two_digit_year = false }));
try expectEqual(Year.from_number(1969), try Year.from_string("69", .{}));
try expectEqual(Year.from_number(123), try Year.from_string("123", .{}));
try expectEqual(Year.from_number(4), try Year.from_string(" 4 ", .{}));
try expectError(error.InvalidFormat, Year.from_string("'24", .{ .allow_two_digit_year = false, .allow_non_two_digit_year = false }));
try expect(Year.from_number(2000).is_leap());
try expect(!Year.from_number(2001).is_leap());
try expect(!Year.from_number(2002).is_leap());
try expect(!Year.from_number(2003).is_leap());
try expect(Year.from_number(1996).is_leap());
try expect(!Year.from_number(1997).is_leap());
try expect(!Year.from_number(1998).is_leap());
try expect(!Year.from_number(1999).is_leap());
try expect(!Year.from_number(1900).is_leap());
try expect(!Year.from_number(1901).is_leap());
try expect(!Year.from_number(1902).is_leap());
try expect(!Year.from_number(1903).is_leap());
try expect(Year.from_number(1904).is_leap());
try expect(Year.from_number(2016).is_leap());
try expect(Year.from_number(2020).is_leap());
try expect(Year.from_number(2024).is_leap());
try expect(!Year.from_number(2017).is_leap());
try expect(!Year.from_number(2018).is_leap());
try expect(!Year.from_number(2019).is_leap());
try expect(!Year.from_number(2021).is_leap());
try expect(!Year.from_number(2022).is_leap());
try expect(!Year.from_number(2023).is_leap());
}
const expect = std.testing.expect;
const expectEqual = std.testing.expectEqual;
const expectError = std.testing.expectError;
const Date = @import("date.zig").Date;
const formatting = @import("formatting.zig");
const std = @import("std");
|
0 | repos/tempora | repos/tempora/src/ordinal_week.zig | // N.B. this is not the same as https://en.wikipedia.org/wiki/ISO_week_date
pub const Ordinal_Week = enum (u6) {
first = 1,
_,
pub fn from_number(day: i32) Ordinal_Week {
std.debug.assert(day >= 1);
std.debug.assert(day <= 53);
return @enumFromInt(day);
}
pub fn as_number(self: Ordinal_Week) i32 {
return @intFromEnum(self);
}
pub fn as_unsigned(self: Ordinal_Week) u32 {
return @intFromEnum(self);
}
pub fn starting_day(self: Ordinal_Week) Ordinal_Day {
return Ordinal_Day.from_number(@intCast((self.as_unsigned() - 1) * 7 + 1));
}
pub fn is_before(self: Ordinal_Week, other: Ordinal_Week) bool {
return @intFromEnum(self) < @intFromEnum(other);
}
pub fn is_after(self: Ordinal_Week, other: Ordinal_Week) bool {
return @intFromEnum(self) < @intFromEnum(other);
}
};
const Ordinal_Day = @import("ordinal_day.zig").Ordinal_Day;
const std = @import("std");
|
0 | repos/tempora | repos/tempora/src/Date_Time.zig |
date: Date,
time: Time,
const Date_Time = @This();
pub const epoch = Date_Time{
.date = .epoch,
.time = .midnight,
};
pub fn with_offset(self: Date_Time, utc_offset_ms: i32) With_Offset {
return .{
.dt = self,
.utc_offset_ms = utc_offset_ms,
.timezone = null,
};
}
/// Assumes this is a local Date_Time from the timezone provided
/// Note that timezones with an annual DST cycle pose a problem here,
/// e.g. 2023-11-05 01:30 central time could refer to either 2023-11-05 06:30 +00:00 or 2023-11-05 07:30 +00:00
/// This function will always pick the earlier option in these cases.
pub fn with_timezone(self: Date_Time, timezone: *const Timezone) With_Offset {
const offset_ts = self.with_offset(0).timestamp_ms();
var zi = timezone.zone_info(@divFloor(offset_ts, 1000));
var offset = zi.offset * 1000;
var ts = offset_ts - offset;
if (zi.begin_ts) |begin_ts| {
if (ts < begin_ts * 1000) {
zi = timezone.zone_info(@divFloor(ts, 1000));
offset = zi.offset * 1000;
ts = offset_ts - offset;
if (zi.end_ts) |end_ts| {
if (ts >= end_ts * 1000) {
const zi2 = timezone.zone_info(@divFloor(ts, 1000));
const offset2 = zi2.offset * 1000;
const ts2 = offset_ts - offset;
if (ts2 < ts) {
ts = ts2;
offset = offset2;
}
}
}
return .{
.dt = self,
.utc_offset_ms = offset,
.timezone = timezone,
};
}
}
if (zi.end_ts) |end_ts| {
if (ts >= end_ts * 1000) {
zi = timezone.zone_info(@divFloor(ts, 1000));
offset = zi.offset * 1000;
ts = offset_ts - offset;
if (zi.begin_ts) |begin_ts| {
if (ts < begin_ts * 1000) {
const zi2 = timezone.zone_info(@divFloor(ts, 1000));
const offset2 = zi2.offset * 1000;
const ts2 = offset_ts - offset;
if (ts2 < ts) {
ts = ts2;
offset = offset2;
}
}
}
}
}
return .{
.dt = self,
.utc_offset_ms = offset,
.timezone = timezone,
};
}
/// N.B. this does not include leap seconds, and assumes both date/times are from the same timezone
pub fn ms_since(self: Date_Time, past: Date_Time) i64 {
const date_diff: i64 = @intFromEnum(self.date) - @intFromEnum(past.date);
const time_diff: i64 = self.time.ms_since_midnight() - past.time.ms_since_midnight();
return date_diff * std.time.ms_per_day + time_diff;
}
pub fn is_before(self: Date_Time, other: Date_Time) bool {
if (self.date.is_before(other.date)) return true;
if (self.date.is_after(other.date)) return false;
return self.time.is_before(other.time);
}
pub fn is_after(self: Date_Time, other: Date_Time) bool {
if (self.date.is_after(other.date)) return true;
if (self.date.is_before(other.date)) return false;
return self.time.is_after(other.time);
}
pub fn plus_days_and_ms(self: Date_Time, days: i32, ms: i64) Date_Time {
var new_date: i64 = @intFromEnum(self.date) + days;
var new_time: i64 = self.time.ms_since_midnight() + ms;
if (new_time < 0) {
const additional_days: i64 = @divTrunc(new_time - std.time.ms_per_day + 1, std.time.ms_per_day);
new_date += additional_days;
new_time -= additional_days * std.time.ms_per_day;
} else if (new_time >= std.time.ms_per_day) {
const additional_days: i64 = @divTrunc(new_time, std.time.ms_per_day);
new_date += additional_days;
new_time -= additional_days * std.time.ms_per_day;
}
return .{
.date = @enumFromInt(new_date),
.time = @enumFromInt(new_time),
};
}
pub const With_Offset = struct {
dt: Date_Time,
utc_offset_ms: i32,
timezone: ?*const Timezone,
pub fn from_timestamp_ms(ts: i64, timezone: ?*const Timezone) With_Offset {
var utc_offset_ms: i32 = 0;
if (timezone) |tz| utc_offset_ms = tz.zone_info(@divFloor(ts, 1000)).offset * 1000;
const offset_ts = ts + utc_offset_ms;
const days = @divFloor(offset_ts, std.time.ms_per_day);
const adjusted: i32 = @intCast(days - 10957);
const date: Date = @enumFromInt(adjusted);
const ms_since_midnight = offset_ts - days * std.time.ms_per_day;
const time: Time = @enumFromInt(ms_since_midnight);
return .{
.dt = .{
.date = date,
.time = time,
},
.utc_offset_ms = utc_offset_ms,
.timezone = timezone,
};
}
pub fn from_timestamp_s(ts: i64, timezone: ?*const Timezone) With_Offset {
return from_timestamp_ms(ts * 1000, timezone);
}
pub fn timestamp_ms(self: With_Offset) i64 {
const days: i64 = @intFromEnum(self.dt.date) + 10957;
const ms_since_midnight = self.dt.time.ms_since_midnight();
return days * std.time.ms_per_day + ms_since_midnight - self.utc_offset_ms;
}
pub fn timestamp_s(self: With_Offset) i64 {
return @divFloor(self.timestamp_ms(), 1000);
}
pub fn in_timezone(self: With_Offset, timezone: ?*const Timezone) With_Offset {
return from_timestamp_ms(self.timestamp_ms(), timezone);
}
/// N.B. this does not include leap seconds
pub fn ms_since(self: With_Offset, past: With_Offset) i64 {
return self.timestamp_ms() - past.timestamp_ms();
}
pub const iso8601 = "{" ++ fmt_iso8601 ++ "}";
pub const iso8601_local = "{" ++ fmt_iso8601_local ++ "}";
pub const rfc2822 = "{" ++ fmt_rfc2822 ++ "}";
pub const http = "{" ++ fmt_http ++ "}";
pub const sql_ms = "{" ++ fmt_sql_ms ++ "}";
pub const sql_ms_local = "{" ++ fmt_sql_ms_local ++ "}";
pub const sql = "{" ++ fmt_sql ++ "}";
pub const sql_local = "{" ++ fmt_sql_local ++ "}";
pub const fmt_iso8601 = "YYYY-MM-DDTHH;mm;ss.SSSZ";
pub const fmt_iso8601_local = "YYYY-MM-DDTHH;mm;ss.SSS";
pub const fmt_rfc2822 = "ddd, DD MMM YYYY HH;mm;ss ZZ";
pub const fmt_http = "ddd, DD MMM YYYY HH;mm;ss [GMT]"; // timezone must be GMT
pub const fmt_sql_ms = "YYYY-MM-DD HH;mm;ss.SSS z";
pub const fmt_sql_ms_local = "YYYY-MM-DD HH;mm;ss.SSS";
pub const fmt_sql = "YYYY-MM-DD HH;mm;ss z";
pub const fmt_sql_local = "YYYY-MM-DD HH;mm;ss";
pub fn format(self: With_Offset, comptime fmt: []const u8, options: std.fmt.FormatOptions, writer: anytype) !void {
_ = options;
try formatting.format(self, if (fmt.len == 0) fmt_iso8601 else fmt, writer);
}
pub fn from_string(comptime fmt: []const u8, str: []const u8) !With_Offset {
return from_string_tz(fmt, str, null);
}
pub fn from_string_tz(comptime fmt: []const u8, str: []const u8, timezone: ?*const Timezone) !With_Offset {
var stream = std.io.fixedBufferStream(str);
const pi = formatting.parse(if (fmt.len == 0) fmt_iso8601 else fmt, &stream) catch return error.InvalidFormat;
var dt: Date_Time = .{
.date = .epoch,
.time = .midnight,
};
if (pi.timestamp) |ts| {
dt = from_timestamp_ms(ts, null).dt;
} else {
if (pi.year) |pi_y| {
const y = if (pi.negate_year) Year.from_number(-pi_y.as_number()) else pi_y;
if (pi.ordinal_day) |od| {
dt.date = Date.from_yd(y, od);
} else if (pi.ordinal_week) |ow| {
const d = Date.from_yd(y, ow.starting_day());
dt.date = if (pi.week_day) |wd| d.advance_to_week_day(wd) else d;
} else if (pi.month) |m| {
dt.date = if (pi.day) |d| Date.from_ymd(y, m, d) else Date.from_yd(y, .first);
} else {
dt.date = Date.from_yd(y, .first);
}
} else return error.InvalidFormat;
if (pi.hours) |raw_h| {
const h = @mod(if (pi.hours_is_pm) raw_h + 12 else raw_h, 24);
const m = pi.minutes orelse 0;
const s = pi.seconds orelse 0;
const milli = pi.ms orelse 0;
dt.time = Time.from_hmsm(h, m, s, milli);
} else return error.InvalidFormat;
}
var dto: With_Offset = .{
.dt = dt,
.utc_offset_ms = pi.utc_offset_ms orelse 0,
.timezone = null,
};
if (timezone) |tz| {
const tz_utc_offset_ms = tz.zone_info(dto.timestamp_s()).offset * 1000;
if (pi.utc_offset_ms) |pi_utc_offset_ms| {
if (tz_utc_offset_ms != pi_utc_offset_ms) {
return dto.in_timezone(tz);
}
}
dto.utc_offset_ms = tz_utc_offset_ms;
dto.timezone = tz;
}
return dto;
}
};
test "Date_Time" {
try tzdb.init_cache(std.testing.allocator);
defer tzdb.deinit_cache();
const tz = (try tzdb.timezone("America/Chicago")).?;
const gmt = (try tzdb.timezone("GMT")).?;
const dt1 = (Date_Time {
.date = Date.from_ymd(Year.from_number(2024), .february, .first),
.time = Time.from_hmsm(12, 34, 56, 789),
}).with_offset(0);
const dt2 = (Date_Time {
.date = Date.from_ymd(Year.from_number(1928), .december, Day.from_number(24)),
.time = Time.from_hmsm(0, 30, 0, 0),
}).with_offset(0);
try expectFmt("2024-02-01 12:34:56.789 +00:00", With_Offset.sql_ms, .{ dt1 });
try expectFmt("2024-02-01 12:34:56.789 GMT", With_Offset.sql_ms, .{ dt1.in_timezone(gmt) });
try expectFmt("2024-02-01 06:34:56.789 CST", With_Offset.sql_ms, .{ dt1.in_timezone(tz) });
try expectFmt("Mon, 24 Dec 1928 00:30:00 +0000", With_Offset.rfc2822, .{ dt2.in_timezone(gmt) });
try expectFmt("Mon, 24 Dec 1928 00:30:00 GMT", With_Offset.http, .{ dt2.in_timezone(gmt) });
try expectFmt("1928-12-24T00:30:00.000+00:00", With_Offset.iso8601, .{ dt2.in_timezone(gmt) });
try expectEqual(dt1, try With_Offset.from_string(With_Offset.fmt_sql_ms, "2024-02-01 12:34:56.789 +00:00"));
try expectEqual(dt1, (try With_Offset.from_string(With_Offset.fmt_sql_ms, "2024-02-01 06:34:56.789 CST")).in_timezone(null));
}
const expectFmt = std.testing.expectFmt;
const expectEqual = std.testing.expectEqual;
const Date = @import("date.zig").Date;
const Time = @import("time.zig").Time;
const Day = @import("day.zig").Day;
const Year = @import("year.zig").Year;
const Timezone = @import("Timezone.zig");
const tzdb = @import("tzdb.zig");
const formatting = @import("formatting.zig");
const std = @import("std");
|
0 | repos/tempora | repos/tempora/src/Timezone.zig | // Parts of this file are based on https://github.com/leroycep/zig-tzif:
// MIT License
// Copyright (c) 2021 LeRoyce Pearson
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
id: []const u8,
transitions: std.MultiArrayList(Transition),
zones: []const Zone_Info,
posix_tz: ?POSIX_TZ,
designations: []const u8,
const Timezone = @This();
pub fn deinit(self: *Timezone, allocator: std.mem.Allocator) void {
allocator.free(self.id);
self.transitions.deinit(allocator);
allocator.free(self.zones);
allocator.free(self.designations);
}
pub fn zone_info(this: Timezone, utc: i64) Zone_Info {
const transitions = this.transitions.slice();
const transition_ts = transitions.items(.ts);
const next_transition = std.sort.upperBound(i64, utc, transition_ts, {}, ts_less_than);
if (next_transition == transitions.len) {
if (this.posix_tz) |posix_tz| {
const last_transition_ts: ?i64 = if (transitions.len > 0) transition_ts[transitions.len - 1] else null;
return posix_tz.zone_info(utc, last_transition_ts);
}
}
if (next_transition == 0) {
return this.zones[0];
}
const transition = transitions.get(next_transition - 1);
var zone = this.zones[transition.zone_index];
zone.begin_ts = transition.ts;
if (next_transition < transitions.len) {
zone.end_ts = transitions.items(.ts)[next_transition];
}
return zone;
}
pub const Zone_Info = struct {
/// A timezone designation string, e.g. EST or EDT
/// Note this string MAY be empty.
designation: []const u8,
/// UTC timestamp for the first instant when this ZoneInfo became valid.
begin_ts: ?i64,
/// UTC timestamp for the first instant when this ZoneInfo is no longer valid.
end_ts: ?i64,
/// An i32 specifying the number of seconds to be added to UTC in order to determine local time.
/// The value MUST NOT be -2**31 and SHOULD be in the range
/// [-89999, 93599] (i.e., its value SHOULD be more than -25 hours
/// and less than 26 hours). Avoiding -2**31 allows 32-bit clients
/// to negate the value without overflow. Restricting it to
/// [-89999, 93599] allows easy support by implementations that
/// already support the POSIX-required range [-24:59:59, 25:59:59].
offset: i32,
/// A value indicating whether local time should be considered Daylight Saving Time (DST).
///
/// A value of `true` indicates that this type of time is DST.
/// A value of `false` indicates that this time type is standard time.
is_dst: bool,
/// This field indicates whether transitions for this local time type
/// were specified in the original tzdata as one of:
/// * UTC
/// * Standard time (local time, ignoring DST)
/// * Wall clock time (local time, possibly including DST)
/// * POSIX TZ string (predicted future transitions)
source: enum {
tzdata_utc,
tzdata_standard,
tzdata_wall,
posix_tz,
},
};
pub const Transition = struct {
ts: i64,
zone_index: u8,
};
/// This is based on Posix definition of the TZ environment variable
pub const POSIX_TZ = struct {
std_designation: []const u8,
std_offset: i32,
dst_designation: ?[]const u8 = null,
/// This field is ignored when dst is null
dst_offset: i32 = 0,
dst_range: ?struct {
start: Rule,
end: Rule,
} = null,
pub const Rule = union(enum) {
julian_day: struct {
/// 1 <= day <= 365. Leap days are not counted and are impossible to refer to
day: u16,
/// The default DST transition time is 02:00:00 local time
time: i32 = 2 * std.time.s_per_hour,
},
julian_day_zero: struct {
/// 0 <= day <= 365. Leap days are counted, and can be referred to.
day: u16,
/// The default DST transition time is 02:00:00 local time
time: i32 = 2 * std.time.s_per_hour,
},
/// In the format of "Mm.n.d", where m = month, n = n, and d = day.
month_nth_week_day: struct {
/// Month of the year. 1 <= month <= 12
month: u8,
/// Specifies which of the weekdays should be used. Does NOT specify the week of the month! 1 <= week <= 5.
///
/// Let's use M3.2.0 as an example. The month is 3, which translates to March.
/// The day is 0, which means Sunday. `n` is 2, which means the second Sunday
/// in the month, NOT Sunday of the second week!
///
/// In 2021, this is difference between 2023-03-07 (Sunday of the second week of March)
/// and 2023-03-14 (the Second Sunday of March).
///
/// * When n is 1, it means the first week in which the day `day` occurs.
/// * 5 is a special case. When n is 5, it means "the last day `day` in the month", which may occur in either the fourth or the fifth week.
n: u8,
/// Day of the week. 0 <= day <= 6. Day zero is Sunday.
day: u8,
/// The default DST transition time is 02:00:00 local time
time: i32 = 2 * std.time.s_per_hour,
},
pub fn is_at_start_of_year(self: Rule) bool {
switch (self) {
.julian_day => |j| return j.day == 1 and j.time == 0,
.julian_day_zero => |j| return j.day == 0 and j.time == 0,
.month_nth_week_day => |mwd| return mwd.month == 1 and mwd.n == 1 and mwd.day == 0 and mwd.time == 0,
}
}
pub fn is_at_end_of_year(self: Rule) bool {
switch (self) {
.julian_day => |j| return j.day == 365 and j.time >= 24,
// Since julian_day_zero dates account for leap year, it would vary depending on the year.
.julian_day_zero => return false,
// There is also no way to specify "end of the year" with month_nth_week_day rules
.month_nth_week_day => return false,
}
}
/// Returned value is the local timestamp when the timezone will transition in the given year.
pub fn to_secs(self: Rule, yi: Year.Info) i64 {
switch (self) {
.julian_day => |j| {
var x: i32 = j.day;
if (x < 60 or !yi.is_leap) x -= 1;
const dt = yi.starting_date.plus_days(x).with_time(.midnight).with_offset(0);
return dt.timestamp_s() + j.time;
},
.julian_day_zero => |j| {
const dt = yi.starting_date.plus_days(j.day).with_time(.midnight).with_offset(0);
return dt.timestamp_s() + j.time;
},
.month_nth_week_day => |mwd| {
const month = Month.from_number(mwd.month);
const target_week_day = Week_Day.from_number(mwd.day + 1);
const first_of_month = Ordinal_Day.from_yimd(yi, month, .first).date_from_yi(yi);
var day = first_of_month;
if (day.week_day() != target_week_day) {
day = day.advance_to_week_day(target_week_day);
}
day = day.plus_days(7 * (mwd.n - 1));
const first_of_next_month = first_of_month.plus_days(month.days_from_yi(yi));
if (!day.is_before(first_of_next_month)) {
day = day.plus_days(-7);
}
const dt = day.with_time(@enumFromInt(mwd.time * 1000)).with_offset(0);
return dt.timestamp_s();
},
}
}
pub fn format(self: Rule, comptime fmt: []const u8, options: std.fmt.FormatOptions, writer: anytype) !void {
_ = fmt;
_ = options;
switch (self) {
.julian_day => |julian_day| {
try std.fmt.format(writer, "J{}", .{julian_day.day});
},
.julian_day_zero => |julian_day_zero| {
try std.fmt.format(writer, "{}", .{julian_day_zero.day});
},
.month_nth_week_day => |month_week_day| {
try std.fmt.format(writer, "M{}.{}.{}", .{
month_week_day.month,
month_week_day.n,
month_week_day.day,
});
},
}
const time = switch (self) {
inline else => |rule| rule.time,
};
// Only write out the time if it is not the default time of 02:00
if (time != 2 * std.time.s_per_hour) {
const seconds = @mod(time, std.time.s_per_min);
const minutes = @mod(@divTrunc(time, std.time.s_per_min), 60);
const hours = @divTrunc(@divTrunc(time, std.time.s_per_min), 60);
try std.fmt.format(writer, "/{}", .{hours});
if (minutes != 0 or seconds != 0) {
try std.fmt.format(writer, ":{}", .{minutes});
}
if (seconds != 0) {
try std.fmt.format(writer, ":{}", .{seconds});
}
}
}
};
pub fn zone_info(self: POSIX_TZ, utc: i64, last_transition_ts: ?i64) Zone_Info {
const dst_designation = self.dst_designation orelse {
std.debug.assert(self.dst_range == null);
return .{
.designation = self.std_designation,
.begin_ts = last_transition_ts,
.end_ts = null,
.offset = self.std_offset,
.is_dst = false,
.source = .posix_tz,
};
};
if (self.dst_range) |range| {
const dt = Date_Time.With_Offset.from_timestamp_s(utc, null).dt;
const yi = dt.date.year_info();
const start_dst = range.start.to_secs(yi) - self.std_offset;
const end_dst = range.end.to_secs(yi) - self.dst_offset;
const is_dst_all_year = range.start.is_at_start_of_year() and range.end.is_at_end_of_year();
if (is_dst_all_year) {
return .{
.designation = dst_designation,
.begin_ts = last_transition_ts,
.end_ts = null,
.offset = self.dst_offset,
.is_dst = true,
.source = .posix_tz,
};
}
if (start_dst < end_dst) {
if (utc >= start_dst and utc < end_dst) {
return .{
.designation = dst_designation,
.begin_ts = @max(last_transition_ts orelse start_dst, start_dst),
.end_ts = end_dst,
.offset = self.dst_offset,
.is_dst = true,
.source = .posix_tz,
};
} else if (utc < start_dst) {
const prev_yi = Year.from_number(yi.year - 1).info();
const prev_end_dst = range.end.to_secs(prev_yi) - self.dst_offset;
return .{
.designation = self.std_designation,
.begin_ts = @max(last_transition_ts orelse prev_end_dst, prev_end_dst),
.end_ts = start_dst,
.offset = self.std_offset,
.is_dst = false,
.source = .posix_tz,
};
} else { // utc >= end_dst
const next_yi = Year.from_number(yi.year + 1).info();
const next_start_dst = range.start.to_secs(next_yi) - self.std_offset;
return .{
.designation = self.std_designation,
.begin_ts = @max(last_transition_ts orelse end_dst, end_dst),
.end_ts = next_start_dst,
.offset = self.std_offset,
.is_dst = false,
.source = .posix_tz,
};
}
} else {
if (utc >= end_dst and utc < start_dst) {
return .{
.designation = self.std_designation,
.begin_ts = @max(last_transition_ts orelse end_dst, end_dst),
.end_ts = start_dst,
.offset = self.std_offset,
.is_dst = false,
.source = .posix_tz,
};
} else if (utc < end_dst) {
const prev_yi = Year.from_number(yi.year - 1).info();
const prev_start_dst = range.start.to_secs(prev_yi) - self.std_offset;
return .{
.designation = dst_designation,
.begin_ts = @max(last_transition_ts orelse prev_start_dst, prev_start_dst),
.end_ts = end_dst,
.offset = self.dst_offset,
.is_dst = true,
.source = .posix_tz,
};
} else { // utc >= start_dst
const next_yi = Year.from_number(yi.year + 1).info();
const next_end_dst = range.end.to_secs(next_yi) - self.dst_offset;
return .{
.designation = dst_designation,
.begin_ts = @max(last_transition_ts orelse start_dst, start_dst),
.end_ts = next_end_dst,
.offset = self.dst_offset,
.is_dst = true,
.source = .posix_tz,
};
}
}
} else {
return .{
.designation = self.std_designation,
.begin_ts = last_transition_ts,
.end_ts = null,
.offset = self.std_offset,
.is_dst = false,
.source = .posix_tz,
};
}
}
pub fn format(self: POSIX_TZ, comptime fmt: []const u8, options: std.fmt.FormatOptions, writer: anytype) !void {
_ = fmt;
_ = options;
const should_quote_std_designation = for (self.std_designation) |character| {
if (!std.ascii.isAlphabetic(character)) {
break true;
}
} else false;
if (should_quote_std_designation) {
try writer.writeAll("<");
try writer.writeAll(self.std_designation);
try writer.writeAll(">");
} else {
try writer.writeAll(self.std_designation);
}
const std_offset_west = -self.std_offset;
const std_seconds = @rem(std_offset_west, std.time.s_per_min);
const std_minutes = @rem(@divTrunc(std_offset_west, std.time.s_per_min), 60);
const std_hours = @divTrunc(@divTrunc(std_offset_west, std.time.s_per_min), 60);
try std.fmt.format(writer, "{}", .{std_hours});
if (std_minutes != 0 or std_seconds != 0) {
try std.fmt.format(writer, ":{}", .{if (std_minutes < 0) -std_minutes else std_minutes});
}
if (std_seconds != 0) {
try std.fmt.format(writer, ":{}", .{if (std_seconds < 0) -std_seconds else std_seconds});
}
if (self.dst_designation) |dst_designation| {
const should_quote_dst_designation = for (dst_designation) |character| {
if (!std.ascii.isAlphabetic(character)) {
break true;
}
} else false;
if (should_quote_dst_designation) {
try writer.writeAll("<");
try writer.writeAll(dst_designation);
try writer.writeAll(">");
} else {
try writer.writeAll(dst_designation);
}
// Only write out the DST offset if it is not just the standard offset plus an hour
if (self.dst_offset != self.std_offset + std.time.s_per_hour) {
const dst_offset_west = -self.dst_offset;
const dst_seconds = @rem(dst_offset_west, std.time.s_per_min);
const dst_minutes = @rem(@divTrunc(dst_offset_west, std.time.s_per_min), 60);
const dst_hours = @divTrunc(@divTrunc(dst_offset_west, std.time.s_per_min), 60);
try std.fmt.format(writer, "{}", .{dst_hours});
if (dst_minutes != 0 or dst_seconds != 0) {
try std.fmt.format(writer, ":{}", .{if (dst_minutes < 0) -dst_minutes else dst_minutes});
}
if (dst_seconds != 0) {
try std.fmt.format(writer, ":{}", .{if (dst_seconds < 0) -dst_seconds else dst_seconds});
}
}
}
if (self.dst_range) |dst_range| {
try std.fmt.format(writer, ",{},{}", .{ dst_range.start, dst_range.end });
}
}
test format {
const america_denver = POSIX_TZ{
.std_designation = "MST",
.std_offset = -25200,
.dst_designation = "MDT",
.dst_offset = -21600,
.dst_range = .{
.start = .{
.month_nth_week_day = .{
.month = 3,
.n = 2,
.day = 0,
.time = 2 * std.time.s_per_hour,
},
},
.end = .{
.month_nth_week_day = .{
.month = 11,
.n = 1,
.day = 0,
.time = 2 * std.time.s_per_hour,
},
},
},
};
try std.testing.expectFmt("MST7MDT,M3.2.0,M11.1.0", "{}", .{america_denver});
const europe_berlin = POSIX_TZ{
.std_designation = "CET",
.std_offset = 3600,
.dst_designation = "CEST",
.dst_offset = 7200,
.dst_range = .{
.start = .{
.month_nth_week_day = .{
.month = 3,
.n = 5,
.day = 0,
.time = 2 * std.time.s_per_hour,
},
},
.end = .{
.month_nth_week_day = .{
.month = 10,
.n = 5,
.day = 0,
.time = 3 * std.time.s_per_hour,
},
},
},
};
try std.testing.expectFmt("CET-1CEST,M3.5.0,M10.5.0/3", "{}", .{europe_berlin});
const antarctica_syowa = POSIX_TZ{
.std_designation = "+03",
.std_offset = 3 * std.time.s_per_hour,
.dst_designation = null,
.dst_offset = undefined,
.dst_range = null,
};
try std.testing.expectFmt("<+03>-3", "{}", .{antarctica_syowa});
const pacific_chatham = POSIX_TZ{
.std_designation = "+1245",
.std_offset = 12 * std.time.s_per_hour + 45 * std.time.s_per_min,
.dst_designation = "+1345",
.dst_offset = 13 * std.time.s_per_hour + 45 * std.time.s_per_min,
.dst_range = .{
.start = .{
.month_nth_week_day = .{
.month = 9,
.n = 5,
.day = 0,
.time = 2 * std.time.s_per_hour + 45 * std.time.s_per_min,
},
},
.end = .{
.month_nth_week_day = .{
.month = 4,
.n = 1,
.day = 0,
.time = 3 * std.time.s_per_hour + 45 * std.time.s_per_min,
},
},
},
};
try std.testing.expectFmt("<+1245>-12:45<+1345>,M9.5.0/2:45,M4.1.0/3:45", "{}", .{pacific_chatham});
}
};
fn ts_less_than(_: void, a: i64, b: i64) bool {
return a < b;
}
const Date_Time = @import("Date_Time.zig");
const Date = @import("date.zig").Date;
const Week_Day = @import("week_day.zig").Week_Day;
const Ordinal_Day = @import("ordinal_day.zig").Ordinal_Day;
const Month = @import("month.zig").Month;
const Year = @import("year.zig").Year;
const testing = std.testing;
const std = @import("std");
|
0 | repos/tempora | repos/tempora/src/time.zig | pub const Time = enum (i32) {
midnight = 0,
@"1am" = 1 * 60 * 60 * 1000,
@"2am" = 2 * 60 * 60 * 1000,
@"3am" = 3 * 60 * 60 * 1000,
@"4am" = 4 * 60 * 60 * 1000,
@"5am" = 5 * 60 * 60 * 1000,
@"6am" = 6 * 60 * 60 * 1000,
@"7am" = 7 * 60 * 60 * 1000,
@"8am" = 8 * 60 * 60 * 1000,
@"9am" = 9 * 60 * 60 * 1000,
@"10am" = 10 * 60 * 60 * 1000,
@"11am" = 11 * 60 * 60 * 1000,
noon = 12 * 60 * 60 * 1000,
@"1pm" = 13 * 60 * 60 * 1000,
@"2pm" = 14 * 60 * 60 * 1000,
@"3pm" = 15 * 60 * 60 * 1000,
@"4pm" = 16 * 60 * 60 * 1000,
@"5pm" = 17 * 60 * 60 * 1000,
@"6pm" = 18 * 60 * 60 * 1000,
@"7pm" = 19 * 60 * 60 * 1000,
@"8pm" = 20 * 60 * 60 * 1000,
@"9pm" = 21 * 60 * 60 * 1000,
@"10pm" = 22 * 60 * 60 * 1000,
@"11pm" = 23 * 60 * 60 * 1000,
midnight_eod = 24 * 60 * 60 * 1000,
_,
pub fn from_hmsm(h: i32, m: i32, s: i32, milli: i32) Time {
const mm = h * 60 + m;
const ss = mm * 60 + s;
const msms = ss * 1000 + milli;
std.debug.assert(msms >= 0);
std.debug.assert(msms < @intFromEnum(Time.midnight_eod));
return @enumFromInt(msms);
}
pub fn with_date(self: Time, date: Date) Date_Time {
return .{
.date = date,
.time = self,
};
}
pub fn with_offset(self: Time, utc_offset_ms: i32) With_Offset {
return .{
.time = self,
.utc_offset_ms = utc_offset_ms,
.timezone = null,
};
}
pub fn with_timezone(self: Time, timezone: *const Timezone, utc_offset_ms: i32) With_Offset {
return .{
.time = self,
.utc_offset_ms = utc_offset_ms,
.timezone = timezone,
};
}
pub fn hours(self: Time) i32 {
const raw = self.ms_since_midnight();
return @divFloor(raw, 60 * 60 * 1000);
}
pub fn minutes_since_midnight(self: Time) i32 {
const raw = self.ms_since_midnight();
return @divFloor(raw, 60 * 1000);
}
pub fn minutes(self: Time) i32 {
const raw = self.ms_since_midnight();
const delta = raw - self.hours() * 60 * 60 * 1000;
return @divFloor(delta, 60 * 1000);
}
pub fn seconds_since_midnight(self: Time) i32 {
const raw = self.ms_since_midnight();
return @divFloor(raw, 1000);
}
pub fn seconds(self: Time) i32 {
const raw = self.ms_since_midnight();
const delta = raw - self.minutes_since_midnight() * 60 * 1000;
return @divFloor(delta, 1000);
}
pub fn ms_since_midnight(self: Time) i32 {
return @intFromEnum(self);
}
pub fn ms(self: Time) i32 {
const raw = self.ms_since_midnight();
return @mod(raw, 1000);
}
pub fn is_before(self: Time, other: Time) bool {
return @intFromEnum(self) < @intFromEnum(other);
}
pub fn is_after(self: Time, other: Time) bool {
return @intFromEnum(self) > @intFromEnum(other);
}
pub fn plus_ms(self: Time, milli: i32) Date {
return @enumFromInt(@intFromEnum(self) + milli);
}
pub fn plus_seconds(self: Time, s: i32) Date {
return @enumFromInt(@intFromEnum(self) + s * 1000);
}
pub fn plus_minutes(self: Time, m: i32) Date {
return @enumFromInt(@intFromEnum(self) + m * 60 * 1000);
}
pub fn plus_hours(self: Time, h: i32) Date {
return @enumFromInt(@intFromEnum(self) + h * 60 * 60 * 1000);
}
pub const With_Offset = struct {
time: Time,
utc_offset_ms: i32,
timezone: ?*const Timezone,
pub fn with_date(self: With_Offset, date: Date) Date_Time.With_Offset {
return .{
.dt = .{
.date = date,
.time = self.time,
},
.utc_offset_ms = self.utc_offset_ms,
.timezone = self.timezone,
};
}
pub fn in_timezone(self: With_Offset, timezone: ?*const Timezone, utc_offset_ms: i32) With_Offset {
return .{
.time = self.time.plus_ms(utc_offset_ms - self.utc_offset_ms),
.utc_offset_ms = utc_offset_ms,
.timezone = timezone,
};
}
pub const iso8601 = "{" ++ fmt_iso8601 ++ "}";
pub const iso8601_local = "{" ++ fmt_iso8601_local ++ "}";
pub const rfc2822 = "{" ++ fmt_rfc2822 ++ "}";
pub const sql_ms = "{" ++ fmt_sql_ms ++ "}";
pub const sql = "{" ++ fmt_sql ++ "}";
pub const hms = "{" ++ fmt_hms ++ "}";
pub const hm = "{" ++ fmt_hm ++ "}";
pub const fmt_iso8601 = "HH;mm;ss.SSSZ";
pub const fmt_iso8601_local = "HH;mm;ss.SSS";
pub const fmt_rfc2822 = "HH;mm;ss ZZ";
pub const fmt_sql_ms = "HH;mm;ss.SSS z";
pub const fmt_sql = "HH;mm;ss z";
pub const fmt_hms = "h;mm;ss a";
pub const fmt_hm = "h;mm a";
pub fn format(self: With_Offset, comptime fmt: []const u8, options: std.fmt.FormatOptions, writer: anytype) !void {
_ = options;
try formatting.format(self.with_date(.epoch), if (fmt.len == 0) fmt_iso8601 else fmt, writer);
}
pub fn from_string(comptime fmt: []const u8, str: []const u8) !With_Offset {
return from_string_tz(fmt, str, null);
}
pub fn from_string_tz(comptime fmt: []const u8, str: []const u8, timezone: ?*const Timezone) !With_Offset {
var stream = std.io.fixedBufferStream(str);
const pi = formatting.parse(if (fmt.len == 0) fmt_iso8601 else fmt, &stream) catch return error.InvalidFormat;
if (pi.timestamp) |ts| {
const dto = Date_Time.With_Offset.from_timestamp_ms(ts, timezone);
return .{
.time = dto.dt.time,
.utc_offset_ms = dto.utc_offset_ms,
.timezone = dto.timezone,
};
} else if (pi.hours) |raw_h| {
const h = @mod(if (pi.hours_is_pm) raw_h + 12 else raw_h, 24);
const m = pi.minutes orelse 0;
const s = pi.seconds orelse 0;
const milli = pi.ms orelse 0;
return .{
.time = Time.from_hmsm(h, m, s, milli),
.utc_offset_ms = pi.utc_offset_ms orelse if (timezone) |tz| tz.zone_info(std.time.timestamp()).offset * 1000 else 0,
.timezone = timezone,
};
} else return error.InvalidFormat;
}
};
};
const Date_Time = @import("Date_Time.zig");
const Timezone = @import("Timezone.zig");
const Date = @import("date.zig").Date;
const formatting = @import("formatting.zig");
const std = @import("std");
|
0 | repos/tempora | repos/tempora/src/date.zig |
pub const Date = enum (i32) {
epoch = 0,
_,
pub const epoch_year = 2000;
// In the gregorian calendar, there are 97 leap years per 400 years,
// so the average length of a year is 365 + 97/400 = 365.2425
const average_days_per_year_x400 = 365 * 400 + 97;
pub fn from_ymd(y: Year, m: Month, d: Day) Date {
return from_yd(y, Ordinal_Day.from_ymd(y, m, d));
}
pub fn from_yd(y: Year, d: Ordinal_Day) Date {
const raw = @intFromEnum(y.starting_date()) + d.as_number() - 1;
return @enumFromInt(raw);
}
pub fn year(self: Date) Year {
return Year.from_number(self.year_info().year);
}
pub fn year_info(self: Date) Year.Info {
var yi: Year.Info = undefined;
const raw = @intFromEnum(self);
const four_hundreths: i64 = @as(i64, raw) * 400;
// Due to leap years this starts as only a guess; but it will be
// correct within +/- one year.
yi.year = @intCast(epoch_year + @divFloor(four_hundreths, average_days_per_year_x400));
yi.starting_date = Year.from_number(yi.year).starting_date();
const delta = raw - @intFromEnum(yi.starting_date);
if (delta < 0) {
yi.year -= 1;
yi.is_leap = Year.from_number(yi.year).is_leap();
yi.starting_date = yi.starting_date.plus_days(if (yi.is_leap) -366 else -365);
} else if (delta >= 365) {
if (Year.from_number(yi.year).is_leap()) {
if (delta >= 366) {
yi.starting_date = yi.starting_date.plus_days(366);
yi.year += 1;
yi.is_leap = Year.from_number(yi.year).is_leap();
} else {
yi.is_leap = true;
}
} else {
yi.starting_date = yi.starting_date.plus_days(365);
yi.year += 1;
yi.is_leap = Year.from_number(yi.year).is_leap();
}
} else {
yi.is_leap = Year.from_number(yi.year).is_leap();
}
return yi;
}
pub fn month(self: Date) Month {
return self.month_from_yi(self.year_info());
}
fn month_from_yi(self: Date, yi: Year.Info) Month {
var od = self.ordinal_day_from_yi(yi);
const noleap_od = Ordinal_Day.from_md_assume_non_leap_year;
if (od.is_before(comptime noleap_od(.march, .first))) {
if (od.is_before(comptime noleap_od(.february, .first))) {
return .january;
} else {
return .february;
}
} else {
if (yi.is_leap) {
if (od == comptime noleap_od(.march, .first)) {
return .february;
} else {
od = Ordinal_Day.from_number(od.as_number() - 1);
}
}
if (od.is_before(comptime noleap_od(.june, .first))) {
if (od.is_before(comptime noleap_od(.april, .first))) {
return .march;
} else if (od.is_before(comptime noleap_od(.may, .first))) {
return .april;
} else {
return .may;
}
} else if (od.is_before(comptime noleap_od(.september, .first))) {
if (od.is_before(comptime noleap_od(.july, .first))) {
return .june;
} else if (od.is_before(comptime noleap_od(.august, .first))) {
return .july;
} else {
return .august;
}
} else if (od.is_before(comptime noleap_od(.november, .first))) {
if (od.is_before(comptime noleap_od(.october, .first))) {
return .september;
} else {
return .october;
}
} else if (od.is_before(comptime noleap_od(.december, .first))) {
return .november;
} else {
return .december;
}
}
}
pub fn day(self: Date) Day {
return self.day_from_yi(self.year_info());
}
fn day_from_yi(self: Date, yi: Year.Info) Day {
var od = self.ordinal_day_from_yi(yi);
const noleap_od = Ordinal_Day.from_md_assume_non_leap_year;
const offset: i32 = blk: {
if (od.is_before(comptime noleap_od(.march, .first))) {
if (od.is_before(comptime noleap_od(.february, .first))) {
break :blk 0;
} else {
break :blk comptime -noleap_od(.february, .first).as_number() + 1;
}
} else {
if (yi.is_leap) {
if (od == comptime noleap_od(.march, .first)) {
break :blk comptime -noleap_od(.february, .first).as_number() + 1;
} else {
od = Ordinal_Day.from_number(od.as_number() - 1);
}
}
if (od.is_before(comptime noleap_od(.june, .first))) {
if (od.is_before(comptime noleap_od(.april, .first))) {
break :blk comptime -noleap_od(.march, .first).as_number() + 1;
} else if (od.is_before(comptime noleap_od(.may, .first))) {
break :blk comptime -noleap_od(.april, .first).as_number() + 1;
} else {
break :blk comptime -noleap_od(.may, .first).as_number() + 1;
}
} else if (od.is_before(comptime noleap_od(.september, .first))) {
if (od.is_before(comptime noleap_od(.july, .first))) {
break :blk comptime -noleap_od(.june, .first).as_number() + 1;
} else if (od.is_before(comptime noleap_od(.august, .first))) {
break :blk comptime -noleap_od(.july, .first).as_number() + 1;
} else {
break :blk comptime -noleap_od(.august, .first).as_number() + 1;
}
} else if (od.is_before(comptime noleap_od(.november, .first))) {
if (od.is_before(comptime noleap_od(.october, .first))) {
break :blk comptime -noleap_od(.september, .first).as_number() + 1;
} else {
break :blk comptime -noleap_od(.october, .first).as_number() + 1;
}
} else if (od.is_before(comptime noleap_od(.december, .first))) {
break :blk comptime -noleap_od(.november, .first).as_number() + 1;
} else {
break :blk comptime -noleap_od(.december, .first).as_number() + 1;
}
}
};
return Day.from_number(od.as_number() + offset);
}
pub fn ordinal_day(self: Date) Ordinal_Day {
return self.ordinal_day_from_yi(self.year_info());
}
fn ordinal_day_from_yi(self: Date, yi: Year.Info) Ordinal_Day {
return Ordinal_Day.from_number(@intFromEnum(self) - @intFromEnum(yi.starting_date) + 1);
}
pub fn ordinal_week(self: Date) Ordinal_Week {
return self.ordinal_week_from_yi(self.year_info());
}
fn ordinal_week_from_yi(self: Date, yi: Year.Info) Ordinal_Week {
return Ordinal_Week.from_od(self.ordinal_day_from_yi(yi));
}
pub fn week_day(self: Date) Week_Day {
// epoch (2000-01-01) was a saturday (7).
var raw: i32 = @intFromEnum(self);
raw = @mod(raw - 1, 7) + 1;
return Week_Day.from_number(raw);
}
pub const Info = Date_Info;
pub fn info(self: Date) Info {
var di: Date_Info = undefined;
di.raw = @intFromEnum(self);
const yi = self.year_info();
di.start_of_year = yi.starting_date;
di.year = Year.from_number(yi.year);
di.is_leap_year = yi.is_leap;
di.month = self.month_from_yi(yi);
di.day = self.day_from_yi(yi);
di.week_day = self.week_day();
di.ordinal_day = self.ordinal_day_from_yi(yi);
di.start_of_month = @enumFromInt(di.raw - di.day.as_number() + 1);
di.start_of_week = @enumFromInt(di.raw - di.week_day.as_number() + 1);
return di;
}
pub fn is_before(self: Date, other: Date) bool {
return @intFromEnum(self) < @intFromEnum(other);
}
pub fn is_after(self: Date, other: Date) bool {
return @intFromEnum(self) > @intFromEnum(other);
}
pub fn plus_days(self: Date, days: i32) Date {
return @enumFromInt(@intFromEnum(self) + days);
}
pub fn advance_to_week_day(self: Date, d: Week_Day) Date {
const current: i32 = self.week_day().as_number();
const target: i32 = d.as_number();
if (current < target) {
return self.plus_days(target - current);
} else {
return self.plus_days(7 + target - current);
}
}
pub fn advance_to_day(self: Date, d: Day) Date {
const di = self.info();
const current = di.day.as_number();
const target = d.as_number();
if (current < target) {
return self.plus_days(target - current);
} else {
var y = di.year;
const m = switch (di.month) {
.december => blk: {
y = Year.from_number(y.as_number() + 1);
break :blk .january;
},
else => Month.from_number(di.month.as_number() + 1),
};
return Date.from_ymd(y, m, d);
}
}
pub fn advance_to_month_and_day(self: Date, m: Month, d: Day) Date {
const di = self.info();
const current = di.ordinal_day.as_number();
const target = Ordinal_Day.from_ymd(di.year, m, d).as_number();
if (current < target) {
return self.plus_days(target - current);
} else {
const y = Year.from_number(di.year.as_number() + 1);
return Date.from_ymd(y, m, d);
}
}
pub fn with_time(self: Date, time: Time) Date_Time {
return .{
.date = self,
.time = time,
};
}
pub const iso8601 = "{" ++ fmt_iso8601 ++ "}";
pub const rfc2822 = "{" ++ fmt_rfc2822 ++ "}";
pub const us = "{" ++ fmt_us ++ "}";
pub const us_numeric = "{" ++ fmt_us_numeric ++ "}";
pub const fmt_iso8601 = "YYYY-MM-DD";
pub const fmt_rfc2822 = "ddd, DD MMM YYYY";
pub const fmt_us = "MMMM D, Y";
pub const fmt_us_numeric = "M/D/Y";
pub fn format(self: Date, comptime fmt: []const u8, options: std.fmt.FormatOptions, writer: anytype) !void {
_ = options;
try formatting.format(self.with_time(.midnight).with_offset(0), if (fmt.len == 0) fmt_iso8601 else fmt, writer);
}
pub fn from_string(comptime fmt: []const u8, str: []const u8) !Date {
var stream = std.io.fixedBufferStream(str);
const pi = formatting.parse(if (fmt.len == 0) fmt_iso8601 else fmt, &stream) catch return error.InvalidFormat;
if (pi.timestamp) |ts| {
return Date_Time.With_Offset.from_timestamp_ms(ts, null).dt.date;
}
if (pi.year) |pi_y| {
const y = if (pi.negate_year) Year.from_number(-pi_y.as_number()) else pi_y;
if (pi.ordinal_day) |od| return from_yd(y, od);
if (pi.ordinal_week) |ow| {
const d = from_yd(y, ow.starting_day());
if (pi.week_day) |wd| {
return d.advance_to_week_day(wd);
}
return d;
}
if (pi.month) |m| {
if (pi.day) |d| return from_ymd(y, m, d);
}
return from_yd(y, .first);
}
return error.InvalidFormat;
}
};
pub const Date_Info = struct {
raw: i32,
start_of_year: Date,
start_of_month: Date,
start_of_week: Date,
is_leap_year: bool,
year: Year,
month: Month,
day: Day,
week_day: Week_Day,
ordinal_day: Ordinal_Day,
pub fn year_info(self: Date_Info) Year.Info {
return .{
.year = self.year.as_number(),
.starting_date = self.start_of_year,
.is_leap = self.is_leap_year,
};
}
};
test "Date" {
const date1 = Date.from_ymd(Year.from_number(2024), .february, .first);
const date2 = Date.from_ymd(Year.from_number(1928), .december, Day.from_number(24));
const date3 = Date.from_ymd(Year.from_number(0), .december, Day.from_number(24));
const date4 = Date.from_ymd(Year.from_number(12), .december, Day.from_number(24));
const date5 = Date.from_ymd(Year.from_number(-123), .december, Day.from_number(24));
const date6 = Date.from_ymd(Year.from_number(1970), .january, .first);
try expectFmt("2024-02-01", "{YYYY-MM-DD}", .{ date1 });
try expectFmt("2024-02-01", "{}", .{ date1 });
try expectFmt("2 2nd Feb February", "{M Mo MMM MMMM}", .{ date1 });
try expectFmt("1.1st", "{Q.Qo}", .{ date1 });
try expectFmt("24 Do 24th", "{D [Do] Do}", .{ date2 });
try expectFmt("359 359th 359", "{DDD DDDo DDDD}", .{ date2 });
try expectFmt("32 32nd 032", "{DDD DDDo DDDD}", .{ date1 });
try expectFmt("4 4 4th Th Thu Thursday", "{d e do dd ddd dddd}", .{ date1 });
try expectFmt("5 5th", "{E Eo}", .{ date1 });
try expectFmt("52 52nd 52", "{w wo ww}", .{ date2 });
try expectFmt("2024 24 2024 2024 +002024", "{Y YY YYY YYYY YYYYYY}", .{ date1 });
try expectFmt("1928 28 1928 1928 +001928", "{Y YY YYY YYYY YYYYYY}", .{ date2 });
try expectFmt("0 00 0 0000 000000", "{Y YY YYY YYYY YYYYYY}", .{ date3 });
try expectFmt("12 12 12 0012 +000012", "{Y YY YYY YYYY YYYYYY}", .{ date4 });
try expectFmt("-123 -123 -123 -0123 -000123", "{Y YY YYY YYYY YYYYYY}", .{ date5 });
try expectFmt("AD AD", "{N NN}", .{ date1 });
try expectFmt("2000", "{Y}", .{ @as(Date, @enumFromInt(0)) });
try expectFmt("2000", "{Y}", .{ Year.from_number(2000).starting_date() });
try expectFmt("2000", "{Y}", .{ Date.from_yd(Year.from_number(2000), .first) });
try expectFmt("2020", "{Y}", .{ Date.from_yd(Year.from_number(2020), .first) });
try expectFmt("1999", "{Y}", .{ Date.from_yd(Year.from_number(1999), .first) });
try expectEqual(date1, try Date.from_string("YYYY-MM-DD", "2024-02-01"));
try expectEqual(date1, try Date.from_string("Y DDDo", "2024 32nd"));
try expectEqual(date1, try Date.from_string("Y MMM D", "+002024 feb 1"));
try expectEqual(date6, try Date.from_string("YY", "70"));
try expectEqual(date6, (try Date.from_string("YYYY-MM-DD", "1969-12-31")).plus_days(1));
try expectEqual(date6, try Date.from_string("x", "0"));
try expectEqual(date6, try Date.from_string("X", "0"));
try expectError(error.InvalidFormat, Date.from_string("MM", "12"));
var d = Date.from_ymd(Year.from_number(2000), .january, .first);
try expectEqual(.first, d.ordinal_day());
try expectEqual(d, (try Date.from_string("YYYY-MM-DD", "1999-12-31")).plus_days(1));
try expect(!d.is_after(d));
try expect(!d.is_before(d));
try expect(d.is_after(try Date.from_string("YYYY-MM-DD", "1999-12-31")));
try expect(d.is_before(try Date.from_string("YYYY-MM-DD", "2000-01-02")));
d = d.advance_to_day(Day.from_number(14));
try expectEqual(d, try Date.from_string("YYYY-MM-DD", "2000-01-14"));
d = d.advance_to_day(Day.from_number(14));
try expectEqual(d, try Date.from_string("YYYY-MM-DD", "2000-02-14"));
d = d.advance_to_day(Day.from_number(13));
try expectEqual(d, try Date.from_string("YYYY-MM-DD", "2000-03-13"));
d = d.advance_to_day(Day.from_number(14));
try expectEqual(d, try Date.from_string("YYYY-MM-DD", "2000-03-14"));
try expectEqual(.tuesday, d.week_day());
d = d.advance_to_week_day(.friday);
try expectEqual(d, try Date.from_string("YYYY-MM-DD", "2000-03-17"));
d = d.advance_to_week_day(.friday);
try expectEqual(d, try Date.from_string("YYYY-MM-DD", "2000-03-24"));
d = d.advance_to_month_and_day(.october, Day.from_number(18));
try expectEqual(d, try Date.from_string("YYYY-MM-DD", "2000-10-18"));
d = d.advance_to_month_and_day(.october, Day.from_number(18));
try expectEqual(d, try Date.from_string("YYYY-MM-DD", "2001-10-18"));
d = d.advance_to_month_and_day(.september, Day.from_number(18));
try expectEqual(d, try Date.from_string("YYYY-MM-DD", "2002-09-18"));
}
const expect = std.testing.expect;
const expectError = std.testing.expectError;
const expectEqual = std.testing.expectEqual;
const expectFmt = std.testing.expectFmt;
const Year = @import("year.zig").Year;
const Month = @import("month.zig").Month;
const Day = @import("day.zig").Day;
const Week_Day = @import("week_day.zig").Week_Day;
const Ordinal_Day = @import("ordinal_day.zig").Ordinal_Day;
const Ordinal_Week = @import("ordinal_week.zig").Ordinal_Week;
const Date_Time = @import("Date_Time.zig");
const Time = @import("time.zig").Time;
const formatting = @import("formatting.zig");
const std = @import("std");
|
0 | repos/tempora | repos/tempora/src/formatting.zig | pub const default_from_string_trim = std.ascii.whitespace ++ "-/,._'";
pub fn format(dto: Date_Time.With_Offset, comptime fmt: []const u8, writer: anytype) !void {
@setEvalBranchQuota(100000);
comptime var iter = Token.iterator(fmt);
const need_date_info = inline while (comptime iter.next()) |token| {
switch (token) {
.y, .Y, .YY, .YYY, .YYYY, .YYYYYY,
.MM, .M, .Mo, .MMM, .MMMM, .Q, .Qo,
.D, .Do, .DD, .DDD, .DDDo, .DDDD, .d, .e, .do, .dd, .ddd, .dddd, .E, .Eo,
.w, .wo, .ww, .N, .NN,
=> break true,
else => {},
}
} else false;
var di: Date.Info = if (need_date_info) dto.dt.date.info() else .{
.raw = 0,
.start_of_year = .epoch,
.start_of_month = .epoch,
.start_of_week = .epoch,
.is_leap_year = false,
.year = .epoch,
.month = .january,
.day = .first,
.week_day = .sunday,
.ordinal_day = .first,
};
iter = comptime Token.iterator(fmt);
inline while (comptime iter.next()) |token| switch (token) {
.MM => try writer.print("{:0>2}", .{ di.month.as_unsigned() }),
.M => try writer.print("{}", .{ di.month.as_unsigned() }),
.Mo => try write_ordinal(writer, di.month.as_unsigned()),
.MMM => try writer.writeAll(di.month.short_name()),
.MMMM => try writer.writeAll(di.month.name()),
.Q => try writer.print("{}", .{ (di.month.as_unsigned() - 1) / 3 + 1 }),
.Qo => try write_ordinal(writer, (di.month.as_unsigned() - 1) / 3 + 1),
.D => try writer.print("{}", .{ di.day.as_unsigned() }),
.Do => try write_ordinal(writer, di.day.as_unsigned()),
.DD => try writer.print("{:0>2}", .{ di.day.as_unsigned() }),
.DDD => try writer.print("{}", .{ di.ordinal_day.as_unsigned() }),
.DDDo => try write_ordinal(writer, di.ordinal_day.as_unsigned()),
.DDDD => try writer.print("{:0>3}", .{ di.ordinal_day.as_unsigned() }),
.d, .e => try writer.print("{}", .{ di.week_day.as_unsigned() - 1 }),
.do => try write_ordinal(writer, di.week_day.as_unsigned() - 1),
.dd => try writer.writeAll(di.week_day.name()[0..2]),
.ddd => try writer.writeAll(di.week_day.short_name()),
.dddd => try writer.writeAll(di.week_day.name()),
.E => try writer.print("{}", .{ di.week_day.as_unsigned() }),
.Eo => try write_ordinal(writer, di.week_day.as_unsigned()),
.w => try writer.print("{}", .{ di.ordinal_day.ordinal_week().as_unsigned() }),
.wo => try write_ordinal(writer, di.ordinal_day.ordinal_week().as_unsigned()),
.ww => try writer.print("{:0>2}", .{ di.ordinal_day.ordinal_week().as_unsigned() }),
.y => try writer.print("{}", .{ @as(u32, @intCast(@abs(di.year.as_number()))) }),
.Y => if (di.year.as_number() > 9999) {
try writer.print("{}", .{ di.year.as_number() });
} else if (di.year.as_number() < 0) {
try writer.print("-{}", .{ @as(u32, @intCast(-di.year.as_number())) });
} else {
try writer.print("{}", .{ di.year.as_unsigned() });
},
.YY => if (di.year.as_number() >= 0) {
try writer.print("{:0>2}", .{ di.year.as_unsigned() % 100 });
} else {
try writer.print("{}", .{ di.year.as_number() });
},
.YYY => if (di.year.as_number() >= 0) {
try writer.print("{}", .{ di.year.as_unsigned() });
} else {
try writer.print("{}", .{ di.year.as_number() });
},
.YYYY => if (di.year.as_number() >= 0) {
try writer.print("{:0>4}", .{ di.year.as_unsigned() });
} else {
try writer.print("-{:0>4}", .{ @as(u32, @intCast(-di.year.as_number())) });
},
.YYYYYY => if (di.year.as_number() > 0) {
try writer.print("+{:0>6}", .{ di.year.as_unsigned() });
} else if (di.year.as_number() < 0) {
try writer.print("-{:0>6}", .{ @as(u32, @intCast(-di.year.as_number())) });
} else {
try writer.writeAll("000000");
},
.N, .NN => if (di.year.as_number() > 0) {
try writer.writeAll("AD");
} else if (di.year.as_number() < 0) {
try writer.writeAll("BC");
},
.literal => |text| {
try writer.writeAll(text);
},
.A => try writer.writeAll(if (dto.dt.time.hours() < 12) "AM" else "PM"),
.a => try writer.writeAll(if (dto.dt.time.hours() < 12) "am" else "pm"),
.H => try writer.print("{}", .{ @as(u32, @intCast(dto.dt.time.hours())) }),
.HH => try writer.print("{:0>2}", .{ @as(u32, @intCast(dto.dt.time.hours())) }),
.h => try writer.print("{}", .{ @as(u32, switch (dto.dt.time.hours()) {
0 => 12,
1...12 => |h| h,
else => |h| h - 12,
}) }),
.hh => try writer.print("{:0>2}", .{ @as(u32, switch (dto.dt.time.hours()) {
0 => 12,
1...12 => |h| h,
else => |h| h - 12,
}) }),
.k => try writer.print("{}", .{ @as(u32, switch (dto.dt.time.hours()) {
0 => 24,
else => |h| h,
}) }),
.kk => try writer.print("{:0>2}", .{ @as(u32, switch (dto.dt.time.hours()) {
0 => 24,
else => |h| h,
}) }),
.m => try writer.print("{}", .{ @as(u32, @intCast(dto.dt.time.minutes())) }),
.mm => try writer.print("{:0>2}", .{ @as(u32, @intCast(dto.dt.time.minutes())) }),
.s => try writer.print("{}", .{ @as(u32, @intCast(dto.dt.time.seconds())) }),
.ss => try writer.print("{:0>2}", .{ @as(u32, @intCast(dto.dt.time.seconds())) }),
.S => try writer.print("{}", .{ @as(u32, @intCast(@divFloor(dto.dt.time.ms(), 100))) }),
.SS => try writer.print("{:0>2}", .{ @as(u32, @intCast(@divFloor(dto.dt.time.ms(), 10))) }),
.SSS => try writer.print("{:0>3}", .{ @as(u32, @intCast(dto.dt.time.ms())) }),
.z, .zz, .Z, .ZZ => done: {
if (token == .z or token == .zz) {
if (dto.timezone) |tz| {
const utc = dto.timestamp_ms();
const zi = tz.zone_info(@divFloor(utc, 1000));
if (zi.designation.len > 0) {
try writer.writeAll(zi.designation);
break :done;
}
}
}
const minutes: u32 = @intCast(@divFloor(@abs(dto.utc_offset_ms), 60 * 1000));
if (token == .zz or token == .ZZ) {
if (dto.utc_offset_ms < 0) {
try writer.print("-{:0>4}", .{ minutes });
} else {
try writer.print("+{:0>4}", .{ minutes });
}
} else {
if (dto.utc_offset_ms < 0) {
try writer.print("-{:0>2}:{:0>2}", .{ @divFloor(minutes, 60), @mod(minutes, 60) });
} else {
try writer.print("+{:0>2}:{:0>2}", .{ @divFloor(minutes, 60), @mod(minutes, 60) });
}
}
},
.x => try writer.print("{}", .{ dto.timestamp_ms() }),
.X => try writer.print("{}", .{ dto.timestamp_s() }),
};
}
const Parse_Info = struct {
timestamp: ?i64 = null,
negate_year: bool = false,
year: ?Year = null,
month: ?Month = null,
day: ?Day = null,
ordinal_day: ?Ordinal_Day = null,
ordinal_week: ?Ordinal_Week = null,
week_day: ?Week_Day = null,
hours_is_pm: bool = false,
hours: ?i32 = null,
minutes: ?i32 = null,
seconds: ?i32 = null,
ms: ?i32 = null,
utc_offset_ms: ?i32 = null,
};
pub fn parse(comptime fmt: []const u8, stream: *std.io.FixedBufferStream([]const u8)) !Parse_Info {
var reader = stream.reader();
var parsed: Parse_Info = .{};
@setEvalBranchQuota(100000);
comptime var iter = Token.iterator(fmt);
inline while (comptime iter.next()) |token| {
switch (token) {
.MM => {
var buf: [2]u8 = undefined;
if (2 == try reader.readAll(&buf)) {
parsed.month = try Month.from_string(&buf, .{
.trim = "",
.allow_short = false,
.allow_long = false,
});
} else return error.InvalidFormat;
},
.M, .Mo => {
const raw = try read_int(u4, stream);
if (raw < 1 or raw > 12) return error.InvalidFormat;
parsed.month = Month.from_number(raw);
if (token == .Mo) {
_ = try reader.readByte();
_ = try reader.readByte();
}
},
.MMM, .MMMM => {
var buf: [3]u8 = undefined;
if (3 == try reader.readAll(&buf)) {
const m = try Month.from_string(&buf, .{
.trim = "",
.allow_long = false,
.allow_numeric = false,
});
parsed.month = m;
if (token == .MMMM) {
const remaining = m.name()[3..];
var buf2: [16]u8 = undefined;
if (remaining.len == try reader.readAll(buf2[0..remaining.len])) {
if (!std.ascii.eqlIgnoreCase(remaining, buf2[0..remaining.len])) {
return error.InvalidFormat;
}
} else return error.InvalidFormat;
}
} else return error.InvalidFormat;
},
.Q, .Qo => {},
.D, .Do => {
const raw = try read_int(u5, stream);
if (raw < 1 or raw > 31) return error.InvalidFormat;
parsed.day = Day.from_number(raw);
if (token == .Do) {
_ = try reader.readByte();
_ = try reader.readByte();
}
},
.DD => {
var buf: [2]u8 = undefined;
if (2 == try reader.readAll(&buf)) {
const num = try std.fmt.parseInt(u5, &buf, 10);
if (num < 1 or num > 31) return error.InvalidFormat;
parsed.day = Day.from_number(num);
} else return error.InvalidFormat;
},
.DDD, .DDDo => {
const raw = try read_int(u9, stream);
if (raw < 1 or raw > 366) return error.InvalidFormat;
parsed.ordinal_day = Ordinal_Day.from_number(raw);
if (token == .DDDo) {
_ = try reader.readByte();
_ = try reader.readByte();
}
},
.DDDD => {
var buf: [3]u8 = undefined;
if (3 == try reader.readAll(&buf)) {
const num = try std.fmt.parseInt(u5, &buf, 10);
if (num < 1 or num > 366) return error.InvalidFormat;
parsed.ordinal_day = Ordinal_Day.from_number(num);
} else return error.InvalidFormat;
},
.d, .do, .e, .E, .Eo => {
var raw = try read_int(u3, stream);
if (token != .E and token != .Eo) raw += 1;
if (raw < 1 or raw > 7) return error.InvalidFormat;
parsed.week_day = Week_Day.from_number(raw);
if (token == .do or token == .Eo) {
_ = try reader.readByte();
_ = try reader.readByte();
}
},
.dd, .ddd, .dddd => {
var buf: [2]u8 = undefined;
if (2 == try reader.readAll(&buf)) {
const d = try Week_Day.from_string(&buf, .{
.trim = "",
.allow_long = false,
.allow_numeric = false,
});
parsed.week_day = d;
if (token == .ddd) {
if (d.name()[2] != std.ascii.toLower(try reader.readByte())) {
return error.InvalidFormat;
}
} else if (token == .dddd) {
const remaining = d.name()[2..];
var buf2: [16]u8 = undefined;
if (remaining.len == try reader.readAll(buf2[0..remaining.len])) {
if (!std.ascii.eqlIgnoreCase(remaining, buf2[0..remaining.len])) {
return error.InvalidFormat;
}
} else return error.InvalidFormat;
}
} else return error.InvalidFormat;
},
.w, .wo => {
const raw = try read_int(u6, stream);
if (raw < 1 or raw > 53) return error.InvalidFormat;
parsed.ordinal_week = Ordinal_Week.from_number(raw);
if (token == .wo) {
_ = try reader.readByte();
_ = try reader.readByte();
}
},
.ww => {
var buf: [2]u8 = undefined;
if (2 == try reader.readAll(&buf)) {
const num = try std.fmt.parseInt(u6, &buf, 10);
if (num < 1 or num > 53) return error.InvalidFormat;
parsed.ordinal_week = Ordinal_Week.from_number(num);
} else return error.InvalidFormat;
},
.y, .Y, .YYY, .YYYYYY => {
const raw = try read_int(i32, stream);
parsed.year = Year.from_number(raw);
},
.YY => {
var buf: [2]u8 = undefined;
if (2 == try reader.readAll(&buf)) {
parsed.year = try Year.from_string(&buf, .{
.trim = "",
.allow_non_two_digit_year = false,
});
} else return error.InvalidFormat;
},
.YYYY => {
var buf: [4]u8 = undefined;
if (4 == try reader.readAll(&buf)) {
parsed.year = try Year.from_string(&buf, .{
.trim = "",
.allow_two_digit_year = false,
});
} else return error.InvalidFormat;
},
.N, .NN => {
var buf: [2]u8 = undefined;
if (2 == try reader.readAll(&buf)) {
parsed.negate_year = std.ascii.eqlIgnoreCase(&buf, "BC");
} else return error.InvalidFormat;
},
.A, .a => {
var buf: [2]u8 = undefined;
if (2 == try reader.readAll(&buf)) {
parsed.hours_is_pm = std.ascii.eqlIgnoreCase(&buf, "PM");
} else return error.InvalidFormat;
},
.H, .h, .k => {
const raw = try read_int(u5, stream);
if (raw > 24) return error.InvalidFormat;
parsed.hours = raw;
},
.HH, .hh, .kk => {
var buf: [2]u8 = undefined;
if (2 == try reader.readAll(&buf)) {
const num = try std.fmt.parseInt(u5, &buf, 10);
if (num > 24) return error.InvalidFormat;
parsed.hours = num;
} else return error.InvalidFormat;
},
.m => {
const raw = try read_int(u6, stream);
if (raw > 59) return error.InvalidFormat;
parsed.minutes = raw;
},
.mm => {
var buf: [2]u8 = undefined;
if (2 == try reader.readAll(&buf)) {
const num = try std.fmt.parseInt(u6, &buf, 10);
if (num > 59) return error.InvalidFormat;
parsed.minutes = num;
} else return error.InvalidFormat;
},
.s => {
const raw = try read_int(u6, stream);
if (raw > 59) return error.InvalidFormat;
parsed.seconds = raw;
},
.ss => {
var buf: [2]u8 = undefined;
if (2 == try reader.readAll(&buf)) {
const num = try std.fmt.parseInt(u6, &buf, 10);
if (num > 59) return error.InvalidFormat;
parsed.seconds = num;
} else return error.InvalidFormat;
},
.S => {
var buf: [1]u8 = undefined;
if (1 == try reader.readAll(&buf)) {
const num = try std.fmt.parseInt(u10, &buf, 10);
if (num > 9) return error.InvalidFormat;
parsed.ms = num;
} else return error.InvalidFormat;
},
.SS => {
var buf: [2]u8 = undefined;
if (2 == try reader.readAll(&buf)) {
const num = try std.fmt.parseInt(u10, &buf, 10);
if (num > 99) return error.InvalidFormat;
parsed.ms = num;
} else return error.InvalidFormat;
},
.SSS => {
var buf: [3]u8 = undefined;
if (3 == try reader.readAll(&buf)) {
const num = try std.fmt.parseInt(u10, &buf, 10);
if (num > 999) return error.InvalidFormat;
parsed.ms = num;
} else return error.InvalidFormat;
},
.Z, .ZZ => parsed.utc_offset_ms = try read_utc_offset(reader, token == .Z),
.z, .zz => {
var buf: [5]u8 = undefined;
const designation: []const u8 = for (0..buf.len) |i| {
if (0 == try reader.read(buf[i..i+1])) {
break buf[0..i];
}
if (!std.ascii.isAlphabetic(buf[i])) {
stream.pos -= 1;
break buf[0..i];
}
} else &buf;
if (designation.len == 0) {
parsed.utc_offset_ms = try read_utc_offset(reader, token == .z);
} else if (try tzdb.designation_offset_ms(designation)) |offset_ms| {
parsed.utc_offset_ms = offset_ms;
} else return error.InvalidFormat;
},
.x, .X => {
var raw = try read_int(i64, stream);
if (token == .X) {
raw = try std.math.mul(i64, raw, 1000);
}
parsed.timestamp = raw;
},
.literal => |text| {
var buf: [text.len]u8 = undefined;
if (text.len == try reader.readAll(&buf)) {
if (!std.ascii.eqlIgnoreCase(&buf, text)) {
//std.log.err("Expected {s}; found {s}", .{ text, &buf });
return error.InvalidFormat;
}
} else return error.InvalidFormat;
},
}
}
return parsed;
}
// moment.js style format specifiers, see https://momentjs.com/docs/#/displaying/format/
const Token = union (enum) {
M, // 1 2 ... 11 12
Mo, // 1st 2nd ... 11th 12th
MM, // 01 02 ... 11 12
MMM, // Jan Feb ... Nov Dec
MMMM, // January February ... November December
Q, // 1 2 3 4
Qo, // 1st 2nd 3rd 4th
D, // 1 2 ... 30 31
Do, // 1st 2nd ... 30th 31st
DD, // 01 02 ... 30 31
DDD, // 1 2 ... 364 365
DDDo, // 1st 2nd ... 364th 365th
DDDD, // 001 002 ... 364 365
d, // 0 1 ... 5 6
do, // 0th 1st ... 5th 6th
dd, // Su Mo ... Fr Sa
ddd, // Sun Mon ... Fri Sat
dddd, // Sunday Monday ... Friday Saturday
e, // 0 1 ... 5 6 (locale)
E, // 1 2 ... 6 7 (ISO)
Eo, // 1st 2nd ... 6th 7th
w, // 1 2 ... 52 53
wo, // 1st 2nd ... 52nd 53rd
ww, // 01 02 ... 52 53
y, // 1 2 ... 2020 ...
Y, // 11970 11971 ... 19999 20000 20001 (Holocene calendar)
YY, // 70 71 ... 29 30
YYY, // 1 2 ... 1970 1971 ... 2029 2030
YYYY, // 0001 0002 ... 1970 1971 ... 2029 2030
YYYYYY, // e.g. -000123 ... +002024
N, // BC AD
NN, // Before Christ ... Anno Domini
A, // AM PM
a, // am pm
H, // 0 1 ... 22 23
HH, // 00 01 ... 22 23
h, // 1 2 ... 11 12
hh, // 01 02 ... 11 12
k, // 1 2 ... 23 24
kk, // 01 02 ... 23 24
m, // 0 1 ... 58 59
mm, // 00 01 ... 58 59
s, // 0 1 ... 58 59
ss, // 00 01 ... 58 59
S, // 0 1 ... 8 9 (second fraction)
SS, // 00 01 ... 98 99
SSS, // 000 001 ... 998 999
z, // EST CST ... MST PST (falls back to Z if no timezone)
zz, // EST CST ... MST PST (falls back to ZZ if no timezone)
Z, // -07:00 -06:00 ... +06:00 +07:00
ZZ, // -0700 -0600 ... +0600 +0700
x, // unix millis
X, // unix seconds
literal: []const u8,
pub fn iterator(fmt: []const u8) Iterator {
return .{ .remaining = fmt };
}
pub const Iterator = struct {
remaining: []const u8,
pub fn next(self: *Iterator) ?Token {
const remaining = self.remaining;
if (remaining.len == 0) return null;
var literal_chars_used: usize = 0;
const token: Token = switch (remaining[0]) {
'M' => if (remaining.len > 1) switch (remaining[1]) {
'o' => .Mo,
'M' => blk: {
if (remaining.len > 3 and remaining[2] == 'M' and remaining[3] == 'M') break :blk .MMMM;
if (remaining.len > 2 and remaining[2] == 'M') break :blk .MMM;
break :blk .MM;
},
else => .M,
} else .M,
'Q' => if (remaining.len > 1 and remaining[1] == 'o') .Qo else .Q,
'D' => if (remaining.len > 1) switch (remaining[1]) {
'o' => .Do,
'D' => blk: {
if (remaining.len > 3 and remaining[2] == 'D') {
break :blk switch (remaining[3]) {
'o' => .DDDo,
'D' => .DDDD,
else => .DDD,
};
}
if (remaining.len > 2 and remaining[2] == 'D') break :blk .DDD;
break :blk .DD;
},
else => .D,
} else .D,
'd' => if (remaining.len > 1) switch (remaining[1]) {
'o' => .do,
'd' => blk: {
if (remaining.len > 3 and remaining[2] == 'd' and remaining[3] == 'd') break :blk .dddd;
if (remaining.len > 2 and remaining[2] == 'd') break :blk .ddd;
break :blk .dd;
},
else => .d,
} else .d,
'e' => .e,
'E' => if (remaining.len > 1 and remaining[1] == 'o') .Eo else .E,
'w' => if (remaining.len > 1) switch (remaining[1]) {
'o' => .wo,
'w' => .ww,
else => .w,
} else .w,
'y' => .y,
'Y' => blk: {
if (remaining.len >= 6 and std.mem.eql(u8, remaining[1..6], "YYYYY")) break :blk .YYYYYY;
if (remaining.len >= 4 and std.mem.eql(u8, remaining[1..4], "YYY")) break :blk .YYYY;
if (remaining.len >= 3 and remaining[1] == 'Y' and remaining[2] == 'Y') break :blk .YYY;
if (remaining.len >= 2 and remaining[1] == 'Y') break :blk .YY;
break :blk .Y;
},
'N' => if (remaining.len > 1 and remaining[1] == 'N') .NN else .N,
'A' => .A,
'a' => .a,
'H' => if (remaining.len > 1 and remaining[1] == 'H') .HH else .H,
'h' => if (remaining.len > 1 and remaining[1] == 'h') .hh else .h,
'k' => if (remaining.len > 1 and remaining[1] == 'k') .kk else .k,
'm' => if (remaining.len > 1 and remaining[1] == 'm') .mm else .m,
's' => if (remaining.len > 1 and remaining[1] == 's') .ss else .s,
'S' => if (remaining.len > 2 and remaining[1] == 'S' and remaining[2] == 'S') .SSS
else if (remaining.len > 1 and remaining[1] == 'S') .SS
else .S,
'z' => if (remaining.len > 1 and remaining[1] == 'z') .zz else .z,
'Z' => if (remaining.len > 1 and remaining[1] == 'Z') .ZZ else .Z,
'x' => .x,
'X' => .X,
'[' => blk: {
if (std.mem.indexOfScalar(u8, remaining, ']')) |end| {
literal_chars_used = end + 1;
break :blk .{ .literal = remaining[1..end] };
} else {
literal_chars_used = remaining.len;
break :blk .{ .literal = remaining[1..] };
}
},
';' => blk: {
// Since std.fmt treats ':' as a special character in format strings, we support using
// semicolons in the format string, which will be treated as colons instead.
