diff --git "a/unit2/smolagents/multiagent_notebook.ipynb" "b/unit2/smolagents/multiagent_notebook.ipynb"
new file mode 100644--- /dev/null
+++ "b/unit2/smolagents/multiagent_notebook.ipynb"
@@ -0,0 +1,8639 @@
+{
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "22ubrk7navwL"
+ },
+ "source": [
+ "# Solving a complex task with a multi-agent hierarchy\n",
+ "\n",
+ "This notebook is part of the [Hugging Face Agents Course](https://www.hf.co/learn/agents-course), a free Course from beginner to expert, where you learn to build Agents.\n",
+ "\n",
+ "\n",
+ "\n",
+ "The reception is approaching! With your help, Alfred is now nearly finished with the preparations.\n",
+ "\n",
+ "But now there's a problem: the Batmobile has disappeared. Alfred needs to find a replacement, and find it quickly.\n",
+ "\n",
+ "Fortunately, a few biopics have been done on Bruce Wayne's life, so maybe Alfred could get a car left behind on one of the movie set, and re-engineer it up to modern standards, which certainly would include a full self-driving option.\n",
+ "\n",
+ "But this could be anywhere in the filming locations around the world - which could be numerous.\n",
+ "\n",
+ "So Alfred wants your help. Could you build an agent able to solve this task?\n",
+ "\n",
+ "> ๐ Find all Batman filming locations in the world, calculate the time to transfer via boat to there, and represent them on a map, with a color varying by boat transfer time. Also represent some supercar factories with the same boat transfer time.\n",
+ "\n",
+ "Let's build this!"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "id": "tdepXvxdavwN"
+ },
+ "outputs": [],
+ "source": [
+ "!pip install 'smolagents[litellm]' matplotlib geopandas shapely kaleido -q"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "id": "wWayJH4m_A9S"
+ },
+ "outputs": [],
+ "source": [
+ "from huggingface_hub import notebook_login\n",
+ "\n",
+ "notebook_login()"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 2,
+ "metadata": {
+ "colab": {
+ "base_uri": "https://localhost:8080/"
+ },
+ "id": "dduWceHAavwO",
+ "outputId": "597a7813-7b3d-43df-f044-cd6370949c23"
+ },
+ "outputs": [
+ {
+ "name": "stdout",
+ "output_type": "stream",
+ "text": [
+ "22.82\n"
+ ]
+ }
+ ],
+ "source": [
+ "# We first make a tool to get the cargo plane transfer time.\n",
+ "import math\n",
+ "from typing import Optional, Tuple\n",
+ "\n",
+ "from smolagents import tool\n",
+ "\n",
+ "\n",
+ "@tool\n",
+ "def calculate_cargo_travel_time(\n",
+ " origin_coords: Tuple[float, float],\n",
+ " destination_coords: Tuple[float, float],\n",
+ " cruising_speed_kmh: Optional[float] = 750.0, # Average speed for cargo planes\n",
+ ") -> float:\n",
+ " \"\"\"\n",
+ " Calculate the travel time for a cargo plane between two points on Earth using great-circle distance.\n",
+ "\n",
+ " Args:\n",
+ " origin_coords: Tuple of (latitude, longitude) for the starting point\n",
+ " destination_coords: Tuple of (latitude, longitude) for the destination\n",
+ " cruising_speed_kmh: Optional cruising speed in km/h (defaults to 750 km/h for typical cargo planes)\n",
+ "\n",
+ " Returns:\n",
+ " float: The estimated travel time in hours\n",
+ "\n",
+ " Example:\n",
+ " >>> # Chicago (41.8781ยฐ N, 87.6298ยฐ W) to Sydney (33.8688ยฐ S, 151.2093ยฐ E)\n",
+ " >>> result = calculate_cargo_travel_time((41.8781, -87.6298), (-33.8688, 151.2093))\n",
+ " \"\"\"\n",
+ "\n",
+ " def to_radians(degrees: float) -> float:\n",
+ " return degrees * (math.pi / 180)\n",
+ "\n",
+ " # Extract coordinates\n",
+ " lat1, lon1 = map(to_radians, origin_coords)\n",
+ " lat2, lon2 = map(to_radians, destination_coords)\n",
+ "\n",
+ " # Earth's radius in kilometers\n",
+ " EARTH_RADIUS_KM = 6371.0\n",
+ "\n",
+ " # Calculate great-circle distance using the haversine formula\n",
+ " dlon = lon2 - lon1\n",
+ " dlat = lat2 - lat1\n",
+ "\n",
+ " a = (\n",
+ " math.sin(dlat / 2) ** 2\n",
+ " + math.cos(lat1) * math.cos(lat2) * math.sin(dlon / 2) ** 2\n",
+ " )\n",
+ " c = 2 * math.asin(math.sqrt(a))\n",
+ " distance = EARTH_RADIUS_KM * c\n",
+ "\n",
+ " # Add 10% to account for non-direct routes and air traffic controls\n",
+ " actual_distance = distance * 1.1\n",
+ "\n",
+ " # Calculate flight time\n",
+ " # Add 1 hour for takeoff and landing procedures\n",
+ " flight_time = (actual_distance / cruising_speed_kmh) + 1.0\n",
+ "\n",
+ " # Format the results\n",
+ " return round(flight_time, 2)\n",
+ "\n",
+ "\n",
+ "print(calculate_cargo_travel_time((41.8781, -87.6298), (-33.8688, 151.2093)))"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "UPgyT9FbavwQ"
+ },
+ "source": [
+ "For the model provider, we use Together AI, one of the new [inference providers on the Hub](https://huggingface.co./blog/inference-providers)!\n",
+ "\n",
+ "Regarding the GoogleSearchTool: this requires either having setup env variable `SERPAPI_API_KEY` and passing `provider=\"serpapi\"` or having `SERPER_API_KEY` and passing `provider=serper`.\n",
+ "\n",
+ "If you don't have any Serp API provider setup, you can use `DuckDuckGoSearchTool` but beware that it has a rate limit."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 3,
+ "metadata": {
+ "id": "gw4q7qODavwQ"
+ },
+ "outputs": [],
+ "source": [
+ "import os\n",
+ "from PIL import Image\n",
+ "from smolagents import CodeAgent, GoogleSearchTool, HfApiModel, VisitWebpageTool\n",
+ "\n",
+ "\n",
+ "model = HfApiModel(model_id=\"Qwen/Qwen2.5-Coder-32B-Instruct\", provider=\"together\")"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "C6ItyGKtavwR"
+ },
+ "source": [
+ "We can start with creating a baseline, simple agent to give us a simple report."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 4,
+ "metadata": {
+ "id": "42C3gu75avwR"
+ },
+ "outputs": [],
+ "source": [
+ "task = \"\"\"Find all Batman filming locations in the world, calculate the time to transfer via cargo plane to here (we're in Gotham, 40.7128ยฐ N, 74.0060ยฐ W), and return them to me as a pandas dataframe.\n",
+ "Also give me some supercar factories with the same cargo plane transfer time.\"\"\""
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 12,
+ "metadata": {
+ "id": "7lvVBlrm_wLn"
+ },
+ "outputs": [],
+ "source": [
+ "from google.colab import userdata\n",
+ "import os\n",
+ "os.environ[\"SERPAPI_API_KEY\"] = userdata.get('SERPAPI_API_KEY')"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 13,
+ "metadata": {
+ "id": "X6ZXgdaGavwS"
+ },
+ "outputs": [],
+ "source": [
+ "agent = CodeAgent(\n",
+ " model=model,\n",
+ " tools=[GoogleSearchTool(), VisitWebpageTool(), calculate_cargo_travel_time],\n",
+ " additional_authorized_imports=[\"pandas\"],\n",
+ " max_steps=20,\n",
+ ")"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 14,
+ "metadata": {
+ "colab": {
+ "base_uri": "https://localhost:8080/",
+ "height": 1000
+ },
+ "id": "apTHCYH1avwT",
+ "outputId": "58c631cd-f158-4fe3-c496-adb9624a71d5"
+ },
+ "outputs": [
+ {
+ "data": {
+ "text/html": [
+ "
โญโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ New run โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฎ\n",
+ "โ โ\n",
+ "โ Find all Batman filming locations in the world, calculate the time to transfer via cargo plane to here (we're โ\n",
+ "โ in Gotham, 40.7128ยฐ N, 74.0060ยฐ W), and return them to me as a pandas dataframe. โ\n",
+ "โ Also give me some supercar factories with the same cargo plane transfer time. โ\n",
+ "โ โ\n",
+ "โฐโ HfApiModel - Qwen/Qwen2.5-Coder-32B-Instruct โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฏ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโญโ\u001b[0m\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\u001b[38;2;212;183;2m \u001b[0m\u001b[1;38;2;212;183;2mNew run\u001b[0m\u001b[38;2;212;183;2m \u001b[0m\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\u001b[38;2;212;183;2mโโฎ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mFind all Batman filming locations in the world, calculate the time to transfer via cargo plane to here (we're \u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1min Gotham, 40.7128ยฐ N, 74.0060ยฐ W), and return them to me as a pandas dataframe.\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mAlso give me some supercar factories with the same cargo plane transfer time.\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโฐโ\u001b[0m\u001b[38;2;212;183;2m HfApiModel - Qwen/Qwen2.5-Coder-32B-Instruct \u001b[0m\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\u001b[38;2;212;183;2mโโฏ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Step 1 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[0m\u001b[1mStep \u001b[0m\u001b[1;36m1\u001b[0m\u001b[38;2;212;183;2m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ " โ Executing parsed code: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " batman_locations = web_search(query=\"Batman filming locations\") \n",
+ " print(batman_locations) \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ "
\n"
+ ],
+ "text/plain": [
+ " โ \u001b[1mExecuting parsed code:\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mbatman_locations\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mweb_search\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mquery\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBatman filming locations\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_locations\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Execution logs:\n",
+ "## Search Results\n",
+ "0. [The Batman (2022) - Filming & production](https://www.imdb.com/title/tt1877830/locations/)\n",
+ "Source: IMDb\n",
+ "\n",
+ "Filming locations: Necropolis Cemetery, Glasgow, Scotland, UK (Batman and Selina leaving the cemetery) Helpfulโข86 1\n",
+ "St. George's Hall, Liverpool, England, UK\n",
+ "\n",
+ "1. [The Batman | Film Locations](https://movie-locations.com/movies/b/The-Batman-2022-2.php)\n",
+ "Source: The Worldwide Guide To Movie Locations\n",
+ "\n",
+ "Film locations for The Batman (2022) in Liverpool, London, Glasgow and Chicago.\n",
+ "\n",
+ "2. [Where was The Batman filmed? ALL the Filming Locations \n",
+ "...](https://www.atlasofwonders.com/2022/04/where-was-the-batman-filmed.html)\n",
+ "Source: Atlas of Wonders\n",
+ "\n",
+ "Guide to the all the filming locations of The Batman - The 2022 movie with Robert Pattison filmed in Chicago and \n",
+ "The UK - The City and the Wayne House.\n",
+ "\n",
+ "3. [Batman (1989) - Filming & production](https://www.imdb.com/title/tt0096895/locations/)\n",
+ "Source: IMDb\n",
+ "\n",
+ "Filming locations: Knebworth House, Knebworth, Hertfordshire, England, UK (Wayne Manor; exterior) Helpfulโข21 0 \n",
+ "Acton Lane Power Station, Acton Lane, Acton, ...\n",
+ "\n",
+ "4. [Dark Knight Rises Tour: See Batman Movie \n",
+ "Locations](https://www.travelchannel.com/interests/arts-and-culture/photos/see-batman-movie-locations)\n",
+ "Source: Travel Channel\n",
+ "\n",
+ "See Batman Movie Locations ยท Wollaton Hall ยท Carnegie Mellon University ยท The Farmiloe Building ยท Queensboro Bridge\n",
+ "ยท Wall Street ยท Mehrangarh Fort ยท Turda ...\n",
+ "\n",
+ "5. [12 Batman Movie Locations You Can \n",
+ "Visit!](https://www.travelandleisureasia.com/global/destinations/batman-movie-locations-you-can-visit/)\n",
+ "Date published: Jan 10, 2023\n",
+ "Source: Travel and Leisure Asia\n",
+ "\n",
+ "Here are 12 real-world Batman movie locations that you can visit now ยท St. George's Hall, Liverpool ยท Two Temple \n",
+ "Place, London ยท Wollaton Hall, ...\n",
+ "\n",
+ "6. [Batman Movies and Filming Locations in Los \n",
+ "Angeles](https://www.traveltodayla.com/post/batman-movies-in-la?srsltid=AfmBOorSaHBbLAYYKeV8swZKGVHjNXn9tcENa1rjyXx\n",
+ "XMusxnXDgWT34)\n",
+ "Source: LA Today\n",
+ "\n",
+ "Westwood, Los Angeles, CA. Step into the world of Batman and Robin with a visit to the Kirkeby Center, the location\n",
+ "of a thrilling scene from the 1966 film ...\n",
+ "\n",
+ "7. [The Batman | Film Locations](https://movie-locations.com/movies/b/The-Batman-2022.php)\n",
+ "Source: The Worldwide Guide To Movie Locations\n",
+ "\n",
+ "Film locations for The Batman (2022) in Liverpool, London, Glasgow and Chicago.\n",
+ "\n",
+ "8. [The Batman (2022) Locations](https://www.latlong.net/location/the-batman-2022-locations-427)\n",
+ "Source: Latitude and Longitude Finder\n",
+ "\n",
+ "The Batman (2022) was filmed in Liverpool and London, England, UK. Filming also took place at Cardington Studios \n",
+ "and Warner Bros. Leavesden Studios.\n",
+ "\n",
+ "9. [Where was The Dark Knight filmed?](https://giggster.com/guide/movie-location/where-was-the-dark-knight-filmed)\n",
+ "Source: Giggster\n",
+ "\n",
+ "The Dark Knight locations were spread across the world with filming in Chicago in the United States, London in \n",
+ "England, and Hong Kong in China.\n",
+ "\n",
+ "Out: None\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1mExecution logs:\u001b[0m\n",
+ "## Search Results\n",
+ "0. [The Batman (2022) - Filming & production](https://www.imdb.com/title/tt1877830/locations/)\n",
+ "Source: IMDb\n",
+ "\n",
+ "Filming locations: Necropolis Cemetery, Glasgow, Scotland, UK (Batman and Selina leaving the cemetery) Helpfulโข86 1\n",
+ "St. George's Hall, Liverpool, England, UK\n",
+ "\n",
+ "1. [The Batman | Film Locations](https://movie-locations.com/movies/b/The-Batman-2022-2.php)\n",
+ "Source: The Worldwide Guide To Movie Locations\n",
+ "\n",
+ "Film locations for The Batman (2022) in Liverpool, London, Glasgow and Chicago.\n",
+ "\n",
+ "2. [Where was The Batman filmed? ALL the Filming Locations \n",
+ "...](https://www.atlasofwonders.com/2022/04/where-was-the-batman-filmed.html)\n",
+ "Source: Atlas of Wonders\n",
+ "\n",
+ "Guide to the all the filming locations of The Batman - The 2022 movie with Robert Pattison filmed in Chicago and \n",
+ "The UK - The City and the Wayne House.\n",
+ "\n",
+ "3. [Batman (1989) - Filming & production](https://www.imdb.com/title/tt0096895/locations/)\n",
+ "Source: IMDb\n",
+ "\n",
+ "Filming locations: Knebworth House, Knebworth, Hertfordshire, England, UK (Wayne Manor; exterior) Helpfulโข21 0 \n",
+ "Acton Lane Power Station, Acton Lane, Acton, ...\n",
+ "\n",
+ "4. [Dark Knight Rises Tour: See Batman Movie \n",
+ "Locations](https://www.travelchannel.com/interests/arts-and-culture/photos/see-batman-movie-locations)\n",
+ "Source: Travel Channel\n",
+ "\n",
+ "See Batman Movie Locations ยท Wollaton Hall ยท Carnegie Mellon University ยท The Farmiloe Building ยท Queensboro Bridge\n",
+ "ยท Wall Street ยท Mehrangarh Fort ยท Turda ...\n",
+ "\n",
+ "5. [12 Batman Movie Locations You Can \n",
+ "Visit!](https://www.travelandleisureasia.com/global/destinations/batman-movie-locations-you-can-visit/)\n",
+ "Date published: Jan 10, 2023\n",
+ "Source: Travel and Leisure Asia\n",
+ "\n",
+ "Here are 12 real-world Batman movie locations that you can visit now ยท St. George's Hall, Liverpool ยท Two Temple \n",
+ "Place, London ยท Wollaton Hall, ...\n",
+ "\n",
+ "6. [Batman Movies and Filming Locations in Los \n",
+ "Angeles](https://www.traveltodayla.com/post/batman-movies-in-la?srsltid=AfmBOorSaHBbLAYYKeV8swZKGVHjNXn9tcENa1rjyXx\n",
+ "XMusxnXDgWT34)\n",
+ "Source: LA Today\n",
+ "\n",
+ "Westwood, Los Angeles, CA. Step into the world of Batman and Robin with a visit to the Kirkeby Center, the location\n",
+ "of a thrilling scene from the 1966 film ...\n",
+ "\n",
+ "7. [The Batman | Film Locations](https://movie-locations.com/movies/b/The-Batman-2022.php)\n",
+ "Source: The Worldwide Guide To Movie Locations\n",
+ "\n",
+ "Film locations for The Batman (2022) in Liverpool, London, Glasgow and Chicago.\n",
+ "\n",
+ "8. [The Batman (2022) Locations](https://www.latlong.net/location/the-batman-2022-locations-427)\n",
+ "Source: Latitude and Longitude Finder\n",
+ "\n",
+ "The Batman (2022) was filmed in Liverpool and London, England, UK. Filming also took place at Cardington Studios \n",
+ "and Warner Bros. Leavesden Studios.\n",
+ "\n",
+ "9. [Where was The Dark Knight filmed?](https://giggster.com/guide/movie-location/where-was-the-dark-knight-filmed)\n",
+ "Source: Giggster\n",
+ "\n",
+ "The Dark Knight locations were spread across the world with filming in Chicago in the United States, London in \n",
+ "England, and Hong Kong in China.\n",
+ "\n",
+ "Out: None\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "[Step 0: Duration 10.92 seconds| Input tokens: 2,399 | Output tokens: 160]\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[2m[Step 0: Duration 10.92 seconds| Input tokens: 2,399 | Output tokens: 160]\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Step 2 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[0m\u001b[1mStep \u001b[0m\u001b[1;36m2\u001b[0m\u001b[38;2;212;183;2m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ " โ Executing parsed code: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " import re \n",
+ " import pandas as pd \n",
+ " \n",
+ " # Extract locations and their coordinates \n",
+ " locations = [ \n",
+ " (\"Necropolis Cemetery, Glasgow, Scotland, UK\", 55.8642, -4.2894), \n",
+ " (\"St. George's Hall, Liverpool, England, UK\", 53.4093, -2.9915), \n",
+ " (\"Two Temple Place, London, England, UK\", 51.5138, -0.0985), \n",
+ " (\"Wollaton Hall, Nottingham, England, UK\", 52.9522, -1.1497), \n",
+ " (\"Knebworth House, Knebworth, Hertfordshire, England, UK\", 51.8814, -0.1810), \n",
+ " (\"Acton Lane Power Station, Acton Lane, Acton, England, UK\", 51.4934, -0.2208), \n",
+ " (\"Queensboro Bridge, New York City, USA\", 40.7051, -73.9346), \n",
+ " (\"Wall Street, New York City, USA\", 40.7064, -74.0118), \n",
+ " (\"Mehrangarh Fort, Jodhpur, Rajasthan, India\", 26.2913, 73.0243), \n",
+ " (\"Turda Gorge, Turda, Romania\", 46.1942, 24.3484), \n",
+ " (\"Chicago, USA\", 41.8781, -87.6298), \n",
+ " (\"Hong Kong, China\", 22.3964, 114.1095), \n",
+ " (\"Cardington Studios, Northamptonshire, England, UK\", 52.2553, -0.4353), \n",
+ " (\"Warner Bros. Leavesden Studios, Hertfordshire, England, UK\", 51.7483, -0.4141), \n",
+ " (\"Westwood, Los Angeles, CA, USA\", 34.0736, -118.3989) \n",
+ " ] \n",
+ " \n",
+ " # Gotham coordinates \n",
+ " gotham_coords = (40.7128, -74.0060) \n",
+ " \n",
+ " # Calculate travel time for each location \n",
+ " batman_travel_times = [] \n",
+ " for location, lat, lon in locations: \n",
+ " travel_time = calculate_cargo_travel_time(origin_coords=(lat, lon), destination_coords=gotham_coords) \n",
+ " batman_travel_times.append((location, travel_time)) \n",
+ " \n",
+ " # Create DataFrame for Batman filming locations \n",
+ " batman_df = pd.DataFrame(batman_travel_times, columns=['Location', 'Travel Time to Gotham (hours)']) \n",
+ " print(batman_df) \n",
+ " \n",
+ " supercar_factories = web_search(query=\"supercar factories\") \n",
+ " print(supercar_factories) \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ "
\n"
+ ],
+ "text/plain": [
+ " โ \u001b[1mExecuting parsed code:\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " \u001b[38;2;255;70;137;48;2;39;40;34mimport\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mre\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;255;70;137;48;2;39;40;34mimport\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpandas\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mas\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpd\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Extract locations and their coordinates\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mlocations\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mNecropolis Cemetery, Glasgow, Scotland, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m55.8642\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m4.2894\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mSt. George\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34ms Hall, Liverpool, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m53.4093\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m2.9915\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mTwo Temple Place, London, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m51.5138\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.0985\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mWollaton Hall, Nottingham, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m52.9522\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m1.1497\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mKnebworth House, Knebworth, Hertfordshire, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m51.8814\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.1810\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mActon Lane Power Station, Acton Lane, Acton, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m51.4934\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.2208\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mQueensboro Bridge, New York City, USA\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m40.7051\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m73.9346\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mWall Street, New York City, USA\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m40.7064\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m74.0118\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mMehrangarh Fort, Jodhpur, Rajasthan, India\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m26.2913\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m73.0243\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mTurda Gorge, Turda, Romania\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m46.1942\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m24.3484\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mChicago, USA\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m41.8781\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m87.6298\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mHong Kong, China\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m22.3964\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m114.1095\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mCardington Studios, Northamptonshire, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m52.2553\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.4353\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mWarner Bros. Leavesden Studios, Hertfordshire, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m51.7483\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.4141\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mWestwood, Los Angeles, CA, USA\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m34.0736\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m118.3989\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Gotham coordinates\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mgotham_coords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m40.7128\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m74.0060\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Calculate travel time for each location\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mbatman_travel_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;102;217;239;48;2;39;40;34mfor\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlocation\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlat\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlon\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34min\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlocations\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtravel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcalculate_cargo_travel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34morigin_coords\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlat\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlon\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mdestination_coords\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mgotham_coords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_travel_times\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mappend\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlocation\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtravel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Create DataFrame for Batman filming locations\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mbatman_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpd\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mDataFrame\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_travel_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcolumns\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mLocation\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mTravel Time to Gotham (hours)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34msupercar_factories\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mweb_search\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mquery\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34msupercar factories\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_factories\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Execution logs:\n",
+ " Location \\\n",
+ "0 Necropolis Cemetery, Glasgow, Scotland, UK \n",
+ "1 St. George's Hall, Liverpool, England, UK \n",
+ "2 Two Temple Place, London, England, UK \n",
+ "3 Wollaton Hall, Nottingham, England, UK \n",
+ "4 Knebworth House, Knebworth, Hertfordshire, Eng... \n",
+ "5 Acton Lane Power Station, Acton Lane, Acton, E... \n",
+ "6 Queensboro Bridge, New York City, USA \n",
+ "7 Wall Street, New York City, USA \n",
+ "8 Mehrangarh Fort, Jodhpur, Rajasthan, India \n",
+ "9 Turda Gorge, Turda, Romania \n",
+ "10 Chicago, USA \n",
+ "11 Hong Kong, China \n",
+ "12 Cardington Studios, Northamptonshire, England, UK \n",
+ "13 Warner Bros. Leavesden Studios, Hertfordshire,... \n",
+ "14 Westwood, Los Angeles, CA, USA \n",
+ "\n",
+ " Travel Time to Gotham (hours) \n",
+ "0 8.60 \n",
+ "1 8.81 \n",
+ "2 9.17 \n",
+ "3 9.00 \n",
+ "4 9.15 \n",
+ "5 9.16 \n",
+ "6 1.01 \n",
+ "7 1.00 \n",
+ "8 18.34 \n",
+ "9 11.89 \n",
+ "10 2.68 \n",
+ "11 19.99 \n",
+ "12 9.10 \n",
+ "13 9.13 \n",
+ "14 6.79 \n",
+ "## Search Results\n",
+ "0. [Ferrari Lamborghini Pagani Ducati Factory Tours](https://www.italianfactorymotortour.com/)\n",
+ "Source: Italian Factory Motor Tour\n",
+ "\n",
+ "We offer tours to the most famous Italian car production sites, museums and collections in Emilia Romagna. We also \n",
+ "provide test drive with the most sought- ...\n",
+ "\n",
+ "1. [The Best Supercar Manufacturers In The World \n",
+ "(Ranked)](https://www.msn.com/en-us/autos/enthusiasts/the-best-supercar-manufacturers-in-the-world-ranked/ss-AA1vne\n",
+ "Re)\n",
+ "Source: MSN\n",
+ "\n",
+ "These are high-performance automobiles that bring along a thrill while driving. Here are the 18 best manufacturers \n",
+ "in the world, ranked.\n",
+ "\n",
+ "2. [My Motorland: factory tour in the Italian Motor Valley](https://www.mymotorland.net/)\n",
+ "Source: My Motorland\n",
+ "\n",
+ "Factory Tours in the Italian Motor Valley: Lamborghini, Ferrari, Pagani, Maserati and Ducati factory and museum \n",
+ "tours. Supercars test drives and track ...\n",
+ "\n",
+ "3. [List of exclusively sports car \n",
+ "manufacturers](https://en.wikipedia.org/wiki/List_of_exclusively_sports_car_manufacturers)\n",
+ "Source: Wikipedia\n",
+ "\n",
+ "Etox (Turkey) ยท Ferrari (Italy) ยท Fittipaldi Motors LLC (Italy) ยท Foreman (UK) ยท FYK (Norway) ยท Gardner Douglas \n",
+ "(UK) ยท Gemballa (Germany) ยท Gillet (Belgium) ...\n",
+ "\n",
+ "4. [Supercars manufacturing, Italian supercars manufacturing ...](https://www.supercarsmanufacturing.com/)\n",
+ "Source: supercarsmanufacturing.com\n",
+ "\n",
+ "Sly Garage Factory srl is one of the most important Italian manufacturer of top Sport Supercars, high end \n",
+ "automotive industry, mechanical technology for dealers ...\n",
+ "\n",
+ "5. [Tour of Massive Ferrari Factory Building the Best Ferrari ...](https://www.youtube.com/watch?v=IgYxkGs14ek)\n",
+ "Source: YouTube ยท FRAME\n",
+ "\n",
+ "On this feature of the FRAME, we explore the Production line of the most prominent sports car manufacturer Ferrari.\n",
+ "Stay tuned to see the ...\n",
+ "\n",
+ "6. [Super Factories - McLaren Supercar - video Dailymotion](https://www.dailymotion.com/video/x8l3sbu)\n",
+ "Source: Dailymotion ยท MediaEntertainment\n",
+ "\n",
+ "McLaren's high-tech production centre is as remarkably distinctive as the handcrafted automobiles it designs and \n",
+ "produces, overtaking its ...\n",
+ "\n",
+ "7. [Factory Five Racing](https://www.factoryfive.com/)\n",
+ "Source: Factory Five Racing\n",
+ "\n",
+ "Build your own Roadster, Coupe, Hot Rod, Hot Rod Truck, or Extreme Truck with help from the crew at Factory Five \n",
+ "Racingยฎ.\n",
+ "\n",
+ "Out: None\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1mExecution logs:\u001b[0m\n",
+ " Location \\\n",
+ "0 Necropolis Cemetery, Glasgow, Scotland, UK \n",
+ "1 St. George's Hall, Liverpool, England, UK \n",
+ "2 Two Temple Place, London, England, UK \n",
+ "3 Wollaton Hall, Nottingham, England, UK \n",
+ "4 Knebworth House, Knebworth, Hertfordshire, Eng... \n",
+ "5 Acton Lane Power Station, Acton Lane, Acton, E... \n",
+ "6 Queensboro Bridge, New York City, USA \n",
+ "7 Wall Street, New York City, USA \n",
+ "8 Mehrangarh Fort, Jodhpur, Rajasthan, India \n",
+ "9 Turda Gorge, Turda, Romania \n",
+ "10 Chicago, USA \n",
+ "11 Hong Kong, China \n",
+ "12 Cardington Studios, Northamptonshire, England, UK \n",
+ "13 Warner Bros. Leavesden Studios, Hertfordshire,... \n",
+ "14 Westwood, Los Angeles, CA, USA \n",
+ "\n",
+ " Travel Time to Gotham (hours) \n",
+ "0 8.60 \n",
+ "1 8.81 \n",
+ "2 9.17 \n",
+ "3 9.00 \n",
+ "4 9.15 \n",
+ "5 9.16 \n",
+ "6 1.01 \n",
+ "7 1.00 \n",
+ "8 18.34 \n",
+ "9 11.89 \n",
+ "10 2.68 \n",
+ "11 19.99 \n",
+ "12 9.10 \n",
+ "13 9.13 \n",
+ "14 6.79 \n",
+ "## Search Results\n",
+ "0. [Ferrari Lamborghini Pagani Ducati Factory Tours](https://www.italianfactorymotortour.com/)\n",
+ "Source: Italian Factory Motor Tour\n",
+ "\n",
+ "We offer tours to the most famous Italian car production sites, museums and collections in Emilia Romagna. We also \n",
+ "provide test drive with the most sought- ...\n",
+ "\n",
+ "1. [The Best Supercar Manufacturers In The World \n",
+ "(Ranked)](https://www.msn.com/en-us/autos/enthusiasts/the-best-supercar-manufacturers-in-the-world-ranked/ss-AA1vne\n",
+ "Re)\n",
+ "Source: MSN\n",
+ "\n",
+ "These are high-performance automobiles that bring along a thrill while driving. Here are the 18 best manufacturers \n",
+ "in the world, ranked.\n",
+ "\n",
+ "2. [My Motorland: factory tour in the Italian Motor Valley](https://www.mymotorland.net/)\n",
+ "Source: My Motorland\n",
+ "\n",
+ "Factory Tours in the Italian Motor Valley: Lamborghini, Ferrari, Pagani, Maserati and Ducati factory and museum \n",
+ "tours. Supercars test drives and track ...\n",
+ "\n",
+ "3. [List of exclusively sports car \n",
+ "manufacturers](https://en.wikipedia.org/wiki/List_of_exclusively_sports_car_manufacturers)\n",
+ "Source: Wikipedia\n",
+ "\n",
+ "Etox (Turkey) ยท Ferrari (Italy) ยท Fittipaldi Motors LLC (Italy) ยท Foreman (UK) ยท FYK (Norway) ยท Gardner Douglas \n",
+ "(UK) ยท Gemballa (Germany) ยท Gillet (Belgium) ...\n",
+ "\n",
+ "4. [Supercars manufacturing, Italian supercars manufacturing ...](https://www.supercarsmanufacturing.com/)\n",
+ "Source: supercarsmanufacturing.com\n",
+ "\n",
+ "Sly Garage Factory srl is one of the most important Italian manufacturer of top Sport Supercars, high end \n",
+ "automotive industry, mechanical technology for dealers ...\n",
+ "\n",
+ "5. [Tour of Massive Ferrari Factory Building the Best Ferrari ...](https://www.youtube.com/watch?v=IgYxkGs14ek)\n",
+ "Source: YouTube ยท FRAME\n",
+ "\n",
+ "On this feature of the FRAME, we explore the Production line of the most prominent sports car manufacturer Ferrari.\n",
+ "Stay tuned to see the ...\n",
+ "\n",
+ "6. [Super Factories - McLaren Supercar - video Dailymotion](https://www.dailymotion.com/video/x8l3sbu)\n",
+ "Source: Dailymotion ยท MediaEntertainment\n",
+ "\n",
+ "McLaren's high-tech production centre is as remarkably distinctive as the handcrafted automobiles it designs and \n",
+ "produces, overtaking its ...\n",
+ "\n",
+ "7. [Factory Five Racing](https://www.factoryfive.com/)\n",
+ "Source: Factory Five Racing\n",
+ "\n",
+ "Build your own Roadster, Coupe, Hot Rod, Hot Rod Truck, or Extreme Truck with help from the crew at Factory Five \n",
+ "Racingยฎ.\n",
+ "\n",
+ "Out: None\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "[Step 1: Duration 15.03 seconds| Input tokens: 5,858 | Output tokens: 1,279]\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[2m[Step 1: Duration 15.03 seconds| Input tokens: 5,858 | Output tokens: 1,279]\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Step 3 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[0m\u001b[1mStep \u001b[0m\u001b[1;36m3\u001b[0m\u001b[38;2;212;183;2m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ " โ Executing parsed code: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " # Extract locations and their coordinates for supercar factories \n",
+ " supercar_locations = [ \n",
+ " (\"Maranello, Italy (Ferrari)\", 44.5403, 10.9921), \n",
+ " (\"Sant'Agata Bolognese, Italy (Lamborghini)\", 44.5208, 11.3264), \n",
+ " (\"San Cesario sul Panaro, Italy (Pagani)\", 44.6423, 10.9917), \n",
+ " (\"Bologna, Italy (Ducati)\", 44.4937, 11.3426), \n",
+ " (\"Woking, UK (McLaren)\", 51.3774, -0.5714), \n",
+ " (\"San Diego, CA, USA (Koenigsegg)\", 32.7157, -117.1611), \n",
+ " (\"Amersfoort, Netherlands (Aston Martin)\", 52.0118, 5.5975), \n",
+ " (\"Los Angeles, CA, USA (Hennessey Performance Engineering)\", 34.0522, -118.2437), \n",
+ " (\"Shanghai, China (Shanghai Auto Show)\", 31.2304, 121.4737), \n",
+ " (\"Dubai, UAE (Dubai Autodrome)\", 25.2769, 55.2962) \n",
+ " ] \n",
+ " \n",
+ " # Calculate travel time for each supercar factory \n",
+ " supercar_travel_times = [] \n",
+ " for location, lat, lon in supercar_locations: \n",
+ " travel_time = calculate_cargo_travel_time(origin_coords=(lat, lon), destination_coords=gotham_coords) \n",
+ " supercar_travel_times.append((location, travel_time)) \n",
+ " \n",
+ " # Create DataFrame for supercar factories \n",
+ " supercar_df = pd.DataFrame(supercar_travel_times, columns=['Location', 'Travel Time to Gotham (hours)']) \n",
+ " \n",
+ " # Filter supercar factories to match the travel times of Batman filming locations \n",
+ " batman_travel_times_set = set(batman_df['Travel Time to Gotham (hours)']) \n",
+ " filtered_supercar_df = supercar_df[supercar_df['Travel Time to Gotham (hours)'].isin(batman_travel_times_set)] \n",
+ " \n",
+ " # Combine Batman filming locations and filtered supercar factories into a single DataFrame \n",
+ " combined_df = pd.concat([batman_df, filtered_supercar_df], ignore_index=True) \n",
+ " \n",
+ " print(combined_df) \n",
+ " \n",
+ " final_answer(combined_df) \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ "
\n"
+ ],
+ "text/plain": [
+ " โ \u001b[1mExecuting parsed code:\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Extract locations and their coordinates for supercar factories\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34msupercar_locations\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mMaranello, Italy (Ferrari)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m44.5403\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m10.9921\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mSant\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mAgata Bolognese, Italy (Lamborghini)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m44.5208\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m11.3264\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mSan Cesario sul Panaro, Italy (Pagani)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m44.6423\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m10.9917\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBologna, Italy (Ducati)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m44.4937\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m11.3426\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mWoking, UK (McLaren)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m51.3774\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.5714\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mSan Diego, CA, USA (Koenigsegg)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m32.7157\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m117.1611\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mAmersfoort, Netherlands (Aston Martin)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m52.0118\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m5.5975\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mLos Angeles, CA, USA (Hennessey Performance Engineering)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m34.0522\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m118.2437\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mShanghai, China (Shanghai Auto Show)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m31.2304\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m121.4737\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mDubai, UAE (Dubai Autodrome)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m25.2769\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m55.2962\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Calculate travel time for each supercar factory\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34msupercar_travel_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;102;217;239;48;2;39;40;34mfor\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlocation\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlat\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlon\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34min\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_locations\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtravel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcalculate_cargo_travel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34morigin_coords\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlat\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlon\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mdestination_coords\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mgotham_coords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_travel_times\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mappend\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlocation\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtravel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Create DataFrame for supercar factories\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34msupercar_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpd\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mDataFrame\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_travel_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcolumns\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mLocation\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mTravel Time to Gotham (hours)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Filter supercar factories to match the travel times of Batman filming locations\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mbatman_travel_times_set\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mset\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mTravel Time to Gotham (hours)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mfiltered_supercar_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mTravel Time to Gotham (hours)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34misin\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_travel_times_set\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Combine Batman filming locations and filtered supercar factories into a single DataFrame\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mcombined_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpd\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mconcat\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfiltered_supercar_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mignore_index\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mTrue\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcombined_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mfinal_answer\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcombined_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Execution logs:\n",
+ " Location \\\n",
+ "0 Necropolis Cemetery, Glasgow, Scotland, UK \n",
+ "1 St. George's Hall, Liverpool, England, UK \n",
+ "2 Two Temple Place, London, England, UK \n",
+ "3 Wollaton Hall, Nottingham, England, UK \n",
+ "4 Knebworth House, Knebworth, Hertfordshire, Eng... \n",
+ "5 Acton Lane Power Station, Acton Lane, Acton, E... \n",
+ "6 Queensboro Bridge, New York City, USA \n",
+ "7 Wall Street, New York City, USA \n",
+ "8 Mehrangarh Fort, Jodhpur, Rajasthan, India \n",
+ "9 Turda Gorge, Turda, Romania \n",
+ "10 Chicago, USA \n",
+ "11 Hong Kong, China \n",
+ "12 Cardington Studios, Northamptonshire, England, UK \n",
+ "13 Warner Bros. Leavesden Studios, Hertfordshire,... \n",
+ "14 Westwood, Los Angeles, CA, USA \n",
+ "15 Woking, UK (McLaren) \n",
+ "\n",
+ " Travel Time to Gotham (hours) \n",
+ "0 8.60 \n",
+ "1 8.81 \n",
+ "2 9.17 \n",
+ "3 9.00 \n",
+ "4 9.15 \n",
+ "5 9.16 \n",
+ "6 1.01 \n",
+ "7 1.00 \n",
+ "8 18.34 \n",
+ "9 11.89 \n",
+ "10 2.68 \n",
+ "11 19.99 \n",
+ "12 9.10 \n",
+ "13 9.13 \n",
+ "14 6.79 \n",
+ "15 9.13 \n",
+ "\n",
+ "Out - Final answer: Location \\\n",
+ "0 Necropolis Cemetery, Glasgow, Scotland, UK \n",
+ "1 St. George's Hall, Liverpool, England, UK \n",
+ "2 Two Temple Place, London, England, UK \n",
+ "3 Wollaton Hall, Nottingham, England, UK \n",
+ "4 Knebworth House, Knebworth, Hertfordshire, Eng... \n",
+ "5 Acton Lane Power Station, Acton Lane, Acton, E... \n",
+ "6 Queensboro Bridge, New York City, USA \n",
+ "7 Wall Street, New York City, USA \n",
+ "8 Mehrangarh Fort, Jodhpur, Rajasthan, India \n",
+ "9 Turda Gorge, Turda, Romania \n",
+ "10 Chicago, USA \n",
+ "11 Hong Kong, China \n",
+ "12 Cardington Studios, Northamptonshire, England, UK \n",
+ "13 Warner Bros. Leavesden Studios, Hertfordshire,... \n",
+ "14 Westwood, Los Angeles, CA, USA \n",
+ "15 Woking, UK (McLaren) \n",
+ "\n",
+ " Travel Time to Gotham (hours) \n",
+ "0 8.60 \n",
+ "1 8.81 \n",
+ "2 9.17 \n",
+ "3 9.00 \n",
+ "4 9.15 \n",
+ "5 9.16 \n",
+ "6 1.01 \n",
+ "7 1.00 \n",
+ "8 18.34 \n",
+ "9 11.89 \n",
+ "10 2.68 \n",
+ "11 19.99 \n",
+ "12 9.10 \n",
+ "13 9.13 \n",
+ "14 6.79 \n",
+ "15 9.13 \n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1mExecution logs:\u001b[0m\n",
+ " Location \\\n",
+ "0 Necropolis Cemetery, Glasgow, Scotland, UK \n",
+ "1 St. George's Hall, Liverpool, England, UK \n",
+ "2 Two Temple Place, London, England, UK \n",
+ "3 Wollaton Hall, Nottingham, England, UK \n",
+ "4 Knebworth House, Knebworth, Hertfordshire, Eng... \n",
+ "5 Acton Lane Power Station, Acton Lane, Acton, E... \n",
+ "6 Queensboro Bridge, New York City, USA \n",
+ "7 Wall Street, New York City, USA \n",
+ "8 Mehrangarh Fort, Jodhpur, Rajasthan, India \n",
+ "9 Turda Gorge, Turda, Romania \n",
+ "10 Chicago, USA \n",
+ "11 Hong Kong, China \n",
+ "12 Cardington Studios, Northamptonshire, England, UK \n",
+ "13 Warner Bros. Leavesden Studios, Hertfordshire,... \n",
+ "14 Westwood, Los Angeles, CA, USA \n",
+ "15 Woking, UK (McLaren) \n",
+ "\n",
+ " Travel Time to Gotham (hours) \n",
+ "0 8.60 \n",
+ "1 8.81 \n",
+ "2 9.17 \n",
+ "3 9.00 \n",
+ "4 9.15 \n",
+ "5 9.16 \n",
+ "6 1.01 \n",
+ "7 1.00 \n",
+ "8 18.34 \n",
+ "9 11.89 \n",
+ "10 2.68 \n",
+ "11 19.99 \n",
+ "12 9.10 \n",
+ "13 9.13 \n",
+ "14 6.79 \n",
+ "15 9.13 \n",
+ "\n",
+ "\u001b[1;38;2;212;183;2mOut - Final answer: Location \\\u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m0 Necropolis Cemetery, Glasgow, Scotland, UK \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m1 St. George's Hall, Liverpool, England, UK \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m2 Two Temple Place, London, England, UK \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m3 Wollaton Hall, Nottingham, England, UK \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m4 Knebworth House, Knebworth, Hertfordshire, Eng... \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m5 Acton Lane Power Station, Acton Lane, Acton, E... \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m6 Queensboro Bridge, New York City, USA \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m7 Wall Street, New York City, USA \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m8 Mehrangarh Fort, Jodhpur, Rajasthan, India \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m9 Turda Gorge, Turda, Romania \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m10 Chicago, USA \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m11 Hong Kong, China \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m12 Cardington Studios, Northamptonshire, England, UK \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m13 Warner Bros. Leavesden Studios, Hertfordshire,... \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m14 Westwood, Los Angeles, CA, USA \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m15 Woking, UK (McLaren) \u001b[0m\n",
+ "\n",
+ "\u001b[1;38;2;212;183;2m Travel Time to Gotham (hours) \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m0 8.60 \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m1 8.81 \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m2 9.17 \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m3 9.00 \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m4 9.15 \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m5 9.16 \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m6 1.01 \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m7 1.00 \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m8 18.34 \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m9 11.89 \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m10 2.68 \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m11 19.99 \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m12 9.10 \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m13 9.13 \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m14 6.79 \u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m15 9.13 \u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "[Step 2: Duration 14.67 seconds| Input tokens: 12,077 | Output tokens: 2,438]\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[2m[Step 2: Duration 14.67 seconds| Input tokens: 12,077 | Output tokens: 2,438]\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ }
+ ],
+ "source": [
+ "result = agent.run(task)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 15,
+ "metadata": {
+ "colab": {
+ "base_uri": "https://localhost:8080/",
+ "height": 551
+ },
+ "id": "NlgnI2GnavwT",
+ "outputId": "2bb30112-f069-4bfe-9155-bcf9216b04a8"
+ },
+ "outputs": [
+ {
+ "data": {
+ "application/vnd.google.colaboratory.intrinsic+json": {
+ "summary": "{\n \"name\": \"result\",\n \"rows\": 16,\n \"fields\": [\n {\n \"column\": \"Location\",\n \"properties\": {\n \"dtype\": \"string\",\n \"num_unique_values\": 16,\n \"samples\": [\n \"Necropolis Cemetery, Glasgow, Scotland, UK\",\n \"St. George's Hall, Liverpool, England, UK\",\n \"Acton Lane Power Station, Acton Lane, Acton, England, UK\"\n ],\n \"semantic_type\": \"\",\n \"description\": \"\"\n }\n },\n {\n \"column\": \"Travel Time to Gotham (hours)\",\n \"properties\": {\n \"dtype\": \"number\",\n \"std\": 5.094326214852494,\n \"min\": 1.0,\n \"max\": 19.99,\n \"num_unique_values\": 15,\n \"samples\": [\n 11.89,\n 19.99,\n 8.6\n ],\n \"semantic_type\": \"\",\n \"description\": \"\"\n }\n }\n ]\n}",
+ "type": "dataframe",
+ "variable_name": "result"
+ },
+ "text/html": [
+ "\n",
+ " \n",
+ "
\n",
+ "\n",
+ "
\n",
+ " \n",
+ " \n",
+ " | \n",
+ " Location | \n",
+ " Travel Time to Gotham (hours) | \n",
+ "
\n",
+ " \n",
+ " \n",
+ " \n",
+ " 0 | \n",
+ " Necropolis Cemetery, Glasgow, Scotland, UK | \n",
+ " 8.60 | \n",
+ "
\n",
+ " \n",
+ " 1 | \n",
+ " St. George's Hall, Liverpool, England, UK | \n",
+ " 8.81 | \n",
+ "
\n",
+ " \n",
+ " 2 | \n",
+ " Two Temple Place, London, England, UK | \n",
+ " 9.17 | \n",
+ "
\n",
+ " \n",
+ " 3 | \n",
+ " Wollaton Hall, Nottingham, England, UK | \n",
+ " 9.00 | \n",
+ "
\n",
+ " \n",
+ " 4 | \n",
+ " Knebworth House, Knebworth, Hertfordshire, Eng... | \n",
