Upload 2 files
Browse files- README.md +97 -0
- result.png +0 -0
README.md
ADDED
@@ -0,0 +1,97 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
---
|
2 |
+
library_name: transformers.js
|
3 |
+
pipeline_tag: object-detection
|
4 |
+
---
|
5 |
+
|
6 |
+
ONNX weights for https://huggingface.co/hantian/yolo-doclaynet yolo10b
|
7 |
+
|
8 |
+
## Usage (Transformers.js)
|
9 |
+
|
10 |
+
If you haven't already, you can install the [Transformers.js](https://huggingface.co/docs/transformers.js) JavaScript library from [NPM](https://www.npmjs.com/package/@huggingface/transformers) using:
|
11 |
+
|
12 |
+
```bash
|
13 |
+
npm i @huggingface/transformers
|
14 |
+
```
|
15 |
+
|
16 |
+
**Example:** Perform object-detection with `Oblix/yolov10m-doclaynet_ONNX_document-layout-analysis`.
|
17 |
+
|
18 |
+
```js
|
19 |
+
const model = await AutoModel.from_pretrained(
|
20 |
+
"Oblix/yolov10m-doclaynet_ONNX_document-layout-analysis",
|
21 |
+
{
|
22 |
+
dtype: "fp32"
|
23 |
+
}
|
24 |
+
);
|
25 |
+
const processor = await AutoProcessor.from_pretrained(
|
26 |
+
"Oblix/yolov10m-doclaynet_ONNX_document-layout-analysis"
|
27 |
+
);
|
28 |
+
const url =
|
29 |
+
"https://huggingface.co/DILHTWD/documentlayoutsegmentation_YOLOv8_ondoclaynet/resolve/main/sample1.png";
|
30 |
+
const image = await RawImage.read(url);
|
31 |
+
const { pixel_values, reshaped_input_sizes } = await processor(image);
|
32 |
+
|
33 |
+
// Run object detection
|
34 |
+
const { output0 } = await model({ images: pixel_values });
|
35 |
+
const predictions = output0.tolist()[0];
|
36 |
+
|
37 |
+
const threshold = 0.35;
|
38 |
+
const [newHeight, newWidth] = reshaped_input_sizes[0]; // Reshaped height and width
|
39 |
+
const [xs, ys] = [image.width / newWidth, image.height / newHeight]; // x and y resize scales
|
40 |
+
for (const [xmin, ymin, xmax, ymax, score, id] of predictions) {
|
41 |
+
if (score < threshold) continue;
|
42 |
+
|
43 |
+
// Convert to original image coordinates
|
44 |
+
const bbox = [xmin * xs, ymin * ys, xmax * xs, ymax * ys]
|
45 |
+
.map((x) => x.toFixed(2))
|
46 |
+
.join(", ");
|
47 |
+
console.log(
|
48 |
+
// eslint-disable-next-line @typescript-eslint/no-explicit-any
|
49 |
+
`Found "${(model.config as any).id2label[id]}" at [${bbox}] with score ${score.toFixed(
|
50 |
+
2
|
51 |
+
)}.`
|
52 |
+
);
|
53 |
+
}
|
54 |
+
```
|
55 |
+
|
56 |
+
**Result**
|
57 |
+
|
58 |
+
```
|
59 |
+
Found "Text" at [53.75, 478.56, 623.46, 562.13] with score 0.98.
|
60 |
+
Found "Text" at [54.20, 593.64, 609.42, 637.15] with score 0.98.
|
61 |
+
Found "Text" at [53.98, 715.41, 621.06, 759.33] with score 0.98.
|
62 |
+
Found "Text" at [53.98, 247.44, 610.82, 277.49] with score 0.97.
|
63 |
+
Found "Title" at [53.64, 75.40, 551.96, 159.72] with score 0.97.
|
64 |
+
Found "List-item" at [55.56, 761.62, 607.48, 792.06] with score 0.97.
|
65 |
+
Found "List-item" at [56.05, 657.97, 614.57, 701.79] with score 0.97.