literal_chars_used = 1;
break :blk .{ .literal = ":" };
},
else => blk: {
literal_chars_used = std.mem.indexOfAny(u8, remaining, "MQDdeEwYNAaHhkmsSzZxX[") orelse remaining.len;
break :blk .{ .literal = remaining[0..literal_chars_used] };
// literal_chars_used = 1;
// break :blk .{ .literal = remaining[0..1] };
},
};
if (token != .literal) {
self.remaining = remaining[@tagName(token).len..];
} else {
self.remaining = remaining[literal_chars_used..];
}
return token;
}
};
};
fn write_ordinal(writer: anytype, num: u32) !void {
try writer.print("{}", .{num});
try writer.writeAll(switch(num % 100) {
11, 12, 13 => "th",
else => switch (num % 10) {
1 => "st",
2 => "nd",
3 => "rd",
else => "th",
},
});
}
fn read_int(comptime T: type, stream: *std.io.FixedBufferStream([]const u8)) !T {
const reader = stream.reader();
var sign: T = 1;
var value: T = 0;
if (@typeInfo(T).Int.signedness == .signed) switch(try reader.readByte()) {
'-' => sign = -1,
'+' => {},
else => stream.pos -= 1,
};
while (true) {
const c = reader.readByte() catch |err| switch (err) {
error.EndOfStream => break,
else => return err,
};
switch (c) {
'0'...'9' => {
const digit = std.math.cast(T, c - '0') orelse return error.Overflow;
value = try std.math.add(T, try std.math.mul(T, value, 10), digit);
},
else => {
stream.pos -= 1;
break;
},
}
}
return try std.math.mul(T, sign, value);
}
fn read_utc_offset(reader: anytype, colon: bool) !i32 {
const mult: i32 = switch (try reader.readByte()) {
'+' => 1,
'-' => -1,
else => return error.InvalidFormat,
};
var hours: [2]u8 = undefined;
hours[0] = try reader.readByte();
hours[1] = try reader.readByte();
if (colon and ':' != try reader.readByte()) return error.InvalidFormat;
var minutes: [2]u8 = undefined;
minutes[0] = try reader.readByte();
minutes[1] = try reader.readByte();
const h: i32 = try std.fmt.parseInt(u5, &hours, 10);
const m: i32 = try std.fmt.parseInt(u5, &minutes, 10);
if (h > 23) return error.InvalidFormat;
if (m > 59) return error.InvalidFormat;
return (h * 60 + m) * 60 * 1000 * mult;
}
const Date_Time = @import("Date_Time.zig");
const Date = @import("date.zig").Date;
const Year = @import("year.zig").Year;
const Month = @import("month.zig").Month;
const Day = @import("day.zig").Day;
const Ordinal_Day = @import("ordinal_day.zig").Ordinal_Day;
const Ordinal_Week = @import("ordinal_week.zig").Ordinal_Week;
const Week_Day = @import("week_day.zig").Week_Day;
const Time = @import("time.zig").Time;
const Timezone = @import("Timezone.zig");
const tzdb = @import("tzdb.zig");
const std = @import("std");
|
0 | repos/tempora | repos/tempora/src/tzdb.zig | pub const parse_tzif = @import("tzdb/parse_tzif.zig");
var cache: ?Cache = null;
const Cache = struct {
mutex: std.Thread.Mutex = .{},
arena: std.heap.ArenaAllocator,
designation_offsets: std.StringArrayHashMap(i32),
db: std.StringArrayHashMap([]const u8),
cache: std.StringHashMap(*const Timezone),
temp: std.ArrayList(u8),
current: ?*const Timezone = null,
};
pub fn init_cache(gpa: std.mem.Allocator) !void {
std.debug.assert(cache == null);
var arena = std.heap.ArenaAllocator.init(std.heap.page_allocator);
var designation_offsets = try data.designations(arena.allocator());
errdefer designation_offsets.deinit();
var db = try data.db(arena.allocator());
errdefer db.deinit();
cache = .{
.arena = arena,
.designation_offsets = designation_offsets,
.db = db,
.cache = std.StringHashMap(*const Timezone).init(gpa),
.temp = std.ArrayList(u8).init(gpa),
};
}
pub fn deinit_cache() void {
if (cache) |*c| {
c.arena.deinit();
c.cache.deinit();
c.temp.deinit();
cache = null;
}
}
pub fn timezone(id: []const u8) !?*const Timezone {
if (cache) |*c| {
c.mutex.lock();
defer c.mutex.unlock();
return timezone_from_cache(id, c);
} else return error.TzdbCacheNotInitialized; // call tempora.tzdb.init_cache(allocator) once during program initialization
}
fn timezone_from_cache(id: []const u8, c: *Cache) !?*const Timezone {
if (c.cache.get(id)) |tz| {
return tz;
}
if (c.db.getEntry(id)) |entry| {
var stream = std.io.fixedBufferStream(entry.value_ptr.*);
c.temp.clearRetainingCapacity();
try std.compress.zlib.decompress(stream.reader(), c.temp.writer());
const tz = try parse_tzif.parse_memory(c.arena.allocator(), c.temp.items);
const stable_id = entry.key_ptr.*;
var stable_tz = try c.arena.allocator().create(Timezone);
stable_tz.* = tz;
stable_tz.id = stable_id;
const result = try c.cache.getOrPut(id);
result.key_ptr.* = stable_id;
result.value_ptr.* = stable_tz;
return stable_tz;
}
return null;
}
test "timezone" {
try init_cache(std.testing.allocator);
defer deinit_cache();
const ct = (try timezone("America/Chicago")).?;
try expectEqualStrings("America/Chicago", ct.id);
try expect(ct.zones.len > 1);
try expectEqualStrings("CST", ct.posix_tz.?.std_designation);
try expectEqualStrings("CDT", ct.posix_tz.?.dst_designation.?);
const utc = (try timezone("UTC")).?;
try expectEqualStrings("UTC", utc.id);
try expectEqualStrings("UTC", utc.posix_tz.?.std_designation);
try expect(utc.posix_tz.?.dst_designation == null);
}
pub const current_timezone_id = current.current_timezone_id;
pub fn current_timezone() !?*const Timezone {
if (cache) |*c| {
c.mutex.lock();
defer c.mutex.unlock();
if (c.current) |tz| return tz;
const id = try current_timezone_id();
const tz = try timezone_from_cache(id, c);
c.current = tz;
return tz;
} else return error.TzdbCacheNotInitialized; // call tempora.tzdb.init_cache(allocator) once during program initialization
}
test "current_timezone" {
try init_cache(std.testing.allocator);
defer deinit_cache();
var current_tz_id: []const u8 = undefined;
current_tz_id = try current_timezone_id();
try expect(current_tz_id.len > 0);
//try expectEqualStrings("America/New_York", current_tz_id);
//try expectEqualStrings("America/Chicago", current_tz_id);
//try expectEqualStrings("America/Denver", current_tz_id);
//try expectEqualStrings("America/Los_Angeles", current_tz_id);
try expect(null != try current_timezone());
}
pub const ids = data.ids;
pub fn designation_offset_ms(designation: []const u8) !?i32 {
if (cache) |*c| {
// designation_offsets is immutable, so we don't grab the mutex for this
if (c.designation_offsets.get(designation)) |offset_s| {
return offset_s * 1000;
} else return null;
} else return error.TzdbCacheNotInitialized; // call tempora.tzdb.init_cache(allocator) once during program initialization
}
test "designation_offset_ms" {
try init_cache(std.testing.allocator);
defer deinit_cache();
try expectEqual(-6 * 60 * 60 * 1000, (try designation_offset_ms("CST")).?);
try expectEqual(1 * 60 * 60 * 1000, (try designation_offset_ms("BST")).?);
try expectEqual(13 * 60 * 60 * 1000, (try designation_offset_ms("NZDT")).?);
}
const expect = std.testing.expect;
const expectEqual = std.testing.expectEqual;
const expectEqualStrings = std.testing.expectEqualStrings;
const current = @import("tzdb/current.zig");
const data = @import("tzdb/data.zig");
const Timezone = @import("Timezone.zig");
const std = @import("std");
|
0 | repos/tempora | repos/tempora/src/tempora.zig | pub const Date_Time = @import("Date_Time.zig");
pub const Date = @import("date.zig").Date;
pub const Time = @import("time.zig").Time;
pub const Year = @import("year.zig").Year;
pub const Month = @import("month.zig").Month;
pub const Day = @import("day.zig").Day;
pub const Week_Day = @import("week_day.zig").Week_Day;
pub const Ordinal_Week = @import("ordinal_week.zig").Ordinal_Week;
pub const Ordinal_Day = @import("ordinal_day.zig").Ordinal_Day;
pub const Timezone = @import("Timezone.zig");
pub const tzdb = @import("tzdb.zig");
pub fn now() Date_Time.With_Offset {
return now_tz(null);
}
pub fn now_local() !Date_Time.With_Offset {
return now_tz(try tzdb.current_timezone());
}
pub fn now_tz(tz: ?*const Timezone) Date_Time.With_Offset {
return Date_Time.With_Offset.from_timestamp_ms(std.time.milliTimestamp(), tz);
}
const std = @import("std");
test {
_ = tzdb;
_ = Date_Time;
_ = Date;
}
|
0 | repos/tempora/src | repos/tempora/src/tzdb/current.zig | const SYSTEMTIME = extern struct {
year: win.WORD,
month: win.WORD,
day_of_week: win.WORD,
day: win.WORD,
hour: win.WORD,
minute: win.WORD,
second: win.WORD,
milliseconds: win.WORD,
};
const DYNAMIC_TIME_ZONE_INFORMATION = extern struct {
bias: win.LONG,
standard_name: [32]win.WCHAR,
standard_date: SYSTEMTIME,
standard_bias: win.LONG,
daylight_name: [32]win.WCHAR,
daylight_date: SYSTEMTIME,
daylight_bias: win.LONG,
time_zone_key_name: [128]win.WCHAR,
dynamic_daylight_time_disabled: win.BOOLEAN,
};
const TIME_ZONE_ID_INVALID: win.DWORD = 0xffffffff;
extern "kernel32" fn GetDynamicTimeZoneInformation(time_zone_information: *DYNAMIC_TIME_ZONE_INFORMATION) callconv(win.WINAPI) win.DWORD;
fn current_timezone_id_windows() ![]const u8 {
var tzinfo: DYNAMIC_TIME_ZONE_INFORMATION = undefined;
if (GetDynamicTimeZoneInformation(&tzinfo) == TIME_ZONE_ID_INVALID) {
return win.unexpectedError(win.kernel32.GetLastError());
}
const wide = std.mem.sliceTo(&tzinfo.time_zone_key_name, 0);
const codepoints = try std.unicode.utf16CountCodepoints(wide);
if (codepoints <= 40) {
var buf: [160]u8 = undefined;
const end = try std.unicode.utf16leToUtf8(&buf, wide);
const narrow = buf[0..end];
// based on territory 001 data from https://github.com/unicode-org/cldr/blob/main/common/supplemental/windowsZones.xml
if (std.mem.eql(u8, narrow, "Dateline Standard Time")) return "GMT-12";
if (std.mem.eql(u8, narrow, "UTC-11")) return "GMT-11";
if (std.mem.eql(u8, narrow, "Aleutian Standard Time")) return "America/Adak";
if (std.mem.eql(u8, narrow, "Hawaiian Standard Time")) return "Pacific/Honolulu";
if (std.mem.eql(u8, narrow, "Marquesas Standard Time")) return "Pacific/Marquesas";
if (std.mem.eql(u8, narrow, "Alaskan Standard Time")) return "America/Anchorage";
if (std.mem.eql(u8, narrow, "UTC-09")) return "GMT-9";
if (std.mem.eql(u8, narrow, "UTC-08")) return "GMT-8";
if (std.mem.eql(u8, narrow, "Pacific Standard Time (Mexico)")) return "America/Tijuana";
if (std.mem.eql(u8, narrow, "Pacific Standard Time")) return "America/Los_Angeles";
if (std.mem.eql(u8, narrow, "US Mountain Standard Time")) return "America/Phoenix";
if (std.mem.eql(u8, narrow, "Mountain Standard Time")) return "America/Denver";
if (std.mem.eql(u8, narrow, "Mountain Standard Time (Mexico)")) return "America/Mazatlan";
if (std.mem.eql(u8, narrow, "Yukon Standard Time")) return "America/Whitehorse";
if (std.mem.eql(u8, narrow, "Central America Standard Time")) return "America/Guatemala";
if (std.mem.eql(u8, narrow, "Central Standard Time")) return "America/Chicago";
if (std.mem.eql(u8, narrow, "Easter Island Standard Time")) return "Pacific/Easter";
if (std.mem.eql(u8, narrow, "Central Standard Time (Mexico)")) return "America/Mexico_City";
if (std.mem.eql(u8, narrow, "Canada Central Standard Time")) return "America/Regina";
if (std.mem.eql(u8, narrow, "SA Pacific Standard Time")) return "America/Bogota";
if (std.mem.eql(u8, narrow, "Eastern Standard Time (Mexico)")) return "America/Cancun";
if (std.mem.eql(u8, narrow, "Eastern Standard Time")) return "America/New_York";
if (std.mem.eql(u8, narrow, "Haiti Standard Time")) return "America/Port-au-Prince";
if (std.mem.eql(u8, narrow, "Cuba Standard Time")) return "America/Havana";
if (std.mem.eql(u8, narrow, "US Eastern Standard Time")) return "America/Indianapolis";
if (std.mem.eql(u8, narrow, "Turks And Caicos Standard Time")) return "America/Grand_Turk";
if (std.mem.eql(u8, narrow, "Paraguay Standard Time")) return "America/Asuncion";
if (std.mem.eql(u8, narrow, "Atlantic Standard Time")) return "America/Halifax";
if (std.mem.eql(u8, narrow, "Venezuela Standard Time")) return "America/Caracas";
if (std.mem.eql(u8, narrow, "Central Brazilian Standard Time")) return "America/Cuiaba";
if (std.mem.eql(u8, narrow, "SA Western Standard Time")) return "America/La_Paz";
if (std.mem.eql(u8, narrow, "Pacific SA Standard Time")) return "America/Santiago";
if (std.mem.eql(u8, narrow, "Newfoundland Standard Time")) return "America/St_Johns";
if (std.mem.eql(u8, narrow, "Tocantins Standard Time")) return "America/Araguaina";
if (std.mem.eql(u8, narrow, "E. South America Standard Time")) return "America/Sao_Paulo";
if (std.mem.eql(u8, narrow, "SA Eastern Standard Time")) return "America/Cayenne";
if (std.mem.eql(u8, narrow, "Argentina Standard Time")) return "America/Buenos_Aires";
if (std.mem.eql(u8, narrow, "Greenland Standard Time")) return "America/Godthab";
if (std.mem.eql(u8, narrow, "Montevideo Standard Time")) return "America/Montevideo";
if (std.mem.eql(u8, narrow, "Magallanes Standard Time")) return "America/Punta_Arenas";
if (std.mem.eql(u8, narrow, "Saint Pierre Standard Time")) return "America/Miquelon";
if (std.mem.eql(u8, narrow, "Bahia Standard Time")) return "America/Bahia";
if (std.mem.eql(u8, narrow, "UTC-02")) return "GMT-2";
if (std.mem.eql(u8, narrow, "Azores Standard Time")) return "Atlantic/Azores";
if (std.mem.eql(u8, narrow, "Cape Verde Standard Time")) return "Atlantic/Cape_Verde";
if (std.mem.eql(u8, narrow, "UTC")) return "UTC";
if (std.mem.eql(u8, narrow, "Coordinated Universal Time")) return "GMT";
if (std.mem.eql(u8, narrow, "GMT Standard Time")) return "Europe/London";
if (std.mem.eql(u8, narrow, "Greenwich Standard Time")) return "Atlantic/Reykjavik";
if (std.mem.eql(u8, narrow, "Sao Tome Standard Time")) return "Africa/Sao_Tome";
if (std.mem.eql(u8, narrow, "Morocco Standard Time")) return "Africa/Casablanca";
if (std.mem.eql(u8, narrow, "W. Europe Standard Time")) return "Europe/Berlin";
if (std.mem.eql(u8, narrow, "Central Europe Standard Time")) return "Europe/Budapest";
if (std.mem.eql(u8, narrow, "Romance Standard Time")) return "Europe/Paris";
if (std.mem.eql(u8, narrow, "Central European Standard Time")) return "Europe/Warsaw";
if (std.mem.eql(u8, narrow, "W. Central Africa Standard Time")) return "Africa/Lagos";
if (std.mem.eql(u8, narrow, "Jordan Standard Time")) return "Asia/Amman";
if (std.mem.eql(u8, narrow, "GTB Standard Time")) return "Europe/Bucharest";
if (std.mem.eql(u8, narrow, "Middle East Standard Time")) return "Asia/Beirut";
if (std.mem.eql(u8, narrow, "Egypt Standard Time")) return "Africa/Cairo";
if (std.mem.eql(u8, narrow, "E. Europe Standard Time")) return "Europe/Chisinau";
if (std.mem.eql(u8, narrow, "Syria Standard Time")) return "Asia/Damascus";
if (std.mem.eql(u8, narrow, "West Bank Standard Time")) return "Asia/Hebron";
if (std.mem.eql(u8, narrow, "South Africa Standard Time")) return "Africa/Johannesburg";
if (std.mem.eql(u8, narrow, "FLE Standard Time")) return "Europe/Kiev";
if (std.mem.eql(u8, narrow, "Israel Standard Time")) return "Asia/Jerusalem";
if (std.mem.eql(u8, narrow, "South Sudan Standard Time")) return "Africa/Juba";
if (std.mem.eql(u8, narrow, "Kaliningrad Standard Time")) return "Europe/Kaliningrad";
if (std.mem.eql(u8, narrow, "Sudan Standard Time")) return "Africa/Khartoum";
if (std.mem.eql(u8, narrow, "Libya Standard Time")) return "Africa/Tripoli";
if (std.mem.eql(u8, narrow, "Namibia Standard Time")) return "Africa/Windhoek";
if (std.mem.eql(u8, narrow, "Arabic Standard Time")) return "Asia/Baghdad";
if (std.mem.eql(u8, narrow, "Turkey Standard Time")) return "Europe/Istanbul";
if (std.mem.eql(u8, narrow, "Arab Standard Time")) return "Asia/Riyadh";
if (std.mem.eql(u8, narrow, "Belarus Standard Time")) return "Europe/Minsk";
if (std.mem.eql(u8, narrow, "Russian Standard Time")) return "Europe/Moscow";
if (std.mem.eql(u8, narrow, "E. Africa Standard Time")) return "Africa/Nairobi";
if (std.mem.eql(u8, narrow, "Iran Standard Time")) return "Asia/Tehran";
if (std.mem.eql(u8, narrow, "Arabian Standard Time")) return "Asia/Dubai";
if (std.mem.eql(u8, narrow, "Astrakhan Standard Time")) return "Europe/Astrakhan";
if (std.mem.eql(u8, narrow, "Azerbaijan Standard Time")) return "Asia/Baku";
if (std.mem.eql(u8, narrow, "Russia Time Zone 3")) return "Europe/Samara";
if (std.mem.eql(u8, narrow, "Mauritius Standard Time")) return "Indian/Mauritius";
if (std.mem.eql(u8, narrow, "Saratov Standard Time")) return "Europe/Saratov";
if (std.mem.eql(u8, narrow, "Georgian Standard Time")) return "Asia/Tbilisi";
if (std.mem.eql(u8, narrow, "Volgograd Standard Time")) return "Europe/Volgograd";
if (std.mem.eql(u8, narrow, "Caucasus Standard Time")) return "Asia/Yerevan";
if (std.mem.eql(u8, narrow, "Afghanistan Standard Time")) return "Asia/Kabul";
if (std.mem.eql(u8, narrow, "West Asia Standard Time")) return "Asia/Tashkent";
if (std.mem.eql(u8, narrow, "Ekaterinburg Standard Time")) return "Asia/Yekaterinburg";
if (std.mem.eql(u8, narrow, "Pakistan Standard Time")) return "Asia/Karachi";
if (std.mem.eql(u8, narrow, "Qyzylorda Standard Time")) return "Asia/Qyzylorda";
if (std.mem.eql(u8, narrow, "India Standard Time")) return "Asia/Calcutta";
if (std.mem.eql(u8, narrow, "Sri Lanka Standard Time")) return "Asia/Colombo";
if (std.mem.eql(u8, narrow, "Nepal Standard Time")) return "Asia/Katmandu";
if (std.mem.eql(u8, narrow, "Central Asia Standard Time")) return "Asia/Almaty";
if (std.mem.eql(u8, narrow, "Bangladesh Standard Time")) return "Asia/Dhaka";
if (std.mem.eql(u8, narrow, "Omsk Standard Time")) return "Asia/Omsk";
if (std.mem.eql(u8, narrow, "Myanmar Standard Time")) return "Asia/Rangoon";
if (std.mem.eql(u8, narrow, "SE Asia Standard Time")) return "Asia/Bangkok";
if (std.mem.eql(u8, narrow, "Altai Standard Time")) return "Asia/Barnaul";
if (std.mem.eql(u8, narrow, "W. Mongolia Standard Time")) return "Asia/Hovd";
if (std.mem.eql(u8, narrow, "North Asia Standard Time")) return "Asia/Krasnoyarsk";
if (std.mem.eql(u8, narrow, "N. Central Asia Standard Time")) return "Asia/Novosibirsk";
if (std.mem.eql(u8, narrow, "Tomsk Standard Time")) return "Asia/Tomsk";
if (std.mem.eql(u8, narrow, "China Standard Time")) return "Asia/Shanghai";
if (std.mem.eql(u8, narrow, "North Asia East Standard Time")) return "Asia/Irkutsk";
if (std.mem.eql(u8, narrow, "Singapore Standard Time")) return "Asia/Singapore";
if (std.mem.eql(u8, narrow, "W. Australia Standard Time")) return "Australia/Perth";
if (std.mem.eql(u8, narrow, "Taipei Standard Time")) return "Asia/Taipei";
if (std.mem.eql(u8, narrow, "Ulaanbaatar Standard Time")) return "Asia/Ulaanbaatar";
if (std.mem.eql(u8, narrow, "Aus Central W. Standard Time")) return "Australia/Eucla";
if (std.mem.eql(u8, narrow, "Transbaikal Standard Time")) return "Asia/Chita";
if (std.mem.eql(u8, narrow, "Tokyo Standard Time")) return "Asia/Tokyo";
if (std.mem.eql(u8, narrow, "North Korea Standard Time")) return "Asia/Pyongyang";
if (std.mem.eql(u8, narrow, "Korea Standard Time")) return "Asia/Seoul";
if (std.mem.eql(u8, narrow, "Yakutsk Standard Time")) return "Asia/Yakutsk";
if (std.mem.eql(u8, narrow, "Cen. Australia Standard Time")) return "Australia/Adelaide";
if (std.mem.eql(u8, narrow, "AUS Central Standard Time")) return "Australia/Darwin";
if (std.mem.eql(u8, narrow, "E. Australia Standard Time")) return "Australia/Brisbane";
if (std.mem.eql(u8, narrow, "AUS Eastern Standard Time")) return "Australia/Sydney";
if (std.mem.eql(u8, narrow, "West Pacific Standard Time")) return "Pacific/Port_Moresby";
if (std.mem.eql(u8, narrow, "Tasmania Standard Time")) return "Australia/Hobart";
if (std.mem.eql(u8, narrow, "Vladivostok Standard Time")) return "Asia/Vladivostok";
if (std.mem.eql(u8, narrow, "Lord Howe Standard Time")) return "Australia/Lord_Howe";
if (std.mem.eql(u8, narrow, "Bougainville Standard Time")) return "Pacific/Bougainville";
if (std.mem.eql(u8, narrow, "Russia Time Zone 10")) return "Asia/Srednekolymsk";
if (std.mem.eql(u8, narrow, "Magadan Standard Time")) return "Asia/Magadan";
if (std.mem.eql(u8, narrow, "Norfolk Standard Time")) return "Pacific/Norfolk";
if (std.mem.eql(u8, narrow, "Sakhalin Standard Time")) return "Asia/Sakhalin";
if (std.mem.eql(u8, narrow, "Central Pacific Standard Time")) return "Pacific/Guadalcanal";
if (std.mem.eql(u8, narrow, "Russia Time Zone 11")) return "Asia/Kamchatka";
if (std.mem.eql(u8, narrow, "New Zealand Standard Time")) return "Pacific/Auckland";
if (std.mem.eql(u8, narrow, "UTC+12")) return "GMT+12";
if (std.mem.eql(u8, narrow, "Fiji Standard Time")) return "Pacific/Fiji";
if (std.mem.eql(u8, narrow, "Chatham Islands Standard Time")) return "Pacific/Chatham";
if (std.mem.eql(u8, narrow, "UTC+13")) return "GMT+13";
if (std.mem.eql(u8, narrow, "Tonga Standard Time")) return "Pacific/Tongatapu";
if (std.mem.eql(u8, narrow, "Samoa Standard Time")) return "Pacific/Apia";
if (std.mem.eql(u8, narrow, "Line Islands Standard Time")) return "Pacific/Kiritimati";
log.warn("No mapping for Windows timezone key '{s}'", .{ narrow });
} else {
log.warn("Windows timezone key name too long ({} codepoints; expected < {})", .{ codepoints, 40 });
}
const id = switch (std.math.clamp(-@divFloor(tzinfo.bias + 29, 60), -12, 14)) {
-12 => "GMT-12",
-11 => "GMT-11",
-10 => "GMT-10",
-9 => "GMT-9",
-8 => "GMT-8",
-7 => "GMT-7",
-6 => "GMT-6",
-5 => "GMT-5",
-4 => "GMT-4",
-3 => "GMT-3",
-2 => "GMT-2",
-1 => "GMT-1",
0 => "GMT",
1 => "GMT+1",
2 => "GMT+2",
3 => "GMT+3",
4 => "GMT+4",
5 => "GMT+5",
6 => "GMT+6",
7 => "GMT+7",
8 => "GMT+8",
9 => "GMT+9",
10 => "GMT+10",
11 => "GMT+11",
12 => "GMT+12",
13 => "GMT+13",
14 => "GMT+14",
else => unreachable,
};
log.info("Falling back to closest GMT offset: {s}", .{ id });
return id;
}
fn current_timezone_id_unix() ![]const u8 {
const buf: [std.fs.MAX_PATH_BYTES]u8 = undefined;
const raw = try std.fs.cwd().readLink("/etc/localtime", &buf);
const prefix = "/usr/share/zoneinfo/";
if (std.mem.startsWith(u8, raw, prefix)) {
return raw[prefix.len..];
}
log.err("Expected /etc/localtime to be a link into {s}; but found {s}", .{ prefix, raw });
return error.InvalidTimezone;
}
pub fn current_timezone_id() ![]const u8 {
if (builtin.os.tag == .windows) {
return current_timezone_id_windows();
} else {
return current_timezone_id_unix();
}
}
const log = std.log.scoped(.tempora);
const win = std.os.windows;
const std = @import("std");
const builtin = @import("builtin");
|
0 | repos/tempora/src | repos/tempora/src/tzdb/data.zig | pub const ids = [_][]const u8 {
"Africa/Abidjan", "Africa/Accra", "Africa/Addis_Ababa", "Africa/Algiers", "Africa/Asmara", "Africa/Asmera",
"Africa/Bamako", "Africa/Bangui", "Africa/Banjul", "Africa/Bissau", "Africa/Blantyre",
"Africa/Brazzaville", "Africa/Bujumbura", "Africa/Cairo", "Africa/Casablanca", "Africa/Ceuta",
"Africa/Conakry", "Africa/Dakar", "Africa/Dar_es_Salaam", "Africa/Djibouti", "Africa/Douala",
"Africa/El_Aaiun", "Africa/Freetown", "Africa/Gaborone", "Africa/Harare", "Africa/Johannesburg",
"Africa/Juba", "Africa/Kampala", "Africa/Khartoum", "Africa/Kigali", "Africa/Kinshasa", "Africa/Lagos",
"Africa/Libreville", "Africa/Lome", "Africa/Luanda", "Africa/Lubumbashi", "Africa/Lusaka",
"Africa/Malabo", "Africa/Maputo", "Africa/Maseru", "Africa/Mbabane", "Africa/Mogadishu",
"Africa/Monrovia", "Africa/Nairobi", "Africa/Ndjamena", "Africa/Niamey", "Africa/Nouakchott",
"Africa/Ouagadougou", "Africa/Porto-Novo", "Africa/Sao_Tome", "Africa/Timbuktu", "Africa/Tripoli",
"Africa/Tunis", "Africa/Windhoek", "America/Adak", "America/Anchorage", "America/Anguilla",
"America/Antigua", "America/Araguaina", "America/Argentina/Buenos_Aires", "America/Argentina/Catamarca",
"America/Argentina/ComodRivadavia", "America/Argentina/Cordoba", "America/Argentina/Jujuy",
"America/Argentina/La_Rioja", "America/Argentina/Mendoza", "America/Argentina/Rio_Gallegos",
"America/Argentina/Salta", "America/Argentina/San_Juan", "America/Argentina/San_Luis",
"America/Argentina/Tucuman", "America/Argentina/Ushuaia", "America/Aruba", "America/Asuncion",
"America/Atikokan", "America/Atka", "America/Bahia", "America/Bahia_Banderas", "America/Barbados",
"America/Belem", "America/Belize", "America/Blanc-Sablon", "America/Boa_Vista", "America/Bogota",
"America/Boise", "America/Buenos_Aires", "America/Cambridge_Bay", "America/Campo_Grande",
"America/Cancun", "America/Caracas", "America/Catamarca", "America/Cayenne", "America/Cayman",
"America/Chicago", "America/Chihuahua", "America/Ciudad_Juarez", "America/Coral_Harbour",
"America/Cordoba", "America/Costa_Rica", "America/Creston", "America/Cuiaba", "America/Curacao",
"America/Danmarkshavn", "America/Dawson", "America/Dawson_Creek", "America/Denver", "America/Detroit",
"America/Dominica", "America/Edmonton", "America/Eirunepe", "America/El_Salvador", "America/Ensenada",
"America/Fort_Nelson", "America/Fort_Wayne", "America/Fortaleza", "America/Glace_Bay", "America/Godthab",
"America/Goose_Bay", "America/Grand_Turk", "America/Grenada", "America/Guadeloupe", "America/Guatemala",
"America/Guayaquil", "America/Guyana", "America/Halifax", "America/Havana", "America/Hermosillo",
"America/Indiana/Indianapolis", "America/Indiana/Knox", "America/Indiana/Marengo",
"America/Indiana/Petersburg", "America/Indiana/Tell_City", "America/Indiana/Vevay",
"America/Indiana/Vincennes", "America/Indiana/Winamac", "America/Indianapolis", "America/Inuvik",
"America/Iqaluit", "America/Jamaica", "America/Jujuy", "America/Juneau", "America/Kentucky/Louisville",
"America/Kentucky/Monticello", "America/Knox_IN", "America/Kralendijk", "America/La_Paz", "America/Lima",
"America/Los_Angeles", "America/Louisville", "America/Lower_Princes", "America/Maceio", "America/Managua",
"America/Manaus", "America/Marigot", "America/Martinique", "America/Matamoros", "America/Mazatlan",
"America/Mendoza", "America/Menominee", "America/Merida", "America/Metlakatla", "America/Mexico_City",
"America/Miquelon", "America/Moncton", "America/Monterrey", "America/Montevideo", "America/Montreal",
"America/Montserrat", "America/Nassau", "America/New_York", "America/Nipigon", "America/Nome",
"America/Noronha", "America/North_Dakota/Beulah", "America/North_Dakota/Center",
"America/North_Dakota/New_Salem", "America/Nuuk", "America/Ojinaga", "America/Panama",
"America/Pangnirtung", "America/Paramaribo", "America/Phoenix", "America/Port-au-Prince",
"America/Port_of_Spain", "America/Porto_Acre", "America/Porto_Velho", "America/Puerto_Rico",
"America/Punta_Arenas", "America/Rainy_River", "America/Rankin_Inlet", "America/Recife", "America/Regina",
"America/Resolute", "America/Rio_Branco", "America/Rosario", "America/Santa_Isabel", "America/Santarem",
"America/Santiago", "America/Santo_Domingo", "America/Sao_Paulo", "America/Scoresbysund",
"America/Shiprock", "America/Sitka", "America/St_Barthelemy", "America/St_Johns", "America/St_Kitts",
"America/St_Lucia", "America/St_Thomas", "America/St_Vincent", "America/Swift_Current",
"America/Tegucigalpa", "America/Thule", "America/Thunder_Bay", "America/Tijuana", "America/Toronto",
"America/Tortola", "America/Vancouver", "America/Virgin", "America/Whitehorse", "America/Winnipeg",
"America/Yakutat", "America/Yellowknife", "Antarctica/Casey", "Antarctica/Davis",
"Antarctica/DumontDUrville", "Antarctica/Macquarie", "Antarctica/Mawson", "Antarctica/McMurdo",
"Antarctica/Palmer", "Antarctica/Rothera", "Antarctica/South_Pole", "Antarctica/Syowa",
"Antarctica/Troll", "Antarctica/Vostok", "Arctic/Longyearbyen", "Asia/Aden", "Asia/Almaty", "Asia/Amman",
"Asia/Anadyr", "Asia/Aqtau", "Asia/Aqtobe", "Asia/Ashgabat", "Asia/Ashkhabad", "Asia/Atyrau",
"Asia/Baghdad", "Asia/Bahrain", "Asia/Baku", "Asia/Bangkok", "Asia/Barnaul", "Asia/Beirut",
"Asia/Bishkek", "Asia/Brunei", "Asia/Calcutta", "Asia/Chita", "Asia/Choibalsan", "Asia/Chongqing",
"Asia/Chungking", "Asia/Colombo", "Asia/Dacca", "Asia/Damascus", "Asia/Dhaka", "Asia/Dili", "Asia/Dubai",
"Asia/Dushanbe", "Asia/Famagusta", "Asia/Gaza", "Asia/Harbin", "Asia/Hebron", "Asia/Ho_Chi_Minh",
"Asia/Hong_Kong", "Asia/Hovd", "Asia/Irkutsk", "Asia/Istanbul", "Asia/Jakarta", "Asia/Jayapura",
"Asia/Jerusalem", "Asia/Kabul", "Asia/Kamchatka", "Asia/Karachi", "Asia/Kashgar", "Asia/Kathmandu",
"Asia/Katmandu", "Asia/Khandyga", "Asia/Kolkata", "Asia/Krasnoyarsk", "Asia/Kuala_Lumpur", "Asia/Kuching",
"Asia/Kuwait", "Asia/Macao", "Asia/Macau", "Asia/Magadan", "Asia/Makassar", "Asia/Manila", "Asia/Muscat",
"Asia/Nicosia", "Asia/Novokuznetsk", "Asia/Novosibirsk", "Asia/Omsk", "Asia/Oral", "Asia/Phnom_Penh",
"Asia/Pontianak", "Asia/Pyongyang", "Asia/Qatar", "Asia/Qostanay", "Asia/Qyzylorda", "Asia/Rangoon",
"Asia/Riyadh", "Asia/Saigon", "Asia/Sakhalin", "Asia/Samarkand", "Asia/Seoul", "Asia/Shanghai",
"Asia/Singapore", "Asia/Srednekolymsk", "Asia/Taipei", "Asia/Tashkent", "Asia/Tbilisi", "Asia/Tehran",
"Asia/Tel_Aviv", "Asia/Thimbu", "Asia/Thimphu", "Asia/Tokyo", "Asia/Tomsk", "Asia/Ujung_Pandang",
"Asia/Ulaanbaatar", "Asia/Ulan_Bator", "Asia/Urumqi", "Asia/Ust-Nera", "Asia/Vientiane",
"Asia/Vladivostok", "Asia/Yakutsk", "Asia/Yangon", "Asia/Yekaterinburg", "Asia/Yerevan",
"Atlantic/Azores", "Atlantic/Bermuda", "Atlantic/Canary", "Atlantic/Cape_Verde", "Atlantic/Faeroe",
"Atlantic/Faroe", "Atlantic/Jan_Mayen", "Atlantic/Madeira", "Atlantic/Reykjavik",
"Atlantic/South_Georgia", "Atlantic/St_Helena", "Atlantic/Stanley", "Australia/ACT", "Australia/Adelaide",
"Australia/Brisbane", "Australia/Broken_Hill", "Australia/Canberra", "Australia/Currie",
"Australia/Darwin", "Australia/Eucla", "Australia/Hobart", "Australia/LHI", "Australia/Lindeman",
"Australia/Lord_Howe", "Australia/Melbourne", "Australia/NSW", "Australia/North", "Australia/Perth",
"Australia/Queensland", "Australia/South", "Australia/Sydney", "Australia/Tasmania", "Australia/Victoria",
"Australia/West", "Australia/Yancowinna", "Brazil/Acre", "Brazil/DeNoronha", "Brazil/East", "Brazil/West",
"CET", "CST6CDT", "Canada/Atlantic", "Canada/Central", "Canada/Eastern", "Canada/Mountain",
"Canada/Newfoundland", "Canada/Pacific", "Canada/Saskatchewan", "Canada/Yukon", "Chile/Continental",
"Chile/EasterIsland", "Cuba", "EET", "EST", "EST5EDT", "Egypt", "Eire", "Etc/GMT", "Etc/GMT+0",
"Etc/GMT+1", "Etc/GMT+10", "Etc/GMT+11", "Etc/GMT+12", "Etc/GMT+2", "Etc/GMT+3", "Etc/GMT+4", "Etc/GMT+5",
"Etc/GMT+6", "Etc/GMT+7", "Etc/GMT+8", "Etc/GMT+9", "Etc/GMT-0", "Etc/GMT-1", "Etc/GMT-10", "Etc/GMT-11",
"Etc/GMT-12", "Etc/GMT-13", "Etc/GMT-14", "Etc/GMT-2", "Etc/GMT-3", "Etc/GMT-4", "Etc/GMT-5", "Etc/GMT-6",
"Etc/GMT-7", "Etc/GMT-8", "Etc/GMT-9", "Etc/GMT0", "Etc/Greenwich", "Etc/UCT", "Etc/UTC", "Etc/Universal",
"Etc/Zulu", "Europe/Amsterdam", "Europe/Andorra", "Europe/Astrakhan", "Europe/Athens", "Europe/Belfast",
"Europe/Belgrade", "Europe/Berlin", "Europe/Bratislava", "Europe/Brussels", "Europe/Bucharest",
"Europe/Budapest", "Europe/Busingen", "Europe/Chisinau", "Europe/Copenhagen", "Europe/Dublin",
"Europe/Gibraltar", "Europe/Guernsey", "Europe/Helsinki", "Europe/Isle_of_Man", "Europe/Istanbul",
"Europe/Jersey", "Europe/Kaliningrad", "Europe/Kiev", "Europe/Kirov", "Europe/Kyiv", "Europe/Lisbon",
"Europe/Ljubljana", "Europe/London", "Europe/Luxembourg", "Europe/Madrid", "Europe/Malta",
"Europe/Mariehamn", "Europe/Minsk", "Europe/Monaco", "Europe/Moscow", "Europe/Nicosia", "Europe/Oslo",
"Europe/Paris", "Europe/Podgorica", "Europe/Prague", "Europe/Riga", "Europe/Rome", "Europe/Samara",
"Europe/San_Marino", "Europe/Sarajevo", "Europe/Saratov", "Europe/Simferopol", "Europe/Skopje",
"Europe/Sofia", "Europe/Stockholm", "Europe/Tallinn", "Europe/Tirane", "Europe/Tiraspol",
"Europe/Ulyanovsk", "Europe/Uzhgorod", "Europe/Vaduz", "Europe/Vatican", "Europe/Vienna",
"Europe/Vilnius", "Europe/Volgograd", "Europe/Warsaw", "Europe/Zagreb", "Europe/Zaporozhye",
"Europe/Zurich", "Factory", "GB", "GB-Eire", "GMT", "GMT+0", "GMT+1", "GMT+10", "GMT+11", "GMT+12",
"GMT+13", "GMT+14", "GMT+2", "GMT+3", "GMT+4", "GMT+5", "GMT+6", "GMT+7", "GMT+8", "GMT+9", "GMT-0",
"GMT-1", "GMT-10", "GMT-11", "GMT-12", "GMT-2", "GMT-3", "GMT-4", "GMT-5", "GMT-6", "GMT-7", "GMT-8",
"GMT-9", "GMT0", "Greenwich", "HST", "Hongkong", "Iceland", "Indian/Antananarivo", "Indian/Chagos",
"Indian/Christmas", "Indian/Cocos", "Indian/Comoro", "Indian/Kerguelen", "Indian/Mahe", "Indian/Maldives",
"Indian/Mauritius", "Indian/Mayotte", "Indian/Reunion", "Iran", "Israel", "Jamaica", "Japan", "Kwajalein",
"Libya", "MET", "MST", "MST7MDT", "Mexico/BajaNorte", "Mexico/BajaSur", "Mexico/General", "NZ", "NZ-CHAT",
"Navajo", "PRC", "PST8PDT", "Pacific/Apia", "Pacific/Auckland", "Pacific/Bougainville", "Pacific/Chatham",
"Pacific/Chuuk", "Pacific/Easter", "Pacific/Efate", "Pacific/Enderbury", "Pacific/Fakaofo",
"Pacific/Fiji", "Pacific/Funafuti", "Pacific/Galapagos", "Pacific/Gambier", "Pacific/Guadalcanal",
"Pacific/Guam", "Pacific/Honolulu", "Pacific/Johnston", "Pacific/Kanton", "Pacific/Kiritimati",
"Pacific/Kosrae", "Pacific/Kwajalein", "Pacific/Majuro", "Pacific/Marquesas", "Pacific/Midway",
"Pacific/Nauru", "Pacific/Niue", "Pacific/Norfolk", "Pacific/Noumea", "Pacific/Pago_Pago",
"Pacific/Palau", "Pacific/Pitcairn", "Pacific/Pohnpei", "Pacific/Ponape", "Pacific/Port_Moresby",
"Pacific/Rarotonga", "Pacific/Saipan", "Pacific/Samoa", "Pacific/Tahiti", "Pacific/Tarawa",
"Pacific/Tongatapu", "Pacific/Truk", "Pacific/Wake", "Pacific/Wallis", "Pacific/Yap", "Poland",
"Portugal", "ROC", "ROK", "Singapore", "Turkey", "UCT", "US/Alaska", "US/Aleutian", "US/Arizona",
"US/Central", "US/East-Indiana", "US/Eastern", "US/Hawaii", "US/Indiana-Starke", "US/Michigan",
"US/Mountain", "US/Pacific", "US/Samoa", "UTC", "Universal", "W-SU", "WET", "Zulu", "posixrules"
};
pub fn designations(allocator: std.mem.Allocator) !std.StringArrayHashMap(i32) {
var m = std.StringArrayHashMap(i32).init(allocator);
errdefer m.deinit();
try m.ensureUnusedCapacity(105);
try m.put("ACDT", 37800);
try m.put("ACST", 34200);
try m.put("ADDT", -7200);
try m.put("ADT", -10800);
try m.put("AEDT", 39600);
try m.put("AEST", 36000);
try m.put("AHDT", -32400);
try m.put("AHST", -36000);
try m.put("AKDT", -28800);
try m.put("AKST", -32400);
try m.put("AMT", 14400);
try m.put("AST", -14400);
try m.put("AWDT", 32400);
try m.put("AWST", 28800);
try m.put("BDST", 7200);
try m.put("BDT", -36000);
try m.put("BST", 3600);
try m.put("CAST", 10800);
try m.put("CAT", 7200);
try m.put("CDT", -18000);
try m.put("CEMT", 10800);
try m.put("CEST", 7200);
try m.put("CET", 3600);
try m.put("CPT", -18000);
try m.put("CST", -21600);
try m.put("CWT", -18000);
try m.put("ChST", 36000);
try m.put("DMT", -1521);
try m.put("EAT", 10800);
try m.put("EDT", -14400);
try m.put("EEST", 10800);
try m.put("EET", 7200);
try m.put("EMT", -26248);
try m.put("EPT", -14400);
try m.put("EST", -18000);
try m.put("EWT", -14400);
try m.put("FFMT", -14660);
try m.put("FMT", -4056);
try m.put("GDT", 39600);
try m.put("GMT", 0);
try m.put("GST", 36000);
try m.put("HDT", -32400);
try m.put("HKST", 32400);
try m.put("HKT", 28800);
try m.put("HKWT", 30600);
try m.put("HMT", 18000);
try m.put("HPT", -34200);
try m.put("HST", -36000);
try m.put("HWT", -34200);
try m.put("IDDT", 14400);
try m.put("IDT", 10800);
try m.put("IST", 19800);
try m.put("JDT", 36000);
try m.put("JMT", 8440);
try m.put("JST", 32400);
try m.put("KST", 32400);
try m.put("LST", 9394);
try m.put("MDST", 16279);
try m.put("MDT", -21600);
try m.put("MEST", 7200);
try m.put("MET", 3600);
try m.put("MMT", 23400);
try m.put("MPT", -21600);
try m.put("MSD", 14400);
try m.put("MSK", 10800);
try m.put("MST", -25200);
try m.put("MWT", -21600);
try m.put("NDDT", -5400);
try m.put("NDT", -9000);
try m.put("NPT", 20700);
try m.put("NST", -12600);
try m.put("NZDT", 46800);
try m.put("NZMT", 41400);
try m.put("NZST", 43200);
try m.put("PDT", -25200);
try m.put("PKST", 21600);
try m.put("PKT", 18000);
try m.put("PLMT", 25590);
try m.put("PMMT", 35312);
try m.put("PPMT", -17340);
try m.put("PPT", -25200);
try m.put("PST", -28800);
try m.put("PWT", -25200);
try m.put("QMT", -18840);
try m.put("SAST", 7200);
try m.put("SDMT", -16800);
try m.put("SJMT", -20173);
try m.put("SST", -39600);
try m.put("TBMT", 10751);
try m.put("TMT", 18000);
try m.put("UTC", 0);
try m.put("WAST", 7200);
try m.put("WAT", 3600);
try m.put("WEMT", 7200);
try m.put("WEST", 3600);
try m.put("WET", 0);
try m.put("WIB", 25200);
try m.put("WIT", 32400);
try m.put("WITA", 28800);
try m.put("WMT", 5040);
try m.put("YDDT", -25200);
try m.put("YDT", -28800);
try m.put("YPT", -28800);
try m.put("YST", -32400);
try m.put("YWT", -28800);
return m;
}
pub fn db(allocator: std.mem.Allocator) !std.StringArrayHashMap([]const u8) {
var m = std.StringArrayHashMap([]const u8).init(allocator);
errdefer m.deinit();
try m.ensureUnusedCapacity(624);
try m.put("Africa/Abidjan", data._d37f5b0e4017c5c540c81dc7a09e9cbbd91d2662de0bd589ff855052a271078e);
try m.put("Africa/Accra", data._d37f5b0e4017c5c540c81dc7a09e9cbbd91d2662de0bd589ff855052a271078e);
try m.put("Africa/Addis_Ababa", data._b5096d10cda1ef86614ac0dc9b159edcf57b5609c7f2f041a04718f860df1c6c);
try m.put("Africa/Algiers", data._fb151678d30c302661f1c63f5675b733ea12f6d55a331e5a3ba5b5707f9dc35d);
try m.put("Africa/Asmara", data._b5096d10cda1ef86614ac0dc9b159edcf57b5609c7f2f041a04718f860df1c6c);
try m.put("Africa/Asmera", data._b5096d10cda1ef86614ac0dc9b159edcf57b5609c7f2f041a04718f860df1c6c);
try m.put("Africa/Bamako", data._d37f5b0e4017c5c540c81dc7a09e9cbbd91d2662de0bd589ff855052a271078e);
try m.put("Africa/Bangui", data._4067756a503dd1568625ded15848ea9e694df0244525b6006cb5fbb2d998db74);
try m.put("Africa/Banjul", data._d37f5b0e4017c5c540c81dc7a09e9cbbd91d2662de0bd589ff855052a271078e);
try m.put("Africa/Bissau", data._7d29c692fdb4c721e209b622c78bdb1b95b4a9846d33236ae361a5a20ee3bc4d);
try m.put("Africa/Blantyre", data._7abe0c67a15f97931c72cbeaef702111cf901c46359cff966a701a65e815c225);
try m.put("Africa/Brazzaville", data._4067756a503dd1568625ded15848ea9e694df0244525b6006cb5fbb2d998db74);
try m.put("Africa/Bujumbura", data._7abe0c67a15f97931c72cbeaef702111cf901c46359cff966a701a65e815c225);
try m.put("Africa/Cairo", data._915f7ccb36fce7a8af1a8eac657bfeec904267612bee130bd5a36abaa81ba9d8);
try m.put("Africa/Casablanca", data._592c651dff09cdf966e7ad09543cceb46e0e872cdd265f8f48d6151a142bef6d);
try m.put("Africa/Ceuta", data._6ec8e023033e73c2df92acc118482aec6b5c8b4b985f50a891dd38521e2c60df);
try m.put("Africa/Conakry", data._d37f5b0e4017c5c540c81dc7a09e9cbbd91d2662de0bd589ff855052a271078e);
try m.put("Africa/Dakar", data._d37f5b0e4017c5c540c81dc7a09e9cbbd91d2662de0bd589ff855052a271078e);
try m.put("Africa/Dar_es_Salaam", data._b5096d10cda1ef86614ac0dc9b159edcf57b5609c7f2f041a04718f860df1c6c);
try m.put("Africa/Djibouti", data._b5096d10cda1ef86614ac0dc9b159edcf57b5609c7f2f041a04718f860df1c6c);
try m.put("Africa/Douala", data._4067756a503dd1568625ded15848ea9e694df0244525b6006cb5fbb2d998db74);
try m.put("Africa/El_Aaiun", data._2e9438e10ad4ef3612a9483f5bd51e7676e7aaf6bdcfac100d41b253cebee175);
try m.put("Africa/Freetown", data._d37f5b0e4017c5c540c81dc7a09e9cbbd91d2662de0bd589ff855052a271078e);
try m.put("Africa/Gaborone", data._7abe0c67a15f97931c72cbeaef702111cf901c46359cff966a701a65e815c225);
try m.put("Africa/Harare", data._7abe0c67a15f97931c72cbeaef702111cf901c46359cff966a701a65e815c225);
try m.put("Africa/Johannesburg", data._9d37dc0a548ed1ef7e2735c2c57fda9eb92112de951996ca6225383b214a3f42);
try m.put("Africa/Juba", data._37b6f1fd4c94a1187b705970b9935f12190aa44fe342640be274f5287b7d0d7a);
try m.put("Africa/Kampala", data._b5096d10cda1ef86614ac0dc9b159edcf57b5609c7f2f041a04718f860df1c6c);
try m.put("Africa/Khartoum", data._fd41a35969ac5ba1cf3501fc579549361f978dd86cfbc789246ae9756da7ef38);
try m.put("Africa/Kigali", data._7abe0c67a15f97931c72cbeaef702111cf901c46359cff966a701a65e815c225);
try m.put("Africa/Kinshasa", data._4067756a503dd1568625ded15848ea9e694df0244525b6006cb5fbb2d998db74);
try m.put("Africa/Lagos", data._4067756a503dd1568625ded15848ea9e694df0244525b6006cb5fbb2d998db74);
try m.put("Africa/Libreville", data._4067756a503dd1568625ded15848ea9e694df0244525b6006cb5fbb2d998db74);
try m.put("Africa/Lome", data._d37f5b0e4017c5c540c81dc7a09e9cbbd91d2662de0bd589ff855052a271078e);
try m.put("Africa/Luanda", data._4067756a503dd1568625ded15848ea9e694df0244525b6006cb5fbb2d998db74);
try m.put("Africa/Lubumbashi", data._7abe0c67a15f97931c72cbeaef702111cf901c46359cff966a701a65e815c225);
try m.put("Africa/Lusaka", data._7abe0c67a15f97931c72cbeaef702111cf901c46359cff966a701a65e815c225);
try m.put("Africa/Malabo", data._4067756a503dd1568625ded15848ea9e694df0244525b6006cb5fbb2d998db74);
try m.put("Africa/Maputo", data._7abe0c67a15f97931c72cbeaef702111cf901c46359cff966a701a65e815c225);
try m.put("Africa/Maseru", data._9d37dc0a548ed1ef7e2735c2c57fda9eb92112de951996ca6225383b214a3f42);
try m.put("Africa/Mbabane", data._9d37dc0a548ed1ef7e2735c2c57fda9eb92112de951996ca6225383b214a3f42);
try m.put("Africa/Mogadishu", data._b5096d10cda1ef86614ac0dc9b159edcf57b5609c7f2f041a04718f860df1c6c);
try m.put("Africa/Monrovia", data._e5b32f8c976cdb4b5789ec123323bc7d919b9036cdec082e63602ed7cd1e6076);
try m.put("Africa/Nairobi", data._b5096d10cda1ef86614ac0dc9b159edcf57b5609c7f2f041a04718f860df1c6c);
try m.put("Africa/Ndjamena", data._92a5eea850481eb1764915fa596b828866c1ea0e0a5aa7d0a7608162b67fcaeb);
try m.put("Africa/Niamey", data._4067756a503dd1568625ded15848ea9e694df0244525b6006cb5fbb2d998db74);
try m.put("Africa/Nouakchott", data._d37f5b0e4017c5c540c81dc7a09e9cbbd91d2662de0bd589ff855052a271078e);
try m.put("Africa/Ouagadougou", data._d37f5b0e4017c5c540c81dc7a09e9cbbd91d2662de0bd589ff855052a271078e);
try m.put("Africa/Porto-Novo", data._4067756a503dd1568625ded15848ea9e694df0244525b6006cb5fbb2d998db74);
try m.put("Africa/Sao_Tome", data._8622c2eed0b8b7ed3104f10dd751d53f44080a8628401bf4def9005e4d677f56);
try m.put("Africa/Timbuktu", data._d37f5b0e4017c5c540c81dc7a09e9cbbd91d2662de0bd589ff855052a271078e);
try m.put("Africa/Tripoli", data._9625341a543c54499448cd0f801e96dd2e559ab12d593c5d9afc011ce7082e32);
try m.put("Africa/Tunis", data._dae515a00547753794c537185a71bbf4ad2e0e65c03a7e2f992cde47da507e40);
try m.put("Africa/Windhoek", data._31117aed4d2ebf73765b0d9e19777868c20e856133752f41d7d73ac3c4a10a02);
try m.put("America/Adak", data._0998fd951c89609ff550cdfd5ca473b50cc38590071248c14345bc49f121745f);
try m.put("America/Anchorage", data._5fa2890205b6a6b80b63a26ff1c93db12e2e703543ca4155381d925248d524f5);
try m.put("America/Anguilla", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/Antigua", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/Araguaina", data._27302ed548c6bfb82fd2add242e9c6f7fb7b4573f8c5fbe7f70017374c8e31c3);
try m.put("America/Argentina/Buenos_Aires", data._601765e7857812510e2b07045baa8d31314a5ebc1b84af8ce54f5894ffcfe7de);
try m.put("America/Argentina/Catamarca", data._53b102cbf92851d2a71636a05c80b77f47c881ee0fb889e6dd178dcf17661745);
try m.put("America/Argentina/ComodRivadavia", data._53b102cbf92851d2a71636a05c80b77f47c881ee0fb889e6dd178dcf17661745);
try m.put("America/Argentina/Cordoba", data._809628c05c6334babb840db042b3a56c884a43ba942a68accaa0d3eae948029e);
try m.put("America/Argentina/Jujuy", data._34acaa1f27473b955187c76d59d19db2912201ddd6fdace47897157beaa1561e);
try m.put("America/Argentina/La_Rioja", data._fc711b8fd6e8961ea759e378358d29558eaabf6182eb0d464a2ab43d2c745f7c);
try m.put("America/Argentina/Mendoza", data._4550ee00bf9231cb9a235dbe100032cd500d6762d8c1bbaf864c12e79f7cee15);
try m.put("America/Argentina/Rio_Gallegos", data._4056563ef128e08e9ac61406b2981c8834bf7e421bb5ef4ca506e9002f7e216e);
try m.put("America/Argentina/Salta", data._0bcce19ac1b0db072f47dd0a1e7251f69331821ab6f5981d3a035acf6213b948);
try m.put("America/Argentina/San_Juan", data._b580ec37dc520ddaab43359f86e95865d3d54bcd8b31476c2117e88a2a452377);
try m.put("America/Argentina/San_Luis", data._1f4993f3de1b17765d05ac54b116a1725a2174e90044dc767bb52b4ec405c8ff);
try m.put("America/Argentina/Tucuman", data._ac43686e96a694176bc30782e67b9a4b64e933b6c4867bd91034372cd48214e9);
try m.put("America/Argentina/Ushuaia", data._9d354e27f6efdeab8997aa3cb264e1c5e35d92a31b7b2971ce3e53f78a49db41);
try m.put("America/Aruba", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/Asuncion", data._653a908d7b24b9de17e309e7fc88c8c10b1b99e6bd7a112f58de92a774b53e32);
try m.put("America/Atikokan", data._6d216fa790b3cb4f62218ec3523a18de4b46fccfa5d744c09c07b699b16b3f3e);
try m.put("America/Atka", data._0998fd951c89609ff550cdfd5ca473b50cc38590071248c14345bc49f121745f);
try m.put("America/Bahia", data._170f865cbe75433db6ae4a20a960fe377958176048e32ac2bdfa48796681b1d9);
try m.put("America/Bahia_Banderas", data._ad46879d8669e69e15cf3c47ef6b66d05b80c0b15f9e1f3fd9301e4c0dceaa26);
try m.put("America/Barbados", data._1737c860058fc8ba47552d2435f088998cd6ef351ce9d7128347f31873f5218f);
try m.put("America/Belem", data._dd6a3e22de59efb208689316799f0447f06f1b62b61b6f2fdfa9005482525ba0);
try m.put("America/Belize", data._ab31d8e304b60d24a3445f72d4ac823a43bf8703d6c13687b1547da3092c85c0);
try m.put("America/Blanc-Sablon", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/Boa_Vista", data._35ca02dd06cc40028fd71fa68045c18ab7907a9d07702466baef418fad0e293c);
try m.put("America/Bogota", data._57f017c91dbca3db7c2d962b8f039cb9245f095f0a1b79150840daffdf02342d);
try m.put("America/Boise", data._85c44a067162404593383d823cb12b927664b1f7b1034bbafbdc1e1294ad50b0);
try m.put("America/Buenos_Aires", data._601765e7857812510e2b07045baa8d31314a5ebc1b84af8ce54f5894ffcfe7de);
try m.put("America/Cambridge_Bay", data._28b6e959c8a837e1984b7aa9620ffc62d7282a7cae3622483b368e6858c593b3);
try m.put("America/Campo_Grande", data._5f1e711079002e90deda04fef199d275bb6865c863cc127ea007e94286a98ded);
try m.put("America/Cancun", data._b8d65426b5ca5217fdb3617aa87fec53a25a5188dc99ba893cfd026d1e9984d1);
try m.put("America/Caracas", data._d9955367bce76132b68dc34b1e6466e2931053563687d7e9f08e8d93b89d6500);
try m.put("America/Catamarca", data._53b102cbf92851d2a71636a05c80b77f47c881ee0fb889e6dd178dcf17661745);
try m.put("America/Cayenne", data._5823bde86e4d44ca1f4dfbe982599c9229f05f6aa1fb5ba7c6808f60add895fe);
try m.put("America/Cayman", data._6d216fa790b3cb4f62218ec3523a18de4b46fccfa5d744c09c07b699b16b3f3e);
try m.put("America/Chicago", data._7a4a63c31779b1f6a0bcca5db33702f69e7d4c0da79734d05f6090ad20e8ddb2);
try m.put("America/Chihuahua", data._5ed2b0363781e15a4b82f5d589f37f213b77e8264a590c5ca1d9f313e05ea194);
try m.put("America/Ciudad_Juarez", data._394f6718862e083f14b9a10b957056afe63d6b5276e562e78bf5ab233abed3b5);
try m.put("America/Coral_Harbour", data._6d216fa790b3cb4f62218ec3523a18de4b46fccfa5d744c09c07b699b16b3f3e);
try m.put("America/Cordoba", data._809628c05c6334babb840db042b3a56c884a43ba942a68accaa0d3eae948029e);
try m.put("America/Costa_Rica", data._ad05cca9f9af104bc798f152839f4ab6106993262884018eb86bfa2446ee13ab);
try m.put("America/Creston", data._8d222052bfcfa7d7b6e7e969d9d1c5f670e14cc33d53eb18ae5e36545a874375);
try m.put("America/Cuiaba", data._9eaa70e420830caa7529bd751be5e88a5dc8265821202e18f1ac1bfc91841f91);
try m.put("America/Curacao", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/Danmarkshavn", data._f206f44f6083cdc56e2b7a3470c99ecf92b9f2c6d08782f4050656a501614302);
try m.put("America/Dawson", data._5ed0953fab07109c208369210cde02f3614adb2a2f2942ad58fec08894e86eef);
try m.put("America/Dawson_Creek", data._5c124863e0ed6e95da8e87e12db342c5f22f6a635adc1de2da2112cd4c06cae1);
try m.put("America/Denver", data._2aa33d91e874baba8938f3db942cfbbde619699bd14ee7c57b85e1acbbd6dac7);
try m.put("America/Detroit", data._fd3d734ca90c78759ba857f154294a622541977ff7be4acda87b19e9301bcf06);
try m.put("America/Dominica", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/Edmonton", data._c89417d1bce49ab286b616aeb5b70ab95791da32c1b2ca6a5cc44f3e849bd919);
try m.put("America/Eirunepe", data._56a31fa69e8a101704c34458d49a379a6ff3f1bc8b17255d7bbabffc50ec03ae);
try m.put("America/El_Salvador", data._557f2acbf08bb9790b6610df60186c224f9c0b0d473026363248c50afad06597);
try m.put("America/Ensenada", data._5e09d1a662ce94cbc7d44e41db7fdb1a2c572623b3e6c5ba4a01749c4b83930e);
try m.put("America/Fort_Nelson", data._b60d1bbe676ea5587202a1774ba69aa24035e2346dd4bba6b29d1a7598d989ab);
try m.put("America/Fort_Wayne", data._a00098f3fe81be064938995161025cf389765f0b1fc3a0378ddb0b5d3718e4de);
try m.put("America/Fortaleza", data._811851500e7db105049fdfa32ded4655347d479757e5d006681f620d5fdb5019);
try m.put("America/Glace_Bay", data._fc1b912d2c912dc7f059ee84eac62638ed3ea0dd9916ac891316a8a868ff5bfa);
try m.put("America/Godthab", data._de914caf495676f65ce02da17e208e0631ecbc5f6ac74555e8a2fdb5a45e3262);
try m.put("America/Goose_Bay", data._ea178a34ae8580f5d01f1250b3405678239a246ac7d46506fcb51529ae2d0207);
try m.put("America/Grand_Turk", data._45b97e4c64fec5ab5cb396927df2dca9fc54e1dc3b553e4dbdd2ed717dd3d168);
try m.put("America/Grenada", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/Guadeloupe", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/Guatemala", data._35ed715689400d8919726dd815924fdfcd58a4ec87c5e1d4759b160eb26d0632);
try m.put("America/Guayaquil", data._39adbf12cbe849e69506eca72299624a587dd412438d3e70e319ee985e4bd280);
try m.put("America/Guyana", data._5cdbbe4615c48ca39f7f923e2a457e809724ba4089a7d360438ef67b85c07d20);
try m.put("America/Halifax", data._ed7d957524fcf4e3fde70a77ea29ccdf48164dabdc206cc1a713580ebaf34a95);
try m.put("America/Havana", data._c77043d5ca087c0c202233d1737389655eed08bec417aaea63bdac35383690ca);
try m.put("America/Hermosillo", data._7f31e5732d1305c58a2683219eaeed318bf9485342a76670c5f14d045a293fdf);
try m.put("America/Indiana/Indianapolis", data._a00098f3fe81be064938995161025cf389765f0b1fc3a0378ddb0b5d3718e4de);
try m.put("America/Indiana/Knox", data._a4d5afdcbec62f4c3bc777484f79d5be0ae8537d4d4263d1f8b253c62a0c95c6);
try m.put("America/Indiana/Marengo", data._1e0e05e101b2ab7f22313862c026f4f35724e77a77b832325a44c19d7c7848ce);
try m.put("America/Indiana/Petersburg", data._dbfb59d82a776ee2a1625b340d35ab3e98f18c959d3705438284ee89d7ee90f2);
try m.put("America/Indiana/Tell_City", data._c9d0f09cffcd6a2ae8f24da938a0658e3045df2dd962c9e01f00663bbc9f2c3a);
try m.put("America/Indiana/Vevay", data._65b63c107a6e73534d0c8844fcb140516038d1135231a228f0cd2b9c96096690);
try m.put("America/Indiana/Vincennes", data._f5a127efc3ae28fd8646b1a284c513a49a40d6f425c0479fdb7feaa8b0081feb);
try m.put("America/Indiana/Winamac", data._68103acfd0f77528065662e74cf9fed1b063a54e9da94fa2720b47e6b9cba8e4);
try m.put("America/Indianapolis", data._a00098f3fe81be064938995161025cf389765f0b1fc3a0378ddb0b5d3718e4de);
try m.put("America/Inuvik", data._1e65ad0b2b311e5f0aa08327413d197742f6363955d1ad09f1925f4a5667e89f);
try m.put("America/Iqaluit", data._0d61b2f04e66ef2a07fc1a2b8b3093835f09f64030c771cb079017a772ffac64);
try m.put("America/Jamaica", data._74d2dee47633591a71751a0aac5810cc077f09680a872e251f230a2da3fae742);
try m.put("America/Jujuy", data._34acaa1f27473b955187c76d59d19db2912201ddd6fdace47897157beaa1561e);
try m.put("America/Juneau", data._c4a3000417e2883b34da657264f973c0fc81e334a4980457d82645ab34549d1d);
try m.put("America/Kentucky/Louisville", data._ff1241305dfd3e9fed854a114c7cdf296baf443de6f4d7e6a56503ed922aea09);
try m.put("America/Kentucky/Monticello", data._ffb9477cc36bf36b1de89c9451e69e515c0432eeaa28b2cf942399cfa69000cb);
try m.put("America/Knox_IN", data._a4d5afdcbec62f4c3bc777484f79d5be0ae8537d4d4263d1f8b253c62a0c95c6);
try m.put("America/Kralendijk", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/La_Paz", data._3bddc50aaa323edaabff725449816f41a8ca7abc35f588b87ab54ce978c81941);
try m.put("America/Lima", data._664375d096600358d868fd88f645c0c022ff92bde9895f61491768073bdaa1b9);
try m.put("America/Los_Angeles", data._ea0e14e16facb1ab5bfc83ddc49d9cb6bf2dd1f60dc13baad67cf4bd3b6a70f9);
try m.put("America/Louisville", data._ff1241305dfd3e9fed854a114c7cdf296baf443de6f4d7e6a56503ed922aea09);
try m.put("America/Lower_Princes", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/Maceio", data._f4c2359bbdfc94b9bd4953e507fbc4ab3b8495b20d9ce6497a413eb8390ca010);
try m.put("America/Managua", data._8cc7858aaae420b88522d3b170d969cb88c7ff11b460d360bdb8ef8ae26cb51c);
try m.put("America/Manaus", data._77406c896720b6740ceb9bc7f992ef8cb6eee56ac5b900dc7981f1f98727733e);
try m.put("America/Marigot", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/Martinique", data._23c2fa5c339647796e2097201e7e51bc5a0ffe295c17201805a663a89508eee7);
try m.put("America/Matamoros", data._067a4cb88d9577fbe956eec3074d1557f17efe6d9ce5bd8c78f53ba76e2e4829);
try m.put("America/Mazatlan", data._43431ca9253ec139b4a575f7f5f2bc86ededbb0aa5e87e471d3c7a2e3eff387c);
try m.put("America/Mendoza", data._4550ee00bf9231cb9a235dbe100032cd500d6762d8c1bbaf864c12e79f7cee15);
try m.put("America/Menominee", data._7ecc31d7f08b79393678f1519b7db436d2a7aa485107730007757a5f5446fad8);
try m.put("America/Merida", data._484edc43d71168231fa26d7f41cca3aaf95b21e809d28f19976ff56da663dc4f);
try m.put("America/Metlakatla", data._fcfac5dd64737bf0a6a805641af375a0407d348d77cd9b9fa95d10e324a0c5a2);
try m.put("America/Mexico_City", data._3d530d778e7bb0b4c2ec14a86ddc032f97e527dd22f85a6ea9ef055178667d8c);
try m.put("America/Miquelon", data._d8edf1760ad7a621b31b8afcb784c791fc31e4ae9b8d302a4d919566517a5bb5);
try m.put("America/Moncton", data._1ca593a5260fe7c5c77dbf3acc2ea87c7a6fa1afcd7043429794cccfe2b9b738);
try m.put("America/Monterrey", data._ac50bce03378f4aacbbe0ddabf68f670f0dc8dd2c223e84091f60278dc1acaef);
try m.put("America/Montevideo", data._a6b12eb23f7c4861e37a5436b888e1b49708e32e0789c08a4c0b0bbe55edcd06);
try m.put("America/Montreal", data._8683fb72d087ca979341bbd1165cfa032a7d8665733a6887ee97fd08c462c441);
try m.put("America/Montserrat", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/Nassau", data._8683fb72d087ca979341bbd1165cfa032a7d8665733a6887ee97fd08c462c441);
try m.put("America/New_York", data._958219f7ddc3dbb9f933e40468a2df2f8b27aabb244ad4f31db12be50dfdbd78);
try m.put("America/Nipigon", data._8683fb72d087ca979341bbd1165cfa032a7d8665733a6887ee97fd08c462c441);
try m.put("America/Nome", data._a9f966695411b9d328a6d7584db1fa569f58c3f15d0696b335de529bcaf8ca20);
try m.put("America/Noronha", data._c2ebd6ba5c1ba8f8ff8e45ec2ee6d1d7f6ef169956f6fa8c4abfcd66ed1fc4b9);
try m.put("America/North_Dakota/Beulah", data._172912824cec856ca262aac283493bf6db061574ce65997c4d4d358091492a4a);
try m.put("America/North_Dakota/Center", data._1106a22664c32919ad97278984cbe357dff38fdf7f6a6399e1842104066995cf);
try m.put("America/North_Dakota/New_Salem", data._906689f327946706422421789061bc7e1bb40c22772d6ba8caa7d46c3ed9aa21);
try m.put("America/Nuuk", data._de914caf495676f65ce02da17e208e0631ecbc5f6ac74555e8a2fdb5a45e3262);
try m.put("America/Ojinaga", data._e48e3912bf7df8410664ba40c811978dfe2e80666f13dddd6f87aa74d006abf7);
try m.put("America/Panama", data._6d216fa790b3cb4f62218ec3523a18de4b46fccfa5d744c09c07b699b16b3f3e);
try m.put("America/Pangnirtung", data._0d61b2f04e66ef2a07fc1a2b8b3093835f09f64030c771cb079017a772ffac64);
try m.put("America/Paramaribo", data._50dd1ee5d1828f880607bc278d58200e66f7b892d0a3f1cc553804c998b33f99);
try m.put("America/Phoenix", data._8d222052bfcfa7d7b6e7e969d9d1c5f670e14cc33d53eb18ae5e36545a874375);
try m.put("America/Port-au-Prince", data._853f9724bc31b92eff2c9b4e659ef1ee931a38a97ef01e9018e4293f62c06311);
try m.put("America/Port_of_Spain", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/Porto_Acre", data._450fb5c3f23b038d6b381db98c73dd84c70a2857ef4a241620f824dabe8d0d63);
try m.put("America/Porto_Velho", data._bea6075613c0d990a14ad73a5d1cf698ca0f2c64a4a1b96cd9fe29fab50e956f);
try m.put("America/Puerto_Rico", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/Punta_Arenas", data._577f9b3fe1e20c3994409ca23bcfb38c3306659d3e5394828a5ee642c677779c);
try m.put("America/Rainy_River", data._be001324d6d4690155d92e2e692b10194ac2cc3ed9cb8d1574e2c4b451ae724a);
try m.put("America/Rankin_Inlet", data._585c5d1f10aea645ced8fb497b1a84759fbf5a39c104543502ba779552a92a93);
try m.put("America/Recife", data._a3d0885358e38dc135b841a6c839f8d106719e249fc54f1d080299ea01ada56a);
try m.put("America/Regina", data._7e3e4291f891f115f4c8796f6b5e68a27236e674c9fc7f1b2d06c3ca249dff36);
try m.put("America/Resolute", data._1cff22b594f581018824b5c4ea6269d3a4b424b691697fb1e7b9f85fed5f9daa);
try m.put("America/Rio_Branco", data._450fb5c3f23b038d6b381db98c73dd84c70a2857ef4a241620f824dabe8d0d63);
try m.put("America/Rosario", data._809628c05c6334babb840db042b3a56c884a43ba942a68accaa0d3eae948029e);
try m.put("America/Santa_Isabel", data._5e09d1a662ce94cbc7d44e41db7fdb1a2c572623b3e6c5ba4a01749c4b83930e);
try m.put("America/Santarem", data._d63c8033ef20a986b514b33befe2a5658246506dfdaace8272eae548bd66b419);
try m.put("America/Santiago", data._68b4fffbfef9b9d5e29bac1e92ad7f8ee97d4755a887595ecea9c36512b954ce);
try m.put("America/Santo_Domingo", data._3643da818d632050584cf3212877c2e9e6ba900cdeca653a49dcbe1b2e8da43b);
try m.put("America/Sao_Paulo", data._cd8a8d81d0ae4fd9b528e77cb02ca5ba10d1ba8bc98bafbbaf4be184d0a4031d);
try m.put("America/Scoresbysund", data._f94de0f37bebaaca486720aa99d92a560c9c158c5d29cd5c4c29f5f117ec2e49);
try m.put("America/Shiprock", data._2aa33d91e874baba8938f3db942cfbbde619699bd14ee7c57b85e1acbbd6dac7);
try m.put("America/Sitka", data._31e0edd9e0def26b9afc1f1dceb6af9169d977cafa37504f943d0f973c966255);
try m.put("America/St_Barthelemy", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/St_Johns", data._07d5b8ce712e4367f9e77d40c7c89f9a50febffbefd0dde4ecd9bd3a64841aee);
try m.put("America/St_Kitts", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/St_Lucia", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/St_Thomas", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/St_Vincent", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/Swift_Current", data._215ca14a45d9d6decbe4546d35a343bb0bb6f511f890b87464f6c5f5e58bb7f9);
try m.put("America/Tegucigalpa", data._b9be868d2070f9e549a64c5a7ff85f8508959d70f7449ac75fa239fa8defe692);
try m.put("America/Thule", data._d2746d4bc6f1d4b49b703c0120fff705702f9d291600686a06860e52e562bf4e);
try m.put("America/Thunder_Bay", data._8683fb72d087ca979341bbd1165cfa032a7d8665733a6887ee97fd08c462c441);
try m.put("America/Tijuana", data._5e09d1a662ce94cbc7d44e41db7fdb1a2c572623b3e6c5ba4a01749c4b83930e);
try m.put("America/Toronto", data._8683fb72d087ca979341bbd1165cfa032a7d8665733a6887ee97fd08c462c441);
try m.put("America/Tortola", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/Vancouver", data._e34b61c903ac69cd51c4bd0b3a5a6f72a551fc31c3ca17be8806a93a0f05b5dc);
try m.put("America/Virgin", data._657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f);
try m.put("America/Whitehorse", data._1ceb8b36e0b7a7565e7b5313400f89dd900e2312d8bdfa1bd134234b460bbd61);
try m.put("America/Winnipeg", data._be001324d6d4690155d92e2e692b10194ac2cc3ed9cb8d1574e2c4b451ae724a);
try m.put("America/Yakutat", data._115781dbefe43a145a581df3329998f125549bff90723f7124885f8bd764e431);
try m.put("America/Yellowknife", data._16342064f8a75ebf10ceb23e29bc99aea427457e4f26d164d03cb28cda03b6b1);
try m.put("Antarctica/Casey", data._4a1cf455fc98ec6ac52256f79f7d7edd9bd247ca50aa752554ee9f349493e9ff);
try m.put("Antarctica/Davis", data._0fd667c94bfefe34799dc233f2ccc5032e6faf7375c413d7b00b514cd2346aa3);
try m.put("Antarctica/DumontDUrville", data._3d760812cdafc26bd745543b1b879ffec432686280982930ae3666ce555c4e99);
try m.put("Antarctica/Macquarie", data._fa096e00cbda9923c62ed0e7e7efd9f485304eadf6c6d5358aa42188059995bc);
try m.put("Antarctica/Mawson", data._050a3f142f71e7cb5a152ce629dbc2b33385dbe644678bc9341c06802bc7ff2c);
try m.put("Antarctica/McMurdo", data._0cd2b2fe7d7a598f8040ac0adab206a92851f628e787a92f620514c1191a188f);
try m.put("Antarctica/Palmer", data._a890551b21d3f0b6e7c6111827e96322fb97fb8c23cc20d59b9147b1470a61b0);
try m.put("Antarctica/Rothera", data._639f8ccec735a17a0e690ffab6b12ccda137bc7feb28522d782b89fbd9c88c8b);
try m.put("Antarctica/South_Pole", data._0cd2b2fe7d7a598f8040ac0adab206a92851f628e787a92f620514c1191a188f);
try m.put("Antarctica/Syowa", data._2223def648f5c0d35e7b4a1c16ce7c60f909cc3e53c93fdfe821093671827539);
try m.put("Antarctica/Troll", data._d006a1fe239ec07c6308f15255f27677c1786c4f6d279d0e34bc5e9cff711e30);
try m.put("Antarctica/Vostok", data._981f14e3b6c442bab84b51ea8f108f19305ff794a654f400f810e4842d6f75d6);
try m.put("Arctic/Longyearbyen", data._221ac897654a1be77bf916269d63462fdba20fb73c6762de8351dfb552701fe1);
try m.put("Asia/Aden", data._2223def648f5c0d35e7b4a1c16ce7c60f909cc3e53c93fdfe821093671827539);
try m.put("Asia/Almaty", data._a7fd7a566ed380d6d8c076ac42ae73304bad66b38812d27838a73f594ddf1b39);
try m.put("Asia/Amman", data._177f4a37284621004e7a7f28e658ab33db1991759c58a554a680f9825e7bf10e);
try m.put("Asia/Anadyr", data._75031dd85336b8cade20000093b9e9c51c7e65ad20034aa38b81881d44e034c6);
try m.put("Asia/Aqtau", data._9dbcedb67be3e1f0beaa6651eaf448b11de2c8795235cc0c267f78cff5946824);
try m.put("Asia/Aqtobe", data._f2a1991f627f8121c95929b407c16fef74d9648f2ab7d20cfab86381c61bb9d7);
try m.put("Asia/Ashgabat", data._c346dbe3b4d0ea64561c999695015f7bdd7d858a15ab8a2855f72e01ca0540b8);
try m.put("Asia/Ashkhabad", data._c346dbe3b4d0ea64561c999695015f7bdd7d858a15ab8a2855f72e01ca0540b8);
try m.put("Asia/Atyrau", data._d42bfd8c9537278cc463b5ce2db10c26af47d3311c632c0e95c7605361e06d90);
try m.put("Asia/Baghdad", data._37f3b764c455f6ce24f115476391a5f9a5d0cc420acecd9a5efa614b8194c4c0);
try m.put("Asia/Bahrain", data._83975017ffba92cf3e0f0ae32a0d9ea20f414bbcb9daadaea5813c5d55f0a439);
try m.put("Asia/Baku", data._37c79b4aec18c117296aa226b8d37be2dbde9ed3347b2aedbf2aaa4aa25b726b);
try m.put("Asia/Bangkok", data._dfed901ef8430760fae944464e6c7b6351a381ed2438567480243aa019640758);
try m.put("Asia/Barnaul", data._6ed0c60488298f2cf38e319fad62118a59db5eb69f27d73b95706b6f1e4fe618);
try m.put("Asia/Beirut", data._3bfae174a9b0d3c7cc68d40484bdbc9ea2c73c40a2add55014062150b1ce9e24);
try m.put("Asia/Bishkek", data._c3cbc3e896bd7d358c01aa7f92c124d43925adef64252026dece43dfcf863c6d);
try m.put("Asia/Brunei", data._7cac3432bb23604830c161ca86ed6e33d5f9e9c2e0d842954110b0089e1efbc8);
try m.put("Asia/Calcutta", data._2eed3d92d50018f597bcf8aa7b4cb5d9026271bc93fded839e51330919ddce8b);
try m.put("Asia/Chita", data._e5e484256a3225d4a44b8052b531855316c0397cf82c53b06a8284fcb312a319);
try m.put("Asia/Choibalsan", data._d7cd0546f7758efe92b29ccd906d34a7184caad22fd10384dd8841078c0018e3);
try m.put("Asia/Chongqing", data._8441e95b648bc455abd54cf5c4a4799fcfbbcfa0dbabdff23a75d3f17c4914df);
try m.put("Asia/Chungking", data._8441e95b648bc455abd54cf5c4a4799fcfbbcfa0dbabdff23a75d3f17c4914df);
try m.put("Asia/Colombo", data._1711f7859d8c9c6e50f828b4bd9b0c6562ea997d1f6f31170f09b14e9a2a1053);
try m.put("Asia/Dacca", data._7c2ce26a2839d5f073cdb7cbf3a61448af85308f9761e5aab8bc6566ccaa468d);
try m.put("Asia/Damascus", data._f815c741fa0e8b3287a9b4c7288f4e7f8125af0c567426ab9e48281feffd4866);
try m.put("Asia/Dhaka", data._7c2ce26a2839d5f073cdb7cbf3a61448af85308f9761e5aab8bc6566ccaa468d);
try m.put("Asia/Dili", data._a4ad6d34eaa53c55f1f4a7420a3b8784d9d41f6c5eecc88b9e0a93155b96a9f4);
try m.put("Asia/Dubai", data._f8cdaa940dd364d0e732833f2fa03816a68b1b589e750a14687c8e57555cbb92);
try m.put("Asia/Dushanbe", data._cbeaff0d11483a3817361e39115f3ef4493fe174a5252a8dc8789d8b8f564aaa);
try m.put("Asia/Famagusta", data._e26a45216e9764c69eba16d0329266c5d65b13175f8552162eb09253bd6d90c4);
try m.put("Asia/Gaza", data._8eea6c329c6d76cf88375cedb33855ba1b9a839c9abb759d146e7900c1489a73);
try m.put("Asia/Harbin", data._8441e95b648bc455abd54cf5c4a4799fcfbbcfa0dbabdff23a75d3f17c4914df);
try m.put("Asia/Hebron", data._38797b93d2275a5af47dabe4116adb28021a80658d779d9007181a94ccb593c6);
try m.put("Asia/Ho_Chi_Minh", data._0173eddcfa771c8c8c06c05e22735040a4ccde312b3e0ead2317b094a8d5435a);
try m.put("Asia/Hong_Kong", data._7f21ddc20d4f7043961b668d78b73294204f1495514d744f1b759598fb7e7df5);
try m.put("Asia/Hovd", data._0abdac16bf4770cc2680e5300d7280aa21ea87758c80fd97c0677b47592ac5f3);
try m.put("Asia/Irkutsk", data._d16c9eb04a5de3a1565d2ba85319b7380646f466dceb2e276ec066df6580e17e);
try m.put("Asia/Istanbul", data._6d634443f5ec2060e4bce83e4a65a7f2f8087438896c6005991295c37085f686);
try m.put("Asia/Jakarta", data._2afa86183d490a938db2bcf64d3f7b5509d2a0fcfe9cbaef9875a1c05cdae04e);
try m.put("Asia/Jayapura", data._18fc98dc623cea9ddf5365652e1dee21fcde9db2bb12967e8c09936ecdee6758);
try m.put("Asia/Jerusalem", data._d9985dc4801b5b8c6a6f11017792a119a74d07baaafd0f1fb65d1b49d276fa4e);
try m.put("Asia/Kabul", data._0dd9e702000666c53780d67d80e104b5139035e5437a5351676e642d24fcadc6);
try m.put("Asia/Kamchatka", data._8a84e660cca469956134bb165734358f4a98b13abb44e75ea83aee538d11cc95);
try m.put("Asia/Karachi", data._911c8aabbacf27c53f22ceadc965d9f85855e6869a39252b5fc581067d4677b8);
try m.put("Asia/Kashgar", data._981f14e3b6c442bab84b51ea8f108f19305ff794a654f400f810e4842d6f75d6);
try m.put("Asia/Kathmandu", data._40c78ac09b9af96927f472354272c8066323766bd1813c53eb7e8cc1036433fc);
try m.put("Asia/Katmandu", data._40c78ac09b9af96927f472354272c8066323766bd1813c53eb7e8cc1036433fc);
try m.put("Asia/Khandyga", data._b1f20c3917b748de2161a8f379526eca431852fd376075fc2ec948f0a2dbb1c3);
try m.put("Asia/Kolkata", data._2eed3d92d50018f597bcf8aa7b4cb5d9026271bc93fded839e51330919ddce8b);
try m.put("Asia/Krasnoyarsk", data._e26e811fb1cde2b0bb570067c531f111a1b9357227dde4bcd31464b3402b2499);
try m.put("Asia/Kuala_Lumpur", data._6a669875e8518ef461a6a56e541d753fffcf8146d8bb43d9b90e25808ce638ab);
try m.put("Asia/Kuching", data._7cac3432bb23604830c161ca86ed6e33d5f9e9c2e0d842954110b0089e1efbc8);
try m.put("Asia/Kuwait", data._2223def648f5c0d35e7b4a1c16ce7c60f909cc3e53c93fdfe821093671827539);
try m.put("Asia/Macao", data._5dcb2cced3dbfbf92c0b836c5d2773f3101342cce8eb976bbf64cd271a4c446c);
try m.put("Asia/Macau", data._5dcb2cced3dbfbf92c0b836c5d2773f3101342cce8eb976bbf64cd271a4c446c);
try m.put("Asia/Magadan", data._78e7fbb1ba166c9cd09a23940f65ad21e457bd16413a0414d7ccc316222c0496);
try m.put("Asia/Makassar", data._fdc369e7f136358be156833320334420c3842f7427153b504c7eeb7268c3577c);
try m.put("Asia/Manila", data._b72f593788c54c82df85b45547c127700dea3576a539bd34e7c69021c9faac0a);
try m.put("Asia/Muscat", data._f8cdaa940dd364d0e732833f2fa03816a68b1b589e750a14687c8e57555cbb92);
try m.put("Asia/Nicosia", data._674b7a6a1e33e9f17f69972d76ec8c9d68bfa869def5c4adb0e38780c10a28f8);
try m.put("Asia/Novokuznetsk", data._78033539ff2723002ac4fc542b995597f209453ecdc8bdc68d628fdea4ff3472);
try m.put("Asia/Novosibirsk", data._2944153ad63b0aace49557b19296ce31b64d3ea45b55da87559c1ccb6a4160da);
try m.put("Asia/Omsk", data._091bdc3f8397061491d43a0e1cea9ed1536f813c1b1a4b36b787541c74143db8);
try m.put("Asia/Oral", data._d522fa224b772edf13a3f03b60914a16de77d0ffabd13d70cc344e1cf4224443);
try m.put("Asia/Phnom_Penh", data._dfed901ef8430760fae944464e6c7b6351a381ed2438567480243aa019640758);
try m.put("Asia/Pontianak", data._855c38eba6183b106b09982c87c4f4c6c55a6c37cb3f51f49aed80d8a0630249);
try m.put("Asia/Pyongyang", data._2e42b47546e87b1518b953b0e97c0bb18f6a33b363cdd2111776f6d9c0028cc8);
try m.put("Asia/Qatar", data._83975017ffba92cf3e0f0ae32a0d9ea20f414bbcb9daadaea5813c5d55f0a439);
try m.put("Asia/Qostanay", data._10c1d6cb0ee38f98d04346fdb2bb9a9f703cbdb92db14acf3a0181d56a43c5f9);
try m.put("Asia/Qyzylorda", data._b7a70a732fa1678e4bebd4a457932c670b1f084d3bc55a8a18e91f9341e9998f);
try m.put("Asia/Rangoon", data._2e91532d80e1642564a1303b77ae1a32d5b9fddb3a1d21e1dea5e1995665590d);
try m.put("Asia/Riyadh", data._2223def648f5c0d35e7b4a1c16ce7c60f909cc3e53c93fdfe821093671827539);
try m.put("Asia/Saigon", data._0173eddcfa771c8c8c06c05e22735040a4ccde312b3e0ead2317b094a8d5435a);
try m.put("Asia/Sakhalin", data._1d335a57a51c755055350989a57d20389967002e75b28ddeddffba7640410042);
try m.put("Asia/Samarkand", data._c307d9e6cd395db132902d2433bd4cf18c9b80a86d8bb51d213aaff00322e4e8);
try m.put("Asia/Seoul", data._63851746376d1a2bbd03c5552c555c121c5d274e5de6ed294ab72884b0b58f4b);
try m.put("Asia/Shanghai", data._8441e95b648bc455abd54cf5c4a4799fcfbbcfa0dbabdff23a75d3f17c4914df);
try m.put("Asia/Singapore", data._6a669875e8518ef461a6a56e541d753fffcf8146d8bb43d9b90e25808ce638ab);
try m.put("Asia/Srednekolymsk", data._8f332db547246069364959a600cecc889e17dc91e552544b1d46047826f9bda7);
try m.put("Asia/Taipei", data._47bdd3b5f5ed4ce498c1cd16aa042e381883104b5e39406d894f6cdd99d90690);
try m.put("Asia/Tashkent", data._ac5369e613f8fda204f42a8b11cea0f34e8f02b936dbd8d76aff9cb0527b7814);
try m.put("Asia/Tbilisi", data._e5c78249e670ecbee72532585e4e7dd2b5a61c8c06f15d58edc7f2c7c96bc45e);
try m.put("Asia/Tehran", data._12b62f3b6255ec562cb288a0acf1807c996fcbe1aa695364e77d8d56308409d9);
try m.put("Asia/Tel_Aviv", data._d9985dc4801b5b8c6a6f11017792a119a74d07baaafd0f1fb65d1b49d276fa4e);
try m.put("Asia/Thimbu", data._87b0e3254ce0c02e5eb7f68581d4664c2904f0fc364572686dfd0141f7eb9bb7);
try m.put("Asia/Thimphu", data._87b0e3254ce0c02e5eb7f68581d4664c2904f0fc364572686dfd0141f7eb9bb7);
try m.put("Asia/Tokyo", data._770ae87519ad4e8ffcd51b313ad10c7b723e69cf34842bd8b2dad1d10364c058);
try m.put("Asia/Tomsk", data._77e4d24042d9761f306d76c4ad51ea1336f2ef435d1c35f9285f97385cb1c1c2);
try m.put("Asia/Ujung_Pandang", data._fdc369e7f136358be156833320334420c3842f7427153b504c7eeb7268c3577c);
try m.put("Asia/Ulaanbaatar", data._79a13f2d0fbb637984739f6f75c6a9512657f2bc78061f2cdac17772df1533a1);
try m.put("Asia/Ulan_Bator", data._79a13f2d0fbb637984739f6f75c6a9512657f2bc78061f2cdac17772df1533a1);
try m.put("Asia/Urumqi", data._981f14e3b6c442bab84b51ea8f108f19305ff794a654f400f810e4842d6f75d6);
try m.put("Asia/Ust-Nera", data._371039399380245bf063f334df5b96ce1ec0ac4874090b6f96f82fcd0ff684c2);
try m.put("Asia/Vientiane", data._dfed901ef8430760fae944464e6c7b6351a381ed2438567480243aa019640758);
try m.put("Asia/Vladivostok", data._e672da1c4f98da2d2c7c972895914d213fc718b1a05a1a9bdece3b696ff2de09);
try m.put("Asia/Yakutsk", data._d63aaa5d5221e59129c3beb6ee79bbad46fb2f5d88ac3eb24448ca439f6f8e8d);
try m.put("Asia/Yangon", data._2e91532d80e1642564a1303b77ae1a32d5b9fddb3a1d21e1dea5e1995665590d);
try m.put("Asia/Yekaterinburg", data._279a9dedb277978a369499bb3591fbb6cfcb1e63bb812da5f73f164f9e3973db);
try m.put("Asia/Yerevan", data._eb4b43b95b1118b9375388a9af8d2430cb05ac3af53c4258feec479396b9ce7b);
try m.put("Atlantic/Azores", data._4ae350e636208b6837d9bd02d5daf2d20672de37add0b5a71215b454cea19fda);
try m.put("Atlantic/Bermuda", data._7dd8ca6b5d1c46ddc83776f31ef0ae150c07936a30a72b1bdd294457b22e64b5);
try m.put("Atlantic/Canary", data._1fe292d5a766736243e091b45709ec0851994423cc384230b75ab18e7a5eeade);
try m.put("Atlantic/Cape_Verde", data._ea03f272e068b5abd16925d1cc9c5cecaf26e86ec22ec4910b0e3234a296bd52);
try m.put("Atlantic/Faeroe", data._36b55646ede432b1b13cb1b08c36625d37132d4dfe5c44fd68c059c6ac343d7e);
try m.put("Atlantic/Faroe", data._36b55646ede432b1b13cb1b08c36625d37132d4dfe5c44fd68c059c6ac343d7e);
try m.put("Atlantic/Jan_Mayen", data._221ac897654a1be77bf916269d63462fdba20fb73c6762de8351dfb552701fe1);
try m.put("Atlantic/Madeira", data._622eb9f044da5fbd3806b48d14ca207a53e1f5c65dd55807ce68196d16653384);
try m.put("Atlantic/Reykjavik", data._d37f5b0e4017c5c540c81dc7a09e9cbbd91d2662de0bd589ff855052a271078e);
try m.put("Atlantic/South_Georgia", data._f62bcca38178fe7119918cbd07a03c14054e9b2b778273977ac40737bf8806d7);
try m.put("Atlantic/St_Helena", data._d37f5b0e4017c5c540c81dc7a09e9cbbd91d2662de0bd589ff855052a271078e);
try m.put("Atlantic/Stanley", data._ebd9e671d5a0e2d099620556695d174d9e29658afe26ff32caeb9dbc2b806ae0);
try m.put("Australia/ACT", data._73e21fb954b10bc52ca7bd0cb75f23f9da561d0db5294fd81f23f9161f4b94a9);
try m.put("Australia/Adelaide", data._ba4d37856b86a6c120217d5c2ed4d7016d0e1741fe8a1d4f2691fc2c5bec16d0);
try m.put("Australia/Brisbane", data._6696d891077b7a3c0a811b0f1c0b2eb85adb0a76a68b049409e99208c0b04f10);
try m.put("Australia/Broken_Hill", data._afc7210ebf21a84cff6b45c6609b56effe787a322fb7266679ae2c803cdaeeba);
try m.put("Australia/Canberra", data._73e21fb954b10bc52ca7bd0cb75f23f9da561d0db5294fd81f23f9161f4b94a9);
try m.put("Australia/Currie", data._adcbfa719567258d90ab4be773c3056f0e9c2e68dfc0f39bd82e940ed3853fba);
try m.put("Australia/Darwin", data._1827d87f6b26d9de1e27ee2c72aea6a3fd31bb08b5a6056a1efa07e5d539df8f);
try m.put("Australia/Eucla", data._2b230687e7da5c271dea54dc5d1dabc0effab8908245af661cb57d1458dd18b1);
try m.put("Australia/Hobart", data._adcbfa719567258d90ab4be773c3056f0e9c2e68dfc0f39bd82e940ed3853fba);
try m.put("Australia/LHI", data._8db861acfac7d1a865d26a53f95fdb04de211e157f4f3de5f97583f040ce0c67);
try m.put("Australia/Lindeman", data._b19b347246119e2c76842d3cfc1b1d5f81b487ed1eb518a5d4935648e5f1db6e);
try m.put("Australia/Lord_Howe", data._8db861acfac7d1a865d26a53f95fdb04de211e157f4f3de5f97583f040ce0c67);
try m.put("Australia/Melbourne", data._fabe3cd0365e79817a8648d2a4e27500bd251d11848ae94a778136ef0aac2d2e);
try m.put("Australia/NSW", data._73e21fb954b10bc52ca7bd0cb75f23f9da561d0db5294fd81f23f9161f4b94a9);
try m.put("Australia/North", data._1827d87f6b26d9de1e27ee2c72aea6a3fd31bb08b5a6056a1efa07e5d539df8f);
try m.put("Australia/Perth", data._016e88408fe029a8617b65006e14cc2e572c6dd3f7b32417a11df47ec701c2e0);
try m.put("Australia/Queensland", data._6696d891077b7a3c0a811b0f1c0b2eb85adb0a76a68b049409e99208c0b04f10);
try m.put("Australia/South", data._ba4d37856b86a6c120217d5c2ed4d7016d0e1741fe8a1d4f2691fc2c5bec16d0);
try m.put("Australia/Sydney", data._73e21fb954b10bc52ca7bd0cb75f23f9da561d0db5294fd81f23f9161f4b94a9);
try m.put("Australia/Tasmania", data._adcbfa719567258d90ab4be773c3056f0e9c2e68dfc0f39bd82e940ed3853fba);
try m.put("Australia/Victoria", data._fabe3cd0365e79817a8648d2a4e27500bd251d11848ae94a778136ef0aac2d2e);
try m.put("Australia/West", data._016e88408fe029a8617b65006e14cc2e572c6dd3f7b32417a11df47ec701c2e0);
try m.put("Australia/Yancowinna", data._afc7210ebf21a84cff6b45c6609b56effe787a322fb7266679ae2c803cdaeeba);
try m.put("Brazil/Acre", data._450fb5c3f23b038d6b381db98c73dd84c70a2857ef4a241620f824dabe8d0d63);
try m.put("Brazil/DeNoronha", data._c2ebd6ba5c1ba8f8ff8e45ec2ee6d1d7f6ef169956f6fa8c4abfcd66ed1fc4b9);