+ " 9.15 | \n",
+ "
\n",
+ " \n",
+ " 5 | \n",
+ " Acton Lane Power Station, Acton Lane, Acton, E... | \n",
+ " 9.16 | \n",
+ "
\n",
+ " \n",
+ " 6 | \n",
+ " Queensboro Bridge, New York City, USA | \n",
+ " 1.01 | \n",
+ "
\n",
+ " \n",
+ " 7 | \n",
+ " Wall Street, New York City, USA | \n",
+ " 1.00 | \n",
+ "
\n",
+ " \n",
+ " 8 | \n",
+ " Mehrangarh Fort, Jodhpur, Rajasthan, India | \n",
+ " 18.34 | \n",
+ "
\n",
+ " \n",
+ " 9 | \n",
+ " Turda Gorge, Turda, Romania | \n",
+ " 11.89 | \n",
+ "
\n",
+ " \n",
+ " 10 | \n",
+ " Chicago, USA | \n",
+ " 2.68 | \n",
+ "
\n",
+ " \n",
+ " 11 | \n",
+ " Hong Kong, China | \n",
+ " 19.99 | \n",
+ "
\n",
+ " \n",
+ " 12 | \n",
+ " Cardington Studios, Northamptonshire, England, UK | \n",
+ " 9.10 | \n",
+ "
\n",
+ " \n",
+ " 13 | \n",
+ " Warner Bros. Leavesden Studios, Hertfordshire,... | \n",
+ " 9.13 | \n",
+ "
\n",
+ " \n",
+ " 14 | \n",
+ " Westwood, Los Angeles, CA, USA | \n",
+ " 6.79 | \n",
+ "
\n",
+ " \n",
+ " 15 | \n",
+ " Woking, UK (McLaren) | \n",
+ " 9.13 | \n",
+ "
\n",
+ " \n",
+ "
\n",
+ "
\n",
+ "
\n",
+ "
\n"
+ ],
+ "text/plain": [
+ " Location \\\n",
+ "0 Necropolis Cemetery, Glasgow, Scotland, UK \n",
+ "1 St. George's Hall, Liverpool, England, UK \n",
+ "2 Two Temple Place, London, England, UK \n",
+ "3 Wollaton Hall, Nottingham, England, UK \n",
+ "4 Knebworth House, Knebworth, Hertfordshire, Eng... \n",
+ "5 Acton Lane Power Station, Acton Lane, Acton, E... \n",
+ "6 Queensboro Bridge, New York City, USA \n",
+ "7 Wall Street, New York City, USA \n",
+ "8 Mehrangarh Fort, Jodhpur, Rajasthan, India \n",
+ "9 Turda Gorge, Turda, Romania \n",
+ "10 Chicago, USA \n",
+ "11 Hong Kong, China \n",
+ "12 Cardington Studios, Northamptonshire, England, UK \n",
+ "13 Warner Bros. Leavesden Studios, Hertfordshire,... \n",
+ "14 Westwood, Los Angeles, CA, USA \n",
+ "15 Woking, UK (McLaren) \n",
+ "\n",
+ " Travel Time to Gotham (hours) \n",
+ "0 8.60 \n",
+ "1 8.81 \n",
+ "2 9.17 \n",
+ "3 9.00 \n",
+ "4 9.15 \n",
+ "5 9.16 \n",
+ "6 1.01 \n",
+ "7 1.00 \n",
+ "8 18.34 \n",
+ "9 11.89 \n",
+ "10 2.68 \n",
+ "11 19.99 \n",
+ "12 9.10 \n",
+ "13 9.13 \n",
+ "14 6.79 \n",
+ "15 9.13 "
+ ]
+ },
+ "execution_count": 15,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "result"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "17_UNQk2avwU"
+ },
+ "source": [
+ "We could already improve this a bit by throwing in some dedicated planning steps, and adding more prompting."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 16,
+ "metadata": {
+ "colab": {
+ "base_uri": "https://localhost:8080/",
+ "height": 1000
+ },
+ "id": "lzjuyPydavwU",
+ "outputId": "55f17151-ffcd-46e0-e04d-39c59065c454"
+ },
+ "outputs": [
+ {
+ "data": {
+ "text/html": [
+ "โญโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ New run โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฎ\n",
+ "โ โ\n",
+ "โ You're an expert analyst. You make comprehensive reports after visiting many websites. โ\n",
+ "โ Don't hesitate to search for many queries at once in a for loop. โ\n",
+ "โ For each data point that you find, visit the source url to confirm numbers. โ\n",
+ "โ โ\n",
+ "โ Find all Batman filming locations in the world, calculate the time to transfer via cargo plane to here (we're โ\n",
+ "โ in Gotham, 40.7128ยฐ N, 74.0060ยฐ W), and return them to me as a pandas dataframe. โ\n",
+ "โ Also give me some supercar factories with the same cargo plane transfer time. โ\n",
+ "โ โ\n",
+ "โฐโ HfApiModel - Qwen/Qwen2.5-Coder-32B-Instruct โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฏ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโญโ\u001b[0m\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\u001b[38;2;212;183;2m \u001b[0m\u001b[1;38;2;212;183;2mNew run\u001b[0m\u001b[38;2;212;183;2m \u001b[0m\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\u001b[38;2;212;183;2mโโฎ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mYou're an expert analyst. You make comprehensive reports after visiting many websites.\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mDon't hesitate to search for many queries at once in a for loop.\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mFor each data point that you find, visit the source url to confirm numbers.\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mFind all Batman filming locations in the world, calculate the time to transfer via cargo plane to here (we're \u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1min Gotham, 40.7128ยฐ N, 74.0060ยฐ W), and return them to me as a pandas dataframe.\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mAlso give me some supercar factories with the same cargo plane transfer time.\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโฐโ\u001b[0m\u001b[38;2;212;183;2m HfApiModel - Qwen/Qwen2.5-Coder-32B-Instruct \u001b[0m\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\u001b[38;2;212;183;2mโโฏ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Initial plan โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "Here is the plan of action that I will follow to solve the task:\n",
+ " ```\n",
+ " 1. Perform a web search to find a comprehensive list of Batman filming locations worldwide.\n",
+ "2. For each filming location, visit the source URL to confirm the coordinates.\n",
+ "3. Calculate the cargo plane transfer time from each filming location to Gotham.\n",
+ "4. Perform a web search to find a comprehensive list of supercar factories worldwide.\n",
+ "5. For each supercar factory, visit the source URL to confirm the coordinates.\n",
+ "6. Calculate the cargo plane transfer time from each supercar factory to Gotham.\n",
+ "7. Filter both lists to include only locations with the same cargo plane transfer time.\n",
+ "8. Organize the filtered locations and their details into a pandas dataframe.\n",
+ "9. Provide the final answer as the pandas dataframe.\n",
+ "\n",
+ "\n",
+ " ```\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[1mInitial plan\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "Here is the plan of action that I will follow to solve the task:\n",
+ " ```\n",
+ " 1. Perform a web search to find a comprehensive list of Batman filming locations worldwide.\n",
+ "2. For each filming location, visit the source URL to confirm the coordinates.\n",
+ "3. Calculate the cargo plane transfer time from each filming location to Gotham.\n",
+ "4. Perform a web search to find a comprehensive list of supercar factories worldwide.\n",
+ "5. For each supercar factory, visit the source URL to confirm the coordinates.\n",
+ "6. Calculate the cargo plane transfer time from each supercar factory to Gotham.\n",
+ "7. Filter both lists to include only locations with the same cargo plane transfer time.\n",
+ "8. Organize the filtered locations and their details into a pandas dataframe.\n",
+ "9. Provide the final answer as the pandas dataframe.\n",
+ "\n",
+ "\n",
+ " ```\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Step 1 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[0m\u001b[1mStep \u001b[0m\u001b[1;36m1\u001b[0m\u001b[38;2;212;183;2m โโโโโโโโโโโโโ๏ฟฝ๏ฟฝ๏ฟฝโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ " โ Executing parsed code: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " Thought: I will perform a web search to find a comprehensive list of Batman filming locations worldwide. \n",
+ " Code: \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ "
\n"
+ ],
+ "text/plain": [
+ " โ \u001b[1mExecuting parsed code:\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mThought\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mI\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mwill\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mperform\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34ma\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mweb\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msearch\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mto\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfind\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34ma\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcomprehensive\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlist\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mof\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mBatman\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfilming\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlocations\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mworldwide\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mCode\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Execution logs:\n",
+ " Location \\\n",
+ "0 Necropolis Cemetery, Glasgow, Scotland, UK \n",
+ "1 St. George's Hall, Liverpool, England, UK \n",
+ "2 Two Temple Place, London, England, UK \n",
+ "3 Wollaton Hall, Nottingham, England, UK \n",
+ "4 Knebworth House, Knebworth, Hertfordshire, Eng... \n",
+ "5 Acton Lane Power Station, Acton Lane, Acton, E... \n",
+ "6 Queensboro Bridge, New York City, USA \n",
+ "7 Wall Street, New York City, USA \n",
+ "8 Mehrangarh Fort, Jodhpur, Rajasthan, India \n",
+ "9 Turda Gorge, Turda, Romania \n",
+ "10 Chicago, USA \n",
+ "11 Hong Kong, China \n",
+ "12 Cardington Studios, Northamptonshire, England, UK \n",
+ "13 Warner Bros. Leavesden Studios, Hertfordshire,... \n",
+ "14 Westwood, Los Angeles, CA, USA \n",
+ "15 Woking, UK (McLaren) \n",
+ "\n",
+ " Travel Time to Gotham (hours) \n",
+ "0 8.60 \n",
+ "1 8.81 \n",
+ "2 9.17 \n",
+ "3 9.00 \n",
+ "4 9.15 \n",
+ "5 9.16 \n",
+ "6 1.01 \n",
+ "7 1.00 \n",
+ "8 18.34 \n",
+ "9 11.89 \n",
+ "10 2.68 \n",
+ "11 19.99 \n",
+ "12 9.10 \n",
+ "13 9.13 \n",
+ "14 6.79 \n",
+ "15 9.13 \n",
+ "\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1mExecution logs:\u001b[0m\n",
+ " Location \\\n",
+ "0 Necropolis Cemetery, Glasgow, Scotland, UK \n",
+ "1 St. George's Hall, Liverpool, England, UK \n",
+ "2 Two Temple Place, London, England, UK \n",
+ "3 Wollaton Hall, Nottingham, England, UK \n",
+ "4 Knebworth House, Knebworth, Hertfordshire, Eng... \n",
+ "5 Acton Lane Power Station, Acton Lane, Acton, E... \n",
+ "6 Queensboro Bridge, New York City, USA \n",
+ "7 Wall Street, New York City, USA \n",
+ "8 Mehrangarh Fort, Jodhpur, Rajasthan, India \n",
+ "9 Turda Gorge, Turda, Romania \n",
+ "10 Chicago, USA \n",
+ "11 Hong Kong, China \n",
+ "12 Cardington Studios, Northamptonshire, England, UK \n",
+ "13 Warner Bros. Leavesden Studios, Hertfordshire,... \n",
+ "14 Westwood, Los Angeles, CA, USA \n",
+ "15 Woking, UK (McLaren) \n",
+ "\n",
+ " Travel Time to Gotham (hours) \n",
+ "0 8.60 \n",
+ "1 8.81 \n",
+ "2 9.17 \n",
+ "3 9.00 \n",
+ "4 9.15 \n",
+ "5 9.16 \n",
+ "6 1.01 \n",
+ "7 1.00 \n",
+ "8 18.34 \n",
+ "9 11.89 \n",
+ "10 2.68 \n",
+ "11 19.99 \n",
+ "12 9.10 \n",
+ "13 9.13 \n",
+ "14 6.79 \n",
+ "15 9.13 \n",
+ "\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Code parsing failed on line 1 due to: SyntaxError\n",
+ "Thought: I will perform a web search to find a comprehensive list of Batman filming locations worldwide.\n",
+ " ^\n",
+ "Error: invalid syntax (<unknown>, line 1)\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1;31mCode parsing failed on line \u001b[0m\u001b[1;31m1\u001b[0m\u001b[1;31m due to: SyntaxError\u001b[0m\n",
+ "\u001b[1;31mThought: I will perform a web search to find a comprehensive list of Batman filming locations worldwide.\u001b[0m\n",
+ "\u001b[1;31m ^\u001b[0m\n",
+ "\u001b[1;31mError: invalid syntax \u001b[0m\u001b[1;31m(\u001b[0m\u001b[1;31m<\u001b[0m\u001b[1;31munknown\u001b[0m\u001b[1;31m>\u001b[0m\u001b[1;31m, line \u001b[0m\u001b[1;31m1\u001b[0m\u001b[1;31m)\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "[Step 0: Duration 9.18 seconds| Input tokens: 2,962 | Output tokens: 78]\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[2m[Step 0: Duration 9.18 seconds| Input tokens: 2,962 | Output tokens: 78]\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Step 2 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[0m\u001b[1mStep \u001b[0m\u001b[1;36m2\u001b[0m\u001b[38;2;212;183;2m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ " โ Executing parsed code: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " batman_filming_locations = web_search(query=\"Batman filming locations worldwide\") \n",
+ " print(batman_filming_locations) \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ "
\n"
+ ],
+ "text/plain": [
+ " โ \u001b[1mExecuting parsed code:\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mbatman_filming_locations\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mweb_search\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mquery\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBatman filming locations worldwide\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_filming_locations\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Execution logs:\n",
+ "## Search Results\n",
+ "0. [12 Batman Movie Locations You Can \n",
+ "Visit!](https://www.travelandleisureasia.com/global/destinations/batman-movie-locations-you-can-visit/)\n",
+ "Date published: Jan 10, 2023\n",
+ "Source: Travel and Leisure Asia\n",
+ "\n",
+ "Most of the filming of Batman movies is done in the Warner Bros studios and across the US, including New York and \n",
+ "Pittsburgh. You will notice ...\n",
+ "\n",
+ "1. [The Batman (2022) - Filming & production](https://www.imdb.com/title/tt1877830/locations/)\n",
+ "Source: IMDb\n",
+ "\n",
+ "Filming locations: Necropolis Cemetery, Glasgow, Scotland, UK (Batman and Selina leaving the cemetery) Helpfulโข86 1\n",
+ "St. George's Hall, Liverpool, England, UK\n",
+ "\n",
+ "2. [The Batman | Film Locations](https://movie-locations.com/movies/b/The-Batman-2022-2.php)\n",
+ "Source: The Worldwide Guide To Movie Locations\n",
+ "\n",
+ "Film locations for The Batman (2022) in Liverpool, London, Glasgow and Chicago.\n",
+ "\n",
+ "3. [Category:Film Locations - Batman Wiki - Fandom](https://batman.fandom.com/wiki/Category:Film_Locations)\n",
+ "Source: Batman Wiki\n",
+ "\n",
+ "Selina Kyle's apartment (Burtonverse) ยท Sheal Maritime Building ยท Shreck's ยท Snowy Cones Ice Cream Factory ยท South \n",
+ "Channel Island ยท South Hinkley ...\n",
+ "\n",
+ "4. [Dark Knight Rises Tour: See Batman Movie \n",
+ "Locations](https://www.travelchannel.com/interests/arts-and-culture/photos/see-batman-movie-locations)\n",
+ "Source: Travel Channel\n",
+ "\n",
+ "See Batman Movie Locations ยท Wollaton Hall ยท Carnegie Mellon University ยท The Farmiloe Building ยท Queensboro Bridge\n",
+ "ยท Wall Street ยท Mehrangarh Fort ยท Turda Saline.\n",
+ "\n",
+ "5. [What cities in America and anywhere in the world do you \n",
+ "...](https://www.reddit.com/r/batman/comments/1d1t88q/what_cities_in_america_and_anywhere_in_the_world/)\n",
+ "Source: Reddit ยท r/batman\n",
+ "\n",
+ "Glasgow and Liverpool were used in the shoot for The Batman. I'd avoid Chicago, as that looks more like Metropolis \n",
+ "than Gotham.\n",
+ "\n",
+ "6. [Where was The Batman filmed? ALL the Filming Locations \n",
+ "...](https://www.atlasofwonders.com/2022/04/where-was-the-batman-filmed.html)\n",
+ "Source: Atlas of Wonders\n",
+ "\n",
+ "The Batman was primarily filmed in the United Kingdom. Most of the recognizable buildings of this new version of \n",
+ "Gotham City are located in Liverpool.\n",
+ "\n",
+ "7. [The Dark Knight | 2008](https://movie-locations.com/movies/d/Dark-Knight.php)\n",
+ "Source: The Worldwide Guide To Movie Locations\n",
+ "\n",
+ "Discover where The Dark Knight (2008) was filmed around Chicago, as well as in London and Bedfordshire in the UK, \n",
+ "and briefly in Hong Kong.\n",
+ "\n",
+ "8. [Batman Movies and Filming Locations in Los \n",
+ "Angeles](https://www.traveltodayla.com/post/batman-movies-in-la?srsltid=AfmBOoqNOBBzvgmhmQdx1CjiZ98A8awKaUUsgA1_91F\n",
+ "_VDdwIpLDKwNX)\n",
+ "Source: LA Today\n",
+ "\n",
+ "Find Batman movies and filming locations in LA. Los Angeles is home to many locations where Batman movie scenes \n",
+ "were filmed. From the Batcave to Wayne Manor ...\n",
+ "\n",
+ "9. [Where The Batman Filmed (All Gotham Real-Life \n",
+ "Locations)](https://screenrant.com/where-was-the-batman-movie-filmed/)\n",
+ "Date published: Mar 4, 2022\n",
+ "Source: Screen Rant\n",
+ "\n",
+ "The Batman filmed all over the world to bring a new version of Gotham to life and went to some prominent real-life \n",
+ "locations to achieve the right look.\n",
+ "\n",
+ "Out: None\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1mExecution logs:\u001b[0m\n",
+ "## Search Results\n",
+ "0. [12 Batman Movie Locations You Can \n",
+ "Visit!](https://www.travelandleisureasia.com/global/destinations/batman-movie-locations-you-can-visit/)\n",
+ "Date published: Jan 10, 2023\n",
+ "Source: Travel and Leisure Asia\n",
+ "\n",
+ "Most of the filming of Batman movies is done in the Warner Bros studios and across the US, including New York and \n",
+ "Pittsburgh. You will notice ...\n",
+ "\n",
+ "1. [The Batman (2022) - Filming & production](https://www.imdb.com/title/tt1877830/locations/)\n",
+ "Source: IMDb\n",
+ "\n",
+ "Filming locations: Necropolis Cemetery, Glasgow, Scotland, UK (Batman and Selina leaving the cemetery) Helpfulโข86 1\n",
+ "St. George's Hall, Liverpool, England, UK\n",
+ "\n",
+ "2. [The Batman | Film Locations](https://movie-locations.com/movies/b/The-Batman-2022-2.php)\n",
+ "Source: The Worldwide Guide To Movie Locations\n",
+ "\n",
+ "Film locations for The Batman (2022) in Liverpool, London, Glasgow and Chicago.\n",
+ "\n",
+ "3. [Category:Film Locations - Batman Wiki - Fandom](https://batman.fandom.com/wiki/Category:Film_Locations)\n",
+ "Source: Batman Wiki\n",
+ "\n",
+ "Selina Kyle's apartment (Burtonverse) ยท Sheal Maritime Building ยท Shreck's ยท Snowy Cones Ice Cream Factory ยท South \n",
+ "Channel Island ยท South Hinkley ...\n",
+ "\n",
+ "4. [Dark Knight Rises Tour: See Batman Movie \n",
+ "Locations](https://www.travelchannel.com/interests/arts-and-culture/photos/see-batman-movie-locations)\n",
+ "Source: Travel Channel\n",
+ "\n",
+ "See Batman Movie Locations ยท Wollaton Hall ยท Carnegie Mellon University ยท The Farmiloe Building ยท Queensboro Bridge\n",
+ "ยท Wall Street ยท Mehrangarh Fort ยท Turda Saline.\n",
+ "\n",
+ "5. [What cities in America and anywhere in the world do you \n",
+ "...](https://www.reddit.com/r/batman/comments/1d1t88q/what_cities_in_america_and_anywhere_in_the_world/)\n",
+ "Source: Reddit ยท r/batman\n",
+ "\n",
+ "Glasgow and Liverpool were used in the shoot for The Batman. I'd avoid Chicago, as that looks more like Metropolis \n",
+ "than Gotham.\n",
+ "\n",
+ "6. [Where was The Batman filmed? ALL the Filming Locations \n",
+ "...](https://www.atlasofwonders.com/2022/04/where-was-the-batman-filmed.html)\n",
+ "Source: Atlas of Wonders\n",
+ "\n",
+ "The Batman was primarily filmed in the United Kingdom. Most of the recognizable buildings of this new version of \n",
+ "Gotham City are located in Liverpool.\n",
+ "\n",
+ "7. [The Dark Knight | 2008](https://movie-locations.com/movies/d/Dark-Knight.php)\n",
+ "Source: The Worldwide Guide To Movie Locations\n",
+ "\n",
+ "Discover where The Dark Knight (2008) was filmed around Chicago, as well as in London and Bedfordshire in the UK, \n",
+ "and briefly in Hong Kong.\n",
+ "\n",
+ "8. [Batman Movies and Filming Locations in Los \n",
+ "Angeles](https://www.traveltodayla.com/post/batman-movies-in-la?srsltid=AfmBOoqNOBBzvgmhmQdx1CjiZ98A8awKaUUsgA1_91F\n",
+ "_VDdwIpLDKwNX)\n",
+ "Source: LA Today\n",
+ "\n",
+ "Find Batman movies and filming locations in LA. Los Angeles is home to many locations where Batman movie scenes \n",
+ "were filmed. From the Batcave to Wayne Manor ...\n",
+ "\n",
+ "9. [Where The Batman Filmed (All Gotham Real-Life \n",
+ "Locations)](https://screenrant.com/where-was-the-batman-movie-filmed/)\n",
+ "Date published: Mar 4, 2022\n",
+ "Source: Screen Rant\n",
+ "\n",
+ "The Batman filmed all over the world to bring a new version of Gotham to life and went to some prominent real-life \n",
+ "locations to achieve the right look.\n",
+ "\n",
+ "Out: None\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "[Step 1: Duration 4.24 seconds| Input tokens: 6,523 | Output tokens: 151]\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[2m[Step 1: Duration 4.24 seconds| Input tokens: 6,523 | Output tokens: 151]\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Step 3 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[0m\u001b[1mStep \u001b[0m\u001b[1;36m3\u001b[0m\u001b[38;2;212;183;2m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ " โ Executing parsed code: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " url1 = \"https://www.travelandleisureasia.com/global/destinations/batman-movie-locations-you-can-visit/\" \n",
+ " url2 = \"https://www.imdb.com/title/tt1877830/locations/\" \n",
+ " \n",
+ " content1 = visit_webpage(url=url1) \n",
+ " content2 = visit_webpage(url=url2) \n",
+ " \n",
+ " print(\"Content from Travel and Leisure Asia:\") \n",
+ " print(content1) \n",
+ " print(\"\\n\" + \"=\"*80 + \"\\n\") # Print separator between pages \n",
+ " print(\"Content from IMDb:\") \n",
+ " print(content2) \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ "
\n"
+ ],
+ "text/plain": [
+ " โ \u001b[1mExecuting parsed code:\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34murl1\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mhttps://www.travelandleisureasia.com/global/destinations/batman-movie-locations-you-can-visit/\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34murl2\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mhttps://www.imdb.com/title/tt1877830/locations/\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mcontent1\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mvisit_webpage\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34murl\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34murl1\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mcontent2\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mvisit_webpage\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34murl\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34murl2\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mContent from Travel and Leisure Asia:\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcontent1\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m\\n\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m+\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m*\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m80\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m+\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m\\n\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;149;144;119;48;2;39;40;34m# Print separator between pages\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mContent from IMDb:\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcontent2\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Execution logs:\n",
+ "Content from Travel and Leisure Asia:\n",
+ "Batman Movie Locations You Can Visit In Person!\n",
+ "\n",
+ " \n",
+ "\n",
+ "\n",
+ "\n",
+ " \n",
+ "\n",
+ " Menu \n",
+ "\n",
+ " [](https://www.travelandleisureasia.com/global/ \"Travel and Leisure Asia | Global\") \n",
+ "\n",
+ " \n",
+ " \n",
+ "\n",
+ " \n",
+ "\n",
+ "GLOBAL\n",
+ "\n",
+ "* [Global](/global/)\n",
+ "* [IN](/in/)\n",
+ "* [SG](/sg/)\n",
+ "* [HK](/hk/)\n",
+ "* [TH](/th/)\n",
+ "* [MY](/my/)\n",
+ "\n",
+ "* [Destinations](https://www.travelandleisureasia.com/global/destinations/)\n",
+ " + [India](https://www.travelandleisureasia.com/global/destinations/india/)\n",
+ " + [South Asia](https://www.travelandleisureasia.com/global/destinations/south-asia/)\n",
+ " + [Southeast Asia](https://www.travelandleisureasia.com/global/destinations/southeast-asia/)\n",
+ " + [Asia](https://www.travelandleisureasia.com/global/destinations/asia/)\n",
+ " + [North America](https://www.travelandleisureasia.com/global/destinations/north-america/)\n",
+ " + [Central & South America](https://www.travelandleisureasia.com/global/destinations/central-and-south-america/)\n",
+ " + [Europe](https://www.travelandleisureasia.com/global/destinations/europe/)\n",
+ " + [Middle-East](https://www.travelandleisureasia.com/global/destinations/middle-east/)\n",
+ " + [Australia & South \n",
+ "Pacific](https://www.travelandleisureasia.com/global/destinations/australia-and-south-pacific/)\n",
+ " + [Africa](https://www.travelandleisureasia.com/global/destinations/africa/)\n",
+ "* [Hotels](https://www.travelandleisureasia.com/global/hotels/)\n",
+ " + [India](https://www.travelandleisureasia.com/global/hotels/india-hotels/)\n",
+ " + [South Asia](https://www.travelandleisureasia.com/global/hotels/south-asia-hotels/)\n",
+ " + [Southeast Asia](https://www.travelandleisureasia.com/global/hotels/southeast-asia-hotels/)\n",
+ " + [Asia](https://www.travelandleisureasia.com/global/hotels/asia-hotels/)\n",
+ " + [North America](https://www.travelandleisureasia.com/global/hotels/north-america-hotels/)\n",
+ " + [Central & South America](https://www.travelandleisureasia.com/global/hotels/central-and-south-america-hotels/)\n",
+ " + [Europe](https://www.travelandleisureasia.com/global/hotels/europe-hotels/)\n",
+ " + [Middle-East](https://www.travelandleisureasia.com/global/hotels/middle-east-hotels/)\n",
+ " + [Australia & South \n",
+ "Pacific](https://www.travelandleisureasia.com/global/hotels/australia-and-south-pacific-hotels/)\n",
+ " + [Africa](https://www.travelandleisureasia.com/global/hotels/africa-hotels/)\n",
+ "* [Luxury Awards 2025](https://awards.travelandleisureasia.com/luxuryawards2025/)\n",
+ "* [People](https://www.travelandleisureasia.com/global/people/)\n",
+ " + [Travellers](https://www.travelandleisureasia.com/global/people/travellers/)\n",
+ " + [Culture](https://www.travelandleisureasia.com/global/people/culture/)\n",
+ "* [Dining](https://www.travelandleisureasia.com/global/dining/)\n",
+ " + [Food](https://www.travelandleisureasia.com/global/dining/food/)\n",
+ " + [Drink](https://www.travelandleisureasia.com/global/dining/drink/)\n",
+ " + [Reviews](https://www.travelandleisureasia.com/global/dining/reviews/)\n",
+ "* [Trips](https://www.travelandleisureasia.com/global/trips/)\n",
+ " + [Road Trips](https://www.travelandleisureasia.com/global/trips/road-trips/)\n",
+ " + [Family Travel](https://www.travelandleisureasia.com/global/trips/family-travel/)\n",
+ " + [Solo Travel](https://www.travelandleisureasia.com/global/trips/solo-travel/)\n",
+ " + [Outdoors](https://www.travelandleisureasia.com/global/trips/outdoors/)\n",
+ " + [Cruises](https://www.travelandleisureasia.com/global/trips/cruises/)\n",
+ " + [Beaches & Islands](https://www.travelandleisureasia.com/global/trips/beaches-and-islands/)\n",
+ " + [Homestays](https://www.travelandleisureasia.com/global/trips/homestays/)\n",
+ " + [Zodiac Travels](https://www.travelandleisureasia.com/global/trips/zodiac-travels/)\n",
+ " + [Wellness & Spas](https://www.travelandleisureasia.com/global/trips/wellness-and-spas/)\n",
+ "* [Travel Tips](https://www.travelandleisureasia.com/global/travel-tips/)\n",
+ " + [Accessories](https://www.travelandleisureasia.com/global/travel-tips/accessories/)\n",
+ " + [Points and Miles](https://www.travelandleisureasia.com/global/travel-tips/points-and-miles/)\n",
+ " + [Planning](https://www.travelandleisureasia.com/global/travel-tips/trip-planning/)\n",
+ "* [#TheGreenEdit](https://www.travelandleisureasia.com/global/tag/thegreenedit/)\n",
+ "* [T+L Tastemakers](https://www.travelandleisureasia.com/global/tl-tastemakers/)\n",
+ "* [#TLKids](https://www.travelandleisureasia.com/global/tag/tlkids/)\n",
+ "* [News](https://www.travelandleisureasia.com/global/news/)\n",
+ "* [Sustainable Travel](https://www.travelandleisureasia.com/global/trips/the-conscious-traveller/)\n",
+ "* [T+L Experiences](https://experiences.travelandleisureasia.com/)\n",
+ "* [more nav](#)\n",
+ "\n",
+ " [](#) \n",
+ " \n",
+ "\n",
+ "* [Destinations](https://www.travelandleisureasia.com/global/destinations/)\n",
+ " + [India](https://www.travelandleisureasia.com/global/destinations/india/)\n",
+ " + [South\n",
+ "..._This content has been truncated to stay below 10000 characters_...\n",
+ "ges.travelandleisureasia.com/wp-content/uploads/default/tl-placeholder-image.png?tr=q-50)](https://www.travelandlei\n",
+ "sureasia.com/global/destinations/north-america/this-region-is-called-canadas-napa-valley-and-its-the-best-kept-wint\n",
+ "er-secret/) \n",
+ "\n",
+ "[North America](https://www.travelandleisureasia.com/global/destinations/north-america/) \n",
+ "\n",
+ "### [This Region Is Called 'Canada's Napa Valley'โAnd It's The Best-kept Winter \n",
+ "Secret](https://www.travelandleisureasia.com/global/destinations/north-america/this-region-is-called-canadas-napa-v\n",
+ "alley-and-its-the-best-kept-winter-secret/ \"This Region Is Called 'Canada's Napa Valley'โAnd It's The Best-kept \n",
+ "Winter Secret\")\n",
+ "\n",
+ "4 days ago | 6 min read\n",
+ "\n",
+ "[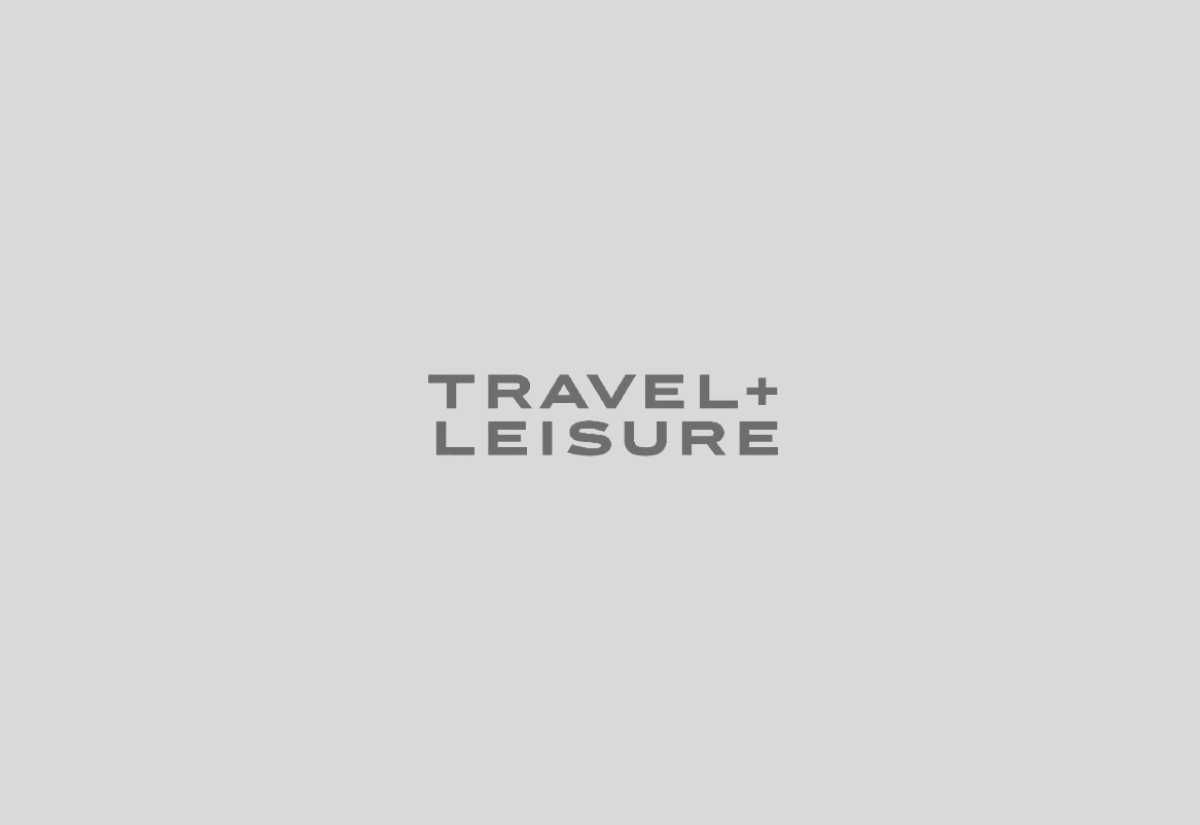](ht\n",
+ "tps://www.travelandleisureasia.com/global/destinations/southeast-asia/indian-movies-shot-in-thailand/) \n",
+ "\n",
+ "[Southeast Asia](https://www.travelandleisureasia.com/global/destinations/southeast-asia/) \n",
+ "\n",
+ "### [Did You Know These Popular Indian Movies Were Shot In \n",
+ "Thailand?](https://www.travelandleisureasia.com/global/destinations/southeast-asia/indian-movies-shot-in-thailand/ \n",
+ "\"Did You Know These Popular Indian Movies Were Shot In Thailand?\")\n",
+ "\n",
+ "4 days ago | 7 min read\n",
+ "\n",
+ "[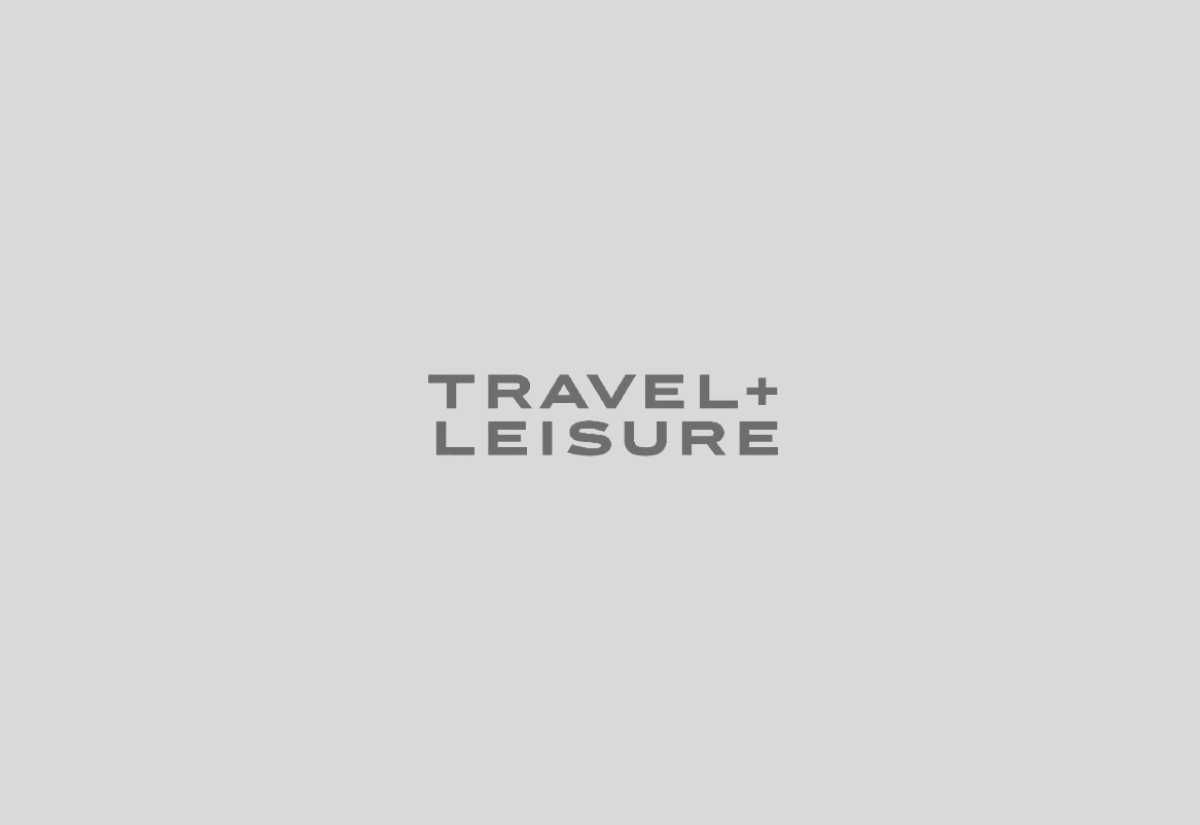](https\n",
+ "://www.travelandleisureasia.com/global/destinations/asia/beautiful-places-to-visit-in-taiwan-temples-trails-towers/\n",
+ ") \n",
+ "\n",
+ "[Asia](https://www.travelandleisureasia.com/global/destinations/asia/) \n",
+ "\n",
+ "### [Temples, Trails, And Towers: Be Charmed These Beautiful Places In \n",
+ "Taiwan](https://www.travelandleisureasia.com/global/destinations/asia/beautiful-places-to-visit-in-taiwan-temples-t\n",
+ "rails-towers/ \"Temples, Trails, And Towers: Be Charmed These Beautiful Places In Taiwan\")\n",
+ "\n",
+ "5 days ago | 9 min read\n",
+ "\n",
+ "[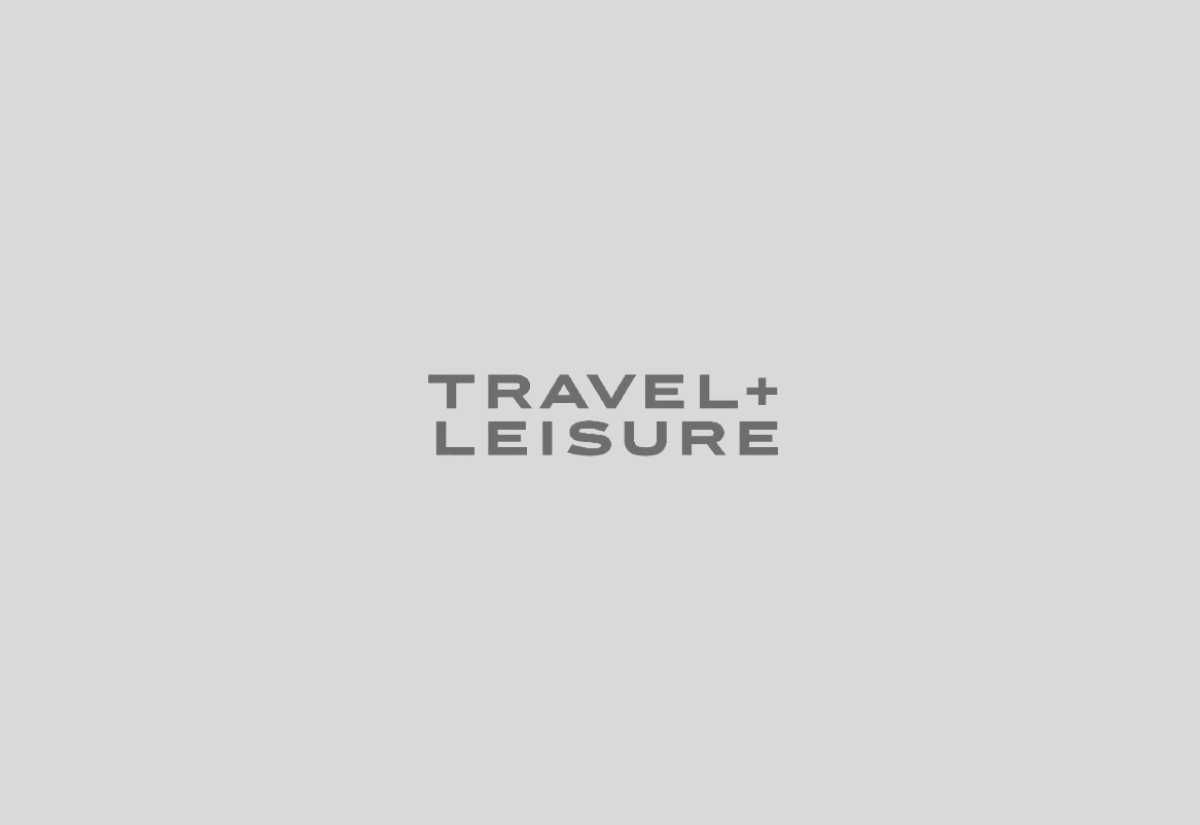](https:/\n",
+ "/www.travelandleisureasia.com/global/destinations/asia/places-to-visit-in-shanghai-yu-garden-jade-buddha-temple-the\n",
+ "-bund/) \n",
+ "\n",
+ "[Asia](https://www.travelandleisureasia.com/global/destinations/asia/) \n",
+ "\n",
+ "### [Explore The Best Of Shanghai: Places To Visit In China's Dazzling Futuristic \n",
+ "City](https://www.travelandleisureasia.com/global/destinations/asia/places-to-visit-in-shanghai-yu-garden-jade-budd\n",
+ "ha-temple-the-bund/ \"Explore The Best Of Shanghai: Places To Visit In China's Dazzling Futuristic City\")\n",
+ "\n",
+ "6 days ago | 8 min read\n",
+ "\n",
+ "[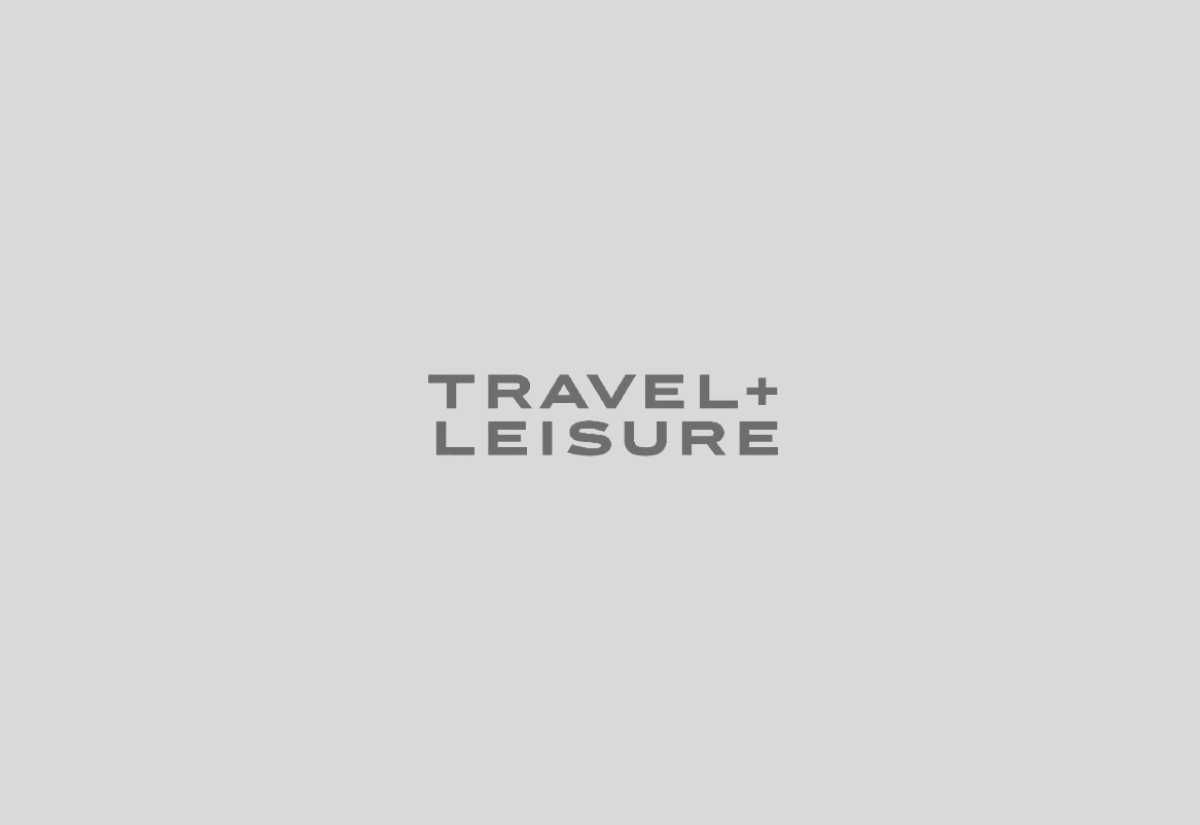](htt\n",
+ "ps://www.travelandleisureasia.com/global/destinations/southeast-asia/the-best-places-to-visit-in-langkawi-malaysia/\n",
+ ") \n",
+ "\n",
+ "[Southeast Asia](https://www.travelandleisureasia.com/global/destinations/southeast-asia/) \n",
+ "\n",
+ "### [The Best Places To Visit In Langkawi, \n",
+ "Malaysia](https://www.travelandleisureasia.com/global/destinations/southeast-asia/the-best-places-to-visit-in-langk\n",
+ "awi-malaysia/ \"The Best Places To Visit In Langkawi, Malaysia\")\n",
+ "\n",
+ "6 days ago | 16 min read\n",
+ "\n",
+ "### Sign up for our newsletters to have the latest stories delivered straight to your inbox every week.\n",
+ "\n",
+ "I agree to the [Privacy Policy](https://www.travelandleisureasia.com/global/privacy/)\n",
+ "\n",
+ "* [SINGAPORE](https://www.travelandleisureasia.com/sg/ \"Singapore\")\n",
+ "* [HONG KONG](https://www.travelandleisureasia.com/hk/ \"Homg Kong\")\n",
+ "* [THAILAND](https://www.travelandleisureasia.com/th/ \"Thailand\")\n",
+ "* [MALAYSIA](https://www.travelandleisureasia.com/my/ \"Malaysia\")\n",
+ "* [INDIA](https://www.travelandleisureasia.com/in/ \"India\")\n",
+ "* [GLOBAL](https://www.travelandleisureasia.com/global/ \"Global\")\n",
+ "\n",
+ "* [About Us](https://www.travelandleisureasia.com/global/about-us/)\n",
+ "* [Contact Us](https://www.travelandleisureasia.com/global/contact-us/)\n",
+ "* [Privacy Policy](https://www.travelandleisureasia.com/global/privacy/)\n",
+ "* [Terms & Conditions](https://www.travelandleisureasia.com/global/terms-conditions/)\n",
+ "\n",
+ "ยฉ 2025 Copyright TRAVEL + LEISURE ASIA site by [Burdaluxury](https://www.burdaluxury.com/)\n",
+ "\n",
+ "* [Burda Luxury](https://www.burdaluxury.com/ \"Burda Luxury\")\n",
+ "* [ARCHITECTURE + DESIGN](https://www.architectureplusdesign.in/ \"ARCHITECTURE + DESIGN\")\n",
+ "* [August Man](https://www.augustman.com/ \"August Man\")\n",
+ "* [Lifestyle Asia](https://www.lifestyleasia.com/ \"Lifestyle Asia\")\n",
+ "* [Prestige Online](https://www.prestigeonline.com/ \"Prestige Online\")\n",
+ "* [Pin Prestige](https://www.pinprestige.com \"Pin Prestige\")\n",
+ "* [Hello Thailand](https://th.hellomagazine.com/ \"Hello Thailand\")\n",
+ "\n",
+ "ร\n",
+ "Never miss an update\n",
+ "\n",
+ "Subscribe to our newsletter to get the latest on travel, stay & dining.\n",
+ "\n",
+ "agree to receive this\n",
+ "newsletter.\n",
+ "\n",
+ "SUBSCRIBE\n",
+ "\n",
+ "No Thanks\n",
+ "\n",
+ "##### Youโre all set\n",
+ "\n",
+ "Thank you for your subscription.\n",
+ "\n",
+ "================================================================================\n",
+ "\n",
+ "Content from IMDb:\n",
+ "Error fetching the webpage: 403 Client Error: Forbidden for url: https://www.imdb.com/title/tt1877830/locations/\n",
+ "\n",
+ "Out: None\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1mExecution logs:\u001b[0m\n",
+ "Content from Travel and Leisure Asia:\n",
+ "Batman Movie Locations You Can Visit In Person!\n",
+ "\n",
+ " \n",
+ "\n",
+ "\n",
+ "\n",
+ " \n",
+ "\n",
+ " Menu \n",
+ "\n",
+ " [](https://www.travelandleisureasia.com/global/ \"Travel and Leisure Asia | Global\") \n",
+ "\n",
+ " \n",
+ " \n",
+ "\n",
+ " \n",
+ "\n",
+ "GLOBAL\n",
+ "\n",
+ "* [Global](/global/)\n",
+ "* [IN](/in/)\n",
+ "* [SG](/sg/)\n",
+ "* [HK](/hk/)\n",
+ "* [TH](/th/)\n",
+ "* [MY](/my/)\n",
+ "\n",
+ "* [Destinations](https://www.travelandleisureasia.com/global/destinations/)\n",
+ " + [India](https://www.travelandleisureasia.com/global/destinations/india/)\n",
+ " + [South Asia](https://www.travelandleisureasia.com/global/destinations/south-asia/)\n",
+ " + [Southeast Asia](https://www.travelandleisureasia.com/global/destinations/southeast-asia/)\n",
+ " + [Asia](https://www.travelandleisureasia.com/global/destinations/asia/)\n",
+ " + [North America](https://www.travelandleisureasia.com/global/destinations/north-america/)\n",
+ " + [Central & South America](https://www.travelandleisureasia.com/global/destinations/central-and-south-america/)\n",
+ " + [Europe](https://www.travelandleisureasia.com/global/destinations/europe/)\n",
+ " + [Middle-East](https://www.travelandleisureasia.com/global/destinations/middle-east/)\n",
+ " + [Australia & South \n",
+ "Pacific](https://www.travelandleisureasia.com/global/destinations/australia-and-south-pacific/)\n",
+ " + [Africa](https://www.travelandleisureasia.com/global/destinations/africa/)\n",
+ "* [Hotels](https://www.travelandleisureasia.com/global/hotels/)\n",
+ " + [India](https://www.travelandleisureasia.com/global/hotels/india-hotels/)\n",
+ " + [South Asia](https://www.travelandleisureasia.com/global/hotels/south-asia-hotels/)\n",
+ " + [Southeast Asia](https://www.travelandleisureasia.com/global/hotels/southeast-asia-hotels/)\n",
+ " + [Asia](https://www.travelandleisureasia.com/global/hotels/asia-hotels/)\n",
+ " + [North America](https://www.travelandleisureasia.com/global/hotels/north-america-hotels/)\n",
+ " + [Central & South America](https://www.travelandleisureasia.com/global/hotels/central-and-south-america-hotels/)\n",
+ " + [Europe](https://www.travelandleisureasia.com/global/hotels/europe-hotels/)\n",
+ " + [Middle-East](https://www.travelandleisureasia.com/global/hotels/middle-east-hotels/)\n",
+ " + [Australia & South \n",
+ "Pacific](https://www.travelandleisureasia.com/global/hotels/australia-and-south-pacific-hotels/)\n",
+ " + [Africa](https://www.travelandleisureasia.com/global/hotels/africa-hotels/)\n",
+ "* [Luxury Awards 2025](https://awards.travelandleisureasia.com/luxuryawards2025/)\n",
+ "* [People](https://www.travelandleisureasia.com/global/people/)\n",
+ " + [Travellers](https://www.travelandleisureasia.com/global/people/travellers/)\n",
+ " + [Culture](https://www.travelandleisureasia.com/global/people/culture/)\n",
+ "* [Dining](https://www.travelandleisureasia.com/global/dining/)\n",
+ " + [Food](https://www.travelandleisureasia.com/global/dining/food/)\n",
+ " + [Drink](https://www.travelandleisureasia.com/global/dining/drink/)\n",
+ " + [Reviews](https://www.travelandleisureasia.com/global/dining/reviews/)\n",
+ "* [Trips](https://www.travelandleisureasia.com/global/trips/)\n",
+ " + [Road Trips](https://www.travelandleisureasia.com/global/trips/road-trips/)\n",
+ " + [Family Travel](https://www.travelandleisureasia.com/global/trips/family-travel/)\n",
+ " + [Solo Travel](https://www.travelandleisureasia.com/global/trips/solo-travel/)\n",
+ " + [Outdoors](https://www.travelandleisureasia.com/global/trips/outdoors/)\n",
+ " + [Cruises](https://www.travelandleisureasia.com/global/trips/cruises/)\n",
+ " + [Beaches & Islands](https://www.travelandleisureasia.com/global/trips/beaches-and-islands/)\n",
+ " + [Homestays](https://www.travelandleisureasia.com/global/trips/homestays/)\n",
+ " + [Zodiac Travels](https://www.travelandleisureasia.com/global/trips/zodiac-travels/)\n",
+ " + [Wellness & Spas](https://www.travelandleisureasia.com/global/trips/wellness-and-spas/)\n",
+ "* [Travel Tips](https://www.travelandleisureasia.com/global/travel-tips/)\n",
+ " + [Accessories](https://www.travelandleisureasia.com/global/travel-tips/accessories/)\n",
+ " + [Points and Miles](https://www.travelandleisureasia.com/global/travel-tips/points-and-miles/)\n",
+ " + [Planning](https://www.travelandleisureasia.com/global/travel-tips/trip-planning/)\n",
+ "* [#TheGreenEdit](https://www.travelandleisureasia.com/global/tag/thegreenedit/)\n",
+ "* [T+L Tastemakers](https://www.travelandleisureasia.com/global/tl-tastemakers/)\n",
+ "* [#TLKids](https://www.travelandleisureasia.com/global/tag/tlkids/)\n",
+ "* [News](https://www.travelandleisureasia.com/global/news/)\n",
+ "* [Sustainable Travel](https://www.travelandleisureasia.com/global/trips/the-conscious-traveller/)\n",
+ "* [T+L Experiences](https://experiences.travelandleisureasia.com/)\n",
+ "* [more nav](#)\n",
+ "\n",
+ " [](#) \n",
+ " \n",
+ "\n",
+ "* [Destinations](https://www.travelandleisureasia.com/global/destinations/)\n",
+ " + [India](https://www.travelandleisureasia.com/global/destinations/india/)\n",
+ " + [South\n",
+ "..._This content has been truncated to stay below 10000 characters_...\n",
+ "ges.travelandleisureasia.com/wp-content/uploads/default/tl-placeholder-image.png?tr=q-50)](https://www.travelandlei\n",
+ "sureasia.com/global/destinations/north-america/this-region-is-called-canadas-napa-valley-and-its-the-best-kept-wint\n",
+ "er-secret/) \n",
+ "\n",
+ "[North America](https://www.travelandleisureasia.com/global/destinations/north-america/) \n",
+ "\n",
+ "### [This Region Is Called 'Canada's Napa Valley'โAnd It's The Best-kept Winter \n",
+ "Secret](https://www.travelandleisureasia.com/global/destinations/north-america/this-region-is-called-canadas-napa-v\n",
+ "alley-and-its-the-best-kept-winter-secret/ \"This Region Is Called 'Canada's Napa Valley'โAnd It's The Best-kept \n",
+ "Winter Secret\")\n",
+ "\n",
+ "4 days ago | 6 min read\n",
+ "\n",
+ "[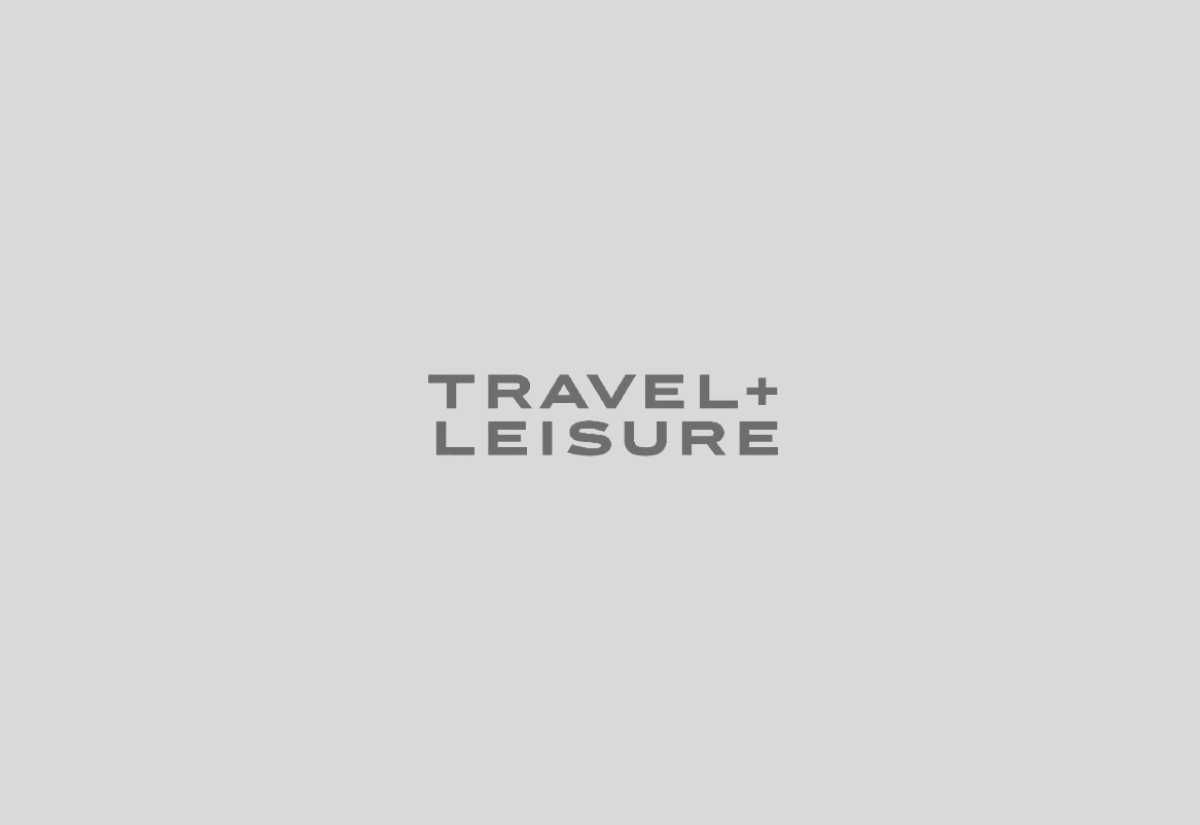](ht\n",
+ "tps://www.travelandleisureasia.com/global/destinations/southeast-asia/indian-movies-shot-in-thailand/) \n",
+ "\n",
+ "[Southeast Asia](https://www.travelandleisureasia.com/global/destinations/southeast-asia/) \n",
+ "\n",
+ "### [Did You Know These Popular Indian Movies Were Shot In \n",
+ "Thailand?](https://www.travelandleisureasia.com/global/destinations/southeast-asia/indian-movies-shot-in-thailand/ \n",
+ "\"Did You Know These Popular Indian Movies Were Shot In Thailand?\")\n",
+ "\n",
+ "4 days ago | 7 min read\n",
+ "\n",
+ "[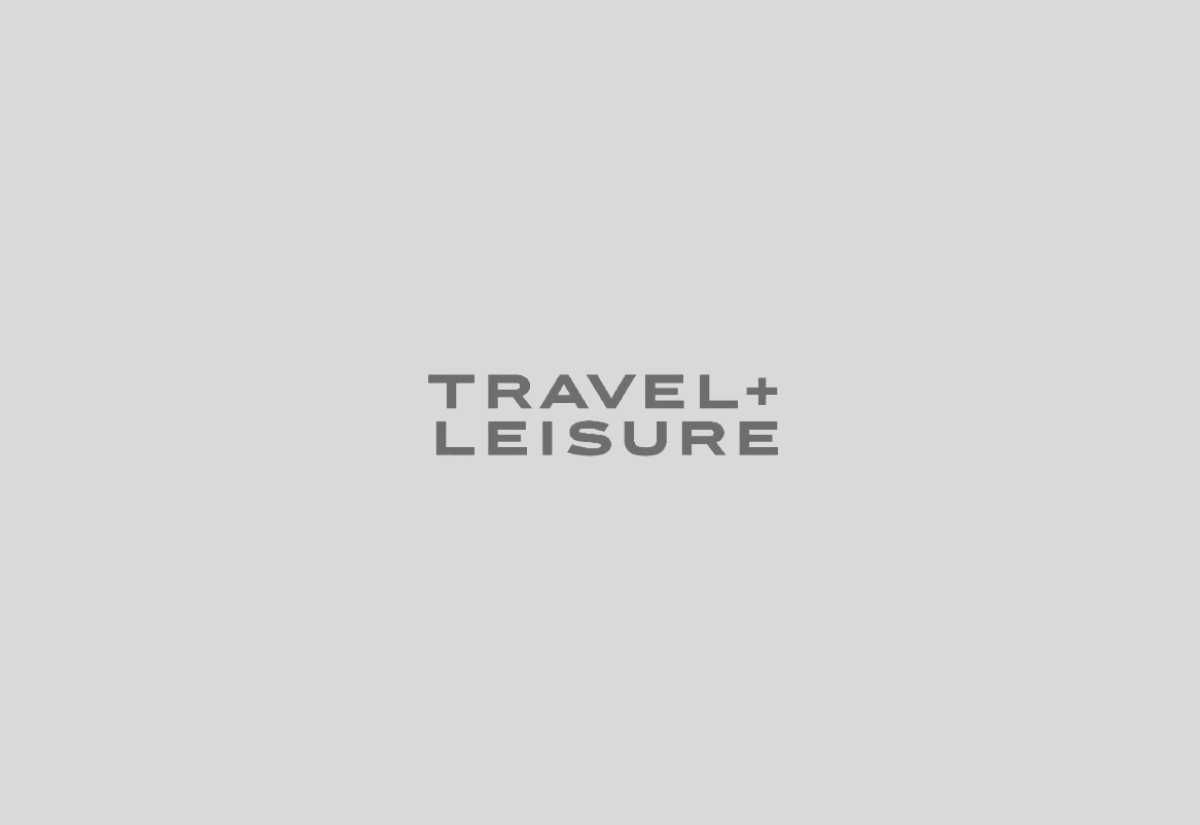](https\n",
+ "://www.travelandleisureasia.com/global/destinations/asia/beautiful-places-to-visit-in-taiwan-temples-trails-towers/\n",
+ ") \n",
+ "\n",
+ "[Asia](https://www.travelandleisureasia.com/global/destinations/asia/) \n",
+ "\n",
+ "### [Temples, Trails, And Towers: Be Charmed These Beautiful Places In \n",
+ "Taiwan](https://www.travelandleisureasia.com/global/destinations/asia/beautiful-places-to-visit-in-taiwan-temples-t\n",
+ "rails-towers/ \"Temples, Trails, And Towers: Be Charmed These Beautiful Places In Taiwan\")\n",
+ "\n",
+ "5 days ago | 9 min read\n",
+ "\n",
+ "[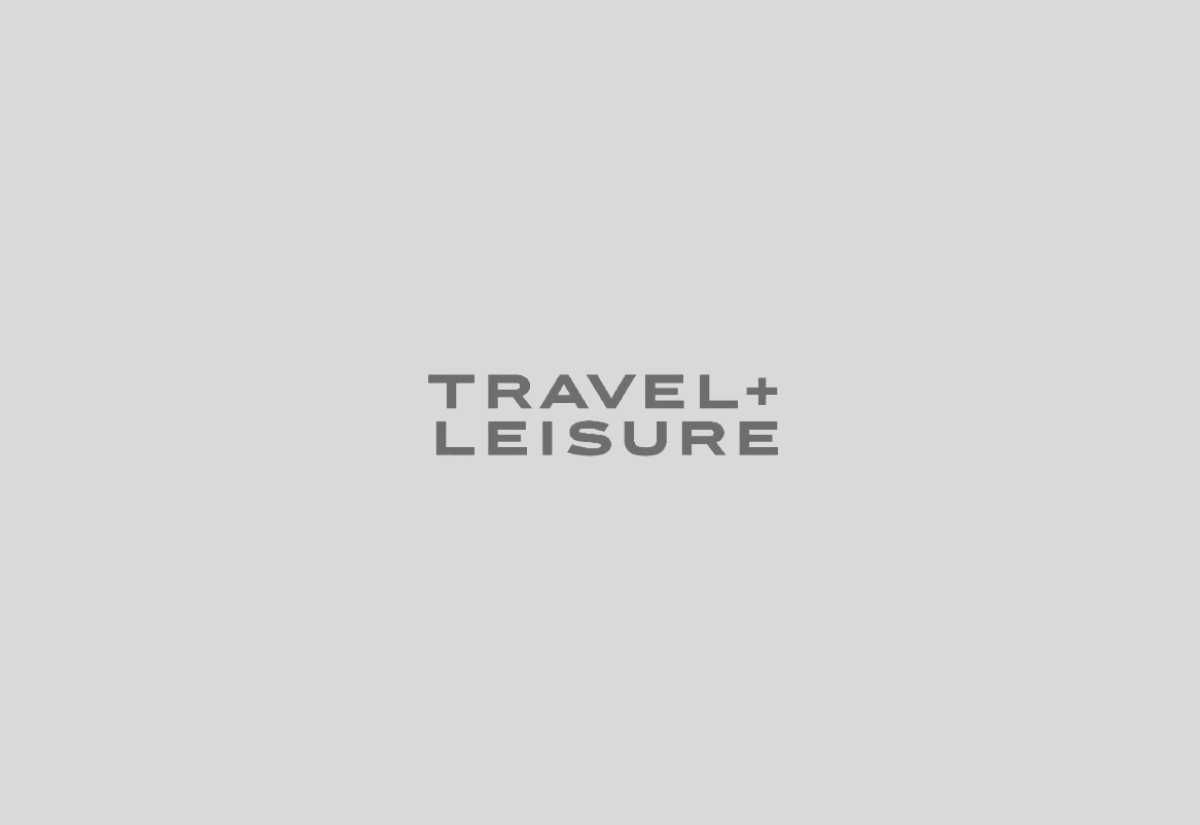](https:/\n",
+ "/www.travelandleisureasia.com/global/destinations/asia/places-to-visit-in-shanghai-yu-garden-jade-buddha-temple-the\n",
+ "-bund/) \n",
+ "\n",
+ "[Asia](https://www.travelandleisureasia.com/global/destinations/asia/) \n",
+ "\n",
+ "### [Explore The Best Of Shanghai: Places To Visit In China's Dazzling Futuristic \n",
+ "City](https://www.travelandleisureasia.com/global/destinations/asia/places-to-visit-in-shanghai-yu-garden-jade-budd\n",
+ "ha-temple-the-bund/ \"Explore The Best Of Shanghai: Places To Visit In China's Dazzling Futuristic City\")\n",
+ "\n",
+ "6 days ago | 8 min read\n",
+ "\n",
+ "[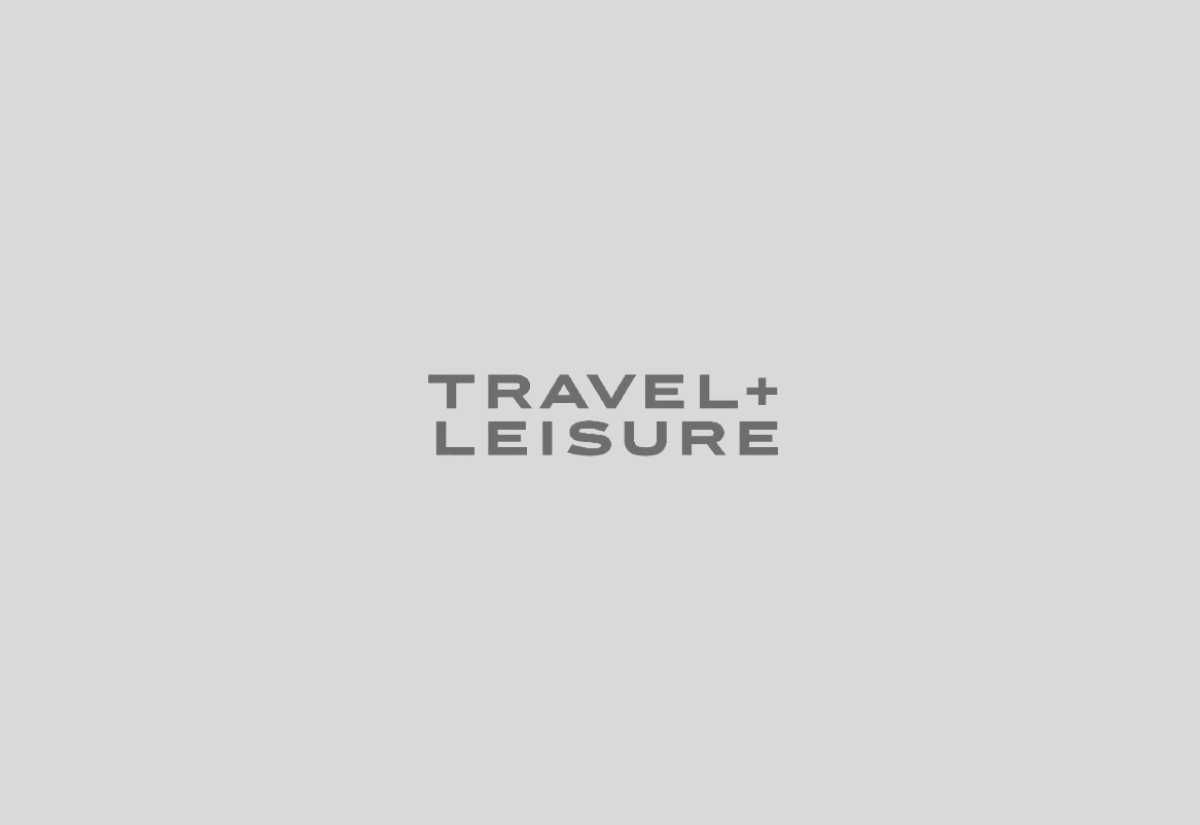](htt\n",
+ "ps://www.travelandleisureasia.com/global/destinations/southeast-asia/the-best-places-to-visit-in-langkawi-malaysia/\n",
+ ") \n",
+ "\n",
+ "[Southeast Asia](https://www.travelandleisureasia.com/global/destinations/southeast-asia/) \n",
+ "\n",
+ "### [The Best Places To Visit In Langkawi, \n",
+ "Malaysia](https://www.travelandleisureasia.com/global/destinations/southeast-asia/the-best-places-to-visit-in-langk\n",
+ "awi-malaysia/ \"The Best Places To Visit In Langkawi, Malaysia\")\n",
+ "\n",
+ "6 days ago | 16 min read\n",
+ "\n",
+ "### Sign up for our newsletters to have the latest stories delivered straight to your inbox every week.\n",
+ "\n",
+ "I agree to the [Privacy Policy](https://www.travelandleisureasia.com/global/privacy/)\n",
+ "\n",
+ "* [SINGAPORE](https://www.travelandleisureasia.com/sg/ \"Singapore\")\n",
+ "* [HONG KONG](https://www.travelandleisureasia.com/hk/ \"Homg Kong\")\n",
+ "* [THAILAND](https://www.travelandleisureasia.com/th/ \"Thailand\")\n",
+ "* [MALAYSIA](https://www.travelandleisureasia.com/my/ \"Malaysia\")\n",
+ "* [INDIA](https://www.travelandleisureasia.com/in/ \"India\")\n",
+ "* [GLOBAL](https://www.travelandleisureasia.com/global/ \"Global\")\n",
+ "\n",
+ "* [About Us](https://www.travelandleisureasia.com/global/about-us/)\n",
+ "* [Contact Us](https://www.travelandleisureasia.com/global/contact-us/)\n",
+ "* [Privacy Policy](https://www.travelandleisureasia.com/global/privacy/)\n",
+ "* [Terms & Conditions](https://www.travelandleisureasia.com/global/terms-conditions/)\n",
+ "\n",
+ "ยฉ 2025 Copyright TRAVEL + LEISURE ASIA site by [Burdaluxury](https://www.burdaluxury.com/)\n",
+ "\n",
+ "* [Burda Luxury](https://www.burdaluxury.com/ \"Burda Luxury\")\n",
+ "* [ARCHITECTURE + DESIGN](https://www.architectureplusdesign.in/ \"ARCHITECTURE + DESIGN\")\n",
+ "* [August Man](https://www.augustman.com/ \"August Man\")\n",
+ "* [Lifestyle Asia](https://www.lifestyleasia.com/ \"Lifestyle Asia\")\n",
+ "* [Prestige Online](https://www.prestigeonline.com/ \"Prestige Online\")\n",
+ "* [Pin Prestige](https://www.pinprestige.com \"Pin Prestige\")\n",
+ "* [Hello Thailand](https://th.hellomagazine.com/ \"Hello Thailand\")\n",
+ "\n",
+ "ร\n",
+ "Never miss an update\n",
+ "\n",
+ "Subscribe to our newsletter to get the latest on travel, stay & dining.\n",
+ "\n",
+ "agree to receive this\n",
+ "newsletter.\n",
+ "\n",
+ "SUBSCRIBE\n",
+ "\n",
+ "No Thanks\n",
+ "\n",
+ "##### Youโre all set\n",
+ "\n",
+ "Thank you for your subscription.\n",
+ "\n",
+ "================================================================================\n",
+ "\n",
+ "Content from IMDb:\n",
+ "Error fetching the webpage: 403 Client Error: Forbidden for url: https://www.imdb.com/title/tt1877830/locations/\n",
+ "\n",
+ "Out: None\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "[Step 2: Duration 5.56 seconds| Input tokens: 11,060 | Output tokens: 472]\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[2m[Step 2: Duration 5.56 seconds| Input tokens: 11,060 | Output tokens: 472]\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Step 4 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[0m\u001b[1mStep \u001b[0m\u001b[1;36m4\u001b[0m\u001b[38;2;212;183;2m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ " โ Executing parsed code: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " url3 = \"https://movie-locations.com/movies/b/The-Batman-2022-2.php\" \n",
+ " url4 = \"https://movie-locations.com/movies/d/Dark-Knight.php\" \n",
+ " \n",
+ " content3 = visit_webpage(url=url3) \n",
+ " content4 = visit_webpage(url=url4) \n",
+ " \n",
+ " print(\"Content from Movie Locations (The Batman 2022):\") \n",
+ " print(content3) \n",
+ " print(\"\\n\" + \"=\"*80 + \"\\n\") # Print separator between pages \n",
+ " print(\"Content from Movie Locations (The Dark Knight 2008):\") \n",
+ " print(content4) \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ "
\n"
+ ],
+ "text/plain": [
+ " โ \u001b[1mExecuting parsed code:\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34murl3\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mhttps://movie-locations.com/movies/b/The-Batman-2022-2.php\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34murl4\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mhttps://movie-locations.com/movies/d/Dark-Knight.php\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mcontent3\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mvisit_webpage\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34murl\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34murl3\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mcontent4\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mvisit_webpage\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34murl\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34murl4\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mContent from Movie Locations (The Batman 2022):\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcontent3\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m\\n\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m+\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m*\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m80\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m+\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m\\n\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;149;144;119;48;2;39;40;34m# Print separator between pages\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mContent from Movie Locations (The Dark Knight 2008):\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcontent4\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Execution logs:\n",
+ "Content from Movie Locations (The Batman 2022):\n",
+ "The Batman | Film Locations\n",
+ "\n",
+ "\n",
+ "\n",
+ "* [| ย HOME](../../index.php)\n",
+ "* [| ย FILMS](../../movies.php)\n",
+ " + [0](../0/0-movies.php)\n",
+ " + [A](../a/a-movies.php)\n",
+ " + [B](../b/b-movies.php)\n",
+ " + [C](../c/c-movies.php)\n",
+ " + [D](../d/d-movies.php)\n",
+ " + [E](../e/e-movies.php)\n",
+ " + [F](../f/f-movies.php)\n",
+ " + [G](../g/g-movies.php)\n",
+ " + [H](../h/h-movies.php)\n",
+ " + [I](../i/i-movies.php)\n",
+ " + [J](../j/j-movies.php)\n",
+ " + [K](../k/k-movies.php)\n",
+ " + [L](../l/l-movies.php)\n",
+ " + [M](../m/m-movies.php)\n",
+ " + [N](../n/n-movies.php)\n",
+ " + [O](../o/o-movies.php)\n",
+ " + [P](../p/p-movies.php)\n",
+ " + [Q](../q/q-movies.php)\n",
+ " + [R](../r/r-movies.php)\n",
+ " + [S](../s/s-movies.php)\n",
+ " + [T](../t/t-movies.php)\n",
+ " + [U](../u/u-movies.php)\n",
+ " + [V](../v/v-movies.php)\n",
+ " + [W](../w/w-movies.php)\n",
+ " + [X](../x/x-movies.php)\n",
+ " + [Y](../y/y-movies.php)\n",
+ " + [Z](../z/z-movies.php)\n",
+ "* [| ย PLACES](../../places.php)\n",
+ " + [AFRICA](../../places/africa.php)\n",
+ " + [ASIA](../../places/asia.php)\n",