|
66 |
+
Found "Text" at [54.10, 195.40, 221.43, 211.88] with score 0.96.
|
67 |
+
Found "Text" at [54.25, 169.14, 95.17, 186.22] with score 0.95.
|
68 |
+
Found "Text" at [54.15, 222.11, 98.62, 237.74] with score 0.95.
|
69 |
+
Found "Text" at [53.73, 429.63, 412.82, 446.28] with score 0.95.
|
70 |
+
Found "Page-header" at [308.98, 10.07, 605.53, 34.59] with score 0.95.
|
71 |
+
Found "Section-header" at [54.18, 338.87, 102.68, 355.16] with score 0.95.
|
72 |
+
Found "List-item" at [55.75, 793.91, 519.29, 810.43] with score 0.95.
|
73 |
+
Found "Section-header" at [54.20, 453.01, 145.02, 469.42] with score 0.94.
|
74 |
+
Found "Text" at [56.76, 309.85, 316.43, 325.71] with score 0.93.
|
75 |
+
Found "List-item" at [55.62, 812.37, 445.03, 829.42] with score 0.92.
|
76 |
+
Found "Page-footer" at [308.43, 907.93, 374.03, 922.28] with score 0.92.
|
77 |
+
Found "Section-header" at [53.70, 567.21, 75.24, 584.85] with score 0.91.
|
78 |
+
Found "Text" at [56.26, 289.47, 415.46, 306.48] with score 0.80.
|
79 |
+
Found "Text" at [54.11, 365.35, 623.46, 407.97] with score 0.79.
|
80 |
+
Found "List-item" at [55.77, 638.84, 382.47, 655.46] with score 0.60.
|
81 |
+
```
|
82 |
+
|
83 |
+
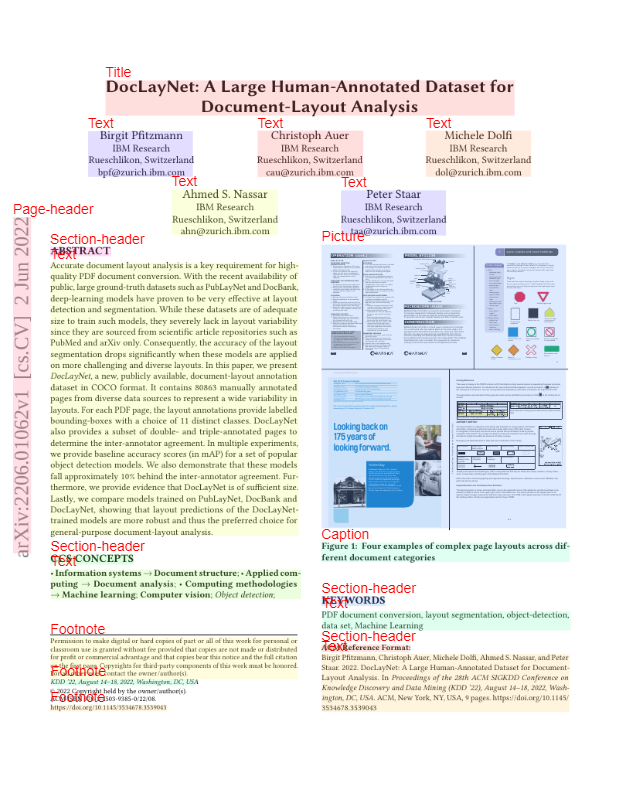
|
84 |
+
|
85 |
+
## Labels
|
86 |
+
|
87 |
+
- Caption [0]
|
88 |
+
- Footnote [1]
|
89 |
+
- Formula [2]
|
90 |
+
- List-item [3]
|
91 |
+
- Page-footer [4]
|
92 |
+
- Page-header [5]
|
93 |
+
- Picture [6]
|
94 |
+
- Section-header [7]
|
95 |
+
- Table [8]
|
96 |
+
- Text [9]
|
97 |
+
- Title [10]
|
result.png
ADDED
![]() |