try m.put("Brazil/East", data._cd8a8d81d0ae4fd9b528e77cb02ca5ba10d1ba8bc98bafbbaf4be184d0a4031d);
try m.put("Brazil/West", data._77406c896720b6740ceb9bc7f992ef8cb6eee56ac5b900dc7981f1f98727733e);
try m.put("CET", data._e87cf18702cb9ec31fc5bce20850fc9381f0563b913b6de92033c84d6dfc4317);
try m.put("CST6CDT", data._1c3cee8685b9a6f4723760005685ccf5a694b8056d2aa26f053578081c6e0c8c);
try m.put("Canada/Atlantic", data._ed7d957524fcf4e3fde70a77ea29ccdf48164dabdc206cc1a713580ebaf34a95);
try m.put("Canada/Central", data._be001324d6d4690155d92e2e692b10194ac2cc3ed9cb8d1574e2c4b451ae724a);
try m.put("Canada/Eastern", data._8683fb72d087ca979341bbd1165cfa032a7d8665733a6887ee97fd08c462c441);
try m.put("Canada/Mountain", data._c89417d1bce49ab286b616aeb5b70ab95791da32c1b2ca6a5cc44f3e849bd919);
try m.put("Canada/Newfoundland", data._07d5b8ce712e4367f9e77d40c7c89f9a50febffbefd0dde4ecd9bd3a64841aee);
try m.put("Canada/Pacific", data._e34b61c903ac69cd51c4bd0b3a5a6f72a551fc31c3ca17be8806a93a0f05b5dc);
try m.put("Canada/Saskatchewan", data._7e3e4291f891f115f4c8796f6b5e68a27236e674c9fc7f1b2d06c3ca249dff36);
try m.put("Canada/Yukon", data._1ceb8b36e0b7a7565e7b5313400f89dd900e2312d8bdfa1bd134234b460bbd61);
try m.put("Chile/Continental", data._68b4fffbfef9b9d5e29bac1e92ad7f8ee97d4755a887595ecea9c36512b954ce);
try m.put("Chile/EasterIsland", data._0971e81ded14ff4c33574eb9afdbc1b393c2660dbf088407f15c5d3de9aa95fc);
try m.put("Cuba", data._c77043d5ca087c0c202233d1737389655eed08bec417aaea63bdac35383690ca);
try m.put("EET", data._1c0450a60bb805ad53c83804bbd7ec50c86264134910a19ed101fefc310fe626);
try m.put("EST", data._68acd323128673bb87a62563f958f123a5a20942d25f6871db7538f0c560b8b3);
try m.put("EST5EDT", data._d93fb9680bc085bd92157b1b47bd9454bff1c8f3b05e754ce17eb3ee006a3d9f);
try m.put("Egypt", data._915f7ccb36fce7a8af1a8eac657bfeec904267612bee130bd5a36abaa81ba9d8);
try m.put("Eire", data._fab2c087e7bca2462c179e67bd74b05da1c608717d66473490b88a46d9e7157f);
try m.put("Etc/GMT", data._98db876d5fea89b556fdcee4af82d59f4ce11297e884c3c6f555d01c522b0ec9);
try m.put("Etc/GMT+0", data._98db876d5fea89b556fdcee4af82d59f4ce11297e884c3c6f555d01c522b0ec9);
try m.put("Etc/GMT+1", data._91b752130690ea10799c3480e12343ac996a68c49d8c5e0bd63033e86d7c3f03);
try m.put("Etc/GMT+10", data._1b8b19935caa992911ad2f210c98bdafe61aa2d4ecc59a694e6d87a8c1d917cc);
try m.put("Etc/GMT+11", data._cdabd98a4b8ebe04eae57fba447b4a32e6c1a7204b2ee0eea42ba4b186079d7b);
try m.put("Etc/GMT+12", data._28aa4b5c73a212e38bfba73b2c5a6fca71dee0d503f9649a16624c8db8a38bd9);
try m.put("Etc/GMT+2", data._2b5a449681ece44f1fc06153a2d037881b737abad75535667bc1e5f4fb3ac967);
try m.put("Etc/GMT+3", data._00f509f1b2dcc15945a5660035c44b471f49af80b409307e4556121c38cef791);
try m.put("Etc/GMT+4", data._e134d54b3929f0d3840bb979bae69359693055221735d211dce874a7360279f5);
try m.put("Etc/GMT+5", data._7026dcf86704c9e66844b4a916a56fee386579bde5de33cf0cf8786e051e6bfa);
try m.put("Etc/GMT+6", data._3bf792d2e9f74f8879f1efd39e573d3ee0261dfdda4f79de25064617869bae83);
try m.put("Etc/GMT+7", data._83b17610c47fad26784167c46c9ea90a628d331fb037459e79056a5e87e2a3f1);
try m.put("Etc/GMT+8", data._2d3bb9056a516694bb27591604f39a7f6c8ba92f58d66514983da75e5de15c24);
try m.put("Etc/GMT+9", data._261c0a4defbea54dc9deb1d3e4778e7b3b0e451b908be96c0aeda6f755ee172d);
try m.put("Etc/GMT-0", data._98db876d5fea89b556fdcee4af82d59f4ce11297e884c3c6f555d01c522b0ec9);
try m.put("Etc/GMT-1", data._41fafea33ed497604db08982e3ef5e3c896c552b5418a63f0111c4f25dda83d0);
try m.put("Etc/GMT-10", data._d7cc91273897c46444de9a58fc6a99122948151674a4546ef4bd4521bab1a6a1);
try m.put("Etc/GMT-11", data._0b2b400cc71281f356f56ad5f63c859de36b98321c1a7b07b2e95efadb09ab75);
try m.put("Etc/GMT-12", data._d010accc1c7959802703e3ce6b8365ebd07612f4373a1e12ba873a115428fc9e);
try m.put("Etc/GMT-13", data._928528ecf93f4f79e24ebd0e258e723803849a909999537f6b956ed20e269295);
try m.put("Etc/GMT-14", data._81e78e78cd87e28e62c6e65d2d8842c801b06181afddd910cceeced176ffe719);
try m.put("Etc/GMT-2", data._84c92ccd2c97da49076d62139755314ff127b25ec6b7f369dcf2fc970d21ab86);
try m.put("Etc/GMT-3", data._61696de705ca524597f7ba8e6efeb0999abb2f066ba57fcec04934024ceed9d5);
try m.put("Etc/GMT-4", data._5a94ab6520bef9312c9346213f02d5f78bdf70ac7ee16e3b18467a0dcfce2a52);
try m.put("Etc/GMT-5", data._a9a668b769c96f20df15e5af22341bc3f339e630d7ec2d5d495bc77ac00138b8);
try m.put("Etc/GMT-6", data._d6b3d1c436568f91e9a2f690939f079453547f9d7e2db3ebd3616de1096c0e5e);
try m.put("Etc/GMT-7", data._49ce0def94b7461ff2b86e2c31dc10062eb2cc07ffdcf16c12bf084ea0694dd2);
try m.put("Etc/GMT-8", data._0fafd91a78641b451172e27f36f5e8a21e17e53c3ff0bd596e3e5f75c9a45b00);
try m.put("Etc/GMT-9", data._f455e02ac3971f5f58aef44052180983dbf43e0f2c004a935464cbc2d02c72ee);
try m.put("Etc/GMT0", data._98db876d5fea89b556fdcee4af82d59f4ce11297e884c3c6f555d01c522b0ec9);
try m.put("Etc/Greenwich", data._98db876d5fea89b556fdcee4af82d59f4ce11297e884c3c6f555d01c522b0ec9);
try m.put("Etc/UCT", data._67a0dfbde9c61da219439719631db9982573a201c839556bb29e9448e5e94b13);
try m.put("Etc/UTC", data._67a0dfbde9c61da219439719631db9982573a201c839556bb29e9448e5e94b13);
try m.put("Etc/Universal", data._67a0dfbde9c61da219439719631db9982573a201c839556bb29e9448e5e94b13);
try m.put("Etc/Zulu", data._67a0dfbde9c61da219439719631db9982573a201c839556bb29e9448e5e94b13);
try m.put("Europe/Amsterdam", data._9a90c694240f02e0a010f3b3b5ae7943a61a94d6d5c7bbc147a98e792c6d02bb);
try m.put("Europe/Andorra", data._3be2209487512fc75885f48e6a87e2b953c6352051fe3880b985a81a85ebe2b1);
try m.put("Europe/Astrakhan", data._7dd18a8ec78d3aae39a9e67a0264be64be3679d6db4ff402abd5bfd4cd2cbf51);
try m.put("Europe/Athens", data._331d0e55f2a10dc3cda9ca379ee3173caf6c02ca82405699d0cc4d034278c3e7);
try m.put("Europe/Belfast", data._63879c489ae234760936ed1d074acc1e2e4f785537900ac7854cc6c29303b074);
try m.put("Europe/Belgrade", data._8011374faf92b972f729b49137b75f9d88c76641419030f0d7b63603d4399985);
try m.put("Europe/Berlin", data._221ac897654a1be77bf916269d63462fdba20fb73c6762de8351dfb552701fe1);
try m.put("Europe/Bratislava", data._3d44b9577c44b8fbba05485ee9d47fee842bcbebbdd735ee1417679ec2c92742);
try m.put("Europe/Brussels", data._9a90c694240f02e0a010f3b3b5ae7943a61a94d6d5c7bbc147a98e792c6d02bb);
try m.put("Europe/Bucharest", data._82243cbca1a71eca4d47aabd8e7f84c0536605334c10c2417c96ca600d9c5a32);
try m.put("Europe/Budapest", data._3c1f0795fc22df31dabc4f315069f606730c4cb5140759f27a1bc19e006215c5);
try m.put("Europe/Busingen", data._6106c4fe8472993a4128a90759d57f985701d461f4bee3f8b022b022391630b0);
try m.put("Europe/Chisinau", data._6fc8a3416123200727d5cacc540e37fecdd6cfff6805660d33b6c89e83a025b7);
try m.put("Europe/Copenhagen", data._221ac897654a1be77bf916269d63462fdba20fb73c6762de8351dfb552701fe1);
try m.put("Europe/Dublin", data._fab2c087e7bca2462c179e67bd74b05da1c608717d66473490b88a46d9e7157f);
try m.put("Europe/Gibraltar", data._0c7448eed3df7dc8a1061b17980e2ddb3aeebc46c397e207ad23889f8a386546);
try m.put("Europe/Guernsey", data._63879c489ae234760936ed1d074acc1e2e4f785537900ac7854cc6c29303b074);
try m.put("Europe/Helsinki", data._d0447ba0f30123155a30acb81dda62e3288b5b86d920c00417f5b4dc70ac4d41);
try m.put("Europe/Isle_of_Man", data._63879c489ae234760936ed1d074acc1e2e4f785537900ac7854cc6c29303b074);
try m.put("Europe/Istanbul", data._6d634443f5ec2060e4bce83e4a65a7f2f8087438896c6005991295c37085f686);
try m.put("Europe/Jersey", data._63879c489ae234760936ed1d074acc1e2e4f785537900ac7854cc6c29303b074);
try m.put("Europe/Kaliningrad", data._afba6108a2dd9b1c01f1d3da9628e647e13e441ffc0db542fd494ed99356f5b4);
try m.put("Europe/Kiev", data._ace305bbafe572f8dacaff860f758bfa27d3b22d26bb74077e5eb257d7041d25);
try m.put("Europe/Kirov", data._e4b2e0f3503767985f0e2edeb1378d2a4fd4bc5b146361f524dbbdcf36d7f776);
try m.put("Europe/Kyiv", data._ace305bbafe572f8dacaff860f758bfa27d3b22d26bb74077e5eb257d7041d25);
try m.put("Europe/Lisbon", data._a9919ea8431b7872f9b314af41c6926036f3e30246d2af8c5efabe37ce03cd98);
try m.put("Europe/Ljubljana", data._8011374faf92b972f729b49137b75f9d88c76641419030f0d7b63603d4399985);
try m.put("Europe/London", data._63879c489ae234760936ed1d074acc1e2e4f785537900ac7854cc6c29303b074);
try m.put("Europe/Luxembourg", data._9a90c694240f02e0a010f3b3b5ae7943a61a94d6d5c7bbc147a98e792c6d02bb);
try m.put("Europe/Madrid", data._30579d5cf562d785dccc09e3386645e58ef876027233d1ca3c6e86140adcb0b9);
try m.put("Europe/Malta", data._1885f6dcb030bdfe880d23918ba9addab2763cb6f49b61f20609f1a04116deff);
try m.put("Europe/Mariehamn", data._d0447ba0f30123155a30acb81dda62e3288b5b86d920c00417f5b4dc70ac4d41);
try m.put("Europe/Minsk", data._0529614c5609d47207181f75595514761594a71d50436e1f1c62ad87adfb5446);
try m.put("Europe/Monaco", data._af74d377fc4115febb69b168ea9b78820efe91cb152c802956746c27f3f2ec49);
try m.put("Europe/Moscow", data._c3764207506c205c45f13d832a09fc8510d09ed2e0ef1de4f87b267a94c09bab);
try m.put("Europe/Nicosia", data._674b7a6a1e33e9f17f69972d76ec8c9d68bfa869def5c4adb0e38780c10a28f8);
try m.put("Europe/Oslo", data._221ac897654a1be77bf916269d63462fdba20fb73c6762de8351dfb552701fe1);
try m.put("Europe/Paris", data._af74d377fc4115febb69b168ea9b78820efe91cb152c802956746c27f3f2ec49);
try m.put("Europe/Podgorica", data._8011374faf92b972f729b49137b75f9d88c76641419030f0d7b63603d4399985);
try m.put("Europe/Prague", data._3d44b9577c44b8fbba05485ee9d47fee842bcbebbdd735ee1417679ec2c92742);
try m.put("Europe/Riga", data._7369f93e60d1be145e7834fe9d5723a8a3818ded9190d378cf35702a7d3667bc);
try m.put("Europe/Rome", data._31790b6f1787327b51abaa3c3f303d0e0d084ac97774be05bad67fc7346ec171);
try m.put("Europe/Samara", data._6ede4faf3322f3577701bc5d446779e95b74e13bc9c8e28c090130a81d163dc4);
try m.put("Europe/San_Marino", data._31790b6f1787327b51abaa3c3f303d0e0d084ac97774be05bad67fc7346ec171);
try m.put("Europe/Sarajevo", data._8011374faf92b972f729b49137b75f9d88c76641419030f0d7b63603d4399985);
try m.put("Europe/Saratov", data._d7c810eaccea15b0cd726d0a3fc6ad772161350d850d9561ab81eb30c4b3777c);
try m.put("Europe/Simferopol", data._55698526acd256eb122064cf2b6cb9d932c6f7bb6e74d9f11fda83559e30b7db);
try m.put("Europe/Skopje", data._8011374faf92b972f729b49137b75f9d88c76641419030f0d7b63603d4399985);
try m.put("Europe/Sofia", data._8ede21039ccbb68f8129a2e5d4d0eecfeda00c83d8122d0853e2b9969c8224d7);
try m.put("Europe/Stockholm", data._221ac897654a1be77bf916269d63462fdba20fb73c6762de8351dfb552701fe1);
try m.put("Europe/Tallinn", data._af3dd6f6329ab832d3455dd1a371db0cf8822eef883535ce5bd894e563341eb6);
try m.put("Europe/Tirane", data._5fd24743719e49613863d27ce6fdd4e051955f9e40654b776f61957cbf056ddb);
try m.put("Europe/Tiraspol", data._6fc8a3416123200727d5cacc540e37fecdd6cfff6805660d33b6c89e83a025b7);
try m.put("Europe/Ulyanovsk", data._a173623e0a470791670db57b51a0bb4292b2bb5f42ad6e44b78f7a88dccb42eb);
try m.put("Europe/Uzhgorod", data._ace305bbafe572f8dacaff860f758bfa27d3b22d26bb74077e5eb257d7041d25);
try m.put("Europe/Vaduz", data._6106c4fe8472993a4128a90759d57f985701d461f4bee3f8b022b022391630b0);
try m.put("Europe/Vatican", data._31790b6f1787327b51abaa3c3f303d0e0d084ac97774be05bad67fc7346ec171);
try m.put("Europe/Vienna", data._e00ec75b8c5277d8a2b011901b605c6535a572bc82a64c3664d41f9d41e46fe7);
try m.put("Europe/Vilnius", data._30b75e45c4f20edccf99eb14378335e6a18d5c91a8ddd2ab14d9c7fd1cc53643);
try m.put("Europe/Volgograd", data._0b3c50d87599164155c3cb7fac702d6b8e91c71c68e22135cf6981205546328f);
try m.put("Europe/Warsaw", data._1d8cab681088d868962e794b16821e6129eccbfd05a6b2b74dbf6b30791b6df2);
try m.put("Europe/Zagreb", data._8011374faf92b972f729b49137b75f9d88c76641419030f0d7b63603d4399985);
try m.put("Europe/Zaporozhye", data._ace305bbafe572f8dacaff860f758bfa27d3b22d26bb74077e5eb257d7041d25);
try m.put("Europe/Zurich", data._6106c4fe8472993a4128a90759d57f985701d461f4bee3f8b022b022391630b0);
try m.put("Factory", data._63636022c04f6f52ecd07ea00e8d1782fdf0d6e03a58a76923af4cdef1aa5748);
try m.put("GB", data._63879c489ae234760936ed1d074acc1e2e4f785537900ac7854cc6c29303b074);
try m.put("GB-Eire", data._63879c489ae234760936ed1d074acc1e2e4f785537900ac7854cc6c29303b074);
try m.put("GMT", data._98db876d5fea89b556fdcee4af82d59f4ce11297e884c3c6f555d01c522b0ec9);
try m.put("GMT+0", data._98db876d5fea89b556fdcee4af82d59f4ce11297e884c3c6f555d01c522b0ec9);
try m.put("GMT+1", data._41fafea33ed497604db08982e3ef5e3c896c552b5418a63f0111c4f25dda83d0);
try m.put("GMT+10", data._d7cc91273897c46444de9a58fc6a99122948151674a4546ef4bd4521bab1a6a1);
try m.put("GMT+11", data._0b2b400cc71281f356f56ad5f63c859de36b98321c1a7b07b2e95efadb09ab75);
try m.put("GMT+12", data._d010accc1c7959802703e3ce6b8365ebd07612f4373a1e12ba873a115428fc9e);
try m.put("GMT+13", data._928528ecf93f4f79e24ebd0e258e723803849a909999537f6b956ed20e269295);
try m.put("GMT+14", data._81e78e78cd87e28e62c6e65d2d8842c801b06181afddd910cceeced176ffe719);
try m.put("GMT+2", data._84c92ccd2c97da49076d62139755314ff127b25ec6b7f369dcf2fc970d21ab86);
try m.put("GMT+3", data._61696de705ca524597f7ba8e6efeb0999abb2f066ba57fcec04934024ceed9d5);
try m.put("GMT+4", data._5a94ab6520bef9312c9346213f02d5f78bdf70ac7ee16e3b18467a0dcfce2a52);
try m.put("GMT+5", data._a9a668b769c96f20df15e5af22341bc3f339e630d7ec2d5d495bc77ac00138b8);
try m.put("GMT+6", data._d6b3d1c436568f91e9a2f690939f079453547f9d7e2db3ebd3616de1096c0e5e);
try m.put("GMT+7", data._49ce0def94b7461ff2b86e2c31dc10062eb2cc07ffdcf16c12bf084ea0694dd2);
try m.put("GMT+8", data._0fafd91a78641b451172e27f36f5e8a21e17e53c3ff0bd596e3e5f75c9a45b00);
try m.put("GMT+9", data._f455e02ac3971f5f58aef44052180983dbf43e0f2c004a935464cbc2d02c72ee);
try m.put("GMT-0", data._98db876d5fea89b556fdcee4af82d59f4ce11297e884c3c6f555d01c522b0ec9);
try m.put("GMT-1", data._91b752130690ea10799c3480e12343ac996a68c49d8c5e0bd63033e86d7c3f03);
try m.put("GMT-10", data._1b8b19935caa992911ad2f210c98bdafe61aa2d4ecc59a694e6d87a8c1d917cc);
try m.put("GMT-11", data._cdabd98a4b8ebe04eae57fba447b4a32e6c1a7204b2ee0eea42ba4b186079d7b);
try m.put("GMT-12", data._28aa4b5c73a212e38bfba73b2c5a6fca71dee0d503f9649a16624c8db8a38bd9);
try m.put("GMT-2", data._2b5a449681ece44f1fc06153a2d037881b737abad75535667bc1e5f4fb3ac967);
try m.put("GMT-3", data._00f509f1b2dcc15945a5660035c44b471f49af80b409307e4556121c38cef791);
try m.put("GMT-4", data._e134d54b3929f0d3840bb979bae69359693055221735d211dce874a7360279f5);
try m.put("GMT-5", data._7026dcf86704c9e66844b4a916a56fee386579bde5de33cf0cf8786e051e6bfa);
try m.put("GMT-6", data._3bf792d2e9f74f8879f1efd39e573d3ee0261dfdda4f79de25064617869bae83);
try m.put("GMT-7", data._83b17610c47fad26784167c46c9ea90a628d331fb037459e79056a5e87e2a3f1);
try m.put("GMT-8", data._2d3bb9056a516694bb27591604f39a7f6c8ba92f58d66514983da75e5de15c24);
try m.put("GMT-9", data._261c0a4defbea54dc9deb1d3e4778e7b3b0e451b908be96c0aeda6f755ee172d);
try m.put("GMT0", data._98db876d5fea89b556fdcee4af82d59f4ce11297e884c3c6f555d01c522b0ec9);
try m.put("Greenwich", data._98db876d5fea89b556fdcee4af82d59f4ce11297e884c3c6f555d01c522b0ec9);
try m.put("HST", data._ee2a5cc76e8645c426c00f0ad25e8e76d20163cc403f9187276ff60b03a121f1);
try m.put("Hongkong", data._7f21ddc20d4f7043961b668d78b73294204f1495514d744f1b759598fb7e7df5);
try m.put("Iceland", data._d37f5b0e4017c5c540c81dc7a09e9cbbd91d2662de0bd589ff855052a271078e);
try m.put("Indian/Antananarivo", data._b5096d10cda1ef86614ac0dc9b159edcf57b5609c7f2f041a04718f860df1c6c);
try m.put("Indian/Chagos", data._6cc8361c70a1331176d022518aba4ca1ad84debe8c02a86b5baa03a9cf1642a8);
try m.put("Indian/Christmas", data._dfed901ef8430760fae944464e6c7b6351a381ed2438567480243aa019640758);
try m.put("Indian/Cocos", data._2e91532d80e1642564a1303b77ae1a32d5b9fddb3a1d21e1dea5e1995665590d);
try m.put("Indian/Comoro", data._b5096d10cda1ef86614ac0dc9b159edcf57b5609c7f2f041a04718f860df1c6c);
try m.put("Indian/Kerguelen", data._a49b58e2c1fb7518bd6c45955a1dbf69cda8a47237ed56a6b4b23acaa91db071);
try m.put("Indian/Mahe", data._f8cdaa940dd364d0e732833f2fa03816a68b1b589e750a14687c8e57555cbb92);
try m.put("Indian/Maldives", data._a49b58e2c1fb7518bd6c45955a1dbf69cda8a47237ed56a6b4b23acaa91db071);
try m.put("Indian/Mauritius", data._285c57becf847fb85cc73f06a574661fc7a085e1d438733935fb05ad2f467f5c);
try m.put("Indian/Mayotte", data._b5096d10cda1ef86614ac0dc9b159edcf57b5609c7f2f041a04718f860df1c6c);
try m.put("Indian/Reunion", data._f8cdaa940dd364d0e732833f2fa03816a68b1b589e750a14687c8e57555cbb92);
try m.put("Iran", data._12b62f3b6255ec562cb288a0acf1807c996fcbe1aa695364e77d8d56308409d9);
try m.put("Israel", data._d9985dc4801b5b8c6a6f11017792a119a74d07baaafd0f1fb65d1b49d276fa4e);
try m.put("Jamaica", data._74d2dee47633591a71751a0aac5810cc077f09680a872e251f230a2da3fae742);
try m.put("Japan", data._770ae87519ad4e8ffcd51b313ad10c7b723e69cf34842bd8b2dad1d10364c058);
try m.put("Kwajalein", data._08eb18e5c7aada08b1c01401ab75929fedefbaf489925cdde65605725af00f50);
try m.put("Libya", data._9625341a543c54499448cd0f801e96dd2e559ab12d593c5d9afc011ce7082e32);
try m.put("MET", data._aed559f918b2cfc6e04d2ce5365226d0c3e5e31641294f544796f587f6a06706);
try m.put("MST", data._88db57d643913ed549e190448cefaa5f5c0483ac2e4813472245e4396cad89eb);
try m.put("MST7MDT", data._2b5cb91483afbb09a640147cd901cb388104482afc7644dd70cfad5c483d9590);
try m.put("Mexico/BajaNorte", data._5e09d1a662ce94cbc7d44e41db7fdb1a2c572623b3e6c5ba4a01749c4b83930e);
try m.put("Mexico/BajaSur", data._43431ca9253ec139b4a575f7f5f2bc86ededbb0aa5e87e471d3c7a2e3eff387c);
try m.put("Mexico/General", data._3d530d778e7bb0b4c2ec14a86ddc032f97e527dd22f85a6ea9ef055178667d8c);
try m.put("NZ", data._0cd2b2fe7d7a598f8040ac0adab206a92851f628e787a92f620514c1191a188f);
try m.put("NZ-CHAT", data._8b9cc7427f164a79fcac8323b15048b2eea2fa7392ab9c849843c0f4ed6fb819);
try m.put("Navajo", data._2aa33d91e874baba8938f3db942cfbbde619699bd14ee7c57b85e1acbbd6dac7);
try m.put("PRC", data._8441e95b648bc455abd54cf5c4a4799fcfbbcfa0dbabdff23a75d3f17c4914df);
try m.put("PST8PDT", data._5e27e1ba3db932c6d4540ff56f402033b1eee2d6e8ac9e37e9e214863a33fde4);
try m.put("Pacific/Apia", data._687c5109468b320ba5d8b42d19788620160cfb8a2763fcbdd5a4beb9a7850558);
try m.put("Pacific/Auckland", data._0cd2b2fe7d7a598f8040ac0adab206a92851f628e787a92f620514c1191a188f);
try m.put("Pacific/Bougainville", data._10ee1b552e7c44c43353762b17295eb8dd89003037380ba2bdf07d98a2da0574);
try m.put("Pacific/Chatham", data._8b9cc7427f164a79fcac8323b15048b2eea2fa7392ab9c849843c0f4ed6fb819);
try m.put("Pacific/Chuuk", data._3d760812cdafc26bd745543b1b879ffec432686280982930ae3666ce555c4e99);
try m.put("Pacific/Easter", data._0971e81ded14ff4c33574eb9afdbc1b393c2660dbf088407f15c5d3de9aa95fc);
try m.put("Pacific/Efate", data._b62d78968d87833b38eb21a251e0b66b40651e656d305555f63424c2a80af9b2);
try m.put("Pacific/Enderbury", data._8abe4ea1d46c68bf1a4242c1e2c24720a1dbb59f27d8afe3442dde8a53f2d699);
try m.put("Pacific/Fakaofo", data._b54bd510377c46dac28eff64cbf8171f9b8423132e4f555f459f4d2505606b57);
try m.put("Pacific/Fiji", data._2e22bfaeb7bc1e19b132bd744b92a9818113c057b1aa562bd50530af1caae8e2);
try m.put("Pacific/Funafuti", data._5154a1bf5919fc1c604b9b3e6ba9b409e9364dc36a40b10e4c38575c9079da15);
try m.put("Pacific/Galapagos", data._37c1719843b77507f8e6b4eb6de6ae57d28850afacc9154a8ee3156ed3fb271c);
try m.put("Pacific/Gambier", data._cc3ae7c1e5c3be4f5d6a60ea0b2870e4ea7039eaec3d9ff38407ebdf9fd94a40);
try m.put("Pacific/Guadalcanal", data._4f657bcaf5d4927f688694a65dabe257aac8a4ab0c3bb7680eb2d743b1fa7146);
try m.put("Pacific/Guam", data._b774da2504fba6db4b4149ab308f0485dd582f1d5a028458319ae7182d70c426);
try m.put("Pacific/Honolulu", data._b8f9aeb477851a86419f191b393249cd40762dcb66a18148f12644ff7763ce8a);
try m.put("Pacific/Johnston", data._b8f9aeb477851a86419f191b393249cd40762dcb66a18148f12644ff7763ce8a);
try m.put("Pacific/Kanton", data._8abe4ea1d46c68bf1a4242c1e2c24720a1dbb59f27d8afe3442dde8a53f2d699);
try m.put("Pacific/Kiritimati", data._86e3a8a601361347e3a47d537dfa59755aa8f6ad4dc0f975c9b1f37caff5af58);
try m.put("Pacific/Kosrae", data._a130e88212533dd3c23cc55ebdeaceb7ac116b00a7ca43fb03125a564129c7ac);
try m.put("Pacific/Kwajalein", data._08eb18e5c7aada08b1c01401ab75929fedefbaf489925cdde65605725af00f50);
try m.put("Pacific/Majuro", data._5154a1bf5919fc1c604b9b3e6ba9b409e9364dc36a40b10e4c38575c9079da15);
try m.put("Pacific/Marquesas", data._51c278d3aafd7be6a98d5ace05e1d072f87b3211ff8f61b2742fbd839d05f4b0);
try m.put("Pacific/Midway", data._ff2a59039b4a6d7c7ae6adba0bb384e9e6be99ca71aded3d8ad6ca7e0b127f40);
try m.put("Pacific/Nauru", data._bdbc8dc9a2175ba7c62891f60ccfb72ec681170101d05c0bcb7e428ebadc4560);
try m.put("Pacific/Niue", data._0cb35370389ffb1cd69d1606a8b52d8c47da8f9ad4a25bcd8e12ec58e11db94e);
try m.put("Pacific/Norfolk", data._397bbf2875c86d6e957905a52cc5187c6ba54492b23fd44cf4c62c78520ed5a3);
try m.put("Pacific/Noumea", data._7f77b353ad63f2593bc88e54db2cf2d3554db57d9ea155d657f815f4f0313c74);
try m.put("Pacific/Pago_Pago", data._ff2a59039b4a6d7c7ae6adba0bb384e9e6be99ca71aded3d8ad6ca7e0b127f40);
try m.put("Pacific/Palau", data._f649de7c0428e399e8b90525f952f858af1bfd3c57533a3bae7d098d6a481787);
try m.put("Pacific/Pitcairn", data._28b9522562de6fabf72ca13f7073920dd2ba2735559288f59b4000af1091ef35);
try m.put("Pacific/Pohnpei", data._4f657bcaf5d4927f688694a65dabe257aac8a4ab0c3bb7680eb2d743b1fa7146);
try m.put("Pacific/Ponape", data._4f657bcaf5d4927f688694a65dabe257aac8a4ab0c3bb7680eb2d743b1fa7146);
try m.put("Pacific/Port_Moresby", data._3d760812cdafc26bd745543b1b879ffec432686280982930ae3666ce555c4e99);
try m.put("Pacific/Rarotonga", data._f202cb759da06dce84dd9598b6d33b9ddc53e5eb4ae2869113e7524169a00edc);
try m.put("Pacific/Saipan", data._b774da2504fba6db4b4149ab308f0485dd582f1d5a028458319ae7182d70c426);
try m.put("Pacific/Samoa", data._ff2a59039b4a6d7c7ae6adba0bb384e9e6be99ca71aded3d8ad6ca7e0b127f40);
try m.put("Pacific/Tahiti", data._2e477455ba6559ee24473382eec89aea25f991a33df3dae05fae9535784a9ef9);
try m.put("Pacific/Tarawa", data._5154a1bf5919fc1c604b9b3e6ba9b409e9364dc36a40b10e4c38575c9079da15);
try m.put("Pacific/Tongatapu", data._faf947c275b5ace8fcb30fdef5b51e72a43315c7db08d2002ef472a9e189eb39);
try m.put("Pacific/Truk", data._3d760812cdafc26bd745543b1b879ffec432686280982930ae3666ce555c4e99);
try m.put("Pacific/Wake", data._5154a1bf5919fc1c604b9b3e6ba9b409e9364dc36a40b10e4c38575c9079da15);
try m.put("Pacific/Wallis", data._5154a1bf5919fc1c604b9b3e6ba9b409e9364dc36a40b10e4c38575c9079da15);
try m.put("Pacific/Yap", data._3d760812cdafc26bd745543b1b879ffec432686280982930ae3666ce555c4e99);
try m.put("Poland", data._1d8cab681088d868962e794b16821e6129eccbfd05a6b2b74dbf6b30791b6df2);
try m.put("Portugal", data._a9919ea8431b7872f9b314af41c6926036f3e30246d2af8c5efabe37ce03cd98);
try m.put("ROC", data._47bdd3b5f5ed4ce498c1cd16aa042e381883104b5e39406d894f6cdd99d90690);
try m.put("ROK", data._63851746376d1a2bbd03c5552c555c121c5d274e5de6ed294ab72884b0b58f4b);
try m.put("Singapore", data._6a669875e8518ef461a6a56e541d753fffcf8146d8bb43d9b90e25808ce638ab);
try m.put("Turkey", data._6d634443f5ec2060e4bce83e4a65a7f2f8087438896c6005991295c37085f686);
try m.put("UCT", data._67a0dfbde9c61da219439719631db9982573a201c839556bb29e9448e5e94b13);
try m.put("US/Alaska", data._5fa2890205b6a6b80b63a26ff1c93db12e2e703543ca4155381d925248d524f5);
try m.put("US/Aleutian", data._0998fd951c89609ff550cdfd5ca473b50cc38590071248c14345bc49f121745f);
try m.put("US/Arizona", data._8d222052bfcfa7d7b6e7e969d9d1c5f670e14cc33d53eb18ae5e36545a874375);
try m.put("US/Central", data._7a4a63c31779b1f6a0bcca5db33702f69e7d4c0da79734d05f6090ad20e8ddb2);
try m.put("US/East-Indiana", data._a00098f3fe81be064938995161025cf389765f0b1fc3a0378ddb0b5d3718e4de);
try m.put("US/Eastern", data._958219f7ddc3dbb9f933e40468a2df2f8b27aabb244ad4f31db12be50dfdbd78);
try m.put("US/Hawaii", data._b8f9aeb477851a86419f191b393249cd40762dcb66a18148f12644ff7763ce8a);
try m.put("US/Indiana-Starke", data._a4d5afdcbec62f4c3bc777484f79d5be0ae8537d4d4263d1f8b253c62a0c95c6);
try m.put("US/Michigan", data._fd3d734ca90c78759ba857f154294a622541977ff7be4acda87b19e9301bcf06);
try m.put("US/Mountain", data._2aa33d91e874baba8938f3db942cfbbde619699bd14ee7c57b85e1acbbd6dac7);
try m.put("US/Pacific", data._ea0e14e16facb1ab5bfc83ddc49d9cb6bf2dd1f60dc13baad67cf4bd3b6a70f9);
try m.put("US/Samoa", data._ff2a59039b4a6d7c7ae6adba0bb384e9e6be99ca71aded3d8ad6ca7e0b127f40);
try m.put("UTC", data._67a0dfbde9c61da219439719631db9982573a201c839556bb29e9448e5e94b13);
try m.put("Universal", data._67a0dfbde9c61da219439719631db9982573a201c839556bb29e9448e5e94b13);
try m.put("W-SU", data._c3764207506c205c45f13d832a09fc8510d09ed2e0ef1de4f87b267a94c09bab);
try m.put("WET", data._9e889a4304319045168a0529daab457c060facbd4e1aba393afb0ccdf62797c0);
try m.put("Zulu", data._67a0dfbde9c61da219439719631db9982573a201c839556bb29e9448e5e94b13);
try m.put("posixrules", data._958219f7ddc3dbb9f933e40468a2df2f8b27aabb244ad4f31db12be50dfdbd78);
return m;
}
const data = struct {
pub const _55698526acd256eb122064cf2b6cb9d932c6f7bb6e74d9f11fda83559e30b7db = @embedFile("data/5/55698526acd256eb122064cf2b6cb9d932c6f7bb6e74d9f11fda83559e30b7db");
pub const _8683fb72d087ca979341bbd1165cfa032a7d8665733a6887ee97fd08c462c441 = @embedFile("data/8/8683fb72d087ca979341bbd1165cfa032a7d8665733a6887ee97fd08c462c441");
pub const _484edc43d71168231fa26d7f41cca3aaf95b21e809d28f19976ff56da663dc4f = @embedFile("data/4/484edc43d71168231fa26d7f41cca3aaf95b21e809d28f19976ff56da663dc4f");
pub const _23c2fa5c339647796e2097201e7e51bc5a0ffe295c17201805a663a89508eee7 = @embedFile("data/2/23c2fa5c339647796e2097201e7e51bc5a0ffe295c17201805a663a89508eee7");
pub const _855c38eba6183b106b09982c87c4f4c6c55a6c37cb3f51f49aed80d8a0630249 = @embedFile("data/8/855c38eba6183b106b09982c87c4f4c6c55a6c37cb3f51f49aed80d8a0630249");
pub const _c77043d5ca087c0c202233d1737389655eed08bec417aaea63bdac35383690ca = @embedFile("data/c/c77043d5ca087c0c202233d1737389655eed08bec417aaea63bdac35383690ca");
pub const _6d216fa790b3cb4f62218ec3523a18de4b46fccfa5d744c09c07b699b16b3f3e = @embedFile("data/6/6d216fa790b3cb4f62218ec3523a18de4b46fccfa5d744c09c07b699b16b3f3e");
pub const _63851746376d1a2bbd03c5552c555c121c5d274e5de6ed294ab72884b0b58f4b = @embedFile("data/6/63851746376d1a2bbd03c5552c555c121c5d274e5de6ed294ab72884b0b58f4b");
pub const _35ed715689400d8919726dd815924fdfcd58a4ec87c5e1d4759b160eb26d0632 = @embedFile("data/3/35ed715689400d8919726dd815924fdfcd58a4ec87c5e1d4759b160eb26d0632");
pub const _b62d78968d87833b38eb21a251e0b66b40651e656d305555f63424c2a80af9b2 = @embedFile("data/b/b62d78968d87833b38eb21a251e0b66b40651e656d305555f63424c2a80af9b2");
pub const _2afa86183d490a938db2bcf64d3f7b5509d2a0fcfe9cbaef9875a1c05cdae04e = @embedFile("data/2/2afa86183d490a938db2bcf64d3f7b5509d2a0fcfe9cbaef9875a1c05cdae04e");
pub const _0173eddcfa771c8c8c06c05e22735040a4ccde312b3e0ead2317b094a8d5435a = @embedFile("data/0/0173eddcfa771c8c8c06c05e22735040a4ccde312b3e0ead2317b094a8d5435a");
pub const _ac43686e96a694176bc30782e67b9a4b64e933b6c4867bd91034372cd48214e9 = @embedFile("data/a/ac43686e96a694176bc30782e67b9a4b64e933b6c4867bd91034372cd48214e9");
pub const _1885f6dcb030bdfe880d23918ba9addab2763cb6f49b61f20609f1a04116deff = @embedFile("data/1/1885f6dcb030bdfe880d23918ba9addab2763cb6f49b61f20609f1a04116deff");
pub const _911c8aabbacf27c53f22ceadc965d9f85855e6869a39252b5fc581067d4677b8 = @embedFile("data/9/911c8aabbacf27c53f22ceadc965d9f85855e6869a39252b5fc581067d4677b8");
pub const _61696de705ca524597f7ba8e6efeb0999abb2f066ba57fcec04934024ceed9d5 = @embedFile("data/6/61696de705ca524597f7ba8e6efeb0999abb2f066ba57fcec04934024ceed9d5");
pub const _ad46879d8669e69e15cf3c47ef6b66d05b80c0b15f9e1f3fd9301e4c0dceaa26 = @embedFile("data/a/ad46879d8669e69e15cf3c47ef6b66d05b80c0b15f9e1f3fd9301e4c0dceaa26");
pub const _1ceb8b36e0b7a7565e7b5313400f89dd900e2312d8bdfa1bd134234b460bbd61 = @embedFile("data/1/1ceb8b36e0b7a7565e7b5313400f89dd900e2312d8bdfa1bd134234b460bbd61");
pub const _83b17610c47fad26784167c46c9ea90a628d331fb037459e79056a5e87e2a3f1 = @embedFile("data/8/83b17610c47fad26784167c46c9ea90a628d331fb037459e79056a5e87e2a3f1");
pub const _36b55646ede432b1b13cb1b08c36625d37132d4dfe5c44fd68c059c6ac343d7e = @embedFile("data/3/36b55646ede432b1b13cb1b08c36625d37132d4dfe5c44fd68c059c6ac343d7e");
pub const _e48e3912bf7df8410664ba40c811978dfe2e80666f13dddd6f87aa74d006abf7 = @embedFile("data/e/e48e3912bf7df8410664ba40c811978dfe2e80666f13dddd6f87aa74d006abf7");
pub const _28b9522562de6fabf72ca13f7073920dd2ba2735559288f59b4000af1091ef35 = @embedFile("data/2/28b9522562de6fabf72ca13f7073920dd2ba2735559288f59b4000af1091ef35");
pub const _8622c2eed0b8b7ed3104f10dd751d53f44080a8628401bf4def9005e4d677f56 = @embedFile("data/8/8622c2eed0b8b7ed3104f10dd751d53f44080a8628401bf4def9005e4d677f56");
pub const _3be2209487512fc75885f48e6a87e2b953c6352051fe3880b985a81a85ebe2b1 = @embedFile("data/3/3be2209487512fc75885f48e6a87e2b953c6352051fe3880b985a81a85ebe2b1");
pub const _5e27e1ba3db932c6d4540ff56f402033b1eee2d6e8ac9e37e9e214863a33fde4 = @embedFile("data/5/5e27e1ba3db932c6d4540ff56f402033b1eee2d6e8ac9e37e9e214863a33fde4");
pub const _bea6075613c0d990a14ad73a5d1cf698ca0f2c64a4a1b96cd9fe29fab50e956f = @embedFile("data/b/bea6075613c0d990a14ad73a5d1cf698ca0f2c64a4a1b96cd9fe29fab50e956f");
pub const _fabe3cd0365e79817a8648d2a4e27500bd251d11848ae94a778136ef0aac2d2e = @embedFile("data/f/fabe3cd0365e79817a8648d2a4e27500bd251d11848ae94a778136ef0aac2d2e");
pub const _a9919ea8431b7872f9b314af41c6926036f3e30246d2af8c5efabe37ce03cd98 = @embedFile("data/a/a9919ea8431b7872f9b314af41c6926036f3e30246d2af8c5efabe37ce03cd98");
pub const _fab2c087e7bca2462c179e67bd74b05da1c608717d66473490b88a46d9e7157f = @embedFile("data/f/fab2c087e7bca2462c179e67bd74b05da1c608717d66473490b88a46d9e7157f");
pub const _7d29c692fdb4c721e209b622c78bdb1b95b4a9846d33236ae361a5a20ee3bc4d = @embedFile("data/7/7d29c692fdb4c721e209b622c78bdb1b95b4a9846d33236ae361a5a20ee3bc4d");
pub const _43431ca9253ec139b4a575f7f5f2bc86ededbb0aa5e87e471d3c7a2e3eff387c = @embedFile("data/4/43431ca9253ec139b4a575f7f5f2bc86ededbb0aa5e87e471d3c7a2e3eff387c");
pub const _3d760812cdafc26bd745543b1b879ffec432686280982930ae3666ce555c4e99 = @embedFile("data/3/3d760812cdafc26bd745543b1b879ffec432686280982930ae3666ce555c4e99");
pub const _00f509f1b2dcc15945a5660035c44b471f49af80b409307e4556121c38cef791 = @embedFile("data/0/00f509f1b2dcc15945a5660035c44b471f49af80b409307e4556121c38cef791");
pub const _3d44b9577c44b8fbba05485ee9d47fee842bcbebbdd735ee1417679ec2c92742 = @embedFile("data/3/3d44b9577c44b8fbba05485ee9d47fee842bcbebbdd735ee1417679ec2c92742");
pub const _1fe292d5a766736243e091b45709ec0851994423cc384230b75ab18e7a5eeade = @embedFile("data/1/1fe292d5a766736243e091b45709ec0851994423cc384230b75ab18e7a5eeade");
pub const _f202cb759da06dce84dd9598b6d33b9ddc53e5eb4ae2869113e7524169a00edc = @embedFile("data/f/f202cb759da06dce84dd9598b6d33b9ddc53e5eb4ae2869113e7524169a00edc");
pub const _7abe0c67a15f97931c72cbeaef702111cf901c46359cff966a701a65e815c225 = @embedFile("data/7/7abe0c67a15f97931c72cbeaef702111cf901c46359cff966a701a65e815c225");
pub const _b19b347246119e2c76842d3cfc1b1d5f81b487ed1eb518a5d4935648e5f1db6e = @embedFile("data/b/b19b347246119e2c76842d3cfc1b1d5f81b487ed1eb518a5d4935648e5f1db6e");
pub const _331d0e55f2a10dc3cda9ca379ee3173caf6c02ca82405699d0cc4d034278c3e7 = @embedFile("data/3/331d0e55f2a10dc3cda9ca379ee3173caf6c02ca82405699d0cc4d034278c3e7");
pub const _68103acfd0f77528065662e74cf9fed1b063a54e9da94fa2720b47e6b9cba8e4 = @embedFile("data/6/68103acfd0f77528065662e74cf9fed1b063a54e9da94fa2720b47e6b9cba8e4");
pub const _450fb5c3f23b038d6b381db98c73dd84c70a2857ef4a241620f824dabe8d0d63 = @embedFile("data/4/450fb5c3f23b038d6b381db98c73dd84c70a2857ef4a241620f824dabe8d0d63");
pub const _78033539ff2723002ac4fc542b995597f209453ecdc8bdc68d628fdea4ff3472 = @embedFile("data/7/78033539ff2723002ac4fc542b995597f209453ecdc8bdc68d628fdea4ff3472");
pub const _1d335a57a51c755055350989a57d20389967002e75b28ddeddffba7640410042 = @embedFile("data/1/1d335a57a51c755055350989a57d20389967002e75b28ddeddffba7640410042");
pub const _fa096e00cbda9923c62ed0e7e7efd9f485304eadf6c6d5358aa42188059995bc = @embedFile("data/f/fa096e00cbda9923c62ed0e7e7efd9f485304eadf6c6d5358aa42188059995bc");
pub const _37f3b764c455f6ce24f115476391a5f9a5d0cc420acecd9a5efa614b8194c4c0 = @embedFile("data/3/37f3b764c455f6ce24f115476391a5f9a5d0cc420acecd9a5efa614b8194c4c0");
pub const _10ee1b552e7c44c43353762b17295eb8dd89003037380ba2bdf07d98a2da0574 = @embedFile("data/1/10ee1b552e7c44c43353762b17295eb8dd89003037380ba2bdf07d98a2da0574");
pub const _6a669875e8518ef461a6a56e541d753fffcf8146d8bb43d9b90e25808ce638ab = @embedFile("data/6/6a669875e8518ef461a6a56e541d753fffcf8146d8bb43d9b90e25808ce638ab");
pub const _6cc8361c70a1331176d022518aba4ca1ad84debe8c02a86b5baa03a9cf1642a8 = @embedFile("data/6/6cc8361c70a1331176d022518aba4ca1ad84debe8c02a86b5baa03a9cf1642a8");
pub const _12b62f3b6255ec562cb288a0acf1807c996fcbe1aa695364e77d8d56308409d9 = @embedFile("data/1/12b62f3b6255ec562cb288a0acf1807c996fcbe1aa695364e77d8d56308409d9");
pub const _958219f7ddc3dbb9f933e40468a2df2f8b27aabb244ad4f31db12be50dfdbd78 = @embedFile("data/9/958219f7ddc3dbb9f933e40468a2df2f8b27aabb244ad4f31db12be50dfdbd78");
pub const _afba6108a2dd9b1c01f1d3da9628e647e13e441ffc0db542fd494ed99356f5b4 = @embedFile("data/a/afba6108a2dd9b1c01f1d3da9628e647e13e441ffc0db542fd494ed99356f5b4");
pub const _067a4cb88d9577fbe956eec3074d1557f17efe6d9ce5bd8c78f53ba76e2e4829 = @embedFile("data/0/067a4cb88d9577fbe956eec3074d1557f17efe6d9ce5bd8c78f53ba76e2e4829");
pub const _73e21fb954b10bc52ca7bd0cb75f23f9da561d0db5294fd81f23f9161f4b94a9 = @embedFile("data/7/73e21fb954b10bc52ca7bd0cb75f23f9da561d0db5294fd81f23f9161f4b94a9");
pub const _2e477455ba6559ee24473382eec89aea25f991a33df3dae05fae9535784a9ef9 = @embedFile("data/2/2e477455ba6559ee24473382eec89aea25f991a33df3dae05fae9535784a9ef9");
pub const _8eea6c329c6d76cf88375cedb33855ba1b9a839c9abb759d146e7900c1489a73 = @embedFile("data/8/8eea6c329c6d76cf88375cedb33855ba1b9a839c9abb759d146e7900c1489a73");
pub const _ac5369e613f8fda204f42a8b11cea0f34e8f02b936dbd8d76aff9cb0527b7814 = @embedFile("data/a/ac5369e613f8fda204f42a8b11cea0f34e8f02b936dbd8d76aff9cb0527b7814");
pub const _c3764207506c205c45f13d832a09fc8510d09ed2e0ef1de4f87b267a94c09bab = @embedFile("data/c/c3764207506c205c45f13d832a09fc8510d09ed2e0ef1de4f87b267a94c09bab");
pub const _be001324d6d4690155d92e2e692b10194ac2cc3ed9cb8d1574e2c4b451ae724a = @embedFile("data/b/be001324d6d4690155d92e2e692b10194ac2cc3ed9cb8d1574e2c4b451ae724a");
pub const _1e0e05e101b2ab7f22313862c026f4f35724e77a77b832325a44c19d7c7848ce = @embedFile("data/1/1e0e05e101b2ab7f22313862c026f4f35724e77a77b832325a44c19d7c7848ce");
pub const _5fd24743719e49613863d27ce6fdd4e051955f9e40654b776f61957cbf056ddb = @embedFile("data/5/5fd24743719e49613863d27ce6fdd4e051955f9e40654b776f61957cbf056ddb");
pub const _f8cdaa940dd364d0e732833f2fa03816a68b1b589e750a14687c8e57555cbb92 = @embedFile("data/f/f8cdaa940dd364d0e732833f2fa03816a68b1b589e750a14687c8e57555cbb92");
pub const _ab31d8e304b60d24a3445f72d4ac823a43bf8703d6c13687b1547da3092c85c0 = @embedFile("data/a/ab31d8e304b60d24a3445f72d4ac823a43bf8703d6c13687b1547da3092c85c0");
pub const _3bf792d2e9f74f8879f1efd39e573d3ee0261dfdda4f79de25064617869bae83 = @embedFile("data/3/3bf792d2e9f74f8879f1efd39e573d3ee0261dfdda4f79de25064617869bae83");
pub const _177f4a37284621004e7a7f28e658ab33db1991759c58a554a680f9825e7bf10e = @embedFile("data/1/177f4a37284621004e7a7f28e658ab33db1991759c58a554a680f9825e7bf10e");
pub const _664375d096600358d868fd88f645c0c022ff92bde9895f61491768073bdaa1b9 = @embedFile("data/6/664375d096600358d868fd88f645c0c022ff92bde9895f61491768073bdaa1b9");
pub const _a4d5afdcbec62f4c3bc777484f79d5be0ae8537d4d4263d1f8b253c62a0c95c6 = @embedFile("data/a/a4d5afdcbec62f4c3bc777484f79d5be0ae8537d4d4263d1f8b253c62a0c95c6");
pub const _87b0e3254ce0c02e5eb7f68581d4664c2904f0fc364572686dfd0141f7eb9bb7 = @embedFile("data/8/87b0e3254ce0c02e5eb7f68581d4664c2904f0fc364572686dfd0141f7eb9bb7");
pub const _853f9724bc31b92eff2c9b4e659ef1ee931a38a97ef01e9018e4293f62c06311 = @embedFile("data/8/853f9724bc31b92eff2c9b4e659ef1ee931a38a97ef01e9018e4293f62c06311");
pub const _4067756a503dd1568625ded15848ea9e694df0244525b6006cb5fbb2d998db74 = @embedFile("data/4/4067756a503dd1568625ded15848ea9e694df0244525b6006cb5fbb2d998db74");
pub const _dd6a3e22de59efb208689316799f0447f06f1b62b61b6f2fdfa9005482525ba0 = @embedFile("data/d/dd6a3e22de59efb208689316799f0447f06f1b62b61b6f2fdfa9005482525ba0");
pub const _56a31fa69e8a101704c34458d49a379a6ff3f1bc8b17255d7bbabffc50ec03ae = @embedFile("data/5/56a31fa69e8a101704c34458d49a379a6ff3f1bc8b17255d7bbabffc50ec03ae");
pub const _34acaa1f27473b955187c76d59d19db2912201ddd6fdace47897157beaa1561e = @embedFile("data/3/34acaa1f27473b955187c76d59d19db2912201ddd6fdace47897157beaa1561e");
pub const _3d530d778e7bb0b4c2ec14a86ddc032f97e527dd22f85a6ea9ef055178667d8c = @embedFile("data/3/3d530d778e7bb0b4c2ec14a86ddc032f97e527dd22f85a6ea9ef055178667d8c");
pub const _faf947c275b5ace8fcb30fdef5b51e72a43315c7db08d2002ef472a9e189eb39 = @embedFile("data/f/faf947c275b5ace8fcb30fdef5b51e72a43315c7db08d2002ef472a9e189eb39");
pub const _2944153ad63b0aace49557b19296ce31b64d3ea45b55da87559c1ccb6a4160da = @embedFile("data/2/2944153ad63b0aace49557b19296ce31b64d3ea45b55da87559c1ccb6a4160da");
pub const _79a13f2d0fbb637984739f6f75c6a9512657f2bc78061f2cdac17772df1533a1 = @embedFile("data/7/79a13f2d0fbb637984739f6f75c6a9512657f2bc78061f2cdac17772df1533a1");
pub const _0d61b2f04e66ef2a07fc1a2b8b3093835f09f64030c771cb079017a772ffac64 = @embedFile("data/0/0d61b2f04e66ef2a07fc1a2b8b3093835f09f64030c771cb079017a772ffac64");
pub const _557f2acbf08bb9790b6610df60186c224f9c0b0d473026363248c50afad06597 = @embedFile("data/5/557f2acbf08bb9790b6610df60186c224f9c0b0d473026363248c50afad06597");
pub const _3bfae174a9b0d3c7cc68d40484bdbc9ea2c73c40a2add55014062150b1ce9e24 = @embedFile("data/3/3bfae174a9b0d3c7cc68d40484bdbc9ea2c73c40a2add55014062150b1ce9e24");
pub const _9d354e27f6efdeab8997aa3cb264e1c5e35d92a31b7b2971ce3e53f78a49db41 = @embedFile("data/9/9d354e27f6efdeab8997aa3cb264e1c5e35d92a31b7b2971ce3e53f78a49db41");
pub const _f4c2359bbdfc94b9bd4953e507fbc4ab3b8495b20d9ce6497a413eb8390ca010 = @embedFile("data/f/f4c2359bbdfc94b9bd4953e507fbc4ab3b8495b20d9ce6497a413eb8390ca010");
pub const _5cdbbe4615c48ca39f7f923e2a457e809724ba4089a7d360438ef67b85c07d20 = @embedFile("data/5/5cdbbe4615c48ca39f7f923e2a457e809724ba4089a7d360438ef67b85c07d20");
pub const _d522fa224b772edf13a3f03b60914a16de77d0ffabd13d70cc344e1cf4224443 = @embedFile("data/d/d522fa224b772edf13a3f03b60914a16de77d0ffabd13d70cc344e1cf4224443");
pub const _0dd9e702000666c53780d67d80e104b5139035e5437a5351676e642d24fcadc6 = @embedFile("data/0/0dd9e702000666c53780d67d80e104b5139035e5437a5351676e642d24fcadc6");
pub const _c9d0f09cffcd6a2ae8f24da938a0658e3045df2dd962c9e01f00663bbc9f2c3a = @embedFile("data/c/c9d0f09cffcd6a2ae8f24da938a0658e3045df2dd962c9e01f00663bbc9f2c3a");
pub const _cbeaff0d11483a3817361e39115f3ef4493fe174a5252a8dc8789d8b8f564aaa = @embedFile("data/c/cbeaff0d11483a3817361e39115f3ef4493fe174a5252a8dc8789d8b8f564aaa");
pub const _a3d0885358e38dc135b841a6c839f8d106719e249fc54f1d080299ea01ada56a = @embedFile("data/a/a3d0885358e38dc135b841a6c839f8d106719e249fc54f1d080299ea01ada56a");
pub const _115781dbefe43a145a581df3329998f125549bff90723f7124885f8bd764e431 = @embedFile("data/1/115781dbefe43a145a581df3329998f125549bff90723f7124885f8bd764e431");
pub const _45b97e4c64fec5ab5cb396927df2dca9fc54e1dc3b553e4dbdd2ed717dd3d168 = @embedFile("data/4/45b97e4c64fec5ab5cb396927df2dca9fc54e1dc3b553e4dbdd2ed717dd3d168");
pub const _37c1719843b77507f8e6b4eb6de6ae57d28850afacc9154a8ee3156ed3fb271c = @embedFile("data/3/37c1719843b77507f8e6b4eb6de6ae57d28850afacc9154a8ee3156ed3fb271c");
pub const _2eed3d92d50018f597bcf8aa7b4cb5d9026271bc93fded839e51330919ddce8b = @embedFile("data/2/2eed3d92d50018f597bcf8aa7b4cb5d9026271bc93fded839e51330919ddce8b");
pub const _1e65ad0b2b311e5f0aa08327413d197742f6363955d1ad09f1925f4a5667e89f = @embedFile("data/1/1e65ad0b2b311e5f0aa08327413d197742f6363955d1ad09f1925f4a5667e89f");
pub const _8abe4ea1d46c68bf1a4242c1e2c24720a1dbb59f27d8afe3442dde8a53f2d699 = @embedFile("data/8/8abe4ea1d46c68bf1a4242c1e2c24720a1dbb59f27d8afe3442dde8a53f2d699");
pub const _07d5b8ce712e4367f9e77d40c7c89f9a50febffbefd0dde4ecd9bd3a64841aee = @embedFile("data/0/07d5b8ce712e4367f9e77d40c7c89f9a50febffbefd0dde4ecd9bd3a64841aee");
pub const _397bbf2875c86d6e957905a52cc5187c6ba54492b23fd44cf4c62c78520ed5a3 = @embedFile("data/3/397bbf2875c86d6e957905a52cc5187c6ba54492b23fd44cf4c62c78520ed5a3");
pub const _7c2ce26a2839d5f073cdb7cbf3a61448af85308f9761e5aab8bc6566ccaa468d = @embedFile("data/7/7c2ce26a2839d5f073cdb7cbf3a61448af85308f9761e5aab8bc6566ccaa468d");
pub const _7f31e5732d1305c58a2683219eaeed318bf9485342a76670c5f14d045a293fdf = @embedFile("data/7/7f31e5732d1305c58a2683219eaeed318bf9485342a76670c5f14d045a293fdf");
pub const _a4ad6d34eaa53c55f1f4a7420a3b8784d9d41f6c5eecc88b9e0a93155b96a9f4 = @embedFile("data/a/a4ad6d34eaa53c55f1f4a7420a3b8784d9d41f6c5eecc88b9e0a93155b96a9f4");
pub const _d7cd0546f7758efe92b29ccd906d34a7184caad22fd10384dd8841078c0018e3 = @embedFile("data/d/d7cd0546f7758efe92b29ccd906d34a7184caad22fd10384dd8841078c0018e3");
pub const _ee2a5cc76e8645c426c00f0ad25e8e76d20163cc403f9187276ff60b03a121f1 = @embedFile("data/e/ee2a5cc76e8645c426c00f0ad25e8e76d20163cc403f9187276ff60b03a121f1");
pub const _0b2b400cc71281f356f56ad5f63c859de36b98321c1a7b07b2e95efadb09ab75 = @embedFile("data/0/0b2b400cc71281f356f56ad5f63c859de36b98321c1a7b07b2e95efadb09ab75");
pub const _fc1b912d2c912dc7f059ee84eac62638ed3ea0dd9916ac891316a8a868ff5bfa = @embedFile("data/f/fc1b912d2c912dc7f059ee84eac62638ed3ea0dd9916ac891316a8a868ff5bfa");
pub const _4f657bcaf5d4927f688694a65dabe257aac8a4ab0c3bb7680eb2d743b1fa7146 = @embedFile("data/4/4f657bcaf5d4927f688694a65dabe257aac8a4ab0c3bb7680eb2d743b1fa7146");
pub const _a173623e0a470791670db57b51a0bb4292b2bb5f42ad6e44b78f7a88dccb42eb = @embedFile("data/a/a173623e0a470791670db57b51a0bb4292b2bb5f42ad6e44b78f7a88dccb42eb");
pub const _b1f20c3917b748de2161a8f379526eca431852fd376075fc2ec948f0a2dbb1c3 = @embedFile("data/b/b1f20c3917b748de2161a8f379526eca431852fd376075fc2ec948f0a2dbb1c3");
pub const _585c5d1f10aea645ced8fb497b1a84759fbf5a39c104543502ba779552a92a93 = @embedFile("data/5/585c5d1f10aea645ced8fb497b1a84759fbf5a39c104543502ba779552a92a93");
pub const _2e42b47546e87b1518b953b0e97c0bb18f6a33b363cdd2111776f6d9c0028cc8 = @embedFile("data/2/2e42b47546e87b1518b953b0e97c0bb18f6a33b363cdd2111776f6d9c0028cc8");
pub const _ad05cca9f9af104bc798f152839f4ab6106993262884018eb86bfa2446ee13ab = @embedFile("data/a/ad05cca9f9af104bc798f152839f4ab6106993262884018eb86bfa2446ee13ab");
pub const _9eaa70e420830caa7529bd751be5e88a5dc8265821202e18f1ac1bfc91841f91 = @embedFile("data/9/9eaa70e420830caa7529bd751be5e88a5dc8265821202e18f1ac1bfc91841f91");
pub const _b580ec37dc520ddaab43359f86e95865d3d54bcd8b31476c2117e88a2a452377 = @embedFile("data/b/b580ec37dc520ddaab43359f86e95865d3d54bcd8b31476c2117e88a2a452377");
pub const _a49b58e2c1fb7518bd6c45955a1dbf69cda8a47237ed56a6b4b23acaa91db071 = @embedFile("data/a/a49b58e2c1fb7518bd6c45955a1dbf69cda8a47237ed56a6b4b23acaa91db071");
pub const _28b6e959c8a837e1984b7aa9620ffc62d7282a7cae3622483b368e6858c593b3 = @embedFile("data/2/28b6e959c8a837e1984b7aa9620ffc62d7282a7cae3622483b368e6858c593b3");
pub const _221ac897654a1be77bf916269d63462fdba20fb73c6762de8351dfb552701fe1 = @embedFile("data/2/221ac897654a1be77bf916269d63462fdba20fb73c6762de8351dfb552701fe1");
pub const _31e0edd9e0def26b9afc1f1dceb6af9169d977cafa37504f943d0f973c966255 = @embedFile("data/3/31e0edd9e0def26b9afc1f1dceb6af9169d977cafa37504f943d0f973c966255");
pub const _5f1e711079002e90deda04fef199d275bb6865c863cc127ea007e94286a98ded = @embedFile("data/5/5f1e711079002e90deda04fef199d275bb6865c863cc127ea007e94286a98ded");
pub const _f455e02ac3971f5f58aef44052180983dbf43e0f2c004a935464cbc2d02c72ee = @embedFile("data/f/f455e02ac3971f5f58aef44052180983dbf43e0f2c004a935464cbc2d02c72ee");
pub const _0fd667c94bfefe34799dc233f2ccc5032e6faf7375c413d7b00b514cd2346aa3 = @embedFile("data/0/0fd667c94bfefe34799dc233f2ccc5032e6faf7375c413d7b00b514cd2346aa3");
pub const _86e3a8a601361347e3a47d537dfa59755aa8f6ad4dc0f975c9b1f37caff5af58 = @embedFile("data/8/86e3a8a601361347e3a47d537dfa59755aa8f6ad4dc0f975c9b1f37caff5af58");
pub const _8d222052bfcfa7d7b6e7e969d9d1c5f670e14cc33d53eb18ae5e36545a874375 = @embedFile("data/8/8d222052bfcfa7d7b6e7e969d9d1c5f670e14cc33d53eb18ae5e36545a874375");
pub const _77406c896720b6740ceb9bc7f992ef8cb6eee56ac5b900dc7981f1f98727733e = @embedFile("data/7/77406c896720b6740ceb9bc7f992ef8cb6eee56ac5b900dc7981f1f98727733e");
pub const _cc3ae7c1e5c3be4f5d6a60ea0b2870e4ea7039eaec3d9ff38407ebdf9fd94a40 = @embedFile("data/c/cc3ae7c1e5c3be4f5d6a60ea0b2870e4ea7039eaec3d9ff38407ebdf9fd94a40");
pub const _0998fd951c89609ff550cdfd5ca473b50cc38590071248c14345bc49f121745f = @embedFile("data/0/0998fd951c89609ff550cdfd5ca473b50cc38590071248c14345bc49f121745f");
pub const _a6b12eb23f7c4861e37a5436b888e1b49708e32e0789c08a4c0b0bbe55edcd06 = @embedFile("data/a/a6b12eb23f7c4861e37a5436b888e1b49708e32e0789c08a4c0b0bbe55edcd06");
pub const _91b752130690ea10799c3480e12343ac996a68c49d8c5e0bd63033e86d7c3f03 = @embedFile("data/9/91b752130690ea10799c3480e12343ac996a68c49d8c5e0bd63033e86d7c3f03");
pub const _18fc98dc623cea9ddf5365652e1dee21fcde9db2bb12967e8c09936ecdee6758 = @embedFile("data/1/18fc98dc623cea9ddf5365652e1dee21fcde9db2bb12967e8c09936ecdee6758");
pub const _0abdac16bf4770cc2680e5300d7280aa21ea87758c80fd97c0677b47592ac5f3 = @embedFile("data/0/0abdac16bf4770cc2680e5300d7280aa21ea87758c80fd97c0677b47592ac5f3");
pub const _28aa4b5c73a212e38bfba73b2c5a6fca71dee0d503f9649a16624c8db8a38bd9 = @embedFile("data/2/28aa4b5c73a212e38bfba73b2c5a6fca71dee0d503f9649a16624c8db8a38bd9");
pub const _b5096d10cda1ef86614ac0dc9b159edcf57b5609c7f2f041a04718f860df1c6c = @embedFile("data/b/b5096d10cda1ef86614ac0dc9b159edcf57b5609c7f2f041a04718f860df1c6c");
pub const _2aa33d91e874baba8938f3db942cfbbde619699bd14ee7c57b85e1acbbd6dac7 = @embedFile("data/2/2aa33d91e874baba8938f3db942cfbbde619699bd14ee7c57b85e1acbbd6dac7");
pub const _8cc7858aaae420b88522d3b170d969cb88c7ff11b460d360bdb8ef8ae26cb51c = @embedFile("data/8/8cc7858aaae420b88522d3b170d969cb88c7ff11b460d360bdb8ef8ae26cb51c");
pub const _2d3bb9056a516694bb27591604f39a7f6c8ba92f58d66514983da75e5de15c24 = @embedFile("data/2/2d3bb9056a516694bb27591604f39a7f6c8ba92f58d66514983da75e5de15c24");
pub const _4a1cf455fc98ec6ac52256f79f7d7edd9bd247ca50aa752554ee9f349493e9ff = @embedFile("data/4/4a1cf455fc98ec6ac52256f79f7d7edd9bd247ca50aa752554ee9f349493e9ff");
pub const _4056563ef128e08e9ac61406b2981c8834bf7e421bb5ef4ca506e9002f7e216e = @embedFile("data/4/4056563ef128e08e9ac61406b2981c8834bf7e421bb5ef4ca506e9002f7e216e");
pub const _9dbcedb67be3e1f0beaa6651eaf448b11de2c8795235cc0c267f78cff5946824 = @embedFile("data/9/9dbcedb67be3e1f0beaa6651eaf448b11de2c8795235cc0c267f78cff5946824");
pub const _6106c4fe8472993a4128a90759d57f985701d461f4bee3f8b022b022391630b0 = @embedFile("data/6/6106c4fe8472993a4128a90759d57f985701d461f4bee3f8b022b022391630b0");
pub const _b774da2504fba6db4b4149ab308f0485dd582f1d5a028458319ae7182d70c426 = @embedFile("data/b/b774da2504fba6db4b4149ab308f0485dd582f1d5a028458319ae7182d70c426");
pub const _653a908d7b24b9de17e309e7fc88c8c10b1b99e6bd7a112f58de92a774b53e32 = @embedFile("data/6/653a908d7b24b9de17e309e7fc88c8c10b1b99e6bd7a112f58de92a774b53e32");
pub const _809628c05c6334babb840db042b3a56c884a43ba942a68accaa0d3eae948029e = @embedFile("data/8/809628c05c6334babb840db042b3a56c884a43ba942a68accaa0d3eae948029e");
pub const _fd41a35969ac5ba1cf3501fc579549361f978dd86cfbc789246ae9756da7ef38 = @embedFile("data/f/fd41a35969ac5ba1cf3501fc579549361f978dd86cfbc789246ae9756da7ef38");
pub const _c3cbc3e896bd7d358c01aa7f92c124d43925adef64252026dece43dfcf863c6d = @embedFile("data/c/c3cbc3e896bd7d358c01aa7f92c124d43925adef64252026dece43dfcf863c6d");
pub const _2b5a449681ece44f1fc06153a2d037881b737abad75535667bc1e5f4fb3ac967 = @embedFile("data/2/2b5a449681ece44f1fc06153a2d037881b737abad75535667bc1e5f4fb3ac967");
pub const _0fafd91a78641b451172e27f36f5e8a21e17e53c3ff0bd596e3e5f75c9a45b00 = @embedFile("data/0/0fafd91a78641b451172e27f36f5e8a21e17e53c3ff0bd596e3e5f75c9a45b00");
pub const _d7c810eaccea15b0cd726d0a3fc6ad772161350d850d9561ab81eb30c4b3777c = @embedFile("data/d/d7c810eaccea15b0cd726d0a3fc6ad772161350d850d9561ab81eb30c4b3777c");
pub const _3c1f0795fc22df31dabc4f315069f606730c4cb5140759f27a1bc19e006215c5 = @embedFile("data/3/3c1f0795fc22df31dabc4f315069f606730c4cb5140759f27a1bc19e006215c5");
pub const _51c278d3aafd7be6a98d5ace05e1d072f87b3211ff8f61b2742fbd839d05f4b0 = @embedFile("data/5/51c278d3aafd7be6a98d5ace05e1d072f87b3211ff8f61b2742fbd839d05f4b0");
pub const _2223def648f5c0d35e7b4a1c16ce7c60f909cc3e53c93fdfe821093671827539 = @embedFile("data/2/2223def648f5c0d35e7b4a1c16ce7c60f909cc3e53c93fdfe821093671827539");
pub const _639f8ccec735a17a0e690ffab6b12ccda137bc7feb28522d782b89fbd9c88c8b = @embedFile("data/6/639f8ccec735a17a0e690ffab6b12ccda137bc7feb28522d782b89fbd9c88c8b");
pub const _394f6718862e083f14b9a10b957056afe63d6b5276e562e78bf5ab233abed3b5 = @embedFile("data/3/394f6718862e083f14b9a10b957056afe63d6b5276e562e78bf5ab233abed3b5");
pub const _f5a127efc3ae28fd8646b1a284c513a49a40d6f425c0479fdb7feaa8b0081feb = @embedFile("data/f/f5a127efc3ae28fd8646b1a284c513a49a40d6f425c0479fdb7feaa8b0081feb");
pub const _f815c741fa0e8b3287a9b4c7288f4e7f8125af0c567426ab9e48281feffd4866 = @embedFile("data/f/f815c741fa0e8b3287a9b4c7288f4e7f8125af0c567426ab9e48281feffd4866");
pub const _10c1d6cb0ee38f98d04346fdb2bb9a9f703cbdb92db14acf3a0181d56a43c5f9 = @embedFile("data/1/10c1d6cb0ee38f98d04346fdb2bb9a9f703cbdb92db14acf3a0181d56a43c5f9");
pub const _81e78e78cd87e28e62c6e65d2d8842c801b06181afddd910cceeced176ffe719 = @embedFile("data/8/81e78e78cd87e28e62c6e65d2d8842c801b06181afddd910cceeced176ffe719");
pub const _35ca02dd06cc40028fd71fa68045c18ab7907a9d07702466baef418fad0e293c = @embedFile("data/3/35ca02dd06cc40028fd71fa68045c18ab7907a9d07702466baef418fad0e293c");
pub const _5fa2890205b6a6b80b63a26ff1c93db12e2e703543ca4155381d925248d524f5 = @embedFile("data/5/5fa2890205b6a6b80b63a26ff1c93db12e2e703543ca4155381d925248d524f5");
pub const _39adbf12cbe849e69506eca72299624a587dd412438d3e70e319ee985e4bd280 = @embedFile("data/3/39adbf12cbe849e69506eca72299624a587dd412438d3e70e319ee985e4bd280");
pub const _82243cbca1a71eca4d47aabd8e7f84c0536605334c10c2417c96ca600d9c5a32 = @embedFile("data/8/82243cbca1a71eca4d47aabd8e7f84c0536605334c10c2417c96ca600d9c5a32");
pub const _ff1241305dfd3e9fed854a114c7cdf296baf443de6f4d7e6a56503ed922aea09 = @embedFile("data/f/ff1241305dfd3e9fed854a114c7cdf296baf443de6f4d7e6a56503ed922aea09");
pub const _a00098f3fe81be064938995161025cf389765f0b1fc3a0378ddb0b5d3718e4de = @embedFile("data/a/a00098f3fe81be064938995161025cf389765f0b1fc3a0378ddb0b5d3718e4de");
pub const _92a5eea850481eb1764915fa596b828866c1ea0e0a5aa7d0a7608162b67fcaeb = @embedFile("data/9/92a5eea850481eb1764915fa596b828866c1ea0e0a5aa7d0a7608162b67fcaeb");
pub const _215ca14a45d9d6decbe4546d35a343bb0bb6f511f890b87464f6c5f5e58bb7f9 = @embedFile("data/2/215ca14a45d9d6decbe4546d35a343bb0bb6f511f890b87464f6c5f5e58bb7f9");
pub const _7f21ddc20d4f7043961b668d78b73294204f1495514d744f1b759598fb7e7df5 = @embedFile("data/7/7f21ddc20d4f7043961b668d78b73294204f1495514d744f1b759598fb7e7df5");
pub const _5154a1bf5919fc1c604b9b3e6ba9b409e9364dc36a40b10e4c38575c9079da15 = @embedFile("data/5/5154a1bf5919fc1c604b9b3e6ba9b409e9364dc36a40b10e4c38575c9079da15");
pub const _577f9b3fe1e20c3994409ca23bcfb38c3306659d3e5394828a5ee642c677779c = @embedFile("data/5/577f9b3fe1e20c3994409ca23bcfb38c3306659d3e5394828a5ee642c677779c");
pub const _e26a45216e9764c69eba16d0329266c5d65b13175f8552162eb09253bd6d90c4 = @embedFile("data/e/e26a45216e9764c69eba16d0329266c5d65b13175f8552162eb09253bd6d90c4");
pub const _f206f44f6083cdc56e2b7a3470c99ecf92b9f2c6d08782f4050656a501614302 = @embedFile("data/f/f206f44f6083cdc56e2b7a3470c99ecf92b9f2c6d08782f4050656a501614302");
pub const _279a9dedb277978a369499bb3591fbb6cfcb1e63bb812da5f73f164f9e3973db = @embedFile("data/2/279a9dedb277978a369499bb3591fbb6cfcb1e63bb812da5f73f164f9e3973db");
pub const _d63aaa5d5221e59129c3beb6ee79bbad46fb2f5d88ac3eb24448ca439f6f8e8d = @embedFile("data/d/d63aaa5d5221e59129c3beb6ee79bbad46fb2f5d88ac3eb24448ca439f6f8e8d");
pub const _c89417d1bce49ab286b616aeb5b70ab95791da32c1b2ca6a5cc44f3e849bd919 = @embedFile("data/c/c89417d1bce49ab286b616aeb5b70ab95791da32c1b2ca6a5cc44f3e849bd919");
pub const _c307d9e6cd395db132902d2433bd4cf18c9b80a86d8bb51d213aaff00322e4e8 = @embedFile("data/c/c307d9e6cd395db132902d2433bd4cf18c9b80a86d8bb51d213aaff00322e4e8");
pub const _d2746d4bc6f1d4b49b703c0120fff705702f9d291600686a06860e52e562bf4e = @embedFile("data/d/d2746d4bc6f1d4b49b703c0120fff705702f9d291600686a06860e52e562bf4e");
pub const _31117aed4d2ebf73765b0d9e19777868c20e856133752f41d7d73ac3c4a10a02 = @embedFile("data/3/31117aed4d2ebf73765b0d9e19777868c20e856133752f41d7d73ac3c4a10a02");
pub const _e5c78249e670ecbee72532585e4e7dd2b5a61c8c06f15d58edc7f2c7c96bc45e = @embedFile("data/e/e5c78249e670ecbee72532585e4e7dd2b5a61c8c06f15d58edc7f2c7c96bc45e");
pub const _e134d54b3929f0d3840bb979bae69359693055221735d211dce874a7360279f5 = @embedFile("data/e/e134d54b3929f0d3840bb979bae69359693055221735d211dce874a7360279f5");
pub const _ffb9477cc36bf36b1de89c9451e69e515c0432eeaa28b2cf942399cfa69000cb = @embedFile("data/f/ffb9477cc36bf36b1de89c9451e69e515c0432eeaa28b2cf942399cfa69000cb");
pub const _d8edf1760ad7a621b31b8afcb784c791fc31e4ae9b8d302a4d919566517a5bb5 = @embedFile("data/d/d8edf1760ad7a621b31b8afcb784c791fc31e4ae9b8d302a4d919566517a5bb5");
pub const _fd3d734ca90c78759ba857f154294a622541977ff7be4acda87b19e9301bcf06 = @embedFile("data/f/fd3d734ca90c78759ba857f154294a622541977ff7be4acda87b19e9301bcf06");
pub const _63636022c04f6f52ecd07ea00e8d1782fdf0d6e03a58a76923af4cdef1aa5748 = @embedFile("data/6/63636022c04f6f52ecd07ea00e8d1782fdf0d6e03a58a76923af4cdef1aa5748");
pub const _ebd9e671d5a0e2d099620556695d174d9e29658afe26ff32caeb9dbc2b806ae0 = @embedFile("data/e/ebd9e671d5a0e2d099620556695d174d9e29658afe26ff32caeb9dbc2b806ae0");
pub const _9e889a4304319045168a0529daab457c060facbd4e1aba393afb0ccdf62797c0 = @embedFile("data/9/9e889a4304319045168a0529daab457c060facbd4e1aba393afb0ccdf62797c0");
pub const _e5e484256a3225d4a44b8052b531855316c0397cf82c53b06a8284fcb312a319 = @embedFile("data/e/e5e484256a3225d4a44b8052b531855316c0397cf82c53b06a8284fcb312a319");
pub const _7f77b353ad63f2593bc88e54db2cf2d3554db57d9ea155d657f815f4f0313c74 = @embedFile("data/7/7f77b353ad63f2593bc88e54db2cf2d3554db57d9ea155d657f815f4f0313c74");
pub const _84c92ccd2c97da49076d62139755314ff127b25ec6b7f369dcf2fc970d21ab86 = @embedFile("data/8/84c92ccd2c97da49076d62139755314ff127b25ec6b7f369dcf2fc970d21ab86");
pub const _75031dd85336b8cade20000093b9e9c51c7e65ad20034aa38b81881d44e034c6 = @embedFile("data/7/75031dd85336b8cade20000093b9e9c51c7e65ad20034aa38b81881d44e034c6");
pub const _9625341a543c54499448cd0f801e96dd2e559ab12d593c5d9afc011ce7082e32 = @embedFile("data/9/9625341a543c54499448cd0f801e96dd2e559ab12d593c5d9afc011ce7082e32");
pub const _ac50bce03378f4aacbbe0ddabf68f670f0dc8dd2c223e84091f60278dc1acaef = @embedFile("data/a/ac50bce03378f4aacbbe0ddabf68f670f0dc8dd2c223e84091f60278dc1acaef");
pub const _7ecc31d7f08b79393678f1519b7db436d2a7aa485107730007757a5f5446fad8 = @embedFile("data/7/7ecc31d7f08b79393678f1519b7db436d2a7aa485107730007757a5f5446fad8");
pub const _e4b2e0f3503767985f0e2edeb1378d2a4fd4bc5b146361f524dbbdcf36d7f776 = @embedFile("data/e/e4b2e0f3503767985f0e2edeb1378d2a4fd4bc5b146361f524dbbdcf36d7f776");
pub const _0c7448eed3df7dc8a1061b17980e2ddb3aeebc46c397e207ad23889f8a386546 = @embedFile("data/0/0c7448eed3df7dc8a1061b17980e2ddb3aeebc46c397e207ad23889f8a386546");
pub const _9d37dc0a548ed1ef7e2735c2c57fda9eb92112de951996ca6225383b214a3f42 = @embedFile("data/9/9d37dc0a548ed1ef7e2735c2c57fda9eb92112de951996ca6225383b214a3f42");
pub const _050a3f142f71e7cb5a152ce629dbc2b33385dbe644678bc9341c06802bc7ff2c = @embedFile("data/0/050a3f142f71e7cb5a152ce629dbc2b33385dbe644678bc9341c06802bc7ff2c");
pub const _7dd18a8ec78d3aae39a9e67a0264be64be3679d6db4ff402abd5bfd4cd2cbf51 = @embedFile("data/7/7dd18a8ec78d3aae39a9e67a0264be64be3679d6db4ff402abd5bfd4cd2cbf51");
pub const _ea0e14e16facb1ab5bfc83ddc49d9cb6bf2dd1f60dc13baad67cf4bd3b6a70f9 = @embedFile("data/e/ea0e14e16facb1ab5bfc83ddc49d9cb6bf2dd1f60dc13baad67cf4bd3b6a70f9");
pub const _592c651dff09cdf966e7ad09543cceb46e0e872cdd265f8f48d6151a142bef6d = @embedFile("data/5/592c651dff09cdf966e7ad09543cceb46e0e872cdd265f8f48d6151a142bef6d");
pub const _1106a22664c32919ad97278984cbe357dff38fdf7f6a6399e1842104066995cf = @embedFile("data/1/1106a22664c32919ad97278984cbe357dff38fdf7f6a6399e1842104066995cf");
pub const _77e4d24042d9761f306d76c4ad51ea1336f2ef435d1c35f9285f97385cb1c1c2 = @embedFile("data/7/77e4d24042d9761f306d76c4ad51ea1336f2ef435d1c35f9285f97385cb1c1c2");
pub const _30579d5cf562d785dccc09e3386645e58ef876027233d1ca3c6e86140adcb0b9 = @embedFile("data/3/30579d5cf562d785dccc09e3386645e58ef876027233d1ca3c6e86140adcb0b9");
pub const _928528ecf93f4f79e24ebd0e258e723803849a909999537f6b956ed20e269295 = @embedFile("data/9/928528ecf93f4f79e24ebd0e258e723803849a909999537f6b956ed20e269295");
pub const _de914caf495676f65ce02da17e208e0631ecbc5f6ac74555e8a2fdb5a45e3262 = @embedFile("data/d/de914caf495676f65ce02da17e208e0631ecbc5f6ac74555e8a2fdb5a45e3262");
pub const _b8f9aeb477851a86419f191b393249cd40762dcb66a18148f12644ff7763ce8a = @embedFile("data/b/b8f9aeb477851a86419f191b393249cd40762dcb66a18148f12644ff7763ce8a");
pub const _b7a70a732fa1678e4bebd4a457932c670b1f084d3bc55a8a18e91f9341e9998f = @embedFile("data/b/b7a70a732fa1678e4bebd4a457932c670b1f084d3bc55a8a18e91f9341e9998f");
pub const _f94de0f37bebaaca486720aa99d92a560c9c158c5d29cd5c4c29f5f117ec2e49 = @embedFile("data/f/f94de0f37bebaaca486720aa99d92a560c9c158c5d29cd5c4c29f5f117ec2e49");
pub const _ed7d957524fcf4e3fde70a77ea29ccdf48164dabdc206cc1a713580ebaf34a95 = @embedFile("data/e/ed7d957524fcf4e3fde70a77ea29ccdf48164dabdc206cc1a713580ebaf34a95");
pub const _091bdc3f8397061491d43a0e1cea9ed1536f813c1b1a4b36b787541c74143db8 = @embedFile("data/0/091bdc3f8397061491d43a0e1cea9ed1536f813c1b1a4b36b787541c74143db8");
pub const _af74d377fc4115febb69b168ea9b78820efe91cb152c802956746c27f3f2ec49 = @embedFile("data/a/af74d377fc4115febb69b168ea9b78820efe91cb152c802956746c27f3f2ec49");
pub const _f2a1991f627f8121c95929b407c16fef74d9648f2ab7d20cfab86381c61bb9d7 = @embedFile("data/f/f2a1991f627f8121c95929b407c16fef74d9648f2ab7d20cfab86381c61bb9d7");
pub const _5823bde86e4d44ca1f4dfbe982599c9229f05f6aa1fb5ba7c6808f60add895fe = @embedFile("data/5/5823bde86e4d44ca1f4dfbe982599c9229f05f6aa1fb5ba7c6808f60add895fe");
pub const _3bddc50aaa323edaabff725449816f41a8ca7abc35f588b87ab54ce978c81941 = @embedFile("data/3/3bddc50aaa323edaabff725449816f41a8ca7abc35f588b87ab54ce978c81941");
pub const _1737c860058fc8ba47552d2435f088998cd6ef351ce9d7128347f31873f5218f = @embedFile("data/1/1737c860058fc8ba47552d2435f088998cd6ef351ce9d7128347f31873f5218f");
pub const _172912824cec856ca262aac283493bf6db061574ce65997c4d4d358091492a4a = @embedFile("data/1/172912824cec856ca262aac283493bf6db061574ce65997c4d4d358091492a4a");
pub const _d7cc91273897c46444de9a58fc6a99122948151674a4546ef4bd4521bab1a6a1 = @embedFile("data/d/d7cc91273897c46444de9a58fc6a99122948151674a4546ef4bd4521bab1a6a1");
pub const _261c0a4defbea54dc9deb1d3e4778e7b3b0e451b908be96c0aeda6f755ee172d = @embedFile("data/2/261c0a4defbea54dc9deb1d3e4778e7b3b0e451b908be96c0aeda6f755ee172d");
pub const _1827d87f6b26d9de1e27ee2c72aea6a3fd31bb08b5a6056a1efa07e5d539df8f = @embedFile("data/1/1827d87f6b26d9de1e27ee2c72aea6a3fd31bb08b5a6056a1efa07e5d539df8f");
pub const _d42bfd8c9537278cc463b5ce2db10c26af47d3311c632c0e95c7605361e06d90 = @embedFile("data/d/d42bfd8c9537278cc463b5ce2db10c26af47d3311c632c0e95c7605361e06d90");
pub const _7369f93e60d1be145e7834fe9d5723a8a3818ded9190d378cf35702a7d3667bc = @embedFile("data/7/7369f93e60d1be145e7834fe9d5723a8a3818ded9190d378cf35702a7d3667bc");
pub const _bdbc8dc9a2175ba7c62891f60ccfb72ec681170101d05c0bcb7e428ebadc4560 = @embedFile("data/b/bdbc8dc9a2175ba7c62891f60ccfb72ec681170101d05c0bcb7e428ebadc4560");
pub const _674b7a6a1e33e9f17f69972d76ec8c9d68bfa869def5c4adb0e38780c10a28f8 = @embedFile("data/6/674b7a6a1e33e9f17f69972d76ec8c9d68bfa869def5c4adb0e38780c10a28f8");
pub const _2e9438e10ad4ef3612a9483f5bd51e7676e7aaf6bdcfac100d41b253cebee175 = @embedFile("data/2/2e9438e10ad4ef3612a9483f5bd51e7676e7aaf6bdcfac100d41b253cebee175");
pub const _d006a1fe239ec07c6308f15255f27677c1786c4f6d279d0e34bc5e9cff711e30 = @embedFile("data/d/d006a1fe239ec07c6308f15255f27677c1786c4f6d279d0e34bc5e9cff711e30");
pub const _2b230687e7da5c271dea54dc5d1dabc0effab8908245af661cb57d1458dd18b1 = @embedFile("data/2/2b230687e7da5c271dea54dc5d1dabc0effab8908245af661cb57d1458dd18b1");
pub const _dfed901ef8430760fae944464e6c7b6351a381ed2438567480243aa019640758 = @embedFile("data/d/dfed901ef8430760fae944464e6c7b6351a381ed2438567480243aa019640758");
pub const _657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f = @embedFile("data/6/657f60c19d58bf0727ada5adc62297998b5e45c556176b073272383bed51f03f");
pub const _ea178a34ae8580f5d01f1250b3405678239a246ac7d46506fcb51529ae2d0207 = @embedFile("data/e/ea178a34ae8580f5d01f1250b3405678239a246ac7d46506fcb51529ae2d0207");
pub const _afc7210ebf21a84cff6b45c6609b56effe787a322fb7266679ae2c803cdaeeba = @embedFile("data/a/afc7210ebf21a84cff6b45c6609b56effe787a322fb7266679ae2c803cdaeeba");
pub const _6fc8a3416123200727d5cacc540e37fecdd6cfff6805660d33b6c89e83a025b7 = @embedFile("data/6/6fc8a3416123200727d5cacc540e37fecdd6cfff6805660d33b6c89e83a025b7");
pub const _dae515a00547753794c537185a71bbf4ad2e0e65c03a7e2f992cde47da507e40 = @embedFile("data/d/dae515a00547753794c537185a71bbf4ad2e0e65c03a7e2f992cde47da507e40");
pub const _d9955367bce76132b68dc34b1e6466e2931053563687d7e9f08e8d93b89d6500 = @embedFile("data/d/d9955367bce76132b68dc34b1e6466e2931053563687d7e9f08e8d93b89d6500");
pub const _687c5109468b320ba5d8b42d19788620160cfb8a2763fcbdd5a4beb9a7850558 = @embedFile("data/6/687c5109468b320ba5d8b42d19788620160cfb8a2763fcbdd5a4beb9a7850558");
pub const _b8d65426b5ca5217fdb3617aa87fec53a25a5188dc99ba893cfd026d1e9984d1 = @embedFile("data/b/b8d65426b5ca5217fdb3617aa87fec53a25a5188dc99ba893cfd026d1e9984d1");
pub const _f649de7c0428e399e8b90525f952f858af1bfd3c57533a3bae7d098d6a481787 = @embedFile("data/f/f649de7c0428e399e8b90525f952f858af1bfd3c57533a3bae7d098d6a481787");
pub const _8441e95b648bc455abd54cf5c4a4799fcfbbcfa0dbabdff23a75d3f17c4914df = @embedFile("data/8/8441e95b648bc455abd54cf5c4a4799fcfbbcfa0dbabdff23a75d3f17c4914df");
pub const _9a90c694240f02e0a010f3b3b5ae7943a61a94d6d5c7bbc147a98e792c6d02bb = @embedFile("data/9/9a90c694240f02e0a010f3b3b5ae7943a61a94d6d5c7bbc147a98e792c6d02bb");
pub const _5ed0953fab07109c208369210cde02f3614adb2a2f2942ad58fec08894e86eef = @embedFile("data/5/5ed0953fab07109c208369210cde02f3614adb2a2f2942ad58fec08894e86eef");
pub const _a9f966695411b9d328a6d7584db1fa569f58c3f15d0696b335de529bcaf8ca20 = @embedFile("data/a/a9f966695411b9d328a6d7584db1fa569f58c3f15d0696b335de529bcaf8ca20");
pub const _ff2a59039b4a6d7c7ae6adba0bb384e9e6be99ca71aded3d8ad6ca7e0b127f40 = @embedFile("data/f/ff2a59039b4a6d7c7ae6adba0bb384e9e6be99ca71aded3d8ad6ca7e0b127f40");
pub const _8db861acfac7d1a865d26a53f95fdb04de211e157f4f3de5f97583f040ce0c67 = @embedFile("data/8/8db861acfac7d1a865d26a53f95fdb04de211e157f4f3de5f97583f040ce0c67");
pub const _6d634443f5ec2060e4bce83e4a65a7f2f8087438896c6005991295c37085f686 = @embedFile("data/6/6d634443f5ec2060e4bce83e4a65a7f2f8087438896c6005991295c37085f686");
pub const _ea03f272e068b5abd16925d1cc9c5cecaf26e86ec22ec4910b0e3234a296bd52 = @embedFile("data/e/ea03f272e068b5abd16925d1cc9c5cecaf26e86ec22ec4910b0e3234a296bd52");
pub const _a130e88212533dd3c23cc55ebdeaceb7ac116b00a7ca43fb03125a564129c7ac = @embedFile("data/a/a130e88212533dd3c23cc55ebdeaceb7ac116b00a7ca43fb03125a564129c7ac");
pub const _37c79b4aec18c117296aa226b8d37be2dbde9ed3347b2aedbf2aaa4aa25b726b = @embedFile("data/3/37c79b4aec18c117296aa226b8d37be2dbde9ed3347b2aedbf2aaa4aa25b726b");
pub const _915f7ccb36fce7a8af1a8eac657bfeec904267612bee130bd5a36abaa81ba9d8 = @embedFile("data/9/915f7ccb36fce7a8af1a8eac657bfeec904267612bee130bd5a36abaa81ba9d8");
pub const _e26e811fb1cde2b0bb570067c531f111a1b9357227dde4bcd31464b3402b2499 = @embedFile("data/e/e26e811fb1cde2b0bb570067c531f111a1b9357227dde4bcd31464b3402b2499");
pub const _5e09d1a662ce94cbc7d44e41db7fdb1a2c572623b3e6c5ba4a01749c4b83930e = @embedFile("data/5/5e09d1a662ce94cbc7d44e41db7fdb1a2c572623b3e6c5ba4a01749c4b83930e");
pub const _47bdd3b5f5ed4ce498c1cd16aa042e381883104b5e39406d894f6cdd99d90690 = @embedFile("data/4/47bdd3b5f5ed4ce498c1cd16aa042e381883104b5e39406d894f6cdd99d90690");
pub const _dbfb59d82a776ee2a1625b340d35ab3e98f18c959d3705438284ee89d7ee90f2 = @embedFile("data/d/dbfb59d82a776ee2a1625b340d35ab3e98f18c959d3705438284ee89d7ee90f2");
pub const _af3dd6f6329ab832d3455dd1a371db0cf8822eef883535ce5bd894e563341eb6 = @embedFile("data/a/af3dd6f6329ab832d3455dd1a371db0cf8822eef883535ce5bd894e563341eb6");
pub const _0cd2b2fe7d7a598f8040ac0adab206a92851f628e787a92f620514c1191a188f = @embedFile("data/0/0cd2b2fe7d7a598f8040ac0adab206a92851f628e787a92f620514c1191a188f");
pub const _2b5cb91483afbb09a640147cd901cb388104482afc7644dd70cfad5c483d9590 = @embedFile("data/2/2b5cb91483afbb09a640147cd901cb388104482afc7644dd70cfad5c483d9590");
pub const _c4a3000417e2883b34da657264f973c0fc81e334a4980457d82645ab34549d1d = @embedFile("data/c/c4a3000417e2883b34da657264f973c0fc81e334a4980457d82645ab34549d1d");
pub const _b72f593788c54c82df85b45547c127700dea3576a539bd34e7c69021c9faac0a = @embedFile("data/b/b72f593788c54c82df85b45547c127700dea3576a539bd34e7c69021c9faac0a");
pub const _622eb9f044da5fbd3806b48d14ca207a53e1f5c65dd55807ce68196d16653384 = @embedFile("data/6/622eb9f044da5fbd3806b48d14ca207a53e1f5c65dd55807ce68196d16653384");
pub const _fcfac5dd64737bf0a6a805641af375a0407d348d77cd9b9fa95d10e324a0c5a2 = @embedFile("data/f/fcfac5dd64737bf0a6a805641af375a0407d348d77cd9b9fa95d10e324a0c5a2");
pub const _adcbfa719567258d90ab4be773c3056f0e9c2e68dfc0f39bd82e940ed3853fba = @embedFile("data/a/adcbfa719567258d90ab4be773c3056f0e9c2e68dfc0f39bd82e940ed3853fba");
pub const _67a0dfbde9c61da219439719631db9982573a201c839556bb29e9448e5e94b13 = @embedFile("data/6/67a0dfbde9c61da219439719631db9982573a201c839556bb29e9448e5e94b13");
pub const _e00ec75b8c5277d8a2b011901b605c6535a572bc82a64c3664d41f9d41e46fe7 = @embedFile("data/e/e00ec75b8c5277d8a2b011901b605c6535a572bc82a64c3664d41f9d41e46fe7");
pub const _98db876d5fea89b556fdcee4af82d59f4ce11297e884c3c6f555d01c522b0ec9 = @embedFile("data/9/98db876d5fea89b556fdcee4af82d59f4ce11297e884c3c6f555d01c522b0ec9");
pub const _1d8cab681088d868962e794b16821e6129eccbfd05a6b2b74dbf6b30791b6df2 = @embedFile("data/1/1d8cab681088d868962e794b16821e6129eccbfd05a6b2b74dbf6b30791b6df2");
pub const _5ed2b0363781e15a4b82f5d589f37f213b77e8264a590c5ca1d9f313e05ea194 = @embedFile("data/5/5ed2b0363781e15a4b82f5d589f37f213b77e8264a590c5ca1d9f313e05ea194");
pub const _6ec8e023033e73c2df92acc118482aec6b5c8b4b985f50a891dd38521e2c60df = @embedFile("data/6/6ec8e023033e73c2df92acc118482aec6b5c8b4b985f50a891dd38521e2c60df");
pub const _1ca593a5260fe7c5c77dbf3acc2ea87c7a6fa1afcd7043429794cccfe2b9b738 = @embedFile("data/1/1ca593a5260fe7c5c77dbf3acc2ea87c7a6fa1afcd7043429794cccfe2b9b738");
pub const _d6b3d1c436568f91e9a2f690939f079453547f9d7e2db3ebd3616de1096c0e5e = @embedFile("data/d/d6b3d1c436568f91e9a2f690939f079453547f9d7e2db3ebd3616de1096c0e5e");
pub const _31790b6f1787327b51abaa3c3f303d0e0d084ac97774be05bad67fc7346ec171 = @embedFile("data/3/31790b6f1787327b51abaa3c3f303d0e0d084ac97774be05bad67fc7346ec171");
pub const _7e3e4291f891f115f4c8796f6b5e68a27236e674c9fc7f1b2d06c3ca249dff36 = @embedFile("data/7/7e3e4291f891f115f4c8796f6b5e68a27236e674c9fc7f1b2d06c3ca249dff36");
pub const _65b63c107a6e73534d0c8844fcb140516038d1135231a228f0cd2b9c96096690 = @embedFile("data/6/65b63c107a6e73534d0c8844fcb140516038d1135231a228f0cd2b9c96096690");
pub const _0529614c5609d47207181f75595514761594a71d50436e1f1c62ad87adfb5446 = @embedFile("data/0/0529614c5609d47207181f75595514761594a71d50436e1f1c62ad87adfb5446");
pub const _d9985dc4801b5b8c6a6f11017792a119a74d07baaafd0f1fb65d1b49d276fa4e = @embedFile("data/d/d9985dc4801b5b8c6a6f11017792a119a74d07baaafd0f1fb65d1b49d276fa4e");
pub const _0cb35370389ffb1cd69d1606a8b52d8c47da8f9ad4a25bcd8e12ec58e11db94e = @embedFile("data/0/0cb35370389ffb1cd69d1606a8b52d8c47da8f9ad4a25bcd8e12ec58e11db94e");
pub const _53b102cbf92851d2a71636a05c80b77f47c881ee0fb889e6dd178dcf17661745 = @embedFile("data/5/53b102cbf92851d2a71636a05c80b77f47c881ee0fb889e6dd178dcf17661745");
pub const _fdc369e7f136358be156833320334420c3842f7427153b504c7eeb7268c3577c = @embedFile("data/f/fdc369e7f136358be156833320334420c3842f7427153b504c7eeb7268c3577c");
pub const _eb4b43b95b1118b9375388a9af8d2430cb05ac3af53c4258feec479396b9ce7b = @embedFile("data/e/eb4b43b95b1118b9375388a9af8d2430cb05ac3af53c4258feec479396b9ce7b");
pub const _601765e7857812510e2b07045baa8d31314a5ebc1b84af8ce54f5894ffcfe7de = @embedFile("data/6/601765e7857812510e2b07045baa8d31314a5ebc1b84af8ce54f5894ffcfe7de");
pub const _016e88408fe029a8617b65006e14cc2e572c6dd3f7b32417a11df47ec701c2e0 = @embedFile("data/0/016e88408fe029a8617b65006e14cc2e572c6dd3f7b32417a11df47ec701c2e0");
pub const _41fafea33ed497604db08982e3ef5e3c896c552b5418a63f0111c4f25dda83d0 = @embedFile("data/4/41fafea33ed497604db08982e3ef5e3c896c552b5418a63f0111c4f25dda83d0");
pub const _1cff22b594f581018824b5c4ea6269d3a4b424b691697fb1e7b9f85fed5f9daa = @embedFile("data/1/1cff22b594f581018824b5c4ea6269d3a4b424b691697fb1e7b9f85fed5f9daa");
pub const _6ed0c60488298f2cf38e319fad62118a59db5eb69f27d73b95706b6f1e4fe618 = @embedFile("data/6/6ed0c60488298f2cf38e319fad62118a59db5eb69f27d73b95706b6f1e4fe618");
pub const _d37f5b0e4017c5c540c81dc7a09e9cbbd91d2662de0bd589ff855052a271078e = @embedFile("data/d/d37f5b0e4017c5c540c81dc7a09e9cbbd91d2662de0bd589ff855052a271078e");
pub const _e672da1c4f98da2d2c7c972895914d213fc718b1a05a1a9bdece3b696ff2de09 = @embedFile("data/e/e672da1c4f98da2d2c7c972895914d213fc718b1a05a1a9bdece3b696ff2de09");
pub const _49ce0def94b7461ff2b86e2c31dc10062eb2cc07ffdcf16c12bf084ea0694dd2 = @embedFile("data/4/49ce0def94b7461ff2b86e2c31dc10062eb2cc07ffdcf16c12bf084ea0694dd2");
pub const _981f14e3b6c442bab84b51ea8f108f19305ff794a654f400f810e4842d6f75d6 = @embedFile("data/9/981f14e3b6c442bab84b51ea8f108f19305ff794a654f400f810e4842d6f75d6");
pub const _1b8b19935caa992911ad2f210c98bdafe61aa2d4ecc59a694e6d87a8c1d917cc = @embedFile("data/1/1b8b19935caa992911ad2f210c98bdafe61aa2d4ecc59a694e6d87a8c1d917cc");
pub const _5a94ab6520bef9312c9346213f02d5f78bdf70ac7ee16e3b18467a0dcfce2a52 = @embedFile("data/5/5a94ab6520bef9312c9346213f02d5f78bdf70ac7ee16e3b18467a0dcfce2a52");
pub const _8b9cc7427f164a79fcac8323b15048b2eea2fa7392ab9c849843c0f4ed6fb819 = @embedFile("data/8/8b9cc7427f164a79fcac8323b15048b2eea2fa7392ab9c849843c0f4ed6fb819");
pub const _85c44a067162404593383d823cb12b927664b1f7b1034bbafbdc1e1294ad50b0 = @embedFile("data/8/85c44a067162404593383d823cb12b927664b1f7b1034bbafbdc1e1294ad50b0");
pub const _8f332db547246069364959a600cecc889e17dc91e552544b1d46047826f9bda7 = @embedFile("data/8/8f332db547246069364959a600cecc889e17dc91e552544b1d46047826f9bda7");
pub const _78e7fbb1ba166c9cd09a23940f65ad21e457bd16413a0414d7ccc316222c0496 = @embedFile("data/7/78e7fbb1ba166c9cd09a23940f65ad21e457bd16413a0414d7ccc316222c0496");
pub const _1c0450a60bb805ad53c83804bbd7ec50c86264134910a19ed101fefc310fe626 = @embedFile("data/1/1c0450a60bb805ad53c83804bbd7ec50c86264134910a19ed101fefc310fe626");
pub const _aed559f918b2cfc6e04d2ce5365226d0c3e5e31641294f544796f587f6a06706 = @embedFile("data/a/aed559f918b2cfc6e04d2ce5365226d0c3e5e31641294f544796f587f6a06706");
pub const _c2ebd6ba5c1ba8f8ff8e45ec2ee6d1d7f6ef169956f6fa8c4abfcd66ed1fc4b9 = @embedFile("data/c/c2ebd6ba5c1ba8f8ff8e45ec2ee6d1d7f6ef169956f6fa8c4abfcd66ed1fc4b9");
pub const _d93fb9680bc085bd92157b1b47bd9454bff1c8f3b05e754ce17eb3ee006a3d9f = @embedFile("data/d/d93fb9680bc085bd92157b1b47bd9454bff1c8f3b05e754ce17eb3ee006a3d9f");
pub const _e5b32f8c976cdb4b5789ec123323bc7d919b9036cdec082e63602ed7cd1e6076 = @embedFile("data/e/e5b32f8c976cdb4b5789ec123323bc7d919b9036cdec082e63602ed7cd1e6076");
pub const _7a4a63c31779b1f6a0bcca5db33702f69e7d4c0da79734d05f6090ad20e8ddb2 = @embedFile("data/7/7a4a63c31779b1f6a0bcca5db33702f69e7d4c0da79734d05f6090ad20e8ddb2");
pub const _d63c8033ef20a986b514b33befe2a5658246506dfdaace8272eae548bd66b419 = @embedFile("data/d/d63c8033ef20a986b514b33befe2a5658246506dfdaace8272eae548bd66b419");
pub const _e34b61c903ac69cd51c4bd0b3a5a6f72a551fc31c3ca17be8806a93a0f05b5dc = @embedFile("data/e/e34b61c903ac69cd51c4bd0b3a5a6f72a551fc31c3ca17be8806a93a0f05b5dc");
pub const _37b6f1fd4c94a1187b705970b9935f12190aa44fe342640be274f5287b7d0d7a = @embedFile("data/3/37b6f1fd4c94a1187b705970b9935f12190aa44fe342640be274f5287b7d0d7a");
pub const _2e91532d80e1642564a1303b77ae1a32d5b9fddb3a1d21e1dea5e1995665590d = @embedFile("data/2/2e91532d80e1642564a1303b77ae1a32d5b9fddb3a1d21e1dea5e1995665590d");
pub const _6ede4faf3322f3577701bc5d446779e95b74e13bc9c8e28c090130a81d163dc4 = @embedFile("data/6/6ede4faf3322f3577701bc5d446779e95b74e13bc9c8e28c090130a81d163dc4");
pub const _4ae350e636208b6837d9bd02d5daf2d20672de37add0b5a71215b454cea19fda = @embedFile("data/4/4ae350e636208b6837d9bd02d5daf2d20672de37add0b5a71215b454cea19fda");