+ " + [CANADA](../../places/canada.php)\n",
+ " + [CARIBBEAN](../../places/caribbean.php)\n",
+ " + [CENTRAL AMERICA](../../places/centam.php)\n",
+ " + [EUROPE](../../places/europe.php)\n",
+ " + [MIDDLE EAST](../../places/mideast.php)\n",
+ " + [OCEANIA](../../places/oceania.php)\n",
+ " + [RUSSIA](../../places/russia/russia.php)\n",
+ " + [SOUTH AMERICA](../../places/samerica.php)\n",
+ " + [UNITED KINGDOM](../../places/uk.php)\n",
+ " + [USA](../../places/usa.php)\n",
+ "* [| ย PEOPLE](../../people.php)\n",
+ " + [A](../../people/a/a-people.php)\n",
+ " + [B](../../people/b/b-people.php)\n",
+ " + [C](../../people/c/c-people.php)\n",
+ " + [D](../../people/d/d-people.php)\n",
+ " + [E](../../people/e/e-people.php)\n",
+ " + [F](../../people/f/f-people.php)\n",
+ " + [G](../../people/g/g-people.php)\n",
+ " + [H](../../people/h/h-people.php)\n",
+ " + [I](../../people/i/i-people.php)\n",
+ " + [J](../../people/j/j-people.php)\n",
+ " + [K](../../people/k/k-people.php)\n",
+ " + [L](../../people/l/l-people.php)\n",
+ " + [M](../../people/m/m-people.php)\n",
+ " + [N](../../people/n/n-people.php)\n",
+ " + [O](../../people/o/o-people.php)\n",
+ " + [P](../../people/p/p-people.php)\n",
+ " + [Q](../../people/q/q-people.php)\n",
+ " + [R](../../people/r/r-people.php)\n",
+ " + [S](../../people/s/s-people.php)\n",
+ " + [T](../../people/t/t-people.php)\n",
+ " + [U](../../people/u/u-people.php)\n",
+ " + [V](../../people/v/v-people.php)\n",
+ " + [W](../../people/w/w-people.php)\n",
+ " + [X](../../people/x/x-people.php)\n",
+ " + [Y](../../people/y/y-people.php)\n",
+ " + [Z](../../people/z/z-people.php)\n",
+ "* [| ย MORE](#)\n",
+ " + [ABOUT](../../about.php)\n",
+ " + [TRAVEL](../../travel.php)\n",
+ " + [FEATURES](../../features.php)\n",
+ " + [LINKS](../../links.php)\n",
+ "* [| ย SEARCH](#)\n",
+ "* |\n",
+ "\n",
+ "* [| ย HOME](../../index.php)\n",
+ "* [| ย FILMS](../../movies.php)\n",
+ "* [| ย PLACES](../../places.php)\n",
+ "* [| ย PEOPLE](../../people.php)\n",
+ "\n",
+ "[Home](../../index.php) > [Films](../../movies.php) > [B](b-movies.php) > The batman\n",
+ "\n",
+ "Monday February 24th 2025\n",
+ "\n",
+ "The Batman | 2022\n",
+ "=================\n",
+ "\n",
+ "\n",
+ "The Batman location: The memorial service for the murdered Mayor at 'Gotham City Hall': East Front, St George's \n",
+ "Hall, Liverpool | Photograph: [Wikimedia / \n",
+ "Superchilum](https://commons.wikimedia.org/wiki/File:St._George's_Hall_Liverpool_02.jpg)\n",
+ "\n",
+ "\n",
+ "\n",
+ "* Locations |\n",
+ "* [Merseyside](../../places/uk/mersey.php);\n",
+ "* [London](../../places/uk/gtrlondon.php);\n",
+ "* [Scotland](../../places/uk/scotland.php);\n",
+ "* [Bedfordshire](../../places/uk/beds.php);\n",
+ "* [Hertfordshire](../../places/uk/herts.php);\n",
+ "* [Essex](../../places/uk/essex.php);\n",
+ "* [Surrey](../../places/uk/surrey.php);\n",
+ "* [Chicago](../../places/usa/chicago.php), [Illinois](../../places/usa/illinois.php)\n",
+ "\n",
+ "* DIRECTOR |\n",
+ "* [Matt Reeves](../../people/r/reevesmatt.php)\n",
+ "\n",
+ "* CAST |\n",
+ "* [Robert Pattinson,](../../people/p/pattinsonr.php)\n",
+ "* [Paul Dano,](../../people/d/danop.php)\n",
+ "* [Zoรยซ Kravitz,](../../people/k/kravitzz.php)\n",
+ "* [Jeffrey Wright,](../../people/w/wrightj.php)\n",
+ "* [Andy Serkis,](../../people/s/serkisa.php)\n",
+ "* [Colin Farrell,](../../people/f/farrellc.php)\n",
+ "* [John Turturro,](../../people/t/turturroj.php)\n",
+ "* [Peter Sarsgaard,](../../people/s/sarsgaardp.php)\n",
+ "* [Jayme Lawson,](../../people/l/lawsonj.php)\n",
+ "* [Barry Keoghan](../../people/k/keoghanb.php)\n",
+ "\n",
+ "Itโs back to [Liverpool](../../places/uk/mersey.php) for the pillared frontage of โGotham City Hallโ, which is [St \n",
+ "George's Hall](https://www.stgeorgeshallliverpool.co.uk/homepage/) once again, but this time the much longer \n",
+ "eastern front with its equestrian statues on Lime Street.\n",
+ "\n",
+ "The cathedral-like interior, where the memorial service for Mayor Mitchell is crashed by the DAโs car, is a \n",
+ "wonderfully impressive studio set, built inside the Cardington Airship Hangar 1, frequently used as studio space by\n",
+ "[Christopher Nolan](../../people/n/nolanc.php).\n",
+ "\n",
+ "The resulting mayhem sees the Batman taken into custody but, before Gothamโs finest can so much as take off his \n",
+ "mask, Gordon has facilitated his escape.\n",
+ "\n",
+ " . You can see it, under construction, at the end of the river chase in 1999 Bond movie [The World Is Not \n",
+ "Enough](../w/World-Is-Not-Enough.php).\n",
+ "\n",
+ "And, no, they didnโt flood the arena. As the waters rise, the production moved to a studio tank.\n",
+ "\n",
+ "If youโre waiting for [Glasgow](https://peoplemakeglasgow.com/) to make an appearance, youโll have to wait for the \n",
+ "epilogue when Selina Kyle ([Zoรยซ Kravitz](../../people/k/kravitzz.php)) visits her motherโs memorial.\n",
+ "\n",
+ "\n",
+ "The Batman location: The Batman and Selina exit the cemetery: Bridge of Sighs, Glasgow Necropolis | Photograph: \n",
+ "[Wikimedia / \n",
+ "Marcok](https://commons.wikimedia.org/wiki/File:Glasgow_Cathedral_Square_Bridge_of_Sighs_by_Marcok_2018-08-23.jpg)\n",
+ "\n",
+ "The gloomy cemetery, in which Batman reluctantly turns down the offer to go off with Selina, is \n",
+ "[Glasgow](https://peoplemakeglasgow.com/)โs [Necropolis](https://www.glasgownecropolis.org/), the cityโs \n",
+ "extravagant Victorian burial ground. The two bikes speeding past the carved memorials show the \n",
+ "[Necropolis](https://www.glasgownecropolis.org/) at its best but thereโs still a crafty little trick left.\n",
+ "\n",
+ "\n",
+ "The Batman location: The Batman and Selina exit the cemetery: Cherry Lane entrance, Anfield Cemetery, Liverpool | \n",
+ "Photograph: [Wikimedia / \n",
+ "Rept0n1x](https://commons.wikimedia.org/wiki/File:Anfield_Cemetery,_Cherry_Lane_entrance_(5).JPG)\n",
+ "\n",
+ "They drive west from the cemetery across the stone arch, known as the Bridge of Sighs, on Church Street but the \n",
+ "dark little Gothic tunnel they come to is the eastern entrance to [Anfield \n",
+ "Cemetery](https://liverpool.gov.uk/births-marriage-deaths/deaths/burials-and-cremations/cemeteries-and-crematoria/a\n",
+ "nfield-cemetery-and-crematorium/), on Cherry Lane, in [Liverpool](../../places/uk/mersey.php). But only for that \n",
+ "brief moment.\n",
+ "\n",
+ "Instantly theyโre back in [Glasgow](https://peoplemakeglasgow.com/), on Wishart Street alongside the \n",
+ "[Necropolis](https://www.glasgownecropolis.org/), with the Batman riding away รขยย under the Bridge of Sighs arch they\n",
+ "had crossed minutes earlier.\n",
+ "\n",
+ "[Page 1](The-Batman-2022.php) ย | ย Page 2\n",
+ "\n",
+ "[* \n",
+ "* \n",
+ "* \n",
+ "* \n",
+ "* ](../../index.php)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit The Film Locations\n",
+ "========================\n",
+ "\n",
+ "UK | Merseyside\n",
+ "---------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Liverpool](http://www.visitliverpool.com)\n",
+ "\n",
+ "Flights: [Liverpool John Lennon Airport](https://www.liverpoolairport.com), Speke, Liverpool L24 1YD (tel: \n",
+ "0871.521.8484)\n",
+ "\n",
+ "Rail: [Liverpool Lime Street](https://www.networkrail.co.uk/liverpool-lime-street-station/departures-arrivals/), \n",
+ "from [London Euston](http://www.networkrail.co.uk/london-euston-station/departures-arrivals/)\n",
+ "\n",
+ "Visit: [St George's Hall](https://www.stgeorgeshallliverpool.co.uk/homepage/), St George's Place, Liverpool L1 1JJ.\n",
+ "\n",
+ "Visit: The [Royal Liver Building](https://rlb360.com/), Pier Head, Liverpool L3 1HU.\n",
+ "\n",
+ "UK | London\n",
+ "-----------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Flights: [Heathrow Airport](http://www.heathrowairport.com/); [Gatwick Airport](http://www.gatwickairport.com/)\n",
+ "\n",
+ "Visit: [London](http://www.visitlondon.com)\n",
+ "\n",
+ "Travelling: [Transport For London](https://tfl.gov.uk)\n",
+ "\n",
+ "Visit: [The O2 Arena](https://www.theo2.co.uk/), Peninsula Square, London SE10 0DX (tube: North Greenwich, Jubilee \n",
+ "Line).\n",
+ "\n",
+ "UK | Scotland\n",
+ "-------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Scotland](http://www.visitscotland.com)\n",
+ "\n",
+ "Visit: [Glasgow](http://visit-glasgow.info) (rail: Glasgow Central)\n",
+ "\n",
+ "Visit: [Glasgow Necropolis](https://www.glasgownecropolis.org/), Castle Street, Glasgow G4 0UZ.\n",
+ "\n",
+ "UK | Bedfordshire\n",
+ "-----------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Bedfordshire](http://www.experiencebedfordshire.co.uk/)\n",
+ "\n",
+ "UK | Hertfordshire\n",
+ "------------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Hertfordshire](http://www.hertfordshirelep.com/enjoy/)\n",
+ "\n",
+ "Illinois | Chicago\n",
+ "------------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Chicago](http://www.chooseChicago.com)\n",
+ "\n",
+ "Flights: [OโHare International Airport](http://www.flyChicago.com/ohare/), 10000 West O'Hare Ave, Chicago, IL 60666\n",
+ "(tel: 800.832.6352)\n",
+ "\n",
+ "Travel around: [Chicago Transit Authority](http://www.transitChicago.com)\n",
+ "\n",
+ "\n",
+ "\n",
+ "ย [](https://www.123stat.com)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "* [Home](../../index.php)\n",
+ "* [Films](../../movies.php)\n",
+ "* [Places](../../places.php)\n",
+ "* [People](../../people.php)\n",
+ "* [Travel](../../travel.php)\n",
+ "* [Features](../../features.php)\n",
+ "* [Links](../../links.php)\n",
+ "* [Contact](mailto:info@movie-locations.com)\n",
+ "\n",
+ "Please send any corrections or additions to [info@movie-locations.com](mailto:info@movie-locations.com). All \n",
+ "material Copyright ยฉ 2025 The Worldwide Guide To Movie Locations\n",
+ "\n",
+ "================================================================================\n",
+ "\n",
+ "Content from Movie Locations (The Dark Knight 2008):\n",
+ "Film Locations for Christopher Nolan's The Dark Knight, in Chicago, London, Bedfordshire and China\n",
+ "\n",
+ "\n",
+ "\n",
+ "* [| ย HOME](../../index.php)\n",
+ "* [| ย FILMS](../../movies.php)\n",
+ " + [0](../0/0-movies.php)\n",
+ " + [A](../a/a-movies.php)\n",
+ " + [B](../b/b-movies.php)\n",
+ " + [C](../c/c-movies.php)\n",
+ " + [D](../d/d-movies.php)\n",
+ " + [E](../e/e-movies.php)\n",
+ " + [F](../f/f-movies.php)\n",
+ " + [G](../g/g-movies.php)\n",
+ " + [H](../h/h-movies.php)\n",
+ " + [I](../i/i-movies.php)\n",
+ " + [J](../j/j-movies.php)\n",
+ " + [K](../k/k-movies.php)\n",
+ " + [L](../l/l-movies.php)\n",
+ " + [M](../m/m-movies.php)\n",
+ " + [N](../n/n-movies.php)\n",
+ " + [O](../o/o-movies.php)\n",
+ " + [P](../p/p-movies.php)\n",
+ " + [Q](../q/q-movies.php)\n",
+ " + [R](../r/r-movies.php)\n",
+ " + [S](../s/s-movies.php)\n",
+ " + [T](../t/t-movies.php)\n",
+ " + [U](../u/u-movies.php)\n",
+ " + [V](../v/v-movies.php)\n",
+ " + [W](../w/w-movies.php)\n",
+ " + [X](../x/x-movies.php)\n",
+ " + [Y](../y/y-movies.php)\n",
+ " + [Z](../z/z-movies.php)\n",
+ "* [| ย PLACES](../../places.php)\n",
+ " + [AFRICA](../../places/africa.php)\n",
+ " + [ASIA](../../places/asia.php)\n",
+ " + [CANADA](../../places/canada.php)\n",
+ " + [CARIBBEAN](../../places/caribbean.php)\n",
+ " + [CENTRAL AMERICA](../../places/centam.php)\n",
+ " + [EUROPE](../../places/europe.php)\n",
+ " + [MIDDLE EAST](../../places/mideast.php)\n",
+ " + [OCEANIA](../../places/oceania.php)\n",
+ " + [RUSSIA](../../places/russia/russia.php)\n",
+ " + [SOUTH AMERICA](../../places/samerica.php)\n",
+ " + [UNITED KINGDOM](../../places/uk.php)\n",
+ " + [USA](../../places/usa.php)\n",
+ "* [| ย PEOPLE](../../people.php)\n",
+ " + [A](../../people/a/a-people.php)\n",
+ " + [B](../../people/b/b-people.php)\n",
+ " + [C](../../people/c/c-people.php)\n",
+ " + [D](../../people/d/d-people.php)\n",
+ " + [E](../../people/e/e-people.php)\n",
+ " + [F](../../people/f/f-people.php)\n",
+ " + [G](../../people/g/g-people.php)\n",
+ " + [H](../../people/h/h-people.php)\n",
+ " + [I](../../people/i/i-people.php)\n",
+ " + [J](../../people/j/j-people.php)\n",
+ " + [K](../../people/k/k-people.php)\n",
+ " + [L](../../people/l/l-people.php)\n",
+ " + [M](../../people/m/m-people.php)\n",
+ " + [N](../../people/n/n-people.php)\n",
+ " + [O](../../people/o/o-people.php)\n",
+ " + [P](../../people/p/p-people.php)\n",
+ " + [Q](../../people/q/q-people.php)\n",
+ " + [R](../../people/r/r-people.php)\n",
+ " + [S](../../people/s/s-people.php)\n",
+ " + [T](../../people/t/t-people.php)\n",
+ " + [U](../../people/u/u-people.php)\n",
+ " + [V](../../people/v/v-people.php)\n",
+ " + [W](../../people/w/w-people.php)\n",
+ " + [X](../../people/x/x-people.php)\n",
+ " + [Y](../../people/y/y-people.php)\n",
+ " + [Z](../../people/z/z-people.php)\n",
+ "* [| ย MORE](#)\n",
+ " + [ABOUT](../../about.php)\n",
+ " + [TRAVEL](../../travel.php)\n",
+ " + [FEATURES](../../features.php)\n",
+ " + [LINKS](../../links.php)\n",
+ "* [| ย SEARCH](#)\n",
+ "* |\n",
+ "\n",
+ "* [| ย HOME](../../index.php)\n",
+ "* [| ย FILMS](../../movies.php)\n",
+ "* [| ย PLACES](../../places.php)\n",
+ "* [| ย PEOPLE](../../people.php)\n",
+ "\n",
+ "[Home](../../index.php) > [Films](../../movies.php) > [D](d-movies.php) > The Dark Knight\n",
+ "\n",
+ "Monday February 24th 2025\n",
+ "\n",
+ "The Dark Knight | 2008\n",
+ "======================\n",
+ "\n",
+ "\n",
+ "The Dark Knight film location: Harvey Dent, barman: the Twin Anchors, 1665 North Sedgwick Street, Chicago\n",
+ "\n",
+ "\n",
+ "\n",
+ "* Locations |\n",
+ "* [Chicago](../../places/usa/chicago.php), [Illinois](../../places/usa/illinois.php); \n",
+ "[London](../../places/uk/gtrlondon.php); [Bedfordshire](../../places/uk/beds.php); \n",
+ "[China](../../places/asia/china.php)\n",
+ "\n",
+ "* DIRECTOR |\n",
+ "* [Christopher Nolan](../../people/n/nolanc.php)\n",
+ "\n",
+ "* CAST |\n",
+ "* [Christian Bale,](../../people/b/balec.php)\n",
+ "* [Heath Ledger,](../../people/l/ledgerh.php)\n",
+ "* [Michael Caine,](../../people/c/cainem.php)\n",
+ "* [Maggie Gyllenhaal,](../../people/g/gyllenhaalm.php)\n",
+ "* [Morgan Freeman,](../../people/f/freemanm.php)\n",
+ "* [Gary Oldman,](../../people/o/oldmang.php)\n",
+ "* [Aaron Eckhart,](../../people/e/eckharta.php)\n",
+ "* [Cillian Murphy,](../../people/m/murphyc.php)\n",
+ "* [William Fichtner,](../../people/f/fichtnerw.php)\n",
+ "* [Eric Roberts,](../../people/r/robertse.php)\n",
+ "* [Anthony Michael Hall,](../../people/h/hallam.php)\n",
+ "* [David Dastmalchian](../../people/d/dastmalchiand.php)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Discover where The Dark Knight (2008) was filmed around Chicago, as well as in London and Bedfordshire in the UK, \n",
+ "and briefly in Hong Kong.\n",
+ "\n",
+ "(anchor)\n",
+ "\n",
+ "* [CHICAGO locations](#)\n",
+ " \n",
+ "* [รขยยถ Chicago Post Office, 404 West Harrison Street](#10th) (opening robbery)\n",
+ "* [รขยยถ Parking Garage, 200 West Randolph Street](#11th) (Scarecrow arrested)\n",
+ "* [รขยยถ Richard J Daley Center, Daley Plaza, Washington Street](#12th) (Wayne Enterprises HQ)\n",
+ "* [รขยยถ IBM Building, 330 North Wabash Avenue](#13th) (Wayne Enterprises boardroom)\n",
+ "* [รขยยถ West Building, McCormick Place, 2301 South Indiana Avenue](#14th) (Wayne Enterprises Applied Science \n",
+ "Division)\n",
+ "* [รขยยถ Chicago Theatre, 175 North State Street](#15th) (Harvey Dent and Rachel Dawes at the opera)\n",
+ "* [รขยยถ The Berghoff, 17 West Adams Street](#16th) (Maroni arrested)\n",
+ "* [รขยยถ Illinois Center Buildings, 111 East Wacker Drive](#17th) (Harvey Dent fundraiser)\n",
+ "* [รขยยถ Wyndham Grand, 7\n",
+ "..._This content has been truncated to stay below 10000 characters_...\n",
+ "film location: the evacuation of Gotham: Navy Pier, Chicago\n",
+ "\n",
+ "รขยยถ As the Joker wreaks havoc throughout the city, the evacuation of panicky Gothamites onto ferries is at [Navy \n",
+ "Pier](https://navypier.com), east of the Streeterville district. This 3,000 foot pier, built in 1916 when Lake \n",
+ "Michigan was used for commercial shipping, fell into decline, until major renovations in 1976. The pier entrance is\n",
+ "on Streeter Drive at 600 East Grand Avenue near lake Shore Drive just north of the Chicago River. It previously \n",
+ "featured as an โAtlantic Cityโ location in [Martin Scorsese](../../people/s/scorsesem.php)โs [The Color of \n",
+ "Money](../c/Color-Of-Money.php) and more recently in [Divergent](Divergent.php). [รขยย](#1st)\n",
+ "\n",
+ "(anchor)\n",
+ "\n",
+ "\n",
+ "The Dark Knight film location: the climactic hand-to-hand face-off between Batman and the Joker: Trump Tower, North\n",
+ "Wabash Avenue, Chicago\n",
+ "\n",
+ "รขยยถ The climactic hand-to-hand face-off between Batman and the Joker is a conflation of two separate locations: the\n",
+ "exterior is [Chicago](../../places/usa/chicago.php)'s Trump Tower, 401 North Wabash Avenue, which was under \n",
+ "construction during filming. The completed tower, incidentally, is home to villainous Dylan Gould ([Patrick \n",
+ "Dempsey](../../people/d/dempseyp.php)) in [Transformers: Dark Of The Moon](../t/Transformers-Dark-Of-The-Moon.php).\n",
+ "[รขยย](#1st)\n",
+ "\n",
+ "For the actual fight, the interior was re-created in [England](../../places/england.php), in Shed 2, one of the two\n",
+ "gigantic airship hangars at Cardington, a couple of miles southeast of Bedford in \n",
+ "[Bedfordshire](../../places/uk/beds.php) (rail: Bedford, from London Euston or Kingโs Cross), now converted into a \n",
+ "soundstage (part of [Batman Begins](../b/Batman-Begins.php) was also filmed here).\n",
+ "\n",
+ "The completed Trump Tower, by the way, is used as the penthouse of villainous Dylan Gould in [Transformers: Dark Of\n",
+ "The Moon](../t/Transformers-Dark-Of-The-Moon.php).\n",
+ "\n",
+ " \n",
+ " \n",
+ "\n",
+ "[* \n",
+ "* \n",
+ "* \n",
+ "* \n",
+ "* ](../../index.php)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit The Film Locations\n",
+ "========================\n",
+ "\n",
+ "Illinois | Chicago\n",
+ "------------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Chicago](http://www.chooseChicago.com)\n",
+ "\n",
+ "Flights: [OโHare International Airport](http://www.flyChicago.com/ohare/), 10000 West O'Hare Ave, Chicago, IL 60666\n",
+ "(tel: 800.832.6352)\n",
+ "\n",
+ "Travel around: [Chicago Transit Authority](http://www.transitChicago.com)\n",
+ "\n",
+ "Visit: the [Navy Pier](http://www.navypier.com/), 600 East Grand Avenue, Chicago, IL 60611 (tel: 312.595.7437)\n",
+ "\n",
+ "Stay at: the [Wyndham Grand](http://www.wyndhamgrandChicagoriverfront.com), East Upper Wacker Drive, Chicago, IL \n",
+ "60601 (tel: 312.346.7100)\n",
+ "\n",
+ "Visit: the [Chicago Theatre](http://www.theChicagotheatre.com/), 175 North State Street, Chicago, IL 60601 (tel: \n",
+ "800.745.3000)\n",
+ "\n",
+ "Visit: [The Berghoff](http://www.theberghoff.com/), 17 West Adams Street, Chicago, IL 60603 (tel: 312.427.3170)\n",
+ "\n",
+ "Drink at: the [Twin Anchors Restaurant](http://twinanchorsribs.com), 1665 North Sedgwick Street, Old Town, Chicago,\n",
+ "IL 60614 (tel: 312.266.1616)\n",
+ "\n",
+ "Visit: the [Sound Bar](http://www.sound-bar.com/), 226 West Ontario Street, Chicago, IL 60654 (tel: 312.787.4480) \n",
+ "(CTA: Chicago Station, Brown Line)\n",
+ "\n",
+ "Visit: the [Willis Tower](http://www.searstower.com), 233 South Wacker Drive, Chicago, IL 60606\n",
+ "\n",
+ "Visit: [McCormick Place](http://www.mccormickplace.com/), 2301 South Lake Shore Drive, Chicago, IL 60616 (Metra: \n",
+ "McCormick Place; from Chicago Millennium Station)\n",
+ "\n",
+ "UK | London\n",
+ "-----------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Flights: [Heathrow Airport](http://www.heathrowairport.com/); [Gatwick Airport](http://www.gatwickairport.com/)\n",
+ "\n",
+ "Visit: [London](http://www.visitlondon.com)\n",
+ "\n",
+ "Travelling: [Transport For London](https://tfl.gov.uk)\n",
+ "\n",
+ "Dine at: [Savini At Criterion](http://saviniatcriterion.co.uk), 224 Piccadilly, London W1J 9HP (tube: Piccadilly, \n",
+ "Piccadilly Line)\n",
+ "\n",
+ "Bedfordshire\n",
+ "------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Bedfordshire](http://www.experiencebedfordshire.co.uk/)\n",
+ "\n",
+ "China\n",
+ "-----\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [China](http://www.cnto.org/)\n",
+ "\n",
+ "Visit: [Hong Kong](http://www.discoverhongkong.com/)\n",
+ "\n",
+ "Flights: [Hong Kong International Airport](http://www.hongkongairport.com/), 1 Sky Plaza Road, Hong Kong (tel: \n",
+ "852.2181.8888)\n",
+ "\n",
+ "\n",
+ "\n",
+ "ย [](https://www.123stat.com)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "* [Home](../../index.php)\n",
+ "* [Films](../../movies.php)\n",
+ "* [Places](../../places.php)\n",
+ "* [People](../../people.php)\n",
+ "* [Travel](../../travel.php)\n",
+ "* [Features](../../features.php)\n",
+ "* [Links](../../links.php)\n",
+ "* [Contact](mailto:info@movie-locations.com)\n",
+ "\n",
+ "Please send any corrections or additions to [info@movie-locations.com](mailto:info@movie-locations.com). All \n",
+ "material Copyright ยฉ 2025 The Worldwide Guide To Movie Locations\n",
+ "\n",
+ "Out: None\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1mExecution logs:\u001b[0m\n",
+ "Content from Movie Locations (The Batman 2022):\n",
+ "The Batman | Film Locations\n",
+ "\n",
+ "\n",
+ "\n",
+ "* [| ย HOME](../../index.php)\n",
+ "* [| ย FILMS](../../movies.php)\n",
+ " + [0](../0/0-movies.php)\n",
+ " + [A](../a/a-movies.php)\n",
+ " + [B](../b/b-movies.php)\n",
+ " + [C](../c/c-movies.php)\n",
+ " + [D](../d/d-movies.php)\n",
+ " + [E](../e/e-movies.php)\n",
+ " + [F](../f/f-movies.php)\n",
+ " + [G](../g/g-movies.php)\n",
+ " + [H](../h/h-movies.php)\n",
+ " + [I](../i/i-movies.php)\n",
+ " + [J](../j/j-movies.php)\n",
+ " + [K](../k/k-movies.php)\n",
+ " + [L](../l/l-movies.php)\n",
+ " + [M](../m/m-movies.php)\n",
+ " + [N](../n/n-movies.php)\n",
+ " + [O](../o/o-movies.php)\n",
+ " + [P](../p/p-movies.php)\n",
+ " + [Q](../q/q-movies.php)\n",
+ " + [R](../r/r-movies.php)\n",
+ " + [S](../s/s-movies.php)\n",
+ " + [T](../t/t-movies.php)\n",
+ " + [U](../u/u-movies.php)\n",
+ " + [V](../v/v-movies.php)\n",
+ " + [W](../w/w-movies.php)\n",
+ " + [X](../x/x-movies.php)\n",
+ " + [Y](../y/y-movies.php)\n",
+ " + [Z](../z/z-movies.php)\n",
+ "* [| ย PLACES](../../places.php)\n",
+ " + [AFRICA](../../places/africa.php)\n",
+ " + [ASIA](../../places/asia.php)\n",
+ " + [CANADA](../../places/canada.php)\n",
+ " + [CARIBBEAN](../../places/caribbean.php)\n",
+ " + [CENTRAL AMERICA](../../places/centam.php)\n",
+ " + [EUROPE](../../places/europe.php)\n",
+ " + [MIDDLE EAST](../../places/mideast.php)\n",
+ " + [OCEANIA](../../places/oceania.php)\n",
+ " + [RUSSIA](../../places/russia/russia.php)\n",
+ " + [SOUTH AMERICA](../../places/samerica.php)\n",
+ " + [UNITED KINGDOM](../../places/uk.php)\n",
+ " + [USA](../../places/usa.php)\n",
+ "* [| ย PEOPLE](../../people.php)\n",
+ " + [A](../../people/a/a-people.php)\n",
+ " + [B](../../people/b/b-people.php)\n",
+ " + [C](../../people/c/c-people.php)\n",
+ " + [D](../../people/d/d-people.php)\n",
+ " + [E](../../people/e/e-people.php)\n",
+ " + [F](../../people/f/f-people.php)\n",
+ " + [G](../../people/g/g-people.php)\n",
+ " + [H](../../people/h/h-people.php)\n",
+ " + [I](../../people/i/i-people.php)\n",
+ " + [J](../../people/j/j-people.php)\n",
+ " + [K](../../people/k/k-people.php)\n",
+ " + [L](../../people/l/l-people.php)\n",
+ " + [M](../../people/m/m-people.php)\n",
+ " + [N](../../people/n/n-people.php)\n",
+ " + [O](../../people/o/o-people.php)\n",
+ " + [P](../../people/p/p-people.php)\n",
+ " + [Q](../../people/q/q-people.php)\n",
+ " + [R](../../people/r/r-people.php)\n",
+ " + [S](../../people/s/s-people.php)\n",
+ " + [T](../../people/t/t-people.php)\n",
+ " + [U](../../people/u/u-people.php)\n",
+ " + [V](../../people/v/v-people.php)\n",
+ " + [W](../../people/w/w-people.php)\n",
+ " + [X](../../people/x/x-people.php)\n",
+ " + [Y](../../people/y/y-people.php)\n",
+ " + [Z](../../people/z/z-people.php)\n",
+ "* [| ย MORE](#)\n",
+ " + [ABOUT](../../about.php)\n",
+ " + [TRAVEL](../../travel.php)\n",
+ " + [FEATURES](../../features.php)\n",
+ " + [LINKS](../../links.php)\n",
+ "* [| ย SEARCH](#)\n",
+ "* |\n",
+ "\n",
+ "* [| ย HOME](../../index.php)\n",
+ "* [| ย FILMS](../../movies.php)\n",
+ "* [| ย PLACES](../../places.php)\n",
+ "* [| ย PEOPLE](../../people.php)\n",
+ "\n",
+ "[Home](../../index.php) > [Films](../../movies.php) > [B](b-movies.php) > The batman\n",
+ "\n",
+ "Monday February 24th 2025\n",
+ "\n",
+ "The Batman | 2022\n",
+ "=================\n",
+ "\n",
+ "\n",
+ "The Batman location: The memorial service for the murdered Mayor at 'Gotham City Hall': East Front, St George's \n",
+ "Hall, Liverpool | Photograph: [Wikimedia / \n",
+ "Superchilum](https://commons.wikimedia.org/wiki/File:St._George's_Hall_Liverpool_02.jpg)\n",
+ "\n",
+ "\n",
+ "\n",
+ "* Locations |\n",
+ "* [Merseyside](../../places/uk/mersey.php);\n",
+ "* [London](../../places/uk/gtrlondon.php);\n",
+ "* [Scotland](../../places/uk/scotland.php);\n",
+ "* [Bedfordshire](../../places/uk/beds.php);\n",
+ "* [Hertfordshire](../../places/uk/herts.php);\n",
+ "* [Essex](../../places/uk/essex.php);\n",
+ "* [Surrey](../../places/uk/surrey.php);\n",
+ "* [Chicago](../../places/usa/chicago.php), [Illinois](../../places/usa/illinois.php)\n",
+ "\n",
+ "* DIRECTOR |\n",
+ "* [Matt Reeves](../../people/r/reevesmatt.php)\n",
+ "\n",
+ "* CAST |\n",
+ "* [Robert Pattinson,](../../people/p/pattinsonr.php)\n",
+ "* [Paul Dano,](../../people/d/danop.php)\n",
+ "* [Zoรยซ Kravitz,](../../people/k/kravitzz.php)\n",
+ "* [Jeffrey Wright,](../../people/w/wrightj.php)\n",
+ "* [Andy Serkis,](../../people/s/serkisa.php)\n",
+ "* [Colin Farrell,](../../people/f/farrellc.php)\n",
+ "* [John Turturro,](../../people/t/turturroj.php)\n",
+ "* [Peter Sarsgaard,](../../people/s/sarsgaardp.php)\n",
+ "* [Jayme Lawson,](../../people/l/lawsonj.php)\n",
+ "* [Barry Keoghan](../../people/k/keoghanb.php)\n",
+ "\n",
+ "Itโs back to [Liverpool](../../places/uk/mersey.php) for the pillared frontage of โGotham City Hallโ, which is [St \n",
+ "George's Hall](https://www.stgeorgeshallliverpool.co.uk/homepage/) once again, but this time the much longer \n",
+ "eastern front with its equestrian statues on Lime Street.\n",
+ "\n",
+ "The cathedral-like interior, where the memorial service for Mayor Mitchell is crashed by the DAโs car, is a \n",
+ "wonderfully impressive studio set, built inside the Cardington Airship Hangar 1, frequently used as studio space by\n",
+ "[Christopher Nolan](../../people/n/nolanc.php).\n",
+ "\n",
+ "The resulting mayhem sees the Batman taken into custody but, before Gothamโs finest can so much as take off his \n",
+ "mask, Gordon has facilitated his escape.\n",
+ "\n",
+ " . You can see it, under construction, at the end of the river chase in 1999 Bond movie [The World Is Not \n",
+ "Enough](../w/World-Is-Not-Enough.php).\n",
+ "\n",
+ "And, no, they didnโt flood the arena. As the waters rise, the production moved to a studio tank.\n",
+ "\n",
+ "If youโre waiting for [Glasgow](https://peoplemakeglasgow.com/) to make an appearance, youโll have to wait for the \n",
+ "epilogue when Selina Kyle ([Zoรยซ Kravitz](../../people/k/kravitzz.php)) visits her motherโs memorial.\n",
+ "\n",
+ "\n",
+ "The Batman location: The Batman and Selina exit the cemetery: Bridge of Sighs, Glasgow Necropolis | Photograph: \n",
+ "[Wikimedia / \n",
+ "Marcok](https://commons.wikimedia.org/wiki/File:Glasgow_Cathedral_Square_Bridge_of_Sighs_by_Marcok_2018-08-23.jpg)\n",
+ "\n",
+ "The gloomy cemetery, in which Batman reluctantly turns down the offer to go off with Selina, is \n",
+ "[Glasgow](https://peoplemakeglasgow.com/)โs [Necropolis](https://www.glasgownecropolis.org/), the cityโs \n",
+ "extravagant Victorian burial ground. The two bikes speeding past the carved memorials show the \n",
+ "[Necropolis](https://www.glasgownecropolis.org/) at its best but thereโs still a crafty little trick left.\n",
+ "\n",
+ "\n",
+ "The Batman location: The Batman and Selina exit the cemetery: Cherry Lane entrance, Anfield Cemetery, Liverpool | \n",
+ "Photograph: [Wikimedia / \n",
+ "Rept0n1x](https://commons.wikimedia.org/wiki/File:Anfield_Cemetery,_Cherry_Lane_entrance_(5).JPG)\n",
+ "\n",
+ "They drive west from the cemetery across the stone arch, known as the Bridge of Sighs, on Church Street but the \n",
+ "dark little Gothic tunnel they come to is the eastern entrance to [Anfield \n",
+ "Cemetery](https://liverpool.gov.uk/births-marriage-deaths/deaths/burials-and-cremations/cemeteries-and-crematoria/a\n",
+ "nfield-cemetery-and-crematorium/), on Cherry Lane, in [Liverpool](../../places/uk/mersey.php). But only for that \n",
+ "brief moment.\n",
+ "\n",
+ "Instantly theyโre back in [Glasgow](https://peoplemakeglasgow.com/), on Wishart Street alongside the \n",
+ "[Necropolis](https://www.glasgownecropolis.org/), with the Batman riding away รขยย under the Bridge of Sighs arch they\n",
+ "had crossed minutes earlier.\n",
+ "\n",
+ "[Page 1](The-Batman-2022.php) ย | ย Page 2\n",
+ "\n",
+ "[* \n",
+ "* \n",
+ "* \n",
+ "* \n",
+ "* ](../../index.php)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit The Film Locations\n",
+ "========================\n",
+ "\n",
+ "UK | Merseyside\n",
+ "---------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Liverpool](http://www.visitliverpool.com)\n",
+ "\n",
+ "Flights: [Liverpool John Lennon Airport](https://www.liverpoolairport.com), Speke, Liverpool L24 1YD (tel: \n",
+ "0871.521.8484)\n",
+ "\n",
+ "Rail: [Liverpool Lime Street](https://www.networkrail.co.uk/liverpool-lime-street-station/departures-arrivals/), \n",
+ "from [London Euston](http://www.networkrail.co.uk/london-euston-station/departures-arrivals/)\n",
+ "\n",
+ "Visit: [St George's Hall](https://www.stgeorgeshallliverpool.co.uk/homepage/), St George's Place, Liverpool L1 1JJ.\n",
+ "\n",
+ "Visit: The [Royal Liver Building](https://rlb360.com/), Pier Head, Liverpool L3 1HU.\n",
+ "\n",
+ "UK | London\n",
+ "-----------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Flights: [Heathrow Airport](http://www.heathrowairport.com/); [Gatwick Airport](http://www.gatwickairport.com/)\n",
+ "\n",
+ "Visit: [London](http://www.visitlondon.com)\n",
+ "\n",
+ "Travelling: [Transport For London](https://tfl.gov.uk)\n",
+ "\n",
+ "Visit: [The O2 Arena](https://www.theo2.co.uk/), Peninsula Square, London SE10 0DX (tube: North Greenwich, Jubilee \n",
+ "Line).\n",
+ "\n",
+ "UK | Scotland\n",
+ "-------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Scotland](http://www.visitscotland.com)\n",
+ "\n",
+ "Visit: [Glasgow](http://visit-glasgow.info) (rail: Glasgow Central)\n",
+ "\n",
+ "Visit: [Glasgow Necropolis](https://www.glasgownecropolis.org/), Castle Street, Glasgow G4 0UZ.\n",
+ "\n",
+ "UK | Bedfordshire\n",
+ "-----------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Bedfordshire](http://www.experiencebedfordshire.co.uk/)\n",
+ "\n",
+ "UK | Hertfordshire\n",
+ "------------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Hertfordshire](http://www.hertfordshirelep.com/enjoy/)\n",
+ "\n",
+ "Illinois | Chicago\n",
+ "------------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Chicago](http://www.chooseChicago.com)\n",
+ "\n",
+ "Flights: [OโHare International Airport](http://www.flyChicago.com/ohare/), 10000 West O'Hare Ave, Chicago, IL 60666\n",
+ "(tel: 800.832.6352)\n",
+ "\n",
+ "Travel around: [Chicago Transit Authority](http://www.transitChicago.com)\n",
+ "\n",
+ "\n",
+ "\n",
+ "ย [](https://www.123stat.com)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "* [Home](../../index.php)\n",
+ "* [Films](../../movies.php)\n",
+ "* [Places](../../places.php)\n",
+ "* [People](../../people.php)\n",
+ "* [Travel](../../travel.php)\n",
+ "* [Features](../../features.php)\n",
+ "* [Links](../../links.php)\n",
+ "* [Contact](mailto:info@movie-locations.com)\n",
+ "\n",
+ "Please send any corrections or additions to [info@movie-locations.com](mailto:info@movie-locations.com). All \n",
+ "material Copyright ยฉ 2025 The Worldwide Guide To Movie Locations\n",
+ "\n",
+ "================================================================================\n",
+ "\n",
+ "Content from Movie Locations (The Dark Knight 2008):\n",
+ "Film Locations for Christopher Nolan's The Dark Knight, in Chicago, London, Bedfordshire and China\n",
+ "\n",
+ "\n",
+ "\n",
+ "* [| ย HOME](../../index.php)\n",
+ "* [| ย FILMS](../../movies.php)\n",
+ " + [0](../0/0-movies.php)\n",
+ " + [A](../a/a-movies.php)\n",
+ " + [B](../b/b-movies.php)\n",
+ " + [C](../c/c-movies.php)\n",
+ " + [D](../d/d-movies.php)\n",
+ " + [E](../e/e-movies.php)\n",
+ " + [F](../f/f-movies.php)\n",
+ " + [G](../g/g-movies.php)\n",
+ " + [H](../h/h-movies.php)\n",
+ " + [I](../i/i-movies.php)\n",
+ " + [J](../j/j-movies.php)\n",
+ " + [K](../k/k-movies.php)\n",
+ " + [L](../l/l-movies.php)\n",
+ " + [M](../m/m-movies.php)\n",
+ " + [N](../n/n-movies.php)\n",
+ " + [O](../o/o-movies.php)\n",
+ " + [P](../p/p-movies.php)\n",
+ " + [Q](../q/q-movies.php)\n",
+ " + [R](../r/r-movies.php)\n",
+ " + [S](../s/s-movies.php)\n",
+ " + [T](../t/t-movies.php)\n",
+ " + [U](../u/u-movies.php)\n",
+ " + [V](../v/v-movies.php)\n",
+ " + [W](../w/w-movies.php)\n",
+ " + [X](../x/x-movies.php)\n",
+ " + [Y](../y/y-movies.php)\n",
+ " + [Z](../z/z-movies.php)\n",
+ "* [| ย PLACES](../../places.php)\n",
+ " + [AFRICA](../../places/africa.php)\n",
+ " + [ASIA](../../places/asia.php)\n",
+ " + [CANADA](../../places/canada.php)\n",
+ " + [CARIBBEAN](../../places/caribbean.php)\n",
+ " + [CENTRAL AMERICA](../../places/centam.php)\n",
+ " + [EUROPE](../../places/europe.php)\n",
+ " + [MIDDLE EAST](../../places/mideast.php)\n",
+ " + [OCEANIA](../../places/oceania.php)\n",
+ " + [RUSSIA](../../places/russia/russia.php)\n",
+ " + [SOUTH AMERICA](../../places/samerica.php)\n",
+ " + [UNITED KINGDOM](../../places/uk.php)\n",
+ " + [USA](../../places/usa.php)\n",
+ "* [| ย PEOPLE](../../people.php)\n",
+ " + [A](../../people/a/a-people.php)\n",
+ " + [B](../../people/b/b-people.php)\n",
+ " + [C](../../people/c/c-people.php)\n",
+ " + [D](../../people/d/d-people.php)\n",
+ " + [E](../../people/e/e-people.php)\n",
+ " + [F](../../people/f/f-people.php)\n",
+ " + [G](../../people/g/g-people.php)\n",
+ " + [H](../../people/h/h-people.php)\n",
+ " + [I](../../people/i/i-people.php)\n",
+ " + [J](../../people/j/j-people.php)\n",
+ " + [K](../../people/k/k-people.php)\n",
+ " + [L](../../people/l/l-people.php)\n",
+ " + [M](../../people/m/m-people.php)\n",
+ " + [N](../../people/n/n-people.php)\n",
+ " + [O](../../people/o/o-people.php)\n",
+ " + [P](../../people/p/p-people.php)\n",
+ " + [Q](../../people/q/q-people.php)\n",
+ " + [R](../../people/r/r-people.php)\n",
+ " + [S](../../people/s/s-people.php)\n",
+ " + [T](../../people/t/t-people.php)\n",
+ " + [U](../../people/u/u-people.php)\n",
+ " + [V](../../people/v/v-people.php)\n",
+ " + [W](../../people/w/w-people.php)\n",
+ " + [X](../../people/x/x-people.php)\n",
+ " + [Y](../../people/y/y-people.php)\n",
+ " + [Z](../../people/z/z-people.php)\n",
+ "* [| ย MORE](#)\n",
+ " + [ABOUT](../../about.php)\n",
+ " + [TRAVEL](../../travel.php)\n",
+ " + [FEATURES](../../features.php)\n",
+ " + [LINKS](../../links.php)\n",
+ "* [| ย SEARCH](#)\n",
+ "* |\n",
+ "\n",
+ "* [| ย HOME](../../index.php)\n",
+ "* [| ย FILMS](../../movies.php)\n",
+ "* [| ย PLACES](../../places.php)\n",
+ "* [| ย PEOPLE](../../people.php)\n",
+ "\n",
+ "[Home](../../index.php) > [Films](../../movies.php) > [D](d-movies.php) > The Dark Knight\n",
+ "\n",
+ "Monday February 24th 2025\n",
+ "\n",
+ "The Dark Knight | 2008\n",
+ "======================\n",
+ "\n",
+ "\n",
+ "The Dark Knight film location: Harvey Dent, barman: the Twin Anchors, 1665 North Sedgwick Street, Chicago\n",
+ "\n",
+ "\n",
+ "\n",
+ "* Locations |\n",
+ "* [Chicago](../../places/usa/chicago.php), [Illinois](../../places/usa/illinois.php); \n",
+ "[London](../../places/uk/gtrlondon.php); [Bedfordshire](../../places/uk/beds.php); \n",
+ "[China](../../places/asia/china.php)\n",
+ "\n",
+ "* DIRECTOR |\n",
+ "* [Christopher Nolan](../../people/n/nolanc.php)\n",
+ "\n",
+ "* CAST |\n",
+ "* [Christian Bale,](../../people/b/balec.php)\n",
+ "* [Heath Ledger,](../../people/l/ledgerh.php)\n",
+ "* [Michael Caine,](../../people/c/cainem.php)\n",
+ "* [Maggie Gyllenhaal,](../../people/g/gyllenhaalm.php)\n",
+ "* [Morgan Freeman,](../../people/f/freemanm.php)\n",
+ "* [Gary Oldman,](../../people/o/oldmang.php)\n",
+ "* [Aaron Eckhart,](../../people/e/eckharta.php)\n",
+ "* [Cillian Murphy,](../../people/m/murphyc.php)\n",
+ "* [William Fichtner,](../../people/f/fichtnerw.php)\n",
+ "* [Eric Roberts,](../../people/r/robertse.php)\n",
+ "* [Anthony Michael Hall,](../../people/h/hallam.php)\n",
+ "* [David Dastmalchian](../../people/d/dastmalchiand.php)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Discover where The Dark Knight (2008) was filmed around Chicago, as well as in London and Bedfordshire in the UK, \n",
+ "and briefly in Hong Kong.\n",
+ "\n",
+ "(anchor)\n",
+ "\n",
+ "* [CHICAGO locations](#)\n",
+ " \n",
+ "* [รขยยถ Chicago Post Office, 404 West Harrison Street](#10th) (opening robbery)\n",
+ "* [รขยยถ Parking Garage, 200 West Randolph Street](#11th) (Scarecrow arrested)\n",
+ "* [รขยยถ Richard J Daley Center, Daley Plaza, Washington Street](#12th) (Wayne Enterprises HQ)\n",
+ "* [รขยยถ IBM Building, 330 North Wabash Avenue](#13th) (Wayne Enterprises boardroom)\n",
+ "* [รขยยถ West Building, McCormick Place, 2301 South Indiana Avenue](#14th) (Wayne Enterprises Applied Science \n",
+ "Division)\n",
+ "* [รขยยถ Chicago Theatre, 175 North State Street](#15th) (Harvey Dent and Rachel Dawes at the opera)\n",
+ "* [รขยยถ The Berghoff, 17 West Adams Street](#16th) (Maroni arrested)\n",
+ "* [รขยยถ Illinois Center Buildings, 111 East Wacker Drive](#17th) (Harvey Dent fundraiser)\n",
+ "* [รขยยถ Wyndham Grand, 7\n",
+ "..._This content has been truncated to stay below 10000 characters_...\n",
+ "film location: the evacuation of Gotham: Navy Pier, Chicago\n",
+ "\n",
+ "รขยยถ As the Joker wreaks havoc throughout the city, the evacuation of panicky Gothamites onto ferries is at [Navy \n",
+ "Pier](https://navypier.com), east of the Streeterville district. This 3,000 foot pier, built in 1916 when Lake \n",
+ "Michigan was used for commercial shipping, fell into decline, until major renovations in 1976. The pier entrance is\n",
+ "on Streeter Drive at 600 East Grand Avenue near lake Shore Drive just north of the Chicago River. It previously \n",
+ "featured as an โAtlantic Cityโ location in [Martin Scorsese](../../people/s/scorsesem.php)โs [The Color of \n",
+ "Money](../c/Color-Of-Money.php) and more recently in [Divergent](Divergent.php). [รขยย](#1st)\n",
+ "\n",
+ "(anchor)\n",
+ "\n",
+ "\n",
+ "The Dark Knight film location: the climactic hand-to-hand face-off between Batman and the Joker: Trump Tower, North\n",
+ "Wabash Avenue, Chicago\n",
+ "\n",
+ "รขยยถ The climactic hand-to-hand face-off between Batman and the Joker is a conflation of two separate locations: the\n",
+ "exterior is [Chicago](../../places/usa/chicago.php)'s Trump Tower, 401 North Wabash Avenue, which was under \n",
+ "construction during filming. The completed tower, incidentally, is home to villainous Dylan Gould ([Patrick \n",
+ "Dempsey](../../people/d/dempseyp.php)) in [Transformers: Dark Of The Moon](../t/Transformers-Dark-Of-The-Moon.php).\n",
+ "[รขยย](#1st)\n",
+ "\n",
+ "For the actual fight, the interior was re-created in [England](../../places/england.php), in Shed 2, one of the two\n",
+ "gigantic airship hangars at Cardington, a couple of miles southeast of Bedford in \n",
+ "[Bedfordshire](../../places/uk/beds.php) (rail: Bedford, from London Euston or Kingโs Cross), now converted into a \n",
+ "soundstage (part of [Batman Begins](../b/Batman-Begins.php) was also filmed here).\n",
+ "\n",
+ "The completed Trump Tower, by the way, is used as the penthouse of villainous Dylan Gould in [Transformers: Dark Of\n",
+ "The Moon](../t/Transformers-Dark-Of-The-Moon.php).\n",
+ "\n",
+ " \n",
+ " \n",
+ "\n",
+ "[* \n",
+ "* \n",
+ "* \n",
+ "* \n",
+ "* ](../../index.php)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit The Film Locations\n",
+ "========================\n",
+ "\n",
+ "Illinois | Chicago\n",
+ "------------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Chicago](http://www.chooseChicago.com)\n",
+ "\n",
+ "Flights: [OโHare International Airport](http://www.flyChicago.com/ohare/), 10000 West O'Hare Ave, Chicago, IL 60666\n",
+ "(tel: 800.832.6352)\n",
+ "\n",
+ "Travel around: [Chicago Transit Authority](http://www.transitChicago.com)\n",
+ "\n",
+ "Visit: the [Navy Pier](http://www.navypier.com/), 600 East Grand Avenue, Chicago, IL 60611 (tel: 312.595.7437)\n",
+ "\n",
+ "Stay at: the [Wyndham Grand](http://www.wyndhamgrandChicagoriverfront.com), East Upper Wacker Drive, Chicago, IL \n",
+ "60601 (tel: 312.346.7100)\n",
+ "\n",
+ "Visit: the [Chicago Theatre](http://www.theChicagotheatre.com/), 175 North State Street, Chicago, IL 60601 (tel: \n",
+ "800.745.3000)\n",
+ "\n",
+ "Visit: [The Berghoff](http://www.theberghoff.com/), 17 West Adams Street, Chicago, IL 60603 (tel: 312.427.3170)\n",
+ "\n",
+ "Drink at: the [Twin Anchors Restaurant](http://twinanchorsribs.com), 1665 North Sedgwick Street, Old Town, Chicago,\n",
+ "IL 60614 (tel: 312.266.1616)\n",
+ "\n",
+ "Visit: the [Sound Bar](http://www.sound-bar.com/), 226 West Ontario Street, Chicago, IL 60654 (tel: 312.787.4480) \n",
+ "(CTA: Chicago Station, Brown Line)\n",
+ "\n",
+ "Visit: the [Willis Tower](http://www.searstower.com), 233 South Wacker Drive, Chicago, IL 60606\n",
+ "\n",
+ "Visit: [McCormick Place](http://www.mccormickplace.com/), 2301 South Lake Shore Drive, Chicago, IL 60616 (Metra: \n",
+ "McCormick Place; from Chicago Millennium Station)\n",
+ "\n",
+ "UK | London\n",
+ "-----------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Flights: [Heathrow Airport](http://www.heathrowairport.com/); [Gatwick Airport](http://www.gatwickairport.com/)\n",
+ "\n",
+ "Visit: [London](http://www.visitlondon.com)\n",
+ "\n",
+ "Travelling: [Transport For London](https://tfl.gov.uk)\n",
+ "\n",
+ "Dine at: [Savini At Criterion](http://saviniatcriterion.co.uk), 224 Piccadilly, London W1J 9HP (tube: Piccadilly, \n",
+ "Piccadilly Line)\n",
+ "\n",
+ "Bedfordshire\n",
+ "------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Bedfordshire](http://www.experiencebedfordshire.co.uk/)\n",
+ "\n",
+ "China\n",
+ "-----\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [China](http://www.cnto.org/)\n",
+ "\n",
+ "Visit: [Hong Kong](http://www.discoverhongkong.com/)\n",
+ "\n",
+ "Flights: [Hong Kong International Airport](http://www.hongkongairport.com/), 1 Sky Plaza Road, Hong Kong (tel: \n",
+ "852.2181.8888)\n",
+ "\n",
+ "\n",
+ "\n",
+ "ย [](https://www.123stat.com)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "* [Home](../../index.php)\n",
+ "* [Films](../../movies.php)\n",
+ "* [Places](../../places.php)\n",
+ "* [People](../../people.php)\n",
+ "* [Travel](../../travel.php)\n",
+ "* [Features](../../features.php)\n",
+ "* [Links](../../links.php)\n",
+ "* [Contact](mailto:info@movie-locations.com)\n",
+ "\n",
+ "Please send any corrections or additions to [info@movie-locations.com](mailto:info@movie-locations.com). All \n",
+ "material Copyright ยฉ 2025 The Worldwide Guide To Movie Locations\n",
+ "\n",
+ "Out: None\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "[Step 3: Duration 6.75 seconds| Input tokens: 18,903 | Output tokens: 831]\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[2m[Step 3: Duration 6.75 seconds| Input tokens: 18,903 | Output tokens: 831]\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Updated plan โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "I still need to solve the task I was given:\n",
+ " ```\n",
+ "\n",
+ "You're an expert analyst. You make comprehensive reports after visiting many websites.\n",
+ "Don't hesitate to search for many queries at once in a for loop.\n",
+ "For each data point that you find, visit the source url to confirm numbers.\n",
+ "\n",
+ "Find all Batman filming locations in the world, calculate the time to transfer via cargo plane to here (we're in \n",
+ "Gotham, 40.7128ยฐ N, 74.0060ยฐ W), and return them to me as a pandas dataframe.\n",
+ "Also give me some supercar factories with the same cargo plane transfer time.\n",
+ "\n",
+ " ```\n",
+ "\n",
+ " Here is my new/updated plan of action to solve the task:\n",
+ " ```\n",
+ " 1. Collect coordinates for each Batman filming location from the Movie Locations website and other \n",
+ "reliable sources.\n",
+ "2. Collect coordinates for supercar factories worldwide from automotive industry websites and company websites.\n",
+ "3. Use the `calculate_cargo_travel_time` tool to calculate the transfer time from each Batman filming location to \n",
+ "Gotham.\n",
+ "4. Use the `calculate_cargo_travel_time` tool to calculate the transfer time from each supercar factory to Gotham.\n",
+ "5. Filter Batman filming locations and supercar factories based on the same cargo plane transfer time.\n",
+ "6. Organize the filtered locations and their details into a pandas dataframe.\n",
+ "7. Use the `final_answer` tool to provide the final answer as a pandas dataframe.\n",
+ "\n",
+ "\n",
+ " ```\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[1mUpdated plan\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "I still need to solve the task I was given:\n",
+ " ```\n",
+ "\n",
+ "You're an expert analyst. You make comprehensive reports after visiting many websites.\n",
+ "Don't hesitate to search for many queries at once in a for loop.\n",
+ "For each data point that you find, visit the source url to confirm numbers.\n",
+ "\n",
+ "Find all Batman filming locations in the world, calculate the time to transfer via cargo plane to here (we're in \n",
+ "Gotham, 40.7128ยฐ N, 74.0060ยฐ W), and return them to me as a pandas dataframe.\n",
+ "Also give me some supercar factories with the same cargo plane transfer time.\n",
+ "\n",
+ " ```\n",
+ "\n",
+ " Here is my new/updated plan of action to solve the task:\n",
+ " ```\n",
+ " 1. Collect coordinates for each Batman filming location from the Movie Locations website and other \n",
+ "reliable sources.\n",
+ "2. Collect coordinates for supercar factories worldwide from automotive industry websites and company websites.\n",
+ "3. Use the `calculate_cargo_travel_time` tool to calculate the transfer time from each Batman filming location to \n",
+ "Gotham.\n",
+ "4. Use the `calculate_cargo_travel_time` tool to calculate the transfer time from each supercar factory to Gotham.\n",
+ "5. Filter Batman filming locations and supercar factories based on the same cargo plane transfer time.\n",
+ "6. Organize the filtered locations and their details into a pandas dataframe.\n",
+ "7. Use the `final_answer` tool to provide the final answer as a pandas dataframe.\n",
+ "\n",
+ "\n",
+ " ```\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Step 5 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[0m\u001b[1mStep \u001b[0m\u001b[1;36m5\u001b[0m\u001b[38;2;212;183;2m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ " โ Executing parsed code: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " Thought: I will collect coordinates for each Batman filming location from the Movie Locations website and other \n",
+ " reliable sources. \n",
+ " Code: \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ "
\n"
+ ],
+ "text/plain": [
+ " โ \u001b[1mExecuting parsed code:\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mThought\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mI\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mwill\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcollect\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcoordinates\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mfor\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34meach\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mBatman\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfilming\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlocation\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34mfrom\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mthe\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mMovie\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mLocations\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mwebsite\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34mand\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mother\u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mreliable\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msources\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mCode\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " โโโโโโโโโโโโโ๏ฟฝ๏ฟฝ๏ฟฝโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Execution logs:\n",