pub const _68b4fffbfef9b9d5e29bac1e92ad7f8ee97d4755a887595ecea9c36512b954ce = @embedFile("data/6/68b4fffbfef9b9d5e29bac1e92ad7f8ee97d4755a887595ecea9c36512b954ce");
pub const _6696d891077b7a3c0a811b0f1c0b2eb85adb0a76a68b049409e99208c0b04f10 = @embedFile("data/6/6696d891077b7a3c0a811b0f1c0b2eb85adb0a76a68b049409e99208c0b04f10");
pub const _d16c9eb04a5de3a1565d2ba85319b7380646f466dceb2e276ec066df6580e17e = @embedFile("data/d/d16c9eb04a5de3a1565d2ba85319b7380646f466dceb2e276ec066df6580e17e");
pub const _7cac3432bb23604830c161ca86ed6e33d5f9e9c2e0d842954110b0089e1efbc8 = @embedFile("data/7/7cac3432bb23604830c161ca86ed6e33d5f9e9c2e0d842954110b0089e1efbc8");
pub const _8a84e660cca469956134bb165734358f4a98b13abb44e75ea83aee538d11cc95 = @embedFile("data/8/8a84e660cca469956134bb165734358f4a98b13abb44e75ea83aee538d11cc95");
pub const _371039399380245bf063f334df5b96ce1ec0ac4874090b6f96f82fcd0ff684c2 = @embedFile("data/3/371039399380245bf063f334df5b96ce1ec0ac4874090b6f96f82fcd0ff684c2");
pub const _7026dcf86704c9e66844b4a916a56fee386579bde5de33cf0cf8786e051e6bfa = @embedFile("data/7/7026dcf86704c9e66844b4a916a56fee386579bde5de33cf0cf8786e051e6bfa");
pub const _770ae87519ad4e8ffcd51b313ad10c7b723e69cf34842bd8b2dad1d10364c058 = @embedFile("data/7/770ae87519ad4e8ffcd51b313ad10c7b723e69cf34842bd8b2dad1d10364c058");
pub const _16342064f8a75ebf10ceb23e29bc99aea427457e4f26d164d03cb28cda03b6b1 = @embedFile("data/1/16342064f8a75ebf10ceb23e29bc99aea427457e4f26d164d03cb28cda03b6b1");
pub const _1f4993f3de1b17765d05ac54b116a1725a2174e90044dc767bb52b4ec405c8ff = @embedFile("data/1/1f4993f3de1b17765d05ac54b116a1725a2174e90044dc767bb52b4ec405c8ff");
pub const _fc711b8fd6e8961ea759e378358d29558eaabf6182eb0d464a2ab43d2c745f7c = @embedFile("data/f/fc711b8fd6e8961ea759e378358d29558eaabf6182eb0d464a2ab43d2c745f7c");
pub const _d010accc1c7959802703e3ce6b8365ebd07612f4373a1e12ba873a115428fc9e = @embedFile("data/d/d010accc1c7959802703e3ce6b8365ebd07612f4373a1e12ba873a115428fc9e");
pub const _8ede21039ccbb68f8129a2e5d4d0eecfeda00c83d8122d0853e2b9969c8224d7 = @embedFile("data/8/8ede21039ccbb68f8129a2e5d4d0eecfeda00c83d8122d0853e2b9969c8224d7");
pub const _b54bd510377c46dac28eff64cbf8171f9b8423132e4f555f459f4d2505606b57 = @embedFile("data/b/b54bd510377c46dac28eff64cbf8171f9b8423132e4f555f459f4d2505606b57");
pub const _74d2dee47633591a71751a0aac5810cc077f09680a872e251f230a2da3fae742 = @embedFile("data/7/74d2dee47633591a71751a0aac5810cc077f09680a872e251f230a2da3fae742");
pub const _1711f7859d8c9c6e50f828b4bd9b0c6562ea997d1f6f31170f09b14e9a2a1053 = @embedFile("data/1/1711f7859d8c9c6e50f828b4bd9b0c6562ea997d1f6f31170f09b14e9a2a1053");
pub const _e87cf18702cb9ec31fc5bce20850fc9381f0563b913b6de92033c84d6dfc4317 = @embedFile("data/e/e87cf18702cb9ec31fc5bce20850fc9381f0563b913b6de92033c84d6dfc4317");
pub const _5dcb2cced3dbfbf92c0b836c5d2773f3101342cce8eb976bbf64cd271a4c446c = @embedFile("data/5/5dcb2cced3dbfbf92c0b836c5d2773f3101342cce8eb976bbf64cd271a4c446c");
pub const _cdabd98a4b8ebe04eae57fba447b4a32e6c1a7204b2ee0eea42ba4b186079d7b = @embedFile("data/c/cdabd98a4b8ebe04eae57fba447b4a32e6c1a7204b2ee0eea42ba4b186079d7b");
pub const _a9a668b769c96f20df15e5af22341bc3f339e630d7ec2d5d495bc77ac00138b8 = @embedFile("data/a/a9a668b769c96f20df15e5af22341bc3f339e630d7ec2d5d495bc77ac00138b8");
pub const _68acd323128673bb87a62563f958f123a5a20942d25f6871db7538f0c560b8b3 = @embedFile("data/6/68acd323128673bb87a62563f958f123a5a20942d25f6871db7538f0c560b8b3");
pub const _40c78ac09b9af96927f472354272c8066323766bd1813c53eb7e8cc1036433fc = @embedFile("data/4/40c78ac09b9af96927f472354272c8066323766bd1813c53eb7e8cc1036433fc");
pub const _8011374faf92b972f729b49137b75f9d88c76641419030f0d7b63603d4399985 = @embedFile("data/8/8011374faf92b972f729b49137b75f9d88c76641419030f0d7b63603d4399985");
pub const _fb151678d30c302661f1c63f5675b733ea12f6d55a331e5a3ba5b5707f9dc35d = @embedFile("data/f/fb151678d30c302661f1c63f5675b733ea12f6d55a331e5a3ba5b5707f9dc35d");
pub const _7dd8ca6b5d1c46ddc83776f31ef0ae150c07936a30a72b1bdd294457b22e64b5 = @embedFile("data/7/7dd8ca6b5d1c46ddc83776f31ef0ae150c07936a30a72b1bdd294457b22e64b5");
pub const _a7fd7a566ed380d6d8c076ac42ae73304bad66b38812d27838a73f594ddf1b39 = @embedFile("data/a/a7fd7a566ed380d6d8c076ac42ae73304bad66b38812d27838a73f594ddf1b39");
pub const _4550ee00bf9231cb9a235dbe100032cd500d6762d8c1bbaf864c12e79f7cee15 = @embedFile("data/4/4550ee00bf9231cb9a235dbe100032cd500d6762d8c1bbaf864c12e79f7cee15");
pub const _ba4d37856b86a6c120217d5c2ed4d7016d0e1741fe8a1d4f2691fc2c5bec16d0 = @embedFile("data/b/ba4d37856b86a6c120217d5c2ed4d7016d0e1741fe8a1d4f2691fc2c5bec16d0");
pub const _38797b93d2275a5af47dabe4116adb28021a80658d779d9007181a94ccb593c6 = @embedFile("data/3/38797b93d2275a5af47dabe4116adb28021a80658d779d9007181a94ccb593c6");
pub const _170f865cbe75433db6ae4a20a960fe377958176048e32ac2bdfa48796681b1d9 = @embedFile("data/1/170f865cbe75433db6ae4a20a960fe377958176048e32ac2bdfa48796681b1d9");
pub const _811851500e7db105049fdfa32ded4655347d479757e5d006681f620d5fdb5019 = @embedFile("data/8/811851500e7db105049fdfa32ded4655347d479757e5d006681f620d5fdb5019");
pub const _30b75e45c4f20edccf99eb14378335e6a18d5c91a8ddd2ab14d9c7fd1cc53643 = @embedFile("data/3/30b75e45c4f20edccf99eb14378335e6a18d5c91a8ddd2ab14d9c7fd1cc53643");
pub const _83975017ffba92cf3e0f0ae32a0d9ea20f414bbcb9daadaea5813c5d55f0a439 = @embedFile("data/8/83975017ffba92cf3e0f0ae32a0d9ea20f414bbcb9daadaea5813c5d55f0a439");
pub const _b9be868d2070f9e549a64c5a7ff85f8508959d70f7449ac75fa239fa8defe692 = @embedFile("data/b/b9be868d2070f9e549a64c5a7ff85f8508959d70f7449ac75fa239fa8defe692");
pub const _0b3c50d87599164155c3cb7fac702d6b8e91c71c68e22135cf6981205546328f = @embedFile("data/0/0b3c50d87599164155c3cb7fac702d6b8e91c71c68e22135cf6981205546328f");
pub const _88db57d643913ed549e190448cefaa5f5c0483ac2e4813472245e4396cad89eb = @embedFile("data/8/88db57d643913ed549e190448cefaa5f5c0483ac2e4813472245e4396cad89eb");
pub const _27302ed548c6bfb82fd2add242e9c6f7fb7b4573f8c5fbe7f70017374c8e31c3 = @embedFile("data/2/27302ed548c6bfb82fd2add242e9c6f7fb7b4573f8c5fbe7f70017374c8e31c3");
pub const _cd8a8d81d0ae4fd9b528e77cb02ca5ba10d1ba8bc98bafbbaf4be184d0a4031d = @embedFile("data/c/cd8a8d81d0ae4fd9b528e77cb02ca5ba10d1ba8bc98bafbbaf4be184d0a4031d");
pub const _0bcce19ac1b0db072f47dd0a1e7251f69331821ab6f5981d3a035acf6213b948 = @embedFile("data/0/0bcce19ac1b0db072f47dd0a1e7251f69331821ab6f5981d3a035acf6213b948");
pub const _57f017c91dbca3db7c2d962b8f039cb9245f095f0a1b79150840daffdf02342d = @embedFile("data/5/57f017c91dbca3db7c2d962b8f039cb9245f095f0a1b79150840daffdf02342d");
pub const _285c57becf847fb85cc73f06a574661fc7a085e1d438733935fb05ad2f467f5c = @embedFile("data/2/285c57becf847fb85cc73f06a574661fc7a085e1d438733935fb05ad2f467f5c");
pub const _3643da818d632050584cf3212877c2e9e6ba900cdeca653a49dcbe1b2e8da43b = @embedFile("data/3/3643da818d632050584cf3212877c2e9e6ba900cdeca653a49dcbe1b2e8da43b");
pub const _50dd1ee5d1828f880607bc278d58200e66f7b892d0a3f1cc553804c998b33f99 = @embedFile("data/5/50dd1ee5d1828f880607bc278d58200e66f7b892d0a3f1cc553804c998b33f99");
pub const _d0447ba0f30123155a30acb81dda62e3288b5b86d920c00417f5b4dc70ac4d41 = @embedFile("data/d/d0447ba0f30123155a30acb81dda62e3288b5b86d920c00417f5b4dc70ac4d41");
pub const _2e22bfaeb7bc1e19b132bd744b92a9818113c057b1aa562bd50530af1caae8e2 = @embedFile("data/2/2e22bfaeb7bc1e19b132bd744b92a9818113c057b1aa562bd50530af1caae8e2");
pub const _a890551b21d3f0b6e7c6111827e96322fb97fb8c23cc20d59b9147b1470a61b0 = @embedFile("data/a/a890551b21d3f0b6e7c6111827e96322fb97fb8c23cc20d59b9147b1470a61b0");
pub const _1c3cee8685b9a6f4723760005685ccf5a694b8056d2aa26f053578081c6e0c8c = @embedFile("data/1/1c3cee8685b9a6f4723760005685ccf5a694b8056d2aa26f053578081c6e0c8c");
pub const _c346dbe3b4d0ea64561c999695015f7bdd7d858a15ab8a2855f72e01ca0540b8 = @embedFile("data/c/c346dbe3b4d0ea64561c999695015f7bdd7d858a15ab8a2855f72e01ca0540b8");
pub const _0971e81ded14ff4c33574eb9afdbc1b393c2660dbf088407f15c5d3de9aa95fc = @embedFile("data/0/0971e81ded14ff4c33574eb9afdbc1b393c2660dbf088407f15c5d3de9aa95fc");
pub const _63879c489ae234760936ed1d074acc1e2e4f785537900ac7854cc6c29303b074 = @embedFile("data/6/63879c489ae234760936ed1d074acc1e2e4f785537900ac7854cc6c29303b074");
pub const _ace305bbafe572f8dacaff860f758bfa27d3b22d26bb74077e5eb257d7041d25 = @embedFile("data/a/ace305bbafe572f8dacaff860f758bfa27d3b22d26bb74077e5eb257d7041d25");
pub const _906689f327946706422421789061bc7e1bb40c22772d6ba8caa7d46c3ed9aa21 = @embedFile("data/9/906689f327946706422421789061bc7e1bb40c22772d6ba8caa7d46c3ed9aa21");
pub const _5c124863e0ed6e95da8e87e12db342c5f22f6a635adc1de2da2112cd4c06cae1 = @embedFile("data/5/5c124863e0ed6e95da8e87e12db342c5f22f6a635adc1de2da2112cd4c06cae1");
pub const _b60d1bbe676ea5587202a1774ba69aa24035e2346dd4bba6b29d1a7598d989ab = @embedFile("data/b/b60d1bbe676ea5587202a1774ba69aa24035e2346dd4bba6b29d1a7598d989ab");
pub const _08eb18e5c7aada08b1c01401ab75929fedefbaf489925cdde65605725af00f50 = @embedFile("data/0/08eb18e5c7aada08b1c01401ab75929fedefbaf489925cdde65605725af00f50");
pub const _f62bcca38178fe7119918cbd07a03c14054e9b2b778273977ac40737bf8806d7 = @embedFile("data/f/f62bcca38178fe7119918cbd07a03c14054e9b2b778273977ac40737bf8806d7");
};
const std = @import("std");
|
0 | repos/tempora/src | repos/tempora/src/tzdb/parse_tzif.zig | // Parts of this file are based on https://github.com/leroycep/zig-tzif:
// MIT License
// Copyright (c) 2021 LeRoyce Pearson
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
pub const Version = enum(u8) {
V1 = 0,
V2 = '2',
V3 = '3',
pub fn time_size(self: Version) u32 {
return switch (self) {
.V1 => 4,
.V2, .V3 => 8,
};
}
pub fn leap_size(self: Version) u32 {
return self.time_size() + 4;
}
pub fn string(self: Version) []const u8 {
return switch (self) {
.V1 => "1",
.V2 => "2",
.V3 => "3",
};
}
};
const time_type_size = 6;
pub const TZif_Header = struct {
version: Version,
isutcnt: u32,
isstdcnt: u32,
leapcnt: u32,
timecnt: u32,
typecnt: u32,
charcnt: u32,
pub fn dataSize(self: TZif_Header, data_block_version: Version) u32 {
return self.timecnt * data_block_version.time_size() +
self.timecnt +
self.typecnt * time_type_size +
self.charcnt +
self.leapcnt * data_block_version.leap_size() +
self.isstdcnt +
self.isutcnt;
}
};
pub fn parse_header(reader: anytype, seekableStream: anytype) !TZif_Header {
var magic_buf: [4]u8 = undefined;
try reader.readNoEof(&magic_buf);
if (!std.mem.eql(u8, "TZif", &magic_buf)) {
return error.InvalidFormat; // Magic number "TZif" is missing
}
// Check verison
const version = reader.readEnum(Version, .little) catch |err| switch (err) {
error.InvalidValue => return error.UnsupportedVersion,
else => |e| return e,
};
if (version == .V1) {
return error.UnsupportedVersion;
}
// Seek past reserved bytes
try seekableStream.seekBy(15);
return TZif_Header{
.version = version,
.isutcnt = try reader.readInt(u32, .big),
.isstdcnt = try reader.readInt(u32, .big),
.leapcnt = try reader.readInt(u32, .big),
.timecnt = try reader.readInt(u32, .big),
.typecnt = try reader.readInt(u32, .big),
.charcnt = try reader.readInt(u32, .big),
};
}
/// Parses hh[:mm[:ss]] to a number of seconds. Hours may be one digit long. Minutes and seconds must be two digits.
fn hhmmss_offset_to_s(_string: []const u8, idx: *usize) !i32 {
var string = _string;
var sign: i2 = 1;
if (string[0] == '+') {
sign = 1;
string = string[1..];
idx.* += 1;
} else if (string[0] == '-') {
sign = -1;
string = string[1..];
idx.* += 1;
}
for (string, 0..) |c, i| {
if (!(std.ascii.isDigit(c) or c == ':')) {
string = string[0..i];
break;
}
idx.* += 1;
}
var result: i32 = 0;
var segment_iter = std.mem.split(u8, string, ":");
const hour_string = segment_iter.next() orelse return error.EmptyString;
const hours = std.fmt.parseInt(u32, hour_string, 10) catch |err| switch (err) {
error.InvalidCharacter => return error.InvalidFormat,
error.Overflow => return error.InvalidFormat,
};
if (hours > 167) {
// TODO: use diagnostics mechanism instead of logging
return error.InvalidFormat;
}
result += std.time.s_per_hour * @as(i32, @intCast(hours));
if (segment_iter.next()) |minute_string| {
if (minute_string.len != 2) {
// TODO: Add diagnostics when returning an error.
return error.InvalidFormat;
}
const minutes = try std.fmt.parseInt(u32, minute_string, 10);
if (minutes > 59) return error.InvalidFormat;
result += std.time.s_per_min * @as(i32, @intCast(minutes));
}
if (segment_iter.next()) |second_string| {
if (second_string.len != 2) {
// TODO: Add diagnostics when returning an error.
return error.InvalidFormat;
}
const seconds = try std.fmt.parseInt(u8, second_string, 10);
if (seconds > 59) return error.InvalidFormat;
result += seconds;
}
return result * sign;
}
fn parse_posix_tz_rule(_string: []const u8) !Timezone.POSIX_TZ.Rule {
var string = _string;
if (string.len < 2) return error.InvalidFormat;
const time: i32 = if (std.mem.indexOf(u8, string, "/")) |start_of_time| parse_time: {
const time_string = string[start_of_time + 1 ..];
var i: usize = 0;
const time = try hhmmss_offset_to_s(time_string, &i);
// The time at the end of the rule should be the last thing in the string. Fixes the parsing to return
// an error in cases like "/2/3", where they have some extra characters.
if (i != time_string.len) {
return error.InvalidFormat;
}
string = string[0..start_of_time];
break :parse_time time;
} else 2 * std.time.s_per_hour;
if (string[0] == 'J') {
const julian_day1 = std.fmt.parseInt(u16, string[1..], 10) catch |err| switch (err) {
error.InvalidCharacter => return error.InvalidFormat,
error.Overflow => return error.InvalidFormat,
};
if (julian_day1 < 1 or julian_day1 > 365) return error.InvalidFormat;
return .{ .julian_day = .{ .day = julian_day1, .time = time } };
} else if (std.ascii.isDigit(string[0])) {
const julian_day0 = std.fmt.parseInt(u16, string[0..], 10) catch |err| switch (err) {
error.InvalidCharacter => return error.InvalidFormat,
error.Overflow => return error.InvalidFormat,
};
if (julian_day0 > 365) return error.InvalidFormat;
return .{ .julian_day_zero = .{ .day = julian_day0, .time = time } };
} else if (string[0] == 'M') {
var split_iter = std.mem.split(u8, string[1..], ".");
const m_str = split_iter.next() orelse return error.InvalidFormat;
const n_str = split_iter.next() orelse return error.InvalidFormat;
const d_str = split_iter.next() orelse return error.InvalidFormat;
const m = std.fmt.parseInt(u8, m_str, 10) catch |err| switch (err) {
error.InvalidCharacter => return error.InvalidFormat,
error.Overflow => return error.InvalidFormat,
};
const n = std.fmt.parseInt(u8, n_str, 10) catch |err| switch (err) {
error.InvalidCharacter => return error.InvalidFormat,
error.Overflow => return error.InvalidFormat,
};
const d = std.fmt.parseInt(u8, d_str, 10) catch |err| switch (err) {
error.InvalidCharacter => return error.InvalidFormat,
error.Overflow => return error.InvalidFormat,
};
if (m < 1 or m > 12) return error.InvalidFormat;
if (n < 1 or n > 5) return error.InvalidFormat;
if (d > 6) return error.InvalidFormat;
return .{ .month_nth_week_day = .{ .month = m, .n = n, .day = d, .time = time } };
} else {
return error.InvalidFormat;
}
}
fn parse_posix_tz_designation(string: []const u8, idx: *usize) ![]const u8 {
const quoted = string[idx.*] == '<';
if (quoted) idx.* += 1;
const start = idx.*;
while (idx.* < string.len) : (idx.* += 1) {
if ((quoted and string[idx.*] == '>') or
(!quoted and !std.ascii.isAlphabetic(string[idx.*])))
{
const designation = string[start..idx.*];
// The designation must be at least one character long!
if (designation.len == 0) return error.InvalidFormat;
if (quoted) idx.* += 1;
return designation;
}
}
return error.InvalidFormat;
}
pub fn parse_posix_tz(string: []const u8) !Timezone.POSIX_TZ {
var result: Timezone.POSIX_TZ = .{ .std_designation = undefined, .std_offset = undefined };
var idx: usize = 0;
result.std_designation = try parse_posix_tz_designation(string, &idx);
// multiply by -1 to get offset as seconds East of Greenwich as TZif specifies it:
result.std_offset = try hhmmss_offset_to_s(string[idx..], &idx) * -1;
if (idx >= string.len) {
return result;
}
if (string[idx] != ',') {
result.dst_designation = try parse_posix_tz_designation(string, &idx);
if (idx < string.len and string[idx] != ',') {
// multiply by -1 to get offset as seconds East of Greenwich as TZif specifies it:
result.dst_offset = try hhmmss_offset_to_s(string[idx..], &idx) * -1;
} else {
result.dst_offset = result.std_offset + std.time.s_per_hour;
}
if (idx >= string.len) {
return result;
}
}
std.debug.assert(string[idx] == ',');
idx += 1;
if (std.mem.indexOf(u8, string[idx..], ",")) |_end_of_start_rule| {
const end_of_start_rule = idx + _end_of_start_rule;
result.dst_range = .{
.start = try parse_posix_tz_rule(string[idx..end_of_start_rule]),
.end = try parse_posix_tz_rule(string[end_of_start_rule + 1 ..]),
};
} else {
return error.InvalidFormat;
}
return result;
}
pub fn parse(allocator: std.mem.Allocator, reader: anytype, seekableStream: anytype) !Timezone {
const v1_header = try parse_header(reader, seekableStream);
try seekableStream.seekBy(v1_header.dataSize(.V1));
const v2_header = try parse_header(reader, seekableStream);
var transitions: std.MultiArrayList(Timezone.Transition) = .{};
try transitions.ensureTotalCapacity(allocator, v2_header.timecnt);
errdefer transitions.deinit(allocator);
// Parse transition times
{
var prev: i64 = -(2 << 59); // Earliest time supported, this is earlier than the big bang
for (0..v2_header.timecnt) |_| {
const ts = try reader.readInt(i64, .big);
if (ts <= prev) {
return error.InvalidFormat;
}
transitions.appendAssumeCapacity(.{
.ts = ts,
.zone_index = 0,
});
prev = ts;
}
}
// Parse transition types
const transition_zones = transitions.items(.zone_index);
try reader.readNoEof(transition_zones);
for (transition_zones) |zone_index| {
if (zone_index >= v2_header.typecnt) {
return error.InvalidFormat;
}
}
// Parse local time type records
const zone_infos = try allocator.alloc(Timezone.Zone_Info, v2_header.typecnt);
errdefer allocator.free(zone_infos);
for (zone_infos) |*zi| {
const offset = try reader.readInt(i32, .big);
const is_dst = switch (try reader.readByte()) {
0 => false,
1 => true,
else => return error.InvalidFormat,
};
const designation_index = try reader.readByte(); // will be replaced below
var designation_temp: []const u8 = &.{};
designation_temp.len = designation_index;
zi.* = .{
.designation = designation_temp,
.begin_ts = null,
.end_ts = null,
.offset = offset,
.is_dst = is_dst,
.source = .tzdata_wall,
};
}
// Read designations
const designations = try allocator.alloc(u8, v2_header.charcnt);
errdefer allocator.free(designations);
try reader.readNoEof(designations);
// Update local time type records with a valid designation string
for (zone_infos) |*zi| {
const start_index = zi.designation.len;
if (start_index > v2_header.charcnt) {
return error.InvalidFormat;
}
zi.designation = std.mem.sliceTo(designations[start_index..], 0);
}
// Parse and ignore leap seconds records
for (0..v2_header.leapcnt) |_| {
_ = try reader.readInt(i64, .big);
_ = try reader.readInt(i32, .big);
}
// Parse standard/wall indicators
if (v2_header.isstdcnt > 0) {
if (v2_header.isstdcnt != v2_header.typecnt) {
return error.InvalidFormat;
}
for (zone_infos) |*zi| {
zi.source = switch (try reader.readByte()) {
1 => .tzdata_standard,
0 => .tzdata_wall,
else => return error.InvalidFormat,
};
}
}
// Parse UT/local indicators
if (v2_header.isutcnt > 0) {
if (v2_header.isutcnt != v2_header.typecnt) {
return error.InvalidFormat;
}
for (zone_infos) |*zi| {
zi.source = switch (try reader.readByte()) {
1 => switch (zi.source) {
.tzdata_standard => .tzdata_utc,
else => return error.InvalidFormat,
},
0 => zi.source,
else => return error.InvalidFormat,
};
}
}
// Parse TZ string from footer
if ((try reader.readByte()) != '\n') return error.InvalidFormat;
const tz_string = blk: {
var buf: [60]u8 = undefined;
const tz_string = try reader.readUntilDelimiter(&buf, '\n');
break :blk try allocator.dupe(u8, tz_string);
};
errdefer allocator.free(tz_string);
const posix_tz: ?Timezone.POSIX_TZ = if (tz_string.len > 0)
try parse_posix_tz(tz_string)
else
null;
return Timezone{
.id = tz_string,
.transitions = transitions,
.zones = zone_infos,
.posix_tz = posix_tz,
.designations = designations,
};
}
pub fn parse_file(allocator: std.mem.Allocator, path: []const u8) !Timezone {
const file = try std.fs.cwd().openFile(path, .{});
defer file.close();
return parse(allocator, file.reader(), file.seekableStream());
}
pub fn parse_memory(allocator: std.mem.Allocator, data: []const u8) !Timezone {
var stream = std.io.fixedBufferStream(data);
return parse(allocator, stream.reader(), stream.seekableStream());
}
const log = std.log.scoped(.tempora);
const Timezone = @import("../Timezone.zig");
const testing = std.testing;
const std = @import("std");
|
0 | repos/tempora | repos/tempora/test/parse_tzif.zig |
test "parse invalid bytes" {
try testing.expectError(error.InvalidFormat, parse_memory(std.testing.allocator, "dflkasjreklnlkvnalkfek"));
}
test "parse UTC zoneinfo" {
var res = try parse_memory(std.testing.allocator, @embedFile("zoneinfo/UTC"));
defer res.deinit(std.testing.allocator);
try testing.expectEqual(0, res.transitions.len);
try testing.expectEqualDeep(&[_]Timezone.Zone_Info {
.{
.designation = "UTC",
.begin_ts = null,
.end_ts = null,
.offset = 0,
.is_dst = false,
.source = .tzdata_wall,
}
}, res.zones);
}
test "parse Pacific/Honolulu zoneinfo and calculate local times" {
const transition_times = [7]i64{ -2334101314, -1157283000, -1155436200, -880198200, -769395600, -765376200, -712150200 };
const transition_types = [7]u8{ 1, 2, 1, 3, 4, 1, 5 };
const zones = [6]Timezone.Zone_Info{
.{ .designation = "LMT", .begin_ts = null, .end_ts = null, .offset = -37886, .is_dst = false, .source = .tzdata_wall },
.{ .designation = "HST", .begin_ts = null, .end_ts = null, .offset = -37800, .is_dst = false, .source = .tzdata_wall },
.{ .designation = "HDT", .begin_ts = null, .end_ts = null, .offset = -34200, .is_dst = true, .source = .tzdata_wall },
.{ .designation = "HWT", .begin_ts = null, .end_ts = null, .offset = -34200, .is_dst = true, .source = .tzdata_wall },
.{ .designation = "HPT", .begin_ts = null, .end_ts = null, .offset = -34200, .is_dst = true, .source = .tzdata_utc },
.{ .designation = "HST", .begin_ts = null, .end_ts = null, .offset = -36000, .is_dst = false, .source = .tzdata_wall },
};
var res = try parse_memory(std.testing.allocator, @embedFile("zoneinfo/Pacific/Honolulu"));
defer res.deinit(std.testing.allocator);
try testing.expectEqualStrings("HST10", res.id);
try testing.expectEqualStrings("LMT\x00HST\x00HDT\x00HWT\x00HPT\x00", res.designations);
try testing.expectEqualSlices(i64, &transition_times, res.transitions.items(.ts));
try testing.expectEqualStrings(&transition_types, res.transitions.items(.zone_index));
try testing.expectEqualDeep(&zones, res.zones);
{
const zi = res.zone_info(-1156939200);
try testing.expectEqual(-34200, zi.offset);
try testing.expect(zi.is_dst);
try testing.expectEqualStrings("HDT", zi.designation);
}
{
// A second before the first timezone transition
const zi = res.zone_info(-2334101315);
try testing.expectEqual(-37886, zi.offset);
try testing.expectEqual(false, zi.is_dst);
try testing.expectEqualStrings("LMT", zi.designation);
}
{
// At the first timezone transition
const zi = res.zone_info(-2334101314);
try testing.expectEqual(-37800, zi.offset);
try testing.expectEqual(false, zi.is_dst);
try testing.expectEqualStrings("HST", zi.designation);
}
{
// After the first timezone transition
const zi = res.zone_info(-2334101313);
try testing.expectEqual(-37800, zi.offset);
try testing.expectEqual(false, zi.is_dst);
try testing.expectEqualStrings("HST", zi.designation);
}
{
// After the last timezone transition; conversion should be performed using the Posix TZ footer.
// Taken from RFC8536 Appendix B.2
const zi = res.zone_info(1546300800);
try testing.expectEqual(-10 * std.time.s_per_hour, zi.offset);
try testing.expectEqual(false, zi.is_dst);
try testing.expectEqual(.posix_tz, zi.source);
try testing.expectEqualStrings("HST", zi.designation);
}
}
test "posix TZ string, regular year" {
// IANA identifier America/Denver; default DST transition time at 2 am
var result = try parse_posix_tz("MST7MDT,M3.2.0,M11.1.0");
var stdoff: i32 = -25200;
var dstoff: i32 = -21600;
try testing.expectEqualStrings("MST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("MDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
try testing.expectEqual(Timezone.POSIX_TZ.Rule{ .month_nth_week_day = .{ .month = 3, .n = 2, .day = 0, .time = 2 * std.time.s_per_hour } }, result.dst_range.?.start);
try testing.expectEqual(Timezone.POSIX_TZ.Rule{ .month_nth_week_day = .{ .month = 11, .n = 1, .day = 0, .time = 2 * std.time.s_per_hour } }, result.dst_range.?.end);
try testing.expectEqual(stdoff, result.zone_info(1612734960, 0).offset);
// 2021-03-14T01:59:59-07:00 (2nd Sunday of the 3rd month, MST)
try testing.expectEqual(stdoff, result.zone_info(1615712399, 0).offset);
// 2021-03-14T02:00:00-07:00 (2nd Sunday of the 3rd month, MST)
try testing.expectEqual(dstoff, result.zone_info(1615712400, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1620453601, 0).offset);
// 2021-11-07T01:59:59-06:00 (1st Sunday of the 11th month, MDT)
try testing.expectEqual(dstoff, result.zone_info(1636271999, 0).offset);
// 2021-11-07T02:00:00-06:00 (1st Sunday of the 11th month, MDT)
try testing.expectEqual(stdoff, result.zone_info(1636272000, 0).offset);
// IANA identifier: Europe/Berlin
result = try parse_posix_tz("CET-1CEST,M3.5.0,M10.5.0/3");
stdoff = 3600;
dstoff = 7200;
try testing.expectEqualStrings("CET", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("CEST", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
try testing.expectEqual(Timezone.POSIX_TZ.Rule{ .month_nth_week_day = .{ .month = 3, .n = 5, .day = 0, .time = 2 * std.time.s_per_hour } }, result.dst_range.?.start);
try testing.expectEqual(Timezone.POSIX_TZ.Rule{ .month_nth_week_day = .{ .month = 10, .n = 5, .day = 0, .time = 3 * std.time.s_per_hour } }, result.dst_range.?.end);
// 2023-10-29T00:59:59Z, or 2023-10-29 01:59:59 CEST. Offset should still be CEST.
try testing.expectEqual(dstoff, result.zone_info(1698541199, 0).offset);
// 2023-10-29T01:00:00Z, or 2023-10-29 03:00:00 CEST. Offset should now be CET.
try testing.expectEqual(stdoff, result.zone_info(1698541200, 0).offset);
// IANA identifier: America/New_York
result = try parse_posix_tz("EST5EDT,M3.2.0/02:00:00,M11.1.0");
stdoff = -18000;
dstoff = -14400;
try testing.expectEqualStrings("EST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("EDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2023-03-12T01:59:59-05:00 --> dst 2023-03-12T03:00:00-04:00
try testing.expectEqual(stdoff, result.zone_info(1678604399, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1678604400, 0).offset);
// transition dst 2023-11-05T01:59:59-04:00 --> std 2023-11-05T01:00:00-05:00
try testing.expectEqual(dstoff, result.zone_info(1699163999, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1699164000, 0).offset);
// IANA identifier: America/New_York
result = try parse_posix_tz("EST5EDT,M3.2.0/02:00:00,M11.1.0/02:00:00");
stdoff = -18000;
dstoff = -14400;
try testing.expectEqualStrings("EST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("EDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2023-03-12T01:59:59-05:00 --> dst 2023-03-12T03:00:00-04:00
try testing.expectEqual(stdoff, result.zone_info(1678604399, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1678604400, 0).offset);
// transition dst 2023-11-05T01:59:59-04:00 --> std 2023-11-05T01:00:00-05:00
try testing.expectEqual(dstoff, result.zone_info(1699163999, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1699164000, 0).offset);
// IANA identifier: America/New_York
result = try parse_posix_tz("EST5EDT,M3.2.0,M11.1.0/02:00:00");
stdoff = -18000;
dstoff = -14400;
try testing.expectEqualStrings("EST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("EDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2023-03-12T01:59:59-05:00 --> dst 2023-03-12T03:00:00-04:00
try testing.expectEqual(stdoff, result.zone_info(1678604399, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1678604400, 0).offset);
// transition dst 2023-11-05T01:59:59-04:00 --> std 2023-11-05T01:00:00-05:00
try testing.expectEqual(dstoff, result.zone_info(1699163999, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1699164000, 0).offset);
// IANA identifier: America/Chicago
result = try parse_posix_tz("CST6CDT,M3.2.0/2:00:00,M11.1.0/2:00:00");
stdoff = -21600;
dstoff = -18000;
try testing.expectEqualStrings("CST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("CDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2023-03-12T01:59:59-06:00 --> dst 2023-03-12T03:00:00-05:00
try testing.expectEqual(stdoff, result.zone_info(1678607999, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1678608000, 0).offset);
// transition dst 2023-11-05T01:59:59-05:00 --> std 2023-11-05T01:00:00-06:00
try testing.expectEqual(dstoff, result.zone_info(1699167599, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1699167600, 0).offset);
// IANA identifier: America/Denver
result = try parse_posix_tz("MST7MDT,M3.2.0/2:00:00,M11.1.0/2:00:00");
stdoff = -25200;
dstoff = -21600;
try testing.expectEqualStrings("MST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("MDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2023-03-12T01:59:59-07:00 --> dst 2023-03-12T03:00:00-06:00
try testing.expectEqual(stdoff, result.zone_info(1678611599, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1678611600, 0).offset);
// transition dst 2023-11-05T01:59:59-06:00 --> std 2023-11-05T01:00:00-07:00
try testing.expectEqual(dstoff, result.zone_info(1699171199, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1699171200, 0).offset);
// IANA identifier: America/Los_Angeles
result = try parse_posix_tz("PST8PDT,M3.2.0/2:00:00,M11.1.0/2:00:00");
stdoff = -28800;
dstoff = -25200;
try testing.expectEqualStrings("PST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("PDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2023-03-12T01:59:59-08:00 --> dst 2023-03-12T03:00:00-07:00
try testing.expectEqual(stdoff, result.zone_info(1678615199, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1678615200, 0).offset);
// transition dst 2023-11-05T01:59:59-07:00 --> std 2023-11-05T01:00:00-08:00
try testing.expectEqual(dstoff, result.zone_info(1699174799, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1699174800, 0).offset);
// IANA identifier: America/Sitka
result = try parse_posix_tz("AKST9AKDT,M3.2.0,M11.1.0");
stdoff = -32400;
dstoff = -28800;
try testing.expectEqualStrings("AKST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("AKDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2023-03-12T01:59:59-09:00 --> dst 2023-03-12T03:00:00-08:00
try testing.expectEqual(stdoff, result.zone_info(1678618799, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1678618800, 0).offset);
// transition dst 2023-11-05T01:59:59-08:00 --> std 2023-11-05T01:00:00-09:00
try testing.expectEqual(dstoff, result.zone_info(1699178399, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1699178400, 0).offset);
// IANA identifier: Asia/Jerusalem
result = try parse_posix_tz("IST-2IDT,M3.4.4/26,M10.5.0");
stdoff = 7200;
dstoff = 10800;
try testing.expectEqualStrings("IST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("IDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2023-03-24T01:59:59+02:00 --> dst 2023-03-24T03:00:00+03:00
try testing.expectEqual(stdoff, result.zone_info(1679615999, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1679616000, 0).offset);
// transition dst 2023-10-29T01:59:59+03:00 --> std 2023-10-29T01:00:00+02:00
try testing.expectEqual(dstoff, result.zone_info(1698533999, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1698534000, 0).offset);
// IANA identifier: America/Argentina/Buenos_Aires
result = try parse_posix_tz("WART4WARST,J1/0,J365/25"); // TODO : separate tests for jday ?
stdoff = -10800;
dstoff = -10800;
try testing.expectEqualStrings("WART", result.std_designation);
try testing.expectEqualStrings("WARST", result.dst_designation.?);
// transition std 2023-03-24T01:59:59-03:00 --> dst 2023-03-24T03:00:00-03:00
try testing.expectEqual(stdoff, result.zone_info(1679633999, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1679637600, 0).offset);
// transition dst 2023-10-29T01:59:59-03:00 --> std 2023-10-29T01:00:00-03:00
try testing.expectEqual(dstoff, result.zone_info(1698555599, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1698552000, 0).offset);
// IANA identifier: America/Nuuk
result = try parse_posix_tz("WGT3WGST,M3.5.0/-2,M10.5.0/-1");
stdoff = -10800;
dstoff = -7200;
try testing.expectEqualStrings("WGT", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("WGST", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2021-03-27T21:59:59-03:00 --> dst 2021-03-27T23:00:00-02:00
try testing.expectEqual(stdoff, result.zone_info(1616893199, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1616893200, 0).offset);
// transition dst 2021-10-30T22:59:59-02:00 --> std 2021-10-30T22:00:00-03:00
try testing.expectEqual(dstoff, result.zone_info(1635641999, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1635642000, 0).offset);
}
test "posix TZ string, leap year, America/New_York, start transition time specified" {
// IANA identifier: America/New_York
const result = try parse_posix_tz("EST5EDT,M3.2.0/02:00:00,M11.1.0");
const stdoff: i32 = -18000;
const dstoff: i32 = -14400;
try testing.expectEqualStrings("EST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("EDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2020-03-08T01:59:59-05:00 --> dst 2020-03-08T03:00:00-04:00
try testing.expectEqual(stdoff, result.zone_info(1583650799, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1583650800, 0).offset);
// transition dst 2020-11-01T01:59:59-04:00 --> std 2020-11-01T01:00:00-05:00
try testing.expectEqual(dstoff, result.zone_info(1604210399, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1604210400, 0).offset);
}
test "posix TZ string, leap year, America/New_York, both transition times specified" {
// IANA identifier: America/New_York
const result = try parse_posix_tz("EST5EDT,M3.2.0/02:00:00,M11.1.0/02:00:00");
const stdoff: i32 = -18000;
const dstoff: i32 = -14400;
try testing.expectEqualStrings("EST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("EDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2020-03-08T01:59:59-05:00 --> dst 2020-03-08T03:00:00-04:00
try testing.expectEqual(stdoff, result.zone_info(1583650799, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1583650800, 0).offset);
// transtion dst 2020-11-01T01:59:59-04:00 --> std 2020-11-01T01:00:00-05:00
try testing.expectEqual(dstoff, result.zone_info(1604210399, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1604210400, 0).offset);
}
test "posix TZ string, leap year, America/New_York, end transition time specified" {
// IANA identifier: America/New_York
const result = try parse_posix_tz("EST5EDT,M3.2.0,M11.1.0/02:00:00");
const stdoff: i32 = -18000;
const dstoff: i32 = -14400;
try testing.expectEqualStrings("EST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("EDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2020-03-08T01:59:59-05:00 --> dst 2020-03-08T03:00:00-04:00
try testing.expectEqual(stdoff, result.zone_info(1583650799, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1583650800, 0).offset);
// transtion dst 2020-11-01T01:59:59-04:00 --> std 2020-11-01T01:00:00-05:00
try testing.expectEqual(dstoff, result.zone_info(1604210399, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1604210400, 0).offset);
}
test "posix TZ string, leap year, America/Chicago, both transition times specified" {
// IANA identifier: America/Chicago
const result = try parse_posix_tz("CST6CDT,M3.2.0/2:00:00,M11.1.0/2:00:00");
const stdoff: i32 = -21600;
const dstoff: i32 = -18000;
try testing.expectEqualStrings("CST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("CDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2020-03-08T01:59:59-06:00 --> dst 2020-03-08T03:00:00-05:00
try testing.expectEqual(stdoff, result.zone_info(1583654399, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1583654400, 0).offset);
// transtion dst 2020-11-01T01:59:59-05:00 --> std 2020-11-01T01:00:00-06:00
try testing.expectEqual(dstoff, result.zone_info(1604213999, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1604214000, 0).offset);
}
test "posix TZ string, leap year, America/Denver, both transition times specified" {
// IANA identifier: America/Denver
const result = try parse_posix_tz("MST7MDT,M3.2.0/2:00:00,M11.1.0/2:00:00");
const stdoff: i32 = -25200;
const dstoff: i32 = -21600;
try testing.expectEqualStrings("MST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("MDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2020-03-08T01:59:59-07:00 --> dst 2020-03-08T03:00:00-06:00
try testing.expectEqual(stdoff, result.zone_info(1583657999, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1583658000, 0).offset);
// transtion dst 2020-11-01T01:59:59-06:00 --> std 2020-11-01T01:00:00-07:00
try testing.expectEqual(dstoff, result.zone_info(1604217599, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1604217600, 0).offset);
}
test "posix TZ string, leap year, America/Los_Angeles, both transition times specified" {
// IANA identifier: America/Los_Angeles
const result = try parse_posix_tz("PST8PDT,M3.2.0/2:00:00,M11.1.0/2:00:00");
const stdoff: i32 = -28800;
const dstoff: i32 = -25200;
try testing.expectEqualStrings("PST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("PDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2020-03-08T01:59:59-08:00 --> dst 2020-03-08T03:00:00-07:00
try testing.expectEqual(stdoff, result.zone_info(1583661599, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1583661600, 0).offset);
// transtion dst 2020-11-01T01:59:59-07:00 --> std 2020-11-01T01:00:00-08:00
try testing.expectEqual(dstoff, result.zone_info(1604221199, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1604221200, 0).offset);
}
test "posix TZ string, leap year, America/Sitka" {
// IANA identifier: America/Sitka
const result = try parse_posix_tz("AKST9AKDT,M3.2.0,M11.1.0");
const stdoff: i32 = -32400;
const dstoff: i32 = -28800;
try testing.expectEqualStrings("AKST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("AKDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2020-03-08T01:59:59-09:00 --> dst 2020-03-08T03:00:00-08:00
try testing.expectEqual(stdoff, result.zone_info(1583665199, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1583665200, 0).offset);
// transtion dst 2020-11-01T01:59:59-08:00 --> std 2020-11-01T01:00:00-09:00
try testing.expectEqual(dstoff, result.zone_info(1604224799, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1604224800, 0).offset);
}
test "posix TZ string, leap year, Asia/Jerusalem" {
// IANA identifier: Asia/Jerusalem
const result = try parse_posix_tz("IST-2IDT,M3.4.4/26,M10.5.0");
const stdoff: i32 = 7200;
const dstoff: i32 = 10800;
try testing.expectEqualStrings("IST", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("IDT", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2020-03-27T01:59:59+02:00 --> dst 2020-03-27T03:00:00+03:00
try testing.expectEqual(stdoff, result.zone_info(1585267199, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1585267200, 0).offset);
// transtion dst 2020-10-25T01:59:59+03:00 --> std 2020-10-25T01:00:00+02:00
try testing.expectEqual(dstoff, result.zone_info(1603580399, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1603580400, 0).offset);
}
// Buenos Aires has DST all year long, make sure that it never returns the STD offset
test "posix TZ string, leap year, America/Argentina/Buenos_Aires" {
// IANA identifier: America/Argentina/Buenos_Aires
const result = try parse_posix_tz("WART4WARST,J1/0,J365/25");
const stdoff: i32 = -4 * std.time.s_per_hour;
const dstoff: i32 = -3 * std.time.s_per_hour;
try testing.expectEqualStrings("WART", result.std_designation);
try testing.expectEqualStrings("WARST", result.dst_designation.?);
_ = stdoff;
// transition std 2020-03-27T01:59:59-03:00 --> dst 2020-03-27T03:00:00-03:00
try testing.expectEqual(dstoff, result.zone_info(1585285199, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1585288800, 0).offset);
// transtion dst 2020-10-25T01:59:59-03:00 --> std 2020-10-25T01:00:00-03:00
try testing.expectEqual(dstoff, result.zone_info(1603601999, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1603598400, 0).offset);
// Make sure it returns dstoff at the start of the year
try testing.expectEqual(dstoff, result.zone_info(1577836800, 0).offset); // 2020
try testing.expectEqual(dstoff, result.zone_info(1609459200, 0).offset); // 2021
// Make sure it returns dstoff at the end of the year
try testing.expectEqual(dstoff, result.zone_info(1609459199, 0).offset);
}
test "posix TZ string, leap year, America/Nuuk" {
// IANA identifier: America/Nuuk
const result = try parse_posix_tz("WGT3WGST,M3.5.0/-2,M10.5.0/-1");
const stdoff: i32 = -10800;
const dstoff: i32 = -7200;
try testing.expectEqualStrings("WGT", result.std_designation);
try testing.expectEqual(stdoff, result.std_offset);
try testing.expectEqualStrings("WGST", result.dst_designation.?);
try testing.expectEqual(dstoff, result.dst_offset);
// transition std 2020-03-28T21:59:59-03:00 --> dst 2020-03-28T23:00:00-02:00
try testing.expectEqual(stdoff, result.zone_info(1585443599, 0).offset);
try testing.expectEqual(dstoff, result.zone_info(1585443600, 0).offset);
// transtion dst 2020-10-24T22:59:59-02:00 --> std 2020-10-24T22:00:00-03:00
try testing.expectEqual(dstoff, result.zone_info(1603587599, 0).offset);
try testing.expectEqual(stdoff, result.zone_info(1603587600, 0).offset);
}
test "posix TZ, valid strings" {
// from CPython's zoneinfo tests;
// https://github.com/python/cpython/blob/main/Lib/test/test_zoneinfo/test_zoneinfo.py
const tzstrs = [_][]const u8{
// Extreme offset hour
"AAA24",
"AAA+24",
"AAA-24",
"AAA24BBB,J60/2,J300/2",
"AAA+24BBB,J60/2,J300/2",
"AAA-24BBB,J60/2,J300/2",
"AAA4BBB24,J60/2,J300/2",
"AAA4BBB+24,J60/2,J300/2",
"AAA4BBB-24,J60/2,J300/2",
// Extreme offset minutes
"AAA4:00BBB,J60/2,J300/2",
"AAA4:59BBB,J60/2,J300/2",
"AAA4BBB5:00,J60/2,J300/2",
"AAA4BBB5:59,J60/2,J300/2",
// Extreme offset seconds
"AAA4:00:00BBB,J60/2,J300/2",
"AAA4:00:59BBB,J60/2,J300/2",
"AAA4BBB5:00:00,J60/2,J300/2",
"AAA4BBB5:00:59,J60/2,J300/2",
// Extreme total offset
"AAA24:59:59BBB5,J60/2,J300/2",
"AAA-24:59:59BBB5,J60/2,J300/2",
"AAA4BBB24:59:59,J60/2,J300/2",
"AAA4BBB-24:59:59,J60/2,J300/2",
// Extreme months
"AAA4BBB,M12.1.1/2,M1.1.1/2",
"AAA4BBB,M1.1.1/2,M12.1.1/2",
// Extreme weeks
"AAA4BBB,M1.5.1/2,M1.1.1/2",
"AAA4BBB,M1.1.1/2,M1.5.1/2",
// Extreme weekday
"AAA4BBB,M1.1.6/2,M2.1.1/2",
"AAA4BBB,M1.1.1/2,M2.1.6/2",
// Extreme numeric offset
"AAA4BBB,0/2,20/2",
"AAA4BBB,0/2,0/14",
"AAA4BBB,20/2,365/2",
"AAA4BBB,365/2,365/14",
// Extreme julian offset
"AAA4BBB,J1/2,J20/2",
"AAA4BBB,J1/2,J1/14",
"AAA4BBB,J20/2,J365/2",
"AAA4BBB,J365/2,J365/14",
// Extreme transition hour
"AAA4BBB,J60/167,J300/2",
"AAA4BBB,J60/+167,J300/2",
"AAA4BBB,J60/-167,J300/2",
"AAA4BBB,J60/2,J300/167",
"AAA4BBB,J60/2,J300/+167",
"AAA4BBB,J60/2,J300/-167",
// Extreme transition minutes
"AAA4BBB,J60/2:00,J300/2",
"AAA4BBB,J60/2:59,J300/2",
"AAA4BBB,J60/2,J300/2:00",
"AAA4BBB,J60/2,J300/2:59",
// Extreme transition seconds
"AAA4BBB,J60/2:00:00,J300/2",
"AAA4BBB,J60/2:00:59,J300/2",
"AAA4BBB,J60/2,J300/2:00:00",
"AAA4BBB,J60/2,J300/2:00:59",
// Extreme total transition time
"AAA4BBB,J60/167:59:59,J300/2",
"AAA4BBB,J60/-167:59:59,J300/2",
"AAA4BBB,J60/2,J300/167:59:59",
"AAA4BBB,J60/2,J300/-167:59:59",
};
for (tzstrs) |valid_str| {
_ = try parse_posix_tz(valid_str);
}
}
// The following tests are from CPython's zoneinfo tests;
// https://github.com/python/cpython/blob/main/Lib/test/test_zoneinfo/test_zoneinfo.py
test "posix TZ invalid string, unquoted alphanumeric" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("+11"));
}
test "posix TZ invalid string, unquoted alphanumeric in DST" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("GMT0+11,M3.2.0/2,M11.1.0/3"));
}
test "posix TZ invalid string, DST but no transition specified" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("PST8PDT"));
}
test "posix TZ invalid string, only one transition rule" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("PST8PDT,M3.2.0/2"));
}
test "posix TZ invalid string, transition rule but no DST" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("GMT,M3.2.0/2,M11.1.0/3"));
}
test "posix TZ invalid offset hours" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA168"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA+168"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA-168"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA168BBB,J60/2,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA+168BBB,J60/2,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA-168BBB,J60/2,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB168,J60/2,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB+168,J60/2,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB-168,J60/2,J300/2"));
}
test "posix TZ invalid offset minutes" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4:0BBB,J60/2,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4:100BBB,J60/2,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB5:0,J60/2,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB5:100,J60/2,J300/2"));
}
test "posix TZ invalid offset seconds" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4:00:0BBB,J60/2,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4:00:100BBB,J60/2,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB5:00:0,J60/2,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB5:00:100,J60/2,J300/2"));
}
test "posix TZ completely invalid dates" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,M1443339,M11.1.0/3"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,M3.2.0/2,0349309483959c"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,z,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/2,"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/2,z"));
}
test "posix TZ invalid months" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,M13.1.1/2,M1.1.1/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,M1.1.1/2,M13.1.1/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,M0.1.1/2,M1.1.1/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,M1.1.1/2,M0.1.1/2"));
}
test "posix TZ invalid weeks" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,M1.6.1/2,M1.1.1/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,M1.1.1/2,M1.6.1/2"));
}
test "posix TZ invalid weekday" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,M1.1.7/2,M2.1.1/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,M1.1.1/2,M2.1.7/2"));
}
test "posix TZ invalid numeric offset" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,-1/2,20/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,1/2,-1/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,367,20/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,1/2,367/2"));
}
test "posix TZ invalid julian offset" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J0/2,J20/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J20/2,J366/2"));
}
test "posix TZ invalid transition time" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/2/3,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/2,J300/2/3"));
}
test "posix TZ invalid transition hour" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/168,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/+168,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/-168,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/2,J300/168"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/2,J300/+168"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/2,J300/-168"));
}
test "posix TZ invalid transition minutes" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/2:0,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/2:100,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/2,J300/2:0"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/2,J300/2:100"));
}
test "posix TZ invalid transition seconds" {
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/2:00:0,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/2:00:100,J300/2"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/2,J300/2:00:0"));
try std.testing.expectError(error.InvalidFormat, parse_posix_tz("AAA4BBB,J60/2,J300/2:00:100"));
}
test "posix TZ EST5EDT,M3.2.0/4:00,M11.1.0/3:00 from zoneinfo_test.py" {
// Transition to EDT on the 2nd Sunday in March at 4 AM, and
// transition back on the first Sunday in November at 3AM
const result = try parse_posix_tz("EST5EDT,M3.2.0/4:00,M11.1.0/3:00");
try testing.expectEqual(@as(i32, -18000), result.zone_info(1552107600, 0).offset); // 2019-03-09T00:00:00-05:00
try testing.expectEqual(@as(i32, -18000), result.zone_info(1552208340, 0).offset); // 2019-03-10T03:59:00-05:00
try testing.expectEqual(@as(i32, -14400), result.zone_info(1572667200, 0).offset); // 2019-11-02T00:00:00-04:00
try testing.expectEqual(@as(i32, -14400), result.zone_info(1572760740, 0).offset); // 2019-11-03T01:59:00-04:00
try testing.expectEqual(@as(i32, -14400), result.zone_info(1572760800, 0).offset); // 2019-11-03T02:00:00-04:00
try testing.expectEqual(@as(i32, -18000), result.zone_info(1572764400, 0).offset); // 2019-11-03T02:00:00-05:00
try testing.expectEqual(@as(i32, -18000), result.zone_info(1583657940, 0).offset); // 2020-03-08T03:59:00-05:00
try testing.expectEqual(@as(i32, -14400), result.zone_info(1604210340, 0).offset); // 2020-11-01T01:59:00-04:00
try testing.expectEqual(@as(i32, -14400), result.zone_info(1604210400, 0).offset); // 2020-11-01T02:00:00-04:00
try testing.expectEqual(@as(i32, -18000), result.zone_info(1604214000, 0).offset); // 2020-11-01T02:00:00-05:00
}
test "posix TZ GMT0BST-1,M3.5.0/1:00,M10.5.0/2:00 from zoneinfo_test.py" {
// Transition to BST happens on the last Sunday in March at 1 AM GMT
// and the transition back happens the last Sunday in October at 2AM BST
const result = try parse_posix_tz("GMT0BST-1,M3.5.0/1:00,M10.5.0/2:00");
try testing.expectEqual(@as(i32, 0), result.zone_info(1553904000, 0).offset); // 2019-03-30T00:00:00+00:00
try testing.expectEqual(@as(i32, 0), result.zone_info(1553993940, 0).offset); // 2019-03-31T00:59:00+00:00
try testing.expectEqual(@as(i32, 3600), result.zone_info(1553994000, 0).offset); // 2019-03-31T02:00:00+01:00
try testing.expectEqual(@as(i32, 3600), result.zone_info(1572044400, 0).offset); // 2019-10-26T00:00:00+01:00
try testing.expectEqual(@as(i32, 3600), result.zone_info(1572134340, 0).offset); // 2019-10-27T00:59:00+01:00
try testing.expectEqual(@as(i32, 0), result.zone_info(1585443540, 0).offset); // 2020-03-29T00:59:00+00:00
try testing.expectEqual(@as(i32, 3600), result.zone_info(1585443600, 0).offset); // 2020-03-29T02:00:00+01:00
try testing.expectEqual(@as(i32, 3600), result.zone_info(1603583940, 0).offset); // 2020-10-25T00:59:00+01:00
try testing.expectEqual(@as(i32, 3600), result.zone_info(1603584000, 0).offset); // 2020-10-25T01:00:00+01:00
try testing.expectEqual(@as(i32, 0), result.zone_info(1603591200, 0).offset); // 2020-10-25T02:00:00+00:00
}
test "posix TZ AEST-10AEDT,M10.1.0/2,M4.1.0/3 from zoneinfo_test.py" {
// Austrialian time zone - DST start is chronologically first
const result = try parse_posix_tz("AEST-10AEDT,M10.1.0/2,M4.1.0/3");
try testing.expectEqual(@as(i32, 39600), result.zone_info(1554469200, 0).offset); // 2019-04-06T00:00:00+11:00
try testing.expectEqual(@as(i32, 39600), result.zone_info(1554562740, 0).offset); // 2019-04-07T01:59:00+11:00
try testing.expectEqual(@as(i32, 39600), result.zone_info(1554562740, 0).offset); // 2019-04-07T01:59:00+11:00
try testing.expectEqual(@as(i32, 39600), result.zone_info(1554562800, 0).offset); // 2019-04-07T02:00:00+11:00
try testing.expectEqual(@as(i32, 39600), result.zone_info(1554562860, 0).offset); // 2019-04-07T02:01:00+11:00
try testing.expectEqual(@as(i32, 36000), result.zone_info(1554566400, 0).offset); // 2019-04-07T02:00:00+10:00
try testing.expectEqual(@as(i32, 36000), result.zone_info(1554566460, 0).offset); // 2019-04-07T02:01:00+10:00
try testing.expectEqual(@as(i32, 36000), result.zone_info(1554570000, 0).offset); // 2019-04-07T03:00:00+10:00
try testing.expectEqual(@as(i32, 36000), result.zone_info(1554570000, 0).offset); // 2019-04-07T03:00:00+10:00
try testing.expectEqual(@as(i32, 36000), result.zone_info(1570197600, 0).offset); // 2019-10-05T00:00:00+10:00
try testing.expectEqual(@as(i32, 36000), result.zone_info(1570291140, 0).offset); // 2019-10-06T01:59:00+10:00
try testing.expectEqual(@as(i32, 39600), result.zone_info(1570291200, 0).offset); // 2019-10-06T03:00:00+11:00
}
test "posix TZ IST-1GMT0,M10.5.0,M3.5.0/1 from zoneinfo_test.py" {
// Irish time zone - negative DST
const result = try parse_posix_tz("IST-1GMT0,M10.5.0,M3.5.0/1");
try testing.expectEqual(@as(i32, 0), result.zone_info(1553904000, 0).offset); // 2019-03-30T00:00:00+00:00
try testing.expectEqual(@as(i32, 0), result.zone_info(1553993940, 0).offset); // 2019-03-31T00:59:00+00:00
try testing.expectEqual(true, result.zone_info(1553993940, 0).is_dst); // 2019-03-31T00:59:00+00:00
try testing.expectEqual(@as(i32, 3600), result.zone_info(1553994000, 0).offset); // 2019-03-31T02:00:00+01:00
try testing.expectEqual(false, result.zone_info(1553994000, 0).is_dst); // 2019-03-31T02:00:00+01:00
try testing.expectEqual(@as(i32, 3600), result.zone_info(1572044400, 0).offset); // 2019-10-26T00:00:00+01:00
try testing.expectEqual(@as(i32, 3600), result.zone_info(1572134340, 0).offset); // 2019-10-27T00:59:00+01:00
try testing.expectEqual(@as(i32, 3600), result.zone_info(1572134400, 0).offset); // 2019-10-27T01:00:00+01:00
try testing.expectEqual(@as(i32, 0), result.zone_info(1572138000, 0).offset); // 2019-10-27T01:00:00+00:00
try testing.expectEqual(@as(i32, 0), result.zone_info(1572141600, 0).offset); // 2019-10-27T02:00:00+00:00
try testing.expectEqual(@as(i32, 0), result.zone_info(1585443540, 0).offset); // 2020-03-29T00:59:00+00:00
try testing.expectEqual(@as(i32, 3600), result.zone_info(1585443600, 0).offset); // 2020-03-29T02:00:00+01:00
try testing.expectEqual(@as(i32, 3600), result.zone_info(1603583940, 0).offset); // 2020-10-25T00:59:00+01:00
try testing.expectEqual(@as(i32, 3600), result.zone_info(1603584000, 0).offset); // 2020-10-25T01:00:00+01:00
try testing.expectEqual(@as(i32, 0), result.zone_info(1603591200, 0).offset); // 2020-10-25T02:00:00+00:00
}
test "posix TZ <+11>-11 from zoneinfo_test.py" {
// Pacific/Kosrae: Fixed offset zone with a quoted numerical tzname
const result = try parse_posix_tz("<+11>-11");
try testing.expectEqual(@as(i32, 39600), result.zone_info(1577797200, 0).offset); // 2020-01-01T00:00:00+11:00
}
test "posix TZ <-04>4<-03>,M9.1.6/24,M4.1.6/24 from zoneinfo_test.py" {
// Quoted STD and DST, transitions at 24:00
const result = try parse_posix_tz("<-04>4<-03>,M9.1.6/24,M4.1.6/24");
try testing.expectEqual(@as(i32, -14400), result.zone_info(1588305600, 0).offset); // 2020-05-01T00:00:00-04:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1604199600, 0).offset); // 2020-11-01T00:00:00-03:00
}
test "posix TZ EST5EDT,0/0,J365/25 from zoneinfo_test.py" {
// Permanent daylight saving time is modeled with transitions at 0/0
// and J365/25, as mentioned in RFC 8536 Section 3.3.1
const result = try parse_posix_tz("EST5EDT,0/0,J365/25");
try testing.expectEqual(@as(i32, -14400), result.zone_info(1546315200, 0).offset); // 2019-01-01T00:00:00-04:00
try testing.expectEqual(@as(i32, -14400), result.zone_info(1559361600, 0).offset); // 2019-06-01T00:00:00-04:00
try testing.expectEqual(@as(i32, -14400), result.zone_info(1577851199, 0).offset); // 2019-12-31T23:59:59.999999-04:00
try testing.expectEqual(@as(i32, -14400), result.zone_info(1577851200, 0).offset); // 2020-01-01T00:00:00-04:00
try testing.expectEqual(@as(i32, -14400), result.zone_info(1583035200, 0).offset); // 2020-03-01T00:00:00-04:00
try testing.expectEqual(@as(i32, -14400), result.zone_info(1590984000, 0).offset); // 2020-06-01T00:00:00-04:00
try testing.expectEqual(@as(i32, -14400), result.zone_info(1609473599, 0).offset); // 2020-12-31T23:59:59.999999-04:00
try testing.expectEqual(@as(i32, -14400), result.zone_info(13569480000, 0).offset); // 2400-01-01T00:00:00-04:00
try testing.expectEqual(@as(i32, -14400), result.zone_info(13574664000, 0).offset); // 2400-03-01T00:00:00-04:00
try testing.expectEqual(@as(i32, -14400), result.zone_info(13601102399, 0).offset); // 2400-12-31T23:59:59.999999-04:00
}
test "posix TZ AAA3BBB,J60/12,J305/12 from zoneinfo_test.py" {
// Transitions on March 1st and November 1st of each year
const result = try parse_posix_tz("AAA3BBB,J60/12,J305/12");
try testing.expectEqual(@as(i32, -10800), result.zone_info(1546311600, 0).offset); // 2019-01-01T00:00:00-03:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1551322800, 0).offset); // 2019-02-28T00:00:00-03:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1551452340, 0).offset); // 2019-03-01T11:59:00-03:00
try testing.expectEqual(@as(i32, -7200), result.zone_info(1551452400, 0).offset); // 2019-03-01T13:00:00-02:00
try testing.expectEqual(@as(i32, -7200), result.zone_info(1572613140, 0).offset); // 2019-11-01T10:59:00-02:00
try testing.expectEqual(@as(i32, -7200), result.zone_info(1572613200, 0).offset); // 2019-11-01T11:00:00-02:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1572616800, 0).offset); // 2019-11-01T11:00:00-03:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1572620400, 0).offset); // 2019-11-01T12:00:00-03:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1577847599, 0).offset); // 2019-12-31T23:59:59.999999-03:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1577847600, 0).offset); // 2020-01-01T00:00:00-03:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1582945200, 0).offset); // 2020-02-29T00:00:00-03:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1583074740, 0).offset); // 2020-03-01T11:59:00-03:00
try testing.expectEqual(@as(i32, -7200), result.zone_info(1583074800, 0).offset); // 2020-03-01T13:00:00-02:00
try testing.expectEqual(@as(i32, -7200), result.zone_info(1604235540, 0).offset); // 2020-11-01T10:59:00-02:00
try testing.expectEqual(@as(i32, -7200), result.zone_info(1604235600, 0).offset); // 2020-11-01T11:00:00-02:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1604239200, 0).offset); // 2020-11-01T11:00:00-03:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1604242800, 0).offset); // 2020-11-01T12:00:00-03:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1609469999, 0).offset); // 2020-12-31T23:59:59.999999-03:00
}
test "posix TZ <-03>3<-02>,M3.5.0/-2,M10.5.0/-1 from zoneinfo_test.py" {
// Taken from America/Godthab, this rule has a transition on the
// Saturday before the last Sunday of March and October, at 22:00 and 23:00,
// respectively. This is encoded with negative start and end transition times.
const result = try parse_posix_tz("<-03>3<-02>,M3.5.0/-2,M10.5.0/-1");
try testing.expectEqual(@as(i32, -10800), result.zone_info(1585278000, 0).offset); // 2020-03-27T00:00:00-03:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1585443599, 0).offset); // 2020-03-28T21:59:59-03:00
try testing.expectEqual(@as(i32, -7200), result.zone_info(1585443600, 0).offset); // 2020-03-28T23:00:00-02:00
try testing.expectEqual(@as(i32, -7200), result.zone_info(1603580400, 0).offset); // 2020-10-24T21:00:00-02:00
try testing.expectEqual(@as(i32, -7200), result.zone_info(1603584000, 0).offset); // 2020-10-24T22:00:00-02:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1603587600, 0).offset); // 2020-10-24T22:00:00-03:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1603591200, 0).offset); // 2020-10-24T23:00:00-03:00
}
test "posix TZ AAA3BBB,M3.2.0/01:30,M11.1.0/02:15:45 from zoneinfo_test.py" {
// Transition times with minutes and seconds
const result = try parse_posix_tz("AAA3BBB,M3.2.0/01:30,M11.1.0/02:15:45");
try testing.expectEqual(@as(i32, -10800), result.zone_info(1331438400, 0).offset); // 2012-03-11T01:00:00-03:00
try testing.expectEqual(@as(i32, -7200), result.zone_info(1331440200, 0).offset); // 2012-03-11T02:30:00-02:00
try testing.expectEqual(@as(i32, -7200), result.zone_info(1351998944, 0).offset); // 2012-11-04T01:15:44.999999-02:00
try testing.expectEqual(@as(i32, -7200), result.zone_info(1351998945, 0).offset); // 2012-11-04T01:15:45-02:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1352002545, 0).offset); // 2012-11-04T01:15:45-03:00
try testing.expectEqual(@as(i32, -10800), result.zone_info(1352006145, 0).offset); // 2012-11-04T02:15:45-03:00
}
const parse_memory = parse.parse_memory;
const parse_posix_tz = parse.parse_posix_tz;
const parse = tempora.tzdb.parse_tzif;
const Timezone = tempora.Timezone;
const tempora = @import("tempora");
const testing = std.testing;
const std = @import("std");
|
0 | repos/tempora | repos/tempora/tools/dump.zig | pub fn main() !u8 {
var gpa = std.heap.GeneralPurposeAllocator(.{}){};
defer _ = gpa.deinit();
const allocator = gpa.allocator();
try tempora.tzdb.init_cache(gpa.allocator());
defer tempora.tzdb.deinit_cache();
const args = try std.process.argsAlloc(allocator);
defer std.process.argsFree(allocator, args);
var writer = std.io.getStdOut().writer();
if (args.len < 2) {
std.log.err("Timezone name is required", .{});
return 1;
}
const show_transitions = args.len >= 3 and std.mem.eql(u8, "--transitions", args[2]);
if (try tempora.tzdb.timezone(args[1])) |tz| {
if (tz.posix_tz) |ptz| {
try writer.print("POSIX timezone string: {}\n", .{ ptz });
}
const now = std.time.timestamp();
const zi = tz.zone_info(now);
try writer.print("Current Time: {YYYY-MM-DD HH;mm;ss z} offset={}s dst={} source={s}\n", .{
tempora.Date_Time.With_Offset.from_timestamp_s(now, null).in_timezone(tz),
zi.offset,
zi.is_dst,
@tagName(zi.source),
});
if (zi.end_ts) |end_ts| {
const end_dt = tempora.Date_Time.With_Offset.from_timestamp_s(end_ts, null).in_timezone(tz);
if (zi.begin_ts) |begin_ts| {
const begin_dt = tempora.Date_Time.With_Offset.from_timestamp_s(begin_ts, null).in_timezone(tz);
if (zi.is_dst) {
try writer.print(" DST began: {YYYY-MM-DD HH;mm;ss z}\n", .{ begin_dt });
try writer.print(" DST ends: {YYYY-MM-DD HH;mm;ss z}\n", .{ end_dt });
} else {
try writer.print(" DST ended: {YYYY-MM-DD HH;mm;ss z}\n", .{ begin_dt });
try writer.print(" DST begins: {YYYY-MM-DD HH;mm;ss z}\n", .{ end_dt });
}
} else if (zi.is_dst) {
try writer.print(" DST ends: {YYYY-MM-DD HH;mm;ss z}\n", .{ end_dt });
} else {
try writer.print(" DST begins: {YYYY-MM-DD HH;mm;ss z}\n", .{ end_dt });
}
} else if (zi.begin_ts) |begin_ts| {
const begin_dt = tempora.Date_Time.With_Offset.from_timestamp_s(begin_ts, null).in_timezone(tz);
if (zi.is_dst) {
try writer.print(" This timezone has permanent daylight time\n", .{});
} else {
try writer.print(" This timezone has permanent standard time\n", .{});
}
try writer.print(" The current time rules for this zone began on {YYYY-MM-DD HH;mm;ss z}\n", .{ begin_dt });
} else {
if (zi.is_dst) {
try writer.print(" This timezone has permanent daylight time\n", .{});
} else {
try writer.print(" This timezone has permanent standard time\n", .{});
}
}
if (show_transitions) {
try writer.print("{} transition times\n", .{tz.transitions.len});
for (0.., tz.transitions.items(.ts), tz.transitions.items(.zone_index)) |n, ts, index| {
const info = tz.zones[index];
try writer.print(" [{}]: ts={}s offset={}s dst={} designation={s} source={s}\n", .{
n,
ts,
info.offset,
info.is_dst,
info.designation,
@tagName(info.source),
});
}
}
return 0;
} else {
try writer.print("No timezone found with name {s}. Valid IDs are:\n", .{ args[1] });
for (tempora.tzdb.ids) |id| {
try writer.print(" {s}\n", .{ id });
}
return 1;
}
}
const tempora = @import("tempora");
const std = @import("std");
|
0 | repos/tempora | repos/tempora/tools/update_tzdb.zig |
pub fn main() !void {
var arena = std.heap.ArenaAllocator.init(std.heap.page_allocator);
defer arena.deinit();
const aa = arena.allocator();
var gpa_alloc: std.heap.GeneralPurposeAllocator(.{}) = .{};
defer _ = gpa_alloc.deinit();
const gpa = gpa_alloc.allocator();
const Sha256 = std.crypto.hash.sha2.Sha256;
const Digest = [Sha256.digest_length]u8;
var id_to_digest = std.StringHashMap(Digest).init(gpa);
defer id_to_digest.deinit();
var digest_to_data = std.AutoHashMap(Digest, []const u8).init(gpa);
defer digest_to_data.deinit();
var designation_to_offset = std.StringHashMap(i32).init(gpa);
defer designation_to_offset.deinit();
const designation_override_kvs = .{
.{ "LMT", null }, // "Local mean time" - not a standard designation
.{ "CMT", null },
.{ "PMT", null },
.{ "SMT", null },
.{ "BMT", null },
.{ "RMT", null },
.{ "NWT", null },
.{ "KMT", null },
.{ "KDT", null },
.{ "APT", null },
.{ "AWT", null },
.{ "IMT", null },
.{ "HST", -10 * 60 * 60 }, // Hawaii-Aleutian Standard Time
.{ "HDT", -9 * 60 * 60 }, // Hawaii-Aleutian Standard Time
.{ "PST", -8 * 60 * 60 }, // Pacific Standard Time
.{ "PDT", -7 * 60 * 60 }, // Pacific Daylight Time
.{ "MST", -7 * 60 * 60 }, // Mountain Standard Time
.{ "MDT", -6 * 60 * 60 }, // Mountain Daylight Time
.{ "CST", -6 * 60 * 60 }, // Central Standard Time
.{ "CDT", -5 * 60 * 60 }, // Central Daylight Time
.{ "EST", -5 * 60 * 60 }, // Eastern Standard Time
.{ "EDT", -4 * 60 * 60 }, // Eastern Daylight Time
.{ "AST", -4 * 60 * 60 }, // Atlantic Standard Time
.{ "ADT", -3 * 60 * 60 }, // Atlantic Daylight Time
.{ "NST", -(3 * 60 + 30) * 60 }, // Newfoundland Standard Time
.{ "NDT", -(2 * 60 + 30) * 60 }, // Newfoundland Daylight Time
.{ "BST", 1 * 60 * 60 }, // British Summer Time
.{ "SAST", 2 * 60 * 60 }, // South African Standard Time
.{ "MSK", 3 * 60 * 60 }, // Moscow Time
.{ "AMT", 4 * 60 * 60 }, // Armenia Time
.{ "HMT", 5 * 60 * 60 }, // Heard and McDonald Islands Time
.{ "TMT", 5 * 60 * 60 }, // Turkmenistan Time
.{ "IST", (5 * 60 + 30) * 60 }, // Indian Standard Time
.{ "NPT", (5 * 60 + 45) * 60 }, // Nepal Time
.{ "MMT", (6 * 60 + 30) * 60 }, // Myanmar Standard Time
.{ "KST", 9 * 60 * 60 }, // Korea Standard Time
.{ "ACST", (9 * 60 + 30) * 60 }, // Australian Central Standard Time
.{ "NZST", 12 * 60 * 60 }, // New Zealand Standard Time
};
const designation_overrides = if (comptime @import("builtin").zig_version.minor == 12)
std.ComptimeStringMap(?i32, designation_override_kvs) // TODO remove zig 0.12 support when 0.14 is released
else
std.StaticStringMap(?i32).initComptime(designation_override_kvs);
const zoneinfo = try std.fs.cwd().openDir("/usr/share/zoneinfo", .{ .iterate = true });
var walker = try zoneinfo.walk(gpa);
defer walker.deinit();
while (try walker.next()) |entry| {
if (entry.kind == .file or entry.kind == .sym_link) {
if (std.mem.startsWith(u8, entry.path, "right/")) continue;
if (std.mem.startsWith(u8, entry.path, "posix/")) continue;
if (std.fs.path.extension(entry.path).len > 0) continue;
if (std.mem.eql(u8, entry.path, "localtime")) continue;
if (std.mem.eql(u8, entry.path, "leapseconds")) continue;
//std.log.info("{s}", .{ entry.path });
const id = try aa.dupe(u8, entry.path);
const stat = try zoneinfo.statFile(id);
const data = try zoneinfo.readFileAllocOptions(aa, id, 1_000_000, stat.size, 1, null);
var data_stream = std.io.fixedBufferStream(data);
var compressed = try std.ArrayList(u8).initCapacity(aa, (stat.size / 2) * 3);
try std.compress.zlib.compress(data_stream.reader(), compressed.writer(), .{ .level = .best });
const compressed_data = compressed.items;
var digest: Digest = undefined;
Sha256.hash(compressed_data, &digest, .{});
const tz = try tempora.tzdb.parse_tzif.parse_memory(aa, data);
for (tz.zones) |zi| {
if (zi.designation.len == 0) continue;
if (std.mem.startsWith(u8, zi.designation, "+")) continue;
if (std.mem.startsWith(u8, zi.designation, "-")) continue;
if (designation_overrides.get(zi.designation)) |_| continue;
const result = try designation_to_offset.getOrPut(zi.designation);
if (result.found_existing) {
if (result.value_ptr.* != zi.offset) {
std.log.warn("Multiple offsets found for designation {s}: first saw {}, now {}", .{
zi.designation,
result.value_ptr.*,
zi.offset,
});
}
} else {
result.key_ptr.* = try aa.dupe(u8, zi.designation);
result.value_ptr.* = zi.offset;
}
}
try id_to_digest.put(id, digest);
try digest_to_data.put(digest, compressed_data);
}
}
inline for (designation_override_kvs) |entry| {
if (@typeInfo(@TypeOf(entry[1])) != .Null) {
try designation_to_offset.putNoClobber(entry[0], entry[1]);
}
}
for (1..13) |neg_utc_offset| {
var buf1: [32]u8 = undefined;
var buf2: [32]u8 = undefined;
const src_id = try std.fmt.bufPrint(&buf1, "Etc/GMT+{}", .{ neg_utc_offset });
const dest_id = try std.fmt.bufPrint(&buf2, "GMT-{}", .{ neg_utc_offset });
const duped = try aa.dupe(u8, dest_id);
try id_to_digest.put(duped, id_to_digest.get(src_id).?);
}
for (1..15) |utc_offset| {
var buf1: [32]u8 = undefined;
var buf2: [32]u8 = undefined;
const src_id = try std.fmt.bufPrint(&buf1, "Etc/GMT-{}", .{ utc_offset });
const dest_id = try std.fmt.bufPrint(&buf2, "GMT+{}", .{ utc_offset });
const duped = try aa.dupe(u8, dest_id);
try id_to_digest.put(duped, id_to_digest.get(src_id).?);
}
const sorted_designations: [][]const u8 = try aa.alloc([]const u8, designation_to_offset.count());
{
var iter = designation_to_offset.keyIterator();
var i: usize = 0;
while (iter.next()) |designation| {
sorted_designations[i] = designation.*;
i += 1;
}
std.sort.block([]const u8, sorted_designations, {}, sort_string);
}
const sorted_ids: [][]const u8 = try aa.alloc([]const u8, id_to_digest.count());
{
var iter = id_to_digest.keyIterator();
var i: usize = 0;
while (iter.next()) |id| {
sorted_ids[i] = id.*;
i += 1;
}
std.sort.block([]const u8, sorted_ids, {}, sort_string);
}
const data_dir = try std.fs.cwd().openDir("src/tzdb/data", .{ .iterate = true });
var data_walker = try data_dir.walk(gpa);
defer data_walker.deinit();
while (try data_walker.next()) |entry| {
if (entry.kind == .file) {
try data_dir.deleteFile(entry.path);
}
}
var file = try std.fs.cwd().createFile("src/tzdb/data.zig", .{});
defer file.close();
const writer = file.writer();
try writer.writeAll(
\\pub const ids = [_][]const u8 {
\\
);
var col: usize = 0;
for (sorted_ids) |id| {
if (col > 0) {
try writer.writeAll(", ");
col += 2;
}
if (col + id.len + 2 >= 110) {
try writer.writeAll("\n ");
col = 4;
}
try writer.print("\"{}\"", .{ std.zig.fmtEscapes(id) });
col += id.len + 2;
}
try writer.writeAll(
\\
\\};
\\
\\pub fn designations(allocator: std.mem.Allocator) !std.StringArrayHashMap(i32) {
\\ var m = std.StringArrayHashMap(i32).init(allocator);
\\ errdefer m.deinit();
\\ try m.ensureUnusedCapacity(
);
try writer.print("{}", .{ sorted_designations.len });
try writer.writeAll(
\\);
\\
);
for (sorted_designations) |designation| {
try writer.print(" try m.put(\"{}\", {});\n", .{
std.zig.fmtEscapes(designation),
designation_to_offset.get(designation).?,
});
}
try writer.writeAll(
\\ return m;
\\}
\\
\\pub fn db(allocator: std.mem.Allocator) !std.StringArrayHashMap([]const u8) {
\\ var m = std.StringArrayHashMap([]const u8).init(allocator);
\\ errdefer m.deinit();
\\ try m.ensureUnusedCapacity(
);
try writer.print("{}", .{ sorted_ids.len });
try writer.writeAll(
\\);
\\
);
for (sorted_ids) |id| {
const digest = id_to_digest.get(id).?;
var hex_buf: [digest.len*2]u8 = undefined;
const hex = try std.fmt.bufPrint(&hex_buf, "{s}", .{ std.fmt.fmtSliceHexLower(&digest) });
try writer.print(" try m.put(\"{}\", data._{s});\n", .{
std.zig.fmtEscapes(id),
hex,
});
}
try writer.writeAll(
\\ return m;
\\}
\\
\\const data = struct {
\\
);
var total_size: usize = 0;
{
var iter = digest_to_data.iterator();
while (iter.next()) |entry| {
const digest = entry.key_ptr.*;
const compressed_data = entry.value_ptr.*;
var hex_buf: [digest.len*2]u8 = undefined;
const hex = try std.fmt.bufPrint(&hex_buf, "{s}", .{ std.fmt.fmtSliceHexLower(&digest) });
var dir = try data_dir.makeOpenPath(hex[0..1], .{});
defer dir.close();
const writeFile = if (comptime @import("builtin").zig_version.minor == 12)
std.fs.Dir.writeFile2 // TODO remove zig 0.12 support when 0.14 is released
else
std.fs.Dir.writeFile;
try writeFile(dir, .{
.sub_path = hex,
.data = compressed_data,
});
total_size += compressed_data.len;
try writer.print(" pub const _{s} = @embedFile(\"data/{s}/{s}\");\n", .{
hex,
hex[0..1],
hex,
});
}
}
try writer.writeAll(
\\};
\\
\\const std = @import("std");
\\
);
std.log.info("Found {} designations, {} unique files ({} bytes compressed) and {} ids", .{
designation_to_offset.count(),
digest_to_data.count(),
total_size,
id_to_digest.count(),
});
}
fn sort_string(_: void, left: []const u8, right: []const u8) bool {
return std.mem.lessThan(u8, left, right);
}
const tempora = @import("tempora");
const std = @import("std");
|
0 | repos | repos/update-zig/README.md | # Zig Updater
Fetch the latest Zig master branch build easily with one command. `update-zig` will automatically parse https://ziglang.org/download/index.json, download the latest `x86_64-linux` archive, and unpack the contents to `$HOME/.zig`.
You can run the command again and nothing will happen if a new build is not available so feel free to spam it however you like.
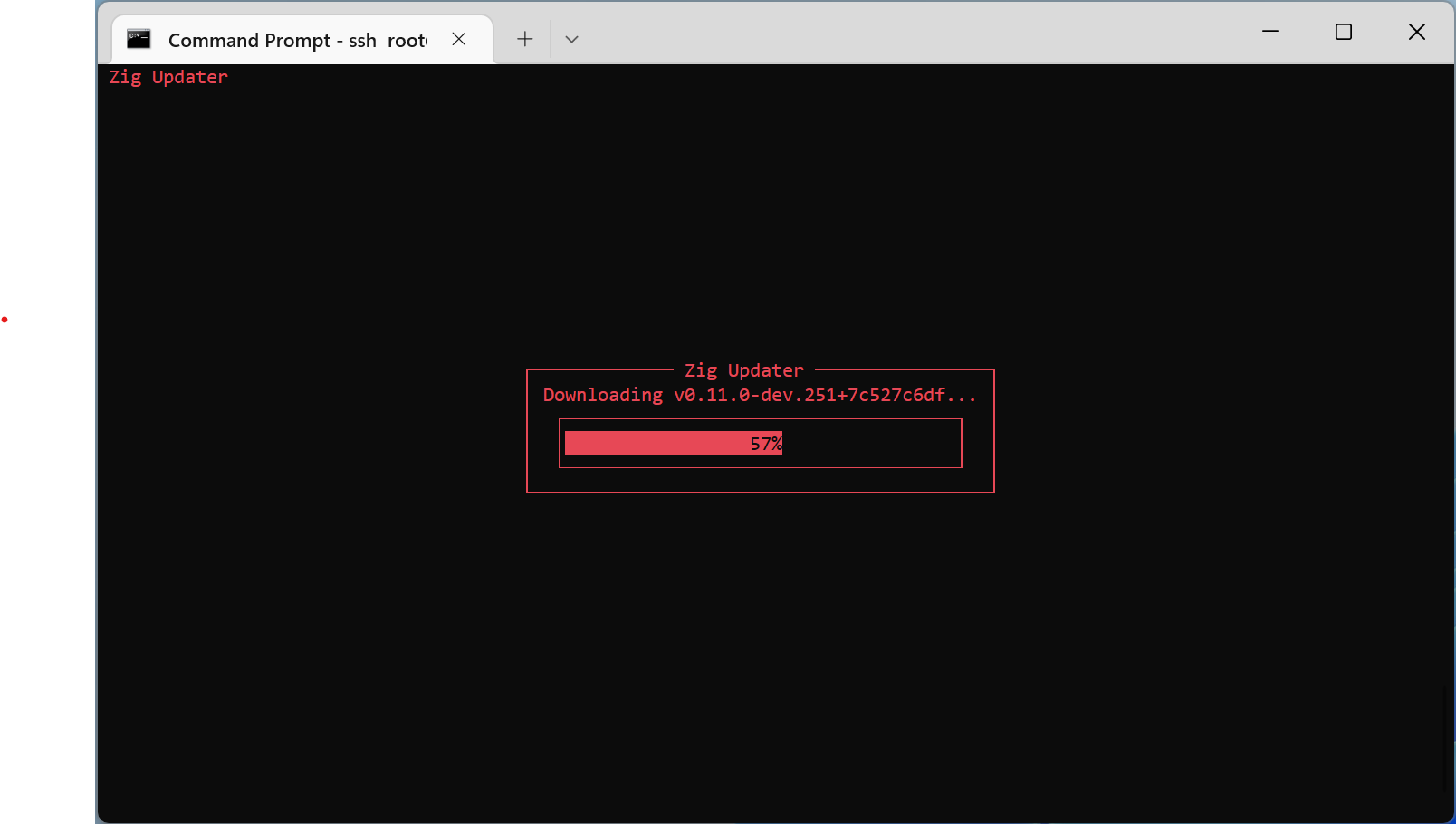
Note: `dialog` colors may be different on your machine.
## Running
`update-zig`
## Prerequisites
Make sure the following packages are installed if any issues arise on your system:
- `gawk`
- `dialog`
- `jq`
- `pv`
- `wget`
- `xz-utils`
## Installing
```
git clone https://github.com/snail23/update-zig $HOME/.zig
chmod +x $HOME/.zig/update-zig
````
Optionally, run `update-zig` on login as well as add `$HOME/.zig` to `PATH`:
```
echo 'PATH="$PATH:$HOME/.zig"' >> $HOME/.profile
echo update-zig >> $HOME/.profile
```
# License
```
MIT License
Copyright (c) 2023 Snail
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
```
|
0 | repos | repos/zCOM/README.md | # zCOM Protocol Stacks
The zCOM library is there to provide a composable stack of protocols, down from osi layer 1 (e.g. [ethernet frames](https://en.wikipedia.org/wiki/Ethernet_frame), [IEEE_802.11](https://en.wikipedia.org/wiki/IEEE_802.11)) up to osi layer 7 ([DNS](https://en.wikipedia.org/wiki/Domain_Name_System), [DHCP](https://en.wikipedia.org/wiki/Dynamic_Host_Configuration_Protocol), [Tiny TP](https://en.wikipedia.org/wiki/Infrared_Data_Association#Tiny_TP), ...).
The library is designed in a way that you can stack each protocol onto a compatible protocol, for example serving Tiny TP over UDP instead of [IrLMP](https://en.wikipedia.org/wiki/Infrared_Data_Association#IrLMP) or use [PPP](https://en.wikipedia.org/wiki/Point-to-Point_Protocol) over a serial line.
## Goals
- Provide a general purpose implementation of a lot of link protocols
- Protocol implementations should be configurable so they are suitable for both high end machines (desktop, server) and embedded devices
- This means that a protocol might require `comptime` configuration to restrict the number of allowed connections (for example, allow only up to 3 TCP connections to save RAM)
- Allow the use of _any_ [physical layer](https://en.wikipedia.org/wiki/Physical_layer)
- Provide APIs that allows the use of `async`, but doesn't enforce it
- Make the APIs be usable for byte-by-byte inputs and don't require any specified packet size. Feeding a recording of 1 MB of data should work the same way as feeding a single byte.
## Project Status
The whole project is just in planning phase, no concrete implementation done yet.
## Supported Protocols
Each protocol with a tick is at least in a somewhat usable state
### Layer 1
- [ ] [Ethernet](https://en.wikipedia.org/wiki/Ethernet_frame)
- [ ] [IEEE802.11](https://en.wikipedia.org/wiki/802.11_Frame_Types) (WLAN)
### Layer 2
- [ ] [ARP](https://en.wikipedia.org/wiki/Address_Resolution_Protocol)
- [ ] [PPP](https://en.wikipedia.org/wiki/Point-to-Point_Protocol)
- [ ] [IrLAP](https://en.wikipedia.org/wiki/Infrared_Data_Association#IrLAP)
### Layer 3
- [ ] [IPv4](https://en.wikipedia.org/wiki/IPv4)
- [ ] [IPv6](https://en.wikipedia.org/wiki/IPv6)
- [ ] [ICMP](https://en.wikipedia.org/wiki/Internet_Control_Message_Protocol)
- [ ] [IGMP](https://en.wikipedia.org/wiki/Internet_Group_Management_Protocol)
- [ ] [IrLMP](https://en.wikipedia.org/wiki/Infrared_Data_Association#IrLMP)
- [ ] [ESP-NOW](https://docs.espressif.com/projects/esp-idf/en/latest/esp32/api-reference/network/esp_now.html)
### Layer 4
- [ ] [TCP](https://en.wikipedia.org/wiki/Transmission_Control_Protocol)
- [ ] [UDP](https://en.wikipedia.org/wiki/User_Datagram_Protocol)
- [ ] [Tiny TP](https://en.wikipedia.org/wiki/Infrared_Data_Association#Tiny_TP)
### Layer 7
- [ ] [DHCP](https://en.wikipedia.org/wiki/Dynamic_Host_Configuration_Protocol)
- [ ] [DNS](https://en.wikipedia.org/wiki/Domain_Name_System)
- [ ] [mDNS](https://en.wikipedia.org/wiki/Multicast_DNS)
- [ ] [NTP](https://en.wikipedia.org/wiki/Network_Time_Protocol)
- [ ] [PTP](https://en.wikipedia.org/wiki/Precision_Time_Protocol)
## Documents / Blogs / Further Reading
- https://www.saminiir.com/lets-code-tcp-ip-stack-1-ethernet-arp/
## Dictionary
### Message
A message is a sequence of octets a user wants to transmit/receive. Messages are application specific and don't contain any protocol headers.
**Examples:**
- A chat message formatted in JSON
### Buffer
A buffer is a sequence of octets that are stored for temporary/implementation reasons.
### Packet
A packet is a delimited sequence of octets with known (but maybe dynamic) length. Packets have a protocol defined format and usually contain protocol headers.
**Examples:**
- UDP sockets transmit/receive packets called datagrams.
- IEEE 802.11 transmits/receives packets called frames.
### Stream
A stream is a unlimited sequence of octets without a well defined length.
**Examples:**
- TCP sockets transmit/receive streams.
- Serial ports transmit/receive streams.
|
0 | repos | repos/zCOM/build.zig | const Builder = @import("std").build.Builder;
pub fn build(b: *Builder) void {
const mode = b.standardReleaseOptions();
var main_tests = b.addTest("src/main.zig");
main_tests.setBuildMode(mode);
const test_step = b.step("test", "Run library tests");
test_step.dependOn(&main_tests.step);
}
|
0 | repos/zCOM | repos/zCOM/src/main.zig | const std = @import("std");
const testing = std.testing;
export fn add(a: i32, b: i32) i32 {
return a + b;
}
test "basic add functionality" {
testing.expect(add(3, 7) == 10);
}
|
0 | repos | repos/log.zig/README.md | # *log.zig*
a cross-platform, thread-safe logging library for [zig](https://ziglang.org/).
## usage
```zig
const io = @import("std").io;
const log = @import("log");
const Logger = log.Logger;
pub fn main() !void {
var logger = Logger.new(try io.getStdOut(), true);
logger.setBright(false);
logger.logWarn("crime is afoot");
logger.setColor(false);
logger.logInfo("crime has been stopped");
}
```
## supports
- window's console
- any terminal supporting ansi escape codes
|
0 | repos | repos/log.zig/build.zig | const Builder = @import("std").build.Builder;
pub fn build(b: *Builder) void {
const mode = b.standardReleaseOptions();
const lib = b.addStaticLibrary("log.zig", "src/index.zig");
lib.setBuildMode(mode);
var main_tests = b.addTest("src/index.zig");
main_tests.setBuildMode(mode);
const test_step = b.step("test", "run library tests");
test_step.dependOn(&main_tests.step);
b.default_step.dependOn(&lib.step);
b.installArtifact(lib);
}
|
0 | repos/log.zig | repos/log.zig/src/index.zig | // Copyright (c) 2018 emekoi
//
// This library is free software; you can redistribute it and/or modify it
// under the terms of the MIT license. See LICENSE for details.
//
const std = @import("std");
const builtin = @import("builtin");
const io = std.io;
const os = std.os;
const fs = std.fs;
const windows = os.windows;
const posix = os.posix;
const Mutex = std.Mutex;
const TtyColor = enum {
Red,
Green,
Yellow,
Magenta,
Cyan,
Blue,
Reset,
};
fn Protected(comptime T: type) type {
return struct {
const Self = @This();
mutex: Mutex,
private_data: T,
const HeldMutex = struct {
value: *T,
held: Mutex.Held,
pub fn release(self: HeldMutex) void {
self.held.release();
}
};
pub fn init(data: T) Self {
return Self{
.mutex = Mutex.init(),
.private_data = data,
};
}
pub fn acquire(self: *Self) HeldMutex {
return HeldMutex{
.held = self.mutex.acquire(),
.value = &self.private_data,
};
}
};
}
const FOREGROUND_BLUE = 1;
const FOREGROUND_GREEN = 2;
const FOREGROUND_AQUA = 3;
const FOREGROUND_RED = 4;
const FOREGROUND_MAGENTA = 5;
const FOREGROUND_YELLOW = 6;
/// different levels of logging
pub const Level = enum {
const Self = @This();
Trace,
Debug,
Info,
Warn,
Error,
Fatal,
fn toString(self: Self) []const u8 {
return switch (self) {
Self.Trace => "TRACE",
Self.Debug => "DEBUG",
Self.Info => "INFO",
Self.Warn => "WARN",
Self.Error => "ERROR",
Self.Fatal => "FATAL",
};
}
fn color(self: Self) TtyColor {
return switch (self) {
Self.Trace => TtyColor.Blue,
Self.Debug => TtyColor.Cyan,
Self.Info => TtyColor.Green,
Self.Warn => TtyColor.Yellow,
Self.Error => TtyColor.Red,
Self.Fatal => TtyColor.Magenta,
};
}
};
/// a simple thread-safe logger
pub const Logger = struct {
const Self = @This();
const ProtectedOutStream = Protected(*fs.File.OutStream);
file: fs.File,
file_stream: fs.File.OutStream,
out_stream: ?ProtectedOutStream,
default_attrs: windows.WORD,
level: Protected(Level),
quiet: Protected(bool),
use_color: bool,
use_bright: bool,
/// create `Logger`.
pub fn new(file: fs.File, use_color: bool) Self {
// TODO handle error
var info: windows.CONSOLE_SCREEN_BUFFER_INFO = undefined;
if (std.Target.current.os.tag == .windows) {
_ = windows.kernel32.GetConsoleScreenBufferInfo(file.handle, &info);
}
return Self{
.file = file,
.file_stream = file.outStream(),
.out_stream = undefined,
.level = Protected(Level).init(Level.Trace),
.quiet = Protected(bool).init(false),
.default_attrs = info.wAttributes,
.use_color = use_color,
.use_bright = true,
};
}
// can't be done in `Logger.new` because of no copy-elision
fn getOutStream(self: *Self) ProtectedOutStream {
if (self.out_stream) |out_stream| {
return out_stream;
} else {
self.out_stream = ProtectedOutStream.init(&self.file_stream);
return self.out_stream.?;
}
}
fn setTtyColorWindows(self: *Self, color: TtyColor) void {
// TODO handle errors
const bright = if (self.use_bright) windows.FOREGROUND_INTENSITY else u16(0);
_ = windows.SetConsoleTextAttribute(self.file.handle, switch (color) {
TtyColor.Red => FOREGROUND_RED | bright,
TtyColor.Green => FOREGROUND_GREEN | bright,
TtyColor.Yellow => FOREGROUND_YELLOW | bright,
TtyColor.Magenta => FOREGROUND_MAGENTA | bright,
TtyColor.Cyan => FOREGROUND_AQUA | bright,
TtyColor.Blue => FOREGROUND_BLUE | bright,
TtyColor.Reset => self.default_attrs,
});
}
fn setTtyColor(self: *Self, color: TtyColor) !void {
if (std.Target.current.os.tag == .windows and !os.supportsAnsiEscapeCodes(self.file.handle)) {
self.setTtyColorWindows(color);
} else {
var out = self.getOutStream();
var out_held = out.acquire();
defer out_held.release();
const bright = if (self.use_bright) "\x1b[1m" else "";
return switch (color) {
TtyColor.Red => out_held.value.*.print("{}\x1b[31m", .{bright}),
TtyColor.Green => out_held.value.*.print("{}\x1b[32m", .{bright}),
TtyColor.Yellow => out_held.value.*.print("{}\x1b[33m", .{bright}),
TtyColor.Magenta => out_held.value.*.print("{}\x1b[35m", .{bright}),
TtyColor.Cyan => out_held.value.*.print("{}\x1b[36m", .{bright}),
TtyColor.Blue => out_held.value.*.print("{}\x1b[34m", .{bright}),
TtyColor.Reset => blk: {
_ = try out_held.value.*.write("\x1b[0m");
},
};
}
}
/// enable or disable color.
pub fn setColor(self: *Self, use_color: bool) void {
self.use_color = use_color;
}
/// enable or disable bright versions of the colors.
pub fn setBright(self: *Self, use_bright: bool) void {
self.use_bright = use_bright;
}
/// Set the minimum logging level.
pub fn setLevel(self: *Self, level: Level) void {
var held = self.level.acquire();
defer held.release();
held.value.* = level;
}
/// Outputs to stderr if true. true by default.
pub fn setQuiet(self: *Self, quiet: bool) void {
var held = self.quiet.acquire();
defer held.release();
held.value.* = quiet;
}
/// General purpose log function.
pub fn log(self: *Self, level: Level, comptime fmt: []const u8, args: var) !void {
const level_held = self.level.acquire();
defer level_held.release();
if (@enumToInt(level) < @enumToInt(level_held.value.*)) {
return;
}
var out = self.getOutStream();
var out_held = out.acquire();
defer out_held.release();
var out_stream = out_held.value.*;
const quiet_held = self.quiet.acquire();
defer quiet_held.release();
// TODO get filename and number
// TODO get time as a string
// time includes the year
if (!quiet_held.value.*) {
if (self.use_color and self.file.isTty()) {
try out_stream.print("{} ", .{std.time.timestamp()});
try self.setTtyColor(level.color());
try out_stream.print("[{}]", .{level.toString()});
try self.setTtyColor(TtyColor.Reset);
try out_stream.print(": ", .{});
// out_stream.print("\x1b[90m{}:{}:", filename, line);
// self.resetTtyColor();
} else {
try out_stream.print("{} [{s}]: ", .{ std.time.timestamp(), level.toString() });
}
if (args.len > 0) {
out_stream.print(fmt, args) catch return;
} else {
_ = out_stream.write(fmt) catch return;
}
_ = out_stream.write("\n") catch return;
}
}
/// log at level `Level.Trace`.
pub fn logTrace(self: *Self, comptime fmt: []const u8, args: var) void {
self.log(Level.Trace, fmt, args) catch return;
}
/// log at level `Level.Debug`.
pub fn logDebug(self: *Self, comptime fmt: []const u8, args: var) void {
self.log(Level.Debug, fmt, args) catch return;
}
/// log at level `Level.Info`.
pub fn logInfo(self: *Self, comptime fmt: []const u8, args: var) void {
self.log(Level.Info, fmt, args) catch return;
}
/// log at level `Level.Warn`.
pub fn logWarn(self: *Self, comptime fmt: []const u8, args: var) void {
self.log(Level.Warn, fmt, args) catch return;
}
/// log at level `Level.Error`.
pub fn logError(self: *Self, comptime fmt: []const u8, args: var) void {
self.log(Level.Error, fmt, args) catch return;
}
/// log at level `Level.Fatal`.
pub fn logFatal(self: *Self, comptime fmt: []const u8, args: var) void {
self.log(Level.Fatal, fmt, args) catch return;
}
};
test "log_with_color" {
var logger = Logger.new(io.getStdOut(), true);
std.debug.warn("\n", .{});
logger.logTrace("hi", .{});
logger.logDebug("hey", .{});
logger.logInfo("hello", .{});
const world = "world";
const num = 12345;
logger.logInfo("hello {} {}", .{ world, num });
logger.logWarn("greetings", .{});
logger.logError("salutations", .{});
logger.logFatal("goodbye", .{});
}
fn worker(logger: *Logger) void {
logger.logTrace("hi", .{});
std.time.sleep(10000);
logger.logDebug("hey", .{});
std.time.sleep(10);
logger.logInfo("hello", .{});
std.time.sleep(100);
logger.logWarn("greetings", .{});
std.time.sleep(1000);
logger.logError("salutations", .{});
std.time.sleep(10000);
logger.logFatal("goodbye", .{});
std.time.sleep(1000000000);
}
test "log_thread_safe" {
var logger = Logger.new(io.getStdOut(), true);
std.debug.warn("\n", .{});
const thread_count = 5;
var threads: [thread_count]*std.Thread = undefined;
for (threads) |*t| {
t.* = try std.Thread.spawn(&logger, worker);
}
for (threads) |t| {
t.wait();
}
}
|
0 | repos | repos/computils/exports.zig | const std = @import("std");
pub const ComptimeArrayList = @import("src/comptime_array_list.zig").ComptimeArrayList;
comptime {
std.meta.refAllDecls(@This());
}
|
0 | repos | repos/computils/README.md | # computils
Zig utilities for all your comptime needs.
## Utilities
### `ComptimeArrayList`
**Example:**
```zig
// Simply replace your ArrayList(T).init(allocator) with ComptimeArrayList(T).init()
// and use `comptime` on all operations
comptime {
var list = ComptimeArrayList(u8).init();
list.append('a');
}
```
## License
MIT
|
0 | repos | repos/computils/LICENSE.md | MIT License
Copyright (c) 2020 Auguste Rame
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
|
0 | repos/computils | repos/computils/src/comptime_array_list.zig | const std = @import("std");
const testing = std.testing;
/// All subfunctions have to be run as `comptime` or in `comptime` blocks.
pub fn ComptimeArrayList(comptime T: type) type {
return struct {
const Self = @This();
items: []T,
pub fn init() Self {
comptime var initial = [_]T{};
return Self{
.items = &initial
};
}
pub fn append(self: *Self, comptime item: T) void {
var new_items: [self.items.len + 1]T = undefined;
std.mem.copy(T, &new_items, self.items);
new_items[self.items.len] = item;
self.items = &new_items;
}
pub fn appendSlice(self: *Self, comptime items: []T) void {
var new_items: [self.items.len + items.len]T = undefined;
std.mem.copy(T, &new_items, self.items);
var i: usize = 0;
while (i < items.len) : (i += 1)
new_items[self.items.len + i] = items[i];
self.items = &new_items;
}
pub fn deinit(self: *Self) void {
var new_items: [0]T = undefined;
self.items = &new_items;
}
pub fn toOwnedSlice(self: *Self) []T {
defer self.deinit();
return self.items;
}
pub fn orderedRemove(self: *Self, comptime index: usize) void {
var new_items: [self.items.len - 1]T = undefined;
var i: usize = 0;
while (i < self.items.len) : (i += 1) {
if (i == index) {
continue;
} else if (i > index) {
new_items[i - 1] = self.items[i];
} else {
new_items[i] = self.items[i];
}
}
self.items = &new_items;
}
/// Adjusts the list's length to `new_len`.
/// On "upsizing", elements that were previously "downsized" and left
/// out of the resize will become undefined
pub fn resize(self: *Self, comptime new_len: usize) void {
if (new_len > self.items.len) {
var new_items: [new_len]T = undefined;
std.mem.copy(u8, &new_items, self.items);
self.items = &new_items;
} else {
self.items.len = new_len;
}
}
/// Shrinks the list's length to `new_len`.
/// This will make elements "left out" of the resize "disappear".
pub fn shrink(self: *Self, comptime new_len: usize) void {
std.debug.assert(new_len <= self.items.len);
var new_items: [new_len]T = undefined;
var i: usize = 0;
while (i < new_len) : (i += 1) {
new_items[i] = self.items[i];
}
self.items = &new_items;
}
/// Caller must free memory.
pub fn toRuntime(self: *Self, allocator: *std.mem.Allocator) !std.ArrayList(T) {
var arr = std.ArrayList(T).init(allocator);
try arr.appendSlice(self.items);
return arr;
}
};
}
test "ComptimeArrayList.append / ComptimeArrayList.appendSlice" {
comptime {
var list = ComptimeArrayList(u8).init();
list.append('a');
list.append('b');
var arr = [_]u8{'c', 'd'};
list.appendSlice(&arr);
var expected = [_]u8{'a', 'b', 'c', 'd'};
var expected_empty = [_]u8{};
std.testing.expectEqualSlices(u8, &expected, list.items);
std.testing.expectEqualSlices(u8, &expected, list.toOwnedSlice());
std.testing.expectEqual(list.items.len, 0);
std.testing.expectEqualSlices(u8, &expected_empty, list.items);
}
}
test "ComptimeArrayList.remove" {
comptime {
var list = ComptimeArrayList(u8).init();
list.append('a');
list.append('b');
list.append('c');
list.append('d');
list.append('e');
list.orderedRemove(1);
list.orderedRemove(3);
list.orderedRemove(1);
var expected = [_]u8{'a', 'd'};
std.testing.expectEqualSlices(u8, &expected, list.toOwnedSlice());
}
}
test "ComptimeArrayList.resize" {
comptime {
var list = ComptimeArrayList(u8).init();
list.append('a');
list.append('b');
list.append('c');
list.append('d');
list.resize(2);
std.testing.expectEqual(2, list.items.len);
}
}
test "ComptimeArrayList.shrink" {
comptime {
var list = ComptimeArrayList(u8).init();
list.append('a');
list.append('b');
list.append('c');
list.append('d');
list.shrink(2);
std.testing.expectEqual(2, list.items.len);
}
}
test "ComptimeArrayList.toRuntime" {
comptime var list = ComptimeArrayList(u8).init();
comptime {
list.append('a');
list.append('b');
list.append('c');
list.append('d');
}
var array_list = try list.toRuntime(std.testing.allocator);
defer array_list.deinit();
var expected = [_]u8{'a', 'b', 'c', 'd'};
std.testing.expectEqualSlices(u8, &expected, array_list.items);
}
|
0 | repos | repos/image-viewer/x11common.zig | const std = @import("std");
const x = @import("x");
const common = @This();
pub const SocketReader = std.io.Reader(std.os.socket_t, std.os.RecvFromError, readSocket);
pub fn send(sock: std.os.socket_t, data: []const u8) !void {
const sent = try x.writeSock(sock, data, 0);
if (sent != data.len) {
std.log.err("send {} only sent {}\n", .{data.len, sent});
return error.DidNotSendAllData;
}
}
pub const ConnectResult = struct {
sock: std.os.socket_t,
setup: x.ConnectSetup,
pub fn reader(self: ConnectResult) SocketReader {
return .{ .context = self.sock };
}
pub fn send(self: ConnectResult, data: []const u8) !void {
try common.send(self.sock, data);
}
};
pub fn connect(allocator: std.mem.Allocator) !ConnectResult {
const display = x.getDisplay();
const parsed_display = x.parseDisplay(display) catch |err| {
std.log.err("invalid display '{s}': {s}", .{display, @errorName(err)});
std.os.exit(0xff);
};
const sock = x.connect(display, parsed_display) catch |err| {
std.log.err("failed to connect to display '{s}': {s}", .{display, @errorName(err)});
std.os.exit(0xff);
};
errdefer x.disconnect(sock);
{
const len = comptime x.connect_setup.getLen(0, 0);
var msg: [len]u8 = undefined;
x.connect_setup.serialize(&msg, 11, 0, .{ .ptr = undefined, .len = 0 }, .{ .ptr = undefined, .len = 0 });
try send(sock, &msg);
}
const reader = SocketReader { .context = sock };
const connect_setup_header = try x.readConnectSetupHeader(reader, .{});
switch (connect_setup_header.status) {
.failed => {
std.log.err("connect setup failed, version={}.{}, reason='{s}'", .{
connect_setup_header.proto_major_ver,
connect_setup_header.proto_minor_ver,
connect_setup_header.readFailReason(reader),
});
return error.ConnectSetupFailed;
},
.authenticate => {
std.log.err("AUTHENTICATE! not implemented", .{});
return error.NotImplemetned;
},
.success => {
// TODO: check version?
std.log.debug("SUCCESS! version {}.{}", .{connect_setup_header.proto_major_ver, connect_setup_header.proto_minor_ver});
},
else => |status| {
std.log.err("Error: expected 0, 1 or 2 as first byte of connect setup reply, but got {}", .{status});
return error.MalformedXReply;
}
}
const connect_setup = x.ConnectSetup {
.buf = try allocator.allocWithOptions(u8, connect_setup_header.getReplyLen(), 4, null),
};
std.log.debug("connect setup reply is {} bytes", .{connect_setup.buf.len});
try x.readFull(reader, connect_setup.buf);
return ConnectResult{ .sock = sock, .setup = connect_setup };
}
pub fn asReply(comptime T: type, msg_bytes: []align(4) u8) !*T {
const generic_msg: *x.ServerMsg.Generic = @ptrCast(msg_bytes.ptr);
if (generic_msg.kind != .reply) {
std.log.err("expected reply but got {}", .{generic_msg});
return error.UnexpectedReply;
}
return @ptrCast(generic_msg);
}
fn readSocket(sock: std.os.socket_t, buffer: []u8) !usize {
return x.readSock(sock, buffer, 0);
}
|
0 | repos | repos/image-viewer/x11backend.zig | const builtin = @import("builtin");
const std = @import("std");
const Allocator = std.mem.Allocator;
const img = @import("img");
const convert = @import("convert.zig");
const x = @import("x");
const common = @import("x11common.zig");
const ContiguousReadBuffer = x.ContiguousReadBuffer;
const XY = @import("xy.zig").XY;
const Image = img.Image;
const Endian = std.builtin.Endian;
fn oom(e: error{OutOfMemory}) noreturn {
std.log.err("{s}", .{@errorName(e)});
@panic("OutOfMemory");
}
const ImageState = union(enum) {
no_file: void,
err: struct {
msg: []const u8,
filename: []const u8,
},
loaded: struct {
filename: []const u8,
size: XY(u16),
},
};
const State = struct {
pixmap: struct {
pending_create: bool = false,
pending_frees: u16 = 0,
},
window_size: XY(u16),
image: ImageState,
pub fn close(
self: *State,
allocator: Allocator,
sock: std.os.socket_t,
ids: Ids,
) !void {
if (self.pixmap.pending_create) {
var msg: [x.free_pixmap.len]u8 = undefined;
x.free_pixmap.serialize(&msg, ids.pixmap());
try common.send(sock, &msg);
self.pixmap.pending_create = false;
self.pixmap.pending_frees += 1;
}
switch (self.image) {
.no_file => {},
.err => |e| {
allocator.free(e.msg);
allocator.free(e.filename);
self.image = .no_file;
},
.loaded => |s| {
allocator.free(s.filename);
self.image = .no_file;
},
}
}
pub fn setError(
self: *State,
allocator: Allocator,
sock: std.os.socket_t,
ids: Ids,
filename: []const u8,
comptime fmt: []const u8,
args: anytype,
) !void {
try self.close(allocator, sock, ids);
const msg = std.fmt.allocPrint(allocator, fmt, args) catch |e| oom(e);
errdefer allocator.free(msg);
self.image = .{
.err = .{
.msg = msg,
.filename = allocator.dupe(u8, filename) catch |e| oom(e),
},
};
}
pub fn loadImage(
self: *State,
allocator: Allocator,
sock: std.os.socket_t,
screen_root: u32,
ids: Ids,
image_format: XImageFormat,
filename: []const u8,
) !void {
try self.close(allocator, sock, ids);
const content = blk: {
var file = std.fs.cwd().openFile(filename, .{}) catch |err| {
try self.setError(
allocator, sock, ids, filename,
"Open image failed with {s}:",
.{@errorName(err)},
);
return;
};
defer file.close();
break :blk file.readToEndAlloc(
allocator,
std.math.maxInt(usize),
) catch |err| {
try self.setError(
allocator, sock, ids, filename,
"Read image failed with {s}:",
.{@errorName(err)},
);
return;
};
};
defer allocator.free(content);
var image = img.Image.fromMemory(
allocator,
content,
) catch |err| {
try self.setError(
allocator, sock, ids, filename,
"Parse image failed with {s}:",
.{@errorName(err)},
);
return;
};
defer image.deinit();
const image_size_u16 = XY(u16){
.x = std.math.cast(u16, image.width) orelse {
try self.setError(
allocator, sock, ids, filename,
"todo: support image width {}",
.{image.width},
);
return;
},
.y = std.math.cast(u16, image.height) orelse {
try self.setError(
allocator, sock, ids, filename,
"todo: support image height {}",
.{image.height},
);
return;
},
};
const rgb32 = allocator.alloc(u8, 4 * image.width * image.height) catch |e| oom(e);
defer allocator.free(rgb32);
convert.toRgb32(rgb32, image);
{
var msg: [x.create_pixmap.len]u8 = undefined;
x.create_pixmap.serialize(&msg, .{
.id = ids.pixmap(),
.drawable_id = screen_root,
.depth = image_format.depth,
.width = image_size_u16.x,
.height = image_size_u16.y,
});
try common.send(sock, &msg);
}
{
const image_bytes_per_pixel = image_format.bits_per_pixel / 8;
const image_stride = std.mem.alignForward(
usize,
image_bytes_per_pixel * image.width,
image_format.scanline_pad / 8,
);
const put_image_line_msg = try allocator.alloc(u8, x.put_image.data_offset + image_stride);
defer allocator.free(put_image_line_msg);
var it = image.iterator();
var line_index: usize = 0;
while (line_index < image_size_u16.y) : (line_index += 1) {
try sendLine(
sock,
image_format,
ids.pixmap(),
ids.fg_gc(),
0,
// TODO: is this cast ok?
@intCast(line_index),
image_size_u16.x,
&it,
put_image_line_msg,
);
}
}
self.image = .{
.loaded = .{
.filename = allocator.dupe(u8, filename) catch |e| oom(e),
.size = .{ .x = @intCast(image.width), .y = @intCast(image.height) },
},
};
}
};
pub const Ids = struct {
base: u32,
pub fn window(self: Ids) u32 { return self.base; }
pub fn fg_gc(self: Ids) u32 { return self.base + 1; }
pub fn pixmap(self: Ids) u32 { return self.base + 2; }
};
const XImageFormat = struct {
endian: Endian,
depth: u8,
bits_per_pixel: u8,
scanline_pad: u8,
};
fn getXImageFormat(
endian: Endian,
formats: []const align(4) x.Format,
root_depth: u8,
) !XImageFormat {
var opt_match_index: ?usize = null;
for (formats, 0..) |format, i| {
if (format.depth == root_depth) {
if (opt_match_index) |_|
return error.MultiplePixmapFormatsSameDepth;
opt_match_index = i;
}
}
const match_index = opt_match_index orelse
return error.MissingPixmapFormat;
return XImageFormat{
.endian = endian,
.depth = root_depth,
.bits_per_pixel = formats[match_index].bits_per_pixel,
.scanline_pad = formats[match_index].scanline_pad,
};
}
const WindowRect = struct {
pos: XY(u16),
size: XY(u16),
};
fn calcStartWindowRect(state: State) WindowRect {
const desired_size: XY(u16) = switch (state.image) {
.no_file => .{ .x = 500, .y = 200 },
.err => .{ .x = 500, .y = 200 },
.loaded => |s| s.size,
};
return .{
.pos = .{ .x = 0, .y = 0 },
.size = desired_size,
};
}
pub fn go(maybe_filename: ?[]const u8) !void {
try x.wsaStartup();
var gpa_instance = std.heap.GeneralPurposeAllocator(.{}){ };
const gpa = gpa_instance.allocator();
const conn = try common.connect(gpa);
defer {
std.os.shutdown(conn.sock, .both) catch {};
conn.setup.deinit(gpa);
}
const fixed = conn.setup.fixed();
inline for (@typeInfo(@TypeOf(fixed.*)).Struct.fields) |field| {
std.log.debug("{s}: {any}", .{field.name, @field(fixed, field.name)});
}
const ids = Ids{ .base = conn.setup.fixed().resource_id_base };
std.log.debug("vendor: {s}", .{try conn.setup.getVendorSlice(fixed.vendor_len)});
const format_list_offset = x.ConnectSetup.getFormatListOffset(fixed.vendor_len);
const format_list_limit = x.ConnectSetup.getFormatListLimit(format_list_offset, fixed.format_count);
std.log.debug("fmt list off={} limit={}", .{format_list_offset, format_list_limit});
const formats = try conn.setup.getFormatList(format_list_offset, format_list_limit);
for (formats, 0..) |format, i| {
std.log.debug("format[{}] depth={:3} bpp={:3} scanpad={:3}", .{i, format.depth, format.bits_per_pixel, format.scanline_pad});
}
const screen = conn.setup.getFirstScreenPtr(format_list_limit);
inline for (@typeInfo(@TypeOf(screen.*)).Struct.fields) |field| {
std.log.debug("SCREEN 0| {s}: {any}", .{field.name, @field(screen, field.name)});
}
const image_format = blk: {
const image_endian: Endian = switch (fixed.image_byte_order) {
.lsb_first => .Little,
.msb_first => .Big,
else => |order| {
std.log.err("unknown image-byte-order {}", .{order});
return error.X11UnexpectedReply;
},
};
break :blk try getXImageFormat(image_endian, formats, screen.root_depth);
};
// TODO: maybe need to call conn.setup.verify or something?
{
var msg_buf: [x.create_gc.max_len]u8 = undefined;
const len = x.create_gc.serialize(&msg_buf, .{
.gc_id = ids.fg_gc(),
.drawable_id = screen.root,
}, .{
.background = screen.black_pixel,
.foreground = x.rgb24To(0xffaadd, screen.root_depth),
// prevent NoExposure events when we CopyArea
.graphics_exposures = false,
});
try conn.send(msg_buf[0..len]);
}
// get some font information
{
const text_literal = [_]u16 { 'm' };
const text = x.Slice(u16, [*]const u16) { .ptr = &text_literal, .len = text_literal.len };
var msg: [x.query_text_extents.getLen(text.len)]u8 = undefined;
x.query_text_extents.serialize(&msg, ids.fg_gc(), text);
try conn.send(&msg);
}
const double_buf = try x.DoubleBuffer.init(
std.mem.alignForward(usize, 1000, std.mem.page_size),
.{ .memfd_name = "ZigX11DoubleBuffer" },
);
defer double_buf.deinit();
std.log.info("read buffer capacity is {}", .{double_buf.half_len});
var buf = double_buf.contiguousReadBuffer();
const font_dims: FontDims = blk: {
_ = try x.readOneMsg(conn.reader(), @alignCast(buf.nextReadBuffer()));
switch (x.serverMsgTaggedUnion(@alignCast(buf.double_buffer_ptr))) {
.reply => |msg_reply| {
const msg: *x.ServerMsg.QueryTextExtents = @ptrCast(msg_reply);
break :blk .{
.width = @intCast(msg.overall_width),
.height = @intCast(msg.font_ascent + msg.font_descent),
.font_left = @intCast(msg.overall_left),
.font_ascent = msg.font_ascent,
};
},
else => |msg| {
std.log.err("expected a reply but got {}", .{msg});
return error.X11UnexpectedReply;
},
}
};
var state: State = .{
.pixmap = .{},
.window_size = .{ .x = 0, .y = 0 },
.image = .no_file,
};
if (maybe_filename) |filename| {
try state.loadImage(gpa, conn.sock, screen.root, ids, image_format, filename);
}
{
const window_rect = calcStartWindowRect(state);
state.window_size = window_rect.size;
var msg_buf: [x.create_window.max_len]u8 = undefined;
const len = x.create_window.serialize(&msg_buf, .{
.window_id = ids.window(),
.parent_window_id = screen.root,
.depth = 0, // we don't care, just inherit from the parent
.x = window_rect.pos.x,
.y = window_rect.pos.y,
.width = window_rect.size.x,
.height = window_rect.size.y,
.border_width = 0, // TODO: what is this?
.class = .input_output,
.visual_id = screen.root_visual,
}, .{
// .bg_pixmap = .copy_from_parent,
.bg_pixel = x.rgb24To(0xbbccdd, screen.root_depth),
// //.border_pixmap =
// .border_pixel = 0x01fa8ec9,
// .bit_gravity = .north_west,
// .win_gravity = .east,
// .backing_store = .when_mapped,
// .backing_planes = 0x1234,
// .backing_pixel = 0xbbeeeeff,
// .override_redirect = true,
// .save_under = true,
.event_mask =
x.event.key_press
| x.event.key_release
| x.event.button_press
| x.event.button_release
| x.event.enter_window
| x.event.leave_window
| x.event.pointer_motion
// | x.event.pointer_motion_hint WHAT THIS DO?
// | x.event.button1_motion WHAT THIS DO?
// | x.event.button2_motion WHAT THIS DO?
// | x.event.button3_motion WHAT THIS DO?
// | x.event.button4_motion WHAT THIS DO?
// | x.event.button5_motion WHAT THIS DO?
// | x.event.button_motion WHAT THIS DO?
| x.event.keymap_state
| x.event.exposure
,
// .dont_propagate = 1,
});
try conn.send(msg_buf[0..len]);
}
// render image to pixmap
// NOTE: if image is too big (width/height can't fit in u16), we will need
// mulitple pixmaps
{
var msg: [x.map_window.len]u8 = undefined;
x.map_window.serialize(&msg, ids.window());
try conn.send(&msg);
}
while (true) {
{
const recv_buf = buf.nextReadBuffer();
if (recv_buf.len == 0) {
std.log.err("buffer size {} not big enough!", .{buf.half_len});
return error.X11BufferTooSmall;
}
const len = try x.readSock(conn.sock, recv_buf, 0);
if (len == 0) {
std.log.info("X server connection closed", .{});
return;
}
buf.reserve(len);
}
while (true) {
const data = buf.nextReservedBuffer();
if (data.len < 32)
break;
const msg_len = x.parseMsgLen(data[0..32].*);
if (msg_len == 0)
break;
buf.release(msg_len);
//buf.resetIfEmpty();
switch (x.serverMsgTaggedUnion(@alignCast(data.ptr))) {
.err => |msg| {
std.log.err("{}", .{msg});
return error.X11Error;
},
.reply => |msg| {
std.log.info("todo: handle a reply message {}", .{msg});
return error.TodoHandleReplyMessage;
},
.key_press => |msg| {
std.log.info("key_press: keycode={}", .{msg.keycode});
},
.key_release => |msg| {
std.log.info("key_release: keycode={}", .{msg.keycode});
},
.button_press => |msg| {
std.log.info("button_press: {}", .{msg});
},
.button_release => |msg| {
std.log.info("button_release: {}", .{msg});
},
.enter_notify => |msg| {
std.log.info("enter_window: {}", .{msg});
},
.leave_notify => |msg| {
std.log.info("leave_window: {}", .{msg});
},
.motion_notify => |msg| {
// too much logging
_ = msg;
//std.log.info("pointer_motion: {}", .{msg});
},
.keymap_notify => |msg| {
std.log.info("keymap_state: {}", .{msg});
},
.expose => |msg| {
std.log.info("expose: {}", .{msg});
try render(conn.sock, ids, font_dims, state);
},
.mapping_notify => |msg| {
std.log.info("mapping_notify: {}", .{msg});
},
.no_exposure => |msg| std.debug.panic("unexpected {}", .{msg}),
.unhandled => |msg| {
std.log.info("todo: server msg {}", .{msg});
return error.UnhandledServerMsg;
},
.map_notify,
.reparent_notify,
.configure_notify,
=> unreachable, // did not register for structure_notify events
}
}
}
}
const FontDims = struct {
width: u8,
height: u8,
font_left: i16, // pixels to the left of the text basepoint
font_ascent: i16, // pixels up from the text basepoint to the top of the text
};
fn render(
sock: std.os.socket_t,
ids: Ids,
font_dims: FontDims,
state: State,
) !void {
switch (state.image) {
.no_file => {
try renderClearBg(sock, ids, state.window_size);
try renderText(sock, ids, font_dims, state.window_size, "No File Opened", 0);
},
.err => |err| {
try renderClearBg(sock, ids, state.window_size);
try renderText(sock, ids, font_dims, state.window_size, err.msg, -1);
try renderText(sock, ids, font_dims, state.window_size, err.filename, 1);
},
.loaded => |s| {
var msg: [x.copy_area.len]u8 = undefined;
x.copy_area.serialize(&msg, .{
.src_drawable_id = ids.pixmap(),
.dst_drawable_id = ids.window(),
.gc_id = ids.fg_gc(),
.src_x = 0,
.src_y = 0,
.dst_x = 0,
.dst_y = 0,
.width = s.size.x,
.height = s.size.y,
});
try common.send(sock, &msg);
},
}
}
fn renderClearBg(sock: std.os.socket_t, ids: Ids, size: XY(u16)) !void {
var msg: [x.clear_area.len]u8 = undefined;
x.clear_area.serialize(&msg, false, ids.window(), .{
.x = 0, .y = 0, .width = size.x, .height = size.y,
});
try common.send(sock, &msg);
}
fn renderText(
sock: std.os.socket_t,
ids: Ids,
font_dims: FontDims,
window_size: XY(u16),
text_slice: []const u8,
y_offset_multiplier: f32,
) !void {
const text = x.Slice(u8, [*]const u8) {
.ptr = text_slice.ptr,
.len = std.math.cast(u8, text_slice.len) orelse
std.debug.panic("todo: implement render message of length {}", .{text_slice.len}),
};
var msg: [x.image_text8.max_len]u8 = undefined;
const text_width = font_dims.width * text_slice.len;
const y_offset: i16 = @intFromFloat(y_offset_multiplier * @as(f32, @floatFromInt(font_dims.height)));
x.image_text8.serialize(&msg, text, .{
.drawable_id = ids.window(),
.gc_id = ids.fg_gc(),
.x = @divTrunc((@as(i16, @intCast(window_size.x)) - @as(i16, @intCast(text_width))), 2) + font_dims.font_left,
.y = @divTrunc((@as(i16, @intCast(window_size.y)) - @as(i16, @intCast(font_dims.height))), 2) + font_dims.font_ascent + y_offset,
});
try common.send(sock, msg[0 .. x.image_text8.getLen(text.len)]);
}
fn sendLine(
sock: std.os.socket_t,
dst_image_format: XImageFormat,
drawable_id: u32,
gc_id: u32,
x_loc: i16,
y: i16,
width: u16,
pixel_it: *img.color.PixelStorageIterator,
msg: []u8,
) !void {
const dst_bytes_per_pixel = dst_image_format.bits_per_pixel / 8;
const dst_stride = std.mem.alignForward(
u18,
dst_bytes_per_pixel * width,
dst_image_format.scanline_pad / 8,
);
std.debug.assert(msg.len == x.put_image.getLen(dst_stride));
x.put_image.serializeNoDataCopy(msg.ptr, dst_stride, .{
.format = .z_pixmap,
.drawable_id = drawable_id,
.gc_id = gc_id,
.width = width,
.height = 1,
.x = x_loc,
.y = y,
.left_pad = 0,
.depth = dst_image_format.depth,
});
{
var msg_off: usize = x.put_image.data_offset;
var col: u16 = 0;
while (col < width) : (col += 1) {
const color_f32 = pixel_it.next().?;
const r: u24 = @as(u24, @intFromFloat(color_f32.r / 1.0 * 0xff)) & 0xff;
const g: u24 = @as(u24, @intFromFloat(color_f32.g / 1.0 * 0xff)) & 0xff;
const b: u24 = @as(u24, @intFromFloat(color_f32.b / 1.0 * 0xff)) & 0xff;
const color = (r << 16) | (g << 8) | (b << 0);
switch (dst_image_format.depth) {
16 => std.mem.writeInt(
u16,
msg[msg_off..][0 .. 2],
x.rgb24To16(color),
dst_image_format.endian,
),
24 => std.mem.writeInt(
u24,
msg[msg_off..][0 .. 3],
color,
dst_image_format.endian,
),
32 => std.mem.writeInt(
u32,
msg[msg_off..][0 .. 4],
color,
dst_image_format.endian,
),
else => std.debug.panic("TODO: implement image depth {}", .{dst_image_format.depth}),
}
msg_off += dst_bytes_per_pixel;
}
}
try common.send(sock, msg);
}
|
0 | repos | repos/image-viewer/gentestimg.zig | const std = @import("std");
pub fn main() !void {
const width = 256;
const height = 256;
var file = try std.fs.cwd().createFile("testimg.ppm", .{});
defer file.close();
var bw = std.io.bufferedWriter(file.writer());
try bw.writer().print("P6\n{} {}\n255\n", .{width, height});
var y: usize = 0;
while (y < height) : (y += 1) {
const mod = y % 100;
var r: u8 = 0;
var g: u8 = 0;
var b: u8 = 0;
if (mod < 20) r = 0xff
else if (mod < 40) g = 0xff
else if (mod < 60) b = 0xff
else if (mod < 80) {}
else { r = 0xff; g = 0xff; b = 0xff; }
var x: usize = 0;
while (x < width) : (x += 1) {
try bw.writer().writeAll(&[3]u8{r, b, g});
}
}
try bw.flush();
}
|
0 | repos | repos/image-viewer/main.zig | const builtin = @import("builtin");
const std = @import("std");
const build_options = @import("build_options");
const img = @import("img");
const XY = @import("xy.zig").XY;
const x11backend = if (build_options.enable_x11_backend) @import("x11backend.zig") else struct {};
const win32backend = @import("win32backend.zig");
pub fn oom(e: error{OutOfMemory}) noreturn {
@panic(@errorName(e));
}
pub fn fatal(comptime fmt: []const u8, args: anytype) noreturn {
if (builtin.os.tag == .windows) {
win32backend.fatal(fmt, args);
} else {
std.log.err(fmt, args);
std.os.exit(0xff);
}
}
var windows_args_arena = if (builtin.os.tag == .windows)
std.heap.ArenaAllocator.init(std.heap.page_allocator) else struct{}{};
pub fn cmdlineArgs() [][*:0]u8 {
if (builtin.os.tag == .windows) {
const slices = std.process.argsAlloc(windows_args_arena.allocator()) catch |err| switch (err) {
error.OutOfMemory => oom(error.OutOfMemory),
error.InvalidCmdLine => @panic("InvalidCmdLine"),
error.Overflow => @panic("Overflow while parsing command line"),
};
const args = windows_args_arena.allocator().alloc([*:0]u8, slices.len - 1) catch |e| oom(e);
for (slices[1..], 0..) |slice, i| {
args[i] = slice.ptr;
}
return args;
}
return std.os.argv.ptr[1 .. std.os.argv.len];
}
pub fn wWinMain(
hInstance: std.os.windows.HINSTANCE,
hPrevInstance: ?std.os.windows.HINSTANCE,
pCmdLine: [*:0]u16,
nCmdShow: u32,
) c_int {
_ = hInstance;
_ = hPrevInstance;
_ = pCmdLine;
_ = nCmdShow;
return std.start.callMain();
}
pub fn main() !u8 {
var cmdline_opt = struct {
x11: if (build_options.enable_x11_backend) bool else void =
if (build_options.enable_x11_backend) false else {},
}{};
const args = blk: {
const all_args = cmdlineArgs();
var non_option_len: usize = 0;
for (all_args) |arg_ptr| {
const arg = std.mem.span(arg_ptr);
if (!std.mem.startsWith(u8, arg, "-")) {
all_args[non_option_len] = arg;
non_option_len += 1;
} else if (std.mem.eql(u8, arg, "--x11")) {
if (build_options.enable_x11_backend) {
cmdline_opt.x11 = true;
} else fatal("the x11 backend was not enabled in this build", .{});
} else {
fatal("unknown cmdline option '{s}'", .{arg});
}
}
break :blk all_args[0 .. non_option_len];
};
const maybe_filename: ?[]const u8 = blk: {
if (args.len == 0) break :blk null;
if (args.len > 1)
fatal("expected 0 or 1 cmdline arguments but got {}", .{args.len});
break :blk std.mem.span(args[0]);
};
if (builtin.os.tag == .windows) {
if (build_options.enable_x11_backend) {
if (cmdline_opt.x11) {
try x11backend.go(maybe_filename);
return 0;
}
}
try win32backend.go(maybe_filename);
} else {
try x11backend.go(maybe_filename);
}
return 0;
}
|
0 | repos | repos/image-viewer/convert.zig | const std = @import("std");
const img = @import("img");
pub fn toRgb32(dst_rgb32: []u8, image: img.Image) void {
const bytes = image.rawBytes();
switch (image.pixelFormat()) {
.rgb24 => {
var src_off: usize = 0;
var dst_off: usize = 0;
var y: usize = 0;
while (y < image.height) : (y += 1) {
var col: usize = 0;
while (col < image.width) : (col += 1) {
dst_rgb32[dst_off + 0] = bytes[src_off + 2];
dst_rgb32[dst_off + 1] = bytes[src_off + 1];
dst_rgb32[dst_off + 2] = bytes[src_off + 0];
dst_rgb32[dst_off + 3] = 0;
dst_off += 4;
src_off += 3;
}
}
},
.rgba32 => {
var src_off: usize = 0;
var dst_off: usize = 0;
var y: usize = 0;
while (y < image.height) : (y += 1) {
var col: usize = 0;
while (col < image.width) : (col += 1) {
dst_rgb32[dst_off + 0] = bytes[src_off + 2];
dst_rgb32[dst_off + 1] = bytes[src_off + 1];
dst_rgb32[dst_off + 2] = bytes[src_off + 0];
dst_rgb32[dst_off + 3] = 0;
dst_off += 4;
src_off += 4;
}
}
},
else => std.debug.panic(
"x11 does not yet support pixel format {s}",
.{@tagName(image.pixelFormat())},
),
}
}
|
0 | repos | repos/image-viewer/README.md | # Image Viewer
An image viewer experiment in Zig.
Currently tested with zig version `0.11.0`.
# TODO
* scale the image with the window size?
* zoom?
* add direct wayland support (not just through Xwayland)
* add support for Mac
|
0 | repos | repos/image-viewer/xy.zig | pub fn XY(comptime T: type) type {
return struct {
x: T,
y: T,
pub fn init(x: T, y: T) @This() {
return .{ .x = x, .y = y };
}
};
}
|
0 | repos | repos/image-viewer/GitRepoStep.zig | //! Publish Date: 2023_03_19
//! This file is hosted at github.com/marler8997/zig-build-repos and is meant to be copied
//! to projects that use it.
const std = @import("std");
const GitRepoStep = @This();
pub const ShaCheck = enum {
none,
warn,
err,
pub fn reportFail(self: ShaCheck, comptime fmt: []const u8, args: anytype) void {
switch (self) {
.none => unreachable,
.warn => std.log.warn(fmt, args),
.err => {
std.log.err(fmt, args);
std.os.exit(0xff);
},
}
}
};
step: std.build.Step,
url: []const u8,
name: []const u8,
branch: ?[]const u8 = null,
sha: []const u8,
path: []const u8,
sha_check: ShaCheck = .warn,
fetch_enabled: bool,
var cached_default_fetch_option: ?bool = null;
pub fn defaultFetchOption(b: *std.build.Builder) bool {
if (cached_default_fetch_option) |_| {} else {
cached_default_fetch_option = if (b.option(bool, "fetch", "automatically fetch network resources")) |o| o else false;
}
return cached_default_fetch_option.?;
}
pub fn create(b: *std.build.Builder, opt: struct {
url: []const u8,
branch: ?[]const u8 = null,
sha: []const u8,
path: ?[]const u8 = null,
sha_check: ShaCheck = .warn,
fetch_enabled: ?bool = null,
first_ret_addr: ?usize = null,
}) *GitRepoStep {
var result = b.allocator.create(GitRepoStep) catch @panic("memory");
const name = std.fs.path.basename(opt.url);
result.* = GitRepoStep{
.step = std.build.Step.init(.{
.id = .custom,
.name = b.fmt("clone git repository '{s}'", .{name}),
.owner = b,
.makeFn = make,
.first_ret_addr = opt.first_ret_addr orelse @returnAddress(),
.max_rss = 0,
}),
.url = opt.url,
.name = name,
.branch = opt.branch,
.sha = opt.sha,
.path = if (opt.path) |p| b.allocator.dupe(u8, p) catch @panic("OOM")
else b.pathFromRoot(b.pathJoin(&.{"dep", name})),
.sha_check = opt.sha_check,
.fetch_enabled = if (opt.fetch_enabled) |fe| fe else defaultFetchOption(b),
};
return result;
}
// TODO: this should be included in std.build, it helps find bugs in build files
fn hasDependency(step: *const std.build.Step, dep_candidate: *const std.build.Step) bool {
for (step.dependencies.items) |dep| {
// TODO: should probably use step.loop_flag to prevent infinite recursion
// when a circular reference is encountered, or maybe keep track of
// the steps encounterd with a hash set
if (dep == dep_candidate or hasDependency(dep, dep_candidate))
return true;
}
return false;
}
fn make(step: *std.Build.Step, prog_node: *std.Progress.Node) !void {
_ = prog_node;
const self = @fieldParentPtr(GitRepoStep, "step", step);
std.fs.accessAbsolute(self.path, .{}) catch {
const branch_args = if (self.branch) |b| &[2][]const u8{ " -b ", b } else &[2][]const u8{ "", "" };
if (!self.fetch_enabled) {
std.debug.print("Error: git repository '{s}' does not exist\n", .{self.path});
std.debug.print(" Use -Dfetch to download it automatically, or run the following to clone it:\n", .{});
std.debug.print(" git clone {s}{s}{s} {s} && git -C {3s} checkout {s} -b fordep\n", .{
self.url,
branch_args[0],
branch_args[1],
self.path,
self.sha,
});
std.os.exit(1);
}
{
var args = std.ArrayList([]const u8).init(self.step.owner.allocator);
defer args.deinit();
try args.append("git");
try args.append("clone");
try args.append(self.url);
// TODO: clone it to a temporary location in case of failure
// also, remove that temporary location before running
try args.append(self.path);
if (self.branch) |branch| {
try args.append("-b");
try args.append(branch);
}
try run(self.step.owner, args.items);
}
try run(self.step.owner, &[_][]const u8{
"git",
"-C",
self.path,
"checkout",
self.sha,
"-b",
"fordep",
});
};
try self.checkSha();
}
fn checkSha(self: GitRepoStep) !void {
if (self.sha_check == .none)
return;
const result: union(enum) { failed: anyerror, output: []const u8 } = blk: {
const result = std.ChildProcess.exec(.{
.allocator = self.step.owner.allocator,
.argv = &[_][]const u8{
"git",
"-C",
self.path,
"rev-parse",
"HEAD",
},
.cwd = self.step.owner.build_root.path,
.env_map = self.step.owner.env_map,
}) catch |e| break :blk .{ .failed = e };
try std.io.getStdErr().writer().writeAll(result.stderr);
switch (result.term) {
.Exited => |code| {
if (code == 0) break :blk .{ .output = result.stdout };
break :blk .{ .failed = error.GitProcessNonZeroExit };
},
.Signal => break :blk .{ .failed = error.GitProcessFailedWithSignal },
.Stopped => break :blk .{ .failed = error.GitProcessWasStopped },
.Unknown => break :blk .{ .failed = error.GitProcessFailed },
}
};
switch (result) {
.failed => |err| {
return self.sha_check.reportFail("failed to retreive sha for repository '{s}': {s}", .{ self.name, @errorName(err) });
},
.output => |output| {
if (!std.mem.eql(u8, std.mem.trimRight(u8, output, "\n\r"), self.sha)) {
return self.sha_check.reportFail("repository '{s}' sha does not match\nexpected: {s}\nactual : {s}\n", .{ self.name, self.sha, output });
}
},
}
}
fn run(builder: *std.build.Builder, argv: []const []const u8) !void {
{
var msg = std.ArrayList(u8).init(builder.allocator);
defer msg.deinit();
const writer = msg.writer();
var prefix: []const u8 = "";
for (argv) |arg| {
try writer.print("{s}\"{s}\"", .{ prefix, arg });
prefix = " ";
}
std.log.info("[RUN] {s}", .{msg.items});
}
var child = std.ChildProcess.init(argv, builder.allocator);
child.stdin_behavior = .Ignore;
child.stdout_behavior = .Inherit;
child.stderr_behavior = .Inherit;
child.cwd = builder.build_root.path;
child.env_map = builder.env_map;
try child.spawn();
const result = try child.wait();
switch (result) {
.Exited => |code| if (code != 0) {
std.log.err("git clone failed with exit code {}", .{code});
std.os.exit(0xff);
},
else => {
std.log.err("git clone failed with: {}", .{result});
std.os.exit(0xff);
},
}
}
// Get's the repository path and also verifies that the step requesting the path
// is dependent on this step.
pub fn getPath(self: *const GitRepoStep, who_wants_to_know: *const std.build.Step) []const u8 {
if (!hasDependency(who_wants_to_know, &self.step))
@panic("a step called GitRepoStep.getPath but has not added it as a dependency");
return self.path;
}
|
0 | repos | repos/image-viewer/build.zig.zon | .{
.name = "image-viewer",
.version = "0.0.1",
.dependencies = .{
.zigimg = .{
.url = "https://github.com/zigimg/zigimg/archive/40ddb16fd246174545b7327a12dce7f0889ada7a.tar.gz",
.hash = "12208c69b00540fe3f38893800fb6c89a9fe27b4a687de5d0462e498a43db4caeec0",
},
.zigx = .{
.url = "https://github.com/marler8997/zigx/archive/5dd695270862619f1a7a2457c4e61295cc9d96de.tar.gz",
.hash = "1220c7a45bd3e3aee922fa79187d6f5876925f11cc6af40af7f2c9331dfed39f76da",
},
.resinator = .{
.url = "https://github.com/tupleapp/resinator/archive/41e67244161337925554f5150fcdea2e816305b3.tar.gz",
.hash = "122090609f5c4d0e1602b7907db170fcc0b3c32e22b08c46f264f12bf74be3dbba1b",
},
},
}
|
0 | repos | repos/image-viewer/build.zig | const std = @import("std");
const GitRepoStep = @import("GitRepoStep.zig");
pub fn build(b: *std.build.Builder) void {
const target = b.standardTargetOptions(.{});
const optimize = b.standardOptimizeOption(.{});
const is_windows = target.getOs().tag == .windows;
const enable_x11_backend = blk: {
if (b.option(bool, "x11", "enable the x11 backend")) |opt| break :blk opt;
break :blk true;
};
const zigwin32_repo = GitRepoStep.create(b, .{
.url = "https://github.com/marlersoft/zigwin32",
.branch = "15.0.2-preview",
.sha = "007649ade45ffb544de3aafbb112de25064d3d92",
.fetch_enabled = true,
});
const build_options = b.addOptions();
build_options.addOption(bool, "enable_x11_backend", enable_x11_backend);
const zigimg_dep = b.dependency("zigimg", .{
.target = target,
.optimize = optimize,
});
const zigwin32 = b.addModule("win32", .{
.source_file = .{ .path = b.pathJoin(&.{zigwin32_repo.path, "win32.zig"}), },
});
const resinator_dep = b.dependency("resinator", .{});
const resinator = resinator_dep.artifact("resinator");
//const exe = b.addExecutable("image-viewer", if (is_windows) "win32main.zig" else "main.zig");
const exe = b.addExecutable(.{
.name = "image-viewer",
.root_source_file = .{ .path = "main.zig" },
.target = target,
.optimize = optimize,
});
exe.addOptions("build_options", build_options);
exe.addModule("img", zigimg_dep.module("zigimg"));
if (enable_x11_backend) {
const zigx_dep = b.dependency("zigx", .{});
exe.addModule("x", zigx_dep.module("zigx"));
}
if (is_windows) {
exe.subsystem = .Windows;
exe.step.dependOn(&zigwin32_repo.step);
exe.addModule("win32", zigwin32);
{
const compile_res = b.addRunArtifact(resinator);
// end of resinator options so it doesn't see absolute paths as options
compile_res.addArg("--");
compile_res.addFileArg(.{ .path = "res/image-viewer.rc" });
const res = compile_res.addOutputFileArg("image-viewer.res");
exe.step.dependOn(&compile_res.step);
exe.link_objects.append(.{
.static_path = res,
}) catch unreachable;
}
}
b.installArtifact(exe);
const run_cmd = b.addRunArtifact(exe);
run_cmd.step.dependOn(b.getInstallStep());
if (b.args) |args| {
run_cmd.addArgs(args);
}
const run_step = b.step("run", "Run the app");
run_step.dependOn(&run_cmd.step);
}
|
0 | repos | repos/image-viewer/win32backend.zig | const builtin = @import("builtin");
const std = @import("std");
const win32 = struct {
usingnamespace @import("win32").zig;
usingnamespace @import("win32").foundation;
usingnamespace @import("win32").system.library_loader;
usingnamespace @import("win32").system.system_services;
usingnamespace @import("win32").ui.hi_dpi;
usingnamespace @import("win32").ui.input.keyboard_and_mouse;
usingnamespace @import("win32").ui.shell;
usingnamespace @import("win32").ui.windows_and_messaging;
usingnamespace @import("win32").graphics.gdi;
};
const win32fix = struct {
pub extern "user32" fn LoadImageW(
hInst: ?win32.HINSTANCE,
name: ?[*:0]const align(1) u16,
type: win32.GDI_IMAGE_TYPE,
cx: i32,
cy: i32,
flags: win32.IMAGE_FLAGS,
) callconv(@import("std").os.windows.WINAPI) ?win32.HANDLE;
};
const L = win32.L;
const HINSTANCE = win32.HINSTANCE;
const CW_USEDEFAULT = win32.CW_USEDEFAULT;
const MSG = win32.MSG;
const HWND = win32.HWND;
const img = @import("img");
const convert = @import("convert.zig");
const XY = @import("xy.zig").XY;
const Image = img.Image;
pub const UNICODE = true;
const window_style = win32.WS_OVERLAPPEDWINDOW;
const window_menu: win32.BOOL = 0;
const window_style_ex = win32.WINDOW_EX_STYLE.initFlags(.{});
pub const State = union(enum) {
no_file: void,
err: struct {
msg: []const u8,
filename: []const u8,
},
loaded: struct {
filename: []const u8,
size: XY(i32),
rgb32: []u8,
},
pub fn close(self: *State) void {
switch (self.*) {
.no_file => {},
.err => |e| {
global.gpa.free(e.msg);
global.gpa.free(e.filename);
self.* = .no_file;
},
.loaded => |s| {
global.gpa.free(s.filename);
global.gpa.free(s.rgb32);
self.* = .no_file;
},
}
}
pub fn setError(
self: *State,
filename: []const u8,
comptime fmt: []const u8,
args: anytype,
) void {
self.close();
const msg = std.fmt.allocPrint(global.gpa, fmt, args) catch |e| oom(e);
errdefer global.gpa.free(msg);
self.* = .{
.err = .{
.msg = msg,
.filename = global.gpa.dupe(u8, filename) catch |e| oom(e),
},
};
}
pub fn loadImage(self: *State, filename: []const u8) void {
self.close();
const content = blk: {
var file = std.fs.cwd().openFile(filename, .{}) catch |err| {
self.setError(
filename,
"Open image failed with {s}:",
.{@errorName(err)},
);
return;
};
defer file.close();
break :blk file.readToEndAlloc(
global.gpa,
std.math.maxInt(usize),
) catch |err| {
self.setError(
filename,
"Read image failed with {s}:",
.{@errorName(err)},
);
return;
};
};
defer global.gpa.free(content);
var image = img.Image.fromMemory(
global.gpa,
content,
) catch |err| {
self.setError(
filename,
"Parse image failed with {s}:",
.{@errorName(err)},
);
return;
};
defer image.deinit();
const rgb32 = global.gpa.alloc(u8, 4 * image.width * image.height) catch |e| oom(e);
errdefer global.gpa.free(rgb32);
convert.toRgb32(rgb32, image);
self.* = .{
.loaded = .{
.filename = global.gpa.dupe(u8, filename) catch |e| oom(e),
.size = .{ .x = @intCast(image.width), .y = @intCast(image.height) },
.rgb32 = rgb32,
},
};
}
};
const global = struct {
pub var gpa_instance = std.heap.GeneralPurposeAllocator(.{}){ };
pub const gpa = gpa_instance.allocator();
pub var state: State = .no_file;
};
fn msgBoxA(msg: [*:0]const u8) void {
_ = win32.MessageBoxA(null, msg, "Image Viewer", win32.MB_OK);
}
fn oom(e: error{OutOfMemory}) noreturn {
std.log.err("{s}", .{@errorName(e)});
msgBoxA("Out of memory");
std.os.exit(0xff);
}
pub fn fatal(comptime fmt: []const u8, args: anytype) noreturn {
std.log.err(fmt, args);
// TODO: detect if there is a console or not, only show message box
// if there is not a console
var arena = std.heap.ArenaAllocator.init(std.heap.page_allocator);
const msg = std.fmt.allocPrintZ(arena.allocator(), fmt, args) catch @panic("Out of memory");
msgBoxA(msg.ptr);
std.os.exit(0xff);
}
fn getWindowDpi(hWnd: HWND) u32 {
const dpi = win32.GetDpiForWindow(hWnd);
// is this possible?
if (dpi == 0) fatal("GetDpiForWindow failed, error={}", .{win32.GetLastError()});
return dpi;
}
const ID_ICON_IMAGE_VIEWER = 1;
const Icons = struct {
small: ?win32.HICON,
large: ?win32.HICON,
};
fn getIcons() Icons {
const size_small = XY(i32){
.x = win32.GetSystemMetrics(win32.SM_CXSMICON),
.y = win32.GetSystemMetrics(win32.SM_CYSMICON)
};
const size_large = XY(i32){
.x = win32.GetSystemMetrics(win32.SM_CXICON),
.y = win32.GetSystemMetrics(win32.SM_CYICON)
};
const small = win32fix.LoadImageW(
win32.GetModuleHandle(null),
@ptrFromInt(ID_ICON_IMAGE_VIEWER),
.ICON,
size_small.x,
size_small.y,
win32.IMAGE_FLAGS.initFlags(.{ .DEFAULTCOLOR=1, .SHARED=1 }),
);
if (small == null) {
std.log.err("LoadImage for small icon failed, error={}", .{win32.GetLastError()});
// not a critical error
}
const large = win32fix.LoadImageW(
win32.GetModuleHandle(null),
@ptrFromInt(ID_ICON_IMAGE_VIEWER),
.ICON,
size_large.x,
size_large.y,
win32.IMAGE_FLAGS.initFlags(.{ .DEFAULTCOLOR=1, .SHARED=1 }),
);
if (large == null) {
std.log.err("LoadImage for large icon failed, error={}", .{win32.GetLastError()});
// not a critical error
}
return .{
.small = @ptrCast(small),
.large = @ptrCast(large),
};
}
pub fn go(maybe_filename: ?[]const u8) !void {
if (maybe_filename) |filename| {
global.state.loadImage(filename);
}
// See https://gist.github.com/marler8997/9f39458d26e2d8521d48e36530fbb459
// for notes about windows DPI scaling.
// {
// var dpi_awareness: win32.PROCESS_DPI_AWARENESS = undefined;
// {
// const result = win32.GetProcessDpiAwareness(null, &dpi_awareness);
// if (result != 0)
// fatal("GetProcessDpiAwareness failed, error={}", .{result});
// }
// if (dpi_awareness != win32.PROCESS_PER_MONITOR_DPI_AWARE)
// // We'll just exit for now until we see if this is possible
// // it *might* be possible on older versions of windows
// fatal("unexpected dpi awareness {}", dpi_awareness);
// }
const icons = getIcons();
const CLASS_NAME = L("ImageViewer");
const wc = win32.WNDCLASSEXW{
.cbSize = @sizeOf(win32.WNDCLASSEXW),
.style = @enumFromInt(0),
.lpfnWndProc = WindowProc,
.cbClsExtra = 0,
.cbWndExtra = 0,
.hInstance = win32.GetModuleHandle(null),
.hIcon = icons.large,
.hIconSm = icons.small,
.hCursor = null,
.hbrBackground = null,
.lpszMenuName = null,
.lpszClassName = CLASS_NAME,
};
const class_id = win32.RegisterClassExW(&wc);
if (class_id == 0) {
std.log.err("RegisterClass failed, error={}", .{win32.GetLastError()});
std.os.exit(0xff);
}
const hwnd = win32.CreateWindowEx(
win32.WINDOW_EX_STYLE.initFlags(.{ .ACCEPTFILES = 1 }),
CLASS_NAME, // Window class
// TODO: use the image name in the title if we have one
L("Image Viewer"),
window_style,
0, 0, // position
0, 0, // size
null, // Parent window
null, // Menu
win32.GetModuleHandle(null),
null // Additional application data
) orelse {
std.log.err("CreateWindow failed with {}", .{win32.GetLastError()});
std.os.exit(0xff);
};
const dpi = getWindowDpi(hwnd);
{
const rect = calcStartWindowRect(hwnd, dpi);
if (0 == win32.SetWindowPos(
hwnd,
null,
rect.left, rect.top,
rect.right - rect.left,
rect.bottom - rect.top,
win32.SET_WINDOW_POS_FLAGS.initFlags(.{}),
))
fatal("SetWindowPos failed, error={}", .{win32.GetLastError()});
}
_ = win32.ShowWindow(hwnd, win32.SW_SHOW);
var msg: MSG = undefined;
while (win32.GetMessage(&msg, null, 0, 0) != 0) {
_ = win32.TranslateMessage(&msg);
_ = win32.DispatchMessage(&msg);
}
}
fn clientToWindowSize(size: XY(i32)) XY(i32) {
var rect = win32.RECT{
.left = 0,
.top = 0,
.right = size.x,
.bottom = size.y,
};
if (0 == win32.AdjustWindowRectEx(&rect, window_style, window_menu, window_style_ex))
std.debug.panic("AdjustWindowRectExForDpi failed, error={}", .{win32.GetLastError()});
return XY(i32){
.x = rect.right - rect.left,
.y = rect.bottom - rect.top,
};
}
fn scaleDpiOnly(comptime T: type, value: T, dpi: u32) T {
switch (@typeInfo(T)) {
.Int => return @intFromFloat(
@as(f32, @floatFromInt(value)) * (@as(f32, @floatFromInt(dpi)) / 96.0)
),
else => @compileError("scaleDpiOnly doesn't support type " ++ @typeName(T)),
}
}
fn calcStartWindowRect(hWnd: HWND, dpi: u32) win32.RECT {
const monitor = win32.MonitorFromWindow(
hWnd, win32.MONITOR_DEFAULTTOPRIMARY
) orelse @panic("unexpected");
var info: win32.MONITORINFO = undefined;
info.cbSize = @sizeOf(@TypeOf(info));
if (0 == win32.GetMonitorInfoW(monitor, &info)) @panic("unexpected");
std.log.info("monitor rect {},{} {},{}", .{
info.rcWork.left, info.rcWork.top,
info.rcWork.right, info.rcWork.bottom,
});
const desktop_size = XY(i32){
.x = info.rcWork.right - info.rcWork.left,
.y = info.rcWork.bottom - info.rcWork.top,
};
const desired_client_size: XY(i32) = switch (global.state) {
.no_file, .err => .{
.x = scaleDpiOnly(i32, 500, dpi),
.y = scaleDpiOnly(i32, 200, dpi),
},
.loaded => |s| s.size,
};
const desired_window_size = clientToWindowSize(desired_client_size);
var window_size = desired_window_size;
if (desktop_size.x < desired_window_size.x) {
std.log.info(
"clamping window width {} to desktop {}",
.{desired_window_size.x, desktop_size.x},
);
window_size.x = desktop_size.x;
}
if (desktop_size.y < desired_window_size.y) {
std.log.info(
"clamping window height {} to desktop {}",
.{desired_window_size.y, desktop_size.y},
);
window_size.y = desktop_size.y;
}
const adjust = XY(i32){
.x = @divTrunc(desktop_size.x - window_size.x, 2),
.y = @divTrunc(desktop_size.y - window_size.y, 2),
};
return .{
.left = info.rcWork.left + adjust.x,
.top = info.rcWork.top + adjust.y,
.right = info.rcWork.right - adjust.x,
.bottom = info.rcWork.bottom - adjust.y,
};
}
fn WindowProc(
hWnd: HWND,
uMsg: u32,
wParam: win32.WPARAM,
lParam: win32.LPARAM,
) callconv(std.os.windows.WINAPI) win32.LRESULT {
switch (uMsg) {
win32.WM_KEYDOWN => {
if (wParam == @intFromEnum(win32.VK_F5)) {
std.log.info("TODO: refresh the file", .{});
}
},
win32.WM_DESTROY => {
win32.PostQuitMessage(0);
return 0;
},
win32.WM_PAINT => {
paint(hWnd);
return 0;
},
win32.WM_DROPFILES => {
onDragDrop(hWnd, @ptrFromInt(wParam));
return 0;
},
win32.WM_SIZE => {
// since we "stretch" the image accross the full window, we
// always invalidate the full client area on each window resize
std.debug.assert(0 != win32.InvalidateRect(hWnd, null, 0));
},
else => {},
}
return win32.DefWindowProc(hWnd, uMsg, wParam, lParam);
}
fn getClientSize(hWnd: HWND) win32.SIZE {
var client_rect: win32.RECT = undefined;
std.debug.assert(0 != win32.GetClientRect(hWnd, &client_rect));
return .{
.cx = client_rect.right - client_rect.left,
.cy = client_rect.bottom - client_rect.top,
};
}
fn onDragDrop(hWnd: HWND, hDrop: win32.HDROP) void {
defer win32.DragFinish(hDrop);
{
const file_count = win32.DragQueryFileA(hDrop, 0xffffffff, null, 0);
if (file_count == 0) {
msgBoxA("Drag and Dropping 0 files... is this possible?");
return;
}
if (file_count > 1) {
msgBoxA("Drag and Dropping multiple files is not supported.");
return;
}
}
const filename_len = win32.DragQueryFileA(hDrop, 0, null, 0);
if (filename_len == 0) {
var msg_buf: [100]u8 = undefined;
const msg = std.fmt.bufPrintZ(
&msg_buf,
"DragQueryFile to get file size failed with {s}",
.{@tagName(win32.GetLastError())},
) catch |e| switch (e) {
error.NoSpaceLeft => unreachable,
};
msgBoxA(msg);
return;
}
const filename = global.gpa.allocSentinel(u8, filename_len, 0) catch |e| oom(e);
defer global.gpa.free(filename);
{
const len2 = win32.DragQueryFileA(hDrop, 0, filename.ptr, filename_len + 1);
if (len2 != filename_len) @panic("codebug");
}
global.state.loadImage(filename);
std.debug.assert(0 != win32.InvalidateRect(hWnd, null, 0));
std.log.warn("TODO: adjust window dimensions to the new aspect ratio!", .{});
}
fn paint(hWnd: HWND) void {
var ps: win32.PAINTSTRUCT = undefined;
const hdc = win32.BeginPaint(hWnd, &ps);
const client_size = getClientSize(hWnd);
switch (global.state) {
.no_file => {
fillBg(hdc, ps.rcPaint);
paintMsg(hdc, client_size, "No File Opened", 0);
},
.err => |err| {
fillBg(hdc, ps.rcPaint);
paintMsg(hdc, client_size, err.msg, -1);
paintMsg(hdc, client_size, err.filename, 1);
},
.loaded => |s| {
std.log.info("PAINT! client rect {}x{} image {}x{}", .{
client_size.cx, client_size.cy,
s.size.x, s.size.y,
});
const bitmap_info = win32.BITMAPINFO{
.bmiHeader = .{
.biSize = @sizeOf(win32.BITMAPINFOHEADER),
.biWidth = @intCast(s.size.x),
.biHeight = -@as(i32, @intCast(s.size.y)),
.biPlanes = 1,
.biBitCount = 32,
.biCompression = win32.BI_RGB,
.biSizeImage = 0,
.biXPelsPerMeter = 0,
.biYPelsPerMeter = 0,
.biClrUsed = 0,
.biClrImportant = 0,
},
.bmiColors = undefined,
};
const result = win32.StretchDIBits(
hdc,
0, 0, client_size.cx, client_size.cy,
0, 0, @intCast(s.size.x), @intCast(s.size.y),
s.rgb32.ptr,
&bitmap_info,
win32.DIB_RGB_COLORS,
win32.SRCCOPY,
);
if (result == 0)
std.debug.panic("StretchDIBits failed with {}", .{win32.GetLastError()});
//std.debug.assert(result == client_size.cx);
//std.log.info("result is {}", .{result});
std.debug.assert(result != 0);
},
}
_ = win32.EndPaint(hWnd, &ps);
}
fn fillBg(hdc: ?win32.HDC, paint_rect: win32.RECT) void {
_ = win32.FillRect(
hdc,
&paint_rect,
@ptrFromInt(@as(usize, @intFromEnum(win32.COLOR_WINDOW)) + 1),
);
}
fn paintMsg(
hdc: ?win32.HDC,
client_size: win32.SIZE,
msg: []const u8,
y_offset_multiplier: f32,
) void {
var size: win32.SIZE = undefined;
std.debug.assert(0 != win32.GetTextExtentPoint32A(
hdc, @ptrCast(msg.ptr), @intCast(msg.len), &size
));
const y_offset: i32 = @intFromFloat(y_offset_multiplier * @as(f32, @floatFromInt(size.cy)));
const x = @divTrunc(client_size.cx - size.cx, 2);
const y = @divTrunc(client_size.cy - size.cy, 2) + y_offset;
_ = win32.TextOutA(hdc, x, y, @ptrCast(msg.ptr), @intCast(msg.len));
}
|
0 | repos/image-viewer | repos/image-viewer/res/image-viewer.rc | // LANG_NEUTRAL(0), SUBLANG_NEUTRAL(0)
LANGUAGE 0, 0
//1 RT_MANIFEST "image-viewer.manifest"
1 24 "image-viewer.manifest"
#define ID_ICON_IMAGE_VIEWER 1
ID_ICON_IMAGE_VIEWER ICON "image-viewer.ico"
VS_VERSION_INFO VERSIONINFO
//FILEVERSION VERSION_MAJOR,VERSION_MINOR,VERSION_PATCH,VERSION_COMMIT_HEIGHT
//PRODUCTVERSION VERSION_MAJOR,VERSION_MINOR,VERSION_PATCH,VERSION_COMMIT_HEIGHT
//FILEFLAGSMASK VS_FFI_FILEFLAGSMASK
//FILEFLAGS VER_DBG
//FILEOS VOS_NT
//FILETYPE VFT_APP
//FILESUBTYPE VFT2_UNKNOWN
BEGIN
BLOCK "StringFileInfo"
BEGIN
BLOCK "040904b0"
BEGIN
VALUE "CompanyName", "Jonathan Marler"
VALUE "FileDescription", "ImageViewer"
//VALUE "FileVersion", VERSION
VALUE "LegalCopyright", "(C) 2023 Jonathan Marler"
VALUE "OriginalFilename", "image-viewer.exe"
VALUE "ProductName", "ImageViewer"
//VALUE "ProductVersion", VERSION
END
END
BLOCK "VarFileInfo"
BEGIN
VALUE "Translation", 0x0409,1200
END
END
|
0 | repos | repos/libvaxis/README.md | # libvaxis
```
It begins with them, but ends with me. Their son, Vaxis
```

Libvaxis _does not use terminfo_. Support for vt features is detected through
terminal queries.
Contributions are welcome.
Vaxis uses zig `0.13.0`.
## Features
libvaxis supports all major platforms: macOS, Windows, Linux/BSD/and other
Unix-likes.
| Feature | libvaxis |
| ------------------------------ | :------: |
| RGB | ✅ |
| Hyperlinks | ✅ |
| Bracketed Paste | ✅ |
| Kitty Keyboard | ✅ |
| Styled Underlines | ✅ |
| Mouse Shapes (OSC 22) | ✅ |
| System Clipboard (OSC 52) | ✅ |
| System Notifications (OSC 9) | ✅ |
| System Notifications (OSC 777) | ✅ |
| Synchronized Output (DEC 2026) | ✅ |
| Unicode Core (DEC 2027) | ✅ |
| Color Mode Updates (DEC 2031) | ✅ |
| [In-Band Resize Reports](https://gist.github.com/rockorager/e695fb2924d36b2bcf1fff4a3704bd83) | ✅ |
| Images (kitty) | ✅ |
## Usage
[Documentation](https://rockorager.github.io/libvaxis/#vaxis.Vaxis)
[Starter repo](https://github.com/rockorager/libvaxis-starter)
Vaxis requires three basic primitives to operate:
1. A TTY instance
2. An instance of Vaxis
3. An event loop
The library provides a general purpose posix TTY implementation, as well as a
multi-threaded event loop implementation. Users of the library are encouraged to
use the event loop of their choice. The event loop is responsible for reading
the TTY, passing the read bytes to the vaxis parser, and handling events.
A core feature of Vaxis is it's ability to detect features via terminal queries
instead of relying on a terminfo database. This requires that the event loop
also handle these query responses and update the Vaxis.caps struct accordingly.
See the `Loop` implementation to see how this is done if writing your own event
loop.
## Example
```zig
const std = @import("std");
const vaxis = @import("vaxis");
const Cell = vaxis.Cell;
const TextInput = vaxis.widgets.TextInput;
const border = vaxis.widgets.border;
// This can contain internal events as well as Vaxis events.
// Internal events can be posted into the same queue as vaxis events to allow
// for a single event loop with exhaustive switching. Booya
const Event = union(enum) {
key_press: vaxis.Key,
winsize: vaxis.Winsize,
focus_in,
foo: u8,
};
pub fn main() !void {
var gpa = std.heap.GeneralPurposeAllocator(.{}){};
defer {
const deinit_status = gpa.deinit();
//fail test; can't try in defer as defer is executed after we return
if (deinit_status == .leak) {
std.log.err("memory leak", .{});
}
}
const alloc = gpa.allocator();
// Initialize a tty
var tty = try vaxis.Tty.init();
defer tty.deinit();
// Initialize Vaxis
var vx = try vaxis.init(alloc, .{});
// deinit takes an optional allocator. If your program is exiting, you can
// choose to pass a null allocator to save some exit time.
defer vx.deinit(alloc, tty.anyWriter());
// The event loop requires an intrusive init. We create an instance with
// stable pointers to Vaxis and our TTY, then init the instance. Doing so
// installs a signal handler for SIGWINCH on posix TTYs
//
// This event loop is thread safe. It reads the tty in a separate thread
var loop: vaxis.Loop(Event) = .{
.tty = &tty,
.vaxis = &vx,
};
try loop.init();
// Start the read loop. This puts the terminal in raw mode and begins
// reading user input
try loop.start();
defer loop.stop();
// Optionally enter the alternate screen
try vx.enterAltScreen(tty.anyWriter());
// We'll adjust the color index every keypress for the border
var color_idx: u8 = 0;
// init our text input widget. The text input widget needs an allocator to
// store the contents of the input
var text_input = TextInput.init(alloc, &vx.unicode);
defer text_input.deinit();
// Sends queries to terminal to detect certain features. This should always
// be called after entering the alt screen, if you are using the alt screen
try vx.queryTerminal(tty.anyWriter(), 1 * std.time.ns_per_s);
while (true) {
// nextEvent blocks until an event is in the queue
const event = loop.nextEvent();
// exhaustive switching ftw. Vaxis will send events if your Event enum
// has the fields for those events (ie "key_press", "winsize")
switch (event) {
.key_press => |key| {
color_idx = switch (color_idx) {
255 => 0,
else => color_idx + 1,
};
if (key.matches('c', .{ .ctrl = true })) {
break;
} else if (key.matches('l', .{ .ctrl = true })) {
vx.queueRefresh();
} else {
try text_input.update(.{ .key_press = key });
}
},
// winsize events are sent to the application to ensure that all
// resizes occur in the main thread. This lets us avoid expensive
// locks on the screen. All applications must handle this event
// unless they aren't using a screen (IE only detecting features)
//
// The allocations are because we keep a copy of each cell to
// optimize renders. When resize is called, we allocated two slices:
// one for the screen, and one for our buffered screen. Each cell in
// the buffered screen contains an ArrayList(u8) to be able to store
// the grapheme for that cell. Each cell is initialized with a size
// of 1, which is sufficient for all of ASCII. Anything requiring
// more than one byte will incur an allocation on the first render
// after it is drawn. Thereafter, it will not allocate unless the
// screen is resized
.winsize => |ws| try vx.resize(alloc, tty.anyWriter(), ws),
else => {},
}
// vx.window() returns the root window. This window is the size of the
// terminal and can spawn child windows as logical areas. Child windows
// cannot draw outside of their bounds
const win = vx.window();
// Clear the entire space because we are drawing in immediate mode.