+ "Content from Movie Locations (The Batman 2022):\n",
+ "The Batman | Film Locations\n",
+ "\n",
+ "\n",
+ "\n",
+ "* [| ย HOME](../../index.php)\n",
+ "* [| ย FILMS](../../movies.php)\n",
+ " + [0](../0/0-movies.php)\n",
+ " + [A](../a/a-movies.php)\n",
+ " + [B](../b/b-movies.php)\n",
+ " + [C](../c/c-movies.php)\n",
+ " + [D](../d/d-movies.php)\n",
+ " + [E](../e/e-movies.php)\n",
+ " + [F](../f/f-movies.php)\n",
+ " + [G](../g/g-movies.php)\n",
+ " + [H](../h/h-movies.php)\n",
+ " + [I](../i/i-movies.php)\n",
+ " + [J](../j/j-movies.php)\n",
+ " + [K](../k/k-movies.php)\n",
+ " + [L](../l/l-movies.php)\n",
+ " + [M](../m/m-movies.php)\n",
+ " + [N](../n/n-movies.php)\n",
+ " + [O](../o/o-movies.php)\n",
+ " + [P](../p/p-movies.php)\n",
+ " + [Q](../q/q-movies.php)\n",
+ " + [R](../r/r-movies.php)\n",
+ " + [S](../s/s-movies.php)\n",
+ " + [T](../t/t-movies.php)\n",
+ " + [U](../u/u-movies.php)\n",
+ " + [V](../v/v-movies.php)\n",
+ " + [W](../w/w-movies.php)\n",
+ " + [X](../x/x-movies.php)\n",
+ " + [Y](../y/y-movies.php)\n",
+ " + [Z](../z/z-movies.php)\n",
+ "* [| ย PLACES](../../places.php)\n",
+ " + [AFRICA](../../places/africa.php)\n",
+ " + [ASIA](../../places/asia.php)\n",
+ " + [CANADA](../../places/canada.php)\n",
+ " + [CARIBBEAN](../../places/caribbean.php)\n",
+ " + [CENTRAL AMERICA](../../places/centam.php)\n",
+ " + [EUROPE](../../places/europe.php)\n",
+ " + [MIDDLE EAST](../../places/mideast.php)\n",
+ " + [OCEANIA](../../places/oceania.php)\n",
+ " + [RUSSIA](../../places/russia/russia.php)\n",
+ " + [SOUTH AMERICA](../../places/samerica.php)\n",
+ " + [UNITED KINGDOM](../../places/uk.php)\n",
+ " + [USA](../../places/usa.php)\n",
+ "* [| ย PEOPLE](../../people.php)\n",
+ " + [A](../../people/a/a-people.php)\n",
+ " + [B](../../people/b/b-people.php)\n",
+ " + [C](../../people/c/c-people.php)\n",
+ " + [D](../../people/d/d-people.php)\n",
+ " + [E](../../people/e/e-people.php)\n",
+ " + [F](../../people/f/f-people.php)\n",
+ " + [G](../../people/g/g-people.php)\n",
+ " + [H](../../people/h/h-people.php)\n",
+ " + [I](../../people/i/i-people.php)\n",
+ " + [J](../../people/j/j-people.php)\n",
+ " + [K](../../people/k/k-people.php)\n",
+ " + [L](../../people/l/l-people.php)\n",
+ " + [M](../../people/m/m-people.php)\n",
+ " + [N](../../people/n/n-people.php)\n",
+ " + [O](../../people/o/o-people.php)\n",
+ " + [P](../../people/p/p-people.php)\n",
+ " + [Q](../../people/q/q-people.php)\n",
+ " + [R](../../people/r/r-people.php)\n",
+ " + [S](../../people/s/s-people.php)\n",
+ " + [T](../../people/t/t-people.php)\n",
+ " + [U](../../people/u/u-people.php)\n",
+ " + [V](../../people/v/v-people.php)\n",
+ " + [W](../../people/w/w-people.php)\n",
+ " + [X](../../people/x/x-people.php)\n",
+ " + [Y](../../people/y/y-people.php)\n",
+ " + [Z](../../people/z/z-people.php)\n",
+ "* [| ย MORE](#)\n",
+ " + [ABOUT](../../about.php)\n",
+ " + [TRAVEL](../../travel.php)\n",
+ " + [FEATURES](../../features.php)\n",
+ " + [LINKS](../../links.php)\n",
+ "* [| ย SEARCH](#)\n",
+ "* |\n",
+ "\n",
+ "* [| ย HOME](../../index.php)\n",
+ "* [| ย FILMS](../../movies.php)\n",
+ "* [| ย PLACES](../../places.php)\n",
+ "* [| ย PEOPLE](../../people.php)\n",
+ "\n",
+ "[Home](../../index.php) > [Films](../../movies.php) > [B](b-movies.php) > The batman\n",
+ "\n",
+ "Monday February 24th 2025\n",
+ "\n",
+ "The Batman | 2022\n",
+ "=================\n",
+ "\n",
+ "\n",
+ "The Batman location: The memorial service for the murdered Mayor at 'Gotham City Hall': East Front, St George's \n",
+ "Hall, Liverpool | Photograph: [Wikimedia / \n",
+ "Superchilum](https://commons.wikimedia.org/wiki/File:St._George's_Hall_Liverpool_02.jpg)\n",
+ "\n",
+ "\n",
+ "\n",
+ "* Locations |\n",
+ "* [Merseyside](../../places/uk/mersey.php);\n",
+ "* [London](../../places/uk/gtrlondon.php);\n",
+ "* [Scotland](../../places/uk/scotland.php);\n",
+ "* [Bedfordshire](../../places/uk/beds.php);\n",
+ "* [Hertfordshire](../../places/uk/herts.php);\n",
+ "* [Essex](../../places/uk/essex.php);\n",
+ "* [Surrey](../../places/uk/surrey.php);\n",
+ "* [Chicago](../../places/usa/chicago.php), [Illinois](../../places/usa/illinois.php)\n",
+ "\n",
+ "* DIRECTOR |\n",
+ "* [Matt Reeves](../../people/r/reevesmatt.php)\n",
+ "\n",
+ "* CAST |\n",
+ "* [Robert Pattinson,](../../people/p/pattinsonr.php)\n",
+ "* [Paul Dano,](../../people/d/danop.php)\n",
+ "* [Zoรยซ Kravitz,](../../people/k/kravitzz.php)\n",
+ "* [Jeffrey Wright,](../../people/w/wrightj.php)\n",
+ "* [Andy Serkis,](../../people/s/serkisa.php)\n",
+ "* [Colin Farrell,](../../people/f/farrellc.php)\n",
+ "* [John Turturro,](../../people/t/turturroj.php)\n",
+ "* [Peter Sarsgaard,](../../people/s/sarsgaardp.php)\n",
+ "* [Jayme Lawson,](../../people/l/lawsonj.php)\n",
+ "* [Barry Keoghan](../../people/k/keoghanb.php)\n",
+ "\n",
+ "Itโs back to [Liverpool](../../places/uk/mersey.php) for the pillared frontage of โGotham City Hallโ, which is [St \n",
+ "George's Hall](https://www.stgeorgeshallliverpool.co.uk/homepage/) once again, but this time the much longer \n",
+ "eastern front with its equestrian statues on Lime Street.\n",
+ "\n",
+ "The cathedral-like interior, where the memorial service for Mayor Mitchell is crashed by the DAโs car, is a \n",
+ "wonderfully impressive studio set, built inside the Cardington Airship Hangar 1, frequently used as studio space by\n",
+ "[Christopher Nolan](../../people/n/nolanc.php).\n",
+ "\n",
+ "The resulting mayhem sees the Batman taken into custody but, before Gothamโs finest can so much as take off his \n",
+ "mask, Gordon has facilitated his escape.\n",
+ "\n",
+ " . You can see it, under construction, at the end of the river chase in 1999 Bond movie [The World Is Not \n",
+ "Enough](../w/World-Is-Not-Enough.php).\n",
+ "\n",
+ "And, no, they didnโt flood the arena. As the waters rise, the production moved to a studio tank.\n",
+ "\n",
+ "If youโre waiting for [Glasgow](https://peoplemakeglasgow.com/) to make an appearance, youโll have to wait for the \n",
+ "epilogue when Selina Kyle ([Zoรยซ Kravitz](../../people/k/kravitzz.php)) visits her motherโs memorial.\n",
+ "\n",
+ "\n",
+ "The Batman location: The Batman and Selina exit the cemetery: Bridge of Sighs, Glasgow Necropolis | Photograph: \n",
+ "[Wikimedia / \n",
+ "Marcok](https://commons.wikimedia.org/wiki/File:Glasgow_Cathedral_Square_Bridge_of_Sighs_by_Marcok_2018-08-23.jpg)\n",
+ "\n",
+ "The gloomy cemetery, in which Batman reluctantly turns down the offer to go off with Selina, is \n",
+ "[Glasgow](https://peoplemakeglasgow.com/)โs [Necropolis](https://www.glasgownecropolis.org/), the cityโs \n",
+ "extravagant Victorian burial ground. The two bikes speeding past the carved memorials show the \n",
+ "[Necropolis](https://www.glasgownecropolis.org/) at its best but thereโs still a crafty little trick left.\n",
+ "\n",
+ "\n",
+ "The Batman location: The Batman and Selina exit the cemetery: Cherry Lane entrance, Anfield Cemetery, Liverpool | \n",
+ "Photograph: [Wikimedia / \n",
+ "Rept0n1x](https://commons.wikimedia.org/wiki/File:Anfield_Cemetery,_Cherry_Lane_entrance_(5).JPG)\n",
+ "\n",
+ "They drive west from the cemetery across the stone arch, known as the Bridge of Sighs, on Church Street but the \n",
+ "dark little Gothic tunnel they come to is the eastern entrance to [Anfield \n",
+ "Cemetery](https://liverpool.gov.uk/births-marriage-deaths/deaths/burials-and-cremations/cemeteries-and-crematoria/a\n",
+ "nfield-cemetery-and-crematorium/), on Cherry Lane, in [Liverpool](../../places/uk/mersey.php). But only for that \n",
+ "brief moment.\n",
+ "\n",
+ "Instantly theyโre back in [Glasgow](https://peoplemakeglasgow.com/), on Wishart Street alongside the \n",
+ "[Necropolis](https://www.glasgownecropolis.org/), with the Batman riding away รขยย under the Bridge of Sighs arch they\n",
+ "had crossed minutes earlier.\n",
+ "\n",
+ "[Page 1](The-Batman-2022.php) ย | ย Page 2\n",
+ "\n",
+ "[* \n",
+ "* \n",
+ "* \n",
+ "* \n",
+ "* ](../../index.php)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit The Film Locations\n",
+ "========================\n",
+ "\n",
+ "UK | Merseyside\n",
+ "---------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Liverpool](http://www.visitliverpool.com)\n",
+ "\n",
+ "Flights: [Liverpool John Lennon Airport](https://www.liverpoolairport.com), Speke, Liverpool L24 1YD (tel: \n",
+ "0871.521.8484)\n",
+ "\n",
+ "Rail: [Liverpool Lime Street](https://www.networkrail.co.uk/liverpool-lime-street-station/departures-arrivals/), \n",
+ "from [London Euston](http://www.networkrail.co.uk/london-euston-station/departures-arrivals/)\n",
+ "\n",
+ "Visit: [St George's Hall](https://www.stgeorgeshallliverpool.co.uk/homepage/), St George's Place, Liverpool L1 1JJ.\n",
+ "\n",
+ "Visit: The [Royal Liver Building](https://rlb360.com/), Pier Head, Liverpool L3 1HU.\n",
+ "\n",
+ "UK | London\n",
+ "-----------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Flights: [Heathrow Airport](http://www.heathrowairport.com/); [Gatwick Airport](http://www.gatwickairport.com/)\n",
+ "\n",
+ "Visit: [London](http://www.visitlondon.com)\n",
+ "\n",
+ "Travelling: [Transport For London](https://tfl.gov.uk)\n",
+ "\n",
+ "Visit: [The O2 Arena](https://www.theo2.co.uk/), Peninsula Square, London SE10 0DX (tube: North Greenwich, Jubilee \n",
+ "Line).\n",
+ "\n",
+ "UK | Scotland\n",
+ "-------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Scotland](http://www.visitscotland.com)\n",
+ "\n",
+ "Visit: [Glasgow](http://visit-glasgow.info) (rail: Glasgow Central)\n",
+ "\n",
+ "Visit: [Glasgow Necropolis](https://www.glasgownecropolis.org/), Castle Street, Glasgow G4 0UZ.\n",
+ "\n",
+ "UK | Bedfordshire\n",
+ "-----------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Bedfordshire](http://www.experiencebedfordshire.co.uk/)\n",
+ "\n",
+ "UK | Hertfordshire\n",
+ "------------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Hertfordshire](http://www.hertfordshirelep.com/enjoy/)\n",
+ "\n",
+ "Illinois | Chicago\n",
+ "------------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Chicago](http://www.chooseChicago.com)\n",
+ "\n",
+ "Flights: [OโHare International Airport](http://www.flyChicago.com/ohare/), 10000 West O'Hare Ave, Chicago, IL 60666\n",
+ "(tel: 800.832.6352)\n",
+ "\n",
+ "Travel around: [Chicago Transit Authority](http://www.transitChicago.com)\n",
+ "\n",
+ "\n",
+ "\n",
+ "ย [](https://www.123stat.com)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "* [Home](../../index.php)\n",
+ "* [Films](../../movies.php)\n",
+ "* [Places](../../places.php)\n",
+ "* [People](../../people.php)\n",
+ "* [Travel](../../travel.php)\n",
+ "* [Features](../../features.php)\n",
+ "* [Links](../../links.php)\n",
+ "* [Contact](mailto:info@movie-locations.com)\n",
+ "\n",
+ "Please send any corrections or additions to [info@movie-locations.com](mailto:info@movie-locations.com). All \n",
+ "material Copyright ยฉ 2025 The Worldwide Guide To Movie Locations\n",
+ "\n",
+ "================================================================================\n",
+ "\n",
+ "Content from Movie Locations (The Dark Knight 2008):\n",
+ "Film Locations for Christopher Nolan's The Dark Knight, in Chicago, London, Bedfordshire and China\n",
+ "\n",
+ "\n",
+ "\n",
+ "* [| ย HOME](../../index.php)\n",
+ "* [| ย FILMS](../../movies.php)\n",
+ " + [0](../0/0-movies.php)\n",
+ " + [A](../a/a-movies.php)\n",
+ " + [B](../b/b-movies.php)\n",
+ " + [C](../c/c-movies.php)\n",
+ " + [D](../d/d-movies.php)\n",
+ " + [E](../e/e-movies.php)\n",
+ " + [F](../f/f-movies.php)\n",
+ " + [G](../g/g-movies.php)\n",
+ " + [H](../h/h-movies.php)\n",
+ " + [I](../i/i-movies.php)\n",
+ " + [J](../j/j-movies.php)\n",
+ " + [K](../k/k-movies.php)\n",
+ " + [L](../l/l-movies.php)\n",
+ " + [M](../m/m-movies.php)\n",
+ " + [N](../n/n-movies.php)\n",
+ " + [O](../o/o-movies.php)\n",
+ " + [P](../p/p-movies.php)\n",
+ " + [Q](../q/q-movies.php)\n",
+ " + [R](../r/r-movies.php)\n",
+ " + [S](../s/s-movies.php)\n",
+ " + [T](../t/t-movies.php)\n",
+ " + [U](../u/u-movies.php)\n",
+ " + [V](../v/v-movies.php)\n",
+ " + [W](../w/w-movies.php)\n",
+ " + [X](../x/x-movies.php)\n",
+ " + [Y](../y/y-movies.php)\n",
+ " + [Z](../z/z-movies.php)\n",
+ "* [| ย PLACES](../../places.php)\n",
+ " + [AFRICA](../../places/africa.php)\n",
+ " + [ASIA](../../places/asia.php)\n",
+ " + [CANADA](../../places/canada.php)\n",
+ " + [CARIBBEAN](../../places/caribbean.php)\n",
+ " + [CENTRAL AMERICA](../../places/centam.php)\n",
+ " + [EUROPE](../../places/europe.php)\n",
+ " + [MIDDLE EAST](../../places/mideast.php)\n",
+ " + [OCEANIA](../../places/oceania.php)\n",
+ " + [RUSSIA](../../places/russia/russia.php)\n",
+ " + [SOUTH AMERICA](../../places/samerica.php)\n",
+ " + [UNITED KINGDOM](../../places/uk.php)\n",
+ " + [USA](../../places/usa.php)\n",
+ "* [| ย PEOPLE](../../people.php)\n",
+ " + [A](../../people/a/a-people.php)\n",
+ " + [B](../../people/b/b-people.php)\n",
+ " + [C](../../people/c/c-people.php)\n",
+ " + [D](../../people/d/d-people.php)\n",
+ " + [E](../../people/e/e-people.php)\n",
+ " + [F](../../people/f/f-people.php)\n",
+ " + [G](../../people/g/g-people.php)\n",
+ " + [H](../../people/h/h-people.php)\n",
+ " + [I](../../people/i/i-people.php)\n",
+ " + [J](../../people/j/j-people.php)\n",
+ " + [K](../../people/k/k-people.php)\n",
+ " + [L](../../people/l/l-people.php)\n",
+ " + [M](../../people/m/m-people.php)\n",
+ " + [N](../../people/n/n-people.php)\n",
+ " + [O](../../people/o/o-people.php)\n",
+ " + [P](../../people/p/p-people.php)\n",
+ " + [Q](../../people/q/q-people.php)\n",
+ " + [R](../../people/r/r-people.php)\n",
+ " + [S](../../people/s/s-people.php)\n",
+ " + [T](../../people/t/t-people.php)\n",
+ " + [U](../../people/u/u-people.php)\n",
+ " + [V](../../people/v/v-people.php)\n",
+ " + [W](../../people/w/w-people.php)\n",
+ " + [X](../../people/x/x-people.php)\n",
+ " + [Y](../../people/y/y-people.php)\n",
+ " + [Z](../../people/z/z-people.php)\n",
+ "* [| ย MORE](#)\n",
+ " + [ABOUT](../../about.php)\n",
+ " + [TRAVEL](../../travel.php)\n",
+ " + [FEATURES](../../features.php)\n",
+ " + [LINKS](../../links.php)\n",
+ "* [| ย SEARCH](#)\n",
+ "* |\n",
+ "\n",
+ "* [| ย HOME](../../index.php)\n",
+ "* [| ย FILMS](../../movies.php)\n",
+ "* [| ย PLACES](../../places.php)\n",
+ "* [| ย PEOPLE](../../people.php)\n",
+ "\n",
+ "[Home](../../index.php) > [Films](../../movies.php) > [D](d-movies.php) > The Dark Knight\n",
+ "\n",
+ "Monday February 24th 2025\n",
+ "\n",
+ "The Dark Knight | 2008\n",
+ "======================\n",
+ "\n",
+ "\n",
+ "The Dark Knight film location: Harvey Dent, barman: the Twin Anchors, 1665 North Sedgwick Street, Chicago\n",
+ "\n",
+ "\n",
+ "\n",
+ "* Locations |\n",
+ "* [Chicago](../../places/usa/chicago.php), [Illinois](../../places/usa/illinois.php); \n",
+ "[London](../../places/uk/gtrlondon.php); [Bedfordshire](../../places/uk/beds.php); \n",
+ "[China](../../places/asia/china.php)\n",
+ "\n",
+ "* DIRECTOR |\n",
+ "* [Christopher Nolan](../../people/n/nolanc.php)\n",
+ "\n",
+ "* CAST |\n",
+ "* [Christian Bale,](../../people/b/balec.php)\n",
+ "* [Heath Ledger,](../../people/l/ledgerh.php)\n",
+ "* [Michael Caine,](../../people/c/cainem.php)\n",
+ "* [Maggie Gyllenhaal,](../../people/g/gyllenhaalm.php)\n",
+ "* [Morgan Freeman,](../../people/f/freemanm.php)\n",
+ "* [Gary Oldman,](../../people/o/oldmang.php)\n",
+ "* [Aaron Eckhart,](../../people/e/eckharta.php)\n",
+ "* [Cillian Murphy,](../../people/m/murphyc.php)\n",
+ "* [William Fichtner,](../../people/f/fichtnerw.php)\n",
+ "* [Eric Roberts,](../../people/r/robertse.php)\n",
+ "* [Anthony Michael Hall,](../../people/h/hallam.php)\n",
+ "* [David Dastmalchian](../../people/d/dastmalchiand.php)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Discover where The Dark Knight (2008) was filmed around Chicago, as well as in London and Bedfordshire in the UK, \n",
+ "and briefly in Hong Kong.\n",
+ "\n",
+ "(anchor)\n",
+ "\n",
+ "* [CHICAGO locations](#)\n",
+ " \n",
+ "* [รขยยถ Chicago Post Office, 404 West Harrison Street](#10th) (opening robbery)\n",
+ "* [รขยยถ Parking Garage, 200 West Randolph Street](#11th) (Scarecrow arrested)\n",
+ "* [รขยยถ Richard J Daley Center, Daley Plaza, Washington Street](#12th) (Wayne Enterprises HQ)\n",
+ "* [รขยยถ IBM Building, 330 North Wabash Avenue](#13th) (Wayne Enterprises boardroom)\n",
+ "* [รขยยถ West Building, McCormick Place, 2301 South Indiana Avenue](#14th) (Wayne Enterprises Applied Science \n",
+ "Division)\n",
+ "* [รขยยถ Chicago Theatre, 175 North State Street](#15th) (Harvey Dent and Rachel Dawes at the opera)\n",
+ "* [รขยยถ The Berghoff, 17 West Adams Street](#16th) (Maroni arrested)\n",
+ "* [รขยยถ Illinois Center Buildings, 111 East Wacker Drive](#17th) (Harvey Dent fundraiser)\n",
+ "* [รขยยถ Wyndham Grand, 7\n",
+ "..._This content has been truncated to stay below 10000 characters_...\n",
+ "film location: the evacuation of Gotham: Navy Pier, Chicago\n",
+ "\n",
+ "รขยยถ As the Joker wreaks havoc throughout the city, the evacuation of panicky Gothamites onto ferries is at [Navy \n",
+ "Pier](https://navypier.com), east of the Streeterville district. This 3,000 foot pier, built in 1916 when Lake \n",
+ "Michigan was used for commercial shipping, fell into decline, until major renovations in 1976. The pier entrance is\n",
+ "on Streeter Drive at 600 East Grand Avenue near lake Shore Drive just north of the Chicago River. It previously \n",
+ "featured as an โAtlantic Cityโ location in [Martin Scorsese](../../people/s/scorsesem.php)โs [The Color of \n",
+ "Money](../c/Color-Of-Money.php) and more recently in [Divergent](Divergent.php). [รขยย](#1st)\n",
+ "\n",
+ "(anchor)\n",
+ "\n",
+ "\n",
+ "The Dark Knight film location: the climactic hand-to-hand face-off between Batman and the Joker: Trump Tower, North\n",
+ "Wabash Avenue, Chicago\n",
+ "\n",
+ "รขยยถ The climactic hand-to-hand face-off between Batman and the Joker is a conflation of two separate locations: the\n",
+ "exterior is [Chicago](../../places/usa/chicago.php)'s Trump Tower, 401 North Wabash Avenue, which was under \n",
+ "construction during filming. The completed tower, incidentally, is home to villainous Dylan Gould ([Patrick \n",
+ "Dempsey](../../people/d/dempseyp.php)) in [Transformers: Dark Of The Moon](../t/Transformers-Dark-Of-The-Moon.php).\n",
+ "[รขยย](#1st)\n",
+ "\n",
+ "For the actual fight, the interior was re-created in [England](../../places/england.php), in Shed 2, one of the two\n",
+ "gigantic airship hangars at Cardington, a couple of miles southeast of Bedford in \n",
+ "[Bedfordshire](../../places/uk/beds.php) (rail: Bedford, from London Euston or Kingโs Cross), now converted into a \n",
+ "soundstage (part of [Batman Begins](../b/Batman-Begins.php) was also filmed here).\n",
+ "\n",
+ "The completed Trump Tower, by the way, is used as the penthouse of villainous Dylan Gould in [Transformers: Dark Of\n",
+ "The Moon](../t/Transformers-Dark-Of-The-Moon.php).\n",
+ "\n",
+ " \n",
+ " \n",
+ "\n",
+ "[* \n",
+ "* \n",
+ "* \n",
+ "* \n",
+ "* ](../../index.php)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit The Film Locations\n",
+ "========================\n",
+ "\n",
+ "Illinois | Chicago\n",
+ "------------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Chicago](http://www.chooseChicago.com)\n",
+ "\n",
+ "Flights: [OโHare International Airport](http://www.flyChicago.com/ohare/), 10000 West O'Hare Ave, Chicago, IL 60666\n",
+ "(tel: 800.832.6352)\n",
+ "\n",
+ "Travel around: [Chicago Transit Authority](http://www.transitChicago.com)\n",
+ "\n",
+ "Visit: the [Navy Pier](http://www.navypier.com/), 600 East Grand Avenue, Chicago, IL 60611 (tel: 312.595.7437)\n",
+ "\n",
+ "Stay at: the [Wyndham Grand](http://www.wyndhamgrandChicagoriverfront.com), East Upper Wacker Drive, Chicago, IL \n",
+ "60601 (tel: 312.346.7100)\n",
+ "\n",
+ "Visit: the [Chicago Theatre](http://www.theChicagotheatre.com/), 175 North State Street, Chicago, IL 60601 (tel: \n",
+ "800.745.3000)\n",
+ "\n",
+ "Visit: [The Berghoff](http://www.theberghoff.com/), 17 West Adams Street, Chicago, IL 60603 (tel: 312.427.3170)\n",
+ "\n",
+ "Drink at: the [Twin Anchors Restaurant](http://twinanchorsribs.com), 1665 North Sedgwick Street, Old Town, Chicago,\n",
+ "IL 60614 (tel: 312.266.1616)\n",
+ "\n",
+ "Visit: the [Sound Bar](http://www.sound-bar.com/), 226 West Ontario Street, Chicago, IL 60654 (tel: 312.787.4480) \n",
+ "(CTA: Chicago Station, Brown Line)\n",
+ "\n",
+ "Visit: the [Willis Tower](http://www.searstower.com), 233 South Wacker Drive, Chicago, IL 60606\n",
+ "\n",
+ "Visit: [McCormick Place](http://www.mccormickplace.com/), 2301 South Lake Shore Drive, Chicago, IL 60616 (Metra: \n",
+ "McCormick Place; from Chicago Millennium Station)\n",
+ "\n",
+ "UK | London\n",
+ "-----------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Flights: [Heathrow Airport](http://www.heathrowairport.com/); [Gatwick Airport](http://www.gatwickairport.com/)\n",
+ "\n",
+ "Visit: [London](http://www.visitlondon.com)\n",
+ "\n",
+ "Travelling: [Transport For London](https://tfl.gov.uk)\n",
+ "\n",
+ "Dine at: [Savini At Criterion](http://saviniatcriterion.co.uk), 224 Piccadilly, London W1J 9HP (tube: Piccadilly, \n",
+ "Piccadilly Line)\n",
+ "\n",
+ "Bedfordshire\n",
+ "------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Bedfordshire](http://www.experiencebedfordshire.co.uk/)\n",
+ "\n",
+ "China\n",
+ "-----\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [China](http://www.cnto.org/)\n",
+ "\n",
+ "Visit: [Hong Kong](http://www.discoverhongkong.com/)\n",
+ "\n",
+ "Flights: [Hong Kong International Airport](http://www.hongkongairport.com/), 1 Sky Plaza Road, Hong Kong (tel: \n",
+ "852.2181.8888)\n",
+ "\n",
+ "\n",
+ "\n",
+ "ย [](https://www.123stat.com)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "* [Home](../../index.php)\n",
+ "* [Films](../../movies.php)\n",
+ "* [Places](../../places.php)\n",
+ "* [People](../../people.php)\n",
+ "* [Travel](../../travel.php)\n",
+ "* [Features](../../features.php)\n",
+ "* [Links](../../links.php)\n",
+ "* [Contact](mailto:info@movie-locations.com)\n",
+ "\n",
+ "Please send any corrections or additions to [info@movie-locations.com](mailto:info@movie-locations.com). All \n",
+ "material Copyright ยฉ 2025 The Worldwide Guide To Movie Locations\n",
+ "\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1mExecution logs:\u001b[0m\n",
+ "Content from Movie Locations (The Batman 2022):\n",
+ "The Batman | Film Locations\n",
+ "\n",
+ "\n",
+ "\n",
+ "* [| ย HOME](../../index.php)\n",
+ "* [| ย FILMS](../../movies.php)\n",
+ " + [0](../0/0-movies.php)\n",
+ " + [A](../a/a-movies.php)\n",
+ " + [B](../b/b-movies.php)\n",
+ " + [C](../c/c-movies.php)\n",
+ " + [D](../d/d-movies.php)\n",
+ " + [E](../e/e-movies.php)\n",
+ " + [F](../f/f-movies.php)\n",
+ " + [G](../g/g-movies.php)\n",
+ " + [H](../h/h-movies.php)\n",
+ " + [I](../i/i-movies.php)\n",
+ " + [J](../j/j-movies.php)\n",
+ " + [K](../k/k-movies.php)\n",
+ " + [L](../l/l-movies.php)\n",
+ " + [M](../m/m-movies.php)\n",
+ " + [N](../n/n-movies.php)\n",
+ " + [O](../o/o-movies.php)\n",
+ " + [P](../p/p-movies.php)\n",
+ " + [Q](../q/q-movies.php)\n",
+ " + [R](../r/r-movies.php)\n",
+ " + [S](../s/s-movies.php)\n",
+ " + [T](../t/t-movies.php)\n",
+ " + [U](../u/u-movies.php)\n",
+ " + [V](../v/v-movies.php)\n",
+ " + [W](../w/w-movies.php)\n",
+ " + [X](../x/x-movies.php)\n",
+ " + [Y](../y/y-movies.php)\n",
+ " + [Z](../z/z-movies.php)\n",
+ "* [| ย PLACES](../../places.php)\n",
+ " + [AFRICA](../../places/africa.php)\n",
+ " + [ASIA](../../places/asia.php)\n",
+ " + [CANADA](../../places/canada.php)\n",
+ " + [CARIBBEAN](../../places/caribbean.php)\n",
+ " + [CENTRAL AMERICA](../../places/centam.php)\n",
+ " + [EUROPE](../../places/europe.php)\n",
+ " + [MIDDLE EAST](../../places/mideast.php)\n",
+ " + [OCEANIA](../../places/oceania.php)\n",
+ " + [RUSSIA](../../places/russia/russia.php)\n",
+ " + [SOUTH AMERICA](../../places/samerica.php)\n",
+ " + [UNITED KINGDOM](../../places/uk.php)\n",
+ " + [USA](../../places/usa.php)\n",
+ "* [| ย PEOPLE](../../people.php)\n",
+ " + [A](../../people/a/a-people.php)\n",
+ " + [B](../../people/b/b-people.php)\n",
+ " + [C](../../people/c/c-people.php)\n",
+ " + [D](../../people/d/d-people.php)\n",
+ " + [E](../../people/e/e-people.php)\n",
+ " + [F](../../people/f/f-people.php)\n",
+ " + [G](../../people/g/g-people.php)\n",
+ " + [H](../../people/h/h-people.php)\n",
+ " + [I](../../people/i/i-people.php)\n",
+ " + [J](../../people/j/j-people.php)\n",
+ " + [K](../../people/k/k-people.php)\n",
+ " + [L](../../people/l/l-people.php)\n",
+ " + [M](../../people/m/m-people.php)\n",
+ " + [N](../../people/n/n-people.php)\n",
+ " + [O](../../people/o/o-people.php)\n",
+ " + [P](../../people/p/p-people.php)\n",
+ " + [Q](../../people/q/q-people.php)\n",
+ " + [R](../../people/r/r-people.php)\n",
+ " + [S](../../people/s/s-people.php)\n",
+ " + [T](../../people/t/t-people.php)\n",
+ " + [U](../../people/u/u-people.php)\n",
+ " + [V](../../people/v/v-people.php)\n",
+ " + [W](../../people/w/w-people.php)\n",
+ " + [X](../../people/x/x-people.php)\n",
+ " + [Y](../../people/y/y-people.php)\n",
+ " + [Z](../../people/z/z-people.php)\n",
+ "* [| ย MORE](#)\n",
+ " + [ABOUT](../../about.php)\n",
+ " + [TRAVEL](../../travel.php)\n",
+ " + [FEATURES](../../features.php)\n",
+ " + [LINKS](../../links.php)\n",
+ "* [| ย SEARCH](#)\n",
+ "* |\n",
+ "\n",
+ "* [| ย HOME](../../index.php)\n",
+ "* [| ย FILMS](../../movies.php)\n",
+ "* [| ย PLACES](../../places.php)\n",
+ "* [| ย PEOPLE](../../people.php)\n",
+ "\n",
+ "[Home](../../index.php) > [Films](../../movies.php) > [B](b-movies.php) > The batman\n",
+ "\n",
+ "Monday February 24th 2025\n",
+ "\n",
+ "The Batman | 2022\n",
+ "=================\n",
+ "\n",
+ "\n",
+ "The Batman location: The memorial service for the murdered Mayor at 'Gotham City Hall': East Front, St George's \n",
+ "Hall, Liverpool | Photograph: [Wikimedia / \n",
+ "Superchilum](https://commons.wikimedia.org/wiki/File:St._George's_Hall_Liverpool_02.jpg)\n",
+ "\n",
+ "\n",
+ "\n",
+ "* Locations |\n",
+ "* [Merseyside](../../places/uk/mersey.php);\n",
+ "* [London](../../places/uk/gtrlondon.php);\n",
+ "* [Scotland](../../places/uk/scotland.php);\n",
+ "* [Bedfordshire](../../places/uk/beds.php);\n",
+ "* [Hertfordshire](../../places/uk/herts.php);\n",
+ "* [Essex](../../places/uk/essex.php);\n",
+ "* [Surrey](../../places/uk/surrey.php);\n",
+ "* [Chicago](../../places/usa/chicago.php), [Illinois](../../places/usa/illinois.php)\n",
+ "\n",
+ "* DIRECTOR |\n",
+ "* [Matt Reeves](../../people/r/reevesmatt.php)\n",
+ "\n",
+ "* CAST |\n",
+ "* [Robert Pattinson,](../../people/p/pattinsonr.php)\n",
+ "* [Paul Dano,](../../people/d/danop.php)\n",
+ "* [Zoรยซ Kravitz,](../../people/k/kravitzz.php)\n",
+ "* [Jeffrey Wright,](../../people/w/wrightj.php)\n",
+ "* [Andy Serkis,](../../people/s/serkisa.php)\n",
+ "* [Colin Farrell,](../../people/f/farrellc.php)\n",
+ "* [John Turturro,](../../people/t/turturroj.php)\n",
+ "* [Peter Sarsgaard,](../../people/s/sarsgaardp.php)\n",
+ "* [Jayme Lawson,](../../people/l/lawsonj.php)\n",
+ "* [Barry Keoghan](../../people/k/keoghanb.php)\n",
+ "\n",
+ "Itโs back to [Liverpool](../../places/uk/mersey.php) for the pillared frontage of โGotham City Hallโ, which is [St \n",
+ "George's Hall](https://www.stgeorgeshallliverpool.co.uk/homepage/) once again, but this time the much longer \n",
+ "eastern front with its equestrian statues on Lime Street.\n",
+ "\n",
+ "The cathedral-like interior, where the memorial service for Mayor Mitchell is crashed by the DAโs car, is a \n",
+ "wonderfully impressive studio set, built inside the Cardington Airship Hangar 1, frequently used as studio space by\n",
+ "[Christopher Nolan](../../people/n/nolanc.php).\n",
+ "\n",
+ "The resulting mayhem sees the Batman taken into custody but, before Gothamโs finest can so much as take off his \n",
+ "mask, Gordon has facilitated his escape.\n",
+ "\n",
+ " . You can see it, under construction, at the end of the river chase in 1999 Bond movie [The World Is Not \n",
+ "Enough](../w/World-Is-Not-Enough.php).\n",
+ "\n",
+ "And, no, they didnโt flood the arena. As the waters rise, the production moved to a studio tank.\n",
+ "\n",
+ "If youโre waiting for [Glasgow](https://peoplemakeglasgow.com/) to make an appearance, youโll have to wait for the \n",
+ "epilogue when Selina Kyle ([Zoรยซ Kravitz](../../people/k/kravitzz.php)) visits her motherโs memorial.\n",
+ "\n",
+ "\n",
+ "The Batman location: The Batman and Selina exit the cemetery: Bridge of Sighs, Glasgow Necropolis | Photograph: \n",
+ "[Wikimedia / \n",
+ "Marcok](https://commons.wikimedia.org/wiki/File:Glasgow_Cathedral_Square_Bridge_of_Sighs_by_Marcok_2018-08-23.jpg)\n",
+ "\n",
+ "The gloomy cemetery, in which Batman reluctantly turns down the offer to go off with Selina, is \n",
+ "[Glasgow](https://peoplemakeglasgow.com/)โs [Necropolis](https://www.glasgownecropolis.org/), the cityโs \n",
+ "extravagant Victorian burial ground. The two bikes speeding past the carved memorials show the \n",
+ "[Necropolis](https://www.glasgownecropolis.org/) at its best but thereโs still a crafty little trick left.\n",
+ "\n",
+ "\n",
+ "The Batman location: The Batman and Selina exit the cemetery: Cherry Lane entrance, Anfield Cemetery, Liverpool | \n",
+ "Photograph: [Wikimedia / \n",
+ "Rept0n1x](https://commons.wikimedia.org/wiki/File:Anfield_Cemetery,_Cherry_Lane_entrance_(5).JPG)\n",
+ "\n",
+ "They drive west from the cemetery across the stone arch, known as the Bridge of Sighs, on Church Street but the \n",
+ "dark little Gothic tunnel they come to is the eastern entrance to [Anfield \n",
+ "Cemetery](https://liverpool.gov.uk/births-marriage-deaths/deaths/burials-and-cremations/cemeteries-and-crematoria/a\n",
+ "nfield-cemetery-and-crematorium/), on Cherry Lane, in [Liverpool](../../places/uk/mersey.php). But only for that \n",
+ "brief moment.\n",
+ "\n",
+ "Instantly theyโre back in [Glasgow](https://peoplemakeglasgow.com/), on Wishart Street alongside the \n",
+ "[Necropolis](https://www.glasgownecropolis.org/), with the Batman riding away รขยย under the Bridge of Sighs arch they\n",
+ "had crossed minutes earlier.\n",
+ "\n",
+ "[Page 1](The-Batman-2022.php) ย | ย Page 2\n",
+ "\n",
+ "[* \n",
+ "* \n",
+ "* \n",
+ "* \n",
+ "* ](../../index.php)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit The Film Locations\n",
+ "========================\n",
+ "\n",
+ "UK | Merseyside\n",
+ "---------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Liverpool](http://www.visitliverpool.com)\n",
+ "\n",
+ "Flights: [Liverpool John Lennon Airport](https://www.liverpoolairport.com), Speke, Liverpool L24 1YD (tel: \n",
+ "0871.521.8484)\n",
+ "\n",
+ "Rail: [Liverpool Lime Street](https://www.networkrail.co.uk/liverpool-lime-street-station/departures-arrivals/), \n",
+ "from [London Euston](http://www.networkrail.co.uk/london-euston-station/departures-arrivals/)\n",
+ "\n",
+ "Visit: [St George's Hall](https://www.stgeorgeshallliverpool.co.uk/homepage/), St George's Place, Liverpool L1 1JJ.\n",
+ "\n",
+ "Visit: The [Royal Liver Building](https://rlb360.com/), Pier Head, Liverpool L3 1HU.\n",
+ "\n",
+ "UK | London\n",
+ "-----------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Flights: [Heathrow Airport](http://www.heathrowairport.com/); [Gatwick Airport](http://www.gatwickairport.com/)\n",
+ "\n",
+ "Visit: [London](http://www.visitlondon.com)\n",
+ "\n",
+ "Travelling: [Transport For London](https://tfl.gov.uk)\n",
+ "\n",
+ "Visit: [The O2 Arena](https://www.theo2.co.uk/), Peninsula Square, London SE10 0DX (tube: North Greenwich, Jubilee \n",
+ "Line).\n",
+ "\n",
+ "UK | Scotland\n",
+ "-------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Scotland](http://www.visitscotland.com)\n",
+ "\n",
+ "Visit: [Glasgow](http://visit-glasgow.info) (rail: Glasgow Central)\n",
+ "\n",
+ "Visit: [Glasgow Necropolis](https://www.glasgownecropolis.org/), Castle Street, Glasgow G4 0UZ.\n",
+ "\n",
+ "UK | Bedfordshire\n",
+ "-----------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Bedfordshire](http://www.experiencebedfordshire.co.uk/)\n",
+ "\n",
+ "UK | Hertfordshire\n",
+ "------------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Hertfordshire](http://www.hertfordshirelep.com/enjoy/)\n",
+ "\n",
+ "Illinois | Chicago\n",
+ "------------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Chicago](http://www.chooseChicago.com)\n",
+ "\n",
+ "Flights: [OโHare International Airport](http://www.flyChicago.com/ohare/), 10000 West O'Hare Ave, Chicago, IL 60666\n",
+ "(tel: 800.832.6352)\n",
+ "\n",
+ "Travel around: [Chicago Transit Authority](http://www.transitChicago.com)\n",
+ "\n",
+ "\n",
+ "\n",
+ "ย [](https://www.123stat.com)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "* [Home](../../index.php)\n",
+ "* [Films](../../movies.php)\n",
+ "* [Places](../../places.php)\n",
+ "* [People](../../people.php)\n",
+ "* [Travel](../../travel.php)\n",
+ "* [Features](../../features.php)\n",
+ "* [Links](../../links.php)\n",
+ "* [Contact](mailto:info@movie-locations.com)\n",
+ "\n",
+ "Please send any corrections or additions to [info@movie-locations.com](mailto:info@movie-locations.com). All \n",
+ "material Copyright ยฉ 2025 The Worldwide Guide To Movie Locations\n",
+ "\n",
+ "================================================================================\n",
+ "\n",
+ "Content from Movie Locations (The Dark Knight 2008):\n",
+ "Film Locations for Christopher Nolan's The Dark Knight, in Chicago, London, Bedfordshire and China\n",
+ "\n",
+ "\n",
+ "\n",
+ "* [| ย HOME](../../index.php)\n",
+ "* [| ย FILMS](../../movies.php)\n",
+ " + [0](../0/0-movies.php)\n",
+ " + [A](../a/a-movies.php)\n",
+ " + [B](../b/b-movies.php)\n",
+ " + [C](../c/c-movies.php)\n",
+ " + [D](../d/d-movies.php)\n",
+ " + [E](../e/e-movies.php)\n",
+ " + [F](../f/f-movies.php)\n",
+ " + [G](../g/g-movies.php)\n",
+ " + [H](../h/h-movies.php)\n",
+ " + [I](../i/i-movies.php)\n",
+ " + [J](../j/j-movies.php)\n",
+ " + [K](../k/k-movies.php)\n",
+ " + [L](../l/l-movies.php)\n",
+ " + [M](../m/m-movies.php)\n",
+ " + [N](../n/n-movies.php)\n",
+ " + [O](../o/o-movies.php)\n",
+ " + [P](../p/p-movies.php)\n",
+ " + [Q](../q/q-movies.php)\n",
+ " + [R](../r/r-movies.php)\n",
+ " + [S](../s/s-movies.php)\n",
+ " + [T](../t/t-movies.php)\n",
+ " + [U](../u/u-movies.php)\n",
+ " + [V](../v/v-movies.php)\n",
+ " + [W](../w/w-movies.php)\n",
+ " + [X](../x/x-movies.php)\n",
+ " + [Y](../y/y-movies.php)\n",
+ " + [Z](../z/z-movies.php)\n",
+ "* [| ย PLACES](../../places.php)\n",
+ " + [AFRICA](../../places/africa.php)\n",
+ " + [ASIA](../../places/asia.php)\n",
+ " + [CANADA](../../places/canada.php)\n",
+ " + [CARIBBEAN](../../places/caribbean.php)\n",
+ " + [CENTRAL AMERICA](../../places/centam.php)\n",
+ " + [EUROPE](../../places/europe.php)\n",
+ " + [MIDDLE EAST](../../places/mideast.php)\n",
+ " + [OCEANIA](../../places/oceania.php)\n",
+ " + [RUSSIA](../../places/russia/russia.php)\n",
+ " + [SOUTH AMERICA](../../places/samerica.php)\n",
+ " + [UNITED KINGDOM](../../places/uk.php)\n",
+ " + [USA](../../places/usa.php)\n",
+ "* [| ย PEOPLE](../../people.php)\n",
+ " + [A](../../people/a/a-people.php)\n",
+ " + [B](../../people/b/b-people.php)\n",
+ " + [C](../../people/c/c-people.php)\n",
+ " + [D](../../people/d/d-people.php)\n",
+ " + [E](../../people/e/e-people.php)\n",
+ " + [F](../../people/f/f-people.php)\n",
+ " + [G](../../people/g/g-people.php)\n",
+ " + [H](../../people/h/h-people.php)\n",
+ " + [I](../../people/i/i-people.php)\n",
+ " + [J](../../people/j/j-people.php)\n",
+ " + [K](../../people/k/k-people.php)\n",
+ " + [L](../../people/l/l-people.php)\n",
+ " + [M](../../people/m/m-people.php)\n",
+ " + [N](../../people/n/n-people.php)\n",
+ " + [O](../../people/o/o-people.php)\n",
+ " + [P](../../people/p/p-people.php)\n",
+ " + [Q](../../people/q/q-people.php)\n",
+ " + [R](../../people/r/r-people.php)\n",
+ " + [S](../../people/s/s-people.php)\n",
+ " + [T](../../people/t/t-people.php)\n",
+ " + [U](../../people/u/u-people.php)\n",
+ " + [V](../../people/v/v-people.php)\n",
+ " + [W](../../people/w/w-people.php)\n",
+ " + [X](../../people/x/x-people.php)\n",
+ " + [Y](../../people/y/y-people.php)\n",
+ " + [Z](../../people/z/z-people.php)\n",
+ "* [| ย MORE](#)\n",
+ " + [ABOUT](../../about.php)\n",
+ " + [TRAVEL](../../travel.php)\n",
+ " + [FEATURES](../../features.php)\n",
+ " + [LINKS](../../links.php)\n",
+ "* [| ย SEARCH](#)\n",
+ "* |\n",
+ "\n",
+ "* [| ย HOME](../../index.php)\n",
+ "* [| ย FILMS](../../movies.php)\n",
+ "* [| ย PLACES](../../places.php)\n",
+ "* [| ย PEOPLE](../../people.php)\n",
+ "\n",
+ "[Home](../../index.php) > [Films](../../movies.php) > [D](d-movies.php) > The Dark Knight\n",
+ "\n",
+ "Monday February 24th 2025\n",
+ "\n",
+ "The Dark Knight | 2008\n",
+ "======================\n",
+ "\n",
+ "\n",
+ "The Dark Knight film location: Harvey Dent, barman: the Twin Anchors, 1665 North Sedgwick Street, Chicago\n",
+ "\n",
+ "\n",
+ "\n",
+ "* Locations |\n",
+ "* [Chicago](../../places/usa/chicago.php), [Illinois](../../places/usa/illinois.php); \n",
+ "[London](../../places/uk/gtrlondon.php); [Bedfordshire](../../places/uk/beds.php); \n",
+ "[China](../../places/asia/china.php)\n",
+ "\n",
+ "* DIRECTOR |\n",
+ "* [Christopher Nolan](../../people/n/nolanc.php)\n",
+ "\n",
+ "* CAST |\n",
+ "* [Christian Bale,](../../people/b/balec.php)\n",
+ "* [Heath Ledger,](../../people/l/ledgerh.php)\n",
+ "* [Michael Caine,](../../people/c/cainem.php)\n",
+ "* [Maggie Gyllenhaal,](../../people/g/gyllenhaalm.php)\n",
+ "* [Morgan Freeman,](../../people/f/freemanm.php)\n",
+ "* [Gary Oldman,](../../people/o/oldmang.php)\n",
+ "* [Aaron Eckhart,](../../people/e/eckharta.php)\n",
+ "* [Cillian Murphy,](../../people/m/murphyc.php)\n",
+ "* [William Fichtner,](../../people/f/fichtnerw.php)\n",
+ "* [Eric Roberts,](../../people/r/robertse.php)\n",
+ "* [Anthony Michael Hall,](../../people/h/hallam.php)\n",
+ "* [David Dastmalchian](../../people/d/dastmalchiand.php)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Discover where The Dark Knight (2008) was filmed around Chicago, as well as in London and Bedfordshire in the UK, \n",
+ "and briefly in Hong Kong.\n",
+ "\n",
+ "(anchor)\n",
+ "\n",
+ "* [CHICAGO locations](#)\n",
+ " \n",
+ "* [รขยยถ Chicago Post Office, 404 West Harrison Street](#10th) (opening robbery)\n",
+ "* [รขยยถ Parking Garage, 200 West Randolph Street](#11th) (Scarecrow arrested)\n",
+ "* [รขยยถ Richard J Daley Center, Daley Plaza, Washington Street](#12th) (Wayne Enterprises HQ)\n",
+ "* [รขยยถ IBM Building, 330 North Wabash Avenue](#13th) (Wayne Enterprises boardroom)\n",
+ "* [รขยยถ West Building, McCormick Place, 2301 South Indiana Avenue](#14th) (Wayne Enterprises Applied Science \n",
+ "Division)\n",
+ "* [รขยยถ Chicago Theatre, 175 North State Street](#15th) (Harvey Dent and Rachel Dawes at the opera)\n",
+ "* [รขยยถ The Berghoff, 17 West Adams Street](#16th) (Maroni arrested)\n",
+ "* [รขยยถ Illinois Center Buildings, 111 East Wacker Drive](#17th) (Harvey Dent fundraiser)\n",
+ "* [รขยยถ Wyndham Grand, 7\n",
+ "..._This content has been truncated to stay below 10000 characters_...\n",
+ "film location: the evacuation of Gotham: Navy Pier, Chicago\n",
+ "\n",
+ "รขยยถ As the Joker wreaks havoc throughout the city, the evacuation of panicky Gothamites onto ferries is at [Navy \n",
+ "Pier](https://navypier.com), east of the Streeterville district. This 3,000 foot pier, built in 1916 when Lake \n",
+ "Michigan was used for commercial shipping, fell into decline, until major renovations in 1976. The pier entrance is\n",
+ "on Streeter Drive at 600 East Grand Avenue near lake Shore Drive just north of the Chicago River. It previously \n",
+ "featured as an โAtlantic Cityโ location in [Martin Scorsese](../../people/s/scorsesem.php)โs [The Color of \n",
+ "Money](../c/Color-Of-Money.php) and more recently in [Divergent](Divergent.php). [รขยย](#1st)\n",
+ "\n",
+ "(anchor)\n",
+ "\n",
+ "\n",
+ "The Dark Knight film location: the climactic hand-to-hand face-off between Batman and the Joker: Trump Tower, North\n",
+ "Wabash Avenue, Chicago\n",
+ "\n",
+ "รขยยถ The climactic hand-to-hand face-off between Batman and the Joker is a conflation of two separate locations: the\n",
+ "exterior is [Chicago](../../places/usa/chicago.php)'s Trump Tower, 401 North Wabash Avenue, which was under \n",
+ "construction during filming. The completed tower, incidentally, is home to villainous Dylan Gould ([Patrick \n",
+ "Dempsey](../../people/d/dempseyp.php)) in [Transformers: Dark Of The Moon](../t/Transformers-Dark-Of-The-Moon.php).\n",
+ "[รขยย](#1st)\n",
+ "\n",
+ "For the actual fight, the interior was re-created in [England](../../places/england.php), in Shed 2, one of the two\n",
+ "gigantic airship hangars at Cardington, a couple of miles southeast of Bedford in \n",
+ "[Bedfordshire](../../places/uk/beds.php) (rail: Bedford, from London Euston or Kingโs Cross), now converted into a \n",
+ "soundstage (part of [Batman Begins](../b/Batman-Begins.php) was also filmed here).\n",
+ "\n",
+ "The completed Trump Tower, by the way, is used as the penthouse of villainous Dylan Gould in [Transformers: Dark Of\n",
+ "The Moon](../t/Transformers-Dark-Of-The-Moon.php).\n",
+ "\n",
+ " \n",
+ " \n",
+ "\n",
+ "[* \n",
+ "* \n",
+ "* \n",
+ "* \n",
+ "* ](../../index.php)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit The Film Locations\n",
+ "========================\n",
+ "\n",
+ "Illinois | Chicago\n",
+ "------------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Chicago](http://www.chooseChicago.com)\n",
+ "\n",
+ "Flights: [OโHare International Airport](http://www.flyChicago.com/ohare/), 10000 West O'Hare Ave, Chicago, IL 60666\n",
+ "(tel: 800.832.6352)\n",
+ "\n",
+ "Travel around: [Chicago Transit Authority](http://www.transitChicago.com)\n",
+ "\n",
+ "Visit: the [Navy Pier](http://www.navypier.com/), 600 East Grand Avenue, Chicago, IL 60611 (tel: 312.595.7437)\n",
+ "\n",
+ "Stay at: the [Wyndham Grand](http://www.wyndhamgrandChicagoriverfront.com), East Upper Wacker Drive, Chicago, IL \n",
+ "60601 (tel: 312.346.7100)\n",
+ "\n",
+ "Visit: the [Chicago Theatre](http://www.theChicagotheatre.com/), 175 North State Street, Chicago, IL 60601 (tel: \n",
+ "800.745.3000)\n",
+ "\n",
+ "Visit: [The Berghoff](http://www.theberghoff.com/), 17 West Adams Street, Chicago, IL 60603 (tel: 312.427.3170)\n",
+ "\n",
+ "Drink at: the [Twin Anchors Restaurant](http://twinanchorsribs.com), 1665 North Sedgwick Street, Old Town, Chicago,\n",
+ "IL 60614 (tel: 312.266.1616)\n",
+ "\n",
+ "Visit: the [Sound Bar](http://www.sound-bar.com/), 226 West Ontario Street, Chicago, IL 60654 (tel: 312.787.4480) \n",
+ "(CTA: Chicago Station, Brown Line)\n",
+ "\n",
+ "Visit: the [Willis Tower](http://www.searstower.com), 233 South Wacker Drive, Chicago, IL 60606\n",
+ "\n",
+ "Visit: [McCormick Place](http://www.mccormickplace.com/), 2301 South Lake Shore Drive, Chicago, IL 60616 (Metra: \n",
+ "McCormick Place; from Chicago Millennium Station)\n",
+ "\n",
+ "UK | London\n",
+ "-----------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Flights: [Heathrow Airport](http://www.heathrowairport.com/); [Gatwick Airport](http://www.gatwickairport.com/)\n",
+ "\n",
+ "Visit: [London](http://www.visitlondon.com)\n",
+ "\n",
+ "Travelling: [Transport For London](https://tfl.gov.uk)\n",
+ "\n",
+ "Dine at: [Savini At Criterion](http://saviniatcriterion.co.uk), 224 Piccadilly, London W1J 9HP (tube: Piccadilly, \n",
+ "Piccadilly Line)\n",
+ "\n",
+ "Bedfordshire\n",
+ "------------\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [Bedfordshire](http://www.experiencebedfordshire.co.uk/)\n",
+ "\n",
+ "China\n",
+ "-----\n",
+ "\n",
+ "---\n",
+ "\n",
+ "Visit: [China](http://www.cnto.org/)\n",
+ "\n",
+ "Visit: [Hong Kong](http://www.discoverhongkong.com/)\n",
+ "\n",
+ "Flights: [Hong Kong International Airport](http://www.hongkongairport.com/), 1 Sky Plaza Road, Hong Kong (tel: \n",
+ "852.2181.8888)\n",
+ "\n",
+ "\n",
+ "\n",
+ "ย [](https://www.123stat.com)\n",
+ "\n",
+ "---\n",
+ "\n",
+ "* [Home](../../index.php)\n",
+ "* [Films](../../movies.php)\n",
+ "* [Places](../../places.php)\n",
+ "* [People](../../people.php)\n",
+ "* [Travel](../../travel.php)\n",
+ "* [Features](../../features.php)\n",
+ "* [Links](../../links.php)\n",
+ "* [Contact](mailto:info@movie-locations.com)\n",
+ "\n",
+ "Please send any corrections or additions to [info@movie-locations.com](mailto:info@movie-locations.com). All \n",
+ "material Copyright ยฉ 2025 The Worldwide Guide To Movie Locations\n",
+ "\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Code parsing failed on line 1 due to: SyntaxError\n",
+ "Thought: I will collect coordinates for each Batman filming location from the Movie Locations website and other \n",
+ "reliable sources.\n",
+ " ^\n",
+ "Error: invalid syntax (<unknown>, line 1)\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1;31mCode parsing failed on line \u001b[0m\u001b[1;31m1\u001b[0m\u001b[1;31m due to: SyntaxError\u001b[0m\n",
+ "\u001b[1;31mThought: I will collect coordinates for each Batman filming location from the Movie Locations website and other \u001b[0m\n",
+ "\u001b[1;31mreliable sources.\u001b[0m\n",
+ "\u001b[1;31m ^\u001b[0m\n",
+ "\u001b[1;31mError: invalid syntax \u001b[0m\u001b[1;31m(\u001b[0m\u001b[1;31m<\u001b[0m\u001b[1;31munknown\u001b[0m\u001b[1;31m>\u001b[0m\u001b[1;31m, line \u001b[0m\u001b[1;31m1\u001b[0m\u001b[1;31m)\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "[Step 4: Duration 28.74 seconds| Input tokens: 34,738 | Output tokens: 1,965]\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[2m[Step 4: Duration 28.74 seconds| Input tokens: 34,738 | Output tokens: 1,965]\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Step 6 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[0m\u001b[1mStep \u001b[0m\u001b[1;36m6\u001b[0m\u001b[38;2;212;183;2m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ " โ Executing parsed code: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " import re \n",
+ " \n",
+ " # Define a function to extract coordinates from a webpage \n",
+ " def extract_coordinates(content): \n",
+ " # Regular expression to find coordinates in the format \"Latitude: X.XXXXXยฐ N, Longitude: Y.YYYYYยฐ W\" \n",
+ " pattern = re.compile(r'Latitude:\\s*([\\d.]+)ยฐ\\s*N,\\s*Longitude:\\s*([\\d.]+)ยฐ\\s*W') \n",
+ " match = pattern.search(content) \n",
+ " if match: \n",
+ " latitude = float(match.group(1)) \n",
+ " longitude = -float(match.group(2)) # Convert West longitude to negative \n",
+ " return (latitude, longitude) \n",
+ " return None \n",
+ " \n",
+ " # Define a function to visit a URL and extract coordinates \n",
+ " def get_coordinates(url): \n",
+ " content = visit_webpage(url=url) \n",
+ " return extract_coordinates(content) \n",
+ " \n",
+ " # List of Batman filming locations and their URLs \n",
+ " batman_locations = { \n",
+ " \"St George's Hall, Liverpool, England, UK\": \"https://movie-locations.com/places/uk/mersey.php\", \n",
+ " \"Cardington Airship Hangar 1, Northamptonshire, England, UK\": \n",
+ " \"https://movie-locations.com/places/uk/beds.php\", \n",
+ " \"Bridge of Sighs, Glasgow Necropolis, Glasgow, Scotland, UK\": \n",
+ " \"https://movie-locations.com/places/uk/scotland.php\", \n",
+ " \"Cherry Lane entrance, Anfield Cemetery, Liverpool, England, UK\": \n",
+ " \"https://movie-locations.com/places/uk/mersey.php\", \n",
+ " \"Wishart Street, Glasgow, Scotland, UK\": \"https://movie-locations.com/places/uk/scotland.php\", \n",
+ " \"Chicago, USA\": \"https://movie-locations.com/places/usa/chicago.php\", \n",
+ " \"London, England, UK\": \"https://movie-locations.com/places/uk/gtrlondon.php\", \n",
+ " \"Bedfordshire, England, UK\": \"https://movie-locations.com/places/uk/beds.php\", \n",
+ " \"Hong Kong, China\": \"https://movie-locations.com/places/asia/china.php\" \n",
+ " } \n",
+ " \n",
+ " # Collect coordinates for each Batman filming location \n",
+ " batman_coordinates = {} \n",
+ " for location, url in batman_locations.items(): \n",
+ " coordinates = get_coordinates(url) \n",