// vaxis double buffers the screen. This new frame will be compared to
// the old and only updated cells will be drawn
win.clear();
// Create a style
const style: vaxis.Style = .{
.fg = .{ .index = color_idx },
};
// Create a bordered child window
const child = win.child(.{
.x_off = win.width / 2 - 20,
.y_off = win.height / 2 - 3,
.width = .{ .limit = 40 },
.height = .{ .limit = 3 },
.border = .{
.where = .all,
.style = style,
},
});
// Draw the text_input in the child window
text_input.draw(child);
// Render the screen. Using a buffered writer will offer much better
// performance, but is not required
try vx.render(tty.anyWriter());
}
}
```
## Contributing
Contributions are welcome. Please submit a PR on Github or a patch on the
[mailing list](mailto:~rockorager/[email protected])
|
0 | repos | repos/libvaxis/build.zig.zon | .{
.name = "vaxis",
.version = "0.1.0",
.minimum_zig_version = "0.13.0",
.dependencies = .{
.zigimg = .{
.url = "git+https://github.com/zigimg/zigimg#3a667bdb3d7f0955a5a51c8468eac83210c1439e",
.hash = "1220dd654ef941fc76fd96f9ec6adadf83f69b9887a0d3f4ee5ac0a1a3e11be35cf5",
.lazy = true,
},
.zg = .{
.url = "git+https://codeberg.org/dude_the_builder/zg?ref=master#689ab6b83d08c02724b99d199d650ff731250998",
.hash = "12200d1ce5f9733a9437415d85665ad5fbc85a4d27689fd337fecad8014acffe3aa5",
},
.libxev = .{
.url = "git+https://github.com/mitchellh/libxev#f6a672a78436d8efee1aa847a43a900ad773618b",
.hash = "12207b7a5b538ffb7fb18f954ae17d2f8490b6e3778a9e30564ad82c58ee8da52361",
.lazy = true,
},
.aio = .{
.url = "git+https://github.com/Cloudef/zig-aio#b5a407344379508466c5dcbe4c74438a6166e2ca",
.hash = "1220a55aedabdd10578d0c514719ea39ae1bc6d7ed990f508dc100db7f0ccf391437",
.lazy = true,
},
},
.paths = .{
"LICENSE",
"build.zig",
"build.zig.zon",
"src",
},
}
|
0 | repos | repos/libvaxis/build.zig | const std = @import("std");
pub fn build(b: *std.Build) void {
const include_libxev = b.option(bool, "libxev", "Enable support for libxev library (default: true)") orelse true;
const include_images = b.option(bool, "images", "Enable support for images (default: true)") orelse true;
const include_aio = b.option(bool, "aio", "Enable support for zig-aio library (default: false)") orelse false;
const options = b.addOptions();
options.addOption(bool, "libxev", include_libxev);
options.addOption(bool, "images", include_images);
options.addOption(bool, "aio", include_aio);
const options_mod = options.createModule();
const target = b.standardTargetOptions(.{});
const optimize = b.standardOptimizeOption(.{});
const root_source_file = b.path("src/main.zig");
// Dependencies
const zg_dep = b.dependency("zg", .{
.optimize = optimize,
.target = target,
});
const zigimg_dep = if (include_images) b.lazyDependency("zigimg", .{
.optimize = optimize,
.target = target,
}) else null;
const xev_dep = if (include_libxev) b.lazyDependency("libxev", .{
.optimize = optimize,
.target = target,
}) else null;
const aio_dep = if (include_aio) b.lazyDependency("aio", .{
.optimize = optimize,
.target = target,
}) else null;
// Module
const vaxis_mod = b.addModule("vaxis", .{
.root_source_file = root_source_file,
.target = target,
.optimize = optimize,
});
vaxis_mod.addImport("code_point", zg_dep.module("code_point"));
vaxis_mod.addImport("grapheme", zg_dep.module("grapheme"));
vaxis_mod.addImport("DisplayWidth", zg_dep.module("DisplayWidth"));
if (zigimg_dep) |dep| vaxis_mod.addImport("zigimg", dep.module("zigimg"));
if (xev_dep) |dep| vaxis_mod.addImport("xev", dep.module("xev"));
if (aio_dep) |dep| vaxis_mod.addImport("aio", dep.module("aio"));
if (aio_dep) |dep| vaxis_mod.addImport("coro", dep.module("coro"));
vaxis_mod.addImport("build_options", options_mod);
// Examples
const Example = enum {
cli,
image,
main,
nvim,
table,
text_input,
vaxis,
vt,
xev,
aio,
};
const example_option = b.option(Example, "example", "Example to run (default: text_input)") orelse .text_input;
const example_step = b.step("example", "Run example");
const example = b.addExecutable(.{
.name = "example",
// future versions should use b.path, see zig PR #19597
.root_source_file = b.path(
b.fmt("examples/{s}.zig", .{@tagName(example_option)}),
),
.target = target,
.optimize = optimize,
});
example.root_module.addImport("vaxis", vaxis_mod);
if (xev_dep) |dep| example.root_module.addImport("xev", dep.module("xev"));
if (aio_dep) |dep| example.root_module.addImport("aio", dep.module("aio"));
if (aio_dep) |dep| example.root_module.addImport("coro", dep.module("coro"));
const example_run = b.addRunArtifact(example);
example_step.dependOn(&example_run.step);
// Tests
const tests_step = b.step("test", "Run tests");
const tests = b.addTest(.{
.root_source_file = b.path("src/main.zig"),
.target = target,
.optimize = optimize,
});
tests.root_module.addImport("code_point", zg_dep.module("code_point"));
tests.root_module.addImport("grapheme", zg_dep.module("grapheme"));
tests.root_module.addImport("DisplayWidth", zg_dep.module("DisplayWidth"));
if (zigimg_dep) |dep| tests.root_module.addImport("zigimg", dep.module("zigimg"));
tests.root_module.addImport("build_options", options_mod);
const tests_run = b.addRunArtifact(tests);
b.installArtifact(tests);
tests_step.dependOn(&tests_run.step);
// Lints
const lints_step = b.step("lint", "Run lints");
const lints = b.addFmt(.{
.paths = &.{ "src", "build.zig" },
.check = true,
});
lints_step.dependOn(&lints.step);
b.default_step.dependOn(lints_step);
// Docs
const docs_step = b.step("docs", "Build the vaxis library docs");
const docs_obj = b.addObject(.{
.name = "vaxis",
.root_source_file = root_source_file,
.target = target,
.optimize = optimize,
});
const docs = docs_obj.getEmittedDocs();
docs_step.dependOn(&b.addInstallDirectory(.{
.source_dir = docs,
.install_dir = .prefix,
.install_subdir = "docs",
}).step);
}
|
0 | repos/libvaxis | repos/libvaxis/src/GraphemeCache.zig | const std = @import("std");
const GraphemeCache = @This();
/// the underlying storage for graphemes. Right now 8kb
buf: [1024 * 8]u8 = undefined,
// the start index of the next grapheme
idx: usize = 0,
/// put a slice of bytes in the cache as a grapheme
pub fn put(self: *GraphemeCache, bytes: []const u8) []u8 {
// reset the idx to 0 if we would overflow
if (self.idx + bytes.len > self.buf.len) self.idx = 0;
defer self.idx += bytes.len;
// copy the grapheme to our storage
@memcpy(self.buf[self.idx .. self.idx + bytes.len], bytes);
// return the slice
return self.buf[self.idx .. self.idx + bytes.len];
}
|
0 | repos/libvaxis | repos/libvaxis/src/Screen.zig | const std = @import("std");
const assert = std.debug.assert;
const Cell = @import("Cell.zig");
const Shape = @import("Mouse.zig").Shape;
const Image = @import("Image.zig");
const Winsize = @import("main.zig").Winsize;
const Unicode = @import("Unicode.zig");
const Method = @import("gwidth.zig").Method;
const Screen = @This();
width: usize = 0,
height: usize = 0,
width_pix: usize = 0,
height_pix: usize = 0,
buf: []Cell = undefined,
cursor_row: usize = 0,
cursor_col: usize = 0,
cursor_vis: bool = false,
unicode: *const Unicode = undefined,
width_method: Method = .wcwidth,
mouse_shape: Shape = .default,
cursor_shape: Cell.CursorShape = .default,
pub fn init(alloc: std.mem.Allocator, winsize: Winsize, unicode: *const Unicode) !Screen {
const w = winsize.cols;
const h = winsize.rows;
const self = Screen{
.buf = try alloc.alloc(Cell, w * h),
.width = w,
.height = h,
.width_pix = winsize.x_pixel,
.height_pix = winsize.y_pixel,
.unicode = unicode,
};
const base_cell: Cell = .{};
@memset(self.buf, base_cell);
return self;
}
pub fn deinit(self: *Screen, alloc: std.mem.Allocator) void {
alloc.free(self.buf);
}
/// writes a cell to a location. 0 indexed
pub fn writeCell(self: *Screen, col: usize, row: usize, cell: Cell) void {
if (self.width <= col) {
// column out of bounds
return;
}
if (self.height <= row) {
// height out of bounds
return;
}
const i = (row * self.width) + col;
assert(i < self.buf.len);
self.buf[i] = cell;
}
pub fn readCell(self: *Screen, col: usize, row: usize) ?Cell {
if (self.width <= col) {
// column out of bounds
return null;
}
if (self.height <= row) {
// height out of bounds
return null;
}
const i = (row * self.width) + col;
assert(i < self.buf.len);
return self.buf[i];
}
|
0 | repos/libvaxis | repos/libvaxis/src/Window.zig | const std = @import("std");
const Screen = @import("Screen.zig");
const Cell = @import("Cell.zig");
const Mouse = @import("Mouse.zig");
const Segment = @import("Cell.zig").Segment;
const Unicode = @import("Unicode.zig");
const gw = @import("gwidth.zig");
const Window = @This();
pub const Size = union(enum) {
expand,
limit: usize,
};
/// horizontal offset from the screen
x_off: usize,
/// vertical offset from the screen
y_off: usize,
/// width of the window. This can't be larger than the terminal screen
width: usize,
/// height of the window. This can't be larger than the terminal screen
height: usize,
screen: *Screen,
/// Deprecated. Use `child` instead
///
/// Creates a new window with offset relative to parent and size clamped to the
/// parent's size. Windows do not retain a reference to their parent and are
/// unaware of resizes.
pub fn initChild(
self: Window,
x_off: usize,
y_off: usize,
width: Size,
height: Size,
) Window {
const resolved_width = switch (width) {
.expand => self.width -| x_off,
.limit => |w| blk: {
if (w + x_off > self.width) {
break :blk self.width -| x_off;
}
break :blk w;
},
};
const resolved_height = switch (height) {
.expand => self.height -| y_off,
.limit => |h| blk: {
if (h + y_off > self.height) {
break :blk self.height -| y_off;
}
break :blk h;
},
};
return Window{
.x_off = x_off + self.x_off,
.y_off = y_off + self.y_off,
.width = resolved_width,
.height = resolved_height,
.screen = self.screen,
};
}
pub const ChildOptions = struct {
x_off: usize = 0,
y_off: usize = 0,
/// the width of the resulting child, including any borders
width: Size = .expand,
/// the height of the resulting child, including any borders
height: Size = .expand,
border: BorderOptions = .{},
};
pub const BorderOptions = struct {
style: Cell.Style = .{},
where: union(enum) {
none,
all,
top,
right,
bottom,
left,
other: Locations,
} = .none,
glyphs: Glyphs = .single_rounded,
pub const Locations = packed struct {
top: bool = false,
right: bool = false,
bottom: bool = false,
left: bool = false,
};
pub const Glyphs = union(enum) {
single_rounded,
single_square,
/// custom border glyphs. each glyph should be one cell wide and the
/// following indices apply:
/// [0] = top left
/// [1] = horizontal
/// [2] = top right
/// [3] = vertical
/// [4] = bottom right
/// [5] = bottom left
custom: [6][]const u8,
};
const single_rounded: [6][]const u8 = .{ "╭", "─", "╮", "│", "╯", "╰" };
const single_square: [6][]const u8 = .{ "┌", "─", "┐", "│", "┘", "└" };
};
/// create a child window
pub fn child(self: Window, opts: ChildOptions) Window {
var result = self.initChild(opts.x_off, opts.y_off, opts.width, opts.height);
const glyphs = switch (opts.border.glyphs) {
.single_rounded => BorderOptions.single_rounded,
.single_square => BorderOptions.single_square,
.custom => |custom| custom,
};
const top_left: Cell.Character = .{ .grapheme = glyphs[0], .width = 1 };
const horizontal: Cell.Character = .{ .grapheme = glyphs[1], .width = 1 };
const top_right: Cell.Character = .{ .grapheme = glyphs[2], .width = 1 };
const vertical: Cell.Character = .{ .grapheme = glyphs[3], .width = 1 };
const bottom_right: Cell.Character = .{ .grapheme = glyphs[4], .width = 1 };
const bottom_left: Cell.Character = .{ .grapheme = glyphs[5], .width = 1 };
const style = opts.border.style;
const h = result.height;
const w = result.width;
const loc: BorderOptions.Locations = switch (opts.border.where) {
.none => return result,
.all => .{ .top = true, .bottom = true, .right = true, .left = true },
.bottom => .{ .bottom = true },
.right => .{ .right = true },
.left => .{ .left = true },
.top => .{ .top = true },
.other => |loc| loc,
};
if (loc.top) {
var i: usize = 0;
while (i < w) : (i += 1) {
result.writeCell(i, 0, .{ .char = horizontal, .style = style });
}
}
if (loc.bottom) {
var i: usize = 0;
while (i < w) : (i += 1) {
result.writeCell(i, h -| 1, .{ .char = horizontal, .style = style });
}
}
if (loc.left) {
var i: usize = 0;
while (i < h) : (i += 1) {
result.writeCell(0, i, .{ .char = vertical, .style = style });
}
}
if (loc.right) {
var i: usize = 0;
while (i < h) : (i += 1) {
result.writeCell(w -| 1, i, .{ .char = vertical, .style = style });
}
}
// draw corners
if (loc.top and loc.left)
result.writeCell(0, 0, .{ .char = top_left, .style = style });
if (loc.top and loc.right)
result.writeCell(w - 1, 0, .{ .char = top_right, .style = style });
if (loc.bottom and loc.left)
result.writeCell(0, h -| 1, .{ .char = bottom_left, .style = style });
if (loc.bottom and loc.right)
result.writeCell(w - 1, h -| 1, .{ .char = bottom_right, .style = style });
const x_off: usize = if (loc.left) 1 else 0;
const y_off: usize = if (loc.top) 1 else 0;
const h_delt: usize = if (loc.bottom) 1 else 0;
const w_delt: usize = if (loc.right) 1 else 0;
const h_ch: usize = h -| y_off -| h_delt;
const w_ch: usize = w -| x_off -| w_delt;
return result.initChild(x_off, y_off, .{ .limit = w_ch }, .{ .limit = h_ch });
}
/// writes a cell to the location in the window
pub fn writeCell(self: Window, col: usize, row: usize, cell: Cell) void {
if (self.height == 0 or self.width == 0) return;
if (self.height <= row or self.width <= col) return;
self.screen.writeCell(col + self.x_off, row + self.y_off, cell);
}
/// reads a cell at the location in the window
pub fn readCell(self: Window, col: usize, row: usize) ?Cell {
if (self.height == 0 or self.width == 0) return null;
if (self.height <= row or self.width <= col) return null;
return self.screen.readCell(col + self.x_off, row + self.y_off);
}
/// fills the window with the default cell
pub fn clear(self: Window) void {
self.fill(.{ .default = true });
}
/// returns the width of the grapheme. This depends on the terminal capabilities
pub fn gwidth(self: Window, str: []const u8) usize {
return gw.gwidth(str, self.screen.width_method, &self.screen.unicode.width_data) catch 1;
}
/// fills the window with the provided cell
pub fn fill(self: Window, cell: Cell) void {
if (self.screen.width < self.x_off)
return;
if (self.screen.height < self.y_off)
return;
if (self.x_off == 0 and self.width == self.screen.width) {
// we have a full width window, therefore contiguous memory.
const start = @min(self.y_off * self.width, self.screen.buf.len);
const end = @min(start + (self.height * self.width), self.screen.buf.len);
@memset(self.screen.buf[start..end], cell);
} else {
// Non-contiguous. Iterate over rows an memset
var row: usize = self.y_off;
const last_row = @min(self.height + self.y_off, self.screen.height);
while (row < last_row) : (row += 1) {
const start = @min(self.x_off + (row * self.screen.width), self.screen.buf.len);
var end = @min(start + self.width, start + (self.screen.width - self.x_off));
end = @min(end, self.screen.buf.len);
@memset(self.screen.buf[start..end], cell);
}
}
}
/// hide the cursor
pub fn hideCursor(self: Window) void {
self.screen.cursor_vis = false;
}
/// show the cursor at the given coordinates, 0 indexed
pub fn showCursor(self: Window, col: usize, row: usize) void {
if (self.height == 0 or self.width == 0) return;
if (self.height <= row or self.width <= col) return;
self.screen.cursor_vis = true;
self.screen.cursor_row = row + self.y_off;
self.screen.cursor_col = col + self.x_off;
}
pub fn setCursorShape(self: Window, shape: Cell.CursorShape) void {
self.screen.cursor_shape = shape;
}
/// Options to use when printing Segments to a window
pub const PrintOptions = struct {
/// vertical offset to start printing at
row_offset: usize = 0,
/// horizontal offset to start printing at
col_offset: usize = 0,
/// wrap behavior for printing
wrap: enum {
/// wrap at grapheme boundaries
grapheme,
/// wrap at word boundaries
word,
/// stop printing after one line
none,
} = .grapheme,
/// when true, print will write to the screen for rendering. When false,
/// nothing is written. The return value describes the size of the wrapped
/// text
commit: bool = true,
};
pub const PrintResult = struct {
col: usize,
row: usize,
overflow: bool,
};
/// prints segments to the window. returns true if the text overflowed with the
/// given wrap strategy and size.
pub fn print(self: Window, segments: []const Segment, opts: PrintOptions) !PrintResult {
var row = opts.row_offset;
switch (opts.wrap) {
.grapheme => {
var col: usize = opts.col_offset;
const overflow: bool = blk: for (segments) |segment| {
var iter = self.screen.unicode.graphemeIterator(segment.text);
while (iter.next()) |grapheme| {
if (col >= self.width) {
row += 1;
col = 0;
}
if (row >= self.height) break :blk true;
const s = grapheme.bytes(segment.text);
if (std.mem.eql(u8, s, "\n")) {
row +|= 1;
col = 0;
continue;
}
const w = self.gwidth(s);
if (w == 0) continue;
if (opts.commit) self.writeCell(col, row, .{
.char = .{
.grapheme = s,
.width = w,
},
.style = segment.style,
.link = segment.link,
.wrapped = col + w >= self.width,
});
col += w;
}
} else false;
if (col >= self.width) {
row += 1;
col = 0;
}
return .{
.row = row,
.col = col,
.overflow = overflow,
};
},
.word => {
var col: usize = opts.col_offset;
var overflow: bool = false;
var soft_wrapped: bool = false;
outer: for (segments) |segment| {
var line_iter: LineIterator = .{ .buf = segment.text };
while (line_iter.next()) |line| {
defer {
// We only set soft_wrapped to false if a segment actually contains a linebreak
if (line_iter.has_break) {
soft_wrapped = false;
row += 1;
col = 0;
}
}
var iter: WhitespaceTokenizer = .{ .buf = line };
while (iter.next()) |token| {
switch (token) {
.whitespace => |len| {
if (soft_wrapped) continue;
for (0..len) |_| {
if (col >= self.width) {
col = 0;
row += 1;
break;
}
if (opts.commit) {
self.writeCell(col, row, .{
.char = .{
.grapheme = " ",
.width = 1,
},
.style = segment.style,
.link = segment.link,
});
}
col += 1;
}
},
.word => |word| {
const width = self.gwidth(word);
if (width + col > self.width and width < self.width) {
row += 1;
col = 0;
}
var grapheme_iterator = self.screen.unicode.graphemeIterator(word);
while (grapheme_iterator.next()) |grapheme| {
soft_wrapped = false;
if (row >= self.height) {
overflow = true;
break :outer;
}
const s = grapheme.bytes(word);
const w = self.gwidth(s);
if (opts.commit) self.writeCell(col, row, .{
.char = .{
.grapheme = s,
.width = w,
},
.style = segment.style,
.link = segment.link,
});
col += w;
if (col >= self.width) {
row += 1;
col = 0;
soft_wrapped = true;
}
}
},
}
}
}
}
return .{
// remove last row counter
.row = row,
.col = col,
.overflow = overflow,
};
},
.none => {
var col: usize = opts.col_offset;
const overflow: bool = blk: for (segments) |segment| {
var iter = self.screen.unicode.graphemeIterator(segment.text);
while (iter.next()) |grapheme| {
if (col >= self.width) break :blk true;
const s = grapheme.bytes(segment.text);
if (std.mem.eql(u8, s, "\n")) break :blk true;
const w = self.gwidth(s);
if (w == 0) continue;
if (opts.commit) self.writeCell(col, row, .{
.char = .{
.grapheme = s,
.width = w,
},
.style = segment.style,
.link = segment.link,
});
col +|= w;
}
} else false;
return .{
.row = row,
.col = col,
.overflow = overflow,
};
},
}
return false;
}
/// print a single segment. This is just a shortcut for print(&.{segment}, opts)
pub fn printSegment(self: Window, segment: Segment, opts: PrintOptions) !PrintResult {
return self.print(&.{segment}, opts);
}
/// scrolls the window down one row (IE inserts a blank row at the bottom of the
/// screen and shifts all rows up one)
pub fn scroll(self: Window, n: usize) void {
if (n > self.height) return;
var row = self.y_off;
while (row < self.height - n) : (row += 1) {
const dst_start = (row * self.width) + self.x_off;
const dst_end = dst_start + self.width;
const src_start = ((row + n) * self.width) + self.x_off;
const src_end = src_start + self.width;
@memcpy(self.screen.buf[dst_start..dst_end], self.screen.buf[src_start..src_end]);
}
const last_row = self.child(.{
.y_off = self.height - n,
});
last_row.clear();
}
/// returns the mouse event if the mouse event occurred within the window. If
/// the mouse event occurred outside the window, null is returned
pub fn hasMouse(win: Window, mouse: ?Mouse) ?Mouse {
const event = mouse orelse return null;
if (event.col >= win.x_off and
event.col < (win.x_off + win.width) and
event.row >= win.y_off and
event.row < (win.y_off + win.height)) return event else return null;
}
test "Window size set" {
var parent = Window{
.x_off = 0,
.y_off = 0,
.width = 20,
.height = 20,
.screen = undefined,
};
const ch = parent.initChild(1, 1, .expand, .expand);
try std.testing.expectEqual(19, ch.width);
try std.testing.expectEqual(19, ch.height);
}
test "Window size set too big" {
var parent = Window{
.x_off = 0,
.y_off = 0,
.width = 20,
.height = 20,
.screen = undefined,
};
const ch = parent.initChild(0, 0, .{ .limit = 21 }, .{ .limit = 21 });
try std.testing.expectEqual(20, ch.width);
try std.testing.expectEqual(20, ch.height);
}
test "Window size set too big with offset" {
var parent = Window{
.x_off = 0,
.y_off = 0,
.width = 20,
.height = 20,
.screen = undefined,
};
const ch = parent.initChild(10, 10, .{ .limit = 21 }, .{ .limit = 21 });
try std.testing.expectEqual(10, ch.width);
try std.testing.expectEqual(10, ch.height);
}
test "Window size nested offsets" {
var parent = Window{
.x_off = 1,
.y_off = 1,
.width = 20,
.height = 20,
.screen = undefined,
};
const ch = parent.initChild(10, 10, .{ .limit = 21 }, .{ .limit = 21 });
try std.testing.expectEqual(11, ch.x_off);
try std.testing.expectEqual(11, ch.y_off);
}
test "print: grapheme" {
const alloc = std.testing.allocator_instance.allocator();
const unicode = try Unicode.init(alloc);
defer unicode.deinit();
var screen: Screen = .{ .width_method = .unicode, .unicode = &unicode };
const win: Window = .{
.x_off = 0,
.y_off = 0,
.width = 4,
.height = 2,
.screen = &screen,
};
const opts: PrintOptions = .{
.commit = false,
.wrap = .grapheme,
};
{
var segments = [_]Segment{
.{ .text = "a" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(1, result.col);
try std.testing.expectEqual(0, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "abcd" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(0, result.col);
try std.testing.expectEqual(1, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "abcde" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(1, result.col);
try std.testing.expectEqual(1, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "abcdefgh" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(0, result.col);
try std.testing.expectEqual(2, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "abcdefghi" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(0, result.col);
try std.testing.expectEqual(2, result.row);
try std.testing.expectEqual(true, result.overflow);
}
}
test "print: word" {
const alloc = std.testing.allocator_instance.allocator();
const unicode = try Unicode.init(alloc);
defer unicode.deinit();
var screen: Screen = .{
.width_method = .unicode,
.unicode = &unicode,
};
const win: Window = .{
.x_off = 0,
.y_off = 0,
.width = 4,
.height = 2,
.screen = &screen,
};
const opts: PrintOptions = .{
.commit = false,
.wrap = .word,
};
{
var segments = [_]Segment{
.{ .text = "a" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(1, result.col);
try std.testing.expectEqual(0, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = " " },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(1, result.col);
try std.testing.expectEqual(0, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = " a" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(2, result.col);
try std.testing.expectEqual(0, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "a b" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(3, result.col);
try std.testing.expectEqual(0, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "a b c" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(1, result.col);
try std.testing.expectEqual(1, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "hello" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(1, result.col);
try std.testing.expectEqual(1, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "hi tim" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(3, result.col);
try std.testing.expectEqual(1, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "hello tim" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(0, result.col);
try std.testing.expectEqual(2, result.row);
try std.testing.expectEqual(true, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "hello ti" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(0, result.col);
try std.testing.expectEqual(2, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "h" },
.{ .text = "e" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(2, result.col);
try std.testing.expectEqual(0, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "h" },
.{ .text = "e" },
.{ .text = "l" },
.{ .text = "l" },
.{ .text = "o" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(1, result.col);
try std.testing.expectEqual(1, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "he\n" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(0, result.col);
try std.testing.expectEqual(1, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "he\n\n" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(0, result.col);
try std.testing.expectEqual(2, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "not now" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(3, result.col);
try std.testing.expectEqual(1, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "note now" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(3, result.col);
try std.testing.expectEqual(1, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "note" },
.{ .text = " now" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(3, result.col);
try std.testing.expectEqual(1, result.row);
try std.testing.expectEqual(false, result.overflow);
}
{
var segments = [_]Segment{
.{ .text = "note " },
.{ .text = "now" },
};
const result = try win.print(&segments, opts);
try std.testing.expectEqual(3, result.col);
try std.testing.expectEqual(1, result.row);
try std.testing.expectEqual(false, result.overflow);
}
}
/// Iterates a slice of bytes by linebreaks. Lines are split by '\r', '\n', or '\r\n'
const LineIterator = struct {
buf: []const u8,
index: usize = 0,
has_break: bool = true,
fn next(self: *LineIterator) ?[]const u8 {
if (self.index >= self.buf.len) return null;
const start = self.index;
const end = std.mem.indexOfAnyPos(u8, self.buf, self.index, "\r\n") orelse {
if (start == 0) self.has_break = false;
self.index = self.buf.len;
return self.buf[start..];
};
self.index = end;
self.consumeCR();
self.consumeLF();
return self.buf[start..end];
}
// consumes a \n byte
fn consumeLF(self: *LineIterator) void {
if (self.index >= self.buf.len) return;
if (self.buf[self.index] == '\n') self.index += 1;
}
// consumes a \r byte
fn consumeCR(self: *LineIterator) void {
if (self.index >= self.buf.len) return;
if (self.buf[self.index] == '\r') self.index += 1;
}
};
/// Returns tokens of text and whitespace
const WhitespaceTokenizer = struct {
buf: []const u8,
index: usize = 0,
const Token = union(enum) {
// the length of whitespace. Tab = 8
whitespace: usize,
word: []const u8,
};
fn next(self: *WhitespaceTokenizer) ?Token {
if (self.index >= self.buf.len) return null;
const Mode = enum {
whitespace,
word,
};
const first = self.buf[self.index];
const mode: Mode = if (first == ' ' or first == '\t') .whitespace else .word;
switch (mode) {
.whitespace => {
var len: usize = 0;
while (self.index < self.buf.len) : (self.index += 1) {
switch (self.buf[self.index]) {
' ' => len += 1,
'\t' => len += 8,
else => break,
}
}
return .{ .whitespace = len };
},
.word => {
const start = self.index;
while (self.index < self.buf.len) : (self.index += 1) {
switch (self.buf[self.index]) {
' ', '\t' => break,
else => {},
}
}
return .{ .word = self.buf[start..self.index] };
},
}
}
};
|
0 | repos/libvaxis | repos/libvaxis/src/event.zig | pub const Key = @import("Key.zig");
pub const Mouse = @import("Mouse.zig");
pub const Color = @import("Cell.zig").Color;
pub const Winsize = @import("main.zig").Winsize;
/// The events that Vaxis emits internally
pub const Event = union(enum) {
key_press: Key,
key_release: Key,
mouse: Mouse,
focus_in,
focus_out,
paste_start, // bracketed paste start
paste_end, // bracketed paste end
paste: []const u8, // osc 52 paste, caller must free
color_report: Color.Report, // osc 4, 10, 11, 12 response
color_scheme: Color.Scheme,
winsize: Winsize,
// these are delivered as discovered terminal capabilities
cap_kitty_keyboard,
cap_kitty_graphics,
cap_rgb,
cap_sgr_pixels,
cap_unicode,
cap_da1,
cap_color_scheme_updates,
};
|
0 | repos/libvaxis | repos/libvaxis/src/Loop.zig | const std = @import("std");
const builtin = @import("builtin");
const grapheme = @import("grapheme");
const GraphemeCache = @import("GraphemeCache.zig");
const Parser = @import("Parser.zig");
const Queue = @import("queue.zig").Queue;
const vaxis = @import("main.zig");
const Tty = vaxis.Tty;
const Vaxis = @import("Vaxis.zig");
const log = std.log.scoped(.vaxis);
pub fn Loop(comptime T: type) type {
return struct {
const Self = @This();
const Event = T;
tty: *Tty,
vaxis: *Vaxis,
queue: Queue(T, 512) = .{},
thread: ?std.Thread = null,
should_quit: bool = false,
/// Initialize the event loop. This is an intrusive init so that we have
/// a stable pointer to register signal callbacks with posix TTYs
pub fn init(self: *Self) !void {
switch (builtin.os.tag) {
.windows => {},
else => {
const handler: Tty.SignalHandler = .{
.context = self,
.callback = Self.winsizeCallback,
};
try Tty.notifyWinsize(handler);
},
}
}
/// spawns the input thread to read input from the tty
pub fn start(self: *Self) !void {
if (self.thread) |_| return;
self.thread = try std.Thread.spawn(.{}, Self.ttyRun, .{
self,
&self.vaxis.unicode.grapheme_data,
self.vaxis.opts.system_clipboard_allocator,
});
}
/// stops reading from the tty.
pub fn stop(self: *Self) void {
// If we don't have a thread, we have nothing to stop
if (self.thread == null) return;
self.should_quit = true;
// trigger a read
self.vaxis.deviceStatusReport(self.tty.anyWriter()) catch {};
if (self.thread) |thread| {
thread.join();
self.thread = null;
self.should_quit = false;
}
}
/// returns the next available event, blocking until one is available
pub fn nextEvent(self: *Self) T {
return self.queue.pop();
}
/// blocks until an event is available. Useful when your application is
/// operating on a poll + drain architecture (see tryEvent)
pub fn pollEvent(self: *Self) void {
self.queue.poll();
}
/// returns an event if one is available, otherwise null. Non-blocking.
pub fn tryEvent(self: *Self) ?T {
return self.queue.tryPop();
}
/// posts an event into the event queue. Will block if there is not
/// capacity for the event
pub fn postEvent(self: *Self, event: T) void {
self.queue.push(event);
}
pub fn tryPostEvent(self: *Self, event: T) bool {
return self.queue.tryPush(event);
}
pub fn winsizeCallback(ptr: *anyopaque) void {
const self: *Self = @ptrCast(@alignCast(ptr));
// We will be receiving winsize updates in-band
if (self.vaxis.state.in_band_resize) return;
const winsize = Tty.getWinsize(self.tty.fd) catch return;
if (@hasField(Event, "winsize")) {
self.postEvent(.{ .winsize = winsize });
}
}
/// read input from the tty. This is run in a separate thread
fn ttyRun(
self: *Self,
grapheme_data: *const grapheme.GraphemeData,
paste_allocator: ?std.mem.Allocator,
) !void {
// initialize a grapheme cache
var cache: GraphemeCache = .{};
switch (builtin.os.tag) {
.windows => {
var parser: Parser = .{
.grapheme_data = grapheme_data,
};
while (!self.should_quit) {
const event = try self.tty.nextEvent(&parser, paste_allocator);
try handleEventGeneric(self, self.vaxis, &cache, Event, event, null);
}
},
else => {
// get our initial winsize
const winsize = try Tty.getWinsize(self.tty.fd);
if (@hasField(Event, "winsize")) {
self.postEvent(.{ .winsize = winsize });
}
var parser: Parser = .{
.grapheme_data = grapheme_data,
};
// initialize the read buffer
var buf: [1024]u8 = undefined;
var read_start: usize = 0;
// read loop
read_loop: while (!self.should_quit) {
const n = try self.tty.read(buf[read_start..]);
var seq_start: usize = 0;
while (seq_start < n) {
const result = try parser.parse(buf[seq_start..n], paste_allocator);
if (result.n == 0) {
// copy the read to the beginning. We don't use memcpy because
// this could be overlapping, and it's also rare
const initial_start = seq_start;
while (seq_start < n) : (seq_start += 1) {
buf[seq_start - initial_start] = buf[seq_start];
}
read_start = seq_start - initial_start + 1;
continue :read_loop;
}
read_start = 0;
seq_start += result.n;
const event = result.event orelse continue;
try handleEventGeneric(self, self.vaxis, &cache, Event, event, paste_allocator);
}
}
},
}
}
};
}
// Use return on the self.postEvent's so it can either return error union or void
pub fn handleEventGeneric(self: anytype, vx: *Vaxis, cache: *GraphemeCache, Event: type, event: anytype, paste_allocator: ?std.mem.Allocator) !void {
switch (builtin.os.tag) {
.windows => {
switch (event) {
.winsize => |ws| {
if (@hasField(Event, "winsize")) {
return self.postEvent(.{ .winsize = ws });
}
},
.key_press => |key| {
if (@hasField(Event, "key_press")) {
// HACK: yuck. there has to be a better way
var mut_key = key;
if (key.text) |text| {
mut_key.text = cache.put(text);
}
return self.postEvent(.{ .key_press = mut_key });
}
},
.key_release => |key| {
if (@hasField(Event, "key_release")) {
// HACK: yuck. there has to be a better way
var mut_key = key;
if (key.text) |text| {
mut_key.text = cache.put(text);
}
return self.postEvent(.{ .key_release = mut_key });
}
},
.cap_da1 => {
std.Thread.Futex.wake(&vx.query_futex, 10);
},
.mouse => {}, // Unsupported currently
else => {},
}
},
else => {
switch (event) {
.key_press => |key| {
if (@hasField(Event, "key_press")) {
// HACK: yuck. there has to be a better way
var mut_key = key;
if (key.text) |text| {
mut_key.text = cache.put(text);
}
return self.postEvent(.{ .key_press = mut_key });
}
},
.key_release => |key| {
if (@hasField(Event, "key_release")) {
// HACK: yuck. there has to be a better way
var mut_key = key;
if (key.text) |text| {
mut_key.text = cache.put(text);
}
return self.postEvent(.{ .key_release = mut_key });
}
},
.mouse => |mouse| {
if (@hasField(Event, "mouse")) {
return self.postEvent(.{ .mouse = vx.translateMouse(mouse) });
}
},
.focus_in => {
if (@hasField(Event, "focus_in")) {
return self.postEvent(.focus_in);
}
},
.focus_out => {
if (@hasField(Event, "focus_out")) {
return self.postEvent(.focus_out);
}
},
.paste_start => {
if (@hasField(Event, "paste_start")) {
return self.postEvent(.paste_start);
}
},
.paste_end => {
if (@hasField(Event, "paste_end")) {
return self.postEvent(.paste_end);
}
},
.paste => |text| {
if (@hasField(Event, "paste")) {
return self.postEvent(.{ .paste = text });
} else {
if (paste_allocator) |_|
paste_allocator.?.free(text);
}
},
.color_report => |report| {
if (@hasField(Event, "color_report")) {
return self.postEvent(.{ .color_report = report });
}
},
.color_scheme => |scheme| {
if (@hasField(Event, "color_scheme")) {
return self.postEvent(.{ .color_scheme = scheme });
}
},
.cap_kitty_keyboard => {
log.info("kitty keyboard capability detected", .{});
vx.caps.kitty_keyboard = true;
},
.cap_kitty_graphics => {
if (!vx.caps.kitty_graphics) {
log.info("kitty graphics capability detected", .{});
vx.caps.kitty_graphics = true;
}
},
.cap_rgb => {
log.info("rgb capability detected", .{});
vx.caps.rgb = true;
},
.cap_unicode => {
log.info("unicode capability detected", .{});
vx.caps.unicode = .unicode;
vx.screen.width_method = .unicode;
},
.cap_sgr_pixels => {
log.info("pixel mouse capability detected", .{});
vx.caps.sgr_pixels = true;
},
.cap_color_scheme_updates => {
log.info("color_scheme_updates capability detected", .{});
vx.caps.color_scheme_updates = true;
},
.cap_da1 => {
std.Thread.Futex.wake(&vx.query_futex, 10);
},
.winsize => |winsize| {
vx.state.in_band_resize = true;
if (@hasField(Event, "winsize")) {
return self.postEvent(.{ .winsize = winsize });
}
},
}
},
}
}
|
0 | repos/libvaxis | repos/libvaxis/src/main.zig | const std = @import("std");
const builtin = @import("builtin");
const build_options = @import("build_options");
pub const Vaxis = @import("Vaxis.zig");
pub const Loop = @import("Loop.zig").Loop;
pub const xev = @import("xev.zig");
pub const aio = @import("aio.zig");
pub const zigimg = @import("zigimg");
pub const Queue = @import("queue.zig").Queue;
pub const Key = @import("Key.zig");
pub const Cell = @import("Cell.zig");
pub const Segment = Cell.Segment;
pub const PrintOptions = Window.PrintOptions;
pub const Style = Cell.Style;
pub const Color = Cell.Color;
pub const Image = @import("Image.zig");
pub const Mouse = @import("Mouse.zig");
pub const Screen = @import("Screen.zig");
pub const AllocatingScreen = @import("InternalScreen.zig");
pub const Parser = @import("Parser.zig");
pub const Window = @import("Window.zig");
pub const widgets = @import("widgets.zig");
pub const gwidth = @import("gwidth.zig");
pub const ctlseqs = @import("ctlseqs.zig");
pub const GraphemeCache = @import("GraphemeCache.zig");
pub const grapheme = @import("grapheme");
pub const Event = @import("event.zig").Event;
pub const Unicode = @import("Unicode.zig");
/// The target TTY implementation
pub const Tty = switch (builtin.os.tag) {
.windows => @import("windows/Tty.zig"),
else => @import("posix/Tty.zig"),
};
/// The size of the terminal screen
pub const Winsize = struct {
rows: usize,
cols: usize,
x_pixel: usize,
y_pixel: usize,
};
/// Initialize a Vaxis application.
pub fn init(alloc: std.mem.Allocator, opts: Vaxis.Options) !Vaxis {
return Vaxis.init(alloc, opts);
}
/// Resets terminal state on a panic, then calls the default zig panic handler
pub fn panic_handler(msg: []const u8, error_return_trace: ?*std.builtin.StackTrace, ret_addr: ?usize) noreturn {
if (Tty.global_tty) |gty| {
const reset: []const u8 = ctlseqs.csi_u_pop ++
ctlseqs.mouse_reset ++
ctlseqs.bp_reset ++
ctlseqs.rmcup;
gty.anyWriter().writeAll(reset) catch {};
gty.deinit();
}
std.builtin.default_panic(msg, error_return_trace, ret_addr);
}
pub const log_scopes = enum {
vaxis,
};
/// the vaxis logo. In PixelCode
pub const logo =
\\▄ ▄ ▄▄▄ ▄ ▄ ▄▄▄ ▄▄▄
\\█ █ █▄▄▄█ ▀▄ ▄▀ █ █ ▀
\\▀▄ ▄▀ █ █ ▄▀▄ █ ▀▀▀▄
\\ ▀▄▀ █ █ █ █ ▄█▄ ▀▄▄▄▀
;
test {
_ = @import("gwidth.zig");
_ = @import("Cell.zig");
_ = @import("Key.zig");
_ = @import("Parser.zig");
_ = @import("Window.zig");
_ = @import("gwidth.zig");
_ = @import("queue.zig");
_ = @import("widgets/TextInput.zig");
}
|
0 | repos/libvaxis | repos/libvaxis/src/Image.zig | const std = @import("std");
const fmt = std.fmt;
const math = std.math;
const base64 = std.base64.standard.Encoder;
const zigimg = @import("zigimg");
const Window = @import("Window.zig");
const Image = @This();
const transmit_opener = "\x1b_Gf=32,i={d},s={d},v={d},m={d};";
pub const Source = union(enum) {
path: []const u8,
mem: []const u8,
};
pub const TransmitFormat = enum {
rgb,
rgba,
png,
};
pub const TransmitMedium = enum {
file,
temp_file,
shared_mem,
};
pub const Placement = struct {
img_id: u32,
options: Image.DrawOptions,
};
pub const CellSize = struct {
rows: usize,
cols: usize,
};
pub const DrawOptions = struct {
/// an offset into the top left cell, in pixels, with where to place the
/// origin of the image. These must be less than the pixel size of a single
/// cell
pixel_offset: ?struct {
x: usize,
y: usize,
} = null,
/// the vertical stacking order
/// < 0: Drawn beneath text
/// < -1_073_741_824: Drawn beneath "default" background cells
z_index: ?i32 = null,
/// A clip region of the source image to draw.
clip_region: ?struct {
x: ?usize = null,
y: ?usize = null,
width: ?usize = null,
height: ?usize = null,
} = null,
/// Scaling to apply to the Image
scale: enum {
/// no scaling applied. the image may extend beyond the window
none,
/// Stretch / shrink the image to fill the window
fill,
/// Scale the image to fit the window, maintaining aspect ratio
fit,
/// Scale the image to fit the window, only if needed.
contain,
} = .none,
/// the size to render the image. Generally you will not need to use this
/// field, and should prefer to use scale. `draw` will fill in this field with
/// the correct values if a scale method is applied.
size: ?struct {
rows: ?usize = null,
cols: ?usize = null,
} = null,
};
/// unique identifier for this image. This will be managed by the screen.
id: u32,
/// width in pixels
width: usize,
/// height in pixels
height: usize,
pub fn draw(self: Image, win: Window, opts: DrawOptions) !void {
var p_opts = opts;
switch (opts.scale) {
.none => {},
.fill => {
p_opts.size = .{
.rows = win.height,
.cols = win.width,
};
},
.fit,
.contain,
=> contain: {
// cell geometry
const x_pix = win.screen.width_pix;
const y_pix = win.screen.height_pix;
const w = win.screen.width;
const h = win.screen.height;
const pix_per_col = try std.math.divCeil(usize, x_pix, w);
const pix_per_row = try std.math.divCeil(usize, y_pix, h);
const win_width_pix = pix_per_col * win.width;
const win_height_pix = pix_per_row * win.height;
const fit_x: bool = if (win_width_pix >= self.width) true else false;
const fit_y: bool = if (win_height_pix >= self.height) true else false;
// Does the image fit with no scaling?
if (opts.scale == .contain and fit_x and fit_y) break :contain;
// Does the image require vertical scaling?
if (fit_x and !fit_y)
p_opts.size = .{
.rows = win.height,
}
// Does the image require horizontal scaling?
else if (!fit_x and fit_y)
p_opts.size = .{
.cols = win.width,
}
else if (!fit_x and !fit_y) {
const diff_x = self.width - win_width_pix;
const diff_y = self.height - win_height_pix;
// The width difference is larger than the height difference.
// Scale by width
if (diff_x > diff_y)
p_opts.size = .{
.cols = win.width,
}
else
// The height difference is larger than the width difference.
// Scale by height
p_opts.size = .{
.rows = win.height,
};
} else {
std.debug.assert(opts.scale == .fit);
std.debug.assert(win_width_pix >= self.width);
std.debug.assert(win_height_pix >= self.height);
// Fits in both directions. Find the closer direction
const diff_x = win_width_pix - self.width;
const diff_y = win_height_pix - self.height;
// The width is closer in dimension. Scale by that
if (diff_x < diff_y)
p_opts.size = .{
.cols = win.width,
}
else
p_opts.size = .{
.rows = win.height,
};
}
},
}
const p = Placement{
.img_id = self.id,
.options = p_opts,
};
win.writeCell(0, 0, .{ .image = p });
}
/// the size of the image, in cells
pub fn cellSize(self: Image, win: Window) !CellSize {
// cell geometry
const x_pix = win.screen.width_pix;
const y_pix = win.screen.height_pix;
const w = win.screen.width;
const h = win.screen.height;
const pix_per_col = try std.math.divCeil(usize, x_pix, w);
const pix_per_row = try std.math.divCeil(usize, y_pix, h);
const cell_width = std.math.divCeil(usize, self.width, pix_per_col) catch 0;
const cell_height = std.math.divCeil(usize, self.height, pix_per_row) catch 0;
return .{
.rows = cell_height,
.cols = cell_width,
};
}
|
0 | repos/libvaxis | repos/libvaxis/src/widgets.zig | //! Specialized TUI Widgets
const opts = @import("build_options");
pub const border = @import("widgets/border.zig");
pub const alignment = @import("widgets/alignment.zig");
pub const Scrollbar = @import("widgets/Scrollbar.zig");
pub const Table = @import("widgets/Table.zig");
pub const ScrollView = @import("widgets/ScrollView.zig");
pub const LineNumbers = @import("widgets/LineNumbers.zig");
pub const TextView = @import("widgets/TextView.zig");
pub const CodeView = @import("widgets/CodeView.zig");
pub const Terminal = @import("widgets/terminal/Terminal.zig");
pub const TextInput = @import("widgets/TextInput.zig");
|
0 | repos/libvaxis | repos/libvaxis/src/Vaxis.zig | const std = @import("std");
const builtin = @import("builtin");
const atomic = std.atomic;
const base64Encoder = std.base64.standard.Encoder;
const zigimg = @import("zigimg");
const Cell = @import("Cell.zig");
const Image = @import("Image.zig");
const InternalScreen = @import("InternalScreen.zig");
const Key = @import("Key.zig");
const Mouse = @import("Mouse.zig");
const Screen = @import("Screen.zig");
const Unicode = @import("Unicode.zig");
const Window = @import("Window.zig");
const AnyWriter = std.io.AnyWriter;
const Hyperlink = Cell.Hyperlink;
const KittyFlags = Key.KittyFlags;
const Shape = Mouse.Shape;
const Style = Cell.Style;
const Winsize = @import("main.zig").Winsize;
const ctlseqs = @import("ctlseqs.zig");
const gwidth = @import("gwidth.zig");
const Vaxis = @This();
const log = std.log.scoped(.vaxis);
pub const Capabilities = struct {
kitty_keyboard: bool = false,
kitty_graphics: bool = false,
rgb: bool = false,
unicode: gwidth.Method = .wcwidth,
sgr_pixels: bool = false,
color_scheme_updates: bool = false,
};
pub const Options = struct {
kitty_keyboard_flags: KittyFlags = .{},
/// When supplied, this allocator will be used for system clipboard
/// requests. If not supplied, it won't be possible to request the system
/// clipboard
system_clipboard_allocator: ?std.mem.Allocator = null,
};
/// the screen we write to
screen: Screen,
/// The last screen we drew. We keep this so we can efficiently update on
/// the next render
screen_last: InternalScreen = undefined,
caps: Capabilities = .{},
opts: Options = .{},
/// if we should redraw the entire screen on the next render
refresh: bool = false,
/// blocks the main thread until a DA1 query has been received, or the
/// futex times out
query_futex: atomic.Value(u32) = atomic.Value(u32).init(0),
// images
next_img_id: u32 = 1,
unicode: Unicode,
// statistics
renders: usize = 0,
render_dur: u64 = 0,
render_timer: std.time.Timer,
sgr: enum {
standard,
legacy,
} = .standard,
state: struct {
/// if we are in the alt screen
alt_screen: bool = false,
/// if we have entered kitty keyboard
kitty_keyboard: bool = false,
bracketed_paste: bool = false,
mouse: bool = false,
pixel_mouse: bool = false,
color_scheme_updates: bool = false,
in_band_resize: bool = false,
changed_default_fg: bool = false,
changed_default_bg: bool = false,
cursor: struct {
row: usize = 0,
col: usize = 0,
} = .{},
} = .{},
/// Initialize Vaxis with runtime options
pub fn init(alloc: std.mem.Allocator, opts: Options) !Vaxis {
return .{
.opts = opts,
.screen = .{},
.screen_last = .{},
.render_timer = try std.time.Timer.start(),
.unicode = try Unicode.init(alloc),
};
}
/// Resets the terminal to it's original state. If an allocator is
/// passed, this will free resources associated with Vaxis. This is left as an
/// optional so applications can choose to not free resources when the
/// application will be exiting anyways
pub fn deinit(self: *Vaxis, alloc: ?std.mem.Allocator, tty: AnyWriter) void {
self.resetState(tty) catch {};
// always show the cursor on exit
tty.writeAll(ctlseqs.show_cursor) catch {};
if (alloc) |a| {
self.screen.deinit(a);
self.screen_last.deinit(a);
}
if (self.renders > 0) {
const tpr = @divTrunc(self.render_dur, self.renders);
log.debug("total renders = {d}\r", .{self.renders});
log.debug("microseconds per render = {d}\r", .{tpr});
}
self.unicode.deinit();
}
/// resets enabled features, sends cursor to home and clears below cursor
pub fn resetState(self: *Vaxis, tty: AnyWriter) !void {
if (self.state.kitty_keyboard) {
try tty.writeAll(ctlseqs.csi_u_pop);
self.state.kitty_keyboard = false;
}
if (self.state.mouse) {
try self.setMouseMode(tty, false);
}
if (self.state.bracketed_paste) {
try self.setBracketedPaste(tty, false);
}
if (self.state.alt_screen) {
try tty.writeAll(ctlseqs.home);
try tty.writeAll(ctlseqs.erase_below_cursor);
try self.exitAltScreen(tty);
} else {
try tty.writeByte('\r');
var i: usize = 0;
while (i < self.state.cursor.row) : (i += 1) {
try tty.writeAll(ctlseqs.ri);
}
try tty.writeAll(ctlseqs.erase_below_cursor);
}
if (self.state.color_scheme_updates) {
try tty.writeAll(ctlseqs.color_scheme_reset);
self.state.color_scheme_updates = false;
}
if (self.state.in_band_resize) {
try tty.writeAll(ctlseqs.in_band_resize_reset);
self.state.in_band_resize = false;
}
if (self.state.changed_default_fg) {
try tty.writeAll(ctlseqs.osc10_reset);
self.state.changed_default_fg = false;
}
if (self.state.changed_default_bg) {
try tty.writeAll(ctlseqs.osc11_reset);
self.state.changed_default_bg = false;
}
}
/// resize allocates a slice of cells equal to the number of cells
/// required to display the screen (ie width x height). Any previous screen is
/// freed when resizing. The cursor will be sent to it's home position and a
/// hardware clear-below-cursor will be sent
pub fn resize(
self: *Vaxis,
alloc: std.mem.Allocator,
tty: AnyWriter,
winsize: Winsize,
) !void {
log.debug("resizing screen: width={d} height={d}", .{ winsize.cols, winsize.rows });
self.screen.deinit(alloc);
self.screen = try Screen.init(alloc, winsize, &self.unicode);
self.screen.width_method = self.caps.unicode;
// try self.screen.int(alloc, winsize.cols, winsize.rows);
// we only init our current screen. This has the effect of redrawing
// every cell
self.screen_last.deinit(alloc);
self.screen_last = try InternalScreen.init(alloc, winsize.cols, winsize.rows);
if (self.state.alt_screen)
try tty.writeAll(ctlseqs.home)
else {
try tty.writeBytesNTimes(ctlseqs.ri, self.state.cursor.row);
try tty.writeByte('\r');
}
self.state.cursor.row = 0;
self.state.cursor.col = 0;
try tty.writeAll(ctlseqs.sgr_reset ++ ctlseqs.erase_below_cursor);
}
/// returns a Window comprising of the entire terminal screen
pub fn window(self: *Vaxis) Window {
return .{
.x_off = 0,
.y_off = 0,
.width = self.screen.width,
.height = self.screen.height,
.screen = &self.screen,
};
}
/// enter the alternate screen. The alternate screen will automatically
/// be exited if calling deinit while in the alt screen
pub fn enterAltScreen(self: *Vaxis, tty: AnyWriter) !void {
try tty.writeAll(ctlseqs.smcup);
self.state.alt_screen = true;
}
/// exit the alternate screen
pub fn exitAltScreen(self: *Vaxis, tty: AnyWriter) !void {
try tty.writeAll(ctlseqs.rmcup);
self.state.alt_screen = false;
}
/// write queries to the terminal to determine capabilities. Individual
/// capabilities will be delivered to the client and possibly intercepted by
/// Vaxis to enable features.
///
/// This call will block until Vaxis.query_futex is woken up, or the timeout.
/// Event loops can wake up this futex when cap_da1 is received
pub fn queryTerminal(self: *Vaxis, tty: AnyWriter, timeout_ns: u64) !void {
try self.queryTerminalSend(tty);
// 1 second timeout
std.Thread.Futex.timedWait(&self.query_futex, 0, timeout_ns) catch {};
try self.enableDetectedFeatures(tty);
}
/// write queries to the terminal to determine capabilities. This function
/// is only for use with a custom main loop. Call Vaxis.queryTerminal() if
/// you are using Loop.run()
pub fn queryTerminalSend(_: Vaxis, tty: AnyWriter) !void {
// TODO: re-enable this
// const colorterm = std.posix.getenv("COLORTERM") orelse "";
// if (std.mem.eql(u8, colorterm, "truecolor") or
// std.mem.eql(u8, colorterm, "24bit"))
// {
// if (@hasField(Event, "cap_rgb")) {
// self.postEvent(.cap_rgb);
// }
// }
// TODO: XTGETTCAP queries ("RGB", "Smulx")
// TODO: decide if we actually want to query for focus and sync. It
// doesn't hurt to blindly use them
// _ = try tty.write(ctlseqs.decrqm_focus);
// _ = try tty.write(ctlseqs.decrqm_sync);
try tty.writeAll(ctlseqs.decrqm_sgr_pixels ++
ctlseqs.decrqm_unicode ++
ctlseqs.decrqm_color_scheme ++
ctlseqs.in_band_resize_set ++
ctlseqs.xtversion ++
ctlseqs.csi_u_query ++
ctlseqs.kitty_graphics_query ++
ctlseqs.primary_device_attrs);
}
/// Enable features detected by responses to queryTerminal. This function
/// is only for use with a custom main loop. Call Vaxis.queryTerminal() if
/// you are using Loop.run()
pub fn enableDetectedFeatures(self: *Vaxis, tty: AnyWriter) !void {
switch (builtin.os.tag) {
.windows => {
// No feature detection on windows. We just hard enable some knowns for ConPTY
self.sgr = .legacy;
},
else => {
// Apply any environment variables
if (std.posix.getenv("TERMUX_VERSION")) |_|
self.sgr = .legacy;
if (std.posix.getenv("VHS_RECORD")) |_| {
self.caps.unicode = .wcwidth;
self.caps.kitty_keyboard = false;
self.sgr = .legacy;
}
if (std.posix.getenv("TERM_PROGRAM")) |prg| {
if (std.mem.eql(u8, prg, "vscode"))
self.sgr = .legacy;
}
if (std.posix.getenv("VAXIS_FORCE_LEGACY_SGR")) |_|
self.sgr = .legacy;
if (std.posix.getenv("VAXIS_FORCE_WCWIDTH")) |_|
self.caps.unicode = .wcwidth;
if (std.posix.getenv("VAXIS_FORCE_UNICODE")) |_|
self.caps.unicode = .unicode;
// enable detected features
if (self.caps.kitty_keyboard) {
try self.enableKittyKeyboard(tty, self.opts.kitty_keyboard_flags);
}
if (self.caps.unicode == .unicode) {
try tty.writeAll(ctlseqs.unicode_set);
}
},
}
}
// the next render call will refresh the entire screen
pub fn queueRefresh(self: *Vaxis) void {
self.refresh = true;
}
/// draws the screen to the terminal
pub fn render(self: *Vaxis, tty: AnyWriter) !void {
self.renders += 1;
self.render_timer.reset();
defer {
self.render_dur += self.render_timer.read() / std.time.ns_per_us;
}
defer self.refresh = false;
// Set up sync before we write anything
// TODO: optimize sync so we only sync _when we have changes_. This
// requires a smarter buffered writer, we'll probably have to write
// our own
try tty.writeAll(ctlseqs.sync_set);
defer tty.writeAll(ctlseqs.sync_reset) catch {};
// Send the cursor to 0,0
// TODO: this needs to move after we optimize writes. We only do
// this if we have an update to make. We also need to hide cursor
// and then reshow it if needed
try tty.writeAll(ctlseqs.hide_cursor);
if (self.state.alt_screen)
try tty.writeAll(ctlseqs.home)
else {
try tty.writeByte('\r');
try tty.writeBytesNTimes(ctlseqs.ri, self.state.cursor.row);
}
try tty.writeAll(ctlseqs.sgr_reset);
// initialize some variables
var reposition: bool = false;
var row: usize = 0;
var col: usize = 0;
var cursor: Style = .{};
var link: Hyperlink = .{};
var cursor_pos: struct {
row: usize = 0,
col: usize = 0,
} = .{};
// Clear all images
if (self.caps.kitty_graphics)
try tty.writeAll(ctlseqs.kitty_graphics_clear);
var i: usize = 0;
while (i < self.screen.buf.len) {
const cell = self.screen.buf[i];
const w = blk: {
if (cell.char.width != 0) break :blk cell.char.width;
const method: gwidth.Method = self.caps.unicode;
const width = gwidth.gwidth(cell.char.grapheme, method, &self.unicode.width_data) catch 1;
break :blk @max(1, width);
};
defer {
// advance by the width of this char mod 1
std.debug.assert(w > 0);
var j = i + 1;
while (j < i + w) : (j += 1) {
if (j >= self.screen_last.buf.len) break;
self.screen_last.buf[j].skipped = true;
}
col += w;
i += w;
}
if (col >= self.screen.width) {
row += 1;
col = 0;
// Rely on terminal wrapping to reposition into next row instead of forcing it
if (!cell.wrapped)
reposition = true;
}
// If cell is the same as our last frame, we don't need to do
// anything
const last = self.screen_last.buf[i];
if (!self.refresh and last.eql(cell) and !last.skipped and cell.image == null) {
reposition = true;
// Close any osc8 sequence we might be in before
// repositioning
if (link.uri.len > 0) {
try tty.writeAll(ctlseqs.osc8_clear);
}
continue;
}
self.screen_last.buf[i].skipped = false;
defer {
cursor = cell.style;
link = cell.link;
}
// Set this cell in the last frame
self.screen_last.writeCell(col, row, cell);
// reposition the cursor, if needed
if (reposition) {
reposition = false;
link = .{};
if (self.state.alt_screen)
try tty.print(ctlseqs.cup, .{ row + 1, col + 1 })
else {
if (cursor_pos.row == row) {
const n = col - cursor_pos.col;
if (n > 0)
try tty.print(ctlseqs.cuf, .{n});
} else {
const n = row - cursor_pos.row;
try tty.writeByteNTimes('\n', n);
try tty.writeByte('\r');
if (col > 0)
try tty.print(ctlseqs.cuf, .{col});
}
}
}
if (cell.image) |img| {
try tty.print(
ctlseqs.kitty_graphics_preamble,
.{img.img_id},
);
if (img.options.pixel_offset) |offset| {
try tty.print(
",X={d},Y={d}",
.{ offset.x, offset.y },
);
}
if (img.options.clip_region) |clip| {
if (clip.x) |x|
try tty.print(",x={d}", .{x});
if (clip.y) |y|
try tty.print(",y={d}", .{y});
if (clip.width) |width|
try tty.print(",w={d}", .{width});
if (clip.height) |height|
try tty.print(",h={d}", .{height});
}
if (img.options.size) |size| {
if (size.rows) |rows|
try tty.print(",r={d}", .{rows});
if (size.cols) |cols|
try tty.print(",c={d}", .{cols});
}
if (img.options.z_index) |z| {
try tty.print(",z={d}", .{z});
}
try tty.writeAll(ctlseqs.kitty_graphics_closing);
}
// something is different, so let's loop through everything and
// find out what
// foreground
if (!Cell.Color.eql(cursor.fg, cell.style.fg)) {
switch (cell.style.fg) {
.default => try tty.writeAll(ctlseqs.fg_reset),
.index => |idx| {
switch (idx) {
0...7 => try tty.print(ctlseqs.fg_base, .{idx}),
8...15 => try tty.print(ctlseqs.fg_bright, .{idx - 8}),
else => {
switch (self.sgr) {
.standard => try tty.print(ctlseqs.fg_indexed, .{idx}),
.legacy => try tty.print(ctlseqs.fg_indexed_legacy, .{idx}),
}
},
}
},
.rgb => |rgb| {
switch (self.sgr) {
.standard => try tty.print(ctlseqs.fg_rgb, .{ rgb[0], rgb[1], rgb[2] }),
.legacy => try tty.print(ctlseqs.fg_rgb_legacy, .{ rgb[0], rgb[1], rgb[2] }),
}
},
}
}
// background
if (!Cell.Color.eql(cursor.bg, cell.style.bg)) {
switch (cell.style.bg) {
.default => try tty.writeAll(ctlseqs.bg_reset),
.index => |idx| {
switch (idx) {
0...7 => try tty.print(ctlseqs.bg_base, .{idx}),
8...15 => try tty.print(ctlseqs.bg_bright, .{idx - 8}),
else => {
switch (self.sgr) {
.standard => try tty.print(ctlseqs.bg_indexed, .{idx}),
.legacy => try tty.print(ctlseqs.bg_indexed_legacy, .{idx}),
}
},
}
},
.rgb => |rgb| {
switch (self.sgr) {
.standard => try tty.print(ctlseqs.bg_rgb, .{ rgb[0], rgb[1], rgb[2] }),
.legacy => try tty.print(ctlseqs.bg_rgb_legacy, .{ rgb[0], rgb[1], rgb[2] }),
}
},
}
}
// underline color
if (!Cell.Color.eql(cursor.ul, cell.style.ul)) {
switch (cell.style.ul) {
.default => try tty.writeAll(ctlseqs.ul_reset),
.index => |idx| {
switch (self.sgr) {
.standard => try tty.print(ctlseqs.ul_indexed, .{idx}),
.legacy => try tty.print(ctlseqs.ul_indexed_legacy, .{idx}),
}
},
.rgb => |rgb| {
switch (self.sgr) {
.standard => try tty.print(ctlseqs.ul_rgb, .{ rgb[0], rgb[1], rgb[2] }),
.legacy => try tty.print(ctlseqs.ul_rgb_legacy, .{ rgb[0], rgb[1], rgb[2] }),
}
},
}
}
// underline style
if (cursor.ul_style != cell.style.ul_style) {
const seq = switch (cell.style.ul_style) {
.off => ctlseqs.ul_off,
.single => ctlseqs.ul_single,
.double => ctlseqs.ul_double,
.curly => ctlseqs.ul_curly,
.dotted => ctlseqs.ul_dotted,
.dashed => ctlseqs.ul_dashed,
};
try tty.writeAll(seq);
}
// bold
if (cursor.bold != cell.style.bold) {
const seq = switch (cell.style.bold) {
true => ctlseqs.bold_set,
false => ctlseqs.bold_dim_reset,
};
try tty.writeAll(seq);
if (cell.style.dim) {
try tty.writeAll(ctlseqs.dim_set);
}
}
// dim
if (cursor.dim != cell.style.dim) {
const seq = switch (cell.style.dim) {
true => ctlseqs.dim_set,
false => ctlseqs.bold_dim_reset,
};
try tty.writeAll(seq);
if (cell.style.bold) {
try tty.writeAll(ctlseqs.bold_set);
}
}
// dim
if (cursor.italic != cell.style.italic) {
const seq = switch (cell.style.italic) {
true => ctlseqs.italic_set,
false => ctlseqs.italic_reset,
};
try tty.writeAll(seq);
}
// dim
if (cursor.blink != cell.style.blink) {
const seq = switch (cell.style.blink) {
true => ctlseqs.blink_set,
false => ctlseqs.blink_reset,
};
try tty.writeAll(seq);
}
// reverse
if (cursor.reverse != cell.style.reverse) {
const seq = switch (cell.style.reverse) {
true => ctlseqs.reverse_set,
false => ctlseqs.reverse_reset,
};
try tty.writeAll(seq);
}
// invisible
if (cursor.invisible != cell.style.invisible) {
const seq = switch (cell.style.invisible) {
true => ctlseqs.invisible_set,
false => ctlseqs.invisible_reset,
};
try tty.writeAll(seq);
}
// strikethrough
if (cursor.strikethrough != cell.style.strikethrough) {
const seq = switch (cell.style.strikethrough) {
true => ctlseqs.strikethrough_set,
false => ctlseqs.strikethrough_reset,
};
try tty.writeAll(seq);
}
// url
if (!std.mem.eql(u8, link.uri, cell.link.uri)) {
var ps = cell.link.params;
if (cell.link.uri.len == 0) {
// Empty out the params no matter what if we don't have
// a url
ps = "";
}
try tty.print(ctlseqs.osc8, .{ ps, cell.link.uri });
}
try tty.writeAll(cell.char.grapheme);
cursor_pos.col = col + w;
cursor_pos.row = row;
}
if (self.screen.cursor_vis) {
if (self.state.alt_screen) {
try tty.print(
ctlseqs.cup,
.{
self.screen.cursor_row + 1,
self.screen.cursor_col + 1,
},
);
} else {
// TODO: position cursor relative to current location
try tty.writeByte('\r');
if (self.screen.cursor_row >= cursor_pos.row)
try tty.writeByteNTimes('\n', self.screen.cursor_row - cursor_pos.row)
else
try tty.writeBytesNTimes(ctlseqs.ri, cursor_pos.row - self.screen.cursor_row);
if (self.screen.cursor_col > 0)
try tty.print(ctlseqs.cuf, .{self.screen.cursor_col});
}
self.state.cursor.row = self.screen.cursor_row;
self.state.cursor.col = self.screen.cursor_col;
try tty.writeAll(ctlseqs.show_cursor);
} else {
self.state.cursor.row = cursor_pos.row;
self.state.cursor.col = cursor_pos.col;
}
if (self.screen.mouse_shape != self.screen_last.mouse_shape) {
try tty.print(
ctlseqs.osc22_mouse_shape,
.{@tagName(self.screen.mouse_shape)},
);
self.screen_last.mouse_shape = self.screen.mouse_shape;
}
if (self.screen.cursor_shape != self.screen_last.cursor_shape) {
try tty.print(
ctlseqs.cursor_shape,
.{@intFromEnum(self.screen.cursor_shape)},
);
self.screen_last.cursor_shape = self.screen.cursor_shape;
}
}
fn enableKittyKeyboard(self: *Vaxis, tty: AnyWriter, flags: Key.KittyFlags) !void {
const flag_int: u5 = @bitCast(flags);
try tty.print(ctlseqs.csi_u_push, .{flag_int});
self.state.kitty_keyboard = true;
}
/// send a system notification
pub fn notify(_: *Vaxis, tty: AnyWriter, title: ?[]const u8, body: []const u8) !void {
if (title) |t|
try tty.print(ctlseqs.osc777_notify, .{ t, body })
else
try tty.print(ctlseqs.osc9_notify, .{body});
}
/// sets the window title
pub fn setTitle(_: *Vaxis, tty: AnyWriter, title: []const u8) !void {
try tty.print(ctlseqs.osc2_set_title, .{title});
}
// turn bracketed paste on or off. An event will be sent at the
// beginning and end of a detected paste. All keystrokes between these
// events were pasted
pub fn setBracketedPaste(self: *Vaxis, tty: AnyWriter, enable: bool) !void {
const seq = if (enable)
ctlseqs.bp_set
else
ctlseqs.bp_reset;
try tty.writeAll(seq);
self.state.bracketed_paste = enable;
}
/// set the mouse shape
pub fn setMouseShape(self: *Vaxis, shape: Shape) void {
self.screen.mouse_shape = shape;
}
/// Change the mouse reporting mode
pub fn setMouseMode(self: *Vaxis, tty: AnyWriter, enable: bool) !void {
if (enable) {
self.state.mouse = true;
if (self.caps.sgr_pixels) {
log.debug("enabling mouse mode: pixel coordinates", .{});
self.state.pixel_mouse = true;
try tty.writeAll(ctlseqs.mouse_set_pixels);
} else {
log.debug("enabling mouse mode: cell coordinates", .{});
try tty.writeAll(ctlseqs.mouse_set);
}
} else {
try tty.writeAll(ctlseqs.mouse_reset);
}
}
/// Translate pixel mouse coordinates to cell + offset
pub fn translateMouse(self: Vaxis, mouse: Mouse) Mouse {
if (self.screen.width == 0 or self.screen.height == 0) return mouse;
var result = mouse;
if (self.state.pixel_mouse) {
std.debug.assert(mouse.xoffset == 0);
std.debug.assert(mouse.yoffset == 0);
const xpos = mouse.col;
const ypos = mouse.row;
const xextra = self.screen.width_pix % self.screen.width;
const yextra = self.screen.height_pix % self.screen.height;
const xcell = (self.screen.width_pix - xextra) / self.screen.width;
const ycell = (self.screen.height_pix - yextra) / self.screen.height;
result.col = xpos / xcell;
result.row = ypos / ycell;
result.xoffset = xpos % xcell;
result.yoffset = ypos % ycell;
}
return result;
}
/// Transmit an image using the local filesystem. Allocates only for base64 encoding
pub fn transmitLocalImagePath(
self: *Vaxis,
allocator: std.mem.Allocator,
tty: AnyWriter,
payload: []const u8,
width: usize,
height: usize,
medium: Image.TransmitMedium,
format: Image.TransmitFormat,
) !Image {
defer self.next_img_id += 1;
const id = self.next_img_id;
const size = base64Encoder.calcSize(payload.len);
if (size >= 4096) return error.PathTooLong;
const buf = try allocator.alloc(u8, size);
const encoded = base64Encoder.encode(buf, payload);
defer allocator.free(buf);
const medium_char: u8 = switch (medium) {
.file => 'f',
.temp_file => 't',
.shared_mem => 's',
};
switch (format) {
.rgb => {
try tty.print(
"\x1b_Gf=24,s={d},v={d},i={d},t={c};{s}\x1b\\",
.{ width, height, id, medium_char, encoded },
);
},
.rgba => {
try tty.print(
"\x1b_Gf=32,s={d},v={d},i={d},t={c};{s}\x1b\\",
.{ width, height, id, medium_char, encoded },
);
},
.png => {
try tty.print(
"\x1b_Gf=100,i={d},t={c};{s}\x1b\\",
.{ id, medium_char, encoded },
);
},
}
return .{
.id = id,
.width = width,
.height = height,
};
}
/// Transmit an image which has been pre-base64 encoded
pub fn transmitPreEncodedImage(
self: *Vaxis,
tty: AnyWriter,
bytes: []const u8,
width: usize,
height: usize,
format: Image.TransmitFormat,
) !Image {
defer self.next_img_id += 1;
const id = self.next_img_id;
const fmt: u8 = switch (format) {
.rgb => 24,
.rgba => 32,
.png => 100,
};
if (bytes.len < 4096) {
try tty.print(
"\x1b_Gf={d},s={d},v={d},i={d};{s}\x1b\\",
.{
fmt,
width,
height,
id,
bytes,
},
);
} else {
var n: usize = 4096;
try tty.print(
"\x1b_Gf={d},s={d},v={d},i={d},m=1;{s}\x1b\\",
.{ fmt, width, height, id, bytes[0..n] },
);
while (n < bytes.len) : (n += 4096) {
const end: usize = @min(n + 4096, bytes.len);
const m: u2 = if (end == bytes.len) 0 else 1;
try tty.print(
"\x1b_Gm={d};{s}\x1b\\",
.{
m,
bytes[n..end],
},
);
}
}
return .{
.id = id,
.width = width,
.height = height,
};
}
pub fn transmitImage(
self: *Vaxis,
alloc: std.mem.Allocator,
tty: AnyWriter,
img: *zigimg.Image,
format: Image.TransmitFormat,
) !Image {
if (!self.caps.kitty_graphics) return error.NoGraphicsCapability;
var arena = std.heap.ArenaAllocator.init(alloc);
defer arena.deinit();
const buf = switch (format) {
.png => png: {
const png_buf = try arena.allocator().alloc(u8, img.imageByteSize());
const png = try img.writeToMemory(png_buf, .{ .png = .{} });
break :png png;
},
.rgb => rgb: {
try img.convert(.rgb24);
break :rgb img.rawBytes();
},
.rgba => rgba: {
try img.convert(.rgba32);
break :rgba img.rawBytes();
},
};
const b64_buf = try arena.allocator().alloc(u8, base64Encoder.calcSize(buf.len));
const encoded = base64Encoder.encode(b64_buf, buf);
return self.transmitPreEncodedImage(tty, encoded, img.width, img.height, format);
}
pub fn loadImage(
self: *Vaxis,
alloc: std.mem.Allocator,
tty: AnyWriter,
src: Image.Source,
) !Image {
if (!self.caps.kitty_graphics) return error.NoGraphicsCapability;
var img = switch (src) {
.path => |path| try zigimg.Image.fromFilePath(alloc, path),
.mem => |bytes| try zigimg.Image.fromMemory(alloc, bytes),
};
defer img.deinit();
return self.transmitImage(alloc, tty, &img, .png);
}
/// deletes an image from the terminal's memory
pub fn freeImage(_: Vaxis, tty: AnyWriter, id: u32) void {
tty.print("\x1b_Ga=d,d=I,i={d};\x1b\\", .{id}) catch |err| {
log.err("couldn't delete image {d}: {}", .{ id, err });
return;
};
}
pub fn copyToSystemClipboard(_: Vaxis, tty: AnyWriter, text: []const u8, encode_allocator: std.mem.Allocator) !void {
const encoder = std.base64.standard.Encoder;
const size = encoder.calcSize(text.len);
const buf = try encode_allocator.alloc(u8, size);
const b64 = encoder.encode(buf, text);
defer encode_allocator.free(buf);
try tty.print(
ctlseqs.osc52_clipboard_copy,
.{b64},
);
}
pub fn requestSystemClipboard(self: Vaxis, tty: AnyWriter) !void {
if (self.opts.system_clipboard_allocator == null) return error.NoClipboardAllocator;
try tty.print(
ctlseqs.osc52_clipboard_request,
.{},
);
}
/// Set the default terminal foreground color
pub fn setTerminalForegroundColor(self: *Vaxis, tty: AnyWriter, rgb: [3]u8) !void {
try tty.print(ctlseqs.osc10_set, .{ rgb[0], rgb[0], rgb[1], rgb[1], rgb[2], rgb[2] });
self.state.changed_default_fg = true;
}
/// Set the default terminal background color
pub fn setTerminalBackgroundColor(self: *Vaxis, tty: AnyWriter, rgb: [3]u8) !void {
try tty.print(ctlseqs.osc11_set, .{ rgb[0], rgb[0], rgb[1], rgb[1], rgb[2], rgb[2] });
self.state.changed_default_bg = true;
}
/// Request a color report from the terminal. Note: not all terminals support
/// reporting colors. It is always safe to try, but you may not receive a
/// response.
pub fn queryColor(_: Vaxis, tty: AnyWriter, kind: Cell.Color.Kind) !void {
switch (kind) {
.fg => try tty.writeAll(ctlseqs.osc10_query),
.bg => try tty.writeAll(ctlseqs.osc11_query),
.cursor => try tty.writeAll(ctlseqs.osc12_query),
.index => |idx| try tty.print(ctlseqs.osc4_query, .{idx}),
}
}
/// Subscribe to color theme updates. A `color_scheme: Color.Scheme` tag must
/// exist on your Event type to receive the response. This is a queried
/// capability. Support can be detected by checking the value of
/// vaxis.caps.color_scheme_updates. The initial scheme will be reported when
/// subscribing.
pub fn subscribeToColorSchemeUpdates(self: Vaxis, tty: AnyWriter) !void {
try tty.writeAll(ctlseqs.color_scheme_request);
try tty.writeAll(ctlseqs.color_scheme_set);
self.state.color_scheme_updates = true;
}
pub fn deviceStatusReport(_: Vaxis, tty: AnyWriter) !void {
try tty.writeAll(ctlseqs.device_status_report);
}
/// prettyPrint is used to print the contents of the Screen to the tty. The state is not stored, and
/// the cursor will be put on the next line after the last line is printed. This is useful to
/// sequentially print data in a styled format to eg. stdout. This function returns an error if you
/// are not in the alt screen. The cursor is always hidden, and mouse shapes are not available
pub fn prettyPrint(self: *Vaxis, tty: AnyWriter) !void {
if (self.state.alt_screen) return error.NotInPrimaryScreen;
try tty.writeAll(ctlseqs.hide_cursor);
try tty.writeAll(ctlseqs.sync_set);
defer tty.writeAll(ctlseqs.sync_reset) catch {};
try tty.writeAll(ctlseqs.sgr_reset);
var reposition: bool = false;
var row: usize = 0;
var col: usize = 0;
var cursor: Style = .{};
var link: Hyperlink = .{};
var cursor_pos: struct {
row: usize = 0,
col: usize = 0,
} = .{};
var i: usize = 0;
while (i < self.screen.buf.len) {
const cell = self.screen.buf[i];
const w = blk: {
if (cell.char.width != 0) break :blk cell.char.width;
const method: gwidth.Method = self.caps.unicode;
const width = gwidth.gwidth(cell.char.grapheme, method, &self.unicode.width_data) catch 1;
break :blk @max(1, width);
};
defer {
// advance by the width of this char mod 1
std.debug.assert(w > 0);
var j = i + 1;
while (j < i + w) : (j += 1) {
if (j >= self.screen_last.buf.len) break;
self.screen_last.buf[j].skipped = true;
}
col += w;
i += w;
}
if (col >= self.screen.width) {
row += 1;
col = 0;
// Rely on terminal wrapping to reposition into next row instead of forcing it
if (!cell.wrapped)
reposition = true;
}
if (cell.default) {
reposition = true;
continue;
}
defer {
cursor = cell.style;
link = cell.link;
}
// reposition the cursor, if needed
if (reposition) {
reposition = false;
link = .{};
if (cursor_pos.row == row) {
const n = col - cursor_pos.col;
if (n > 0)
try tty.print(ctlseqs.cuf, .{n});
} else {
const n = row - cursor_pos.row;
try tty.writeByteNTimes('\n', n);
try tty.writeByte('\r');
if (col > 0)
try tty.print(ctlseqs.cuf, .{col});
}
}
if (cell.image) |img| {
try tty.print(
ctlseqs.kitty_graphics_preamble,
.{img.img_id},
);
if (img.options.pixel_offset) |offset| {
try tty.print(
",X={d},Y={d}",
.{ offset.x, offset.y },
);
}
if (img.options.clip_region) |clip| {
if (clip.x) |x|
try tty.print(",x={d}", .{x});
if (clip.y) |y|
try tty.print(",y={d}", .{y});
if (clip.width) |width|
try tty.print(",w={d}", .{width});
if (clip.height) |height|
try tty.print(",h={d}", .{height});
}
if (img.options.size) |size| {
if (size.rows) |rows|
try tty.print(",r={d}", .{rows});
if (size.cols) |cols|
try tty.print(",c={d}", .{cols});
}
if (img.options.z_index) |z| {
try tty.print(",z={d}", .{z});
}
try tty.writeAll(ctlseqs.kitty_graphics_closing);
}
// something is different, so let's loop through everything and
// find out what
// foreground
if (!Cell.Color.eql(cursor.fg, cell.style.fg)) {
switch (cell.style.fg) {
.default => try tty.writeAll(ctlseqs.fg_reset),
.index => |idx| {
switch (idx) {
0...7 => try tty.print(ctlseqs.fg_base, .{idx}),
8...15 => try tty.print(ctlseqs.fg_bright, .{idx - 8}),
else => {
switch (self.sgr) {
.standard => try tty.print(ctlseqs.fg_indexed, .{idx}),
.legacy => try tty.print(ctlseqs.fg_indexed_legacy, .{idx}),
}
},
}
},
.rgb => |rgb| {
switch (self.sgr) {
.standard => try tty.print(ctlseqs.fg_rgb, .{ rgb[0], rgb[1], rgb[2] }),
.legacy => try tty.print(ctlseqs.fg_rgb_legacy, .{ rgb[0], rgb[1], rgb[2] }),
}
},
}
}
// background
if (!Cell.Color.eql(cursor.bg, cell.style.bg)) {
switch (cell.style.bg) {
.default => try tty.writeAll(ctlseqs.bg_reset),
.index => |idx| {
switch (idx) {
0...7 => try tty.print(ctlseqs.bg_base, .{idx}),
8...15 => try tty.print(ctlseqs.bg_bright, .{idx - 8}),
else => {
switch (self.sgr) {
.standard => try tty.print(ctlseqs.bg_indexed, .{idx}),
.legacy => try tty.print(ctlseqs.bg_indexed_legacy, .{idx}),
}
},
}
},
.rgb => |rgb| {
switch (self.sgr) {
.standard => try tty.print(ctlseqs.bg_rgb, .{ rgb[0], rgb[1], rgb[2] }),
.legacy => try tty.print(ctlseqs.bg_rgb_legacy, .{ rgb[0], rgb[1], rgb[2] }),
}
},
}
}
// underline color
if (!Cell.Color.eql(cursor.ul, cell.style.ul)) {
switch (cell.style.ul) {
.default => try tty.writeAll(ctlseqs.ul_reset),
.index => |idx| {
switch (self.sgr) {
.standard => try tty.print(ctlseqs.ul_indexed, .{idx}),
.legacy => try tty.print(ctlseqs.ul_indexed_legacy, .{idx}),
}
},
.rgb => |rgb| {
switch (self.sgr) {
.standard => try tty.print(ctlseqs.ul_rgb, .{ rgb[0], rgb[1], rgb[2] }),
.legacy => try tty.print(ctlseqs.ul_rgb_legacy, .{ rgb[0], rgb[1], rgb[2] }),
}
},
}
}
// underline style
if (cursor.ul_style != cell.style.ul_style) {
const seq = switch (cell.style.ul_style) {
.off => ctlseqs.ul_off,
.single => ctlseqs.ul_single,
.double => ctlseqs.ul_double,
.curly => ctlseqs.ul_curly,
.dotted => ctlseqs.ul_dotted,
.dashed => ctlseqs.ul_dashed,
};
try tty.writeAll(seq);
}
// bold
if (cursor.bold != cell.style.bold) {
const seq = switch (cell.style.bold) {
true => ctlseqs.bold_set,
false => ctlseqs.bold_dim_reset,
};
try tty.writeAll(seq);
if (cell.style.dim) {
try tty.writeAll(ctlseqs.dim_set);
}
}
// dim
if (cursor.dim != cell.style.dim) {
const seq = switch (cell.style.dim) {
true => ctlseqs.dim_set,
false => ctlseqs.bold_dim_reset,
};
try tty.writeAll(seq);
if (cell.style.bold) {
try tty.writeAll(ctlseqs.bold_set);
}
}
// dim
if (cursor.italic != cell.style.italic) {
const seq = switch (cell.style.italic) {
true => ctlseqs.italic_set,
false => ctlseqs.italic_reset,
};
try tty.writeAll(seq);
}
// dim
if (cursor.blink != cell.style.blink) {
const seq = switch (cell.style.blink) {
true => ctlseqs.blink_set,
false => ctlseqs.blink_reset,
};
try tty.writeAll(seq);
}
// reverse
if (cursor.reverse != cell.style.reverse) {
const seq = switch (cell.style.reverse) {
true => ctlseqs.reverse_set,
false => ctlseqs.reverse_reset,
};
try tty.writeAll(seq);
}
// invisible
if (cursor.invisible != cell.style.invisible) {
const seq = switch (cell.style.invisible) {
true => ctlseqs.invisible_set,
false => ctlseqs.invisible_reset,
};
try tty.writeAll(seq);
}
// strikethrough
if (cursor.strikethrough != cell.style.strikethrough) {
const seq = switch (cell.style.strikethrough) {
true => ctlseqs.strikethrough_set,
false => ctlseqs.strikethrough_reset,
};
try tty.writeAll(seq);
}
// url
if (!std.mem.eql(u8, link.uri, cell.link.uri)) {
var ps = cell.link.params;
if (cell.link.uri.len == 0) {
// Empty out the params no matter what if we don't have
// a url
ps = "";
}
try tty.print(ctlseqs.osc8, .{ ps, cell.link.uri });
}
try tty.writeAll(cell.char.grapheme);
cursor_pos.col = col + w;
cursor_pos.row = row;
}
try tty.writeAll("\r\n");
}
/// Set the terminal's current working directory
pub fn setTerminalWorkingDirectory(_: *Vaxis, tty: AnyWriter, path: []const u8) !void {
if (path.len == 0 or path[0] != '/')
return error.InvalidAbsolutePath;
const hostname = switch (builtin.os.tag) {
.windows => null,
else => std.posix.getenv("HOSTNAME"),
} orelse "localhost";
const uri: std.Uri = .{
.scheme = "file",
.host = .{ .raw = hostname },
.path = .{ .raw = path },
};
try tty.print(ctlseqs.osc7, .{uri});
}
|
0 | repos/libvaxis | repos/libvaxis/src/Key.zig | const std = @import("std");
const testing = std.testing;
const Key = @This();
/// Modifier Keys for a Key Match Event.
pub const Modifiers = packed struct(u8) {
shift: bool = false,
alt: bool = false,
ctrl: bool = false,
super: bool = false,
hyper: bool = false,
meta: bool = false,
caps_lock: bool = false,
num_lock: bool = false,
};
/// Flags for the Kitty Protocol.
pub const KittyFlags = packed struct(u5) {
disambiguate: bool = true,
report_events: bool = false,
report_alternate_keys: bool = true,
report_all_as_ctl_seqs: bool = true,
report_text: bool = true,
};
/// the unicode codepoint of the key event.
codepoint: u21,
/// the text generated from the key event. The underlying slice has a limited
/// lifetime. Vaxis maintains an internal ring buffer to temporarily store text.
/// If the application needs these values longer than the lifetime of the event
/// it must copy the data.
text: ?[]const u8 = null,
/// the shifted codepoint of this key event. This will only be present if the
/// Shift modifier was used to generate the event
shifted_codepoint: ?u21 = null,
/// the key that would have been pressed on a standard keyboard layout. This is
/// useful for shortcut matching
base_layout_codepoint: ?u21 = null,
mods: Modifiers = .{},
// matches follows a loose matching algorithm for key matches.
// 1. If the codepoint and modifiers are exact matches, after removing caps_lock
// and num_lock
// 2. If the utf8 encoding of the codepoint matches the text, after removing
// num_lock
// 3. If there is a shifted codepoint and it matches after removing the shift
// modifier from self, after removing caps_lock and num_lock
pub fn matches(self: Key, cp: u21, mods: Modifiers) bool {
// rule 1
if (self.matchExact(cp, mods)) return true;
// rule 2
if (self.matchText(cp, mods)) return true;
// rule 3
if (self.matchShiftedCodepoint(cp, mods)) return true;
return false;
}
/// matches against any of the provided codepoints.
pub fn matchesAny(self: Key, cps: []const u21, mods: Modifiers) bool {
for (cps) |cp| {
if (self.matches(cp, mods)) return true;
}
return false;
}
/// matches base layout codes, useful for shortcut matching when an alternate key
/// layout is used
pub fn matchShortcut(self: Key, cp: u21, mods: Modifiers) bool {
if (self.base_layout_codepoint == null) return false;
return cp == self.base_layout_codepoint.? and std.meta.eql(self.mods, mods);
}
/// matches keys that aren't upper case versions when shifted. For example, shift
/// + semicolon produces a colon. The key can be matched against shift +
/// semicolon or just colon...or shift + ctrl + ; or just ctrl + :
pub fn matchShiftedCodepoint(self: Key, cp: u21, mods: Modifiers) bool {
if (self.shifted_codepoint == null) return false;
if (!self.mods.shift) return false;
var self_mods = self.mods;
self_mods.shift = false;
self_mods.caps_lock = false;
self_mods.num_lock = false;
var tgt_mods = mods;
tgt_mods.caps_lock = false;
tgt_mods.num_lock = false;
return cp == self.shifted_codepoint.? and std.meta.eql(self_mods, mods);
}
/// matches when the utf8 encoding of the codepoint and relevant mods matches the
/// text of the key. This function will consume Shift and Caps Lock when matching
pub fn matchText(self: Key, cp: u21, mods: Modifiers) bool {
// return early if we have no text
if (self.text == null) return false;
var self_mods = self.mods;
self_mods.num_lock = false;
self_mods.shift = false;
self_mods.caps_lock = false;
var arg_mods = mods;
arg_mods.num_lock = false;
arg_mods.shift = false;
arg_mods.caps_lock = false;
var buf: [4]u8 = undefined;
const n = std.unicode.utf8Encode(cp, buf[0..]) catch return false;
return std.mem.eql(u8, self.text.?, buf[0..n]) and std.meta.eql(self_mods, arg_mods);
}
// The key must exactly match the codepoint and modifiers. caps_lock and
// num_lock are removed before matching
pub fn matchExact(self: Key, cp: u21, mods: Modifiers) bool {
var self_mods = self.mods;
self_mods.caps_lock = false;
self_mods.num_lock = false;
var tgt_mods = mods;
tgt_mods.caps_lock = false;
tgt_mods.num_lock = false;
return self.codepoint == cp and std.meta.eql(self_mods, tgt_mods);
}
/// True if the key is a single modifier (ie: left_shift)
pub fn isModifier(self: Key) bool {
return self.codepoint == left_shift or
self.codepoint == left_alt or
self.codepoint == left_super or
self.codepoint == left_hyper or
self.codepoint == left_control or
self.codepoint == left_meta or
self.codepoint == right_shift or
self.codepoint == right_alt or
self.codepoint == right_super or
self.codepoint == right_hyper or
self.codepoint == right_control or
self.codepoint == right_meta;
}
// a few special keys that we encode as their actual ascii value
pub const tab: u21 = 0x09;
pub const enter: u21 = 0x0D;
pub const escape: u21 = 0x1B;
pub const space: u21 = 0x20;
pub const backspace: u21 = 0x7F;
/// multicodepoint is a key which generated text but cannot be expressed as a
/// single codepoint. The value is the maximum unicode codepoint + 1
pub const multicodepoint: u21 = 1_114_112 + 1;
// kitty encodes these keys directly in the private use area. We reuse those
// mappings
pub const insert: u21 = 57348;
pub const delete: u21 = 57349;
pub const left: u21 = 57350;
pub const right: u21 = 57351;
pub const up: u21 = 57352;
pub const down: u21 = 57353;
pub const page_up: u21 = 57354;
pub const page_down: u21 = 57355;
pub const home: u21 = 57356;
pub const end: u21 = 57357;
pub const caps_lock: u21 = 57358;
pub const scroll_lock: u21 = 57359;
pub const num_lock: u21 = 57360;
pub const print_screen: u21 = 57361;
pub const pause: u21 = 57362;
pub const menu: u21 = 57363;
pub const f1: u21 = 57364;
pub const f2: u21 = 57365;
pub const f3: u21 = 57366;
pub const f4: u21 = 57367;
pub const f5: u21 = 57368;
pub const f6: u21 = 57369;
pub const f7: u21 = 57370;
pub const f8: u21 = 57371;
pub const f9: u21 = 57372;
pub const f10: u21 = 57373;
pub const f11: u21 = 57374;
pub const f12: u21 = 57375;
pub const f13: u21 = 57376;
pub const f14: u21 = 57377;
pub const f15: u21 = 57378;
pub const @"f16": u21 = 57379;
pub const f17: u21 = 57380;
pub const f18: u21 = 57381;
pub const f19: u21 = 57382;
pub const f20: u21 = 57383;
pub const f21: u21 = 57384;
pub const f22: u21 = 57385;
pub const f23: u21 = 57386;
pub const f24: u21 = 57387;
pub const f25: u21 = 57388;
pub const f26: u21 = 57389;
pub const f27: u21 = 57390;
pub const f28: u21 = 57391;
pub const f29: u21 = 57392;
pub const f30: u21 = 57393;
pub const f31: u21 = 57394;
pub const @"f32": u21 = 57395;
pub const f33: u21 = 57396;
pub const f34: u21 = 57397;
pub const f35: u21 = 57398;
pub const kp_0: u21 = 57399;
pub const kp_1: u21 = 57400;
pub const kp_2: u21 = 57401;
pub const kp_3: u21 = 57402;
pub const kp_4: u21 = 57403;
pub const kp_5: u21 = 57404;
pub const kp_6: u21 = 57405;
pub const kp_7: u21 = 57406;
pub const kp_8: u21 = 57407;
pub const kp_9: u21 = 57408;
pub const kp_decimal: u21 = 57409;
pub const kp_divide: u21 = 57410;
pub const kp_multiply: u21 = 57411;
pub const kp_subtract: u21 = 57412;
pub const kp_add: u21 = 57413;
pub const kp_enter: u21 = 57414;
pub const kp_equal: u21 = 57415;
pub const kp_separator: u21 = 57416;
pub const kp_left: u21 = 57417;
pub const kp_right: u21 = 57418;
pub const kp_up: u21 = 57419;
pub const kp_down: u21 = 57420;
pub const kp_page_up: u21 = 57421;
pub const kp_page_down: u21 = 57422;
pub const kp_home: u21 = 57423;
pub const kp_end: u21 = 57424;
pub const kp_insert: u21 = 57425;
pub const kp_delete: u21 = 57426;
pub const kp_begin: u21 = 57427;
pub const media_play: u21 = 57428;
pub const media_pause: u21 = 57429;
pub const media_play_pause: u21 = 57430;
pub const media_reverse: u21 = 57431;
pub const media_stop: u21 = 57432;
pub const media_fast_forward: u21 = 57433;
pub const media_rewind: u21 = 57434;
pub const media_track_next: u21 = 57435;
pub const media_track_previous: u21 = 57436;
pub const media_record: u21 = 57437;
pub const lower_volume: u21 = 57438;
pub const raise_volume: u21 = 57439;
pub const mute_volume: u21 = 57440;
pub const left_shift: u21 = 57441;
pub const left_control: u21 = 57442;
pub const left_alt: u21 = 57443;
pub const left_super: u21 = 57444;
pub const left_hyper: u21 = 57445;
pub const left_meta: u21 = 57446;
pub const right_shift: u21 = 57447;
pub const right_control: u21 = 57448;
pub const right_alt: u21 = 57449;
pub const right_super: u21 = 57450;
pub const right_hyper: u21 = 57451;
pub const right_meta: u21 = 57452;
pub const iso_level_3_shift: u21 = 57453;
pub const iso_level_5_shift: u21 = 57454;
pub const name_map = blk: {
@setEvalBranchQuota(2000);
break :blk std.StaticStringMap(u21).initComptime(.{
// common names
.{ "plus", '+' },
.{ "minus", '-' },
.{ "colon", ':' },
.{ "semicolon", ';' },
.{ "comma", ',' },
// special keys
.{ "insert", insert },
.{ "delete", delete },
.{ "left", left },
.{ "right", right },
.{ "up", up },
.{ "down", down },
.{ "page_up", page_up },
.{ "page_down", page_down },
.{ "home", home },
.{ "end", end },
.{ "caps_lock", caps_lock },
.{ "scroll_lock", scroll_lock },
.{ "num_lock", num_lock },
.{ "print_screen", print_screen },
.{ "pause", pause },
.{ "menu", menu },
.{ "f1", f1 },
.{ "f2", f2 },
.{ "f3", f3 },
.{ "f4", f4 },
.{ "f5", f5 },
.{ "f6", f6 },
.{ "f7", f7 },
.{ "f8", f8 },
.{ "f9", f9 },
.{ "f10", f10 },
.{ "f11", f11 },
.{ "f12", f12 },
.{ "f13", f13 },
.{ "f14", f14 },
.{ "f15", f15 },
.{ "f16", @"f16" },
.{ "f17", f17 },
.{ "f18", f18 },
.{ "f19", f19 },
.{ "f20", f20 },
.{ "f21", f21 },
.{ "f22", f22 },
.{ "f23", f23 },
.{ "f24", f24 },
.{ "f25", f25 },
.{ "f26", f26 },
.{ "f27", f27 },
.{ "f28", f28 },
.{ "f29", f29 },
.{ "f30", f30 },
.{ "f31", f31 },
.{ "f32", @"f32" },
.{ "f33", f33 },
.{ "f34", f34 },
.{ "f35", f35 },
.{ "kp_0", kp_0 },
.{ "kp_1", kp_1 },
.{ "kp_2", kp_2 },
.{ "kp_3", kp_3 },
.{ "kp_4", kp_4 },
.{ "kp_5", kp_5 },
.{ "kp_6", kp_6 },
.{ "kp_7", kp_7 },
.{ "kp_8", kp_8 },
.{ "kp_9", kp_9 },
.{ "kp_decimal", kp_decimal },
.{ "kp_divide", kp_divide },
.{ "kp_multiply", kp_multiply },
.{ "kp_subtract", kp_subtract },
.{ "kp_add", kp_add },
.{ "kp_enter", kp_enter },
.{ "kp_equal", kp_equal },
.{ "kp_separator", kp_separator },
.{ "kp_left", kp_left },
.{ "kp_right", kp_right },
.{ "kp_up", kp_up },
.{ "kp_down", kp_down },
.{ "kp_page_up", kp_page_up },
.{ "kp_page_down", kp_page_down },
.{ "kp_home", kp_home },
.{ "kp_end", kp_end },
.{ "kp_insert", kp_insert },
.{ "kp_delete", kp_delete },
.{ "kp_begin", kp_begin },
.{ "media_play", media_play },
.{ "media_pause", media_pause },
.{ "media_play_pause", media_play_pause },
.{ "media_reverse", media_reverse },
.{ "media_stop", media_stop },
.{ "media_fast_forward", media_fast_forward },
.{ "media_rewind", media_rewind },
.{ "media_track_next", media_track_next },
.{ "media_track_previous", media_track_previous },
.{ "media_record", media_record },
.{ "lower_volume", lower_volume },
.{ "raise_volume", raise_volume },
.{ "mute_volume", mute_volume },
.{ "left_shift", left_shift },
.{ "left_control", left_control },
.{ "left_alt", left_alt },
.{ "left_super", left_super },
.{ "left_hyper", left_hyper },
.{ "left_meta", left_meta },
.{ "right_shift", right_shift },
.{ "right_control", right_control },
.{ "right_alt", right_alt },
.{ "right_super", right_super },
.{ "right_hyper", right_hyper },
.{ "right_meta", right_meta },
.{ "iso_level_3_shift", iso_level_3_shift },
.{ "iso_level_5_shift", iso_level_5_shift },
});
};
test "matches 'a'" {
const key: Key = .{
.codepoint = 'a',
.mods = .{ .num_lock = true },
};
try testing.expect(key.matches('a', .{}));
}
test "matches 'shift+a'" {
const key: Key = .{
.codepoint = 'a',
.mods = .{ .shift = true },
.text = "A",
};
try testing.expect(key.matches('a', .{ .shift = true }));
try testing.expect(key.matches('A', .{}));
try testing.expect(!key.matches('A', .{ .ctrl = true }));
}
test "matches 'shift+tab'" {
const key: Key = .{
.codepoint = Key.tab,
.mods = .{ .shift = true, .num_lock = true },
};
try testing.expect(key.matches(Key.tab, .{ .shift = true }));
try testing.expect(!key.matches(Key.tab, .{}));
}
test "matches 'shift+;'" {
const key: Key = .{
.codepoint = ';',
.shifted_codepoint = ':',
.mods = .{ .shift = true },
.text = ":",
};
try testing.expect(key.matches(';', .{ .shift = true }));
try testing.expect(key.matches(':', .{}));
const colon: Key = .{
.codepoint = ':',
.mods = .{},
};
try testing.expect(colon.matches(':', .{}));
}
test "name_map" {
try testing.expectEqual(insert, name_map.get("insert"));
}
|
0 | repos/libvaxis | repos/libvaxis/src/xev.zig | const std = @import("std");
const xev = @import("xev");
const Tty = @import("main.zig").Tty;
const Winsize = @import("main.zig").Winsize;
const Vaxis = @import("Vaxis.zig");
const Parser = @import("Parser.zig");
const Key = @import("Key.zig");
const Mouse = @import("Mouse.zig");
const Color = @import("Cell.zig").Color;
const log = std.log.scoped(.vaxis_xev);
pub const Event = union(enum) {
key_press: Key,
key_release: Key,
mouse: Mouse,
focus_in,
focus_out,
paste_start, // bracketed paste start
paste_end, // bracketed paste end
paste: []const u8, // osc 52 paste, caller must free
color_report: Color.Report, // osc 4, 10, 11, 12 response
color_scheme: Color.Scheme,
winsize: Winsize,
};
pub fn TtyWatcher(comptime Userdata: type) type {
return struct {
const Self = @This();
file: xev.File,
tty: *Tty,
read_buf: [4096]u8,
read_buf_start: usize,
read_cmp: xev.Completion,
winsize_wakeup: xev.Async,
winsize_cmp: xev.Completion,
callback: *const fn (
ud: ?*Userdata,
loop: *xev.Loop,
watcher: *Self,
event: Event,
) xev.CallbackAction,
ud: ?*Userdata,
vx: *Vaxis,
parser: Parser,
pub fn init(
self: *Self,
tty: *Tty,
vaxis: *Vaxis,
loop: *xev.Loop,
userdata: ?*Userdata,
callback: *const fn (
ud: ?*Userdata,
loop: *xev.Loop,
watcher: *Self,
event: Event,
) xev.CallbackAction,
) !void {
self.* = .{
.tty = tty,
.file = xev.File.initFd(tty.fd),
.read_buf = undefined,
.read_buf_start = 0,
.read_cmp = .{},
.winsize_wakeup = try xev.Async.init(),
.winsize_cmp = .{},
.callback = callback,
.ud = userdata,
.vx = vaxis,
.parser = .{ .grapheme_data = &vaxis.unicode.grapheme_data },
};
self.file.read(
loop,
&self.read_cmp,
.{ .slice = &self.read_buf },
Self,
self,
Self.ttyReadCallback,
);
self.winsize_wakeup.wait(
loop,
&self.winsize_cmp,
Self,
self,
winsizeCallback,
);
const handler: Tty.SignalHandler = .{
.context = self,
.callback = Self.signalCallback,
};
try Tty.notifyWinsize(handler);
}
fn signalCallback(ptr: *anyopaque) void {
const self: *Self = @ptrCast(@alignCast(ptr));
self.winsize_wakeup.notify() catch |err| {
log.warn("couldn't wake up winsize callback: {}", .{err});
};
}
fn ttyReadCallback(
ud: ?*Self,
loop: *xev.Loop,
c: *xev.Completion,
_: xev.File,
buf: xev.ReadBuffer,
r: xev.ReadError!usize,
) xev.CallbackAction {
const n = r catch |err| {
log.err("read error: {}", .{err});
return .disarm;
};
const self = ud orelse unreachable;
// reset read start state
self.read_buf_start = 0;
var seq_start: usize = 0;
parse_loop: while (seq_start < n) {
const result = self.parser.parse(buf.slice[seq_start..n], null) catch |err| {
log.err("couldn't parse input: {}", .{err});
return .disarm;
};
if (result.n == 0) {
// copy the read to the beginning. We don't use memcpy because
// this could be overlapping, and it's also rare
const initial_start = seq_start;
while (seq_start < n) : (seq_start += 1) {
self.read_buf[seq_start - initial_start] = self.read_buf[seq_start];
}
self.read_buf_start = seq_start - initial_start + 1;
return .rearm;
}
seq_start += n;
const event_inner = result.event orelse {
log.debug("unknown event: {s}", .{self.read_buf[seq_start - n + 1 .. seq_start]});
continue :parse_loop;
};
// Capture events we want to bubble up
const event: ?Event = switch (event_inner) {
.key_press => |key| .{ .key_press = key },
.key_release => |key| .{ .key_release = key },
.mouse => |mouse| .{ .mouse = mouse },
.focus_in => .focus_in,
.focus_out => .focus_out,
.paste_start => .paste_start,
.paste_end => .paste_end,
.paste => |paste| .{ .paste = paste },
.color_report => |report| .{ .color_report = report },
.color_scheme => |scheme| .{ .color_scheme = scheme },
.winsize => |ws| .{ .winsize = ws },
// capability events which we handle below
.cap_kitty_keyboard,
.cap_kitty_graphics,
.cap_rgb,
.cap_unicode,
.cap_sgr_pixels,
.cap_color_scheme_updates,
.cap_da1,
=> null, // handled below
};
if (event) |ev| {
const action = self.callback(self.ud, loop, self, ev);
switch (action) {
.disarm => return .disarm,
else => continue :parse_loop,
}
}
switch (event_inner) {
.key_press,
.key_release,
.mouse,
.focus_in,
.focus_out,
.paste_start,
.paste_end,
.paste,
.color_report,
.color_scheme,
.winsize,
=> unreachable, // handled above
.cap_kitty_keyboard => {
log.info("kitty keyboard capability detected", .{});
self.vx.caps.kitty_keyboard = true;
},
.cap_kitty_graphics => {
if (!self.vx.caps.kitty_graphics) {
log.info("kitty graphics capability detected", .{});
self.vx.caps.kitty_graphics = true;
}
},
.cap_rgb => {
log.info("rgb capability detected", .{});
self.vx.caps.rgb = true;
},
.cap_unicode => {
log.info("unicode capability detected", .{});
self.vx.caps.unicode = .unicode;
self.vx.screen.width_method = .unicode;
},
.cap_sgr_pixels => {
log.info("pixel mouse capability detected", .{});
self.vx.caps.sgr_pixels = true;
},
.cap_color_scheme_updates => {
log.info("color_scheme_updates capability detected", .{});
self.vx.caps.color_scheme_updates = true;
},
.cap_da1 => {
self.vx.enableDetectedFeatures(self.tty.anyWriter()) catch |err| {
log.err("couldn't enable features: {}", .{err});
};
},
}
}
self.file.read(
loop,
c,
.{ .slice = &self.read_buf },
Self,
self,
Self.ttyReadCallback,
);
return .disarm;
}
fn winsizeCallback(
ud: ?*Self,
l: *xev.Loop,
c: *xev.Completion,
r: xev.Async.WaitError!void,
) xev.CallbackAction {
_ = r catch |err| {
log.err("async error: {}", .{err});
return .disarm;
};
const self = ud orelse unreachable; // no userdata
const winsize = Tty.getWinsize(self.tty.fd) catch |err| {
log.err("couldn't get winsize: {}", .{err});
return .disarm;
};
const ret = self.callback(self.ud, l, self, .{ .winsize = winsize });
if (ret == .disarm) return .disarm;
self.winsize_wakeup.wait(
l,
c,
Self,
self,
winsizeCallback,
);
return .disarm;
}
};
}
|
0 | repos/libvaxis | repos/libvaxis/src/Cell.zig | const std = @import("std");
const Image = @import("Image.zig");
char: Character = .{},
style: Style = .{},
link: Hyperlink = .{},
image: ?Image.Placement = null,
default: bool = false,
/// Set to true if this cell is the last cell printed in a row before wrap. Vaxis will determine if
/// it should rely on the terminal's autowrap feature which can help with primary screen resizes
wrapped: bool = false,
/// Segment is a contiguous run of text that has a constant style
pub const Segment = struct {
text: []const u8,
style: Style = .{},
link: Hyperlink = .{},
};
pub const Character = struct {
grapheme: []const u8 = " ",
/// width should only be provided when the application is sure the terminal
/// will measure the same width. This can be ensure by using the gwidth method
/// included in libvaxis. If width is 0, libvaxis will measure the glyph at
/// render time
width: usize = 1,
};
pub const CursorShape = enum {
default,
block_blink,
block,
underline_blink,
underline,
beam_blink,
beam,
};
pub const Hyperlink = struct {
uri: []const u8 = "",
/// ie "id=app-1234"
params: []const u8 = "",
};
pub const Style = struct {
pub const Underline = enum {
off,
single,
double,
curly,
dotted,
dashed,
};
fg: Color = .default,
bg: Color = .default,
ul: Color = .default,
ul_style: Underline = .off,
bold: bool = false,
dim: bool = false,
italic: bool = false,
blink: bool = false,
reverse: bool = false,
invisible: bool = false,
strikethrough: bool = false,
pub fn eql(a: Style, b: Style) bool {
const SGRBits = packed struct {
bold: bool,
dim: bool,
italic: bool,
blink: bool,
reverse: bool,
invisible: bool,
strikethrough: bool,
};
const a_sgr: SGRBits = .{
.bold = a.bold,
.dim = a.dim,
.italic = a.italic,
.blink = a.blink,
.reverse = a.reverse,
.invisible = a.invisible,
.strikethrough = a.strikethrough,
};
const b_sgr: SGRBits = .{
.bold = b.bold,
.dim = b.dim,
.italic = b.italic,
.blink = b.blink,
.reverse = b.reverse,
.invisible = b.invisible,
.strikethrough = b.strikethrough,
};
const a_cast: u7 = @bitCast(a_sgr);
const b_cast: u7 = @bitCast(b_sgr);
return a_cast == b_cast and
Color.eql(a.fg, b.fg) and
Color.eql(a.bg, b.bg) and
Color.eql(a.ul, b.ul) and
a.ul_style == b.ul_style;
}
};
pub const Color = union(enum) {
default,
index: u8,
rgb: [3]u8,
pub const Kind = union(enum) {
fg,
bg,
cursor,
index: u8,
};
/// Returned when querying a color from the terminal
pub const Report = struct {
kind: Kind,
value: [3]u8,
};
pub const Scheme = enum {
dark,
light,
};
pub fn eql(a: Color, b: Color) bool {
switch (a) {
.default => return b == .default,
.index => |a_idx| {
switch (b) {
.index => |b_idx| return a_idx == b_idx,
else => return false,
}
},
.rgb => |a_rgb| {
switch (b) {
.rgb => |b_rgb| return a_rgb[0] == b_rgb[0] and
a_rgb[1] == b_rgb[1] and
a_rgb[2] == b_rgb[2],
else => return false,
}
},
}
}
pub fn rgbFromUint(val: u24) Color {
const r_bits = val & 0b11111111_00000000_00000000;
const g_bits = val & 0b00000000_11111111_00000000;
const b_bits = val & 0b00000000_00000000_11111111;
const rgb = [_]u8{
@truncate(r_bits >> 16),
@truncate(g_bits >> 8),
@truncate(b_bits),
};
return .{ .rgb = rgb };
}
/// parse an XParseColor-style rgb specification into an rgb Color. The spec
/// is of the form: rgb:rrrr/gggg/bbbb. Generally, the high two bits will always
/// be the same as the low two bits.
pub fn rgbFromSpec(spec: []const u8) !Color {
var iter = std.mem.splitScalar(u8, spec, ':');
const prefix = iter.next() orelse return error.InvalidColorSpec;
if (!std.mem.eql(u8, "rgb", prefix)) return error.InvalidColorSpec;
const spec_str = iter.next() orelse return error.InvalidColorSpec;
var spec_iter = std.mem.splitScalar(u8, spec_str, '/');
const r_raw = spec_iter.next() orelse return error.InvalidColorSpec;
if (r_raw.len != 4) return error.InvalidColorSpec;
const g_raw = spec_iter.next() orelse return error.InvalidColorSpec;
if (g_raw.len != 4) return error.InvalidColorSpec;
const b_raw = spec_iter.next() orelse return error.InvalidColorSpec;
if (b_raw.len != 4) return error.InvalidColorSpec;
const r = try std.fmt.parseUnsigned(u8, r_raw[2..], 16);
const g = try std.fmt.parseUnsigned(u8, g_raw[2..], 16);
const b = try std.fmt.parseUnsigned(u8, b_raw[2..], 16);
return .{
.rgb = [_]u8{ r, g, b },
};
}
test "rgbFromSpec" {
const spec = "rgb:aaaa/bbbb/cccc";
const actual = try rgbFromSpec(spec);
switch (actual) {
.rgb => |rgb| {
try std.testing.expectEqual(0xAA, rgb[0]);
try std.testing.expectEqual(0xBB, rgb[1]);
try std.testing.expectEqual(0xCC, rgb[2]);
},
else => try std.testing.expect(false),
}
}
};
|
0 | repos/libvaxis | repos/libvaxis/src/gwidth.zig | const std = @import("std");
const unicode = std.unicode;
const testing = std.testing;
const DisplayWidth = @import("DisplayWidth");
const code_point = @import("code_point");
/// the method to use when calculating the width of a grapheme
pub const Method = enum {
unicode,
wcwidth,
no_zwj,
};
/// returns the width of the provided string, as measured by the method chosen
pub fn gwidth(str: []const u8, method: Method, data: *const DisplayWidth.DisplayWidthData) !usize {
switch (method) {
.unicode => {
const dw: DisplayWidth = .{ .data = data };
return dw.strWidth(str);
},
.wcwidth => {
var total: usize = 0;
var iter: code_point.Iterator = .{ .bytes = str };
while (iter.next()) |cp| {
const w = switch (cp.code) {
// undo an override in zg for emoji skintone selectors
0x1f3fb...0x1f3ff,
=> 2,
else => data.codePointWidth(cp.code),
};
if (w < 0) continue;
total += @intCast(w);
}
return total;
},
.no_zwj => {
var out: [256]u8 = undefined;
if (str.len > out.len) return error.OutOfMemory;
const n = std.mem.replacementSize(u8, str, "\u{200D}", "");
_ = std.mem.replace(u8, str, "\u{200D}", "", &out);
return gwidth(out[0..n], .unicode, data);
},
}
}
test "gwidth: a" {
const alloc = testing.allocator_instance.allocator();
const data = try DisplayWidth.DisplayWidthData.init(alloc);
defer data.deinit();
try testing.expectEqual(1, try gwidth("a", .unicode, &data));
try testing.expectEqual(1, try gwidth("a", .wcwidth, &data));
try testing.expectEqual(1, try gwidth("a", .no_zwj, &data));
}
test "gwidth: emoji with ZWJ" {
const alloc = testing.allocator_instance.allocator();
const data = try DisplayWidth.DisplayWidthData.init(alloc);
defer data.deinit();
try testing.expectEqual(2, try gwidth("👩🚀", .unicode, &data));
try testing.expectEqual(4, try gwidth("👩🚀", .wcwidth, &data));
try testing.expectEqual(4, try gwidth("👩🚀", .no_zwj, &data));
}
test "gwidth: emoji with VS16 selector" {
const alloc = testing.allocator_instance.allocator();
const data = try DisplayWidth.DisplayWidthData.init(alloc);
defer data.deinit();
try testing.expectEqual(2, try gwidth("\xE2\x9D\xA4\xEF\xB8\x8F", .unicode, &data));
try testing.expectEqual(1, try gwidth("\xE2\x9D\xA4\xEF\xB8\x8F", .wcwidth, &data));
try testing.expectEqual(2, try gwidth("\xE2\x9D\xA4\xEF\xB8\x8F", .no_zwj, &data));
}
test "gwidth: emoji with skin tone selector" {
const alloc = testing.allocator_instance.allocator();
const data = try DisplayWidth.DisplayWidthData.init(alloc);
defer data.deinit();
try testing.expectEqual(2, try gwidth("👋🏿", .unicode, &data));
try testing.expectEqual(4, try gwidth("👋🏿", .wcwidth, &data));
try testing.expectEqual(2, try gwidth("👋🏿", .no_zwj, &data));
}
|
0 | repos/libvaxis | repos/libvaxis/src/Parser.zig | const std = @import("std");
const testing = std.testing;
const Color = @import("Cell.zig").Color;
const Event = @import("event.zig").Event;
const Key = @import("Key.zig");
const Mouse = @import("Mouse.zig");
const code_point = @import("code_point");
const grapheme = @import("grapheme");
const Winsize = @import("main.zig").Winsize;
const log = std.log.scoped(.vaxis_parser);
const Parser = @This();
/// The return type of our parse method. Contains an Event and the number of
/// bytes read from the buffer.
pub const Result = struct {
event: ?Event,
n: usize,
};
const mouse_bits = struct {
const motion: u8 = 0b00100000;
const buttons: u8 = 0b11000011;
const shift: u8 = 0b00000100;
const alt: u8 = 0b00001000;
const ctrl: u8 = 0b00010000;
};
// the state of the parser
const State = enum {
ground,
escape,
csi,
osc,
dcs,
sos,
pm,
apc,
ss2,
ss3,
};
// a buffer to temporarily store text in. We need this to encode
// text-as-codepoints
buf: [128]u8 = undefined,
grapheme_data: *const grapheme.GraphemeData,
/// Parse the first event from the input buffer. If a completion event is not
/// present, Result.event will be null and Result.n will be 0
///
/// If an unknown event is found, Result.event will be null and Result.n will be
/// greater than 0
pub fn parse(self: *Parser, input: []const u8, paste_allocator: ?std.mem.Allocator) !Result {
std.debug.assert(input.len > 0);
// We gate this for len > 1 so we can detect singular escape key presses
if (input[0] == 0x1b and input.len > 1) {
switch (input[1]) {
0x4F => return parseSs3(input),
0x50 => return skipUntilST(input), // DCS
0x58 => return skipUntilST(input), // SOS
0x5B => return parseCsi(input, &self.buf), // CSI
0x5D => return parseOsc(input, paste_allocator),
0x5E => return skipUntilST(input), // PM
0x5F => return parseApc(input),
else => {
// Anything else is an "alt + <char>" keypress
const key: Key = .{
.codepoint = input[1],
.mods = .{ .alt = true },
};
return .{
.event = .{ .key_press = key },
.n = 2,
};
},
}
} else return parseGround(input, self.grapheme_data);
}
/// Parse ground state
inline fn parseGround(input: []const u8, data: *const grapheme.GraphemeData) !Result {
std.debug.assert(input.len > 0);
const b = input[0];
var n: usize = 1;
// ground state generates keypresses when parsing input. We
// generally get ascii characters, but anything less than
// 0x20 is a Ctrl+<c> keypress. We map these to lowercase
// ascii characters when we can
const key: Key = switch (b) {
0x00 => .{ .codepoint = '@', .mods = .{ .ctrl = true } },
0x08 => .{ .codepoint = Key.backspace },
0x09 => .{ .codepoint = Key.tab },
0x0A,
0x0D,
=> .{ .codepoint = Key.enter },
0x01...0x07,
0x0B...0x0C,
0x0E...0x1A,
=> .{ .codepoint = b + 0x60, .mods = .{ .ctrl = true } },
0x1B => escape: {
std.debug.assert(input.len == 1); // parseGround expects len == 1 with 0x1b
break :escape .{
.codepoint = Key.escape,
};
},
0x7F => .{ .codepoint = Key.backspace },
else => blk: {
var iter: code_point.Iterator = .{ .bytes = input };
// return null if we don't have a valid codepoint
const cp = iter.next() orelse return error.InvalidUTF8;
n = cp.len;
// Check if we have a multi-codepoint grapheme
var code = cp.code;
var g_state: grapheme.State = .{};
var prev_cp = code;
while (iter.next()) |next_cp| {
if (grapheme.graphemeBreak(prev_cp, next_cp.code, data, &g_state)) {
break;
}
prev_cp = next_cp.code;
code = Key.multicodepoint;
n += next_cp.len;
}
break :blk .{ .codepoint = code, .text = input[0..n] };
},
};
return .{
.event = .{ .key_press = key },
.n = n,
};
}
inline fn parseSs3(input: []const u8) Result {
if (input.len < 3) {
return .{
.event = null,
.n = 0,
};
}
const key: Key = switch (input[2]) {
0x1B => return .{
.event = null,
.n = 2,
},
'A' => .{ .codepoint = Key.up },
'B' => .{ .codepoint = Key.down },
'C' => .{ .codepoint = Key.right },
'D' => .{ .codepoint = Key.left },
'E' => .{ .codepoint = Key.kp_begin },
'F' => .{ .codepoint = Key.end },
'H' => .{ .codepoint = Key.home },
'P' => .{ .codepoint = Key.f1 },
'Q' => .{ .codepoint = Key.f2 },
'R' => .{ .codepoint = Key.f3 },
'S' => .{ .codepoint = Key.f4 },
else => {
log.warn("unhandled ss3: {x}", .{input[2]});
return .{
.event = null,
.n = 3,
};
},
};
return .{
.event = .{ .key_press = key },
.n = 3,
};
}
inline fn parseApc(input: []const u8) Result {
if (input.len < 3) {
return .{
.event = null,
.n = 0,
};
}
const end = std.mem.indexOfScalarPos(u8, input, 2, 0x1b) orelse return .{
.event = null,
.n = 0,
};
const sequence = input[0 .. end + 1 + 1];
switch (input[2]) {
'G' => return .{
.event = .cap_kitty_graphics,
.n = sequence.len,
},
else => return .{
.event = null,
.n = sequence.len,
},
}
}
/// Skips sequences until we see an ST (String Terminator, ESC \)
inline fn skipUntilST(input: []const u8) Result {
if (input.len < 3) {
return .{
.event = null,
.n = 0,
};
}
const end = std.mem.indexOfScalarPos(u8, input, 2, 0x1b) orelse return .{
.event = null,
.n = 0,
};
if (input.len < end + 1 + 1) {
return .{
.event = null,
.n = 0,
};
}
const sequence = input[0 .. end + 1 + 1];
return .{
.event = null,
.n = sequence.len,
};
}
/// Parses an OSC sequence
inline fn parseOsc(input: []const u8, paste_allocator: ?std.mem.Allocator) !Result {
if (input.len < 3) {
return .{
.event = null,
.n = 0,
};
}
var bel_terminated: bool = false;
// end is the index of the terminating byte(s) (either the last byte of an
// ST or BEL)
const end: usize = blk: {
const esc_result = skipUntilST(input);
if (esc_result.n > 0) break :blk esc_result.n;
// No escape, could be BEL terminated
const bel = std.mem.indexOfScalarPos(u8, input, 2, 0x07) orelse return .{
.event = null,
.n = 0,
};
bel_terminated = true;
break :blk bel + 1;
};
// The complete OSC sequence
const sequence = input[0..end];
const null_event: Result = .{ .event = null, .n = sequence.len };
const semicolon_idx = std.mem.indexOfScalarPos(u8, input, 2, ';') orelse return null_event;
const ps = std.fmt.parseUnsigned(u8, input[2..semicolon_idx], 10) catch return null_event;
switch (ps) {
4 => {
const color_idx_delim = std.mem.indexOfScalarPos(u8, input, semicolon_idx + 1, ';') orelse return null_event;
const ps_idx = std.fmt.parseUnsigned(u8, input[semicolon_idx + 1 .. color_idx_delim], 10) catch return null_event;
const color_spec = if (bel_terminated)
input[color_idx_delim + 1 .. sequence.len - 1]
else
input[color_idx_delim + 1 .. sequence.len - 2];
const color = try Color.rgbFromSpec(color_spec);
const event: Color.Report = .{
.kind = .{ .index = ps_idx },
.value = color.rgb,
};
return .{
.event = .{ .color_report = event },
.n = sequence.len,
};
},
10,
11,
12,
=> {
const color_spec = if (bel_terminated)
input[semicolon_idx + 1 .. sequence.len - 1]
else
input[semicolon_idx + 1 .. sequence.len - 2];
const color = try Color.rgbFromSpec(color_spec);
const event: Color.Report = .{
.kind = switch (ps) {
10 => .fg,
11 => .bg,
12 => .cursor,
else => unreachable,
},
.value = color.rgb,
};
return .{
.event = .{ .color_report = event },
.n = sequence.len,
};
},
52 => {
if (input[semicolon_idx + 1] != 'c') return null_event;
const payload = if (bel_terminated)
input[semicolon_idx + 3 .. sequence.len - 1]
else
input[semicolon_idx + 3 .. sequence.len - 2];
const decoder = std.base64.standard.Decoder;
const text = try paste_allocator.?.alloc(u8, try decoder.calcSizeForSlice(payload));
try decoder.decode(text, payload);
log.debug("decoded paste: {s}", .{text});
return .{
.event = .{ .paste = text },
.n = sequence.len,
};
},
else => return null_event,
}
}
inline fn parseCsi(input: []const u8, text_buf: []u8) Result {
if (input.len < 3) {
return .{
.event = null,
.n = 0,
};
}
// We start iterating at index 2 to get past the '['
const sequence = for (input[2..], 2..) |b, i| {
switch (b) {
0x40...0xFF => break input[0 .. i + 1],
else => continue,
}
} else return .{ .event = null, .n = 0 };
const null_event: Result = .{ .event = null, .n = sequence.len };
const final = sequence[sequence.len - 1];
switch (final) {
'A', 'B', 'C', 'D', 'E', 'F', 'H', 'P', 'Q', 'R', 'S' => {
// Legacy keys
// CSI {ABCDEFHPQS}
// CSI 1 ; modifier:event_type {ABCDEFHPQS}
// Split first into fields delimited by ';'
var field_iter = std.mem.splitScalar(u8, sequence[2 .. sequence.len - 1], ';');
// skip the first field
_ = field_iter.next(); //
var is_release: bool = false;
var key: Key = .{
.codepoint = switch (final) {
'A' => Key.up,
'B' => Key.down,
'C' => Key.right,
'D' => Key.left,
'E' => Key.kp_begin,
'F' => Key.end,
'H' => Key.home,
'P' => Key.f1,
'Q' => Key.f2,
'R' => Key.f3,
'S' => Key.f4,
else => return null_event,
},
};
field2: {
// modifier_mask:event_type
const field_buf = field_iter.next() orelse break :field2;
var param_iter = std.mem.splitScalar(u8, field_buf, ':');
const modifier_buf = param_iter.next() orelse unreachable;
const modifier_mask = parseParam(u8, modifier_buf, 1) orelse return null_event;
key.mods = @bitCast(modifier_mask -| 1);
if (param_iter.next()) |event_type_buf| {
is_release = std.mem.eql(u8, event_type_buf, "3");
}
}
field3: {
// text_as_codepoint[:text_as_codepoint]
const field_buf = field_iter.next() orelse break :field3;
var param_iter = std.mem.splitScalar(u8, field_buf, ':');
var total: usize = 0;
while (param_iter.next()) |cp_buf| {
const cp = parseParam(u21, cp_buf, null) orelse return null_event;
total += std.unicode.utf8Encode(cp, text_buf[total..]) catch return null_event;
}
key.text = text_buf[0..total];
}
const event: Event = if (is_release) .{ .key_release = key } else .{ .key_press = key };
return .{
.event = event,
.n = sequence.len,
};
},
'~' => {
// Legacy keys
// CSI number ~
// CSI number ; modifier ~
// CSI number ; modifier:event_type ; text_as_codepoint ~
var field_iter = std.mem.splitScalar(u8, sequence[2 .. sequence.len - 1], ';');
const number_buf = field_iter.next() orelse unreachable; // always will have one field
const number = parseParam(u16, number_buf, null) orelse return null_event;
var key: Key = .{
.codepoint = switch (number) {
2 => Key.insert,
3 => Key.delete,
5 => Key.page_up,
6 => Key.page_down,
7 => Key.home,
8 => Key.end,
11 => Key.f1,
12 => Key.f2,
13 => Key.f3,
14 => Key.f4,
15 => Key.f5,
17 => Key.f6,
18 => Key.f7,
19 => Key.f8,
20 => Key.f9,
21 => Key.f10,
23 => Key.f11,
24 => Key.f12,
200 => return .{ .event = .paste_start, .n = sequence.len },
201 => return .{ .event = .paste_end, .n = sequence.len },
57427 => Key.kp_begin,
else => return null_event,
},
};
var is_release: bool = false;
field2: {
// modifier_mask:event_type
const field_buf = field_iter.next() orelse break :field2;
var param_iter = std.mem.splitScalar(u8, field_buf, ':');
const modifier_buf = param_iter.next() orelse unreachable;
const modifier_mask = parseParam(u8, modifier_buf, 1) orelse return null_event;
key.mods = @bitCast(modifier_mask -| 1);
if (param_iter.next()) |event_type_buf| {
is_release = std.mem.eql(u8, event_type_buf, "3");
}
}
field3: {
// text_as_codepoint[:text_as_codepoint]
const field_buf = field_iter.next() orelse break :field3;
var param_iter = std.mem.splitScalar(u8, field_buf, ':');
var total: usize = 0;
while (param_iter.next()) |cp_buf| {
const cp = parseParam(u21, cp_buf, null) orelse return null_event;
total += std.unicode.utf8Encode(cp, text_buf[total..]) catch return null_event;
}
key.text = text_buf[0..total];
}
const event: Event = if (is_release) .{ .key_release = key } else .{ .key_press = key };
return .{
.event = event,
.n = sequence.len,
};
},
'I' => return .{ .event = .focus_in, .n = sequence.len },
'O' => return .{ .event = .focus_out, .n = sequence.len },
'M', 'm' => return parseMouse(sequence),
'c' => {
// Primary DA (CSI ? Pm c)
std.debug.assert(sequence.len >= 4); // ESC [ ? c == 4 bytes
switch (input[2]) {
'?' => return .{ .event = .cap_da1, .n = sequence.len },
else => return null_event,
}
},
'n' => {
// Device Status Report
// CSI Ps n
// CSI ? Ps n
std.debug.assert(sequence.len >= 3);
switch (sequence[2]) {
'?' => {
const delim_idx = std.mem.indexOfScalarPos(u8, input, 3, ';') orelse return null_event;
const ps = std.fmt.parseUnsigned(u16, input[3..delim_idx], 10) catch return null_event;
switch (ps) {
997 => {
// Color scheme update (CSI 997 ; Ps n)
// See https://github.com/contour-terminal/contour/blob/master/docs/vt-extensions/color-palette-update-notifications.md
switch (sequence[delim_idx + 1]) {
'1' => return .{
.event = .{ .color_scheme = .dark },
.n = sequence.len,
},
'2' => return .{
.event = .{ .color_scheme = .light },
.n = sequence.len,
},
else => return null_event,
}
},
else => return null_event,
}
},
else => return null_event,
}
},
't' => {
// XTWINOPS
// Split first into fields delimited by ';'
var iter = std.mem.splitScalar(u8, sequence[2 .. sequence.len - 1], ';');
const ps = iter.first();
if (std.mem.eql(u8, "48", ps)) {
// in band window resize
// CSI 48 ; height ; width ; height_pix ; width_pix t
const height_char = iter.next() orelse return null_event;
const width_char = iter.next() orelse return null_event;
const height_pix = iter.next() orelse "0";
const width_pix = iter.next() orelse "0";
const winsize: Winsize = .{
.rows = std.fmt.parseUnsigned(usize, height_char, 10) catch return null_event,
.cols = std.fmt.parseUnsigned(usize, width_char, 10) catch return null_event,
.x_pixel = std.fmt.parseUnsigned(usize, width_pix, 10) catch return null_event,
.y_pixel = std.fmt.parseUnsigned(usize, height_pix, 10) catch return null_event,
};
return .{
.event = .{ .winsize = winsize },
.n = sequence.len,
};
}
return null_event;
},
'u' => {
// Kitty keyboard
// CSI unicode-key-code:alternate-key-codes ; modifiers:event-type ; text-as-codepoints u
// Not all fields will be present. Only unicode-key-code is
// mandatory
if (sequence.len > 2 and sequence[2] == '?') return .{
.event = .cap_kitty_keyboard,
.n = sequence.len,
};
var key: Key = .{
.codepoint = undefined,
};
// Split first into fields delimited by ';'
var field_iter = std.mem.splitScalar(u8, sequence[2 .. sequence.len - 1], ';');
{ // field 1
// unicode-key-code:shifted_codepoint:base_layout_codepoint
const field_buf = field_iter.next() orelse unreachable; // There will always be at least one field
var param_iter = std.mem.splitScalar(u8, field_buf, ':');
const codepoint_buf = param_iter.next() orelse unreachable;
key.codepoint = parseParam(u21, codepoint_buf, null) orelse return null_event;
if (param_iter.next()) |shifted_cp_buf| {
key.shifted_codepoint = parseParam(u21, shifted_cp_buf, null);
}
if (param_iter.next()) |base_layout_buf| {
key.base_layout_codepoint = parseParam(u21, base_layout_buf, null);
}
}
var is_release: bool = false;
field2: {
// modifier_mask:event_type
const field_buf = field_iter.next() orelse break :field2;
var param_iter = std.mem.splitScalar(u8, field_buf, ':');
const modifier_buf = param_iter.next() orelse unreachable;
const modifier_mask = parseParam(u8, modifier_buf, 1) orelse return null_event;
key.mods = @bitCast(modifier_mask -| 1);
if (param_iter.next()) |event_type_buf| {
is_release = std.mem.eql(u8, event_type_buf, "3");
}
}
field3: {
// text_as_codepoint[:text_as_codepoint]
const field_buf = field_iter.next() orelse break :field3;
var param_iter = std.mem.splitScalar(u8, field_buf, ':');
var total: usize = 0;
while (param_iter.next()) |cp_buf| {
const cp = parseParam(u21, cp_buf, null) orelse return null_event;
total += std.unicode.utf8Encode(cp, text_buf[total..]) catch return null_event;
}
key.text = text_buf[0..total];
}
const event: Event = if (is_release)
.{ .key_release = key }
else
.{ .key_press = key };
return .{ .event = event, .n = sequence.len };
},
'y' => {
// DECRPM (CSI ? Ps ; Pm $ y)
const delim_idx = std.mem.indexOfScalarPos(u8, input, 3, ';') orelse return null_event;
const ps = std.fmt.parseUnsigned(u16, input[3..delim_idx], 10) catch return null_event;
const pm = std.fmt.parseUnsigned(u8, input[delim_idx + 1 .. sequence.len - 2], 10) catch return null_event;
switch (ps) {
// Mouse Pixel reporting
1016 => switch (pm) {
0, 4 => return null_event,
else => return .{ .event = .cap_sgr_pixels, .n = sequence.len },
},
// Unicode Core, see https://github.com/contour-terminal/terminal-unicode-core
2027 => switch (pm) {
0, 4 => return null_event,
else => return .{ .event = .cap_unicode, .n = sequence.len },
},
// Color scheme reportnig, see https://github.com/contour-terminal/contour/blob/master/docs/vt-extensions/color-palette-update-notifications.md
2031 => switch (pm) {
0, 4 => return null_event,
else => return .{ .event = .cap_color_scheme_updates, .n = sequence.len },
},
else => return null_event,
}
},
else => return null_event,
}
}
/// Parse a param buffer, returning a default value if the param was empty
inline fn parseParam(comptime T: type, buf: []const u8, default: ?T) ?T {
if (buf.len == 0) return default;
return std.fmt.parseUnsigned(T, buf, 10) catch return null;
}
/// Parse a mouse event
inline fn parseMouse(input: []const u8) Result {
std.debug.assert(input.len >= 4); // ESC [ < [Mm]
const null_event: Result = .{ .event = null, .n = input.len };
if (input[2] != '<') return null_event;
const delim1 = std.mem.indexOfScalarPos(u8, input, 3, ';') orelse return null_event;
const button_mask = parseParam(u16, input[3..delim1], null) orelse return null_event;
const delim2 = std.mem.indexOfScalarPos(u8, input, delim1 + 1, ';') orelse return null_event;
const px = parseParam(u16, input[delim1 + 1 .. delim2], 1) orelse return null_event;
const py = parseParam(u16, input[delim2 + 1 .. input.len - 1], 1) orelse return null_event;
const button: Mouse.Button = @enumFromInt(button_mask & mouse_bits.buttons);
const motion = button_mask & mouse_bits.motion > 0;
const shift = button_mask & mouse_bits.shift > 0;
const alt = button_mask & mouse_bits.alt > 0;
const ctrl = button_mask & mouse_bits.ctrl > 0;
const mouse = Mouse{
.button = button,
.mods = .{
.shift = shift,
.alt = alt,
.ctrl = ctrl,
},
.col = px -| 1,
.row = py -| 1,
.type = blk: {
if (motion and button != Mouse.Button.none) {
break :blk .drag;
}
if (motion and button == Mouse.Button.none) {
break :blk .motion;
}
if (input[input.len - 1] == 'm') break :blk .release;
break :blk .press;
},
};
return .{ .event = .{ .mouse = mouse }, .n = input.len };
}
test "parse: single xterm keypress" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "a";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{
.codepoint = 'a',
.text = "a",
};
const expected_event: Event = .{ .key_press = expected_key };
try testing.expectEqual(1, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: single xterm keypress backspace" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "\x08";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{
.codepoint = Key.backspace,
};
const expected_event: Event = .{ .key_press = expected_key };
try testing.expectEqual(1, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: single xterm keypress with more buffer" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "ab";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{
.codepoint = 'a',
.text = "a",
};
const expected_event: Event = .{ .key_press = expected_key };
try testing.expectEqual(1, result.n);
try testing.expectEqualStrings(expected_key.text.?, result.event.?.key_press.text.?);
try testing.expectEqualDeep(expected_event, result.event);
}
test "parse: xterm escape keypress" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "\x1b";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{ .codepoint = Key.escape };
const expected_event: Event = .{ .key_press = expected_key };
try testing.expectEqual(1, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: xterm ctrl+a" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "\x01";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{ .codepoint = 'a', .mods = .{ .ctrl = true } };
const expected_event: Event = .{ .key_press = expected_key };
try testing.expectEqual(1, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: xterm alt+a" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "\x1ba";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{ .codepoint = 'a', .mods = .{ .alt = true } };
const expected_event: Event = .{ .key_press = expected_key };
try testing.expectEqual(2, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: xterm key up" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
{
// normal version
const input = "\x1b[A";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{ .codepoint = Key.up };
const expected_event: Event = .{ .key_press = expected_key };
try testing.expectEqual(3, result.n);
try testing.expectEqual(expected_event, result.event);
}
{
// application keys version
const input = "\x1bOA";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{ .codepoint = Key.up };
const expected_event: Event = .{ .key_press = expected_key };
try testing.expectEqual(3, result.n);
try testing.expectEqual(expected_event, result.event);
}
}
test "parse: xterm shift+up" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "\x1b[1;2A";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{ .codepoint = Key.up, .mods = .{ .shift = true } };
const expected_event: Event = .{ .key_press = expected_key };
try testing.expectEqual(6, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: xterm insert" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "\x1b[2~";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{ .codepoint = Key.insert, .mods = .{} };
const expected_event: Event = .{ .key_press = expected_key };
try testing.expectEqual(input.len, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: paste_start" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "\x1b[200~";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_event: Event = .paste_start;
try testing.expectEqual(6, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: paste_end" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "\x1b[201~";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_event: Event = .paste_end;
try testing.expectEqual(6, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: osc52 paste" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "\x1b]52;c;b3NjNTIgcGFzdGU=\x1b\\";
const expected_text = "osc52 paste";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
try testing.expectEqual(25, result.n);
switch (result.event.?) {
.paste => |text| {
defer alloc.free(text);
try testing.expectEqualStrings(expected_text, text);
},
else => try testing.expect(false),
}
}
test "parse: focus_in" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "\x1b[I";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_event: Event = .focus_in;
try testing.expectEqual(3, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: focus_out" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "\x1b[O";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_event: Event = .focus_out;
try testing.expectEqual(3, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: kitty: shift+a without text reporting" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "\x1b[97:65;2u";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{
.codepoint = 'a',
.shifted_codepoint = 'A',
.mods = .{ .shift = true },
};
const expected_event: Event = .{ .key_press = expected_key };
try testing.expectEqual(10, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: kitty: alt+shift+a without text reporting" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "\x1b[97:65;4u";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{
.codepoint = 'a',
.shifted_codepoint = 'A',
.mods = .{ .shift = true, .alt = true },
};
const expected_event: Event = .{ .key_press = expected_key };
try testing.expectEqual(10, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: kitty: a without text reporting" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "\x1b[97u";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{
.codepoint = 'a',
};
const expected_event: Event = .{ .key_press = expected_key };
try testing.expectEqual(5, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: kitty: release event" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "\x1b[97;1:3u";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{
.codepoint = 'a',
};
const expected_event: Event = .{ .key_release = expected_key };
try testing.expectEqual(9, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: single codepoint" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "🙂";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{
.codepoint = 0x1F642,
.text = input,
};
const expected_event: Event = .{ .key_press = expected_key };
try testing.expectEqual(4, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: single codepoint with more in buffer" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "🙂a";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{
.codepoint = 0x1F642,
.text = "🙂",
};
const expected_event: Event = .{ .key_press = expected_key };
try testing.expectEqual(4, result.n);
try testing.expectEqualDeep(expected_event, result.event);
}
test "parse: multiple codepoint grapheme" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "👩🚀";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{
.codepoint = Key.multicodepoint,
.text = input,
};
const expected_event: Event = .{ .key_press = expected_key };
try testing.expectEqual(input.len, result.n);
try testing.expectEqual(expected_event, result.event);
}
test "parse: multiple codepoint grapheme with more after" {
const alloc = testing.allocator_instance.allocator();
const grapheme_data = try grapheme.GraphemeData.init(alloc);
defer grapheme_data.deinit();
const input = "👩🚀abc";
var parser: Parser = .{ .grapheme_data = &grapheme_data };
const result = try parser.parse(input, alloc);
const expected_key: Key = .{
.codepoint = Key.multicodepoint,
.text = "👩🚀",
};
try testing.expectEqual(expected_key.text.?.len, result.n);
const actual = result.event.?.key_press;
try testing.expectEqualStrings(expected_key.text.?, actual.text.?);
try testing.expectEqual(expected_key.codepoint, actual.codepoint);
}
test "parse(csi): decrpm" {
var buf: [1]u8 = undefined;
{
const input = "\x1b[?1016;1$y";
const result = parseCsi(input, &buf);
const expected: Result = .{
.event = .cap_sgr_pixels,
.n = input.len,
};
try testing.expectEqual(expected.n, result.n);
try testing.expectEqual(expected.event, result.event);
}
{
const input = "\x1b[?1016;0$y";
const result = parseCsi(input, &buf);
const expected: Result = .{
.event = null,
.n = input.len,
};
try testing.expectEqual(expected.n, result.n);
try testing.expectEqual(expected.event, result.event);
}
}
test "parse(csi): primary da" {
var buf: [1]u8 = undefined;
const input = "\x1b[?c";
const result = parseCsi(input, &buf);
const expected: Result = .{
.event = .cap_da1,
.n = input.len,
};
try testing.expectEqual(expected.n, result.n);
try testing.expectEqual(expected.event, result.event);
}
test "parse(csi): dsr" {
var buf: [1]u8 = undefined;
{
const input = "\x1b[?997;1n";
const result = parseCsi(input, &buf);
const expected: Result = .{
.event = .{ .color_scheme = .dark },
.n = input.len,
};
try testing.expectEqual(expected.n, result.n);
try testing.expectEqual(expected.event, result.event);
}
{
const input = "\x1b[?997;2n";
const result = parseCsi(input, &buf);
const expected: Result = .{
.event = .{ .color_scheme = .light },
.n = input.len,
};
try testing.expectEqual(expected.n, result.n);
try testing.expectEqual(expected.event, result.event);
}
{
const input = "\x1b[0n";
const result = parseCsi(input, &buf);
const expected: Result = .{
.event = null,
.n = input.len,
};
try testing.expectEqual(expected.n, result.n);
try testing.expectEqual(expected.event, result.event);
}
}
test "parse(csi): mouse" {
var buf: [1]u8 = undefined;
const input = "\x1b[<35;1;1m";
const result = parseCsi(input, &buf);
const expected: Result = .{
.event = .{ .mouse = .{
.col = 0,
.row = 0,
.button = .none,
.type = .motion,
.mods = .{},
} },
.n = input.len,
};
try testing.expectEqual(expected.n, result.n);
try testing.expectEqual(expected.event, result.event);
}
|
0 | repos/libvaxis | repos/libvaxis/src/Mouse.zig | /// A mouse event
pub const Mouse = @This();
pub const Shape = enum {
default,
text,
pointer,
help,
progress,
wait,
@"ew-resize",
@"ns-resize",
cell,
};
pub const Button = enum(u8) {
left,
middle,
right,
none,
wheel_up = 64,
wheel_down = 65,
wheel_right = 66,
wheel_left = 67,
button_8 = 128,
button_9 = 129,
button_10 = 130,
button_11 = 131,
};
pub const Modifiers = packed struct(u3) {
shift: bool = false,
alt: bool = false,
ctrl: bool = false,
};
pub const Type = enum {
press,
release,
motion,
drag,
};
col: usize,
row: usize,
xoffset: usize = 0,
yoffset: usize = 0,
button: Button,
mods: Modifiers,
type: Type,
|
0 | repos/libvaxis | repos/libvaxis/src/Unicode.zig | const std = @import("std");
const grapheme = @import("grapheme");
const DisplayWidth = @import("DisplayWidth");
/// A thin wrapper around zg data
const Unicode = @This();
grapheme_data: grapheme.GraphemeData,
width_data: DisplayWidth.DisplayWidthData,
/// initialize all unicode data vaxis may possibly need
pub fn init(alloc: std.mem.Allocator) !Unicode {
return .{
.grapheme_data = try grapheme.GraphemeData.init(alloc),
.width_data = try DisplayWidth.DisplayWidthData.init(alloc),
};
}
/// free all data
pub fn deinit(self: *const Unicode) void {
self.grapheme_data.deinit();
self.width_data.deinit();
}
/// creates a grapheme iterator based on str
pub fn graphemeIterator(self: *const Unicode, str: []const u8) grapheme.Iterator {
return grapheme.Iterator.init(str, &self.grapheme_data);
}
|
0 | repos/libvaxis | repos/libvaxis/src/InternalScreen.zig | const std = @import("std");
const assert = std.debug.assert;
const Style = @import("Cell.zig").Style;
const Cell = @import("Cell.zig");
const MouseShape = @import("Mouse.zig").Shape;
const CursorShape = Cell.CursorShape;
const log = std.log.scoped(.vaxis);
const InternalScreen = @This();
pub const InternalCell = struct {
char: std.ArrayList(u8) = undefined,
style: Style = .{},
uri: std.ArrayList(u8) = undefined,
uri_id: std.ArrayList(u8) = undefined,
// if we got skipped because of a wide character
skipped: bool = false,
default: bool = true,
pub fn eql(self: InternalCell, cell: Cell) bool {
// fastpath when both cells are default
if (self.default and cell.default) return true;
// this is actually faster than std.meta.eql on the individual items.
// Our strings are always small, usually less than 4 bytes so the simd
// usage in std.mem.eql has too much overhead vs looping the bytes
if (!std.mem.eql(u8, self.char.items, cell.char.grapheme)) return false;
if (!Style.eql(self.style, cell.style)) return false;
if (!std.mem.eql(u8, self.uri.items, cell.link.uri)) return false;
if (!std.mem.eql(u8, self.uri_id.items, cell.link.params)) return false;
return true;
}
};
width: usize = 0,
height: usize = 0,
buf: []InternalCell = undefined,
cursor_row: usize = 0,
cursor_col: usize = 0,
cursor_vis: bool = false,
cursor_shape: CursorShape = .default,
mouse_shape: MouseShape = .default,
/// sets each cell to the default cell
pub fn init(alloc: std.mem.Allocator, w: usize, h: usize) !InternalScreen {
var screen = InternalScreen{
.buf = try alloc.alloc(InternalCell, w * h),
};
for (screen.buf, 0..) |_, i| {
screen.buf[i] = .{
.char = try std.ArrayList(u8).initCapacity(alloc, 1),
.uri = std.ArrayList(u8).init(alloc),
.uri_id = std.ArrayList(u8).init(alloc),
};
try screen.buf[i].char.append(' ');
}
screen.width = w;
screen.height = h;
return screen;
}
pub fn deinit(self: *InternalScreen, alloc: std.mem.Allocator) void {
for (self.buf, 0..) |_, i| {
self.buf[i].char.deinit();
self.buf[i].uri.deinit();
self.buf[i].uri_id.deinit();
}
alloc.free(self.buf);
}
/// writes a cell to a location. 0 indexed
pub fn writeCell(
self: *InternalScreen,
col: usize,
row: usize,
cell: Cell,
) void {
if (self.width < col) {
// column out of bounds
return;
}
if (self.height < row) {
// height out of bounds
return;
}
const i = (row * self.width) + col;
assert(i < self.buf.len);
self.buf[i].char.clearRetainingCapacity();
self.buf[i].char.appendSlice(cell.char.grapheme) catch {
log.warn("couldn't write grapheme", .{});
};
self.buf[i].uri.clearRetainingCapacity();
self.buf[i].uri.appendSlice(cell.link.uri) catch {
log.warn("couldn't write uri", .{});
};
self.buf[i].uri_id.clearRetainingCapacity();
self.buf[i].uri_id.appendSlice(cell.link.params) catch {
log.warn("couldn't write uri_id", .{});
};
self.buf[i].style = cell.style;
self.buf[i].default = cell.default;
}
pub fn readCell(self: *InternalScreen, col: usize, row: usize) ?Cell {
if (self.width < col) {
// column out of bounds
return null;
}
if (self.height < row) {
// height out of bounds
return null;
}
const i = (row * self.width) + col;
assert(i < self.buf.len);
return .{
.char = .{ .grapheme = self.buf[i].char.items },
.style = self.buf[i].style,
};
}
|
0 | repos/libvaxis | repos/libvaxis/src/queue.zig | const std = @import("std");
const assert = std.debug.assert;
const atomic = std.atomic;
const Condition = std.Thread.Condition;
/// Thread safe. Fixed size. Blocking push and pop.
pub fn Queue(
comptime T: type,
comptime size: usize,
) type {
return struct {
buf: [size]T = undefined,
read_index: usize = 0,
write_index: usize = 0,
mutex: std.Thread.Mutex = .{},
// blocks when the buffer is full
not_full: Condition = .{},
// ...or empty
not_empty: Condition = .{},
const Self = @This();
/// Pop an item from the queue. Blocks until an item is available.
pub fn pop(self: *Self) T {
self.mutex.lock();
defer self.mutex.unlock();
while (self.isEmptyLH()) {
self.not_empty.wait(&self.mutex);
}
std.debug.assert(!self.isEmptyLH());
if (self.isFullLH()) {
// If we are full, wake up a push that might be
// waiting here.
self.not_full.signal();
}
const result = self.buf[self.mask(self.read_index)];
self.read_index = self.mask2(self.read_index + 1);
return result;
}
/// Push an item into the queue. Blocks until an item has been
/// put in the queue.
pub fn push(self: *Self, item: T) void {
self.mutex.lock();
defer self.mutex.unlock();
while (self.isFullLH()) {
self.not_full.wait(&self.mutex);
}
if (self.isEmptyLH()) {
// If we were empty, wake up a pop if it was waiting.
self.not_empty.signal();
}
std.debug.assert(!self.isFullLH());
self.buf[self.mask(self.write_index)] = item;
self.write_index = self.mask2(self.write_index + 1);
}
/// Push an item into the queue. Returns true when the item
/// was successfully placed in the queue, false if the queue
/// was full.
pub fn tryPush(self: *Self, item: T) bool {
self.mutex.lock();
if (self.isFullLH()) {
self.mutex.unlock();
return false;
}
self.mutex.unlock();
self.push(item);
return true;
}
/// Pop an item from the queue. Returns null when no item is
/// available.
pub fn tryPop(self: *Self) ?T {
self.mutex.lock();
if (self.isEmptyLH()) {
self.mutex.unlock();
return null;
}
self.mutex.unlock();
return self.pop();
}
/// Poll the queue. This call blocks until events are in the queue
pub fn poll(self: *Self) void {
self.mutex.lock();
defer self.mutex.unlock();
while (self.isEmptyLH()) {
self.not_empty.wait(&self.mutex);
}
std.debug.assert(!self.isEmptyLH());
}
fn isEmptyLH(self: Self) bool {
return self.write_index == self.read_index;
}
fn isFullLH(self: Self) bool {
return self.mask2(self.write_index + self.buf.len) ==
self.read_index;
}
/// Returns `true` if the queue is empty and `false` otherwise.
pub fn isEmpty(self: *Self) bool {
self.mutex.lock();
defer self.mutex.unlock();
return self.isEmptyLH();
}
/// Returns `true` if the queue is full and `false` otherwise.
pub fn isFull(self: *Self) bool {
self.mutex.lock();
defer self.mutex.unlock();
return self.isFullLH();
}
/// Returns the length
fn len(self: Self) usize {
const wrap_offset = 2 * self.buf.len *
@intFromBool(self.write_index < self.read_index);
const adjusted_write_index = self.write_index + wrap_offset;
return adjusted_write_index - self.read_index;
}
/// Returns `index` modulo the length of the backing slice.
fn mask(self: Self, index: usize) usize {
return index % self.buf.len;
}
/// Returns `index` modulo twice the length of the backing slice.
fn mask2(self: Self, index: usize) usize {
return index % (2 * self.buf.len);
}
};
}
const testing = std.testing;
const cfg = Thread.SpawnConfig{ .allocator = testing.allocator };
test "Queue: simple push / pop" {
var queue: Queue(u8, 16) = .{};
queue.push(1);
queue.push(2);
const pop = queue.pop();
try testing.expectEqual(1, pop);
try testing.expectEqual(2, queue.pop());
}
const Thread = std.Thread;
fn testPushPop(q: *Queue(u8, 2)) !void {
q.push(3);
try testing.expectEqual(2, q.pop());
}
test "Fill, wait to push, pop once in another thread" {
var queue: Queue(u8, 2) = .{};
queue.push(1);
queue.push(2);
const t = try Thread.spawn(cfg, testPushPop, .{&queue});
try testing.expectEqual(false, queue.tryPush(3));
try testing.expectEqual(1, queue.pop());
t.join();
try testing.expectEqual(3, queue.pop());
try testing.expectEqual(null, queue.tryPop());
}
fn testPush(q: *Queue(u8, 2)) void {
q.push(0);
q.push(1);
q.push(2);
q.push(3);
q.push(4);
}
test "Try to pop, fill from another thread" {
var queue: Queue(u8, 2) = .{};
const thread = try Thread.spawn(cfg, testPush, .{&queue});
for (0..5) |idx| {
try testing.expectEqual(@as(u8, @intCast(idx)), queue.pop());
}
thread.join();
}
fn sleepyPop(q: *Queue(u8, 2)) !void {
// First we wait for the queue to be full.
while (!q.isFull())
try Thread.yield();
// Then we spuriously wake it up, because that's a thing that can
// happen.
q.not_full.signal();
q.not_empty.signal();
// Then give the other thread a good chance of waking up. It's not
// clear that yield guarantees the other thread will be scheduled,
// so we'll throw a sleep in here just to be sure. The queue is
// still full and the push in the other thread is still blocked
// waiting for space.
try Thread.yield();
std.time.sleep(std.time.ns_per_s);
// Finally, let that other thread go.
try std.testing.expectEqual(1, q.pop());
// This won't continue until the other thread has had a chance to
// put at least one item in the queue.
while (!q.isFull())
try Thread.yield();
// But we want to ensure that there's a second push waiting, so
// here's another sleep.
std.time.sleep(std.time.ns_per_s / 2);
// Another spurious wake...
q.not_full.signal();
q.not_empty.signal();
// And another chance for the other thread to see that it's
// spurious and go back to sleep.
try Thread.yield();
std.time.sleep(std.time.ns_per_s / 2);
// Pop that thing and we're done.
try std.testing.expectEqual(2, q.pop());
}
test "Fill, block, fill, block" {
// Fill the queue, block while trying to write another item, have
// a background thread unblock us, then block while trying to
// write yet another thing. Have the background thread unblock
// that too (after some time) then drain the queue. This test
// fails if the while loop in `push` is turned into an `if`.
var queue: Queue(u8, 2) = .{};
const thread = try Thread.spawn(cfg, sleepyPop, .{&queue});
queue.push(1);
queue.push(2);
const now = std.time.milliTimestamp();
queue.push(3); // This one should block.
const then = std.time.milliTimestamp();
// Just to make sure the sleeps are yielding to this thread, make
// sure it took at least 900ms to do the push.
try std.testing.expect(then - now > 900);
// This should block again, waiting for the other thread.
queue.push(4);
// And once that push has gone through, the other thread's done.
thread.join();
try std.testing.expectEqual(3, queue.pop());
try std.testing.expectEqual(4, queue.pop());
}
fn sleepyPush(q: *Queue(u8, 1)) !void {
// Try to ensure the other thread has already started trying to pop.
try Thread.yield();
std.time.sleep(std.time.ns_per_s / 2);
// Spurious wake
q.not_full.signal();
q.not_empty.signal();
try Thread.yield();
std.time.sleep(std.time.ns_per_s / 2);
// Stick something in the queue so it can be popped.
q.push(1);
// Ensure it's been popped.
while (!q.isEmpty())
try Thread.yield();
// Give the other thread time to block again.
try Thread.yield();
std.time.sleep(std.time.ns_per_s / 2);
// Spurious wake
q.not_full.signal();
q.not_empty.signal();
q.push(2);
}
test "Drain, block, drain, block" {
// This is like fill/block/fill/block, but on the pop end. This
// test should fail if the `while` loop in `pop` is turned into an
// `if`.
var queue: Queue(u8, 1) = .{};
const thread = try Thread.spawn(cfg, sleepyPush, .{&queue});
try std.testing.expectEqual(1, queue.pop());
try std.testing.expectEqual(2, queue.pop());
thread.join();
}
fn readerThread(q: *Queue(u8, 1)) !void {
try testing.expectEqual(1, q.pop());
}
test "2 readers" {
// 2 threads read, one thread writes
var queue: Queue(u8, 1) = .{};
const t1 = try Thread.spawn(cfg, readerThread, .{&queue});
const t2 = try Thread.spawn(cfg, readerThread, .{&queue});
try Thread.yield();
std.time.sleep(std.time.ns_per_s / 2);
queue.push(1);
queue.push(1);
t1.join();
t2.join();
}
fn writerThread(q: *Queue(u8, 1)) !void {
q.push(1);
}
test "2 writers" {
var queue: Queue(u8, 1) = .{};
const t1 = try Thread.spawn(cfg, writerThread, .{&queue});
const t2 = try Thread.spawn(cfg, writerThread, .{&queue});
try testing.expectEqual(1, queue.pop());
try testing.expectEqual(1, queue.pop());
t1.join();
t2.join();
}
|
0 | repos/libvaxis | repos/libvaxis/src/aio.zig | const build_options = @import("build_options");
const builtin = @import("builtin");
const std = @import("std");
const vaxis = @import("main.zig");
const handleEventGeneric = @import("Loop.zig").handleEventGeneric;
const log = std.log.scoped(.vaxis_aio);
const Yield = enum { no_state, took_event };
pub fn Loop(T: type) type {
if (!build_options.aio) {
@compileError(
\\build_options.aio is not enabled.
\\Use `LoopWithModules` instead to provide `aio` and `coro` modules from outside vaxis.
);
}
return LoopWithModules(T, @import("aio"), @import("coro"));
}
/// zig-aio based event loop
/// <https://github.com/Cloudef/zig-aio>
pub fn LoopWithModules(T: type, aio: type, coro: type) type {
return struct {
const Event = T;
winsize_task: ?coro.Task.Generic2(winsizeTask) = null,
reader_task: ?coro.Task.Generic2(ttyReaderTask) = null,
queue: std.BoundedArray(T, 512) = .{},
source: aio.EventSource,
fatal: bool = false,
pub fn init() !@This() {
return .{ .source = try aio.EventSource.init() };
}
pub fn deinit(self: *@This(), vx: *vaxis.Vaxis, tty: *vaxis.Tty) void {
vx.deviceStatusReport(tty.anyWriter()) catch {};
if (self.winsize_task) |task| task.cancel();
if (self.reader_task) |task| task.cancel();
self.source.deinit();
self.* = undefined;
}
fn winsizeInner(self: *@This(), tty: *vaxis.Tty) !void {
const Context = struct {
loop: *@TypeOf(self.*),
tty: *vaxis.Tty,
winsize: ?vaxis.Winsize = null,
fn cb(ptr: *anyopaque) void {
std.debug.assert(coro.current() == null);
const ctx: *@This() = @ptrCast(@alignCast(ptr));
ctx.winsize = vaxis.Tty.getWinsize(ctx.tty.fd) catch return;
ctx.loop.source.notify();
}
};
// keep on stack
var ctx: Context = .{ .loop = self, .tty = tty };
if (builtin.target.os.tag != .windows) {
if (@hasField(Event, "winsize")) {
const handler: vaxis.Tty.SignalHandler = .{ .context = &ctx, .callback = Context.cb };
try vaxis.Tty.notifyWinsize(handler);
}
}
while (true) {
try coro.io.single(aio.WaitEventSource{ .source = &self.source });
if (ctx.winsize) |winsize| {
if (!@hasField(Event, "winsize")) unreachable;
ctx.loop.postEvent(.{ .winsize = winsize }) catch {};
ctx.winsize = null;
}
}
}
fn winsizeTask(self: *@This(), tty: *vaxis.Tty) void {
self.winsizeInner(tty) catch |err| {
if (err != error.Canceled) log.err("winsize: {}", .{err});
self.fatal = true;
};
}
fn windowsReadEvent(tty: *vaxis.Tty) !vaxis.Event {
var state: vaxis.Tty.EventState = .{};
while (true) {
var bytes_read: usize = 0;
var input_record: vaxis.Tty.INPUT_RECORD = undefined;
try coro.io.single(aio.ReadTty{
.tty = .{ .handle = tty.stdin },
.buffer = std.mem.asBytes(&input_record),
.out_read = &bytes_read,
});
if (try tty.eventFromRecord(&input_record, &state)) |ev| {
return ev;
}
}
}
fn ttyReaderWindows(self: *@This(), vx: *vaxis.Vaxis, tty: *vaxis.Tty) !void {
var cache: vaxis.GraphemeCache = .{};
while (true) {
const event = try windowsReadEvent(tty);
try handleEventGeneric(self, vx, &cache, Event, event, null);
}
}
fn ttyReaderPosix(self: *@This(), vx: *vaxis.Vaxis, tty: *vaxis.Tty, paste_allocator: ?std.mem.Allocator) !void {
// initialize a grapheme cache
var cache: vaxis.GraphemeCache = .{};
// get our initial winsize
const winsize = try vaxis.Tty.getWinsize(tty.fd);
if (@hasField(Event, "winsize")) {
try self.postEvent(.{ .winsize = winsize });
}
var parser: vaxis.Parser = .{
.grapheme_data = &vx.unicode.grapheme_data,
};
const file: std.fs.File = .{ .handle = tty.fd };
while (true) {
var buf: [4096]u8 = undefined;
var n: usize = undefined;
var read_start: usize = 0;
try coro.io.single(aio.ReadTty{ .tty = file, .buffer = buf[read_start..], .out_read = &n });
var seq_start: usize = 0;
while (seq_start < n) {
const result = try parser.parse(buf[seq_start..n], paste_allocator);
if (result.n == 0) {
// copy the read to the beginning. We don't use memcpy because
// this could be overlapping, and it's also rare
const initial_start = seq_start;
while (seq_start < n) : (seq_start += 1) {
buf[seq_start - initial_start] = buf[seq_start];
}
read_start = seq_start - initial_start + 1;
continue;
}
read_start = 0;
seq_start += result.n;
const event = result.event orelse continue;
try handleEventGeneric(self, vx, &cache, Event, event, paste_allocator);
}
}
}
fn ttyReaderTask(self: *@This(), vx: *vaxis.Vaxis, tty: *vaxis.Tty, paste_allocator: ?std.mem.Allocator) void {
return switch (builtin.target.os.tag) {
.windows => self.ttyReaderWindows(vx, tty),
else => self.ttyReaderPosix(vx, tty, paste_allocator),
} catch |err| {
if (err != error.Canceled) log.err("ttyReader: {}", .{err});
self.fatal = true;
};
}
/// Spawns tasks to handle winsize signal and tty
pub fn spawn(
self: *@This(),
scheduler: *coro.Scheduler,
vx: *vaxis.Vaxis,
tty: *vaxis.Tty,
paste_allocator: ?std.mem.Allocator,
spawn_options: coro.Scheduler.SpawnOptions,
) coro.Scheduler.SpawnError!void {
if (self.reader_task) |_| unreachable; // programming error
// This is required even if app doesn't care about winsize
// It is because it consumes the EventSource, so it can wakeup the scheduler
// Without that custom `postEvent`'s wouldn't wake up the scheduler and UI wouldn't update
self.winsize_task = try scheduler.spawn(winsizeTask, .{ self, tty }, spawn_options);
self.reader_task = try scheduler.spawn(ttyReaderTask, .{ self, vx, tty, paste_allocator }, spawn_options);
}
pub const PopEventError = error{TtyCommunicationSevered};
/// Call this in a while loop in the main event handler until it returns null
pub fn popEvent(self: *@This()) PopEventError!?T {
if (self.fatal) return error.TtyCommunicationSevered;
defer self.winsize_task.?.wakeupIf(Yield.took_event);
defer self.reader_task.?.wakeupIf(Yield.took_event);
return self.queue.popOrNull();
}
pub const PostEventError = error{Overflow};
pub fn postEvent(self: *@This(), event: T) !void {
if (coro.current()) |_| {
while (true) {
self.queue.insert(0, event) catch {
// wait for the app to take event
try coro.yield(Yield.took_event);
continue;
};
break;
}
} else {
// queue can be full, app could handle this error by spinning the scheduler
try self.queue.insert(0, event);
}
// wakes up the scheduler, so custom events update UI
self.source.notify();
}
};
}
|
0 | repos/libvaxis | repos/libvaxis/src/ctlseqs.zig | // Queries
pub const primary_device_attrs = "\x1b[c";
pub const tertiary_device_attrs = "\x1b[=c";
pub const device_status_report = "\x1b[5n";
pub const xtversion = "\x1b[>0q";
pub const decrqm_focus = "\x1b[?1004$p";
pub const decrqm_sgr_pixels = "\x1b[?1016$p";
pub const decrqm_sync = "\x1b[?2026$p";
pub const decrqm_unicode = "\x1b[?2027$p";
pub const decrqm_color_scheme = "\x1b[?2031$p";
pub const csi_u_query = "\x1b[?u";
pub const kitty_graphics_query = "\x1b_Gi=1,a=q\x1b\\";
pub const sixel_geometry_query = "\x1b[?2;1;0S";
// mouse. We try for button motion and any motion. terminals will enable the
// last one we tried (any motion). This was added because zellij doesn't
// support any motion currently
// See: https://github.com/zellij-org/zellij/issues/1679
pub const mouse_set = "\x1b[?1002;1003;1004;1006h";
pub const mouse_set_pixels = "\x1b[?1002;1003;1004;1016h";
pub const mouse_reset = "\x1b[?1002;1003;1004;1006;1016l";
// in-band window size reports
pub const in_band_resize_set = "\x1b[?2048h";
pub const in_band_resize_reset = "\x1b[?2048l";
// sync
pub const sync_set = "\x1b[?2026h";
pub const sync_reset = "\x1b[?2026l";
// unicode
pub const unicode_set = "\x1b[?2027h";
pub const unicode_reset = "\x1b[?2027l";
// bracketed paste
pub const bp_set = "\x1b[?2004h";
pub const bp_reset = "\x1b[?2004l";
// color scheme updates
pub const color_scheme_request = "\x1b[?996n";
pub const color_scheme_set = "\x1b[?2031h";
pub const color_scheme_reset = "\x1b[?2031l";
// Key encoding
pub const csi_u_push = "\x1b[>{d}u";
pub const csi_u_pop = "\x1b[<u";
// Cursor
pub const home = "\x1b[H";
pub const cup = "\x1b[{d};{d}H";
pub const hide_cursor = "\x1b[?25l";
pub const show_cursor = "\x1b[?25h";
pub const cursor_shape = "\x1b[{d} q";
pub const ri = "\x1bM";
pub const ind = "\n";
pub const cuf = "\x1b[{d}C";
pub const cub = "\x1b[{d}D";
// Erase
pub const erase_below_cursor = "\x1b[J";
// alt screen
pub const smcup = "\x1b[?1049h";
pub const rmcup = "\x1b[?1049l";
// sgr reset all
pub const sgr_reset = "\x1b[m";
// colors
pub const fg_base = "\x1b[3{d}m";
pub const fg_bright = "\x1b[9{d}m";
pub const bg_base = "\x1b[4{d}m";
pub const bg_bright = "\x1b[10{d}m";
pub const fg_reset = "\x1b[39m";
pub const bg_reset = "\x1b[49m";
pub const ul_reset = "\x1b[59m";
pub const fg_indexed = "\x1b[38:5:{d}m";
pub const bg_indexed = "\x1b[48:5:{d}m";
pub const ul_indexed = "\x1b[58:5:{d}m";
pub const fg_rgb = "\x1b[38:2:{d}:{d}:{d}m";
pub const bg_rgb = "\x1b[48:2:{d}:{d}:{d}m";
pub const ul_rgb = "\x1b[58:2:{d}:{d}:{d}m";
pub const fg_indexed_legacy = "\x1b[38;5;{d}m";
pub const bg_indexed_legacy = "\x1b[48;5;{d}m";
pub const ul_indexed_legacy = "\x1b[58;5;{d}m";
pub const fg_rgb_legacy = "\x1b[38;2;{d};{d};{d}m";
pub const bg_rgb_legacy = "\x1b[48;2;{d};{d};{d}m";
pub const ul_rgb_legacy = "\x1b[58;2;{d};{d};{d}m";
// Underlines
pub const ul_off = "\x1b[24m"; // NOTE: this could be \x1b[4:0m but is not as widely supported
pub const ul_single = "\x1b[4m";
pub const ul_double = "\x1b[4:2m";
pub const ul_curly = "\x1b[4:3m";
pub const ul_dotted = "\x1b[4:4m";
pub const ul_dashed = "\x1b[4:5m";
// Attributes
pub const bold_set = "\x1b[1m";
pub const dim_set = "\x1b[2m";
pub const italic_set = "\x1b[3m";
pub const blink_set = "\x1b[5m";
pub const reverse_set = "\x1b[7m";
pub const invisible_set = "\x1b[8m";
pub const strikethrough_set = "\x1b[9m";
pub const bold_dim_reset = "\x1b[22m";
pub const italic_reset = "\x1b[23m";
pub const blink_reset = "\x1b[25m";
pub const reverse_reset = "\x1b[27m";
pub const invisible_reset = "\x1b[28m";
pub const strikethrough_reset = "\x1b[29m";
// OSC sequences
pub const osc2_set_title = "\x1b]2;{s}\x1b\\";
pub const osc7 = "\x1b]7;{;+/}\x1b\\";
pub const osc8 = "\x1b]8;{s};{s}\x1b\\";
pub const osc8_clear = "\x1b]8;;\x1b\\";
pub const osc9_notify = "\x1b]9;{s}\x1b\\";
pub const osc777_notify = "\x1b]777;notify;{s};{s}\x1b\\";
pub const osc22_mouse_shape = "\x1b]22;{s}\x1b\\";
pub const osc52_clipboard_copy = "\x1b]52;c;{s}\x1b\\";
pub const osc52_clipboard_request = "\x1b]52;c;?\x1b\\";
// Kitty graphics
pub const kitty_graphics_clear = "\x1b_Ga=d\x1b\\";
pub const kitty_graphics_preamble = "\x1b_Ga=p,i={d}";
pub const kitty_graphics_closing = ",C=1\x1b\\";
// Color control sequences
pub const osc4_query = "\x1b]4;{d};?\x1b\\"; // color index {d}
pub const osc4_reset = "\x1b]104\x1b\\"; // this resets _all_ color indexes
pub const osc10_query = "\x1b]10;?\x1b\\"; // fg
pub const osc10_set = "\x1b]10;rgb:{x:0>2}{x:0>2}/{x:0>2}{x:0>2}/{x:0>2}{x:0>2}\x1b\\"; // set default terminal fg
pub const osc10_reset = "\x1b]110\x1b\\"; // reset fg to terminal default
pub const osc11_query = "\x1b]11;?\x1b\\"; // bg
pub const osc11_set = "\x1b]11;rgb:{x:0>2}{x:0>2}/{x:0>2}{x:0>2}/{x:0>2}{x:0>2}\x1b\\"; // set default terminal bg
pub const osc11_reset = "\x1b]111\x1b\\"; // reset bg to terminal default
pub const osc12_query = "\x1b]12;?\x1b\\"; // cursor color
pub const osc12_reset = "\x1b]112\x1b\\"; // reset cursor to terminal default
|
0 | repos/libvaxis/src | repos/libvaxis/src/posix/Tty.zig | //! TTY implementation conforming to posix standards
const Posix = @This();
const std = @import("std");
const builtin = @import("builtin");
const posix = std.posix;
const Winsize = @import("../main.zig").Winsize;
/// the original state of the terminal, prior to calling makeRaw
termios: posix.termios,
/// The file descriptor of the tty
fd: posix.fd_t,
pub const SignalHandler = struct {
context: *anyopaque,
callback: *const fn (context: *anyopaque) void,
};
/// global signal handlers
var handlers: [8]SignalHandler = undefined;
var handler_mutex: std.Thread.Mutex = .{};
var handler_idx: usize = 0;
/// global tty instance, used in case of a panic. Not guaranteed to work if
/// for some reason there are multiple TTYs open under a single vaxis
/// compilation unit - but this is better than nothing
pub var global_tty: ?Posix = null;
/// initializes a Tty instance by opening /dev/tty and "making it raw". A
/// signal handler is installed for SIGWINCH. No callbacks are installed, be
/// sure to register a callback when initializing the event loop
pub fn init() !Posix {
// Open our tty
const fd = try posix.open("/dev/tty", .{ .ACCMODE = .RDWR }, 0);
// Set the termios of the tty
const termios = try makeRaw(fd);
var act = posix.Sigaction{
.handler = .{ .handler = Posix.handleWinch },
.mask = switch (builtin.os.tag) {
.macos => 0,
.linux => posix.empty_sigset,
.freebsd => posix.empty_sigset,
else => @compileError("os not supported"),
},
.flags = 0,
};
try posix.sigaction(posix.SIG.WINCH, &act, null);
const self: Posix = .{
.fd = fd,
.termios = termios,
};
global_tty = self;
return self;
}
/// release resources associated with the Tty return it to its original state
pub fn deinit(self: Posix) void {
posix.tcsetattr(self.fd, .FLUSH, self.termios) catch |err| {
std.log.err("couldn't restore terminal: {}", .{err});
};
if (builtin.os.tag != .macos) // closing /dev/tty may block indefinitely on macos
posix.close(self.fd);
}
/// Write bytes to the tty
pub fn write(self: *const Posix, bytes: []const u8) !usize {
return posix.write(self.fd, bytes);
}
pub fn opaqueWrite(ptr: *const anyopaque, bytes: []const u8) !usize {
const self: *const Posix = @ptrCast(@alignCast(ptr));
return posix.write(self.fd, bytes);
}
pub fn anyWriter(self: *const Posix) std.io.AnyWriter {
return .{
.context = self,
.writeFn = Posix.opaqueWrite,
};
}
pub fn read(self: *const Posix, buf: []u8) !usize {
return posix.read(self.fd, buf);
}
pub fn opaqueRead(ptr: *const anyopaque, buf: []u8) !usize {
const self: *const Posix = @ptrCast(@alignCast(ptr));
return posix.read(self.fd, buf);
}
pub fn anyReader(self: *const Posix) std.io.AnyReader {
return .{
.context = self,
.readFn = Posix.opaqueRead,
};
}
/// Install a signal handler for winsize. A maximum of 8 handlers may be
/// installed
pub fn notifyWinsize(handler: SignalHandler) !void {
handler_mutex.lock();
defer handler_mutex.unlock();
if (handler_idx == handlers.len) return error.OutOfMemory;
handlers[handler_idx] = handler;
handler_idx += 1;
}
fn handleWinch(_: c_int) callconv(.C) void {
handler_mutex.lock();
defer handler_mutex.unlock();
var i: usize = 0;
while (i < handler_idx) : (i += 1) {
const handler = handlers[i];
handler.callback(handler.context);
}
}
/// makeRaw enters the raw state for the terminal.