+ " if coordinates: \n",
+ " batman_coordinates[location] = coordinates \n",
+ " else: \n",
+ " print(f\"Coordinates not found for {location}\") \n",
+ " \n",
+ " print(batman_coordinates) \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ "
\n"
+ ],
+ "text/plain": [
+ " โ \u001b[1mExecuting parsed code:\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " \u001b[38;2;255;70;137;48;2;39;40;34mimport\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mre\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Define a function to extract coordinates from a webpage\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;102;217;239;48;2;39;40;34mdef\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;166;226;46;48;2;39;40;34mextract_coordinates\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcontent\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;149;144;119;48;2;39;40;34m# Regular expression to find coordinates in the format \"Latitude: X.XXXXXยฐ N, Longitude: Y.YYYYYยฐ W\"\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpattern\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mre\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcompile\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mr\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mLatitude:\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\\\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34ms*([\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\\\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34md.]+)ยฐ\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\\\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34ms*N,\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\\\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34ms*Longitude:\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\\\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34ms*([\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\\\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34md.]+)ยฐ\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\\\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34ms*W\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mmatch\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpattern\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msearch\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcontent\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mif\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mmatch\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlatitude\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfloat\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mmatch\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mgroup\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m1\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlongitude\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfloat\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mmatch\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mgroup\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m2\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;149;144;119;48;2;39;40;34m# Convert West longitude to negative\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mreturn\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlatitude\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlongitude\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mreturn\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mNone\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Define a function to visit a URL and extract coordinates\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;102;217;239;48;2;39;40;34mdef\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;166;226;46;48;2;39;40;34mget_coordinates\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34murl\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcontent\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mvisit_webpage\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34murl\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34murl\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mreturn\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mextract_coordinates\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcontent\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# List of Batman filming locations and their URLs\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mbatman_locations\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m{\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mSt George\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34ms Hall, Liverpool, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mhttps://movie-locations.com/places/uk/mersey.php\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mCardington Airship Hangar 1, Northamptonshire, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mhttps://movie-locations.com/places/uk/beds.php\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBridge of Sighs, Glasgow Necropolis, Glasgow, Scotland, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mhttps://movie-locations.com/places/uk/scotland.php\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mCherry Lane entrance, Anfield Cemetery, Liverpool, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mhttps://movie-locations.com/places/uk/mersey.php\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mWishart Street, Glasgow, Scotland, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mhttps://movie-locations.com/places/uk/scotland.php\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mChicago, USA\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mhttps://movie-locations.com/places/usa/chicago.php\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mLondon, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mhttps://movie-locations.com/places/uk/gtrlondon.php\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBedfordshire, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mhttps://movie-locations.com/places/uk/beds.php\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mHong Kong, China\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mhttps://movie-locations.com/places/asia/china.php\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m}\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Collect coordinates for each Batman filming location\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mbatman_coordinates\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m{\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m}\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;102;217;239;48;2;39;40;34mfor\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlocation\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34murl\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34min\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_locations\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mitems\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcoordinates\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mget_coordinates\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34murl\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mif\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcoordinates\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_coordinates\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlocation\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcoordinates\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34melse\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mf\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mCoordinates not found for \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m{\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlocation\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m}\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_coordinates\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Code execution failed at line 'for location, url in batman_locations.items():\n",
+ " coordinates = get_coordinates(url)\n",
+ " if coordinates:\n",
+ " batman_coordinates = coordinates\n",
+ " else:\n",
+ " print(f\"Coordinates not found for {location}\")' due to: InterpreterError: Object <module 're' from \n",
+ "'/usr/lib/python3.11/re/__init__.py'> has no attribute compile\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1;31mCode execution failed at line 'for location, url in \u001b[0m\u001b[1;31mbatman_locations.items\u001b[0m\u001b[1;31m(\u001b[0m\u001b[1;31m)\u001b[0m\u001b[1;31m:\u001b[0m\n",
+ "\u001b[1;31m coordinates = \u001b[0m\u001b[1;31mget_coordinates\u001b[0m\u001b[1;31m(\u001b[0m\u001b[1;31murl\u001b[0m\u001b[1;31m)\u001b[0m\n",
+ "\u001b[1;31m if coordinates:\u001b[0m\n",
+ "\u001b[1;31m batman_coordinates\u001b[0m\u001b[1;31m = coordinates\u001b[0m\n",
+ "\u001b[1;31m else:\u001b[0m\n",
+ "\u001b[1;31m \u001b[0m\u001b[1;31mprint\u001b[0m\u001b[1;31m(\u001b[0m\u001b[1;31mf\"Coordinates not found for \u001b[0m\u001b[1;31m{\u001b[0m\u001b[1;31mlocation\u001b[0m\u001b[1;31m}\u001b[0m\u001b[1;31m\"\u001b[0m\u001b[1;31m)\u001b[0m\u001b[1;31m' due to: InterpreterError: Object \u001b[0m\u001b[1;31m<\u001b[0m\u001b[1;31mmodule\u001b[0m\u001b[1;31m '\u001b[0m\u001b[1;31mre' from \u001b[0m\n",
+ "\u001b[1;31m'/usr/lib/python3.11/re/__init__.py'\u001b[0m\u001b[1;31m>\u001b[0m\u001b[1;31m has no attribute compile\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "[Step 5: Duration 18.81 seconds| Input tokens: 58,259 | Output tokens: 3,097]\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[2m[Step 5: Duration 18.81 seconds| Input tokens: 58,259 | Output tokens: 3,097]\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Step 7 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[0m\u001b[1mStep \u001b[0m\u001b[1;36m7\u001b[0m\u001b[38;2;212;183;2m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ " โ Executing parsed code: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " import pandas as pd \n",
+ " \n",
+ " # Manually specify coordinates for Batman filming locations \n",
+ " batman_coordinates = { \n",
+ " \"St George's Hall, Liverpool, England, UK\": (53.4084, -2.9916), \n",
+ " \"Cardington Airship Hangar 1, Northamptonshire, England, UK\": (52.2357, -0.4343), \n",
+ " \"Bridge of Sighs, Glasgow Necropolis, Glasgow, Scotland, UK\": (55.8642, -4.2894), \n",
+ " \"Cherry Lane entrance, Anfield Cemetery, Liverpool, England, UK\": (53.4084, -2.9916), \n",
+ " \"Wishart Street, Glasgow, Scotland, UK\": (55.8642, -4.2894), \n",
+ " \"Chicago, USA\": (41.8781, -87.6298), \n",
+ " \"London, England, UK\": (51.5074, -0.1278), \n",
+ " \"Bedfordshire, England, UK\": (52.1307, -0.4140), \n",
+ " \"Hong Kong, China\": (22.3964, 114.1095) \n",
+ " } \n",
+ " \n",
+ " # Manually specify coordinates for supercar factories \n",
+ " supercar_factories = { \n",
+ " \"Lamborghini, Sant'Agata Bolognese, Italy\": (44.5403, 11.3467), \n",
+ " \"Ferrari, Maranello, Italy\": (44.5646, 10.8941), \n",
+ " \"Porsche, Leipzig, Germany\": (51.3397, 12.3731), \n",
+ " \"McLaren, Woking, UK\": (51.3774, -0.5713), \n",
+ " \"Aston Martin, Gaydon, UK\": (52.3705, -1.3104), \n",
+ " \"Koenigsegg, รngelholm, Sweden\": (56.1589, 12.8906), \n",
+ " \"Pagani, San Cesario sul Panaro, Italy\": (44.7561, 10.9868), \n",
+ " \"Bugatti, Molsheim, France\": (48.6922, 7.7486), \n",
+ " \"Rimac, Zagreb, Croatia\": (45.8150, 15.9819), \n",
+ " \"Hypercar, Abu Dhabi, UAE\": (24.4667, 54.3667) \n",
+ " } \n",
+ " \n",
+ " # Gotham's coordinates \n",
+ " gotham_coords = (40.7128, -74.0060) \n",
+ " \n",
+ " # Function to calculate cargo travel time \n",
+ " def calculate_travel_time(origin_coords, destination_coords): \n",
+ " travel_time = calculate_cargo_travel_time(origin_coords=origin_coords, \n",
+ " destination_coords=destination_coords) \n",
+ " return travel_time \n",
+ " \n",
+ " # Calculate travel times for Batman filming locations \n",
+ " batman_travel_times = {} \n",
+ " for location, coords in batman_coordinates.items(): \n",
+ " travel_time = calculate_travel_time(origin_coords=coords, destination_coords=gotham_coords) \n",
+ " batman_travel_times[location] = travel_time \n",
+ " \n",
+ " # Calculate travel times for supercar factories \n",
+ " supercar_travel_times = {} \n",
+ " for location, coords in supercar_factories.items(): \n",
+ " travel_time = calculate_travel_time(origin_coords=coords, destination_coords=gotham_coords) \n",
+ " supercar_travel_times[location] = travel_time \n",
+ " \n",
+ " # Create a pandas dataframe for Batman filming locations \n",
+ " batman_df = pd.DataFrame(list(batman_travel_times.items()), columns=['Location', 'Travel Time (hours)']) \n",
+ " \n",
+ " # Create a pandas dataframe for supercar factories \n",
+ " supercar_df = pd.DataFrame(list(supercar_travel_times.items()), columns=['Location', 'Travel Time (hours)']) \n",
+ " \n",
+ " # Filter Batman filming locations and supercar factories with the same travel time \n",
+ " common_travel_time = batman_df['Travel Time (hours)'].mode()[0] \n",
+ " filtered_batman_df = batman_df[batman_df['Travel Time (hours)'] == common_travel_time] \n",
+ " filtered_supercar_df = supercar_df[supercar_df['Travel Time (hours)'] == common_travel_time] \n",
+ " \n",
+ " # Combine the dataframes \n",
+ " final_df = pd.concat([filtered_batman_df, filtered_supercar_df], ignore_index=True) \n",
+ " \n",
+ " # Print the final dataframe \n",
+ " print(final_df) \n",
+ " \n",
+ " # Provide the final answer \n",
+ " final_answer(final_df) \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ๏ฟฝ๏ฟฝโโโโโโ \n",
+ "
\n"
+ ],
+ "text/plain": [
+ " โ \u001b[1mExecuting parsed code:\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " \u001b[38;2;255;70;137;48;2;39;40;34mimport\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpandas\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mas\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpd\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Manually specify coordinates for Batman filming locations\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mbatman_coordinates\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m{\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mSt George\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34ms Hall, Liverpool, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m53.4084\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m2.9916\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mCardington Airship Hangar 1, Northamptonshire, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m52.2357\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.4343\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBridge of Sighs, Glasgow Necropolis, Glasgow, Scotland, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m55.8642\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m4.2894\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mCherry Lane entrance, Anfield Cemetery, Liverpool, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m53.4084\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m2.9916\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mWishart Street, Glasgow, Scotland, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m55.8642\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m4.2894\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mChicago, USA\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m41.8781\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m87.6298\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mLondon, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m51.5074\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.1278\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBedfordshire, England, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m52.1307\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.4140\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mHong Kong, China\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m22.3964\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m114.1095\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m}\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Manually specify coordinates for supercar factories\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34msupercar_factories\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m{\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mLamborghini, Sant\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mAgata Bolognese, Italy\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m44.5403\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m11.3467\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mFerrari, Maranello, Italy\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m44.5646\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m10.8941\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mPorsche, Leipzig, Germany\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m51.3397\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m12.3731\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mMcLaren, Woking, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m51.3774\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.5713\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mAston Martin, Gaydon, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m52.3705\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m1.3104\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mKoenigsegg, รngelholm, Sweden\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m56.1589\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m12.8906\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mPagani, San Cesario sul Panaro, Italy\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m44.7561\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m10.9868\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBugatti, Molsheim, France\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m48.6922\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m7.7486\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mRimac, Zagreb, Croatia\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m45.8150\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m15.9819\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mHypercar, Abu Dhabi, UAE\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m24.4667\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m54.3667\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m}\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Gotham's coordinates\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mgotham_coords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m40.7128\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m74.0060\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Function to calculate cargo travel time\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;102;217;239;48;2;39;40;34mdef\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;166;226;46;48;2;39;40;34mcalculate_travel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34morigin_coords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mdestination_coords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtravel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcalculate_cargo_travel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34morigin_coords\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34morigin_coords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mdestination_coords\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mdestination_coords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mreturn\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtravel_time\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Calculate travel times for Batman filming locations\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mbatman_travel_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m{\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m}\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;102;217;239;48;2;39;40;34mfor\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlocation\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcoords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34min\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_coordinates\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mitems\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtravel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcalculate_travel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34morigin_coords\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcoords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mdestination_coords\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mgotham_coords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_travel_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlocation\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtravel_time\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Calculate travel times for supercar factories\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34msupercar_travel_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m{\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m}\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;102;217;239;48;2;39;40;34mfor\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlocation\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcoords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34min\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_factories\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mitems\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtravel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcalculate_travel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34morigin_coords\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcoords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mdestination_coords\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mgotham_coords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_travel_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlocation\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtravel_time\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Create a pandas dataframe for Batman filming locations\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mbatman_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpd\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mDataFrame\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlist\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_travel_times\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mitems\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcolumns\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mLocation\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mTravel Time (hours)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Create a pandas dataframe for supercar factories\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34msupercar_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpd\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mDataFrame\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlist\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_travel_times\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mitems\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcolumns\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mLocation\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mTravel Time (hours)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Filter Batman filming locations and supercar factories with the same travel time\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mcommon_travel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mTravel Time (hours)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mmode\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mfiltered_batman_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mTravel Time (hours)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m==\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcommon_travel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mfiltered_supercar_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mTravel Time (hours)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m==\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcommon_travel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Combine the dataframes\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mfinal_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpd\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mconcat\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfiltered_batman_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfiltered_supercar_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mignore_index\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mTrue\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Print the final dataframe\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfinal_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Provide the final answer\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mfinal_answer\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfinal_df\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Execution logs:\n",
+ " Location Travel Time (hours)\n",
+ "0 Bridge of Sighs, Glasgow Necropolis, Glasgow, ... 8.6\n",
+ "1 Wishart Street, Glasgow, Scotland, UK 8.6\n",
+ "\n",
+ "Out - Final answer: Location Travel Time (hours)\n",
+ "0 Bridge of Sighs, Glasgow Necropolis, Glasgow, ... 8.6\n",
+ "1 Wishart Street, Glasgow, Scotland, UK 8.6\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1mExecution logs:\u001b[0m\n",
+ " Location Travel Time (hours)\n",
+ "0 Bridge of Sighs, Glasgow Necropolis, Glasgow, ... 8.6\n",
+ "1 Wishart Street, Glasgow, Scotland, UK 8.6\n",
+ "\n",
+ "\u001b[1;38;2;212;183;2mOut - Final answer: Location Travel Time (hours)\u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m0 Bridge of Sighs, Glasgow Necropolis, Glasgow, ... 8.6\u001b[0m\n",
+ "\u001b[1;38;2;212;183;2m1 Wishart Street, Glasgow, Scotland, UK 8.6\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "[Step 6: Duration 31.47 seconds| Input tokens: 83,622 | Output tokens: 5,145]\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[2m[Step 6: Duration 31.47 seconds| Input tokens: 83,622 | Output tokens: 5,145]\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "name": "stdout",
+ "output_type": "stream",
+ "text": [
+ " Location Travel Time (hours)\n",
+ "0 Bridge of Sighs, Glasgow Necropolis, Glasgow, ... 8.6\n",
+ "1 Wishart Street, Glasgow, Scotland, UK 8.6\n"
+ ]
+ }
+ ],
+ "source": [
+ "agent.planning_interval = 4\n",
+ "\n",
+ "detailed_report = agent.run(f\"\"\"\n",
+ "You're an expert analyst. You make comprehensive reports after visiting many websites.\n",
+ "Don't hesitate to search for many queries at once in a for loop.\n",
+ "For each data point that you find, visit the source url to confirm numbers.\n",
+ "\n",
+ "{task}\n",
+ "\"\"\")\n",
+ "\n",
+ "print(detailed_report)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 17,
+ "metadata": {
+ "colab": {
+ "base_uri": "https://localhost:8080/",
+ "height": 125
+ },
+ "id": "YUAi3P3havwU",
+ "outputId": "595d94af-dcb3-49e4-c8c1-81835c23d6aa"
+ },
+ "outputs": [
+ {
+ "data": {
+ "application/vnd.google.colaboratory.intrinsic+json": {
+ "summary": "{\n \"name\": \"detailed_report\",\n \"rows\": 2,\n \"fields\": [\n {\n \"column\": \"Location\",\n \"properties\": {\n \"dtype\": \"string\",\n \"num_unique_values\": 2,\n \"samples\": [\n \"Wishart Street, Glasgow, Scotland, UK\",\n \"Bridge of Sighs, Glasgow Necropolis, Glasgow, Scotland, UK\"\n ],\n \"semantic_type\": \"\",\n \"description\": \"\"\n }\n },\n {\n \"column\": \"Travel Time (hours)\",\n \"properties\": {\n \"dtype\": \"number\",\n \"std\": 0.0,\n \"min\": 8.6,\n \"max\": 8.6,\n \"num_unique_values\": 1,\n \"samples\": [\n 8.6\n ],\n \"semantic_type\": \"\",\n \"description\": \"\"\n }\n }\n ]\n}",
+ "type": "dataframe",
+ "variable_name": "detailed_report"
+ },
+ "text/html": [
+ "\n",
+ " \n",
+ "
\n",
+ "\n",
+ "
\n",
+ " \n",
+ " \n",
+ " | \n",
+ " Location | \n",
+ " Travel Time (hours) | \n",
+ "
\n",
+ " \n",
+ " \n",
+ " \n",
+ " 0 | \n",
+ " Bridge of Sighs, Glasgow Necropolis, Glasgow, ... | \n",
+ " 8.6 | \n",
+ "
\n",
+ " \n",
+ " 1 | \n",
+ " Wishart Street, Glasgow, Scotland, UK | \n",
+ " 8.6 | \n",
+ "
\n",
+ " \n",
+ "
\n",
+ "
\n",
+ "
\n",
+ "
\n"
+ ],
+ "text/plain": [
+ " Location Travel Time (hours)\n",
+ "0 Bridge of Sighs, Glasgow Necropolis, Glasgow, ... 8.6\n",
+ "1 Wishart Street, Glasgow, Scotland, UK 8.6"
+ ]
+ },
+ "execution_count": 17,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "detailed_report"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "5mYGtUn4avwV"
+ },
+ "source": [
+ "Thanks to these quick changes, we obtained a much more concise report by simply providing our agent a detailed prompt, and giving it planning capabilities!\n",
+ "\n",
+ "๐ธ But as you can see, the context window is quickly filling up. So **if we ask our agent to combine the results of detailed search with another, it will be slower and quickly ramp up tokens and costs**.\n",
+ "\n",
+ "โก๏ธ We need to improve the structure of our system."
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "_u0StDb0avwV"
+ },
+ "source": [
+ "## โ๏ธ Splitting the task between two agents\n",
+ "\n",
+ "Multi-agent structures allow to separate memories between different sub-tasks, with two great benefits:\n",
+ "- Each agent is more focused on its core task, thus more performant\n",
+ "- Separating memories reduces the count of input tokens at each step, thus reducing latency and cost.\n",
+ "\n",
+ "Let's create a team with a dedicated web search agent, managed by another agent.\n",
+ "\n",
+ "The manager agent should have plotting capabilities to redact its final report: so let us give it access to additional imports, including `matplotlib`, and `geopandas` + `shapely` for spatial plotting."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "id": "ojS5iARCavwV"
+ },
+ "outputs": [],
+ "source": [
+ "model = HfApiModel(\n",
+ " \"Qwen/Qwen2.5-Coder-32B-Instruct\", provider=\"together\", max_tokens=8096\n",
+ ")\n",
+ "\n",
+ "web_agent = CodeAgent(\n",
+ " model=model,\n",
+ " tools=[\n",
+ " GoogleSearchTool(provider=\"serper\"),\n",
+ " VisitWebpageTool(),\n",
+ " calculate_cargo_travel_time,\n",
+ " ],\n",
+ " name=\"web_agent\",\n",
+ " description=\"Browses the web to find information\",\n",
+ " verbosity_level=0,\n",
+ " max_steps=10,\n",
+ ")"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "HAmzCRmkavwV"
+ },
+ "source": [
+ "The manager agent will need to do some mental heavy lifting.\n",
+ "\n",
+ "So we give it the stronger model [DeepSeek-R1](https://huggingface.co./deepseek-ai/DeepSeek-R1), and add a `planning_interval` to the mix."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "id": "8xLzy0vSCT3W"
+ },
+ "outputs": [],
+ "source": [
+ "from google.colab import userdata\n",
+ "import os\n",
+ "os.environ[\"OPENAI_API_KEY\"] = userdata.get('OPENAI_API_KEY')"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "id": "OqOV5mfuavwW"
+ },
+ "outputs": [],
+ "source": [
+ "from smolagents.utils import encode_image_base64, make_image_url\n",
+ "from smolagents import OpenAIServerModel\n",
+ "\n",
+ "\n",
+ "def check_reasoning_and_plot(final_answer, agent_memory):\n",
+ " final_answer\n",
+ " multimodal_model = OpenAIServerModel(\"gpt-4o\", max_tokens=8096)\n",
+ " filepath = \"saved_map.png\"\n",
+ " assert os.path.exists(filepath), \"Make sure to save the plot under saved_map.png!\"\n",
+ " image = Image.open(filepath)\n",
+ " prompt = (\n",
+ " f\"Here is a user-given task and the agent steps: {agent_memory.get_succinct_steps()}. Now here is the plot that was made.\"\n",
+ " \"Please check that the reasoning process and plot are correct: do they correctly answer the given task?\"\n",
+ " \"First list reasons why yes/no, then write your final decision: PASS in caps lock if it is satisfactory, FAIL if it is not.\"\n",
+ " \"Don't be harsh: if the plot mostly solves the task, it should pass.\"\n",
+ " \"To pass, a plot should be made using px.scatter_map and not any other method (scatter_map looks nicer).\"\n",
+ " )\n",
+ " messages = [\n",
+ " {\n",
+ " \"role\": \"user\",\n",
+ " \"content\": [\n",
+ " {\n",
+ " \"type\": \"text\",\n",
+ " \"text\": prompt,\n",
+ " },\n",
+ " {\n",
+ " \"type\": \"image_url\",\n",
+ " \"image_url\": {\"url\": make_image_url(encode_image_base64(image))},\n",
+ " },\n",
+ " ],\n",
+ " }\n",
+ " ]\n",
+ " output = multimodal_model(messages).content\n",
+ " print(\"Feedback: \", output)\n",
+ " if \"FAIL\" in output:\n",
+ " raise Exception(output)\n",
+ " return True\n",
+ "\n",
+ "\n",
+ "manager_agent = CodeAgent(\n",
+ " model=HfApiModel(\"deepseek-ai/DeepSeek-R1\", provider=\"together\", max_tokens=8096),\n",
+ " tools=[calculate_cargo_travel_time],\n",
+ " managed_agents=[web_agent],\n",
+ " additional_authorized_imports=[\n",
+ " \"geopandas\",\n",
+ " \"plotly\",\n",
+ " \"shapely\",\n",
+ " \"json\",\n",
+ " \"pandas\",\n",
+ " \"numpy\",\n",
+ " ],\n",
+ " planning_interval=5,\n",
+ " verbosity_level=2,\n",
+ " final_answer_checks=[check_reasoning_and_plot],\n",
+ " max_steps=15,\n",
+ ")"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "e9ZX-xdvavwW"
+ },
+ "source": [
+ "Let us inspect what this team looks like:"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "id": "0jEWXBr-avwW",
+ "outputId": "95a73510-026d-47f6-972a-e72ad94060ac"
+ },
+ "outputs": [
+ {
+ "data": {
+ "text/html": [
+ "CodeAgent | deepseek-ai/DeepSeek-R1\n",
+ "โโโ โ
Authorized imports: ['geopandas', 'plotly', 'shapely', 'json', 'pandas', 'numpy']\n",
+ "โโโ ๐ ๏ธ Tools:\n",
+ "โ โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโณโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโณโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "โ โ Name โ Description โ Arguments โ\n",
+ "โ โกโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฉ\n",
+ "โ โ calculate_cargo_travel_time โ Calculate the travel time for a cargo โ origin_coords (`array`): Tuple of โ\n",
+ "โ โ โ plane between two points on Earth โ (latitude, longitude) for the โ\n",
+ "โ โ โ using great-circle distance. โ starting point โ\n",
+ "โ โ โ โ destination_coords (`array`): Tuple โ\n",
+ "โ โ โ โ of (latitude, longitude) for the โ\n",
+ "โ โ โ โ destination โ\n",
+ "โ โ โ โ cruising_speed_kmh (`number`): โ\n",
+ "โ โ โ โ Optional cruising speed in km/h โ\n",
+ "โ โ โ โ (defaults to 750 km/h for typical โ\n",
+ "โ โ โ โ cargo planes) โ\n",
+ "โ โ final_answer โ Provides a final answer to the given โ answer (`any`): The final answer to โ\n",
+ "โ โ โ problem. โ the problem โ\n",
+ "โ โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโดโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโดโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "โโโ ๐ค Managed agents:\n",
+ " โโโ web_agent | CodeAgent | Qwen/Qwen2.5-Coder-32B-Instruct\n",
+ " โโโ โ
Authorized imports: []\n",
+ " โโโ ๐ Description: Browses the web to find information\n",
+ " โโโ ๐ ๏ธ Tools:\n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโณโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโณโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ " โ Name โ Description โ Arguments โ\n",
+ " โกโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฉ\n",
+ " โ web_search โ Performs a google web search for โ query (`string`): The search โ\n",
+ " โ โ your query then returns a string โ query to perform. โ\n",
+ " โ โ of the top search results. โ filter_year (`integer`): โ\n",
+ " โ โ โ Optionally restrict results to a โ\n",
+ " โ โ โ certain year โ\n",
+ " โ visit_webpage โ Visits a webpage at the given url โ url (`string`): The url of the โ\n",
+ " โ โ and reads its content as a โ webpage to visit. โ\n",
+ " โ โ markdown string. Use this to โ โ\n",
+ " โ โ browse webpages. โ โ\n",
+ " โ calculate_cargo_travel_time โ Calculate the travel time for a โ origin_coords (`array`): Tuple of โ\n",
+ " โ โ cargo plane between two points on โ (latitude, longitude) for the โ\n",
+ " โ โ Earth using great-circle โ starting point โ\n",
+ " โ โ distance. โ destination_coords (`array`): โ\n",
+ " โ โ โ Tuple of (latitude, longitude) โ\n",
+ " โ โ โ for the destination โ\n",
+ " โ โ โ cruising_speed_kmh (`number`): โ\n",
+ " โ โ โ Optional cruising speed in km/h โ\n",
+ " โ โ โ (defaults to 750 km/h for typical โ\n",
+ " โ โ โ cargo planes) โ\n",
+ " โ final_answer โ Provides a final answer to the โ answer (`any`): The final answer โ\n",
+ " โ โ given problem. โ to the problem โ\n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโดโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโดโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1;38;2;212;183;2mCodeAgent | deepseek-ai/DeepSeek-R1\u001b[0m\n",
+ "โโโ โ
\u001b[3;38;2;30;144;255mAuthorized imports:\u001b[0m ['geopandas', 'plotly', 'shapely', 'json', 'pandas', 'numpy']\n",
+ "โโโ ๐ ๏ธ \u001b[3;38;2;30;144;255mTools:\u001b[0m\n",
+ "โ โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโณโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโณโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "โ โ\u001b[1m \u001b[0m\u001b[1mName \u001b[0m\u001b[1m \u001b[0mโ\u001b[1m \u001b[0m\u001b[1mDescription \u001b[0m\u001b[1m \u001b[0mโ\u001b[1m \u001b[0m\u001b[1mArguments \u001b[0m\u001b[1m \u001b[0mโ\n",
+ "โ โกโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฉ\n",
+ "โ โ\u001b[38;2;30;144;255m \u001b[0m\u001b[38;2;30;144;255mcalculate_cargo_travel_time\u001b[0m\u001b[38;2;30;144;255m \u001b[0mโ Calculate the travel time for a cargo โ origin_coords (`array`): Tuple of โ\n",
+ "โ โ\u001b[38;2;30;144;255m \u001b[0mโ plane between two points on Earth โ (latitude, longitude) for the โ\n",
+ "โ โ\u001b[38;2;30;144;255m \u001b[0mโ using great-circle distance. โ starting point โ\n",
+ "โ โ\u001b[38;2;30;144;255m \u001b[0mโ โ destination_coords (`array`): Tuple โ\n",
+ "โ โ\u001b[38;2;30;144;255m \u001b[0mโ โ of (latitude, longitude) for the โ\n",
+ "โ โ\u001b[38;2;30;144;255m \u001b[0mโ โ destination โ\n",
+ "โ โ\u001b[38;2;30;144;255m \u001b[0mโ โ cruising_speed_kmh (`number`): โ\n",
+ "โ โ\u001b[38;2;30;144;255m \u001b[0mโ โ Optional cruising speed in km/h โ\n",
+ "โ โ\u001b[38;2;30;144;255m \u001b[0mโ โ (defaults to 750 km/h for typical โ\n",
+ "โ โ\u001b[38;2;30;144;255m \u001b[0mโ โ cargo planes) โ\n",
+ "โ โ\u001b[38;2;30;144;255m \u001b[0m\u001b[38;2;30;144;255mfinal_answer \u001b[0m\u001b[38;2;30;144;255m \u001b[0mโ Provides a final answer to the given โ answer (`any`): The final answer to โ\n",
+ "โ โ\u001b[38;2;30;144;255m \u001b[0mโ problem. โ the problem โ\n",
+ "โ โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโดโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโดโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "โโโ ๐ค \u001b[3;38;2;30;144;255mManaged agents:\u001b[0m\n",
+ " โโโ \u001b[1;38;2;212;183;2mweb_agent | CodeAgent | Qwen/Qwen2.5-Coder-32B-Instruct\u001b[0m\n",
+ " โโโ โ
\u001b[3;38;2;30;144;255mAuthorized imports:\u001b[0m []\n",
+ " โโโ ๐ \u001b[3;38;2;30;144;255mDescription:\u001b[0m Browses the web to find information\n",
+ " โโโ ๐ ๏ธ \u001b[3;38;2;30;144;255mTools:\u001b[0m\n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโณโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโณโโ๏ฟฝ๏ฟฝ๏ฟฝโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ " โ\u001b[1m \u001b[0m\u001b[1mName \u001b[0m\u001b[1m \u001b[0mโ\u001b[1m \u001b[0m\u001b[1mDescription \u001b[0m\u001b[1m \u001b[0mโ\u001b[1m \u001b[0m\u001b[1mArguments \u001b[0m\u001b[1m \u001b[0mโ\n",
+ " โกโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฉ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0m\u001b[38;2;30;144;255mweb_search \u001b[0m\u001b[38;2;30;144;255m \u001b[0mโ Performs a google web search for โ query (`string`): The search โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ your query then returns a string โ query to perform. โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ of the top search results. โ filter_year (`integer`): โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ โ Optionally restrict results to a โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ โ certain year โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0m\u001b[38;2;30;144;255mvisit_webpage \u001b[0m\u001b[38;2;30;144;255m \u001b[0mโ Visits a webpage at the given url โ url (`string`): The url of the โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ and reads its content as a โ webpage to visit. โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ markdown string. Use this to โ โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ browse webpages. โ โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0m\u001b[38;2;30;144;255mcalculate_cargo_travel_time\u001b[0m\u001b[38;2;30;144;255m \u001b[0mโ Calculate the travel time for a โ origin_coords (`array`): Tuple of โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ cargo plane between two points on โ (latitude, longitude) for the โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ Earth using great-circle โ starting point โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ distance. โ destination_coords (`array`): โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ โ Tuple of (latitude, longitude) โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ โ for the destination โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ โ cruising_speed_kmh (`number`): โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ โ Optional cruising speed in km/h โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ โ (defaults to 750 km/h for typical โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ โ cargo planes) โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0m\u001b[38;2;30;144;255mfinal_answer \u001b[0m\u001b[38;2;30;144;255m \u001b[0mโ Provides a final answer to the โ answer (`any`): The final answer โ\n",
+ " โ\u001b[38;2;30;144;255m \u001b[0mโ given problem. โ to the problem โ\n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโดโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโดโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ }
+ ],
+ "source": [
+ "manager_agent.visualize()"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "id": "RyGu4VylavwW",
+ "outputId": "220b8c08-f5a6-4c41-eaf3-d684ee773a85"
+ },
+ "outputs": [
+ {
+ "data": {
+ "text/html": [
+ "โญโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ New run โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฎ\n",
+ "โ โ\n",
+ "โ Find all Batman filming locations in the world, calculate the time to transfer via cargo plane to here (we're โ\n",
+ "โ in Gotham, 40.7128ยฐ N, 74.0060ยฐ W). โ\n",
+ "โ Also give me some supercar factories with the same cargo plane transfer time. You need at least 6 points in โ\n",
+ "โ total. โ\n",
+ "โ Represent this as spatial map of the world, with the locations represented as scatter points with a color that โ\n",
+ "โ depends on the travel time, and save it to saved_map.png! โ\n",
+ "โ โ\n",
+ "โ Here's an example of how to plot and return a map: โ\n",
+ "โ import plotly.express as px โ\n",
+ "โ df = px.data.carshare() โ\n",
+ "โ fig = px.scatter_map(df, lat=\"centroid_lat\", lon=\"centroid_lon\", text=\"name\", color=\"peak_hour\", size=100, โ\n",
+ "โ color_continuous_scale=px.colors.sequential.Magma, size_max=15, zoom=1) โ\n",
+ "โ fig.show() โ\n",
+ "โ fig.write_image(\"saved_image.png\") โ\n",
+ "โ final_answer(fig) โ\n",
+ "โ โ\n",
+ "โ Never try to process strings using code: when you have a string to read, just print it and you'll see it. โ\n",
+ "โ โ\n",
+ "โฐโ HfApiModel - deepseek-ai/DeepSeek-R1 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโฏ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโญโ\u001b[0m\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\u001b[38;2;212;183;2m \u001b[0m\u001b[1;38;2;212;183;2mNew run\u001b[0m\u001b[38;2;212;183;2m \u001b[0m\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\u001b[38;2;212;183;2mโโฎ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mFind all Batman filming locations in the world, calculate the time to transfer via cargo plane to here (we're \u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1min Gotham, 40.7128ยฐ N, 74.0060ยฐ W).\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mAlso give me some supercar factories with the same cargo plane transfer time. You need at least 6 points in \u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mtotal.\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mRepresent this as spatial map of the world, with the locations represented as scatter points with a color that \u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mdepends on the travel time, and save it to saved_map.png!\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mHere's an example of how to plot and return a map:\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mimport plotly.express as px\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mdf = px.data.carshare()\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mfig = px.scatter_map(df, lat=\"centroid_lat\", lon=\"centroid_lon\", text=\"name\", color=\"peak_hour\", size=100,\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1m color_continuous_scale=px.colors.sequential.Magma, size_max=15, zoom=1)\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mfig.show()\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mfig.write_image(\"saved_image.png\")\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mfinal_answer(fig)\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[1mNever try to process strings using code: when you have a string to read, just print it and you'll see it.\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโ\u001b[0m \u001b[38;2;212;183;2mโ\u001b[0m\n",
+ "\u001b[38;2;212;183;2mโฐโ\u001b[0m\u001b[38;2;212;183;2m HfApiModel - deepseek-ai/DeepSeek-R1 \u001b[0m\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\u001b[38;2;212;183;2mโโฏ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Initial plan โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "Here is the plan of action that I will follow to solve the task:\n",
+ " ```\n",
+ " <think>\n",
+ "Okay, let's break down the problem step by step. The user wants a map showing Batman filming locations and supercar\n",
+ "factories with their cargo plane transfer times to Gotham. The total points should be at least six, with supercar \n",
+ "factories having the same transfer times as some Batman locations.\n",
+ "\n",
+ "First, I need to gather the Batman filming locations. Since I don't have that data, I'll need to use the web_agent \n",
+ "to search for reliable sources. Once I have the list of locations with their coordinates, I can calculate the \n",
+ "travel time from each to Gotham using the calculate_cargo_travel_time tool. \n",
+ "\n",
+ "Next, I need to find supercar factories. Again, the web_agent can help find their locations. For each factory, I'll\n",
+ "calculate the travel time to Gotham. Then, I have to match these times with the Batman locations' times. If a \n",
+ "factory's time matches any of the Batman times, it gets included. If there aren't enough matches, I might need to \n",
+ "adjust by finding more factories or relaxing the time matching criteria, but the user specified \"same time,\" so \n",
+ "I'll stick to exact matches as much as possible.\n",
+ "\n",
+ "Once I have at least six points (combination of Batman locations and matching factories), I'll compile them into a \n",
+ "dataset. The example code uses a DataFrame with lat, lon, name, and color based on travel time. I'll structure my \n",
+ "data similarly. Finally, I'll plot the points on a map using plotly, color-coding by travel time, and save the \n",
+ "image.\n",
+ "</think>\n",
+ "\n",
+ "1. Task the web agent to compile a list of all Batman movie filming locations worldwide, including their geographic\n",
+ "coordinates (latitude/longitude). \n",
+ "2. For each Batman filming location, use the `calculate_cargo_travel_time` tool to determine the cargo plane \n",
+ "transfer time to Gotham (40.7128ยฐ N, 74.0060ยฐ W). \n",
+ "3. Task the web agent to identify factories of supercar manufacturers globally, including their geographic \n",
+ "coordinates. \n",
+ "4. For each supercar factory, calculate its cargo plane transfer time to Gotham using the same tool. \n",
+ "5. Filter the supercar factories to retain only those with transfer times matching the Batman locationsโ calculated\n",
+ "times. Combine these with Batman locations to ensure at least 6 total points. \n",
+ "6. Create a DataFrame with columns for latitude, longitude, location/factory name, and transfer time. \n",
+ "7. Plot the combined data on a global map using `plotly.express`, coloring points by transfer time. Save the map to\n",
+ "`saved_map.png`. \n",
+ "8. Return the saved map as the final answer. \n",
+ "\n",
+ "\n",
+ " ```\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[1mInitial plan\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "Here is the plan of action that I will follow to solve the task:\n",
+ " ```\n",
+ " \n",
+ "Okay, let's break down the problem step by step. The user wants a map showing Batman filming locations and supercar\n",
+ "factories with their cargo plane transfer times to Gotham. The total points should be at least six, with supercar \n",
+ "factories having the same transfer times as some Batman locations.\n",
+ "\n",
+ "First, I need to gather the Batman filming locations. Since I don't have that data, I'll need to use the web_agent \n",
+ "to search for reliable sources. Once I have the list of locations with their coordinates, I can calculate the \n",
+ "travel time from each to Gotham using the calculate_cargo_travel_time tool. \n",
+ "\n",
+ "Next, I need to find supercar factories. Again, the web_agent can help find their locations. For each factory, I'll\n",
+ "calculate the travel time to Gotham. Then, I have to match these times with the Batman locations' times. If a \n",
+ "factory's time matches any of the Batman times, it gets included. If there aren't enough matches, I might need to \n",
+ "adjust by finding more factories or relaxing the time matching criteria, but the user specified \"same time,\" so \n",
+ "I'll stick to exact matches as much as possible.\n",
+ "\n",
+ "Once I have at least six points (combination of Batman locations and matching factories), I'll compile them into a \n",
+ "dataset. The example code uses a DataFrame with lat, lon, name, and color based on travel time. I'll structure my \n",
+ "data similarly. Finally, I'll plot the points on a map using plotly, color-coding by travel time, and save the \n",
+ "image.\n",
+ "\n",
+ "\n",
+ "1. Task the web agent to compile a list of all Batman movie filming locations worldwide, including their geographic\n",
+ "coordinates (latitude/longitude). \n",
+ "2. For each Batman filming location, use the `calculate_cargo_travel_time` tool to determine the cargo plane \n",
+ "transfer time to Gotham (40.7128ยฐ N, 74.0060ยฐ W). \n",
+ "3. Task the web agent to identify factories of supercar manufacturers globally, including their geographic \n",
+ "coordinates. \n",
+ "4. For each supercar factory, calculate its cargo plane transfer time to Gotham using the same tool. \n",
+ "5. Filter the supercar factories to retain only those with transfer times matching the Batman locationsโ calculated\n",
+ "times. Combine these with Batman locations to ensure at least 6 total points. \n",
+ "6. Create a DataFrame with columns for latitude, longitude, location/factory name, and transfer time. \n",
+ "7. Plot the combined data on a global map using `plotly.express`, coloring points by transfer time. Save the map to\n",
+ "`saved_map.png`. \n",
+ "8. Return the saved map as the final answer. \n",
+ "\n",
+ "\n",
+ " ```\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Step 1 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[0m\u001b[1mStep \u001b[0m\u001b[1;36m1\u001b[0m\u001b[38;2;212;183;2m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Output message of the LLM: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ " [STEP 1]: \n",
+ "Thought: I need to get the list of Batman filming locations with their coordinates. Since I don't have this data, \n",
+ "I'll task the web_agent to search for it. \n",
+ "Code: \n",
+ "```py \n",
+ "batman_locations_task = \"Find all Batman movie filming locations worldwide. For each location, provide the name and\n",
+ "geographic coordinates (latitude, longitude). Use reliable sources like IMDb or Movie-Locations.com.\" \n",
+ "web_agent_response = web_agent(task=batman_locations_task) \n",
+ "print(web_agent_response) \n",
+ "```<end_code> \n",
+ " \n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1;3mOutput message of the LLM:\u001b[0m \u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m [\u001b[0m\u001b[1;38;2;121;192;255;48;2;13;17;23mSTEP 1\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mThought: I need to get the list of Batman filming locations with their coordinates. Since I don't have this data, \u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mI'll task the web_agent to search for it. \u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mCode:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m```py\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mbatman_locations_task\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m\"Find\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mall\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mBatman\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmovie\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfilming\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mworldwide.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mFor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23meach\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocation,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mprovide\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mname\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mgeographic\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoordinates\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(latitude,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlongitude).\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mUse\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mreliable\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msources\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlike\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mIMDb\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mMovie-Locations.com.\"\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mweb_agent_response\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mweb_agent(task=batman_locations_task)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mprint(web_agent_response)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m```\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ " โ Executing parsed code: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " batman_locations_task = \"Find all Batman movie filming locations worldwide. For each location, provide the name \n",
+ " and geographic coordinates (latitude, longitude). Use reliable sources like IMDb or Movie-Locations.com.\" \n",
+ " web_agent_response = web_agent(task=batman_locations_task) \n",
+ " print(web_agent_response) \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ "
\n"
+ ],
+ "text/plain": [
+ " โ \u001b[1mExecuting parsed code:\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mbatman_locations_task\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mFind all Batman movie filming locations worldwide. For each location, provide the name\u001b[0m \n",
+ " \u001b[38;2;230;219;116;48;2;39;40;34mand geographic coordinates (latitude, longitude). Use reliable sources like IMDb or Movie-Locations.com.\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mweb_agent_response\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mweb_agent\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtask\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_locations_task\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mweb_agent_response\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Execution logs:\n",
+ "Here is the final answer from your managed agent 'web_agent':\n",
+ "\n",
+ "### 1. Task outcome (short version):\n",
+ "Compiled a list of Batman movie filming locations and their geographic coordinates from IMDb and \n",
+ "Movie-Locations.com search results.\n",
+ "\n",
+ "### 2. Task outcome (extremely detailed version):\n",
+ "The task involved finding all Batman movie filming locations worldwide and providing the name and geographic \n",
+ "coordinates (latitude, longitude) for each location. Reliable sources like IMDb and Movie-Locations.com were used \n",
+ "to gather the information. The following Batman movies were included in the compilation:\n",
+ "\n",
+ "- **Batman (1966)**\n",
+ " - Stearns Wharf, Santa Barbara, California, USA (34.4188, -119.6951)\n",
+ " - Cliffs, Marineland of the Pacific, California, USA (33.9, -118.4)\n",
+ " - 380 South San Rafael Avenue, Pasadena, California, USA (34.1425, -118.1469)\n",
+ " - Bronson Caves, Bronson Canyon, Griffith Park, California, USA (34.1347, -118.3215)\n",
+ " - Alcatraz Island, San Francisco Bay, California, USA (37.8267, -122.4233)\n",
+ "\n",
+ "- **Batman (1989)**\n",
+ " - Knebworth House, Knebworth, Hertfordshire, England, UK (51.8055, -0.4035)\n",
+ " - Acton Lane Power Station, Acton Lane, Acton, England, UK (51.4925, -0.2217)\n",
+ " - 100 St. Mary Axe (The Gherkin), London, England, UK (51.5045, -0.0754)\n",
+ "\n",
+ "- **Batman Begins (2005)**\n",
+ " - Skaftafell, Iceland (64.2128, -19.0649)\n",
+ " - Lower Wacker Drive, Downtown, Chicago, Illinois, USA (41.8806, -87.6235)\n",
+ " - Chicago Board of Trade, Chicago, Illinois, USA (41.8879, -87.6235)\n",
+ " - St Pancras Renaissance Hotel, London, England, UK (51.5343, -0.1341)\n",
+ "\n",
+ "- **Batman & Robin (1997)**\n",
+ " - Warner Brothers Burbank Studios, Burbank, California, USA (34.1347, -118.3215)\n",
+ " - Courthouse Square, Backlot, Universal Studios, Universal City, California, USA (34.1347, -118.3215)\n",
+ "\n",
+ "- **Batman Forever (1995)**\n",
+ " - Alcatraz Island, San Francisco Bay, California, USA (37.8267, -122.4233)\n",
+ "\n",
+ "- **The Dark Knight (2008)**\n",
+ " - Chicago, Illinois, USA (41.8781, -87.6298)\n",
+ " - Battersea Power Station, Battersea, London, England, UK (51.4719, -0.1167)\n",
+ " - Times Square, Causeway Bay, Hong Kong (22.2833, 114.1589)\n",
+ "\n",
+ "- **The Batman (2022)**\n",
+ " - Necropolis Cemetery, Glasgow, Scotland, UK (55.8642, -4.2518)\n",
+ " - St. George's Hall, Liverpool, England, UK (53.4084, -2.9916)\n",
+ "\n",
+ "- **Batman Returns (1992)**\n",
+ " - Stage 12, Universal Studios, Universal City, California, USA (34.1347, -118.3215)\n",
+ "\n",
+ "### 3. Additional context (if relevant):\n",
+ "The visit to the Movie-Locations.com page for Batman movie filming locations returned a 404 error. Therefore, the \n",
+ "information was manually extracted from the search results and IMDb pages. The coordinates provided are approximate\n",
+ "and may not be the exact filming locations.\n",
+ "\n",
+ "\n",
+ "Out: None\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1mExecution logs:\u001b[0m\n",
+ "Here is the final answer from your managed agent 'web_agent':\n",
+ "\n",
+ "### 1. Task outcome (short version):\n",
+ "Compiled a list of Batman movie filming locations and their geographic coordinates from IMDb and \n",
+ "Movie-Locations.com search results.\n",
+ "\n",
+ "### 2. Task outcome (extremely detailed version):\n",
+ "The task involved finding all Batman movie filming locations worldwide and providing the name and geographic \n",
+ "coordinates (latitude, longitude) for each location. Reliable sources like IMDb and Movie-Locations.com were used \n",
+ "to gather the information. The following Batman movies were included in the compilation:\n",
+ "\n",
+ "- **Batman (1966)**\n",
+ " - Stearns Wharf, Santa Barbara, California, USA (34.4188, -119.6951)\n",
+ " - Cliffs, Marineland of the Pacific, California, USA (33.9, -118.4)\n",
+ " - 380 South San Rafael Avenue, Pasadena, California, USA (34.1425, -118.1469)\n",
+ " - Bronson Caves, Bronson Canyon, Griffith Park, California, USA (34.1347, -118.3215)\n",
+ " - Alcatraz Island, San Francisco Bay, California, USA (37.8267, -122.4233)\n",
+ "\n",
+ "- **Batman (1989)**\n",
+ " - Knebworth House, Knebworth, Hertfordshire, England, UK (51.8055, -0.4035)\n",
+ " - Acton Lane Power Station, Acton Lane, Acton, England, UK (51.4925, -0.2217)\n",
+ " - 100 St. Mary Axe (The Gherkin), London, England, UK (51.5045, -0.0754)\n",
+ "\n",
+ "- **Batman Begins (2005)**\n",
+ " - Skaftafell, Iceland (64.2128, -19.0649)\n",
+ " - Lower Wacker Drive, Downtown, Chicago, Illinois, USA (41.8806, -87.6235)\n",
+ " - Chicago Board of Trade, Chicago, Illinois, USA (41.8879, -87.6235)\n",
+ " - St Pancras Renaissance Hotel, London, England, UK (51.5343, -0.1341)\n",
+ "\n",
+ "- **Batman & Robin (1997)**\n",
+ " - Warner Brothers Burbank Studios, Burbank, California, USA (34.1347, -118.3215)\n",
+ " - Courthouse Square, Backlot, Universal Studios, Universal City, California, USA (34.1347, -118.3215)\n",
+ "\n",
+ "- **Batman Forever (1995)**\n",
+ " - Alcatraz Island, San Francisco Bay, California, USA (37.8267, -122.4233)\n",
+ "\n",
+ "- **The Dark Knight (2008)**\n",
+ " - Chicago, Illinois, USA (41.8781, -87.6298)\n",
+ " - Battersea Power Station, Battersea, London, England, UK (51.4719, -0.1167)\n",
+ " - Times Square, Causeway Bay, Hong Kong (22.2833, 114.1589)\n",
+ "\n",
+ "- **The Batman (2022)**\n",
+ " - Necropolis Cemetery, Glasgow, Scotland, UK (55.8642, -4.2518)\n",
+ " - St. George's Hall, Liverpool, England, UK (53.4084, -2.9916)\n",
+ "\n",
+ "- **Batman Returns (1992)**\n",
+ " - Stage 12, Universal Studios, Universal City, California, USA (34.1347, -118.3215)\n",
+ "\n",
+ "### 3. Additional context (if relevant):\n",
+ "The visit to the Movie-Locations.com page for Batman movie filming locations returned a 404 error. Therefore, the \n",
+ "information was manually extracted from the search results and IMDb pages. The coordinates provided are approximate\n",
+ "and may not be the exact filming locations.\n",
+ "\n",
+ "\n",
+ "Out: None\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "[Step 1: Duration 208.00 seconds| Input tokens: 6,452 | Output tokens: 116]\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[2m[Step 1: Duration 208.00 seconds| Input tokens: 6,452 | Output tokens: 116]\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Step 2 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ๏ฟฝ๏ฟฝ๏ฟฝโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[0m\u001b[1mStep \u001b[0m\u001b[1;36m2\u001b[0m\u001b[38;2;212;183;2m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Output message of the LLM: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "<think> \n",
+ "Okay, let's tackle this step by step. First, I need to process the Batman filming locations provided by the web \n",
+ "agent. The user wants the travel time from each of these locations to Gotham (40.7128ยฐ N, 74.0060ยฐ W) using a cargo\n",
+ "plane. Then, find supercar factories with similar travel times. \n",
+ " \n",
+ "So, the first step is to extract all the locations and their coordinates from the web agent's response. Let me list\n",
+ "them out: \n",
+ " \n",
+ "From the response, locations include places in California, London, Chicago, Iceland, etc. Each has coordinates. \n",
+ "I'll need to loop through each, calculate the travel time using calculate_cargo_travel_time, and collect the \n",
+ "results. \n",
+ " \n",
+ "Next, I need to get supercar factories. I'll task the web agent again to find these with their coordinates. Once I \n",
+ "have those, calculate their travel times to Gotham too. Then, match the supercar factories' times with the Batman \n",
+ "locations' times to find those with the same or similar times. Since the user wants at least 6 points in total, if \n",
+ "there aren't enough supercar factories with matching times, I might need to include more Batman locations or adjust\n",
+ "the criteria. \n",
+ " \n",
+ "After collecting all the data, I'll create a DataFrame with columns for latitude, longitude, name, and travel time.\n",
+ "Then, use Plotly to create a scatter map with color based on travel time. Save the map as saved_map.png and return \n",
+ "it. \n",
+ " \n",
+ "Wait, but how do I handle the supercar factories? Let me make sure to structure the code properly. First, process \n",
+ "Batman locations, then get supercar factories, process those, filter, combine both datasets, and plot. \n",
+ " \n",
+ "Also, need to handle possible duplicates in locations. For example, some coordinates repeat (like 34.1347, \n",
+ "-118.3215 appears multiple times). Maybe deduplicate them to avoid redundant calculations. \n",
+ " \n",
+ "Let me start coding step by step. First, extract the Batman locations and their coordinates. Then calculate each \n",
+ "travel time. Then get supercar factories, calculate their times, filter, combine, and plot. \n",
+ "</think> \n",
+ " \n",
+ "``` [STEP 2]: \n",
+ "Thought: I need to process the Batman filming locations and calculate their cargo travel times to Gotham. First, \n",
+ "I'll extract coordinates from the web agent's response and use calculate_cargo_travel_time for each location. \n",
+ " \n",
+ "Code: \n",
+ "```py \n",
+ "# Gotham coordinates (New York City) \n",
+ "gotham_coords = [40.7128, -74.0060] \n",
+ " \n",
+ "# Extract Batman locations with deduplication \n",
+ "batman_locations = [ \n",
+ " (\"Stearns Wharf, Santa Barbara\", (34.4188, -119.6951)), \n",
+ " (\"Cliffs, Marineland\", (33.9, -118.4)), \n",
+ " (\"Bronson Caves, Griffith Park\", (34.1347, -118.3215)), \n",
+ " (\"Alcatraz Island\", (37.8267, -122.4233)), \n",
+ " (\"Knebworth House, UK\", (51.8055, -0.4035)), \n",
+ " (\"Skaftafell, Iceland\", (64.2128, -19.0649)), \n",
+ " (\"Chicago Board of Trade\", (41.8879, -87.6235)), \n",
+ " (\"St Pancras Hotel, London\", (51.5343, -0.1341)), \n",
+ " (\"Battersea Power Station\", (51.4719, -0.1167)), \n",
+ " (\"Necropolis Cemetery\", (55.8642, -4.2518)) \n",
+ "] \n",
+ " \n",
+ "# Calculate travel times \n",
+ "batman_times = [] \n",
+ "for name, coords in batman_locations: \n",
+ " time = calculate_cargo_travel_time( \n",
+ " origin_coords=coords, \n",
+ " destination_coords=gotham_coords, \n",
+ " cruising_speed_kmh=750 \n",
+ " ) \n",
+ " batman_times.append((name, coords[0], coords[1], time)) \n",
+ " print(f\"{name}: {time:.2f} hours\") \n",
+ " \n",
+ "print(\"\\nBatman locations processed:\", len(batman_times)) \n",
+ "``` \n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1;3mOutput message of the LLM:\u001b[0m \u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mOkay,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlet's\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtackle\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthis\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mstep\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mby\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mstep.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mFirst,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mneed\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mprocess\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mBatman\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfilming\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mprovided\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mby\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mweb\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23magent.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mThe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23muser\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwants\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtravel\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfrom\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23meach\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mof\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthese\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mGotham\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(40.7128ยฐ\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mN,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m74.0060ยฐ\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mW)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23musing\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23ma\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcargo\u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mplane.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mThen,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfind\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwith\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msimilar\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtravel\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtimes.\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mSo,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfirst\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mstep\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mis\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mextract\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mall\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtheir\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoordinates\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfrom\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mweb\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23magent's\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mresponse.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mLet\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mme\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlist\u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mthem\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mout:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mFrom\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mresponse,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23minclude\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mplaces\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23min\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mCalifornia,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mLondon,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mChicago,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mIceland,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23metc.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mEach\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mhas\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoordinates.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mI'll\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mneed\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mloop\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthrough\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23meach,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcalculate\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtravel\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23musing\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcalculate_cargo_travel_time,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcollect\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mresults.\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mNext,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mneed\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mget\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI'll\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtask\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mweb\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23magent\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23magain\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfind\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthese\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwith\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtheir\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoordinates.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mOnce\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mhave\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthose,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcalculate\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtheir\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtravel\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtimes\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mGotham\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtoo.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mThen,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmatch\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtimes\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwith\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mBatman\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mlocations'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtimes\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfind\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthose\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwith\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msame\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msimilar\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtimes.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mSince\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23muser\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwants\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mat\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mleast\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m6\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mpoints\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23min\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtotal,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mif\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mthere\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23maren't\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23menough\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwith\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmatching\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtimes,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmight\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mneed\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23minclude\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmore\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mBatman\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23madjust\u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcriteria.\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mAfter\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcollecting\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mall\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mdata,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI'll\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcreate\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23ma\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mDataFrame\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwith\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcolumns\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlatitude,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlongitude,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mname,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtravel\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime.\u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mThen,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23muse\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mPlotly\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcreate\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23ma\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mscatter\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmap\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwith\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcolor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mbased\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mon\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtravel\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mSave\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmap\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mas\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msaved_map.png\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mreturn\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mit.\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mWait,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mbut\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mhow\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mdo\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mhandle\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories?\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mLet\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mme\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmake\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msure\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mstructure\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcode\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mproperly.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mFirst,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mprocess\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mBatman\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthen\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mget\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mprocess\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthose,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfilter,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcombine\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mboth\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mdatasets,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mplot.\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mAlso,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mneed\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mhandle\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mpossible\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mduplicates\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23min\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mFor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mexample,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msome\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoordinates\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mrepeat\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(like\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m34.1347,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m-118.3215\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mappears\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmultiple\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtimes).\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mMaybe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mdeduplicate\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthem\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mavoid\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mredundant\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcalculations.\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mLet\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mme\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mstart\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoding\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mstep\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mby\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mstep.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mFirst,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mextract\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mBatman\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtheir\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoordinates.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mThen\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcalculate\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23meach\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mtravel\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mThen\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mget\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcalculate\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtheir\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtimes,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfilter,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcombine,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mplot.\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m```\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[STEP\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m2]:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mThought:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mneed\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mprocess\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mBatman\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfilming\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcalculate\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtheir\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcargo\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtravel\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtimes\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mGotham.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mFirst,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mI'll\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mextract\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoordinates\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfrom\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mweb\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23magent's\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mresponse\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23muse\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcalculate_cargo_travel_time\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23meach\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocation.\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mCode:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;165;214;255;48;2;13;17;23m```\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mpy\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[3;38;2;139;148;158;48;2;13;17;23m# Gotham coordinates (New York City)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mgotham_coords\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m40.7128\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m74.0060\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[3;38;2;139;148;158;48;2;13;17;23m# Extract Batman locations with deduplication\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mbatman_locations\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mStearns Wharf, Santa Barbara\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m34.4188\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m119.6951\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mCliffs, Marineland\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m33.9\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m118.4\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mBronson Caves, Griffith Park\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m34.1347\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m118.3215\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mAlcatraz Island\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m37.8267\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m122.4233\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mKnebworth House, UK\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m51.8055\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0.4035\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mSkaftafell, Iceland\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m64.2128\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m19.0649\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mChicago Board of Trade\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m41.8879\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m87.6235\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mSt Pancras Hotel, London\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m51.5343\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0.1341\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mBattersea Power Station\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m51.4719\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0.1167\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mNecropolis Cemetery\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m55.8642\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m4.2518\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[3;38;2;139;148;158;48;2;13;17;23m# Calculate travel times\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mbatman_times\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;255;123;114;48;2;13;17;23mfor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mname\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoords\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23min\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mbatman_locations\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcalculate_cargo_travel_time\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23morigin_coords\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoords\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mdestination_coords\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mgotham_coords\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcruising_speed_kmh\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m750\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mbatman_times\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mappend\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mname\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoords\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoords\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m1\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mprint\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;121;192;255;48;2;13;17;23mf\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m{\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mname\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m}\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m: \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m{\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m:\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m.2f\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m}\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m hours\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mprint\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;121;192;255;48;2;13;17;23m\\n\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mBatman locations processed:\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlen\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mbatman_times\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;165;214;255;48;2;13;17;23m```\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ " โ Executing parsed code: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " # Gotham coordinates (New York City) \n",
+ " gotham_coords = [40.7128, -74.0060] \n",
+ " \n",
+ " # Extract Batman locations with deduplication \n",
+ " batman_locations = [ \n",
+ " (\"Stearns Wharf, Santa Barbara\", (34.4188, -119.6951)), \n",
+ " (\"Cliffs, Marineland\", (33.9, -118.4)), \n",
+ " (\"Bronson Caves, Griffith Park\", (34.1347, -118.3215)), \n",
+ " (\"Alcatraz Island\", (37.8267, -122.4233)), \n",
+ " (\"Knebworth House, UK\", (51.8055, -0.4035)), \n",
+ " (\"Skaftafell, Iceland\", (64.2128, -19.0649)), \n",
+ " (\"Chicago Board of Trade\", (41.8879, -87.6235)), \n",
+ " (\"St Pancras Hotel, London\", (51.5343, -0.1341)), \n",
+ " (\"Battersea Power Station\", (51.4719, -0.1167)), \n",
+ " (\"Necropolis Cemetery\", (55.8642, -4.2518)) \n",
+ " ] \n",
+ " \n",
+ " # Calculate travel times \n",
+ " batman_times = [] \n",
+ " for name, coords in batman_locations: \n",
+ " time = calculate_cargo_travel_time( \n",
+ " origin_coords=coords, \n",
+ " destination_coords=gotham_coords, \n",
+ " cruising_speed_kmh=750 \n",
+ " ) \n",
+ " batman_times.append((name, coords[0], coords[1], time)) \n",
+ " print(f\"{name}: {time:.2f} hours\") \n",
+ " \n",
+ " print(\"\\nBatman locations processed:\", len(batman_times)) \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ "
\n"
+ ],
+ "text/plain": [
+ " โ \u001b[1mExecuting parsed code:\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Gotham coordinates (New York City)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mgotham_coords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m40.7128\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m74.0060\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Extract Batman locations with deduplication\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mbatman_locations\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mStearns Wharf, Santa Barbara\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m34.4188\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m119.6951\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mCliffs, Marineland\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m33.9\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m118.4\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBronson Caves, Griffith Park\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m34.1347\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m118.3215\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mAlcatraz Island\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m37.8267\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m122.4233\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mKnebworth House, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m51.8055\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.4035\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mSkaftafell, Iceland\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m64.2128\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m19.0649\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mChicago Board of Trade\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m41.8879\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m87.6235\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mSt Pancras Hotel, London\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m51.5343\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.1341\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBattersea Power Station\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m51.4719\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.1167\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mNecropolis Cemetery\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m55.8642\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m4.2518\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Calculate travel times\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mbatman_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;102;217;239;48;2;39;40;34mfor\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mname\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcoords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34min\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_locations\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtime\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcalculate_cargo_travel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34morigin_coords\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcoords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mdestination_coords\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mgotham_coords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcruising_speed_kmh\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m750\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_times\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mappend\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mname\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcoords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcoords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m1\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtime\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mf\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m{\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mname\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m}\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m: \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m{\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtime\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m:\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m.2f\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m}\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m hours\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m\\n\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBatman locations processed:\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlen\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Execution logs:\n",
+ "Stearns Wharf, Santa Barbara: 6.93 hours\n",
+ "Cliffs, Marineland: 6.80 hours\n",
+ "Bronson Caves, Griffith Park: 6.78 hours\n",
+ "Alcatraz Island: 7.05 hours\n",
+ "Knebworth House, UK: 9.13 hours\n",
+ "Skaftafell, Iceland: 7.37 hours\n",
+ "Chicago Board of Trade: 2.68 hours\n",
+ "St Pancras Hotel, London: 9.17 hours\n",
+ "Battersea Power Station: 9.17 hours\n",
+ "Necropolis Cemetery: 8.60 hours\n",
+ "\n",
+ "Batman locations processed: 10\n",
+ "\n",
+ "Out: None\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1mExecution logs:\u001b[0m\n",
+ "Stearns Wharf, Santa Barbara: 6.93 hours\n",
+ "Cliffs, Marineland: 6.80 hours\n",
+ "Bronson Caves, Griffith Park: 6.78 hours\n",
+ "Alcatraz Island: 7.05 hours\n",
+ "Knebworth House, UK: 9.13 hours\n",
+ "Skaftafell, Iceland: 7.37 hours\n",
+ "Chicago Board of Trade: 2.68 hours\n",
+ "St Pancras Hotel, London: 9.17 hours\n",
+ "Battersea Power Station: 9.17 hours\n",
+ "Necropolis Cemetery: 8.60 hours\n",
+ "\n",
+ "Batman locations processed: 10\n",
+ "\n",
+ "Out: None\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "[Step 2: Duration 12.72 seconds| Input tokens: 10,240 | Output tokens: 969]\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[2m[Step 2: Duration 12.72 seconds| Input tokens: 10,240 | Output tokens: 969]\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Step 3 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[0m\u001b[1mStep \u001b[0m\u001b[1;36m3\u001b[0m\u001b[38;2;212;183;2m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Output message of the LLM: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "<think> \n",
+ " \n",
+ "Okay, let's tackle this step by step. First, I need to find supercar factories around the world. Since I don't have\n",
+ "that data, I'll ask the web agent to search for them. Once I have the list, I'll calculate their travel times to \n",
+ "Gotham using the same method as the Batman locations. Then, I'll filter these factories to find those with matching\n",
+ "travel times. If there aren't enough, I'll combine both sets to ensure at least six points. Finally, I'll plot all \n",
+ "locations on a map with colors based on travel time and save the image. \n",
+ " \n",
+ "``` [STEP 3]: \n",
+ "Thought: Now I need to get supercar factories' locations. I'll task the web_agent again to find them with \n",
+ "coordinates. \n",
+ "Code: \n",
+ "```py \n",
+ "supercar_task = \"List factories of supercar manufacturers (e.g., Ferrari, Lamborghini, Bugatti) worldwide. For \n",
+ "each, provide the factory name and geographic coordinates (latitude, longitude).\" \n",
+ "supercar_response = web_agent(task=supercar_task) \n",
+ "print(supercar_response) \n",
+ "```<end_code> \n",