pub fn makeRaw(fd: posix.fd_t) !posix.termios {
const state = try posix.tcgetattr(fd);
var raw = state;
// see termios(3)
raw.iflag.IGNBRK = false;
raw.iflag.BRKINT = false;
raw.iflag.PARMRK = false;
raw.iflag.ISTRIP = false;
raw.iflag.INLCR = false;
raw.iflag.IGNCR = false;
raw.iflag.ICRNL = false;
raw.iflag.IXON = false;
raw.oflag.OPOST = false;
raw.lflag.ECHO = false;
raw.lflag.ECHONL = false;
raw.lflag.ICANON = false;
raw.lflag.ISIG = false;
raw.lflag.IEXTEN = false;
raw.cflag.CSIZE = .CS8;
raw.cflag.PARENB = false;
raw.cc[@intFromEnum(posix.V.MIN)] = 1;
raw.cc[@intFromEnum(posix.V.TIME)] = 0;
try posix.tcsetattr(fd, .FLUSH, raw);
return state;
}
/// Get the window size from the kernel
pub fn getWinsize(fd: posix.fd_t) !Winsize {
var winsize = posix.winsize{
.ws_row = 0,
.ws_col = 0,
.ws_xpixel = 0,
.ws_ypixel = 0,
};
const err = posix.system.ioctl(fd, posix.T.IOCGWINSZ, @intFromPtr(&winsize));
if (posix.errno(err) == .SUCCESS)
return Winsize{
.rows = winsize.ws_row,
.cols = winsize.ws_col,
.x_pixel = winsize.ws_xpixel,
.y_pixel = winsize.ws_ypixel,
};
return error.IoctlError;
}
pub fn bufferedWriter(self: *const Posix) std.io.BufferedWriter(4096, std.io.AnyWriter) {
return std.io.bufferedWriter(self.anyWriter());
}
|
0 | repos/libvaxis/src | repos/libvaxis/src/windows/Tty.zig | //! A Windows TTY implementation, using virtual terminal process output and
//! native windows input
const Tty = @This();
const std = @import("std");
const Event = @import("../event.zig").Event;
const Key = @import("../Key.zig");
const Mouse = @import("../Mouse.zig");
const Parser = @import("../Parser.zig");
const windows = std.os.windows;
stdin: windows.HANDLE,
stdout: windows.HANDLE,
initial_codepage: c_uint,
initial_input_mode: u32,
initial_output_mode: u32,
// a buffer to write key text into
buf: [4]u8 = undefined,
/// The last mouse button that was pressed. We store the previous state of button presses on each
/// mouse event so we can detect which button was released
last_mouse_button_press: u16 = 0,
pub var global_tty: ?Tty = null;
const utf8_codepage: c_uint = 65001;
const InputMode = struct {
const enable_window_input: u32 = 0x0008; // resize events
const enable_mouse_input: u32 = 0x0010;
const enable_extended_flags: u32 = 0x0080; // allows mouse events
pub fn rawMode() u32 {
return enable_window_input | enable_mouse_input | enable_extended_flags;
}
};
const OutputMode = struct {
const enable_processed_output: u32 = 0x0001; // handle control sequences
const enable_virtual_terminal_processing: u32 = 0x0004; // handle ANSI sequences
const disable_newline_auto_return: u32 = 0x0008; // disable inserting a new line when we write at the last column
const enable_lvb_grid_worldwide: u32 = 0x0010; // enables reverse video and underline
fn rawMode() u32 {
return enable_processed_output |
enable_virtual_terminal_processing |
disable_newline_auto_return |
enable_lvb_grid_worldwide;
}
};
pub fn init() !Tty {
const stdin = try windows.GetStdHandle(windows.STD_INPUT_HANDLE);
const stdout = try windows.GetStdHandle(windows.STD_OUTPUT_HANDLE);
// get initial modes
var initial_input_mode: windows.DWORD = undefined;
var initial_output_mode: windows.DWORD = undefined;
const initial_output_codepage = windows.kernel32.GetConsoleOutputCP();
{
if (windows.kernel32.GetConsoleMode(stdin, &initial_input_mode) == 0) {
return windows.unexpectedError(windows.kernel32.GetLastError());
}
if (windows.kernel32.GetConsoleMode(stdout, &initial_output_mode) == 0) {
return windows.unexpectedError(windows.kernel32.GetLastError());
}
}
// set new modes
{
if (SetConsoleMode(stdin, InputMode.rawMode()) == 0)
return windows.unexpectedError(windows.kernel32.GetLastError());
if (SetConsoleMode(stdout, OutputMode.rawMode()) == 0)
return windows.unexpectedError(windows.kernel32.GetLastError());
if (windows.kernel32.SetConsoleOutputCP(utf8_codepage) == 0)
return windows.unexpectedError(windows.kernel32.GetLastError());
}
const self: Tty = .{
.stdin = stdin,
.stdout = stdout,
.initial_codepage = initial_output_codepage,
.initial_input_mode = initial_input_mode,
.initial_output_mode = initial_output_mode,
};
// save a copy of this tty as the global_tty for panic handling
global_tty = self;
return self;
}
pub fn deinit(self: Tty) void {
_ = windows.kernel32.SetConsoleOutputCP(self.initial_codepage);
_ = SetConsoleMode(self.stdin, self.initial_input_mode);
_ = SetConsoleMode(self.stdout, self.initial_output_mode);
windows.CloseHandle(self.stdin);
windows.CloseHandle(self.stdout);
}
pub fn opaqueWrite(ptr: *const anyopaque, bytes: []const u8) !usize {
const self: *const Tty = @ptrCast(@alignCast(ptr));
return windows.WriteFile(self.stdout, bytes, null);
}
pub fn anyWriter(self: *const Tty) std.io.AnyWriter {
return .{
.context = self,
.writeFn = Tty.opaqueWrite,
};
}
pub fn bufferedWriter(self: *const Tty) std.io.BufferedWriter(4096, std.io.AnyWriter) {
return std.io.bufferedWriter(self.anyWriter());
}
pub fn nextEvent(self: *Tty, parser: *Parser, paste_allocator: ?std.mem.Allocator) !Event {
// We use a loop so we can ignore certain events
var state: EventState = .{};
while (true) {
var event_count: u32 = 0;
var input_record: INPUT_RECORD = undefined;
if (ReadConsoleInputW(self.stdin, &input_record, 1, &event_count) == 0)
return windows.unexpectedError(windows.kernel32.GetLastError());
if (try self.eventFromRecord(&input_record, &state, parser, paste_allocator)) |ev| {
return ev;
}
}
}
pub const EventState = struct {
ansi_buf: [128]u8 = undefined,
ansi_idx: usize = 0,
utf16_buf: [2]u16 = undefined,
utf16_half: bool = false,
};
pub fn eventFromRecord(self: *Tty, record: *const INPUT_RECORD, state: *EventState, parser: *Parser, paste_allocator: ?std.mem.Allocator) !?Event {
switch (record.EventType) {
0x0001 => { // Key event
const event = record.Event.KeyEvent;
if (state.utf16_half) half: {
state.utf16_half = false;
state.utf16_buf[1] = event.uChar.UnicodeChar;
const codepoint: u21 = std.unicode.utf16DecodeSurrogatePair(&state.utf16_buf) catch break :half;
const n = std.unicode.utf8Encode(codepoint, &self.buf) catch return null;
const key: Key = .{
.codepoint = codepoint,
.base_layout_codepoint = codepoint,
.mods = translateMods(event.dwControlKeyState),
.text = self.buf[0..n],
};
switch (event.bKeyDown) {
0 => return .{ .key_release = key },
else => return .{ .key_press = key },
}
}
const base_layout: u16 = switch (event.wVirtualKeyCode) {
0x00 => blk: { // delivered when we get an escape sequence or a unicode codepoint
if (state.ansi_idx == 0 and event.uChar.AsciiChar != 27)
break :blk event.uChar.UnicodeChar;
state.ansi_buf[state.ansi_idx] = event.uChar.AsciiChar;
state.ansi_idx += 1;
if (state.ansi_idx <= 2) return null;
const result = try parser.parse(state.ansi_buf[0..state.ansi_idx], paste_allocator);
return if (result.n == 0) null else evt: {
state.ansi_idx = 0;
break :evt result.event;
};
},
0x08 => Key.backspace,
0x09 => Key.tab,
0x0D => Key.enter,
0x13 => Key.pause,
0x14 => Key.caps_lock,
0x1B => Key.escape,
0x20 => Key.space,
0x21 => Key.page_up,
0x22 => Key.page_down,
0x23 => Key.end,
0x24 => Key.home,
0x25 => Key.left,
0x26 => Key.up,
0x27 => Key.right,
0x28 => Key.down,
0x2c => Key.print_screen,
0x2d => Key.insert,
0x2e => Key.delete,
0x30...0x39 => |k| k,
0x41...0x5a => |k| k + 0x20, // translate to lowercase
0x5b => Key.left_meta,
0x5c => Key.right_meta,
0x60 => Key.kp_0,
0x61 => Key.kp_1,
0x62 => Key.kp_2,
0x63 => Key.kp_3,
0x64 => Key.kp_4,
0x65 => Key.kp_5,
0x66 => Key.kp_6,
0x67 => Key.kp_7,
0x68 => Key.kp_8,
0x69 => Key.kp_9,
0x6a => Key.kp_multiply,
0x6b => Key.kp_add,
0x6c => Key.kp_separator,
0x6d => Key.kp_subtract,
0x6e => Key.kp_decimal,
0x6f => Key.kp_divide,
0x70 => Key.f1,
0x71 => Key.f2,
0x72 => Key.f3,
0x73 => Key.f4,
0x74 => Key.f5,
0x75 => Key.f6,
0x76 => Key.f8,
0x77 => Key.f8,
0x78 => Key.f9,
0x79 => Key.f10,
0x7a => Key.f11,
0x7b => Key.f12,
0x7c => Key.f13,
0x7d => Key.f14,
0x7e => Key.f15,
0x7f => Key.f16,
0x80 => Key.f17,
0x81 => Key.f18,
0x82 => Key.f19,
0x83 => Key.f20,
0x84 => Key.f21,
0x85 => Key.f22,
0x86 => Key.f23,
0x87 => Key.f24,
0x90 => Key.num_lock,
0x91 => Key.scroll_lock,
0xa0 => Key.left_shift,
0xa1 => Key.right_shift,
0xa2 => Key.left_control,
0xa3 => Key.right_control,
0xa4 => Key.left_alt,
0xa5 => Key.right_alt,
0xad => Key.mute_volume,
0xae => Key.lower_volume,
0xaf => Key.raise_volume,
0xb0 => Key.media_track_next,
0xb1 => Key.media_track_previous,
0xb2 => Key.media_stop,
0xb3 => Key.media_play_pause,
0xba => ';',
0xbb => '+',
0xbc => ',',
0xbd => '-',
0xbe => '.',
0xbf => '/',
0xc0 => '`',
0xdb => '[',
0xdc => '\\',
0xdd => ']',
0xde => '\'',
else => return null,
};
if (std.unicode.utf16IsHighSurrogate(base_layout)) {
state.utf16_buf[0] = base_layout;
state.utf16_half = true;
return null;
}
if (std.unicode.utf16IsLowSurrogate(base_layout)) {
return null;
}
var codepoint: u21 = base_layout;
var text: ?[]const u8 = null;
switch (event.uChar.UnicodeChar) {
0x00...0x1F => {},
else => |cp| {
codepoint = cp;
const n = try std.unicode.utf8Encode(codepoint, &self.buf);
text = self.buf[0..n];
},
}
const key: Key = .{
.codepoint = codepoint,
.base_layout_codepoint = base_layout,
.mods = translateMods(event.dwControlKeyState),
.text = text,
};
switch (event.bKeyDown) {
0 => return .{ .key_release = key },
else => return .{ .key_press = key },
}
},
0x0002 => { // Mouse event
// see https://learn.microsoft.com/en-us/windows/console/mouse-event-record-str
const event = record.Event.MouseEvent;
// High word of dwButtonState represents mouse wheel. Positive is wheel_up, negative
// is wheel_down
// Low word represents button state
const mouse_wheel_direction: i16 = blk: {
const wheelu32: u32 = event.dwButtonState >> 16;
const wheelu16: u16 = @truncate(wheelu32);
break :blk @bitCast(wheelu16);
};
const buttons: u16 = @truncate(event.dwButtonState);
// save the current state when we are done
defer self.last_mouse_button_press = buttons;
const button_xor = self.last_mouse_button_press ^ buttons;
var event_type: Mouse.Type = .press;
const btn: Mouse.Button = switch (button_xor) {
0x0000 => blk: {
// Check wheel event
if (event.dwEventFlags & 0x0004 > 0) {
if (mouse_wheel_direction > 0)
break :blk .wheel_up
else
break :blk .wheel_down;
}
// If we have no change but one of the buttons is still pressed we have a
// drag event. Find out which button is held down
if (buttons > 0 and event.dwEventFlags & 0x0001 > 0) {
event_type = .drag;
if (buttons & 0x0001 > 0) break :blk .left;
if (buttons & 0x0002 > 0) break :blk .right;
if (buttons & 0x0004 > 0) break :blk .middle;
if (buttons & 0x0008 > 0) break :blk .button_8;
if (buttons & 0x0010 > 0) break :blk .button_9;
}
if (event.dwEventFlags & 0x0001 > 0) event_type = .motion;
break :blk .none;
},
0x0001 => blk: {
if (buttons & 0x0001 == 0) event_type = .release;
break :blk .left;
},
0x0002 => blk: {
if (buttons & 0x0002 == 0) event_type = .release;
break :blk .right;
},
0x0004 => blk: {
if (buttons & 0x0004 == 0) event_type = .release;
break :blk .middle;
},
0x0008 => blk: {
if (buttons & 0x0008 == 0) event_type = .release;
break :blk .button_8;
},
0x0010 => blk: {
if (buttons & 0x0010 == 0) event_type = .release;
break :blk .button_9;
},
else => {
std.log.warn("unknown mouse event: {}", .{event});
return null;
},
};
const shift: u32 = 0x0010;
const alt: u32 = 0x0001 | 0x0002;
const ctrl: u32 = 0x0004 | 0x0008;
const mods: Mouse.Modifiers = .{
.shift = event.dwControlKeyState & shift > 0,
.alt = event.dwControlKeyState & alt > 0,
.ctrl = event.dwControlKeyState & ctrl > 0,
};
const mouse: Mouse = .{
.col = @as(u16, @bitCast(event.dwMousePosition.X)), // Windows reports with 0 index
.row = @as(u16, @bitCast(event.dwMousePosition.Y)), // Windows reports with 0 index
.mods = mods,
.type = event_type,
.button = btn,
};
return .{ .mouse = mouse };
},
0x0004 => { // Screen resize events
// NOTE: Even though the event comes with a size, it may not be accurate. We ask for
// the size directly when we get this event
var console_info: windows.CONSOLE_SCREEN_BUFFER_INFO = undefined;
if (windows.kernel32.GetConsoleScreenBufferInfo(self.stdout, &console_info) == 0) {
return windows.unexpectedError(windows.kernel32.GetLastError());
}
const window_rect = console_info.srWindow;
const width = window_rect.Right - window_rect.Left + 1;
const height = window_rect.Bottom - window_rect.Top + 1;
return .{
.winsize = .{
.cols = @intCast(width),
.rows = @intCast(height),
.x_pixel = 0,
.y_pixel = 0,
},
};
},
0x0010 => { // Focus events
switch (record.Event.FocusEvent.bSetFocus) {
0 => return .focus_out,
else => return .focus_in,
}
},
else => {},
}
return null;
}
fn translateMods(mods: u32) Key.Modifiers {
const left_alt: u32 = 0x0002;
const right_alt: u32 = 0x0001;
const left_ctrl: u32 = 0x0008;
const right_ctrl: u32 = 0x0004;
const caps: u32 = 0x0080;
const num_lock: u32 = 0x0020;
const shift: u32 = 0x0010;
const alt: u32 = left_alt | right_alt;
const ctrl: u32 = left_ctrl | right_ctrl;
return .{
.shift = mods & shift > 0,
.alt = mods & alt > 0,
.ctrl = mods & ctrl > 0,
.caps_lock = mods & caps > 0,
.num_lock = mods & num_lock > 0,
};
}
// From gitub.com/ziglibs/zig-windows-console. Thanks :)
//
// Events
const union_unnamed_248 = extern union {
UnicodeChar: windows.WCHAR,
AsciiChar: windows.CHAR,
};
pub const KEY_EVENT_RECORD = extern struct {
bKeyDown: windows.BOOL,
wRepeatCount: windows.WORD,
wVirtualKeyCode: windows.WORD,
wVirtualScanCode: windows.WORD,
uChar: union_unnamed_248,
dwControlKeyState: windows.DWORD,
};
pub const PKEY_EVENT_RECORD = *KEY_EVENT_RECORD;
pub const MOUSE_EVENT_RECORD = extern struct {
dwMousePosition: windows.COORD,
dwButtonState: windows.DWORD,
dwControlKeyState: windows.DWORD,
dwEventFlags: windows.DWORD,
};
pub const PMOUSE_EVENT_RECORD = *MOUSE_EVENT_RECORD;
pub const WINDOW_BUFFER_SIZE_RECORD = extern struct {
dwSize: windows.COORD,
};
pub const PWINDOW_BUFFER_SIZE_RECORD = *WINDOW_BUFFER_SIZE_RECORD;
pub const MENU_EVENT_RECORD = extern struct {
dwCommandId: windows.UINT,
};
pub const PMENU_EVENT_RECORD = *MENU_EVENT_RECORD;
pub const FOCUS_EVENT_RECORD = extern struct {
bSetFocus: windows.BOOL,
};
pub const PFOCUS_EVENT_RECORD = *FOCUS_EVENT_RECORD;
const union_unnamed_249 = extern union {
KeyEvent: KEY_EVENT_RECORD,
MouseEvent: MOUSE_EVENT_RECORD,
WindowBufferSizeEvent: WINDOW_BUFFER_SIZE_RECORD,
MenuEvent: MENU_EVENT_RECORD,
FocusEvent: FOCUS_EVENT_RECORD,
};
pub const INPUT_RECORD = extern struct {
EventType: windows.WORD,
Event: union_unnamed_249,
};
pub const PINPUT_RECORD = *INPUT_RECORD;
pub extern "kernel32" fn ReadConsoleInputW(hConsoleInput: windows.HANDLE, lpBuffer: PINPUT_RECORD, nLength: windows.DWORD, lpNumberOfEventsRead: *windows.DWORD) callconv(windows.WINAPI) windows.BOOL;
// TODO: remove this in zig 0.13.0
pub extern "kernel32" fn SetConsoleMode(in_hConsoleHandle: windows.HANDLE, in_dwMode: windows.DWORD) callconv(windows.WINAPI) windows.BOOL;
|
0 | repos/libvaxis/src | repos/libvaxis/src/widgets/Scrollbar.zig | const std = @import("std");
const vaxis = @import("../main.zig");
const Scrollbar = @This();
/// character to use for the scrollbar
character: vaxis.Cell.Character = .{ .grapheme = "▐", .width = 1 },
/// style to draw the bar character with
style: vaxis.Style = .{},
/// index of the top of the visible area
top: usize = 0,
/// total items in the list
total: usize,
/// total items that fit within the view area
view_size: usize,
pub fn draw(self: Scrollbar, win: vaxis.Window) void {
// don't draw when 0 items
if (self.total < 1) return;
// don't draw when all items can be shown
if (self.view_size >= self.total) return;
const bar_height = @max(std.math.divCeil(usize, self.view_size * win.height, self.total) catch unreachable, 1);
const bar_top = self.top * win.height / self.total;
var i: usize = 0;
while (i < bar_height) : (i += 1)
win.writeCell(0, i + bar_top, .{ .char = self.character, .style = self.style });
}
|
0 | repos/libvaxis/src | repos/libvaxis/src/widgets/LineNumbers.zig | const std = @import("std");
const vaxis = @import("../main.zig");
const digits = "0123456789";
num_lines: usize = std.math.maxInt(usize),
highlighted_line: usize = 0,
style: vaxis.Style = .{ .dim = true },
highlighted_style: vaxis.Style = .{ .dim = true, .bg = .{ .index = 0 } },
pub fn extractDigit(v: usize, n: usize) usize {
return (v / (std.math.powi(usize, 10, n) catch unreachable)) % 10;
}
pub fn numDigits(v: usize) usize {
return switch (v) {
0...9 => 1,
10...99 => 2,
100...999 => 3,
1000...9999 => 4,
10000...99999 => 5,
100000...999999 => 6,
1000000...9999999 => 7,
10000000...99999999 => 8,
else => 0,
};
}
pub fn draw(self: @This(), win: vaxis.Window, y_scroll: usize) void {
for (1 + y_scroll..self.num_lines) |line| {
if (line - 1 >= y_scroll +| win.height) {
break;
}
const highlighted = line == self.highlighted_line;
const num_digits = numDigits(line);
for (0..num_digits) |i| {
const digit = extractDigit(line, i);
win.writeCell(win.width -| (i + 2), line -| (y_scroll +| 1), .{
.char = .{
.width = 1,
.grapheme = digits[digit .. digit + 1],
},
.style = if (highlighted) self.highlighted_style else self.style,
});
}
if (highlighted) {
for (num_digits + 1..win.width) |i| {
win.writeCell(i, line -| (y_scroll +| 1), .{
.style = if (highlighted) self.highlighted_style else self.style,
});
}
}
}
}
|
0 | repos/libvaxis/src | repos/libvaxis/src/widgets/ScrollView.zig | const std = @import("std");
const vaxis = @import("../main.zig");
pub const Scroll = struct {
x: usize = 0,
y: usize = 0,
pub fn restrictTo(self: *@This(), w: usize, h: usize) void {
self.x = @min(self.x, w);
self.y = @min(self.y, h);
}
};
pub const VerticalScrollbar = struct {
character: vaxis.Cell.Character = .{ .grapheme = "▐", .width = 1 },
fg: vaxis.Style = .{},
bg: vaxis.Style = .{ .fg = .{ .index = 8 } },
};
scroll: Scroll = .{},
vertical_scrollbar: ?VerticalScrollbar = .{},
/// Standard input mappings.
/// It is not neccessary to use this, you can set `scroll` manually.
pub fn input(self: *@This(), key: vaxis.Key) void {
if (key.matches(vaxis.Key.right, .{})) {
self.scroll.x +|= 1;
} else if (key.matches(vaxis.Key.right, .{ .shift = true })) {
self.scroll.x +|= 32;
} else if (key.matches(vaxis.Key.left, .{})) {
self.scroll.x -|= 1;
} else if (key.matches(vaxis.Key.left, .{ .shift = true })) {
self.scroll.x -|= 32;
} else if (key.matches(vaxis.Key.up, .{})) {
self.scroll.y -|= 1;
} else if (key.matches(vaxis.Key.page_up, .{})) {
self.scroll.y -|= 32;
} else if (key.matches(vaxis.Key.down, .{})) {
self.scroll.y +|= 1;
} else if (key.matches(vaxis.Key.page_down, .{})) {
self.scroll.y +|= 32;
} else if (key.matches(vaxis.Key.end, .{})) {
self.scroll.y = std.math.maxInt(usize);
} else if (key.matches(vaxis.Key.home, .{})) {
self.scroll.y = 0;
}
}
/// Must be called before doing any `writeCell` calls.
pub fn draw(self: *@This(), parent: vaxis.Window, content_size: struct {
cols: usize,
rows: usize,
}) void {
const content_cols = if (self.vertical_scrollbar) |_| content_size.cols +| 1 else content_size.cols;
const max_scroll_x = content_cols -| parent.width;
const max_scroll_y = content_size.rows -| parent.height;
self.scroll.restrictTo(max_scroll_x, max_scroll_y);
if (self.vertical_scrollbar) |opts| {
const vbar: vaxis.widgets.Scrollbar = .{
.character = opts.character,
.style = opts.fg,
.total = content_size.rows,
.view_size = parent.height,
.top = self.scroll.y,
};
const bg = parent.child(.{
.x_off = parent.width -| opts.character.width,
.width = .{ .limit = opts.character.width },
.height = .{ .limit = parent.height },
});
bg.fill(.{ .char = opts.character, .style = opts.bg });
vbar.draw(bg);
}
}
pub const BoundingBox = struct {
x1: usize,
y1: usize,
x2: usize,
y2: usize,
pub inline fn below(self: @This(), row: usize) bool {
return row < self.y1;
}
pub inline fn above(self: @This(), row: usize) bool {
return row >= self.y2;
}
pub inline fn rowInside(self: @This(), row: usize) bool {
return row >= self.y1 and row < self.y2;
}
pub inline fn colInside(self: @This(), col: usize) bool {
return col >= self.x1 and col < self.x2;
}
pub inline fn inside(self: @This(), col: usize, row: usize) bool {
return self.rowInside(row) and self.colInside(col);
}
};
/// Boundary of the content, useful for culling to improve draw performance.
pub fn bounds(self: *@This(), parent: vaxis.Window) BoundingBox {
const right_pad: usize = if (self.vertical_scrollbar != null) 1 else 0;
return .{
.x1 = self.scroll.x,
.y1 = self.scroll.y,
.x2 = self.scroll.x +| parent.width -| right_pad,
.y2 = self.scroll.y +| parent.height,
};
}
/// Use this function instead of `Window.writeCell` to draw your cells and they will magically scroll.
pub fn writeCell(self: *@This(), parent: vaxis.Window, col: usize, row: usize, cell: vaxis.Cell) void {
const b = self.bounds(parent);
if (!b.inside(col, row)) return;
const win = parent.child(.{ .width = .{ .limit = b.x2 - b.x1 }, .height = .{ .limit = b.y2 - b.y1 } });
win.writeCell(col -| self.scroll.x, row -| self.scroll.y, cell);
}
/// Use this function instead of `Window.readCell` to read the correct cell in scrolling context.
pub fn readCell(self: *@This(), parent: vaxis.Window, col: usize, row: usize) ?vaxis.Cell {
const b = self.bounds(parent);
if (!b.inside(col, row)) return;
const win = parent.child(.{ .width = .{ .limit = b.width }, .height = .{ .limit = b.height } });
return win.readCell(col -| self.scroll.x, row -| self.scroll.y);
}
|
0 | repos/libvaxis/src | repos/libvaxis/src/widgets/TextInput.zig | const std = @import("std");
const assert = std.debug.assert;
const Key = @import("../Key.zig");
const Cell = @import("../Cell.zig");
const Window = @import("../Window.zig");
const Unicode = @import("../Unicode.zig");
const TextInput = @This();
/// The events that this widget handles
const Event = union(enum) {
key_press: Key,
};
const ellipsis: Cell.Character = .{ .grapheme = "…", .width = 1 };
// Index of our cursor
buf: Buffer,
/// the number of graphemes to skip when drawing. Used for horizontal scrolling
draw_offset: usize = 0,
/// the column we placed the cursor the last time we drew
prev_cursor_col: usize = 0,
/// the grapheme index of the cursor the last time we drew
prev_cursor_idx: usize = 0,
/// approximate distance from an edge before we scroll
scroll_offset: usize = 4,
unicode: *const Unicode,
pub fn init(alloc: std.mem.Allocator, unicode: *const Unicode) TextInput {
return TextInput{
.buf = Buffer.init(alloc),
.unicode = unicode,
};
}
pub fn deinit(self: *TextInput) void {
self.buf.deinit();
}
pub fn update(self: *TextInput, event: Event) !void {
switch (event) {
.key_press => |key| {
if (key.matches(Key.backspace, .{})) {
self.deleteBeforeCursor();
} else if (key.matches(Key.delete, .{}) or key.matches('d', .{ .ctrl = true })) {
self.deleteAfterCursor();
} else if (key.matches(Key.left, .{}) or key.matches('b', .{ .ctrl = true })) {
self.cursorLeft();
} else if (key.matches(Key.right, .{}) or key.matches('f', .{ .ctrl = true })) {
self.cursorRight();
} else if (key.matches('a', .{ .ctrl = true }) or key.matches(Key.home, .{})) {
self.buf.moveGapLeft(self.buf.firstHalf().len);
} else if (key.matches('e', .{ .ctrl = true }) or key.matches(Key.end, .{})) {
self.buf.moveGapRight(self.buf.secondHalf().len);
} else if (key.matches('k', .{ .ctrl = true })) {
self.deleteToEnd();
} else if (key.matches('u', .{ .ctrl = true })) {
self.deleteToStart();
} else if (key.matches('b', .{ .alt = true }) or key.matches(Key.left, .{ .alt = true })) {
self.moveBackwardWordwise();
} else if (key.matches('f', .{ .alt = true }) or key.matches(Key.right, .{ .alt = true })) {
self.moveForwardWordwise();
} else if (key.matches('w', .{ .ctrl = true }) or key.matches(Key.backspace, .{ .alt = true })) {
self.deleteWordBefore();
} else if (key.matches('d', .{ .alt = true })) {
self.deleteWordAfter();
} else if (key.text) |text| {
try self.insertSliceAtCursor(text);
}
},
}
}
/// insert text at the cursor position
pub fn insertSliceAtCursor(self: *TextInput, data: []const u8) std.mem.Allocator.Error!void {
var iter = self.unicode.graphemeIterator(data);
while (iter.next()) |text| {
try self.buf.insertSliceAtCursor(text.bytes(data));
}
}
pub fn sliceToCursor(self: *TextInput, buf: []u8) []const u8 {
assert(buf.len >= self.buf.cursor);
@memcpy(buf[0..self.buf.cursor], self.buf.firstHalf());
return buf[0..self.buf.cursor];
}
/// calculates the display width from the draw_offset to the cursor
pub fn widthToCursor(self: *TextInput, win: Window) usize {
var width: usize = 0;
const first_half = self.buf.firstHalf();
var first_iter = self.unicode.graphemeIterator(first_half);
var i: usize = 0;
while (first_iter.next()) |grapheme| {
defer i += 1;
if (i < self.draw_offset) {
continue;
}
const g = grapheme.bytes(first_half);
width += win.gwidth(g);
}
return width;
}
pub fn cursorLeft(self: *TextInput) void {
// We need to find the size of the last grapheme in the first half
var iter = self.unicode.graphemeIterator(self.buf.firstHalf());
var len: usize = 0;
while (iter.next()) |grapheme| {
len = grapheme.len;
}
self.buf.moveGapLeft(len);
}
pub fn cursorRight(self: *TextInput) void {
var iter = self.unicode.graphemeIterator(self.buf.secondHalf());
const grapheme = iter.next() orelse return;
self.buf.moveGapRight(grapheme.len);
}
pub fn graphemesBeforeCursor(self: *const TextInput) usize {
const first_half = self.buf.firstHalf();
var first_iter = self.unicode.graphemeIterator(first_half);
var i: usize = 0;
while (first_iter.next()) |_| {
i += 1;
}
return i;
}
pub fn draw(self: *TextInput, win: Window) void {
const cursor_idx = self.graphemesBeforeCursor();
if (cursor_idx < self.draw_offset) self.draw_offset = cursor_idx;
if (win.width == 0) return;
while (true) {
const width = self.widthToCursor(win);
if (width >= win.width) {
self.draw_offset +|= width - win.width + 1;
continue;
} else break;
}
self.prev_cursor_idx = cursor_idx;
self.prev_cursor_col = 0;
// assumption!! the gap is never within a grapheme
// one way to _ensure_ this is to move the gap... but that's a cost we probably don't want to pay.
const first_half = self.buf.firstHalf();
var first_iter = self.unicode.graphemeIterator(first_half);
var col: usize = 0;
var i: usize = 0;
while (first_iter.next()) |grapheme| {
if (i < self.draw_offset) {
i += 1;
continue;
}
const g = grapheme.bytes(first_half);
const w = win.gwidth(g);
if (col + w >= win.width) {
win.writeCell(win.width - 1, 0, .{ .char = ellipsis });
break;
}
win.writeCell(col, 0, .{
.char = .{
.grapheme = g,
.width = w,
},
});
col += w;
i += 1;
if (i == cursor_idx) self.prev_cursor_col = col;
}
const second_half = self.buf.secondHalf();
var second_iter = self.unicode.graphemeIterator(second_half);
while (second_iter.next()) |grapheme| {
if (i < self.draw_offset) {
i += 1;
continue;
}
const g = grapheme.bytes(second_half);
const w = win.gwidth(g);
if (col + w > win.width) {
win.writeCell(win.width - 1, 0, .{ .char = ellipsis });
break;
}
win.writeCell(col, 0, .{
.char = .{
.grapheme = g,
.width = w,
},
});
col += w;
i += 1;
if (i == cursor_idx) self.prev_cursor_col = col;
}
if (self.draw_offset > 0) {
win.writeCell(0, 0, .{ .char = ellipsis });
}
win.showCursor(self.prev_cursor_col, 0);
}
pub fn clearAndFree(self: *TextInput) void {
self.buf.clearAndFree();
self.reset();
}
pub fn clearRetainingCapacity(self: *TextInput) void {
self.buf.clearRetainingCapacity();
self.reset();
}
pub fn toOwnedSlice(self: *TextInput) ![]const u8 {
defer self.reset();
return self.buf.toOwnedSlice();
}
pub fn reset(self: *TextInput) void {
self.draw_offset = 0;
self.prev_cursor_col = 0;
self.prev_cursor_idx = 0;
}
// returns the number of bytes before the cursor
pub fn byteOffsetToCursor(self: TextInput) usize {
return self.buf.cursor;
}
pub fn deleteToEnd(self: *TextInput) void {
self.buf.growGapRight(self.buf.secondHalf().len);
}
pub fn deleteToStart(self: *TextInput) void {
self.buf.growGapLeft(self.buf.cursor);
}
pub fn deleteBeforeCursor(self: *TextInput) void {
// We need to find the size of the last grapheme in the first half
var iter = self.unicode.graphemeIterator(self.buf.firstHalf());
var len: usize = 0;
while (iter.next()) |grapheme| {
len = grapheme.len;
}
self.buf.growGapLeft(len);
}
pub fn deleteAfterCursor(self: *TextInput) void {
var iter = self.unicode.graphemeIterator(self.buf.secondHalf());
const grapheme = iter.next() orelse return;
self.buf.growGapRight(grapheme.len);
}
/// Moves the cursor backward by words. If the character before the cursor is a space, the cursor is
/// positioned just after the next previous space
pub fn moveBackwardWordwise(self: *TextInput) void {
const trimmed = std.mem.trimRight(u8, self.buf.firstHalf(), " ");
const idx = if (std.mem.lastIndexOfScalar(u8, trimmed, ' ')) |last|
last + 1
else
0;
self.buf.moveGapLeft(self.buf.cursor - idx);
}
pub fn moveForwardWordwise(self: *TextInput) void {
const second_half = self.buf.secondHalf();
var i: usize = 0;
while (i < second_half.len and second_half[i] == ' ') : (i += 1) {}
const idx = std.mem.indexOfScalarPos(u8, second_half, i, ' ') orelse second_half.len;
self.buf.moveGapRight(idx);
}
pub fn deleteWordBefore(self: *TextInput) void {
// Store current cursor position. Move one word backward. Delete after the cursor the bytes we
// moved
const pre = self.buf.cursor;
self.moveBackwardWordwise();
self.buf.growGapRight(pre - self.buf.cursor);
}
pub fn deleteWordAfter(self: *TextInput) void {
// Store current cursor position. Move one word backward. Delete after the cursor the bytes we
// moved
const second_half = self.buf.secondHalf();
var i: usize = 0;
while (i < second_half.len and second_half[i] == ' ') : (i += 1) {}
const idx = std.mem.indexOfScalarPos(u8, second_half, i, ' ') orelse second_half.len;
self.buf.growGapRight(idx);
}
test "assertion" {
const alloc = std.testing.allocator_instance.allocator();
const unicode = try Unicode.init(alloc);
defer unicode.deinit();
const astronaut = "👩🚀";
const astronaut_emoji: Key = .{
.text = astronaut,
.codepoint = try std.unicode.utf8Decode(astronaut[0..4]),
};
var input = TextInput.init(std.testing.allocator, &unicode);
defer input.deinit();
for (0..6) |_| {
try input.update(.{ .key_press = astronaut_emoji });
}
}
test "sliceToCursor" {
const alloc = std.testing.allocator_instance.allocator();
const unicode = try Unicode.init(alloc);
defer unicode.deinit();
var input = init(alloc, &unicode);
defer input.deinit();
try input.insertSliceAtCursor("hello, world");
input.cursorLeft();
input.cursorLeft();
input.cursorLeft();
var buf: [32]u8 = undefined;
try std.testing.expectEqualStrings("hello, wo", input.sliceToCursor(&buf));
input.cursorRight();
try std.testing.expectEqualStrings("hello, wor", input.sliceToCursor(&buf));
}
pub const Buffer = struct {
allocator: std.mem.Allocator,
buffer: []u8,
cursor: usize,
gap_size: usize,
pub fn init(allocator: std.mem.Allocator) Buffer {
return .{
.allocator = allocator,
.buffer = &.{},
.cursor = 0,
.gap_size = 0,
};
}
pub fn deinit(self: *Buffer) void {
self.allocator.free(self.buffer);
}
pub fn firstHalf(self: Buffer) []const u8 {
return self.buffer[0..self.cursor];
}
pub fn secondHalf(self: Buffer) []const u8 {
return self.buffer[self.cursor + self.gap_size ..];
}
pub fn grow(self: *Buffer, n: usize) std.mem.Allocator.Error!void {
// Always grow by 512 bytes
const new_size = self.buffer.len + n + 512;
// Allocate the new memory
const new_memory = try self.allocator.alloc(u8, new_size);
// Copy the first half
@memcpy(new_memory[0..self.cursor], self.firstHalf());
// Copy the second half
const second_half = self.secondHalf();
@memcpy(new_memory[new_size - second_half.len ..], second_half);
self.allocator.free(self.buffer);
self.buffer = new_memory;
self.gap_size = new_size - second_half.len - self.cursor;
}
pub fn insertSliceAtCursor(self: *Buffer, slice: []const u8) std.mem.Allocator.Error!void {
if (slice.len == 0) return;
if (self.gap_size <= slice.len) try self.grow(slice.len);
@memcpy(self.buffer[self.cursor .. self.cursor + slice.len], slice);
self.cursor += slice.len;
self.gap_size -= slice.len;
}
/// Move the gap n bytes to the left
pub fn moveGapLeft(self: *Buffer, n: usize) void {
const new_idx = self.cursor -| n;
const dst = self.buffer[new_idx + self.gap_size ..];
const src = self.buffer[new_idx..self.cursor];
std.mem.copyForwards(u8, dst, src);
self.cursor = new_idx;
}
pub fn moveGapRight(self: *Buffer, n: usize) void {
const new_idx = self.cursor + n;
const dst = self.buffer[self.cursor..];
const src = self.buffer[self.cursor + self.gap_size .. new_idx + self.gap_size];
std.mem.copyForwards(u8, dst, src);
self.cursor = new_idx;
}
/// grow the gap by moving the cursor n bytes to the left
pub fn growGapLeft(self: *Buffer, n: usize) void {
// gap grows by the delta
self.gap_size += n;
self.cursor -|= n;
}
/// grow the gap by removing n bytes after the cursor
pub fn growGapRight(self: *Buffer, n: usize) void {
self.gap_size = @min(self.gap_size + n, self.buffer.len - self.cursor);
}
pub fn clearAndFree(self: *Buffer) void {
self.cursor = 0;
self.allocator.free(self.buffer);
self.buffer = &.{};
self.gap_size = 0;
}
pub fn clearRetainingCapacity(self: *Buffer) void {
self.cursor = 0;
self.gap_size = self.buffer.len;
}
pub fn toOwnedSlice(self: *Buffer) std.mem.Allocator.Error![]const u8 {
const first_half = self.firstHalf();
const second_half = self.secondHalf();
const buf = try self.allocator.alloc(u8, first_half.len + second_half.len);
@memcpy(buf[0..first_half.len], first_half);
@memcpy(buf[first_half.len..], second_half);
self.clearAndFree();
return buf;
}
pub fn realLength(self: *const Buffer) usize {
return self.firstHalf().len + self.secondHalf().len;
}
};
test "TextInput.zig: Buffer" {
var gap_buf = Buffer.init(std.testing.allocator);
defer gap_buf.deinit();
try gap_buf.insertSliceAtCursor("abc");
try std.testing.expectEqualStrings("abc", gap_buf.firstHalf());
try std.testing.expectEqualStrings("", gap_buf.secondHalf());
gap_buf.moveGapLeft(1);
try std.testing.expectEqualStrings("ab", gap_buf.firstHalf());
try std.testing.expectEqualStrings("c", gap_buf.secondHalf());
try gap_buf.insertSliceAtCursor(" ");
try std.testing.expectEqualStrings("ab ", gap_buf.firstHalf());
try std.testing.expectEqualStrings("c", gap_buf.secondHalf());
gap_buf.growGapLeft(1);
try std.testing.expectEqualStrings("ab", gap_buf.firstHalf());
try std.testing.expectEqualStrings("c", gap_buf.secondHalf());
try std.testing.expectEqual(2, gap_buf.cursor);
gap_buf.growGapRight(1);
try std.testing.expectEqualStrings("ab", gap_buf.firstHalf());
try std.testing.expectEqualStrings("", gap_buf.secondHalf());
try std.testing.expectEqual(2, gap_buf.cursor);
}
|
0 | repos/libvaxis/src | repos/libvaxis/src/widgets/border.zig | const Cell = @import("../Cell.zig");
const Window = @import("../Window.zig");
const Style = Cell.Style;
const Character = Cell.Character;
const horizontal = Character{ .grapheme = "─", .width = 1 };
const vertical = Character{ .grapheme = "│", .width = 1 };
const top_left = Character{ .grapheme = "╭", .width = 1 };
const top_right = Character{ .grapheme = "╮", .width = 1 };
const bottom_right = Character{ .grapheme = "╯", .width = 1 };
const bottom_left = Character{ .grapheme = "╰", .width = 1 };
pub fn all(win: Window, style: Style) Window {
const h = win.height;
const w = win.width;
win.writeCell(0, 0, .{ .char = top_left, .style = style });
win.writeCell(0, h -| 1, .{ .char = bottom_left, .style = style });
win.writeCell(w -| 1, 0, .{ .char = top_right, .style = style });
win.writeCell(w -| 1, h -| 1, .{ .char = bottom_right, .style = style });
var i: usize = 1;
while (i < (h -| 1)) : (i += 1) {
win.writeCell(0, i, .{ .char = vertical, .style = style });
win.writeCell(w -| 1, i, .{ .char = vertical, .style = style });
}
i = 1;
while (i < w -| 1) : (i += 1) {
win.writeCell(i, 0, .{ .char = horizontal, .style = style });
win.writeCell(i, h -| 1, .{ .char = horizontal, .style = style });
}
return win.initChild(1, 1, .{ .limit = w -| 2 }, .{ .limit = h -| 2 });
}
pub fn right(win: Window, style: Style) Window {
const h = win.height;
const w = win.width;
var i: usize = 0;
while (i < h) : (i += 1) {
win.writeCell(w -| 1, i, .{ .char = vertical, .style = style });
}
return win.initChild(0, 0, .{ .limit = w -| 1 }, .expand);
}
pub fn bottom(win: Window, style: Style) Window {
const h = win.height;
const w = win.width;
var i: usize = 0;
while (i < w) : (i += 1) {
win.writeCell(i, h -| 1, .{ .char = horizontal, .style = style });
}
return win.initChild(0, 0, .expand, .{ .limit = h -| 1 });
}
|
0 | repos/libvaxis/src | repos/libvaxis/src/widgets/TextView.zig | const std = @import("std");
const vaxis = @import("../main.zig");
const grapheme = @import("grapheme");
const DisplayWidth = @import("DisplayWidth");
const ScrollView = vaxis.widgets.ScrollView;
pub const BufferWriter = struct {
pub const Error = error{OutOfMemory};
pub const Writer = std.io.GenericWriter(@This(), Error, write);
allocator: std.mem.Allocator,
buffer: *Buffer,
gd: *const grapheme.GraphemeData,
wd: *const DisplayWidth.DisplayWidthData,
pub fn write(self: @This(), bytes: []const u8) Error!usize {
try self.buffer.append(self.allocator, .{
.bytes = bytes,
.gd = self.gd,
.wd = self.wd,
});
return bytes.len;
}
pub fn writer(self: @This()) Writer {
return .{ .context = self };
}
};
pub const Buffer = struct {
const StyleList = std.ArrayListUnmanaged(vaxis.Style);
const StyleMap = std.HashMapUnmanaged(usize, usize, std.hash_map.AutoContext(usize), std.hash_map.default_max_load_percentage);
pub const Content = struct {
bytes: []const u8,
gd: *const grapheme.GraphemeData,
wd: *const DisplayWidth.DisplayWidthData,
};
pub const Style = struct {
begin: usize,
end: usize,
style: vaxis.Style,
};
pub const Error = error{OutOfMemory};
grapheme: std.MultiArrayList(grapheme.Grapheme) = .{},
content: std.ArrayListUnmanaged(u8) = .{},
style_list: StyleList = .{},
style_map: StyleMap = .{},
rows: usize = 0,
cols: usize = 0,
// used when appending to a buffer
last_cols: usize = 0,
pub fn deinit(self: *@This(), allocator: std.mem.Allocator) void {
self.style_map.deinit(allocator);
self.style_list.deinit(allocator);
self.grapheme.deinit(allocator);
self.content.deinit(allocator);
self.* = undefined;
}
/// Clears all buffer data.
pub fn clear(self: *@This(), allocator: std.mem.Allocator) void {
self.deinit(allocator);
self.* = .{};
}
/// Replaces contents of the buffer, all previous buffer data is lost.
pub fn update(self: *@This(), allocator: std.mem.Allocator, content: Content) Error!void {
self.clear(allocator);
errdefer self.clear(allocator);
try self.append(allocator, content);
}
/// Appends content to the buffer.
pub fn append(self: *@This(), allocator: std.mem.Allocator, content: Content) Error!void {
var cols: usize = self.last_cols;
var iter = grapheme.Iterator.init(content.bytes, content.gd);
const dw: DisplayWidth = .{ .data = content.wd };
while (iter.next()) |g| {
try self.grapheme.append(allocator, .{
.len = g.len,
.offset = @as(u32, @intCast(self.content.items.len)) + g.offset,
});
const cluster = g.bytes(content.bytes);
if (std.mem.eql(u8, cluster, "\n")) {
self.cols = @max(self.cols, cols);
cols = 0;
continue;
}
cols +|= dw.strWidth(cluster);
}
try self.content.appendSlice(allocator, content.bytes);
self.last_cols = cols;
self.cols = @max(self.cols, cols);
self.rows +|= std.mem.count(u8, content.bytes, "\n");
}
/// Clears all styling data.
pub fn clearStyle(self: *@This(), allocator: std.mem.Allocator) void {
self.style_list.deinit(allocator);
self.style_map.deinit(allocator);
}
/// Update style for range of the buffer contents.
pub fn updateStyle(self: *@This(), allocator: std.mem.Allocator, style: Style) Error!void {
const style_index = blk: {
for (self.style_list.items, 0..) |s, i| {
if (std.meta.eql(s, style.style)) {
break :blk i;
}
}
try self.style_list.append(allocator, style.style);
break :blk self.style_list.items.len - 1;
};
for (style.begin..style.end) |i| {
try self.style_map.put(allocator, i, style_index);
}
}
pub fn writer(
self: *@This(),
allocator: std.mem.Allocator,
gd: *const grapheme.GraphemeData,
wd: *const DisplayWidth.DisplayWidthData,
) BufferWriter.Writer {
return .{
.context = .{
.allocator = allocator,
.buffer = self,
.gd = gd,
.wd = wd,
},
};
}
};
scroll_view: ScrollView = .{},
pub fn input(self: *@This(), key: vaxis.Key) void {
self.scroll_view.input(key);
}
pub fn draw(self: *@This(), win: vaxis.Window, buffer: Buffer) void {
self.scroll_view.draw(win, .{ .cols = buffer.cols, .rows = buffer.rows });
const Pos = struct { x: usize = 0, y: usize = 0 };
var pos: Pos = .{};
var byte_index: usize = 0;
const bounds = self.scroll_view.bounds(win);
for (buffer.grapheme.items(.len), buffer.grapheme.items(.offset), 0..) |g_len, g_offset, index| {
if (bounds.above(pos.y)) {
break;
}
const cluster = buffer.content.items[g_offset..][0..g_len];
defer byte_index += cluster.len;
if (std.mem.eql(u8, cluster, "\n")) {
if (index == buffer.grapheme.len - 1) {
break;
}
pos.y +|= 1;
pos.x = 0;
continue;
} else if (bounds.below(pos.y)) {
continue;
}
const width = win.gwidth(cluster);
defer pos.x +|= width;
if (!bounds.colInside(pos.x)) {
continue;
}
const style: vaxis.Style = blk: {
if (buffer.style_map.get(byte_index)) |style_index| {
break :blk buffer.style_list.items[style_index];
}
break :blk .{};
};
self.scroll_view.writeCell(win, pos.x, pos.y, .{
.char = .{ .grapheme = cluster, .width = width },
.style = style,
});
}
}
|
0 | repos/libvaxis/src | repos/libvaxis/src/widgets/alignment.zig | const Window = @import("../Window.zig");
pub fn center(parent: Window, cols: usize, rows: usize) Window {
const y_off = (parent.height / 2) -| (rows / 2);
const x_off = (parent.width / 2) -| (cols / 2);
return parent.initChild(x_off, y_off, .{ .limit = cols }, .{ .limit = rows });
}
|
0 | repos/libvaxis/src | repos/libvaxis/src/widgets/Table.zig | const std = @import("std");
const fmt = std.fmt;
const heap = std.heap;
const mem = std.mem;
const meta = std.meta;
const vaxis = @import("../main.zig");
/// Table Context for maintaining state and drawing Tables with `drawTable()`.
pub const TableContext = struct {
/// Current active Row of the Table.
row: usize = 0,
/// Current active Column of the Table.
col: usize = 0,
/// Starting point within the Data List.
start: usize = 0,
/// Selected Rows.
sel_rows: ?[]usize = null,
/// Active status of the Table.
active: bool = false,
/// Active Content Callback Function.
/// If available, this will be called to vertically expand the active row with additional info.
active_content_fn: ?*const fn (*vaxis.Window, *const anyopaque) anyerror!usize = null,
/// Active Content Context
/// This will be provided to the `active_content` callback when called.
active_ctx: *const anyopaque = &{},
/// Y Offset for rows beyond the Active Content.
/// (This will be calculated automatically)
active_y_off: usize = 0,
/// The Background Color for the Active Row and Column Header.
selected_bg: vaxis.Cell.Color,
/// The Background Color for Selected Rows.
active_bg: vaxis.Cell.Color,
/// First Column Header Background Color
hdr_bg_1: vaxis.Cell.Color = .{ .rgb = [_]u8{ 64, 64, 64 } },
/// Second Column Header Background Color
hdr_bg_2: vaxis.Cell.Color = .{ .rgb = [_]u8{ 8, 8, 24 } },
/// First Row Background Color
row_bg_1: vaxis.Cell.Color = .{ .rgb = [_]u8{ 32, 32, 32 } },
/// Second Row Background Color
row_bg_2: vaxis.Cell.Color = .{ .rgb = [_]u8{ 8, 8, 8 } },
/// Y Offset for drawing to the parent Window.
y_off: usize = 0,
/// Column Width
/// Note, if this is left `null` the Column Width will be dynamically calculated during `drawTable()`.
//col_width: ?usize = null,
col_width: WidthStyle = .dynamic_fill,
// Header Names
header_names: HeaderNames = .field_names,
// Column Indexes
col_indexes: ColumnIndexes = .all,
};
/// Width Styles for `col_width`.
pub const WidthStyle = union(enum) {
/// Dynamically calculate Column Widths such that the entire (or most) of the screen is filled horizontally.
dynamic_fill,
/// Dynamically calculate the Column Width for each Column based on its Header Length and the provided Padding length.
dynamic_header_len: usize,
/// Statically set all Column Widths to the same value.
static_all: usize,
/// Statically set individual Column Widths to specific values.
static_individual: []const usize,
};
/// Column Indexes
pub const ColumnIndexes = union(enum) {
/// Use all of the Columns.
all,
/// Use Columns from the specified indexes.
by_idx: []const usize,
};
/// Header Names
pub const HeaderNames = union(enum) {
/// Use Field Names as Headers
field_names,
/// Custom
custom: []const []const u8,
};
/// Draw a Table for the TUI.
pub fn drawTable(
/// This should be an ArenaAllocator that can be deinitialized after each event call.
/// The Allocator is only used in three cases:
/// 1. If a cell is a non-String. (If the Allocator is not provided, those cells will show "[unsupported (TypeName)]".)
/// 2. To show that a value is too large to fit into a cell using '...'. (If the Allocator is not provided, they'll just be cutoff.)
/// 3. To copy a MultiArrayList into a normal slice. (Note, this is an expensive operation. Prefer to pass a Slice or ArrayList if possible.)
alloc: ?mem.Allocator,
/// The parent Window to draw to.
win: vaxis.Window,
/// This must be a Slice, ArrayList, or MultiArrayList.
/// Note, MultiArrayList support currently requires allocation.
data_list: anytype,
// The Table Context for this Table.
table_ctx: *TableContext,
) !void {
var di_is_mal = false;
const data_items = getData: {
const DataListT = @TypeOf(data_list);
const data_ti = @typeInfo(DataListT);
switch (data_ti) {
.Pointer => |ptr| {
if (ptr.size != .Slice) return error.UnsupportedTableDataType;
break :getData data_list;
},
.Struct => {
const di_fields = meta.fields(DataListT);
const al_fields = meta.fields(std.ArrayList([]const u8));
const mal_fields = meta.fields(std.MultiArrayList(struct { a: u8 = 0, b: u32 = 0 }));
// Probably an ArrayList
const is_al = comptime if (mem.indexOf(u8, @typeName(DataListT), "MultiArrayList") == null and
mem.indexOf(u8, @typeName(DataListT), "ArrayList") != null and
al_fields.len == di_fields.len)
isAL: {
var is = true;
for (al_fields, di_fields) |al_field, di_field|
is = is and mem.eql(u8, al_field.name, di_field.name);
break :isAL is;
} else false;
if (is_al) break :getData data_list.items;
// Probably a MultiArrayList
const is_mal = if (mem.indexOf(u8, @typeName(DataListT), "MultiArrayList") != null and
mal_fields.len == di_fields.len)
isMAL: {
var is = true;
inline for (mal_fields, di_fields) |mal_field, di_field|
is = is and mem.eql(u8, mal_field.name, di_field.name);
break :isMAL is;
} else false;
if (!is_mal) return error.UnsupportedTableDataType;
if (alloc) |_alloc| {
di_is_mal = true;
const mal_slice = data_list.slice();
const DataT = @TypeOf(mal_slice.get(0));
var data_out_list = std.ArrayList(DataT).init(_alloc);
for (0..mal_slice.len) |idx| try data_out_list.append(mal_slice.get(idx));
break :getData try data_out_list.toOwnedSlice();
}
return error.UnsupportedTableDataType;
},
else => return error.UnsupportedTableDataType,
}
};
defer if (di_is_mal) alloc.?.free(data_items);
// Headers for the Table
var hdrs_buf: [100][]const u8 = undefined;
const headers = hdrs: {
switch (table_ctx.header_names) {
.field_names => {
const DataT = @TypeOf(data_items[0]);
const fields = meta.fields(DataT);
var num_hdrs: usize = 0;
inline for (fields, 0..) |field, idx| contFields: {
switch (table_ctx.col_indexes) {
.all => {},
.by_idx => |idxs| {
if (mem.indexOfScalar(usize, idxs, idx) == null) break :contFields;
},
}
num_hdrs += 1;
hdrs_buf[idx] = field.name;
}
break :hdrs hdrs_buf[0..num_hdrs];
},
.custom => |hdrs| break :hdrs hdrs,
}
};
const table_win = win.initChild(
0,
table_ctx.y_off,
.{ .limit = win.width },
.{ .limit = win.height },
);
if (table_ctx.col > headers.len - 1) table_ctx.col = headers.len - 1;
var col_start: usize = 0;
for (headers[0..], 0..) |hdr_txt, idx| {
const col_width = try calcColWidth(
idx,
headers,
table_ctx.col_width,
table_win,
);
defer col_start += col_width;
const hdr_bg =
if (table_ctx.active and idx == table_ctx.col) table_ctx.active_bg else if (idx % 2 == 0) table_ctx.hdr_bg_1 else table_ctx.hdr_bg_2;
const hdr_win = table_win.child(.{
.x_off = col_start,
.y_off = 0,
.width = .{ .limit = col_width },
.height = .{ .limit = 1 },
});
var hdr = vaxis.widgets.alignment.center(hdr_win, @min(col_width -| 1, hdr_txt.len +| 1), 1);
hdr_win.fill(.{ .style = .{ .bg = hdr_bg } });
var seg = [_]vaxis.Cell.Segment{.{
.text = if (hdr_txt.len > col_width and alloc != null) try fmt.allocPrint(alloc.?, "{s}...", .{hdr_txt[0..(col_width -| 4)]}) else hdr_txt,
.style = .{
.bg = hdr_bg,
.bold = true,
.ul_style = if (idx == table_ctx.col) .single else .dotted,
},
}};
_ = try hdr.print(seg[0..], .{ .wrap = .word });
}
if (table_ctx.active_content_fn == null) table_ctx.active_y_off = 0;
const max_items =
if (data_items.len > table_win.height -| 1) table_win.height -| 1 else data_items.len;
var end = table_ctx.start + max_items;
if (table_ctx.row + table_ctx.active_y_off >= end -| 1)
end -|= table_ctx.active_y_off;
if (end > data_items.len) end = data_items.len;
table_ctx.start = tableStart: {
if (table_ctx.row == 0)
break :tableStart 0;
if (table_ctx.row < table_ctx.start)
break :tableStart table_ctx.start - (table_ctx.start - table_ctx.row);
if (table_ctx.row >= data_items.len - 1)
table_ctx.row = data_items.len - 1;
if (table_ctx.row >= end)
break :tableStart table_ctx.start + (table_ctx.row - end + 1);
break :tableStart table_ctx.start;
};
end = table_ctx.start + max_items;
if (table_ctx.row + table_ctx.active_y_off >= end -| 1)
end -|= table_ctx.active_y_off;
if (end > data_items.len) end = data_items.len;
table_ctx.active_y_off = 0;
for (data_items[table_ctx.start..end], 0..) |data, row| {
const row_bg = rowBG: {
if (table_ctx.active and table_ctx.start + row == table_ctx.row)
break :rowBG table_ctx.active_bg;
if (table_ctx.sel_rows) |rows| {
if (mem.indexOfScalar(usize, rows, table_ctx.start + row) != null) break :rowBG table_ctx.selected_bg;
}
if (row % 2 == 0) break :rowBG table_ctx.row_bg_1;
break :rowBG table_ctx.row_bg_2;
};
var row_win = table_win.child(.{
.x_off = 0,
.y_off = 1 + row + table_ctx.active_y_off,
.width = .{ .limit = table_win.width },
.height = .{ .limit = 1 },
});
if (table_ctx.start + row == table_ctx.row) {
table_ctx.active_y_off = if (table_ctx.active_content_fn) |content| try content(&row_win, table_ctx.active_ctx) else 0;
}
const DataT = @TypeOf(data);
col_start = 0;
const item_fields = meta.fields(DataT);
inline for (item_fields[0..], 0..) |item_field, item_idx| contFields: {
switch (table_ctx.col_indexes) {
.all => {},
.by_idx => |idxs| {
if (mem.indexOfScalar(usize, idxs, item_idx) == null) break :contFields;
},
}
const col_width = try calcColWidth(
item_idx,
headers,
table_ctx.col_width,
table_win,
);
defer col_start += col_width;
const item = @field(data, item_field.name);
const ItemT = @TypeOf(item);
const item_win = row_win.child(.{
.x_off = col_start,
.y_off = 0,
.width = .{ .limit = col_width },
.height = .{ .limit = 1 },
});
const item_txt = switch (ItemT) {
[]const u8 => item,
[][]const u8, []const []const u8 => strSlice: {
if (alloc) |_alloc| break :strSlice try fmt.allocPrint(_alloc, "{s}", .{item});
break :strSlice item;
},
else => nonStr: {
switch (@typeInfo(ItemT)) {
.Enum => break :nonStr @tagName(item),
.Optional => {
const opt_item = item orelse break :nonStr "-";
switch (@typeInfo(ItemT).Optional.child) {
[]const u8 => break :nonStr opt_item,
[][]const u8, []const []const u8 => {
break :nonStr if (alloc) |_alloc| try fmt.allocPrint(_alloc, "{s}", .{opt_item}) else fmt.comptimePrint("[unsupported ({s})]", .{@typeName(DataT)});
},
else => {
break :nonStr if (alloc) |_alloc| try fmt.allocPrint(_alloc, "{any}", .{opt_item}) else fmt.comptimePrint("[unsupported ({s})]", .{@typeName(DataT)});
},
}
},
else => {
break :nonStr if (alloc) |_alloc| try fmt.allocPrint(_alloc, "{any}", .{item}) else fmt.comptimePrint("[unsupported ({s})]", .{@typeName(DataT)});
},
}
},
};
item_win.fill(.{ .style = .{ .bg = row_bg } });
var seg = [_]vaxis.Cell.Segment{.{
.text = if (item_txt.len > col_width and alloc != null) try fmt.allocPrint(alloc.?, "{s}...", .{item_txt[0..(col_width -| 4)]}) else item_txt,
.style = .{ .bg = row_bg },
}};
_ = try item_win.print(seg[0..], .{ .wrap = .word });
}
}
}
/// Calculate the Column Width of `col` using the provided Number of Headers (`num_hdrs`), Width Style (`style`), and Table Window (`table_win`).
pub fn calcColWidth(
col: usize,
headers: []const []const u8,
style: WidthStyle,
table_win: vaxis.Window,
) !usize {
return switch (style) {
.dynamic_fill => dynFill: {
var cw = table_win.width / headers.len;
if (cw % 2 != 0) cw +|= 1;
while (cw * headers.len < table_win.width - 1) cw +|= 1;
break :dynFill cw;
},
.dynamic_header_len => dynHdrs: {
if (col >= headers.len) break :dynHdrs error.NotEnoughStaticWidthsProvided;
break :dynHdrs headers[col].len + (style.dynamic_header_len * 2);
},
.static_all => style.static_all,
.static_individual => statInd: {
if (col >= headers.len) break :statInd error.NotEnoughStaticWidthsProvided;
break :statInd style.static_individual[col];
},
};
}
|
0 | repos/libvaxis/src | repos/libvaxis/src/widgets/CodeView.zig | const std = @import("std");
const vaxis = @import("../main.zig");
const ScrollView = vaxis.widgets.ScrollView;
const LineNumbers = vaxis.widgets.LineNumbers;
pub const DrawOptions = struct {
highlighted_line: usize = 0,
draw_line_numbers: bool = true,
indentation: usize = 0,
};
pub const Buffer = vaxis.widgets.TextView.Buffer;
scroll_view: ScrollView = .{ .vertical_scrollbar = null },
highlighted_style: vaxis.Style = .{ .bg = .{ .index = 0 } },
indentation_cell: vaxis.Cell = .{
.char = .{
.grapheme = "┆",
.width = 1,
},
.style = .{ .dim = true },
},
pub fn input(self: *@This(), key: vaxis.Key) void {
self.scroll_view.input(key);
}
pub fn draw(self: *@This(), win: vaxis.Window, buffer: Buffer, opts: DrawOptions) void {
const pad_left: usize = if (opts.draw_line_numbers) LineNumbers.numDigits(buffer.rows) +| 1 else 0;
self.scroll_view.draw(win, .{
.cols = buffer.cols + pad_left,
.rows = buffer.rows,
});
if (opts.draw_line_numbers) {
var nl: LineNumbers = .{
.highlighted_line = opts.highlighted_line,
.num_lines = buffer.rows +| 1,
};
nl.draw(win.child(.{
.x_off = 0,
.y_off = 0,
.width = .{ .limit = pad_left },
.height = .{ .limit = win.height },
}), self.scroll_view.scroll.y);
}
self.drawCode(win.child(.{ .x_off = pad_left }), buffer, opts);
}
fn drawCode(self: *@This(), win: vaxis.Window, buffer: Buffer, opts: DrawOptions) void {
const Pos = struct { x: usize = 0, y: usize = 0 };
var pos: Pos = .{};
var byte_index: usize = 0;
var is_indentation = true;
const bounds = self.scroll_view.bounds(win);
for (buffer.grapheme.items(.len), buffer.grapheme.items(.offset), 0..) |g_len, g_offset, index| {
if (bounds.above(pos.y)) {
break;
}
const cluster = buffer.content.items[g_offset..][0..g_len];
defer byte_index += cluster.len;
if (std.mem.eql(u8, cluster, "\n")) {
if (index == buffer.grapheme.len - 1) {
break;
}
pos.y += 1;
pos.x = 0;
is_indentation = true;
continue;
} else if (bounds.below(pos.y)) {
continue;
}
const highlighted_line = pos.y +| 1 == opts.highlighted_line;
var style: vaxis.Style = if (highlighted_line) self.highlighted_style else .{};
if (buffer.style_map.get(byte_index)) |meta| {
const tmp = style.bg;
style = buffer.style_list.items[meta];
style.bg = tmp;
}
const width = win.gwidth(cluster);
defer pos.x +|= width;
if (!bounds.colInside(pos.x)) {
continue;
}
if (opts.indentation > 0 and !std.mem.eql(u8, cluster, " ")) {
is_indentation = false;
}
if (is_indentation and opts.indentation > 0 and pos.x % opts.indentation == 0) {
var cell = self.indentation_cell;
cell.style.bg = style.bg;
self.scroll_view.writeCell(win, pos.x, pos.y, cell);
} else {
self.scroll_view.writeCell(win, pos.x, pos.y, .{
.char = .{ .grapheme = cluster, .width = width },
.style = style,
});
}
if (highlighted_line) {
for (pos.x +| width..bounds.x2) |x| {
self.scroll_view.writeCell(win, x, pos.y, .{ .style = style });
}
}
}
}
|
0 | repos/libvaxis/src/widgets | repos/libvaxis/src/widgets/terminal/key.zig | const std = @import("std");
const vaxis = @import("../../main.zig");
pub fn encode(
writer: std.io.AnyWriter,
key: vaxis.Key,
press: bool,
kitty_flags: vaxis.Key.KittyFlags,
) !void {
const flags: u5 = @bitCast(kitty_flags);
switch (press) {
true => {
switch (flags) {
0 => try legacy(writer, key),
else => unreachable, // TODO: kitty encodings
}
},
false => {},
}
}
fn legacy(writer: std.io.AnyWriter, key: vaxis.Key) !void {
// If we have text, we always write it directly
if (key.text) |text| {
try writer.writeAll(text);
return;
}
const shift = 0b00000001;
const alt = 0b00000010;
const ctrl = 0b00000100;
const effective_mods: u8 = blk: {
const mods: u8 = @bitCast(key.mods);
break :blk mods & (shift | alt | ctrl);
};
// If we have no mods and an ascii byte, write it directly
if (effective_mods == 0 and key.codepoint <= 0x7F) {
const b: u8 = @truncate(key.codepoint);
try writer.writeByte(b);
return;
}
// If we are lowercase ascii and ctrl, we map to a control byte
if (effective_mods == ctrl and key.codepoint >= 'a' and key.codepoint <= 'z') {
const b: u8 = @truncate(key.codepoint);
try writer.writeByte(b -| 0x60);
return;
}
// If we are printable ascii + alt
if (effective_mods == alt and key.codepoint >= ' ' and key.codepoint < 0x7F) {
const b: u8 = @truncate(key.codepoint);
try writer.print("\x1b{c}", .{b});
return;
}
// If we are ctrl + alt + lowercase ascii
if (effective_mods == (ctrl | alt) and key.codepoint >= 'a' and key.codepoint <= 'z') {
// convert to control sequence
try writer.print("\x1b{d}", .{key.codepoint - 0x60});
}
const def = switch (key.codepoint) {
vaxis.Key.escape => escape,
vaxis.Key.enter,
vaxis.Key.kp_enter,
=> enter,
vaxis.Key.tab => tab,
vaxis.Key.backspace => backspace,
vaxis.Key.insert,
vaxis.Key.kp_insert,
=> insert,
vaxis.Key.delete,
vaxis.Key.kp_delete,
=> delete,
vaxis.Key.left,
vaxis.Key.kp_left,
=> left,
vaxis.Key.right,
vaxis.Key.kp_right,
=> right,
vaxis.Key.up,
vaxis.Key.kp_up,
=> up,
vaxis.Key.down,
vaxis.Key.kp_down,
=> down,
vaxis.Key.page_up,
vaxis.Key.kp_page_up,
=> page_up,
vaxis.Key.page_down,
vaxis.Key.kp_page_down,
=> page_down,
vaxis.Key.home,
vaxis.Key.kp_home,
=> home,
vaxis.Key.end,
vaxis.Key.kp_end,
=> end,
vaxis.Key.f1 => f1,
vaxis.Key.f2 => f2,
vaxis.Key.f3 => f3_legacy,
vaxis.Key.f4 => f4,
vaxis.Key.f5 => f5,
vaxis.Key.f6 => f6,
vaxis.Key.f7 => f7,
vaxis.Key.f8 => f8,
vaxis.Key.f9 => f9,
vaxis.Key.f10 => f10,
vaxis.Key.f11 => f11,
vaxis.Key.f12 => f12,
else => return, // TODO: more keys
};
switch (effective_mods) {
0 => {
if (def.number == 1)
switch (key.codepoint) {
vaxis.Key.f1,
vaxis.Key.f2,
vaxis.Key.f3,
vaxis.Key.f4,
=> try writer.print("\x1bO{c}", .{def.suffix}),
else => try writer.print("\x1b[{c}", .{def.suffix}),
}
else
try writer.print("\x1b[{d}{c}", .{ def.number, def.suffix });
},
else => try writer.print("\x1b[{d};{d}{c}", .{ def.number, effective_mods + 1, def.suffix }),
}
}
const Definition = struct {
number: u21,
suffix: u8,
};
const escape: Definition = .{ .number = 27, .suffix = 'u' };
const enter: Definition = .{ .number = 13, .suffix = 'u' };
const tab: Definition = .{ .number = 9, .suffix = 'u' };
const backspace: Definition = .{ .number = 127, .suffix = 'u' };
const insert: Definition = .{ .number = 2, .suffix = '~' };
const delete: Definition = .{ .number = 3, .suffix = '~' };
const left: Definition = .{ .number = 1, .suffix = 'D' };
const right: Definition = .{ .number = 1, .suffix = 'C' };
const up: Definition = .{ .number = 1, .suffix = 'A' };
const down: Definition = .{ .number = 1, .suffix = 'B' };
const page_up: Definition = .{ .number = 5, .suffix = '~' };
const page_down: Definition = .{ .number = 6, .suffix = '~' };
const home: Definition = .{ .number = 1, .suffix = 'H' };
const end: Definition = .{ .number = 1, .suffix = 'F' };
const caps_lock: Definition = .{ .number = 57358, .suffix = 'u' };
const scroll_lock: Definition = .{ .number = 57359, .suffix = 'u' };
const num_lock: Definition = .{ .number = 57360, .suffix = 'u' };
const print_screen: Definition = .{ .number = 57361, .suffix = 'u' };
const pause: Definition = .{ .number = 57362, .suffix = 'u' };
const menu: Definition = .{ .number = 57363, .suffix = 'u' };
const f1: Definition = .{ .number = 1, .suffix = 'P' };
const f2: Definition = .{ .number = 1, .suffix = 'Q' };
const f3: Definition = .{ .number = 13, .suffix = '~' };
const f3_legacy: Definition = .{ .number = 1, .suffix = 'R' };
const f4: Definition = .{ .number = 1, .suffix = 'S' };
const f5: Definition = .{ .number = 15, .suffix = '~' };
const f6: Definition = .{ .number = 17, .suffix = '~' };
const f7: Definition = .{ .number = 18, .suffix = '~' };
const f8: Definition = .{ .number = 19, .suffix = '~' };
const f9: Definition = .{ .number = 20, .suffix = '~' };
const f10: Definition = .{ .number = 21, .suffix = '~' };
const f11: Definition = .{ .number = 23, .suffix = '~' };
const f12: Definition = .{ .number = 24, .suffix = '~' };
|
0 | repos/libvaxis/src/widgets | repos/libvaxis/src/widgets/terminal/Screen.zig | const std = @import("std");
const assert = std.debug.assert;
const vaxis = @import("../../main.zig");
const ansi = @import("ansi.zig");
const log = std.log.scoped(.vaxis_terminal);
const Screen = @This();
pub const Cell = struct {
char: std.ArrayList(u8) = undefined,
style: vaxis.Style = .{},
uri: std.ArrayList(u8) = undefined,
uri_id: std.ArrayList(u8) = undefined,
width: u8 = 1,
wrapped: bool = false,
dirty: bool = true,
pub fn erase(self: *Cell, bg: vaxis.Color) void {
self.char.clearRetainingCapacity();
self.char.append(' ') catch unreachable; // we never completely free this list
self.style = .{};
self.style.bg = bg;
self.uri.clearRetainingCapacity();
self.uri_id.clearRetainingCapacity();
self.width = 1;
self.wrapped = false;
self.dirty = true;
}
pub fn copyFrom(self: *Cell, src: Cell) !void {
self.char.clearRetainingCapacity();
try self.char.appendSlice(src.char.items);
self.style = src.style;
self.uri.clearRetainingCapacity();
try self.uri.appendSlice(src.uri.items);
self.uri_id.clearRetainingCapacity();
try self.uri_id.appendSlice(src.uri_id.items);
self.width = src.width;
self.wrapped = src.wrapped;
self.dirty = true;
}
};
pub const Cursor = struct {
style: vaxis.Style = .{},
uri: std.ArrayList(u8) = undefined,
uri_id: std.ArrayList(u8) = undefined,
col: usize = 0,
row: usize = 0,
pending_wrap: bool = false,
shape: vaxis.Cell.CursorShape = .default,
visible: bool = true,
pub fn isOutsideScrollingRegion(self: Cursor, sr: ScrollingRegion) bool {
return self.row < sr.top or
self.row > sr.bottom or
self.col < sr.left or
self.col > sr.right;
}
pub fn isInsideScrollingRegion(self: Cursor, sr: ScrollingRegion) bool {
return !self.isOutsideScrollingRegion(sr);
}
};
pub const ScrollingRegion = struct {
top: usize,
bottom: usize,
left: usize,
right: usize,
pub fn contains(self: ScrollingRegion, col: usize, row: usize) bool {
return col >= self.left and
col <= self.right and
row >= self.top and
row <= self.bottom;
}
};
width: usize = 0,
height: usize = 0,
scrolling_region: ScrollingRegion,
buf: []Cell = undefined,
cursor: Cursor = .{},
csi_u_flags: vaxis.Key.KittyFlags = @bitCast(@as(u5, 0)),
/// sets each cell to the default cell
pub fn init(alloc: std.mem.Allocator, w: usize, h: usize) !Screen {
var screen = Screen{
.buf = try alloc.alloc(Cell, w * h),
.scrolling_region = .{
.top = 0,
.bottom = h - 1,
.left = 0,
.right = w - 1,
},
.width = w,
.height = h,
};
for (screen.buf, 0..) |_, i| {
screen.buf[i] = .{
.char = try std.ArrayList(u8).initCapacity(alloc, 1),
.uri = std.ArrayList(u8).init(alloc),
.uri_id = std.ArrayList(u8).init(alloc),
};
try screen.buf[i].char.append(' ');
}
return screen;
}
pub fn deinit(self: *Screen, alloc: std.mem.Allocator) void {
for (self.buf, 0..) |_, i| {
self.buf[i].char.deinit();
self.buf[i].uri.deinit();
self.buf[i].uri_id.deinit();
}
alloc.free(self.buf);
}
/// copies the visible area to the destination screen
pub fn copyTo(self: *Screen, dst: *Screen) !void {
dst.cursor = self.cursor;
for (self.buf, 0..) |cell, i| {
if (!cell.dirty) continue;
self.buf[i].dirty = false;
const grapheme = cell.char.items;
dst.buf[i].char.clearRetainingCapacity();
try dst.buf[i].char.appendSlice(grapheme);
dst.buf[i].width = cell.width;
dst.buf[i].style = cell.style;
}
}
pub fn readCell(self: *Screen, col: usize, row: usize) ?vaxis.Cell {
if (self.width < col) {
// column out of bounds
return null;
}
if (self.height < row) {
// height out of bounds
return null;
}
const i = (row * self.width) + col;
assert(i < self.buf.len);
const cell = self.buf[i];
return .{
.char = .{ .grapheme = cell.char.items, .width = cell.width },
.style = cell.style,
};
}
/// returns true if the current cursor position is within the scrolling region
pub fn withinScrollingRegion(self: Screen) bool {
return self.scrolling_region.contains(self.cursor.col, self.cursor.row);
}
/// writes a cell to a location. 0 indexed
pub fn print(
self: *Screen,
grapheme: []const u8,
width: u8,
wrap: bool,
) !void {
if (self.cursor.pending_wrap) {
try self.index();
self.cursor.col = self.scrolling_region.left;
}
if (self.cursor.col >= self.width) return;
if (self.cursor.row >= self.height) return;
const col = self.cursor.col;
const row = self.cursor.row;
const i = (row * self.width) + col;
assert(i < self.buf.len);
self.buf[i].char.clearRetainingCapacity();
self.buf[i].char.appendSlice(grapheme) catch {
log.warn("couldn't write grapheme", .{});
};
self.buf[i].uri.clearRetainingCapacity();
self.buf[i].uri.appendSlice(self.cursor.uri.items) catch {
log.warn("couldn't write uri", .{});
};
self.buf[i].uri_id.clearRetainingCapacity();
self.buf[i].uri_id.appendSlice(self.cursor.uri_id.items) catch {
log.warn("couldn't write uri_id", .{});
};
self.buf[i].style = self.cursor.style;
self.buf[i].width = width;
self.buf[i].dirty = true;
if (wrap and self.cursor.col >= self.width - 1) self.cursor.pending_wrap = true;
self.cursor.col += width;
}
/// IND
pub fn index(self: *Screen) !void {
self.cursor.pending_wrap = false;
if (self.cursor.isOutsideScrollingRegion(self.scrolling_region)) {
// Outside, we just move cursor down one
self.cursor.row = @min(self.height - 1, self.cursor.row + 1);
return;
}
// We are inside the scrolling region
if (self.cursor.row == self.scrolling_region.bottom) {
// Inside scrolling region *and* at bottom of screen, we scroll contents up and insert a
// blank line
// TODO: scrollback if scrolling region is entire visible screen
try self.deleteLine(1);
return;
}
self.cursor.row += 1;
}
pub fn sgr(self: *Screen, seq: ansi.CSI) void {
if (seq.params.len == 0) {
self.cursor.style = .{};
return;
}
var iter = seq.iterator(u8);
while (iter.next()) |ps| {
switch (ps) {
0 => self.cursor.style = .{},
1 => self.cursor.style.bold = true,
2 => self.cursor.style.dim = true,
3 => self.cursor.style.italic = true,
4 => {
const kind: vaxis.Style.Underline = if (iter.next_is_sub)
@enumFromInt(iter.next() orelse 1)
else
.single;
self.cursor.style.ul_style = kind;
},
5 => self.cursor.style.blink = true,
7 => self.cursor.style.reverse = true,
8 => self.cursor.style.invisible = true,
9 => self.cursor.style.strikethrough = true,
21 => self.cursor.style.ul_style = .double,
22 => {
self.cursor.style.bold = false;
self.cursor.style.dim = false;
},
23 => self.cursor.style.italic = false,
24 => self.cursor.style.ul_style = .off,
25 => self.cursor.style.blink = false,
27 => self.cursor.style.reverse = false,
28 => self.cursor.style.invisible = false,
29 => self.cursor.style.strikethrough = false,
30...37 => self.cursor.style.fg = .{ .index = ps - 30 },
38 => {
// must have another parameter
const kind = iter.next() orelse return;
switch (kind) {
2 => { // rgb
const r = r: {
// First param can be empty
var ps_r = iter.next() orelse return;
if (iter.is_empty)
ps_r = iter.next() orelse return;
break :r ps_r;
};
const g = iter.next() orelse return;
const b = iter.next() orelse return;
self.cursor.style.fg = .{ .rgb = .{ r, g, b } };
},
5 => {
const idx = iter.next() orelse return;
self.cursor.style.fg = .{ .index = idx };
}, // index
else => return,
}
},
39 => self.cursor.style.fg = .default,
40...47 => self.cursor.style.bg = .{ .index = ps - 40 },
48 => {
// must have another parameter
const kind = iter.next() orelse return;
switch (kind) {
2 => { // rgb
const r = r: {
// First param can be empty
var ps_r = iter.next() orelse return;
if (iter.is_empty)
ps_r = iter.next() orelse return;
break :r ps_r;
};
const g = iter.next() orelse return;
const b = iter.next() orelse return;
self.cursor.style.bg = .{ .rgb = .{ r, g, b } };
},
5 => {
const idx = iter.next() orelse return;
self.cursor.style.bg = .{ .index = idx };
}, // index
else => return,
}
},
49 => self.cursor.style.bg = .default,
90...97 => self.cursor.style.fg = .{ .index = ps - 90 + 8 },
100...107 => self.cursor.style.bg = .{ .index = ps - 100 + 8 },
else => continue,
}
}
}
pub fn cursorUp(self: *Screen, n: usize) void {
self.cursor.pending_wrap = false;
if (self.withinScrollingRegion())
self.cursor.row = @max(
self.cursor.row -| n,
self.scrolling_region.top,
)
else
self.cursor.row -|= n;
}
pub fn cursorLeft(self: *Screen, n: usize) void {
self.cursor.pending_wrap = false;
if (self.withinScrollingRegion())
self.cursor.col = @max(
self.cursor.col -| n,
self.scrolling_region.left,
)
else
self.cursor.col = self.cursor.col -| n;
}
pub fn cursorRight(self: *Screen, n: usize) void {
self.cursor.pending_wrap = false;
if (self.withinScrollingRegion())
self.cursor.col = @min(
self.cursor.col + n,
self.scrolling_region.right,
)
else
self.cursor.col = @min(
self.cursor.col + n,
self.width - 1,
);
}
pub fn cursorDown(self: *Screen, n: usize) void {
self.cursor.pending_wrap = false;
if (self.withinScrollingRegion())
self.cursor.row = @min(
self.scrolling_region.bottom,
self.cursor.row + n,
)
else
self.cursor.row = @min(
self.height -| 1,
self.cursor.row + n,
);
}
pub fn eraseRight(self: *Screen) void {
self.cursor.pending_wrap = false;
const end = (self.cursor.row * self.width) + (self.width);
var i = (self.cursor.row * self.width) + self.cursor.col;
while (i < end) : (i += 1) {
self.buf[i].erase(self.cursor.style.bg);
}
}
pub fn eraseLeft(self: *Screen) void {
self.cursor.pending_wrap = false;
const start = self.cursor.row * self.width;
const end = start + self.cursor.col + 1;
var i = start;
while (i < end) : (i += 1) {
self.buf[i].erase(self.cursor.style.bg);
}
}
pub fn eraseLine(self: *Screen) void {
self.cursor.pending_wrap = false;
const start = self.cursor.row * self.width;
const end = start + self.width;
var i = start;
while (i < end) : (i += 1) {
self.buf[i].erase(self.cursor.style.bg);
}
}
/// delete n lines from the bottom of the scrolling region
pub fn deleteLine(self: *Screen, n: usize) !void {
if (n == 0) return;
// Don't delete if outside scroll region
if (!self.withinScrollingRegion()) return;
self.cursor.pending_wrap = false;
// Number of rows from here to bottom of scroll region or n
const cnt = @min(self.scrolling_region.bottom - self.cursor.row + 1, n);
const stride = (self.width) * cnt;
var row: usize = self.scrolling_region.top;
while (row <= self.scrolling_region.bottom) : (row += 1) {
var col: usize = self.scrolling_region.left;
while (col <= self.scrolling_region.right) : (col += 1) {
const i = (row * self.width) + col;
if (row + cnt > self.scrolling_region.bottom)
self.buf[i].erase(self.cursor.style.bg)
else
try self.buf[i].copyFrom(self.buf[i + stride]);
}
}
}
/// insert n lines at the top of the scrolling region
pub fn insertLine(self: *Screen, n: usize) !void {
if (n == 0) return;
self.cursor.pending_wrap = false;
// Don't insert if outside scroll region
if (!self.withinScrollingRegion()) return;
const adjusted_n = @min(self.scrolling_region.bottom - self.cursor.row, n);
const stride = (self.width) * adjusted_n;
var row: usize = self.scrolling_region.bottom;
while (row >= self.scrolling_region.top + adjusted_n) : (row -|= 1) {
var col: usize = self.scrolling_region.left;
while (col <= self.scrolling_region.right) : (col += 1) {
const i = (row * self.width) + col;
try self.buf[i].copyFrom(self.buf[i - stride]);
}
}
row = self.scrolling_region.top;
while (row < self.scrolling_region.top + adjusted_n) : (row += 1) {
var col: usize = self.scrolling_region.left;
while (col <= self.scrolling_region.right) : (col += 1) {
const i = (row * self.width) + col;
self.buf[i].erase(self.cursor.style.bg);
}
}
}
pub fn eraseBelow(self: *Screen) void {
self.eraseRight();
// start is the first column of the row below us
const start = (self.cursor.row * self.width) + (self.width);
var i = start;
while (i < self.buf.len) : (i += 1) {
self.buf[i].erase(self.cursor.style.bg);
}
}
pub fn eraseAbove(self: *Screen) void {
self.eraseLeft();
// start is the first column of the row below us
const start: usize = 0;
const end = self.cursor.row * self.width;
var i = start;
while (i < end) : (i += 1) {
self.buf[i].erase(self.cursor.style.bg);
}
}
pub fn eraseAll(self: *Screen) void {
var i: usize = 0;
while (i < self.buf.len) : (i += 1) {
self.buf[i].erase(self.cursor.style.bg);
}
}
pub fn deleteCharacters(self: *Screen, n: usize) !void {
if (!self.withinScrollingRegion()) return;
self.cursor.pending_wrap = false;
var col = self.cursor.col;
while (col <= self.scrolling_region.right) : (col += 1) {
if (col + n <= self.scrolling_region.right)
try self.buf[col].copyFrom(self.buf[col + n])
else
self.buf[col].erase(self.cursor.style.bg);
}
}
pub fn reverseIndex(self: *Screen) !void {
if (self.cursor.row != self.scrolling_region.top or
self.cursor.col < self.scrolling_region.left or
self.cursor.col > self.scrolling_region.right)
self.cursorUp(1)
else
try self.scrollDown(1);
}
pub fn scrollDown(self: *Screen, n: usize) !void {
const cur_row = self.cursor.row;
const cur_col = self.cursor.col;
const wrap = self.cursor.pending_wrap;
defer {
self.cursor.row = cur_row;
self.cursor.col = cur_col;
self.cursor.pending_wrap = wrap;
}
self.cursor.col = self.scrolling_region.left;
self.cursor.row = self.scrolling_region.top;
try self.insertLine(n);
}
|
0 | repos/libvaxis/src/widgets | repos/libvaxis/src/widgets/terminal/Command.zig | const Command = @This();
const std = @import("std");
const builtin = @import("builtin");
const Pty = @import("Pty.zig");
const Terminal = @import("Terminal.zig");
const posix = std.posix;
argv: []const []const u8,
working_directory: ?[]const u8,
// Set after spawn()
pid: ?std.posix.pid_t = null,
env_map: *const std.process.EnvMap,
pty: Pty,
pub fn spawn(self: *Command, allocator: std.mem.Allocator) !void {
var arena_allocator = std.heap.ArenaAllocator.init(allocator);
defer arena_allocator.deinit();
const arena = arena_allocator.allocator();
const argv_buf = try arena.allocSentinel(?[*:0]const u8, self.argv.len, null);
for (self.argv, 0..) |arg, i| argv_buf[i] = (try arena.dupeZ(u8, arg)).ptr;
const envp = try createEnvironFromMap(arena, self.env_map);
const pid = try std.posix.fork();
if (pid == 0) {
// we are the child
_ = std.os.linux.setsid();
// set the controlling terminal
var u: c_uint = std.posix.STDIN_FILENO;
if (posix.system.ioctl(self.pty.tty, posix.T.IOCSCTTY, @intFromPtr(&u)) != 0) return error.IoctlError;
// set up io
try posix.dup2(self.pty.tty, std.posix.STDIN_FILENO);
try posix.dup2(self.pty.tty, std.posix.STDOUT_FILENO);
try posix.dup2(self.pty.tty, std.posix.STDERR_FILENO);
posix.close(self.pty.tty);
if (self.pty.pty > 2) posix.close(self.pty.pty);
if (self.working_directory) |wd| {
try std.posix.chdir(wd);
}
// exec
const err = std.posix.execvpeZ(argv_buf.ptr[0].?, argv_buf.ptr, envp);
_ = err catch {};
}
// we are the parent
self.pid = @intCast(pid);
if (!Terminal.global_sigchild_installed) {
Terminal.global_sigchild_installed = true;
var act = posix.Sigaction{
.handler = .{ .handler = handleSigChild },
.mask = switch (builtin.os.tag) {
.macos => 0,
.linux => posix.empty_sigset,
else => @compileError("os not supported"),
},
.flags = 0,
};
try posix.sigaction(posix.SIG.CHLD, &act, null);
}
return;
}
fn handleSigChild(_: c_int) callconv(.C) void {
const result = std.posix.waitpid(-1, 0);
Terminal.global_vt_mutex.lock();
defer Terminal.global_vt_mutex.unlock();
if (Terminal.global_vts) |vts| {
var vt = vts.get(result.pid) orelse return;
vt.event_queue.push(.exited);
}
}
pub fn kill(self: *Command) void {
if (self.pid) |pid| {
std.posix.kill(pid, std.posix.SIG.TERM) catch {};
self.pid = null;
}
}
/// Creates a null-deliminated environment variable block in the format expected by POSIX, from a
/// hash map plus options.
fn createEnvironFromMap(
arena: std.mem.Allocator,
map: *const std.process.EnvMap,
) ![:null]?[*:0]u8 {
const envp_count: usize = map.count();
const envp_buf = try arena.allocSentinel(?[*:0]u8, envp_count, null);
var i: usize = 0;
{
var it = map.iterator();
while (it.next()) |pair| {
envp_buf[i] = try std.fmt.allocPrintZ(arena, "{s}={s}", .{ pair.key_ptr.*, pair.value_ptr.* });
i += 1;
}
}
std.debug.assert(i == envp_count);
return envp_buf;
}
|
0 | repos/libvaxis/src/widgets | repos/libvaxis/src/widgets/terminal/ansi.zig | const std = @import("std");
/// Control bytes. See man 7 ascii
pub const C0 = enum(u8) {
NUL = 0x00,
SOH = 0x01,
STX = 0x02,
ETX = 0x03,
EOT = 0x04,
ENQ = 0x05,
ACK = 0x06,
BEL = 0x07,
BS = 0x08,
HT = 0x09,
LF = 0x0a,
VT = 0x0b,
FF = 0x0c,
CR = 0x0d,
SO = 0x0e,
SI = 0x0f,
DLE = 0x10,
DC1 = 0x11,
DC2 = 0x12,
DC3 = 0x13,
DC4 = 0x14,
NAK = 0x15,
SYN = 0x16,
ETB = 0x17,
CAN = 0x18,
EM = 0x19,
SUB = 0x1a,
ESC = 0x1b,
FS = 0x1c,
GS = 0x1d,
RS = 0x1e,
US = 0x1f,
};
pub const CSI = struct {
intermediate: ?u8 = null,
private_marker: ?u8 = null,
final: u8,
params: []const u8,
pub fn hasIntermediate(self: CSI, b: u8) bool {
return b == self.intermediate orelse return false;
}
pub fn hasPrivateMarker(self: CSI, b: u8) bool {
return b == self.private_marker orelse return false;
}
pub fn iterator(self: CSI, comptime T: type) ParamIterator(T) {
return .{ .bytes = self.params };
}
pub fn format(
self: CSI,
comptime layout: []const u8,
opts: std.fmt.FormatOptions,
writer: anytype,
) !void {
_ = layout;
_ = opts;
if (self.private_marker == null and self.intermediate == null)
try std.fmt.format(writer, "CSI {s} {c}", .{
self.params,
self.final,
})
else if (self.private_marker != null and self.intermediate == null)
try std.fmt.format(writer, "CSI {c} {s} {c}", .{
self.private_marker.?,
self.params,
self.final,
})
else if (self.private_marker == null and self.intermediate != null)
try std.fmt.format(writer, "CSI {s} {c} {c}", .{
self.params,
self.intermediate.?,
self.final,
})
else
try std.fmt.format(writer, "CSI {c} {s} {c} {c}", .{
self.private_marker.?,
self.params,
self.intermediate.?,
self.final,
});
}
};
pub fn ParamIterator(T: type) type {
return struct {
const Self = @This();
bytes: []const u8,
idx: usize = 0,
/// indicates the next parameter will be a sub parameter of the current
next_is_sub: bool = false,
/// indicates the current parameter was an empty string
is_empty: bool = false,
pub fn next(self: *Self) ?T {
// reset state
self.next_is_sub = false;
self.is_empty = false;
const start = self.idx;
var val: T = 0;
while (self.idx < self.bytes.len) {
defer self.idx += 1; // defer so we trigger on return as well
const b = self.bytes[self.idx];
switch (b) {
0x30...0x39 => {
val = (val * 10) + (b - 0x30);
if (self.idx == self.bytes.len - 1) return val;
},
':', ';' => {
self.next_is_sub = b == ':';
self.is_empty = self.idx == start;
return val;
},
else => return null,
}
}
return null;
}
/// verifies there are at least n more parameters
pub fn hasAtLeast(self: *Self, n: usize) bool {
const start = self.idx;
defer self.idx = start;
var i: usize = 0;
while (self.next()) |_| {
i += 1;
if (i >= n) return true;
}
return i >= n;
}
};
}
|