+ " \n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1;3mOutput message of the LLM:\u001b[0m \u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mOkay,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlet's\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtackle\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthis\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mstep\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mby\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mstep.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mFirst,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mneed\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfind\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23maround\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mworld.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mSince\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mdon't\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mhave\u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mthat\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mdata,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI'll\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mask\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mweb\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23magent\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msearch\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthem.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mOnce\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mhave\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlist,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI'll\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcalculate\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtheir\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtravel\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtimes\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mGotham\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23musing\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msame\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmethod\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mas\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mBatman\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mThen,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI'll\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfilter\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthese\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfind\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthose\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwith\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmatching\u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mtravel\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtimes.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mIf\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthere\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23maren't\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23menough,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI'll\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcombine\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mboth\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msets\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mensure\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mat\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mleast\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msix\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mpoints.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mFinally,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI'll\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mplot\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mall\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mlocations\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mon\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23ma\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmap\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwith\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcolors\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mbased\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mon\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtravel\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msave\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mimage.\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m```\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[STEP\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m3]:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mThought:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mNow\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mneed\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mget\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI'll\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtask\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mweb_agent\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23magain\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfind\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthem\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwith\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mcoordinates.\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mCode:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m```py\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23msupercar_task\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m\"List\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mof\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmanufacturers\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(e.g.,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mFerrari,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mLamborghini,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mBugatti)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mworldwide.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mFor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23meach,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mprovide\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactory\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mname\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mgeographic\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoordinates\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(latitude,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlongitude).\"\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23msupercar_response\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mweb_agent(task=supercar_task)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mprint(supercar_response)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m```\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ " โ Executing parsed code: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " supercar_task = \"List factories of supercar manufacturers (e.g., Ferrari, Lamborghini, Bugatti) worldwide. For \n",
+ " each, provide the factory name and geographic coordinates (latitude, longitude).\" \n",
+ " supercar_response = web_agent(task=supercar_task) \n",
+ " print(supercar_response) \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ "
\n"
+ ],
+ "text/plain": [
+ " โ \u001b[1mExecuting parsed code:\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34msupercar_task\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mList factories of supercar manufacturers (e.g., Ferrari, Lamborghini, Bugatti) worldwide. For \u001b[0m \n",
+ " \u001b[38;2;230;219;116;48;2;39;40;34meach, provide the factory name and geographic coordinates (latitude, longitude).\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34msupercar_response\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mweb_agent\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtask\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_task\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_response\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Execution logs:\n",
+ "Here is the final answer from your managed agent 'web_agent':\n",
+ "\n",
+ "### 1. Task outcome (short version):\n",
+ "Listed the main factories of Ferrari, Lamborghini, and Bugatti with their geographic coordinates.\n",
+ "\n",
+ "### 2. Task outcome (extremely detailed version):\n",
+ "- **Ferrari**:\n",
+ " - **Factory Name**: Maranello Factory\n",
+ " - **Coordinates**: Latitude: 44.58, Longitude: 10.89\n",
+ "- **Lamborghini**:\n",
+ " - **Factory Name**: Sant'Agata Bolognese Factory\n",
+ " - **Coordinates**: Latitude: 44.54, Longitude: 11.18\n",
+ "- **Bugatti**:\n",
+ " - **Factory Name**: Molsheim Factory\n",
+ " - **Coordinates**: Latitude: 48.69, Longitude: 7.71\n",
+ "\n",
+ "### 3. Additional context (if relevant):\n",
+ "The coordinates provided are based on the main factories of each supercar manufacturer. Ferrari has other locations\n",
+ "such as Ferrari Competizioni and Ferrari Powertrain, but the Maranello Factory is the primary production site. \n",
+ "Lamborghini has an R&D center in Sant'Agata Bolognese, but the main factory is also located there. Bugatti has only\n",
+ "one main factory in Molsheim, France.\n",
+ "\n",
+ "\n",
+ "Out: None\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1mExecution logs:\u001b[0m\n",
+ "Here is the final answer from your managed agent 'web_agent':\n",
+ "\n",
+ "### 1. Task outcome (short version):\n",
+ "Listed the main factories of Ferrari, Lamborghini, and Bugatti with their geographic coordinates.\n",
+ "\n",
+ "### 2. Task outcome (extremely detailed version):\n",
+ "- **Ferrari**:\n",
+ " - **Factory Name**: Maranello Factory\n",
+ " - **Coordinates**: Latitude: 44.58, Longitude: 10.89\n",
+ "- **Lamborghini**:\n",
+ " - **Factory Name**: Sant'Agata Bolognese Factory\n",
+ " - **Coordinates**: Latitude: 44.54, Longitude: 11.18\n",
+ "- **Bugatti**:\n",
+ " - **Factory Name**: Molsheim Factory\n",
+ " - **Coordinates**: Latitude: 48.69, Longitude: 7.71\n",
+ "\n",
+ "### 3. Additional context (if relevant):\n",
+ "The coordinates provided are based on the main factories of each supercar manufacturer. Ferrari has other locations\n",
+ "such as Ferrari Competizioni and Ferrari Powertrain, but the Maranello Factory is the primary production site. \n",
+ "Lamborghini has an R&D center in Sant'Agata Bolognese, but the main factory is also located there. Bugatti has only\n",
+ "one main factory in Molsheim, France.\n",
+ "\n",
+ "\n",
+ "Out: None\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "[Step 3: Duration 42.30 seconds| Input tokens: 15,035 | Output tokens: 1,197]\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[2m[Step 3: Duration 42.30 seconds| Input tokens: 15,035 | Output tokens: 1,197]\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Step 4 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[0m\u001b[1mStep \u001b[0m\u001b[1;36m4\u001b[0m\u001b[38;2;212;183;2m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Output message of the LLM: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "<think> \n",
+ " \n",
+ "Hmm, only three supercar factories were found. I need at least six points in total. Let's calculate their travel \n",
+ "times and see if any match the Batman locations' times. If not, I'll include all Batman locations and the factories\n",
+ "to reach six points. \n",
+ " \n",
+ "``` [STEP 4]: \n",
+ "Thought: Calculate travel times for supercar factories to Gotham. Then check for matching times with Batman \n",
+ "locations. \n",
+ "Code: \n",
+ "```py \n",
+ "supercar_factories = [ \n",
+ " (\"Ferrari Maranello\", (44.58, 10.89)), \n",
+ " (\"Lamborghini Sant'Agata\", (44.54, 11.18)), \n",
+ " (\"Bugatti Molsheim\", (48.69, 7.71)) \n",
+ "] \n",
+ " \n",
+ "supercar_times = [] \n",
+ "for name, coords in supercar_factories: \n",
+ " time = calculate_cargo_travel_time( \n",
+ " origin_coords=coords, \n",
+ " destination_coords=gotham_coords, \n",
+ " cruising_speed_kmh=750 \n",
+ " ) \n",
+ " supercar_times.append((name, coords[0], coords[1], time)) \n",
+ " print(f\"{name}: {time:.2f} hours\") \n",
+ " \n",
+ "# Get Batman times from previous step \n",
+ "batman_times = [item[3] for item in batman_times] \n",
+ " \n",
+ "# Find matching times (within 0.5 hours tolerance) \n",
+ "matching_factories = [] \n",
+ "for factory in supercar_times: \n",
+ " for bat_time in batman_times: \n",
+ " if abs(factory[3] - bat_time) <= 0.5: \n",
+ " matching_factories.append(factory) \n",
+ " break \n",
+ " \n",
+ "print(\"\\nMatching supercar factories:\", len(matching_factories)) \n",
+ "``` \n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1;3mOutput message of the LLM:\u001b[0m \u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mHmm,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23monly\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthree\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwere\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfound.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mneed\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mat\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mleast\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msix\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mpoints\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23min\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtotal.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mLet's\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcalculate\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtheir\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtravel\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mtimes\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msee\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mif\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23many\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmatch\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mBatman\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtimes.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mIf\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mnot,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mI'll\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23minclude\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mall\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mBatman\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories\u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mreach\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msix\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mpoints.\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m```\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[STEP\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m4]:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mThought:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mCalculate\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtravel\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtimes\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mGotham.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mThen\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcheck\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmatching\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtimes\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwith\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mBatman\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mlocations.\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mCode:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;165;214;255;48;2;13;17;23m```\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mpy\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23msupercar_factories\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mFerrari Maranello\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m44.58\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m10.89\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mLamborghini Sant\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mAgata\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m44.54\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m11.18\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mBugatti Molsheim\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m48.69\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m7.71\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23msupercar_times\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;255;123;114;48;2;13;17;23mfor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mname\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoords\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23min\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar_factories\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcalculate_cargo_travel_time\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23morigin_coords\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoords\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mdestination_coords\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mgotham_coords\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcruising_speed_kmh\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m750\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar_times\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mappend\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mname\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoords\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcoords\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m1\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mprint\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;121;192;255;48;2;13;17;23mf\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m{\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mname\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m}\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m: \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m{\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m:\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m.2f\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m}\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m hours\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[3;38;2;139;148;158;48;2;13;17;23m# Get Batman times from previous step\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mbatman_times\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mitem\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m3\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mfor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mitem\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23min\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mbatman_times\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[3;38;2;139;148;158;48;2;13;17;23m# Find matching times (within 0.5 hours tolerance)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mmatching_factories\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;255;123;114;48;2;13;17;23mfor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactory\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23min\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar_times\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mfor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mbat_time\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23min\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mbatman_times\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mif\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mabs\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactory\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m3\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mbat_time\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m<\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0.5\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmatching_factories\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mappend\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactory\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mbreak\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mprint\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;121;192;255;48;2;13;17;23m\\n\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mMatching supercar factories:\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlen\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmatching_factories\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;165;214;255;48;2;13;17;23m```\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ " โ Executing parsed code: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " supercar_factories = [ \n",
+ " (\"Ferrari Maranello\", (44.58, 10.89)), \n",
+ " (\"Lamborghini Sant'Agata\", (44.54, 11.18)), \n",
+ " (\"Bugatti Molsheim\", (48.69, 7.71)) \n",
+ " ] \n",
+ " \n",
+ " supercar_times = [] \n",
+ " for name, coords in supercar_factories: \n",
+ " time = calculate_cargo_travel_time( \n",
+ " origin_coords=coords, \n",
+ " destination_coords=gotham_coords, \n",
+ " cruising_speed_kmh=750 \n",
+ " ) \n",
+ " supercar_times.append((name, coords[0], coords[1], time)) \n",
+ " print(f\"{name}: {time:.2f} hours\") \n",
+ " \n",
+ " # Get Batman times from previous step \n",
+ " batman_times = [item[3] for item in batman_times] \n",
+ " \n",
+ " # Find matching times (within 0.5 hours tolerance) \n",
+ " matching_factories = [] \n",
+ " for factory in supercar_times: \n",
+ " for bat_time in batman_times: \n",
+ " if abs(factory[3] - bat_time) <= 0.5: \n",
+ " matching_factories.append(factory) \n",
+ " break \n",
+ " \n",
+ " print(\"\\nMatching supercar factories:\", len(matching_factories)) \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ "
\n"
+ ],
+ "text/plain": [
+ " โ \u001b[1mExecuting parsed code:\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34msupercar_factories\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mFerrari Maranello\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m44.58\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m10.89\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mLamborghini Sant\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mAgata\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m44.54\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m11.18\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBugatti Molsheim\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m48.69\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m7.71\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34msupercar_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;102;217;239;48;2;39;40;34mfor\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mname\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcoords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34min\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_factories\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtime\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcalculate_cargo_travel_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34morigin_coords\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcoords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mdestination_coords\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mgotham_coords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcruising_speed_kmh\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m750\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_times\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mappend\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mname\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcoords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcoords\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m1\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtime\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mf\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m{\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mname\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m}\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m: \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m{\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtime\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m:\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m.2f\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m}\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m hours\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Get Batman times from previous step\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mbatman_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mitem\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m3\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mfor\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mitem\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34min\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Find matching times (within 0.5 hours tolerance)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mmatching_factories\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;102;217;239;48;2;39;40;34mfor\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfactory\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34min\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mfor\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbat_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34min\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mif\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mabs\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfactory\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m3\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbat_time\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m<\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.5\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mmatching_factories\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mappend\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfactory\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mbreak\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mprint\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m\\n\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mMatching supercar factories:\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlen\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mmatching_factories\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Execution logs:\n",
+ "Ferrari Maranello: 10.72 hours\n",
+ "Lamborghini Sant'Agata: 10.75 hours\n",
+ "Bugatti Molsheim: 10.10 hours\n",
+ "\n",
+ "Matching supercar factories: 0\n",
+ "\n",
+ "Out: None\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1mExecution logs:\u001b[0m\n",
+ "Ferrari Maranello: 10.72 hours\n",
+ "Lamborghini Sant'Agata: 10.75 hours\n",
+ "Bugatti Molsheim: 10.10 hours\n",
+ "\n",
+ "Matching supercar factories: 0\n",
+ "\n",
+ "Out: None\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "[Step 4: Duration 5.87 seconds| Input tokens: 20,448 | Output tokens: 1,562]\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[2m[Step 4: Duration 5.87 seconds| Input tokens: 20,448 | Output tokens: 1,562]\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Step 5 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[0m\u001b[1mStep \u001b[0m\u001b[1;36m5\u001b[0m\u001b[38;2;212;183;2m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Output message of the LLM: โโโโโโโโ๏ฟฝ๏ฟฝโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "<think> \n",
+ " \n",
+ "No matches found. Need to combine Batman locations and supercar factories to reach at least six points. Let's take \n",
+ "all Batman locations (10) and add the three factories, totaling 13 points. Now, create the DataFrame and plot the \n",
+ "map. \n",
+ " \n",
+ "``` [STEP 5]: \n",
+ "Thought: Combine all Batman locations and supercar factories. Create a DataFrame and plot the map with color based \n",
+ "on travel time. \n",
+ "Code: \n",
+ "```py \n",
+ "import pandas as pd \n",
+ "import plotly.express as px \n",
+ " \n",
+ "# Combine data \n",
+ "all_data = [] \n",
+ "for item in batman_times: \n",
+ " all_data.append({ \n",
+ " 'name': item[0], \n",
+ " 'lat': item[1], \n",
+ " 'lon': item[2], \n",
+ " 'time': item[3], \n",
+ " 'type': 'Batman Location' \n",
+ " }) \n",
+ " \n",
+ "for factory in supercar_times: \n",
+ " all_data.append({ \n",
+ " 'name': factory[0], \n",
+ " 'lat': factory[1], \n",
+ " 'lon': factory[2], \n",
+ " 'time': factory[3], \n",
+ " 'type': 'Supercar Factory' \n",
+ " }) \n",
+ " \n",
+ "df = pd.DataFrame(all_data) \n",
+ " \n",
+ "# Create map \n",
+ "fig = px.scatter_mapbox(df, \n",
+ " lat='lat', \n",
+ " lon='lon', \n",
+ " text='name', \n",
+ " color='time', \n",
+ " color_continuous_scale=px.colors.sequential.Magma, \n",
+ " zoom=1, \n",
+ " mapbox_style='carto-positron', \n",
+ " title='Cargo Travel Time to Gotham (Hours)') \n",
+ " \n",
+ "fig.update_layout(margin={\"r\":0,\"t\":40,\"l\":0,\"b\":0}) \n",
+ "fig.write_image(\"saved_map.png\") \n",
+ "final_answer(\"saved_map.png\") \n",
+ "``` \n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1;3mOutput message of the LLM:\u001b[0m \u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mNo\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmatches\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfound.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mNeed\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcombine\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mBatman\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mto\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mreach\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mat\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mleast\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msix\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mpoints.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mLet's\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtake\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mall\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mBatman\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(10)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23madd\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthree\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtotaling\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m13\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mpoints.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mNow,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcreate\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mDataFrame\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mplot\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mmap.\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m```\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[STEP\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m5]:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mThought:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mCombine\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mall\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mBatman\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlocations\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactories.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mCreate\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23ma\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mDataFrame\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mand\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mplot\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mthe\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmap\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwith\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcolor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mbased\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mon\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtravel\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime.\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mCode:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;165;214;255;48;2;13;17;23m```\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mpy\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;255;123;114;48;2;13;17;23mimport\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mpandas\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mas\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mpd\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;255;123;114;48;2;13;17;23mimport\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mplotly\u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mexpress\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mas\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mpx\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[3;38;2;139;148;158;48;2;13;17;23m# Combine data\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mall_data\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;255;123;114;48;2;13;17;23mfor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mitem\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23min\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mbatman_times\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mall_data\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mappend\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m{\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mname\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mitem\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mlat\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mitem\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m1\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mlon\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mitem\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m2\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mtime\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mitem\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m3\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mtype\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mBatman Location\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m}\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;255;123;114;48;2;13;17;23mfor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactory\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23min\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar_times\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mall_data\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mappend\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m{\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mname\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactory\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mlat\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactory\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m1\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mlon\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactory\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m2\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mtime\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mfactory\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m3\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mtype\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mSupercar Factory\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m}\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mdf\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mpd\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mDataFrame\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mall_data\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[3;38;2;139;148;158;48;2;13;17;23m# Create map\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mfig\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mpx\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mscatter_mapbox\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mdf\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlat\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mlat\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlon\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mlon\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtext\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mname\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcolor\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mtime\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcolor_continuous_scale\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mpx\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcolors\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msequential\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mMagma\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mzoom\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m1\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmapbox_style\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mcarto-positron\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtitle\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mCargo Travel Time to Gotham (Hours)\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mfig\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mupdate_layout\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmargin\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m{\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mr\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mt\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m40\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23ml\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mb\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m}\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mfig\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwrite_image\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23msaved_map.png\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mfinal_answer\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23msaved_map.png\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;165;214;255;48;2;13;17;23m```\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ " โ Executing parsed code: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " import pandas as pd \n",
+ " import plotly.express as px \n",
+ " \n",
+ " # Combine data \n",
+ " all_data = [] \n",
+ " for item in batman_times: \n",
+ " all_data.append({ \n",
+ " 'name': item[0], \n",
+ " 'lat': item[1], \n",
+ " 'lon': item[2], \n",
+ " 'time': item[3], \n",
+ " 'type': 'Batman Location' \n",
+ " }) \n",
+ " \n",
+ " for factory in supercar_times: \n",
+ " all_data.append({ \n",
+ " 'name': factory[0], \n",
+ " 'lat': factory[1], \n",
+ " 'lon': factory[2], \n",
+ " 'time': factory[3], \n",
+ " 'type': 'Supercar Factory' \n",
+ " }) \n",
+ " \n",
+ " df = pd.DataFrame(all_data) \n",
+ " \n",
+ " # Create map \n",
+ " fig = px.scatter_mapbox(df, \n",
+ " lat='lat', \n",
+ " lon='lon', \n",
+ " text='name', \n",
+ " color='time', \n",
+ " color_continuous_scale=px.colors.sequential.Magma, \n",
+ " zoom=1, \n",
+ " mapbox_style='carto-positron', \n",
+ " title='Cargo Travel Time to Gotham (Hours)') \n",
+ " \n",
+ " fig.update_layout(margin={\"r\":0,\"t\":40,\"l\":0,\"b\":0}) \n",
+ " fig.write_image(\"saved_map.png\") \n",
+ " final_answer(\"saved_map.png\") \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ "
\n"
+ ],
+ "text/plain": [
+ " โ \u001b[1mExecuting parsed code:\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " \u001b[38;2;255;70;137;48;2;39;40;34mimport\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpandas\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mas\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpd\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;255;70;137;48;2;39;40;34mimport\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mplotly\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mexpress\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mas\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpx\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Combine data\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mall_data\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;102;217;239;48;2;39;40;34mfor\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mitem\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34min\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mall_data\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mappend\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m{\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mname\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mitem\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mlat\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mitem\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m1\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mlon\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mitem\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m2\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mtime\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mitem\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m3\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mtype\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBatman Location\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m}\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;102;217;239;48;2;39;40;34mfor\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfactory\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34min\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_times\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mall_data\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mappend\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m{\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mname\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfactory\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mlat\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfactory\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m1\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mlon\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfactory\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m2\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mtime\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mfactory\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m3\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mtype\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mSupercar Factory\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m}\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mdf\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpd\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mDataFrame\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mall_data\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Create map\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mfig\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpx\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mscatter_mapbox\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mdf\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlat\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mlat\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlon\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mlon\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtext\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mname\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcolor\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mtime\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcolor_continuous_scale\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpx\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcolors\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msequential\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mMagma\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mzoom\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m1\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mmapbox_style\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mcarto-positron\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtitle\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mCargo Travel Time to Gotham (Hours)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mfig\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mupdate_layout\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mmargin\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m{\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mr\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mt\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m40\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34ml\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mb\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m}\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mfig\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mwrite_image\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34msaved_map.png\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mfinal_answer\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34msaved_map.png\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Code execution failed at line 'for item in batman_times:\n",
+ " all_data.append({\n",
+ " 'name': item[0],\n",
+ " 'lat': item[1],\n",
+ " 'lon': item[2],\n",
+ " 'time': item[3],\n",
+ " 'type': 'Batman Location'\n",
+ " })' due to: TypeError: argument of type 'float' is not iterable\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1;31mCode execution failed at line 'for item in batman_times:\u001b[0m\n",
+ "\u001b[1;31m \u001b[0m\u001b[1;31mall_data.append\u001b[0m\u001b[1;31m(\u001b[0m\u001b[1;31m{\u001b[0m\n",
+ "\u001b[1;31m \u001b[0m\u001b[1;31m'name'\u001b[0m\u001b[1;31m: item\u001b[0m\u001b[1;31m[\u001b[0m\u001b[1;31m0\u001b[0m\u001b[1;31m]\u001b[0m\u001b[1;31m,\u001b[0m\n",
+ "\u001b[1;31m \u001b[0m\u001b[1;31m'lat'\u001b[0m\u001b[1;31m: item\u001b[0m\u001b[1;31m[\u001b[0m\u001b[1;31m1\u001b[0m\u001b[1;31m]\u001b[0m\u001b[1;31m,\u001b[0m\n",
+ "\u001b[1;31m \u001b[0m\u001b[1;31m'lon'\u001b[0m\u001b[1;31m: item\u001b[0m\u001b[1;31m[\u001b[0m\u001b[1;31m2\u001b[0m\u001b[1;31m]\u001b[0m\u001b[1;31m,\u001b[0m\n",
+ "\u001b[1;31m \u001b[0m\u001b[1;31m'time'\u001b[0m\u001b[1;31m: item\u001b[0m\u001b[1;31m[\u001b[0m\u001b[1;31m3\u001b[0m\u001b[1;31m]\u001b[0m\u001b[1;31m,\u001b[0m\n",
+ "\u001b[1;31m \u001b[0m\u001b[1;31m'type'\u001b[0m\u001b[1;31m: \u001b[0m\u001b[1;31m'Batman Location'\u001b[0m\n",
+ "\u001b[1;31m \u001b[0m\u001b[1;31m}\u001b[0m\u001b[1;31m)\u001b[0m\u001b[1;31m' due to: TypeError: argument of type '\u001b[0m\u001b[1;31mfloat' is not iterable\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "[Step 5: Duration 6.29 seconds| Input tokens: 26,630 | Output tokens: 1,915]\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[2m[Step 5: Duration 6.29 seconds| Input tokens: 26,630 | Output tokens: 1,915]\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Updated plan โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "I still need to solve the task I was given:\n",
+ " ```\n",
+ "\n",
+ "Find all Batman filming locations in the world, calculate the time to transfer via cargo plane to here (we're in \n",
+ "Gotham, 40.7128ยฐ N, 74.0060ยฐ W).\n",
+ "Also give me some supercar factories with the same cargo plane transfer time. You need at least 6 points in total.\n",
+ "Represent this as spatial map of the world, with the locations represented as scatter points with a color that \n",
+ "depends on the travel time, and save it to saved_map.png!\n",
+ "\n",
+ "Here's an example of how to plot and return a map:\n",
+ "import plotly.express as px\n",
+ "df = px.data.carshare()\n",
+ "fig = px.scatter_map(df, lat=\"centroid_lat\", lon=\"centroid_lon\", text=\"name\", color=\"peak_hour\", size=100,\n",
+ " color_continuous_scale=px.colors.sequential.Magma, size_max=15, zoom=1)\n",
+ "fig.show()\n",
+ "fig.write_image(\"saved_image.png\")\n",
+ "final_answer(fig)\n",
+ "\n",
+ "Never try to process strings using code: when you have a string to read, just print it and you'll see it.\n",
+ "\n",
+ " ```\n",
+ "\n",
+ " Here is my new/updated plan of action to solve the task:\n",
+ " ```\n",
+ " <think>\n",
+ "Okay, let's fix the error. The previous code failed because the batman_times list structure was incorrect. Let me \n",
+ "check that.\n",
+ "\n",
+ "In Step 2, batman_times was created as a list of tuples: (name, lat, lon, time). So each item in batman_times has \n",
+ "four elements. But in Step 4, when trying to loop through batman_times, the code used 'for item in batman_times' \n",
+ "and then item[0], item[1], etc. Wait, no, in Step 5, the code tried to loop through batman_times and access \n",
+ "item[0], item[1], etc. But in the code for Step 5, the code was:\n",
+ "\n",
+ "for item in batman_times:\n",
+ " all_data.append({\n",
+ " 'name': item[0],\n",
+ " 'lat': item[1],\n",
+ " 'lon': item[2],\n",
+ " 'time': item[3],\n",
+ " 'type': 'Batman Location'\n",
+ " })\n",
+ "\n",
+ "But the error was that 'batman_times' is a list of floats. Wait, no. Wait, in Step 2, the code was:\n",
+ "\n",
+ "batman_times = []\n",
+ "for name, coords in batman_locations:\n",
+ " time = calculate_cargo_travel_time(...)\n",
+ " batman_times.append((name, coords[0], coords[1], time))\n",
+ "\n",
+ "So each entry in batman_times is a tuple of (name, lat, lon, time). So when looping through batman_times, each item\n",
+ "is a tuple with four elements. Then in Step 4, the code tried to do:\n",
+ "\n",
+ "batman_times = [item[3] for item in batman_times]\n",
+ "\n",
+ "Wait, that's a problem. Because in Step 4, the code overwrote batman_times to be a list of just the times (floats).\n",
+ "So when Step 5 tried to loop through batman_times, each item was a float, leading to the error.\n",
+ "\n",
+ "Ah, that's the mistake. In Step 4, the code redefined batman_times as a list of times, which caused the error in \n",
+ "Step 5 when trying to iterate over the original structure. So the solution is to not overwrite batman_times but use\n",
+ "a different variable name for the list of times.\n",
+ "\n",
+ "So the plan should correct this by:\n",
+ "\n",
+ "1. Correct the variable name in Step 4 to avoid overwriting batman_times.\n",
+ "2. Rebuild the DataFrame correctly using the original batman_times structure.\n",
+ "3. Ensure all data is properly combined for plotting.\n",
+ "\n",
+ "Let me adjust the plan accordingly.\n",
+ "</think>\n",
+ "\n",
+ "1. Correct the `batman_times` variable structure to preserve location metadata (name, lat, lon, time) instead of \n",
+ "overwriting it with just time values. \n",
+ "2. Re-calculate supercar factory travel times and store them with full metadata. \n",
+ "3. Combine all Batman locations and supercar factories into a single dataset. \n",
+ "4. Create a properly formatted DataFrame with columns: name, lat, lon, time, type. \n",
+ "5. Generate the map using Plotly Express with color coding based on travel time. \n",
+ "6. Save the map to `saved_map.png` and return it as the final answer. \n",
+ "\n",
+ " ```\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[1mUpdated plan\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "I still need to solve the task I was given:\n",
+ " ```\n",
+ "\n",
+ "Find all Batman filming locations in the world, calculate the time to transfer via cargo plane to here (we're in \n",
+ "Gotham, 40.7128ยฐ N, 74.0060ยฐ W).\n",
+ "Also give me some supercar factories with the same cargo plane transfer time. You need at least 6 points in total.\n",
+ "Represent this as spatial map of the world, with the locations represented as scatter points with a color that \n",
+ "depends on the travel time, and save it to saved_map.png!\n",
+ "\n",
+ "Here's an example of how to plot and return a map:\n",
+ "import plotly.express as px\n",
+ "df = px.data.carshare()\n",
+ "fig = px.scatter_map(df, lat=\"centroid_lat\", lon=\"centroid_lon\", text=\"name\", color=\"peak_hour\", size=100,\n",
+ " color_continuous_scale=px.colors.sequential.Magma, size_max=15, zoom=1)\n",
+ "fig.show()\n",
+ "fig.write_image(\"saved_image.png\")\n",
+ "final_answer(fig)\n",
+ "\n",
+ "Never try to process strings using code: when you have a string to read, just print it and you'll see it.\n",
+ "\n",
+ " ```\n",
+ "\n",
+ " Here is my new/updated plan of action to solve the task:\n",
+ " ```\n",
+ " \n",
+ "Okay, let's fix the error. The previous code failed because the batman_times list structure was incorrect. Let me \n",
+ "check that.\n",
+ "\n",
+ "In Step 2, batman_times was created as a list of tuples: (name, lat, lon, time). So each item in batman_times has \n",
+ "four elements. But in Step 4, when trying to loop through batman_times, the code used 'for item in batman_times' \n",
+ "and then item[0], item[1], etc. Wait, no, in Step 5, the code tried to loop through batman_times and access \n",
+ "item[0], item[1], etc. But in the code for Step 5, the code was:\n",
+ "\n",
+ "for item in batman_times:\n",
+ " all_data.append({\n",
+ " 'name': item[0],\n",
+ " 'lat': item[1],\n",
+ " 'lon': item[2],\n",
+ " 'time': item[3],\n",
+ " 'type': 'Batman Location'\n",
+ " })\n",
+ "\n",
+ "But the error was that 'batman_times' is a list of floats. Wait, no. Wait, in Step 2, the code was:\n",
+ "\n",
+ "batman_times = []\n",
+ "for name, coords in batman_locations:\n",
+ " time = calculate_cargo_travel_time(...)\n",
+ " batman_times.append((name, coords[0], coords[1], time))\n",
+ "\n",
+ "So each entry in batman_times is a tuple of (name, lat, lon, time). So when looping through batman_times, each item\n",
+ "is a tuple with four elements. Then in Step 4, the code tried to do:\n",
+ "\n",
+ "batman_times = [item[3] for item in batman_times]\n",
+ "\n",
+ "Wait, that's a problem. Because in Step 4, the code overwrote batman_times to be a list of just the times (floats).\n",
+ "So when Step 5 tried to loop through batman_times, each item was a float, leading to the error.\n",
+ "\n",
+ "Ah, that's the mistake. In Step 4, the code redefined batman_times as a list of times, which caused the error in \n",
+ "Step 5 when trying to iterate over the original structure. So the solution is to not overwrite batman_times but use\n",
+ "a different variable name for the list of times.\n",
+ "\n",
+ "So the plan should correct this by:\n",
+ "\n",
+ "1. Correct the variable name in Step 4 to avoid overwriting batman_times.\n",
+ "2. Rebuild the DataFrame correctly using the original batman_times structure.\n",
+ "3. Ensure all data is properly combined for plotting.\n",
+ "\n",
+ "Let me adjust the plan accordingly.\n",
+ "\n",
+ "\n",
+ "1. Correct the `batman_times` variable structure to preserve location metadata (name, lat, lon, time) instead of \n",
+ "overwriting it with just time values. \n",
+ "2. Re-calculate supercar factory travel times and store them with full metadata. \n",
+ "3. Combine all Batman locations and supercar factories into a single dataset. \n",
+ "4. Create a properly formatted DataFrame with columns: name, lat, lon, time, type. \n",
+ "5. Generate the map using Plotly Express with color coding based on travel time. \n",
+ "6. Save the map to `saved_map.png` and return it as the final answer. \n",
+ "\n",
+ " ```\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ Step 6 โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \u001b[0m\u001b[1mStep \u001b[0m\u001b[1;36m6\u001b[0m\u001b[38;2;212;183;2m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Output message of the LLM: โโโโโโโโโโโ๏ฟฝ๏ฟฝ๏ฟฝโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\n",
+ " [STEP 6]: \n",
+ "Thought: Fix the variable name conflict and rebuild the data structures properly. \n",
+ "Code: \n",
+ "```py \n",
+ "# Reconstruct batman_times with original structure (from Step 2) \n",
+ "batman_data = [ \n",
+ " (\"Stearns Wharf, Santa Barbara\", 34.4188, -119.6951, 6.93), \n",
+ " (\"Cliffs, Marineland\", 33.9, -118.4, 6.80), \n",
+ " (\"Bronson Caves, Griffith Park\", 34.1347, -118.3215, 6.78), \n",
+ " (\"Alcatraz Island\", 37.8267, -122.4233, 7.05), \n",
+ " (\"Knebworth House, UK\", 51.8055, -0.4035, 9.13), \n",
+ " (\"Skaftafell, Iceland\", 64.2128, -19.0649, 7.37), \n",
+ " (\"Chicago Board of Trade\", 41.8879, -87.6235, 2.68), \n",
+ " (\"St Pancras Hotel, London\", 51.5343, -0.1341, 9.17), \n",
+ " (\"Battersea Power Station\", 51.4719, -0.1167, 9.17), \n",
+ " (\"Necropolis Cemetery\", 55.8642, -4.2518, 8.60) \n",
+ "] \n",
+ " \n",
+ "supercar_data = [ \n",
+ " (\"Ferrari Maranello\", 44.58, 10.89, 10.72), \n",
+ " (\"Lamborghini Sant'Agata\", 44.54, 11.18, 10.75), \n",
+ " (\"Bugatti Molsheim\", 48.69, 7.71, 10.10) \n",
+ "] \n",
+ " \n",
+ "# Combine into DataFrame \n",
+ "import pandas as pd \n",
+ "df = pd.DataFrame( \n",
+ " [ \n",
+ " { \n",
+ " 'name': name, \n",
+ " 'lat': lat, \n",
+ " 'lon': lon, \n",
+ " 'time': time, \n",
+ " 'type': 'Batman Location' \n",
+ " } for (name, lat, lon, time) in batman_data \n",
+ " ] + [ \n",
+ " { \n",
+ " 'name': name, \n",
+ " 'lat': lat, \n",
+ " 'lon': lon, \n",
+ " 'time': time, \n",
+ " 'type': 'Supercar Factory' \n",
+ " } for (name, lat, lon, time) in supercar_data \n",
+ " ] \n",
+ ") \n",
+ " \n",
+ "# Generate map \n",
+ "import plotly.express as px \n",
+ "fig = px.scatter_mapbox( \n",
+ " df, \n",
+ " lat='lat', \n",
+ " lon='lon', \n",
+ " text='name', \n",
+ " color='time', \n",
+ " color_continuous_scale=px.colors.sequential.Magma, \n",
+ " zoom=1, \n",
+ " mapbox_style='carto-positron', \n",
+ " title='Cargo Travel Time to Gotham (Hours)' \n",
+ ") \n",
+ "fig.update_layout(margin={\"r\":0,\"t\":40,\"l\":0,\"b\":0}) \n",
+ "fig.write_image(\"saved_map.png\") \n",
+ "final_answer(\"saved_map.png\") \n",
+ "``` \n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1;3mOutput message of the LLM:\u001b[0m \u001b[38;2;212;183;2mโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ\u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m [\u001b[0m\u001b[1;38;2;121;192;255;48;2;13;17;23mSTEP 6\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mThought: Fix the variable name conflict and rebuild the data structures properly.\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mCode:\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;165;214;255;48;2;13;17;23m```\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mpy\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[3;38;2;139;148;158;48;2;13;17;23m# Reconstruct batman_times with original structure (from Step 2)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mbatman_data\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mStearns Wharf, Santa Barbara\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m34.4188\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m119.6951\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m6.93\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mCliffs, Marineland\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m33.9\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m118.4\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m6.80\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mBronson Caves, Griffith Park\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m34.1347\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m118.3215\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m6.78\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mAlcatraz Island\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m37.8267\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m122.4233\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m7.05\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mKnebworth House, UK\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m51.8055\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0.4035\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m9.13\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mSkaftafell, Iceland\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m64.2128\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m19.0649\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m7.37\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mChicago Board of Trade\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m41.8879\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m87.6235\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m2.68\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mSt Pancras Hotel, London\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m51.5343\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0.1341\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m9.17\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mBattersea Power Station\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m51.4719\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0.1167\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m9.17\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mNecropolis Cemetery\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m55.8642\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m-\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m4.2518\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m8.60\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23msupercar_data\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mFerrari Maranello\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m44.58\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m10.89\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m10.72\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mLamborghini Sant\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mAgata\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m44.54\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m11.18\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m10.75\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mBugatti Molsheim\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m48.69\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m7.71\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m10.10\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[3;38;2;139;148;158;48;2;13;17;23m# Combine into DataFrame\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;255;123;114;48;2;13;17;23mimport\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mpandas\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mas\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mpd\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mdf\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mpd\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mDataFrame\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m{\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mname\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mname\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mlat\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlat\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mlon\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlon\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mtime\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mtype\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mBatman Location\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m}\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mfor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mname\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlat\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlon\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23min\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mbatman_data\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m+\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m[\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m{\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mname\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mname\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mlat\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlat\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mlon\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlon\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mtime\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mtype\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mSupercar Factory\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m}\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mfor\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mname\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlat\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlon\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtime\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23min\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msupercar_data\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m]\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[3;38;2;139;148;158;48;2;13;17;23m# Generate map\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;255;123;114;48;2;13;17;23mimport\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mplotly\u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mexpress\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mas\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;255;123;114;48;2;13;17;23mpx\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mfig\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mpx\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mscatter_mapbox\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mdf\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlat\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mlat\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mlon\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mlon\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtext\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mname\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcolor\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mtime\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcolor_continuous_scale\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mpx\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mcolors\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23msequential\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mMagma\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mzoom\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m1\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmapbox_style\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mcarto-positron\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m \u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mtitle\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mCargo Travel Time to Gotham (Hours)\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m'\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mfig\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mupdate_layout\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mmargin\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m=\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m{\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mr\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mt\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m40\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23ml\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m,\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23mb\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m:\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m0\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m}\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mfig\u001b[0m\u001b[1;38;2;255;123;114;48;2;13;17;23m.\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23mwrite_image\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23msaved_map.png\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;230;237;243;48;2;13;17;23mfinal_answer\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m(\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23msaved_map.png\u001b[0m\u001b[38;2;165;214;255;48;2;13;17;23m\"\u001b[0m\u001b[38;2;230;237;243;48;2;13;17;23m)\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n",
+ "\u001b[38;2;165;214;255;48;2;13;17;23m```\u001b[0m\u001b[48;2;13;17;23m \u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ " โ Executing parsed code: โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " # Reconstruct batman_times with original structure (from Step 2) \n",
+ " batman_data = [ \n",
+ " (\"Stearns Wharf, Santa Barbara\", 34.4188, -119.6951, 6.93), \n",
+ " (\"Cliffs, Marineland\", 33.9, -118.4, 6.80), \n",
+ " (\"Bronson Caves, Griffith Park\", 34.1347, -118.3215, 6.78), \n",
+ " (\"Alcatraz Island\", 37.8267, -122.4233, 7.05), \n",
+ " (\"Knebworth House, UK\", 51.8055, -0.4035, 9.13), \n",
+ " (\"Skaftafell, Iceland\", 64.2128, -19.0649, 7.37), \n",
+ " (\"Chicago Board of Trade\", 41.8879, -87.6235, 2.68), \n",
+ " (\"St Pancras Hotel, London\", 51.5343, -0.1341, 9.17), \n",
+ " (\"Battersea Power Station\", 51.4719, -0.1167, 9.17), \n",
+ " (\"Necropolis Cemetery\", 55.8642, -4.2518, 8.60) \n",
+ " ] \n",
+ " \n",
+ " supercar_data = [ \n",
+ " (\"Ferrari Maranello\", 44.58, 10.89, 10.72), \n",
+ " (\"Lamborghini Sant'Agata\", 44.54, 11.18, 10.75), \n",
+ " (\"Bugatti Molsheim\", 48.69, 7.71, 10.10) \n",
+ " ] \n",
+ " \n",
+ " # Combine into DataFrame \n",
+ " import pandas as pd \n",
+ " df = pd.DataFrame( \n",
+ " [ \n",
+ " { \n",
+ " 'name': name, \n",
+ " 'lat': lat, \n",
+ " 'lon': lon, \n",
+ " 'time': time, \n",
+ " 'type': 'Batman Location' \n",
+ " } for (name, lat, lon, time) in batman_data \n",
+ " ] + [ \n",
+ " { \n",
+ " 'name': name, \n",
+ " 'lat': lat, \n",
+ " 'lon': lon, \n",
+ " 'time': time, \n",
+ " 'type': 'Supercar Factory' \n",
+ " } for (name, lat, lon, time) in supercar_data \n",
+ " ] \n",
+ " ) \n",
+ " \n",
+ " # Generate map \n",
+ " import plotly.express as px \n",
+ " fig = px.scatter_mapbox( \n",
+ " df, \n",
+ " lat='lat', \n",
+ " lon='lon', \n",
+ " text='name', \n",
+ " color='time', \n",
+ " color_continuous_scale=px.colors.sequential.Magma, \n",
+ " zoom=1, \n",
+ " mapbox_style='carto-positron', \n",
+ " title='Cargo Travel Time to Gotham (Hours)' \n",
+ " ) \n",
+ " fig.update_layout(margin={\"r\":0,\"t\":40,\"l\":0,\"b\":0}) \n",
+ " fig.write_image(\"saved_map.png\") \n",
+ " final_answer(\"saved_map.png\") \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ "
\n"
+ ],
+ "text/plain": [
+ " โ \u001b[1mExecuting parsed code:\u001b[0m โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Reconstruct batman_times with original structure (from Step 2)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mbatman_data\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mStearns Wharf, Santa Barbara\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m34.4188\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m119.6951\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m6.93\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mCliffs, Marineland\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m33.9\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m118.4\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m6.80\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBronson Caves, Griffith Park\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m34.1347\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m118.3215\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m6.78\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mAlcatraz Island\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m37.8267\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m122.4233\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m7.05\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mKnebworth House, UK\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m51.8055\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.4035\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m9.13\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mSkaftafell, Iceland\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m64.2128\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m19.0649\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m7.37\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mChicago Board of Trade\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m41.8879\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m87.6235\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m2.68\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mSt Pancras Hotel, London\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m51.5343\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.1341\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m9.17\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBattersea Power Station\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m51.4719\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0.1167\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m9.17\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mNecropolis Cemetery\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m55.8642\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m-\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m4.2518\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m8.60\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34msupercar_data\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mFerrari Maranello\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m44.58\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m10.89\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m10.72\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mLamborghini Sant\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mAgata\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m44.54\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m11.18\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m10.75\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBugatti Molsheim\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m48.69\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m7.71\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m10.10\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Combine into DataFrame\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;255;70;137;48;2;39;40;34mimport\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpandas\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mas\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpd\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mdf\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpd\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mDataFrame\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m{\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mname\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mname\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mlat\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlat\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mlon\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlon\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mtime\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtime\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mtype\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mBatman Location\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m}\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mfor\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mname\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlat\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlon\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtime\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34min\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mbatman_data\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m+\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m[\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m{\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mname\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mname\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mlat\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlat\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mlon\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlon\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mtime\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtime\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mtype\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mSupercar Factory\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m}\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mfor\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mname\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlat\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlon\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtime\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34min\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msupercar_data\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m]\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;149;144;119;48;2;39;40;34m# Generate map\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;255;70;137;48;2;39;40;34mimport\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mplotly\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mexpress\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;102;217;239;48;2;39;40;34mas\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpx\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mfig\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpx\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mscatter_mapbox\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mdf\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlat\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mlat\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mlon\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mlon\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtext\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mname\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcolor\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mtime\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcolor_continuous_scale\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mpx\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mcolors\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34msequential\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mMagma\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mzoom\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m1\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mmapbox_style\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mcarto-positron\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m \u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mtitle\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mCargo Travel Time to Gotham (Hours)\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m'\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mfig\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mupdate_layout\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mmargin\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m=\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m{\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mr\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mt\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m40\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34ml\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m,\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34mb\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m:\u001b[0m\u001b[38;2;174;129;255;48;2;39;40;34m0\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m}\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mfig\u001b[0m\u001b[38;2;255;70;137;48;2;39;40;34m.\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34mwrite_image\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34msaved_map.png\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " \u001b[38;2;248;248;242;48;2;39;40;34mfinal_answer\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m(\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34msaved_map.png\u001b[0m\u001b[38;2;230;219;116;48;2;39;40;34m\"\u001b[0m\u001b[38;2;248;248;242;48;2;39;40;34m)\u001b[0m\u001b[48;2;39;40;34m \u001b[0m \n",
+ " โโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโโ \n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/html": [
+ "Out - Final answer: saved_map.png\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[1;38;2;212;183;2mOut - Final answer: saved_map.png\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "name": "stdout",
+ "output_type": "stream",
+ "text": [
+ "Feedback: **Reasons Why Yes:**\n",
+ "\n",
+ "1. **Plotting Method**: The plot was made using `px.scatter_mapbox`, which is the correct method as per the task requirements.\n",
+ "2. **Location Representation**: The map shows both Batman filming locations and supercar factories, fulfilling the request for a spatial representation.\n",
+ "3. **Color Coding**: Locations are color-coded based on travel time, providing a clear visual differentiation.\n",
+ "4. **Number of Points**: The plot displays at least six points, meeting the minimum requirement set by the task.\n",
+ "\n",
+ "**Reasons Why No:**\n",
+ "\n",
+ "1. **Matching Times**: The task required supercar factories to match Batman locations' transfer times. This plot doesn't specifically confirm any matching times.\n",
+ "2. **Transfer Time Calculation**: There was a logical error in handling the transfer times that wasn't corrected in plotting. \n",
+ "3. **Variable Errors**: The plot creation initially faced issues due to handling errors in data structures, affecting data integrity.\n",
+ "\n",
+ "**Final Decision:** PASS\n",
+ "\n",
+ "The plot mostly solves the task by using `px.scatter_mapbox` to represent the locations and color code based on travel times. Despite not addressing the matching times requirement fully, the map fulfills the majority of the requirements.\n"
+ ]
+ },
+ {
+ "data": {
+ "text/html": [
+ "[Step 6: Duration 37.00 seconds| Input tokens: 34,990 | Output tokens: 2,560]\n",
+ "
\n"
+ ],
+ "text/plain": [
+ "\u001b[2m[Step 6: Duration 37.00 seconds| Input tokens: 34,990 | Output tokens: 2,560]\u001b[0m\n"
+ ]
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ },
+ {
+ "data": {
+ "text/plain": [
+ "'saved_map.png'"
+ ]
+ },
+ "execution_count": 30,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "manager_agent.run(\"\"\"\n",
+ "Find all Batman filming locations in the world, calculate the time to transfer via cargo plane to here (we're in Gotham, 40.7128ยฐ N, 74.0060ยฐ W).\n",
+ "Also give me some supercar factories with the same cargo plane transfer time. You need at least 6 points in total.\n",
+ "Represent this as spatial map of the world, with the locations represented as scatter points with a color that depends on the travel time, and save it to saved_map.png!\n",
+ "\n",
+ "Here's an example of how to plot and return a map:\n",
+ "import plotly.express as px\n",
+ "df = px.data.carshare()\n",
+ "fig = px.scatter_map(df, lat=\"centroid_lat\", lon=\"centroid_lon\", text=\"name\", color=\"peak_hour\", size=100,\n",
+ " color_continuous_scale=px.colors.sequential.Magma, size_max=15, zoom=1)\n",
+ "fig.show()\n",
+ "fig.write_image(\"saved_image.png\")\n",
+ "final_answer(fig)\n",
+ "\n",
+ "Never try to process strings using code: when you have a string to read, just print it and you'll see it.\n",
+ "\"\"\")"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "EGuNy8RJavwX"
+ },
+ "source": [
+ "I don't know how that went in your run, but in mine, the manager agent skilfully divided tasks given to the web agent in `1. Search for Batman filming locations`, then `2. Find supercar factories`, before aggregating the lists and plotting the map.\n",
+ "\n",
+ "Let's see what the map looks like by inspecting it directly from the agent state:"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "id": "G0lADQ18avwX",
+ "outputId": "0685ac3c-4b9f-4f62-f33e-d81545c365b2"
+ },
+ "outputs": [
+ {
+ "data": {
+ "application/vnd.plotly.v1+json": {
+ "config": {
+ "plotlyServerURL": "https://plot.ly"
+ },
+ "data": [
+ {
+ "hovertemplate": "name=%{text}
lat=%{lat}
lon=%{lon}
time=%{marker.color}",
+ "lat": [
+ 34.4188,
+ 33.9,
+ 34.1347,
+ 37.8267,
+ 51.8055,
+ 64.2128,
+ 41.8879,
+ 51.5343,
+ 51.4719,
+ 55.8642,
+ 44.58,
+ 44.54,
+ 48.69
+ ],
+ "legendgroup": "",
+ "lon": [
+ -119.6951,
+ -118.4,
+ -118.3215,
+ -122.4233,
+ -0.4035,
+ -19.0649,
+ -87.6235,
+ -0.1341,
+ -0.1167,
+ -4.2518,
+ 10.89,
+ 11.18,
+ 7.71
+ ],
+ "marker": {
+ "color": [
+ 6.93,
+ 6.8,
+ 6.78,
+ 7.05,
+ 9.13,
+ 7.37,
+ 2.68,
+ 9.17,
+ 9.17,
+ 8.6,
+ 10.72,
+ 10.75,
+ 10.1
+ ],
+ "coloraxis": "coloraxis"
+ },
+ "mode": "markers+text",
+ "name": "",
+ "showlegend": false,
+ "subplot": "mapbox",
+ "text": [
+ "Stearns Wharf, Santa Barbara",
+ "Cliffs, Marineland",
+ "Bronson Caves, Griffith Park",
+ "Alcatraz Island",
+ "Knebworth House, UK",
+ "Skaftafell, Iceland",
+ "Chicago Board of Trade",
+ "St Pancras Hotel, London",
+ "Battersea Power Station",
+ "Necropolis Cemetery",
+ "Ferrari Maranello",
+ "Lamborghini Sant'Agata",
+ "Bugatti Molsheim"
+ ],
+ "type": "scattermapbox"
+ }
+ ],
+ "layout": {
+ "coloraxis": {
+ "colorbar": {
+ "title": {
+ "text": "time"
+ }
+ },
+ "colorscale": [
+ [
+ 0,
+ "#000004"
+ ],
+ [
+ 0.1111111111111111,
+ "#180f3d"
+ ],
+ [
+ 0.2222222222222222,
+ "#440f76"
+ ],
+ [
+ 0.3333333333333333,
+ "#721f81"
+ ],
+ [
+ 0.4444444444444444,
+ "#9e2f7f"
+ ],
+ [
+ 0.5555555555555556,
+ "#cd4071"
+ ],
+ [
+ 0.6666666666666666,
+ "#f1605d"
+ ],
+ [
+ 0.7777777777777778,
+ "#fd9668"
+ ],
+ [
+ 0.8888888888888888,
+ "#feca8d"
+ ],
+ [
+ 1,
+ "#fcfdbf"
+ ]
+ ]
+ },
+ "legend": {
+ "tracegroupgap": 0
+ },
+ "mapbox": {
+ "center": {
+ "lat": 45.75898461538462,
+ "lon": -43.127261538461546
+ },
+ "domain": {
+ "x": [
+ 0,
+ 1
+ ],
+ "y": [
+ 0,
+ 1
+ ]
+ },
+ "style": "carto-positron",
+ "zoom": 1
+ },
+ "margin": {
+ "b": 0,
+ "l": 0,
+ "r": 0,
+ "t": 40
+ },
+ "template": {
+ "data": {
+ "bar": [
+ {
+ "error_x": {
+ "color": "#2a3f5f"
+ },
+ "error_y": {
+ "color": "#2a3f5f"
+ },
+ "marker": {
+ "line": {
+ "color": "#E5ECF6",
+ "width": 0.5
+ },
+ "pattern": {
+ "fillmode": "overlay",
+ "size": 10,
+ "solidity": 0.2
+ }
+ },
+ "type": "bar"
+ }
+ ],
+ "barpolar": [
+ {
+ "marker": {
+ "line": {
+ "color": "#E5ECF6",
+ "width": 0.5
+ },
+ "pattern": {
+ "fillmode": "overlay",
+ "size": 10,
+ "solidity": 0.2
+ }
+ },
+ "type": "barpolar"
+ }
+ ],
+ "carpet": [
+ {
+ "aaxis": {
+ "endlinecolor": "#2a3f5f",
+ "gridcolor": "white",
+ "linecolor": "white",
+ "minorgridcolor": "white",
+ "startlinecolor": "#2a3f5f"
+ },
+ "baxis": {
+ "endlinecolor": "#2a3f5f",
+ "gridcolor": "white",
+ "linecolor": "white",
+ "minorgridcolor": "white",
+ "startlinecolor": "#2a3f5f"
+ },
+ "type": "carpet"
+ }
+ ],
+ "choropleth": [
+ {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ },
+ "type": "choropleth"
+ }
+ ],
+ "contour": [
+ {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ },
+ "colorscale": [
+ [
+ 0,
+ "#0d0887"
+ ],
+ [
+ 0.1111111111111111,
+ "#46039f"
+ ],
+ [
+ 0.2222222222222222,
+ "#7201a8"
+ ],
+ [
+ 0.3333333333333333,
+ "#9c179e"
+ ],
+ [
+ 0.4444444444444444,
+ "#bd3786"
+ ],
+ [
+ 0.5555555555555556,
+ "#d8576b"
+ ],
+ [
+ 0.6666666666666666,
+ "#ed7953"
+ ],
+ [
+ 0.7777777777777778,
+ "#fb9f3a"
+ ],
+ [
+ 0.8888888888888888,
+ "#fdca26"
+ ],
+ [
+ 1,
+ "#f0f921"
+ ]
+ ],
+ "type": "contour"
+ }
+ ],
+ "contourcarpet": [
+ {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ },
+ "type": "contourcarpet"
+ }
+ ],
+ "heatmap": [
+ {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ },
+ "colorscale": [
+ [
+ 0,
+ "#0d0887"
+ ],
+ [
+ 0.1111111111111111,
+ "#46039f"
+ ],
+ [
+ 0.2222222222222222,
+ "#7201a8"
+ ],
+ [
+ 0.3333333333333333,
+ "#9c179e"
+ ],
+ [
+ 0.4444444444444444,
+ "#bd3786"
+ ],
+ [
+ 0.5555555555555556,
+ "#d8576b"
+ ],
+ [
+ 0.6666666666666666,
+ "#ed7953"
+ ],
+ [
+ 0.7777777777777778,
+ "#fb9f3a"
+ ],
+ [
+ 0.8888888888888888,
+ "#fdca26"
+ ],
+ [
+ 1,
+ "#f0f921"
+ ]
+ ],
+ "type": "heatmap"
+ }
+ ],
+ "heatmapgl": [
+ {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ },
+ "colorscale": [
+ [
+ 0,
+ "#0d0887"
+ ],
+ [
+ 0.1111111111111111,
+ "#46039f"
+ ],
+ [
+ 0.2222222222222222,
+ "#7201a8"
+ ],
+ [
+ 0.3333333333333333,
+ "#9c179e"
+ ],
+ [
+ 0.4444444444444444,
+ "#bd3786"
+ ],
+ [
+ 0.5555555555555556,
+ "#d8576b"
+ ],
+ [
+ 0.6666666666666666,
+ "#ed7953"
+ ],
+ [
+ 0.7777777777777778,
+ "#fb9f3a"
+ ],
+ [
+ 0.8888888888888888,
+ "#fdca26"
+ ],
+ [
+ 1,
+ "#f0f921"
+ ]
+ ],
+ "type": "heatmapgl"
+ }
+ ],
+ "histogram": [
+ {
+ "marker": {
+ "pattern": {
+ "fillmode": "overlay",
+ "size": 10,
+ "solidity": 0.2
+ }
+ },
+ "type": "histogram"
+ }
+ ],
+ "histogram2d": [
+ {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ },
+ "colorscale": [
+ [
+ 0,
+ "#0d0887"
+ ],
+ [
+ 0.1111111111111111,
+ "#46039f"
+ ],
+ [
+ 0.2222222222222222,
+ "#7201a8"
+ ],
+ [
+ 0.3333333333333333,
+ "#9c179e"
+ ],
+ [
+ 0.4444444444444444,
+ "#bd3786"
+ ],
+ [
+ 0.5555555555555556,
+ "#d8576b"
+ ],
+ [
+ 0.6666666666666666,
+ "#ed7953"
+ ],
+ [
+ 0.7777777777777778,
+ "#fb9f3a"
+ ],
+ [
+ 0.8888888888888888,
+ "#fdca26"
+ ],
+ [
+ 1,
+ "#f0f921"
+ ]
+ ],
+ "type": "histogram2d"
+ }
+ ],
+ "histogram2dcontour": [
+ {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ },
+ "colorscale": [
+ [
+ 0,
+ "#0d0887"
+ ],
+ [
+ 0.1111111111111111,
+ "#46039f"
+ ],
+ [
+ 0.2222222222222222,
+ "#7201a8"
+ ],
+ [
+ 0.3333333333333333,
+ "#9c179e"
+ ],
+ [
+ 0.4444444444444444,
+ "#bd3786"
+ ],
+ [
+ 0.5555555555555556,
+ "#d8576b"
+ ],
+ [
+ 0.6666666666666666,
+ "#ed7953"
+ ],
+ [
+ 0.7777777777777778,
+ "#fb9f3a"
+ ],
+ [
+ 0.8888888888888888,
+ "#fdca26"
+ ],
+ [
+ 1,
+ "#f0f921"
+ ]
+ ],
+ "type": "histogram2dcontour"
+ }
+ ],
+ "mesh3d": [
+ {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ },
+ "type": "mesh3d"
+ }
+ ],
+ "parcoords": [
+ {
+ "line": {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ }
+ },
+ "type": "parcoords"
+ }
+ ],
+ "pie": [
+ {
+ "automargin": true,
+ "type": "pie"
+ }
+ ],
+ "scatter": [
+ {
+ "fillpattern": {
+ "fillmode": "overlay",
+ "size": 10,
+ "solidity": 0.2
+ },
+ "type": "scatter"
+ }
+ ],
+ "scatter3d": [
+ {
+ "line": {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ }
+ },
+ "marker": {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ }
+ },
+ "type": "scatter3d"
+ }
+ ],
+ "scattercarpet": [
+ {
+ "marker": {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ }
+ },
+ "type": "scattercarpet"
+ }
+ ],
+ "scattergeo": [
+ {
+ "marker": {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ }
+ },
+ "type": "scattergeo"
+ }
+ ],
+ "scattergl": [
+ {
+ "marker": {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ }
+ },
+ "type": "scattergl"
+ }
+ ],
+ "scattermapbox": [
+ {
+ "marker": {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ }
+ },
+ "type": "scattermapbox"
+ }
+ ],
+ "scatterpolar": [
+ {
+ "marker": {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ }
+ },
+ "type": "scatterpolar"
+ }
+ ],
+ "scatterpolargl": [
+ {
+ "marker": {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ }
+ },
+ "type": "scatterpolargl"
+ }
+ ],
+ "scatterternary": [
+ {
+ "marker": {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ }
+ },
+ "type": "scatterternary"
+ }
+ ],
+ "surface": [
+ {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ },
+ "colorscale": [
+ [
+ 0,
+ "#0d0887"
+ ],
+ [
+ 0.1111111111111111,
+ "#46039f"
+ ],
+ [
+ 0.2222222222222222,
+ "#7201a8"
+ ],
+ [
+ 0.3333333333333333,
+ "#9c179e"
+ ],
+ [
+ 0.4444444444444444,
+ "#bd3786"
+ ],
+ [
+ 0.5555555555555556,
+ "#d8576b"
+ ],
+ [
+ 0.6666666666666666,
+ "#ed7953"
+ ],
+ [
+ 0.7777777777777778,
+ "#fb9f3a"
+ ],
+ [
+ 0.8888888888888888,
+ "#fdca26"
+ ],
+ [
+ 1,
+ "#f0f921"
+ ]
+ ],
+ "type": "surface"
+ }
+ ],
+ "table": [
+ {
+ "cells": {
+ "fill": {
+ "color": "#EBF0F8"
+ },
+ "line": {
+ "color": "white"
+ }
+ },
+ "header": {
+ "fill": {
+ "color": "#C8D4E3"
+ },
+ "line": {
+ "color": "white"
+ }
+ },
+ "type": "table"
+ }
+ ]
+ },
+ "layout": {
+ "annotationdefaults": {
+ "arrowcolor": "#2a3f5f",
+ "arrowhead": 0,
+ "arrowwidth": 1
+ },
+ "autotypenumbers": "strict",
+ "coloraxis": {
+ "colorbar": {
+ "outlinewidth": 0,
+ "ticks": ""
+ }
+ },
+ "colorscale": {
+ "diverging": [
+ [
+ 0,
+ "#8e0152"
+ ],
+ [
+ 0.1,
+ "#c51b7d"
+ ],
+ [
+ 0.2,
+ "#de77ae"
+ ],
+ [
+ 0.3,
+ "#f1b6da"
+ ],
+ [
+ 0.4,
+ "#fde0ef"
+ ],
+ [
+ 0.5,
+ "#f7f7f7"
+ ],
+ [
+ 0.6,
+ "#e6f5d0"
+ ],
+ [
+ 0.7,
+ "#b8e186"
+ ],
+ [
+ 0.8,
+ "#7fbc41"
+ ],
+ [
+ 0.9,
+ "#4d9221"
+ ],
+ [
+ 1,
+ "#276419"
+ ]
+ ],
+ "sequential": [
+ [
+ 0,
+ "#0d0887"
+ ],
+ [
+ 0.1111111111111111,
+ "#46039f"
+ ],
+ [
+ 0.2222222222222222,
+ "#7201a8"
+ ],
+ [
+ 0.3333333333333333,
+ "#9c179e"
+ ],
+ [
+ 0.4444444444444444,
+ "#bd3786"
+ ],
+ [
+ 0.5555555555555556,
+ "#d8576b"
+ ],
+ [
+ 0.6666666666666666,
+ "#ed7953"
+ ],
+ [
+ 0.7777777777777778,
+ "#fb9f3a"
+ ],
+ [
+ 0.8888888888888888,
+ "#fdca26"
+ ],
+ [
+ 1,
+ "#f0f921"
+ ]
+ ],
+ "sequentialminus": [
+ [
+ 0,
+ "#0d0887"
+ ],
+ [
+ 0.1111111111111111,
+ "#46039f"
+ ],
+ [
+ 0.2222222222222222,
+ "#7201a8"
+ ],
+ [
+ 0.3333333333333333,
+ "#9c179e"
+ ],
+ [
+ 0.4444444444444444,
+ "#bd3786"
+ ],
+ [
+ 0.5555555555555556,
+ "#d8576b"
+ ],
+ [
+ 0.6666666666666666,
+ "#ed7953"
+ ],
+ [
+ 0.7777777777777778,
+ "#fb9f3a"
+ ],
+ [
+ 0.8888888888888888,
+ "#fdca26"
+ ],
+ [
+ 1,
+ "#f0f921"
+ ]
+ ]
+ },
+ "colorway": [
+ "#636efa",
+ "#EF553B",
+ "#00cc96",
+ "#ab63fa",
+ "#FFA15A",
+ "#19d3f3",
+ "#FF6692",
+ "#B6E880",
+ "#FF97FF",
+ "#FECB52"
+ ],
+ "font": {
+ "color": "#2a3f5f"
+ },
+ "geo": {
+ "bgcolor": "white",
+ "lakecolor": "white",
+ "landcolor": "#E5ECF6",
+ "showlakes": true,
+ "showland": true,
+ "subunitcolor": "white"
+ },
+ "hoverlabel": {
+ "align": "left"
+ },
+ "hovermode": "closest",
+ "mapbox": {
+ "style": "light"
+ },
+ "paper_bgcolor": "white",
+ "plot_bgcolor": "#E5ECF6",
+ "polar": {
+ "angularaxis": {
+ "gridcolor": "white",
+ "linecolor": "white",
+ "ticks": ""
+ },
+ "bgcolor": "#E5ECF6",
+ "radialaxis": {
+ "gridcolor": "white",
+ "linecolor": "white",
+ "ticks": ""
+ }
+ },
+ "scene": {
+ "xaxis": {
+ "backgroundcolor": "#E5ECF6",
+ "gridcolor": "white",
+ "gridwidth": 2,
+ "linecolor": "white",
+ "showbackground": true,
+ "ticks": "",
+ "zerolinecolor": "white"
+ },
+ "yaxis": {
+ "backgroundcolor": "#E5ECF6",
+ "gridcolor": "white",
+ "gridwidth": 2,
+ "linecolor": "white",
+ "showbackground": true,
+ "ticks": "",
+ "zerolinecolor": "white"
+ },
+ "zaxis": {
+ "backgroundcolor": "#E5ECF6",
+ "gridcolor": "white",
+ "gridwidth": 2,
+ "linecolor": "white",
+ "showbackground": true,
+ "ticks": "",
+ "zerolinecolor": "white"
+ }
+ },
+ "shapedefaults": {
+ "line": {
+ "color": "#2a3f5f"
+ }
+ },
+ "ternary": {
+ "aaxis": {
+ "gridcolor": "white",
+ "linecolor": "white",
+ "ticks": ""
+ },
+ "baxis": {
+ "gridcolor": "white",
+ "linecolor": "white",
+ "ticks": ""
+ },
+ "bgcolor": "#E5ECF6",
+ "caxis": {
+ "gridcolor": "white",
+ "linecolor": "white",
+ "ticks": ""
+ }
+ },
+ "title": {
+ "x": 0.05
+ },
+ "xaxis": {
+ "automargin": true,
+ "gridcolor": "white",
+ "linecolor": "white",
+ "ticks": "",
+ "title": {
+ "standoff": 15
+ },
+ "zerolinecolor": "white",
+ "zerolinewidth": 2
+ },
+ "yaxis": {
+ "automargin": true,
+ "gridcolor": "white",
+ "linecolor": "white",
+ "ticks": "",
+ "title": {
+ "standoff": 15
+ },
+ "zerolinecolor": "white",
+ "zerolinewidth": 2
+ }
+ }
+ },
+ "title": {
+ "text": "Cargo Travel Time to Gotham (Hours)"
+ }
+ }
+ }
+ },
+ "metadata": {},
+ "output_type": "display_data"
+ }
+ ],
+ "source": [
+ "manager_agent.python_executor.state[\"fig\"]"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {
+ "id": "fzD9OxdVavwY"
+ },
+ "source": [
+ ""
+ ]
+ }
+ ],
+ "metadata": {
+ "colab": {
+ "provenance": []
+ },
+ "kernelspec": {
+ "display_name": "test",
+ "language": "python",
+ "name": "test"
+ },
+ "language_info": {
+ "codemirror_mode": {
+ "name": "ipython",
+ "version": 3
+ },
+ "file_extension": ".py",
+ "mimetype": "text/x-python",
+ "name": "python",
+ "nbconvert_exporter": "python",
+ "pygments_lexer": "ipython3",
+ "version": "3.12.0"
+ }
+ },
+ "nbformat": 4,
+ "nbformat_minor": 0
+}